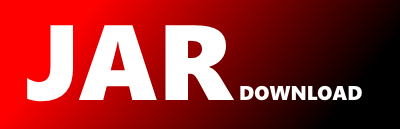
nstream.adapter.common.NstreamAgent Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of nstream-adapter-common Show documentation
Show all versions of nstream-adapter-common Show documentation
General server-side data flow templates with Swim
// Copyright 2015-2024 Nstream, inc.
//
// Licensed under the Redis Source Available License 2.0 (RSALv2) Agreement;
// you may not use this file except in compliance with the License.
// You may obtain a copy of the License at
//
// https://redis.com/legal/rsalv2-agreement/
//
// Unless required by applicable law or agreed to in writing, software
// distributed under the License is distributed on an "AS IS" BASIS,
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
// See the License for the specific language governing permissions and
// limitations under the License.
package nstream.adapter.common;
import java.util.function.BiFunction;
import java.util.function.Function;
import java.util.function.Supplier;
import nstream.adapter.common.amenity.LabeledLogAmenity;
import nstream.adapter.common.amenity.StageAmenity;
import nstream.adapter.common.amenity.SummaryAmenity;
import nstream.adapter.common.schedule.StageService;
import swim.api.SwimLane;
import swim.api.agent.AbstractAgent;
import swim.api.http.HttpLane;
import swim.codec.Decoder;
import swim.concurrent.TaskRef;
import swim.concurrent.TimerRef;
import swim.http.HttpRequest;
import swim.http.HttpResponse;
public class NstreamAgent extends AbstractAgent implements StageService {
protected final LabeledLogAmenity labeledLog;
protected final SummaryAmenity summary;
protected final StageAmenity stageAmenity;
public NstreamAgent() {
this.labeledLog = new LabeledLogAmenity(this);
this.summary = new SummaryAmenity(this);
this.stageAmenity = new StageAmenity(this);
}
// labeledLog delegates
@Override
public void trace(Object message) {
this.labeledLog.trace(message);
}
@Override
public void debug(Object message) {
this.labeledLog.debug(message);
}
@Override
public void info(Object message) {
this.labeledLog.info(message);
}
@Override
public void warn(Object message) {
this.labeledLog.warn(message);
}
@Override
public void fail(Object message) {
this.labeledLog.fail(message);
}
// summary delegates
@SwimLane(SummaryAmenity.SUMMARY_LANE_URI)
protected HttpLane> apiLaneSummary = httpLane()
.decodeRequest(this::apiLaneSummaryDecodeRequest)
.doRespond(this::apiLaneSummaryDoRespond);
protected Decoder
© 2015 - 2025 Weber Informatics LLC | Privacy Policy