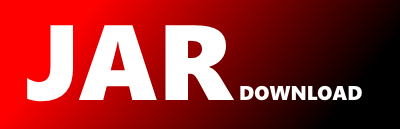
nstream.adapter.common.content.CsvRowAssembler Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of nstream-adapter-common Show documentation
Show all versions of nstream-adapter-common Show documentation
General server-side data flow templates with Swim
// Copyright 2015-2024 Nstream, inc.
//
// Licensed under the Redis Source Available License 2.0 (RSALv2) Agreement;
// you may not use this file except in compliance with the License.
// You may obtain a copy of the License at
//
// https://redis.com/legal/rsalv2-agreement/
//
// Unless required by applicable law or agreed to in writing, software
// distributed under the License is distributed on an "AS IS" BASIS,
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
// See the License for the specific language governing permissions and
// limitations under the License.
package nstream.adapter.common.content;
import java.io.IOException;
import java.io.InputStream;
import java.util.Locale;
import nstream.adapter.common.ingress.AssemblyException;
import nstream.adapter.common.ingress.ContentAssembler;
import swim.csv.Csv;
import swim.csv.structure.CsvStructure;
import swim.csv.structure.CsvStructureCol;
import swim.csv.structure.CsvStructureHeader;
import swim.structure.Attr;
import swim.structure.Item;
import swim.structure.Record;
import swim.structure.Text;
import swim.structure.Value;
public class CsvRowAssembler extends ContentAssembler {
private final CsvStructureHeader header;
public CsvRowAssembler(Value settings) {
super(settings);
if (settings.head() instanceof Attr) {
final String head = settings.head().key().stringValue(null);
if ("csvRowAssembler".equals(head) || "valueAssembler".equals(head)) {
this.header = headerFromTail(settings.tail());
return;
}
}
throw new IllegalArgumentException("Invalid CsvContentMolder structure: " + settings);
}
@Override
public String contentType() {
return "csv";
}
@Override
public Value assembleStream(InputStream decodedStream) throws AssemblyException, IOException {
return assembleBytes(decodedStream.readAllBytes());
}
@Override
public Value assemble(String raw) {
return Csv.parseRow(raw, this.header);
}
private static CsvStructureHeader headerFromTail(Record tail) {
final CsvStructureCol[] arr = tail.stream()
.map(Item::toValue)
.map(Col::fromValue)
.map(Col::toStructureCol)
.toArray(CsvStructureCol[]::new);
if (arr.length != 0) {
return CsvStructure.header(arr);
}
throw new IllegalArgumentException("Invalid CsvContentMolder structure: " + tail);
}
private static final class Col {
private final String name;
private final ColType type;
private final boolean mandatory;
private Col(String name, ColType type, boolean mandatory) {
this.name = name;
this.type = type;
this.mandatory = mandatory;
}
private CsvStructureCol toStructureCol() {
final boolean optional = !this.mandatory;
switch (this.type) {
case STRING:
return this.name == null
? this.mandatory ? MANDATORY_STRING_NAMELESS : OPTIONAL_STRING_NAMELESS
: CsvStructure.stringCol(this.name).optional(optional);
case NUMBER:
return this.name == null
? this.mandatory ? MANDATORY_NUMBER_NAMELESS : OPTIONAL_NUMBER_NAMELESS
: CsvStructure.numberCol(this.name).optional(optional);
case NULL:
default:
return this.name == null
? this.mandatory ? MANDATORY_NULL_NAMELESS : OPTIONAL_NULL_NAMELESS
: CsvStructure.nullCol(this.name).optional(optional);
}
}
private static Col fromValue(Value structure) {
if (structure == null || !structure.isDistinct()) {
return DEFAULT;
}
if (structure instanceof Text) {
return new Col(structure.stringValue(), ColType.STRING, false);
} else if (structure instanceof Record) {
final Item head = structure.head();
if (head instanceof Attr && "col".equals(head.key().stringValue(null))) {
final String name = !head.toValue().isDistinct() ? null : head.toValue().stringValue(null);
String type = structure.get("type").stringValue("string").toUpperCase(Locale.ROOT);
if (!"NUMBER".equals(type) && !"STRING".equals(type) && !"NULL".equals(type)) {
type = "STRING";
}
final boolean mandatory = structure.get("mandatory").booleanValue(false);
return name == null && "STRING".equals(type) && mandatory
? DEFAULT
: new Col(name, ColType.valueOf(type), mandatory);
}
}
return DEFAULT;
}
private static final Col DEFAULT = new Col(null, ColType.STRING, false);
private static final CsvStructureCol MANDATORY_STRING_NAMELESS = CsvStructure.stringCol().optional(false);
private static final CsvStructureCol OPTIONAL_STRING_NAMELESS = CsvStructure.stringCol().optional(true);
private static final CsvStructureCol MANDATORY_NUMBER_NAMELESS = CsvStructure.numberCol().optional(false);
private static final CsvStructureCol OPTIONAL_NUMBER_NAMELESS = CsvStructure.numberCol().optional(true);
private static final CsvStructureCol MANDATORY_NULL_NAMELESS = CsvStructure.numberCol().optional(false);
private static final CsvStructureCol OPTIONAL_NULL_NAMELESS = CsvStructure.numberCol().optional(true);
}
private enum ColType {
STRING, NUMBER, NULL
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy