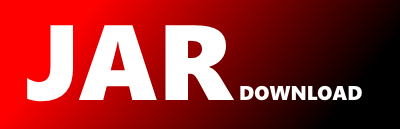
nstream.adapter.common.ext.PubSubIngressSettings Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of nstream-adapter-common Show documentation
Show all versions of nstream-adapter-common Show documentation
General server-side data flow templates with Swim
// Copyright 2015-2024 Nstream, inc.
//
// Licensed under the Redis Source Available License 2.0 (RSALv2) Agreement;
// you may not use this file except in compliance with the License.
// You may obtain a copy of the License at
//
// https://redis.com/legal/rsalv2-agreement/
//
// Unless required by applicable law or agreed to in writing, software
// distributed under the License is distributed on an "AS IS" BASIS,
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
// See the License for the specific language governing permissions and
// limitations under the License.
package nstream.adapter.common.ext;
import java.util.Properties;
import nstream.adapter.common.AdapterSettings;
import swim.codec.Format;
import swim.codec.Output;
import swim.structure.Form;
import swim.structure.Kind;
import swim.structure.Record;
import swim.structure.Tag;
import swim.structure.Value;
import swim.util.Log;
/**
* Provides a concrete class to parse and store agent configuration
* used for Google Pub/Sub ingress by {@code nstream.adapter.pubsub.PubSubIngestingAgent} and
* {@code PubSubIngestingPatch}.
*/
@Tag("pubsubIngressSettings")
public class PubSubIngressSettings implements AdapterSettings {
private static final PubSubIngressSettings DEFAULT = new PubSubIngressSettings();
@Kind
private static Form form;
private final String topicName;
private final String subscriptionId;
private final String subscriberProvisionName;
private final String contentTypeOverride;
private final String contentEncodingOverride;
private final String avroSchemaFilePath; // Avro schema support
private final Value valueMolder;
private final Value relaySchema;
private final long firstFetchDelayMillis;
private final long fetchIntervalMillis;
private final int pollTimeoutMillis;
private final String credentialsFilePath;
private final String protoFilePath;
public PubSubIngressSettings(long firstFetchDelayMillis, long fetchIntervalMillis, int pollTimeoutMillis,
String subscriberProvisionName, String subscriptionId, String topicName,
String credentialsFilePath, String contentTypeOverride, String contentEncodingOverride,
Value valueMolder, Value relaySchema, String avroSchemaFilePath, String protoFilePath) {
this.topicName = topicName;
this.subscriptionId = subscriptionId;
this.subscriberProvisionName = subscriberProvisionName;
this.contentTypeOverride = contentTypeOverride;
this.contentEncodingOverride = contentEncodingOverride;
this.avroSchemaFilePath = avroSchemaFilePath;
this.valueMolder = valueMolder;
this.relaySchema = relaySchema;
this.credentialsFilePath = credentialsFilePath;
this.firstFetchDelayMillis = firstFetchDelayMillis;
this.fetchIntervalMillis = fetchIntervalMillis;
this.pollTimeoutMillis = pollTimeoutMillis;
this.protoFilePath = protoFilePath;
}
public PubSubIngressSettings() {
this(2000L, 120000L, 60000, "subscriberProvision", "mySubscriptionId", "myTopicName", "", "json", null, null,
Value.fromObject(""), "", "");
}
public static Form form() {
if (PubSubIngressSettings.form == null) {
PubSubIngressSettings.form = Form.forClass(PubSubIngressSettings.class);
}
return PubSubIngressSettings.form;
}
public static PubSubIngressSettings defaultSettings() {
return PubSubIngressSettings.DEFAULT;
}
public static Record moldFromProperties(Log log, Properties p) {
return Record.create(); // Implement logic if required
}
public String getSubscriberProvisionName() {
return this.subscriberProvisionName;
}
public String getTopicName() {
return this.topicName;
}
public String getSubscriptionId() {
return this.subscriptionId;
}
public String getCredentialsFilePath() {
return this.credentialsFilePath;
}
public String getAvroSchemaFilePath() {
return this.avroSchemaFilePath;
}
public String getProtoFilePath() {
return this.protoFilePath;
}
public long firstFetchDelayMillis() {
return this.firstFetchDelayMillis;
}
public long fetchIntervalMillis() {
return this.fetchIntervalMillis;
}
public int pollTimeoutMillis() {
return this.pollTimeoutMillis;
}
public String contentTypeOverride() {
return this.contentTypeOverride;
}
public String contentEncodingOverride() {
return this.contentEncodingOverride;
}
public Value valueMolder() {
return this.valueMolder;
}
public Value relaySchema() {
return this.relaySchema;
}
@Override
public Output debug(Output output) {
output = output.write("PubSubIngressSettings").write('.').write("of").write('(').write(')')
.write('.').write("firstFetchDelayMillis").write('(').debug(this.firstFetchDelayMillis).write(')')
.write('.').write("fetchIntervalMillis").write('(').debug(this.fetchIntervalMillis).write(')')
.write('.').write("pollTimeoutMillis").write('(').debug(this.pollTimeoutMillis).write(')')
.write('.').write("subscriptionId").write('(').debug(this.subscriptionId).write(')')
.write('.').write("topicName").write('(').debug(this.topicName).write(')')
.write('.').write("subscriberProvisionName").write('(').debug(this.subscriberProvisionName).write(')')
.write('.').write("credentialsFilePath").write('(').debug(this.credentialsFilePath).write(')')
.write('.').write("contentTypeOverride").write('(').debug(this.contentTypeOverride).write(')')
.write('.').write("contentEncodingOverride").write('(').debug(this.contentEncodingOverride).write(')')
.write('.').write("valueMolder").write('(').debug(this.valueMolder).write(')')
.write('.').write("relaySchema").write('(').debug(this.relaySchema).write(')')
.write('.').write("avroSchemaFilePath").write('(').debug(this.avroSchemaFilePath).write(')')
.write('.').write("protoFilePath").write('(').debug(this.protoFilePath).write(')');
return output;
}
@Override
public String toString() {
return Format.debug(this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy