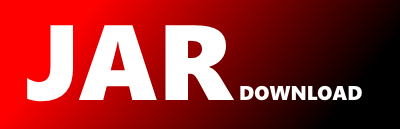
nstream.adapter.common.ext.RedisIngressSettings Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of nstream-adapter-common Show documentation
Show all versions of nstream-adapter-common Show documentation
General server-side data flow templates with Swim
// Copyright 2015-2024 Nstream, inc.
//
// Licensed under the Redis Source Available License 2.0 (RSALv2) Agreement;
// you may not use this file except in compliance with the License.
// You may obtain a copy of the License at
//
// https://redis.com/legal/rsalv2-agreement/
//
// Unless required by applicable law or agreed to in writing, software
// distributed under the License is distributed on an "AS IS" BASIS,
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
// See the License for the specific language governing permissions and
// limitations under the License.
package nstream.adapter.common.ext;
import java.util.Properties;
import nstream.adapter.common.AdapterSettings;
import swim.codec.Format;
import swim.codec.Output;
import swim.structure.Form;
import swim.structure.Kind;
import swim.structure.Record;
import swim.structure.Tag;
import swim.structure.Value;
import swim.util.Log;
/**
* Provides a concrete class to parse and store agent configuration
* used for Redis ingress by various ingesting agents and patches.
*/
@Tag("redisIngressSettings")
public class RedisIngressSettings implements AdapterSettings {
private static final RedisIngressJetTranslator JET_TRANSLATOR = new RedisIngressJetTranslator();
private final long firstFetchDelayMillis;
private final long fetchIntervalMillis;
private final String poolProvisionName;
private final String key;
private final String type;
private final String match;
private final String streams;
private final String groupName;
private final String consumer;
private final Integer block;
private final Integer count;
private final Value relaySchema;
public RedisIngressSettings(long firstFetchDelayMillis, long fetchIntervalMillis,
String poolProvisionName, String key,
String type, String match,
String streams, String groupName,
String consumer, Integer block,
Integer count, Value relaySchema) {
this.firstFetchDelayMillis = firstFetchDelayMillis;
this.fetchIntervalMillis = fetchIntervalMillis;
this.poolProvisionName = poolProvisionName;
this.key = key;
this.type = type;
this.match = match;
this.streams = streams;
this.groupName = groupName;
this.consumer = consumer;
this.block = block;
this.count = count;
this.relaySchema = relaySchema;
}
public RedisIngressSettings() {
this(2000L, 120000L, null, null, null, null, null, null, null, 0, null, null);
}
/**
* Initial delay on agent load before starting ingestion.
*
* @return the delay in ms
*/
public long firstFetchDelayMillis() {
return this.firstFetchDelayMillis;
}
/**
* Interval between fetches/polls in milliseconds.
*
* @return the interval in ms
*/
public long fetchIntervalMillis() {
return this.fetchIntervalMillis;
}
/**
* Name of Jedis pool provision to be used for ingestion.
*
* @return the client provision name
*/
public String poolProvisionName() {
return this.poolProvisionName;
}
/**
* The key of the collection to scan.
*
* @return the collection key
*/
public String key() {
return this.key;
}
/**
* The type of the scan to perform.
*
* @return the scan type
*/
public String type() {
return this.type;
}
/**
* The match pattern for the scan operation on the collection.
*
* @return the match pattern
*/
public String match() {
return this.match;
}
/**
* The streams to ingress and the starting IDs.
*
* @return the streams string
*/
public String streams() {
return this.streams;
}
/**
* The group name of the consumer group.
*
* @return consumer group name
*/
public String groupName() {
return this.groupName;
}
/**
* Consumer name within consumer group.
*
* @return consumer name
*/
public String consumer() {
return this.consumer;
}
/**
* The block value in ms.
*
* @return block (ms)
*/
public Integer block() {
return this.block;
}
/**
* The count value.
*
* @return count
*/
public Integer count() {
return this.count;
}
/**
* Relay schema definition.
*
* @return the relay schema
*/
public Value relaySchema() {
return this.relaySchema;
}
@Override
public Output debug(Output output) {
output = output.write("RedisIngressSettings").write('.').write("of").write('(').write(')')
.write('.').write("firstFetchDelay").write('(').debug(this.firstFetchDelayMillis).write(')')
.write('.').write("fetchInterval").write('(').debug(this.fetchIntervalMillis).write(')')
.write('.').write("poolProvisionName").write('(').debug(this.poolProvisionName).write(')')
.write('.').write("key").write('(').debug(this.key).write(')')
.write('.').write("type").write('(').debug(this.type).write(')')
.write('.').write("match").write('(').debug(this.match).write(')')
.write('.').write("streams").write('(').debug(this.streams).write(')')
.write('.').write("groupName").write('(').debug(this.groupName).write(')')
.write('.').write("consumer").write('(').debug(this.consumer).write(')')
.write('.').write("block").write('(').debug(this.block).write(')')
.write('.').write("count").write('(').debug(this.count).write(')')
.write('.').write("relaySchema").write('(').debug(this.relaySchema).write(')');
return output;
}
@Override
public String toString() {
return Format.debug(this);
}
@Kind
private static Form form;
private static final RedisIngressSettings DEFAULT = new RedisIngressSettings();
public static Form form() {
if (RedisIngressSettings.form == null) {
RedisIngressSettings.form = Form.forClass(RedisIngressSettings.class);
}
return RedisIngressSettings.form;
}
public static RedisIngressSettings defaultSettings() {
return RedisIngressSettings.DEFAULT;
}
public static Record moldFromProperties(Log log, Properties p) {
return JET_TRANSLATOR.moldFromProperties(log, p);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy