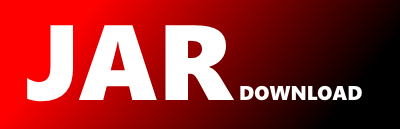
nstream.adapter.common.ingress.IngestorAgent Maven / Gradle / Ivy
Show all versions of nstream-adapter-common Show documentation
// Copyright 2015-2024 Nstream, inc.
//
// Licensed under the Redis Source Available License 2.0 (RSALv2) Agreement;
// you may not use this file except in compliance with the License.
// You may obtain a copy of the License at
//
// https://redis.com/legal/rsalv2-agreement/
//
// Unless required by applicable law or agreed to in writing, software
// distributed under the License is distributed on an "AS IS" BASIS,
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
// See the License for the specific language governing permissions and
// limitations under the License.
package nstream.adapter.common.ingress;
import nstream.adapter.common.AdapterSettings;
import nstream.adapter.common.NstreamAgent;
import nstream.adapter.common.schedule.DeferrableException;
import swim.structure.Value;
/**
* An abstract Web Agent that can pull or receive state from an external process.
* Requirements specific to the external process will be handled by the
* implementing subclasses.
* The {@link IngestorAgent} class encapsulates the settings object required to
* configure ingestion and provides methods to load these settings.
*
* Scheduling of asynchronous tasks can be achieved using the base
* {@link NstreamAgent}.
*
* @param the settings used to configure ingestion
* @param the type to be ingested from the external process
*/
public abstract class IngestorAgent extends NstreamAgent {
protected volatile S ingressSettings;
public IngestorAgent() {
}
/**
* Get the settings agent property and load value as settings.
*
* @param propName agent property name of the settings
* @see #loadSettings(Value)
*/
protected final void loadSettings(String propName) {
loadSettings(getProp(propName));
}
/**
* Parse and set the settings {@link Value} from agent configuration.
*
* @param prop agent settings value to be loaded
* @see #parseIngressSettings(Value)
* @see #loadSettings(S)
*/
protected final void loadSettings(Value prop) {
loadSettings(parseIngressSettings(prop));
}
/**
* Parse the agent settings {@link Value} and cast to a {@link S}
* object.
*
* @param prop agent settings value to be parsed to {@link S}
* @return settings object
*/
protected abstract S parseIngressSettings(Value prop);
/**
* Set the settings that have been loaded from agent configuration.
*
* @param ingressSettings settings loaded from agent configuration
*/
protected void loadSettings(S ingressSettings) {
this.ingressSettings = ingressSettings;
}
/**
* Ingest a {@link V} from an external process.
* Will likely convert the {@link V} object to a {@link Value} and
* relay using schema in settings.
*
* @param unstructured the object to be ingested
* @throws DeferrableException an exception that will be handled without aborting ingestion
* @throws RuntimeException an exception that will abort ingestion
*/
protected abstract void ingest(V unstructured) throws DeferrableException;
/**
* Prepare the agent for reception.
* Load settings object and schedule ingestion as required.
*/
protected abstract void stageReception();
/**
* Callback after successful staging.
*/
protected void didStageReception() {
// Hook
}
/**
* Cancel ingestion.
*/
protected void cancel() {
// Hook
}
/**
* Stage reception on agent start.
*/
@Override
public void didStart() {
info("didStart");
execute(() -> {
stageReception();
didStageReception();
});
}
/**
* Close any open resources on agent close.
*/
@Override
public void willStop() {
info("willStop");
cancel();
}
}