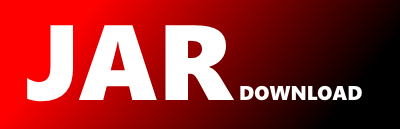
nstream.adapter.common.patches.HistoryPatch Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of nstream-adapter-common Show documentation
Show all versions of nstream-adapter-common Show documentation
General server-side data flow templates with Swim
// Copyright 2015-2024 Nstream, inc.
//
// Licensed under the Redis Source Available License 2.0 (RSALv2) Agreement;
// you may not use this file except in compliance with the License.
// You may obtain a copy of the License at
//
// https://redis.com/legal/rsalv2-agreement/
//
// Unless required by applicable law or agreed to in writing, software
// distributed under the License is distributed on an "AS IS" BASIS,
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
// See the License for the specific language governing permissions and
// limitations under the License.
package nstream.adapter.common.patches;
import java.util.Map;
import nstream.adapter.common.NstreamAgent;
import swim.api.SwimLane;
import swim.api.lane.CommandLane;
import swim.api.lane.MapLane;
import swim.structure.Selector;
import swim.structure.Value;
public class HistoryPatch extends NstreamAgent {
public HistoryPatch() {
}
protected long extractEpochMillisFromEvent(Value event) {
final Value extractDef = getProp("extractEpochMillisFromEvent");
if (extractDef.isDistinct() && extractDef instanceof Selector) {
return extractDef.evaluate(event).longValue();
} else {
return defaultExtractEpochMillisFromEvent(event);
}
}
protected long defaultExtractEpochMillisFromEvent(Value event) {
return System.currentTimeMillis();
}
protected Value extractValueFromEvent(Value event) {
final Value extractDef = getProp("extractValueFromEvent");
if (extractDef.isDistinct() && extractDef instanceof Selector) {
return extractDef.evaluate(event).toValue();
} else {
return defaultExtractValueFromEvent(event);
}
}
protected Value defaultExtractValueFromEvent(Value event) {
return event;
}
protected boolean shouldDropOldEntry(Map.Entry entry) {
return false;
}
protected int maxHistorySize() {
return getProp("maxHistorySize").intValue(Integer.MAX_VALUE);
}
protected void clearUnwantedHistory() {
int dropCount = 0;
for (Map.Entry entry : this.history.entrySet()) {
while (shouldDropOldEntry(entry)) {
dropCount++;
}
}
dropCount = Math.max(this.history.size() - maxHistorySize(), dropCount);
this.history.drop(dropCount);
}
@SwimLane("history")
protected MapLane history = this.mapLane()
.didUpdate((k, n, o) -> {
trace("(HistoryPatch) " + nodeUri() + ".history#didUpdate(): "
+ "key=" + k + ", newValue=" + n + ", oldValue=" + o);
clearUnwantedHistory();
})
.didDrop(lower -> {
info("(HistoryPatch) " + nodeUri() + ".history#didDrop(): "
+ "lower=" + lower);
});
@SwimLane("addEvent")
protected CommandLane addEvent = this.commandLane()
.onCommand(v -> {
trace("(HistoryPatch) " + nodeUri()
+ ".addEvent#onCommand(): value=" + v);
this.history.put(extractEpochMillisFromEvent(v), extractValueFromEvent(v));
});
@Override
public void didStart() {
info("(HistoryPatch) " + nodeUri() + ": didStart");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy