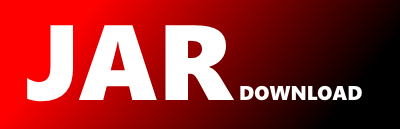
nstream.adapter.common.provision.AbstractProvision Maven / Gradle / Ivy
Show all versions of nstream-adapter-common Show documentation
// Copyright 2015-2024 Nstream, inc.
//
// Licensed under the Redis Source Available License 2.0 (RSALv2) Agreement;
// you may not use this file except in compliance with the License.
// You may obtain a copy of the License at
//
// https://redis.com/legal/rsalv2-agreement/
//
// Unless required by applicable law or agreed to in writing, software
// distributed under the License is distributed on an "AS IS" BASIS,
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
// See the License for the specific language governing permissions and
// limitations under the License.
package nstream.adapter.common.provision;
import java.util.Properties;
import swim.util.Log;
/**
* A resource that is utilized by but possibly loaded independently of a Swim
* server.
*
* Every {@code Provision} must be configurable by some {@link
* java.util.Properties} object.
*
* @param the type of resource wrapped by this {@code Provision}
*/
public abstract class AbstractProvision {
protected T value;
private Properties def;
protected AbstractProvision() {
}
/**
* The {@code Properties} representing the structure of this {@code Provision}
* ({@code null} indicates staleness).
*/
public final Properties def() {
if (this.def == null) {
return null;
} else {
final Properties clone = new Properties();
clone.putAll(this.def);
return clone;
}
}
public final boolean isLive() {
return this.def != null;
}
public final void load(Log log, Properties properties) {
if (this.def != null) {
throw new IllegalStateException("load() invocations without intermediary unload() are forbidden");
}
if (properties == null) {
throw new IllegalArgumentException("Cannot invoke load() with null properties");
} else {
loadValue(log, properties);
this.def = properties;
}
}
protected abstract void loadValue(Log log, Properties properties);
public final T value() {
return this.value;
}
public final void unload(Log log) {
if (this.def != null) {
try {
unloadValue(log);
} catch (RuntimeException e) {
e.printStackTrace();
log.warn("Possible resource leak: incurred exception while unloading Provision value, but "
+ this.getClass().getName() + " was unloaded regardless");
} finally {
this.def = null;
}
}
}
protected abstract void unloadValue(Log log);
}