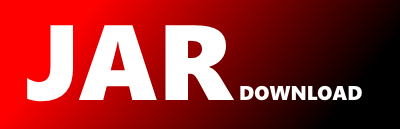
nstream.adapter.common.relay.If Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of nstream-adapter-common Show documentation
Show all versions of nstream-adapter-common Show documentation
General server-side data flow templates with Swim
// Copyright 2015-2024 Nstream, inc.
//
// Licensed under the Redis Source Available License 2.0 (RSALv2) Agreement;
// you may not use this file except in compliance with the License.
// You may obtain a copy of the License at
//
// https://redis.com/legal/rsalv2-agreement/
//
// Unless required by applicable law or agreed to in writing, software
// distributed under the License is distributed on an "AS IS" BASIS,
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
// See the License for the specific language governing permissions and
// limitations under the License.
package nstream.adapter.common.relay;
import swim.api.agent.AgentContext;
import swim.structure.Bool;
import swim.structure.Item;
import swim.structure.Value;
import swim.util.Log;
/**
* {@code Directive} to conditionally invoke logic.
*/
public final class If extends Directive {
private final Value condition;
private final Value conditionLiteral;
private final Value then;
private final Value thenLiteral;
private final Value orElse;
private final Value orElseLiteral;
public If(Directive> parent, AgentContext context, Log log, Item scope,
Value condition, Value conditionLiteral,
Value then, Value thenLiteral,
Value orElse, Value orElseLiteral) {
super(parent, context, log, scope);
this.condition = condition;
this.conditionLiteral = conditionLiteral;
this.then = then;
this.thenLiteral = thenLiteral;
this.orElse = orElse;
this.orElseLiteral = orElseLiteral;
}
static If fromSchema(Directive> parent, AgentContext context, Log log, Item scope,
Value schema) {
return new If(parent, context, log, scope,
schema.get("condition"), schema.get("conditionLiteral"),
schema.get("then"), schema.get("thenLiteral"),
schema.get("else"), schema.get("elseLiteral"));
}
@Override
public Value evaluate() throws InterpretException {
final Value condVal = DslInterpret.evaluateHintedValue(this, this.condition, this.conditionLiteral, "condition");
if (condVal == null || !condVal.isDefined()) {
throw new InterpretException("Evaluated undefined condition " + condVal);
}
final boolean cond;
try {
final Bool mayThrow = (Bool) condVal;
cond = mayThrow.booleanValue();
} catch (RuntimeException e) {
throw new InterpretException("Evaluated condition " + condVal + " does not yield boolean", e);
}
return cond ? DslInterpret.evaluateHintedValue(this, this.then, this.thenLiteral, "then")
: DslInterpret.evaluateHintedValue(this, this.orElse, this.orElseLiteral, "else");
}
@Override
public String toString() {
return "If{"
+ hintOrLiteralToString(this.condition, this.conditionLiteral, "condition", true)
+ hintOrLiteralToString(this.then, this.thenLiteral, "then")
+ hintOrLiteralToString(this.orElse, this.orElseLiteral, "else")
+ '}';
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy