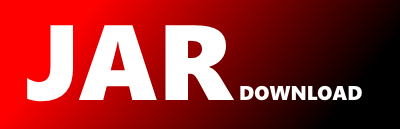
nstream.adapter.common.relay.Substring Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of nstream-adapter-common Show documentation
Show all versions of nstream-adapter-common Show documentation
General server-side data flow templates with Swim
// Copyright 2015-2024 Nstream, inc.
//
// Licensed under the Redis Source Available License 2.0 (RSALv2) Agreement;
// you may not use this file except in compliance with the License.
// You may obtain a copy of the License at
//
// https://redis.com/legal/rsalv2-agreement/
//
// Unless required by applicable law or agreed to in writing, software
// distributed under the License is distributed on an "AS IS" BASIS,
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
// See the License for the specific language governing permissions and
// limitations under the License.
package nstream.adapter.common.relay;
import swim.api.agent.AgentContext;
import swim.structure.Item;
import swim.structure.Text;
import swim.structure.Value;
import swim.util.Log;
// TODO: consider making this capable of accepting dynamic arguments
// (easy enough to do, but is it actually useful?)
public final class Substring extends Directive {
private final int lower;
private final int upper;
public Substring(Directive> parent, AgentContext context, Log log, Item scope,
int lower, int upper) {
super(parent, context, log, scope);
this.lower = lower;
this.upper = upper;
}
static Substring fromSchema(Directive> parent, AgentContext context, Log log, Item scope,
Value schema) {
return new Substring(parent, context, log, scope,
schema.get("lower").intValue(-1),
schema.get("upper").intValue(-1));
}
@Override
public Text evaluate() throws InterpretException {
final String str;
try {
str = this.scope.stringValue();
} catch (RuntimeException e) {
final String msg = "scope " + this.scope + " does not have a String representation;"
+ " consider adding a coercion-type Directive";
throw new InterpretException(msg, e);
}
try {
if (this.lower < 0) {
if (this.upper < 0) {
return Text.from(str);
} else {
return Text.from(str.substring(0, this.upper));
}
} else if (this.upper < 0) {
return Text.from(str.substring(this.lower));
} else {
return Text.from(str.substring(this.lower, this.upper));
}
} catch (RuntimeException e) {
final String msg = "Unsuitable bounds [" + Math.max(this.lower, 0) + ","
+ (this.upper < 0 ? str.length() : this.upper) + ") provided for scope " + str;
throw new InterpretException(msg, e);
}
}
@Override
public String toString() {
return "Substring{"
+ "scope=" + this.scope
+ (this.lower < 0 ? "" : ", lower=" + this.lower)
+ (this.upper < 0 ? "" : ", upper=" + this.upper)
+ '}';
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy