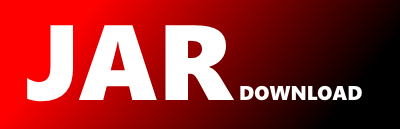
nstream.adapter.http.HttpIngestingAgent Maven / Gradle / Ivy
// Copyright 2015-2024 Nstream, inc.
//
// Licensed under the Redis Source Available License 2.0 (RSALv2) Agreement;
// you may not use this file except in compliance with the License.
// You may obtain a copy of the License at
//
// https://redis.com/legal/rsalv2-agreement/
//
// Unless required by applicable law or agreed to in writing, software
// distributed under the License is distributed on an "AS IS" BASIS,
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
// See the License for the specific language governing permissions and
// limitations under the License.
package nstream.adapter.http;
import java.io.IOException;
import java.io.InputStream;
import java.net.URISyntaxException;
import java.net.http.HttpClient;
import java.net.http.HttpRequest;
import java.net.http.HttpResponse;
import nstream.adapter.common.ext.HttpIngressSettings;
import nstream.adapter.common.ingress.ExchangeRelay;
import nstream.adapter.common.ingress.IngestorMetricsAgent;
import nstream.adapter.common.schedule.DeferrableException;
import swim.concurrent.TimerRef;
import swim.structure.Value;
public abstract class HttpIngestingAgent
extends IngestorMetricsAgent>
implements ExchangeRelay> {
protected TimerRef exchangeTimer;
public HttpIngestingAgent() {
}
protected TimerRef exchangeTimer() {
return this.exchangeTimer;
}
protected HttpClient httpClient() {
return HttpAdapterUtils.defaultHttpClient();
}
protected void fetchAndRelay() throws DeferrableException {
final HttpRequest request = prepareRequest(this.ingressSettings);
final HttpResponse response = executeRequest(request);
relayResponse(response);
}
@Override
public HttpRequest prepareRequest(HttpIngressSettings ingressSettings) {
final HttpRequest request;
try {
request = HttpAdapterUtils.buildHttpRequest("GET",
ingressSettings.endpointUrl(), ingressSettings.headers(),
HttpRequest.BodyPublishers.noBody(), ingressSettings.timeoutMillis());
} catch (URISyntaxException e) {
// Unrecoverable; StageService should cancel recurring task
throw new RuntimeException(e);
}
return request;
}
@Override
public HttpResponse executeRequest(HttpRequest request)
throws DeferrableException {
final HttpResponse response;
try {
response = HttpAdapterUtils.executeHttpRequest(httpClient(), request);
} catch (IOException | InterruptedException e) {
// If this is a recurring task, next iteration may have better luck
throw new DeferrableException("Failed to execute request (timer remains active)", e);
}
return response;
}
protected boolean responseIsHealthy(HttpResponse> response) {
return response.statusCode() / 100 == 2;
}
@Override
public void relayResponse(HttpResponse response) {
ingestOrContinue(response);
}
@Override
protected HttpIngressSettings parseIngressSettings(Value prop) {
return HttpAdapterUtils.ingressSettingsFromProp(prop);
}
@Override
protected void stageReception() {
loadSettings("httpIngressConf");
this.exchangeTimer = scheduleAtFixedRate(this::exchangeTimer,
this.ingressSettings.firstPollDelayMillis(), this.ingressSettings.pollIntervalMillis(),
this::fetchAndRelay);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy