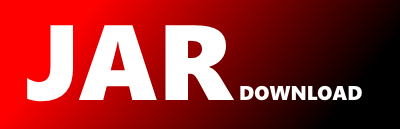
nstream.adapter.http.HttpIngestingPatch Maven / Gradle / Ivy
// Copyright 2015-2024 Nstream, inc.
//
// Licensed under the Redis Source Available License 2.0 (RSALv2) Agreement;
// you may not use this file except in compliance with the License.
// You may obtain a copy of the License at
//
// https://redis.com/legal/rsalv2-agreement/
//
// Unless required by applicable law or agreed to in writing, software
// distributed under the License is distributed on an "AS IS" BASIS,
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
// See the License for the specific language governing permissions and
// limitations under the License.
package nstream.adapter.http;
import java.io.IOException;
import java.io.InputStream;
import java.net.http.HttpResponse;
import nstream.adapter.common.AdapterUtils;
import nstream.adapter.common.ext.HttpIngressSettings;
import nstream.adapter.common.schedule.DeferrableException;
import swim.http.MediaType;
import swim.structure.Value;
/**
* A no-code version of {@link HttpIngestingAgent} that defines its ingestion
* logic through {@link HttpIngressSettings#relaySchema()}.
*/
public class HttpIngestingPatch extends HttpIngestingAgent {
public HttpIngestingPatch() {
}
@Override
public void ingest(HttpResponse response)
throws DeferrableException {
if (responseIsHealthy(response)) {
final Value v = assembleResponse(response);
ingestStructure(v);
} else {
throw new DeferrableException(nodeUri() + ": response saw unhealthy status code " + response.statusCode()
+ " (timer remains active). Headers: " + response.headers());
}
}
protected Value assembleResponse(HttpResponse response)
throws DeferrableException {
final String contentEncoding = HttpAdapterUtils.contentEncoding(this.ingressSettings, response.headers());
final InputStream bodyStream;
try {
bodyStream = HttpAdapterUtils.responseBodyStream(response, contentEncoding);
} catch (IOException e) {
// If this is a recurring task, next iteration may have better luck
throw new DeferrableException("Failed to decode response (timer remains active)", e);
}
final MediaType contentType = HttpAdapterUtils.contentType(this.ingressSettings, response.headers());
try {
return HttpAdapterUtils.responseBodyStructure(bodyStream, contentType);
} catch (Exception e) {
error(e.getMessage());
// If this is a recurring task, next iteration may have better luck
throw new DeferrableException("Failed to assemble response (timer remains active)", e);
}
}
protected void ingestStructure(Value responseStructure)
throws DeferrableException {
AdapterUtils.ingressDslRelay(this.ingressSettings.relaySchema(),
agentContext(), responseStructure);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy