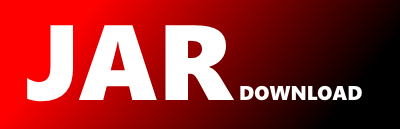
nstream.adapter.jms.ConnectionFactoryProvision Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of nstream-adapter-jms Show documentation
Show all versions of nstream-adapter-jms Show documentation
Templates for consuming from and producing to JMS with Swim
// Copyright 2015-2024 Nstream, inc.
//
// Licensed under the Redis Source Available License 2.0 (RSALv2) Agreement;
// you may not use this file except in compliance with the License.
// You may obtain a copy of the License at
//
// https://redis.com/legal/rsalv2-agreement/
//
// Unless required by applicable law or agreed to in writing, software
// distributed under the License is distributed on an "AS IS" BASIS,
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
// See the License for the specific language governing permissions and
// limitations under the License.
package nstream.adapter.jms;
import java.lang.reflect.InvocationTargetException;
import java.lang.reflect.Method;
import java.util.Properties;
import javax.jms.ConnectionFactory;
import nstream.adapter.common.provision.AbstractProvision;
import swim.util.Log;
/**
* Provision providing a JMS {@link ConnectionFactory} object.
* Required properties:
*
* - connectionFactoryClass - class name of the {@link ConnectionFactory},
* will be specific to the JMS provider used.
*
*
*
* Other properties will depend on the JMS provider and implementation of
* {@link ConnectionFactory} being used.
* Properties of the {@link ConnectionFactory} can also be set here
* assuming the implementation follows the Java Bean naming convention
* and has a no-arg constructor.
*/
public class ConnectionFactoryProvision extends AbstractProvision {
public ConnectionFactoryProvision() {
}
@Override
public void loadValue(Log log, Properties properties) {
final Class extends ConnectionFactory> clazz;
try {
clazz = loadConnectionFactoryClass(properties);
} catch (RuntimeException e) {
log.error("Did not load ConnectionFactoryProvision due to invalid connectionFactoryClass");
throw new RuntimeException("Failed to process connectionFactoryClass", e);
}
final ConnectionFactory target;
try {
target = initConnectionFactory(clazz);
loadConnectionFactory(target, clazz, properties, log);
} catch (RuntimeException e) {
log.error("Did not load ConnectionFactoryProvision due to error initializing connectionFactoryClass instance");
throw new RuntimeException("Failed to initialize connectionFactoryClass instance", e);
}
this.value = target;
}
@SuppressWarnings("unchecked")
private Class extends ConnectionFactory> loadConnectionFactoryClass(final Properties properties) {
final String className = properties.getProperty("connectionFactoryClass");
if (className == null) {
throw new IllegalArgumentException("connectionFactoryClass property not specified");
}
try {
return (Class extends ConnectionFactory>) Class.forName(className);
} catch (ClassNotFoundException | ClassCastException classNotFoundException) {
throw new IllegalArgumentException("connectionFactoryClass not found: " + className, classNotFoundException);
}
}
private ConnectionFactory initConnectionFactory(final Class extends ConnectionFactory> clazz) {
try {
return clazz.getDeclaredConstructor().newInstance();
} catch (NoSuchMethodException | InvocationTargetException | IllegalAccessException | InstantiationException exception) {
throw new IllegalArgumentException(clazz.getName() + " does not have a no-arg constructor", exception);
}
}
private void loadConnectionFactory(final ConnectionFactory target, final Class extends ConnectionFactory> clazz, final Properties properties, final Log log) {
try {
for (final String propertyName : properties.stringPropertyNames()) {
if ("connectionFactoryClass".equals(propertyName)) {
continue;
}
final Method method = findPropertySetterMethod(clazz, propertyName);
if (method == null) {
log.warn("Setter method not found on ConnectionFactory for property: " + propertyName);
continue;
}
loadProperty(target, method, properties.getProperty(propertyName));
}
} catch (IllegalAccessException | InvocationTargetException | IllegalArgumentException | UnsupportedOperationException exception) {
throw new IllegalArgumentException("ConnectionFactoryProvision failed setting properties for class: " + clazz.getName(), exception);
}
}
private Method findPropertySetterMethod(final Class extends ConnectionFactory> clazz, final String propertyName) {
final String setterName = "set" + Character.toUpperCase(propertyName.charAt(0)) + propertyName.substring(1);
final Method[] methods = clazz.getMethods();
for (final Method method : methods) {
if (setterName.equals(method.getName()) && method.getParameterTypes().length == 1) {
return method;
}
}
return null;
}
private void loadProperty(final ConnectionFactory target, final Method setter, final String value)
throws IllegalAccessException, InvocationTargetException {
final Class> type = setter.getParameterTypes()[0];
if (type == String.class) {
setter.invoke(target, value);
} else if (type == Integer.TYPE) {
setter.invoke(target, Integer.parseInt(value));
} else if (type == Long.TYPE) {
setter.invoke(target, Long.parseLong(value));
} else if (type == Boolean.TYPE) {
setter.invoke(target, Boolean.parseBoolean(value));
} else {
throw new UnsupportedOperationException("Unsupported property type: " + type.getName());
}
}
@Override
public void unloadValue(Log log) {
this.value = null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy