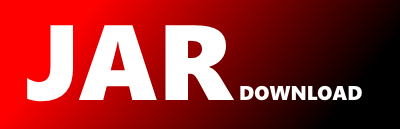
nstream.persist.api.kv.IterationStatus Maven / Gradle / Ivy
// Copyright 2015-2024 Nstream, inc.
//
// Licensed under the Apache License, Version 2.0 (the "License");
// you may not use this file except in compliance with the License.
// You may obtain a copy of the License at
//
// http://www.apache.org/licenses/LICENSE-2.0
//
// Unless required by applicable law or agreed to in writing, software
// distributed under the License is distributed on an "AS IS" BASIS,
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
// See the License for the specific language governing permissions and
// limitations under the License.
package nstream.persist.api.kv;
import java.util.OptionalInt;
/**
* The status returned from an attempt to read the state of a {@link MapIterator} into provided buffers.
*/
@SuppressWarnings("unused")
public class IterationStatus {
private static final byte GOOD = 0x00;
private static final byte KEY_OVERFLOW = 0x01;
private static final byte VALUE_OVERFLOW = 0x10;
private static final int UNKNOWN = -1;
private byte flags;
private int keyRequired;
private int valueRequired;
public IterationStatus() {
this.flags = GOOD;
this.keyRequired = 0;
this.valueRequired = 0;
}
/**
* Reset the status to a good state.
*/
public void reset() {
this.flags = GOOD;
this.keyRequired = 0;
this.valueRequired = 0;
}
/**
* Indicate that the key buffer overflowed.
* @param required The required number of bytes for the buffer.
*/
public void setKeyOverflow(int required) {
if (required <= 0) {
throw new IllegalArgumentException("Required buffer size must be positive.");
}
this.flags |= KEY_OVERFLOW;
this.keyRequired = required;
}
/**
* Indicate that the key buffer overflowed without specifying the required number of bytes.
*/
public void setKeyOverflow() {
this.flags |= KEY_OVERFLOW;
this.keyRequired = UNKNOWN;
}
/**
* Indicate that the value buffer overflowed.
* @param required The required number of bytes for the buffer.
*/
public void setValueOverflow(int required) {
if (required <= 0) {
throw new IllegalArgumentException("Required buffer size must be positive.");
}
this.flags |= VALUE_OVERFLOW;
this.valueRequired = required;
}
/**
* Indicate that the value buffer overflowed without specifying the required number of bytes.
*/
public void setValueOverflow() {
this.flags |= VALUE_OVERFLOW;
this.valueRequired = UNKNOWN;
}
/**
* @return Get the overall result of the operation.
*/
public IoResult getResult() {
if (this.flags == GOOD) {
return IoResult.Success;
} else {
return IoResult.BufferOverflow;
}
}
/**
* @return Following a failed read, get the number of bytes required for the key buffer, if known.
*/
public OptionalInt getKeyRequired() {
if (this.keyRequired <= 0) {
return OptionalInt.empty();
} else {
return OptionalInt.of(this.keyRequired);
}
}
/**
* @return Following a failed read, get the number of bytes required for the value buffer, if known.
*/
public OptionalInt getValueRequired() {
if (this.valueRequired <= 0) {
return OptionalInt.empty();
} else {
return OptionalInt.of(this.valueRequired);
}
}
/**
* @return Whether the key buffer overflowed.
*/
public boolean keyOverflowed() {
return (this.flags & KEY_OVERFLOW) != 0;
}
/**
* @return Whether the value buffer overflowed.
*/
public boolean valueOverflowed() {
return (this.flags & VALUE_OVERFLOW) != 0;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy