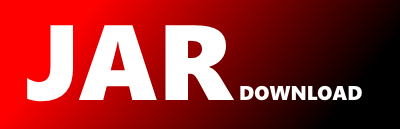
nstream.persist.api.kv.KeyValueBuffers Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of nstream-persist-api Show documentation
Show all versions of nstream-persist-api Show documentation
API for web agent persistence support
The newest version!
// Copyright 2015-2024 Nstream, inc.
//
// Licensed under the Apache License, Version 2.0 (the "License");
// you may not use this file except in compliance with the License.
// You may obtain a copy of the License at
//
// http://www.apache.org/licenses/LICENSE-2.0
//
// Unless required by applicable law or agreed to in writing, software
// distributed under the License is distributed on an "AS IS" BASIS,
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
// See the License for the specific language governing permissions and
// limitations under the License.
package nstream.persist.api.kv;
import java.nio.ByteBuffer;
/**
* Wrapper around a pair of buffers to read the current key-value pair from a {@link MapIterator}.
*/
public class KeyValueBuffers {
private ByteBuffer keyBuffer;
private ByteBuffer valueBuffer;
/**
* @param keyBuffer Initial key buffer.
* @param valueBuffer Initial value buffer.
*/
public KeyValueBuffers(ByteBuffer keyBuffer, ByteBuffer valueBuffer) {
if (keyBuffer == null || valueBuffer == null) {
throw new NullPointerException("Buffers must be non-null.");
}
this.keyBuffer = keyBuffer;
this.valueBuffer = valueBuffer;
}
public ByteBuffer getKeyBuffer() {
return this.keyBuffer;
}
public ByteBuffer getValueBuffer() {
return this.valueBuffer;
}
public void setKeyBuffer(ByteBuffer buffer) {
if (buffer == null) {
throw new NullPointerException("Buffers must no non-null.");
}
this.keyBuffer = buffer;
}
public void setValueBuffer(ByteBuffer buffer) {
if (buffer == null) {
throw new NullPointerException("Buffers must no non-null.");
}
this.valueBuffer = buffer;
}
private static final int MIN_BUFFER = 8;
private static final int MULTIPLE = 2;
private static ByteBuffer grow(ByteBuffer buf, int required) {
final var newSize = Integer.max(Integer.max(MIN_BUFFER, required), buf.capacity() * MULTIPLE);
if (buf.isDirect()) {
return ByteBuffer.allocateDirect(newSize);
} else {
return ByteBuffer.allocate(newSize);
}
}
/**
* Grow the key buffer to be able to accept the specified number of bytes.
* @param n The number of bytes.
*/
public void growKey(int n) {
this.keyBuffer = grow(this.keyBuffer, n);
}
/**
* Grow the key buffer by a default multiplier (when the number of bytes required is unknown).
*/
public void growKey() {
this.growKey(-1);
}
/**
* Grow the value buffer to be able to accept the specified number of bytes.
* @param n The number of bytes.
*/
public void growValue(int n) {
this.valueBuffer = grow(this.valueBuffer, n);
}
/**
* Grow the value buffer by a default multiplier (when the number of bytes required is unknown).
*/
public void growValue() {
this.growValue(-1);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy