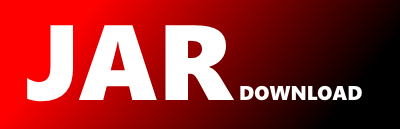
nstream.persist.kv.KvAdapterConfig Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of nstream-persist-kv Show documentation
Show all versions of nstream-persist-kv Show documentation
Adapter for persistence implementations based on key-value stores
The newest version!
// Copyright 2015-2024 Nstream, inc.
//
// Licensed under the Apache License, Version 2.0 (the "License");
// you may not use this file except in compliance with the License.
// You may obtain a copy of the License at
//
// http://www.apache.org/licenses/LICENSE-2.0
//
// Unless required by applicable law or agreed to in writing, software
// distributed under the License is distributed on an "AS IS" BASIS,
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
// See the License for the specific language governing permissions and
// limitations under the License.
package nstream.persist.kv;
import java.util.Objects;
import java.util.Optional;
import java.util.OptionalLong;
import nstream.persist.api.kv.KvStoreFactory;
import swim.structure.Form;
import swim.structure.Kind;
import swim.structure.Value;
/**
* Configuration parameters for the {@link KvAdapter}.
*/
class KvAdapterConfig {
private final long minCommitInterval;
private final long maxCommitInterval;
private final long minCommitSize;
private final long shutdownTimeout;
private final long maxBatchSize;
private final String implName;
private final Value parameters;
KvAdapterConfig(long minCommitInterval,
long maxCommitInterval,
long minCommitSize,
long shutdownTimeout,
long maxBatchSize,
String implName,
Value parameters) {
if (minCommitInterval <= 0) {
throw new IllegalArgumentException("Minimum commit interval must be positive.");
}
if (maxCommitInterval > 0 && maxCommitInterval < minCommitInterval) {
throw new IllegalArgumentException("Minimum commit interval cannot be larger than maximum commit interval.");
}
if (minCommitSize <= 0) {
throw new IllegalArgumentException("Minimum commit size must be positive.");
}
if (shutdownTimeout <= 0) {
throw new IllegalArgumentException("Shutdown timeout must be positive.");
}
if (maxBatchSize <= 0) {
throw new IllegalArgumentException("Maximum batch size must be positive.");
}
this.minCommitInterval = minCommitInterval;
if (maxCommitInterval < 0) {
this.maxCommitInterval = -1;
} else {
this.maxCommitInterval = maxCommitInterval;
}
this.minCommitSize = minCommitSize;
this.shutdownTimeout = shutdownTimeout;
this.maxBatchSize = maxBatchSize;
this.implName = implName;
this.parameters = parameters;
}
/**
* @return Minimum time between commits to the database, in ms.
*/
public long getMinCommitInterval() {
return this.minCommitInterval;
}
/**
* @return Maximum time between commits to the database (disregarding minimum commit size), in ms.
*/
public OptionalLong getMaxCommitInterval() {
if (this.maxCommitInterval < 0) {
return OptionalLong.empty();
} else {
return OptionalLong.of(this.maxCommitInterval);
}
}
/**
* @return The minimum size of a commit to the database in bytes.
*/
public long getMinCommitSize() {
return this.minCommitSize;
}
/**
* @return Timeout for the the store task shutdown process in ms.
*/
public long getShutdownTimeout() {
return this.shutdownTimeout;
}
/**
* @return The maximum size of single commit to the database in bytes..
*/
public long getMaxBatchSize() {
return this.maxBatchSize;
}
/**
* The name of the implementation of {@link KvStoreFactory} to use when more than one is present
* on the module path. If this is not specified, it will be assumed that the choice is unambiguous.
* @return The name of the implementation (as returned by {@link KvStoreFactory#getName()}).
*/
public Optional getImplName() {
return Optional.ofNullable(this.implName);
}
/**
* @return Configuration parameters to be passed to the {@link KvStoreFactory}.
*/
public Optional getParameters() {
return Optional.ofNullable(this.parameters);
}
@Kind
public static final Form FORM = new KvAdapterConfigForm();
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
final var that = (KvAdapterConfig) o;
return this.minCommitInterval == that.minCommitInterval && this.maxCommitInterval == that.maxCommitInterval && this.minCommitSize == that.minCommitSize && this.shutdownTimeout == that.shutdownTimeout && this.maxBatchSize == that.maxBatchSize && Objects.equals(this.implName, that.implName) && Objects.equals(this.parameters, that.parameters);
}
@Override
public int hashCode() {
return Objects.hash(this.minCommitInterval, this.maxCommitInterval, this.minCommitSize, this.shutdownTimeout, this.maxBatchSize, this.implName, this.parameters);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy