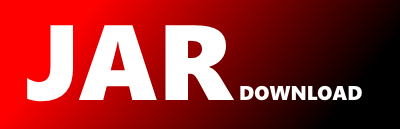
io.nuls.core.thread.commom.ThreadCache Maven / Gradle / Ivy
package io.nuls.core.thread.commom;
import java.util.*;
import java.util.concurrent.ThreadPoolExecutor;
/**
* @author tag
*/
public class ThreadCache {
private static ThreadCache INSTANCE = null;
/**
* key : poolName
* value: pool
*/
private final Map POOL_EXECUTOR_MAP = new HashMap<>();
/**
* key : threadName
* value: thread
*/
private final Map THREAD_MAP = new HashMap<>();
/**
* key : poolName
* value: Set
*/
private final Map> POOL_THREAD_MAP = new HashMap<>();
private ThreadCache() {
}
public static final ThreadCache getInstance() {
if (INSTANCE == null) {
synchronized (ThreadCache.class) {
if (INSTANCE == null) {
INSTANCE = new ThreadCache();
}
}
}
return INSTANCE;
}
public final void putPool(String poolName, ThreadPoolExecutor pool) {
POOL_EXECUTOR_MAP.put(poolName, pool);
}
public final void putThread(String poolName, String threadName, Thread thread) {
THREAD_MAP.put(threadName, thread);
Set set = POOL_THREAD_MAP.get(poolName);
if (null == set) {
set = new HashSet<>();
}
set.add(threadName);
POOL_THREAD_MAP.put(poolName, set);
}
public final Thread getThread(String threadName) {
return THREAD_MAP.get(threadName);
}
public final ThreadPoolExecutor getPool(String poolName) {
return POOL_EXECUTOR_MAP.get(poolName);
}
public final List getThreadList() {
return new ArrayList(THREAD_MAP.values());
}
public final List getThreadList(String poolName) {
Set set = POOL_THREAD_MAP.get(poolName);
if (null == set) {
return null;
}
List list = new ArrayList<>();
for (String threadName : set) {
list.add(THREAD_MAP.get(threadName));
}
return list;
}
public final List getPoolList() {
return new ArrayList<>(POOL_EXECUTOR_MAP.values());
}
public void removeAllThread() {
THREAD_MAP.clear();
POOL_EXECUTOR_MAP.clear();
POOL_THREAD_MAP.clear();
}
public void removePoolThread(String poolName) {
POOL_THREAD_MAP.remove(poolName);
}
public void removeThread(String threadName) {
THREAD_MAP.remove(threadName);
for (Set tset : POOL_THREAD_MAP.values()) {
tset.remove(threadName);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy