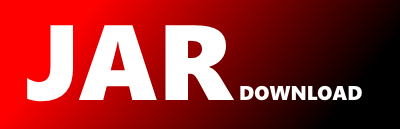
io.numaproj.numaflow.sideinput.v1.SideInputGrpc Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of numaflow-java Show documentation
Show all versions of numaflow-java Show documentation
SDK to implement Numaflow Source or User Defined Functions or Sinks in Java.
package io.numaproj.numaflow.sideinput.v1;
import static io.grpc.MethodDescriptor.generateFullMethodName;
/**
*
* SideInput is the gRPC service for Side Inputs.
* It is used to propagate changes in the values of the provided Side Inputs
* which allows access to slow updated data or configuration without needing to retrieve
* it during each message processing.
* Through this service we should should be able to:-
* 1) Invoke retrieval request for a single Side Input parameter, which in turn should
* check for updates and return its latest value.
* 2) Provide a health check endpoint to indicate whether the service is ready to be used.
*
*/
@javax.annotation.Generated(
value = "by gRPC proto compiler (version 1.50.2)",
comments = "Source: sideinput/v1/sideinput.proto")
@io.grpc.stub.annotations.GrpcGenerated
public final class SideInputGrpc {
private SideInputGrpc() {}
public static final String SERVICE_NAME = "sideinput.v1.SideInput";
// Static method descriptors that strictly reflect the proto.
private static volatile io.grpc.MethodDescriptor getRetrieveSideInputMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "RetrieveSideInput",
requestType = com.google.protobuf.Empty.class,
responseType = io.numaproj.numaflow.sideinput.v1.Sideinput.SideInputResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getRetrieveSideInputMethod() {
io.grpc.MethodDescriptor getRetrieveSideInputMethod;
if ((getRetrieveSideInputMethod = SideInputGrpc.getRetrieveSideInputMethod) == null) {
synchronized (SideInputGrpc.class) {
if ((getRetrieveSideInputMethod = SideInputGrpc.getRetrieveSideInputMethod) == null) {
SideInputGrpc.getRetrieveSideInputMethod = getRetrieveSideInputMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "RetrieveSideInput"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.google.protobuf.Empty.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
io.numaproj.numaflow.sideinput.v1.Sideinput.SideInputResponse.getDefaultInstance()))
.setSchemaDescriptor(new SideInputMethodDescriptorSupplier("RetrieveSideInput"))
.build();
}
}
}
return getRetrieveSideInputMethod;
}
private static volatile io.grpc.MethodDescriptor getIsReadyMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "IsReady",
requestType = com.google.protobuf.Empty.class,
responseType = io.numaproj.numaflow.sideinput.v1.Sideinput.ReadyResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getIsReadyMethod() {
io.grpc.MethodDescriptor getIsReadyMethod;
if ((getIsReadyMethod = SideInputGrpc.getIsReadyMethod) == null) {
synchronized (SideInputGrpc.class) {
if ((getIsReadyMethod = SideInputGrpc.getIsReadyMethod) == null) {
SideInputGrpc.getIsReadyMethod = getIsReadyMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "IsReady"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.google.protobuf.Empty.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
io.numaproj.numaflow.sideinput.v1.Sideinput.ReadyResponse.getDefaultInstance()))
.setSchemaDescriptor(new SideInputMethodDescriptorSupplier("IsReady"))
.build();
}
}
}
return getIsReadyMethod;
}
/**
* Creates a new async stub that supports all call types for the service
*/
public static SideInputStub newStub(io.grpc.Channel channel) {
io.grpc.stub.AbstractStub.StubFactory factory =
new io.grpc.stub.AbstractStub.StubFactory() {
@java.lang.Override
public SideInputStub newStub(io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new SideInputStub(channel, callOptions);
}
};
return SideInputStub.newStub(factory, channel);
}
/**
* Creates a new blocking-style stub that supports unary and streaming output calls on the service
*/
public static SideInputBlockingStub newBlockingStub(
io.grpc.Channel channel) {
io.grpc.stub.AbstractStub.StubFactory factory =
new io.grpc.stub.AbstractStub.StubFactory() {
@java.lang.Override
public SideInputBlockingStub newStub(io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new SideInputBlockingStub(channel, callOptions);
}
};
return SideInputBlockingStub.newStub(factory, channel);
}
/**
* Creates a new ListenableFuture-style stub that supports unary calls on the service
*/
public static SideInputFutureStub newFutureStub(
io.grpc.Channel channel) {
io.grpc.stub.AbstractStub.StubFactory factory =
new io.grpc.stub.AbstractStub.StubFactory() {
@java.lang.Override
public SideInputFutureStub newStub(io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new SideInputFutureStub(channel, callOptions);
}
};
return SideInputFutureStub.newStub(factory, channel);
}
/**
*
* SideInput is the gRPC service for Side Inputs.
* It is used to propagate changes in the values of the provided Side Inputs
* which allows access to slow updated data or configuration without needing to retrieve
* it during each message processing.
* Through this service we should should be able to:-
* 1) Invoke retrieval request for a single Side Input parameter, which in turn should
* check for updates and return its latest value.
* 2) Provide a health check endpoint to indicate whether the service is ready to be used.
*
*/
public static abstract class SideInputImplBase implements io.grpc.BindableService {
/**
*
* RetrieveSideInput is the endpoint to retrieve the latest value of a given Side Input.
*
*/
public void retrieveSideInput(com.google.protobuf.Empty request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getRetrieveSideInputMethod(), responseObserver);
}
/**
*
* IsReady is the health check endpoint to indicate whether the service is ready to be used.
*
*/
public void isReady(com.google.protobuf.Empty request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getIsReadyMethod(), responseObserver);
}
@java.lang.Override public final io.grpc.ServerServiceDefinition bindService() {
return io.grpc.ServerServiceDefinition.builder(getServiceDescriptor())
.addMethod(
getRetrieveSideInputMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
com.google.protobuf.Empty,
io.numaproj.numaflow.sideinput.v1.Sideinput.SideInputResponse>(
this, METHODID_RETRIEVE_SIDE_INPUT)))
.addMethod(
getIsReadyMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
com.google.protobuf.Empty,
io.numaproj.numaflow.sideinput.v1.Sideinput.ReadyResponse>(
this, METHODID_IS_READY)))
.build();
}
}
/**
*
* SideInput is the gRPC service for Side Inputs.
* It is used to propagate changes in the values of the provided Side Inputs
* which allows access to slow updated data or configuration without needing to retrieve
* it during each message processing.
* Through this service we should should be able to:-
* 1) Invoke retrieval request for a single Side Input parameter, which in turn should
* check for updates and return its latest value.
* 2) Provide a health check endpoint to indicate whether the service is ready to be used.
*
*/
public static final class SideInputStub extends io.grpc.stub.AbstractAsyncStub {
private SideInputStub(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
super(channel, callOptions);
}
@java.lang.Override
protected SideInputStub build(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new SideInputStub(channel, callOptions);
}
/**
*
* RetrieveSideInput is the endpoint to retrieve the latest value of a given Side Input.
*
*/
public void retrieveSideInput(com.google.protobuf.Empty request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getRetrieveSideInputMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* IsReady is the health check endpoint to indicate whether the service is ready to be used.
*
*/
public void isReady(com.google.protobuf.Empty request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getIsReadyMethod(), getCallOptions()), request, responseObserver);
}
}
/**
*
* SideInput is the gRPC service for Side Inputs.
* It is used to propagate changes in the values of the provided Side Inputs
* which allows access to slow updated data or configuration without needing to retrieve
* it during each message processing.
* Through this service we should should be able to:-
* 1) Invoke retrieval request for a single Side Input parameter, which in turn should
* check for updates and return its latest value.
* 2) Provide a health check endpoint to indicate whether the service is ready to be used.
*
*/
public static final class SideInputBlockingStub extends io.grpc.stub.AbstractBlockingStub {
private SideInputBlockingStub(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
super(channel, callOptions);
}
@java.lang.Override
protected SideInputBlockingStub build(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new SideInputBlockingStub(channel, callOptions);
}
/**
*
* RetrieveSideInput is the endpoint to retrieve the latest value of a given Side Input.
*
*/
public io.numaproj.numaflow.sideinput.v1.Sideinput.SideInputResponse retrieveSideInput(com.google.protobuf.Empty request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getRetrieveSideInputMethod(), getCallOptions(), request);
}
/**
*
* IsReady is the health check endpoint to indicate whether the service is ready to be used.
*
*/
public io.numaproj.numaflow.sideinput.v1.Sideinput.ReadyResponse isReady(com.google.protobuf.Empty request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getIsReadyMethod(), getCallOptions(), request);
}
}
/**
*
* SideInput is the gRPC service for Side Inputs.
* It is used to propagate changes in the values of the provided Side Inputs
* which allows access to slow updated data or configuration without needing to retrieve
* it during each message processing.
* Through this service we should should be able to:-
* 1) Invoke retrieval request for a single Side Input parameter, which in turn should
* check for updates and return its latest value.
* 2) Provide a health check endpoint to indicate whether the service is ready to be used.
*
*/
public static final class SideInputFutureStub extends io.grpc.stub.AbstractFutureStub {
private SideInputFutureStub(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
super(channel, callOptions);
}
@java.lang.Override
protected SideInputFutureStub build(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new SideInputFutureStub(channel, callOptions);
}
/**
*
* RetrieveSideInput is the endpoint to retrieve the latest value of a given Side Input.
*
*/
public com.google.common.util.concurrent.ListenableFuture retrieveSideInput(
com.google.protobuf.Empty request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getRetrieveSideInputMethod(), getCallOptions()), request);
}
/**
*
* IsReady is the health check endpoint to indicate whether the service is ready to be used.
*
*/
public com.google.common.util.concurrent.ListenableFuture isReady(
com.google.protobuf.Empty request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getIsReadyMethod(), getCallOptions()), request);
}
}
private static final int METHODID_RETRIEVE_SIDE_INPUT = 0;
private static final int METHODID_IS_READY = 1;
private static final class MethodHandlers implements
io.grpc.stub.ServerCalls.UnaryMethod,
io.grpc.stub.ServerCalls.ServerStreamingMethod,
io.grpc.stub.ServerCalls.ClientStreamingMethod,
io.grpc.stub.ServerCalls.BidiStreamingMethod {
private final SideInputImplBase serviceImpl;
private final int methodId;
MethodHandlers(SideInputImplBase serviceImpl, int methodId) {
this.serviceImpl = serviceImpl;
this.methodId = methodId;
}
@java.lang.Override
@java.lang.SuppressWarnings("unchecked")
public void invoke(Req request, io.grpc.stub.StreamObserver responseObserver) {
switch (methodId) {
case METHODID_RETRIEVE_SIDE_INPUT:
serviceImpl.retrieveSideInput((com.google.protobuf.Empty) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_IS_READY:
serviceImpl.isReady((com.google.protobuf.Empty) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
default:
throw new AssertionError();
}
}
@java.lang.Override
@java.lang.SuppressWarnings("unchecked")
public io.grpc.stub.StreamObserver invoke(
io.grpc.stub.StreamObserver responseObserver) {
switch (methodId) {
default:
throw new AssertionError();
}
}
}
private static abstract class SideInputBaseDescriptorSupplier
implements io.grpc.protobuf.ProtoFileDescriptorSupplier, io.grpc.protobuf.ProtoServiceDescriptorSupplier {
SideInputBaseDescriptorSupplier() {}
@java.lang.Override
public com.google.protobuf.Descriptors.FileDescriptor getFileDescriptor() {
return io.numaproj.numaflow.sideinput.v1.Sideinput.getDescriptor();
}
@java.lang.Override
public com.google.protobuf.Descriptors.ServiceDescriptor getServiceDescriptor() {
return getFileDescriptor().findServiceByName("SideInput");
}
}
private static final class SideInputFileDescriptorSupplier
extends SideInputBaseDescriptorSupplier {
SideInputFileDescriptorSupplier() {}
}
private static final class SideInputMethodDescriptorSupplier
extends SideInputBaseDescriptorSupplier
implements io.grpc.protobuf.ProtoMethodDescriptorSupplier {
private final String methodName;
SideInputMethodDescriptorSupplier(String methodName) {
this.methodName = methodName;
}
@java.lang.Override
public com.google.protobuf.Descriptors.MethodDescriptor getMethodDescriptor() {
return getServiceDescriptor().findMethodByName(methodName);
}
}
private static volatile io.grpc.ServiceDescriptor serviceDescriptor;
public static io.grpc.ServiceDescriptor getServiceDescriptor() {
io.grpc.ServiceDescriptor result = serviceDescriptor;
if (result == null) {
synchronized (SideInputGrpc.class) {
result = serviceDescriptor;
if (result == null) {
serviceDescriptor = result = io.grpc.ServiceDescriptor.newBuilder(SERVICE_NAME)
.setSchemaDescriptor(new SideInputFileDescriptorSupplier())
.addMethod(getRetrieveSideInputMethod())
.addMethod(getIsReadyMethod())
.build();
}
}
}
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy