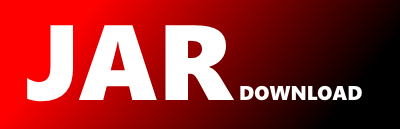
io.numaproj.numaflow.mapper.MapSupervisorActor Maven / Gradle / Ivy
Show all versions of numaflow-java Show documentation
package io.numaproj.numaflow.mapper;
import akka.actor.AbstractActor;
import akka.actor.ActorRef;
import akka.actor.AllDeadLetters;
import akka.actor.AllForOneStrategy;
import akka.actor.Props;
import akka.actor.SupervisorStrategy;
import akka.japi.pf.DeciderBuilder;
import akka.japi.pf.ReceiveBuilder;
import io.grpc.Status;
import io.grpc.stub.StreamObserver;
import io.numaproj.numaflow.map.v1.MapOuterClass;
import lombok.extern.slf4j.Slf4j;
import java.util.Optional;
import java.util.concurrent.CompletableFuture;
/**
* MapSupervisorActor actor is responsible for distributing the messages to actors and handling failure.
* It creates a MapperActor for each incoming request and listens to the responses from the MapperActor.
*
* MapSupervisorActor
* │
* ├── Creates MapperActor instances for each incoming MapRequest
* │ │
* │ ├── MapperActor 1
* │ │ ├── Processes MapRequest
* │ │ ├── Sends MapResponse to MapSupervisorActor
* │ │ └── Stops itself after processing
* │ │
* │ ├── MapperActor 2
* │ │ ├── Processes MapRequest
* │ │ ├── Sends MapResponse to MapSupervisorActor
* │ │ └── Stops itself after processing
* │ │
* ├── Listens to the responses from the MapperActor instances
* │ ├── On receiving a MapResponse, writes the response back to the client
* │
* ├── If any MapperActor fails (throws an exception):
* │ ├── Sends the exception back to the client
* │ ├── Initiates a shutdown by completing the CompletableFuture exceptionally
* │ └── Stops all child actors (AllForOneStrategy)
*/
@Slf4j
class MapSupervisorActor extends AbstractActor {
private final Mapper mapper;
private final StreamObserver responseObserver;
private final CompletableFuture shutdownSignal;
private int activeMapperCount;
private Exception userException;
public MapSupervisorActor(
Mapper mapper,
StreamObserver responseObserver,
CompletableFuture failureFuture) {
this.mapper = mapper;
this.responseObserver = responseObserver;
this.shutdownSignal = failureFuture;
this.userException = null;
this.activeMapperCount = 0;
}
public static Props props(
Mapper mapper,
StreamObserver responseObserver,
CompletableFuture shutdownSignal) {
return Props.create(MapSupervisorActor.class, mapper, responseObserver, shutdownSignal);
}
@Override
public void preRestart(Throwable reason, Optional