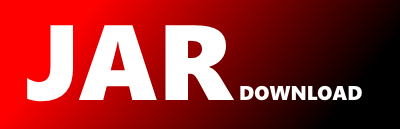
io.numaproj.numaflow.sourcetransformer.TransformSupervisorActor Maven / Gradle / Ivy
Show all versions of numaflow-java Show documentation
package io.numaproj.numaflow.sourcetransformer;
import akka.actor.AbstractActor;
import akka.actor.ActorRef;
import akka.actor.AllDeadLetters;
import akka.actor.AllForOneStrategy;
import akka.actor.Props;
import akka.actor.SupervisorStrategy;
import akka.japi.pf.DeciderBuilder;
import akka.japi.pf.ReceiveBuilder;
import io.grpc.Status;
import io.grpc.stub.StreamObserver;
import io.numaproj.numaflow.sourcetransformer.v1.Sourcetransformer;
import lombok.extern.slf4j.Slf4j;
import java.util.Optional;
import java.util.concurrent.CompletableFuture;
/**
* TransformSupervisorActor actor is responsible for distributing the messages to actors and handling failure.
* It creates a TransformerActor for each incoming request and listens to the responses from the TransformerActor.
*
* TransformSupervisorActor
* │
* ├── Creates TransformerActor instances for each incoming SourceTransformRequest
* │ │
* │ ├── TransformerActor 1
* │ │ ├── Processes SourceTransformRequest
* │ │ ├── Sends SourceTransformResponse to TransformSupervisorActor
* │ │ └── Stops itself after processing
* │ │
* │ ├── TransformerActor 2
* │ │ ├── Processes SourceTransformRequest
* │ │ ├── Sends SourceTransformResponse to TransformSupervisorActor
* │ │ └── Stops itself after processing
* │ │
* ├── Listens to the responses from the TransformerActor instances
* │ ├── On receiving a SourceTransformResponse, writes the response back to the client
* │
* ├── If any TransformerActor fails (throws an exception):
* │ ├── Sends the exception back to the client
* │ ├── Initiates a shutdown by completing the CompletableFuture exceptionally
* │ └── Stops all child actors (AllForOneStrategy)
*/
@Slf4j
class TransformSupervisorActor extends AbstractActor {
private final SourceTransformer transformer;
private final StreamObserver responseObserver;
private final CompletableFuture shutdownSignal;
private int activeTransformersCount;
private Exception userException;
/**
* Constructor for TransformSupervisorActor.
*
* @param transformer The transformer to be used for processing the request.
* @param responseObserver The StreamObserver to be used for sending the responses.
* @param shutdownSignal The CompletableFuture to be completed exceptionally in case of any failure.
*/
public TransformSupervisorActor(
SourceTransformer transformer,
StreamObserver responseObserver,
CompletableFuture shutdownSignal) {
this.transformer = transformer;
this.responseObserver = responseObserver;
this.shutdownSignal = shutdownSignal;
this.userException = null;
this.activeTransformersCount = 0;
}
/**
* Creates Props for a TransformSupervisorActor.
*
* @param transformer The transformer to be used for processing the request.
* @param responseObserver The StreamObserver to be used for sending the responses.
* @param shutdownSignal The CompletableFuture to be completed exceptionally in case of any failure.
*
* @return a Props for creating a TransformSupervisorActor.
*/
public static Props props(
SourceTransformer transformer,
StreamObserver responseObserver,
CompletableFuture shutdownSignal) {
return Props.create(
TransformSupervisorActor.class,
transformer,
responseObserver,
shutdownSignal);
}
/**
* Defines the behavior of the actor when it is restarted due to an exception.
*
* @param reason The exception that caused the restart.
* @param message The message that was being processed when the exception was thrown.
*/
@Override
public void preRestart(Throwable reason, Optional