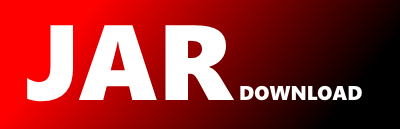
io.openapiprocessor.jsonschema.converter.MapSetStringsOrEmptyConverter Maven / Gradle / Ivy
/*
* Copyright 2022 https://github.com/openapi-processor/openapi-parser
* PDX-License-Identifier: Apache-2.0
*/
package io.openapiprocessor.jsonschema.converter;
import io.openapiprocessor.jsonschema.schema.JsonPointer;
import io.openapiprocessor.jsonschema.support.Types;
import org.checkerframework.checker.nullness.qual.Nullable;
import java.util.*;
import static java.util.Collections.unmodifiableMap;
import static java.util.Collections.unmodifiableSet;
/**
* get a {@link Map} of {@link Set}<{@link String}> from the {@code name} property
* {@code value}.
*/
public class MapSetStringsOrEmptyConverter implements PropertyConverter
© 2015 - 2025 Weber Informatics LLC | Privacy Policy