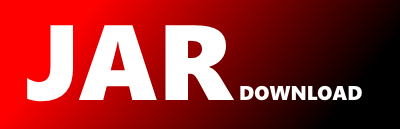
io.openapitools.swagger.config.SwaggerSecurityScheme Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of swagger-maven-plugin Show documentation
Show all versions of swagger-maven-plugin Show documentation
Maven plugin to generate OpenAPI documentation using Swagger.
The newest version!
package io.openapitools.swagger.config;
import java.util.Map;
import org.apache.maven.plugins.annotations.Parameter;
import io.swagger.v3.oas.models.security.SecurityScheme;
import io.swagger.v3.oas.models.security.SecurityScheme.In;
import io.swagger.v3.oas.models.security.SecurityScheme.Type;
public class SwaggerSecurityScheme {
/**
* REQUIRED. The type of the security scheme. Valid values are "apiKey", "http",
* "oauth2", "openIdConnect".
*/
@Parameter
private String type;
/**
* A short description for security scheme. CommonMark syntax MAY be used for
* rich text representation.
*/
@Parameter
private String description;
/**
* If type is apiKey: REQUIRED. The name of the header, query or cookie
* parameter to be used.
*/
@Parameter
private String name;
/**
* If type is apiKey: REQUIRED. The location of the API key. Valid values are
* "query", "header" or "cookie".
*/
@Parameter
private String in;
/**
* If type is http: REQUIRED. The name of the HTTP Authorization scheme to be
* used in the Authorization header as defined in RFC7235.
*/
@Parameter
private String scheme;
/**
* If type is http/"bearer": A hint to the client to identify how the bearer
* token is formatted. Bearer tokens are usually generated by an authorization
* server, so this information is primarily for documentation purposes.
*/
@Parameter
private String bearerFormat;
/**
* If type is oauth2: REQUIRED. An object containing configuration information
* for the flow types supported.
*/
@Parameter
private SwaggerFlows flows;
/**
* If type is openIdConnect: REQUIRED. OpenId Connect URL to discover OAuth2
* configuration values. This MUST be in the form of a URL.
*/
@Parameter
private String openIdConnectUrl;
@Parameter
private Map extensions;
@Parameter
private String $ref;
public SecurityScheme createSecuritySchemaModel() {
SecurityScheme securityScheme = new SecurityScheme();
if (type != null) {
securityScheme.setType(Type.valueOf(type.toUpperCase()));
}
securityScheme.setDescription(description);
securityScheme.setName(name);
if (in != null) {
securityScheme.setIn(In.valueOf(in.toUpperCase()));
}
securityScheme.setScheme(scheme);
securityScheme.setBearerFormat(bearerFormat);
if (flows != null) {
securityScheme.setFlows(flows.createOAuthFlowsModel());
}
securityScheme.setOpenIdConnectUrl(openIdConnectUrl);
if (extensions != null && !extensions.isEmpty()) {
securityScheme.setExtensions(extensions);
}
// Alternative to setting all above properties: reference to other component
securityScheme.set$ref($ref);
return securityScheme;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy