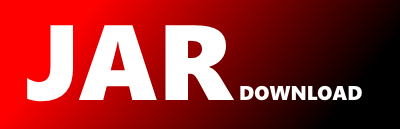
io.openlineage.client.OpenLineageClientUtils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of openlineage-java Show documentation
Show all versions of openlineage-java Show documentation
Java library for OpenLineage
/*
/* Copyright 2018-2023 contributors to the OpenLineage project
/* SPDX-License-Identifier: Apache-2.0
*/
package io.openlineage.client;
import static com.fasterxml.jackson.databind.DeserializationFeature.FAIL_ON_UNKNOWN_PROPERTIES;
import com.fasterxml.jackson.annotation.JsonFilter;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.core.JsonFactory;
import com.fasterxml.jackson.core.JsonProcessingException;
import com.fasterxml.jackson.core.type.TypeReference;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.fasterxml.jackson.databind.SerializationFeature;
import com.fasterxml.jackson.databind.ser.impl.SimpleBeanPropertyFilter;
import com.fasterxml.jackson.databind.ser.impl.SimpleFilterProvider;
import com.fasterxml.jackson.dataformat.yaml.YAMLFactory;
import com.fasterxml.jackson.datatype.jdk8.Jdk8Module;
import com.fasterxml.jackson.datatype.jsr310.JavaTimeModule;
import java.io.IOException;
import java.io.InputStream;
import java.io.UncheckedIOException;
import java.net.URI;
import java.net.URISyntaxException;
import java.nio.file.Files;
import java.nio.file.Path;
import java.util.Map;
import lombok.NonNull;
/** Utilities class for {@link OpenLineageClient}. */
public final class OpenLineageClientUtils {
private OpenLineageClientUtils() {}
private static final ObjectMapper MAPPER = newObjectMapper();
private static final ObjectMapper YML = new ObjectMapper(new YAMLFactory());
private static final ObjectMapper JSON = new ObjectMapper(new JsonFactory());
@JsonFilter("disabledFacets")
public class DisabledFacetsMixin {}
/** Returns a new {@link ObjectMapper} instance. */
public static ObjectMapper newObjectMapper() {
final ObjectMapper mapper = new ObjectMapper();
mapper.registerModule(new Jdk8Module());
mapper.registerModule(new JavaTimeModule());
mapper.setSerializationInclusion(JsonInclude.Include.NON_NULL);
mapper.disable(FAIL_ON_UNKNOWN_PROPERTIES);
mapper.disable(SerializationFeature.WRITE_DATES_AS_TIMESTAMPS);
return mapper;
}
/**
* Configure object mapper to exclude specified facets from being serialized
*
* @param disableFacets
*/
public static void configureObjectMapper(String[] disableFacets) {
if (disableFacets == null) {
return;
}
SimpleFilterProvider simpleFilterProvider =
new SimpleFilterProvider()
.addFilter(
"disabledFacets", SimpleBeanPropertyFilter.serializeAllExcept(disableFacets));
MAPPER.setFilterProvider(simpleFilterProvider);
MAPPER.addMixIn(Object.class, DisabledFacetsMixin.class);
}
/** Converts the provided {@code value} to a Json {@code string}. */
public static String toJson(@NonNull final Object value) {
try {
return MAPPER.writeValueAsString(value);
} catch (JsonProcessingException e) {
throw new UncheckedIOException(e);
}
}
/** Converts the provided Json {@code string} to the specified {@code type}. */
public static T fromJson(@NonNull final String json, @NonNull final TypeReference type) {
try {
return MAPPER.readValue(json, type);
} catch (IOException e) {
throw new UncheckedIOException(e);
}
}
public static T convertValue(Object fromValue, Class toValueType) {
return MAPPER.convertValue(fromValue, toValueType);
}
/**
* Create a new instance of the facets container with all values merged from the original
* facetsContainer and the given facets Map, with precedence given to the facets Map.
*/
public static T mergeFacets(Map facetsMap, T facetsContainer, Class klass) {
if (facetsContainer == null) {
return MAPPER.convertValue(facetsMap, klass);
}
Map targetMap =
MAPPER.convertValue(facetsContainer, new TypeReference
© 2015 - 2025 Weber Informatics LLC | Privacy Policy