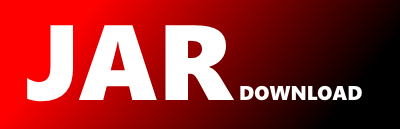
io.openlineage.client.OpenLineage Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of openlineage-java Show documentation
Show all versions of openlineage-java Show documentation
Java library for OpenLineage
package io.openlineage.client;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.fasterxml.jackson.databind.annotation.JsonDeserialize;
import io.openlineage.client.OpenLineage.LifecycleStateChangeDatasetFacet.LifecycleStateChange;
import io.openlineage.client.OpenLineage.RunEvent.EventType;
import java.lang.Boolean;
import java.lang.Double;
import java.lang.Long;
import java.lang.Object;
import java.lang.Override;
import java.lang.String;
import java.net.URI;
import java.time.ZonedDateTime;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
import java.util.UUID;
/**
* Usage:
* {@code
* URI producer = URI.create("http://my.producer/uri");
* OpenLineage ol = new OpenLineage(producer);
* UUID runId = UUID.randomUUID();
* RunFacets runFacets =
* ol.newRunFacetsBuilder().nominalTime(ol.newNominalTimeRunFacet(now, now)).build();
* Run run = ol.newRun(runId, runFacets);
* String name = "jobName";
* String namespace = "namespace";
* JobFacets jobFacets = ol.newJobFacetsBuilder().build();
* Job job = ol.newJob(namespace, name, jobFacets);
* List inputs = Arrays.asList(ol.newInputDataset("ins", "input", null, null));
* List outputs = Arrays.asList(ol.newOutputDataset("ons", "output", null, null));
* RunEvent runStateUpdate =
* ol.newRunEvent(now, OpenLineage.RunEvent.EventType.START, run, job, inputs, outputs);
* }
*/
public final class OpenLineage {
private final URI producer;
/**
* Starting point to create OpenLineage objects. Use the OpenLineage instance to create events and facets
* @param producer the identifier of the library using the client to generate OpenLineage events
*/
public OpenLineage(URI producer) {
this.producer = producer;
}
/**
* Factory method for RunEvent@param eventTime the time the event occurred at
* @param eventType the current transition of the run state. It is required to issue 1 START event and 1 of [ COMPLETE, ABORT, FAIL ] event per run. Additional events with OTHER eventType can be added to the same run. For example to send additional metadata after the run is complete
* @param run the run
* @param job the job
* @param inputs The set of **input** datasets.
* @param outputs The set of **output** datasets.
* @return RunEvent
*/
public RunEvent newRunEvent(ZonedDateTime eventTime, RunEvent.EventType eventType, Run run,
Job job, List inputs, List outputs) {
return new RunEvent(eventTime, this.producer, eventType, run, job, inputs, outputs);
}
/**
* Creates a builder for RunEvent
* @return a new builder for RunEvent
*/
public RunEventBuilder newRunEventBuilder() {
return new RunEventBuilder();
}
/**
* Factory method for SchemaDatasetFacetFields@param name The name of the field.
* @param type The type of the field.
* @param description The description of the field.
* @param fields Nested struct fields.
* @return SchemaDatasetFacetFields
*/
public SchemaDatasetFacetFields newSchemaDatasetFacetFields(String name, String type,
String description, List fields) {
return new SchemaDatasetFacetFields(name, type, description, fields);
}
/**
* Creates a builder for SchemaDatasetFacetFields
* @return a new builder for SchemaDatasetFacetFields
*/
public SchemaDatasetFacetFieldsBuilder newSchemaDatasetFacetFieldsBuilder() {
return new SchemaDatasetFacetFieldsBuilder();
}
/**
* Factory method for ParentRunFacet@param run the run
* @param job the job
* @return ParentRunFacet
*/
public ParentRunFacet newParentRunFacet(ParentRunFacetRun run, ParentRunFacetJob job) {
return new ParentRunFacet(this.producer, run, job);
}
/**
* Creates a builder for ParentRunFacet
* @return a new builder for ParentRunFacet
*/
public ParentRunFacetBuilder newParentRunFacetBuilder() {
return new ParentRunFacetBuilder();
}
/**
* Factory method for OutputStatisticsOutputDatasetFacet@param rowCount The number of rows written to the dataset
* @param size The size in bytes written to the dataset
* @param fileCount The number of files written to the dataset
* @return OutputStatisticsOutputDatasetFacet
*/
public OutputStatisticsOutputDatasetFacet newOutputStatisticsOutputDatasetFacet(Long rowCount,
Long size, Long fileCount) {
return new OutputStatisticsOutputDatasetFacet(this.producer, rowCount, size, fileCount);
}
/**
* Creates a builder for OutputStatisticsOutputDatasetFacet
* @return a new builder for OutputStatisticsOutputDatasetFacet
*/
public OutputStatisticsOutputDatasetFacetBuilder newOutputStatisticsOutputDatasetFacetBuilder() {
return new OutputStatisticsOutputDatasetFacetBuilder();
}
/**
* Factory method for ExtractionErrorRunFacetErrors@param errorMessage Text representation of extraction error message.
* @param stackTrace Stack trace of extraction error message
* @param task Text representation of task that failed. This can be, for example, SQL statement that parser could not interpret.
* @param taskNumber Order of task (counted from 0).
* @return ExtractionErrorRunFacetErrors
*/
public ExtractionErrorRunFacetErrors newExtractionErrorRunFacetErrors(String errorMessage,
String stackTrace, String task, Long taskNumber) {
return new ExtractionErrorRunFacetErrors(errorMessage, stackTrace, task, taskNumber);
}
/**
* Creates a builder for ExtractionErrorRunFacetErrors
* @return a new builder for ExtractionErrorRunFacetErrors
*/
public ExtractionErrorRunFacetErrorsBuilder newExtractionErrorRunFacetErrorsBuilder() {
return new ExtractionErrorRunFacetErrorsBuilder();
}
/**
* Factory method for JobTypeJobFacet@param processingType Job processing type like: BATCH or STREAMING
* @param integration OpenLineage integration type of this job: SPARK|DBT|AIRFLOW|FLINK
* @param jobType Run type like: QUERY|COMMAND|DAG|TASK|JOB|MODEL
* @return JobTypeJobFacet
*/
public JobTypeJobFacet newJobTypeJobFacet(String processingType, String integration,
String jobType) {
return new JobTypeJobFacet(this.producer, processingType, integration, jobType);
}
/**
* Creates a builder for JobTypeJobFacet
* @return a new builder for JobTypeJobFacet
*/
public JobTypeJobFacetBuilder newJobTypeJobFacetBuilder() {
return new JobTypeJobFacetBuilder();
}
/**
* Factory method for Run@param runId The globally unique ID of the run associated with the job.
* @param facets The run facets.
* @return Run
*/
public Run newRun(UUID runId, RunFacets facets) {
return new Run(runId, facets);
}
/**
* Creates a builder for Run
* @return a new builder for Run
*/
public RunBuilder newRunBuilder() {
return new RunBuilder();
}
/**
* Factory method for DataQualityMetricsInputDatasetFacetColumnMetricsAdditionalQuantiles@return DataQualityMetricsInputDatasetFacetColumnMetricsAdditionalQuantiles
*/
public DataQualityMetricsInputDatasetFacetColumnMetricsAdditionalQuantiles newDataQualityMetricsInputDatasetFacetColumnMetricsAdditionalQuantiles(
) {
return new DataQualityMetricsInputDatasetFacetColumnMetricsAdditionalQuantiles();
}
/**
* Creates a builder for DataQualityMetricsInputDatasetFacetColumnMetricsAdditionalQuantiles
* @return a new builder for DataQualityMetricsInputDatasetFacetColumnMetricsAdditionalQuantiles
*/
public DataQualityMetricsInputDatasetFacetColumnMetricsAdditionalQuantilesBuilder newDataQualityMetricsInputDatasetFacetColumnMetricsAdditionalQuantilesBuilder(
) {
return new DataQualityMetricsInputDatasetFacetColumnMetricsAdditionalQuantilesBuilder();
}
/**
* Factory method for ExternalQueryRunFacet@param externalQueryId Identifier for the external system
* @param source source of the external query
* @return ExternalQueryRunFacet
*/
public ExternalQueryRunFacet newExternalQueryRunFacet(String externalQueryId, String source) {
return new ExternalQueryRunFacet(this.producer, externalQueryId, source);
}
/**
* Creates a builder for ExternalQueryRunFacet
* @return a new builder for ExternalQueryRunFacet
*/
public ExternalQueryRunFacetBuilder newExternalQueryRunFacetBuilder() {
return new ExternalQueryRunFacetBuilder();
}
/**
* Factory method for OutputDatasetOutputFacets@param outputStatistics the outputStatistics
* @return OutputDatasetOutputFacets
*/
public OutputDatasetOutputFacets newOutputDatasetOutputFacets(
OutputStatisticsOutputDatasetFacet outputStatistics) {
return new OutputDatasetOutputFacets(outputStatistics);
}
/**
* Creates a builder for OutputDatasetOutputFacets
* @return a new builder for OutputDatasetOutputFacets
*/
public OutputDatasetOutputFacetsBuilder newOutputDatasetOutputFacetsBuilder() {
return new OutputDatasetOutputFacetsBuilder();
}
/**
* Factory method for NominalTimeRunFacet@param nominalStartTime An [ISO-8601](https://en.wikipedia.org/wiki/ISO_8601) timestamp representing the nominal start time (included) of the run. AKA the schedule time
* @param nominalEndTime An [ISO-8601](https://en.wikipedia.org/wiki/ISO_8601) timestamp representing the nominal end time (excluded) of the run. (Should be the nominal start time of the next run)
* @return NominalTimeRunFacet
*/
public NominalTimeRunFacet newNominalTimeRunFacet(ZonedDateTime nominalStartTime,
ZonedDateTime nominalEndTime) {
return new NominalTimeRunFacet(this.producer, nominalStartTime, nominalEndTime);
}
/**
* Creates a builder for NominalTimeRunFacet
* @return a new builder for NominalTimeRunFacet
*/
public NominalTimeRunFacetBuilder newNominalTimeRunFacetBuilder() {
return new NominalTimeRunFacetBuilder();
}
/**
* @return RunFacet
*/
public RunFacet newRunFacet() {
return new DefaultRunFacet(this.producer);
}
/**
* Factory method for OwnershipJobFacetOwners@param name the identifier of the owner of the Job. It is recommended to define this as a URN. For example application:foo, user:jdoe, team:data
* @param type The type of ownership (optional)
* @return OwnershipJobFacetOwners
*/
public OwnershipJobFacetOwners newOwnershipJobFacetOwners(String name, String type) {
return new OwnershipJobFacetOwners(name, type);
}
/**
* Creates a builder for OwnershipJobFacetOwners
* @return a new builder for OwnershipJobFacetOwners
*/
public OwnershipJobFacetOwnersBuilder newOwnershipJobFacetOwnersBuilder() {
return new OwnershipJobFacetOwnersBuilder();
}
/**
* @return InputDatasetFacet
*/
public InputDatasetFacet newInputDatasetFacet() {
return new DefaultInputDatasetFacet(this.producer);
}
/**
* Factory method for JobEvent@param eventTime the time the event occurred at
* @param job the job
* @param inputs The set of **input** datasets.
* @param outputs The set of **output** datasets.
* @return JobEvent
*/
public JobEvent newJobEvent(ZonedDateTime eventTime, Job job, List inputs,
List outputs) {
return new JobEvent(eventTime, this.producer, job, inputs, outputs);
}
/**
* Creates a builder for JobEvent
* @return a new builder for JobEvent
*/
public JobEventBuilder newJobEventBuilder() {
return new JobEventBuilder();
}
/**
* Factory method for ColumnLineageDatasetFacetFieldsAdditional@param inputFields the inputFields
* @param transformationDescription a string representation of the transformation applied
* @param transformationType IDENTITY|MASKED reflects a clearly defined behavior. IDENTITY: exact same as input; MASKED: no original data available (like a hash of PII for example)
* @return ColumnLineageDatasetFacetFieldsAdditional
*/
public ColumnLineageDatasetFacetFieldsAdditional newColumnLineageDatasetFacetFieldsAdditional(
List inputFields,
String transformationDescription, String transformationType) {
return new ColumnLineageDatasetFacetFieldsAdditional(inputFields, transformationDescription, transformationType);
}
/**
* Creates a builder for ColumnLineageDatasetFacetFieldsAdditional
* @return a new builder for ColumnLineageDatasetFacetFieldsAdditional
*/
public ColumnLineageDatasetFacetFieldsAdditionalBuilder newColumnLineageDatasetFacetFieldsAdditionalBuilder(
) {
return new ColumnLineageDatasetFacetFieldsAdditionalBuilder();
}
/**
* Factory method for ExtractionErrorRunFacet@param totalTasks The number of distinguishable tasks in a run that were processed by OpenLineage, whether successfully or not. Those could be, for example, distinct SQL statements.
* @param failedTasks The number of distinguishable tasks in a run that were processed not successfully by OpenLineage. Those could be, for example, distinct SQL statements.
* @param errors the errors
* @return ExtractionErrorRunFacet
*/
public ExtractionErrorRunFacet newExtractionErrorRunFacet(Long totalTasks, Long failedTasks,
List errors) {
return new ExtractionErrorRunFacet(this.producer, totalTasks, failedTasks, errors);
}
/**
* Creates a builder for ExtractionErrorRunFacet
* @return a new builder for ExtractionErrorRunFacet
*/
public ExtractionErrorRunFacetBuilder newExtractionErrorRunFacetBuilder() {
return new ExtractionErrorRunFacetBuilder();
}
/**
* Factory method for OwnershipDatasetFacetOwners@param name the identifier of the owner of the Dataset. It is recommended to define this as a URN. For example application:foo, user:jdoe, team:data
* @param type The type of ownership (optional)
* @return OwnershipDatasetFacetOwners
*/
public OwnershipDatasetFacetOwners newOwnershipDatasetFacetOwners(String name, String type) {
return new OwnershipDatasetFacetOwners(name, type);
}
/**
* Creates a builder for OwnershipDatasetFacetOwners
* @return a new builder for OwnershipDatasetFacetOwners
*/
public OwnershipDatasetFacetOwnersBuilder newOwnershipDatasetFacetOwnersBuilder() {
return new OwnershipDatasetFacetOwnersBuilder();
}
/**
* Factory method for DataQualityMetricsInputDatasetFacetColumnMetricsAdditional@param nullCount The number of null values in this column for the rows evaluated
* @param distinctCount The number of distinct values in this column for the rows evaluated
* @param sum The total sum of values in this column for the rows evaluated
* @param count The number of values in this column
* @param min the min
* @param max the max
* @param quantiles The property key is the quantile. Examples: 0.1 0.25 0.5 0.75 1
* @return DataQualityMetricsInputDatasetFacetColumnMetricsAdditional
*/
public DataQualityMetricsInputDatasetFacetColumnMetricsAdditional newDataQualityMetricsInputDatasetFacetColumnMetricsAdditional(
Long nullCount, Long distinctCount, Double sum, Double count, Double min, Double max,
DataQualityMetricsInputDatasetFacetColumnMetricsAdditionalQuantiles quantiles) {
return new DataQualityMetricsInputDatasetFacetColumnMetricsAdditional(nullCount, distinctCount, sum, count, min, max, quantiles);
}
/**
* Creates a builder for DataQualityMetricsInputDatasetFacetColumnMetricsAdditional
* @return a new builder for DataQualityMetricsInputDatasetFacetColumnMetricsAdditional
*/
public DataQualityMetricsInputDatasetFacetColumnMetricsAdditionalBuilder newDataQualityMetricsInputDatasetFacetColumnMetricsAdditionalBuilder(
) {
return new DataQualityMetricsInputDatasetFacetColumnMetricsAdditionalBuilder();
}
/**
* Factory method for DataQualityMetricsInputDatasetFacetColumnMetrics@return DataQualityMetricsInputDatasetFacetColumnMetrics
*/
public DataQualityMetricsInputDatasetFacetColumnMetrics newDataQualityMetricsInputDatasetFacetColumnMetrics(
) {
return new DataQualityMetricsInputDatasetFacetColumnMetrics();
}
/**
* Creates a builder for DataQualityMetricsInputDatasetFacetColumnMetrics
* @return a new builder for DataQualityMetricsInputDatasetFacetColumnMetrics
*/
public DataQualityMetricsInputDatasetFacetColumnMetricsBuilder newDataQualityMetricsInputDatasetFacetColumnMetricsBuilder(
) {
return new DataQualityMetricsInputDatasetFacetColumnMetricsBuilder();
}
/**
* Factory method for DataQualityMetricsInputDatasetFacet@param rowCount The number of rows evaluated
* @param bytes The size in bytes
* @param fileCount The number of files evaluated
* @param columnMetrics The property key is the column name
* @return DataQualityMetricsInputDatasetFacet
*/
public DataQualityMetricsInputDatasetFacet newDataQualityMetricsInputDatasetFacet(Long rowCount,
Long bytes, Long fileCount, DataQualityMetricsInputDatasetFacetColumnMetrics columnMetrics) {
return new DataQualityMetricsInputDatasetFacet(this.producer, rowCount, bytes, fileCount, columnMetrics);
}
/**
* Creates a builder for DataQualityMetricsInputDatasetFacet
* @return a new builder for DataQualityMetricsInputDatasetFacet
*/
public DataQualityMetricsInputDatasetFacetBuilder newDataQualityMetricsInputDatasetFacetBuilder(
) {
return new DataQualityMetricsInputDatasetFacetBuilder();
}
/**
* Factory method for DocumentationJobFacet@param description The description of the job.
* @return DocumentationJobFacet
*/
public DocumentationJobFacet newDocumentationJobFacet(String description) {
return new DocumentationJobFacet(this.producer, description);
}
/**
* Creates a builder for DocumentationJobFacet
* @return a new builder for DocumentationJobFacet
*/
public DocumentationJobFacetBuilder newDocumentationJobFacetBuilder() {
return new DocumentationJobFacetBuilder();
}
/**
* Factory method for JobFacets@param jobType the jobType
* @param sourceCode the sourceCode
* @param ownership the ownership
* @param sql the sql
* @param sourceCodeLocation the sourceCodeLocation
* @param documentation the documentation
* @return JobFacets
*/
public JobFacets newJobFacets(JobTypeJobFacet jobType, SourceCodeJobFacet sourceCode,
OwnershipJobFacet ownership, SQLJobFacet sql, SourceCodeLocationJobFacet sourceCodeLocation,
DocumentationJobFacet documentation) {
return new JobFacets(jobType, sourceCode, ownership, sql, sourceCodeLocation, documentation);
}
/**
* Creates a builder for JobFacets
* @return a new builder for JobFacets
*/
public JobFacetsBuilder newJobFacetsBuilder() {
return new JobFacetsBuilder();
}
/**
* Factory method for InputDatasetInputFacets@param dataQualityAssertions the dataQualityAssertions
* @param dataQualityMetrics the dataQualityMetrics
* @return InputDatasetInputFacets
*/
public InputDatasetInputFacets newInputDatasetInputFacets(
DataQualityAssertionsDatasetFacet dataQualityAssertions,
DataQualityMetricsInputDatasetFacet dataQualityMetrics) {
return new InputDatasetInputFacets(dataQualityAssertions, dataQualityMetrics);
}
/**
* Creates a builder for InputDatasetInputFacets
* @return a new builder for InputDatasetInputFacets
*/
public InputDatasetInputFacetsBuilder newInputDatasetInputFacetsBuilder() {
return new InputDatasetInputFacetsBuilder();
}
/**
* @return DatasetFacet
*/
public DatasetFacet newDatasetFacet() {
return new DefaultDatasetFacet(this.producer, null);
}
/**
* @return a deleted DatasetFacet
*/
public DatasetFacet newDeletedDatasetFacet() {
return new DefaultDatasetFacet(this.producer, true);
}
/**
* Factory method for OwnershipDatasetFacet@param owners The owners of the dataset.
* @return OwnershipDatasetFacet
*/
public OwnershipDatasetFacet newOwnershipDatasetFacet(List owners) {
return new OwnershipDatasetFacet(this.producer, owners);
}
/**
* Creates a builder for OwnershipDatasetFacet
* @return a new builder for OwnershipDatasetFacet
*/
public OwnershipDatasetFacetBuilder newOwnershipDatasetFacetBuilder() {
return new OwnershipDatasetFacetBuilder();
}
/**
* Factory method for SQLJobFacet@param query the query
* @return SQLJobFacet
*/
public SQLJobFacet newSQLJobFacet(String query) {
return new SQLJobFacet(this.producer, query);
}
/**
* Creates a builder for SQLJobFacet
* @return a new builder for SQLJobFacet
*/
public SQLJobFacetBuilder newSQLJobFacetBuilder() {
return new SQLJobFacetBuilder();
}
/**
* Factory method for DatasetFacets@param documentation the documentation
* @param dataSource the dataSource
* @param version the version
* @param schema the schema
* @param ownership the ownership
* @param storage the storage
* @param columnLineage the columnLineage
* @param symlinks the symlinks
* @param lifecycleStateChange the lifecycleStateChange
* @return DatasetFacets
*/
public DatasetFacets newDatasetFacets(DocumentationDatasetFacet documentation,
DatasourceDatasetFacet dataSource, DatasetVersionDatasetFacet version,
SchemaDatasetFacet schema, OwnershipDatasetFacet ownership, StorageDatasetFacet storage,
ColumnLineageDatasetFacet columnLineage, SymlinksDatasetFacet symlinks,
LifecycleStateChangeDatasetFacet lifecycleStateChange) {
return new DatasetFacets(documentation, dataSource, version, schema, ownership, storage, columnLineage, symlinks, lifecycleStateChange);
}
/**
* Creates a builder for DatasetFacets
* @return a new builder for DatasetFacets
*/
public DatasetFacetsBuilder newDatasetFacetsBuilder() {
return new DatasetFacetsBuilder();
}
/**
* Factory method for OwnershipJobFacet@param owners The owners of the job.
* @return OwnershipJobFacet
*/
public OwnershipJobFacet newOwnershipJobFacet(List owners) {
return new OwnershipJobFacet(this.producer, owners);
}
/**
* Creates a builder for OwnershipJobFacet
* @return a new builder for OwnershipJobFacet
*/
public OwnershipJobFacetBuilder newOwnershipJobFacetBuilder() {
return new OwnershipJobFacetBuilder();
}
/**
* Factory method for ParentRunFacetJob@param namespace The namespace containing that job
* @param name The unique name for that job within that namespace
* @return ParentRunFacetJob
*/
public ParentRunFacetJob newParentRunFacetJob(String namespace, String name) {
return new ParentRunFacetJob(namespace, name);
}
/**
* Creates a builder for ParentRunFacetJob
* @return a new builder for ParentRunFacetJob
*/
public ParentRunFacetJobBuilder newParentRunFacetJobBuilder() {
return new ParentRunFacetJobBuilder();
}
/**
* Factory method for OutputDataset@param namespace The namespace containing that dataset
* @param name The unique name for that dataset within that namespace
* @param facets The facets for this dataset
* @param outputFacets The output facets for this dataset
* @return OutputDataset
*/
public OutputDataset newOutputDataset(String namespace, String name, DatasetFacets facets,
OutputDatasetOutputFacets outputFacets) {
return new OutputDataset(namespace, name, facets, outputFacets);
}
/**
* Creates a builder for OutputDataset
* @return a new builder for OutputDataset
*/
public OutputDatasetBuilder newOutputDatasetBuilder() {
return new OutputDatasetBuilder();
}
/**
* Factory method for ErrorMessageRunFacet@param message A human-readable string representing error message generated by observed system
* @param programmingLanguage Programming language the observed system uses.
* @param stackTrace A language-specific stack trace generated by observed system
* @return ErrorMessageRunFacet
*/
public ErrorMessageRunFacet newErrorMessageRunFacet(String message, String programmingLanguage,
String stackTrace) {
return new ErrorMessageRunFacet(this.producer, message, programmingLanguage, stackTrace);
}
/**
* Creates a builder for ErrorMessageRunFacet
* @return a new builder for ErrorMessageRunFacet
*/
public ErrorMessageRunFacetBuilder newErrorMessageRunFacetBuilder() {
return new ErrorMessageRunFacetBuilder();
}
/**
* @return JobFacet
*/
public JobFacet newJobFacet() {
return new DefaultJobFacet(this.producer, null);
}
/**
* @return a deleted JobFacet
*/
public JobFacet newDeletedJobFacet() {
return new DefaultJobFacet(this.producer, true);
}
/**
* Factory method for RunFacets@param errorMessage the errorMessage
* @param externalQuery the externalQuery
* @param extractionError the extractionError
* @param parent the parent
* @param nominalTime the nominalTime
* @param processing_engine the processing_engine
* @return RunFacets
*/
public RunFacets newRunFacets(ErrorMessageRunFacet errorMessage,
ExternalQueryRunFacet externalQuery, ExtractionErrorRunFacet extractionError,
ParentRunFacet parent, NominalTimeRunFacet nominalTime,
ProcessingEngineRunFacet processing_engine) {
return new RunFacets(errorMessage, externalQuery, extractionError, parent, nominalTime, processing_engine);
}
/**
* Creates a builder for RunFacets
* @return a new builder for RunFacets
*/
public RunFacetsBuilder newRunFacetsBuilder() {
return new RunFacetsBuilder();
}
/**
* Factory method for SchemaDatasetFacet@param fields The fields of the data source.
* @return SchemaDatasetFacet
*/
public SchemaDatasetFacet newSchemaDatasetFacet(List fields) {
return new SchemaDatasetFacet(this.producer, fields);
}
/**
* Creates a builder for SchemaDatasetFacet
* @return a new builder for SchemaDatasetFacet
*/
public SchemaDatasetFacetBuilder newSchemaDatasetFacetBuilder() {
return new SchemaDatasetFacetBuilder();
}
/**
* Factory method for SourceCodeJobFacet@param language Language in which source code of this job was written.
* @param sourceCode Source code of this job.
* @return SourceCodeJobFacet
*/
public SourceCodeJobFacet newSourceCodeJobFacet(String language, String sourceCode) {
return new SourceCodeJobFacet(this.producer, language, sourceCode);
}
/**
* Creates a builder for SourceCodeJobFacet
* @return a new builder for SourceCodeJobFacet
*/
public SourceCodeJobFacetBuilder newSourceCodeJobFacetBuilder() {
return new SourceCodeJobFacetBuilder();
}
/**
* Factory method for DocumentationDatasetFacet@param description The description of the dataset.
* @return DocumentationDatasetFacet
*/
public DocumentationDatasetFacet newDocumentationDatasetFacet(String description) {
return new DocumentationDatasetFacet(this.producer, description);
}
/**
* Creates a builder for DocumentationDatasetFacet
* @return a new builder for DocumentationDatasetFacet
*/
public DocumentationDatasetFacetBuilder newDocumentationDatasetFacetBuilder() {
return new DocumentationDatasetFacetBuilder();
}
/**
* Factory method for SourceCodeLocationJobFacet@param type the source control system
* @param url the full http URL to locate the file
* @param repoUrl the URL to the repository
* @param path the path in the repo containing the source files
* @param version the current version deployed (not a branch name, the actual unique version)
* @param tag optional tag name
* @param branch optional branch name
* @return SourceCodeLocationJobFacet
*/
public SourceCodeLocationJobFacet newSourceCodeLocationJobFacet(String type, URI url,
String repoUrl, String path, String version, String tag, String branch) {
return new SourceCodeLocationJobFacet(this.producer, type, url, repoUrl, path, version, tag, branch);
}
/**
* Creates a builder for SourceCodeLocationJobFacet
* @return a new builder for SourceCodeLocationJobFacet
*/
public SourceCodeLocationJobFacetBuilder newSourceCodeLocationJobFacetBuilder() {
return new SourceCodeLocationJobFacetBuilder();
}
/**
* Factory method for ColumnLineageDatasetFacet@param fields Column level lineage that maps output fields into input fields used to evaluate them.
* @return ColumnLineageDatasetFacet
*/
public ColumnLineageDatasetFacet newColumnLineageDatasetFacet(
ColumnLineageDatasetFacetFields fields) {
return new ColumnLineageDatasetFacet(this.producer, fields);
}
/**
* Creates a builder for ColumnLineageDatasetFacet
* @return a new builder for ColumnLineageDatasetFacet
*/
public ColumnLineageDatasetFacetBuilder newColumnLineageDatasetFacetBuilder() {
return new ColumnLineageDatasetFacetBuilder();
}
/**
* Factory method for DataQualityAssertionsDatasetFacet@param assertions the assertions
* @return DataQualityAssertionsDatasetFacet
*/
public DataQualityAssertionsDatasetFacet newDataQualityAssertionsDatasetFacet(
List assertions) {
return new DataQualityAssertionsDatasetFacet(this.producer, assertions);
}
/**
* Creates a builder for DataQualityAssertionsDatasetFacet
* @return a new builder for DataQualityAssertionsDatasetFacet
*/
public DataQualityAssertionsDatasetFacetBuilder newDataQualityAssertionsDatasetFacetBuilder() {
return new DataQualityAssertionsDatasetFacetBuilder();
}
/**
* Factory method for DatasetEvent@param eventTime the time the event occurred at
* @param dataset the dataset
* @return DatasetEvent
*/
public DatasetEvent newDatasetEvent(ZonedDateTime eventTime, StaticDataset dataset) {
return new DatasetEvent(eventTime, this.producer, dataset);
}
/**
* Creates a builder for DatasetEvent
* @return a new builder for DatasetEvent
*/
public DatasetEventBuilder newDatasetEventBuilder() {
return new DatasetEventBuilder();
}
/**
* Factory method for ProcessingEngineRunFacet@param version Processing engine version. Might be Airflow or Spark version.
* @param name Processing engine name, e.g. Airflow or Spark
* @param openlineageAdapterVersion OpenLineage adapter package version. Might be e.g. OpenLineage Airflow integration package version
* @return ProcessingEngineRunFacet
*/
public ProcessingEngineRunFacet newProcessingEngineRunFacet(String version, String name,
String openlineageAdapterVersion) {
return new ProcessingEngineRunFacet(this.producer, version, name, openlineageAdapterVersion);
}
/**
* Creates a builder for ProcessingEngineRunFacet
* @return a new builder for ProcessingEngineRunFacet
*/
public ProcessingEngineRunFacetBuilder newProcessingEngineRunFacetBuilder() {
return new ProcessingEngineRunFacetBuilder();
}
/**
* Factory method for LifecycleStateChangeDatasetFacetPreviousIdentifier@param name the name
* @param namespace the namespace
* @return LifecycleStateChangeDatasetFacetPreviousIdentifier
*/
public LifecycleStateChangeDatasetFacetPreviousIdentifier newLifecycleStateChangeDatasetFacetPreviousIdentifier(
String name, String namespace) {
return new LifecycleStateChangeDatasetFacetPreviousIdentifier(name, namespace);
}
/**
* Creates a builder for LifecycleStateChangeDatasetFacetPreviousIdentifier
* @return a new builder for LifecycleStateChangeDatasetFacetPreviousIdentifier
*/
public LifecycleStateChangeDatasetFacetPreviousIdentifierBuilder newLifecycleStateChangeDatasetFacetPreviousIdentifierBuilder(
) {
return new LifecycleStateChangeDatasetFacetPreviousIdentifierBuilder();
}
/**
* Factory method for Job@param namespace The namespace containing that job
* @param name The unique name for that job within that namespace
* @param facets The job facets.
* @return Job
*/
public Job newJob(String namespace, String name, JobFacets facets) {
return new Job(namespace, name, facets);
}
/**
* Creates a builder for Job
* @return a new builder for Job
*/
public JobBuilder newJobBuilder() {
return new JobBuilder();
}
/**
* Factory method for DatasetVersionDatasetFacet@param datasetVersion The version of the dataset.
* @return DatasetVersionDatasetFacet
*/
public DatasetVersionDatasetFacet newDatasetVersionDatasetFacet(String datasetVersion) {
return new DatasetVersionDatasetFacet(this.producer, datasetVersion);
}
/**
* Creates a builder for DatasetVersionDatasetFacet
* @return a new builder for DatasetVersionDatasetFacet
*/
public DatasetVersionDatasetFacetBuilder newDatasetVersionDatasetFacetBuilder() {
return new DatasetVersionDatasetFacetBuilder();
}
/**
* Factory method for SymlinksDatasetFacetIdentifiers@param namespace The dataset namespace
* @param name The dataset name
* @param type Identifier type
* @return SymlinksDatasetFacetIdentifiers
*/
public SymlinksDatasetFacetIdentifiers newSymlinksDatasetFacetIdentifiers(String namespace,
String name, String type) {
return new SymlinksDatasetFacetIdentifiers(namespace, name, type);
}
/**
* Creates a builder for SymlinksDatasetFacetIdentifiers
* @return a new builder for SymlinksDatasetFacetIdentifiers
*/
public SymlinksDatasetFacetIdentifiersBuilder newSymlinksDatasetFacetIdentifiersBuilder() {
return new SymlinksDatasetFacetIdentifiersBuilder();
}
/**
* Factory method for DataQualityAssertionsDatasetFacetAssertions@param assertion Type of expectation test that dataset is subjected to
* @param success the success
* @param column Column that expectation is testing. It should match the name provided in SchemaDatasetFacet. If column field is empty, then expectation refers to whole dataset.
* @return DataQualityAssertionsDatasetFacetAssertions
*/
public DataQualityAssertionsDatasetFacetAssertions newDataQualityAssertionsDatasetFacetAssertions(
String assertion, Boolean success, String column) {
return new DataQualityAssertionsDatasetFacetAssertions(assertion, success, column);
}
/**
* Creates a builder for DataQualityAssertionsDatasetFacetAssertions
* @return a new builder for DataQualityAssertionsDatasetFacetAssertions
*/
public DataQualityAssertionsDatasetFacetAssertionsBuilder newDataQualityAssertionsDatasetFacetAssertionsBuilder(
) {
return new DataQualityAssertionsDatasetFacetAssertionsBuilder();
}
/**
* Factory method for ColumnLineageDatasetFacetFieldsAdditionalInputFields@param namespace The input dataset namespace
* @param name The input dataset name
* @param field The input field
* @return ColumnLineageDatasetFacetFieldsAdditionalInputFields
*/
public ColumnLineageDatasetFacetFieldsAdditionalInputFields newColumnLineageDatasetFacetFieldsAdditionalInputFields(
String namespace, String name, String field) {
return new ColumnLineageDatasetFacetFieldsAdditionalInputFields(namespace, name, field);
}
/**
* Creates a builder for ColumnLineageDatasetFacetFieldsAdditionalInputFields
* @return a new builder for ColumnLineageDatasetFacetFieldsAdditionalInputFields
*/
public ColumnLineageDatasetFacetFieldsAdditionalInputFieldsBuilder newColumnLineageDatasetFacetFieldsAdditionalInputFieldsBuilder(
) {
return new ColumnLineageDatasetFacetFieldsAdditionalInputFieldsBuilder();
}
/**
* Factory method for ParentRunFacetRun@param runId The globally unique ID of the run associated with the job.
* @return ParentRunFacetRun
*/
public ParentRunFacetRun newParentRunFacetRun(UUID runId) {
return new ParentRunFacetRun(runId);
}
/**
* Creates a builder for ParentRunFacetRun
* @return a new builder for ParentRunFacetRun
*/
public ParentRunFacetRunBuilder newParentRunFacetRunBuilder() {
return new ParentRunFacetRunBuilder();
}
/**
* @return OutputDatasetFacet
*/
public OutputDatasetFacet newOutputDatasetFacet() {
return new DefaultOutputDatasetFacet(this.producer);
}
/**
* Factory method for StaticDataset@param namespace The namespace containing that dataset
* @param name The unique name for that dataset within that namespace
* @param facets The facets for this dataset
* @return StaticDataset
*/
public StaticDataset newStaticDataset(String namespace, String name, DatasetFacets facets) {
return new StaticDataset(namespace, name, facets);
}
/**
* Creates a builder for StaticDataset
* @return a new builder for StaticDataset
*/
public StaticDatasetBuilder newStaticDatasetBuilder() {
return new StaticDatasetBuilder();
}
/**
* Factory method for ColumnLineageDatasetFacetFields@return ColumnLineageDatasetFacetFields
*/
public ColumnLineageDatasetFacetFields newColumnLineageDatasetFacetFields() {
return new ColumnLineageDatasetFacetFields();
}
/**
* Creates a builder for ColumnLineageDatasetFacetFields
* @return a new builder for ColumnLineageDatasetFacetFields
*/
public ColumnLineageDatasetFacetFieldsBuilder newColumnLineageDatasetFacetFieldsBuilder() {
return new ColumnLineageDatasetFacetFieldsBuilder();
}
/**
* Factory method for SymlinksDatasetFacet@param identifiers the identifiers
* @return SymlinksDatasetFacet
*/
public SymlinksDatasetFacet newSymlinksDatasetFacet(
List identifiers) {
return new SymlinksDatasetFacet(this.producer, identifiers);
}
/**
* Creates a builder for SymlinksDatasetFacet
* @return a new builder for SymlinksDatasetFacet
*/
public SymlinksDatasetFacetBuilder newSymlinksDatasetFacetBuilder() {
return new SymlinksDatasetFacetBuilder();
}
/**
* Factory method for StorageDatasetFacet@param storageLayer Storage layer provider with allowed values: iceberg, delta.
* @param fileFormat File format with allowed values: parquet, orc, avro, json, csv, text, xml.
* @return StorageDatasetFacet
*/
public StorageDatasetFacet newStorageDatasetFacet(String storageLayer, String fileFormat) {
return new StorageDatasetFacet(this.producer, storageLayer, fileFormat);
}
/**
* Creates a builder for StorageDatasetFacet
* @return a new builder for StorageDatasetFacet
*/
public StorageDatasetFacetBuilder newStorageDatasetFacetBuilder() {
return new StorageDatasetFacetBuilder();
}
/**
* Factory method for InputDataset@param namespace The namespace containing that dataset
* @param name The unique name for that dataset within that namespace
* @param facets The facets for this dataset
* @param inputFacets The input facets for this dataset.
* @return InputDataset
*/
public InputDataset newInputDataset(String namespace, String name, DatasetFacets facets,
InputDatasetInputFacets inputFacets) {
return new InputDataset(namespace, name, facets, inputFacets);
}
/**
* Creates a builder for InputDataset
* @return a new builder for InputDataset
*/
public InputDatasetBuilder newInputDatasetBuilder() {
return new InputDatasetBuilder();
}
/**
* Factory method for DatasourceDatasetFacet@param name the name
* @param uri the uri
* @return DatasourceDatasetFacet
*/
public DatasourceDatasetFacet newDatasourceDatasetFacet(String name, URI uri) {
return new DatasourceDatasetFacet(this.producer, name, uri);
}
/**
* Creates a builder for DatasourceDatasetFacet
* @return a new builder for DatasourceDatasetFacet
*/
public DatasourceDatasetFacetBuilder newDatasourceDatasetFacetBuilder() {
return new DatasourceDatasetFacetBuilder();
}
/**
* Factory method for LifecycleStateChangeDatasetFacet@param lifecycleStateChange The lifecycle state change.
* @param previousIdentifier Previous name of the dataset in case of renaming it.
* @return LifecycleStateChangeDatasetFacet
*/
public LifecycleStateChangeDatasetFacet newLifecycleStateChangeDatasetFacet(
LifecycleStateChangeDatasetFacet.LifecycleStateChange lifecycleStateChange,
LifecycleStateChangeDatasetFacetPreviousIdentifier previousIdentifier) {
return new LifecycleStateChangeDatasetFacet(this.producer, lifecycleStateChange, previousIdentifier);
}
/**
* Creates a builder for LifecycleStateChangeDatasetFacet
* @return a new builder for LifecycleStateChangeDatasetFacet
*/
public LifecycleStateChangeDatasetFacetBuilder newLifecycleStateChangeDatasetFacetBuilder() {
return new LifecycleStateChangeDatasetFacetBuilder();
}
public interface Builder {
/**
* @return the constructed type
*/
T build();
}
/**
* model class for RunEvent
*/
@JsonDeserialize(
as = RunEvent.class
)
@JsonPropertyOrder({
"eventTime",
"producer",
"schemaURL",
"eventType",
"run",
"job",
"inputs",
"outputs"
})
public static final class RunEvent implements BaseEvent {
private final ZonedDateTime eventTime;
private final URI producer;
private final URI schemaURL;
private final RunEvent.EventType eventType;
private final Run run;
private final Job job;
private final List inputs;
private final List outputs;
/**
* @param eventTime the time the event occurred at
* @param producer URI identifying the producer of this metadata. For example this could be a git url with a given tag or sha
* @param eventType the current transition of the run state. It is required to issue 1 START event and 1 of [ COMPLETE, ABORT, FAIL ] event per run. Additional events with OTHER eventType can be added to the same run. For example to send additional metadata after the run is complete
* @param run the run
* @param job the job
* @param inputs The set of **input** datasets.
* @param outputs The set of **output** datasets.
*/
@JsonCreator
private RunEvent(@JsonProperty("eventTime") ZonedDateTime eventTime,
@JsonProperty("producer") URI producer,
@JsonProperty("eventType") RunEvent.EventType eventType, @JsonProperty("run") Run run,
@JsonProperty("job") Job job, @JsonProperty("inputs") List inputs,
@JsonProperty("outputs") List outputs) {
this.eventTime = eventTime;
this.producer = producer;
this.schemaURL = URI.create("https://openlineage.io/spec/2-0-2/OpenLineage.json#/$defs/RunEvent");
this.eventType = eventType;
this.run = run;
this.job = job;
this.inputs = inputs;
this.outputs = outputs;
}
/**
* @return the time the event occurred at
*/
@Override
public ZonedDateTime getEventTime() {
return eventTime;
}
/**
* @return URI identifying the producer of this metadata. For example this could be a git url with a given tag or sha
*/
@Override
public URI getProducer() {
return producer;
}
/**
* @return The JSON Pointer (https://tools.ietf.org/html/rfc6901) URL to the corresponding version of the schema definition for this RunEvent
*/
@Override
public URI getSchemaURL() {
return schemaURL;
}
/**
* @return the current transition of the run state. It is required to issue 1 START event and 1 of [ COMPLETE, ABORT, FAIL ] event per run. Additional events with OTHER eventType can be added to the same run. For example to send additional metadata after the run is complete
*/
public RunEvent.EventType getEventType() {
return eventType;
}
public Run getRun() {
return run;
}
public Job getJob() {
return job;
}
/**
* @return The set of **input** datasets.
*/
public List getInputs() {
return inputs;
}
/**
* @return The set of **output** datasets.
*/
public List getOutputs() {
return outputs;
}
public enum EventType {
START,
RUNNING,
COMPLETE,
ABORT,
FAIL,
OTHER
}
}
/**
* builder class for RunEvent
*/
public final class RunEventBuilder implements Builder {
private ZonedDateTime eventTime;
private RunEvent.EventType eventType;
private Run run;
private Job job;
private List inputs;
private List outputs;
/**
* @param eventTime the time the event occurred at
* @return this
*/
public RunEventBuilder eventTime(ZonedDateTime eventTime) {
this.eventTime = eventTime;
return this;
}
/**
* @param eventType the current transition of the run state. It is required to issue 1 START event and 1 of [ COMPLETE, ABORT, FAIL ] event per run. Additional events with OTHER eventType can be added to the same run. For example to send additional metadata after the run is complete
* @return this
*/
public RunEventBuilder eventType(RunEvent.EventType eventType) {
this.eventType = eventType;
return this;
}
/**
* @param run the run
* @return this
*/
public RunEventBuilder run(Run run) {
this.run = run;
return this;
}
/**
* @param job the job
* @return this
*/
public RunEventBuilder job(Job job) {
this.job = job;
return this;
}
/**
* @param inputs The set of **input** datasets.
* @return this
*/
public RunEventBuilder inputs(List inputs) {
this.inputs = inputs;
return this;
}
/**
* @param outputs The set of **output** datasets.
* @return this
*/
public RunEventBuilder outputs(List outputs) {
this.outputs = outputs;
return this;
}
/**
* build an instance of RunEvent from the fields set in the builder
*/
@Override
public RunEvent build() {
RunEvent __result = new RunEvent(eventTime, OpenLineage.this.producer, eventType, run, job, inputs, outputs);
return __result;
}
}
/**
* Interface for BaseEvent
*/
public interface BaseEvent {
/**
* @return the time the event occurred at
*/
ZonedDateTime getEventTime();
/**
* @return URI identifying the producer of this metadata. For example this could be a git url with a given tag or sha
*/
URI getProducer();
/**
* @return The JSON Pointer (https://tools.ietf.org/html/rfc6901) URL to the corresponding version of the schema definition for this RunEvent
*/
URI getSchemaURL();
}
/**
* model class for SchemaDatasetFacetFields
*/
@JsonDeserialize(
as = SchemaDatasetFacetFields.class
)
@JsonPropertyOrder({
"name",
"type",
"description",
"fields"
})
public static final class SchemaDatasetFacetFields {
private final String name;
private final String type;
private final String description;
private final List fields;
/**
* @param name The name of the field.
* @param type The type of the field.
* @param description The description of the field.
* @param fields Nested struct fields.
*/
@JsonCreator
private SchemaDatasetFacetFields(@JsonProperty("name") String name,
@JsonProperty("type") String type, @JsonProperty("description") String description,
@JsonProperty("fields") List fields) {
this.name = name;
this.type = type;
this.description = description;
this.fields = fields;
}
/**
* @return The name of the field.
*/
public String getName() {
return name;
}
/**
* @return The type of the field.
*/
public String getType() {
return type;
}
/**
* @return The description of the field.
*/
public String getDescription() {
return description;
}
/**
* @return Nested struct fields.
*/
public List getFields() {
return fields;
}
}
/**
* builder class for SchemaDatasetFacetFields
*/
public static final class SchemaDatasetFacetFieldsBuilder implements Builder {
private String name;
private String type;
private String description;
private List fields;
/**
* @param name The name of the field.
* @return this
*/
public SchemaDatasetFacetFieldsBuilder name(String name) {
this.name = name;
return this;
}
/**
* @param type The type of the field.
* @return this
*/
public SchemaDatasetFacetFieldsBuilder type(String type) {
this.type = type;
return this;
}
/**
* @param description The description of the field.
* @return this
*/
public SchemaDatasetFacetFieldsBuilder description(String description) {
this.description = description;
return this;
}
/**
* @param fields Nested struct fields.
* @return this
*/
public SchemaDatasetFacetFieldsBuilder fields(List fields) {
this.fields = fields;
return this;
}
/**
* build an instance of SchemaDatasetFacetFields from the fields set in the builder
*/
@Override
public SchemaDatasetFacetFields build() {
SchemaDatasetFacetFields __result = new SchemaDatasetFacetFields(name, type, description, fields);
return __result;
}
}
/**
* model class for ParentRunFacet
*/
@JsonDeserialize(
as = ParentRunFacet.class
)
@JsonPropertyOrder({
"_producer",
"_schemaURL",
"run",
"job"
})
public static final class ParentRunFacet implements RunFacet {
private final URI _producer;
private final URI _schemaURL;
private final ParentRunFacetRun run;
private final ParentRunFacetJob job;
@JsonAnySetter
private final Map additionalProperties;
/**
* @param _producer URI identifying the producer of this metadata. For example this could be a git url with a given tag or sha
* @param run the run
* @param job the job
*/
@JsonCreator
private ParentRunFacet(@JsonProperty("_producer") URI _producer,
@JsonProperty("run") ParentRunFacetRun run, @JsonProperty("job") ParentRunFacetJob job) {
this._producer = _producer;
this._schemaURL = URI.create("https://openlineage.io/spec/facets/1-0-0/ParentRunFacet.json#/$defs/ParentRunFacet");
this.run = run;
this.job = job;
this.additionalProperties = new LinkedHashMap<>();
}
/**
* @return URI identifying the producer of this metadata. For example this could be a git url with a given tag or sha
*/
@Override
public URI get_producer() {
return _producer;
}
/**
* @return The JSON Pointer (https://tools.ietf.org/html/rfc6901) URL to the corresponding version of the schema definition for this facet
*/
@Override
public URI get_schemaURL() {
return _schemaURL;
}
public ParentRunFacetRun getRun() {
return run;
}
public ParentRunFacetJob getJob() {
return job;
}
/**
* @return additional properties
*/
@JsonAnyGetter
@Override
public Map getAdditionalProperties() {
return additionalProperties;
}
/**
* Get object with additional properties
*/
void withAdditionalProperties() {
}
}
/**
* builder class for ParentRunFacet
*/
public final class ParentRunFacetBuilder implements Builder {
private ParentRunFacetRun run;
private ParentRunFacetJob job;
private final Map additionalProperties = new LinkedHashMap<>();
/**
* @param run the run
* @return this
*/
public ParentRunFacetBuilder run(ParentRunFacetRun run) {
this.run = run;
return this;
}
/**
* @param job the job
* @return this
*/
public ParentRunFacetBuilder job(ParentRunFacetJob job) {
this.job = job;
return this;
}
/**
* add additional properties
* @param key the additional property name
* @param value the additional property value
* @return this
*/
public ParentRunFacetBuilder put(String key, Object value) {
this.additionalProperties.put(key, value);return this;
}
/**
* build an instance of ParentRunFacet from the fields set in the builder
*/
@Override
public ParentRunFacet build() {
ParentRunFacet __result = new ParentRunFacet(OpenLineage.this.producer, run, job);
__result.getAdditionalProperties().putAll(additionalProperties);
return __result;
}
}
/**
* model class for OutputStatisticsOutputDatasetFacet
*/
@JsonDeserialize(
as = OutputStatisticsOutputDatasetFacet.class
)
@JsonPropertyOrder({
"_producer",
"_schemaURL",
"rowCount",
"size",
"fileCount"
})
public static final class OutputStatisticsOutputDatasetFacet implements OutputDatasetFacet {
private final URI _producer;
private final URI _schemaURL;
private final Long rowCount;
private final Long size;
private final Long fileCount;
@JsonAnySetter
private final Map additionalProperties;
/**
* @param _producer URI identifying the producer of this metadata. For example this could be a git url with a given tag or sha
* @param rowCount The number of rows written to the dataset
* @param size The size in bytes written to the dataset
* @param fileCount The number of files written to the dataset
*/
@JsonCreator
private OutputStatisticsOutputDatasetFacet(@JsonProperty("_producer") URI _producer,
@JsonProperty("rowCount") Long rowCount, @JsonProperty("size") Long size,
@JsonProperty("fileCount") Long fileCount) {
this._producer = _producer;
this._schemaURL = URI.create("https://openlineage.io/spec/facets/1-0-1/OutputStatisticsOutputDatasetFacet.json#/$defs/OutputStatisticsOutputDatasetFacet");
this.rowCount = rowCount;
this.size = size;
this.fileCount = fileCount;
this.additionalProperties = new LinkedHashMap<>();
}
/**
* @return URI identifying the producer of this metadata. For example this could be a git url with a given tag or sha
*/
@Override
public URI get_producer() {
return _producer;
}
/**
* @return The JSON Pointer (https://tools.ietf.org/html/rfc6901) URL to the corresponding version of the schema definition for this facet
*/
@Override
public URI get_schemaURL() {
return _schemaURL;
}
/**
* @return The number of rows written to the dataset
*/
public Long getRowCount() {
return rowCount;
}
/**
* @return The size in bytes written to the dataset
*/
public Long getSize() {
return size;
}
/**
* @return The number of files written to the dataset
*/
public Long getFileCount() {
return fileCount;
}
/**
* @return additional properties
*/
@JsonAnyGetter
@Override
public Map getAdditionalProperties() {
return additionalProperties;
}
/**
* Get object with additional properties
*/
void withAdditionalProperties() {
}
}
/**
* builder class for OutputStatisticsOutputDatasetFacet
*/
public final class OutputStatisticsOutputDatasetFacetBuilder implements Builder {
private Long rowCount;
private Long size;
private Long fileCount;
private final Map additionalProperties = new LinkedHashMap<>();
/**
* @param rowCount The number of rows written to the dataset
* @return this
*/
public OutputStatisticsOutputDatasetFacetBuilder rowCount(Long rowCount) {
this.rowCount = rowCount;
return this;
}
/**
* @param size The size in bytes written to the dataset
* @return this
*/
public OutputStatisticsOutputDatasetFacetBuilder size(Long size) {
this.size = size;
return this;
}
/**
* @param fileCount The number of files written to the dataset
* @return this
*/
public OutputStatisticsOutputDatasetFacetBuilder fileCount(Long fileCount) {
this.fileCount = fileCount;
return this;
}
/**
* add additional properties
* @param key the additional property name
* @param value the additional property value
* @return this
*/
public OutputStatisticsOutputDatasetFacetBuilder put(String key, Object value) {
this.additionalProperties.put(key, value);return this;
}
/**
* build an instance of OutputStatisticsOutputDatasetFacet from the fields set in the builder
*/
@Override
public OutputStatisticsOutputDatasetFacet build() {
OutputStatisticsOutputDatasetFacet __result = new OutputStatisticsOutputDatasetFacet(OpenLineage.this.producer, rowCount, size, fileCount);
__result.getAdditionalProperties().putAll(additionalProperties);
return __result;
}
}
/**
* model class for ExtractionErrorRunFacetErrors
*/
@JsonDeserialize(
as = ExtractionErrorRunFacetErrors.class
)
@JsonPropertyOrder({
"errorMessage",
"stackTrace",
"task",
"taskNumber"
})
public static final class ExtractionErrorRunFacetErrors {
private final String errorMessage;
private final String stackTrace;
private final String task;
private final Long taskNumber;
/**
* @param errorMessage Text representation of extraction error message.
* @param stackTrace Stack trace of extraction error message
* @param task Text representation of task that failed. This can be, for example, SQL statement that parser could not interpret.
* @param taskNumber Order of task (counted from 0).
*/
@JsonCreator
private ExtractionErrorRunFacetErrors(@JsonProperty("errorMessage") String errorMessage,
@JsonProperty("stackTrace") String stackTrace, @JsonProperty("task") String task,
@JsonProperty("taskNumber") Long taskNumber) {
this.errorMessage = errorMessage;
this.stackTrace = stackTrace;
this.task = task;
this.taskNumber = taskNumber;
}
/**
* @return Text representation of extraction error message.
*/
public String getErrorMessage() {
return errorMessage;
}
/**
* @return Stack trace of extraction error message
*/
public String getStackTrace() {
return stackTrace;
}
/**
* @return Text representation of task that failed. This can be, for example, SQL statement that parser could not interpret.
*/
public String getTask() {
return task;
}
/**
* @return Order of task (counted from 0).
*/
public Long getTaskNumber() {
return taskNumber;
}
}
/**
* builder class for ExtractionErrorRunFacetErrors
*/
public static final class ExtractionErrorRunFacetErrorsBuilder implements Builder {
private String errorMessage;
private String stackTrace;
private String task;
private Long taskNumber;
/**
* @param errorMessage Text representation of extraction error message.
* @return this
*/
public ExtractionErrorRunFacetErrorsBuilder errorMessage(String errorMessage) {
this.errorMessage = errorMessage;
return this;
}
/**
* @param stackTrace Stack trace of extraction error message
* @return this
*/
public ExtractionErrorRunFacetErrorsBuilder stackTrace(String stackTrace) {
this.stackTrace = stackTrace;
return this;
}
/**
* @param task Text representation of task that failed. This can be, for example, SQL statement that parser could not interpret.
* @return this
*/
public ExtractionErrorRunFacetErrorsBuilder task(String task) {
this.task = task;
return this;
}
/**
* @param taskNumber Order of task (counted from 0).
* @return this
*/
public ExtractionErrorRunFacetErrorsBuilder taskNumber(Long taskNumber) {
this.taskNumber = taskNumber;
return this;
}
/**
* build an instance of ExtractionErrorRunFacetErrors from the fields set in the builder
*/
@Override
public ExtractionErrorRunFacetErrors build() {
ExtractionErrorRunFacetErrors __result = new ExtractionErrorRunFacetErrors(errorMessage, stackTrace, task, taskNumber);
return __result;
}
}
/**
* model class for JobTypeJobFacet
*/
@JsonDeserialize(
as = JobTypeJobFacet.class
)
@JsonPropertyOrder({
"_producer",
"_schemaURL",
"_deleted",
"processingType",
"integration",
"jobType"
})
public static final class JobTypeJobFacet implements JobFacet {
private final URI _producer;
private final URI _schemaURL;
private final Boolean _deleted;
private final String processingType;
private final String integration;
private final String jobType;
@JsonAnySetter
private final Map additionalProperties;
/**
* @param _producer URI identifying the producer of this metadata. For example this could be a git url with a given tag or sha
* @param processingType Job processing type like: BATCH or STREAMING
* @param integration OpenLineage integration type of this job: SPARK|DBT|AIRFLOW|FLINK
* @param jobType Run type like: QUERY|COMMAND|DAG|TASK|JOB|MODEL
*/
@JsonCreator
private JobTypeJobFacet(@JsonProperty("_producer") URI _producer,
@JsonProperty("processingType") String processingType,
@JsonProperty("integration") String integration, @JsonProperty("jobType") String jobType) {
this._producer = _producer;
this._schemaURL = URI.create("https://openlineage.io/spec/facets/2-0-2/JobTypeJobFacet.json#/$defs/JobTypeJobFacet");
this._deleted = null;
this.processingType = processingType;
this.integration = integration;
this.jobType = jobType;
this.additionalProperties = new LinkedHashMap<>();
}
/**
* @return URI identifying the producer of this metadata. For example this could be a git url with a given tag or sha
*/
@Override
public URI get_producer() {
return _producer;
}
/**
* @return The JSON Pointer (https://tools.ietf.org/html/rfc6901) URL to the corresponding version of the schema definition for this facet
*/
@Override
public URI get_schemaURL() {
return _schemaURL;
}
/**
* @return set to true to delete a facet
*/
@Override
public Boolean get_deleted() {
return _deleted;
}
/**
* @return Job processing type like: BATCH or STREAMING
*/
public String getProcessingType() {
return processingType;
}
/**
* @return OpenLineage integration type of this job: SPARK|DBT|AIRFLOW|FLINK
*/
public String getIntegration() {
return integration;
}
/**
* @return Run type like: QUERY|COMMAND|DAG|TASK|JOB|MODEL
*/
public String getJobType() {
return jobType;
}
/**
* @return additional properties
*/
@JsonAnyGetter
@Override
public Map getAdditionalProperties() {
return additionalProperties;
}
/**
* Get object with additional properties
*/
void withAdditionalProperties() {
}
}
/**
* builder class for JobTypeJobFacet
*/
public final class JobTypeJobFacetBuilder implements Builder {
private String processingType;
private String integration;
private String jobType;
private final Map additionalProperties = new LinkedHashMap<>();
/**
* @param processingType Job processing type like: BATCH or STREAMING
* @return this
*/
public JobTypeJobFacetBuilder processingType(String processingType) {
this.processingType = processingType;
return this;
}
/**
* @param integration OpenLineage integration type of this job: SPARK|DBT|AIRFLOW|FLINK
* @return this
*/
public JobTypeJobFacetBuilder integration(String integration) {
this.integration = integration;
return this;
}
/**
* @param jobType Run type like: QUERY|COMMAND|DAG|TASK|JOB|MODEL
* @return this
*/
public JobTypeJobFacetBuilder jobType(String jobType) {
this.jobType = jobType;
return this;
}
/**
* add additional properties
* @param key the additional property name
* @param value the additional property value
* @return this
*/
public JobTypeJobFacetBuilder put(String key, Object value) {
this.additionalProperties.put(key, value);return this;
}
/**
* build an instance of JobTypeJobFacet from the fields set in the builder
*/
@Override
public JobTypeJobFacet build() {
JobTypeJobFacet __result = new JobTypeJobFacet(OpenLineage.this.producer, processingType, integration, jobType);
__result.getAdditionalProperties().putAll(additionalProperties);
return __result;
}
}
/**
* model class for Run
*/
@JsonDeserialize(
as = Run.class
)
@JsonPropertyOrder({
"runId",
"facets"
})
public static final class Run {
private final UUID runId;
private final RunFacets facets;
/**
* @param runId The globally unique ID of the run associated with the job.
* @param facets The run facets.
*/
@JsonCreator
private Run(@JsonProperty("runId") UUID runId, @JsonProperty("facets") RunFacets facets) {
this.runId = runId;
this.facets = facets;
}
/**
* @return The globally unique ID of the run associated with the job.
*/
public UUID getRunId() {
return runId;
}
/**
* @return The run facets.
*/
public RunFacets getFacets() {
return facets;
}
}
/**
* builder class for Run
*/
public static final class RunBuilder implements Builder {
private UUID runId;
private RunFacets facets;
/**
* @param runId The globally unique ID of the run associated with the job.
* @return this
*/
public RunBuilder runId(UUID runId) {
this.runId = runId;
return this;
}
/**
* @param facets The run facets.
* @return this
*/
public RunBuilder facets(RunFacets facets) {
this.facets = facets;
return this;
}
/**
* build an instance of Run from the fields set in the builder
*/
@Override
public Run build() {
Run __result = new Run(runId, facets);
return __result;
}
}
/**
* model class for DataQualityMetricsInputDatasetFacetColumnMetricsAdditionalQuantiles
*/
@JsonDeserialize(
as = DataQualityMetricsInputDatasetFacetColumnMetricsAdditionalQuantiles.class
)
@JsonPropertyOrder
public static final class DataQualityMetricsInputDatasetFacetColumnMetricsAdditionalQuantiles {
@JsonAnySetter
private final Map additionalProperties;
@JsonCreator
private DataQualityMetricsInputDatasetFacetColumnMetricsAdditionalQuantiles() {
this.additionalProperties = new LinkedHashMap<>();
}
/**
* @return additional properties
*/
@JsonAnyGetter
public Map getAdditionalProperties() {
return additionalProperties;
}
/**
* Get object with additional properties
*/
void withAdditionalProperties() {
}
}
/**
* builder class for DataQualityMetricsInputDatasetFacetColumnMetricsAdditionalQuantiles
*/
public static final class DataQualityMetricsInputDatasetFacetColumnMetricsAdditionalQuantilesBuilder implements Builder {
private final Map additionalProperties = new LinkedHashMap<>();
/**
* add additional properties
* @param key the additional property name
* @param value the additional property value
* @return this
*/
public DataQualityMetricsInputDatasetFacetColumnMetricsAdditionalQuantilesBuilder put(
String key, Double value) {
this.additionalProperties.put(key, value);return this;
}
/**
* build an instance of DataQualityMetricsInputDatasetFacetColumnMetricsAdditionalQuantiles from the fields set in the builder
*/
@Override
public DataQualityMetricsInputDatasetFacetColumnMetricsAdditionalQuantiles build() {
DataQualityMetricsInputDatasetFacetColumnMetricsAdditionalQuantiles __result = new DataQualityMetricsInputDatasetFacetColumnMetricsAdditionalQuantiles();
__result.getAdditionalProperties().putAll(additionalProperties);
return __result;
}
}
/**
* model class for ExternalQueryRunFacet
*/
@JsonDeserialize(
as = ExternalQueryRunFacet.class
)
@JsonPropertyOrder({
"_producer",
"_schemaURL",
"externalQueryId",
"source"
})
public static final class ExternalQueryRunFacet implements RunFacet {
private final URI _producer;
private final URI _schemaURL;
private final String externalQueryId;
private final String source;
@JsonAnySetter
private final Map additionalProperties;
/**
* @param _producer URI identifying the producer of this metadata. For example this could be a git url with a given tag or sha
* @param externalQueryId Identifier for the external system
* @param source source of the external query
*/
@JsonCreator
private ExternalQueryRunFacet(@JsonProperty("_producer") URI _producer,
@JsonProperty("externalQueryId") String externalQueryId,
@JsonProperty("source") String source) {
this._producer = _producer;
this._schemaURL = URI.create("https://openlineage.io/spec/facets/1-0-0/ExternalQueryRunFacet.json#/$defs/ExternalQueryRunFacet");
this.externalQueryId = externalQueryId;
this.source = source;
this.additionalProperties = new LinkedHashMap<>();
}
/**
* @return URI identifying the producer of this metadata. For example this could be a git url with a given tag or sha
*/
@Override
public URI get_producer() {
return _producer;
}
/**
* @return The JSON Pointer (https://tools.ietf.org/html/rfc6901) URL to the corresponding version of the schema definition for this facet
*/
@Override
public URI get_schemaURL() {
return _schemaURL;
}
/**
* @return Identifier for the external system
*/
public String getExternalQueryId() {
return externalQueryId;
}
/**
* @return source of the external query
*/
public String getSource() {
return source;
}
/**
* @return additional properties
*/
@JsonAnyGetter
@Override
public Map getAdditionalProperties() {
return additionalProperties;
}
/**
* Get object with additional properties
*/
void withAdditionalProperties() {
}
}
/**
* builder class for ExternalQueryRunFacet
*/
public final class ExternalQueryRunFacetBuilder implements Builder {
private String externalQueryId;
private String source;
private final Map additionalProperties = new LinkedHashMap<>();
/**
* @param externalQueryId Identifier for the external system
* @return this
*/
public ExternalQueryRunFacetBuilder externalQueryId(String externalQueryId) {
this.externalQueryId = externalQueryId;
return this;
}
/**
* @param source source of the external query
* @return this
*/
public ExternalQueryRunFacetBuilder source(String source) {
this.source = source;
return this;
}
/**
* add additional properties
* @param key the additional property name
* @param value the additional property value
* @return this
*/
public ExternalQueryRunFacetBuilder put(String key, Object value) {
this.additionalProperties.put(key, value);return this;
}
/**
* build an instance of ExternalQueryRunFacet from the fields set in the builder
*/
@Override
public ExternalQueryRunFacet build() {
ExternalQueryRunFacet __result = new ExternalQueryRunFacet(OpenLineage.this.producer, externalQueryId, source);
__result.getAdditionalProperties().putAll(additionalProperties);
return __result;
}
}
/**
* model class for OutputDatasetOutputFacets
*/
@JsonDeserialize(
as = OutputDatasetOutputFacets.class
)
@JsonPropertyOrder("outputStatistics")
public static final class OutputDatasetOutputFacets {
private final OutputStatisticsOutputDatasetFacet outputStatistics;
@JsonAnySetter
private final Map additionalProperties;
/**
* @param outputStatistics the outputStatistics
*/
@JsonCreator
private OutputDatasetOutputFacets(
@JsonProperty("outputStatistics") OutputStatisticsOutputDatasetFacet outputStatistics) {
this.outputStatistics = outputStatistics;
this.additionalProperties = new LinkedHashMap<>();
}
public OutputStatisticsOutputDatasetFacet getOutputStatistics() {
return outputStatistics;
}
/**
* @return additional properties
*/
@JsonAnyGetter
public Map getAdditionalProperties() {
return additionalProperties;
}
/**
* Get object with additional properties
*/
void withAdditionalProperties() {
}
}
/**
* builder class for OutputDatasetOutputFacets
*/
public static final class OutputDatasetOutputFacetsBuilder implements Builder {
private OutputStatisticsOutputDatasetFacet outputStatistics;
private final Map additionalProperties = new LinkedHashMap<>();
/**
* @param outputStatistics the outputStatistics
* @return this
*/
public OutputDatasetOutputFacetsBuilder outputStatistics(
OutputStatisticsOutputDatasetFacet outputStatistics) {
this.outputStatistics = outputStatistics;
return this;
}
/**
* add additional properties
* @param key the additional property name
* @param value the additional property value
* @return this
*/
public OutputDatasetOutputFacetsBuilder put(String key, OutputDatasetFacet value) {
this.additionalProperties.put(key, value);return this;
}
/**
* build an instance of OutputDatasetOutputFacets from the fields set in the builder
*/
@Override
public OutputDatasetOutputFacets build() {
OutputDatasetOutputFacets __result = new OutputDatasetOutputFacets(outputStatistics);
__result.getAdditionalProperties().putAll(additionalProperties);
return __result;
}
}
/**
* model class for NominalTimeRunFacet
*/
@JsonDeserialize(
as = NominalTimeRunFacet.class
)
@JsonPropertyOrder({
"_producer",
"_schemaURL",
"nominalStartTime",
"nominalEndTime"
})
public static final class NominalTimeRunFacet implements RunFacet {
private final URI _producer;
private final URI _schemaURL;
private final ZonedDateTime nominalStartTime;
private final ZonedDateTime nominalEndTime;
@JsonAnySetter
private final Map additionalProperties;
/**
* @param _producer URI identifying the producer of this metadata. For example this could be a git url with a given tag or sha
* @param nominalStartTime An [ISO-8601](https://en.wikipedia.org/wiki/ISO_8601) timestamp representing the nominal start time (included) of the run. AKA the schedule time
* @param nominalEndTime An [ISO-8601](https://en.wikipedia.org/wiki/ISO_8601) timestamp representing the nominal end time (excluded) of the run. (Should be the nominal start time of the next run)
*/
@JsonCreator
private NominalTimeRunFacet(@JsonProperty("_producer") URI _producer,
@JsonProperty("nominalStartTime") ZonedDateTime nominalStartTime,
@JsonProperty("nominalEndTime") ZonedDateTime nominalEndTime) {
this._producer = _producer;
this._schemaURL = URI.create("https://openlineage.io/spec/facets/1-0-0/NominalTimeRunFacet.json#/$defs/NominalTimeRunFacet");
this.nominalStartTime = nominalStartTime;
this.nominalEndTime = nominalEndTime;
this.additionalProperties = new LinkedHashMap<>();
}
/**
* @return URI identifying the producer of this metadata. For example this could be a git url with a given tag or sha
*/
@Override
public URI get_producer() {
return _producer;
}
/**
* @return The JSON Pointer (https://tools.ietf.org/html/rfc6901) URL to the corresponding version of the schema definition for this facet
*/
@Override
public URI get_schemaURL() {
return _schemaURL;
}
/**
* @return An [ISO-8601](https://en.wikipedia.org/wiki/ISO_8601) timestamp representing the nominal start time (included) of the run. AKA the schedule time
*/
public ZonedDateTime getNominalStartTime() {
return nominalStartTime;
}
/**
* @return An [ISO-8601](https://en.wikipedia.org/wiki/ISO_8601) timestamp representing the nominal end time (excluded) of the run. (Should be the nominal start time of the next run)
*/
public ZonedDateTime getNominalEndTime() {
return nominalEndTime;
}
/**
* @return additional properties
*/
@JsonAnyGetter
@Override
public Map getAdditionalProperties() {
return additionalProperties;
}
/**
* Get object with additional properties
*/
void withAdditionalProperties() {
}
}
/**
* builder class for NominalTimeRunFacet
*/
public final class NominalTimeRunFacetBuilder implements Builder {
private ZonedDateTime nominalStartTime;
private ZonedDateTime nominalEndTime;
private final Map additionalProperties = new LinkedHashMap<>();
/**
* @param nominalStartTime An [ISO-8601](https://en.wikipedia.org/wiki/ISO_8601) timestamp representing the nominal start time (included) of the run. AKA the schedule time
* @return this
*/
public NominalTimeRunFacetBuilder nominalStartTime(ZonedDateTime nominalStartTime) {
this.nominalStartTime = nominalStartTime;
return this;
}
/**
* @param nominalEndTime An [ISO-8601](https://en.wikipedia.org/wiki/ISO_8601) timestamp representing the nominal end time (excluded) of the run. (Should be the nominal start time of the next run)
* @return this
*/
public NominalTimeRunFacetBuilder nominalEndTime(ZonedDateTime nominalEndTime) {
this.nominalEndTime = nominalEndTime;
return this;
}
/**
* add additional properties
* @param key the additional property name
* @param value the additional property value
* @return this
*/
public NominalTimeRunFacetBuilder put(String key, Object value) {
this.additionalProperties.put(key, value);return this;
}
/**
* build an instance of NominalTimeRunFacet from the fields set in the builder
*/
@Override
public NominalTimeRunFacet build() {
NominalTimeRunFacet __result = new NominalTimeRunFacet(OpenLineage.this.producer, nominalStartTime, nominalEndTime);
__result.getAdditionalProperties().putAll(additionalProperties);
return __result;
}
}
public static class DefaultRunFacet implements RunFacet {
private final URI _producer;
private final URI _schemaURL;
@JsonAnySetter
private final Map additionalProperties;
/**
* @param _producer URI identifying the producer of this metadata. For example this could be a git url with a given tag or sha
*/
@JsonCreator
public DefaultRunFacet(@JsonProperty("_producer") URI _producer) {
this._producer = _producer;
this._schemaURL = URI.create("https://openlineage.io/spec/2-0-2/OpenLineage.json#/$defs/RunFacet");
this.additionalProperties = new LinkedHashMap<>();
}
/**
* @return URI identifying the producer of this metadata. For example this could be a git url with a given tag or sha
*/
@Override
public URI get_producer() {
return _producer;
}
/**
* @return The JSON Pointer (https://tools.ietf.org/html/rfc6901) URL to the corresponding version of the schema definition for this facet
*/
@Override
public URI get_schemaURL() {
return _schemaURL;
}
/**
* @return additional properties
*/
@JsonAnyGetter
@Override
public Map getAdditionalProperties() {
return additionalProperties;
}
}
/**
* Interface for RunFacet
*/
@JsonDeserialize(
as = DefaultRunFacet.class
)
public interface RunFacet {
/**
* @return URI identifying the producer of this metadata. For example this could be a git url with a given tag or sha
*/
URI get_producer();
/**
* @return The JSON Pointer (https://tools.ietf.org/html/rfc6901) URL to the corresponding version of the schema definition for this facet
*/
URI get_schemaURL();
/**
* @return additional properties
*/
Map getAdditionalProperties();
}
/**
* Interface for BaseFacet
*/
public interface BaseFacet {
/**
* @return URI identifying the producer of this metadata. For example this could be a git url with a given tag or sha
*/
URI get_producer();
/**
* @return The JSON Pointer (https://tools.ietf.org/html/rfc6901) URL to the corresponding version of the schema definition for this facet
*/
URI get_schemaURL();
/**
* @return additional properties
*/
Map getAdditionalProperties();
}
/**
* model class for OwnershipJobFacetOwners
*/
@JsonDeserialize(
as = OwnershipJobFacetOwners.class
)
@JsonPropertyOrder({
"name",
"type"
})
public static final class OwnershipJobFacetOwners {
private final String name;
private final String type;
/**
* @param name the identifier of the owner of the Job. It is recommended to define this as a URN. For example application:foo, user:jdoe, team:data
* @param type The type of ownership (optional)
*/
@JsonCreator
private OwnershipJobFacetOwners(@JsonProperty("name") String name,
@JsonProperty("type") String type) {
this.name = name;
this.type = type;
}
/**
* @return the identifier of the owner of the Job. It is recommended to define this as a URN. For example application:foo, user:jdoe, team:data
*/
public String getName() {
return name;
}
/**
* @return The type of ownership (optional)
*/
public String getType() {
return type;
}
}
/**
* builder class for OwnershipJobFacetOwners
*/
public static final class OwnershipJobFacetOwnersBuilder implements Builder {
private String name;
private String type;
/**
* @param name the identifier of the owner of the Job. It is recommended to define this as a URN. For example application:foo, user:jdoe, team:data
* @return this
*/
public OwnershipJobFacetOwnersBuilder name(String name) {
this.name = name;
return this;
}
/**
* @param type The type of ownership (optional)
* @return this
*/
public OwnershipJobFacetOwnersBuilder type(String type) {
this.type = type;
return this;
}
/**
* build an instance of OwnershipJobFacetOwners from the fields set in the builder
*/
@Override
public OwnershipJobFacetOwners build() {
OwnershipJobFacetOwners __result = new OwnershipJobFacetOwners(name, type);
return __result;
}
}
public static class DefaultInputDatasetFacet implements InputDatasetFacet {
private final URI _producer;
private final URI _schemaURL;
@JsonAnySetter
private final Map additionalProperties;
/**
* @param _producer URI identifying the producer of this metadata. For example this could be a git url with a given tag or sha
*/
@JsonCreator
public DefaultInputDatasetFacet(@JsonProperty("_producer") URI _producer) {
this._producer = _producer;
this._schemaURL = URI.create("https://openlineage.io/spec/2-0-2/OpenLineage.json#/$defs/InputDatasetFacet");
this.additionalProperties = new LinkedHashMap<>();
}
/**
* @return URI identifying the producer of this metadata. For example this could be a git url with a given tag or sha
*/
@Override
public URI get_producer() {
return _producer;
}
/**
* @return The JSON Pointer (https://tools.ietf.org/html/rfc6901) URL to the corresponding version of the schema definition for this facet
*/
@Override
public URI get_schemaURL() {
return _schemaURL;
}
/**
* @return additional properties
*/
@JsonAnyGetter
@Override
public Map getAdditionalProperties() {
return additionalProperties;
}
}
/**
* Interface for InputDatasetFacet
*/
@JsonDeserialize(
as = DefaultInputDatasetFacet.class
)
public interface InputDatasetFacet {
/**
* @return URI identifying the producer of this metadata. For example this could be a git url with a given tag or sha
*/
URI get_producer();
/**
* @return The JSON Pointer (https://tools.ietf.org/html/rfc6901) URL to the corresponding version of the schema definition for this facet
*/
URI get_schemaURL();
/**
* @return additional properties
*/
Map getAdditionalProperties();
}
/**
* model class for JobEvent
*/
@JsonDeserialize(
as = JobEvent.class
)
@JsonPropertyOrder({
"eventTime",
"producer",
"schemaURL",
"job",
"inputs",
"outputs"
})
public static final class JobEvent implements BaseEvent {
private final ZonedDateTime eventTime;
private final URI producer;
private final URI schemaURL;
private final Job job;
private final List inputs;
private final List outputs;
/**
* @param eventTime the time the event occurred at
* @param producer URI identifying the producer of this metadata. For example this could be a git url with a given tag or sha
* @param job the job
* @param inputs The set of **input** datasets.
* @param outputs The set of **output** datasets.
*/
@JsonCreator
private JobEvent(@JsonProperty("eventTime") ZonedDateTime eventTime,
@JsonProperty("producer") URI producer, @JsonProperty("job") Job job,
@JsonProperty("inputs") List inputs,
@JsonProperty("outputs") List outputs) {
this.eventTime = eventTime;
this.producer = producer;
this.schemaURL = URI.create("https://openlineage.io/spec/2-0-2/OpenLineage.json#/$defs/JobEvent");
this.job = job;
this.inputs = inputs;
this.outputs = outputs;
}
/**
* @return the time the event occurred at
*/
@Override
public ZonedDateTime getEventTime() {
return eventTime;
}
/**
* @return URI identifying the producer of this metadata. For example this could be a git url with a given tag or sha
*/
@Override
public URI getProducer() {
return producer;
}
/**
* @return The JSON Pointer (https://tools.ietf.org/html/rfc6901) URL to the corresponding version of the schema definition for this RunEvent
*/
@Override
public URI getSchemaURL() {
return schemaURL;
}
public Job getJob() {
return job;
}
/**
* @return The set of **input** datasets.
*/
public List getInputs() {
return inputs;
}
/**
* @return The set of **output** datasets.
*/
public List getOutputs() {
return outputs;
}
}
/**
* builder class for JobEvent
*/
public final class JobEventBuilder implements Builder {
private ZonedDateTime eventTime;
private Job job;
private List inputs;
private List outputs;
/**
* @param eventTime the time the event occurred at
* @return this
*/
public JobEventBuilder eventTime(ZonedDateTime eventTime) {
this.eventTime = eventTime;
return this;
}
/**
* @param job the job
* @return this
*/
public JobEventBuilder job(Job job) {
this.job = job;
return this;
}
/**
* @param inputs The set of **input** datasets.
* @return this
*/
public JobEventBuilder inputs(List inputs) {
this.inputs = inputs;
return this;
}
/**
* @param outputs The set of **output** datasets.
* @return this
*/
public JobEventBuilder outputs(List outputs) {
this.outputs = outputs;
return this;
}
/**
* build an instance of JobEvent from the fields set in the builder
*/
@Override
public JobEvent build() {
JobEvent __result = new JobEvent(eventTime, OpenLineage.this.producer, job, inputs, outputs);
return __result;
}
}
/**
* model class for ColumnLineageDatasetFacetFieldsAdditional
*/
@JsonDeserialize(
as = ColumnLineageDatasetFacetFieldsAdditional.class
)
@JsonPropertyOrder({
"inputFields",
"transformationDescription",
"transformationType"
})
public static final class ColumnLineageDatasetFacetFieldsAdditional {
private final List inputFields;
private final String transformationDescription;
private final String transformationType;
@JsonAnySetter
private final Map additionalProperties;
/**
* @param inputFields the inputFields
* @param transformationDescription a string representation of the transformation applied
* @param transformationType IDENTITY|MASKED reflects a clearly defined behavior. IDENTITY: exact same as input; MASKED: no original data available (like a hash of PII for example)
*/
@JsonCreator
private ColumnLineageDatasetFacetFieldsAdditional(
@JsonProperty("inputFields") List inputFields,
@JsonProperty("transformationDescription") String transformationDescription,
@JsonProperty("transformationType") String transformationType) {
this.inputFields = inputFields;
this.transformationDescription = transformationDescription;
this.transformationType = transformationType;
this.additionalProperties = new LinkedHashMap<>();
}
public List getInputFields() {
return inputFields;
}
/**
* @return a string representation of the transformation applied
*/
public String getTransformationDescription() {
return transformationDescription;
}
/**
* @return IDENTITY|MASKED reflects a clearly defined behavior. IDENTITY: exact same as input; MASKED: no original data available (like a hash of PII for example)
*/
public String getTransformationType() {
return transformationType;
}
/**
* @return additional properties
*/
@JsonAnyGetter
public Map getAdditionalProperties() {
return additionalProperties;
}
/**
* Get object with additional properties
*/
void withAdditionalProperties() {
}
}
/**
* builder class for ColumnLineageDatasetFacetFieldsAdditional
*/
public static final class ColumnLineageDatasetFacetFieldsAdditionalBuilder implements Builder {
private List inputFields;
private String transformationDescription;
private String transformationType;
private final Map additionalProperties = new LinkedHashMap<>();
/**
* @param inputFields the inputFields
* @return this
*/
public ColumnLineageDatasetFacetFieldsAdditionalBuilder inputFields(
List inputFields) {
this.inputFields = inputFields;
return this;
}
/**
* @param transformationDescription a string representation of the transformation applied
* @return this
*/
public ColumnLineageDatasetFacetFieldsAdditionalBuilder transformationDescription(
String transformationDescription) {
this.transformationDescription = transformationDescription;
return this;
}
/**
* @param transformationType IDENTITY|MASKED reflects a clearly defined behavior. IDENTITY: exact same as input; MASKED: no original data available (like a hash of PII for example)
* @return this
*/
public ColumnLineageDatasetFacetFieldsAdditionalBuilder transformationType(
String transformationType) {
this.transformationType = transformationType;
return this;
}
/**
* add additional properties
* @param key the additional property name
* @param value the additional property value
* @return this
*/
public ColumnLineageDatasetFacetFieldsAdditionalBuilder put(String key, Object value) {
this.additionalProperties.put(key, value);return this;
}
/**
* build an instance of ColumnLineageDatasetFacetFieldsAdditional from the fields set in the builder
*/
@Override
public ColumnLineageDatasetFacetFieldsAdditional build() {
ColumnLineageDatasetFacetFieldsAdditional __result = new ColumnLineageDatasetFacetFieldsAdditional(inputFields, transformationDescription, transformationType);
__result.getAdditionalProperties().putAll(additionalProperties);
return __result;
}
}
/**
* model class for ExtractionErrorRunFacet
*/
@JsonDeserialize(
as = ExtractionErrorRunFacet.class
)
@JsonPropertyOrder({
"_producer",
"_schemaURL",
"totalTasks",
"failedTasks",
"errors"
})
public static final class ExtractionErrorRunFacet implements RunFacet {
private final URI _producer;
private final URI _schemaURL;
private final Long totalTasks;
private final Long failedTasks;
private final List errors;
@JsonAnySetter
private final Map additionalProperties;
/**
* @param _producer URI identifying the producer of this metadata. For example this could be a git url with a given tag or sha
* @param totalTasks The number of distinguishable tasks in a run that were processed by OpenLineage, whether successfully or not. Those could be, for example, distinct SQL statements.
* @param failedTasks The number of distinguishable tasks in a run that were processed not successfully by OpenLineage. Those could be, for example, distinct SQL statements.
* @param errors the errors
*/
@JsonCreator
private ExtractionErrorRunFacet(@JsonProperty("_producer") URI _producer,
@JsonProperty("totalTasks") Long totalTasks, @JsonProperty("failedTasks") Long failedTasks,
@JsonProperty("errors") List errors) {
this._producer = _producer;
this._schemaURL = URI.create("https://openlineage.io/spec/facets/1-1-0/ExtractionErrorRunFacet.json#/$defs/ExtractionErrorRunFacet");
this.totalTasks = totalTasks;
this.failedTasks = failedTasks;
this.errors = errors;
this.additionalProperties = new LinkedHashMap<>();
}
/**
* @return URI identifying the producer of this metadata. For example this could be a git url with a given tag or sha
*/
@Override
public URI get_producer() {
return _producer;
}
/**
* @return The JSON Pointer (https://tools.ietf.org/html/rfc6901) URL to the corresponding version of the schema definition for this facet
*/
@Override
public URI get_schemaURL() {
return _schemaURL;
}
/**
* @return The number of distinguishable tasks in a run that were processed by OpenLineage, whether successfully or not. Those could be, for example, distinct SQL statements.
*/
public Long getTotalTasks() {
return totalTasks;
}
/**
* @return The number of distinguishable tasks in a run that were processed not successfully by OpenLineage. Those could be, for example, distinct SQL statements.
*/
public Long getFailedTasks() {
return failedTasks;
}
public List getErrors() {
return errors;
}
/**
* @return additional properties
*/
@JsonAnyGetter
@Override
public Map getAdditionalProperties() {
return additionalProperties;
}
/**
* Get object with additional properties
*/
void withAdditionalProperties() {
}
}
/**
* builder class for ExtractionErrorRunFacet
*/
public final class ExtractionErrorRunFacetBuilder implements Builder {
private Long totalTasks;
private Long failedTasks;
private List errors;
private final Map additionalProperties = new LinkedHashMap<>();
/**
* @param totalTasks The number of distinguishable tasks in a run that were processed by OpenLineage, whether successfully or not. Those could be, for example, distinct SQL statements.
* @return this
*/
public ExtractionErrorRunFacetBuilder totalTasks(Long totalTasks) {
this.totalTasks = totalTasks;
return this;
}
/**
* @param failedTasks The number of distinguishable tasks in a run that were processed not successfully by OpenLineage. Those could be, for example, distinct SQL statements.
* @return this
*/
public ExtractionErrorRunFacetBuilder failedTasks(Long failedTasks) {
this.failedTasks = failedTasks;
return this;
}
/**
* @param errors the errors
* @return this
*/
public ExtractionErrorRunFacetBuilder errors(List errors) {
this.errors = errors;
return this;
}
/**
* add additional properties
* @param key the additional property name
* @param value the additional property value
* @return this
*/
public ExtractionErrorRunFacetBuilder put(String key, Object value) {
this.additionalProperties.put(key, value);return this;
}
/**
* build an instance of ExtractionErrorRunFacet from the fields set in the builder
*/
@Override
public ExtractionErrorRunFacet build() {
ExtractionErrorRunFacet __result = new ExtractionErrorRunFacet(OpenLineage.this.producer, totalTasks, failedTasks, errors);
__result.getAdditionalProperties().putAll(additionalProperties);
return __result;
}
}
/**
* model class for OwnershipDatasetFacetOwners
*/
@JsonDeserialize(
as = OwnershipDatasetFacetOwners.class
)
@JsonPropertyOrder({
"name",
"type"
})
public static final class OwnershipDatasetFacetOwners {
private final String name;
private final String type;
/**
* @param name the identifier of the owner of the Dataset. It is recommended to define this as a URN. For example application:foo, user:jdoe, team:data
* @param type The type of ownership (optional)
*/
@JsonCreator
private OwnershipDatasetFacetOwners(@JsonProperty("name") String name,
@JsonProperty("type") String type) {
this.name = name;
this.type = type;
}
/**
* @return the identifier of the owner of the Dataset. It is recommended to define this as a URN. For example application:foo, user:jdoe, team:data
*/
public String getName() {
return name;
}
/**
* @return The type of ownership (optional)
*/
public String getType() {
return type;
}
}
/**
* builder class for OwnershipDatasetFacetOwners
*/
public static final class OwnershipDatasetFacetOwnersBuilder implements Builder {
private String name;
private String type;
/**
* @param name the identifier of the owner of the Dataset. It is recommended to define this as a URN. For example application:foo, user:jdoe, team:data
* @return this
*/
public OwnershipDatasetFacetOwnersBuilder name(String name) {
this.name = name;
return this;
}
/**
* @param type The type of ownership (optional)
* @return this
*/
public OwnershipDatasetFacetOwnersBuilder type(String type) {
this.type = type;
return this;
}
/**
* build an instance of OwnershipDatasetFacetOwners from the fields set in the builder
*/
@Override
public OwnershipDatasetFacetOwners build() {
OwnershipDatasetFacetOwners __result = new OwnershipDatasetFacetOwners(name, type);
return __result;
}
}
/**
* model class for DataQualityMetricsInputDatasetFacetColumnMetricsAdditional
*/
@JsonDeserialize(
as = DataQualityMetricsInputDatasetFacetColumnMetricsAdditional.class
)
@JsonPropertyOrder({
"nullCount",
"distinctCount",
"sum",
"count",
"min",
"max",
"quantiles"
})
public static final class DataQualityMetricsInputDatasetFacetColumnMetricsAdditional {
private final Long nullCount;
private final Long distinctCount;
private final Double sum;
private final Double count;
private final Double min;
private final Double max;
private final DataQualityMetricsInputDatasetFacetColumnMetricsAdditionalQuantiles quantiles;
/**
* @param nullCount The number of null values in this column for the rows evaluated
* @param distinctCount The number of distinct values in this column for the rows evaluated
* @param sum The total sum of values in this column for the rows evaluated
* @param count The number of values in this column
* @param min the min
* @param max the max
* @param quantiles The property key is the quantile. Examples: 0.1 0.25 0.5 0.75 1
*/
@JsonCreator
private DataQualityMetricsInputDatasetFacetColumnMetricsAdditional(
@JsonProperty("nullCount") Long nullCount,
@JsonProperty("distinctCount") Long distinctCount, @JsonProperty("sum") Double sum,
@JsonProperty("count") Double count, @JsonProperty("min") Double min,
@JsonProperty("max") Double max,
@JsonProperty("quantiles") DataQualityMetricsInputDatasetFacetColumnMetricsAdditionalQuantiles quantiles) {
this.nullCount = nullCount;
this.distinctCount = distinctCount;
this.sum = sum;
this.count = count;
this.min = min;
this.max = max;
this.quantiles = quantiles;
}
/**
* @return The number of null values in this column for the rows evaluated
*/
public Long getNullCount() {
return nullCount;
}
/**
* @return The number of distinct values in this column for the rows evaluated
*/
public Long getDistinctCount() {
return distinctCount;
}
/**
* @return The total sum of values in this column for the rows evaluated
*/
public Double getSum() {
return sum;
}
/**
* @return The number of values in this column
*/
public Double getCount() {
return count;
}
public Double getMin() {
return min;
}
public Double getMax() {
return max;
}
/**
* @return The property key is the quantile. Examples: 0.1 0.25 0.5 0.75 1
*/
public DataQualityMetricsInputDatasetFacetColumnMetricsAdditionalQuantiles getQuantiles() {
return quantiles;
}
}
/**
* builder class for DataQualityMetricsInputDatasetFacetColumnMetricsAdditional
*/
public static final class DataQualityMetricsInputDatasetFacetColumnMetricsAdditionalBuilder implements Builder {
private Long nullCount;
private Long distinctCount;
private Double sum;
private Double count;
private Double min;
private Double max;
private DataQualityMetricsInputDatasetFacetColumnMetricsAdditionalQuantiles quantiles;
/**
* @param nullCount The number of null values in this column for the rows evaluated
* @return this
*/
public DataQualityMetricsInputDatasetFacetColumnMetricsAdditionalBuilder nullCount(
Long nullCount) {
this.nullCount = nullCount;
return this;
}
/**
* @param distinctCount The number of distinct values in this column for the rows evaluated
* @return this
*/
public DataQualityMetricsInputDatasetFacetColumnMetricsAdditionalBuilder distinctCount(
Long distinctCount) {
this.distinctCount = distinctCount;
return this;
}
/**
* @param sum The total sum of values in this column for the rows evaluated
* @return this
*/
public DataQualityMetricsInputDatasetFacetColumnMetricsAdditionalBuilder sum(Double sum) {
this.sum = sum;
return this;
}
/**
* @param count The number of values in this column
* @return this
*/
public DataQualityMetricsInputDatasetFacetColumnMetricsAdditionalBuilder count(Double count) {
this.count = count;
return this;
}
/**
* @param min the min
* @return this
*/
public DataQualityMetricsInputDatasetFacetColumnMetricsAdditionalBuilder min(Double min) {
this.min = min;
return this;
}
/**
* @param max the max
* @return this
*/
public DataQualityMetricsInputDatasetFacetColumnMetricsAdditionalBuilder max(Double max) {
this.max = max;
return this;
}
/**
* @param quantiles The property key is the quantile. Examples: 0.1 0.25 0.5 0.75 1
* @return this
*/
public DataQualityMetricsInputDatasetFacetColumnMetricsAdditionalBuilder quantiles(
DataQualityMetricsInputDatasetFacetColumnMetricsAdditionalQuantiles quantiles) {
this.quantiles = quantiles;
return this;
}
/**
* build an instance of DataQualityMetricsInputDatasetFacetColumnMetricsAdditional from the fields set in the builder
*/
@Override
public DataQualityMetricsInputDatasetFacetColumnMetricsAdditional build() {
DataQualityMetricsInputDatasetFacetColumnMetricsAdditional __result = new DataQualityMetricsInputDatasetFacetColumnMetricsAdditional(nullCount, distinctCount, sum, count, min, max, quantiles);
return __result;
}
}
/**
* model class for DataQualityMetricsInputDatasetFacetColumnMetrics
*/
@JsonDeserialize(
as = DataQualityMetricsInputDatasetFacetColumnMetrics.class
)
@JsonPropertyOrder
public static final class DataQualityMetricsInputDatasetFacetColumnMetrics {
@JsonAnySetter
private final Map additionalProperties;
@JsonCreator
private DataQualityMetricsInputDatasetFacetColumnMetrics() {
this.additionalProperties = new LinkedHashMap<>();
}
/**
* @return additional properties
*/
@JsonAnyGetter
public Map getAdditionalProperties(
) {
return additionalProperties;
}
/**
* Get object with additional properties
*/
void withAdditionalProperties() {
}
}
/**
* builder class for DataQualityMetricsInputDatasetFacetColumnMetrics
*/
public static final class DataQualityMetricsInputDatasetFacetColumnMetricsBuilder implements Builder {
private final Map additionalProperties = new LinkedHashMap<>();
/**
* add additional properties
* @param key the additional property name
* @param value the additional property value
* @return this
*/
public DataQualityMetricsInputDatasetFacetColumnMetricsBuilder put(String key,
DataQualityMetricsInputDatasetFacetColumnMetricsAdditional value) {
this.additionalProperties.put(key, value);return this;
}
/**
* build an instance of DataQualityMetricsInputDatasetFacetColumnMetrics from the fields set in the builder
*/
@Override
public DataQualityMetricsInputDatasetFacetColumnMetrics build() {
DataQualityMetricsInputDatasetFacetColumnMetrics __result = new DataQualityMetricsInputDatasetFacetColumnMetrics();
__result.getAdditionalProperties().putAll(additionalProperties);
return __result;
}
}
/**
* model class for DataQualityMetricsInputDatasetFacet
*/
@JsonDeserialize(
as = DataQualityMetricsInputDatasetFacet.class
)
@JsonPropertyOrder({
"_producer",
"_schemaURL",
"rowCount",
"bytes",
"fileCount",
"columnMetrics"
})
public static final class DataQualityMetricsInputDatasetFacet implements InputDatasetFacet {
private final URI _producer;
private final URI _schemaURL;
private final Long rowCount;
private final Long bytes;
private final Long fileCount;
private final DataQualityMetricsInputDatasetFacetColumnMetrics columnMetrics;
@JsonAnySetter
private final Map additionalProperties;
/**
* @param _producer URI identifying the producer of this metadata. For example this could be a git url with a given tag or sha
* @param rowCount The number of rows evaluated
* @param bytes The size in bytes
* @param fileCount The number of files evaluated
* @param columnMetrics The property key is the column name
*/
@JsonCreator
private DataQualityMetricsInputDatasetFacet(@JsonProperty("_producer") URI _producer,
@JsonProperty("rowCount") Long rowCount, @JsonProperty("bytes") Long bytes,
@JsonProperty("fileCount") Long fileCount,
@JsonProperty("columnMetrics") DataQualityMetricsInputDatasetFacetColumnMetrics columnMetrics) {
this._producer = _producer;
this._schemaURL = URI.create("https://openlineage.io/spec/facets/1-0-1/DataQualityMetricsInputDatasetFacet.json#/$defs/DataQualityMetricsInputDatasetFacet");
this.rowCount = rowCount;
this.bytes = bytes;
this.fileCount = fileCount;
this.columnMetrics = columnMetrics;
this.additionalProperties = new LinkedHashMap<>();
}
/**
* @return URI identifying the producer of this metadata. For example this could be a git url with a given tag or sha
*/
@Override
public URI get_producer() {
return _producer;
}
/**
* @return The JSON Pointer (https://tools.ietf.org/html/rfc6901) URL to the corresponding version of the schema definition for this facet
*/
@Override
public URI get_schemaURL() {
return _schemaURL;
}
/**
* @return The number of rows evaluated
*/
public Long getRowCount() {
return rowCount;
}
/**
* @return The size in bytes
*/
public Long getBytes() {
return bytes;
}
/**
* @return The number of files evaluated
*/
public Long getFileCount() {
return fileCount;
}
/**
* @return The property key is the column name
*/
public DataQualityMetricsInputDatasetFacetColumnMetrics getColumnMetrics() {
return columnMetrics;
}
/**
* @return additional properties
*/
@JsonAnyGetter
@Override
public Map getAdditionalProperties() {
return additionalProperties;
}
/**
* Get object with additional properties
*/
void withAdditionalProperties() {
}
}
/**
* builder class for DataQualityMetricsInputDatasetFacet
*/
public final class DataQualityMetricsInputDatasetFacetBuilder implements Builder {
private Long rowCount;
private Long bytes;
private Long fileCount;
private DataQualityMetricsInputDatasetFacetColumnMetrics columnMetrics;
private final Map additionalProperties = new LinkedHashMap<>();
/**
* @param rowCount The number of rows evaluated
* @return this
*/
public DataQualityMetricsInputDatasetFacetBuilder rowCount(Long rowCount) {
this.rowCount = rowCount;
return this;
}
/**
* @param bytes The size in bytes
* @return this
*/
public DataQualityMetricsInputDatasetFacetBuilder bytes(Long bytes) {
this.bytes = bytes;
return this;
}
/**
* @param fileCount The number of files evaluated
* @return this
*/
public DataQualityMetricsInputDatasetFacetBuilder fileCount(Long fileCount) {
this.fileCount = fileCount;
return this;
}
/**
* @param columnMetrics The property key is the column name
* @return this
*/
public DataQualityMetricsInputDatasetFacetBuilder columnMetrics(
DataQualityMetricsInputDatasetFacetColumnMetrics columnMetrics) {
this.columnMetrics = columnMetrics;
return this;
}
/**
* add additional properties
* @param key the additional property name
* @param value the additional property value
* @return this
*/
public DataQualityMetricsInputDatasetFacetBuilder put(String key, Object value) {
this.additionalProperties.put(key, value);return this;
}
/**
* build an instance of DataQualityMetricsInputDatasetFacet from the fields set in the builder
*/
@Override
public DataQualityMetricsInputDatasetFacet build() {
DataQualityMetricsInputDatasetFacet __result = new DataQualityMetricsInputDatasetFacet(OpenLineage.this.producer, rowCount, bytes, fileCount, columnMetrics);
__result.getAdditionalProperties().putAll(additionalProperties);
return __result;
}
}
/**
* model class for DocumentationJobFacet
*/
@JsonDeserialize(
as = DocumentationJobFacet.class
)
@JsonPropertyOrder({
"_producer",
"_schemaURL",
"_deleted",
"description"
})
public static final class DocumentationJobFacet implements JobFacet {
private final URI _producer;
private final URI _schemaURL;
private final Boolean _deleted;
private final String description;
@JsonAnySetter
private final Map additionalProperties;
/**
* @param _producer URI identifying the producer of this metadata. For example this could be a git url with a given tag or sha
* @param description The description of the job.
*/
@JsonCreator
private DocumentationJobFacet(@JsonProperty("_producer") URI _producer,
@JsonProperty("description") String description) {
this._producer = _producer;
this._schemaURL = URI.create("https://openlineage.io/spec/facets/1-0-0/DocumentationJobFacet.json#/$defs/DocumentationJobFacet");
this._deleted = null;
this.description = description;
this.additionalProperties = new LinkedHashMap<>();
}
/**
* @return URI identifying the producer of this metadata. For example this could be a git url with a given tag or sha
*/
@Override
public URI get_producer() {
return _producer;
}
/**
* @return The JSON Pointer (https://tools.ietf.org/html/rfc6901) URL to the corresponding version of the schema definition for this facet
*/
@Override
public URI get_schemaURL() {
return _schemaURL;
}
/**
* @return set to true to delete a facet
*/
@Override
public Boolean get_deleted() {
return _deleted;
}
/**
* @return The description of the job.
*/
public String getDescription() {
return description;
}
/**
* @return additional properties
*/
@JsonAnyGetter
@Override
public Map getAdditionalProperties() {
return additionalProperties;
}
/**
* Get object with additional properties
*/
void withAdditionalProperties() {
}
}
/**
* builder class for DocumentationJobFacet
*/
public final class DocumentationJobFacetBuilder implements Builder {
private String description;
private final Map additionalProperties = new LinkedHashMap<>();
/**
* @param description The description of the job.
* @return this
*/
public DocumentationJobFacetBuilder description(String description) {
this.description = description;
return this;
}
/**
* add additional properties
* @param key the additional property name
* @param value the additional property value
* @return this
*/
public DocumentationJobFacetBuilder put(String key, Object value) {
this.additionalProperties.put(key, value);return this;
}
/**
* build an instance of DocumentationJobFacet from the fields set in the builder
*/
@Override
public DocumentationJobFacet build() {
DocumentationJobFacet __result = new DocumentationJobFacet(OpenLineage.this.producer, description);
__result.getAdditionalProperties().putAll(additionalProperties);
return __result;
}
}
/**
* model class for JobFacets
*/
@JsonDeserialize(
as = JobFacets.class
)
@JsonPropertyOrder({
"jobType",
"sourceCode",
"ownership",
"sql",
"sourceCodeLocation",
"documentation"
})
public static final class JobFacets {
private final JobTypeJobFacet jobType;
private final SourceCodeJobFacet sourceCode;
private final OwnershipJobFacet ownership;
private final SQLJobFacet sql;
private final SourceCodeLocationJobFacet sourceCodeLocation;
private final DocumentationJobFacet documentation;
@JsonAnySetter
private final Map additionalProperties;
/**
* @param jobType the jobType
* @param sourceCode the sourceCode
* @param ownership the ownership
* @param sql the sql
* @param sourceCodeLocation the sourceCodeLocation
* @param documentation the documentation
*/
@JsonCreator
private JobFacets(@JsonProperty("jobType") JobTypeJobFacet jobType,
@JsonProperty("sourceCode") SourceCodeJobFacet sourceCode,
@JsonProperty("ownership") OwnershipJobFacet ownership,
@JsonProperty("sql") SQLJobFacet sql,
@JsonProperty("sourceCodeLocation") SourceCodeLocationJobFacet sourceCodeLocation,
@JsonProperty("documentation") DocumentationJobFacet documentation) {
this.jobType = jobType;
this.sourceCode = sourceCode;
this.ownership = ownership;
this.sql = sql;
this.sourceCodeLocation = sourceCodeLocation;
this.documentation = documentation;
this.additionalProperties = new LinkedHashMap<>();
}
public JobTypeJobFacet getJobType() {
return jobType;
}
public SourceCodeJobFacet getSourceCode() {
return sourceCode;
}
public OwnershipJobFacet getOwnership() {
return ownership;
}
public SQLJobFacet getSql() {
return sql;
}
public SourceCodeLocationJobFacet getSourceCodeLocation() {
return sourceCodeLocation;
}
public DocumentationJobFacet getDocumentation() {
return documentation;
}
/**
* @return additional properties
*/
@JsonAnyGetter
public Map getAdditionalProperties() {
return additionalProperties;
}
/**
* Get object with additional properties
*/
void withAdditionalProperties() {
}
}
/**
* builder class for JobFacets
*/
public static final class JobFacetsBuilder implements Builder {
private JobTypeJobFacet jobType;
private SourceCodeJobFacet sourceCode;
private OwnershipJobFacet ownership;
private SQLJobFacet sql;
private SourceCodeLocationJobFacet sourceCodeLocation;
private DocumentationJobFacet documentation;
private final Map additionalProperties = new LinkedHashMap<>();
/**
* @param jobType the jobType
* @return this
*/
public JobFacetsBuilder jobType(JobTypeJobFacet jobType) {
this.jobType = jobType;
return this;
}
/**
* @param sourceCode the sourceCode
* @return this
*/
public JobFacetsBuilder sourceCode(SourceCodeJobFacet sourceCode) {
this.sourceCode = sourceCode;
return this;
}
/**
* @param ownership the ownership
* @return this
*/
public JobFacetsBuilder ownership(OwnershipJobFacet ownership) {
this.ownership = ownership;
return this;
}
/**
* @param sql the sql
* @return this
*/
public JobFacetsBuilder sql(SQLJobFacet sql) {
this.sql = sql;
return this;
}
/**
* @param sourceCodeLocation the sourceCodeLocation
* @return this
*/
public JobFacetsBuilder sourceCodeLocation(SourceCodeLocationJobFacet sourceCodeLocation) {
this.sourceCodeLocation = sourceCodeLocation;
return this;
}
/**
* @param documentation the documentation
* @return this
*/
public JobFacetsBuilder documentation(DocumentationJobFacet documentation) {
this.documentation = documentation;
return this;
}
/**
* add additional properties
* @param key the additional property name
* @param value the additional property value
* @return this
*/
public JobFacetsBuilder put(String key, JobFacet value) {
this.additionalProperties.put(key, value);return this;
}
/**
* build an instance of JobFacets from the fields set in the builder
*/
@Override
public JobFacets build() {
JobFacets __result = new JobFacets(jobType, sourceCode, ownership, sql, sourceCodeLocation, documentation);
__result.getAdditionalProperties().putAll(additionalProperties);
return __result;
}
}
/**
* model class for InputDatasetInputFacets
*/
@JsonDeserialize(
as = InputDatasetInputFacets.class
)
@JsonPropertyOrder({
"dataQualityAssertions",
"dataQualityMetrics"
})
public static final class InputDatasetInputFacets {
private final DataQualityAssertionsDatasetFacet dataQualityAssertions;
private final DataQualityMetricsInputDatasetFacet dataQualityMetrics;
@JsonAnySetter
private final Map additionalProperties;
/**
* @param dataQualityAssertions the dataQualityAssertions
* @param dataQualityMetrics the dataQualityMetrics
*/
@JsonCreator
private InputDatasetInputFacets(
@JsonProperty("dataQualityAssertions") DataQualityAssertionsDatasetFacet dataQualityAssertions,
@JsonProperty("dataQualityMetrics") DataQualityMetricsInputDatasetFacet dataQualityMetrics) {
this.dataQualityAssertions = dataQualityAssertions;
this.dataQualityMetrics = dataQualityMetrics;
this.additionalProperties = new LinkedHashMap<>();
}
public DataQualityAssertionsDatasetFacet getDataQualityAssertions() {
return dataQualityAssertions;
}
public DataQualityMetricsInputDatasetFacet getDataQualityMetrics() {
return dataQualityMetrics;
}
/**
* @return additional properties
*/
@JsonAnyGetter
public Map getAdditionalProperties() {
return additionalProperties;
}
/**
* Get object with additional properties
*/
void withAdditionalProperties() {
}
}
/**
* builder class for InputDatasetInputFacets
*/
public static final class InputDatasetInputFacetsBuilder implements Builder {
private DataQualityAssertionsDatasetFacet dataQualityAssertions;
private DataQualityMetricsInputDatasetFacet dataQualityMetrics;
private final Map additionalProperties = new LinkedHashMap<>();
/**
* @param dataQualityAssertions the dataQualityAssertions
* @return this
*/
public InputDatasetInputFacetsBuilder dataQualityAssertions(
DataQualityAssertionsDatasetFacet dataQualityAssertions) {
this.dataQualityAssertions = dataQualityAssertions;
return this;
}
/**
* @param dataQualityMetrics the dataQualityMetrics
* @return this
*/
public InputDatasetInputFacetsBuilder dataQualityMetrics(
DataQualityMetricsInputDatasetFacet dataQualityMetrics) {
this.dataQualityMetrics = dataQualityMetrics;
return this;
}
/**
* add additional properties
* @param key the additional property name
* @param value the additional property value
* @return this
*/
public InputDatasetInputFacetsBuilder put(String key, InputDatasetFacet value) {
this.additionalProperties.put(key, value);return this;
}
/**
* build an instance of InputDatasetInputFacets from the fields set in the builder
*/
@Override
public InputDatasetInputFacets build() {
InputDatasetInputFacets __result = new InputDatasetInputFacets(dataQualityAssertions, dataQualityMetrics);
__result.getAdditionalProperties().putAll(additionalProperties);
return __result;
}
}
public static class DefaultDatasetFacet implements DatasetFacet {
private final URI _producer;
private final URI _schemaURL;
private final Boolean _deleted;
@JsonAnySetter
private final Map additionalProperties;
/**
* @param _producer URI identifying the producer of this metadata. For example this could be a git url with a given tag or sha
* @param _deleted set to true to delete a facet
*/
@JsonCreator
public DefaultDatasetFacet(@JsonProperty("_producer") URI _producer,
@JsonProperty("_deleted") Boolean _deleted) {
this._producer = _producer;
this._schemaURL = URI.create("https://openlineage.io/spec/2-0-2/OpenLineage.json#/$defs/DatasetFacet");
this._deleted = _deleted;
this.additionalProperties = new LinkedHashMap<>();
}
/**
* @return URI identifying the producer of this metadata. For example this could be a git url with a given tag or sha
*/
@Override
public URI get_producer() {
return _producer;
}
/**
* @return The JSON Pointer (https://tools.ietf.org/html/rfc6901) URL to the corresponding version of the schema definition for this facet
*/
@Override
public URI get_schemaURL() {
return _schemaURL;
}
/**
* @return set to true to delete a facet
*/
@Override
public Boolean get_deleted() {
return _deleted;
}
/**
* @return additional properties
*/
@JsonAnyGetter
@Override
public Map getAdditionalProperties() {
return additionalProperties;
}
}
/**
* Interface for DatasetFacet
*/
@JsonDeserialize(
as = DefaultDatasetFacet.class
)
public interface DatasetFacet {
/**
* @return URI identifying the producer of this metadata. For example this could be a git url with a given tag or sha
*/
URI get_producer();
/**
* @return The JSON Pointer (https://tools.ietf.org/html/rfc6901) URL to the corresponding version of the schema definition for this facet
*/
URI get_schemaURL();
/**
* @return set to true to delete a facet
*/
Boolean get_deleted();
/**
* @return set to true to delete a facet
*/
@JsonIgnore
default Boolean isDeleted() {
return get_deleted();
}
/**
* @return additional properties
*/
Map getAdditionalProperties();
}
/**
* model class for OwnershipDatasetFacet
*/
@JsonDeserialize(
as = OwnershipDatasetFacet.class
)
@JsonPropertyOrder({
"_producer",
"_schemaURL",
"_deleted",
"owners"
})
public static final class OwnershipDatasetFacet implements DatasetFacet {
private final URI _producer;
private final URI _schemaURL;
private final Boolean _deleted;
private final List owners;
@JsonAnySetter
private final Map additionalProperties;
/**
* @param _producer URI identifying the producer of this metadata. For example this could be a git url with a given tag or sha
* @param owners The owners of the dataset.
*/
@JsonCreator
private OwnershipDatasetFacet(@JsonProperty("_producer") URI _producer,
@JsonProperty("owners") List owners) {
this._producer = _producer;
this._schemaURL = URI.create("https://openlineage.io/spec/facets/1-0-0/OwnershipDatasetFacet.json#/$defs/OwnershipDatasetFacet");
this._deleted = null;
this.owners = owners;
this.additionalProperties = new LinkedHashMap<>();
}
/**
* @return URI identifying the producer of this metadata. For example this could be a git url with a given tag or sha
*/
@Override
public URI get_producer() {
return _producer;
}
/**
* @return The JSON Pointer (https://tools.ietf.org/html/rfc6901) URL to the corresponding version of the schema definition for this facet
*/
@Override
public URI get_schemaURL() {
return _schemaURL;
}
/**
* @return set to true to delete a facet
*/
@Override
public Boolean get_deleted() {
return _deleted;
}
/**
* @return The owners of the dataset.
*/
public List getOwners() {
return owners;
}
/**
* @return additional properties
*/
@JsonAnyGetter
@Override
public Map getAdditionalProperties() {
return additionalProperties;
}
/**
* Get object with additional properties
*/
void withAdditionalProperties() {
}
}
/**
* builder class for OwnershipDatasetFacet
*/
public final class OwnershipDatasetFacetBuilder implements Builder {
private List owners;
private final Map additionalProperties = new LinkedHashMap<>();
/**
* @param owners The owners of the dataset.
* @return this
*/
public OwnershipDatasetFacetBuilder owners(List owners) {
this.owners = owners;
return this;
}
/**
* add additional properties
* @param key the additional property name
* @param value the additional property value
* @return this
*/
public OwnershipDatasetFacetBuilder put(String key, Object value) {
this.additionalProperties.put(key, value);return this;
}
/**
* build an instance of OwnershipDatasetFacet from the fields set in the builder
*/
@Override
public OwnershipDatasetFacet build() {
OwnershipDatasetFacet __result = new OwnershipDatasetFacet(OpenLineage.this.producer, owners);
__result.getAdditionalProperties().putAll(additionalProperties);
return __result;
}
}
/**
* model class for SQLJobFacet
*/
@JsonDeserialize(
as = SQLJobFacet.class
)
@JsonPropertyOrder({
"_producer",
"_schemaURL",
"_deleted",
"query"
})
public static final class SQLJobFacet implements JobFacet {
private final URI _producer;
private final URI _schemaURL;
private final Boolean _deleted;
private final String query;
@JsonAnySetter
private final Map additionalProperties;
/**
* @param _producer URI identifying the producer of this metadata. For example this could be a git url with a given tag or sha
* @param query the query
*/
@JsonCreator
private SQLJobFacet(@JsonProperty("_producer") URI _producer,
@JsonProperty("query") String query) {
this._producer = _producer;
this._schemaURL = URI.create("https://openlineage.io/spec/facets/1-0-0/SQLJobFacet.json#/$defs/SQLJobFacet");
this._deleted = null;
this.query = query;
this.additionalProperties = new LinkedHashMap<>();
}
/**
* @return URI identifying the producer of this metadata. For example this could be a git url with a given tag or sha
*/
@Override
public URI get_producer() {
return _producer;
}
/**
* @return The JSON Pointer (https://tools.ietf.org/html/rfc6901) URL to the corresponding version of the schema definition for this facet
*/
@Override
public URI get_schemaURL() {
return _schemaURL;
}
/**
* @return set to true to delete a facet
*/
@Override
public Boolean get_deleted() {
return _deleted;
}
public String getQuery() {
return query;
}
/**
* @return additional properties
*/
@JsonAnyGetter
@Override
public Map getAdditionalProperties() {
return additionalProperties;
}
/**
* Get object with additional properties
*/
void withAdditionalProperties() {
}
}
/**
* builder class for SQLJobFacet
*/
public final class SQLJobFacetBuilder implements Builder {
private String query;
private final Map additionalProperties = new LinkedHashMap<>();
/**
* @param query the query
* @return this
*/
public SQLJobFacetBuilder query(String query) {
this.query = query;
return this;
}
/**
* add additional properties
* @param key the additional property name
* @param value the additional property value
* @return this
*/
public SQLJobFacetBuilder put(String key, Object value) {
this.additionalProperties.put(key, value);return this;
}
/**
* build an instance of SQLJobFacet from the fields set in the builder
*/
@Override
public SQLJobFacet build() {
SQLJobFacet __result = new SQLJobFacet(OpenLineage.this.producer, query);
__result.getAdditionalProperties().putAll(additionalProperties);
return __result;
}
}
/**
* model class for DatasetFacets
*/
@JsonDeserialize(
as = DatasetFacets.class
)
@JsonPropertyOrder({
"documentation",
"dataSource",
"version",
"schema",
"ownership",
"storage",
"columnLineage",
"symlinks",
"lifecycleStateChange"
})
public static final class DatasetFacets {
private final DocumentationDatasetFacet documentation;
private final DatasourceDatasetFacet dataSource;
private final DatasetVersionDatasetFacet version;
private final SchemaDatasetFacet schema;
private final OwnershipDatasetFacet ownership;
private final StorageDatasetFacet storage;
private final ColumnLineageDatasetFacet columnLineage;
private final SymlinksDatasetFacet symlinks;
private final LifecycleStateChangeDatasetFacet lifecycleStateChange;
@JsonAnySetter
private final Map additionalProperties;
/**
* @param documentation the documentation
* @param dataSource the dataSource
* @param version the version
* @param schema the schema
* @param ownership the ownership
* @param storage the storage
* @param columnLineage the columnLineage
* @param symlinks the symlinks
* @param lifecycleStateChange the lifecycleStateChange
*/
@JsonCreator
private DatasetFacets(@JsonProperty("documentation") DocumentationDatasetFacet documentation,
@JsonProperty("dataSource") DatasourceDatasetFacet dataSource,
@JsonProperty("version") DatasetVersionDatasetFacet version,
@JsonProperty("schema") SchemaDatasetFacet schema,
@JsonProperty("ownership") OwnershipDatasetFacet ownership,
@JsonProperty("storage") StorageDatasetFacet storage,
@JsonProperty("columnLineage") ColumnLineageDatasetFacet columnLineage,
@JsonProperty("symlinks") SymlinksDatasetFacet symlinks,
@JsonProperty("lifecycleStateChange") LifecycleStateChangeDatasetFacet lifecycleStateChange) {
this.documentation = documentation;
this.dataSource = dataSource;
this.version = version;
this.schema = schema;
this.ownership = ownership;
this.storage = storage;
this.columnLineage = columnLineage;
this.symlinks = symlinks;
this.lifecycleStateChange = lifecycleStateChange;
this.additionalProperties = new LinkedHashMap<>();
}
public DocumentationDatasetFacet getDocumentation() {
return documentation;
}
public DatasourceDatasetFacet getDataSource() {
return dataSource;
}
public DatasetVersionDatasetFacet getVersion() {
return version;
}
public SchemaDatasetFacet getSchema() {
return schema;
}
public OwnershipDatasetFacet getOwnership() {
return ownership;
}
public StorageDatasetFacet getStorage() {
return storage;
}
public ColumnLineageDatasetFacet getColumnLineage() {
return columnLineage;
}
public SymlinksDatasetFacet getSymlinks() {
return symlinks;
}
public LifecycleStateChangeDatasetFacet getLifecycleStateChange() {
return lifecycleStateChange;
}
/**
* @return additional properties
*/
@JsonAnyGetter
public Map getAdditionalProperties() {
return additionalProperties;
}
/**
* Get object with additional properties
*/
void withAdditionalProperties() {
}
}
/**
* builder class for DatasetFacets
*/
public static final class DatasetFacetsBuilder implements Builder {
private DocumentationDatasetFacet documentation;
private DatasourceDatasetFacet dataSource;
private DatasetVersionDatasetFacet version;
private SchemaDatasetFacet schema;
private OwnershipDatasetFacet ownership;
private StorageDatasetFacet storage;
private ColumnLineageDatasetFacet columnLineage;
private SymlinksDatasetFacet symlinks;
private LifecycleStateChangeDatasetFacet lifecycleStateChange;
private final Map additionalProperties = new LinkedHashMap<>();
/**
* @param documentation the documentation
* @return this
*/
public DatasetFacetsBuilder documentation(DocumentationDatasetFacet documentation) {
this.documentation = documentation;
return this;
}
/**
* @param dataSource the dataSource
* @return this
*/
public DatasetFacetsBuilder dataSource(DatasourceDatasetFacet dataSource) {
this.dataSource = dataSource;
return this;
}
/**
* @param version the version
* @return this
*/
public DatasetFacetsBuilder version(DatasetVersionDatasetFacet version) {
this.version = version;
return this;
}
/**
* @param schema the schema
* @return this
*/
public DatasetFacetsBuilder schema(SchemaDatasetFacet schema) {
this.schema = schema;
return this;
}
/**
* @param ownership the ownership
* @return this
*/
public DatasetFacetsBuilder ownership(OwnershipDatasetFacet ownership) {
this.ownership = ownership;
return this;
}
/**
* @param storage the storage
* @return this
*/
public DatasetFacetsBuilder storage(StorageDatasetFacet storage) {
this.storage = storage;
return this;
}
/**
* @param columnLineage the columnLineage
* @return this
*/
public DatasetFacetsBuilder columnLineage(ColumnLineageDatasetFacet columnLineage) {
this.columnLineage = columnLineage;
return this;
}
/**
* @param symlinks the symlinks
* @return this
*/
public DatasetFacetsBuilder symlinks(SymlinksDatasetFacet symlinks) {
this.symlinks = symlinks;
return this;
}
/**
* @param lifecycleStateChange the lifecycleStateChange
* @return this
*/
public DatasetFacetsBuilder lifecycleStateChange(
LifecycleStateChangeDatasetFacet lifecycleStateChange) {
this.lifecycleStateChange = lifecycleStateChange;
return this;
}
/**
* add additional properties
* @param key the additional property name
* @param value the additional property value
* @return this
*/
public DatasetFacetsBuilder put(String key, DatasetFacet value) {
this.additionalProperties.put(key, value);return this;
}
/**
* build an instance of DatasetFacets from the fields set in the builder
*/
@Override
public DatasetFacets build() {
DatasetFacets __result = new DatasetFacets(documentation, dataSource, version, schema, ownership, storage, columnLineage, symlinks, lifecycleStateChange);
__result.getAdditionalProperties().putAll(additionalProperties);
return __result;
}
}
/**
* model class for OwnershipJobFacet
*/
@JsonDeserialize(
as = OwnershipJobFacet.class
)
@JsonPropertyOrder({
"_producer",
"_schemaURL",
"_deleted",
"owners"
})
public static final class OwnershipJobFacet implements JobFacet {
private final URI _producer;
private final URI _schemaURL;
private final Boolean _deleted;
private final List owners;
@JsonAnySetter
private final Map additionalProperties;
/**
* @param _producer URI identifying the producer of this metadata. For example this could be a git url with a given tag or sha
* @param owners The owners of the job.
*/
@JsonCreator
private OwnershipJobFacet(@JsonProperty("_producer") URI _producer,
@JsonProperty("owners") List owners) {
this._producer = _producer;
this._schemaURL = URI.create("https://openlineage.io/spec/facets/1-0-0/OwnershipJobFacet.json#/$defs/OwnershipJobFacet");
this._deleted = null;
this.owners = owners;
this.additionalProperties = new LinkedHashMap<>();
}
/**
* @return URI identifying the producer of this metadata. For example this could be a git url with a given tag or sha
*/
@Override
public URI get_producer() {
return _producer;
}
/**
* @return The JSON Pointer (https://tools.ietf.org/html/rfc6901) URL to the corresponding version of the schema definition for this facet
*/
@Override
public URI get_schemaURL() {
return _schemaURL;
}
/**
* @return set to true to delete a facet
*/
@Override
public Boolean get_deleted() {
return _deleted;
}
/**
* @return The owners of the job.
*/
public List getOwners() {
return owners;
}
/**
* @return additional properties
*/
@JsonAnyGetter
@Override
public Map getAdditionalProperties() {
return additionalProperties;
}
/**
* Get object with additional properties
*/
void withAdditionalProperties() {
}
}
/**
* builder class for OwnershipJobFacet
*/
public final class OwnershipJobFacetBuilder implements Builder {
private List owners;
private final Map additionalProperties = new LinkedHashMap<>();
/**
* @param owners The owners of the job.
* @return this
*/
public OwnershipJobFacetBuilder owners(List owners) {
this.owners = owners;
return this;
}
/**
* add additional properties
* @param key the additional property name
* @param value the additional property value
* @return this
*/
public OwnershipJobFacetBuilder put(String key, Object value) {
this.additionalProperties.put(key, value);return this;
}
/**
* build an instance of OwnershipJobFacet from the fields set in the builder
*/
@Override
public OwnershipJobFacet build() {
OwnershipJobFacet __result = new OwnershipJobFacet(OpenLineage.this.producer, owners);
__result.getAdditionalProperties().putAll(additionalProperties);
return __result;
}
}
/**
* model class for ParentRunFacetJob
*/
@JsonDeserialize(
as = ParentRunFacetJob.class
)
@JsonPropertyOrder({
"namespace",
"name"
})
public static final class ParentRunFacetJob {
private final String namespace;
private final String name;
/**
* @param namespace The namespace containing that job
* @param name The unique name for that job within that namespace
*/
@JsonCreator
private ParentRunFacetJob(@JsonProperty("namespace") String namespace,
@JsonProperty("name") String name) {
this.namespace = namespace;
this.name = name;
}
/**
* @return The namespace containing that job
*/
public String getNamespace() {
return namespace;
}
/**
* @return The unique name for that job within that namespace
*/
public String getName() {
return name;
}
}
/**
* builder class for ParentRunFacetJob
*/
public static final class ParentRunFacetJobBuilder implements Builder {
private String namespace;
private String name;
/**
* @param namespace The namespace containing that job
* @return this
*/
public ParentRunFacetJobBuilder namespace(String namespace) {
this.namespace = namespace;
return this;
}
/**
* @param name The unique name for that job within that namespace
* @return this
*/
public ParentRunFacetJobBuilder name(String name) {
this.name = name;
return this;
}
/**
* build an instance of ParentRunFacetJob from the fields set in the builder
*/
@Override
public ParentRunFacetJob build() {
ParentRunFacetJob __result = new ParentRunFacetJob(namespace, name);
return __result;
}
}
/**
* model class for OutputDataset
*/
@JsonDeserialize(
as = OutputDataset.class
)
@JsonPropertyOrder({
"namespace",
"name",
"facets",
"outputFacets"
})
public static final class OutputDataset implements Dataset {
private final String namespace;
private final String name;
private final DatasetFacets facets;
private final OutputDatasetOutputFacets outputFacets;
/**
* @param namespace The namespace containing that dataset
* @param name The unique name for that dataset within that namespace
* @param facets The facets for this dataset
* @param outputFacets The output facets for this dataset
*/
@JsonCreator
private OutputDataset(@JsonProperty("namespace") String namespace,
@JsonProperty("name") String name, @JsonProperty("facets") DatasetFacets facets,
@JsonProperty("outputFacets") OutputDatasetOutputFacets outputFacets) {
this.namespace = namespace;
this.name = name;
this.facets = facets;
this.outputFacets = outputFacets;
}
/**
* @return The namespace containing that dataset
*/
@Override
public String getNamespace() {
return namespace;
}
/**
* @return The unique name for that dataset within that namespace
*/
@Override
public String getName() {
return name;
}
/**
* @return The facets for this dataset
*/
@Override
public DatasetFacets getFacets() {
return facets;
}
/**
* @return The output facets for this dataset
*/
public OutputDatasetOutputFacets getOutputFacets() {
return outputFacets;
}
}
/**
* builder class for OutputDataset
*/
public static final class OutputDatasetBuilder implements Builder {
private String namespace;
private String name;
private DatasetFacets facets;
private OutputDatasetOutputFacets outputFacets;
/**
* @param namespace The namespace containing that dataset
* @return this
*/
public OutputDatasetBuilder namespace(String namespace) {
this.namespace = namespace;
return this;
}
/**
* @param name The unique name for that dataset within that namespace
* @return this
*/
public OutputDatasetBuilder name(String name) {
this.name = name;
return this;
}
/**
* @param facets The facets for this dataset
* @return this
*/
public OutputDatasetBuilder facets(DatasetFacets facets) {
this.facets = facets;
return this;
}
/**
* @param outputFacets The output facets for this dataset
* @return this
*/
public OutputDatasetBuilder outputFacets(OutputDatasetOutputFacets outputFacets) {
this.outputFacets = outputFacets;
return this;
}
/**
* build an instance of OutputDataset from the fields set in the builder
*/
@Override
public OutputDataset build() {
OutputDataset __result = new OutputDataset(namespace, name, facets, outputFacets);
return __result;
}
}
/**
* model class for ErrorMessageRunFacet
*/
@JsonDeserialize(
as = ErrorMessageRunFacet.class
)
@JsonPropertyOrder({
"_producer",
"_schemaURL",
"message",
"programmingLanguage",
"stackTrace"
})
public static final class ErrorMessageRunFacet implements RunFacet {
private final URI _producer;
private final URI _schemaURL;
private final String message;
private final String programmingLanguage;
private final String stackTrace;
@JsonAnySetter
private final Map additionalProperties;
/**
* @param _producer URI identifying the producer of this metadata. For example this could be a git url with a given tag or sha
* @param message A human-readable string representing error message generated by observed system
* @param programmingLanguage Programming language the observed system uses.
* @param stackTrace A language-specific stack trace generated by observed system
*/
@JsonCreator
private ErrorMessageRunFacet(@JsonProperty("_producer") URI _producer,
@JsonProperty("message") String message,
@JsonProperty("programmingLanguage") String programmingLanguage,
@JsonProperty("stackTrace") String stackTrace) {
this._producer = _producer;
this._schemaURL = URI.create("https://openlineage.io/spec/facets/1-0-0/ErrorMessageRunFacet.json#/$defs/ErrorMessageRunFacet");
this.message = message;
this.programmingLanguage = programmingLanguage;
this.stackTrace = stackTrace;
this.additionalProperties = new LinkedHashMap<>();
}
/**
* @return URI identifying the producer of this metadata. For example this could be a git url with a given tag or sha
*/
@Override
public URI get_producer() {
return _producer;
}
/**
* @return The JSON Pointer (https://tools.ietf.org/html/rfc6901) URL to the corresponding version of the schema definition for this facet
*/
@Override
public URI get_schemaURL() {
return _schemaURL;
}
/**
* @return A human-readable string representing error message generated by observed system
*/
public String getMessage() {
return message;
}
/**
* @return Programming language the observed system uses.
*/
public String getProgrammingLanguage() {
return programmingLanguage;
}
/**
* @return A language-specific stack trace generated by observed system
*/
public String getStackTrace() {
return stackTrace;
}
/**
* @return additional properties
*/
@JsonAnyGetter
@Override
public Map getAdditionalProperties() {
return additionalProperties;
}
/**
* Get object with additional properties
*/
void withAdditionalProperties() {
}
}
/**
* builder class for ErrorMessageRunFacet
*/
public final class ErrorMessageRunFacetBuilder implements Builder {
private String message;
private String programmingLanguage;
private String stackTrace;
private final Map additionalProperties = new LinkedHashMap<>();
/**
* @param message A human-readable string representing error message generated by observed system
* @return this
*/
public ErrorMessageRunFacetBuilder message(String message) {
this.message = message;
return this;
}
/**
* @param programmingLanguage Programming language the observed system uses.
* @return this
*/
public ErrorMessageRunFacetBuilder programmingLanguage(String programmingLanguage) {
this.programmingLanguage = programmingLanguage;
return this;
}
/**
* @param stackTrace A language-specific stack trace generated by observed system
* @return this
*/
public ErrorMessageRunFacetBuilder stackTrace(String stackTrace) {
this.stackTrace = stackTrace;
return this;
}
/**
* add additional properties
* @param key the additional property name
* @param value the additional property value
* @return this
*/
public ErrorMessageRunFacetBuilder put(String key, Object value) {
this.additionalProperties.put(key, value);return this;
}
/**
* build an instance of ErrorMessageRunFacet from the fields set in the builder
*/
@Override
public ErrorMessageRunFacet build() {
ErrorMessageRunFacet __result = new ErrorMessageRunFacet(OpenLineage.this.producer, message, programmingLanguage, stackTrace);
__result.getAdditionalProperties().putAll(additionalProperties);
return __result;
}
}
public static class DefaultJobFacet implements JobFacet {
private final URI _producer;
private final URI _schemaURL;
private final Boolean _deleted;
@JsonAnySetter
private final Map additionalProperties;
/**
* @param _producer URI identifying the producer of this metadata. For example this could be a git url with a given tag or sha
* @param _deleted set to true to delete a facet
*/
@JsonCreator
public DefaultJobFacet(@JsonProperty("_producer") URI _producer,
@JsonProperty("_deleted") Boolean _deleted) {
this._producer = _producer;
this._schemaURL = URI.create("https://openlineage.io/spec/2-0-2/OpenLineage.json#/$defs/JobFacet");
this._deleted = _deleted;
this.additionalProperties = new LinkedHashMap<>();
}
/**
* @return URI identifying the producer of this metadata. For example this could be a git url with a given tag or sha
*/
@Override
public URI get_producer() {
return _producer;
}
/**
* @return The JSON Pointer (https://tools.ietf.org/html/rfc6901) URL to the corresponding version of the schema definition for this facet
*/
@Override
public URI get_schemaURL() {
return _schemaURL;
}
/**
* @return set to true to delete a facet
*/
@Override
public Boolean get_deleted() {
return _deleted;
}
/**
* @return additional properties
*/
@JsonAnyGetter
@Override
public Map getAdditionalProperties() {
return additionalProperties;
}
}
/**
* Interface for JobFacet
*/
@JsonDeserialize(
as = DefaultJobFacet.class
)
public interface JobFacet {
/**
* @return URI identifying the producer of this metadata. For example this could be a git url with a given tag or sha
*/
URI get_producer();
/**
* @return The JSON Pointer (https://tools.ietf.org/html/rfc6901) URL to the corresponding version of the schema definition for this facet
*/
URI get_schemaURL();
/**
* @return set to true to delete a facet
*/
Boolean get_deleted();
/**
* @return set to true to delete a facet
*/
@JsonIgnore
default Boolean isDeleted() {
return get_deleted();
}
/**
* @return additional properties
*/
Map getAdditionalProperties();
}
/**
* model class for RunFacets
*/
@JsonDeserialize(
as = RunFacets.class
)
@JsonPropertyOrder({
"errorMessage",
"externalQuery",
"extractionError",
"parent",
"nominalTime",
"processing_engine"
})
public static final class RunFacets {
private final ErrorMessageRunFacet errorMessage;
private final ExternalQueryRunFacet externalQuery;
private final ExtractionErrorRunFacet extractionError;
private final ParentRunFacet parent;
private final NominalTimeRunFacet nominalTime;
private final ProcessingEngineRunFacet processing_engine;
@JsonAnySetter
private final Map additionalProperties;
/**
* @param errorMessage the errorMessage
* @param externalQuery the externalQuery
* @param extractionError the extractionError
* @param parent the parent
* @param nominalTime the nominalTime
* @param processing_engine the processing_engine
*/
@JsonCreator
private RunFacets(@JsonProperty("errorMessage") ErrorMessageRunFacet errorMessage,
@JsonProperty("externalQuery") ExternalQueryRunFacet externalQuery,
@JsonProperty("extractionError") ExtractionErrorRunFacet extractionError,
@JsonProperty("parent") ParentRunFacet parent,
@JsonProperty("nominalTime") NominalTimeRunFacet nominalTime,
@JsonProperty("processing_engine") ProcessingEngineRunFacet processing_engine) {
this.errorMessage = errorMessage;
this.externalQuery = externalQuery;
this.extractionError = extractionError;
this.parent = parent;
this.nominalTime = nominalTime;
this.processing_engine = processing_engine;
this.additionalProperties = new LinkedHashMap<>();
}
public ErrorMessageRunFacet getErrorMessage() {
return errorMessage;
}
public ExternalQueryRunFacet getExternalQuery() {
return externalQuery;
}
public ExtractionErrorRunFacet getExtractionError() {
return extractionError;
}
public ParentRunFacet getParent() {
return parent;
}
public NominalTimeRunFacet getNominalTime() {
return nominalTime;
}
public ProcessingEngineRunFacet getProcessing_engine() {
return processing_engine;
}
/**
* @return additional properties
*/
@JsonAnyGetter
public Map getAdditionalProperties() {
return additionalProperties;
}
/**
* Get object with additional properties
*/
void withAdditionalProperties() {
}
}
/**
* builder class for RunFacets
*/
public static final class RunFacetsBuilder implements Builder {
private ErrorMessageRunFacet errorMessage;
private ExternalQueryRunFacet externalQuery;
private ExtractionErrorRunFacet extractionError;
private ParentRunFacet parent;
private NominalTimeRunFacet nominalTime;
private ProcessingEngineRunFacet processing_engine;
private final Map additionalProperties = new LinkedHashMap<>();
/**
* @param errorMessage the errorMessage
* @return this
*/
public RunFacetsBuilder errorMessage(ErrorMessageRunFacet errorMessage) {
this.errorMessage = errorMessage;
return this;
}
/**
* @param externalQuery the externalQuery
* @return this
*/
public RunFacetsBuilder externalQuery(ExternalQueryRunFacet externalQuery) {
this.externalQuery = externalQuery;
return this;
}
/**
* @param extractionError the extractionError
* @return this
*/
public RunFacetsBuilder extractionError(ExtractionErrorRunFacet extractionError) {
this.extractionError = extractionError;
return this;
}
/**
* @param parent the parent
* @return this
*/
public RunFacetsBuilder parent(ParentRunFacet parent) {
this.parent = parent;
return this;
}
/**
* @param nominalTime the nominalTime
* @return this
*/
public RunFacetsBuilder nominalTime(NominalTimeRunFacet nominalTime) {
this.nominalTime = nominalTime;
return this;
}
/**
* @param processing_engine the processing_engine
* @return this
*/
public RunFacetsBuilder processing_engine(ProcessingEngineRunFacet processing_engine) {
this.processing_engine = processing_engine;
return this;
}
/**
* add additional properties
* @param key the additional property name
* @param value the additional property value
* @return this
*/
public RunFacetsBuilder put(String key, RunFacet value) {
this.additionalProperties.put(key, value);return this;
}
/**
* build an instance of RunFacets from the fields set in the builder
*/
@Override
public RunFacets build() {
RunFacets __result = new RunFacets(errorMessage, externalQuery, extractionError, parent, nominalTime, processing_engine);
__result.getAdditionalProperties().putAll(additionalProperties);
return __result;
}
}
/**
* model class for SchemaDatasetFacet
*/
@JsonDeserialize(
as = SchemaDatasetFacet.class
)
@JsonPropertyOrder({
"_producer",
"_schemaURL",
"_deleted",
"fields"
})
public static final class SchemaDatasetFacet implements DatasetFacet {
private final URI _producer;
private final URI _schemaURL;
private final Boolean _deleted;
private final List fields;
@JsonAnySetter
private final Map additionalProperties;
/**
* @param _producer URI identifying the producer of this metadata. For example this could be a git url with a given tag or sha
* @param fields The fields of the data source.
*/
@JsonCreator
private SchemaDatasetFacet(@JsonProperty("_producer") URI _producer,
@JsonProperty("fields") List fields) {
this._producer = _producer;
this._schemaURL = URI.create("https://openlineage.io/spec/facets/1-1-0/SchemaDatasetFacet.json#/$defs/SchemaDatasetFacet");
this._deleted = null;
this.fields = fields;
this.additionalProperties = new LinkedHashMap<>();
}
/**
* @return URI identifying the producer of this metadata. For example this could be a git url with a given tag or sha
*/
@Override
public URI get_producer() {
return _producer;
}
/**
* @return The JSON Pointer (https://tools.ietf.org/html/rfc6901) URL to the corresponding version of the schema definition for this facet
*/
@Override
public URI get_schemaURL() {
return _schemaURL;
}
/**
* @return set to true to delete a facet
*/
@Override
public Boolean get_deleted() {
return _deleted;
}
/**
* @return The fields of the data source.
*/
public List getFields() {
return fields;
}
/**
* @return additional properties
*/
@JsonAnyGetter
@Override
public Map getAdditionalProperties() {
return additionalProperties;
}
/**
* Get object with additional properties
*/
void withAdditionalProperties() {
}
}
/**
* builder class for SchemaDatasetFacet
*/
public final class SchemaDatasetFacetBuilder implements Builder {
private List fields;
private final Map additionalProperties = new LinkedHashMap<>();
/**
* @param fields The fields of the data source.
* @return this
*/
public SchemaDatasetFacetBuilder fields(List fields) {
this.fields = fields;
return this;
}
/**
* add additional properties
* @param key the additional property name
* @param value the additional property value
* @return this
*/
public SchemaDatasetFacetBuilder put(String key, Object value) {
this.additionalProperties.put(key, value);return this;
}
/**
* build an instance of SchemaDatasetFacet from the fields set in the builder
*/
@Override
public SchemaDatasetFacet build() {
SchemaDatasetFacet __result = new SchemaDatasetFacet(OpenLineage.this.producer, fields);
__result.getAdditionalProperties().putAll(additionalProperties);
return __result;
}
}
/**
* model class for SourceCodeJobFacet
*/
@JsonDeserialize(
as = SourceCodeJobFacet.class
)
@JsonPropertyOrder({
"_producer",
"_schemaURL",
"_deleted",
"language",
"sourceCode"
})
public static final class SourceCodeJobFacet implements JobFacet {
private final URI _producer;
private final URI _schemaURL;
private final Boolean _deleted;
private final String language;
private final String sourceCode;
@JsonAnySetter
private final Map additionalProperties;
/**
* @param _producer URI identifying the producer of this metadata. For example this could be a git url with a given tag or sha
* @param language Language in which source code of this job was written.
* @param sourceCode Source code of this job.
*/
@JsonCreator
private SourceCodeJobFacet(@JsonProperty("_producer") URI _producer,
@JsonProperty("language") String language, @JsonProperty("sourceCode") String sourceCode) {
this._producer = _producer;
this._schemaURL = URI.create("https://openlineage.io/spec/facets/1-0-0/SourceCodeJobFacet.json#/$defs/SourceCodeJobFacet");
this._deleted = null;
this.language = language;
this.sourceCode = sourceCode;
this.additionalProperties = new LinkedHashMap<>();
}
/**
* @return URI identifying the producer of this metadata. For example this could be a git url with a given tag or sha
*/
@Override
public URI get_producer() {
return _producer;
}
/**
* @return The JSON Pointer (https://tools.ietf.org/html/rfc6901) URL to the corresponding version of the schema definition for this facet
*/
@Override
public URI get_schemaURL() {
return _schemaURL;
}
/**
* @return set to true to delete a facet
*/
@Override
public Boolean get_deleted() {
return _deleted;
}
/**
* @return Language in which source code of this job was written.
*/
public String getLanguage() {
return language;
}
/**
* @return Source code of this job.
*/
public String getSourceCode() {
return sourceCode;
}
/**
* @return additional properties
*/
@JsonAnyGetter
@Override
public Map getAdditionalProperties() {
return additionalProperties;
}
/**
* Get object with additional properties
*/
void withAdditionalProperties() {
}
}
/**
* builder class for SourceCodeJobFacet
*/
public final class SourceCodeJobFacetBuilder implements Builder {
private String language;
private String sourceCode;
private final Map additionalProperties = new LinkedHashMap<>();
/**
* @param language Language in which source code of this job was written.
* @return this
*/
public SourceCodeJobFacetBuilder language(String language) {
this.language = language;
return this;
}
/**
* @param sourceCode Source code of this job.
* @return this
*/
public SourceCodeJobFacetBuilder sourceCode(String sourceCode) {
this.sourceCode = sourceCode;
return this;
}
/**
* add additional properties
* @param key the additional property name
* @param value the additional property value
* @return this
*/
public SourceCodeJobFacetBuilder put(String key, Object value) {
this.additionalProperties.put(key, value);return this;
}
/**
* build an instance of SourceCodeJobFacet from the fields set in the builder
*/
@Override
public SourceCodeJobFacet build() {
SourceCodeJobFacet __result = new SourceCodeJobFacet(OpenLineage.this.producer, language, sourceCode);
__result.getAdditionalProperties().putAll(additionalProperties);
return __result;
}
}
/**
* model class for DocumentationDatasetFacet
*/
@JsonDeserialize(
as = DocumentationDatasetFacet.class
)
@JsonPropertyOrder({
"_producer",
"_schemaURL",
"_deleted",
"description"
})
public static final class DocumentationDatasetFacet implements DatasetFacet {
private final URI _producer;
private final URI _schemaURL;
private final Boolean _deleted;
private final String description;
@JsonAnySetter
private final Map additionalProperties;
/**
* @param _producer URI identifying the producer of this metadata. For example this could be a git url with a given tag or sha
* @param description The description of the dataset.
*/
@JsonCreator
private DocumentationDatasetFacet(@JsonProperty("_producer") URI _producer,
@JsonProperty("description") String description) {
this._producer = _producer;
this._schemaURL = URI.create("https://openlineage.io/spec/facets/1-0-0/DocumentationDatasetFacet.json#/$defs/DocumentationDatasetFacet");
this._deleted = null;
this.description = description;
this.additionalProperties = new LinkedHashMap<>();
}
/**
* @return URI identifying the producer of this metadata. For example this could be a git url with a given tag or sha
*/
@Override
public URI get_producer() {
return _producer;
}
/**
* @return The JSON Pointer (https://tools.ietf.org/html/rfc6901) URL to the corresponding version of the schema definition for this facet
*/
@Override
public URI get_schemaURL() {
return _schemaURL;
}
/**
* @return set to true to delete a facet
*/
@Override
public Boolean get_deleted() {
return _deleted;
}
/**
* @return The description of the dataset.
*/
public String getDescription() {
return description;
}
/**
* @return additional properties
*/
@JsonAnyGetter
@Override
public Map getAdditionalProperties() {
return additionalProperties;
}
/**
* Get object with additional properties
*/
void withAdditionalProperties() {
}
}
/**
* builder class for DocumentationDatasetFacet
*/
public final class DocumentationDatasetFacetBuilder implements Builder {
private String description;
private final Map additionalProperties = new LinkedHashMap<>();
/**
* @param description The description of the dataset.
* @return this
*/
public DocumentationDatasetFacetBuilder description(String description) {
this.description = description;
return this;
}
/**
* add additional properties
* @param key the additional property name
* @param value the additional property value
* @return this
*/
public DocumentationDatasetFacetBuilder put(String key, Object value) {
this.additionalProperties.put(key, value);return this;
}
/**
* build an instance of DocumentationDatasetFacet from the fields set in the builder
*/
@Override
public DocumentationDatasetFacet build() {
DocumentationDatasetFacet __result = new DocumentationDatasetFacet(OpenLineage.this.producer, description);
__result.getAdditionalProperties().putAll(additionalProperties);
return __result;
}
}
/**
* model class for SourceCodeLocationJobFacet
*/
@JsonDeserialize(
as = SourceCodeLocationJobFacet.class
)
@JsonPropertyOrder({
"_producer",
"_schemaURL",
"_deleted",
"type",
"url",
"repoUrl",
"path",
"version",
"tag",
"branch"
})
public static final class SourceCodeLocationJobFacet implements JobFacet {
private final URI _producer;
private final URI _schemaURL;
private final Boolean _deleted;
private final String type;
private final URI url;
private final String repoUrl;
private final String path;
private final String version;
private final String tag;
private final String branch;
@JsonAnySetter
private final Map additionalProperties;
/**
* @param _producer URI identifying the producer of this metadata. For example this could be a git url with a given tag or sha
* @param type the source control system
* @param url the full http URL to locate the file
* @param repoUrl the URL to the repository
* @param path the path in the repo containing the source files
* @param version the current version deployed (not a branch name, the actual unique version)
* @param tag optional tag name
* @param branch optional branch name
*/
@JsonCreator
private SourceCodeLocationJobFacet(@JsonProperty("_producer") URI _producer,
@JsonProperty("type") String type, @JsonProperty("url") URI url,
@JsonProperty("repoUrl") String repoUrl, @JsonProperty("path") String path,
@JsonProperty("version") String version, @JsonProperty("tag") String tag,
@JsonProperty("branch") String branch) {
this._producer = _producer;
this._schemaURL = URI.create("https://openlineage.io/spec/facets/1-0-0/SourceCodeLocationJobFacet.json#/$defs/SourceCodeLocationJobFacet");
this._deleted = null;
this.type = type;
this.url = url;
this.repoUrl = repoUrl;
this.path = path;
this.version = version;
this.tag = tag;
this.branch = branch;
this.additionalProperties = new LinkedHashMap<>();
}
/**
* @return URI identifying the producer of this metadata. For example this could be a git url with a given tag or sha
*/
@Override
public URI get_producer() {
return _producer;
}
/**
* @return The JSON Pointer (https://tools.ietf.org/html/rfc6901) URL to the corresponding version of the schema definition for this facet
*/
@Override
public URI get_schemaURL() {
return _schemaURL;
}
/**
* @return set to true to delete a facet
*/
@Override
public Boolean get_deleted() {
return _deleted;
}
/**
* @return the source control system
*/
public String getType() {
return type;
}
/**
* @return the full http URL to locate the file
*/
public URI getUrl() {
return url;
}
/**
* @return the URL to the repository
*/
public String getRepoUrl() {
return repoUrl;
}
/**
* @return the path in the repo containing the source files
*/
public String getPath() {
return path;
}
/**
* @return the current version deployed (not a branch name, the actual unique version)
*/
public String getVersion() {
return version;
}
/**
* @return optional tag name
*/
public String getTag() {
return tag;
}
/**
* @return optional branch name
*/
public String getBranch() {
return branch;
}
/**
* @return additional properties
*/
@JsonAnyGetter
@Override
public Map getAdditionalProperties() {
return additionalProperties;
}
/**
* Get object with additional properties
*/
void withAdditionalProperties() {
}
}
/**
* builder class for SourceCodeLocationJobFacet
*/
public final class SourceCodeLocationJobFacetBuilder implements Builder {
private String type;
private URI url;
private String repoUrl;
private String path;
private String version;
private String tag;
private String branch;
private final Map additionalProperties = new LinkedHashMap<>();
/**
* @param type the source control system
* @return this
*/
public SourceCodeLocationJobFacetBuilder type(String type) {
this.type = type;
return this;
}
/**
* @param url the full http URL to locate the file
* @return this
*/
public SourceCodeLocationJobFacetBuilder url(URI url) {
this.url = url;
return this;
}
/**
* @param repoUrl the URL to the repository
* @return this
*/
public SourceCodeLocationJobFacetBuilder repoUrl(String repoUrl) {
this.repoUrl = repoUrl;
return this;
}
/**
* @param path the path in the repo containing the source files
* @return this
*/
public SourceCodeLocationJobFacetBuilder path(String path) {
this.path = path;
return this;
}
/**
* @param version the current version deployed (not a branch name, the actual unique version)
* @return this
*/
public SourceCodeLocationJobFacetBuilder version(String version) {
this.version = version;
return this;
}
/**
* @param tag optional tag name
* @return this
*/
public SourceCodeLocationJobFacetBuilder tag(String tag) {
this.tag = tag;
return this;
}
/**
* @param branch optional branch name
* @return this
*/
public SourceCodeLocationJobFacetBuilder branch(String branch) {
this.branch = branch;
return this;
}
/**
* add additional properties
* @param key the additional property name
* @param value the additional property value
* @return this
*/
public SourceCodeLocationJobFacetBuilder put(String key, Object value) {
this.additionalProperties.put(key, value);return this;
}
/**
* build an instance of SourceCodeLocationJobFacet from the fields set in the builder
*/
@Override
public SourceCodeLocationJobFacet build() {
SourceCodeLocationJobFacet __result = new SourceCodeLocationJobFacet(OpenLineage.this.producer, type, url, repoUrl, path, version, tag, branch);
__result.getAdditionalProperties().putAll(additionalProperties);
return __result;
}
}
/**
* model class for ColumnLineageDatasetFacet
*/
@JsonDeserialize(
as = ColumnLineageDatasetFacet.class
)
@JsonPropertyOrder({
"_producer",
"_schemaURL",
"_deleted",
"fields"
})
public static final class ColumnLineageDatasetFacet implements DatasetFacet {
private final URI _producer;
private final URI _schemaURL;
private final Boolean _deleted;
private final ColumnLineageDatasetFacetFields fields;
@JsonAnySetter
private final Map additionalProperties;
/**
* @param _producer URI identifying the producer of this metadata. For example this could be a git url with a given tag or sha
* @param fields Column level lineage that maps output fields into input fields used to evaluate them.
*/
@JsonCreator
private ColumnLineageDatasetFacet(@JsonProperty("_producer") URI _producer,
@JsonProperty("fields") ColumnLineageDatasetFacetFields fields) {
this._producer = _producer;
this._schemaURL = URI.create("https://openlineage.io/spec/facets/1-0-1/ColumnLineageDatasetFacet.json#/$defs/ColumnLineageDatasetFacet");
this._deleted = null;
this.fields = fields;
this.additionalProperties = new LinkedHashMap<>();
}
/**
* @return URI identifying the producer of this metadata. For example this could be a git url with a given tag or sha
*/
@Override
public URI get_producer() {
return _producer;
}
/**
* @return The JSON Pointer (https://tools.ietf.org/html/rfc6901) URL to the corresponding version of the schema definition for this facet
*/
@Override
public URI get_schemaURL() {
return _schemaURL;
}
/**
* @return set to true to delete a facet
*/
@Override
public Boolean get_deleted() {
return _deleted;
}
/**
* @return Column level lineage that maps output fields into input fields used to evaluate them.
*/
public ColumnLineageDatasetFacetFields getFields() {
return fields;
}
/**
* @return additional properties
*/
@JsonAnyGetter
@Override
public Map getAdditionalProperties() {
return additionalProperties;
}
/**
* Get object with additional properties
*/
void withAdditionalProperties() {
}
}
/**
* builder class for ColumnLineageDatasetFacet
*/
public final class ColumnLineageDatasetFacetBuilder implements Builder {
private ColumnLineageDatasetFacetFields fields;
private final Map additionalProperties = new LinkedHashMap<>();
/**
* @param fields Column level lineage that maps output fields into input fields used to evaluate them.
* @return this
*/
public ColumnLineageDatasetFacetBuilder fields(ColumnLineageDatasetFacetFields fields) {
this.fields = fields;
return this;
}
/**
* add additional properties
* @param key the additional property name
* @param value the additional property value
* @return this
*/
public ColumnLineageDatasetFacetBuilder put(String key, Object value) {
this.additionalProperties.put(key, value);return this;
}
/**
* build an instance of ColumnLineageDatasetFacet from the fields set in the builder
*/
@Override
public ColumnLineageDatasetFacet build() {
ColumnLineageDatasetFacet __result = new ColumnLineageDatasetFacet(OpenLineage.this.producer, fields);
__result.getAdditionalProperties().putAll(additionalProperties);
return __result;
}
}
/**
* model class for DataQualityAssertionsDatasetFacet
*/
@JsonDeserialize(
as = DataQualityAssertionsDatasetFacet.class
)
@JsonPropertyOrder({
"_producer",
"_schemaURL",
"assertions"
})
public static final class DataQualityAssertionsDatasetFacet implements InputDatasetFacet {
private final URI _producer;
private final URI _schemaURL;
private final List assertions;
@JsonAnySetter
private final Map additionalProperties;
/**
* @param _producer URI identifying the producer of this metadata. For example this could be a git url with a given tag or sha
* @param assertions the assertions
*/
@JsonCreator
private DataQualityAssertionsDatasetFacet(@JsonProperty("_producer") URI _producer,
@JsonProperty("assertions") List assertions) {
this._producer = _producer;
this._schemaURL = URI.create("https://openlineage.io/spec/facets/1-0-0/DataQualityAssertionsDatasetFacet.json#/$defs/DataQualityAssertionsDatasetFacet");
this.assertions = assertions;
this.additionalProperties = new LinkedHashMap<>();
}
/**
* @return URI identifying the producer of this metadata. For example this could be a git url with a given tag or sha
*/
@Override
public URI get_producer() {
return _producer;
}
/**
* @return The JSON Pointer (https://tools.ietf.org/html/rfc6901) URL to the corresponding version of the schema definition for this facet
*/
@Override
public URI get_schemaURL() {
return _schemaURL;
}
public List getAssertions() {
return assertions;
}
/**
* @return additional properties
*/
@JsonAnyGetter
@Override
public Map getAdditionalProperties() {
return additionalProperties;
}
/**
* Get object with additional properties
*/
void withAdditionalProperties() {
}
}
/**
* builder class for DataQualityAssertionsDatasetFacet
*/
public final class DataQualityAssertionsDatasetFacetBuilder implements Builder {
private List assertions;
private final Map additionalProperties = new LinkedHashMap<>();
/**
* @param assertions the assertions
* @return this
*/
public DataQualityAssertionsDatasetFacetBuilder assertions(
List assertions) {
this.assertions = assertions;
return this;
}
/**
* add additional properties
* @param key the additional property name
* @param value the additional property value
* @return this
*/
public DataQualityAssertionsDatasetFacetBuilder put(String key, Object value) {
this.additionalProperties.put(key, value);return this;
}
/**
* build an instance of DataQualityAssertionsDatasetFacet from the fields set in the builder
*/
@Override
public DataQualityAssertionsDatasetFacet build() {
DataQualityAssertionsDatasetFacet __result = new DataQualityAssertionsDatasetFacet(OpenLineage.this.producer, assertions);
__result.getAdditionalProperties().putAll(additionalProperties);
return __result;
}
}
/**
* model class for DatasetEvent
*/
@JsonDeserialize(
as = DatasetEvent.class
)
@JsonPropertyOrder({
"eventTime",
"producer",
"schemaURL",
"dataset"
})
public static final class DatasetEvent implements BaseEvent {
private final ZonedDateTime eventTime;
private final URI producer;
private final URI schemaURL;
private final StaticDataset dataset;
/**
* @param eventTime the time the event occurred at
* @param producer URI identifying the producer of this metadata. For example this could be a git url with a given tag or sha
* @param dataset the dataset
*/
@JsonCreator
private DatasetEvent(@JsonProperty("eventTime") ZonedDateTime eventTime,
@JsonProperty("producer") URI producer, @JsonProperty("dataset") StaticDataset dataset) {
this.eventTime = eventTime;
this.producer = producer;
this.schemaURL = URI.create("https://openlineage.io/spec/2-0-2/OpenLineage.json#/$defs/DatasetEvent");
this.dataset = dataset;
}
/**
* @return the time the event occurred at
*/
@Override
public ZonedDateTime getEventTime() {
return eventTime;
}
/**
* @return URI identifying the producer of this metadata. For example this could be a git url with a given tag or sha
*/
@Override
public URI getProducer() {
return producer;
}
/**
* @return The JSON Pointer (https://tools.ietf.org/html/rfc6901) URL to the corresponding version of the schema definition for this RunEvent
*/
@Override
public URI getSchemaURL() {
return schemaURL;
}
public StaticDataset getDataset() {
return dataset;
}
}
/**
* builder class for DatasetEvent
*/
public final class DatasetEventBuilder implements Builder {
private ZonedDateTime eventTime;
private StaticDataset dataset;
/**
* @param eventTime the time the event occurred at
* @return this
*/
public DatasetEventBuilder eventTime(ZonedDateTime eventTime) {
this.eventTime = eventTime;
return this;
}
/**
* @param dataset the dataset
* @return this
*/
public DatasetEventBuilder dataset(StaticDataset dataset) {
this.dataset = dataset;
return this;
}
/**
* build an instance of DatasetEvent from the fields set in the builder
*/
@Override
public DatasetEvent build() {
DatasetEvent __result = new DatasetEvent(eventTime, OpenLineage.this.producer, dataset);
return __result;
}
}
/**
* Interface for Dataset
*/
public interface Dataset {
/**
* @return The namespace containing that dataset
*/
String getNamespace();
/**
* @return The unique name for that dataset within that namespace
*/
String getName();
/**
* @return The facets for this dataset
*/
DatasetFacets getFacets();
}
/**
* model class for ProcessingEngineRunFacet
*/
@JsonDeserialize(
as = ProcessingEngineRunFacet.class
)
@JsonPropertyOrder({
"_producer",
"_schemaURL",
"version",
"name",
"openlineageAdapterVersion"
})
public static final class ProcessingEngineRunFacet implements RunFacet {
private final URI _producer;
private final URI _schemaURL;
private final String version;
private final String name;
private final String openlineageAdapterVersion;
@JsonAnySetter
private final Map additionalProperties;
/**
* @param _producer URI identifying the producer of this metadata. For example this could be a git url with a given tag or sha
* @param version Processing engine version. Might be Airflow or Spark version.
* @param name Processing engine name, e.g. Airflow or Spark
* @param openlineageAdapterVersion OpenLineage adapter package version. Might be e.g. OpenLineage Airflow integration package version
*/
@JsonCreator
private ProcessingEngineRunFacet(@JsonProperty("_producer") URI _producer,
@JsonProperty("version") String version, @JsonProperty("name") String name,
@JsonProperty("openlineageAdapterVersion") String openlineageAdapterVersion) {
this._producer = _producer;
this._schemaURL = URI.create("https://openlineage.io/spec/facets/1-1-0/ProcessingEngineRunFacet.json#/$defs/ProcessingEngineRunFacet");
this.version = version;
this.name = name;
this.openlineageAdapterVersion = openlineageAdapterVersion;
this.additionalProperties = new LinkedHashMap<>();
}
/**
* @return URI identifying the producer of this metadata. For example this could be a git url with a given tag or sha
*/
@Override
public URI get_producer() {
return _producer;
}
/**
* @return The JSON Pointer (https://tools.ietf.org/html/rfc6901) URL to the corresponding version of the schema definition for this facet
*/
@Override
public URI get_schemaURL() {
return _schemaURL;
}
/**
* @return Processing engine version. Might be Airflow or Spark version.
*/
public String getVersion() {
return version;
}
/**
* @return Processing engine name, e.g. Airflow or Spark
*/
public String getName() {
return name;
}
/**
* @return OpenLineage adapter package version. Might be e.g. OpenLineage Airflow integration package version
*/
public String getOpenlineageAdapterVersion() {
return openlineageAdapterVersion;
}
/**
* @return additional properties
*/
@JsonAnyGetter
@Override
public Map getAdditionalProperties() {
return additionalProperties;
}
/**
* Get object with additional properties
*/
void withAdditionalProperties() {
}
}
/**
* builder class for ProcessingEngineRunFacet
*/
public final class ProcessingEngineRunFacetBuilder implements Builder {
private String version;
private String name;
private String openlineageAdapterVersion;
private final Map additionalProperties = new LinkedHashMap<>();
/**
* @param version Processing engine version. Might be Airflow or Spark version.
* @return this
*/
public ProcessingEngineRunFacetBuilder version(String version) {
this.version = version;
return this;
}
/**
* @param name Processing engine name, e.g. Airflow or Spark
* @return this
*/
public ProcessingEngineRunFacetBuilder name(String name) {
this.name = name;
return this;
}
/**
* @param openlineageAdapterVersion OpenLineage adapter package version. Might be e.g. OpenLineage Airflow integration package version
* @return this
*/
public ProcessingEngineRunFacetBuilder openlineageAdapterVersion(
String openlineageAdapterVersion) {
this.openlineageAdapterVersion = openlineageAdapterVersion;
return this;
}
/**
* add additional properties
* @param key the additional property name
* @param value the additional property value
* @return this
*/
public ProcessingEngineRunFacetBuilder put(String key, Object value) {
this.additionalProperties.put(key, value);return this;
}
/**
* build an instance of ProcessingEngineRunFacet from the fields set in the builder
*/
@Override
public ProcessingEngineRunFacet build() {
ProcessingEngineRunFacet __result = new ProcessingEngineRunFacet(OpenLineage.this.producer, version, name, openlineageAdapterVersion);
__result.getAdditionalProperties().putAll(additionalProperties);
return __result;
}
}
/**
* model class for LifecycleStateChangeDatasetFacetPreviousIdentifier
*/
@JsonDeserialize(
as = LifecycleStateChangeDatasetFacetPreviousIdentifier.class
)
@JsonPropertyOrder({
"name",
"namespace"
})
public static final class LifecycleStateChangeDatasetFacetPreviousIdentifier {
private final String name;
private final String namespace;
/**
* @param name the name
* @param namespace the namespace
*/
@JsonCreator
private LifecycleStateChangeDatasetFacetPreviousIdentifier(@JsonProperty("name") String name,
@JsonProperty("namespace") String namespace) {
this.name = name;
this.namespace = namespace;
}
public String getName() {
return name;
}
public String getNamespace() {
return namespace;
}
}
/**
* builder class for LifecycleStateChangeDatasetFacetPreviousIdentifier
*/
public static final class LifecycleStateChangeDatasetFacetPreviousIdentifierBuilder implements Builder {
private String name;
private String namespace;
/**
* @param name the name
* @return this
*/
public LifecycleStateChangeDatasetFacetPreviousIdentifierBuilder name(String name) {
this.name = name;
return this;
}
/**
* @param namespace the namespace
* @return this
*/
public LifecycleStateChangeDatasetFacetPreviousIdentifierBuilder namespace(String namespace) {
this.namespace = namespace;
return this;
}
/**
* build an instance of LifecycleStateChangeDatasetFacetPreviousIdentifier from the fields set in the builder
*/
@Override
public LifecycleStateChangeDatasetFacetPreviousIdentifier build() {
LifecycleStateChangeDatasetFacetPreviousIdentifier __result = new LifecycleStateChangeDatasetFacetPreviousIdentifier(name, namespace);
return __result;
}
}
/**
* model class for Job
*/
@JsonDeserialize(
as = Job.class
)
@JsonPropertyOrder({
"namespace",
"name",
"facets"
})
public static final class Job {
private final String namespace;
private final String name;
private final JobFacets facets;
/**
* @param namespace The namespace containing that job
* @param name The unique name for that job within that namespace
* @param facets The job facets.
*/
@JsonCreator
private Job(@JsonProperty("namespace") String namespace, @JsonProperty("name") String name,
@JsonProperty("facets") JobFacets facets) {
this.namespace = namespace;
this.name = name;
this.facets = facets;
}
/**
* @return The namespace containing that job
*/
public String getNamespace() {
return namespace;
}
/**
* @return The unique name for that job within that namespace
*/
public String getName() {
return name;
}
/**
* @return The job facets.
*/
public JobFacets getFacets() {
return facets;
}
}
/**
* builder class for Job
*/
public static final class JobBuilder implements Builder {
private String namespace;
private String name;
private JobFacets facets;
/**
* @param namespace The namespace containing that job
* @return this
*/
public JobBuilder namespace(String namespace) {
this.namespace = namespace;
return this;
}
/**
* @param name The unique name for that job within that namespace
* @return this
*/
public JobBuilder name(String name) {
this.name = name;
return this;
}
/**
* @param facets The job facets.
* @return this
*/
public JobBuilder facets(JobFacets facets) {
this.facets = facets;
return this;
}
/**
* build an instance of Job from the fields set in the builder
*/
@Override
public Job build() {
Job __result = new Job(namespace, name, facets);
return __result;
}
}
/**
* model class for DatasetVersionDatasetFacet
*/
@JsonDeserialize(
as = DatasetVersionDatasetFacet.class
)
@JsonPropertyOrder({
"_producer",
"_schemaURL",
"_deleted",
"datasetVersion"
})
public static final class DatasetVersionDatasetFacet implements DatasetFacet {
private final URI _producer;
private final URI _schemaURL;
private final Boolean _deleted;
private final String datasetVersion;
@JsonAnySetter
private final Map additionalProperties;
/**
* @param _producer URI identifying the producer of this metadata. For example this could be a git url with a given tag or sha
* @param datasetVersion The version of the dataset.
*/
@JsonCreator
private DatasetVersionDatasetFacet(@JsonProperty("_producer") URI _producer,
@JsonProperty("datasetVersion") String datasetVersion) {
this._producer = _producer;
this._schemaURL = URI.create("https://openlineage.io/spec/facets/1-0-0/DatasetVersionDatasetFacet.json#/$defs/DatasetVersionDatasetFacet");
this._deleted = null;
this.datasetVersion = datasetVersion;
this.additionalProperties = new LinkedHashMap<>();
}
/**
* @return URI identifying the producer of this metadata. For example this could be a git url with a given tag or sha
*/
@Override
public URI get_producer() {
return _producer;
}
/**
* @return The JSON Pointer (https://tools.ietf.org/html/rfc6901) URL to the corresponding version of the schema definition for this facet
*/
@Override
public URI get_schemaURL() {
return _schemaURL;
}
/**
* @return set to true to delete a facet
*/
@Override
public Boolean get_deleted() {
return _deleted;
}
/**
* @return The version of the dataset.
*/
public String getDatasetVersion() {
return datasetVersion;
}
/**
* @return additional properties
*/
@JsonAnyGetter
@Override
public Map getAdditionalProperties() {
return additionalProperties;
}
/**
* Get object with additional properties
*/
void withAdditionalProperties() {
}
}
/**
* builder class for DatasetVersionDatasetFacet
*/
public final class DatasetVersionDatasetFacetBuilder implements Builder {
private String datasetVersion;
private final Map additionalProperties = new LinkedHashMap<>();
/**
* @param datasetVersion The version of the dataset.
* @return this
*/
public DatasetVersionDatasetFacetBuilder datasetVersion(String datasetVersion) {
this.datasetVersion = datasetVersion;
return this;
}
/**
* add additional properties
* @param key the additional property name
* @param value the additional property value
* @return this
*/
public DatasetVersionDatasetFacetBuilder put(String key, Object value) {
this.additionalProperties.put(key, value);return this;
}
/**
* build an instance of DatasetVersionDatasetFacet from the fields set in the builder
*/
@Override
public DatasetVersionDatasetFacet build() {
DatasetVersionDatasetFacet __result = new DatasetVersionDatasetFacet(OpenLineage.this.producer, datasetVersion);
__result.getAdditionalProperties().putAll(additionalProperties);
return __result;
}
}
/**
* model class for SymlinksDatasetFacetIdentifiers
*/
@JsonDeserialize(
as = SymlinksDatasetFacetIdentifiers.class
)
@JsonPropertyOrder({
"namespace",
"name",
"type"
})
public static final class SymlinksDatasetFacetIdentifiers {
private final String namespace;
private final String name;
private final String type;
/**
* @param namespace The dataset namespace
* @param name The dataset name
* @param type Identifier type
*/
@JsonCreator
private SymlinksDatasetFacetIdentifiers(@JsonProperty("namespace") String namespace,
@JsonProperty("name") String name, @JsonProperty("type") String type) {
this.namespace = namespace;
this.name = name;
this.type = type;
}
/**
* @return The dataset namespace
*/
public String getNamespace() {
return namespace;
}
/**
* @return The dataset name
*/
public String getName() {
return name;
}
/**
* @return Identifier type
*/
public String getType() {
return type;
}
}
/**
* builder class for SymlinksDatasetFacetIdentifiers
*/
public static final class SymlinksDatasetFacetIdentifiersBuilder implements Builder {
private String namespace;
private String name;
private String type;
/**
* @param namespace The dataset namespace
* @return this
*/
public SymlinksDatasetFacetIdentifiersBuilder namespace(String namespace) {
this.namespace = namespace;
return this;
}
/**
* @param name The dataset name
* @return this
*/
public SymlinksDatasetFacetIdentifiersBuilder name(String name) {
this.name = name;
return this;
}
/**
* @param type Identifier type
* @return this
*/
public SymlinksDatasetFacetIdentifiersBuilder type(String type) {
this.type = type;
return this;
}
/**
* build an instance of SymlinksDatasetFacetIdentifiers from the fields set in the builder
*/
@Override
public SymlinksDatasetFacetIdentifiers build() {
SymlinksDatasetFacetIdentifiers __result = new SymlinksDatasetFacetIdentifiers(namespace, name, type);
return __result;
}
}
/**
* model class for DataQualityAssertionsDatasetFacetAssertions
*/
@JsonDeserialize(
as = DataQualityAssertionsDatasetFacetAssertions.class
)
@JsonPropertyOrder({
"assertion",
"success",
"column"
})
public static final class DataQualityAssertionsDatasetFacetAssertions {
private final String assertion;
private final Boolean success;
private final String column;
/**
* @param assertion Type of expectation test that dataset is subjected to
* @param success the success
* @param column Column that expectation is testing. It should match the name provided in SchemaDatasetFacet. If column field is empty, then expectation refers to whole dataset.
*/
@JsonCreator
private DataQualityAssertionsDatasetFacetAssertions(@JsonProperty("assertion") String assertion,
@JsonProperty("success") Boolean success, @JsonProperty("column") String column) {
this.assertion = assertion;
this.success = success;
this.column = column;
}
/**
* @return Type of expectation test that dataset is subjected to
*/
public String getAssertion() {
return assertion;
}
public Boolean getSuccess() {
return success;
}
/**
* @return Column that expectation is testing. It should match the name provided in SchemaDatasetFacet. If column field is empty, then expectation refers to whole dataset.
*/
public String getColumn() {
return column;
}
}
/**
* builder class for DataQualityAssertionsDatasetFacetAssertions
*/
public static final class DataQualityAssertionsDatasetFacetAssertionsBuilder implements Builder {
private String assertion;
private Boolean success;
private String column;
/**
* @param assertion Type of expectation test that dataset is subjected to
* @return this
*/
public DataQualityAssertionsDatasetFacetAssertionsBuilder assertion(String assertion) {
this.assertion = assertion;
return this;
}
/**
* @param success the success
* @return this
*/
public DataQualityAssertionsDatasetFacetAssertionsBuilder success(Boolean success) {
this.success = success;
return this;
}
/**
* @param column Column that expectation is testing. It should match the name provided in SchemaDatasetFacet. If column field is empty, then expectation refers to whole dataset.
* @return this
*/
public DataQualityAssertionsDatasetFacetAssertionsBuilder column(String column) {
this.column = column;
return this;
}
/**
* build an instance of DataQualityAssertionsDatasetFacetAssertions from the fields set in the builder
*/
@Override
public DataQualityAssertionsDatasetFacetAssertions build() {
DataQualityAssertionsDatasetFacetAssertions __result = new DataQualityAssertionsDatasetFacetAssertions(assertion, success, column);
return __result;
}
}
/**
* model class for ColumnLineageDatasetFacetFieldsAdditionalInputFields
*/
@JsonDeserialize(
as = ColumnLineageDatasetFacetFieldsAdditionalInputFields.class
)
@JsonPropertyOrder({
"namespace",
"name",
"field"
})
public static final class ColumnLineageDatasetFacetFieldsAdditionalInputFields {
private final String namespace;
private final String name;
private final String field;
@JsonAnySetter
private final Map additionalProperties;
/**
* @param namespace The input dataset namespace
* @param name The input dataset name
* @param field The input field
*/
@JsonCreator
private ColumnLineageDatasetFacetFieldsAdditionalInputFields(
@JsonProperty("namespace") String namespace, @JsonProperty("name") String name,
@JsonProperty("field") String field) {
this.namespace = namespace;
this.name = name;
this.field = field;
this.additionalProperties = new LinkedHashMap<>();
}
/**
* @return The input dataset namespace
*/
public String getNamespace() {
return namespace;
}
/**
* @return The input dataset name
*/
public String getName() {
return name;
}
/**
* @return The input field
*/
public String getField() {
return field;
}
/**
* @return additional properties
*/
@JsonAnyGetter
public Map getAdditionalProperties() {
return additionalProperties;
}
/**
* Get object with additional properties
*/
void withAdditionalProperties() {
}
}
/**
* builder class for ColumnLineageDatasetFacetFieldsAdditionalInputFields
*/
public static final class ColumnLineageDatasetFacetFieldsAdditionalInputFieldsBuilder implements Builder {
private String namespace;
private String name;
private String field;
private final Map additionalProperties = new LinkedHashMap<>();
/**
* @param namespace The input dataset namespace
* @return this
*/
public ColumnLineageDatasetFacetFieldsAdditionalInputFieldsBuilder namespace(String namespace) {
this.namespace = namespace;
return this;
}
/**
* @param name The input dataset name
* @return this
*/
public ColumnLineageDatasetFacetFieldsAdditionalInputFieldsBuilder name(String name) {
this.name = name;
return this;
}
/**
* @param field The input field
* @return this
*/
public ColumnLineageDatasetFacetFieldsAdditionalInputFieldsBuilder field(String field) {
this.field = field;
return this;
}
/**
* add additional properties
* @param key the additional property name
* @param value the additional property value
* @return this
*/
public ColumnLineageDatasetFacetFieldsAdditionalInputFieldsBuilder put(String key,
Object value) {
this.additionalProperties.put(key, value);return this;
}
/**
* build an instance of ColumnLineageDatasetFacetFieldsAdditionalInputFields from the fields set in the builder
*/
@Override
public ColumnLineageDatasetFacetFieldsAdditionalInputFields build() {
ColumnLineageDatasetFacetFieldsAdditionalInputFields __result = new ColumnLineageDatasetFacetFieldsAdditionalInputFields(namespace, name, field);
__result.getAdditionalProperties().putAll(additionalProperties);
return __result;
}
}
/**
* model class for ParentRunFacetRun
*/
@JsonDeserialize(
as = ParentRunFacetRun.class
)
@JsonPropertyOrder("runId")
public static final class ParentRunFacetRun {
private final UUID runId;
/**
* @param runId The globally unique ID of the run associated with the job.
*/
@JsonCreator
private ParentRunFacetRun(@JsonProperty("runId") UUID runId) {
this.runId = runId;
}
/**
* @return The globally unique ID of the run associated with the job.
*/
public UUID getRunId() {
return runId;
}
}
/**
* builder class for ParentRunFacetRun
*/
public static final class ParentRunFacetRunBuilder implements Builder {
private UUID runId;
/**
* @param runId The globally unique ID of the run associated with the job.
* @return this
*/
public ParentRunFacetRunBuilder runId(UUID runId) {
this.runId = runId;
return this;
}
/**
* build an instance of ParentRunFacetRun from the fields set in the builder
*/
@Override
public ParentRunFacetRun build() {
ParentRunFacetRun __result = new ParentRunFacetRun(runId);
return __result;
}
}
public static class DefaultOutputDatasetFacet implements OutputDatasetFacet {
private final URI _producer;
private final URI _schemaURL;
@JsonAnySetter
private final Map additionalProperties;
/**
* @param _producer URI identifying the producer of this metadata. For example this could be a git url with a given tag or sha
*/
@JsonCreator
public DefaultOutputDatasetFacet(@JsonProperty("_producer") URI _producer) {
this._producer = _producer;
this._schemaURL = URI.create("https://openlineage.io/spec/2-0-2/OpenLineage.json#/$defs/OutputDatasetFacet");
this.additionalProperties = new LinkedHashMap<>();
}
/**
* @return URI identifying the producer of this metadata. For example this could be a git url with a given tag or sha
*/
@Override
public URI get_producer() {
return _producer;
}
/**
* @return The JSON Pointer (https://tools.ietf.org/html/rfc6901) URL to the corresponding version of the schema definition for this facet
*/
@Override
public URI get_schemaURL() {
return _schemaURL;
}
/**
* @return additional properties
*/
@JsonAnyGetter
@Override
public Map getAdditionalProperties() {
return additionalProperties;
}
}
/**
* Interface for OutputDatasetFacet
*/
@JsonDeserialize(
as = DefaultOutputDatasetFacet.class
)
public interface OutputDatasetFacet {
/**
* @return URI identifying the producer of this metadata. For example this could be a git url with a given tag or sha
*/
URI get_producer();
/**
* @return The JSON Pointer (https://tools.ietf.org/html/rfc6901) URL to the corresponding version of the schema definition for this facet
*/
URI get_schemaURL();
/**
* @return additional properties
*/
Map getAdditionalProperties();
}
/**
* model class for StaticDataset
*/
@JsonDeserialize(
as = StaticDataset.class
)
@JsonPropertyOrder({
"namespace",
"name",
"facets"
})
public static final class StaticDataset implements Dataset {
private final String namespace;
private final String name;
private final DatasetFacets facets;
/**
* @param namespace The namespace containing that dataset
* @param name The unique name for that dataset within that namespace
* @param facets The facets for this dataset
*/
@JsonCreator
private StaticDataset(@JsonProperty("namespace") String namespace,
@JsonProperty("name") String name, @JsonProperty("facets") DatasetFacets facets) {
this.namespace = namespace;
this.name = name;
this.facets = facets;
}
/**
* @return The namespace containing that dataset
*/
@Override
public String getNamespace() {
return namespace;
}
/**
* @return The unique name for that dataset within that namespace
*/
@Override
public String getName() {
return name;
}
/**
* @return The facets for this dataset
*/
@Override
public DatasetFacets getFacets() {
return facets;
}
}
/**
* builder class for StaticDataset
*/
public static final class StaticDatasetBuilder implements Builder {
private String namespace;
private String name;
private DatasetFacets facets;
/**
* @param namespace The namespace containing that dataset
* @return this
*/
public StaticDatasetBuilder namespace(String namespace) {
this.namespace = namespace;
return this;
}
/**
* @param name The unique name for that dataset within that namespace
* @return this
*/
public StaticDatasetBuilder name(String name) {
this.name = name;
return this;
}
/**
* @param facets The facets for this dataset
* @return this
*/
public StaticDatasetBuilder facets(DatasetFacets facets) {
this.facets = facets;
return this;
}
/**
* build an instance of StaticDataset from the fields set in the builder
*/
@Override
public StaticDataset build() {
StaticDataset __result = new StaticDataset(namespace, name, facets);
return __result;
}
}
/**
* model class for ColumnLineageDatasetFacetFields
*/
@JsonDeserialize(
as = ColumnLineageDatasetFacetFields.class
)
@JsonPropertyOrder
public static final class ColumnLineageDatasetFacetFields {
@JsonAnySetter
private final Map additionalProperties;
@JsonCreator
private ColumnLineageDatasetFacetFields() {
this.additionalProperties = new LinkedHashMap<>();
}
/**
* @return additional properties
*/
@JsonAnyGetter
public Map getAdditionalProperties() {
return additionalProperties;
}
/**
* Get object with additional properties
*/
void withAdditionalProperties() {
}
}
/**
* builder class for ColumnLineageDatasetFacetFields
*/
public static final class ColumnLineageDatasetFacetFieldsBuilder implements Builder {
private final Map additionalProperties = new LinkedHashMap<>();
/**
* add additional properties
* @param key the additional property name
* @param value the additional property value
* @return this
*/
public ColumnLineageDatasetFacetFieldsBuilder put(String key,
ColumnLineageDatasetFacetFieldsAdditional value) {
this.additionalProperties.put(key, value);return this;
}
/**
* build an instance of ColumnLineageDatasetFacetFields from the fields set in the builder
*/
@Override
public ColumnLineageDatasetFacetFields build() {
ColumnLineageDatasetFacetFields __result = new ColumnLineageDatasetFacetFields();
__result.getAdditionalProperties().putAll(additionalProperties);
return __result;
}
}
/**
* model class for SymlinksDatasetFacet
*/
@JsonDeserialize(
as = SymlinksDatasetFacet.class
)
@JsonPropertyOrder({
"_producer",
"_schemaURL",
"_deleted",
"identifiers"
})
public static final class SymlinksDatasetFacet implements DatasetFacet {
private final URI _producer;
private final URI _schemaURL;
private final Boolean _deleted;
private final List identifiers;
@JsonAnySetter
private final Map additionalProperties;
/**
* @param _producer URI identifying the producer of this metadata. For example this could be a git url with a given tag or sha
* @param identifiers the identifiers
*/
@JsonCreator
private SymlinksDatasetFacet(@JsonProperty("_producer") URI _producer,
@JsonProperty("identifiers") List identifiers) {
this._producer = _producer;
this._schemaURL = URI.create("https://openlineage.io/spec/facets/1-0-0/SymlinksDatasetFacet.json#/$defs/SymlinksDatasetFacet");
this._deleted = null;
this.identifiers = identifiers;
this.additionalProperties = new LinkedHashMap<>();
}
/**
* @return URI identifying the producer of this metadata. For example this could be a git url with a given tag or sha
*/
@Override
public URI get_producer() {
return _producer;
}
/**
* @return The JSON Pointer (https://tools.ietf.org/html/rfc6901) URL to the corresponding version of the schema definition for this facet
*/
@Override
public URI get_schemaURL() {
return _schemaURL;
}
/**
* @return set to true to delete a facet
*/
@Override
public Boolean get_deleted() {
return _deleted;
}
public List getIdentifiers() {
return identifiers;
}
/**
* @return additional properties
*/
@JsonAnyGetter
@Override
public Map getAdditionalProperties() {
return additionalProperties;
}
/**
* Get object with additional properties
*/
void withAdditionalProperties() {
}
}
/**
* builder class for SymlinksDatasetFacet
*/
public final class SymlinksDatasetFacetBuilder implements Builder {
private List identifiers;
private final Map additionalProperties = new LinkedHashMap<>();
/**
* @param identifiers the identifiers
* @return this
*/
public SymlinksDatasetFacetBuilder identifiers(
List identifiers) {
this.identifiers = identifiers;
return this;
}
/**
* add additional properties
* @param key the additional property name
* @param value the additional property value
* @return this
*/
public SymlinksDatasetFacetBuilder put(String key, Object value) {
this.additionalProperties.put(key, value);return this;
}
/**
* build an instance of SymlinksDatasetFacet from the fields set in the builder
*/
@Override
public SymlinksDatasetFacet build() {
SymlinksDatasetFacet __result = new SymlinksDatasetFacet(OpenLineage.this.producer, identifiers);
__result.getAdditionalProperties().putAll(additionalProperties);
return __result;
}
}
/**
* model class for StorageDatasetFacet
*/
@JsonDeserialize(
as = StorageDatasetFacet.class
)
@JsonPropertyOrder({
"_producer",
"_schemaURL",
"_deleted",
"storageLayer",
"fileFormat"
})
public static final class StorageDatasetFacet implements DatasetFacet {
private final URI _producer;
private final URI _schemaURL;
private final Boolean _deleted;
private final String storageLayer;
private final String fileFormat;
@JsonAnySetter
private final Map additionalProperties;
/**
* @param _producer URI identifying the producer of this metadata. For example this could be a git url with a given tag or sha
* @param storageLayer Storage layer provider with allowed values: iceberg, delta.
* @param fileFormat File format with allowed values: parquet, orc, avro, json, csv, text, xml.
*/
@JsonCreator
private StorageDatasetFacet(@JsonProperty("_producer") URI _producer,
@JsonProperty("storageLayer") String storageLayer,
@JsonProperty("fileFormat") String fileFormat) {
this._producer = _producer;
this._schemaURL = URI.create("https://openlineage.io/spec/facets/1-0-0/StorageDatasetFacet.json#/$defs/StorageDatasetFacet");
this._deleted = null;
this.storageLayer = storageLayer;
this.fileFormat = fileFormat;
this.additionalProperties = new LinkedHashMap<>();
}
/**
* @return URI identifying the producer of this metadata. For example this could be a git url with a given tag or sha
*/
@Override
public URI get_producer() {
return _producer;
}
/**
* @return The JSON Pointer (https://tools.ietf.org/html/rfc6901) URL to the corresponding version of the schema definition for this facet
*/
@Override
public URI get_schemaURL() {
return _schemaURL;
}
/**
* @return set to true to delete a facet
*/
@Override
public Boolean get_deleted() {
return _deleted;
}
/**
* @return Storage layer provider with allowed values: iceberg, delta.
*/
public String getStorageLayer() {
return storageLayer;
}
/**
* @return File format with allowed values: parquet, orc, avro, json, csv, text, xml.
*/
public String getFileFormat() {
return fileFormat;
}
/**
* @return additional properties
*/
@JsonAnyGetter
@Override
public Map getAdditionalProperties() {
return additionalProperties;
}
/**
* Get object with additional properties
*/
void withAdditionalProperties() {
}
}
/**
* builder class for StorageDatasetFacet
*/
public final class StorageDatasetFacetBuilder implements Builder {
private String storageLayer;
private String fileFormat;
private final Map additionalProperties = new LinkedHashMap<>();
/**
* @param storageLayer Storage layer provider with allowed values: iceberg, delta.
* @return this
*/
public StorageDatasetFacetBuilder storageLayer(String storageLayer) {
this.storageLayer = storageLayer;
return this;
}
/**
* @param fileFormat File format with allowed values: parquet, orc, avro, json, csv, text, xml.
* @return this
*/
public StorageDatasetFacetBuilder fileFormat(String fileFormat) {
this.fileFormat = fileFormat;
return this;
}
/**
* add additional properties
* @param key the additional property name
* @param value the additional property value
* @return this
*/
public StorageDatasetFacetBuilder put(String key, Object value) {
this.additionalProperties.put(key, value);return this;
}
/**
* build an instance of StorageDatasetFacet from the fields set in the builder
*/
@Override
public StorageDatasetFacet build() {
StorageDatasetFacet __result = new StorageDatasetFacet(OpenLineage.this.producer, storageLayer, fileFormat);
__result.getAdditionalProperties().putAll(additionalProperties);
return __result;
}
}
/**
* model class for InputDataset
*/
@JsonDeserialize(
as = InputDataset.class
)
@JsonPropertyOrder({
"namespace",
"name",
"facets",
"inputFacets"
})
public static final class InputDataset implements Dataset {
private final String namespace;
private final String name;
private final DatasetFacets facets;
private final InputDatasetInputFacets inputFacets;
/**
* @param namespace The namespace containing that dataset
* @param name The unique name for that dataset within that namespace
* @param facets The facets for this dataset
* @param inputFacets The input facets for this dataset.
*/
@JsonCreator
private InputDataset(@JsonProperty("namespace") String namespace,
@JsonProperty("name") String name, @JsonProperty("facets") DatasetFacets facets,
@JsonProperty("inputFacets") InputDatasetInputFacets inputFacets) {
this.namespace = namespace;
this.name = name;
this.facets = facets;
this.inputFacets = inputFacets;
}
/**
* @return The namespace containing that dataset
*/
@Override
public String getNamespace() {
return namespace;
}
/**
* @return The unique name for that dataset within that namespace
*/
@Override
public String getName() {
return name;
}
/**
* @return The facets for this dataset
*/
@Override
public DatasetFacets getFacets() {
return facets;
}
/**
* @return The input facets for this dataset.
*/
public InputDatasetInputFacets getInputFacets() {
return inputFacets;
}
}
/**
* builder class for InputDataset
*/
public static final class InputDatasetBuilder implements Builder {
private String namespace;
private String name;
private DatasetFacets facets;
private InputDatasetInputFacets inputFacets;
/**
* @param namespace The namespace containing that dataset
* @return this
*/
public InputDatasetBuilder namespace(String namespace) {
this.namespace = namespace;
return this;
}
/**
* @param name The unique name for that dataset within that namespace
* @return this
*/
public InputDatasetBuilder name(String name) {
this.name = name;
return this;
}
/**
* @param facets The facets for this dataset
* @return this
*/
public InputDatasetBuilder facets(DatasetFacets facets) {
this.facets = facets;
return this;
}
/**
* @param inputFacets The input facets for this dataset.
* @return this
*/
public InputDatasetBuilder inputFacets(InputDatasetInputFacets inputFacets) {
this.inputFacets = inputFacets;
return this;
}
/**
* build an instance of InputDataset from the fields set in the builder
*/
@Override
public InputDataset build() {
InputDataset __result = new InputDataset(namespace, name, facets, inputFacets);
return __result;
}
}
/**
* model class for DatasourceDatasetFacet
*/
@JsonDeserialize(
as = DatasourceDatasetFacet.class
)
@JsonPropertyOrder({
"_producer",
"_schemaURL",
"_deleted",
"name",
"uri"
})
public static final class DatasourceDatasetFacet implements DatasetFacet {
private final URI _producer;
private final URI _schemaURL;
private final Boolean _deleted;
private final String name;
private final URI uri;
@JsonAnySetter
private final Map additionalProperties;
/**
* @param _producer URI identifying the producer of this metadata. For example this could be a git url with a given tag or sha
* @param name the name
* @param uri the uri
*/
@JsonCreator
private DatasourceDatasetFacet(@JsonProperty("_producer") URI _producer,
@JsonProperty("name") String name, @JsonProperty("uri") URI uri) {
this._producer = _producer;
this._schemaURL = URI.create("https://openlineage.io/spec/facets/1-0-0/DatasourceDatasetFacet.json#/$defs/DatasourceDatasetFacet");
this._deleted = null;
this.name = name;
this.uri = uri;
this.additionalProperties = new LinkedHashMap<>();
}
/**
* @return URI identifying the producer of this metadata. For example this could be a git url with a given tag or sha
*/
@Override
public URI get_producer() {
return _producer;
}
/**
* @return The JSON Pointer (https://tools.ietf.org/html/rfc6901) URL to the corresponding version of the schema definition for this facet
*/
@Override
public URI get_schemaURL() {
return _schemaURL;
}
/**
* @return set to true to delete a facet
*/
@Override
public Boolean get_deleted() {
return _deleted;
}
public String getName() {
return name;
}
public URI getUri() {
return uri;
}
/**
* @return additional properties
*/
@JsonAnyGetter
@Override
public Map getAdditionalProperties() {
return additionalProperties;
}
/**
* Get object with additional properties
*/
void withAdditionalProperties() {
}
}
/**
* builder class for DatasourceDatasetFacet
*/
public final class DatasourceDatasetFacetBuilder implements Builder {
private String name;
private URI uri;
private final Map additionalProperties = new LinkedHashMap<>();
/**
* @param name the name
* @return this
*/
public DatasourceDatasetFacetBuilder name(String name) {
this.name = name;
return this;
}
/**
* @param uri the uri
* @return this
*/
public DatasourceDatasetFacetBuilder uri(URI uri) {
this.uri = uri;
return this;
}
/**
* add additional properties
* @param key the additional property name
* @param value the additional property value
* @return this
*/
public DatasourceDatasetFacetBuilder put(String key, Object value) {
this.additionalProperties.put(key, value);return this;
}
/**
* build an instance of DatasourceDatasetFacet from the fields set in the builder
*/
@Override
public DatasourceDatasetFacet build() {
DatasourceDatasetFacet __result = new DatasourceDatasetFacet(OpenLineage.this.producer, name, uri);
__result.getAdditionalProperties().putAll(additionalProperties);
return __result;
}
}
/**
* model class for LifecycleStateChangeDatasetFacet
*/
@JsonDeserialize(
as = LifecycleStateChangeDatasetFacet.class
)
@JsonPropertyOrder({
"_producer",
"_schemaURL",
"_deleted",
"lifecycleStateChange",
"previousIdentifier"
})
public static final class LifecycleStateChangeDatasetFacet implements DatasetFacet {
private final URI _producer;
private final URI _schemaURL;
private final Boolean _deleted;
private final LifecycleStateChangeDatasetFacet.LifecycleStateChange lifecycleStateChange;
private final LifecycleStateChangeDatasetFacetPreviousIdentifier previousIdentifier;
@JsonAnySetter
private final Map additionalProperties;
/**
* @param _producer URI identifying the producer of this metadata. For example this could be a git url with a given tag or sha
* @param lifecycleStateChange The lifecycle state change.
* @param previousIdentifier Previous name of the dataset in case of renaming it.
*/
@JsonCreator
private LifecycleStateChangeDatasetFacet(@JsonProperty("_producer") URI _producer,
@JsonProperty("lifecycleStateChange") LifecycleStateChangeDatasetFacet.LifecycleStateChange lifecycleStateChange,
@JsonProperty("previousIdentifier") LifecycleStateChangeDatasetFacetPreviousIdentifier previousIdentifier) {
this._producer = _producer;
this._schemaURL = URI.create("https://openlineage.io/spec/facets/1-0-0/LifecycleStateChangeDatasetFacet.json#/$defs/LifecycleStateChangeDatasetFacet");
this._deleted = null;
this.lifecycleStateChange = lifecycleStateChange;
this.previousIdentifier = previousIdentifier;
this.additionalProperties = new LinkedHashMap<>();
}
/**
* @return URI identifying the producer of this metadata. For example this could be a git url with a given tag or sha
*/
@Override
public URI get_producer() {
return _producer;
}
/**
* @return The JSON Pointer (https://tools.ietf.org/html/rfc6901) URL to the corresponding version of the schema definition for this facet
*/
@Override
public URI get_schemaURL() {
return _schemaURL;
}
/**
* @return set to true to delete a facet
*/
@Override
public Boolean get_deleted() {
return _deleted;
}
/**
* @return The lifecycle state change.
*/
public LifecycleStateChangeDatasetFacet.LifecycleStateChange getLifecycleStateChange() {
return lifecycleStateChange;
}
/**
* @return Previous name of the dataset in case of renaming it.
*/
public LifecycleStateChangeDatasetFacetPreviousIdentifier getPreviousIdentifier() {
return previousIdentifier;
}
/**
* @return additional properties
*/
@JsonAnyGetter
@Override
public Map getAdditionalProperties() {
return additionalProperties;
}
/**
* Get object with additional properties
*/
void withAdditionalProperties() {
}
public enum LifecycleStateChange {
ALTER,
CREATE,
DROP,
OVERWRITE,
RENAME,
TRUNCATE
}
}
/**
* builder class for LifecycleStateChangeDatasetFacet
*/
public final class LifecycleStateChangeDatasetFacetBuilder implements Builder {
private LifecycleStateChangeDatasetFacet.LifecycleStateChange lifecycleStateChange;
private LifecycleStateChangeDatasetFacetPreviousIdentifier previousIdentifier;
private final Map additionalProperties = new LinkedHashMap<>();
/**
* @param lifecycleStateChange The lifecycle state change.
* @return this
*/
public LifecycleStateChangeDatasetFacetBuilder lifecycleStateChange(
LifecycleStateChangeDatasetFacet.LifecycleStateChange lifecycleStateChange) {
this.lifecycleStateChange = lifecycleStateChange;
return this;
}
/**
* @param previousIdentifier Previous name of the dataset in case of renaming it.
* @return this
*/
public LifecycleStateChangeDatasetFacetBuilder previousIdentifier(
LifecycleStateChangeDatasetFacetPreviousIdentifier previousIdentifier) {
this.previousIdentifier = previousIdentifier;
return this;
}
/**
* add additional properties
* @param key the additional property name
* @param value the additional property value
* @return this
*/
public LifecycleStateChangeDatasetFacetBuilder put(String key, Object value) {
this.additionalProperties.put(key, value);return this;
}
/**
* build an instance of LifecycleStateChangeDatasetFacet from the fields set in the builder
*/
@Override
public LifecycleStateChangeDatasetFacet build() {
LifecycleStateChangeDatasetFacet __result = new LifecycleStateChangeDatasetFacet(OpenLineage.this.producer, lifecycleStateChange, previousIdentifier);
__result.getAdditionalProperties().putAll(additionalProperties);
return __result;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy