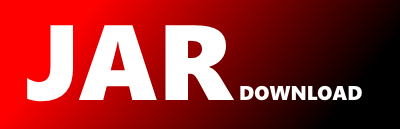
io.openlineage.client.OpenLineageClientUtils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of openlineage-java Show documentation
Show all versions of openlineage-java Show documentation
Java library for OpenLineage
/*
/* Copyright 2018-2024 contributors to the OpenLineage project
/* SPDX-License-Identifier: Apache-2.0
*/
package io.openlineage.client;
import static com.fasterxml.jackson.databind.DeserializationFeature.FAIL_ON_UNKNOWN_PROPERTIES;
import com.fasterxml.jackson.annotation.JsonFilter;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.core.JsonFactory;
import com.fasterxml.jackson.core.JsonProcessingException;
import com.fasterxml.jackson.core.type.TypeReference;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.fasterxml.jackson.databind.SerializationFeature;
import com.fasterxml.jackson.databind.ser.impl.SimpleBeanPropertyFilter;
import com.fasterxml.jackson.databind.ser.impl.SimpleFilterProvider;
import com.fasterxml.jackson.dataformat.yaml.YAMLFactory;
import com.fasterxml.jackson.datatype.jdk8.Jdk8Module;
import com.fasterxml.jackson.datatype.jsr310.JavaTimeModule;
import io.openlineage.client.OpenLineage.RunEvent;
import java.io.FileNotFoundException;
import java.io.IOException;
import java.io.InputStream;
import java.io.UncheckedIOException;
import java.net.URI;
import java.net.URISyntaxException;
import java.nio.file.Files;
import java.nio.file.Path;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.stream.Collectors;
import lombok.NonNull;
import lombok.extern.slf4j.Slf4j;
/**
* Utility class for {@link OpenLineageClient} that provides common functionalities for object
* mapping, JSON and YAML parsing, and URI manipulation.
*/
@Slf4j
public final class OpenLineageClientUtils {
private OpenLineageClientUtils() {}
private static final ObjectMapper MAPPER = newObjectMapper();
private static final ObjectMapper YML = newObjectMapper(new YAMLFactory());
private static final ObjectMapper JSON = newObjectMapper();
@JsonFilter("disabledFacets")
public class DisabledFacetsMixin {}
/**
* Creates a new {@link ObjectMapper} instance configured with modules for JDK8 and JavaTime,
* including settings to ignore unknown properties and to not write dates as timestamps.
*
* @return A configured {@link ObjectMapper} instance.
*/
public static ObjectMapper newObjectMapper() {
return newObjectMapper(new JsonFactory());
}
/**
* Creates a new {@link ObjectMapper} instance configured with modules for JDK8 and JavaTime,
* including settings to ignore unknown properties and to not write dates as timestamps.
*
* @return A configured {@link ObjectMapper} instance.
*/
public static ObjectMapper newObjectMapper(JsonFactory jsonFactory) {
final ObjectMapper mapper = new ObjectMapper(jsonFactory);
mapper.registerModule(new Jdk8Module());
mapper.registerModule(new JavaTimeModule());
mapper.setSerializationInclusion(JsonInclude.Include.NON_NULL);
mapper.disable(FAIL_ON_UNKNOWN_PROPERTIES);
mapper.disable(SerializationFeature.WRITE_DATES_AS_TIMESTAMPS);
return mapper;
}
/**
* Configures the object mapper to exclude specified facets from being serialized.
*
* @param disableFacets Array of facet names to be excluded from serialization.
*/
public static void configureObjectMapper(String... disableFacets) {
if (disableFacets == null) {
return;
}
SimpleFilterProvider simpleFilterProvider =
new SimpleFilterProvider()
.addFilter(
"disabledFacets", SimpleBeanPropertyFilter.serializeAllExcept(disableFacets));
MAPPER.setFilterProvider(simpleFilterProvider);
MAPPER.addMixIn(Object.class, DisabledFacetsMixin.class);
}
/**
* Converts the provided value to a JSON string.
*
* @param value The object to be converted to JSON.
* @return A JSON string representation of the object.
* @throws UncheckedIOException If an I/O error occurs during conversion.
*/
public static String toJson(@NonNull final Object value) throws UncheckedIOException {
try {
return MAPPER.writeValueAsString(value);
} catch (JsonProcessingException e) {
throw new UncheckedIOException(e);
}
}
/**
* Converts the provided JSON string to an instance of the specified type.
*
* @param json The JSON string to be converted.
* @param type The type to convert the JSON string into.
* @param The generic type of the return value.
* @return An instance of the specified type.
* @throws UncheckedIOException If an I/O error occurs during conversion.
*/
public static T fromJson(@NonNull final String json, @NonNull final TypeReference type)
throws UncheckedIOException {
try {
return MAPPER.readValue(json, type);
} catch (IOException e) {
throw new UncheckedIOException(e);
}
}
/**
* Convenience method to convert a JSON string directly into a {@link RunEvent} instance.
*
* @param json The JSON string representing a {@link RunEvent}.
* @return An instance of {@link RunEvent}.
* @throws UncheckedIOException If an I/O error occurs during conversion.
*/
public static RunEvent runEventFromJson(@NonNull final String json) throws UncheckedIOException {
return fromJson(json, new TypeReference() {});
}
/**
* Converts the value of an object from one type to another.
*
* @param fromValue The object whose value is to be converted.
* @param toValueType The target type for the conversion.
* @param The generic type of the target type.
* @return An object of the target type with the value converted from the original object.
*/
public static T convertValue(Object fromValue, Class toValueType) {
return MAPPER.convertValue(fromValue, toValueType);
}
/**
* Merges the given facets map with an existing facets container, giving precedence to the values
* in the facets map.
*
* @param facetsMap A map containing facets to be merged.
* @param facetsContainer The existing container of facets.
* @param klass The class of the facets container.
* @param The type of the facets container.
* @param The type of facets in the map.
* @return A new instance of the facets container with merged values.
*/
public static T mergeFacets(Map facetsMap, T facetsContainer, Class klass) {
if (facetsContainer == null) {
return MAPPER.convertValue(facetsMap, klass);
}
Map targetMap =
MAPPER.convertValue(facetsContainer, new TypeReference
© 2015 - 2024 Weber Informatics LLC | Privacy Policy