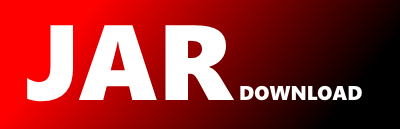
io.opentelemetry.contrib.jmxmetrics.MBeanHelper.groovy Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of opentelemetry-jmx-metrics Show documentation
Show all versions of opentelemetry-jmx-metrics Show documentation
JMX metrics gathering Groovy script runner
/*
* Copyright The OpenTelemetry Authors
* SPDX-License-Identifier: Apache-2.0
*/
package io.opentelemetry.contrib.jmxmetrics
import groovy.jmx.GroovyMBean
import groovy.transform.PackageScope
import javax.management.MBeanServerConnection
import javax.management.ObjectName
import javax.management.AttributeNotFoundException
import java.util.logging.Logger
/**
* A helper for easy {@link GroovyMBean} querying, creation, and protected attribute access.
*
* Basic query functionality is provided by the static queryJmx methods, while MBeanHelper
* instances operate on an underlying GroovyMBean list field.
*
* Specifying if a single underlying GroovyMBean is expected or of interest is done via the
* isSingle argument, where resulting attribute values are only returned for the first
* match.
*
* Intended to be used via the script-bound `otel` instance:
*
* def singleMBean = otel.mbean("com.example:type=SingleType")
* def multipleMBeans = otel.mbeans("com.example:type=MultipleType,*")
* [singleMBean, multipleMBeans].each { it.fetch() }
*
*/
class MBeanHelper {
private static final Logger logger = Logger.getLogger(MBeanHelper.class.getName());
private final JmxClient jmxClient
private final boolean isSingle
private final List objectNames
private final Map attributeTransformation
private List mbeans
MBeanHelper(JmxClient jmxClient, String objectName, boolean isSingle) {
this.jmxClient = jmxClient
this.objectNames = Collections.unmodifiableList([objectName])
this.isSingle = isSingle
this.attributeTransformation = [:] as Map>
}
MBeanHelper(JmxClient jmxClient, List objectNames) {
this.jmxClient = jmxClient
this.objectNames = Collections.unmodifiableList(objectNames)
this.isSingle = false
this.attributeTransformation = [:] as Map>
}
MBeanHelper(JmxClient jmxClient, String objectName, boolean isSingle, Map> attributeTransformation ) {
this.jmxClient = jmxClient
this.objectNames = Collections.unmodifiableList([objectName])
this.isSingle = isSingle
this.attributeTransformation = attributeTransformation
}
MBeanHelper(JmxClient jmxClient, List objectNames, Map> attributeTransformation) {
this.jmxClient = jmxClient
this.objectNames = Collections.unmodifiableList(objectNames)
this.isSingle = false
this.attributeTransformation = attributeTransformation
}
@PackageScope static List queryJmx(JmxClient jmxClient, String objNameStr) {
return queryJmx(jmxClient, new ObjectName(objNameStr))
}
@PackageScope static List queryJmx(JmxClient jmxClient, ObjectName objName) {
List names = jmxClient.query(objName)
MBeanServerConnection server = jmxClient.connection
return names.collect { new GroovyMBean(server, it) }
}
void fetch() {
this.mbeans = []
for(objectName in objectNames){
def tmpMbeans = queryJmx(jmxClient, objectName)
if (tmpMbeans.size() == 0) {
logger.warning("Failed to fetch MBean ${objectName}.")
} else {
this.mbeans.addAll(tmpMbeans)
logger.fine("Fetched ${tmpMbeans.size()} MBeans - ${tmpMbeans}")
}
}
}
@PackageScope List getMBeans() {
if (mbeans == null || mbeans.size() == 0) {
logger.warning("No active MBeans. Be sure to fetch() before updating any applicable instruments.")
return []
}
return isSingle ? [mbeans[0]]: mbeans
}
@PackageScope List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy