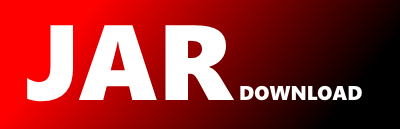
io.opentelemetry.instrumentation.jmx.yaml.RuleParser Maven / Gradle / Ivy
/*
* Copyright The OpenTelemetry Authors
* SPDX-License-Identifier: Apache-2.0
*/
package io.opentelemetry.instrumentation.jmx.yaml;
import static java.util.Collections.emptyList;
import static java.util.logging.Level.INFO;
import static java.util.logging.Level.WARNING;
import io.opentelemetry.instrumentation.jmx.engine.MetricConfiguration;
import java.io.InputStream;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
import java.util.logging.Logger;
import java.util.stream.Collectors;
import javax.annotation.Nullable;
import org.snakeyaml.engine.v2.api.Load;
import org.snakeyaml.engine.v2.api.LoadSettings;
/** Parse a YAML file containing a number of rules. */
public class RuleParser {
// The YAML parser will create and populate objects of the following classes from the
// io.opentelemetry.instrumentation.runtimemetrics.jmx.conf.data package:
// - JmxConfig
// - JmxRule (a subclass of MetricStructure)
// - Metric (a subclass of MetricStructure)
// To populate the objects, the parser will call setter methods for the object fields with
// whatever comes as the result of parsing the YAML file. This means that the arguments for
// the setter calls will be non-null, unless the user will explicitly specify the 'null' literal.
// However, there's hardly any difference in user visible error messages whether the setter
// throws an IllegalArgumentException, or NullPointerException. Therefore, in all above
// classes we skip explicit checks for nullnes in the field setters, and let the setters
// crash with NullPointerException instead.
private static final Logger logger = Logger.getLogger(RuleParser.class.getName());
private static final RuleParser theParser = new RuleParser();
public static RuleParser get() {
return theParser;
}
private RuleParser() {}
@SuppressWarnings("unchecked")
public JmxConfig loadConfig(InputStream is) {
LoadSettings settings = LoadSettings.builder().build();
Load yaml = new Load(settings);
Map data = (Map) yaml.loadFromInputStream(is);
if (data == null) {
return new JmxConfig(emptyList());
}
List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy