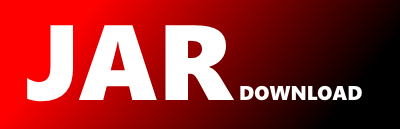
io.opentelemetry.javaagent.extension.StructuredConfigPropertiesBridge Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of opentelemetry-javaagent-extension-api Show documentation
Show all versions of opentelemetry-javaagent-extension-api Show documentation
Instrumentation of Java libraries using OpenTelemetry.
Please wait ...