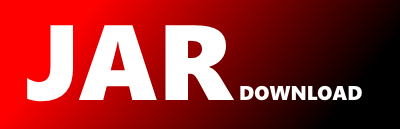
io.opentelemetry.javaagent.extension.matcher.HasSuperMethodMatcher Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of opentelemetry-javaagent-extension-api Show documentation
Show all versions of opentelemetry-javaagent-extension-api Show documentation
Instrumentation of Java libraries using OpenTelemetry.
/*
* Copyright The OpenTelemetry Authors
* SPDX-License-Identifier: Apache-2.0
*/
package io.opentelemetry.javaagent.extension.matcher;
import static io.opentelemetry.javaagent.extension.matcher.SafeHasSuperTypeMatcher.safeGetSuperClass;
import static net.bytebuddy.matcher.ElementMatchers.hasSignature;
import java.util.HashSet;
import java.util.Set;
import javax.annotation.Nullable;
import net.bytebuddy.description.method.MethodDescription;
import net.bytebuddy.description.type.TypeDefinition;
import net.bytebuddy.description.type.TypeList;
import net.bytebuddy.matcher.ElementMatcher;
/**
* Matches a method and all its declarations up the class hierarchy including interfaces using
* provided matcher.
*
* @param Type of the matched method.
*/
class HasSuperMethodMatcher
extends ElementMatcher.Junction.AbstractBase {
private final ElementMatcher super MethodDescription> matcher;
public HasSuperMethodMatcher(ElementMatcher super MethodDescription> matcher) {
this.matcher = matcher;
}
@Override
public boolean matches(MethodDescription target) {
if (target.isConstructor()) {
return false;
}
Junction signatureMatcher = hasSignature(target.asSignatureToken());
TypeDefinition declaringType = target.getDeclaringType();
Set checkedInterfaces = new HashSet<>(8);
while (declaringType != null) {
for (MethodDescription methodDescription : declaringType.getDeclaredMethods()) {
if (signatureMatcher.matches(methodDescription) && matcher.matches(methodDescription)) {
return true;
}
}
if (matchesInterface(declaringType.getInterfaces(), signatureMatcher, checkedInterfaces)) {
return true;
}
declaringType = safeGetSuperClass(declaringType);
}
return false;
}
private boolean matchesInterface(
TypeList.Generic interfaces,
Junction signatureMatcher,
Set checkedInterfaces) {
for (TypeDefinition type : interfaces) {
if (checkedInterfaces.add(type)) {
for (MethodDescription methodDescription : type.getDeclaredMethods()) {
if (signatureMatcher.matches(methodDescription) && matcher.matches(methodDescription)) {
return true;
}
}
if (matchesInterface(type.getInterfaces(), signatureMatcher, checkedInterfaces)) {
return true;
}
}
}
return false;
}
@Override
public String toString() {
return "hasSuperMethodMatcher(" + matcher + ")";
}
@Override
public boolean equals(@Nullable Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof HasSuperMethodMatcher)) {
return false;
}
HasSuperMethodMatcher> other = (HasSuperMethodMatcher>) obj;
return matcher.equals(other.matcher);
}
@Override
public int hashCode() {
return matcher.hashCode();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy