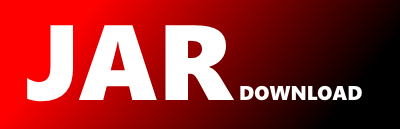
io.opentelemetry.sdk.trace.StringUtils Maven / Gradle / Ivy
Show all versions of opentelemetry-sdk-trace Show documentation
/*
* Copyright The OpenTelemetry Authors
* SPDX-License-Identifier: Apache-2.0
*/
package io.opentelemetry.sdk.trace;
import io.opentelemetry.api.common.AttributeKey;
import io.opentelemetry.api.common.AttributeType;
import java.util.ArrayList;
import java.util.List;
import javax.annotation.Nullable;
final class StringUtils {
/**
* If given attribute is of type STRING and has more characters than given {@code limit} then
* return new value with string truncated to {@code limit} characters.
*
* If given attribute is of type STRING_ARRAY and non-empty then return new value with every
* element truncated to {@code limit} characters.
*
*
Otherwise return given {@code value}
*/
@SuppressWarnings("unchecked")
static T truncateToSize(AttributeKey key, T value, int limit) {
if (value == null
|| ((key.getType() != AttributeType.STRING)
&& (key.getType() != AttributeType.STRING_ARRAY))) {
return value;
}
if (key.getType() == AttributeType.STRING_ARRAY) {
List strings = (List) value;
if (strings.isEmpty()) {
return value;
}
List newStrings = new ArrayList<>(strings.size());
for (String string : strings) {
newStrings.add(truncateToSize(string, limit));
}
return (T) newStrings;
}
return (T) truncateToSize((String) value, limit);
}
@Nullable
private static String truncateToSize(@Nullable String s, int limit) {
if (s == null || s.length() <= limit) {
return s;
}
return s.substring(0, limit);
}
private StringUtils() {}
}