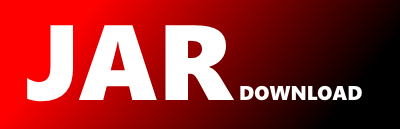
io.opentracing.impl.AbstractTracer Maven / Gradle / Ivy
/**
* Copyright 2016 The OpenTracing Authors
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
package io.opentracing.impl;
import io.opentracing.SpanContext;
import io.opentracing.Tracer;
import io.opentracing.propagation.Extractor;
import io.opentracing.propagation.Format;
import io.opentracing.propagation.Injector;
import java.util.Map;
import java.util.concurrent.ConcurrentHashMap;
import java.util.concurrent.ConcurrentMap;
abstract class AbstractTracer implements Tracer {
static final boolean BAGGAGE_ENABLED = !Boolean.getBoolean("opentracing.propagation.dropBaggage");
private final PropagationRegistry registry = new PropagationRegistry();
protected AbstractTracer() {
registry.register(Format.Builtin.TEXT_MAP, new TextMapInjectorImpl(this));
registry.register(Format.Builtin.TEXT_MAP, new TextMapExtractorImpl(this));
}
abstract AbstractSpanBuilder createSpanBuilder(String operationName);
@Override
public SpanBuilder buildSpan(String operationName){
return createSpanBuilder(operationName);
}
@Override
public void inject(SpanContext spanContext, Format format, C carrier) {
registry.getInjector(format).inject(spanContext, carrier);
}
@Override
public SpanBuilder extract(Format format, C carrier) {
return registry.getExtractor(format).extract(carrier);
}
public Injector register(Format format, Injector injector) {
return registry.register(format, injector);
}
public Extractor register(Format format, Extractor extractor) {
return registry.register(format, extractor);
}
/** @return the minimal set of properties required to propagate this span */
abstract Map getTraceState(SpanContext spanContext);
private static class PropagationRegistry {
private final ConcurrentMap injectors = new ConcurrentHashMap<>();
private final ConcurrentMap extractors = new ConcurrentHashMap<>();
public Injector getInjector(Format format) {
if (injectors.containsKey(format)) {
return injectors.get(format);
}
throw new AssertionError("no registered injector for " + format);
}
public Extractor getExtractor(Format format) {
if (extractors.containsKey(format)) {
return extractors.get(format);
}
throw new AssertionError("no registered extractor for " + format);
}
public Injector register(Format format, Injector injector) {
return injectors.put(format, injector);
}
public Extractor register(Format format, Extractor extractor) {
return extractors.put(format, extractor);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy