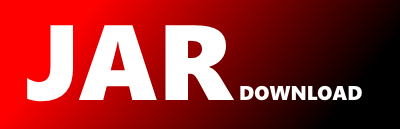
org.geotools.feature.AttributeImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gt-main Show documentation
Show all versions of gt-main Show documentation
The main module contains the GeoTools public interfaces that are used by
other GeoTools modules (and GeoTools applications). Where possible we make
use industry standard terms as provided by OGC and ISO standards.
The formal GeoTools public api consists of gt-metadata, jts and the gt-main module.
The main module contains the default implementations that are available provided
to other GeoTools modules using our factory system. Factories are obtained from
an appropriate FactoryFinder, giving applications a chance configure the factory
used using the Factory Hints facilities.
FilterFactory ff = CommonFactoryFinder.getFilterFactory();
Expression expr = ff.add( expression1, expression2 );
If you find yourself using implementation specific classes chances are you doing it wrong:
Expression expr = new AddImpl( expression1, expressiom2 );
The newest version!
/*
* GeoTools - The Open Source Java GIS Toolkit
* http://geotools.org
*
* (C) 2002-2008, Open Source Geospatial Foundation (OSGeo)
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation;
* version 2.1 of the License.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*/
package org.geotools.feature;
import org.geotools.feature.type.AttributeDescriptorImpl;
import org.geotools.feature.type.Types;
import org.geotools.util.Converters;
import org.geotools.util.Utilities;
import org.opengis.feature.Attribute;
import org.opengis.feature.type.AttributeDescriptor;
import org.opengis.feature.type.AttributeType;
import org.opengis.filter.identity.Identifier;
/**
* Simple, mutable class to store attributes.
*
* @author Rob Hranac, VFNY
* @author Chris Holmes, TOPP
* @author Ian Schneider
* @author Jody Garnett
* @author Gabriel Roldan
* @version $Id$
*/
public class AttributeImpl extends PropertyImpl implements Attribute {
/** id of the attribute. */
protected Identifier id = null;
public AttributeImpl(Object content, AttributeDescriptor descriptor, Identifier id) {
super(content, descriptor);
this.id = id;
}
public AttributeImpl(Object content, AttributeType type, Identifier id) {
this(content, new AttributeDescriptorImpl(type, type.getName(), 1, 1, true, null), id);
}
public Identifier getIdentifier() {
return id;
}
public AttributeDescriptor getDescriptor() {
return (AttributeDescriptor) super.getDescriptor();
}
public AttributeType getType() {
return (AttributeType) super.getType();
}
/** Override of setValue to convert the newValue to specified type if need be. */
public void setValue(Object newValue) throws IllegalArgumentException, IllegalStateException {
newValue = parse(newValue);
super.setValue(newValue);
}
/**
* Override of hashCode.
*
* @return hashCode for this object.
*/
public int hashCode() {
return super.hashCode() + (37 * (id == null ? 0 : id.hashCode()));
}
/**
* Override of equals.
*
* @param obj the object to be tested for equality.
* @return whether other is equal to this attribute Type.
*/
public boolean equals(Object obj) {
if (this == obj) {
return true;
}
if (!(obj instanceof Attribute)) {
return false;
}
if (!super.equals(obj)) {
return false;
}
Attribute att = (Attribute) obj;
return Utilities.equals(id, att.getIdentifier());
}
public void validate() {
Types.validate(this, this.getValue());
}
public String toString() {
StringBuffer sb = new StringBuffer(getClass().getSimpleName()).append(":");
sb.append(getDescriptor().getName().getLocalPart());
if (!getDescriptor()
.getName()
.getLocalPart()
.equals(getDescriptor().getType().getName().getLocalPart())
|| id != null) {
sb.append("<");
sb.append(getDescriptor().getType().getName().getLocalPart());
if (id != null) {
sb.append(" id=");
sb.append(id);
}
sb.append(">");
}
sb.append("=");
sb.append(value);
return sb.toString();
}
/**
* Allows this Attribute to convert an argument to its prefered storage type. If no parsing is
* possible, returns the original value. If a parse is attempted, yet fails (i.e. a poor decimal
* format) throw the Exception. This is mostly for use internally in Features, but implementors
* should simply follow the rules to be safe.
*
* @param value the object to attempt parsing of.
* @return value
converted to the preferred storage of this AttributeType
*
. If no parsing was possible then the same object is returned.
* @throws IllegalArgumentException if parsing is attempted and is unsuccessful.
*/
protected Object parse(Object value) throws IllegalArgumentException {
if (value != null) {
Class target = getType().getBinding();
if (!target.isAssignableFrom(value.getClass())) {
// attempt to convert
Object converted = Converters.convert(value, target);
if (converted != null) {
value = converted;
}
}
}
return value;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy