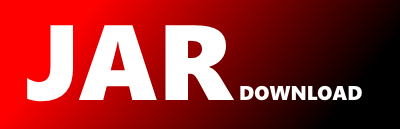
org.geotools.feature.DecoratingFeature Maven / Gradle / Ivy
Show all versions of gt-main Show documentation
/*
* GeoTools - The Open Source Java GIS Toolkit
* http://geotools.org
*
* (C) 2002-2008, Open Source Geospatial Foundation (OSGeo)
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation;
* version 2.1 of the License.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*/
package org.geotools.feature;
import java.util.Collection;
import java.util.List;
import java.util.Map;
import org.locationtech.jts.geom.Geometry;
import org.opengis.feature.GeometryAttribute;
import org.opengis.feature.IllegalAttributeException;
import org.opengis.feature.Property;
import org.opengis.feature.simple.SimpleFeature;
import org.opengis.feature.simple.SimpleFeatureType;
import org.opengis.feature.type.AttributeDescriptor;
import org.opengis.feature.type.Name;
import org.opengis.filter.identity.FeatureId;
import org.opengis.geometry.BoundingBox;
/**
* Base class for feature decorators.
*
* Subclasses should override those methods which are relevant to the decorator.
*
* @author Justin Deoliveira, The Open Planning Project
* @since 2.5
*/
public class DecoratingFeature implements SimpleFeature {
protected SimpleFeature delegate;
public DecoratingFeature(SimpleFeature delegate) {
this.delegate = delegate;
}
public Object getAttribute(int index) {
return delegate.getAttribute(index);
}
public Object getAttribute(Name arg0) {
return delegate.getAttribute(arg0);
}
public Object getAttribute(String path) {
return delegate.getAttribute(path);
}
public int getAttributeCount() {
return delegate.getAttributeCount();
}
public List