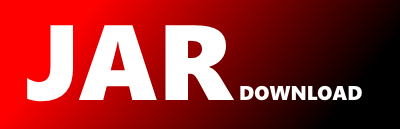
org.geotools.feature.FeatureComparators Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gt-main Show documentation
Show all versions of gt-main Show documentation
The main module contains the GeoTools public interfaces that are used by
other GeoTools modules (and GeoTools applications). Where possible we make
use industry standard terms as provided by OGC and ISO standards.
The formal GeoTools public api consists of gt-metadata, jts and the gt-main module.
The main module contains the default implementations that are available provided
to other GeoTools modules using our factory system. Factories are obtained from
an appropriate FactoryFinder, giving applications a chance configure the factory
used using the Factory Hints facilities.
FilterFactory ff = CommonFactoryFinder.getFilterFactory();
Expression expr = ff.add( expression1, expression2 );
If you find yourself using implementation specific classes chances are you doing it wrong:
Expression expr = new AddImpl( expression1, expressiom2 );
The newest version!
/*
* GeoTools - The Open Source Java GIS Toolkit
* http://geotools.org
*
* (C) 2002-2008, Open Source Geospatial Foundation (OSGeo)
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation;
* version 2.1 of the License.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*/
package org.geotools.feature;
import org.opengis.feature.simple.SimpleFeature;
/**
* A utility class for creating simple Comparators for Features.
*
* @author Ian Schneider
*/
public final class FeatureComparators {
/** A utility comparator for comparison by id. */
public static final java.util.Comparator BY_ID =
new java.util.Comparator() {
public int compare(Object o1, Object o2) {
SimpleFeature f1 = (SimpleFeature) o1;
SimpleFeature f2 = (SimpleFeature) o2;
return f1.getID().compareTo(f2.getID());
}
};
/** Private constructor so default constructor is not available for this utility class. */
private FeatureComparators() {}
/**
* Create a Comparator which compares Features by the attribute at the given index. The
* attribute at the index MUST be Comparable. This will probably not work for heterogenous
* collections, UNLESS the classes at the given index are the same.
*
* @param idx The index to look up attributes at.
* @return A new Comparator.
*/
public static java.util.Comparator byAttributeIndex(final int idx) {
return new Index(idx);
}
/**
* Create a Comparator which compares Features by the attribute found at the given path. The
* attribute found MUST be Comparable. This will probably not work for heterogenous collections,
* UNLESS the attributes found are the same class.
*
* @param name The xpath to use while comparing.
* @return A new Comparator.
*/
public static java.util.Comparator byAttributeName(final String name) {
return new Name(name);
}
/** A Comparator which performs the comparison on attributes at a given index. */
public static class Index implements java.util.Comparator {
/** the index of the attribute to compare against. */
private final int idx;
/**
* Create a new Comparator based on the given index.
*
* @param i The index.
*/
public Index(int i) {
idx = i;
}
/**
* Implementation of Comparator. Calls compareAtts to perform the actual comparison.
*
* @param o1 The first Feature.
* @param o2 The second Feature
* @return A value indicating less than, equal, or greater than.
*/
public int compare(Object o1, Object o2) {
SimpleFeature f1 = (SimpleFeature) o1;
SimpleFeature f2 = (SimpleFeature) o2;
return compareAtts(f1.getAttribute(idx), f2.getAttribute(idx));
}
/**
* Compares the two attributes.
*
* @param att1 The first attribute to compare.
* @param att2 The second attribute to compare.
* @return A value indicating less than, equal, or greater than.
*/
protected int compareAtts(Object att1, Object att2) {
return ((Comparable) att1).compareTo((Comparable) att2);
}
}
/** A Comparator which performs the comparison on attributes with a given name. */
public static class Name implements java.util.Comparator {
/** The name to compare on */
private final String name;
/**
* Create a new Comparator based on the given index.
*
* @param name The attribute name.
*/
public Name(String name) {
this.name = name;
}
/**
* Implementation of Comparator. Calls compareAtts to perform the actual comparison.
*
* @param o1 The first Feature.
* @param o2 The second Feature
* @return A value indicating less than, equal, or greater than.
*/
public int compare(Object o1, Object o2) {
SimpleFeature f1 = (SimpleFeature) o1;
SimpleFeature f2 = (SimpleFeature) o2;
return compareAtts(f1.getAttribute(name), f2.getAttribute(name));
}
/**
* Compares the two attributes.
*
* @param att1 The first attribute to compare.
* @param att2 The second attribute to compare.
* @return A value indicating less than, equal, or greater than.
*/
protected int compareAtts(Object att1, Object att2) {
if ((att1 == null) && (att2 == null)) {
return 0;
}
if (att1 == null) {
return -1;
}
if (att2 == null) {
return 1;
}
return ((Comparable) att1).compareTo((Comparable) att2);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy