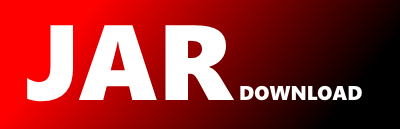
org.geotools.styling.ContrastEnhancementImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gt-main Show documentation
Show all versions of gt-main Show documentation
The main module contains the GeoTools public interfaces that are used by
other GeoTools modules (and GeoTools applications). Where possible we make
use industry standard terms as provided by OGC and ISO standards.
The formal GeoTools public api consists of gt-metadata, jts and the gt-main module.
The main module contains the default implementations that are available provided
to other GeoTools modules using our factory system. Factories are obtained from
an appropriate FactoryFinder, giving applications a chance configure the factory
used using the Factory Hints facilities.
FilterFactory ff = CommonFactoryFinder.getFilterFactory();
Expression expr = ff.add( expression1, expression2 );
If you find yourself using implementation specific classes chances are you doing it wrong:
Expression expr = new AddImpl( expression1, expressiom2 );
The newest version!
/*
* GeoTools - The Open Source Java GIS Toolkit
* http://geotools.org
*
* (C) 2002-2015, Open Source Geospatial Foundation (OSGeo)
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation;
* version 2.1 of the License.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* Created on 13 November 2002, 13:52
*/
package org.geotools.styling;
import java.util.HashMap;
import java.util.Map;
import org.geotools.factory.CommonFactoryFinder;
import org.opengis.filter.FilterFactory;
import org.opengis.filter.FilterFactory2;
import org.opengis.filter.expression.Expression;
import org.opengis.style.ContrastMethod;
/**
* The ContrastEnhancement object defines contrast enhancement for a channel of a false-color image
* or for a color image. Its format is:
*
*
* <xs:element name="ContrastEnhancement">
* <xs:complexType>
* <xs:sequence>
* <xs:choice minOccurs="0">
* <xs:element ref="sld:Normalize"/>
* <xs:element ref="sld:Histogram"/>
* </xs:choice>
* <xs:element ref="sld:GammaValue" minOccurs="0"/>
* </xs:sequence>
* </xs:complexType>
* </xs:element>
* <xs:element name="Normalize">
* <xs:complexType/>
* </xs:element>
* <xs:element name="Histogram">
* <xs:complexType/>
* </xs:element>
* <xs:element name="GammaValue" type="xs:double"/>
*
*
* In the case of a color image, the relative grayscale brightness of a pixel color is used.
* ?Normalize? means to stretch the contrast so that the dimmest color is stretched to black and the
* brightest color is stretched to white, with all colors in between stretched out linearly.
* ?Histogram? means to stretch the contrast based on a histogram of how many colors are at each
* brightness level on input, with the goal of producing equal number of pixels in the image at each
* brightness level on output. This has the effect of revealing many subtle ground features. A
* ?GammaValue? tells how much to brighten (value greater than 1.0) or dim (value less than 1.0) an
* image. The default GammaValue is 1.0 (no change). If none of Normalize, Histogram, or GammaValue
* are selected in a ContrastEnhancement, then no enhancement is performed.
*
* @author iant
*/
public class ContrastEnhancementImpl implements ContrastEnhancement {
@SuppressWarnings("PMD.UnusedPrivateField")
private FilterFactory filterFactory;
private Expression gamma;
private ContrastMethod method;
private Map options;
public ContrastEnhancementImpl() {
this(CommonFactoryFinder.getFilterFactory(null));
}
public ContrastEnhancementImpl(FilterFactory factory) {
this(factory, (ContrastMethod) null);
}
public ContrastEnhancementImpl(FilterFactory factory, ContrastMethod method) {
filterFactory = factory;
this.method = method;
}
public ContrastEnhancementImpl(org.opengis.style.ContrastEnhancement contrastEnhancement) {
filterFactory = CommonFactoryFinder.getFilterFactory2(null);
org.opengis.style.ContrastMethod meth = contrastEnhancement.getMethod();
if (meth != null) {
this.method = ContrastMethod.valueOf(meth.name());
}
this.gamma = contrastEnhancement.getGammaValue();
if (contrastEnhancement instanceof ContrastEnhancement) {
ContrastEnhancement other = (ContrastEnhancement) contrastEnhancement;
if (other.getOptions() != null) {
this.options = new HashMap<>();
this.options.putAll(other.getOptions());
}
}
}
public ContrastEnhancementImpl(
FilterFactory2 factory, Expression gamma, ContrastMethod method) {
this.filterFactory = factory;
this.gamma = gamma;
this.method = method;
}
public void setFilterFactory(FilterFactory factory) {
filterFactory = factory;
}
public Expression getGammaValue() {
return gamma;
}
public void setGammaValue(Expression gamma) {
this.gamma = gamma;
}
public ContrastMethod getMethod() {
return method;
}
public Object accept(org.opengis.style.StyleVisitor visitor, Object extraData) {
return visitor.visit(this, extraData);
}
public void accept(StyleVisitor visitor) {
visitor.visit(this);
}
static ContrastEnhancementImpl cast(org.opengis.style.ContrastEnhancement enhancement) {
if (enhancement == null) {
return null;
} else if (enhancement instanceof ContrastEnhancementImpl) {
return (ContrastEnhancementImpl) enhancement;
} else {
ContrastEnhancementImpl copy = new ContrastEnhancementImpl();
copy.setGammaValue(enhancement.getGammaValue());
copy.setMethod(enhancement.getMethod());
return copy;
}
}
@Override
public void setMethod(ContrastMethod method) {
this.method = method;
}
@Override
public Map getOptions() {
if (this.options == null) {
this.options = new HashMap();
}
return this.options;
}
@Override
public boolean hasOption(String key) {
if (this.options == null) {
this.options = new HashMap();
}
return options.containsKey(key);
}
@Override
public Expression getOption(String key) {
return this.options.get(key);
}
@Override
public void addOption(String key, Expression value) {
if (this.options == null) {
this.options = new HashMap();
}
options.put(key, value);
}
@Override
public void setOptions(Map options) {
this.options = options;
}
@Override
public void setMethod(ContrastMethodStrategy method) {
this.method = method.getMethod();
this.options = method.getOptions();
}
@Override
public int hashCode() {
final int prime = 31;
int result = 1;
result = prime * result + ((gamma == null) ? 0 : gamma.hashCode());
result = prime * result + ((method == null) ? 0 : method.hashCode());
result = prime * result + ((options == null) ? 0 : options.hashCode());
return result;
}
@Override
public boolean equals(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof ContrastEnhancementImpl)) {
return false;
}
ContrastEnhancementImpl other = (ContrastEnhancementImpl) obj;
if (gamma == null) {
if (other.gamma != null) {
return false;
}
} else if (!gamma.equals(other.gamma)) {
return false;
}
if (method == null) {
if (other.method != null) {
return false;
}
} else if (!method.equals(other.method)) {
return false;
}
if (options == null) {
if (other.options != null) {
return false;
}
} else if (!options.equals(other.options)) {
return false;
}
return true;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy