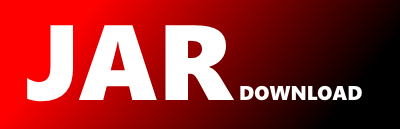
org.geotools.data.DataStoreFactorySpi Maven / Gradle / Ivy
Show all versions of gt-main Show documentation
/*
* GeoTools - The Open Source Java GIS Toolkit
* http://geotools.org
*
* (C) 2008, Open Source Geospatial Foundation (OSGeo)
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation;
* version 2.1 of the License.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*/
package org.geotools.data;
import java.io.IOException;
import java.util.Map;
/**
* Factory used to construct a DataStore from a set of parameters.
*
* The following example shows how a user might connect to a PostGIS database, and maintain the
* resulting DataStore in a Registry:
*
*
*
*
* HashMap params = new HashMap();
* params.put("namespace", "leeds");
* params.put("dbtype", "postgis");
* params.put("host","feathers.leeds.ac.uk");
* params.put("port", "5432");
* params.put("database","postgis_test");
* params.put("user","postgis_ro");
* params.put("passwd","postgis_ro");
*
* DefaultRegistry registry = new DefaultRegistry();
* registry.addDataStore("leeds", params);
*
* DataStore postgis = registry.getDataStore( "leeds" );
* SimpleFeatureSource = postgis.getFeatureSource( "table" );
*
*
* Implementation Notes
*
* An instance of this interface should exist for all data stores which want to take advantage of
* the dynamic plug-in system. In addition to implementing this factory interface each DataStore
* implementation should have a services file:
*
*
META-INF/services/org.geotools.data.DataStoreFactorySpi
*
*
The file should contain a single line which gives the full name of the implementing class.
*
*
example:
* e.g.
* org.geotools.data.mytype.MyTypeDataSourceFacotry
*
*
The factories are never called directly by client code, instead the DataStoreFinder class is
* used.
*
* @author Jody Garnett, Refractions Research
*/
public interface DataStoreFactorySpi extends DataAccessFactory {
/**
* Construct a live data source using the params specifed.
*
*
You can think of this as setting up a connection to the back end data source.
*
*
Magic Params: the following params are magic and are honoured by convention by the
* GeoServer and uDig application.
*
*
* - "user": is taken to be the user name
*
- "passwd": is taken to be the password
*
- "namespace": is taken to be the namespace prefix (and will be kept in sync with
* GeoServer namespace management.
*
*
* When we eventually move over to the use of OpperationalParam we will have to find someway to
* codify this convention.
*
* @param params The full set of information needed to construct a live data store. Typical key
* values for the map include: url - location of a resource, used by file reading
* datasources. dbtype - the type of the database to connect to, e.g. postgis, mysql
* @return The created DataStore, this may be null if the required resource was not found or if
* insufficent parameters were given. Note that canProcess() should have returned false if
* the problem is to do with insuficent parameters.
* @throws IOException if there were any problems setting up (creating or connecting) the
* datasource.
*/
DataStore createDataStore(Map params) throws IOException;
// /**
// * Construct a simple MetadataEntity providing internationlization information
// * for the data source that *would* be created by createDataStore.
// *
// * Suitable for use by CatalogEntry, unknown if this will make
// * a DataStore behind the scenes or not. It is possible it will
// * communicate with the data source though (hense the IOException).
// *
// * @param params The full set of information needed to construct a live
// * data store
// * @return MetadataEntity with descriptive information (including
// * internationlization support).
// */
// DataSourceMetadataEnity createMetadata( Map params ) throws IOException;
DataStore createNewDataStore(Map params) throws IOException;
}