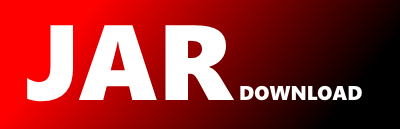
io.outfoxx.sunday.generator.CliktExts.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cli Show documentation
Show all versions of cli Show documentation
Sunday Generator is a code generator for Sunday HTTP clients and JAX-RS server stubs in multiple languages.
/*
* Copyright 2020 Outfox, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.outfoxx.sunday.generator
import com.github.ajalt.clikt.core.BaseCliktCommand
import com.github.ajalt.clikt.core.ParameterHolder
import com.github.ajalt.clikt.parameters.groups.OptionGroup
import com.github.ajalt.clikt.parameters.options.OptionWithValues
import com.github.ajalt.clikt.parameters.options.flag
import com.github.ajalt.clikt.parameters.options.option
import io.outfoxx.sunday.generator.utils.camelCaseToKebabCase
import kotlin.properties.ReadOnlyProperty
import kotlin.reflect.KProperty
typealias EnumOption = OptionWithValues
typealias EnumOptions = Map
class EnumFlagBuilder> {
val flags = mutableMapOf()
infix fun E.to(flag: EnumFlag) = flags.put(this, flag)
fun String.default(default: Boolean = false): EnumFlag = EnumFlag(help = this, default = default)
}
inline fun > ParameterHolder.flags(block: EnumFlagBuilder.() -> Unit): EnumOptions {
val builder = EnumFlagBuilder().apply { block(this) }
return flags(builder.flags)
}
inline fun > ParameterHolder.flags(
vararg entries: Pair,
): EnumOptions = flags(entries.associate { (value, help) -> value to EnumFlag(help = help) })
@JvmName("flagsToHelp")
inline fun > ParameterHolder.flags(
entries: Map,
): EnumOptions {
val enumFlags = entries.mapValues { (_, help) -> EnumFlag(help = help) }
return flags(enumFlags)
}
data class EnumFlag(
var name: String? = null,
var help: String? = null,
var default: Boolean = false,
) {
constructor(help: String) : this(help = help, default = false)
fun name(name: String) = apply { this.name = name }
fun help(help: String) = apply { this.help = help }
fun default(default: Boolean) = apply { this.default = default }
}
inline fun > ParameterHolder.flags(
entries: Map,
): EnumOptions =
entries.mapValues { (value, flag) ->
val enableName = flag.name ?: value.name.camelCaseToKebabCase()
val disableName = "no-$enableName"
val helpDefault = if (flag.default) "enabled" else "disabled"
option("-$enableName", help = flag.help ?: "")
.flag("-$disableName", default = true, defaultForHelp = helpDefault)
}
class EnumFlagsOptionGroup>(
name: String,
help: String? = null,
val options: EnumOptions
) : OptionGroup(name, help) {
init {
options.forEach { (_, option) -> registerOption(option) }
}
}
fun > EnumOptions.grouped(name: String, help: String? = null): EnumFlagsOptionGroup =
EnumFlagsOptionGroup(name, help, this)
operator fun > EnumOptions.provideDelegate(
thisRef: ParameterHolder,
property: KProperty<*>,
): ReadOnlyProperty> {
values.forEach { thisRef.registerOption(it) }
return ReadOnlyProperty { _, _ ->
mapNotNull { (value, option) -> if (option.value) value else null }.toSet()
}
}
operator fun , E : Enum> EnumFlagsOptionGroup.provideDelegate(
thisRef: T,
property: KProperty<*>,
): ReadOnlyProperty> {
thisRef.registerOptionGroup(this)
options.forEach { (_, option) -> thisRef.registerOption(option) }
return ReadOnlyProperty { _, _ ->
options.mapNotNull { (value, option) -> if (option.value) value else null }.toSet()
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy