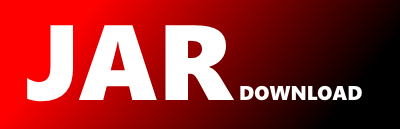
io.pcp.parfait.timing.StepMeasurements Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of parfait-core Show documentation
Show all versions of parfait-core Show documentation
Java performance monitoring framework, including PCP bridge
The newest version!
/*
* Copyright 2009-2017 Aconex
*
* Licensed under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at:
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or
* implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package io.pcp.parfait.timing;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import com.google.common.base.Joiner;
import com.google.common.base.Strings;
public class StepMeasurements {
private final StepMeasurements parent;
private final List children = new ArrayList();
private final List metricInstances = new ArrayList();
private final String eventName;
private final String action;
private volatile boolean started = false;
public StepMeasurements(StepMeasurements parent,
String eventName, String action) {
this.parent = parent;
if (parent != null) {
parent.addChildExecution(this);
}
this.eventName = eventName;
this.action = action;
}
public StepMeasurements getParent() {
return parent;
}
public void addMetricInstance(MetricMeasurement metric) {
metricInstances.add(metric);
}
public void startAll() {
for (MetricMeasurement metric : metricInstances) {
metric.startTimer();
}
started = true;
}
public void stopAll() {
started = false;
for (MetricMeasurement metric : metricInstances) {
metric.stopTimer();
}
}
public void pauseAll() {
for (MetricMeasurement metric : metricInstances) {
metric.pauseOwnTime();
}
}
public void resumeAll() {
for (MetricMeasurement metric : metricInstances) {
metric.resumeOwnTime();
}
}
/**
* @return a nicely-formatted list of all the events taken to reach the one under
* measurement (including that one as the last element)
*/
String getBackTrace() {
if (parent == null) {
return stackTraceElement();
}
return parent.getBackTrace() + "/" + stackTraceElement();
}
/**
* @return a nicely-formatted list of all the events invoked after the one under
* measurement (including that one as the first element)
*/
String getForwardTrace() {
if (children.isEmpty()) {
return stackTraceElement();
} else if (children.size() == 1) {
return stackTraceElement() + "/" + children.get(0).getForwardTrace();
} else {
// Handles the 'freak case' where one event may forward directly to MORE than one
// 'child'. I have no idea if this ever happens, but we might as well handle it.
List childTraces = new ArrayList(children.size());
for (StepMeasurements child : children) {
childTraces.add(child.getForwardTrace());
}
return stackTraceElement() + "/{" + Joiner.on('|').join(childTraces) + "}";
}
}
private void addChildExecution(StepMeasurements newTiming) {
children.add(newTiming);
}
private String stackTraceElement() {
return eventName + (Strings.isNullOrEmpty(action) ? "" : ":" + action);
}
String getEventName() {
return eventName;
}
public Collection getMetricInstances() {
return metricInstances;
}
public Map snapshotValues() {
if (!started) {
return Collections.emptyMap();
}
Collection snapshot = new ArrayList(metricInstances);
Map results = new HashMap();
for (MetricMeasurement measurement : snapshot) {
results.put(measurement.getMetricSource(), measurement.inProgressValue());
}
return results;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy