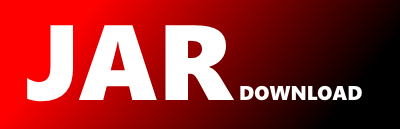
io.pcp.parfait.dropwizard.metricadapters.SamplingAdapter Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of parfait-dropwizard Show documentation
Show all versions of parfait-dropwizard Show documentation
Reporter for Dropwizard (ex Codahale) metrics that publishes to Parfait
package io.pcp.parfait.dropwizard.metricadapters;
import static com.codahale.metrics.MetricRegistry.name;
import static tec.uom.se.AbstractUnit.ONE;
import javax.measure.Unit;
import java.util.Set;
import com.codahale.metrics.Sampling;
import com.codahale.metrics.Snapshot;
import io.pcp.parfait.Monitorable;
import io.pcp.parfait.ValueSemantics;
import io.pcp.parfait.dropwizard.MetricAdapter;
import io.pcp.parfait.dropwizard.NonSelfRegisteringSettableValue;
import com.google.common.collect.Sets;
public class SamplingAdapter implements MetricAdapter {
private final Sampling samplingMetric;
private final NonSelfRegisteringSettableValue mean;
private final NonSelfRegisteringSettableValue max;
private final NonSelfRegisteringSettableValue median;
private final NonSelfRegisteringSettableValue min;
private final NonSelfRegisteringSettableValue stddev;
private final NonSelfRegisteringSettableValue seventyFifthPercentile;
private final NonSelfRegisteringSettableValue ninetyFifthPercentile;
private final NonSelfRegisteringSettableValue ninetyEighthPercentile;
private final NonSelfRegisteringSettableValue ninetyNinthPercentile;
private final NonSelfRegisteringSettableValue threeNinesPercentile;
public SamplingAdapter(Sampling samplingMetric, String name, String description, Unit> unit) {
this.samplingMetric = samplingMetric;
Snapshot snapshot = samplingMetric.getSnapshot();
this.mean = new NonSelfRegisteringSettableValue<>(name(name, "mean"), description + " - Mean", unit, snapshot.getMean(), ValueSemantics.FREE_RUNNING);
this.seventyFifthPercentile = new NonSelfRegisteringSettableValue<>(name(name, "seventyfifth"), description + " - 75th Percentile of recent data", unit, snapshot.get75thPercentile(), ValueSemantics.FREE_RUNNING);
this.ninetyFifthPercentile = new NonSelfRegisteringSettableValue<>(name(name, "ninetyfifth"), description + " - 95th Percentile of recent data", unit, snapshot.get95thPercentile(), ValueSemantics.FREE_RUNNING);
this.ninetyEighthPercentile = new NonSelfRegisteringSettableValue<>(name(name, "ninetyeighth"), description + " - 98th Percentile of recent data", unit, snapshot.get98thPercentile(), ValueSemantics.FREE_RUNNING);
this.ninetyNinthPercentile = new NonSelfRegisteringSettableValue<>(name(name, "ninetynineth"), description + " - 99th Percentile of recent data", unit, snapshot.get99thPercentile(), ValueSemantics.FREE_RUNNING);
this.threeNinesPercentile = new NonSelfRegisteringSettableValue<>(name(name, "threenines"), description + " - 99.9th Percentile of recent data", unit, snapshot.get999thPercentile(), ValueSemantics.FREE_RUNNING);
this.median = new NonSelfRegisteringSettableValue<>(name(name, "median"), description + " - Median", unit, snapshot.getMedian(), ValueSemantics.FREE_RUNNING);
this.max = new NonSelfRegisteringSettableValue<>(name(name, "max"), description + " - Maximum", unit, snapshot.getMax(), ValueSemantics.MONOTONICALLY_INCREASING);
this.min = new NonSelfRegisteringSettableValue<>(name(name, "min"), description + " - Minimum", unit, snapshot.getMin(), ValueSemantics.FREE_RUNNING);
this.stddev = new NonSelfRegisteringSettableValue<>(name(name, "stddev"), description + " - Standard Deviation", unit, snapshot.getStdDev(), ValueSemantics.FREE_RUNNING);
}
public SamplingAdapter(Sampling samplingMetric, String name, String description) {
this(samplingMetric, name, description, ONE);
}
@Override
public Set getMonitorables() {
return Sets.newHashSet(mean, median, max, min, stddev, seventyFifthPercentile, ninetyFifthPercentile, ninetyEighthPercentile, ninetyNinthPercentile, threeNinesPercentile);
}
@Override
public void updateMonitorables() {
Snapshot snapshot = samplingMetric.getSnapshot();
mean.set(snapshot.getMean());
median.set(snapshot.getMedian());
max.set(snapshot.getMax());
min.set(snapshot.getMin());
stddev.set(snapshot.getStdDev());
seventyFifthPercentile.set(snapshot.get75thPercentile());
ninetyFifthPercentile.set(snapshot.get95thPercentile());
ninetyEighthPercentile.set(snapshot.get98thPercentile());
ninetyNinthPercentile.set(snapshot.get99thPercentile());
threeNinesPercentile.set(snapshot.get999thPercentile());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy