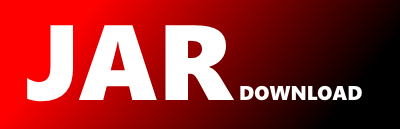
io.pdef.DataTypes Maven / Gradle / Ivy
/*
* Copyright: 2013 Pdef
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at:
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.pdef;
import io.pdef.descriptors.DataTypeDescriptor;
import java.util.*;
/**
* Pdef data types utility methods.
*/
public class DataTypes {
private DataTypes() {}
/**
* Returns a deep copy of a pdef data type (a primitive, a collection, or a message).
*/
public static T copy(final T object) {
if (object == null) return null;
TypeEnum type = TypeEnum.dataTypeOf(object.getClass());
return copy(object, type);
}
/**
* Returns a deep copy of a pdef message.
*/
@SuppressWarnings("unchecked")
public static T copy(final T message) {
return message == null ? null : (T) message.copy();
}
/**
* Returns a deep copy of a pdef list.
*/
public static List copy(final List list) {
if (list == null) {
return null;
}
TypeEnum elementType = null;
List copy = new ArrayList();
for (T element : list) {
T elementCopy = null;
if (element != null) {
if (elementType == null) {
elementType = TypeEnum.dataTypeOf(element.getClass());
}
elementCopy = copy(element, elementType);
}
copy.add(elementCopy);
}
return copy;
}
/**
* Returns a deep copy of a pdef set.
*/
public static Set copy(final Set set) {
if (set == null) {
return null;
}
TypeEnum elementType = null;
Set copy = new HashSet();
for (T element : set) {
T elementCopy = null;
if (element != null) {
if (elementType == null) {
elementType = TypeEnum.dataTypeOf(element.getClass());
}
elementCopy = copy(element, elementType);
}
copy.add(elementCopy);
}
return copy;
}
/**
* Returns a deep copy of a pdef map.
*/
public static Map copy(final Map map) {
if (map == null) {
return null;
}
TypeEnum keyType = null;
TypeEnum valueType = null;
Map copy = new HashMap();
for (Map.Entry entry : map.entrySet()) {
K key = entry.getKey();
V value = entry.getValue();
K keyCopy = null;
if (key != null) {
if (keyType == null) {
keyType = TypeEnum.dataTypeOf(key.getClass());
}
keyCopy = copy(key, keyType);
}
V valueCopy = null;
if (value != null) {
if (valueType == null) {
valueType = TypeEnum.dataTypeOf(value.getClass());
}
valueCopy = copy(value, valueType);
}
copy.put(keyCopy, valueCopy);
}
return copy;
}
/**
* Returns a deep copy of an object.
*/
public static T copy(final T object, final DataTypeDescriptor descriptor) {
if (descriptor == null) {
throw new NullPointerException("descriptor");
}
return copy(object, descriptor.getType());
}
@SuppressWarnings("unchecked")
private static T copy(final T object, final TypeEnum type) {
if (type == null) {
throw new NullPointerException("type");
}
if (object == null) {
return null;
}
switch (type) {
case LIST: return (T) copy((List>) object);
case SET: return (T) copy((Set>) object);
case MAP: return (T) copy((Map, ?>) object);
case MESSAGE: return (T) ((Message) object).copy();
default: return object;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy