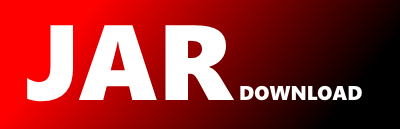
io.pdef.descriptors.InterfaceDescriptor Maven / Gradle / Ivy
/*
* Copyright: 2013 Pdef
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at:
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.pdef.descriptors;
import io.pdef.Message;
import io.pdef.TypeEnum;
import javax.annotation.Nullable;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
public class InterfaceDescriptor extends Descriptor {
private final List> methods;
private final Map> methodMap;
private final MessageDescriptor extends Message> exc;
private InterfaceDescriptor(final Builder builder) {
super(TypeEnum.INTERFACE, builder.javaClass);
exc = builder.exc;
methods = ImmutableCollections.list(builder.methods);
methodMap = ImmutableCollections.map(methodsToMap(methods));
}
public static Builder builder() {
return new Builder();
}
@Override
public String toString() {
return "InterfaceDescriptor{" + getJavaClass().getSimpleName() + '}';
}
/** Returns a list of method descriptors or an empty list. */
public List> getMethods() {
return methods;
}
/** Returns an exception descriptor or {@literal null}. */
@Nullable
public MessageDescriptor extends Message> getExc() {
return exc;
}
/** Returns a method descriptor by its name and returns it or {@literal null}. */
@Nullable
public MethodDescriptor getMethod(final String name) {
return methodMap.get(name);
}
public static class Builder {
private Class javaClass;
private MessageDescriptor extends Message> exc;
private List> methods;
public Builder() {
methods = new ArrayList>();
}
public Builder setJavaClass(final Class javaClass) {
this.javaClass = javaClass;
return this;
}
public Builder setExc(final MessageDescriptor extends Message> exc) {
this.exc = exc;
return this;
}
public Builder addMethod(final MethodDescriptor method) {
this.methods.add(method);
return this;
}
public InterfaceDescriptor build() {
return new InterfaceDescriptor(this);
}
}
private static Map> methodsToMap(
final List> methods) {
Map> map = new HashMap>();
for (MethodDescriptor method : methods) {
map.put(method.getName(), method);
}
return map;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy