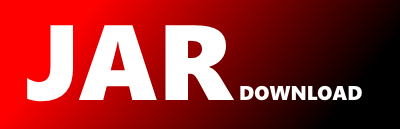
io.pdef.descriptors.MethodDescriptor Maven / Gradle / Ivy
/*
* Copyright: 2013 Pdef
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at:
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.pdef.descriptors;
import io.pdef.Provider;
import io.pdef.Providers;
import io.pdef.TypeEnum;
import java.util.ArrayList;
import java.util.List;
/** MethodDescriptor holds a method name, result, arguments, exception, flags, and its invoker. */
public class MethodDescriptor implements MethodInvoker {
private final String name;
private final MethodInvoker invoker;
private final Provider> resultProvider;
private final List> args;
private final boolean post;
private Descriptor result;
private MethodDescriptor(final Builder builder) {
if (builder.name == null) throw new NullPointerException("name");
if (builder.result == null) throw new NullPointerException("result");
if (builder.invoker == null) throw new NullPointerException("invoker");
name = builder.name;
invoker = builder.invoker;
resultProvider = builder.result;
args = ImmutableCollections.list(builder.args);
post = builder.post;
}
public static Builder builder() {
return new Builder();
}
@Override
public String toString() {
return "MethodDescriptor{" + name + '}';
}
/** Returns a pdef method name. */
public String getName() {
return name;
}
/**
* Returns this method result descriptor.
*
* It can be a {@link DataTypeDescriptor} if this method is terminal or {@link
* io.pdef.descriptors.InterfaceDescriptor} otherwise.
*/
public Descriptor getResult() {
return result != null ? result : (result = resultProvider.get());
}
/** Returns a list of argument descriptors or an empty list. */
public List> getArgs() {
return args;
}
/** Returns whether this method is a post method (annotated with @post annotation). */
public boolean isPost() {
return post;
}
/** Returns whether this method returns a value type or void (not an interface). */
public boolean isTerminal() {
TypeEnum type = getResult().getType();
return type != TypeEnum.INTERFACE;
}
@Override
public R invoke(final T object, final Object[] args) throws Exception {
return invoker.invoke(object, args);
}
public static class Builder {
private String name;
private Provider> result;
private List> args;
private MethodInvoker invoker;
private boolean post;
public Builder() {
args = new ArrayList>();
}
public Builder setName(final String name) {
this.name = name;
return this;
}
public Builder setResult(final Descriptor result) {
if (result == null) throw new NullPointerException("result");
return setResult(Providers.>ofInstance(result));
}
public Builder setInterfaceResult(final Class interfaceClass) {
return setResult(new Provider>() {
@Override
public Descriptor get() {
return Descriptors.findInterfaceDescriptor(interfaceClass);
}
});
}
public Builder setResult(final Provider> result) {
this.result = result;
return this;
}
public Builder addArg(final String name, final DataTypeDescriptor type,
final boolean isQuery, final boolean isPost) {
this.args.add(new ArgumentDescriptor(name, type, isQuery, isPost));
return this;
}
public Builder setInvoker(final MethodInvoker invoker) {
this.invoker = invoker;
return this;
}
public Builder setReflexiveInvoker(final Class interfaceClass) {
this.invoker = MethodInvokers.reflexive(interfaceClass, name);
return this;
}
public Builder setPost(final boolean post) {
this.post = post;
return this;
}
public MethodDescriptor build() {
return new MethodDescriptor(this);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy