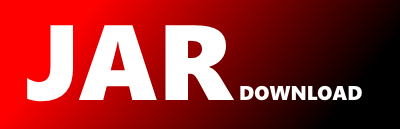
io.pdef.descriptors.Descriptors Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pdef Show documentation
Show all versions of pdef Show documentation
Pdef java descriptors, formats and RPC
/*
* Copyright: 2013 Pdef
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at:
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.pdef.descriptors;
import io.pdef.TypeEnum;
import javax.annotation.Nullable;
import java.lang.reflect.Field;
import java.util.Date;
/** Primitive and collection descriptors. */
public class Descriptors {
public static DataTypeDescriptor bool = primitive(TypeEnum.BOOL, Boolean.class, false);
public static DataTypeDescriptor int16 = primitive(TypeEnum.INT16, Short.class, (short) 0);
public static DataTypeDescriptor int32 = primitive(TypeEnum.INT32, Integer.class, 0);
public static DataTypeDescriptor int64 = primitive(TypeEnum.INT64, Long.class, 0L);
public static DataTypeDescriptor float0 = primitive(TypeEnum.FLOAT, Float.class, 0f);
public static DataTypeDescriptor double0 = primitive(TypeEnum.DOUBLE, Double.class, 0d);
public static DataTypeDescriptor string = primitive(TypeEnum.STRING, String.class, "");
public static DataTypeDescriptor datetime = primitive(TypeEnum.DATETIME, Date.class, null);
public static DataTypeDescriptor void0 = primitive(TypeEnum.VOID, Void.class, null);
private Descriptors() {}
public static ListDescriptor list(final DataTypeDescriptor element) {
return new ListDescriptor(element);
}
public static SetDescriptor set(final DataTypeDescriptor element) {
return new SetDescriptor(element);
}
public static MapDescriptor map(final DataTypeDescriptor key,
final DataTypeDescriptor value) {
return new MapDescriptor(key, value);
}
private static PrimitiveDescriptor primitive(final TypeEnum type, final Class cls,
final T defaultValue) {
return new PrimitiveDescriptor(type, cls, defaultValue);
}
/** Returns an interface descriptor or throws an IllegalArgumentException. */
@Nullable
public static InterfaceDescriptor findInterfaceDescriptor(final Class cls) {
if (!cls.isInterface()) {
throw new IllegalArgumentException("Interface required, got " + cls);
}
Field field;
try {
field = cls.getField("DESCRIPTOR");
} catch (NoSuchFieldException e) {
throw new IllegalArgumentException("No DESCRIPTOR field in " + cls);
}
if (!InterfaceDescriptor.class.isAssignableFrom(field.getType())) {
throw new IllegalArgumentException("Not an InterfaceDescriptor field, " + field);
}
try {
// Get the static TYPE field.
@SuppressWarnings("unchecked")
InterfaceDescriptor descriptor = (InterfaceDescriptor) field.get(null);
return descriptor;
} catch (IllegalAccessException e) {
throw new RuntimeException(e);
}
}
private static class PrimitiveDescriptor extends DataTypeDescriptor {
private final T defaultValue;
private PrimitiveDescriptor(final TypeEnum type, final Class javaClass,
final T defaultValue) {
super(type, javaClass);
this.defaultValue = defaultValue;
}
@Override
public T getDefault() {
return defaultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy