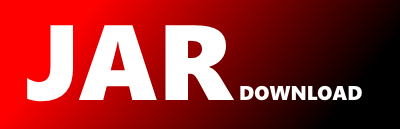
io.pelle.mango.dsl.ui.contentassist.antlr.internal.InternalMangoParser Maven / Gradle / Ivy
package io.pelle.mango.dsl.ui.contentassist.antlr.internal;
import java.io.InputStream;
import org.eclipse.xtext.*;
import org.eclipse.xtext.parser.*;
import org.eclipse.xtext.parser.impl.*;
import org.eclipse.emf.ecore.util.EcoreUtil;
import org.eclipse.emf.ecore.EObject;
import org.eclipse.xtext.parser.antlr.XtextTokenStream;
import org.eclipse.xtext.parser.antlr.XtextTokenStream.HiddenTokens;
import org.eclipse.xtext.ui.editor.contentassist.antlr.internal.AbstractInternalContentAssistParser;
import org.eclipse.xtext.ui.editor.contentassist.antlr.internal.DFA;
import io.pelle.mango.dsl.services.MangoGrammarAccess;
import org.antlr.runtime.*;
import java.util.Stack;
import java.util.List;
import java.util.ArrayList;
import java.util.Map;
import java.util.HashMap;
@SuppressWarnings("all")
interface InternalMangoParserSignatures {
void entryRuleModelRoot() throws RecognitionException;
void ruleModelRoot() throws RecognitionException;
void entryRuleModel() throws RecognitionException;
void ruleModel() throws RecognitionException;
void entryRulePackageDeclaration() throws RecognitionException;
void rulePackageDeclaration() throws RecognitionException;
void entryRuleAbstractElement() throws RecognitionException;
void ruleAbstractElement() throws RecognitionException;
void entryRuleEnumeration() throws RecognitionException;
void ruleEnumeration() throws RecognitionException;
void entryRuleEnumerationValue() throws RecognitionException;
void ruleEnumerationValue() throws RecognitionException;
void entryRuleEntityOptions() throws RecognitionException;
void ruleEntityOptions() throws RecognitionException;
void entryRuleEntityNaturalKeyFields() throws RecognitionException;
void ruleEntityNaturalKeyFields() throws RecognitionException;
void entryRuleEntityHierarchical() throws RecognitionException;
void ruleEntityHierarchical() throws RecognitionException;
void entryRuleEntityDisableIdField() throws RecognitionException;
void ruleEntityDisableIdField() throws RecognitionException;
void entryRuleEntityLabelField() throws RecognitionException;
void ruleEntityLabelField() throws RecognitionException;
void entryRuleEntityPluralLabelField() throws RecognitionException;
void ruleEntityPluralLabelField() throws RecognitionException;
void entryRuleEntityOptionsContainer() throws RecognitionException;
void ruleEntityOptionsContainer() throws RecognitionException;
void entryRuleEntity() throws RecognitionException;
void ruleEntity() throws RecognitionException;
void entryRuleValueObject() throws RecognitionException;
void ruleValueObject() throws RecognitionException;
void entryRuleDatatype() throws RecognitionException;
void ruleDatatype() throws RecognitionException;
void entryRuleSimpleDataType() throws RecognitionException;
void ruleSimpleDataType() throws RecognitionException;
void entryRuleEntityAttribute() throws RecognitionException;
void ruleEntityAttribute() throws RecognitionException;
void entryRuleSimpleDatatypeEntityAttribute() throws RecognitionException;
void ruleSimpleDatatypeEntityAttribute() throws RecognitionException;
void entryRuleBaseDataType() throws RecognitionException;
void ruleBaseDataType() throws RecognitionException;
void entryRuleBaseDataTypeProperties() throws RecognitionException;
void ruleBaseDataTypeProperties() throws RecognitionException;
void entryRuleBaseDataTypeWidth() throws RecognitionException;
void ruleBaseDataTypeWidth() throws RecognitionException;
void entryRuleBaseDataTypeLabel() throws RecognitionException;
void ruleBaseDataTypeLabel() throws RecognitionException;
void entryRuleStringDataType() throws RecognitionException;
void ruleStringDataType() throws RecognitionException;
void entryRuleStringEntityAttribute() throws RecognitionException;
void ruleStringEntityAttribute() throws RecognitionException;
void entryRuleMapEntityAttribute() throws RecognitionException;
void ruleMapEntityAttribute() throws RecognitionException;
void entryRuleBooleanDataType() throws RecognitionException;
void ruleBooleanDataType() throws RecognitionException;
void entryRuleBooleanEntityAttribute() throws RecognitionException;
void ruleBooleanEntityAttribute() throws RecognitionException;
void entryRuleIntegerDataType() throws RecognitionException;
void ruleIntegerDataType() throws RecognitionException;
void entryRuleIntegerEntityAttribute() throws RecognitionException;
void ruleIntegerEntityAttribute() throws RecognitionException;
void entryRuleDateDataType() throws RecognitionException;
void ruleDateDataType() throws RecognitionException;
void entryRuleDateEntityAttribute() throws RecognitionException;
void ruleDateEntityAttribute() throws RecognitionException;
void entryRuleDecimalDataType() throws RecognitionException;
void ruleDecimalDataType() throws RecognitionException;
void entryRuleDecimalEntityAttribute() throws RecognitionException;
void ruleDecimalEntityAttribute() throws RecognitionException;
void entryRuleLongDataType() throws RecognitionException;
void ruleLongDataType() throws RecognitionException;
void entryRuleLongEntityAttribute() throws RecognitionException;
void ruleLongEntityAttribute() throws RecognitionException;
void entryRuleFloatDataType() throws RecognitionException;
void ruleFloatDataType() throws RecognitionException;
void entryRuleFloatEntityAttribute() throws RecognitionException;
void ruleFloatEntityAttribute() throws RecognitionException;
void entryRuleDoubleDataType() throws RecognitionException;
void ruleDoubleDataType() throws RecognitionException;
void entryRuleDoubleEntityAttribute() throws RecognitionException;
void ruleDoubleEntityAttribute() throws RecognitionException;
void entryRuleBinaryDataType() throws RecognitionException;
void ruleBinaryDataType() throws RecognitionException;
void entryRuleBinaryEntityAttribute() throws RecognitionException;
void ruleBinaryEntityAttribute() throws RecognitionException;
void entryRuleEntityDataType() throws RecognitionException;
void ruleEntityDataType() throws RecognitionException;
void entryRuleEntityEntityAttribute() throws RecognitionException;
void ruleEntityEntityAttribute() throws RecognitionException;
void entryRuleValueObjectEntityAttribute() throws RecognitionException;
void ruleValueObjectEntityAttribute() throws RecognitionException;
void entryRuleEnumerationDataType() throws RecognitionException;
void ruleEnumerationDataType() throws RecognitionException;
void entryRuleEnumerationEntityAttribute() throws RecognitionException;
void ruleEnumerationEntityAttribute() throws RecognitionException;
void entryRuleDictionary() throws RecognitionException;
void ruleDictionary() throws RecognitionException;
void entryRuleDictionarySearch() throws RecognitionException;
void ruleDictionarySearch() throws RecognitionException;
void entryRuleDictionaryEditor() throws RecognitionException;
void ruleDictionaryEditor() throws RecognitionException;
void entryRuleDictionaryFilter() throws RecognitionException;
void ruleDictionaryFilter() throws RecognitionException;
void entryRuleDictionaryResult() throws RecognitionException;
void ruleDictionaryResult() throws RecognitionException;
void entryRuleColumnLayout() throws RecognitionException;
void ruleColumnLayout() throws RecognitionException;
void entryRuleColumnLayoutData() throws RecognitionException;
void ruleColumnLayoutData() throws RecognitionException;
void entryRuleDictionaryContainer() throws RecognitionException;
void ruleDictionaryContainer() throws RecognitionException;
void entryRuleDictionaryComposite() throws RecognitionException;
void ruleDictionaryComposite() throws RecognitionException;
void entryRuleDictionaryEditableTable() throws RecognitionException;
void ruleDictionaryEditableTable() throws RecognitionException;
void entryRuleDictionaryAssignmentTable() throws RecognitionException;
void ruleDictionaryAssignmentTable() throws RecognitionException;
void entryRuleDictionaryContainerContent() throws RecognitionException;
void ruleDictionaryContainerContent() throws RecognitionException;
void entryRuleLabels() throws RecognitionException;
void ruleLabels() throws RecognitionException;
void entryRuleBaseDictionaryControl() throws RecognitionException;
void ruleBaseDictionaryControl() throws RecognitionException;
void entryRuleDictionaryControl() throws RecognitionException;
void ruleDictionaryControl() throws RecognitionException;
void entryRuleDictionaryControlGroupOptions() throws RecognitionException;
void ruleDictionaryControlGroupOptions() throws RecognitionException;
void entryRuleDictionaryControlGroupOptionMultiFilterField() throws RecognitionException;
void ruleDictionaryControlGroupOptionMultiFilterField() throws RecognitionException;
void entryRuleDictionaryControlGroupOptionsContainer() throws RecognitionException;
void ruleDictionaryControlGroupOptionsContainer() throws RecognitionException;
void entryRuleDictionaryControlGroup() throws RecognitionException;
void ruleDictionaryControlGroup() throws RecognitionException;
void entryRuleDictionaryHierarchicalControl() throws RecognitionException;
void ruleDictionaryHierarchicalControl() throws RecognitionException;
void entryRuleDictionaryTextControl() throws RecognitionException;
void ruleDictionaryTextControl() throws RecognitionException;
void entryRuleDictionaryIntegerControlInputType() throws RecognitionException;
void ruleDictionaryIntegerControlInputType() throws RecognitionException;
void entryRuleDictionaryIntegerControlOptions() throws RecognitionException;
void ruleDictionaryIntegerControlOptions() throws RecognitionException;
void entryRuleDictionaryIntegerControl() throws RecognitionException;
void ruleDictionaryIntegerControl() throws RecognitionException;
void entryRuleDictionaryBigDecimalControl() throws RecognitionException;
void ruleDictionaryBigDecimalControl() throws RecognitionException;
void entryRuleDictionaryBooleanControl() throws RecognitionException;
void ruleDictionaryBooleanControl() throws RecognitionException;
void entryRuleDictionaryDateControl() throws RecognitionException;
void ruleDictionaryDateControl() throws RecognitionException;
void entryRuleDictionaryEnumerationControl() throws RecognitionException;
void ruleDictionaryEnumerationControl() throws RecognitionException;
void entryRuleDictionaryReferenceControl() throws RecognitionException;
void ruleDictionaryReferenceControl() throws RecognitionException;
void entryRuleDictionaryFileControl() throws RecognitionException;
void ruleDictionaryFileControl() throws RecognitionException;
void entryRuleModule() throws RecognitionException;
void ruleModule() throws RecognitionException;
void entryRuleModuleParameter() throws RecognitionException;
void ruleModuleParameter() throws RecognitionException;
void entryRuleModuleDefinition() throws RecognitionException;
void ruleModuleDefinition() throws RecognitionException;
void entryRuleModuleDefinitionParameter() throws RecognitionException;
void ruleModuleDefinitionParameter() throws RecognitionException;
void entryRuleServiceOptions() throws RecognitionException;
void ruleServiceOptions() throws RecognitionException;
void entryRuleServiceMethod() throws RecognitionException;
void ruleServiceMethod() throws RecognitionException;
void entryRuleService() throws RecognitionException;
void ruleService() throws RecognitionException;
void entryRuleNavigationNode() throws RecognitionException;
void ruleNavigationNode() throws RecognitionException;
void entryRuleXExpression() throws RecognitionException;
void ruleXExpression() throws RecognitionException;
void entryRuleXAssignment() throws RecognitionException;
void ruleXAssignment() throws RecognitionException;
void entryRuleOpSingleAssign() throws RecognitionException;
void ruleOpSingleAssign() throws RecognitionException;
void entryRuleOpMultiAssign() throws RecognitionException;
void ruleOpMultiAssign() throws RecognitionException;
void entryRuleXOrExpression() throws RecognitionException;
void ruleXOrExpression() throws RecognitionException;
void entryRuleOpOr() throws RecognitionException;
void ruleOpOr() throws RecognitionException;
void entryRuleXAndExpression() throws RecognitionException;
void ruleXAndExpression() throws RecognitionException;
void entryRuleOpAnd() throws RecognitionException;
void ruleOpAnd() throws RecognitionException;
void entryRuleXEqualityExpression() throws RecognitionException;
void ruleXEqualityExpression() throws RecognitionException;
void entryRuleOpEquality() throws RecognitionException;
void ruleOpEquality() throws RecognitionException;
void entryRuleXRelationalExpression() throws RecognitionException;
void ruleXRelationalExpression() throws RecognitionException;
void entryRuleOpCompare() throws RecognitionException;
void ruleOpCompare() throws RecognitionException;
void entryRuleXOtherOperatorExpression() throws RecognitionException;
void ruleXOtherOperatorExpression() throws RecognitionException;
void entryRuleOpOther() throws RecognitionException;
void ruleOpOther() throws RecognitionException;
void entryRuleXAdditiveExpression() throws RecognitionException;
void ruleXAdditiveExpression() throws RecognitionException;
void entryRuleOpAdd() throws RecognitionException;
void ruleOpAdd() throws RecognitionException;
void entryRuleXMultiplicativeExpression() throws RecognitionException;
void ruleXMultiplicativeExpression() throws RecognitionException;
void entryRuleOpMulti() throws RecognitionException;
void ruleOpMulti() throws RecognitionException;
void entryRuleXUnaryOperation() throws RecognitionException;
void ruleXUnaryOperation() throws RecognitionException;
void entryRuleOpUnary() throws RecognitionException;
void ruleOpUnary() throws RecognitionException;
void entryRuleXCastedExpression() throws RecognitionException;
void ruleXCastedExpression() throws RecognitionException;
void entryRuleXPostfixOperation() throws RecognitionException;
void ruleXPostfixOperation() throws RecognitionException;
void entryRuleOpPostfix() throws RecognitionException;
void ruleOpPostfix() throws RecognitionException;
void entryRuleXMemberFeatureCall() throws RecognitionException;
void ruleXMemberFeatureCall() throws RecognitionException;
void entryRuleXPrimaryExpression() throws RecognitionException;
void ruleXPrimaryExpression() throws RecognitionException;
void entryRuleXLiteral() throws RecognitionException;
void ruleXLiteral() throws RecognitionException;
void entryRuleXCollectionLiteral() throws RecognitionException;
void ruleXCollectionLiteral() throws RecognitionException;
void entryRuleXSetLiteral() throws RecognitionException;
void ruleXSetLiteral() throws RecognitionException;
void entryRuleXListLiteral() throws RecognitionException;
void ruleXListLiteral() throws RecognitionException;
void entryRuleXClosure() throws RecognitionException;
void ruleXClosure() throws RecognitionException;
void entryRuleXExpressionInClosure() throws RecognitionException;
void ruleXExpressionInClosure() throws RecognitionException;
void entryRuleXShortClosure() throws RecognitionException;
void ruleXShortClosure() throws RecognitionException;
void entryRuleXParenthesizedExpression() throws RecognitionException;
void ruleXParenthesizedExpression() throws RecognitionException;
void entryRuleXIfExpression() throws RecognitionException;
void ruleXIfExpression() throws RecognitionException;
void entryRuleXSwitchExpression() throws RecognitionException;
void ruleXSwitchExpression() throws RecognitionException;
void entryRuleXCasePart() throws RecognitionException;
void ruleXCasePart() throws RecognitionException;
void entryRuleXForLoopExpression() throws RecognitionException;
void ruleXForLoopExpression() throws RecognitionException;
void entryRuleXBasicForLoopExpression() throws RecognitionException;
void ruleXBasicForLoopExpression() throws RecognitionException;
void entryRuleXWhileExpression() throws RecognitionException;
void ruleXWhileExpression() throws RecognitionException;
void entryRuleXDoWhileExpression() throws RecognitionException;
void ruleXDoWhileExpression() throws RecognitionException;
void entryRuleXBlockExpression() throws RecognitionException;
void ruleXBlockExpression() throws RecognitionException;
void entryRuleXExpressionOrVarDeclaration() throws RecognitionException;
void ruleXExpressionOrVarDeclaration() throws RecognitionException;
void entryRuleXVariableDeclaration() throws RecognitionException;
void ruleXVariableDeclaration() throws RecognitionException;
void entryRuleJvmFormalParameter() throws RecognitionException;
void ruleJvmFormalParameter() throws RecognitionException;
void entryRuleFullJvmFormalParameter() throws RecognitionException;
void ruleFullJvmFormalParameter() throws RecognitionException;
void entryRuleXFeatureCall() throws RecognitionException;
void ruleXFeatureCall() throws RecognitionException;
void entryRuleFeatureCallID() throws RecognitionException;
void ruleFeatureCallID() throws RecognitionException;
void entryRuleIdOrSuper() throws RecognitionException;
void ruleIdOrSuper() throws RecognitionException;
void entryRuleXConstructorCall() throws RecognitionException;
void ruleXConstructorCall() throws RecognitionException;
void entryRuleXBooleanLiteral() throws RecognitionException;
void ruleXBooleanLiteral() throws RecognitionException;
void entryRuleXNullLiteral() throws RecognitionException;
void ruleXNullLiteral() throws RecognitionException;
void entryRuleXNumberLiteral() throws RecognitionException;
void ruleXNumberLiteral() throws RecognitionException;
void entryRuleXStringLiteral() throws RecognitionException;
void ruleXStringLiteral() throws RecognitionException;
void entryRuleXTypeLiteral() throws RecognitionException;
void ruleXTypeLiteral() throws RecognitionException;
void entryRuleXThrowExpression() throws RecognitionException;
void ruleXThrowExpression() throws RecognitionException;
void entryRuleXReturnExpression() throws RecognitionException;
void ruleXReturnExpression() throws RecognitionException;
void entryRuleXTryCatchFinallyExpression() throws RecognitionException;
void ruleXTryCatchFinallyExpression() throws RecognitionException;
void entryRuleXSynchronizedExpression() throws RecognitionException;
void ruleXSynchronizedExpression() throws RecognitionException;
void entryRuleXCatchClause() throws RecognitionException;
void ruleXCatchClause() throws RecognitionException;
void entryRuleQualifiedName() throws RecognitionException;
void ruleQualifiedName() throws RecognitionException;
void entryRuleNumber() throws RecognitionException;
void ruleNumber() throws RecognitionException;
void entryRuleJvmTypeReference() throws RecognitionException;
void ruleJvmTypeReference() throws RecognitionException;
void entryRuleArrayBrackets() throws RecognitionException;
void ruleArrayBrackets() throws RecognitionException;
void entryRuleXFunctionTypeRef() throws RecognitionException;
void ruleXFunctionTypeRef() throws RecognitionException;
void entryRuleJvmParameterizedTypeReference() throws RecognitionException;
void ruleJvmParameterizedTypeReference() throws RecognitionException;
void entryRuleJvmArgumentTypeReference() throws RecognitionException;
void ruleJvmArgumentTypeReference() throws RecognitionException;
void entryRuleJvmWildcardTypeReference() throws RecognitionException;
void ruleJvmWildcardTypeReference() throws RecognitionException;
void entryRuleJvmUpperBound() throws RecognitionException;
void ruleJvmUpperBound() throws RecognitionException;
void entryRuleJvmUpperBoundAnded() throws RecognitionException;
void ruleJvmUpperBoundAnded() throws RecognitionException;
void entryRuleJvmLowerBound() throws RecognitionException;
void ruleJvmLowerBound() throws RecognitionException;
void entryRuleJvmLowerBoundAnded() throws RecognitionException;
void ruleJvmLowerBoundAnded() throws RecognitionException;
void entryRuleJvmTypeParameter() throws RecognitionException;
void ruleJvmTypeParameter() throws RecognitionException;
void entryRuleQualifiedNameWithWildcard() throws RecognitionException;
void ruleQualifiedNameWithWildcard() throws RecognitionException;
void entryRuleValidID() throws RecognitionException;
void ruleValidID() throws RecognitionException;
void entryRuleXImportSection() throws RecognitionException;
void ruleXImportSection() throws RecognitionException;
void entryRuleXImportDeclaration() throws RecognitionException;
void ruleXImportDeclaration() throws RecognitionException;
void entryRuleQualifiedNameInStaticImport() throws RecognitionException;
void ruleQualifiedNameInStaticImport() throws RecognitionException;
void ruleIdGeneratorStrategy() throws RecognitionException;
void ruleCardinality() throws RecognitionException;
void ruleSimpleTypes() throws RecognitionException;
void ruleIntegerControlInputType() throws RecognitionException;
void ruleReferenceControlType() throws RecognitionException;
void rule__ModelRoot__Alternatives() throws RecognitionException;
void rule__AbstractElement__Alternatives() throws RecognitionException;
void rule__EntityOptions__Alternatives() throws RecognitionException;
void rule__Datatype__Alternatives() throws RecognitionException;
void rule__SimpleDataType__Alternatives() throws RecognitionException;
void rule__EntityAttribute__Alternatives() throws RecognitionException;
void rule__SimpleDatatypeEntityAttribute__Alternatives() throws RecognitionException;
void rule__BaseDataTypeProperties__Alternatives() throws RecognitionException;
void rule__DictionaryContainer__Alternatives() throws RecognitionException;
void rule__DictionaryContainerContent__Alternatives() throws RecognitionException;
void rule__DictionaryControl__Alternatives() throws RecognitionException;
void rule__XAssignment__Alternatives() throws RecognitionException;
void rule__OpMultiAssign__Alternatives() throws RecognitionException;
void rule__OpEquality__Alternatives() throws RecognitionException;
void rule__XRelationalExpression__Alternatives_1() throws RecognitionException;
void rule__OpCompare__Alternatives() throws RecognitionException;
void rule__OpOther__Alternatives() throws RecognitionException;
void rule__OpOther__Alternatives_5_1() throws RecognitionException;
void rule__OpOther__Alternatives_6_1() throws RecognitionException;
void rule__OpAdd__Alternatives() throws RecognitionException;
void rule__OpMulti__Alternatives() throws RecognitionException;
void rule__XUnaryOperation__Alternatives() throws RecognitionException;
void rule__OpUnary__Alternatives() throws RecognitionException;
void rule__OpPostfix__Alternatives() throws RecognitionException;
void rule__XMemberFeatureCall__Alternatives_1() throws RecognitionException;
void rule__XMemberFeatureCall__Alternatives_1_0_0_0_1() throws RecognitionException;
void rule__XMemberFeatureCall__Alternatives_1_1_0_0_1() throws RecognitionException;
void rule__XMemberFeatureCall__Alternatives_1_1_3_1() throws RecognitionException;
void rule__XPrimaryExpression__Alternatives() throws RecognitionException;
void rule__XLiteral__Alternatives() throws RecognitionException;
void rule__XCollectionLiteral__Alternatives() throws RecognitionException;
void rule__XSwitchExpression__Alternatives_2() throws RecognitionException;
void rule__XCasePart__Alternatives_3() throws RecognitionException;
void rule__XExpressionOrVarDeclaration__Alternatives() throws RecognitionException;
void rule__XVariableDeclaration__Alternatives_1() throws RecognitionException;
void rule__XVariableDeclaration__Alternatives_2() throws RecognitionException;
void rule__XFeatureCall__Alternatives_3_1() throws RecognitionException;
void rule__FeatureCallID__Alternatives() throws RecognitionException;
void rule__IdOrSuper__Alternatives() throws RecognitionException;
void rule__XConstructorCall__Alternatives_4_1() throws RecognitionException;
void rule__XBooleanLiteral__Alternatives_1() throws RecognitionException;
void rule__XTryCatchFinallyExpression__Alternatives_3() throws RecognitionException;
void rule__Number__Alternatives() throws RecognitionException;
void rule__Number__Alternatives_1_0() throws RecognitionException;
void rule__Number__Alternatives_1_1_1() throws RecognitionException;
void rule__JvmTypeReference__Alternatives() throws RecognitionException;
void rule__JvmArgumentTypeReference__Alternatives() throws RecognitionException;
void rule__JvmWildcardTypeReference__Alternatives_2() throws RecognitionException;
void rule__XImportDeclaration__Alternatives_1() throws RecognitionException;
void rule__XImportDeclaration__Alternatives_1_0_3() throws RecognitionException;
void rule__IdGeneratorStrategy__Alternatives() throws RecognitionException;
void rule__Cardinality__Alternatives() throws RecognitionException;
void rule__SimpleTypes__Alternatives() throws RecognitionException;
void rule__IntegerControlInputType__Alternatives() throws RecognitionException;
void rule__ReferenceControlType__Alternatives() throws RecognitionException;
void rule__ModelRoot__Group_0__0() throws RecognitionException;
void rule__ModelRoot__Group_0__0__Impl() throws RecognitionException;
void rule__ModelRoot__Group_0__1() throws RecognitionException;
void rule__ModelRoot__Group_0__1__Impl() throws RecognitionException;
void rule__Model__Group__0() throws RecognitionException;
void rule__Model__Group__0__Impl() throws RecognitionException;
void rule__Model__Group__1() throws RecognitionException;
void rule__Model__Group__1__Impl() throws RecognitionException;
void rule__Model__Group__2() throws RecognitionException;
void rule__Model__Group__2__Impl() throws RecognitionException;
void rule__Model__Group__3() throws RecognitionException;
void rule__Model__Group__3__Impl() throws RecognitionException;
void rule__Model__Group__4() throws RecognitionException;
void rule__Model__Group__4__Impl() throws RecognitionException;
void rule__PackageDeclaration__Group__0() throws RecognitionException;
void rule__PackageDeclaration__Group__0__Impl() throws RecognitionException;
void rule__PackageDeclaration__Group__1() throws RecognitionException;
void rule__PackageDeclaration__Group__1__Impl() throws RecognitionException;
void rule__PackageDeclaration__Group__2() throws RecognitionException;
void rule__PackageDeclaration__Group__2__Impl() throws RecognitionException;
void rule__PackageDeclaration__Group__3() throws RecognitionException;
void rule__PackageDeclaration__Group__3__Impl() throws RecognitionException;
void rule__PackageDeclaration__Group__4() throws RecognitionException;
void rule__PackageDeclaration__Group__4__Impl() throws RecognitionException;
void rule__Enumeration__Group__0() throws RecognitionException;
void rule__Enumeration__Group__0__Impl() throws RecognitionException;
void rule__Enumeration__Group__1() throws RecognitionException;
void rule__Enumeration__Group__1__Impl() throws RecognitionException;
void rule__Enumeration__Group__2() throws RecognitionException;
void rule__Enumeration__Group__2__Impl() throws RecognitionException;
void rule__Enumeration__Group__3() throws RecognitionException;
void rule__Enumeration__Group__3__Impl() throws RecognitionException;
void rule__Enumeration__Group__4() throws RecognitionException;
void rule__Enumeration__Group__4__Impl() throws RecognitionException;
void rule__EnumerationValue__Group__0() throws RecognitionException;
void rule__EnumerationValue__Group__0__Impl() throws RecognitionException;
void rule__EnumerationValue__Group__1() throws RecognitionException;
void rule__EnumerationValue__Group__1__Impl() throws RecognitionException;
void rule__EnumerationValue__Group_1__0() throws RecognitionException;
void rule__EnumerationValue__Group_1__0__Impl() throws RecognitionException;
void rule__EnumerationValue__Group_1__1() throws RecognitionException;
void rule__EnumerationValue__Group_1__1__Impl() throws RecognitionException;
void rule__EntityNaturalKeyFields__Group__0() throws RecognitionException;
void rule__EntityNaturalKeyFields__Group__0__Impl() throws RecognitionException;
void rule__EntityNaturalKeyFields__Group__1() throws RecognitionException;
void rule__EntityNaturalKeyFields__Group__1__Impl() throws RecognitionException;
void rule__EntityNaturalKeyFields__Group__2() throws RecognitionException;
void rule__EntityNaturalKeyFields__Group__2__Impl() throws RecognitionException;
void rule__EntityNaturalKeyFields__Group__3() throws RecognitionException;
void rule__EntityNaturalKeyFields__Group__3__Impl() throws RecognitionException;
void rule__EntityNaturalKeyFields__Group__4() throws RecognitionException;
void rule__EntityNaturalKeyFields__Group__4__Impl() throws RecognitionException;
void rule__EntityNaturalKeyFields__Group__5() throws RecognitionException;
void rule__EntityNaturalKeyFields__Group__5__Impl() throws RecognitionException;
void rule__EntityNaturalKeyFields__Group_4__0() throws RecognitionException;
void rule__EntityNaturalKeyFields__Group_4__0__Impl() throws RecognitionException;
void rule__EntityNaturalKeyFields__Group_4__1() throws RecognitionException;
void rule__EntityNaturalKeyFields__Group_4__1__Impl() throws RecognitionException;
void rule__EntityHierarchical__Group__0() throws RecognitionException;
void rule__EntityHierarchical__Group__0__Impl() throws RecognitionException;
void rule__EntityHierarchical__Group__1() throws RecognitionException;
void rule__EntityHierarchical__Group__1__Impl() throws RecognitionException;
void rule__EntityHierarchical__Group__2() throws RecognitionException;
void rule__EntityHierarchical__Group__2__Impl() throws RecognitionException;
void rule__EntityDisableIdField__Group__0() throws RecognitionException;
void rule__EntityDisableIdField__Group__0__Impl() throws RecognitionException;
void rule__EntityDisableIdField__Group__1() throws RecognitionException;
void rule__EntityDisableIdField__Group__1__Impl() throws RecognitionException;
void rule__EntityDisableIdField__Group__2() throws RecognitionException;
void rule__EntityDisableIdField__Group__2__Impl() throws RecognitionException;
void rule__EntityLabelField__Group__0() throws RecognitionException;
void rule__EntityLabelField__Group__0__Impl() throws RecognitionException;
void rule__EntityLabelField__Group__1() throws RecognitionException;
void rule__EntityLabelField__Group__1__Impl() throws RecognitionException;
void rule__EntityLabelField__Group__2() throws RecognitionException;
void rule__EntityLabelField__Group__2__Impl() throws RecognitionException;
void rule__EntityPluralLabelField__Group__0() throws RecognitionException;
void rule__EntityPluralLabelField__Group__0__Impl() throws RecognitionException;
void rule__EntityPluralLabelField__Group__1() throws RecognitionException;
void rule__EntityPluralLabelField__Group__1__Impl() throws RecognitionException;
void rule__EntityPluralLabelField__Group__2() throws RecognitionException;
void rule__EntityPluralLabelField__Group__2__Impl() throws RecognitionException;
void rule__EntityOptionsContainer__Group__0() throws RecognitionException;
void rule__EntityOptionsContainer__Group__0__Impl() throws RecognitionException;
void rule__EntityOptionsContainer__Group__1() throws RecognitionException;
void rule__EntityOptionsContainer__Group__1__Impl() throws RecognitionException;
void rule__EntityOptionsContainer__Group__2() throws RecognitionException;
void rule__EntityOptionsContainer__Group__2__Impl() throws RecognitionException;
void rule__EntityOptionsContainer__Group__3() throws RecognitionException;
void rule__EntityOptionsContainer__Group__3__Impl() throws RecognitionException;
void rule__EntityOptionsContainer__Group__4() throws RecognitionException;
void rule__EntityOptionsContainer__Group__4__Impl() throws RecognitionException;
void rule__Entity__Group__0() throws RecognitionException;
void rule__Entity__Group__0__Impl() throws RecognitionException;
void rule__Entity__Group__1() throws RecognitionException;
void rule__Entity__Group__1__Impl() throws RecognitionException;
void rule__Entity__Group__2() throws RecognitionException;
void rule__Entity__Group__2__Impl() throws RecognitionException;
void rule__Entity__Group__3() throws RecognitionException;
void rule__Entity__Group__3__Impl() throws RecognitionException;
void rule__Entity__Group__4() throws RecognitionException;
void rule__Entity__Group__4__Impl() throws RecognitionException;
void rule__Entity__Group__5() throws RecognitionException;
void rule__Entity__Group__5__Impl() throws RecognitionException;
void rule__Entity__Group__6() throws RecognitionException;
void rule__Entity__Group__6__Impl() throws RecognitionException;
void rule__Entity__Group__7() throws RecognitionException;
void rule__Entity__Group__7__Impl() throws RecognitionException;
void rule__Entity__Group_2__0() throws RecognitionException;
void rule__Entity__Group_2__0__Impl() throws RecognitionException;
void rule__Entity__Group_2__1() throws RecognitionException;
void rule__Entity__Group_2__1__Impl() throws RecognitionException;
void rule__Entity__Group_3__0() throws RecognitionException;
void rule__Entity__Group_3__0__Impl() throws RecognitionException;
void rule__Entity__Group_3__1() throws RecognitionException;
void rule__Entity__Group_3__1__Impl() throws RecognitionException;
void rule__ValueObject__Group__0() throws RecognitionException;
void rule__ValueObject__Group__0__Impl() throws RecognitionException;
void rule__ValueObject__Group__1() throws RecognitionException;
void rule__ValueObject__Group__1__Impl() throws RecognitionException;
void rule__ValueObject__Group__2() throws RecognitionException;
void rule__ValueObject__Group__2__Impl() throws RecognitionException;
void rule__ValueObject__Group__3() throws RecognitionException;
void rule__ValueObject__Group__3__Impl() throws RecognitionException;
void rule__ValueObject__Group__4() throws RecognitionException;
void rule__ValueObject__Group__4__Impl() throws RecognitionException;
void rule__ValueObject__Group__5() throws RecognitionException;
void rule__ValueObject__Group__5__Impl() throws RecognitionException;
void rule__ValueObject__Group__6() throws RecognitionException;
void rule__ValueObject__Group__6__Impl() throws RecognitionException;
void rule__ValueObject__Group_2__0() throws RecognitionException;
void rule__ValueObject__Group_2__0__Impl() throws RecognitionException;
void rule__ValueObject__Group_2__1() throws RecognitionException;
void rule__ValueObject__Group_2__1__Impl() throws RecognitionException;
void rule__ValueObject__Group_3__0() throws RecognitionException;
void rule__ValueObject__Group_3__0__Impl() throws RecognitionException;
void rule__ValueObject__Group_3__1() throws RecognitionException;
void rule__ValueObject__Group_3__1__Impl() throws RecognitionException;
void rule__BaseDataType__Group__0() throws RecognitionException;
void rule__BaseDataType__Group__0__Impl() throws RecognitionException;
void rule__BaseDataType__Group__1() throws RecognitionException;
void rule__BaseDataType__Group__1__Impl() throws RecognitionException;
void rule__BaseDataTypeWidth__Group__0() throws RecognitionException;
void rule__BaseDataTypeWidth__Group__0__Impl() throws RecognitionException;
void rule__BaseDataTypeWidth__Group__1() throws RecognitionException;
void rule__BaseDataTypeWidth__Group__1__Impl() throws RecognitionException;
void rule__BaseDataTypeWidth__Group__2() throws RecognitionException;
void rule__BaseDataTypeWidth__Group__2__Impl() throws RecognitionException;
void rule__BaseDataTypeLabel__Group__0() throws RecognitionException;
void rule__BaseDataTypeLabel__Group__0__Impl() throws RecognitionException;
void rule__BaseDataTypeLabel__Group__1() throws RecognitionException;
void rule__BaseDataTypeLabel__Group__1__Impl() throws RecognitionException;
void rule__BaseDataTypeLabel__Group__2() throws RecognitionException;
void rule__BaseDataTypeLabel__Group__2__Impl() throws RecognitionException;
void rule__StringDataType__Group__0() throws RecognitionException;
void rule__StringDataType__Group__0__Impl() throws RecognitionException;
void rule__StringDataType__Group__1() throws RecognitionException;
void rule__StringDataType__Group__1__Impl() throws RecognitionException;
void rule__StringDataType__Group__2() throws RecognitionException;
void rule__StringDataType__Group__2__Impl() throws RecognitionException;
void rule__StringDataType__Group__3() throws RecognitionException;
void rule__StringDataType__Group__3__Impl() throws RecognitionException;
void rule__StringDataType__Group__4() throws RecognitionException;
void rule__StringDataType__Group__4__Impl() throws RecognitionException;
void rule__StringDataType__Group__5() throws RecognitionException;
void rule__StringDataType__Group__5__Impl() throws RecognitionException;
void rule__StringDataType__Group__6() throws RecognitionException;
void rule__StringDataType__Group__6__Impl() throws RecognitionException;
void rule__StringDataType__Group__7() throws RecognitionException;
void rule__StringDataType__Group__7__Impl() throws RecognitionException;
void rule__StringDataType__Group_2__0() throws RecognitionException;
void rule__StringDataType__Group_2__0__Impl() throws RecognitionException;
void rule__StringDataType__Group_2__1() throws RecognitionException;
void rule__StringDataType__Group_2__1__Impl() throws RecognitionException;
void rule__StringDataType__Group_5__0() throws RecognitionException;
void rule__StringDataType__Group_5__0__Impl() throws RecognitionException;
void rule__StringDataType__Group_5__1() throws RecognitionException;
void rule__StringDataType__Group_5__1__Impl() throws RecognitionException;
void rule__StringDataType__Group_6__0() throws RecognitionException;
void rule__StringDataType__Group_6__0__Impl() throws RecognitionException;
void rule__StringDataType__Group_6__1() throws RecognitionException;
void rule__StringDataType__Group_6__1__Impl() throws RecognitionException;
void rule__StringEntityAttribute__Group__0() throws RecognitionException;
void rule__StringEntityAttribute__Group__0__Impl() throws RecognitionException;
void rule__StringEntityAttribute__Group__1() throws RecognitionException;
void rule__StringEntityAttribute__Group__1__Impl() throws RecognitionException;
void rule__StringEntityAttribute__Group__2() throws RecognitionException;
void rule__StringEntityAttribute__Group__2__Impl() throws RecognitionException;
void rule__StringEntityAttribute__Group__3() throws RecognitionException;
void rule__StringEntityAttribute__Group__3__Impl() throws RecognitionException;
void rule__MapEntityAttribute__Group__0() throws RecognitionException;
void rule__MapEntityAttribute__Group__0__Impl() throws RecognitionException;
void rule__MapEntityAttribute__Group__1() throws RecognitionException;
void rule__MapEntityAttribute__Group__1__Impl() throws RecognitionException;
void rule__MapEntityAttribute__Group__2() throws RecognitionException;
void rule__MapEntityAttribute__Group__2__Impl() throws RecognitionException;
void rule__MapEntityAttribute__Group__3() throws RecognitionException;
void rule__MapEntityAttribute__Group__3__Impl() throws RecognitionException;
void rule__MapEntityAttribute__Group_2__0() throws RecognitionException;
void rule__MapEntityAttribute__Group_2__0__Impl() throws RecognitionException;
void rule__MapEntityAttribute__Group_2__1() throws RecognitionException;
void rule__MapEntityAttribute__Group_2__1__Impl() throws RecognitionException;
void rule__BooleanDataType__Group__0() throws RecognitionException;
void rule__BooleanDataType__Group__0__Impl() throws RecognitionException;
void rule__BooleanDataType__Group__1() throws RecognitionException;
void rule__BooleanDataType__Group__1__Impl() throws RecognitionException;
void rule__BooleanDataType__Group__2() throws RecognitionException;
void rule__BooleanDataType__Group__2__Impl() throws RecognitionException;
void rule__BooleanDataType__Group__3() throws RecognitionException;
void rule__BooleanDataType__Group__3__Impl() throws RecognitionException;
void rule__BooleanDataType__Group__4() throws RecognitionException;
void rule__BooleanDataType__Group__4__Impl() throws RecognitionException;
void rule__BooleanDataType__Group__5() throws RecognitionException;
void rule__BooleanDataType__Group__5__Impl() throws RecognitionException;
void rule__BooleanDataType__Group_2__0() throws RecognitionException;
void rule__BooleanDataType__Group_2__0__Impl() throws RecognitionException;
void rule__BooleanDataType__Group_2__1() throws RecognitionException;
void rule__BooleanDataType__Group_2__1__Impl() throws RecognitionException;
void rule__BooleanEntityAttribute__Group__0() throws RecognitionException;
void rule__BooleanEntityAttribute__Group__0__Impl() throws RecognitionException;
void rule__BooleanEntityAttribute__Group__1() throws RecognitionException;
void rule__BooleanEntityAttribute__Group__1__Impl() throws RecognitionException;
void rule__BooleanEntityAttribute__Group__2() throws RecognitionException;
void rule__BooleanEntityAttribute__Group__2__Impl() throws RecognitionException;
void rule__IntegerDataType__Group__0() throws RecognitionException;
void rule__IntegerDataType__Group__0__Impl() throws RecognitionException;
void rule__IntegerDataType__Group__1() throws RecognitionException;
void rule__IntegerDataType__Group__1__Impl() throws RecognitionException;
void rule__IntegerDataType__Group__2() throws RecognitionException;
void rule__IntegerDataType__Group__2__Impl() throws RecognitionException;
void rule__IntegerDataType__Group__3() throws RecognitionException;
void rule__IntegerDataType__Group__3__Impl() throws RecognitionException;
void rule__IntegerDataType__Group__4() throws RecognitionException;
void rule__IntegerDataType__Group__4__Impl() throws RecognitionException;
void rule__IntegerDataType__Group__5() throws RecognitionException;
void rule__IntegerDataType__Group__5__Impl() throws RecognitionException;
void rule__IntegerDataType__Group_2__0() throws RecognitionException;
void rule__IntegerDataType__Group_2__0__Impl() throws RecognitionException;
void rule__IntegerDataType__Group_2__1() throws RecognitionException;
void rule__IntegerDataType__Group_2__1__Impl() throws RecognitionException;
void rule__IntegerEntityAttribute__Group__0() throws RecognitionException;
void rule__IntegerEntityAttribute__Group__0__Impl() throws RecognitionException;
void rule__IntegerEntityAttribute__Group__1() throws RecognitionException;
void rule__IntegerEntityAttribute__Group__1__Impl() throws RecognitionException;
void rule__IntegerEntityAttribute__Group__2() throws RecognitionException;
void rule__IntegerEntityAttribute__Group__2__Impl() throws RecognitionException;
void rule__DateDataType__Group__0() throws RecognitionException;
void rule__DateDataType__Group__0__Impl() throws RecognitionException;
void rule__DateDataType__Group__1() throws RecognitionException;
void rule__DateDataType__Group__1__Impl() throws RecognitionException;
void rule__DateDataType__Group__2() throws RecognitionException;
void rule__DateDataType__Group__2__Impl() throws RecognitionException;
void rule__DateDataType__Group__3() throws RecognitionException;
void rule__DateDataType__Group__3__Impl() throws RecognitionException;
void rule__DateDataType__Group__4() throws RecognitionException;
void rule__DateDataType__Group__4__Impl() throws RecognitionException;
void rule__DateDataType__Group__5() throws RecognitionException;
void rule__DateDataType__Group__5__Impl() throws RecognitionException;
void rule__DateDataType__Group_2__0() throws RecognitionException;
void rule__DateDataType__Group_2__0__Impl() throws RecognitionException;
void rule__DateDataType__Group_2__1() throws RecognitionException;
void rule__DateDataType__Group_2__1__Impl() throws RecognitionException;
void rule__DateEntityAttribute__Group__0() throws RecognitionException;
void rule__DateEntityAttribute__Group__0__Impl() throws RecognitionException;
void rule__DateEntityAttribute__Group__1() throws RecognitionException;
void rule__DateEntityAttribute__Group__1__Impl() throws RecognitionException;
void rule__DateEntityAttribute__Group__2() throws RecognitionException;
void rule__DateEntityAttribute__Group__2__Impl() throws RecognitionException;
void rule__DecimalDataType__Group__0() throws RecognitionException;
void rule__DecimalDataType__Group__0__Impl() throws RecognitionException;
void rule__DecimalDataType__Group__1() throws RecognitionException;
void rule__DecimalDataType__Group__1__Impl() throws RecognitionException;
void rule__DecimalDataType__Group__2() throws RecognitionException;
void rule__DecimalDataType__Group__2__Impl() throws RecognitionException;
void rule__DecimalDataType__Group__3() throws RecognitionException;
void rule__DecimalDataType__Group__3__Impl() throws RecognitionException;
void rule__DecimalDataType__Group__4() throws RecognitionException;
void rule__DecimalDataType__Group__4__Impl() throws RecognitionException;
void rule__DecimalDataType__Group__5() throws RecognitionException;
void rule__DecimalDataType__Group__5__Impl() throws RecognitionException;
void rule__DecimalDataType__Group_2__0() throws RecognitionException;
void rule__DecimalDataType__Group_2__0__Impl() throws RecognitionException;
void rule__DecimalDataType__Group_2__1() throws RecognitionException;
void rule__DecimalDataType__Group_2__1__Impl() throws RecognitionException;
void rule__DecimalEntityAttribute__Group__0() throws RecognitionException;
void rule__DecimalEntityAttribute__Group__0__Impl() throws RecognitionException;
void rule__DecimalEntityAttribute__Group__1() throws RecognitionException;
void rule__DecimalEntityAttribute__Group__1__Impl() throws RecognitionException;
void rule__DecimalEntityAttribute__Group__2() throws RecognitionException;
void rule__DecimalEntityAttribute__Group__2__Impl() throws RecognitionException;
void rule__LongDataType__Group__0() throws RecognitionException;
void rule__LongDataType__Group__0__Impl() throws RecognitionException;
void rule__LongDataType__Group__1() throws RecognitionException;
void rule__LongDataType__Group__1__Impl() throws RecognitionException;
void rule__LongDataType__Group__2() throws RecognitionException;
void rule__LongDataType__Group__2__Impl() throws RecognitionException;
void rule__LongDataType__Group__3() throws RecognitionException;
void rule__LongDataType__Group__3__Impl() throws RecognitionException;
void rule__LongDataType__Group__4() throws RecognitionException;
void rule__LongDataType__Group__4__Impl() throws RecognitionException;
void rule__LongDataType__Group__5() throws RecognitionException;
void rule__LongDataType__Group__5__Impl() throws RecognitionException;
void rule__LongDataType__Group_2__0() throws RecognitionException;
void rule__LongDataType__Group_2__0__Impl() throws RecognitionException;
void rule__LongDataType__Group_2__1() throws RecognitionException;
void rule__LongDataType__Group_2__1__Impl() throws RecognitionException;
void rule__LongEntityAttribute__Group__0() throws RecognitionException;
void rule__LongEntityAttribute__Group__0__Impl() throws RecognitionException;
void rule__LongEntityAttribute__Group__1() throws RecognitionException;
void rule__LongEntityAttribute__Group__1__Impl() throws RecognitionException;
void rule__LongEntityAttribute__Group__2() throws RecognitionException;
void rule__LongEntityAttribute__Group__2__Impl() throws RecognitionException;
void rule__FloatDataType__Group__0() throws RecognitionException;
void rule__FloatDataType__Group__0__Impl() throws RecognitionException;
void rule__FloatDataType__Group__1() throws RecognitionException;
void rule__FloatDataType__Group__1__Impl() throws RecognitionException;
void rule__FloatDataType__Group__2() throws RecognitionException;
void rule__FloatDataType__Group__2__Impl() throws RecognitionException;
void rule__FloatDataType__Group__3() throws RecognitionException;
void rule__FloatDataType__Group__3__Impl() throws RecognitionException;
void rule__FloatDataType__Group__4() throws RecognitionException;
void rule__FloatDataType__Group__4__Impl() throws RecognitionException;
void rule__FloatDataType__Group__5() throws RecognitionException;
void rule__FloatDataType__Group__5__Impl() throws RecognitionException;
void rule__FloatDataType__Group_2__0() throws RecognitionException;
void rule__FloatDataType__Group_2__0__Impl() throws RecognitionException;
void rule__FloatDataType__Group_2__1() throws RecognitionException;
void rule__FloatDataType__Group_2__1__Impl() throws RecognitionException;
void rule__FloatEntityAttribute__Group__0() throws RecognitionException;
void rule__FloatEntityAttribute__Group__0__Impl() throws RecognitionException;
void rule__FloatEntityAttribute__Group__1() throws RecognitionException;
void rule__FloatEntityAttribute__Group__1__Impl() throws RecognitionException;
void rule__FloatEntityAttribute__Group__2() throws RecognitionException;
void rule__FloatEntityAttribute__Group__2__Impl() throws RecognitionException;
void rule__DoubleDataType__Group__0() throws RecognitionException;
void rule__DoubleDataType__Group__0__Impl() throws RecognitionException;
void rule__DoubleDataType__Group__1() throws RecognitionException;
void rule__DoubleDataType__Group__1__Impl() throws RecognitionException;
void rule__DoubleDataType__Group__2() throws RecognitionException;
void rule__DoubleDataType__Group__2__Impl() throws RecognitionException;
void rule__DoubleDataType__Group__3() throws RecognitionException;
void rule__DoubleDataType__Group__3__Impl() throws RecognitionException;
void rule__DoubleDataType__Group__4() throws RecognitionException;
void rule__DoubleDataType__Group__4__Impl() throws RecognitionException;
void rule__DoubleDataType__Group__5() throws RecognitionException;
void rule__DoubleDataType__Group__5__Impl() throws RecognitionException;
void rule__DoubleDataType__Group_2__0() throws RecognitionException;
void rule__DoubleDataType__Group_2__0__Impl() throws RecognitionException;
void rule__DoubleDataType__Group_2__1() throws RecognitionException;
void rule__DoubleDataType__Group_2__1__Impl() throws RecognitionException;
void rule__DoubleEntityAttribute__Group__0() throws RecognitionException;
void rule__DoubleEntityAttribute__Group__0__Impl() throws RecognitionException;
void rule__DoubleEntityAttribute__Group__1() throws RecognitionException;
void rule__DoubleEntityAttribute__Group__1__Impl() throws RecognitionException;
void rule__DoubleEntityAttribute__Group__2() throws RecognitionException;
void rule__DoubleEntityAttribute__Group__2__Impl() throws RecognitionException;
void rule__BinaryDataType__Group__0() throws RecognitionException;
void rule__BinaryDataType__Group__0__Impl() throws RecognitionException;
void rule__BinaryDataType__Group__1() throws RecognitionException;
void rule__BinaryDataType__Group__1__Impl() throws RecognitionException;
void rule__BinaryDataType__Group__2() throws RecognitionException;
void rule__BinaryDataType__Group__2__Impl() throws RecognitionException;
void rule__BinaryDataType__Group__3() throws RecognitionException;
void rule__BinaryDataType__Group__3__Impl() throws RecognitionException;
void rule__BinaryDataType__Group__4() throws RecognitionException;
void rule__BinaryDataType__Group__4__Impl() throws RecognitionException;
void rule__BinaryDataType__Group__5() throws RecognitionException;
void rule__BinaryDataType__Group__5__Impl() throws RecognitionException;
void rule__BinaryDataType__Group_2__0() throws RecognitionException;
void rule__BinaryDataType__Group_2__0__Impl() throws RecognitionException;
void rule__BinaryDataType__Group_2__1() throws RecognitionException;
void rule__BinaryDataType__Group_2__1__Impl() throws RecognitionException;
void rule__BinaryEntityAttribute__Group__0() throws RecognitionException;
void rule__BinaryEntityAttribute__Group__0__Impl() throws RecognitionException;
void rule__BinaryEntityAttribute__Group__1() throws RecognitionException;
void rule__BinaryEntityAttribute__Group__1__Impl() throws RecognitionException;
void rule__BinaryEntityAttribute__Group__2() throws RecognitionException;
void rule__BinaryEntityAttribute__Group__2__Impl() throws RecognitionException;
void rule__EntityDataType__Group__0() throws RecognitionException;
void rule__EntityDataType__Group__0__Impl() throws RecognitionException;
void rule__EntityDataType__Group__1() throws RecognitionException;
void rule__EntityDataType__Group__1__Impl() throws RecognitionException;
void rule__EntityDataType__Group__2() throws RecognitionException;
void rule__EntityDataType__Group__2__Impl() throws RecognitionException;
void rule__EntityDataType__Group__3() throws RecognitionException;
void rule__EntityDataType__Group__3__Impl() throws RecognitionException;
void rule__EntityDataType__Group__4() throws RecognitionException;
void rule__EntityDataType__Group__4__Impl() throws RecognitionException;
void rule__EntityDataType__Group__5() throws RecognitionException;
void rule__EntityDataType__Group__5__Impl() throws RecognitionException;
void rule__EntityDataType__Group__6() throws RecognitionException;
void rule__EntityDataType__Group__6__Impl() throws RecognitionException;
void rule__EntityDataType__Group__7() throws RecognitionException;
void rule__EntityDataType__Group__7__Impl() throws RecognitionException;
void rule__EntityDataType__Group_2__0() throws RecognitionException;
void rule__EntityDataType__Group_2__0__Impl() throws RecognitionException;
void rule__EntityDataType__Group_2__1() throws RecognitionException;
void rule__EntityDataType__Group_2__1__Impl() throws RecognitionException;
void rule__EntityEntityAttribute__Group__0() throws RecognitionException;
void rule__EntityEntityAttribute__Group__0__Impl() throws RecognitionException;
void rule__EntityEntityAttribute__Group__1() throws RecognitionException;
void rule__EntityEntityAttribute__Group__1__Impl() throws RecognitionException;
void rule__EntityEntityAttribute__Group__2() throws RecognitionException;
void rule__EntityEntityAttribute__Group__2__Impl() throws RecognitionException;
void rule__EntityEntityAttribute__Group__3() throws RecognitionException;
void rule__EntityEntityAttribute__Group__3__Impl() throws RecognitionException;
void rule__ValueObjectEntityAttribute__Group__0() throws RecognitionException;
void rule__ValueObjectEntityAttribute__Group__0__Impl() throws RecognitionException;
void rule__ValueObjectEntityAttribute__Group__1() throws RecognitionException;
void rule__ValueObjectEntityAttribute__Group__1__Impl() throws RecognitionException;
void rule__ValueObjectEntityAttribute__Group__2() throws RecognitionException;
void rule__ValueObjectEntityAttribute__Group__2__Impl() throws RecognitionException;
void rule__ValueObjectEntityAttribute__Group__3() throws RecognitionException;
void rule__ValueObjectEntityAttribute__Group__3__Impl() throws RecognitionException;
void rule__EnumerationDataType__Group__0() throws RecognitionException;
void rule__EnumerationDataType__Group__0__Impl() throws RecognitionException;
void rule__EnumerationDataType__Group__1() throws RecognitionException;
void rule__EnumerationDataType__Group__1__Impl() throws RecognitionException;
void rule__EnumerationDataType__Group__2() throws RecognitionException;
void rule__EnumerationDataType__Group__2__Impl() throws RecognitionException;
void rule__EnumerationDataType__Group__3() throws RecognitionException;
void rule__EnumerationDataType__Group__3__Impl() throws RecognitionException;
void rule__EnumerationDataType__Group__4() throws RecognitionException;
void rule__EnumerationDataType__Group__4__Impl() throws RecognitionException;
void rule__EnumerationDataType__Group__5() throws RecognitionException;
void rule__EnumerationDataType__Group__5__Impl() throws RecognitionException;
void rule__EnumerationDataType__Group__6() throws RecognitionException;
void rule__EnumerationDataType__Group__6__Impl() throws RecognitionException;
void rule__EnumerationDataType__Group__7() throws RecognitionException;
void rule__EnumerationDataType__Group__7__Impl() throws RecognitionException;
void rule__EnumerationDataType__Group_2__0() throws RecognitionException;
void rule__EnumerationDataType__Group_2__0__Impl() throws RecognitionException;
void rule__EnumerationDataType__Group_2__1() throws RecognitionException;
void rule__EnumerationDataType__Group_2__1__Impl() throws RecognitionException;
void rule__EnumerationEntityAttribute__Group__0() throws RecognitionException;
void rule__EnumerationEntityAttribute__Group__0__Impl() throws RecognitionException;
void rule__EnumerationEntityAttribute__Group__1() throws RecognitionException;
void rule__EnumerationEntityAttribute__Group__1__Impl() throws RecognitionException;
void rule__EnumerationEntityAttribute__Group__2() throws RecognitionException;
void rule__EnumerationEntityAttribute__Group__2__Impl() throws RecognitionException;
void rule__EnumerationEntityAttribute__Group__3() throws RecognitionException;
void rule__EnumerationEntityAttribute__Group__3__Impl() throws RecognitionException;
void rule__Dictionary__Group__0() throws RecognitionException;
void rule__Dictionary__Group__0__Impl() throws RecognitionException;
void rule__Dictionary__Group__1() throws RecognitionException;
void rule__Dictionary__Group__1__Impl() throws RecognitionException;
void rule__Dictionary__Group__2() throws RecognitionException;
void rule__Dictionary__Group__2__Impl() throws RecognitionException;
void rule__Dictionary__Group__3() throws RecognitionException;
void rule__Dictionary__Group__3__Impl() throws RecognitionException;
void rule__Dictionary__Group__4() throws RecognitionException;
void rule__Dictionary__Group__4__Impl() throws RecognitionException;
void rule__Dictionary__Group__5() throws RecognitionException;
void rule__Dictionary__Group__5__Impl() throws RecognitionException;
void rule__Dictionary__Group__6() throws RecognitionException;
void rule__Dictionary__Group__6__Impl() throws RecognitionException;
void rule__Dictionary__Group__7() throws RecognitionException;
void rule__Dictionary__Group__7__Impl() throws RecognitionException;
void rule__Dictionary__Group__8() throws RecognitionException;
void rule__Dictionary__Group__8__Impl() throws RecognitionException;
void rule__Dictionary__Group__9() throws RecognitionException;
void rule__Dictionary__Group__9__Impl() throws RecognitionException;
void rule__Dictionary__Group__10() throws RecognitionException;
void rule__Dictionary__Group__10__Impl() throws RecognitionException;
void rule__Dictionary__Group__11() throws RecognitionException;
void rule__Dictionary__Group__11__Impl() throws RecognitionException;
void rule__Dictionary__Group_5__0() throws RecognitionException;
void rule__Dictionary__Group_5__0__Impl() throws RecognitionException;
void rule__Dictionary__Group_5__1() throws RecognitionException;
void rule__Dictionary__Group_5__1__Impl() throws RecognitionException;
void rule__Dictionary__Group_6__0() throws RecognitionException;
void rule__Dictionary__Group_6__0__Impl() throws RecognitionException;
void rule__Dictionary__Group_6__1() throws RecognitionException;
void rule__Dictionary__Group_6__1__Impl() throws RecognitionException;
void rule__Dictionary__Group_7__0() throws RecognitionException;
void rule__Dictionary__Group_7__0__Impl() throws RecognitionException;
void rule__Dictionary__Group_7__1() throws RecognitionException;
void rule__Dictionary__Group_7__1__Impl() throws RecognitionException;
void rule__Dictionary__Group_7__2() throws RecognitionException;
void rule__Dictionary__Group_7__2__Impl() throws RecognitionException;
void rule__Dictionary__Group_7__3() throws RecognitionException;
void rule__Dictionary__Group_7__3__Impl() throws RecognitionException;
void rule__Dictionary__Group_8__0() throws RecognitionException;
void rule__Dictionary__Group_8__0__Impl() throws RecognitionException;
void rule__Dictionary__Group_8__1() throws RecognitionException;
void rule__Dictionary__Group_8__1__Impl() throws RecognitionException;
void rule__Dictionary__Group_8__2() throws RecognitionException;
void rule__Dictionary__Group_8__2__Impl() throws RecognitionException;
void rule__Dictionary__Group_8__3() throws RecognitionException;
void rule__Dictionary__Group_8__3__Impl() throws RecognitionException;
void rule__DictionarySearch__Group__0() throws RecognitionException;
void rule__DictionarySearch__Group__0__Impl() throws RecognitionException;
void rule__DictionarySearch__Group__1() throws RecognitionException;
void rule__DictionarySearch__Group__1__Impl() throws RecognitionException;
void rule__DictionarySearch__Group__2() throws RecognitionException;
void rule__DictionarySearch__Group__2__Impl() throws RecognitionException;
void rule__DictionarySearch__Group__3() throws RecognitionException;
void rule__DictionarySearch__Group__3__Impl() throws RecognitionException;
void rule__DictionarySearch__Group__4() throws RecognitionException;
void rule__DictionarySearch__Group__4__Impl() throws RecognitionException;
void rule__DictionarySearch__Group__5() throws RecognitionException;
void rule__DictionarySearch__Group__5__Impl() throws RecognitionException;
void rule__DictionarySearch__Group__6() throws RecognitionException;
void rule__DictionarySearch__Group__6__Impl() throws RecognitionException;
void rule__DictionarySearch__Group_3__0() throws RecognitionException;
void rule__DictionarySearch__Group_3__0__Impl() throws RecognitionException;
void rule__DictionarySearch__Group_3__1() throws RecognitionException;
void rule__DictionarySearch__Group_3__1__Impl() throws RecognitionException;
void rule__DictionaryEditor__Group__0() throws RecognitionException;
void rule__DictionaryEditor__Group__0__Impl() throws RecognitionException;
void rule__DictionaryEditor__Group__1() throws RecognitionException;
void rule__DictionaryEditor__Group__1__Impl() throws RecognitionException;
void rule__DictionaryEditor__Group__2() throws RecognitionException;
void rule__DictionaryEditor__Group__2__Impl() throws RecognitionException;
void rule__DictionaryEditor__Group__3() throws RecognitionException;
void rule__DictionaryEditor__Group__3__Impl() throws RecognitionException;
void rule__DictionaryEditor__Group__4() throws RecognitionException;
void rule__DictionaryEditor__Group__4__Impl() throws RecognitionException;
void rule__DictionaryEditor__Group__5() throws RecognitionException;
void rule__DictionaryEditor__Group__5__Impl() throws RecognitionException;
void rule__DictionaryEditor__Group__6() throws RecognitionException;
void rule__DictionaryEditor__Group__6__Impl() throws RecognitionException;
void rule__DictionaryEditor__Group__7() throws RecognitionException;
void rule__DictionaryEditor__Group__7__Impl() throws RecognitionException;
void rule__DictionaryEditor__Group_3__0() throws RecognitionException;
void rule__DictionaryEditor__Group_3__0__Impl() throws RecognitionException;
void rule__DictionaryEditor__Group_3__1() throws RecognitionException;
void rule__DictionaryEditor__Group_3__1__Impl() throws RecognitionException;
void rule__DictionaryFilter__Group__0() throws RecognitionException;
void rule__DictionaryFilter__Group__0__Impl() throws RecognitionException;
void rule__DictionaryFilter__Group__1() throws RecognitionException;
void rule__DictionaryFilter__Group__1__Impl() throws RecognitionException;
void rule__DictionaryFilter__Group__2() throws RecognitionException;
void rule__DictionaryFilter__Group__2__Impl() throws RecognitionException;
void rule__DictionaryFilter__Group__3() throws RecognitionException;
void rule__DictionaryFilter__Group__3__Impl() throws RecognitionException;
void rule__DictionaryFilter__Group__4() throws RecognitionException;
void rule__DictionaryFilter__Group__4__Impl() throws RecognitionException;
void rule__DictionaryFilter__Group__5() throws RecognitionException;
void rule__DictionaryFilter__Group__5__Impl() throws RecognitionException;
void rule__DictionaryFilter__Group__6() throws RecognitionException;
void rule__DictionaryFilter__Group__6__Impl() throws RecognitionException;
void rule__DictionaryResult__Group__0() throws RecognitionException;
void rule__DictionaryResult__Group__0__Impl() throws RecognitionException;
void rule__DictionaryResult__Group__1() throws RecognitionException;
void rule__DictionaryResult__Group__1__Impl() throws RecognitionException;
void rule__DictionaryResult__Group__2() throws RecognitionException;
void rule__DictionaryResult__Group__2__Impl() throws RecognitionException;
void rule__DictionaryResult__Group__3() throws RecognitionException;
void rule__DictionaryResult__Group__3__Impl() throws RecognitionException;
void rule__DictionaryResult__Group__4() throws RecognitionException;
void rule__DictionaryResult__Group__4__Impl() throws RecognitionException;
void rule__ColumnLayout__Group__0() throws RecognitionException;
void rule__ColumnLayout__Group__0__Impl() throws RecognitionException;
void rule__ColumnLayout__Group__1() throws RecognitionException;
void rule__ColumnLayout__Group__1__Impl() throws RecognitionException;
void rule__ColumnLayout__Group__2() throws RecognitionException;
void rule__ColumnLayout__Group__2__Impl() throws RecognitionException;
void rule__ColumnLayout__Group__3() throws RecognitionException;
void rule__ColumnLayout__Group__3__Impl() throws RecognitionException;
void rule__ColumnLayout__Group__4() throws RecognitionException;
void rule__ColumnLayout__Group__4__Impl() throws RecognitionException;
void rule__ColumnLayoutData__Group__0() throws RecognitionException;
void rule__ColumnLayoutData__Group__0__Impl() throws RecognitionException;
void rule__ColumnLayoutData__Group__1() throws RecognitionException;
void rule__ColumnLayoutData__Group__1__Impl() throws RecognitionException;
void rule__ColumnLayoutData__Group__2() throws RecognitionException;
void rule__ColumnLayoutData__Group__2__Impl() throws RecognitionException;
void rule__ColumnLayoutData__Group__3() throws RecognitionException;
void rule__ColumnLayoutData__Group__3__Impl() throws RecognitionException;
void rule__ColumnLayoutData__Group__4() throws RecognitionException;
void rule__ColumnLayoutData__Group__4__Impl() throws RecognitionException;
void rule__DictionaryComposite__Group__0() throws RecognitionException;
void rule__DictionaryComposite__Group__0__Impl() throws RecognitionException;
void rule__DictionaryComposite__Group__1() throws RecognitionException;
void rule__DictionaryComposite__Group__1__Impl() throws RecognitionException;
void rule__DictionaryComposite__Group__2() throws RecognitionException;
void rule__DictionaryComposite__Group__2__Impl() throws RecognitionException;
void rule__DictionaryComposite__Group__3() throws RecognitionException;
void rule__DictionaryComposite__Group__3__Impl() throws RecognitionException;
void rule__DictionaryComposite__Group__4() throws RecognitionException;
void rule__DictionaryComposite__Group__4__Impl() throws RecognitionException;
void rule__DictionaryComposite__Group__5() throws RecognitionException;
void rule__DictionaryComposite__Group__5__Impl() throws RecognitionException;
void rule__DictionaryComposite__Group__6() throws RecognitionException;
void rule__DictionaryComposite__Group__6__Impl() throws RecognitionException;
void rule__DictionaryEditableTable__Group__0() throws RecognitionException;
void rule__DictionaryEditableTable__Group__0__Impl() throws RecognitionException;
void rule__DictionaryEditableTable__Group__1() throws RecognitionException;
void rule__DictionaryEditableTable__Group__1__Impl() throws RecognitionException;
void rule__DictionaryEditableTable__Group__2() throws RecognitionException;
void rule__DictionaryEditableTable__Group__2__Impl() throws RecognitionException;
void rule__DictionaryEditableTable__Group__3() throws RecognitionException;
void rule__DictionaryEditableTable__Group__3__Impl() throws RecognitionException;
void rule__DictionaryEditableTable__Group__4() throws RecognitionException;
void rule__DictionaryEditableTable__Group__4__Impl() throws RecognitionException;
void rule__DictionaryEditableTable__Group__5() throws RecognitionException;
void rule__DictionaryEditableTable__Group__5__Impl() throws RecognitionException;
void rule__DictionaryEditableTable__Group__6() throws RecognitionException;
void rule__DictionaryEditableTable__Group__6__Impl() throws RecognitionException;
void rule__DictionaryEditableTable__Group__7() throws RecognitionException;
void rule__DictionaryEditableTable__Group__7__Impl() throws RecognitionException;
void rule__DictionaryEditableTable__Group__8() throws RecognitionException;
void rule__DictionaryEditableTable__Group__8__Impl() throws RecognitionException;
void rule__DictionaryEditableTable__Group__9() throws RecognitionException;
void rule__DictionaryEditableTable__Group__9__Impl() throws RecognitionException;
void rule__DictionaryEditableTable__Group__10() throws RecognitionException;
void rule__DictionaryEditableTable__Group__10__Impl() throws RecognitionException;
void rule__DictionaryEditableTable__Group__11() throws RecognitionException;
void rule__DictionaryEditableTable__Group__11__Impl() throws RecognitionException;
void rule__DictionaryEditableTable__Group__12() throws RecognitionException;
void rule__DictionaryEditableTable__Group__12__Impl() throws RecognitionException;
void rule__DictionaryEditableTable__Group_3__0() throws RecognitionException;
void rule__DictionaryEditableTable__Group_3__0__Impl() throws RecognitionException;
void rule__DictionaryEditableTable__Group_3__1() throws RecognitionException;
void rule__DictionaryEditableTable__Group_3__1__Impl() throws RecognitionException;
void rule__DictionaryEditableTable__Group_4__0() throws RecognitionException;
void rule__DictionaryEditableTable__Group_4__0__Impl() throws RecognitionException;
void rule__DictionaryEditableTable__Group_4__1() throws RecognitionException;
void rule__DictionaryEditableTable__Group_4__1__Impl() throws RecognitionException;
void rule__DictionaryAssignmentTable__Group__0() throws RecognitionException;
void rule__DictionaryAssignmentTable__Group__0__Impl() throws RecognitionException;
void rule__DictionaryAssignmentTable__Group__1() throws RecognitionException;
void rule__DictionaryAssignmentTable__Group__1__Impl() throws RecognitionException;
void rule__DictionaryAssignmentTable__Group__2() throws RecognitionException;
void rule__DictionaryAssignmentTable__Group__2__Impl() throws RecognitionException;
void rule__DictionaryAssignmentTable__Group__3() throws RecognitionException;
void rule__DictionaryAssignmentTable__Group__3__Impl() throws RecognitionException;
void rule__DictionaryAssignmentTable__Group__4() throws RecognitionException;
void rule__DictionaryAssignmentTable__Group__4__Impl() throws RecognitionException;
void rule__DictionaryAssignmentTable__Group__5() throws RecognitionException;
void rule__DictionaryAssignmentTable__Group__5__Impl() throws RecognitionException;
void rule__DictionaryAssignmentTable__Group__6() throws RecognitionException;
void rule__DictionaryAssignmentTable__Group__6__Impl() throws RecognitionException;
void rule__DictionaryAssignmentTable__Group__7() throws RecognitionException;
void rule__DictionaryAssignmentTable__Group__7__Impl() throws RecognitionException;
void rule__DictionaryAssignmentTable__Group__8() throws RecognitionException;
void rule__DictionaryAssignmentTable__Group__8__Impl() throws RecognitionException;
void rule__DictionaryAssignmentTable__Group__9() throws RecognitionException;
void rule__DictionaryAssignmentTable__Group__9__Impl() throws RecognitionException;
void rule__DictionaryAssignmentTable__Group__10() throws RecognitionException;
void rule__DictionaryAssignmentTable__Group__10__Impl() throws RecognitionException;
void rule__DictionaryAssignmentTable__Group__11() throws RecognitionException;
void rule__DictionaryAssignmentTable__Group__11__Impl() throws RecognitionException;
void rule__DictionaryAssignmentTable__Group__12() throws RecognitionException;
void rule__DictionaryAssignmentTable__Group__12__Impl() throws RecognitionException;
void rule__DictionaryAssignmentTable__Group__13() throws RecognitionException;
void rule__DictionaryAssignmentTable__Group__13__Impl() throws RecognitionException;
void rule__DictionaryAssignmentTable__Group__14() throws RecognitionException;
void rule__DictionaryAssignmentTable__Group__14__Impl() throws RecognitionException;
void rule__DictionaryAssignmentTable__Group_3__0() throws RecognitionException;
void rule__DictionaryAssignmentTable__Group_3__0__Impl() throws RecognitionException;
void rule__DictionaryAssignmentTable__Group_3__1() throws RecognitionException;
void rule__DictionaryAssignmentTable__Group_3__1__Impl() throws RecognitionException;
void rule__DictionaryAssignmentTable__Group_4__0() throws RecognitionException;
void rule__DictionaryAssignmentTable__Group_4__0__Impl() throws RecognitionException;
void rule__DictionaryAssignmentTable__Group_4__1() throws RecognitionException;
void rule__DictionaryAssignmentTable__Group_4__1__Impl() throws RecognitionException;
void rule__Labels__Group__0() throws RecognitionException;
void rule__Labels__Group__0__Impl() throws RecognitionException;
void rule__Labels__Group__1() throws RecognitionException;
void rule__Labels__Group__1__Impl() throws RecognitionException;
void rule__Labels__Group__2() throws RecognitionException;
void rule__Labels__Group__2__Impl() throws RecognitionException;
void rule__Labels__Group__3() throws RecognitionException;
void rule__Labels__Group__3__Impl() throws RecognitionException;
void rule__Labels__Group__4() throws RecognitionException;
void rule__Labels__Group__4__Impl() throws RecognitionException;
void rule__Labels__Group__5() throws RecognitionException;
void rule__Labels__Group__5__Impl() throws RecognitionException;
void rule__Labels__Group_1__0() throws RecognitionException;
void rule__Labels__Group_1__0__Impl() throws RecognitionException;
void rule__Labels__Group_1__1() throws RecognitionException;
void rule__Labels__Group_1__1__Impl() throws RecognitionException;
void rule__Labels__Group_2__0() throws RecognitionException;
void rule__Labels__Group_2__0__Impl() throws RecognitionException;
void rule__Labels__Group_2__1() throws RecognitionException;
void rule__Labels__Group_2__1__Impl() throws RecognitionException;
void rule__Labels__Group_3__0() throws RecognitionException;
void rule__Labels__Group_3__0__Impl() throws RecognitionException;
void rule__Labels__Group_3__1() throws RecognitionException;
void rule__Labels__Group_3__1__Impl() throws RecognitionException;
void rule__Labels__Group_4__0() throws RecognitionException;
void rule__Labels__Group_4__0__Impl() throws RecognitionException;
void rule__Labels__Group_4__1() throws RecognitionException;
void rule__Labels__Group_4__1__Impl() throws RecognitionException;
void rule__Labels__Group_5__0() throws RecognitionException;
void rule__Labels__Group_5__0__Impl() throws RecognitionException;
void rule__Labels__Group_5__1() throws RecognitionException;
void rule__Labels__Group_5__1__Impl() throws RecognitionException;
void rule__BaseDictionaryControl__Group__0() throws RecognitionException;
void rule__BaseDictionaryControl__Group__0__Impl() throws RecognitionException;
void rule__BaseDictionaryControl__Group__1() throws RecognitionException;
void rule__BaseDictionaryControl__Group__1__Impl() throws RecognitionException;
void rule__BaseDictionaryControl__Group__2() throws RecognitionException;
void rule__BaseDictionaryControl__Group__2__Impl() throws RecognitionException;
void rule__BaseDictionaryControl__Group__3() throws RecognitionException;
void rule__BaseDictionaryControl__Group__3__Impl() throws RecognitionException;
void rule__BaseDictionaryControl__Group__4() throws RecognitionException;
void rule__BaseDictionaryControl__Group__4__Impl() throws RecognitionException;
void rule__BaseDictionaryControl__Group__5() throws RecognitionException;
void rule__BaseDictionaryControl__Group__5__Impl() throws RecognitionException;
void rule__BaseDictionaryControl__Group_0__0() throws RecognitionException;
void rule__BaseDictionaryControl__Group_0__0__Impl() throws RecognitionException;
void rule__BaseDictionaryControl__Group_0__1() throws RecognitionException;
void rule__BaseDictionaryControl__Group_0__1__Impl() throws RecognitionException;
void rule__BaseDictionaryControl__Group_1__0() throws RecognitionException;
void rule__BaseDictionaryControl__Group_1__0__Impl() throws RecognitionException;
void rule__BaseDictionaryControl__Group_1__1() throws RecognitionException;
void rule__BaseDictionaryControl__Group_1__1__Impl() throws RecognitionException;
void rule__BaseDictionaryControl__Group_4__0() throws RecognitionException;
void rule__BaseDictionaryControl__Group_4__0__Impl() throws RecognitionException;
void rule__BaseDictionaryControl__Group_4__1() throws RecognitionException;
void rule__BaseDictionaryControl__Group_4__1__Impl() throws RecognitionException;
void rule__BaseDictionaryControl__Group_5__0() throws RecognitionException;
void rule__BaseDictionaryControl__Group_5__0__Impl() throws RecognitionException;
void rule__BaseDictionaryControl__Group_5__1() throws RecognitionException;
void rule__BaseDictionaryControl__Group_5__1__Impl() throws RecognitionException;
void rule__DictionaryControlGroupOptionMultiFilterField__Group__0() throws RecognitionException;
void rule__DictionaryControlGroupOptionMultiFilterField__Group__0__Impl() throws RecognitionException;
void rule__DictionaryControlGroupOptionMultiFilterField__Group__1() throws RecognitionException;
void rule__DictionaryControlGroupOptionMultiFilterField__Group__1__Impl() throws RecognitionException;
void rule__DictionaryControlGroupOptionMultiFilterField__Group_1__0() throws RecognitionException;
void rule__DictionaryControlGroupOptionMultiFilterField__Group_1__0__Impl() throws RecognitionException;
void rule__DictionaryControlGroupOptionMultiFilterField__Group_1__1() throws RecognitionException;
void rule__DictionaryControlGroupOptionMultiFilterField__Group_1__1__Impl() throws RecognitionException;
void rule__DictionaryControlGroupOptionsContainer__Group__0() throws RecognitionException;
void rule__DictionaryControlGroupOptionsContainer__Group__0__Impl() throws RecognitionException;
void rule__DictionaryControlGroupOptionsContainer__Group__1() throws RecognitionException;
void rule__DictionaryControlGroupOptionsContainer__Group__1__Impl() throws RecognitionException;
void rule__DictionaryControlGroupOptionsContainer__Group__2() throws RecognitionException;
void rule__DictionaryControlGroupOptionsContainer__Group__2__Impl() throws RecognitionException;
void rule__DictionaryControlGroupOptionsContainer__Group__3() throws RecognitionException;
void rule__DictionaryControlGroupOptionsContainer__Group__3__Impl() throws RecognitionException;
void rule__DictionaryControlGroupOptionsContainer__Group__4() throws RecognitionException;
void rule__DictionaryControlGroupOptionsContainer__Group__4__Impl() throws RecognitionException;
void rule__DictionaryControlGroup__Group__0() throws RecognitionException;
void rule__DictionaryControlGroup__Group__0__Impl() throws RecognitionException;
void rule__DictionaryControlGroup__Group__1() throws RecognitionException;
void rule__DictionaryControlGroup__Group__1__Impl() throws RecognitionException;
void rule__DictionaryControlGroup__Group__2() throws RecognitionException;
void rule__DictionaryControlGroup__Group__2__Impl() throws RecognitionException;
void rule__DictionaryControlGroup__Group__3() throws RecognitionException;
void rule__DictionaryControlGroup__Group__3__Impl() throws RecognitionException;
void rule__DictionaryControlGroup__Group__4() throws RecognitionException;
void rule__DictionaryControlGroup__Group__4__Impl() throws RecognitionException;
void rule__DictionaryControlGroup__Group_3__0() throws RecognitionException;
void rule__DictionaryControlGroup__Group_3__0__Impl() throws RecognitionException;
void rule__DictionaryControlGroup__Group_3__1() throws RecognitionException;
void rule__DictionaryControlGroup__Group_3__1__Impl() throws RecognitionException;
void rule__DictionaryControlGroup__Group_4__0() throws RecognitionException;
void rule__DictionaryControlGroup__Group_4__0__Impl() throws RecognitionException;
void rule__DictionaryControlGroup__Group_4__1() throws RecognitionException;
void rule__DictionaryControlGroup__Group_4__1__Impl() throws RecognitionException;
void rule__DictionaryControlGroup__Group_4__2() throws RecognitionException;
void rule__DictionaryControlGroup__Group_4__2__Impl() throws RecognitionException;
void rule__DictionaryControlGroup__Group_4__3() throws RecognitionException;
void rule__DictionaryControlGroup__Group_4__3__Impl() throws RecognitionException;
void rule__DictionaryControlGroup__Group_4__4() throws RecognitionException;
void rule__DictionaryControlGroup__Group_4__4__Impl() throws RecognitionException;
void rule__DictionaryHierarchicalControl__Group__0() throws RecognitionException;
void rule__DictionaryHierarchicalControl__Group__0__Impl() throws RecognitionException;
void rule__DictionaryHierarchicalControl__Group__1() throws RecognitionException;
void rule__DictionaryHierarchicalControl__Group__1__Impl() throws RecognitionException;
void rule__DictionaryHierarchicalControl__Group__2() throws RecognitionException;
void rule__DictionaryHierarchicalControl__Group__2__Impl() throws RecognitionException;
void rule__DictionaryHierarchicalControl__Group__3() throws RecognitionException;
void rule__DictionaryHierarchicalControl__Group__3__Impl() throws RecognitionException;
void rule__DictionaryHierarchicalControl__Group__4() throws RecognitionException;
void rule__DictionaryHierarchicalControl__Group__4__Impl() throws RecognitionException;
void rule__DictionaryHierarchicalControl__Group_3__0() throws RecognitionException;
void rule__DictionaryHierarchicalControl__Group_3__0__Impl() throws RecognitionException;
void rule__DictionaryHierarchicalControl__Group_3__1() throws RecognitionException;
void rule__DictionaryHierarchicalControl__Group_3__1__Impl() throws RecognitionException;
void rule__DictionaryHierarchicalControl__Group_4__0() throws RecognitionException;
void rule__DictionaryHierarchicalControl__Group_4__0__Impl() throws RecognitionException;
void rule__DictionaryHierarchicalControl__Group_4__1() throws RecognitionException;
void rule__DictionaryHierarchicalControl__Group_4__1__Impl() throws RecognitionException;
void rule__DictionaryHierarchicalControl__Group_4__2() throws RecognitionException;
void rule__DictionaryHierarchicalControl__Group_4__2__Impl() throws RecognitionException;
void rule__DictionaryHierarchicalControl__Group_4__3() throws RecognitionException;
void rule__DictionaryHierarchicalControl__Group_4__3__Impl() throws RecognitionException;
void rule__DictionaryHierarchicalControl__Group_4__4() throws RecognitionException;
void rule__DictionaryHierarchicalControl__Group_4__4__Impl() throws RecognitionException;
void rule__DictionaryTextControl__Group__0() throws RecognitionException;
void rule__DictionaryTextControl__Group__0__Impl() throws RecognitionException;
void rule__DictionaryTextControl__Group__1() throws RecognitionException;
void rule__DictionaryTextControl__Group__1__Impl() throws RecognitionException;
void rule__DictionaryTextControl__Group__2() throws RecognitionException;
void rule__DictionaryTextControl__Group__2__Impl() throws RecognitionException;
void rule__DictionaryTextControl__Group__3() throws RecognitionException;
void rule__DictionaryTextControl__Group__3__Impl() throws RecognitionException;
void rule__DictionaryTextControl__Group__4() throws RecognitionException;
void rule__DictionaryTextControl__Group__4__Impl() throws RecognitionException;
void rule__DictionaryTextControl__Group_3__0() throws RecognitionException;
void rule__DictionaryTextControl__Group_3__0__Impl() throws RecognitionException;
void rule__DictionaryTextControl__Group_3__1() throws RecognitionException;
void rule__DictionaryTextControl__Group_3__1__Impl() throws RecognitionException;
void rule__DictionaryTextControl__Group_4__0() throws RecognitionException;
void rule__DictionaryTextControl__Group_4__0__Impl() throws RecognitionException;
void rule__DictionaryTextControl__Group_4__1() throws RecognitionException;
void rule__DictionaryTextControl__Group_4__1__Impl() throws RecognitionException;
void rule__DictionaryTextControl__Group_4__2() throws RecognitionException;
void rule__DictionaryTextControl__Group_4__2__Impl() throws RecognitionException;
void rule__DictionaryIntegerControlInputType__Group__0() throws RecognitionException;
void rule__DictionaryIntegerControlInputType__Group__0__Impl() throws RecognitionException;
void rule__DictionaryIntegerControlInputType__Group__1() throws RecognitionException;
void rule__DictionaryIntegerControlInputType__Group__1__Impl() throws RecognitionException;
void rule__DictionaryIntegerControlInputType__Group__2() throws RecognitionException;
void rule__DictionaryIntegerControlInputType__Group__2__Impl() throws RecognitionException;
void rule__DictionaryIntegerControl__Group__0() throws RecognitionException;
void rule__DictionaryIntegerControl__Group__0__Impl() throws RecognitionException;
void rule__DictionaryIntegerControl__Group__1() throws RecognitionException;
void rule__DictionaryIntegerControl__Group__1__Impl() throws RecognitionException;
void rule__DictionaryIntegerControl__Group__2() throws RecognitionException;
void rule__DictionaryIntegerControl__Group__2__Impl() throws RecognitionException;
void rule__DictionaryIntegerControl__Group__3() throws RecognitionException;
void rule__DictionaryIntegerControl__Group__3__Impl() throws RecognitionException;
void rule__DictionaryIntegerControl__Group__4() throws RecognitionException;
void rule__DictionaryIntegerControl__Group__4__Impl() throws RecognitionException;
void rule__DictionaryIntegerControl__Group_3__0() throws RecognitionException;
void rule__DictionaryIntegerControl__Group_3__0__Impl() throws RecognitionException;
void rule__DictionaryIntegerControl__Group_3__1() throws RecognitionException;
void rule__DictionaryIntegerControl__Group_3__1__Impl() throws RecognitionException;
void rule__DictionaryIntegerControl__Group_4__0() throws RecognitionException;
void rule__DictionaryIntegerControl__Group_4__0__Impl() throws RecognitionException;
void rule__DictionaryIntegerControl__Group_4__1() throws RecognitionException;
void rule__DictionaryIntegerControl__Group_4__1__Impl() throws RecognitionException;
void rule__DictionaryIntegerControl__Group_4__2() throws RecognitionException;
void rule__DictionaryIntegerControl__Group_4__2__Impl() throws RecognitionException;
void rule__DictionaryIntegerControl__Group_4__3() throws RecognitionException;
void rule__DictionaryIntegerControl__Group_4__3__Impl() throws RecognitionException;
void rule__DictionaryBigDecimalControl__Group__0() throws RecognitionException;
void rule__DictionaryBigDecimalControl__Group__0__Impl() throws RecognitionException;
void rule__DictionaryBigDecimalControl__Group__1() throws RecognitionException;
void rule__DictionaryBigDecimalControl__Group__1__Impl() throws RecognitionException;
void rule__DictionaryBigDecimalControl__Group__2() throws RecognitionException;
void rule__DictionaryBigDecimalControl__Group__2__Impl() throws RecognitionException;
void rule__DictionaryBigDecimalControl__Group__3() throws RecognitionException;
void rule__DictionaryBigDecimalControl__Group__3__Impl() throws RecognitionException;
void rule__DictionaryBigDecimalControl__Group__4() throws RecognitionException;
void rule__DictionaryBigDecimalControl__Group__4__Impl() throws RecognitionException;
void rule__DictionaryBigDecimalControl__Group_3__0() throws RecognitionException;
void rule__DictionaryBigDecimalControl__Group_3__0__Impl() throws RecognitionException;
void rule__DictionaryBigDecimalControl__Group_3__1() throws RecognitionException;
void rule__DictionaryBigDecimalControl__Group_3__1__Impl() throws RecognitionException;
void rule__DictionaryBigDecimalControl__Group_4__0() throws RecognitionException;
void rule__DictionaryBigDecimalControl__Group_4__0__Impl() throws RecognitionException;
void rule__DictionaryBigDecimalControl__Group_4__1() throws RecognitionException;
void rule__DictionaryBigDecimalControl__Group_4__1__Impl() throws RecognitionException;
void rule__DictionaryBigDecimalControl__Group_4__2() throws RecognitionException;
void rule__DictionaryBigDecimalControl__Group_4__2__Impl() throws RecognitionException;
void rule__DictionaryBooleanControl__Group__0() throws RecognitionException;
void rule__DictionaryBooleanControl__Group__0__Impl() throws RecognitionException;
void rule__DictionaryBooleanControl__Group__1() throws RecognitionException;
void rule__DictionaryBooleanControl__Group__1__Impl() throws RecognitionException;
void rule__DictionaryBooleanControl__Group__2() throws RecognitionException;
void rule__DictionaryBooleanControl__Group__2__Impl() throws RecognitionException;
void rule__DictionaryBooleanControl__Group__3() throws RecognitionException;
void rule__DictionaryBooleanControl__Group__3__Impl() throws RecognitionException;
void rule__DictionaryBooleanControl__Group__4() throws RecognitionException;
void rule__DictionaryBooleanControl__Group__4__Impl() throws RecognitionException;
void rule__DictionaryBooleanControl__Group_3__0() throws RecognitionException;
void rule__DictionaryBooleanControl__Group_3__0__Impl() throws RecognitionException;
void rule__DictionaryBooleanControl__Group_3__1() throws RecognitionException;
void rule__DictionaryBooleanControl__Group_3__1__Impl() throws RecognitionException;
void rule__DictionaryBooleanControl__Group_4__0() throws RecognitionException;
void rule__DictionaryBooleanControl__Group_4__0__Impl() throws RecognitionException;
void rule__DictionaryBooleanControl__Group_4__1() throws RecognitionException;
void rule__DictionaryBooleanControl__Group_4__1__Impl() throws RecognitionException;
void rule__DictionaryBooleanControl__Group_4__2() throws RecognitionException;
void rule__DictionaryBooleanControl__Group_4__2__Impl() throws RecognitionException;
void rule__DictionaryDateControl__Group__0() throws RecognitionException;
void rule__DictionaryDateControl__Group__0__Impl() throws RecognitionException;
void rule__DictionaryDateControl__Group__1() throws RecognitionException;
void rule__DictionaryDateControl__Group__1__Impl() throws RecognitionException;
void rule__DictionaryDateControl__Group__2() throws RecognitionException;
void rule__DictionaryDateControl__Group__2__Impl() throws RecognitionException;
void rule__DictionaryDateControl__Group__3() throws RecognitionException;
void rule__DictionaryDateControl__Group__3__Impl() throws RecognitionException;
void rule__DictionaryDateControl__Group__4() throws RecognitionException;
void rule__DictionaryDateControl__Group__4__Impl() throws RecognitionException;
void rule__DictionaryDateControl__Group_3__0() throws RecognitionException;
void rule__DictionaryDateControl__Group_3__0__Impl() throws RecognitionException;
void rule__DictionaryDateControl__Group_3__1() throws RecognitionException;
void rule__DictionaryDateControl__Group_3__1__Impl() throws RecognitionException;
void rule__DictionaryDateControl__Group_4__0() throws RecognitionException;
void rule__DictionaryDateControl__Group_4__0__Impl() throws RecognitionException;
void rule__DictionaryDateControl__Group_4__1() throws RecognitionException;
void rule__DictionaryDateControl__Group_4__1__Impl() throws RecognitionException;
void rule__DictionaryDateControl__Group_4__2() throws RecognitionException;
void rule__DictionaryDateControl__Group_4__2__Impl() throws RecognitionException;
void rule__DictionaryEnumerationControl__Group__0() throws RecognitionException;
void rule__DictionaryEnumerationControl__Group__0__Impl() throws RecognitionException;
void rule__DictionaryEnumerationControl__Group__1() throws RecognitionException;
void rule__DictionaryEnumerationControl__Group__1__Impl() throws RecognitionException;
void rule__DictionaryEnumerationControl__Group__2() throws RecognitionException;
void rule__DictionaryEnumerationControl__Group__2__Impl() throws RecognitionException;
void rule__DictionaryEnumerationControl__Group__3() throws RecognitionException;
void rule__DictionaryEnumerationControl__Group__3__Impl() throws RecognitionException;
void rule__DictionaryEnumerationControl__Group__4() throws RecognitionException;
void rule__DictionaryEnumerationControl__Group__4__Impl() throws RecognitionException;
void rule__DictionaryEnumerationControl__Group_3__0() throws RecognitionException;
void rule__DictionaryEnumerationControl__Group_3__0__Impl() throws RecognitionException;
void rule__DictionaryEnumerationControl__Group_3__1() throws RecognitionException;
void rule__DictionaryEnumerationControl__Group_3__1__Impl() throws RecognitionException;
void rule__DictionaryEnumerationControl__Group_4__0() throws RecognitionException;
void rule__DictionaryEnumerationControl__Group_4__0__Impl() throws RecognitionException;
void rule__DictionaryEnumerationControl__Group_4__1() throws RecognitionException;
void rule__DictionaryEnumerationControl__Group_4__1__Impl() throws RecognitionException;
void rule__DictionaryEnumerationControl__Group_4__2() throws RecognitionException;
void rule__DictionaryEnumerationControl__Group_4__2__Impl() throws RecognitionException;
void rule__DictionaryReferenceControl__Group__0() throws RecognitionException;
void rule__DictionaryReferenceControl__Group__0__Impl() throws RecognitionException;
void rule__DictionaryReferenceControl__Group__1() throws RecognitionException;
void rule__DictionaryReferenceControl__Group__1__Impl() throws RecognitionException;
void rule__DictionaryReferenceControl__Group__2() throws RecognitionException;
void rule__DictionaryReferenceControl__Group__2__Impl() throws RecognitionException;
void rule__DictionaryReferenceControl__Group__3() throws RecognitionException;
void rule__DictionaryReferenceControl__Group__3__Impl() throws RecognitionException;
void rule__DictionaryReferenceControl__Group__4() throws RecognitionException;
void rule__DictionaryReferenceControl__Group__4__Impl() throws RecognitionException;
void rule__DictionaryReferenceControl__Group_3__0() throws RecognitionException;
void rule__DictionaryReferenceControl__Group_3__0__Impl() throws RecognitionException;
void rule__DictionaryReferenceControl__Group_3__1() throws RecognitionException;
void rule__DictionaryReferenceControl__Group_3__1__Impl() throws RecognitionException;
void rule__DictionaryReferenceControl__Group_4__0() throws RecognitionException;
void rule__DictionaryReferenceControl__Group_4__0__Impl() throws RecognitionException;
void rule__DictionaryReferenceControl__Group_4__1() throws RecognitionException;
void rule__DictionaryReferenceControl__Group_4__1__Impl() throws RecognitionException;
void rule__DictionaryReferenceControl__Group_4__2() throws RecognitionException;
void rule__DictionaryReferenceControl__Group_4__2__Impl() throws RecognitionException;
void rule__DictionaryReferenceControl__Group_4__3() throws RecognitionException;
void rule__DictionaryReferenceControl__Group_4__3__Impl() throws RecognitionException;
void rule__DictionaryReferenceControl__Group_4__4() throws RecognitionException;
void rule__DictionaryReferenceControl__Group_4__4__Impl() throws RecognitionException;
void rule__DictionaryReferenceControl__Group_4__5() throws RecognitionException;
void rule__DictionaryReferenceControl__Group_4__5__Impl() throws RecognitionException;
void rule__DictionaryReferenceControl__Group_4_2__0() throws RecognitionException;
void rule__DictionaryReferenceControl__Group_4_2__0__Impl() throws RecognitionException;
void rule__DictionaryReferenceControl__Group_4_2__1() throws RecognitionException;
void rule__DictionaryReferenceControl__Group_4_2__1__Impl() throws RecognitionException;
void rule__DictionaryReferenceControl__Group_4_3__0() throws RecognitionException;
void rule__DictionaryReferenceControl__Group_4_3__0__Impl() throws RecognitionException;
void rule__DictionaryReferenceControl__Group_4_3__1() throws RecognitionException;
void rule__DictionaryReferenceControl__Group_4_3__1__Impl() throws RecognitionException;
void rule__DictionaryReferenceControl__Group_4_4__0() throws RecognitionException;
void rule__DictionaryReferenceControl__Group_4_4__0__Impl() throws RecognitionException;
void rule__DictionaryReferenceControl__Group_4_4__1() throws RecognitionException;
void rule__DictionaryReferenceControl__Group_4_4__1__Impl() throws RecognitionException;
void rule__DictionaryReferenceControl__Group_4_4__2() throws RecognitionException;
void rule__DictionaryReferenceControl__Group_4_4__2__Impl() throws RecognitionException;
void rule__DictionaryReferenceControl__Group_4_4__3() throws RecognitionException;
void rule__DictionaryReferenceControl__Group_4_4__3__Impl() throws RecognitionException;
void rule__DictionaryFileControl__Group__0() throws RecognitionException;
void rule__DictionaryFileControl__Group__0__Impl() throws RecognitionException;
void rule__DictionaryFileControl__Group__1() throws RecognitionException;
void rule__DictionaryFileControl__Group__1__Impl() throws RecognitionException;
void rule__DictionaryFileControl__Group__2() throws RecognitionException;
void rule__DictionaryFileControl__Group__2__Impl() throws RecognitionException;
void rule__DictionaryFileControl__Group__3() throws RecognitionException;
void rule__DictionaryFileControl__Group__3__Impl() throws RecognitionException;
void rule__DictionaryFileControl__Group__4() throws RecognitionException;
void rule__DictionaryFileControl__Group__4__Impl() throws RecognitionException;
void rule__DictionaryFileControl__Group_3__0() throws RecognitionException;
void rule__DictionaryFileControl__Group_3__0__Impl() throws RecognitionException;
void rule__DictionaryFileControl__Group_3__1() throws RecognitionException;
void rule__DictionaryFileControl__Group_3__1__Impl() throws RecognitionException;
void rule__DictionaryFileControl__Group_4__0() throws RecognitionException;
void rule__DictionaryFileControl__Group_4__0__Impl() throws RecognitionException;
void rule__DictionaryFileControl__Group_4__1() throws RecognitionException;
void rule__DictionaryFileControl__Group_4__1__Impl() throws RecognitionException;
void rule__DictionaryFileControl__Group_4__2() throws RecognitionException;
void rule__DictionaryFileControl__Group_4__2__Impl() throws RecognitionException;
void rule__Module__Group__0() throws RecognitionException;
void rule__Module__Group__0__Impl() throws RecognitionException;
void rule__Module__Group__1() throws RecognitionException;
void rule__Module__Group__1__Impl() throws RecognitionException;
void rule__Module__Group__2() throws RecognitionException;
void rule__Module__Group__2__Impl() throws RecognitionException;
void rule__Module__Group__3() throws RecognitionException;
void rule__Module__Group__3__Impl() throws RecognitionException;
void rule__Module__Group__4() throws RecognitionException;
void rule__Module__Group__4__Impl() throws RecognitionException;
void rule__Module__Group__5() throws RecognitionException;
void rule__Module__Group__5__Impl() throws RecognitionException;
void rule__Module__Group__6() throws RecognitionException;
void rule__Module__Group__6__Impl() throws RecognitionException;
void rule__Module__Group_5__0() throws RecognitionException;
void rule__Module__Group_5__0__Impl() throws RecognitionException;
void rule__Module__Group_5__1() throws RecognitionException;
void rule__Module__Group_5__1__Impl() throws RecognitionException;
void rule__Module__Group_5__2() throws RecognitionException;
void rule__Module__Group_5__2__Impl() throws RecognitionException;
void rule__Module__Group_5__3() throws RecognitionException;
void rule__Module__Group_5__3__Impl() throws RecognitionException;
void rule__ModuleParameter__Group__0() throws RecognitionException;
void rule__ModuleParameter__Group__0__Impl() throws RecognitionException;
void rule__ModuleParameter__Group__1() throws RecognitionException;
void rule__ModuleParameter__Group__1__Impl() throws RecognitionException;
void rule__ModuleParameter__Group__2() throws RecognitionException;
void rule__ModuleParameter__Group__2__Impl() throws RecognitionException;
void rule__ModuleDefinition__Group__0() throws RecognitionException;
void rule__ModuleDefinition__Group__0__Impl() throws RecognitionException;
void rule__ModuleDefinition__Group__1() throws RecognitionException;
void rule__ModuleDefinition__Group__1__Impl() throws RecognitionException;
void rule__ModuleDefinition__Group__2() throws RecognitionException;
void rule__ModuleDefinition__Group__2__Impl() throws RecognitionException;
void rule__ModuleDefinition__Group__3() throws RecognitionException;
void rule__ModuleDefinition__Group__3__Impl() throws RecognitionException;
void rule__ModuleDefinition__Group__4() throws RecognitionException;
void rule__ModuleDefinition__Group__4__Impl() throws RecognitionException;
void rule__ModuleDefinition__Group_3__0() throws RecognitionException;
void rule__ModuleDefinition__Group_3__0__Impl() throws RecognitionException;
void rule__ModuleDefinition__Group_3__1() throws RecognitionException;
void rule__ModuleDefinition__Group_3__1__Impl() throws RecognitionException;
void rule__ModuleDefinition__Group_3__2() throws RecognitionException;
void rule__ModuleDefinition__Group_3__2__Impl() throws RecognitionException;
void rule__ModuleDefinition__Group_3__3() throws RecognitionException;
void rule__ModuleDefinition__Group_3__3__Impl() throws RecognitionException;
void rule__ModuleDefinitionParameter__Group__0() throws RecognitionException;
void rule__ModuleDefinitionParameter__Group__0__Impl() throws RecognitionException;
void rule__ModuleDefinitionParameter__Group__1() throws RecognitionException;
void rule__ModuleDefinitionParameter__Group__1__Impl() throws RecognitionException;
void rule__ModuleDefinitionParameter__Group__2() throws RecognitionException;
void rule__ModuleDefinitionParameter__Group__2__Impl() throws RecognitionException;
void rule__ModuleDefinitionParameter__Group__3() throws RecognitionException;
void rule__ModuleDefinitionParameter__Group__3__Impl() throws RecognitionException;
void rule__ModuleDefinitionParameter__Group__4() throws RecognitionException;
void rule__ModuleDefinitionParameter__Group__4__Impl() throws RecognitionException;
void rule__ModuleDefinitionParameter__Group__5() throws RecognitionException;
void rule__ModuleDefinitionParameter__Group__5__Impl() throws RecognitionException;
void rule__ServiceOptions__Group__0() throws RecognitionException;
void rule__ServiceOptions__Group__0__Impl() throws RecognitionException;
void rule__ServiceOptions__Group__1() throws RecognitionException;
void rule__ServiceOptions__Group__1__Impl() throws RecognitionException;
void rule__ServiceMethod__Group__0() throws RecognitionException;
void rule__ServiceMethod__Group__0__Impl() throws RecognitionException;
void rule__ServiceMethod__Group__1() throws RecognitionException;
void rule__ServiceMethod__Group__1__Impl() throws RecognitionException;
void rule__ServiceMethod__Group__2() throws RecognitionException;
void rule__ServiceMethod__Group__2__Impl() throws RecognitionException;
void rule__ServiceMethod__Group__3() throws RecognitionException;
void rule__ServiceMethod__Group__3__Impl() throws RecognitionException;
void rule__ServiceMethod__Group__4() throws RecognitionException;
void rule__ServiceMethod__Group__4__Impl() throws RecognitionException;
void rule__ServiceMethod__Group__5() throws RecognitionException;
void rule__ServiceMethod__Group__5__Impl() throws RecognitionException;
void rule__ServiceMethod__Group__6() throws RecognitionException;
void rule__ServiceMethod__Group__6__Impl() throws RecognitionException;
void rule__ServiceMethod__Group_1__0() throws RecognitionException;
void rule__ServiceMethod__Group_1__0__Impl() throws RecognitionException;
void rule__ServiceMethod__Group_1__1() throws RecognitionException;
void rule__ServiceMethod__Group_1__1__Impl() throws RecognitionException;
void rule__ServiceMethod__Group_1__2() throws RecognitionException;
void rule__ServiceMethod__Group_1__2__Impl() throws RecognitionException;
void rule__ServiceMethod__Group_5__0() throws RecognitionException;
void rule__ServiceMethod__Group_5__0__Impl() throws RecognitionException;
void rule__ServiceMethod__Group_5__1() throws RecognitionException;
void rule__ServiceMethod__Group_5__1__Impl() throws RecognitionException;
void rule__ServiceMethod__Group_5_1__0() throws RecognitionException;
void rule__ServiceMethod__Group_5_1__0__Impl() throws RecognitionException;
void rule__ServiceMethod__Group_5_1__1() throws RecognitionException;
void rule__ServiceMethod__Group_5_1__1__Impl() throws RecognitionException;
void rule__Service__Group__0() throws RecognitionException;
void rule__Service__Group__0__Impl() throws RecognitionException;
void rule__Service__Group__1() throws RecognitionException;
void rule__Service__Group__1__Impl() throws RecognitionException;
void rule__Service__Group__2() throws RecognitionException;
void rule__Service__Group__2__Impl() throws RecognitionException;
void rule__Service__Group__3() throws RecognitionException;
void rule__Service__Group__3__Impl() throws RecognitionException;
void rule__Service__Group__4() throws RecognitionException;
void rule__Service__Group__4__Impl() throws RecognitionException;
void rule__Service__Group__5() throws RecognitionException;
void rule__Service__Group__5__Impl() throws RecognitionException;
void rule__Service__Group__6() throws RecognitionException;
void rule__Service__Group__6__Impl() throws RecognitionException;
void rule__Service__Group_4__0() throws RecognitionException;
void rule__Service__Group_4__0__Impl() throws RecognitionException;
void rule__Service__Group_4__1() throws RecognitionException;
void rule__Service__Group_4__1__Impl() throws RecognitionException;
void rule__Service__Group_4__2() throws RecognitionException;
void rule__Service__Group_4__2__Impl() throws RecognitionException;
void rule__Service__Group_4__3() throws RecognitionException;
void rule__Service__Group_4__3__Impl() throws RecognitionException;
void rule__NavigationNode__Group__0() throws RecognitionException;
void rule__NavigationNode__Group__0__Impl() throws RecognitionException;
void rule__NavigationNode__Group__1() throws RecognitionException;
void rule__NavigationNode__Group__1__Impl() throws RecognitionException;
void rule__NavigationNode__Group__2() throws RecognitionException;
void rule__NavigationNode__Group__2__Impl() throws RecognitionException;
void rule__NavigationNode__Group__3() throws RecognitionException;
void rule__NavigationNode__Group__3__Impl() throws RecognitionException;
void rule__NavigationNode__Group__4() throws RecognitionException;
void rule__NavigationNode__Group__4__Impl() throws RecognitionException;
void rule__NavigationNode__Group__5() throws RecognitionException;
void rule__NavigationNode__Group__5__Impl() throws RecognitionException;
void rule__NavigationNode__Group__6() throws RecognitionException;
void rule__NavigationNode__Group__6__Impl() throws RecognitionException;
void rule__NavigationNode__Group__7() throws RecognitionException;
void rule__NavigationNode__Group__7__Impl() throws RecognitionException;
void rule__NavigationNode__Group__8() throws RecognitionException;
void rule__NavigationNode__Group__8__Impl() throws RecognitionException;
void rule__NavigationNode__Group__9() throws RecognitionException;
void rule__NavigationNode__Group__9__Impl() throws RecognitionException;
void rule__NavigationNode__Group_3__0() throws RecognitionException;
void rule__NavigationNode__Group_3__0__Impl() throws RecognitionException;
void rule__NavigationNode__Group_3__1() throws RecognitionException;
void rule__NavigationNode__Group_3__1__Impl() throws RecognitionException;
void rule__NavigationNode__Group_4__0() throws RecognitionException;
void rule__NavigationNode__Group_4__0__Impl() throws RecognitionException;
void rule__NavigationNode__Group_4__1() throws RecognitionException;
void rule__NavigationNode__Group_4__1__Impl() throws RecognitionException;
void rule__NavigationNode__Group_5__0() throws RecognitionException;
void rule__NavigationNode__Group_5__0__Impl() throws RecognitionException;
void rule__NavigationNode__Group_5__1() throws RecognitionException;
void rule__NavigationNode__Group_5__1__Impl() throws RecognitionException;
void rule__NavigationNode__Group_6__0() throws RecognitionException;
void rule__NavigationNode__Group_6__0__Impl() throws RecognitionException;
void rule__NavigationNode__Group_6__1() throws RecognitionException;
void rule__NavigationNode__Group_6__1__Impl() throws RecognitionException;
void rule__NavigationNode__Group_7__0() throws RecognitionException;
void rule__NavigationNode__Group_7__0__Impl() throws RecognitionException;
void rule__NavigationNode__Group_7__1() throws RecognitionException;
void rule__NavigationNode__Group_7__1__Impl() throws RecognitionException;
void rule__XAssignment__Group_0__0() throws RecognitionException;
void rule__XAssignment__Group_0__0__Impl() throws RecognitionException;
void rule__XAssignment__Group_0__1() throws RecognitionException;
void rule__XAssignment__Group_0__1__Impl() throws RecognitionException;
void rule__XAssignment__Group_0__2() throws RecognitionException;
void rule__XAssignment__Group_0__2__Impl() throws RecognitionException;
void rule__XAssignment__Group_0__3() throws RecognitionException;
void rule__XAssignment__Group_0__3__Impl() throws RecognitionException;
void rule__XAssignment__Group_1__0() throws RecognitionException;
void rule__XAssignment__Group_1__0__Impl() throws RecognitionException;
void rule__XAssignment__Group_1__1() throws RecognitionException;
void rule__XAssignment__Group_1__1__Impl() throws RecognitionException;
void rule__XAssignment__Group_1_1__0() throws RecognitionException;
void rule__XAssignment__Group_1_1__0__Impl() throws RecognitionException;
void rule__XAssignment__Group_1_1__1() throws RecognitionException;
void rule__XAssignment__Group_1_1__1__Impl() throws RecognitionException;
void rule__XAssignment__Group_1_1_0__0() throws RecognitionException;
void rule__XAssignment__Group_1_1_0__0__Impl() throws RecognitionException;
void rule__XAssignment__Group_1_1_0_0__0() throws RecognitionException;
void rule__XAssignment__Group_1_1_0_0__0__Impl() throws RecognitionException;
void rule__XAssignment__Group_1_1_0_0__1() throws RecognitionException;
void rule__XAssignment__Group_1_1_0_0__1__Impl() throws RecognitionException;
void rule__OpMultiAssign__Group_5__0() throws RecognitionException;
void rule__OpMultiAssign__Group_5__0__Impl() throws RecognitionException;
void rule__OpMultiAssign__Group_5__1() throws RecognitionException;
void rule__OpMultiAssign__Group_5__1__Impl() throws RecognitionException;
void rule__OpMultiAssign__Group_5__2() throws RecognitionException;
void rule__OpMultiAssign__Group_5__2__Impl() throws RecognitionException;
void rule__OpMultiAssign__Group_6__0() throws RecognitionException;
void rule__OpMultiAssign__Group_6__0__Impl() throws RecognitionException;
void rule__OpMultiAssign__Group_6__1() throws RecognitionException;
void rule__OpMultiAssign__Group_6__1__Impl() throws RecognitionException;
void rule__OpMultiAssign__Group_6__2() throws RecognitionException;
void rule__OpMultiAssign__Group_6__2__Impl() throws RecognitionException;
void rule__XOrExpression__Group__0() throws RecognitionException;
void rule__XOrExpression__Group__0__Impl() throws RecognitionException;
void rule__XOrExpression__Group__1() throws RecognitionException;
void rule__XOrExpression__Group__1__Impl() throws RecognitionException;
void rule__XOrExpression__Group_1__0() throws RecognitionException;
void rule__XOrExpression__Group_1__0__Impl() throws RecognitionException;
void rule__XOrExpression__Group_1__1() throws RecognitionException;
void rule__XOrExpression__Group_1__1__Impl() throws RecognitionException;
void rule__XOrExpression__Group_1_0__0() throws RecognitionException;
void rule__XOrExpression__Group_1_0__0__Impl() throws RecognitionException;
void rule__XOrExpression__Group_1_0_0__0() throws RecognitionException;
void rule__XOrExpression__Group_1_0_0__0__Impl() throws RecognitionException;
void rule__XOrExpression__Group_1_0_0__1() throws RecognitionException;
void rule__XOrExpression__Group_1_0_0__1__Impl() throws RecognitionException;
void rule__XAndExpression__Group__0() throws RecognitionException;
void rule__XAndExpression__Group__0__Impl() throws RecognitionException;
void rule__XAndExpression__Group__1() throws RecognitionException;
void rule__XAndExpression__Group__1__Impl() throws RecognitionException;
void rule__XAndExpression__Group_1__0() throws RecognitionException;
void rule__XAndExpression__Group_1__0__Impl() throws RecognitionException;
void rule__XAndExpression__Group_1__1() throws RecognitionException;
void rule__XAndExpression__Group_1__1__Impl() throws RecognitionException;
void rule__XAndExpression__Group_1_0__0() throws RecognitionException;
void rule__XAndExpression__Group_1_0__0__Impl() throws RecognitionException;
void rule__XAndExpression__Group_1_0_0__0() throws RecognitionException;
void rule__XAndExpression__Group_1_0_0__0__Impl() throws RecognitionException;
void rule__XAndExpression__Group_1_0_0__1() throws RecognitionException;
void rule__XAndExpression__Group_1_0_0__1__Impl() throws RecognitionException;
void rule__XEqualityExpression__Group__0() throws RecognitionException;
void rule__XEqualityExpression__Group__0__Impl() throws RecognitionException;
void rule__XEqualityExpression__Group__1() throws RecognitionException;
void rule__XEqualityExpression__Group__1__Impl() throws RecognitionException;
void rule__XEqualityExpression__Group_1__0() throws RecognitionException;
void rule__XEqualityExpression__Group_1__0__Impl() throws RecognitionException;
void rule__XEqualityExpression__Group_1__1() throws RecognitionException;
void rule__XEqualityExpression__Group_1__1__Impl() throws RecognitionException;
void rule__XEqualityExpression__Group_1_0__0() throws RecognitionException;
void rule__XEqualityExpression__Group_1_0__0__Impl() throws RecognitionException;
void rule__XEqualityExpression__Group_1_0_0__0() throws RecognitionException;
void rule__XEqualityExpression__Group_1_0_0__0__Impl() throws RecognitionException;
void rule__XEqualityExpression__Group_1_0_0__1() throws RecognitionException;
void rule__XEqualityExpression__Group_1_0_0__1__Impl() throws RecognitionException;
void rule__XRelationalExpression__Group__0() throws RecognitionException;
void rule__XRelationalExpression__Group__0__Impl() throws RecognitionException;
void rule__XRelationalExpression__Group__1() throws RecognitionException;
void rule__XRelationalExpression__Group__1__Impl() throws RecognitionException;
void rule__XRelationalExpression__Group_1_0__0() throws RecognitionException;
void rule__XRelationalExpression__Group_1_0__0__Impl() throws RecognitionException;
void rule__XRelationalExpression__Group_1_0__1() throws RecognitionException;
void rule__XRelationalExpression__Group_1_0__1__Impl() throws RecognitionException;
void rule__XRelationalExpression__Group_1_0_0__0() throws RecognitionException;
void rule__XRelationalExpression__Group_1_0_0__0__Impl() throws RecognitionException;
void rule__XRelationalExpression__Group_1_0_0_0__0() throws RecognitionException;
void rule__XRelationalExpression__Group_1_0_0_0__0__Impl() throws RecognitionException;
void rule__XRelationalExpression__Group_1_0_0_0__1() throws RecognitionException;
void rule__XRelationalExpression__Group_1_0_0_0__1__Impl() throws RecognitionException;
void rule__XRelationalExpression__Group_1_1__0() throws RecognitionException;
void rule__XRelationalExpression__Group_1_1__0__Impl() throws RecognitionException;
void rule__XRelationalExpression__Group_1_1__1() throws RecognitionException;
void rule__XRelationalExpression__Group_1_1__1__Impl() throws RecognitionException;
void rule__XRelationalExpression__Group_1_1_0__0() throws RecognitionException;
void rule__XRelationalExpression__Group_1_1_0__0__Impl() throws RecognitionException;
void rule__XRelationalExpression__Group_1_1_0_0__0() throws RecognitionException;
void rule__XRelationalExpression__Group_1_1_0_0__0__Impl() throws RecognitionException;
void rule__XRelationalExpression__Group_1_1_0_0__1() throws RecognitionException;
void rule__XRelationalExpression__Group_1_1_0_0__1__Impl() throws RecognitionException;
void rule__OpCompare__Group_1__0() throws RecognitionException;
void rule__OpCompare__Group_1__0__Impl() throws RecognitionException;
void rule__OpCompare__Group_1__1() throws RecognitionException;
void rule__OpCompare__Group_1__1__Impl() throws RecognitionException;
void rule__XOtherOperatorExpression__Group__0() throws RecognitionException;
void rule__XOtherOperatorExpression__Group__0__Impl() throws RecognitionException;
void rule__XOtherOperatorExpression__Group__1() throws RecognitionException;
void rule__XOtherOperatorExpression__Group__1__Impl() throws RecognitionException;
void rule__XOtherOperatorExpression__Group_1__0() throws RecognitionException;
void rule__XOtherOperatorExpression__Group_1__0__Impl() throws RecognitionException;
void rule__XOtherOperatorExpression__Group_1__1() throws RecognitionException;
void rule__XOtherOperatorExpression__Group_1__1__Impl() throws RecognitionException;
void rule__XOtherOperatorExpression__Group_1_0__0() throws RecognitionException;
void rule__XOtherOperatorExpression__Group_1_0__0__Impl() throws RecognitionException;
void rule__XOtherOperatorExpression__Group_1_0_0__0() throws RecognitionException;
void rule__XOtherOperatorExpression__Group_1_0_0__0__Impl() throws RecognitionException;
void rule__XOtherOperatorExpression__Group_1_0_0__1() throws RecognitionException;
void rule__XOtherOperatorExpression__Group_1_0_0__1__Impl() throws RecognitionException;
void rule__OpOther__Group_2__0() throws RecognitionException;
void rule__OpOther__Group_2__0__Impl() throws RecognitionException;
void rule__OpOther__Group_2__1() throws RecognitionException;
void rule__OpOther__Group_2__1__Impl() throws RecognitionException;
void rule__OpOther__Group_5__0() throws RecognitionException;
void rule__OpOther__Group_5__0__Impl() throws RecognitionException;
void rule__OpOther__Group_5__1() throws RecognitionException;
void rule__OpOther__Group_5__1__Impl() throws RecognitionException;
void rule__OpOther__Group_5_1_0__0() throws RecognitionException;
void rule__OpOther__Group_5_1_0__0__Impl() throws RecognitionException;
void rule__OpOther__Group_5_1_0_0__0() throws RecognitionException;
void rule__OpOther__Group_5_1_0_0__0__Impl() throws RecognitionException;
void rule__OpOther__Group_5_1_0_0__1() throws RecognitionException;
void rule__OpOther__Group_5_1_0_0__1__Impl() throws RecognitionException;
void rule__OpOther__Group_6__0() throws RecognitionException;
void rule__OpOther__Group_6__0__Impl() throws RecognitionException;
void rule__OpOther__Group_6__1() throws RecognitionException;
void rule__OpOther__Group_6__1__Impl() throws RecognitionException;
void rule__OpOther__Group_6_1_0__0() throws RecognitionException;
void rule__OpOther__Group_6_1_0__0__Impl() throws RecognitionException;
void rule__OpOther__Group_6_1_0_0__0() throws RecognitionException;
void rule__OpOther__Group_6_1_0_0__0__Impl() throws RecognitionException;
void rule__OpOther__Group_6_1_0_0__1() throws RecognitionException;
void rule__OpOther__Group_6_1_0_0__1__Impl() throws RecognitionException;
void rule__XAdditiveExpression__Group__0() throws RecognitionException;
void rule__XAdditiveExpression__Group__0__Impl() throws RecognitionException;
void rule__XAdditiveExpression__Group__1() throws RecognitionException;
void rule__XAdditiveExpression__Group__1__Impl() throws RecognitionException;
void rule__XAdditiveExpression__Group_1__0() throws RecognitionException;
void rule__XAdditiveExpression__Group_1__0__Impl() throws RecognitionException;
void rule__XAdditiveExpression__Group_1__1() throws RecognitionException;
void rule__XAdditiveExpression__Group_1__1__Impl() throws RecognitionException;
void rule__XAdditiveExpression__Group_1_0__0() throws RecognitionException;
void rule__XAdditiveExpression__Group_1_0__0__Impl() throws RecognitionException;
void rule__XAdditiveExpression__Group_1_0_0__0() throws RecognitionException;
void rule__XAdditiveExpression__Group_1_0_0__0__Impl() throws RecognitionException;
void rule__XAdditiveExpression__Group_1_0_0__1() throws RecognitionException;
void rule__XAdditiveExpression__Group_1_0_0__1__Impl() throws RecognitionException;
void rule__XMultiplicativeExpression__Group__0() throws RecognitionException;
void rule__XMultiplicativeExpression__Group__0__Impl() throws RecognitionException;
void rule__XMultiplicativeExpression__Group__1() throws RecognitionException;
void rule__XMultiplicativeExpression__Group__1__Impl() throws RecognitionException;
void rule__XMultiplicativeExpression__Group_1__0() throws RecognitionException;
void rule__XMultiplicativeExpression__Group_1__0__Impl() throws RecognitionException;
void rule__XMultiplicativeExpression__Group_1__1() throws RecognitionException;
void rule__XMultiplicativeExpression__Group_1__1__Impl() throws RecognitionException;
void rule__XMultiplicativeExpression__Group_1_0__0() throws RecognitionException;
void rule__XMultiplicativeExpression__Group_1_0__0__Impl() throws RecognitionException;
void rule__XMultiplicativeExpression__Group_1_0_0__0() throws RecognitionException;
void rule__XMultiplicativeExpression__Group_1_0_0__0__Impl() throws RecognitionException;
void rule__XMultiplicativeExpression__Group_1_0_0__1() throws RecognitionException;
void rule__XMultiplicativeExpression__Group_1_0_0__1__Impl() throws RecognitionException;
void rule__XUnaryOperation__Group_0__0() throws RecognitionException;
void rule__XUnaryOperation__Group_0__0__Impl() throws RecognitionException;
void rule__XUnaryOperation__Group_0__1() throws RecognitionException;
void rule__XUnaryOperation__Group_0__1__Impl() throws RecognitionException;
void rule__XUnaryOperation__Group_0__2() throws RecognitionException;
void rule__XUnaryOperation__Group_0__2__Impl() throws RecognitionException;
void rule__XCastedExpression__Group__0() throws RecognitionException;
void rule__XCastedExpression__Group__0__Impl() throws RecognitionException;
void rule__XCastedExpression__Group__1() throws RecognitionException;
void rule__XCastedExpression__Group__1__Impl() throws RecognitionException;
void rule__XCastedExpression__Group_1__0() throws RecognitionException;
void rule__XCastedExpression__Group_1__0__Impl() throws RecognitionException;
void rule__XCastedExpression__Group_1__1() throws RecognitionException;
void rule__XCastedExpression__Group_1__1__Impl() throws RecognitionException;
void rule__XCastedExpression__Group_1_0__0() throws RecognitionException;
void rule__XCastedExpression__Group_1_0__0__Impl() throws RecognitionException;
void rule__XCastedExpression__Group_1_0_0__0() throws RecognitionException;
void rule__XCastedExpression__Group_1_0_0__0__Impl() throws RecognitionException;
void rule__XCastedExpression__Group_1_0_0__1() throws RecognitionException;
void rule__XCastedExpression__Group_1_0_0__1__Impl() throws RecognitionException;
void rule__XPostfixOperation__Group__0() throws RecognitionException;
void rule__XPostfixOperation__Group__0__Impl() throws RecognitionException;
void rule__XPostfixOperation__Group__1() throws RecognitionException;
void rule__XPostfixOperation__Group__1__Impl() throws RecognitionException;
void rule__XPostfixOperation__Group_1__0() throws RecognitionException;
void rule__XPostfixOperation__Group_1__0__Impl() throws RecognitionException;
void rule__XPostfixOperation__Group_1_0__0() throws RecognitionException;
void rule__XPostfixOperation__Group_1_0__0__Impl() throws RecognitionException;
void rule__XPostfixOperation__Group_1_0__1() throws RecognitionException;
void rule__XPostfixOperation__Group_1_0__1__Impl() throws RecognitionException;
void rule__XMemberFeatureCall__Group__0() throws RecognitionException;
void rule__XMemberFeatureCall__Group__0__Impl() throws RecognitionException;
void rule__XMemberFeatureCall__Group__1() throws RecognitionException;
void rule__XMemberFeatureCall__Group__1__Impl() throws RecognitionException;
void rule__XMemberFeatureCall__Group_1_0__0() throws RecognitionException;
void rule__XMemberFeatureCall__Group_1_0__0__Impl() throws RecognitionException;
void rule__XMemberFeatureCall__Group_1_0__1() throws RecognitionException;
void rule__XMemberFeatureCall__Group_1_0__1__Impl() throws RecognitionException;
void rule__XMemberFeatureCall__Group_1_0_0__0() throws RecognitionException;
void rule__XMemberFeatureCall__Group_1_0_0__0__Impl() throws RecognitionException;
void rule__XMemberFeatureCall__Group_1_0_0_0__0() throws RecognitionException;
void rule__XMemberFeatureCall__Group_1_0_0_0__0__Impl() throws RecognitionException;
void rule__XMemberFeatureCall__Group_1_0_0_0__1() throws RecognitionException;
void rule__XMemberFeatureCall__Group_1_0_0_0__1__Impl() throws RecognitionException;
void rule__XMemberFeatureCall__Group_1_0_0_0__2() throws RecognitionException;
void rule__XMemberFeatureCall__Group_1_0_0_0__2__Impl() throws RecognitionException;
void rule__XMemberFeatureCall__Group_1_0_0_0__3() throws RecognitionException;
void rule__XMemberFeatureCall__Group_1_0_0_0__3__Impl() throws RecognitionException;
void rule__XMemberFeatureCall__Group_1_1__0() throws RecognitionException;
void rule__XMemberFeatureCall__Group_1_1__0__Impl() throws RecognitionException;
void rule__XMemberFeatureCall__Group_1_1__1() throws RecognitionException;
void rule__XMemberFeatureCall__Group_1_1__1__Impl() throws RecognitionException;
void rule__XMemberFeatureCall__Group_1_1__2() throws RecognitionException;
void rule__XMemberFeatureCall__Group_1_1__2__Impl() throws RecognitionException;
void rule__XMemberFeatureCall__Group_1_1__3() throws RecognitionException;
void rule__XMemberFeatureCall__Group_1_1__3__Impl() throws RecognitionException;
void rule__XMemberFeatureCall__Group_1_1__4() throws RecognitionException;
void rule__XMemberFeatureCall__Group_1_1__4__Impl() throws RecognitionException;
void rule__XMemberFeatureCall__Group_1_1_0__0() throws RecognitionException;
void rule__XMemberFeatureCall__Group_1_1_0__0__Impl() throws RecognitionException;
void rule__XMemberFeatureCall__Group_1_1_0_0__0() throws RecognitionException;
void rule__XMemberFeatureCall__Group_1_1_0_0__0__Impl() throws RecognitionException;
void rule__XMemberFeatureCall__Group_1_1_0_0__1() throws RecognitionException;
void rule__XMemberFeatureCall__Group_1_1_0_0__1__Impl() throws RecognitionException;
void rule__XMemberFeatureCall__Group_1_1_1__0() throws RecognitionException;
void rule__XMemberFeatureCall__Group_1_1_1__0__Impl() throws RecognitionException;
void rule__XMemberFeatureCall__Group_1_1_1__1() throws RecognitionException;
void rule__XMemberFeatureCall__Group_1_1_1__1__Impl() throws RecognitionException;
void rule__XMemberFeatureCall__Group_1_1_1__2() throws RecognitionException;
void rule__XMemberFeatureCall__Group_1_1_1__2__Impl() throws RecognitionException;
void rule__XMemberFeatureCall__Group_1_1_1__3() throws RecognitionException;
void rule__XMemberFeatureCall__Group_1_1_1__3__Impl() throws RecognitionException;
void rule__XMemberFeatureCall__Group_1_1_1_2__0() throws RecognitionException;
void rule__XMemberFeatureCall__Group_1_1_1_2__0__Impl() throws RecognitionException;
void rule__XMemberFeatureCall__Group_1_1_1_2__1() throws RecognitionException;
void rule__XMemberFeatureCall__Group_1_1_1_2__1__Impl() throws RecognitionException;
void rule__XMemberFeatureCall__Group_1_1_3__0() throws RecognitionException;
void rule__XMemberFeatureCall__Group_1_1_3__0__Impl() throws RecognitionException;
void rule__XMemberFeatureCall__Group_1_1_3__1() throws RecognitionException;
void rule__XMemberFeatureCall__Group_1_1_3__1__Impl() throws RecognitionException;
void rule__XMemberFeatureCall__Group_1_1_3__2() throws RecognitionException;
void rule__XMemberFeatureCall__Group_1_1_3__2__Impl() throws RecognitionException;
void rule__XMemberFeatureCall__Group_1_1_3_1_1__0() throws RecognitionException;
void rule__XMemberFeatureCall__Group_1_1_3_1_1__0__Impl() throws RecognitionException;
void rule__XMemberFeatureCall__Group_1_1_3_1_1__1() throws RecognitionException;
void rule__XMemberFeatureCall__Group_1_1_3_1_1__1__Impl() throws RecognitionException;
void rule__XMemberFeatureCall__Group_1_1_3_1_1_1__0() throws RecognitionException;
void rule__XMemberFeatureCall__Group_1_1_3_1_1_1__0__Impl() throws RecognitionException;
void rule__XMemberFeatureCall__Group_1_1_3_1_1_1__1() throws RecognitionException;
void rule__XMemberFeatureCall__Group_1_1_3_1_1_1__1__Impl() throws RecognitionException;
void rule__XSetLiteral__Group__0() throws RecognitionException;
void rule__XSetLiteral__Group__0__Impl() throws RecognitionException;
void rule__XSetLiteral__Group__1() throws RecognitionException;
void rule__XSetLiteral__Group__1__Impl() throws RecognitionException;
void rule__XSetLiteral__Group__2() throws RecognitionException;
void rule__XSetLiteral__Group__2__Impl() throws RecognitionException;
void rule__XSetLiteral__Group__3() throws RecognitionException;
void rule__XSetLiteral__Group__3__Impl() throws RecognitionException;
void rule__XSetLiteral__Group__4() throws RecognitionException;
void rule__XSetLiteral__Group__4__Impl() throws RecognitionException;
void rule__XSetLiteral__Group_3__0() throws RecognitionException;
void rule__XSetLiteral__Group_3__0__Impl() throws RecognitionException;
void rule__XSetLiteral__Group_3__1() throws RecognitionException;
void rule__XSetLiteral__Group_3__1__Impl() throws RecognitionException;
void rule__XSetLiteral__Group_3_1__0() throws RecognitionException;
void rule__XSetLiteral__Group_3_1__0__Impl() throws RecognitionException;
void rule__XSetLiteral__Group_3_1__1() throws RecognitionException;
void rule__XSetLiteral__Group_3_1__1__Impl() throws RecognitionException;
void rule__XListLiteral__Group__0() throws RecognitionException;
void rule__XListLiteral__Group__0__Impl() throws RecognitionException;
void rule__XListLiteral__Group__1() throws RecognitionException;
void rule__XListLiteral__Group__1__Impl() throws RecognitionException;
void rule__XListLiteral__Group__2() throws RecognitionException;
void rule__XListLiteral__Group__2__Impl() throws RecognitionException;
void rule__XListLiteral__Group__3() throws RecognitionException;
void rule__XListLiteral__Group__3__Impl() throws RecognitionException;
void rule__XListLiteral__Group__4() throws RecognitionException;
void rule__XListLiteral__Group__4__Impl() throws RecognitionException;
void rule__XListLiteral__Group_3__0() throws RecognitionException;
void rule__XListLiteral__Group_3__0__Impl() throws RecognitionException;
void rule__XListLiteral__Group_3__1() throws RecognitionException;
void rule__XListLiteral__Group_3__1__Impl() throws RecognitionException;
void rule__XListLiteral__Group_3_1__0() throws RecognitionException;
void rule__XListLiteral__Group_3_1__0__Impl() throws RecognitionException;
void rule__XListLiteral__Group_3_1__1() throws RecognitionException;
void rule__XListLiteral__Group_3_1__1__Impl() throws RecognitionException;
void rule__XClosure__Group__0() throws RecognitionException;
void rule__XClosure__Group__0__Impl() throws RecognitionException;
void rule__XClosure__Group__1() throws RecognitionException;
void rule__XClosure__Group__1__Impl() throws RecognitionException;
void rule__XClosure__Group__2() throws RecognitionException;
void rule__XClosure__Group__2__Impl() throws RecognitionException;
void rule__XClosure__Group__3() throws RecognitionException;
void rule__XClosure__Group__3__Impl() throws RecognitionException;
void rule__XClosure__Group_0__0() throws RecognitionException;
void rule__XClosure__Group_0__0__Impl() throws RecognitionException;
void rule__XClosure__Group_0_0__0() throws RecognitionException;
void rule__XClosure__Group_0_0__0__Impl() throws RecognitionException;
void rule__XClosure__Group_0_0__1() throws RecognitionException;
void rule__XClosure__Group_0_0__1__Impl() throws RecognitionException;
void rule__XClosure__Group_1__0() throws RecognitionException;
void rule__XClosure__Group_1__0__Impl() throws RecognitionException;
void rule__XClosure__Group_1_0__0() throws RecognitionException;
void rule__XClosure__Group_1_0__0__Impl() throws RecognitionException;
void rule__XClosure__Group_1_0__1() throws RecognitionException;
void rule__XClosure__Group_1_0__1__Impl() throws RecognitionException;
void rule__XClosure__Group_1_0_0__0() throws RecognitionException;
void rule__XClosure__Group_1_0_0__0__Impl() throws RecognitionException;
void rule__XClosure__Group_1_0_0__1() throws RecognitionException;
void rule__XClosure__Group_1_0_0__1__Impl() throws RecognitionException;
void rule__XClosure__Group_1_0_0_1__0() throws RecognitionException;
void rule__XClosure__Group_1_0_0_1__0__Impl() throws RecognitionException;
void rule__XClosure__Group_1_0_0_1__1() throws RecognitionException;
void rule__XClosure__Group_1_0_0_1__1__Impl() throws RecognitionException;
void rule__XExpressionInClosure__Group__0() throws RecognitionException;
void rule__XExpressionInClosure__Group__0__Impl() throws RecognitionException;
void rule__XExpressionInClosure__Group__1() throws RecognitionException;
void rule__XExpressionInClosure__Group__1__Impl() throws RecognitionException;
void rule__XExpressionInClosure__Group_1__0() throws RecognitionException;
void rule__XExpressionInClosure__Group_1__0__Impl() throws RecognitionException;
void rule__XExpressionInClosure__Group_1__1() throws RecognitionException;
void rule__XExpressionInClosure__Group_1__1__Impl() throws RecognitionException;
void rule__XShortClosure__Group__0() throws RecognitionException;
void rule__XShortClosure__Group__0__Impl() throws RecognitionException;
void rule__XShortClosure__Group__1() throws RecognitionException;
void rule__XShortClosure__Group__1__Impl() throws RecognitionException;
void rule__XShortClosure__Group_0__0() throws RecognitionException;
void rule__XShortClosure__Group_0__0__Impl() throws RecognitionException;
void rule__XShortClosure__Group_0_0__0() throws RecognitionException;
void rule__XShortClosure__Group_0_0__0__Impl() throws RecognitionException;
void rule__XShortClosure__Group_0_0__1() throws RecognitionException;
void rule__XShortClosure__Group_0_0__1__Impl() throws RecognitionException;
void rule__XShortClosure__Group_0_0__2() throws RecognitionException;
void rule__XShortClosure__Group_0_0__2__Impl() throws RecognitionException;
void rule__XShortClosure__Group_0_0_1__0() throws RecognitionException;
void rule__XShortClosure__Group_0_0_1__0__Impl() throws RecognitionException;
void rule__XShortClosure__Group_0_0_1__1() throws RecognitionException;
void rule__XShortClosure__Group_0_0_1__1__Impl() throws RecognitionException;
void rule__XShortClosure__Group_0_0_1_1__0() throws RecognitionException;
void rule__XShortClosure__Group_0_0_1_1__0__Impl() throws RecognitionException;
void rule__XShortClosure__Group_0_0_1_1__1() throws RecognitionException;
void rule__XShortClosure__Group_0_0_1_1__1__Impl() throws RecognitionException;
void rule__XParenthesizedExpression__Group__0() throws RecognitionException;
void rule__XParenthesizedExpression__Group__0__Impl() throws RecognitionException;
void rule__XParenthesizedExpression__Group__1() throws RecognitionException;
void rule__XParenthesizedExpression__Group__1__Impl() throws RecognitionException;
void rule__XParenthesizedExpression__Group__2() throws RecognitionException;
void rule__XParenthesizedExpression__Group__2__Impl() throws RecognitionException;
void rule__XIfExpression__Group__0() throws RecognitionException;
void rule__XIfExpression__Group__0__Impl() throws RecognitionException;
void rule__XIfExpression__Group__1() throws RecognitionException;
void rule__XIfExpression__Group__1__Impl() throws RecognitionException;
void rule__XIfExpression__Group__2() throws RecognitionException;
void rule__XIfExpression__Group__2__Impl() throws RecognitionException;
void rule__XIfExpression__Group__3() throws RecognitionException;
void rule__XIfExpression__Group__3__Impl() throws RecognitionException;
void rule__XIfExpression__Group__4() throws RecognitionException;
void rule__XIfExpression__Group__4__Impl() throws RecognitionException;
void rule__XIfExpression__Group__5() throws RecognitionException;
void rule__XIfExpression__Group__5__Impl() throws RecognitionException;
void rule__XIfExpression__Group__6() throws RecognitionException;
void rule__XIfExpression__Group__6__Impl() throws RecognitionException;
void rule__XIfExpression__Group_6__0() throws RecognitionException;
void rule__XIfExpression__Group_6__0__Impl() throws RecognitionException;
void rule__XIfExpression__Group_6__1() throws RecognitionException;
void rule__XIfExpression__Group_6__1__Impl() throws RecognitionException;
void rule__XSwitchExpression__Group__0() throws RecognitionException;
void rule__XSwitchExpression__Group__0__Impl() throws RecognitionException;
void rule__XSwitchExpression__Group__1() throws RecognitionException;
void rule__XSwitchExpression__Group__1__Impl() throws RecognitionException;
void rule__XSwitchExpression__Group__2() throws RecognitionException;
void rule__XSwitchExpression__Group__2__Impl() throws RecognitionException;
void rule__XSwitchExpression__Group__3() throws RecognitionException;
void rule__XSwitchExpression__Group__3__Impl() throws RecognitionException;
void rule__XSwitchExpression__Group__4() throws RecognitionException;
void rule__XSwitchExpression__Group__4__Impl() throws RecognitionException;
void rule__XSwitchExpression__Group__5() throws RecognitionException;
void rule__XSwitchExpression__Group__5__Impl() throws RecognitionException;
void rule__XSwitchExpression__Group__6() throws RecognitionException;
void rule__XSwitchExpression__Group__6__Impl() throws RecognitionException;
void rule__XSwitchExpression__Group_2_0__0() throws RecognitionException;
void rule__XSwitchExpression__Group_2_0__0__Impl() throws RecognitionException;
void rule__XSwitchExpression__Group_2_0__1() throws RecognitionException;
void rule__XSwitchExpression__Group_2_0__1__Impl() throws RecognitionException;
void rule__XSwitchExpression__Group_2_0__2() throws RecognitionException;
void rule__XSwitchExpression__Group_2_0__2__Impl() throws RecognitionException;
void rule__XSwitchExpression__Group_2_0_0__0() throws RecognitionException;
void rule__XSwitchExpression__Group_2_0_0__0__Impl() throws RecognitionException;
void rule__XSwitchExpression__Group_2_0_0_0__0() throws RecognitionException;
void rule__XSwitchExpression__Group_2_0_0_0__0__Impl() throws RecognitionException;
void rule__XSwitchExpression__Group_2_0_0_0__1() throws RecognitionException;
void rule__XSwitchExpression__Group_2_0_0_0__1__Impl() throws RecognitionException;
void rule__XSwitchExpression__Group_2_0_0_0__2() throws RecognitionException;
void rule__XSwitchExpression__Group_2_0_0_0__2__Impl() throws RecognitionException;
void rule__XSwitchExpression__Group_2_1__0() throws RecognitionException;
void rule__XSwitchExpression__Group_2_1__0__Impl() throws RecognitionException;
void rule__XSwitchExpression__Group_2_1__1() throws RecognitionException;
void rule__XSwitchExpression__Group_2_1__1__Impl() throws RecognitionException;
void rule__XSwitchExpression__Group_2_1_0__0() throws RecognitionException;
void rule__XSwitchExpression__Group_2_1_0__0__Impl() throws RecognitionException;
void rule__XSwitchExpression__Group_2_1_0_0__0() throws RecognitionException;
void rule__XSwitchExpression__Group_2_1_0_0__0__Impl() throws RecognitionException;
void rule__XSwitchExpression__Group_2_1_0_0__1() throws RecognitionException;
void rule__XSwitchExpression__Group_2_1_0_0__1__Impl() throws RecognitionException;
void rule__XSwitchExpression__Group_5__0() throws RecognitionException;
void rule__XSwitchExpression__Group_5__0__Impl() throws RecognitionException;
void rule__XSwitchExpression__Group_5__1() throws RecognitionException;
void rule__XSwitchExpression__Group_5__1__Impl() throws RecognitionException;
void rule__XSwitchExpression__Group_5__2() throws RecognitionException;
void rule__XSwitchExpression__Group_5__2__Impl() throws RecognitionException;
void rule__XCasePart__Group__0() throws RecognitionException;
void rule__XCasePart__Group__0__Impl() throws RecognitionException;
void rule__XCasePart__Group__1() throws RecognitionException;
void rule__XCasePart__Group__1__Impl() throws RecognitionException;
void rule__XCasePart__Group__2() throws RecognitionException;
void rule__XCasePart__Group__2__Impl() throws RecognitionException;
void rule__XCasePart__Group__3() throws RecognitionException;
void rule__XCasePart__Group__3__Impl() throws RecognitionException;
void rule__XCasePart__Group_2__0() throws RecognitionException;
void rule__XCasePart__Group_2__0__Impl() throws RecognitionException;
void rule__XCasePart__Group_2__1() throws RecognitionException;
void rule__XCasePart__Group_2__1__Impl() throws RecognitionException;
void rule__XCasePart__Group_3_0__0() throws RecognitionException;
void rule__XCasePart__Group_3_0__0__Impl() throws RecognitionException;
void rule__XCasePart__Group_3_0__1() throws RecognitionException;
void rule__XCasePart__Group_3_0__1__Impl() throws RecognitionException;
void rule__XForLoopExpression__Group__0() throws RecognitionException;
void rule__XForLoopExpression__Group__0__Impl() throws RecognitionException;
void rule__XForLoopExpression__Group__1() throws RecognitionException;
void rule__XForLoopExpression__Group__1__Impl() throws RecognitionException;
void rule__XForLoopExpression__Group__2() throws RecognitionException;
void rule__XForLoopExpression__Group__2__Impl() throws RecognitionException;
void rule__XForLoopExpression__Group__3() throws RecognitionException;
void rule__XForLoopExpression__Group__3__Impl() throws RecognitionException;
void rule__XForLoopExpression__Group_0__0() throws RecognitionException;
void rule__XForLoopExpression__Group_0__0__Impl() throws RecognitionException;
void rule__XForLoopExpression__Group_0_0__0() throws RecognitionException;
void rule__XForLoopExpression__Group_0_0__0__Impl() throws RecognitionException;
void rule__XForLoopExpression__Group_0_0__1() throws RecognitionException;
void rule__XForLoopExpression__Group_0_0__1__Impl() throws RecognitionException;
void rule__XForLoopExpression__Group_0_0__2() throws RecognitionException;
void rule__XForLoopExpression__Group_0_0__2__Impl() throws RecognitionException;
void rule__XForLoopExpression__Group_0_0__3() throws RecognitionException;
void rule__XForLoopExpression__Group_0_0__3__Impl() throws RecognitionException;
void rule__XForLoopExpression__Group_0_0__4() throws RecognitionException;
void rule__XForLoopExpression__Group_0_0__4__Impl() throws RecognitionException;
void rule__XBasicForLoopExpression__Group__0() throws RecognitionException;
void rule__XBasicForLoopExpression__Group__0__Impl() throws RecognitionException;
void rule__XBasicForLoopExpression__Group__1() throws RecognitionException;
void rule__XBasicForLoopExpression__Group__1__Impl() throws RecognitionException;
void rule__XBasicForLoopExpression__Group__2() throws RecognitionException;
void rule__XBasicForLoopExpression__Group__2__Impl() throws RecognitionException;
void rule__XBasicForLoopExpression__Group__3() throws RecognitionException;
void rule__XBasicForLoopExpression__Group__3__Impl() throws RecognitionException;
void rule__XBasicForLoopExpression__Group__4() throws RecognitionException;
void rule__XBasicForLoopExpression__Group__4__Impl() throws RecognitionException;
void rule__XBasicForLoopExpression__Group__5() throws RecognitionException;
void rule__XBasicForLoopExpression__Group__5__Impl() throws RecognitionException;
void rule__XBasicForLoopExpression__Group__6() throws RecognitionException;
void rule__XBasicForLoopExpression__Group__6__Impl() throws RecognitionException;
void rule__XBasicForLoopExpression__Group__7() throws RecognitionException;
void rule__XBasicForLoopExpression__Group__7__Impl() throws RecognitionException;
void rule__XBasicForLoopExpression__Group__8() throws RecognitionException;
void rule__XBasicForLoopExpression__Group__8__Impl() throws RecognitionException;
void rule__XBasicForLoopExpression__Group__9() throws RecognitionException;
void rule__XBasicForLoopExpression__Group__9__Impl() throws RecognitionException;
void rule__XBasicForLoopExpression__Group_3__0() throws RecognitionException;
void rule__XBasicForLoopExpression__Group_3__0__Impl() throws RecognitionException;
void rule__XBasicForLoopExpression__Group_3__1() throws RecognitionException;
void rule__XBasicForLoopExpression__Group_3__1__Impl() throws RecognitionException;
void rule__XBasicForLoopExpression__Group_3_1__0() throws RecognitionException;
void rule__XBasicForLoopExpression__Group_3_1__0__Impl() throws RecognitionException;
void rule__XBasicForLoopExpression__Group_3_1__1() throws RecognitionException;
void rule__XBasicForLoopExpression__Group_3_1__1__Impl() throws RecognitionException;
void rule__XBasicForLoopExpression__Group_7__0() throws RecognitionException;
void rule__XBasicForLoopExpression__Group_7__0__Impl() throws RecognitionException;
void rule__XBasicForLoopExpression__Group_7__1() throws RecognitionException;
void rule__XBasicForLoopExpression__Group_7__1__Impl() throws RecognitionException;
void rule__XBasicForLoopExpression__Group_7_1__0() throws RecognitionException;
void rule__XBasicForLoopExpression__Group_7_1__0__Impl() throws RecognitionException;
void rule__XBasicForLoopExpression__Group_7_1__1() throws RecognitionException;
void rule__XBasicForLoopExpression__Group_7_1__1__Impl() throws RecognitionException;
void rule__XWhileExpression__Group__0() throws RecognitionException;
void rule__XWhileExpression__Group__0__Impl() throws RecognitionException;
void rule__XWhileExpression__Group__1() throws RecognitionException;
void rule__XWhileExpression__Group__1__Impl() throws RecognitionException;
void rule__XWhileExpression__Group__2() throws RecognitionException;
void rule__XWhileExpression__Group__2__Impl() throws RecognitionException;
void rule__XWhileExpression__Group__3() throws RecognitionException;
void rule__XWhileExpression__Group__3__Impl() throws RecognitionException;
void rule__XWhileExpression__Group__4() throws RecognitionException;
void rule__XWhileExpression__Group__4__Impl() throws RecognitionException;
void rule__XWhileExpression__Group__5() throws RecognitionException;
void rule__XWhileExpression__Group__5__Impl() throws RecognitionException;
void rule__XDoWhileExpression__Group__0() throws RecognitionException;
void rule__XDoWhileExpression__Group__0__Impl() throws RecognitionException;
void rule__XDoWhileExpression__Group__1() throws RecognitionException;
void rule__XDoWhileExpression__Group__1__Impl() throws RecognitionException;
void rule__XDoWhileExpression__Group__2() throws RecognitionException;
void rule__XDoWhileExpression__Group__2__Impl() throws RecognitionException;
void rule__XDoWhileExpression__Group__3() throws RecognitionException;
void rule__XDoWhileExpression__Group__3__Impl() throws RecognitionException;
void rule__XDoWhileExpression__Group__4() throws RecognitionException;
void rule__XDoWhileExpression__Group__4__Impl() throws RecognitionException;
void rule__XDoWhileExpression__Group__5() throws RecognitionException;
void rule__XDoWhileExpression__Group__5__Impl() throws RecognitionException;
void rule__XDoWhileExpression__Group__6() throws RecognitionException;
void rule__XDoWhileExpression__Group__6__Impl() throws RecognitionException;
void rule__XBlockExpression__Group__0() throws RecognitionException;
void rule__XBlockExpression__Group__0__Impl() throws RecognitionException;
void rule__XBlockExpression__Group__1() throws RecognitionException;
void rule__XBlockExpression__Group__1__Impl() throws RecognitionException;
void rule__XBlockExpression__Group__2() throws RecognitionException;
void rule__XBlockExpression__Group__2__Impl() throws RecognitionException;
void rule__XBlockExpression__Group__3() throws RecognitionException;
void rule__XBlockExpression__Group__3__Impl() throws RecognitionException;
void rule__XBlockExpression__Group_2__0() throws RecognitionException;
void rule__XBlockExpression__Group_2__0__Impl() throws RecognitionException;
void rule__XBlockExpression__Group_2__1() throws RecognitionException;
void rule__XBlockExpression__Group_2__1__Impl() throws RecognitionException;
void rule__XVariableDeclaration__Group__0() throws RecognitionException;
void rule__XVariableDeclaration__Group__0__Impl() throws RecognitionException;
void rule__XVariableDeclaration__Group__1() throws RecognitionException;
void rule__XVariableDeclaration__Group__1__Impl() throws RecognitionException;
void rule__XVariableDeclaration__Group__2() throws RecognitionException;
void rule__XVariableDeclaration__Group__2__Impl() throws RecognitionException;
void rule__XVariableDeclaration__Group__3() throws RecognitionException;
void rule__XVariableDeclaration__Group__3__Impl() throws RecognitionException;
void rule__XVariableDeclaration__Group_2_0__0() throws RecognitionException;
void rule__XVariableDeclaration__Group_2_0__0__Impl() throws RecognitionException;
void rule__XVariableDeclaration__Group_2_0_0__0() throws RecognitionException;
void rule__XVariableDeclaration__Group_2_0_0__0__Impl() throws RecognitionException;
void rule__XVariableDeclaration__Group_2_0_0__1() throws RecognitionException;
void rule__XVariableDeclaration__Group_2_0_0__1__Impl() throws RecognitionException;
void rule__XVariableDeclaration__Group_3__0() throws RecognitionException;
void rule__XVariableDeclaration__Group_3__0__Impl() throws RecognitionException;
void rule__XVariableDeclaration__Group_3__1() throws RecognitionException;
void rule__XVariableDeclaration__Group_3__1__Impl() throws RecognitionException;
void rule__JvmFormalParameter__Group__0() throws RecognitionException;
void rule__JvmFormalParameter__Group__0__Impl() throws RecognitionException;
void rule__JvmFormalParameter__Group__1() throws RecognitionException;
void rule__JvmFormalParameter__Group__1__Impl() throws RecognitionException;
void rule__FullJvmFormalParameter__Group__0() throws RecognitionException;
void rule__FullJvmFormalParameter__Group__0__Impl() throws RecognitionException;
void rule__FullJvmFormalParameter__Group__1() throws RecognitionException;
void rule__FullJvmFormalParameter__Group__1__Impl() throws RecognitionException;
void rule__XFeatureCall__Group__0() throws RecognitionException;
void rule__XFeatureCall__Group__0__Impl() throws RecognitionException;
void rule__XFeatureCall__Group__1() throws RecognitionException;
void rule__XFeatureCall__Group__1__Impl() throws RecognitionException;
void rule__XFeatureCall__Group__2() throws RecognitionException;
void rule__XFeatureCall__Group__2__Impl() throws RecognitionException;
void rule__XFeatureCall__Group__3() throws RecognitionException;
void rule__XFeatureCall__Group__3__Impl() throws RecognitionException;
void rule__XFeatureCall__Group__4() throws RecognitionException;
void rule__XFeatureCall__Group__4__Impl() throws RecognitionException;
void rule__XFeatureCall__Group_1__0() throws RecognitionException;
void rule__XFeatureCall__Group_1__0__Impl() throws RecognitionException;
void rule__XFeatureCall__Group_1__1() throws RecognitionException;
void rule__XFeatureCall__Group_1__1__Impl() throws RecognitionException;
void rule__XFeatureCall__Group_1__2() throws RecognitionException;
void rule__XFeatureCall__Group_1__2__Impl() throws RecognitionException;
void rule__XFeatureCall__Group_1__3() throws RecognitionException;
void rule__XFeatureCall__Group_1__3__Impl() throws RecognitionException;
void rule__XFeatureCall__Group_1_2__0() throws RecognitionException;
void rule__XFeatureCall__Group_1_2__0__Impl() throws RecognitionException;
void rule__XFeatureCall__Group_1_2__1() throws RecognitionException;
void rule__XFeatureCall__Group_1_2__1__Impl() throws RecognitionException;
void rule__XFeatureCall__Group_3__0() throws RecognitionException;
void rule__XFeatureCall__Group_3__0__Impl() throws RecognitionException;
void rule__XFeatureCall__Group_3__1() throws RecognitionException;
void rule__XFeatureCall__Group_3__1__Impl() throws RecognitionException;
void rule__XFeatureCall__Group_3__2() throws RecognitionException;
void rule__XFeatureCall__Group_3__2__Impl() throws RecognitionException;
void rule__XFeatureCall__Group_3_1_1__0() throws RecognitionException;
void rule__XFeatureCall__Group_3_1_1__0__Impl() throws RecognitionException;
void rule__XFeatureCall__Group_3_1_1__1() throws RecognitionException;
void rule__XFeatureCall__Group_3_1_1__1__Impl() throws RecognitionException;
void rule__XFeatureCall__Group_3_1_1_1__0() throws RecognitionException;
void rule__XFeatureCall__Group_3_1_1_1__0__Impl() throws RecognitionException;
void rule__XFeatureCall__Group_3_1_1_1__1() throws RecognitionException;
void rule__XFeatureCall__Group_3_1_1_1__1__Impl() throws RecognitionException;
void rule__XConstructorCall__Group__0() throws RecognitionException;
void rule__XConstructorCall__Group__0__Impl() throws RecognitionException;
void rule__XConstructorCall__Group__1() throws RecognitionException;
void rule__XConstructorCall__Group__1__Impl() throws RecognitionException;
void rule__XConstructorCall__Group__2() throws RecognitionException;
void rule__XConstructorCall__Group__2__Impl() throws RecognitionException;
void rule__XConstructorCall__Group__3() throws RecognitionException;
void rule__XConstructorCall__Group__3__Impl() throws RecognitionException;
void rule__XConstructorCall__Group__4() throws RecognitionException;
void rule__XConstructorCall__Group__4__Impl() throws RecognitionException;
void rule__XConstructorCall__Group__5() throws RecognitionException;
void rule__XConstructorCall__Group__5__Impl() throws RecognitionException;
void rule__XConstructorCall__Group_3__0() throws RecognitionException;
void rule__XConstructorCall__Group_3__0__Impl() throws RecognitionException;
void rule__XConstructorCall__Group_3__1() throws RecognitionException;
void rule__XConstructorCall__Group_3__1__Impl() throws RecognitionException;
void rule__XConstructorCall__Group_3__2() throws RecognitionException;
void rule__XConstructorCall__Group_3__2__Impl() throws RecognitionException;
void rule__XConstructorCall__Group_3__3() throws RecognitionException;
void rule__XConstructorCall__Group_3__3__Impl() throws RecognitionException;
void rule__XConstructorCall__Group_3_2__0() throws RecognitionException;
void rule__XConstructorCall__Group_3_2__0__Impl() throws RecognitionException;
void rule__XConstructorCall__Group_3_2__1() throws RecognitionException;
void rule__XConstructorCall__Group_3_2__1__Impl() throws RecognitionException;
void rule__XConstructorCall__Group_4__0() throws RecognitionException;
void rule__XConstructorCall__Group_4__0__Impl() throws RecognitionException;
void rule__XConstructorCall__Group_4__1() throws RecognitionException;
void rule__XConstructorCall__Group_4__1__Impl() throws RecognitionException;
void rule__XConstructorCall__Group_4__2() throws RecognitionException;
void rule__XConstructorCall__Group_4__2__Impl() throws RecognitionException;
void rule__XConstructorCall__Group_4_1_1__0() throws RecognitionException;
void rule__XConstructorCall__Group_4_1_1__0__Impl() throws RecognitionException;
void rule__XConstructorCall__Group_4_1_1__1() throws RecognitionException;
void rule__XConstructorCall__Group_4_1_1__1__Impl() throws RecognitionException;
void rule__XConstructorCall__Group_4_1_1_1__0() throws RecognitionException;
void rule__XConstructorCall__Group_4_1_1_1__0__Impl() throws RecognitionException;
void rule__XConstructorCall__Group_4_1_1_1__1() throws RecognitionException;
void rule__XConstructorCall__Group_4_1_1_1__1__Impl() throws RecognitionException;
void rule__XBooleanLiteral__Group__0() throws RecognitionException;
void rule__XBooleanLiteral__Group__0__Impl() throws RecognitionException;
void rule__XBooleanLiteral__Group__1() throws RecognitionException;
void rule__XBooleanLiteral__Group__1__Impl() throws RecognitionException;
void rule__XNullLiteral__Group__0() throws RecognitionException;
void rule__XNullLiteral__Group__0__Impl() throws RecognitionException;
void rule__XNullLiteral__Group__1() throws RecognitionException;
void rule__XNullLiteral__Group__1__Impl() throws RecognitionException;
void rule__XNumberLiteral__Group__0() throws RecognitionException;
void rule__XNumberLiteral__Group__0__Impl() throws RecognitionException;
void rule__XNumberLiteral__Group__1() throws RecognitionException;
void rule__XNumberLiteral__Group__1__Impl() throws RecognitionException;
void rule__XStringLiteral__Group__0() throws RecognitionException;
void rule__XStringLiteral__Group__0__Impl() throws RecognitionException;
void rule__XStringLiteral__Group__1() throws RecognitionException;
void rule__XStringLiteral__Group__1__Impl() throws RecognitionException;
void rule__XTypeLiteral__Group__0() throws RecognitionException;
void rule__XTypeLiteral__Group__0__Impl() throws RecognitionException;
void rule__XTypeLiteral__Group__1() throws RecognitionException;
void rule__XTypeLiteral__Group__1__Impl() throws RecognitionException;
void rule__XTypeLiteral__Group__2() throws RecognitionException;
void rule__XTypeLiteral__Group__2__Impl() throws RecognitionException;
void rule__XTypeLiteral__Group__3() throws RecognitionException;
void rule__XTypeLiteral__Group__3__Impl() throws RecognitionException;
void rule__XTypeLiteral__Group__4() throws RecognitionException;
void rule__XTypeLiteral__Group__4__Impl() throws RecognitionException;
void rule__XTypeLiteral__Group__5() throws RecognitionException;
void rule__XTypeLiteral__Group__5__Impl() throws RecognitionException;
void rule__XThrowExpression__Group__0() throws RecognitionException;
void rule__XThrowExpression__Group__0__Impl() throws RecognitionException;
void rule__XThrowExpression__Group__1() throws RecognitionException;
void rule__XThrowExpression__Group__1__Impl() throws RecognitionException;
void rule__XThrowExpression__Group__2() throws RecognitionException;
void rule__XThrowExpression__Group__2__Impl() throws RecognitionException;
void rule__XReturnExpression__Group__0() throws RecognitionException;
void rule__XReturnExpression__Group__0__Impl() throws RecognitionException;
void rule__XReturnExpression__Group__1() throws RecognitionException;
void rule__XReturnExpression__Group__1__Impl() throws RecognitionException;
void rule__XReturnExpression__Group__2() throws RecognitionException;
void rule__XReturnExpression__Group__2__Impl() throws RecognitionException;
void rule__XTryCatchFinallyExpression__Group__0() throws RecognitionException;
void rule__XTryCatchFinallyExpression__Group__0__Impl() throws RecognitionException;
void rule__XTryCatchFinallyExpression__Group__1() throws RecognitionException;
void rule__XTryCatchFinallyExpression__Group__1__Impl() throws RecognitionException;
void rule__XTryCatchFinallyExpression__Group__2() throws RecognitionException;
void rule__XTryCatchFinallyExpression__Group__2__Impl() throws RecognitionException;
void rule__XTryCatchFinallyExpression__Group__3() throws RecognitionException;
void rule__XTryCatchFinallyExpression__Group__3__Impl() throws RecognitionException;
void rule__XTryCatchFinallyExpression__Group_3_0__0() throws RecognitionException;
void rule__XTryCatchFinallyExpression__Group_3_0__0__Impl() throws RecognitionException;
void rule__XTryCatchFinallyExpression__Group_3_0__1() throws RecognitionException;
void rule__XTryCatchFinallyExpression__Group_3_0__1__Impl() throws RecognitionException;
void rule__XTryCatchFinallyExpression__Group_3_0_1__0() throws RecognitionException;
void rule__XTryCatchFinallyExpression__Group_3_0_1__0__Impl() throws RecognitionException;
void rule__XTryCatchFinallyExpression__Group_3_0_1__1() throws RecognitionException;
void rule__XTryCatchFinallyExpression__Group_3_0_1__1__Impl() throws RecognitionException;
void rule__XTryCatchFinallyExpression__Group_3_1__0() throws RecognitionException;
void rule__XTryCatchFinallyExpression__Group_3_1__0__Impl() throws RecognitionException;
void rule__XTryCatchFinallyExpression__Group_3_1__1() throws RecognitionException;
void rule__XTryCatchFinallyExpression__Group_3_1__1__Impl() throws RecognitionException;
void rule__XSynchronizedExpression__Group__0() throws RecognitionException;
void rule__XSynchronizedExpression__Group__0__Impl() throws RecognitionException;
void rule__XSynchronizedExpression__Group__1() throws RecognitionException;
void rule__XSynchronizedExpression__Group__1__Impl() throws RecognitionException;
void rule__XSynchronizedExpression__Group__2() throws RecognitionException;
void rule__XSynchronizedExpression__Group__2__Impl() throws RecognitionException;
void rule__XSynchronizedExpression__Group__3() throws RecognitionException;
void rule__XSynchronizedExpression__Group__3__Impl() throws RecognitionException;
void rule__XSynchronizedExpression__Group_0__0() throws RecognitionException;
void rule__XSynchronizedExpression__Group_0__0__Impl() throws RecognitionException;
void rule__XSynchronizedExpression__Group_0_0__0() throws RecognitionException;
void rule__XSynchronizedExpression__Group_0_0__0__Impl() throws RecognitionException;
void rule__XSynchronizedExpression__Group_0_0__1() throws RecognitionException;
void rule__XSynchronizedExpression__Group_0_0__1__Impl() throws RecognitionException;
void rule__XSynchronizedExpression__Group_0_0__2() throws RecognitionException;
void rule__XSynchronizedExpression__Group_0_0__2__Impl() throws RecognitionException;
void rule__XCatchClause__Group__0() throws RecognitionException;
void rule__XCatchClause__Group__0__Impl() throws RecognitionException;
void rule__XCatchClause__Group__1() throws RecognitionException;
void rule__XCatchClause__Group__1__Impl() throws RecognitionException;
void rule__XCatchClause__Group__2() throws RecognitionException;
void rule__XCatchClause__Group__2__Impl() throws RecognitionException;
void rule__XCatchClause__Group__3() throws RecognitionException;
void rule__XCatchClause__Group__3__Impl() throws RecognitionException;
void rule__XCatchClause__Group__4() throws RecognitionException;
void rule__XCatchClause__Group__4__Impl() throws RecognitionException;
void rule__QualifiedName__Group__0() throws RecognitionException;
void rule__QualifiedName__Group__0__Impl() throws RecognitionException;
void rule__QualifiedName__Group__1() throws RecognitionException;
void rule__QualifiedName__Group__1__Impl() throws RecognitionException;
void rule__QualifiedName__Group_1__0() throws RecognitionException;
void rule__QualifiedName__Group_1__0__Impl() throws RecognitionException;
void rule__QualifiedName__Group_1__1() throws RecognitionException;
void rule__QualifiedName__Group_1__1__Impl() throws RecognitionException;
void rule__Number__Group_1__0() throws RecognitionException;
void rule__Number__Group_1__0__Impl() throws RecognitionException;
void rule__Number__Group_1__1() throws RecognitionException;
void rule__Number__Group_1__1__Impl() throws RecognitionException;
void rule__Number__Group_1_1__0() throws RecognitionException;
void rule__Number__Group_1_1__0__Impl() throws RecognitionException;
void rule__Number__Group_1_1__1() throws RecognitionException;
void rule__Number__Group_1_1__1__Impl() throws RecognitionException;
void rule__JvmTypeReference__Group_0__0() throws RecognitionException;
void rule__JvmTypeReference__Group_0__0__Impl() throws RecognitionException;
void rule__JvmTypeReference__Group_0__1() throws RecognitionException;
void rule__JvmTypeReference__Group_0__1__Impl() throws RecognitionException;
void rule__JvmTypeReference__Group_0_1__0() throws RecognitionException;
void rule__JvmTypeReference__Group_0_1__0__Impl() throws RecognitionException;
void rule__JvmTypeReference__Group_0_1_0__0() throws RecognitionException;
void rule__JvmTypeReference__Group_0_1_0__0__Impl() throws RecognitionException;
void rule__JvmTypeReference__Group_0_1_0__1() throws RecognitionException;
void rule__JvmTypeReference__Group_0_1_0__1__Impl() throws RecognitionException;
void rule__ArrayBrackets__Group__0() throws RecognitionException;
void rule__ArrayBrackets__Group__0__Impl() throws RecognitionException;
void rule__ArrayBrackets__Group__1() throws RecognitionException;
void rule__ArrayBrackets__Group__1__Impl() throws RecognitionException;
void rule__XFunctionTypeRef__Group__0() throws RecognitionException;
void rule__XFunctionTypeRef__Group__0__Impl() throws RecognitionException;
void rule__XFunctionTypeRef__Group__1() throws RecognitionException;
void rule__XFunctionTypeRef__Group__1__Impl() throws RecognitionException;
void rule__XFunctionTypeRef__Group__2() throws RecognitionException;
void rule__XFunctionTypeRef__Group__2__Impl() throws RecognitionException;
void rule__XFunctionTypeRef__Group_0__0() throws RecognitionException;
void rule__XFunctionTypeRef__Group_0__0__Impl() throws RecognitionException;
void rule__XFunctionTypeRef__Group_0__1() throws RecognitionException;
void rule__XFunctionTypeRef__Group_0__1__Impl() throws RecognitionException;
void rule__XFunctionTypeRef__Group_0__2() throws RecognitionException;
void rule__XFunctionTypeRef__Group_0__2__Impl() throws RecognitionException;
void rule__XFunctionTypeRef__Group_0_1__0() throws RecognitionException;
void rule__XFunctionTypeRef__Group_0_1__0__Impl() throws RecognitionException;
void rule__XFunctionTypeRef__Group_0_1__1() throws RecognitionException;
void rule__XFunctionTypeRef__Group_0_1__1__Impl() throws RecognitionException;
void rule__XFunctionTypeRef__Group_0_1_1__0() throws RecognitionException;
void rule__XFunctionTypeRef__Group_0_1_1__0__Impl() throws RecognitionException;
void rule__XFunctionTypeRef__Group_0_1_1__1() throws RecognitionException;
void rule__XFunctionTypeRef__Group_0_1_1__1__Impl() throws RecognitionException;
void rule__JvmParameterizedTypeReference__Group__0() throws RecognitionException;
void rule__JvmParameterizedTypeReference__Group__0__Impl() throws RecognitionException;
void rule__JvmParameterizedTypeReference__Group__1() throws RecognitionException;
void rule__JvmParameterizedTypeReference__Group__1__Impl() throws RecognitionException;
void rule__JvmParameterizedTypeReference__Group_1__0() throws RecognitionException;
void rule__JvmParameterizedTypeReference__Group_1__0__Impl() throws RecognitionException;
void rule__JvmParameterizedTypeReference__Group_1__1() throws RecognitionException;
void rule__JvmParameterizedTypeReference__Group_1__1__Impl() throws RecognitionException;
void rule__JvmParameterizedTypeReference__Group_1__2() throws RecognitionException;
void rule__JvmParameterizedTypeReference__Group_1__2__Impl() throws RecognitionException;
void rule__JvmParameterizedTypeReference__Group_1__3() throws RecognitionException;
void rule__JvmParameterizedTypeReference__Group_1__3__Impl() throws RecognitionException;
void rule__JvmParameterizedTypeReference__Group_1__4() throws RecognitionException;
void rule__JvmParameterizedTypeReference__Group_1__4__Impl() throws RecognitionException;
void rule__JvmParameterizedTypeReference__Group_1_2__0() throws RecognitionException;
void rule__JvmParameterizedTypeReference__Group_1_2__0__Impl() throws RecognitionException;
void rule__JvmParameterizedTypeReference__Group_1_2__1() throws RecognitionException;
void rule__JvmParameterizedTypeReference__Group_1_2__1__Impl() throws RecognitionException;
void rule__JvmParameterizedTypeReference__Group_1_4__0() throws RecognitionException;
void rule__JvmParameterizedTypeReference__Group_1_4__0__Impl() throws RecognitionException;
void rule__JvmParameterizedTypeReference__Group_1_4__1() throws RecognitionException;
void rule__JvmParameterizedTypeReference__Group_1_4__1__Impl() throws RecognitionException;
void rule__JvmParameterizedTypeReference__Group_1_4__2() throws RecognitionException;
void rule__JvmParameterizedTypeReference__Group_1_4__2__Impl() throws RecognitionException;
void rule__JvmParameterizedTypeReference__Group_1_4_0__0() throws RecognitionException;
void rule__JvmParameterizedTypeReference__Group_1_4_0__0__Impl() throws RecognitionException;
void rule__JvmParameterizedTypeReference__Group_1_4_0_0__0() throws RecognitionException;
void rule__JvmParameterizedTypeReference__Group_1_4_0_0__0__Impl() throws RecognitionException;
void rule__JvmParameterizedTypeReference__Group_1_4_0_0__1() throws RecognitionException;
void rule__JvmParameterizedTypeReference__Group_1_4_0_0__1__Impl() throws RecognitionException;
void rule__JvmParameterizedTypeReference__Group_1_4_2__0() throws RecognitionException;
void rule__JvmParameterizedTypeReference__Group_1_4_2__0__Impl() throws RecognitionException;
void rule__JvmParameterizedTypeReference__Group_1_4_2__1() throws RecognitionException;
void rule__JvmParameterizedTypeReference__Group_1_4_2__1__Impl() throws RecognitionException;
void rule__JvmParameterizedTypeReference__Group_1_4_2__2() throws RecognitionException;
void rule__JvmParameterizedTypeReference__Group_1_4_2__2__Impl() throws RecognitionException;
void rule__JvmParameterizedTypeReference__Group_1_4_2__3() throws RecognitionException;
void rule__JvmParameterizedTypeReference__Group_1_4_2__3__Impl() throws RecognitionException;
void rule__JvmParameterizedTypeReference__Group_1_4_2_2__0() throws RecognitionException;
void rule__JvmParameterizedTypeReference__Group_1_4_2_2__0__Impl() throws RecognitionException;
void rule__JvmParameterizedTypeReference__Group_1_4_2_2__1() throws RecognitionException;
void rule__JvmParameterizedTypeReference__Group_1_4_2_2__1__Impl() throws RecognitionException;
void rule__JvmWildcardTypeReference__Group__0() throws RecognitionException;
void rule__JvmWildcardTypeReference__Group__0__Impl() throws RecognitionException;
void rule__JvmWildcardTypeReference__Group__1() throws RecognitionException;
void rule__JvmWildcardTypeReference__Group__1__Impl() throws RecognitionException;
void rule__JvmWildcardTypeReference__Group__2() throws RecognitionException;
void rule__JvmWildcardTypeReference__Group__2__Impl() throws RecognitionException;
void rule__JvmWildcardTypeReference__Group_2_0__0() throws RecognitionException;
void rule__JvmWildcardTypeReference__Group_2_0__0__Impl() throws RecognitionException;
void rule__JvmWildcardTypeReference__Group_2_0__1() throws RecognitionException;
void rule__JvmWildcardTypeReference__Group_2_0__1__Impl() throws RecognitionException;
void rule__JvmWildcardTypeReference__Group_2_1__0() throws RecognitionException;
void rule__JvmWildcardTypeReference__Group_2_1__0__Impl() throws RecognitionException;
void rule__JvmWildcardTypeReference__Group_2_1__1() throws RecognitionException;
void rule__JvmWildcardTypeReference__Group_2_1__1__Impl() throws RecognitionException;
void rule__JvmUpperBound__Group__0() throws RecognitionException;
void rule__JvmUpperBound__Group__0__Impl() throws RecognitionException;
void rule__JvmUpperBound__Group__1() throws RecognitionException;
void rule__JvmUpperBound__Group__1__Impl() throws RecognitionException;
void rule__JvmUpperBoundAnded__Group__0() throws RecognitionException;
void rule__JvmUpperBoundAnded__Group__0__Impl() throws RecognitionException;
void rule__JvmUpperBoundAnded__Group__1() throws RecognitionException;
void rule__JvmUpperBoundAnded__Group__1__Impl() throws RecognitionException;
void rule__JvmLowerBound__Group__0() throws RecognitionException;
void rule__JvmLowerBound__Group__0__Impl() throws RecognitionException;
void rule__JvmLowerBound__Group__1() throws RecognitionException;
void rule__JvmLowerBound__Group__1__Impl() throws RecognitionException;
void rule__JvmLowerBoundAnded__Group__0() throws RecognitionException;
void rule__JvmLowerBoundAnded__Group__0__Impl() throws RecognitionException;
void rule__JvmLowerBoundAnded__Group__1() throws RecognitionException;
void rule__JvmLowerBoundAnded__Group__1__Impl() throws RecognitionException;
void rule__JvmTypeParameter__Group__0() throws RecognitionException;
void rule__JvmTypeParameter__Group__0__Impl() throws RecognitionException;
void rule__JvmTypeParameter__Group__1() throws RecognitionException;
void rule__JvmTypeParameter__Group__1__Impl() throws RecognitionException;
void rule__JvmTypeParameter__Group_1__0() throws RecognitionException;
void rule__JvmTypeParameter__Group_1__0__Impl() throws RecognitionException;
void rule__JvmTypeParameter__Group_1__1() throws RecognitionException;
void rule__JvmTypeParameter__Group_1__1__Impl() throws RecognitionException;
void rule__QualifiedNameWithWildcard__Group__0() throws RecognitionException;
void rule__QualifiedNameWithWildcard__Group__0__Impl() throws RecognitionException;
void rule__QualifiedNameWithWildcard__Group__1() throws RecognitionException;
void rule__QualifiedNameWithWildcard__Group__1__Impl() throws RecognitionException;
void rule__QualifiedNameWithWildcard__Group__2() throws RecognitionException;
void rule__QualifiedNameWithWildcard__Group__2__Impl() throws RecognitionException;
void rule__XImportDeclaration__Group__0() throws RecognitionException;
void rule__XImportDeclaration__Group__0__Impl() throws RecognitionException;
void rule__XImportDeclaration__Group__1() throws RecognitionException;
void rule__XImportDeclaration__Group__1__Impl() throws RecognitionException;
void rule__XImportDeclaration__Group__2() throws RecognitionException;
void rule__XImportDeclaration__Group__2__Impl() throws RecognitionException;
void rule__XImportDeclaration__Group_1_0__0() throws RecognitionException;
void rule__XImportDeclaration__Group_1_0__0__Impl() throws RecognitionException;
void rule__XImportDeclaration__Group_1_0__1() throws RecognitionException;
void rule__XImportDeclaration__Group_1_0__1__Impl() throws RecognitionException;
void rule__XImportDeclaration__Group_1_0__2() throws RecognitionException;
void rule__XImportDeclaration__Group_1_0__2__Impl() throws RecognitionException;
void rule__XImportDeclaration__Group_1_0__3() throws RecognitionException;
void rule__XImportDeclaration__Group_1_0__3__Impl() throws RecognitionException;
void rule__QualifiedNameInStaticImport__Group__0() throws RecognitionException;
void rule__QualifiedNameInStaticImport__Group__0__Impl() throws RecognitionException;
void rule__QualifiedNameInStaticImport__Group__1() throws RecognitionException;
void rule__QualifiedNameInStaticImport__Group__1__Impl() throws RecognitionException;
void rule__ModelRoot__ImportSectionAssignment_0_0() throws RecognitionException;
void rule__ModelRoot__ModelRootAssignment_0_1() throws RecognitionException;
void rule__Model__ModelNameAssignment_1() throws RecognitionException;
void rule__Model__ElementsAssignment_3() throws RecognitionException;
void rule__PackageDeclaration__PackageNameAssignment_1() throws RecognitionException;
void rule__PackageDeclaration__ElementsAssignment_3() throws RecognitionException;
void rule__Enumeration__NameAssignment_1() throws RecognitionException;
void rule__Enumeration__EnumerationValuesAssignment_3() throws RecognitionException;
void rule__EnumerationValue__NameAssignment_0() throws RecognitionException;
void rule__EnumerationValue__ValueAssignment_1_1() throws RecognitionException;
void rule__EntityNaturalKeyFields__NaturalKeyAttributesAssignment_3() throws RecognitionException;
void rule__EntityNaturalKeyFields__NaturalKeyAttributesAssignment_4_1() throws RecognitionException;
void rule__EntityHierarchical__HierarchicalAssignment_2() throws RecognitionException;
void rule__EntityDisableIdField__DisableIdFieldAssignment_2() throws RecognitionException;
void rule__EntityLabelField__LabelAssignment_2() throws RecognitionException;
void rule__EntityPluralLabelField__PluralLabelAssignment_2() throws RecognitionException;
void rule__EntityOptionsContainer__OptionsAssignment_3() throws RecognitionException;
void rule__Entity__NameAssignment_1() throws RecognitionException;
void rule__Entity__ExtendsAssignment_2_1() throws RecognitionException;
void rule__Entity__JvmtypeAssignment_3_1() throws RecognitionException;
void rule__Entity__EntityOptionsAssignment_5() throws RecognitionException;
void rule__Entity__AttributesAssignment_6() throws RecognitionException;
void rule__ValueObject__NameAssignment_1() throws RecognitionException;
void rule__ValueObject__ExtendsAssignment_2_1() throws RecognitionException;
void rule__ValueObject__JvmtypeAssignment_3_1() throws RecognitionException;
void rule__ValueObject__AttributesAssignment_5() throws RecognitionException;
void rule__BaseDataType__BaseDatatypePropertiesAssignment_1() throws RecognitionException;
void rule__BaseDataTypeWidth__WidthAssignment_2() throws RecognitionException;
void rule__BaseDataTypeLabel__LabelAssignment_2() throws RecognitionException;
void rule__StringDataType__NameAssignment_1() throws RecognitionException;
void rule__StringDataType__RefAssignment_2_1() throws RecognitionException;
void rule__StringDataType__BaseDataTypeAssignment_4() throws RecognitionException;
void rule__StringDataType__MaxLengthAssignment_5_1() throws RecognitionException;
void rule__StringDataType__MinLengthAssignment_6_1() throws RecognitionException;
void rule__StringEntityAttribute__TypeAssignment_1() throws RecognitionException;
void rule__StringEntityAttribute__CardinalityAssignment_2() throws RecognitionException;
void rule__StringEntityAttribute__NameAssignment_3() throws RecognitionException;
void rule__MapEntityAttribute__TypeAssignment_1() throws RecognitionException;
void rule__MapEntityAttribute__KeyTypeAssignment_2_0() throws RecognitionException;
void rule__MapEntityAttribute__ValueTypeAssignment_2_1() throws RecognitionException;
void rule__MapEntityAttribute__NameAssignment_3() throws RecognitionException;
void rule__BooleanDataType__NameAssignment_1() throws RecognitionException;
void rule__BooleanDataType__RefAssignment_2_1() throws RecognitionException;
void rule__BooleanDataType__BaseDataTypeAssignment_4() throws RecognitionException;
void rule__BooleanEntityAttribute__TypeAssignment_1() throws RecognitionException;
void rule__BooleanEntityAttribute__NameAssignment_2() throws RecognitionException;
void rule__IntegerDataType__NameAssignment_1() throws RecognitionException;
void rule__IntegerDataType__RefAssignment_2_1() throws RecognitionException;
void rule__IntegerDataType__BaseDataTypeAssignment_4() throws RecognitionException;
void rule__IntegerEntityAttribute__TypeAssignment_1() throws RecognitionException;
void rule__IntegerEntityAttribute__NameAssignment_2() throws RecognitionException;
void rule__DateDataType__NameAssignment_1() throws RecognitionException;
void rule__DateDataType__RefAssignment_2_1() throws RecognitionException;
void rule__DateDataType__BaseDataTypeAssignment_4() throws RecognitionException;
void rule__DateEntityAttribute__TypeAssignment_1() throws RecognitionException;
void rule__DateEntityAttribute__NameAssignment_2() throws RecognitionException;
void rule__DecimalDataType__NameAssignment_1() throws RecognitionException;
void rule__DecimalDataType__RefAssignment_2_1() throws RecognitionException;
void rule__DecimalDataType__BaseDataTypeAssignment_4() throws RecognitionException;
void rule__DecimalEntityAttribute__TypeAssignment_1() throws RecognitionException;
void rule__DecimalEntityAttribute__NameAssignment_2() throws RecognitionException;
void rule__LongDataType__NameAssignment_1() throws RecognitionException;
void rule__LongDataType__RefAssignment_2_1() throws RecognitionException;
void rule__LongDataType__BaseDataTypeAssignment_4() throws RecognitionException;
void rule__LongEntityAttribute__TypeAssignment_1() throws RecognitionException;
void rule__LongEntityAttribute__NameAssignment_2() throws RecognitionException;
void rule__FloatDataType__NameAssignment_1() throws RecognitionException;
void rule__FloatDataType__RefAssignment_2_1() throws RecognitionException;
void rule__FloatDataType__BaseDataTypeAssignment_4() throws RecognitionException;
void rule__FloatEntityAttribute__TypeAssignment_1() throws RecognitionException;
void rule__FloatEntityAttribute__NameAssignment_2() throws RecognitionException;
void rule__DoubleDataType__NameAssignment_1() throws RecognitionException;
void rule__DoubleDataType__RefAssignment_2_1() throws RecognitionException;
void rule__DoubleDataType__BaseDataTypeAssignment_4() throws RecognitionException;
void rule__DoubleEntityAttribute__TypeAssignment_1() throws RecognitionException;
void rule__DoubleEntityAttribute__NameAssignment_2() throws RecognitionException;
void rule__BinaryDataType__NameAssignment_1() throws RecognitionException;
void rule__BinaryDataType__RefAssignment_2_1() throws RecognitionException;
void rule__BinaryDataType__BaseDataTypeAssignment_4() throws RecognitionException;
void rule__BinaryEntityAttribute__TypeAssignment_1() throws RecognitionException;
void rule__BinaryEntityAttribute__NameAssignment_2() throws RecognitionException;
void rule__EntityDataType__NameAssignment_1() throws RecognitionException;
void rule__EntityDataType__RefAssignment_2_1() throws RecognitionException;
void rule__EntityDataType__BaseDataTypeAssignment_4() throws RecognitionException;
void rule__EntityDataType__EntityAssignment_6() throws RecognitionException;
void rule__EntityEntityAttribute__TypeAssignment_1() throws RecognitionException;
void rule__EntityEntityAttribute__CardinalityAssignment_2() throws RecognitionException;
void rule__EntityEntityAttribute__NameAssignment_3() throws RecognitionException;
void rule__ValueObjectEntityAttribute__TypeAssignment_1() throws RecognitionException;
void rule__ValueObjectEntityAttribute__CardinalityAssignment_2() throws RecognitionException;
void rule__ValueObjectEntityAttribute__NameAssignment_3() throws RecognitionException;
void rule__EnumerationDataType__NameAssignment_1() throws RecognitionException;
void rule__EnumerationDataType__RefAssignment_2_1() throws RecognitionException;
void rule__EnumerationDataType__BaseDataTypeAssignment_4() throws RecognitionException;
void rule__EnumerationDataType__EnumerationAssignment_6() throws RecognitionException;
void rule__EnumerationEntityAttribute__TypeAssignment_1() throws RecognitionException;
void rule__EnumerationEntityAttribute__CardinalityAssignment_2() throws RecognitionException;
void rule__EnumerationEntityAttribute__NameAssignment_3() throws RecognitionException;
void rule__Dictionary__NameAssignment_1() throws RecognitionException;
void rule__Dictionary__EntityAssignment_4() throws RecognitionException;
void rule__Dictionary__LabelAssignment_5_1() throws RecognitionException;
void rule__Dictionary__PluralLabelAssignment_6_1() throws RecognitionException;
void rule__Dictionary__DictionarycontrolsAssignment_7_2() throws RecognitionException;
void rule__Dictionary__LabelcontrolsAssignment_8_2() throws RecognitionException;
void rule__Dictionary__DictionarysearchAssignment_9() throws RecognitionException;
void rule__Dictionary__DictionaryeditorAssignment_10() throws RecognitionException;
void rule__DictionarySearch__NameAssignment_1() throws RecognitionException;
void rule__DictionarySearch__LabelAssignment_3_1() throws RecognitionException;
void rule__DictionarySearch__DictionaryfiltersAssignment_4() throws RecognitionException;
void rule__DictionarySearch__DictionaryresultAssignment_5() throws RecognitionException;
void rule__DictionaryEditor__NameAssignment_1() throws RecognitionException;
void rule__DictionaryEditor__LabelAssignment_3_1() throws RecognitionException;
void rule__DictionaryEditor__LayoutdataAssignment_4() throws RecognitionException;
void rule__DictionaryEditor__LayoutAssignment_5() throws RecognitionException;
void rule__DictionaryEditor__ContainercontentsAssignment_6() throws RecognitionException;
void rule__DictionaryFilter__NameAssignment_1() throws RecognitionException;
void rule__DictionaryFilter__LayoutdataAssignment_3() throws RecognitionException;
void rule__DictionaryFilter__LayoutAssignment_4() throws RecognitionException;
void rule__DictionaryFilter__ContainercontentsAssignment_5() throws RecognitionException;
void rule__DictionaryResult__NameAssignment_1() throws RecognitionException;
void rule__DictionaryResult__ResultcolumnsAssignment_3() throws RecognitionException;
void rule__ColumnLayout__ColumnsAssignment_3() throws RecognitionException;
void rule__ColumnLayoutData__ColumnspanAssignment_3() throws RecognitionException;
void rule__DictionaryComposite__NameAssignment_1() throws RecognitionException;
void rule__DictionaryComposite__LayoutdataAssignment_3() throws RecognitionException;
void rule__DictionaryComposite__LayoutAssignment_4() throws RecognitionException;
void rule__DictionaryComposite__ContainercontentsAssignment_5() throws RecognitionException;
void rule__DictionaryEditableTable__NameAssignment_1() throws RecognitionException;
void rule__DictionaryEditableTable__LayoutdataAssignment_3_1() throws RecognitionException;
void rule__DictionaryEditableTable__LayoutAssignment_4_1() throws RecognitionException;
void rule__DictionaryEditableTable__ContainercontentsAssignment_5() throws RecognitionException;
void rule__DictionaryEditableTable__EntityattributeAssignment_7() throws RecognitionException;
void rule__DictionaryEditableTable__ColumncontrolsAssignment_10() throws RecognitionException;
void rule__DictionaryAssignmentTable__NameAssignment_1() throws RecognitionException;
void rule__DictionaryAssignmentTable__LayoutdataAssignment_3_1() throws RecognitionException;
void rule__DictionaryAssignmentTable__LayoutAssignment_4_1() throws RecognitionException;
void rule__DictionaryAssignmentTable__ContainercontentsAssignment_5() throws RecognitionException;
void rule__DictionaryAssignmentTable__EntityattributeAssignment_7() throws RecognitionException;
void rule__DictionaryAssignmentTable__DictionaryAssignment_9() throws RecognitionException;
void rule__DictionaryAssignmentTable__ColumncontrolsAssignment_12() throws RecognitionException;
void rule__Labels__LabelAssignment_1_1() throws RecognitionException;
void rule__Labels__FilterLabelAssignment_2_1() throws RecognitionException;
void rule__Labels__ColumnLabelAssignment_3_1() throws RecognitionException;
void rule__Labels__EditorLabelAssignment_4_1() throws RecognitionException;
void rule__Labels__ToolTipAssignment_5_1() throws RecognitionException;
void rule__BaseDictionaryControl__EntityattributeAssignment_0_1() throws RecognitionException;
void rule__BaseDictionaryControl__TypeAssignment_1_1() throws RecognitionException;
void rule__BaseDictionaryControl__LabelsAssignment_2() throws RecognitionException;
void rule__BaseDictionaryControl__MandatoryAssignment_3() throws RecognitionException;
void rule__BaseDictionaryControl__WidthAssignment_4_1() throws RecognitionException;
void rule__BaseDictionaryControl__ReadonlyAssignment_5_1() throws RecognitionException;
void rule__DictionaryControlGroupOptionMultiFilterField__MultiFilterFieldAssignment_1_1() throws RecognitionException;
void rule__DictionaryControlGroupOptionsContainer__OptionsAssignment_3() throws RecognitionException;
void rule__DictionaryControlGroup__NameAssignment_2() throws RecognitionException;
void rule__DictionaryControlGroup__RefAssignment_3_1() throws RecognitionException;
void rule__DictionaryControlGroup__ControlGroupOptionsAssignment_4_1() throws RecognitionException;
void rule__DictionaryControlGroup__BaseControlAssignment_4_2() throws RecognitionException;
void rule__DictionaryControlGroup__GroupcontrolsAssignment_4_3() throws RecognitionException;
void rule__DictionaryHierarchicalControl__NameAssignment_2() throws RecognitionException;
void rule__DictionaryHierarchicalControl__RefAssignment_3_1() throws RecognitionException;
void rule__DictionaryHierarchicalControl__BaseControlAssignment_4_1() throws RecognitionException;
void rule__DictionaryHierarchicalControl__HierarchicalIdAssignment_4_3() throws RecognitionException;
void rule__DictionaryTextControl__NameAssignment_2() throws RecognitionException;
void rule__DictionaryTextControl__RefAssignment_3_1() throws RecognitionException;
void rule__DictionaryTextControl__BaseControlAssignment_4_1() throws RecognitionException;
void rule__DictionaryIntegerControlInputType__InputtypeAssignment_2() throws RecognitionException;
void rule__DictionaryIntegerControl__NameAssignment_2() throws RecognitionException;
void rule__DictionaryIntegerControl__RefAssignment_3_1() throws RecognitionException;
void rule__DictionaryIntegerControl__BaseControlAssignment_4_1() throws RecognitionException;
void rule__DictionaryIntegerControl__OptionsAssignment_4_2() throws RecognitionException;
void rule__DictionaryBigDecimalControl__NameAssignment_2() throws RecognitionException;
void rule__DictionaryBigDecimalControl__RefAssignment_3_1() throws RecognitionException;
void rule__DictionaryBigDecimalControl__BaseControlAssignment_4_1() throws RecognitionException;
void rule__DictionaryBooleanControl__NameAssignment_2() throws RecognitionException;
void rule__DictionaryBooleanControl__RefAssignment_3_1() throws RecognitionException;
void rule__DictionaryBooleanControl__BaseControlAssignment_4_1() throws RecognitionException;
void rule__DictionaryDateControl__NameAssignment_2() throws RecognitionException;
void rule__DictionaryDateControl__RefAssignment_3_1() throws RecognitionException;
void rule__DictionaryDateControl__BaseControlAssignment_4_1() throws RecognitionException;
void rule__DictionaryEnumerationControl__NameAssignment_2() throws RecognitionException;
void rule__DictionaryEnumerationControl__RefAssignment_3_1() throws RecognitionException;
void rule__DictionaryEnumerationControl__BaseControlAssignment_4_1() throws RecognitionException;
void rule__DictionaryReferenceControl__NameAssignment_2() throws RecognitionException;
void rule__DictionaryReferenceControl__RefAssignment_3_1() throws RecognitionException;
void rule__DictionaryReferenceControl__BaseControlAssignment_4_1() throws RecognitionException;
void rule__DictionaryReferenceControl__DictionaryAssignment_4_2_1() throws RecognitionException;
void rule__DictionaryReferenceControl__ControlTypeAssignment_4_3_1() throws RecognitionException;
void rule__DictionaryReferenceControl__LabelcontrolsAssignment_4_4_2() throws RecognitionException;
void rule__DictionaryFileControl__NameAssignment_2() throws RecognitionException;
void rule__DictionaryFileControl__RefAssignment_3_1() throws RecognitionException;
void rule__DictionaryFileControl__BaseControlAssignment_4_1() throws RecognitionException;
void rule__Module__NameAssignment_1() throws RecognitionException;
void rule__Module__ModuledefinitionAssignment_4() throws RecognitionException;
void rule__Module__ModuleParametersAssignment_5_2() throws RecognitionException;
void rule__ModuleParameter__ModuleDefinitionParameterAssignment_0() throws RecognitionException;
void rule__ModuleParameter__ValueAssignment_2() throws RecognitionException;
void rule__ModuleDefinition__NameAssignment_1() throws RecognitionException;
void rule__ModuleDefinition__ModuleDefinitionParametersAssignment_3_2() throws RecognitionException;
void rule__ModuleDefinitionParameter__NameAssignment_1() throws RecognitionException;
void rule__ModuleDefinitionParameter__TypeAssignment_4() throws RecognitionException;
void rule__ServiceOptions__NonpublicAssignment_1() throws RecognitionException;
void rule__ServiceMethod__TypeParameterAssignment_1_1() throws RecognitionException;
void rule__ServiceMethod__ReturnTypeAssignment_2() throws RecognitionException;
void rule__ServiceMethod__NameAssignment_3() throws RecognitionException;
void rule__ServiceMethod__ParamsAssignment_5_0() throws RecognitionException;
void rule__ServiceMethod__ParamsAssignment_5_1_1() throws RecognitionException;
void rule__Service__NameAssignment_2() throws RecognitionException;
void rule__Service__RemoteServiceOptionsAssignment_4_2() throws RecognitionException;
void rule__Service__RemoteMethodsAssignment_5() throws RecognitionException;
void rule__NavigationNode__NameAssignment_1() throws RecognitionException;
void rule__NavigationNode__LabelAssignment_3_1() throws RecognitionException;
void rule__NavigationNode__ModuleDefinitionAssignment_4_1() throws RecognitionException;
void rule__NavigationNode__ModuleAssignment_5_1() throws RecognitionException;
void rule__NavigationNode__DictionaryEditorAssignment_6_1() throws RecognitionException;
void rule__NavigationNode__DictionarySearchAssignment_7_1() throws RecognitionException;
void rule__NavigationNode__NavigationNodesAssignment_8() throws RecognitionException;
void rule__XAssignment__FeatureAssignment_0_1() throws RecognitionException;
void rule__XAssignment__ValueAssignment_0_3() throws RecognitionException;
void rule__XAssignment__FeatureAssignment_1_1_0_0_1() throws RecognitionException;
void rule__XAssignment__RightOperandAssignment_1_1_1() throws RecognitionException;
void rule__XOrExpression__FeatureAssignment_1_0_0_1() throws RecognitionException;
void rule__XOrExpression__RightOperandAssignment_1_1() throws RecognitionException;
void rule__XAndExpression__FeatureAssignment_1_0_0_1() throws RecognitionException;
void rule__XAndExpression__RightOperandAssignment_1_1() throws RecognitionException;
void rule__XEqualityExpression__FeatureAssignment_1_0_0_1() throws RecognitionException;
void rule__XEqualityExpression__RightOperandAssignment_1_1() throws RecognitionException;
void rule__XRelationalExpression__TypeAssignment_1_0_1() throws RecognitionException;
void rule__XRelationalExpression__FeatureAssignment_1_1_0_0_1() throws RecognitionException;
void rule__XRelationalExpression__RightOperandAssignment_1_1_1() throws RecognitionException;
void rule__XOtherOperatorExpression__FeatureAssignment_1_0_0_1() throws RecognitionException;
void rule__XOtherOperatorExpression__RightOperandAssignment_1_1() throws RecognitionException;
void rule__XAdditiveExpression__FeatureAssignment_1_0_0_1() throws RecognitionException;
void rule__XAdditiveExpression__RightOperandAssignment_1_1() throws RecognitionException;
void rule__XMultiplicativeExpression__FeatureAssignment_1_0_0_1() throws RecognitionException;
void rule__XMultiplicativeExpression__RightOperandAssignment_1_1() throws RecognitionException;
void rule__XUnaryOperation__FeatureAssignment_0_1() throws RecognitionException;
void rule__XUnaryOperation__OperandAssignment_0_2() throws RecognitionException;
void rule__XCastedExpression__TypeAssignment_1_1() throws RecognitionException;
void rule__XPostfixOperation__FeatureAssignment_1_0_1() throws RecognitionException;
void rule__XMemberFeatureCall__ExplicitStaticAssignment_1_0_0_0_1_1() throws RecognitionException;
void rule__XMemberFeatureCall__FeatureAssignment_1_0_0_0_2() throws RecognitionException;
void rule__XMemberFeatureCall__ValueAssignment_1_0_1() throws RecognitionException;
void rule__XMemberFeatureCall__NullSafeAssignment_1_1_0_0_1_1() throws RecognitionException;
void rule__XMemberFeatureCall__ExplicitStaticAssignment_1_1_0_0_1_2() throws RecognitionException;
void rule__XMemberFeatureCall__TypeArgumentsAssignment_1_1_1_1() throws RecognitionException;
void rule__XMemberFeatureCall__TypeArgumentsAssignment_1_1_1_2_1() throws RecognitionException;
void rule__XMemberFeatureCall__FeatureAssignment_1_1_2() throws RecognitionException;
void rule__XMemberFeatureCall__ExplicitOperationCallAssignment_1_1_3_0() throws RecognitionException;
void rule__XMemberFeatureCall__MemberCallArgumentsAssignment_1_1_3_1_0() throws RecognitionException;
void rule__XMemberFeatureCall__MemberCallArgumentsAssignment_1_1_3_1_1_0() throws RecognitionException;
void rule__XMemberFeatureCall__MemberCallArgumentsAssignment_1_1_3_1_1_1_1() throws RecognitionException;
void rule__XMemberFeatureCall__MemberCallArgumentsAssignment_1_1_4() throws RecognitionException;
void rule__XSetLiteral__ElementsAssignment_3_0() throws RecognitionException;
void rule__XSetLiteral__ElementsAssignment_3_1_1() throws RecognitionException;
void rule__XListLiteral__ElementsAssignment_3_0() throws RecognitionException;
void rule__XListLiteral__ElementsAssignment_3_1_1() throws RecognitionException;
void rule__XClosure__DeclaredFormalParametersAssignment_1_0_0_0() throws RecognitionException;
void rule__XClosure__DeclaredFormalParametersAssignment_1_0_0_1_1() throws RecognitionException;
void rule__XClosure__ExplicitSyntaxAssignment_1_0_1() throws RecognitionException;
void rule__XClosure__ExpressionAssignment_2() throws RecognitionException;
void rule__XExpressionInClosure__ExpressionsAssignment_1_0() throws RecognitionException;
void rule__XShortClosure__DeclaredFormalParametersAssignment_0_0_1_0() throws RecognitionException;
void rule__XShortClosure__DeclaredFormalParametersAssignment_0_0_1_1_1() throws RecognitionException;
void rule__XShortClosure__ExplicitSyntaxAssignment_0_0_2() throws RecognitionException;
void rule__XShortClosure__ExpressionAssignment_1() throws RecognitionException;
void rule__XIfExpression__IfAssignment_3() throws RecognitionException;
void rule__XIfExpression__ThenAssignment_5() throws RecognitionException;
void rule__XIfExpression__ElseAssignment_6_1() throws RecognitionException;
void rule__XSwitchExpression__DeclaredParamAssignment_2_0_0_0_1() throws RecognitionException;
void rule__XSwitchExpression__SwitchAssignment_2_0_1() throws RecognitionException;
void rule__XSwitchExpression__DeclaredParamAssignment_2_1_0_0_0() throws RecognitionException;
void rule__XSwitchExpression__SwitchAssignment_2_1_1() throws RecognitionException;
void rule__XSwitchExpression__CasesAssignment_4() throws RecognitionException;
void rule__XSwitchExpression__DefaultAssignment_5_2() throws RecognitionException;
void rule__XCasePart__TypeGuardAssignment_1() throws RecognitionException;
void rule__XCasePart__CaseAssignment_2_1() throws RecognitionException;
void rule__XCasePart__ThenAssignment_3_0_1() throws RecognitionException;
void rule__XCasePart__FallThroughAssignment_3_1() throws RecognitionException;
void rule__XForLoopExpression__DeclaredParamAssignment_0_0_3() throws RecognitionException;
void rule__XForLoopExpression__ForExpressionAssignment_1() throws RecognitionException;
void rule__XForLoopExpression__EachExpressionAssignment_3() throws RecognitionException;
void rule__XBasicForLoopExpression__InitExpressionsAssignment_3_0() throws RecognitionException;
void rule__XBasicForLoopExpression__InitExpressionsAssignment_3_1_1() throws RecognitionException;
void rule__XBasicForLoopExpression__ExpressionAssignment_5() throws RecognitionException;
void rule__XBasicForLoopExpression__UpdateExpressionsAssignment_7_0() throws RecognitionException;
void rule__XBasicForLoopExpression__UpdateExpressionsAssignment_7_1_1() throws RecognitionException;
void rule__XBasicForLoopExpression__EachExpressionAssignment_9() throws RecognitionException;
void rule__XWhileExpression__PredicateAssignment_3() throws RecognitionException;
void rule__XWhileExpression__BodyAssignment_5() throws RecognitionException;
void rule__XDoWhileExpression__BodyAssignment_2() throws RecognitionException;
void rule__XDoWhileExpression__PredicateAssignment_5() throws RecognitionException;
void rule__XBlockExpression__ExpressionsAssignment_2_0() throws RecognitionException;
void rule__XVariableDeclaration__WriteableAssignment_1_0() throws RecognitionException;
void rule__XVariableDeclaration__TypeAssignment_2_0_0_0() throws RecognitionException;
void rule__XVariableDeclaration__NameAssignment_2_0_0_1() throws RecognitionException;
void rule__XVariableDeclaration__NameAssignment_2_1() throws RecognitionException;
void rule__XVariableDeclaration__RightAssignment_3_1() throws RecognitionException;
void rule__JvmFormalParameter__ParameterTypeAssignment_0() throws RecognitionException;
void rule__JvmFormalParameter__NameAssignment_1() throws RecognitionException;
void rule__FullJvmFormalParameter__ParameterTypeAssignment_0() throws RecognitionException;
void rule__FullJvmFormalParameter__NameAssignment_1() throws RecognitionException;
void rule__XFeatureCall__TypeArgumentsAssignment_1_1() throws RecognitionException;
void rule__XFeatureCall__TypeArgumentsAssignment_1_2_1() throws RecognitionException;
void rule__XFeatureCall__FeatureAssignment_2() throws RecognitionException;
void rule__XFeatureCall__ExplicitOperationCallAssignment_3_0() throws RecognitionException;
void rule__XFeatureCall__FeatureCallArgumentsAssignment_3_1_0() throws RecognitionException;
void rule__XFeatureCall__FeatureCallArgumentsAssignment_3_1_1_0() throws RecognitionException;
void rule__XFeatureCall__FeatureCallArgumentsAssignment_3_1_1_1_1() throws RecognitionException;
void rule__XFeatureCall__FeatureCallArgumentsAssignment_4() throws RecognitionException;
void rule__XConstructorCall__ConstructorAssignment_2() throws RecognitionException;
void rule__XConstructorCall__TypeArgumentsAssignment_3_1() throws RecognitionException;
void rule__XConstructorCall__TypeArgumentsAssignment_3_2_1() throws RecognitionException;
void rule__XConstructorCall__ExplicitConstructorCallAssignment_4_0() throws RecognitionException;
void rule__XConstructorCall__ArgumentsAssignment_4_1_0() throws RecognitionException;
void rule__XConstructorCall__ArgumentsAssignment_4_1_1_0() throws RecognitionException;
void rule__XConstructorCall__ArgumentsAssignment_4_1_1_1_1() throws RecognitionException;
void rule__XConstructorCall__ArgumentsAssignment_5() throws RecognitionException;
void rule__XBooleanLiteral__IsTrueAssignment_1_1() throws RecognitionException;
void rule__XNumberLiteral__ValueAssignment_1() throws RecognitionException;
void rule__XStringLiteral__ValueAssignment_1() throws RecognitionException;
void rule__XTypeLiteral__TypeAssignment_3() throws RecognitionException;
void rule__XTypeLiteral__ArrayDimensionsAssignment_4() throws RecognitionException;
void rule__XThrowExpression__ExpressionAssignment_2() throws RecognitionException;
void rule__XReturnExpression__ExpressionAssignment_2() throws RecognitionException;
void rule__XTryCatchFinallyExpression__ExpressionAssignment_2() throws RecognitionException;
void rule__XTryCatchFinallyExpression__CatchClausesAssignment_3_0_0() throws RecognitionException;
void rule__XTryCatchFinallyExpression__FinallyExpressionAssignment_3_0_1_1() throws RecognitionException;
void rule__XTryCatchFinallyExpression__FinallyExpressionAssignment_3_1_1() throws RecognitionException;
void rule__XSynchronizedExpression__ParamAssignment_1() throws RecognitionException;
void rule__XSynchronizedExpression__ExpressionAssignment_3() throws RecognitionException;
void rule__XCatchClause__DeclaredParamAssignment_2() throws RecognitionException;
void rule__XCatchClause__ExpressionAssignment_4() throws RecognitionException;
void rule__XFunctionTypeRef__ParamTypesAssignment_0_1_0() throws RecognitionException;
void rule__XFunctionTypeRef__ParamTypesAssignment_0_1_1_1() throws RecognitionException;
void rule__XFunctionTypeRef__ReturnTypeAssignment_2() throws RecognitionException;
void rule__JvmParameterizedTypeReference__TypeAssignment_0() throws RecognitionException;
void rule__JvmParameterizedTypeReference__ArgumentsAssignment_1_1() throws RecognitionException;
void rule__JvmParameterizedTypeReference__ArgumentsAssignment_1_2_1() throws RecognitionException;
void rule__JvmParameterizedTypeReference__TypeAssignment_1_4_1() throws RecognitionException;
void rule__JvmParameterizedTypeReference__ArgumentsAssignment_1_4_2_1() throws RecognitionException;
void rule__JvmParameterizedTypeReference__ArgumentsAssignment_1_4_2_2_1() throws RecognitionException;
void rule__JvmWildcardTypeReference__ConstraintsAssignment_2_0_0() throws RecognitionException;
void rule__JvmWildcardTypeReference__ConstraintsAssignment_2_0_1() throws RecognitionException;
void rule__JvmWildcardTypeReference__ConstraintsAssignment_2_1_0() throws RecognitionException;
void rule__JvmWildcardTypeReference__ConstraintsAssignment_2_1_1() throws RecognitionException;
void rule__JvmUpperBound__TypeReferenceAssignment_1() throws RecognitionException;
void rule__JvmUpperBoundAnded__TypeReferenceAssignment_1() throws RecognitionException;
void rule__JvmLowerBound__TypeReferenceAssignment_1() throws RecognitionException;
void rule__JvmLowerBoundAnded__TypeReferenceAssignment_1() throws RecognitionException;
void rule__JvmTypeParameter__NameAssignment_0() throws RecognitionException;
void rule__JvmTypeParameter__ConstraintsAssignment_1_0() throws RecognitionException;
void rule__JvmTypeParameter__ConstraintsAssignment_1_1() throws RecognitionException;
void rule__XImportSection__ImportDeclarationsAssignment() throws RecognitionException;
void rule__XImportDeclaration__StaticAssignment_1_0_0() throws RecognitionException;
void rule__XImportDeclaration__ExtensionAssignment_1_0_1() throws RecognitionException;
void rule__XImportDeclaration__ImportedTypeAssignment_1_0_2() throws RecognitionException;
void rule__XImportDeclaration__WildcardAssignment_1_0_3_0() throws RecognitionException;
void rule__XImportDeclaration__MemberNameAssignment_1_0_3_1() throws RecognitionException;
void rule__XImportDeclaration__ImportedTypeAssignment_1_1() throws RecognitionException;
void rule__XImportDeclaration__ImportedNamespaceAssignment_1_2() throws RecognitionException;
void synpred75_InternalMango_fragment() throws RecognitionException;
void synpred76_InternalMango_fragment() throws RecognitionException;
void synpred89_InternalMango_fragment() throws RecognitionException;
void synpred97_InternalMango_fragment() throws RecognitionException;
void synpred98_InternalMango_fragment() throws RecognitionException;
void synpred111_InternalMango_fragment() throws RecognitionException;
void synpred115_InternalMango_fragment() throws RecognitionException;
void synpred116_InternalMango_fragment() throws RecognitionException;
void synpred122_InternalMango_fragment() throws RecognitionException;
void synpred282_InternalMango_fragment() throws RecognitionException;
void synpred284_InternalMango_fragment() throws RecognitionException;
void synpred285_InternalMango_fragment() throws RecognitionException;
void synpred286_InternalMango_fragment() throws RecognitionException;
void synpred287_InternalMango_fragment() throws RecognitionException;
void synpred288_InternalMango_fragment() throws RecognitionException;
void synpred289_InternalMango_fragment() throws RecognitionException;
void synpred290_InternalMango_fragment() throws RecognitionException;
void synpred291_InternalMango_fragment() throws RecognitionException;
void synpred292_InternalMango_fragment() throws RecognitionException;
void synpred293_InternalMango_fragment() throws RecognitionException;
void synpred295_InternalMango_fragment() throws RecognitionException;
void synpred296_InternalMango_fragment() throws RecognitionException;
void synpred304_InternalMango_fragment() throws RecognitionException;
void synpred311_InternalMango_fragment() throws RecognitionException;
void synpred314_InternalMango_fragment() throws RecognitionException;
void synpred327_InternalMango_fragment() throws RecognitionException;
void synpred328_InternalMango_fragment() throws RecognitionException;
void synpred332_InternalMango_fragment() throws RecognitionException;
void synpred333_InternalMango_fragment() throws RecognitionException;
void synpred334_InternalMango_fragment() throws RecognitionException;
void synpred339_InternalMango_fragment() throws RecognitionException;
void synpred340_InternalMango_fragment() throws RecognitionException;
void synpred341_InternalMango_fragment() throws RecognitionException;
void synpred342_InternalMango_fragment() throws RecognitionException;
void synpred344_InternalMango_fragment() throws RecognitionException;
void synpred348_InternalMango_fragment() throws RecognitionException;
void synpred350_InternalMango_fragment() throws RecognitionException;
void synpred351_InternalMango_fragment() throws RecognitionException;
}
@SuppressWarnings("all")
abstract class InternalMangoParser1 extends AbstractInternalContentAssistParser implements InternalMangoParserSignatures {
InternalMangoParser1(TokenStream input) {
this(input, new RecognizerSharedState());
}
InternalMangoParser1(TokenStream input, RecognizerSharedState state) {
super(input, state);
}
public static final String[] tokenNames = new String[] {
"", "", "", "", "RULE_ID", "RULE_HEX", "RULE_INT", "RULE_DECIMAL", "RULE_STRING", "RULE_ML_COMMENT", "RULE_SL_COMMENT", "RULE_WS", "RULE_ANY_OTHER", "'='", "'||'", "'&&'", "'+='", "'-='", "'*='", "'/='", "'%='", "'=='", "'!='", "'==='", "'!=='", "'>='", "'>'", "'<'", "'->'", "'..<'", "'..'", "'=>'", "'<>'", "'?:'", "'+'", "'-'", "'*'", "'**'", "'/'", "'%'", "'!'", "'++'", "'--'", "'.'", "'val'", "'extends'", "'static'", "'import'", "'extension'", "'super'", "'false'", "'TABLE'", "'SEQUENCE'", "'0..1'", "'0..n'", "'n..n'", "'long'", "'integer'", "'bigdecimal'", "'string'", "'boolean'", "'reference'", "'textbox'", "'rating'", "'text'", "'dropdown'", "'project'", "'{'", "'}'", "'package'", "'enumeration'", "'naturalkey'", "', '", "'hierarchicalEntity'", "'disableIdField'", "'label'", "'pluralLabel'", "'entityoptions'", "'entity'", "'jvmtype'", "'valueobject'", "'width'", "'stringdatatype'", "'maxLength'", "'minLength'", "'map'", "'booleandatatype'", "'integerdatatype'", "'datedatatype'", "'date'", "'decimaldatatype'", "'decimal'", "'longdatatype'", "'floatdatatype'", "'float'", "'doubledatatype'", "'double'", "'binarydatatype'", "'binary'", "'entitydatatype'", "'enumerationdatatype'", "'dictionary'", "'dictionarycontrols'", "'labelcontrols'", "'dictionarysearch'", "'dictionaryeditor'", "'dictionaryfilter'", "'dictionaryresult'", "'layout'", "'columns'", "'layoutdata'", "'columnspan'", "'composite'", "'editabletable'", "'entityattribute'", "'columncontrols'", "'assignmenttable'", "'filterLabel'", "'columnLabel'", "'editorLabel'", "'toolTip'", "'type'", "'readonly'", "'multiFilterField'", "'groupoptions'", "'controlgroup'", "'ref'", "'hierarchicalcontrol'", "'hierarchicalId'", "'textcontrol'", "'inputtype'", "'integercontrol'", "'bigdecimalcontrol'", "'booleancontrol'", "'datecontrol'", "'enumerationcontrol'", "'referencecontrol'", "'controlType'", "'filecontrol'", "'module'", "'moduledefinition'", "'parameters'", "'parameter'", "'method'", "'('", "')'", "'service'", "'options1'", "'navigationnode'", "'moduleDefinition'", "'dictionaryEditor'", "'dictionarySearch'", "'instanceof'", "'as'", "','", "'#'", "'['", "']'", "';'", "'if'", "'else'", "'switch'", "':'", "'default'", "'case'", "'for'", "'while'", "'do'", "'new'", "'null'", "'typeof'", "'throw'", "'return'", "'try'", "'finally'", "'synchronized'", "'catch'", "'?'", "'&'", "'mandatory'", "'nonpublic'", "'::'", "'?.'", "'|'", "'var'", "'true'"
};
public static final int RULE_ID=4;
public static final int T__29=29;
public static final int T__28=28;
public static final int T__159=159;
public static final int T__27=27;
public static final int T__158=158;
public static final int T__26=26;
public static final int T__25=25;
public static final int T__24=24;
public static final int T__23=23;
public static final int T__22=22;
public static final int RULE_ANY_OTHER=12;
public static final int T__21=21;
public static final int T__20=20;
public static final int T__160=160;
public static final int T__167=167;
public static final int T__168=168;
public static final int EOF=-1;
public static final int T__165=165;
public static final int T__166=166;
public static final int T__163=163;
public static final int T__164=164;
public static final int T__161=161;
public static final int T__162=162;
public static final int T__93=93;
public static final int T__19=19;
public static final int T__94=94;
public static final int T__91=91;
public static final int RULE_HEX=5;
public static final int T__92=92;
public static final int T__148=148;
public static final int T__16=16;
public static final int T__147=147;
public static final int T__15=15;
public static final int T__90=90;
public static final int T__18=18;
public static final int T__149=149;
public static final int T__17=17;
public static final int T__14=14;
public static final int T__13=13;
public static final int RULE_DECIMAL=7;
public static final int T__154=154;
public static final int T__155=155;
public static final int T__156=156;
public static final int T__157=157;
public static final int T__99=99;
public static final int T__150=150;
public static final int T__98=98;
public static final int T__151=151;
public static final int T__97=97;
public static final int T__152=152;
public static final int T__96=96;
public static final int T__153=153;
public static final int T__95=95;
public static final int T__139=139;
public static final int T__138=138;
public static final int T__137=137;
public static final int T__136=136;
public static final int T__80=80;
public static final int T__81=81;
public static final int T__82=82;
public static final int T__83=83;
public static final int T__141=141;
public static final int T__85=85;
public static final int T__142=142;
public static final int T__84=84;
public static final int T__87=87;
public static final int T__140=140;
public static final int T__86=86;
public static final int T__145=145;
public static final int T__89=89;
public static final int T__146=146;
public static final int T__88=88;
public static final int RULE_ML_COMMENT=9;
public static final int T__143=143;
public static final int T__144=144;
public static final int T__126=126;
public static final int T__125=125;
public static final int T__128=128;
public static final int RULE_STRING=8;
public static final int T__127=127;
public static final int T__71=71;
public static final int T__129=129;
public static final int T__72=72;
public static final int T__70=70;
public static final int T__76=76;
public static final int T__75=75;
public static final int T__130=130;
public static final int T__74=74;
public static final int T__131=131;
public static final int T__73=73;
public static final int T__132=132;
public static final int T__133=133;
public static final int T__79=79;
public static final int T__134=134;
public static final int T__78=78;
public static final int T__135=135;
public static final int T__77=77;
public static final int T__68=68;
public static final int T__69=69;
public static final int T__66=66;
public static final int T__67=67;
public static final int T__64=64;
public static final int T__65=65;
public static final int T__62=62;
public static final int T__63=63;
public static final int T__118=118;
public static final int T__119=119;
public static final int T__116=116;
public static final int T__117=117;
public static final int T__114=114;
public static final int T__115=115;
public static final int T__124=124;
public static final int T__123=123;
public static final int T__122=122;
public static final int T__121=121;
public static final int T__120=120;
public static final int T__61=61;
public static final int T__60=60;
public static final int T__55=55;
public static final int T__56=56;
public static final int T__57=57;
public static final int T__58=58;
public static final int T__51=51;
public static final int T__52=52;
public static final int T__53=53;
public static final int T__54=54;
public static final int T__107=107;
public static final int T__108=108;
public static final int T__109=109;
public static final int T__103=103;
public static final int T__59=59;
public static final int T__104=104;
public static final int T__105=105;
public static final int T__106=106;
public static final int T__111=111;
public static final int T__110=110;
public static final int RULE_INT=6;
public static final int T__113=113;
public static final int T__112=112;
public static final int T__50=50;
public static final int T__184=184;
public static final int T__42=42;
public static final int T__183=183;
public static final int T__43=43;
public static final int T__40=40;
public static final int T__185=185;
public static final int T__41=41;
public static final int T__46=46;
public static final int T__47=47;
public static final int T__44=44;
public static final int T__45=45;
public static final int T__48=48;
public static final int T__49=49;
public static final int T__180=180;
public static final int T__182=182;
public static final int T__181=181;
public static final int T__102=102;
public static final int T__101=101;
public static final int T__100=100;
public static final int RULE_SL_COMMENT=10;
public static final int T__175=175;
public static final int T__174=174;
public static final int T__30=30;
public static final int T__173=173;
public static final int T__31=31;
public static final int T__172=172;
public static final int T__32=32;
public static final int T__179=179;
public static final int T__33=33;
public static final int T__178=178;
public static final int T__34=34;
public static final int T__177=177;
public static final int T__35=35;
public static final int T__176=176;
public static final int T__36=36;
public static final int T__37=37;
public static final int T__38=38;
public static final int T__39=39;
public static final int T__171=171;
public static final int T__170=170;
public static final int RULE_WS=11;
public static final int T__169=169;
public String[] getTokenNames() { return InternalMangoParser.tokenNames; }
public String getGrammarFileName() { return "../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g"; }
protected MangoGrammarAccess grammarAccess;
public void setGrammarAccess(MangoGrammarAccess grammarAccess) {
this.grammarAccess = grammarAccess;
}
@Override
protected Grammar getGrammar() {
return grammarAccess.getGrammar();
}
@Override
protected String getValueForTokenName(String tokenName) {
return tokenName;
}
// Delegated rules
public final boolean synpred290_InternalMango() {
state.backtracking++;
int start = input.mark();
try {
synpred290_InternalMango_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred116_InternalMango() {
state.backtracking++;
int start = input.mark();
try {
synpred116_InternalMango_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred285_InternalMango() {
state.backtracking++;
int start = input.mark();
try {
synpred285_InternalMango_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred348_InternalMango() {
state.backtracking++;
int start = input.mark();
try {
synpred348_InternalMango_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred344_InternalMango() {
state.backtracking++;
int start = input.mark();
try {
synpred344_InternalMango_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred340_InternalMango() {
state.backtracking++;
int start = input.mark();
try {
synpred340_InternalMango_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred311_InternalMango() {
state.backtracking++;
int start = input.mark();
try {
synpred311_InternalMango_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred333_InternalMango() {
state.backtracking++;
int start = input.mark();
try {
synpred333_InternalMango_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred332_InternalMango() {
state.backtracking++;
int start = input.mark();
try {
synpred332_InternalMango_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred296_InternalMango() {
state.backtracking++;
int start = input.mark();
try {
synpred296_InternalMango_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred341_InternalMango() {
state.backtracking++;
int start = input.mark();
try {
synpred341_InternalMango_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred282_InternalMango() {
state.backtracking++;
int start = input.mark();
try {
synpred282_InternalMango_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred295_InternalMango() {
state.backtracking++;
int start = input.mark();
try {
synpred295_InternalMango_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred286_InternalMango() {
state.backtracking++;
int start = input.mark();
try {
synpred286_InternalMango_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred97_InternalMango() {
state.backtracking++;
int start = input.mark();
try {
synpred97_InternalMango_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred339_InternalMango() {
state.backtracking++;
int start = input.mark();
try {
synpred339_InternalMango_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred75_InternalMango() {
state.backtracking++;
int start = input.mark();
try {
synpred75_InternalMango_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred328_InternalMango() {
state.backtracking++;
int start = input.mark();
try {
synpred328_InternalMango_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred89_InternalMango() {
state.backtracking++;
int start = input.mark();
try {
synpred89_InternalMango_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred334_InternalMango() {
state.backtracking++;
int start = input.mark();
try {
synpred334_InternalMango_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred115_InternalMango() {
state.backtracking++;
int start = input.mark();
try {
synpred115_InternalMango_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred287_InternalMango() {
state.backtracking++;
int start = input.mark();
try {
synpred287_InternalMango_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred293_InternalMango() {
state.backtracking++;
int start = input.mark();
try {
synpred293_InternalMango_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred351_InternalMango() {
state.backtracking++;
int start = input.mark();
try {
synpred351_InternalMango_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred76_InternalMango() {
state.backtracking++;
int start = input.mark();
try {
synpred76_InternalMango_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred122_InternalMango() {
state.backtracking++;
int start = input.mark();
try {
synpred122_InternalMango_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred111_InternalMango() {
state.backtracking++;
int start = input.mark();
try {
synpred111_InternalMango_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred284_InternalMango() {
state.backtracking++;
int start = input.mark();
try {
synpred284_InternalMango_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred304_InternalMango() {
state.backtracking++;
int start = input.mark();
try {
synpred304_InternalMango_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred292_InternalMango() {
state.backtracking++;
int start = input.mark();
try {
synpred292_InternalMango_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred288_InternalMango() {
state.backtracking++;
int start = input.mark();
try {
synpred288_InternalMango_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred291_InternalMango() {
state.backtracking++;
int start = input.mark();
try {
synpred291_InternalMango_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred289_InternalMango() {
state.backtracking++;
int start = input.mark();
try {
synpred289_InternalMango_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred314_InternalMango() {
state.backtracking++;
int start = input.mark();
try {
synpred314_InternalMango_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred350_InternalMango() {
state.backtracking++;
int start = input.mark();
try {
synpred350_InternalMango_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred342_InternalMango() {
state.backtracking++;
int start = input.mark();
try {
synpred342_InternalMango_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred98_InternalMango() {
state.backtracking++;
int start = input.mark();
try {
synpred98_InternalMango_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred327_InternalMango() {
state.backtracking++;
int start = input.mark();
try {
synpred327_InternalMango_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
protected DFA19 dfa19 = new DFA19(this);
protected DFA27 dfa27 = new DFA27(this);
protected DFA30 dfa30 = new DFA30(this);
protected DFA31 dfa31 = new DFA31(this);
protected DFA34 dfa34 = new DFA34(this);
protected DFA39 dfa39 = new DFA39(this);
protected DFA42 dfa42 = new DFA42(this);
protected DFA51 dfa51 = new DFA51(this);
protected DFA196 dfa196 = new DFA196(this);
protected DFA202 dfa202 = new DFA202(this);
protected DFA209 dfa209 = new DFA209(this);
protected DFA210 dfa210 = new DFA210(this);
protected DFA218 dfa218 = new DFA218(this);
protected DFA228 dfa228 = new DFA228(this);
protected DFA241 dfa241 = new DFA241(this);
protected DFA242 dfa242 = new DFA242(this);
protected DFA246 dfa246 = new DFA246(this);
protected DFA247 dfa247 = new DFA247(this);
protected DFA248 dfa248 = new DFA248(this);
protected DFA253 dfa253 = new DFA253(this);
protected DFA262 dfa262 = new DFA262(this);
protected DFA265 dfa265 = new DFA265(this);
static final String DFA19_eotS =
"\13\uffff";
static final String DFA19_eofS =
"\13\uffff";
static final String DFA19_minS =
"\1\32\2\uffff\1\32\7\uffff";
static final String DFA19_maxS =
"\1\41\2\uffff\1\36\7\uffff";
static final String DFA19_acceptS =
"\1\uffff\1\1\1\2\1\uffff\1\4\1\5\1\7\1\10\1\11\1\3\1\6";
static final String DFA19_specialS =
"\13\uffff}>";
static final String[] DFA19_transitionS = {
"\1\3\1\6\1\1\1\2\1\4\1\5\1\7\1\10",
"",
"",
"\1\12\3\uffff\1\11",
"",
"",
"",
"",
"",
"",
""
};
static final short[] DFA19_eot = DFA.unpackEncodedString(DFA19_eotS);
static final short[] DFA19_eof = DFA.unpackEncodedString(DFA19_eofS);
static final char[] DFA19_min = DFA.unpackEncodedStringToUnsignedChars(DFA19_minS);
static final char[] DFA19_max = DFA.unpackEncodedStringToUnsignedChars(DFA19_maxS);
static final short[] DFA19_accept = DFA.unpackEncodedString(DFA19_acceptS);
static final short[] DFA19_special = DFA.unpackEncodedString(DFA19_specialS);
static final short[][] DFA19_transition;
static {
int numStates = DFA19_transitionS.length;
DFA19_transition = new short[numStates][];
for (int i=0; i' ) | ( '..<' ) | ( ( rule__OpOther__Group_2__0 ) ) | ( '..' ) | ( '=>' ) | ( ( rule__OpOther__Group_5__0 ) ) | ( ( rule__OpOther__Group_6__0 ) ) | ( '<>' ) | ( '?:' ) );";
}
}
static final String DFA27_eotS =
"\12\uffff";
static final String DFA27_eofS =
"\4\uffff\5\3\1\uffff";
static final String DFA27_minS =
"\1\53\2\4\1\uffff\5\4\1\uffff";
static final String DFA27_maxS =
"\1\u00b6\2\61\1\uffff\5\u00b9\1\uffff";
static final String DFA27_acceptS =
"\3\uffff\1\2\5\uffff\1\1";
static final String DFA27_specialS =
"\12\uffff}>";
static final String[] DFA27_transitionS = {
"\1\1\u0089\uffff\1\2\1\3",
"\1\4\26\uffff\1\3\21\uffff\1\5\1\6\1\7\1\10\1\3",
"\1\4\26\uffff\1\3\21\uffff\1\5\1\6\1\7\1\10\1\3",
"",
"\5\3\4\uffff\1\11\45\3\20\uffff\2\3\113\uffff\2\3\6\uffff\31\3\4\uffff\2\3\1\uffff\2\3",
"\5\3\4\uffff\1\11\45\3\20\uffff\2\3\113\uffff\2\3\6\uffff\31\3\4\uffff\2\3\1\uffff\2\3",
"\5\3\4\uffff\1\11\45\3\20\uffff\2\3\113\uffff\2\3\6\uffff\31\3\4\uffff\2\3\1\uffff\2\3",
"\5\3\4\uffff\1\11\45\3\20\uffff\2\3\113\uffff\2\3\6\uffff\31\3\4\uffff\2\3\1\uffff\2\3",
"\5\3\4\uffff\1\11\45\3\20\uffff\2\3\113\uffff\2\3\6\uffff\31\3\4\uffff\2\3\1\uffff\2\3",
""
};
static final short[] DFA27_eot = DFA.unpackEncodedString(DFA27_eotS);
static final short[] DFA27_eof = DFA.unpackEncodedString(DFA27_eofS);
static final char[] DFA27_min = DFA.unpackEncodedStringToUnsignedChars(DFA27_minS);
static final char[] DFA27_max = DFA.unpackEncodedStringToUnsignedChars(DFA27_maxS);
static final short[] DFA27_accept = DFA.unpackEncodedString(DFA27_acceptS);
static final short[] DFA27_special = DFA.unpackEncodedString(DFA27_specialS);
static final short[][] DFA27_transition;
static {
int numStates = DFA27_transitionS.length;
DFA27_transition = new short[numStates][];
for (int i=0; i=0 ) return s;
break;
case 1 :
int LA30_2 = input.LA(1);
int index30_2 = input.index();
input.rewind();
s = -1;
if ( (synpred89_InternalMango()) ) {s = 3;}
else if ( (true) ) {s = 5;}
input.seek(index30_2);
if ( s>=0 ) return s;
break;
}
if (state.backtracking>0) {state.failed=true; return -1;}
NoViableAltException nvae =
new NoViableAltException(getDescription(), 30, _s, input);
error(nvae);
throw nvae;
}
}
static final String DFA31_eotS =
"\40\uffff";
static final String DFA31_eofS =
"\40\uffff";
static final String DFA31_minS =
"\1\4\26\uffff\1\0\10\uffff";
static final String DFA31_maxS =
"\1\u00b9\26\uffff\1\0\10\uffff";
static final String DFA31_acceptS =
"\1\uffff\1\1\1\2\1\3\1\4\1\5\6\uffff\1\6\11\uffff\1\7\1\uffff\1\12\1\13\1\14\1\15\1\16\1\17\1\10\1\11";
static final String DFA31_specialS =
"\27\uffff\1\0\10\uffff}>";
static final String[] DFA31_transitionS = {
"\1\5\4\14\22\uffff\1\5\21\uffff\5\5\1\14\20\uffff\1\2\114\uffff\1\35\12\uffff\2\14\2\uffff\1\26\1\uffff\1\3\3\uffff\1\27\1\30\1\31\1\1\2\14\1\32\1\33\1\34\1\uffff\1\4\11\uffff\1\14",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"\1\uffff",
"",
"",
"",
"",
"",
"",
"",
""
};
static final short[] DFA31_eot = DFA.unpackEncodedString(DFA31_eotS);
static final short[] DFA31_eof = DFA.unpackEncodedString(DFA31_eofS);
static final char[] DFA31_min = DFA.unpackEncodedStringToUnsignedChars(DFA31_minS);
static final char[] DFA31_max = DFA.unpackEncodedStringToUnsignedChars(DFA31_maxS);
static final short[] DFA31_accept = DFA.unpackEncodedString(DFA31_acceptS);
static final short[] DFA31_special = DFA.unpackEncodedString(DFA31_specialS);
static final short[][] DFA31_transition;
static {
int numStates = DFA31_transitionS.length;
DFA31_transition = new short[numStates][];
for (int i=0; i=0 ) return s;
break;
}
if (state.backtracking>0) {state.failed=true; return -1;}
NoViableAltException nvae =
new NoViableAltException(getDescription(), 31, _s, input);
error(nvae);
throw nvae;
}
}
static final String DFA34_eotS =
"\43\uffff";
static final String DFA34_eofS =
"\43\uffff";
static final String DFA34_minS =
"\1\4\1\0\41\uffff";
static final String DFA34_maxS =
"\1\u00b9\1\0\41\uffff";
static final String DFA34_acceptS =
"\2\uffff\1\2\37\uffff\1\1";
static final String DFA34_specialS =
"\1\uffff\1\0\41\uffff}>";
static final String[] DFA34_transitionS = {
"\5\2\22\uffff\1\2\3\uffff\1\2\2\uffff\2\2\4\uffff\1\2\4\uffff\6\2\20\uffff\1\2\114\uffff\1\1\12\uffff\2\2\2\uffff\1\2\1\uffff\1\2\3\uffff\11\2\1\uffff\1\2\11\uffff\1\2",
"\1\uffff",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
""
};
static final short[] DFA34_eot = DFA.unpackEncodedString(DFA34_eotS);
static final short[] DFA34_eof = DFA.unpackEncodedString(DFA34_eofS);
static final char[] DFA34_min = DFA.unpackEncodedStringToUnsignedChars(DFA34_minS);
static final char[] DFA34_max = DFA.unpackEncodedStringToUnsignedChars(DFA34_maxS);
static final short[] DFA34_accept = DFA.unpackEncodedString(DFA34_acceptS);
static final short[] DFA34_special = DFA.unpackEncodedString(DFA34_specialS);
static final short[][] DFA34_transition;
static {
int numStates = DFA34_transitionS.length;
DFA34_transition = new short[numStates][];
for (int i=0; i=0 ) return s;
break;
}
if (state.backtracking>0) {state.failed=true; return -1;}
NoViableAltException nvae =
new NoViableAltException(getDescription(), 34, _s, input);
error(nvae);
throw nvae;
}
}
static final String DFA39_eotS =
"\43\uffff";
static final String DFA39_eofS =
"\43\uffff";
static final String DFA39_minS =
"\1\4\2\0\40\uffff";
static final String DFA39_maxS =
"\1\u00b9\2\0\40\uffff";
static final String DFA39_acceptS =
"\3\uffff\1\1\1\uffff\1\2\35\uffff";
static final String DFA39_specialS =
"\1\uffff\1\0\1\1\40\uffff}>";
static final String[] DFA39_transitionS = {
"\1\1\4\5\22\uffff\1\5\3\uffff\1\3\2\uffff\2\5\4\uffff\1\5\4\uffff\6\5\20\uffff\1\5\114\uffff\1\2\12\uffff\2\5\2\uffff\1\5\1\uffff\1\5\3\uffff\11\5\1\uffff\1\5\7\uffff\1\3\1\uffff\1\5",
"\1\uffff",
"\1\uffff",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
""
};
static final short[] DFA39_eot = DFA.unpackEncodedString(DFA39_eotS);
static final short[] DFA39_eof = DFA.unpackEncodedString(DFA39_eofS);
static final char[] DFA39_min = DFA.unpackEncodedStringToUnsignedChars(DFA39_minS);
static final char[] DFA39_max = DFA.unpackEncodedStringToUnsignedChars(DFA39_maxS);
static final short[] DFA39_accept = DFA.unpackEncodedString(DFA39_acceptS);
static final short[] DFA39_special = DFA.unpackEncodedString(DFA39_specialS);
static final short[][] DFA39_transition;
static {
int numStates = DFA39_transitionS.length;
DFA39_transition = new short[numStates][];
for (int i=0; i=0 ) return s;
break;
case 1 :
int LA39_2 = input.LA(1);
int index39_2 = input.index();
input.rewind();
s = -1;
if ( (synpred116_InternalMango()) ) {s = 3;}
else if ( (true) ) {s = 5;}
input.seek(index39_2);
if ( s>=0 ) return s;
break;
}
if (state.backtracking>0) {state.failed=true; return -1;}
NoViableAltException nvae =
new NoViableAltException(getDescription(), 39, _s, input);
error(nvae);
throw nvae;
}
}
static final String DFA42_eotS =
"\43\uffff";
static final String DFA42_eofS =
"\43\uffff";
static final String DFA42_minS =
"\1\4\2\0\40\uffff";
static final String DFA42_maxS =
"\1\u00b9\2\0\40\uffff";
static final String DFA42_acceptS =
"\3\uffff\1\1\1\uffff\1\2\35\uffff";
static final String DFA42_specialS =
"\1\uffff\1\0\1\1\40\uffff}>";
static final String[] DFA42_transitionS = {
"\1\1\4\5\22\uffff\1\5\3\uffff\1\3\2\uffff\2\5\4\uffff\1\5\4\uffff\6\5\20\uffff\1\5\114\uffff\1\2\12\uffff\2\5\2\uffff\1\5\1\uffff\1\5\3\uffff\11\5\1\uffff\1\5\7\uffff\1\3\1\uffff\1\5",
"\1\uffff",
"\1\uffff",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
""
};
static final short[] DFA42_eot = DFA.unpackEncodedString(DFA42_eotS);
static final short[] DFA42_eof = DFA.unpackEncodedString(DFA42_eofS);
static final char[] DFA42_min = DFA.unpackEncodedStringToUnsignedChars(DFA42_minS);
static final char[] DFA42_max = DFA.unpackEncodedStringToUnsignedChars(DFA42_maxS);
static final short[] DFA42_accept = DFA.unpackEncodedString(DFA42_acceptS);
static final short[] DFA42_special = DFA.unpackEncodedString(DFA42_specialS);
static final short[][] DFA42_transition;
static {
int numStates = DFA42_transitionS.length;
DFA42_transition = new short[numStates][];
for (int i=0; i=0 ) return s;
break;
case 1 :
int LA42_2 = input.LA(1);
int index42_2 = input.index();
input.rewind();
s = -1;
if ( (synpred122_InternalMango()) ) {s = 3;}
else if ( (true) ) {s = 5;}
input.seek(index42_2);
if ( s>=0 ) return s;
break;
}
if (state.backtracking>0) {state.failed=true; return -1;}
NoViableAltException nvae =
new NoViableAltException(getDescription(), 42, _s, input);
error(nvae);
throw nvae;
}
}
static final String DFA51_eotS =
"\7\uffff";
static final String DFA51_eofS =
"\2\uffff\1\4\2\uffff\1\4\1\uffff";
static final String DFA51_minS =
"\1\4\1\uffff\1\53\1\4\1\uffff\1\53\1\uffff";
static final String DFA51_maxS =
"\1\56\1\uffff\1\u009e\1\44\1\uffff\1\u009e\1\uffff";
static final String DFA51_acceptS =
"\1\uffff\1\1\2\uffff\1\2\1\uffff\1\3";
static final String DFA51_specialS =
"\7\uffff}>";
static final String[] DFA51_transitionS = {
"\1\2\51\uffff\1\1",
"",
"\1\3\3\uffff\1\4\22\uffff\1\4\133\uffff\1\4",
"\1\5\37\uffff\1\6",
"",
"\1\3\3\uffff\1\4\22\uffff\1\4\133\uffff\1\4",
""
};
static final short[] DFA51_eot = DFA.unpackEncodedString(DFA51_eotS);
static final short[] DFA51_eof = DFA.unpackEncodedString(DFA51_eofS);
static final char[] DFA51_min = DFA.unpackEncodedStringToUnsignedChars(DFA51_minS);
static final char[] DFA51_max = DFA.unpackEncodedStringToUnsignedChars(DFA51_maxS);
static final short[] DFA51_accept = DFA.unpackEncodedString(DFA51_acceptS);
static final short[] DFA51_special = DFA.unpackEncodedString(DFA51_specialS);
static final short[][] DFA51_transition;
static {
int numStates = DFA51_transitionS.length;
DFA51_transition = new short[numStates][];
for (int i=0; i=0 ) return s;
break;
case 1 :
int LA196_3 = input.LA(1);
int index196_3 = input.index();
input.rewind();
s = -1;
if ( (synpred282_InternalMango()) ) {s = 9;}
else if ( (true) ) {s = 8;}
input.seek(index196_3);
if ( s>=0 ) return s;
break;
case 2 :
int LA196_1 = input.LA(1);
int index196_1 = input.index();
input.rewind();
s = -1;
if ( (synpred282_InternalMango()) ) {s = 9;}
else if ( (true) ) {s = 8;}
input.seek(index196_1);
if ( s>=0 ) return s;
break;
case 3 :
int LA196_6 = input.LA(1);
int index196_6 = input.index();
input.rewind();
s = -1;
if ( (synpred282_InternalMango()) ) {s = 9;}
else if ( (true) ) {s = 8;}
input.seek(index196_6);
if ( s>=0 ) return s;
break;
case 4 :
int LA196_7 = input.LA(1);
int index196_7 = input.index();
input.rewind();
s = -1;
if ( (synpred282_InternalMango()) ) {s = 9;}
else if ( (true) ) {s = 8;}
input.seek(index196_7);
if ( s>=0 ) return s;
break;
case 5 :
int LA196_2 = input.LA(1);
int index196_2 = input.index();
input.rewind();
s = -1;
if ( (synpred282_InternalMango()) ) {s = 9;}
else if ( (true) ) {s = 8;}
input.seek(index196_2);
if ( s>=0 ) return s;
break;
case 6 :
int LA196_5 = input.LA(1);
int index196_5 = input.index();
input.rewind();
s = -1;
if ( (synpred282_InternalMango()) ) {s = 9;}
else if ( (true) ) {s = 8;}
input.seek(index196_5);
if ( s>=0 ) return s;
break;
}
if (state.backtracking>0) {state.failed=true; return -1;}
NoViableAltException nvae =
new NoViableAltException(getDescription(), 196, _s, input);
error(nvae);
throw nvae;
}
}
static final String DFA202_eotS =
"\13\uffff";
static final String DFA202_eofS =
"\1\1\12\uffff";
static final String DFA202_minS =
"\1\4\1\uffff\10\0\1\uffff";
static final String DFA202_maxS =
"\1\u00b9\1\uffff\10\0\1\uffff";
static final String DFA202_acceptS =
"\1\uffff\1\2\10\uffff\1\1";
static final String DFA202_specialS =
"\2\uffff\1\2\1\7\1\3\1\4\1\1\1\6\1\5\1\0\1\uffff}>";
static final String[] DFA202_transitionS = {
"\5\1\5\uffff\14\1\1\3\1\2\1\4\1\5\1\6\1\7\1\10\1\11\21\1\20\uffff\2\1\113\uffff\2\1\6\uffff\31\1\4\uffff\2\1\1\uffff\2\1",
"",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
""
};
static final short[] DFA202_eot = DFA.unpackEncodedString(DFA202_eotS);
static final short[] DFA202_eof = DFA.unpackEncodedString(DFA202_eofS);
static final char[] DFA202_min = DFA.unpackEncodedStringToUnsignedChars(DFA202_minS);
static final char[] DFA202_max = DFA.unpackEncodedStringToUnsignedChars(DFA202_maxS);
static final short[] DFA202_accept = DFA.unpackEncodedString(DFA202_acceptS);
static final short[] DFA202_special = DFA.unpackEncodedString(DFA202_specialS);
static final short[][] DFA202_transition;
static {
int numStates = DFA202_transitionS.length;
DFA202_transition = new short[numStates][];
for (int i=0; i=0 ) return s;
break;
case 1 :
int LA202_6 = input.LA(1);
int index202_6 = input.index();
input.rewind();
s = -1;
if ( (synpred288_InternalMango()) ) {s = 10;}
else if ( (true) ) {s = 1;}
input.seek(index202_6);
if ( s>=0 ) return s;
break;
case 2 :
int LA202_2 = input.LA(1);
int index202_2 = input.index();
input.rewind();
s = -1;
if ( (synpred288_InternalMango()) ) {s = 10;}
else if ( (true) ) {s = 1;}
input.seek(index202_2);
if ( s>=0 ) return s;
break;
case 3 :
int LA202_4 = input.LA(1);
int index202_4 = input.index();
input.rewind();
s = -1;
if ( (synpred288_InternalMango()) ) {s = 10;}
else if ( (true) ) {s = 1;}
input.seek(index202_4);
if ( s>=0 ) return s;
break;
case 4 :
int LA202_5 = input.LA(1);
int index202_5 = input.index();
input.rewind();
s = -1;
if ( (synpred288_InternalMango()) ) {s = 10;}
else if ( (true) ) {s = 1;}
input.seek(index202_5);
if ( s>=0 ) return s;
break;
case 5 :
int LA202_8 = input.LA(1);
int index202_8 = input.index();
input.rewind();
s = -1;
if ( (synpred288_InternalMango()) ) {s = 10;}
else if ( (true) ) {s = 1;}
input.seek(index202_8);
if ( s>=0 ) return s;
break;
case 6 :
int LA202_7 = input.LA(1);
int index202_7 = input.index();
input.rewind();
s = -1;
if ( (synpred288_InternalMango()) ) {s = 10;}
else if ( (true) ) {s = 1;}
input.seek(index202_7);
if ( s>=0 ) return s;
break;
case 7 :
int LA202_3 = input.LA(1);
int index202_3 = input.index();
input.rewind();
s = -1;
if ( (synpred288_InternalMango()) ) {s = 10;}
else if ( (true) ) {s = 1;}
input.seek(index202_3);
if ( s>=0 ) return s;
break;
}
if (state.backtracking>0) {state.failed=true; return -1;}
NoViableAltException nvae =
new NoViableAltException(getDescription(), 202, _s, input);
error(nvae);
throw nvae;
}
}
static final String DFA209_eotS =
"\116\uffff";
static final String DFA209_eofS =
"\1\2\115\uffff";
static final String DFA209_minS =
"\1\4\1\0\114\uffff";
static final String DFA209_maxS =
"\1\u00b9\1\0\114\uffff";
static final String DFA209_acceptS =
"\2\uffff\1\2\112\uffff\1\1";
static final String DFA209_specialS =
"\1\uffff\1\0\114\uffff}>";
static final String[] DFA209_transitionS = {
"\5\2\5\uffff\45\2\20\uffff\2\2\113\uffff\1\1\1\2\6\uffff\31\2\4\uffff\2\2\1\uffff\2\2",
"\1\uffff",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
""
};
static final short[] DFA209_eot = DFA.unpackEncodedString(DFA209_eotS);
static final short[] DFA209_eof = DFA.unpackEncodedString(DFA209_eofS);
static final char[] DFA209_min = DFA.unpackEncodedStringToUnsignedChars(DFA209_minS);
static final char[] DFA209_max = DFA.unpackEncodedStringToUnsignedChars(DFA209_maxS);
static final short[] DFA209_accept = DFA.unpackEncodedString(DFA209_acceptS);
static final short[] DFA209_special = DFA.unpackEncodedString(DFA209_specialS);
static final short[][] DFA209_transition;
static {
int numStates = DFA209_transitionS.length;
DFA209_transition = new short[numStates][];
for (int i=0; i=0 ) return s;
break;
}
if (state.backtracking>0) {state.failed=true; return -1;}
NoViableAltException nvae =
new NoViableAltException(getDescription(), 209, _s, input);
error(nvae);
throw nvae;
}
}
static final String DFA210_eotS =
"\116\uffff";
static final String DFA210_eofS =
"\1\2\115\uffff";
static final String DFA210_minS =
"\1\4\1\0\114\uffff";
static final String DFA210_maxS =
"\1\u00b9\1\0\114\uffff";
static final String DFA210_acceptS =
"\2\uffff\1\2\112\uffff\1\1";
static final String DFA210_specialS =
"\1\uffff\1\0\114\uffff}>";
static final String[] DFA210_transitionS = {
"\5\2\5\uffff\45\2\20\uffff\2\2\113\uffff\2\2\6\uffff\4\2\1\1\24\2\4\uffff\2\2\1\uffff\2\2",
"\1\uffff",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
""
};
static final short[] DFA210_eot = DFA.unpackEncodedString(DFA210_eotS);
static final short[] DFA210_eof = DFA.unpackEncodedString(DFA210_eofS);
static final char[] DFA210_min = DFA.unpackEncodedStringToUnsignedChars(DFA210_minS);
static final char[] DFA210_max = DFA.unpackEncodedStringToUnsignedChars(DFA210_maxS);
static final short[] DFA210_accept = DFA.unpackEncodedString(DFA210_acceptS);
static final short[] DFA210_special = DFA.unpackEncodedString(DFA210_specialS);
static final short[][] DFA210_transition;
static {
int numStates = DFA210_transitionS.length;
DFA210_transition = new short[numStates][];
for (int i=0; i=0 ) return s;
break;
}
if (state.backtracking>0) {state.failed=true; return -1;}
NoViableAltException nvae =
new NoViableAltException(getDescription(), 210, _s, input);
error(nvae);
throw nvae;
}
}
static final String DFA218_eotS =
"\46\uffff";
static final String DFA218_eofS =
"\46\uffff";
static final String DFA218_minS =
"\1\4\2\0\43\uffff";
static final String DFA218_maxS =
"\1\u00b9\2\0\43\uffff";
static final String DFA218_acceptS =
"\3\uffff\1\1\1\uffff\1\2\40\uffff";
static final String DFA218_specialS =
"\1\uffff\1\0\1\1\43\uffff}>";
static final String[] DFA218_transitionS = {
"\1\1\4\5\22\uffff\1\5\3\uffff\1\3\2\uffff\2\5\4\uffff\1\5\3\uffff\7\5\20\uffff\1\5\114\uffff\1\2\12\uffff\3\5\1\uffff\1\5\1\uffff\1\5\3\uffff\11\5\1\uffff\1\5\7\uffff\1\3\2\5",
"\1\uffff",
"\1\uffff",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
""
};
static final short[] DFA218_eot = DFA.unpackEncodedString(DFA218_eotS);
static final short[] DFA218_eof = DFA.unpackEncodedString(DFA218_eofS);
static final char[] DFA218_min = DFA.unpackEncodedStringToUnsignedChars(DFA218_minS);
static final char[] DFA218_max = DFA.unpackEncodedStringToUnsignedChars(DFA218_maxS);
static final short[] DFA218_accept = DFA.unpackEncodedString(DFA218_acceptS);
static final short[] DFA218_special = DFA.unpackEncodedString(DFA218_specialS);
static final short[][] DFA218_transition;
static {
int numStates = DFA218_transitionS.length;
DFA218_transition = new short[numStates][];
for (int i=0; i=0 ) return s;
break;
case 1 :
int LA218_2 = input.LA(1);
int index218_2 = input.index();
input.rewind();
s = -1;
if ( (synpred304_InternalMango()) ) {s = 3;}
else if ( (true) ) {s = 5;}
input.seek(index218_2);
if ( s>=0 ) return s;
break;
}
if (state.backtracking>0) {state.failed=true; return -1;}
NoViableAltException nvae =
new NoViableAltException(getDescription(), 218, _s, input);
error(nvae);
throw nvae;
}
}
static final String DFA228_eotS =
"\42\uffff";
static final String DFA228_eofS =
"\42\uffff";
static final String DFA228_minS =
"\1\4\2\0\37\uffff";
static final String DFA228_maxS =
"\1\u00b9\2\0\37\uffff";
static final String DFA228_acceptS =
"\3\uffff\1\1\1\2\35\uffff";
static final String DFA228_specialS =
"\1\uffff\1\0\1\1\37\uffff}>";
static final String[] DFA228_transitionS = {
"\1\1\4\4\22\uffff\1\4\3\uffff\1\3\2\uffff\2\4\4\uffff\1\4\4\uffff\6\4\20\uffff\1\4\114\uffff\1\2\12\uffff\2\4\2\uffff\1\4\1\uffff\1\4\3\uffff\11\4\1\uffff\1\4\11\uffff\1\4",
"\1\uffff",
"\1\uffff",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
""
};
static final short[] DFA228_eot = DFA.unpackEncodedString(DFA228_eotS);
static final short[] DFA228_eof = DFA.unpackEncodedString(DFA228_eofS);
static final char[] DFA228_min = DFA.unpackEncodedStringToUnsignedChars(DFA228_minS);
static final char[] DFA228_max = DFA.unpackEncodedStringToUnsignedChars(DFA228_maxS);
static final short[] DFA228_accept = DFA.unpackEncodedString(DFA228_acceptS);
static final short[] DFA228_special = DFA.unpackEncodedString(DFA228_specialS);
static final short[][] DFA228_transition;
static {
int numStates = DFA228_transitionS.length;
DFA228_transition = new short[numStates][];
for (int i=0; i=0 ) return s;
break;
case 1 :
int LA228_2 = input.LA(1);
int index228_2 = input.index();
input.rewind();
s = -1;
if ( (synpred314_InternalMango()) ) {s = 3;}
else if ( (true) ) {s = 4;}
input.seek(index228_2);
if ( s>=0 ) return s;
break;
}
if (state.backtracking>0) {state.failed=true; return -1;}
NoViableAltException nvae =
new NoViableAltException(getDescription(), 228, _s, input);
error(nvae);
throw nvae;
}
}
static final String DFA241_eotS =
"\116\uffff";
static final String DFA241_eofS =
"\1\2\115\uffff";
static final String DFA241_minS =
"\1\4\1\0\114\uffff";
static final String DFA241_maxS =
"\1\u00b9\1\0\114\uffff";
static final String DFA241_acceptS =
"\2\uffff\1\2\112\uffff\1\1";
static final String DFA241_specialS =
"\1\uffff\1\0\114\uffff}>";
static final String[] DFA241_transitionS = {
"\5\2\5\uffff\45\2\20\uffff\2\2\113\uffff\1\1\1\2\6\uffff\31\2\4\uffff\2\2\1\uffff\2\2",
"\1\uffff",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
""
};
static final short[] DFA241_eot = DFA.unpackEncodedString(DFA241_eotS);
static final short[] DFA241_eof = DFA.unpackEncodedString(DFA241_eofS);
static final char[] DFA241_min = DFA.unpackEncodedStringToUnsignedChars(DFA241_minS);
static final char[] DFA241_max = DFA.unpackEncodedStringToUnsignedChars(DFA241_maxS);
static final short[] DFA241_accept = DFA.unpackEncodedString(DFA241_acceptS);
static final short[] DFA241_special = DFA.unpackEncodedString(DFA241_specialS);
static final short[][] DFA241_transition;
static {
int numStates = DFA241_transitionS.length;
DFA241_transition = new short[numStates][];
for (int i=0; i=0 ) return s;
break;
}
if (state.backtracking>0) {state.failed=true; return -1;}
NoViableAltException nvae =
new NoViableAltException(getDescription(), 241, _s, input);
error(nvae);
throw nvae;
}
}
static final String DFA242_eotS =
"\116\uffff";
static final String DFA242_eofS =
"\1\2\115\uffff";
static final String DFA242_minS =
"\1\4\1\0\114\uffff";
static final String DFA242_maxS =
"\1\u00b9\1\0\114\uffff";
static final String DFA242_acceptS =
"\2\uffff\1\2\112\uffff\1\1";
static final String DFA242_specialS =
"\1\uffff\1\0\114\uffff}>";
static final String[] DFA242_transitionS = {
"\5\2\5\uffff\45\2\20\uffff\2\2\113\uffff\2\2\6\uffff\4\2\1\1\24\2\4\uffff\2\2\1\uffff\2\2",
"\1\uffff",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
""
};
static final short[] DFA242_eot = DFA.unpackEncodedString(DFA242_eotS);
static final short[] DFA242_eof = DFA.unpackEncodedString(DFA242_eofS);
static final char[] DFA242_min = DFA.unpackEncodedStringToUnsignedChars(DFA242_minS);
static final char[] DFA242_max = DFA.unpackEncodedStringToUnsignedChars(DFA242_maxS);
static final short[] DFA242_accept = DFA.unpackEncodedString(DFA242_acceptS);
static final short[] DFA242_special = DFA.unpackEncodedString(DFA242_specialS);
static final short[][] DFA242_transition;
static {
int numStates = DFA242_transitionS.length;
DFA242_transition = new short[numStates][];
for (int i=0; i=0 ) return s;
break;
}
if (state.backtracking>0) {state.failed=true; return -1;}
NoViableAltException nvae =
new NoViableAltException(getDescription(), 242, _s, input);
error(nvae);
throw nvae;
}
}
static final String DFA246_eotS =
"\116\uffff";
static final String DFA246_eofS =
"\1\2\115\uffff";
static final String DFA246_minS =
"\1\4\1\0\114\uffff";
static final String DFA246_maxS =
"\1\u00b9\1\0\114\uffff";
static final String DFA246_acceptS =
"\2\uffff\1\2\112\uffff\1\1";
static final String DFA246_specialS =
"\1\uffff\1\0\114\uffff}>";
static final String[] DFA246_transitionS = {
"\5\2\5\uffff\15\2\1\1\27\2\20\uffff\2\2\113\uffff\2\2\6\uffff\31\2\4\uffff\2\2\1\uffff\2\2",
"\1\uffff",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
""
};
static final short[] DFA246_eot = DFA.unpackEncodedString(DFA246_eotS);
static final short[] DFA246_eof = DFA.unpackEncodedString(DFA246_eofS);
static final char[] DFA246_min = DFA.unpackEncodedStringToUnsignedChars(DFA246_minS);
static final char[] DFA246_max = DFA.unpackEncodedStringToUnsignedChars(DFA246_maxS);
static final short[] DFA246_accept = DFA.unpackEncodedString(DFA246_acceptS);
static final short[] DFA246_special = DFA.unpackEncodedString(DFA246_specialS);
static final short[][] DFA246_transition;
static {
int numStates = DFA246_transitionS.length;
DFA246_transition = new short[numStates][];
for (int i=0; i=0 ) return s;
break;
}
if (state.backtracking>0) {state.failed=true; return -1;}
NoViableAltException nvae =
new NoViableAltException(getDescription(), 246, _s, input);
error(nvae);
throw nvae;
}
}
static final String DFA247_eotS =
"\116\uffff";
static final String DFA247_eofS =
"\1\2\115\uffff";
static final String DFA247_minS =
"\1\4\1\0\114\uffff";
static final String DFA247_maxS =
"\1\u00b9\1\0\114\uffff";
static final String DFA247_acceptS =
"\2\uffff\1\2\112\uffff\1\1";
static final String DFA247_specialS =
"\1\uffff\1\0\114\uffff}>";
static final String[] DFA247_transitionS = {
"\5\2\5\uffff\45\2\20\uffff\2\2\113\uffff\1\1\1\2\6\uffff\31\2\4\uffff\2\2\1\uffff\2\2",
"\1\uffff",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
""
};
static final short[] DFA247_eot = DFA.unpackEncodedString(DFA247_eotS);
static final short[] DFA247_eof = DFA.unpackEncodedString(DFA247_eofS);
static final char[] DFA247_min = DFA.unpackEncodedStringToUnsignedChars(DFA247_minS);
static final char[] DFA247_max = DFA.unpackEncodedStringToUnsignedChars(DFA247_maxS);
static final short[] DFA247_accept = DFA.unpackEncodedString(DFA247_acceptS);
static final short[] DFA247_special = DFA.unpackEncodedString(DFA247_specialS);
static final short[][] DFA247_transition;
static {
int numStates = DFA247_transitionS.length;
DFA247_transition = new short[numStates][];
for (int i=0; i=0 ) return s;
break;
}
if (state.backtracking>0) {state.failed=true; return -1;}
NoViableAltException nvae =
new NoViableAltException(getDescription(), 247, _s, input);
error(nvae);
throw nvae;
}
}
static final String DFA248_eotS =
"\116\uffff";
static final String DFA248_eofS =
"\1\2\115\uffff";
static final String DFA248_minS =
"\1\4\1\0\114\uffff";
static final String DFA248_maxS =
"\1\u00b9\1\0\114\uffff";
static final String DFA248_acceptS =
"\2\uffff\1\2\112\uffff\1\1";
static final String DFA248_specialS =
"\1\uffff\1\0\114\uffff}>";
static final String[] DFA248_transitionS = {
"\5\2\5\uffff\45\2\20\uffff\2\2\113\uffff\2\2\6\uffff\4\2\1\1\24\2\4\uffff\2\2\1\uffff\2\2",
"\1\uffff",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
""
};
static final short[] DFA248_eot = DFA.unpackEncodedString(DFA248_eotS);
static final short[] DFA248_eof = DFA.unpackEncodedString(DFA248_eofS);
static final char[] DFA248_min = DFA.unpackEncodedStringToUnsignedChars(DFA248_minS);
static final char[] DFA248_max = DFA.unpackEncodedStringToUnsignedChars(DFA248_maxS);
static final short[] DFA248_accept = DFA.unpackEncodedString(DFA248_acceptS);
static final short[] DFA248_special = DFA.unpackEncodedString(DFA248_specialS);
static final short[][] DFA248_transition;
static {
int numStates = DFA248_transitionS.length;
DFA248_transition = new short[numStates][];
for (int i=0; i=0 ) return s;
break;
}
if (state.backtracking>0) {state.failed=true; return -1;}
NoViableAltException nvae =
new NoViableAltException(getDescription(), 248, _s, input);
error(nvae);
throw nvae;
}
}
static final String DFA253_eotS =
"\116\uffff";
static final String DFA253_eofS =
"\1\41\115\uffff";
static final String DFA253_minS =
"\1\4\40\0\55\uffff";
static final String DFA253_maxS =
"\1\u00b9\40\0\55\uffff";
static final String DFA253_acceptS =
"\41\uffff\1\2\53\uffff\1\1";
static final String DFA253_specialS =
"\1\uffff\1\0\1\1\1\2\1\3\1\4\1\5\1\6\1\7\1\10\1\11\1\12\1\13\1\14\1\15\1\16\1\17\1\20\1\21\1\22\1\23\1\24\1\25\1\26\1\27\1\30\1\31\1\32\1\33\1\34\1\35\1\36\1\37\55\uffff}>";
static final String[] DFA253_transitionS = {
"\1\1\1\23\1\24\1\25\1\27\5\uffff\15\41\1\15\6\41\1\10\1\7\4\41\1\6\4\41\1\2\1\3\1\4\1\5\1\16\1\21\20\uffff\1\12\1\41\113\uffff\1\40\1\41\6\uffff\3\41\1\17\1\20\2\41\1\31\1\41\1\13\3\41\1\32\1\33\1\34\1\11\1\26\1\30\1\35\1\36\1\37\1\41\1\14\1\41\4\uffff\2\41\1\uffff\1\41\1\22",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
""
};
static final short[] DFA253_eot = DFA.unpackEncodedString(DFA253_eotS);
static final short[] DFA253_eof = DFA.unpackEncodedString(DFA253_eofS);
static final char[] DFA253_min = DFA.unpackEncodedStringToUnsignedChars(DFA253_minS);
static final char[] DFA253_max = DFA.unpackEncodedStringToUnsignedChars(DFA253_maxS);
static final short[] DFA253_accept = DFA.unpackEncodedString(DFA253_acceptS);
static final short[] DFA253_special = DFA.unpackEncodedString(DFA253_specialS);
static final short[][] DFA253_transition;
static {
int numStates = DFA253_transitionS.length;
DFA253_transition = new short[numStates][];
for (int i=0; i=0 ) return s;
break;
case 1 :
int LA253_2 = input.LA(1);
int index253_2 = input.index();
input.rewind();
s = -1;
if ( (synpred339_InternalMango()) ) {s = 77;}
else if ( (true) ) {s = 33;}
input.seek(index253_2);
if ( s>=0 ) return s;
break;
case 2 :
int LA253_3 = input.LA(1);
int index253_3 = input.index();
input.rewind();
s = -1;
if ( (synpred339_InternalMango()) ) {s = 77;}
else if ( (true) ) {s = 33;}
input.seek(index253_3);
if ( s>=0 ) return s;
break;
case 3 :
int LA253_4 = input.LA(1);
int index253_4 = input.index();
input.rewind();
s = -1;
if ( (synpred339_InternalMango()) ) {s = 77;}
else if ( (true) ) {s = 33;}
input.seek(index253_4);
if ( s>=0 ) return s;
break;
case 4 :
int LA253_5 = input.LA(1);
int index253_5 = input.index();
input.rewind();
s = -1;
if ( (synpred339_InternalMango()) ) {s = 77;}
else if ( (true) ) {s = 33;}
input.seek(index253_5);
if ( s>=0 ) return s;
break;
case 5 :
int LA253_6 = input.LA(1);
int index253_6 = input.index();
input.rewind();
s = -1;
if ( (synpred339_InternalMango()) ) {s = 77;}
else if ( (true) ) {s = 33;}
input.seek(index253_6);
if ( s>=0 ) return s;
break;
case 6 :
int LA253_7 = input.LA(1);
int index253_7 = input.index();
input.rewind();
s = -1;
if ( (synpred339_InternalMango()) ) {s = 77;}
else if ( (true) ) {s = 33;}
input.seek(index253_7);
if ( s>=0 ) return s;
break;
case 7 :
int LA253_8 = input.LA(1);
int index253_8 = input.index();
input.rewind();
s = -1;
if ( (synpred339_InternalMango()) ) {s = 77;}
else if ( (true) ) {s = 33;}
input.seek(index253_8);
if ( s>=0 ) return s;
break;
case 8 :
int LA253_9 = input.LA(1);
int index253_9 = input.index();
input.rewind();
s = -1;
if ( (synpred339_InternalMango()) ) {s = 77;}
else if ( (true) ) {s = 33;}
input.seek(index253_9);
if ( s>=0 ) return s;
break;
case 9 :
int LA253_10 = input.LA(1);
int index253_10 = input.index();
input.rewind();
s = -1;
if ( (synpred339_InternalMango()) ) {s = 77;}
else if ( (true) ) {s = 33;}
input.seek(index253_10);
if ( s>=0 ) return s;
break;
case 10 :
int LA253_11 = input.LA(1);
int index253_11 = input.index();
input.rewind();
s = -1;
if ( (synpred339_InternalMango()) ) {s = 77;}
else if ( (true) ) {s = 33;}
input.seek(index253_11);
if ( s>=0 ) return s;
break;
case 11 :
int LA253_12 = input.LA(1);
int index253_12 = input.index();
input.rewind();
s = -1;
if ( (synpred339_InternalMango()) ) {s = 77;}
else if ( (true) ) {s = 33;}
input.seek(index253_12);
if ( s>=0 ) return s;
break;
case 12 :
int LA253_13 = input.LA(1);
int index253_13 = input.index();
input.rewind();
s = -1;
if ( (synpred339_InternalMango()) ) {s = 77;}
else if ( (true) ) {s = 33;}
input.seek(index253_13);
if ( s>=0 ) return s;
break;
case 13 :
int LA253_14 = input.LA(1);
int index253_14 = input.index();
input.rewind();
s = -1;
if ( (synpred339_InternalMango()) ) {s = 77;}
else if ( (true) ) {s = 33;}
input.seek(index253_14);
if ( s>=0 ) return s;
break;
case 14 :
int LA253_15 = input.LA(1);
int index253_15 = input.index();
input.rewind();
s = -1;
if ( (synpred339_InternalMango()) ) {s = 77;}
else if ( (true) ) {s = 33;}
input.seek(index253_15);
if ( s>=0 ) return s;
break;
case 15 :
int LA253_16 = input.LA(1);
int index253_16 = input.index();
input.rewind();
s = -1;
if ( (synpred339_InternalMango()) ) {s = 77;}
else if ( (true) ) {s = 33;}
input.seek(index253_16);
if ( s>=0 ) return s;
break;
case 16 :
int LA253_17 = input.LA(1);
int index253_17 = input.index();
input.rewind();
s = -1;
if ( (synpred339_InternalMango()) ) {s = 77;}
else if ( (true) ) {s = 33;}
input.seek(index253_17);
if ( s>=0 ) return s;
break;
case 17 :
int LA253_18 = input.LA(1);
int index253_18 = input.index();
input.rewind();
s = -1;
if ( (synpred339_InternalMango()) ) {s = 77;}
else if ( (true) ) {s = 33;}
input.seek(index253_18);
if ( s>=0 ) return s;
break;
case 18 :
int LA253_19 = input.LA(1);
int index253_19 = input.index();
input.rewind();
s = -1;
if ( (synpred339_InternalMango()) ) {s = 77;}
else if ( (true) ) {s = 33;}
input.seek(index253_19);
if ( s>=0 ) return s;
break;
case 19 :
int LA253_20 = input.LA(1);
int index253_20 = input.index();
input.rewind();
s = -1;
if ( (synpred339_InternalMango()) ) {s = 77;}
else if ( (true) ) {s = 33;}
input.seek(index253_20);
if ( s>=0 ) return s;
break;
case 20 :
int LA253_21 = input.LA(1);
int index253_21 = input.index();
input.rewind();
s = -1;
if ( (synpred339_InternalMango()) ) {s = 77;}
else if ( (true) ) {s = 33;}
input.seek(index253_21);
if ( s>=0 ) return s;
break;
case 21 :
int LA253_22 = input.LA(1);
int index253_22 = input.index();
input.rewind();
s = -1;
if ( (synpred339_InternalMango()) ) {s = 77;}
else if ( (true) ) {s = 33;}
input.seek(index253_22);
if ( s>=0 ) return s;
break;
case 22 :
int LA253_23 = input.LA(1);
int index253_23 = input.index();
input.rewind();
s = -1;
if ( (synpred339_InternalMango()) ) {s = 77;}
else if ( (true) ) {s = 33;}
input.seek(index253_23);
if ( s>=0 ) return s;
break;
case 23 :
int LA253_24 = input.LA(1);
int index253_24 = input.index();
input.rewind();
s = -1;
if ( (synpred339_InternalMango()) ) {s = 77;}
else if ( (true) ) {s = 33;}
input.seek(index253_24);
if ( s>=0 ) return s;
break;
case 24 :
int LA253_25 = input.LA(1);
int index253_25 = input.index();
input.rewind();
s = -1;
if ( (synpred339_InternalMango()) ) {s = 77;}
else if ( (true) ) {s = 33;}
input.seek(index253_25);
if ( s>=0 ) return s;
break;
case 25 :
int LA253_26 = input.LA(1);
int index253_26 = input.index();
input.rewind();
s = -1;
if ( (synpred339_InternalMango()) ) {s = 77;}
else if ( (true) ) {s = 33;}
input.seek(index253_26);
if ( s>=0 ) return s;
break;
case 26 :
int LA253_27 = input.LA(1);
int index253_27 = input.index();
input.rewind();
s = -1;
if ( (synpred339_InternalMango()) ) {s = 77;}
else if ( (true) ) {s = 33;}
input.seek(index253_27);
if ( s>=0 ) return s;
break;
case 27 :
int LA253_28 = input.LA(1);
int index253_28 = input.index();
input.rewind();
s = -1;
if ( (synpred339_InternalMango()) ) {s = 77;}
else if ( (true) ) {s = 33;}
input.seek(index253_28);
if ( s>=0 ) return s;
break;
case 28 :
int LA253_29 = input.LA(1);
int index253_29 = input.index();
input.rewind();
s = -1;
if ( (synpred339_InternalMango()) ) {s = 77;}
else if ( (true) ) {s = 33;}
input.seek(index253_29);
if ( s>=0 ) return s;
break;
case 29 :
int LA253_30 = input.LA(1);
int index253_30 = input.index();
input.rewind();
s = -1;
if ( (synpred339_InternalMango()) ) {s = 77;}
else if ( (true) ) {s = 33;}
input.seek(index253_30);
if ( s>=0 ) return s;
break;
case 30 :
int LA253_31 = input.LA(1);
int index253_31 = input.index();
input.rewind();
s = -1;
if ( (synpred339_InternalMango()) ) {s = 77;}
else if ( (true) ) {s = 33;}
input.seek(index253_31);
if ( s>=0 ) return s;
break;
case 31 :
int LA253_32 = input.LA(1);
int index253_32 = input.index();
input.rewind();
s = -1;
if ( (synpred339_InternalMango()) ) {s = 77;}
else if ( (true) ) {s = 33;}
input.seek(index253_32);
if ( s>=0 ) return s;
break;
}
if (state.backtracking>0) {state.failed=true; return -1;}
NoViableAltException nvae =
new NoViableAltException(getDescription(), 253, _s, input);
error(nvae);
throw nvae;
}
}
static final String DFA262_eotS =
"\117\uffff";
static final String DFA262_eofS =
"\1\2\116\uffff";
static final String DFA262_minS =
"\1\4\1\0\115\uffff";
static final String DFA262_maxS =
"\1\u00b9\1\0\115\uffff";
static final String DFA262_acceptS =
"\2\uffff\1\2\113\uffff\1\1";
static final String DFA262_specialS =
"\1\uffff\1\0\115\uffff}>";
static final String[] DFA262_transitionS = {
"\5\2\5\uffff\15\2\1\1\27\2\20\uffff\2\2\113\uffff\2\2\6\uffff\31\2\1\uffff\1\2\2\uffff\2\2\1\uffff\2\2",
"\1\uffff",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
""
};
static final short[] DFA262_eot = DFA.unpackEncodedString(DFA262_eotS);
static final short[] DFA262_eof = DFA.unpackEncodedString(DFA262_eofS);
static final char[] DFA262_min = DFA.unpackEncodedStringToUnsignedChars(DFA262_minS);
static final char[] DFA262_max = DFA.unpackEncodedStringToUnsignedChars(DFA262_maxS);
static final short[] DFA262_accept = DFA.unpackEncodedString(DFA262_acceptS);
static final short[] DFA262_special = DFA.unpackEncodedString(DFA262_specialS);
static final short[][] DFA262_transition;
static {
int numStates = DFA262_transitionS.length;
DFA262_transition = new short[numStates][];
for (int i=0; i=0 ) return s;
break;
}
if (state.backtracking>0) {state.failed=true; return -1;}
NoViableAltException nvae =
new NoViableAltException(getDescription(), 262, _s, input);
error(nvae);
throw nvae;
}
}
static final String DFA265_eotS =
"\117\uffff";
static final String DFA265_eofS =
"\1\2\116\uffff";
static final String DFA265_minS =
"\1\4\1\0\115\uffff";
static final String DFA265_maxS =
"\1\u00b9\1\0\115\uffff";
static final String DFA265_acceptS =
"\2\uffff\1\2\113\uffff\1\1";
static final String DFA265_specialS =
"\1\uffff\1\0\115\uffff}>";
static final String[] DFA265_transitionS = {
"\5\2\5\uffff\15\2\1\1\27\2\20\uffff\2\2\113\uffff\2\2\6\uffff\31\2\1\uffff\1\2\2\uffff\2\2\1\uffff\2\2",
"\1\uffff",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
""
};
static final short[] DFA265_eot = DFA.unpackEncodedString(DFA265_eotS);
static final short[] DFA265_eof = DFA.unpackEncodedString(DFA265_eofS);
static final char[] DFA265_min = DFA.unpackEncodedStringToUnsignedChars(DFA265_minS);
static final char[] DFA265_max = DFA.unpackEncodedStringToUnsignedChars(DFA265_maxS);
static final short[] DFA265_accept = DFA.unpackEncodedString(DFA265_acceptS);
static final short[] DFA265_special = DFA.unpackEncodedString(DFA265_specialS);
static final short[][] DFA265_transition;
static {
int numStates = DFA265_transitionS.length;
DFA265_transition = new short[numStates][];
for (int i=0; i=0 ) return s;
break;
}
if (state.backtracking>0) {state.failed=true; return -1;}
NoViableAltException nvae =
new NoViableAltException(getDescription(), 265, _s, input);
error(nvae);
throw nvae;
}
}
protected static class FollowSets000 {
public static final BitSet FOLLOW_ruleModelRoot_in_entryRuleModelRoot67 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleModelRoot74 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__ModelRoot__Alternatives_in_ruleModelRoot100 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleModel_in_entryRuleModel127 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleModel134 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Model__Group__0_in_ruleModel160 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rulePackageDeclaration_in_entryRulePackageDeclaration187 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRulePackageDeclaration194 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__PackageDeclaration__Group__0_in_rulePackageDeclaration220 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleAbstractElement_in_entryRuleAbstractElement247 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleAbstractElement254 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__AbstractElement__Alternatives_in_ruleAbstractElement280 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleEnumeration_in_entryRuleEnumeration307 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleEnumeration314 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Enumeration__Group__0_in_ruleEnumeration340 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleEnumerationValue_in_entryRuleEnumerationValue367 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleEnumerationValue374 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__EnumerationValue__Group__0_in_ruleEnumerationValue400 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleEntityOptions_in_entryRuleEntityOptions427 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleEntityOptions434 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__EntityOptions__Alternatives_in_ruleEntityOptions460 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleEntityNaturalKeyFields_in_entryRuleEntityNaturalKeyFields487 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleEntityNaturalKeyFields494 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__EntityNaturalKeyFields__Group__0_in_ruleEntityNaturalKeyFields520 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleEntityHierarchical_in_entryRuleEntityHierarchical547 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleEntityHierarchical554 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__EntityHierarchical__Group__0_in_ruleEntityHierarchical580 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleEntityDisableIdField_in_entryRuleEntityDisableIdField607 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleEntityDisableIdField614 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__EntityDisableIdField__Group__0_in_ruleEntityDisableIdField640 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleEntityLabelField_in_entryRuleEntityLabelField667 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleEntityLabelField674 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__EntityLabelField__Group__0_in_ruleEntityLabelField700 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleEntityPluralLabelField_in_entryRuleEntityPluralLabelField727 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleEntityPluralLabelField734 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__EntityPluralLabelField__Group__0_in_ruleEntityPluralLabelField760 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleEntityOptionsContainer_in_entryRuleEntityOptionsContainer789 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleEntityOptionsContainer796 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__EntityOptionsContainer__Group__0_in_ruleEntityOptionsContainer822 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleEntity_in_entryRuleEntity849 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleEntity856 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Entity__Group__0_in_ruleEntity882 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleValueObject_in_entryRuleValueObject909 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleValueObject916 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__ValueObject__Group__0_in_ruleValueObject942 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleDatatype_in_entryRuleDatatype971 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleDatatype978 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Datatype__Alternatives_in_ruleDatatype1004 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleSimpleDataType_in_entryRuleSimpleDataType1031 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleSimpleDataType1038 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__SimpleDataType__Alternatives_in_ruleSimpleDataType1064 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleEntityAttribute_in_entryRuleEntityAttribute1091 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleEntityAttribute1098 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__EntityAttribute__Alternatives_in_ruleEntityAttribute1124 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleSimpleDatatypeEntityAttribute_in_entryRuleSimpleDatatypeEntityAttribute1151 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleSimpleDatatypeEntityAttribute1158 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__SimpleDatatypeEntityAttribute__Alternatives_in_ruleSimpleDatatypeEntityAttribute1184 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleBaseDataType_in_entryRuleBaseDataType1213 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleBaseDataType1220 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__BaseDataType__Group__0_in_ruleBaseDataType1246 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleBaseDataTypeProperties_in_entryRuleBaseDataTypeProperties1273 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleBaseDataTypeProperties1280 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__BaseDataTypeProperties__Alternatives_in_ruleBaseDataTypeProperties1306 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleBaseDataTypeWidth_in_entryRuleBaseDataTypeWidth1333 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleBaseDataTypeWidth1340 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__BaseDataTypeWidth__Group__0_in_ruleBaseDataTypeWidth1366 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleBaseDataTypeLabel_in_entryRuleBaseDataTypeLabel1393 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleBaseDataTypeLabel1400 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__BaseDataTypeLabel__Group__0_in_ruleBaseDataTypeLabel1426 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleStringDataType_in_entryRuleStringDataType1453 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleStringDataType1460 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__StringDataType__Group__0_in_ruleStringDataType1486 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleStringEntityAttribute_in_entryRuleStringEntityAttribute1513 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleStringEntityAttribute1520 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__StringEntityAttribute__Group__0_in_ruleStringEntityAttribute1546 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleMapEntityAttribute_in_entryRuleMapEntityAttribute1575 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleMapEntityAttribute1582 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__MapEntityAttribute__Group__0_in_ruleMapEntityAttribute1608 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleBooleanDataType_in_entryRuleBooleanDataType1635 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleBooleanDataType1642 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__BooleanDataType__Group__0_in_ruleBooleanDataType1668 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleBooleanEntityAttribute_in_entryRuleBooleanEntityAttribute1695 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleBooleanEntityAttribute1702 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__BooleanEntityAttribute__Group__0_in_ruleBooleanEntityAttribute1728 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleIntegerDataType_in_entryRuleIntegerDataType1755 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleIntegerDataType1762 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__IntegerDataType__Group__0_in_ruleIntegerDataType1788 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleIntegerEntityAttribute_in_entryRuleIntegerEntityAttribute1815 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleIntegerEntityAttribute1822 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__IntegerEntityAttribute__Group__0_in_ruleIntegerEntityAttribute1848 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleDateDataType_in_entryRuleDateDataType1875 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleDateDataType1882 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DateDataType__Group__0_in_ruleDateDataType1908 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleDateEntityAttribute_in_entryRuleDateEntityAttribute1935 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleDateEntityAttribute1942 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DateEntityAttribute__Group__0_in_ruleDateEntityAttribute1968 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleDecimalDataType_in_entryRuleDecimalDataType1995 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleDecimalDataType2002 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DecimalDataType__Group__0_in_ruleDecimalDataType2028 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleDecimalEntityAttribute_in_entryRuleDecimalEntityAttribute2055 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleDecimalEntityAttribute2062 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DecimalEntityAttribute__Group__0_in_ruleDecimalEntityAttribute2088 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleLongDataType_in_entryRuleLongDataType2115 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleLongDataType2122 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__LongDataType__Group__0_in_ruleLongDataType2148 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleLongEntityAttribute_in_entryRuleLongEntityAttribute2175 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleLongEntityAttribute2182 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__LongEntityAttribute__Group__0_in_ruleLongEntityAttribute2208 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleFloatDataType_in_entryRuleFloatDataType2235 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleFloatDataType2242 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__FloatDataType__Group__0_in_ruleFloatDataType2268 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleFloatEntityAttribute_in_entryRuleFloatEntityAttribute2295 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleFloatEntityAttribute2302 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__FloatEntityAttribute__Group__0_in_ruleFloatEntityAttribute2328 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleDoubleDataType_in_entryRuleDoubleDataType2355 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleDoubleDataType2362 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DoubleDataType__Group__0_in_ruleDoubleDataType2388 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleDoubleEntityAttribute_in_entryRuleDoubleEntityAttribute2415 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleDoubleEntityAttribute2422 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DoubleEntityAttribute__Group__0_in_ruleDoubleEntityAttribute2448 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleBinaryDataType_in_entryRuleBinaryDataType2475 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleBinaryDataType2482 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__BinaryDataType__Group__0_in_ruleBinaryDataType2508 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleBinaryEntityAttribute_in_entryRuleBinaryEntityAttribute2535 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleBinaryEntityAttribute2542 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__BinaryEntityAttribute__Group__0_in_ruleBinaryEntityAttribute2568 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleEntityDataType_in_entryRuleEntityDataType2595 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleEntityDataType2602 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__EntityDataType__Group__0_in_ruleEntityDataType2628 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleEntityEntityAttribute_in_entryRuleEntityEntityAttribute2657 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleEntityEntityAttribute2664 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__EntityEntityAttribute__Group__0_in_ruleEntityEntityAttribute2690 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleValueObjectEntityAttribute_in_entryRuleValueObjectEntityAttribute2717 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleValueObjectEntityAttribute2724 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__ValueObjectEntityAttribute__Group__0_in_ruleValueObjectEntityAttribute2750 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleEnumerationDataType_in_entryRuleEnumerationDataType2777 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleEnumerationDataType2784 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__EnumerationDataType__Group__0_in_ruleEnumerationDataType2810 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleEnumerationEntityAttribute_in_entryRuleEnumerationEntityAttribute2839 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleEnumerationEntityAttribute2846 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__EnumerationEntityAttribute__Group__0_in_ruleEnumerationEntityAttribute2872 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleDictionary_in_entryRuleDictionary2899 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleDictionary2906 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Dictionary__Group__0_in_ruleDictionary2932 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleDictionarySearch_in_entryRuleDictionarySearch2959 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleDictionarySearch2966 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionarySearch__Group__0_in_ruleDictionarySearch2992 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleDictionaryEditor_in_entryRuleDictionaryEditor3019 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleDictionaryEditor3026 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryEditor__Group__0_in_ruleDictionaryEditor3052 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleDictionaryFilter_in_entryRuleDictionaryFilter3079 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleDictionaryFilter3086 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryFilter__Group__0_in_ruleDictionaryFilter3112 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleDictionaryResult_in_entryRuleDictionaryResult3139 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleDictionaryResult3146 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryResult__Group__0_in_ruleDictionaryResult3172 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleColumnLayout_in_entryRuleColumnLayout3199 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleColumnLayout3206 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__ColumnLayout__Group__0_in_ruleColumnLayout3232 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleColumnLayoutData_in_entryRuleColumnLayoutData3259 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleColumnLayoutData3266 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__ColumnLayoutData__Group__0_in_ruleColumnLayoutData3292 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleDictionaryContainer_in_entryRuleDictionaryContainer3319 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleDictionaryContainer3326 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryContainer__Alternatives_in_ruleDictionaryContainer3352 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleDictionaryComposite_in_entryRuleDictionaryComposite3379 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleDictionaryComposite3386 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryComposite__Group__0_in_ruleDictionaryComposite3412 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleDictionaryEditableTable_in_entryRuleDictionaryEditableTable3439 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleDictionaryEditableTable3446 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryEditableTable__Group__0_in_ruleDictionaryEditableTable3472 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleDictionaryAssignmentTable_in_entryRuleDictionaryAssignmentTable3499 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleDictionaryAssignmentTable3506 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryAssignmentTable__Group__0_in_ruleDictionaryAssignmentTable3532 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleDictionaryContainerContent_in_entryRuleDictionaryContainerContent3559 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleDictionaryContainerContent3566 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryContainerContent__Alternatives_in_ruleDictionaryContainerContent3592 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleLabels_in_entryRuleLabels3619 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleLabels3626 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Labels__Group__0_in_ruleLabels3652 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleBaseDictionaryControl_in_entryRuleBaseDictionaryControl3679 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleBaseDictionaryControl3686 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__BaseDictionaryControl__Group__0_in_ruleBaseDictionaryControl3712 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleDictionaryControl_in_entryRuleDictionaryControl3739 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleDictionaryControl3746 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryControl__Alternatives_in_ruleDictionaryControl3772 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleDictionaryControlGroupOptions_in_entryRuleDictionaryControlGroupOptions3799 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleDictionaryControlGroupOptions3806 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleDictionaryControlGroupOptionMultiFilterField_in_ruleDictionaryControlGroupOptions3832 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleDictionaryControlGroupOptionMultiFilterField_in_entryRuleDictionaryControlGroupOptionMultiFilterField3858 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleDictionaryControlGroupOptionMultiFilterField3865 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryControlGroupOptionMultiFilterField__Group__0_in_ruleDictionaryControlGroupOptionMultiFilterField3891 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleDictionaryControlGroupOptionsContainer_in_entryRuleDictionaryControlGroupOptionsContainer3918 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleDictionaryControlGroupOptionsContainer3925 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryControlGroupOptionsContainer__Group__0_in_ruleDictionaryControlGroupOptionsContainer3951 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleDictionaryControlGroup_in_entryRuleDictionaryControlGroup3978 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleDictionaryControlGroup3985 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryControlGroup__Group__0_in_ruleDictionaryControlGroup4011 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleDictionaryHierarchicalControl_in_entryRuleDictionaryHierarchicalControl4038 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleDictionaryHierarchicalControl4045 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryHierarchicalControl__Group__0_in_ruleDictionaryHierarchicalControl4071 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleDictionaryTextControl_in_entryRuleDictionaryTextControl4098 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleDictionaryTextControl4105 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryTextControl__Group__0_in_ruleDictionaryTextControl4131 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleDictionaryIntegerControlInputType_in_entryRuleDictionaryIntegerControlInputType4158 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleDictionaryIntegerControlInputType4165 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryIntegerControlInputType__Group__0_in_ruleDictionaryIntegerControlInputType4191 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleDictionaryIntegerControlOptions_in_entryRuleDictionaryIntegerControlOptions4218 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleDictionaryIntegerControlOptions4225 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleDictionaryIntegerControlInputType_in_ruleDictionaryIntegerControlOptions4251 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleDictionaryIntegerControl_in_entryRuleDictionaryIntegerControl4277 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleDictionaryIntegerControl4284 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryIntegerControl__Group__0_in_ruleDictionaryIntegerControl4310 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleDictionaryBigDecimalControl_in_entryRuleDictionaryBigDecimalControl4337 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleDictionaryBigDecimalControl4344 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryBigDecimalControl__Group__0_in_ruleDictionaryBigDecimalControl4370 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleDictionaryBooleanControl_in_entryRuleDictionaryBooleanControl4397 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleDictionaryBooleanControl4404 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryBooleanControl__Group__0_in_ruleDictionaryBooleanControl4430 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleDictionaryDateControl_in_entryRuleDictionaryDateControl4457 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleDictionaryDateControl4464 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryDateControl__Group__0_in_ruleDictionaryDateControl4490 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleDictionaryEnumerationControl_in_entryRuleDictionaryEnumerationControl4517 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleDictionaryEnumerationControl4524 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryEnumerationControl__Group__0_in_ruleDictionaryEnumerationControl4550 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleDictionaryReferenceControl_in_entryRuleDictionaryReferenceControl4577 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleDictionaryReferenceControl4584 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryReferenceControl__Group__0_in_ruleDictionaryReferenceControl4610 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleDictionaryFileControl_in_entryRuleDictionaryFileControl4637 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleDictionaryFileControl4644 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryFileControl__Group__0_in_ruleDictionaryFileControl4670 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleModule_in_entryRuleModule4697 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleModule4704 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Module__Group__0_in_ruleModule4730 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleModuleParameter_in_entryRuleModuleParameter4757 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleModuleParameter4764 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__ModuleParameter__Group__0_in_ruleModuleParameter4790 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleModuleDefinition_in_entryRuleModuleDefinition4817 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleModuleDefinition4824 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__ModuleDefinition__Group__0_in_ruleModuleDefinition4850 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleModuleDefinitionParameter_in_entryRuleModuleDefinitionParameter4877 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleModuleDefinitionParameter4884 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__ModuleDefinitionParameter__Group__0_in_ruleModuleDefinitionParameter4910 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleServiceOptions_in_entryRuleServiceOptions4937 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleServiceOptions4944 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__ServiceOptions__Group__0_in_ruleServiceOptions4970 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleServiceMethod_in_entryRuleServiceMethod4997 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleServiceMethod5004 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__ServiceMethod__Group__0_in_ruleServiceMethod5030 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleService_in_entryRuleService5057 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleService5064 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Service__Group__0_in_ruleService5090 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleNavigationNode_in_entryRuleNavigationNode5117 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleNavigationNode5124 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__NavigationNode__Group__0_in_ruleNavigationNode5150 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXExpression_in_entryRuleXExpression5177 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleXExpression5184 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXAssignment_in_ruleXExpression5210 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXAssignment_in_entryRuleXAssignment5236 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleXAssignment5243 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XAssignment__Alternatives_in_ruleXAssignment5269 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleOpSingleAssign_in_entryRuleOpSingleAssign5296 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleOpSingleAssign5303 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_13_in_ruleOpSingleAssign5330 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleOpMultiAssign_in_entryRuleOpMultiAssign5358 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleOpMultiAssign5365 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__OpMultiAssign__Alternatives_in_ruleOpMultiAssign5391 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXOrExpression_in_entryRuleXOrExpression5418 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleXOrExpression5425 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XOrExpression__Group__0_in_ruleXOrExpression5451 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleOpOr_in_entryRuleOpOr5478 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleOpOr5485 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_14_in_ruleOpOr5512 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXAndExpression_in_entryRuleXAndExpression5540 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleXAndExpression5547 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XAndExpression__Group__0_in_ruleXAndExpression5573 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleOpAnd_in_entryRuleOpAnd5600 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleOpAnd5607 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_15_in_ruleOpAnd5634 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXEqualityExpression_in_entryRuleXEqualityExpression5662 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleXEqualityExpression5669 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XEqualityExpression__Group__0_in_ruleXEqualityExpression5695 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleOpEquality_in_entryRuleOpEquality5722 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleOpEquality5729 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__OpEquality__Alternatives_in_ruleOpEquality5755 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXRelationalExpression_in_entryRuleXRelationalExpression5782 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleXRelationalExpression5789 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XRelationalExpression__Group__0_in_ruleXRelationalExpression5815 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleOpCompare_in_entryRuleOpCompare5842 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleOpCompare5849 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__OpCompare__Alternatives_in_ruleOpCompare5875 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXOtherOperatorExpression_in_entryRuleXOtherOperatorExpression5902 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleXOtherOperatorExpression5909 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XOtherOperatorExpression__Group__0_in_ruleXOtherOperatorExpression5935 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleOpOther_in_entryRuleOpOther5962 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleOpOther5969 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__OpOther__Alternatives_in_ruleOpOther5995 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXAdditiveExpression_in_entryRuleXAdditiveExpression6022 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleXAdditiveExpression6029 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XAdditiveExpression__Group__0_in_ruleXAdditiveExpression6055 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleOpAdd_in_entryRuleOpAdd6082 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleOpAdd6089 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__OpAdd__Alternatives_in_ruleOpAdd6115 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXMultiplicativeExpression_in_entryRuleXMultiplicativeExpression6142 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleXMultiplicativeExpression6149 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XMultiplicativeExpression__Group__0_in_ruleXMultiplicativeExpression6175 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleOpMulti_in_entryRuleOpMulti6202 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleOpMulti6209 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__OpMulti__Alternatives_in_ruleOpMulti6235 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXUnaryOperation_in_entryRuleXUnaryOperation6262 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleXUnaryOperation6269 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XUnaryOperation__Alternatives_in_ruleXUnaryOperation6295 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleOpUnary_in_entryRuleOpUnary6322 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleOpUnary6329 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__OpUnary__Alternatives_in_ruleOpUnary6355 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXCastedExpression_in_entryRuleXCastedExpression6382 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleXCastedExpression6389 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XCastedExpression__Group__0_in_ruleXCastedExpression6415 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXPostfixOperation_in_entryRuleXPostfixOperation6442 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleXPostfixOperation6449 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XPostfixOperation__Group__0_in_ruleXPostfixOperation6475 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleOpPostfix_in_entryRuleOpPostfix6502 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleOpPostfix6509 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__OpPostfix__Alternatives_in_ruleOpPostfix6535 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXMemberFeatureCall_in_entryRuleXMemberFeatureCall6562 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleXMemberFeatureCall6569 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XMemberFeatureCall__Group__0_in_ruleXMemberFeatureCall6595 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXPrimaryExpression_in_entryRuleXPrimaryExpression6622 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleXPrimaryExpression6629 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XPrimaryExpression__Alternatives_in_ruleXPrimaryExpression6655 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXLiteral_in_entryRuleXLiteral6682 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleXLiteral6689 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XLiteral__Alternatives_in_ruleXLiteral6715 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXCollectionLiteral_in_entryRuleXCollectionLiteral6742 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleXCollectionLiteral6749 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XCollectionLiteral__Alternatives_in_ruleXCollectionLiteral6775 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXSetLiteral_in_entryRuleXSetLiteral6802 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleXSetLiteral6809 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XSetLiteral__Group__0_in_ruleXSetLiteral6835 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXListLiteral_in_entryRuleXListLiteral6862 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleXListLiteral6869 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XListLiteral__Group__0_in_ruleXListLiteral6895 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXClosure_in_entryRuleXClosure6922 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleXClosure6929 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XClosure__Group__0_in_ruleXClosure6955 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXExpressionInClosure_in_entryRuleXExpressionInClosure6982 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleXExpressionInClosure6989 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XExpressionInClosure__Group__0_in_ruleXExpressionInClosure7015 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXShortClosure_in_entryRuleXShortClosure7042 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleXShortClosure7049 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XShortClosure__Group__0_in_ruleXShortClosure7075 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXParenthesizedExpression_in_entryRuleXParenthesizedExpression7102 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleXParenthesizedExpression7109 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XParenthesizedExpression__Group__0_in_ruleXParenthesizedExpression7135 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXIfExpression_in_entryRuleXIfExpression7162 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleXIfExpression7169 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XIfExpression__Group__0_in_ruleXIfExpression7195 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXSwitchExpression_in_entryRuleXSwitchExpression7222 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleXSwitchExpression7229 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XSwitchExpression__Group__0_in_ruleXSwitchExpression7255 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXCasePart_in_entryRuleXCasePart7282 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleXCasePart7289 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XCasePart__Group__0_in_ruleXCasePart7315 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXForLoopExpression_in_entryRuleXForLoopExpression7342 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleXForLoopExpression7349 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XForLoopExpression__Group__0_in_ruleXForLoopExpression7375 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXBasicForLoopExpression_in_entryRuleXBasicForLoopExpression7402 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleXBasicForLoopExpression7409 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XBasicForLoopExpression__Group__0_in_ruleXBasicForLoopExpression7435 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXWhileExpression_in_entryRuleXWhileExpression7462 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleXWhileExpression7469 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XWhileExpression__Group__0_in_ruleXWhileExpression7495 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXDoWhileExpression_in_entryRuleXDoWhileExpression7522 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleXDoWhileExpression7529 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XDoWhileExpression__Group__0_in_ruleXDoWhileExpression7555 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXBlockExpression_in_entryRuleXBlockExpression7582 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleXBlockExpression7589 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XBlockExpression__Group__0_in_ruleXBlockExpression7615 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXExpressionOrVarDeclaration_in_entryRuleXExpressionOrVarDeclaration7642 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleXExpressionOrVarDeclaration7649 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XExpressionOrVarDeclaration__Alternatives_in_ruleXExpressionOrVarDeclaration7675 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXVariableDeclaration_in_entryRuleXVariableDeclaration7702 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleXVariableDeclaration7709 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XVariableDeclaration__Group__0_in_ruleXVariableDeclaration7735 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleJvmFormalParameter_in_entryRuleJvmFormalParameter7762 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleJvmFormalParameter7769 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__JvmFormalParameter__Group__0_in_ruleJvmFormalParameter7795 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleFullJvmFormalParameter_in_entryRuleFullJvmFormalParameter7822 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleFullJvmFormalParameter7829 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__FullJvmFormalParameter__Group__0_in_ruleFullJvmFormalParameter7855 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXFeatureCall_in_entryRuleXFeatureCall7882 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleXFeatureCall7889 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XFeatureCall__Group__0_in_ruleXFeatureCall7915 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleFeatureCallID_in_entryRuleFeatureCallID7942 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleFeatureCallID7949 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__FeatureCallID__Alternatives_in_ruleFeatureCallID7975 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleIdOrSuper_in_entryRuleIdOrSuper8002 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleIdOrSuper8009 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__IdOrSuper__Alternatives_in_ruleIdOrSuper8035 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXConstructorCall_in_entryRuleXConstructorCall8062 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleXConstructorCall8069 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XConstructorCall__Group__0_in_ruleXConstructorCall8095 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXBooleanLiteral_in_entryRuleXBooleanLiteral8122 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleXBooleanLiteral8129 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XBooleanLiteral__Group__0_in_ruleXBooleanLiteral8155 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXNullLiteral_in_entryRuleXNullLiteral8182 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleXNullLiteral8189 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XNullLiteral__Group__0_in_ruleXNullLiteral8215 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXNumberLiteral_in_entryRuleXNumberLiteral8242 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleXNumberLiteral8249 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XNumberLiteral__Group__0_in_ruleXNumberLiteral8275 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXStringLiteral_in_entryRuleXStringLiteral8302 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleXStringLiteral8309 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XStringLiteral__Group__0_in_ruleXStringLiteral8335 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXTypeLiteral_in_entryRuleXTypeLiteral8362 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleXTypeLiteral8369 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XTypeLiteral__Group__0_in_ruleXTypeLiteral8395 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXThrowExpression_in_entryRuleXThrowExpression8422 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleXThrowExpression8429 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XThrowExpression__Group__0_in_ruleXThrowExpression8455 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXReturnExpression_in_entryRuleXReturnExpression8482 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleXReturnExpression8489 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XReturnExpression__Group__0_in_ruleXReturnExpression8515 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXTryCatchFinallyExpression_in_entryRuleXTryCatchFinallyExpression8542 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleXTryCatchFinallyExpression8549 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XTryCatchFinallyExpression__Group__0_in_ruleXTryCatchFinallyExpression8575 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXSynchronizedExpression_in_entryRuleXSynchronizedExpression8602 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleXSynchronizedExpression8609 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XSynchronizedExpression__Group__0_in_ruleXSynchronizedExpression8635 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXCatchClause_in_entryRuleXCatchClause8662 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleXCatchClause8669 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XCatchClause__Group__0_in_ruleXCatchClause8695 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleQualifiedName_in_entryRuleQualifiedName8722 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleQualifiedName8729 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__QualifiedName__Group__0_in_ruleQualifiedName8755 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleNumber_in_entryRuleNumber8787 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleNumber8794 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Number__Alternatives_in_ruleNumber8824 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleJvmTypeReference_in_entryRuleJvmTypeReference8853 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleJvmTypeReference8860 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__JvmTypeReference__Alternatives_in_ruleJvmTypeReference8886 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleArrayBrackets_in_entryRuleArrayBrackets8913 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleArrayBrackets8920 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__ArrayBrackets__Group__0_in_ruleArrayBrackets8946 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXFunctionTypeRef_in_entryRuleXFunctionTypeRef8973 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleXFunctionTypeRef8980 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XFunctionTypeRef__Group__0_in_ruleXFunctionTypeRef9006 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleJvmParameterizedTypeReference_in_entryRuleJvmParameterizedTypeReference9033 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleJvmParameterizedTypeReference9040 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__JvmParameterizedTypeReference__Group__0_in_ruleJvmParameterizedTypeReference9066 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleJvmArgumentTypeReference_in_entryRuleJvmArgumentTypeReference9093 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleJvmArgumentTypeReference9100 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__JvmArgumentTypeReference__Alternatives_in_ruleJvmArgumentTypeReference9126 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleJvmWildcardTypeReference_in_entryRuleJvmWildcardTypeReference9153 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleJvmWildcardTypeReference9160 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__JvmWildcardTypeReference__Group__0_in_ruleJvmWildcardTypeReference9186 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleJvmUpperBound_in_entryRuleJvmUpperBound9213 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleJvmUpperBound9220 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__JvmUpperBound__Group__0_in_ruleJvmUpperBound9246 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleJvmUpperBoundAnded_in_entryRuleJvmUpperBoundAnded9273 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleJvmUpperBoundAnded9280 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__JvmUpperBoundAnded__Group__0_in_ruleJvmUpperBoundAnded9306 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleJvmLowerBound_in_entryRuleJvmLowerBound9333 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleJvmLowerBound9340 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__JvmLowerBound__Group__0_in_ruleJvmLowerBound9366 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleJvmLowerBoundAnded_in_entryRuleJvmLowerBoundAnded9393 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleJvmLowerBoundAnded9400 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__JvmLowerBoundAnded__Group__0_in_ruleJvmLowerBoundAnded9426 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleJvmTypeParameter_in_entryRuleJvmTypeParameter9453 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleJvmTypeParameter9460 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__JvmTypeParameter__Group__0_in_ruleJvmTypeParameter9486 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleQualifiedNameWithWildcard_in_entryRuleQualifiedNameWithWildcard9513 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleQualifiedNameWithWildcard9520 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__QualifiedNameWithWildcard__Group__0_in_ruleQualifiedNameWithWildcard9546 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleValidID_in_entryRuleValidID9573 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleValidID9580 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_RULE_ID_in_ruleValidID9606 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXImportSection_in_entryRuleXImportSection9632 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleXImportSection9639 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XImportSection__ImportDeclarationsAssignment_in_ruleXImportSection9667 = new BitSet(new long[]{0x0000800000000002L});
public static final BitSet FOLLOW_rule__XImportSection__ImportDeclarationsAssignment_in_ruleXImportSection9679 = new BitSet(new long[]{0x0000800000000002L});
public static final BitSet FOLLOW_ruleXImportDeclaration_in_entryRuleXImportDeclaration9709 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleXImportDeclaration9716 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XImportDeclaration__Group__0_in_ruleXImportDeclaration9742 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleQualifiedNameInStaticImport_in_entryRuleQualifiedNameInStaticImport9769 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_entryRuleQualifiedNameInStaticImport9776 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__QualifiedNameInStaticImport__Group__0_in_ruleQualifiedNameInStaticImport9804 = new BitSet(new long[]{0x0000000000000012L});
public static final BitSet FOLLOW_rule__QualifiedNameInStaticImport__Group__0_in_ruleQualifiedNameInStaticImport9816 = new BitSet(new long[]{0x0000000000000012L});
public static final BitSet FOLLOW_rule__IdGeneratorStrategy__Alternatives_in_ruleIdGeneratorStrategy9856 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Cardinality__Alternatives_in_ruleCardinality9892 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__SimpleTypes__Alternatives_in_ruleSimpleTypes9928 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__IntegerControlInputType__Alternatives_in_ruleIntegerControlInputType9964 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__ReferenceControlType__Alternatives_in_ruleReferenceControlType10000 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__ModelRoot__Group_0__0_in_rule__ModelRoot__Alternatives10035 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rulePackageDeclaration_in_rule__ModelRoot__Alternatives10053 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rulePackageDeclaration_in_rule__AbstractElement__Alternatives10085 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleValueObject_in_rule__AbstractElement__Alternatives10102 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleDatatype_in_rule__AbstractElement__Alternatives10119 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleEntity_in_rule__AbstractElement__Alternatives10136 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleEnumeration_in_rule__AbstractElement__Alternatives10153 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleModuleDefinition_in_rule__AbstractElement__Alternatives10170 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleModule_in_rule__AbstractElement__Alternatives10187 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleService_in_rule__AbstractElement__Alternatives10204 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleDictionary_in_rule__AbstractElement__Alternatives10221 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleNavigationNode_in_rule__AbstractElement__Alternatives10238 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleEntityDisableIdField_in_rule__EntityOptions__Alternatives10270 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleEntityLabelField_in_rule__EntityOptions__Alternatives10287 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleEntityPluralLabelField_in_rule__EntityOptions__Alternatives10304 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleEntityNaturalKeyFields_in_rule__EntityOptions__Alternatives10321 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleEntityHierarchical_in_rule__EntityOptions__Alternatives10338 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleSimpleDataType_in_rule__Datatype__Alternatives10370 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleEntityDataType_in_rule__Datatype__Alternatives10387 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleStringDataType_in_rule__SimpleDataType__Alternatives10419 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleDecimalDataType_in_rule__SimpleDataType__Alternatives10436 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleDateDataType_in_rule__SimpleDataType__Alternatives10453 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleIntegerDataType_in_rule__SimpleDataType__Alternatives10470 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleBinaryDataType_in_rule__SimpleDataType__Alternatives10487 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleEnumerationDataType_in_rule__SimpleDataType__Alternatives10504 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleBooleanDataType_in_rule__SimpleDataType__Alternatives10521 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleDoubleDataType_in_rule__SimpleDataType__Alternatives10538 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleFloatDataType_in_rule__SimpleDataType__Alternatives10555 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleLongDataType_in_rule__SimpleDataType__Alternatives10572 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleSimpleDatatypeEntityAttribute_in_rule__EntityAttribute__Alternatives10604 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleEntityEntityAttribute_in_rule__EntityAttribute__Alternatives10621 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleValueObjectEntityAttribute_in_rule__EntityAttribute__Alternatives10638 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleMapEntityAttribute_in_rule__SimpleDatatypeEntityAttribute__Alternatives10670 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleLongEntityAttribute_in_rule__SimpleDatatypeEntityAttribute__Alternatives10687 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleDateEntityAttribute_in_rule__SimpleDatatypeEntityAttribute__Alternatives10704 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleDecimalEntityAttribute_in_rule__SimpleDatatypeEntityAttribute__Alternatives10721 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleDoubleEntityAttribute_in_rule__SimpleDatatypeEntityAttribute__Alternatives10738 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleFloatEntityAttribute_in_rule__SimpleDatatypeEntityAttribute__Alternatives10755 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleStringEntityAttribute_in_rule__SimpleDatatypeEntityAttribute__Alternatives10772 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleBooleanEntityAttribute_in_rule__SimpleDatatypeEntityAttribute__Alternatives10789 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleIntegerEntityAttribute_in_rule__SimpleDatatypeEntityAttribute__Alternatives10806 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleBinaryEntityAttribute_in_rule__SimpleDatatypeEntityAttribute__Alternatives10823 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleEnumerationEntityAttribute_in_rule__SimpleDatatypeEntityAttribute__Alternatives10840 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleBaseDataTypeWidth_in_rule__BaseDataTypeProperties__Alternatives10872 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleBaseDataTypeLabel_in_rule__BaseDataTypeProperties__Alternatives10889 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleDictionaryComposite_in_rule__DictionaryContainer__Alternatives10923 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleDictionaryEditableTable_in_rule__DictionaryContainer__Alternatives10940 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleDictionaryAssignmentTable_in_rule__DictionaryContainer__Alternatives10957 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleDictionaryContainer_in_rule__DictionaryContainerContent__Alternatives10989 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleDictionaryControl_in_rule__DictionaryContainerContent__Alternatives11006 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleDictionaryControlGroup_in_rule__DictionaryControl__Alternatives11038 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleDictionaryTextControl_in_rule__DictionaryControl__Alternatives11055 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleDictionaryIntegerControl_in_rule__DictionaryControl__Alternatives11072 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleDictionaryHierarchicalControl_in_rule__DictionaryControl__Alternatives11089 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleDictionaryBigDecimalControl_in_rule__DictionaryControl__Alternatives11106 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleDictionaryBooleanControl_in_rule__DictionaryControl__Alternatives11123 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleDictionaryDateControl_in_rule__DictionaryControl__Alternatives11140 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleDictionaryEnumerationControl_in_rule__DictionaryControl__Alternatives11157 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleDictionaryFileControl_in_rule__DictionaryControl__Alternatives11174 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleDictionaryReferenceControl_in_rule__DictionaryControl__Alternatives11191 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XAssignment__Group_0__0_in_rule__XAssignment__Alternatives11223 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XAssignment__Group_1__0_in_rule__XAssignment__Alternatives11241 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_16_in_rule__OpMultiAssign__Alternatives11275 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_17_in_rule__OpMultiAssign__Alternatives11295 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_18_in_rule__OpMultiAssign__Alternatives11315 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_19_in_rule__OpMultiAssign__Alternatives11335 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_20_in_rule__OpMultiAssign__Alternatives11355 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__OpMultiAssign__Group_5__0_in_rule__OpMultiAssign__Alternatives11374 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__OpMultiAssign__Group_6__0_in_rule__OpMultiAssign__Alternatives11392 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_21_in_rule__OpEquality__Alternatives11426 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_22_in_rule__OpEquality__Alternatives11446 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_23_in_rule__OpEquality__Alternatives11466 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_24_in_rule__OpEquality__Alternatives11486 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XRelationalExpression__Group_1_0__0_in_rule__XRelationalExpression__Alternatives_111520 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XRelationalExpression__Group_1_1__0_in_rule__XRelationalExpression__Alternatives_111538 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_25_in_rule__OpCompare__Alternatives11572 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__OpCompare__Group_1__0_in_rule__OpCompare__Alternatives11591 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_26_in_rule__OpCompare__Alternatives11610 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_27_in_rule__OpCompare__Alternatives11630 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_28_in_rule__OpOther__Alternatives11665 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_29_in_rule__OpOther__Alternatives11685 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__OpOther__Group_2__0_in_rule__OpOther__Alternatives11704 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_30_in_rule__OpOther__Alternatives11723 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_31_in_rule__OpOther__Alternatives11743 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__OpOther__Group_5__0_in_rule__OpOther__Alternatives11762 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__OpOther__Group_6__0_in_rule__OpOther__Alternatives11780 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_32_in_rule__OpOther__Alternatives11799 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_33_in_rule__OpOther__Alternatives11819 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__OpOther__Group_5_1_0__0_in_rule__OpOther__Alternatives_5_111853 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_26_in_rule__OpOther__Alternatives_5_111872 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__OpOther__Group_6_1_0__0_in_rule__OpOther__Alternatives_6_111906 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_27_in_rule__OpOther__Alternatives_6_111925 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_31_in_rule__OpOther__Alternatives_6_111945 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_34_in_rule__OpAdd__Alternatives11980 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_35_in_rule__OpAdd__Alternatives12000 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_36_in_rule__OpMulti__Alternatives12035 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_37_in_rule__OpMulti__Alternatives12055 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_38_in_rule__OpMulti__Alternatives12075 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_39_in_rule__OpMulti__Alternatives12095 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XUnaryOperation__Group_0__0_in_rule__XUnaryOperation__Alternatives12129 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXCastedExpression_in_rule__XUnaryOperation__Alternatives12147 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_40_in_rule__OpUnary__Alternatives12180 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_35_in_rule__OpUnary__Alternatives12200 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_34_in_rule__OpUnary__Alternatives12220 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_41_in_rule__OpPostfix__Alternatives12255 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_42_in_rule__OpPostfix__Alternatives12275 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XMemberFeatureCall__Group_1_0__0_in_rule__XMemberFeatureCall__Alternatives_112309 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XMemberFeatureCall__Group_1_1__0_in_rule__XMemberFeatureCall__Alternatives_112327 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_43_in_rule__XMemberFeatureCall__Alternatives_1_0_0_0_112361 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XMemberFeatureCall__ExplicitStaticAssignment_1_0_0_0_1_1_in_rule__XMemberFeatureCall__Alternatives_1_0_0_0_112380 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_43_in_rule__XMemberFeatureCall__Alternatives_1_1_0_0_112414 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XMemberFeatureCall__NullSafeAssignment_1_1_0_0_1_1_in_rule__XMemberFeatureCall__Alternatives_1_1_0_0_112433 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XMemberFeatureCall__ExplicitStaticAssignment_1_1_0_0_1_2_in_rule__XMemberFeatureCall__Alternatives_1_1_0_0_112451 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XMemberFeatureCall__MemberCallArgumentsAssignment_1_1_3_1_0_in_rule__XMemberFeatureCall__Alternatives_1_1_3_112484 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XMemberFeatureCall__Group_1_1_3_1_1__0_in_rule__XMemberFeatureCall__Alternatives_1_1_3_112502 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXConstructorCall_in_rule__XPrimaryExpression__Alternatives12535 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXBlockExpression_in_rule__XPrimaryExpression__Alternatives12552 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXSwitchExpression_in_rule__XPrimaryExpression__Alternatives12569 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXSynchronizedExpression_in_rule__XPrimaryExpression__Alternatives12587 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXFeatureCall_in_rule__XPrimaryExpression__Alternatives12605 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXLiteral_in_rule__XPrimaryExpression__Alternatives12622 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXIfExpression_in_rule__XPrimaryExpression__Alternatives12639 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXForLoopExpression_in_rule__XPrimaryExpression__Alternatives12657 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXBasicForLoopExpression_in_rule__XPrimaryExpression__Alternatives12675 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXWhileExpression_in_rule__XPrimaryExpression__Alternatives12692 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXDoWhileExpression_in_rule__XPrimaryExpression__Alternatives12709 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXThrowExpression_in_rule__XPrimaryExpression__Alternatives12726 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXReturnExpression_in_rule__XPrimaryExpression__Alternatives12743 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXTryCatchFinallyExpression_in_rule__XPrimaryExpression__Alternatives12760 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXParenthesizedExpression_in_rule__XPrimaryExpression__Alternatives12777 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXCollectionLiteral_in_rule__XLiteral__Alternatives12809 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXClosure_in_rule__XLiteral__Alternatives12827 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXBooleanLiteral_in_rule__XLiteral__Alternatives12845 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXNumberLiteral_in_rule__XLiteral__Alternatives12862 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXNullLiteral_in_rule__XLiteral__Alternatives12879 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXStringLiteral_in_rule__XLiteral__Alternatives12896 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXTypeLiteral_in_rule__XLiteral__Alternatives12913 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXSetLiteral_in_rule__XCollectionLiteral__Alternatives12945 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXListLiteral_in_rule__XCollectionLiteral__Alternatives12962 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XSwitchExpression__Group_2_0__0_in_rule__XSwitchExpression__Alternatives_212994 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XSwitchExpression__Group_2_1__0_in_rule__XSwitchExpression__Alternatives_213012 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XCasePart__Group_3_0__0_in_rule__XCasePart__Alternatives_313045 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XCasePart__FallThroughAssignment_3_1_in_rule__XCasePart__Alternatives_313063 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXVariableDeclaration_in_rule__XExpressionOrVarDeclaration__Alternatives13096 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXExpression_in_rule__XExpressionOrVarDeclaration__Alternatives13113 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XVariableDeclaration__WriteableAssignment_1_0_in_rule__XVariableDeclaration__Alternatives_113145 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_44_in_rule__XVariableDeclaration__Alternatives_113164 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XVariableDeclaration__Group_2_0__0_in_rule__XVariableDeclaration__Alternatives_213198 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XVariableDeclaration__NameAssignment_2_1_in_rule__XVariableDeclaration__Alternatives_213216 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XFeatureCall__FeatureCallArgumentsAssignment_3_1_0_in_rule__XFeatureCall__Alternatives_3_113249 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XFeatureCall__Group_3_1_1__0_in_rule__XFeatureCall__Alternatives_3_113267 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleValidID_in_rule__FeatureCallID__Alternatives13300 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_45_in_rule__FeatureCallID__Alternatives13318 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_46_in_rule__FeatureCallID__Alternatives13338 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_47_in_rule__FeatureCallID__Alternatives13358 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_48_in_rule__FeatureCallID__Alternatives13378 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleFeatureCallID_in_rule__IdOrSuper__Alternatives13412 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_49_in_rule__IdOrSuper__Alternatives13430 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XConstructorCall__ArgumentsAssignment_4_1_0_in_rule__XConstructorCall__Alternatives_4_113464 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XConstructorCall__Group_4_1_1__0_in_rule__XConstructorCall__Alternatives_4_113482 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_50_in_rule__XBooleanLiteral__Alternatives_113516 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XBooleanLiteral__IsTrueAssignment_1_1_in_rule__XBooleanLiteral__Alternatives_113535 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XTryCatchFinallyExpression__Group_3_0__0_in_rule__XTryCatchFinallyExpression__Alternatives_313568 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XTryCatchFinallyExpression__Group_3_1__0_in_rule__XTryCatchFinallyExpression__Alternatives_313586 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_RULE_HEX_in_rule__Number__Alternatives13619 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Number__Group_1__0_in_rule__Number__Alternatives13636 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_RULE_INT_in_rule__Number__Alternatives_1_013669 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_RULE_DECIMAL_in_rule__Number__Alternatives_1_013686 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_RULE_INT_in_rule__Number__Alternatives_1_1_113718 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_RULE_DECIMAL_in_rule__Number__Alternatives_1_1_113735 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__JvmTypeReference__Group_0__0_in_rule__JvmTypeReference__Alternatives13767 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXFunctionTypeRef_in_rule__JvmTypeReference__Alternatives13785 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleJvmTypeReference_in_rule__JvmArgumentTypeReference__Alternatives13817 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleJvmWildcardTypeReference_in_rule__JvmArgumentTypeReference__Alternatives13834 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__JvmWildcardTypeReference__Group_2_0__0_in_rule__JvmWildcardTypeReference__Alternatives_213866 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__JvmWildcardTypeReference__Group_2_1__0_in_rule__JvmWildcardTypeReference__Alternatives_213884 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XImportDeclaration__Group_1_0__0_in_rule__XImportDeclaration__Alternatives_113917 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XImportDeclaration__ImportedTypeAssignment_1_1_in_rule__XImportDeclaration__Alternatives_113935 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XImportDeclaration__ImportedNamespaceAssignment_1_2_in_rule__XImportDeclaration__Alternatives_113953 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XImportDeclaration__WildcardAssignment_1_0_3_0_in_rule__XImportDeclaration__Alternatives_1_0_313986 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XImportDeclaration__MemberNameAssignment_1_0_3_1_in_rule__XImportDeclaration__Alternatives_1_0_314004 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_51_in_rule__IdGeneratorStrategy__Alternatives14038 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_52_in_rule__IdGeneratorStrategy__Alternatives14059 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_53_in_rule__Cardinality__Alternatives14095 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_54_in_rule__Cardinality__Alternatives14116 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_55_in_rule__Cardinality__Alternatives14137 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_56_in_rule__SimpleTypes__Alternatives14173 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_57_in_rule__SimpleTypes__Alternatives14194 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_58_in_rule__SimpleTypes__Alternatives14215 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_59_in_rule__SimpleTypes__Alternatives14236 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_60_in_rule__SimpleTypes__Alternatives14257 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_61_in_rule__SimpleTypes__Alternatives14278 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_62_in_rule__IntegerControlInputType__Alternatives14314 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_63_in_rule__IntegerControlInputType__Alternatives14335 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_64_in_rule__ReferenceControlType__Alternatives14371 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_65_in_rule__ReferenceControlType__Alternatives14392 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__ModelRoot__Group_0__0__Impl_in_rule__ModelRoot__Group_0__014425 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000004L});
public static final BitSet FOLLOW_rule__ModelRoot__Group_0__1_in_rule__ModelRoot__Group_0__014428 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__ModelRoot__ImportSectionAssignment_0_0_in_rule__ModelRoot__Group_0__0__Impl14455 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__ModelRoot__Group_0__1__Impl_in_rule__ModelRoot__Group_0__114486 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__ModelRoot__ModelRootAssignment_0_1_in_rule__ModelRoot__Group_0__1__Impl14513 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Model__Group__0__Impl_in_rule__Model__Group__014547 = new BitSet(new long[]{0x0000000000000010L});
public static final BitSet FOLLOW_rule__Model__Group__1_in_rule__Model__Group__014550 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_66_in_rule__Model__Group__0__Impl14578 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Model__Group__1__Impl_in_rule__Model__Group__114609 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000008L});
public static final BitSet FOLLOW_rule__Model__Group__2_in_rule__Model__Group__114612 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Model__ModelNameAssignment_1_in_rule__Model__Group__1__Impl14639 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Model__Group__2__Impl_in_rule__Model__Group__214669 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000030L});
public static final BitSet FOLLOW_rule__Model__Group__3_in_rule__Model__Group__214672 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_67_in_rule__Model__Group__2__Impl14700 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Model__Group__3__Impl_in_rule__Model__Group__314731 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000030L});
public static final BitSet FOLLOW_rule__Model__Group__4_in_rule__Model__Group__314734 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Model__ElementsAssignment_3_in_rule__Model__Group__3__Impl14761 = new BitSet(new long[]{0x0000000000000002L,0x0000000000000020L});
public static final BitSet FOLLOW_rule__Model__Group__4__Impl_in_rule__Model__Group__414792 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_68_in_rule__Model__Group__4__Impl14820 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__PackageDeclaration__Group__0__Impl_in_rule__PackageDeclaration__Group__014861 = new BitSet(new long[]{0x0000000000000010L});
public static final BitSet FOLLOW_rule__PackageDeclaration__Group__1_in_rule__PackageDeclaration__Group__014864 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_69_in_rule__PackageDeclaration__Group__0__Impl14892 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__PackageDeclaration__Group__1__Impl_in_rule__PackageDeclaration__Group__114923 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000008L});
public static final BitSet FOLLOW_rule__PackageDeclaration__Group__2_in_rule__PackageDeclaration__Group__114926 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__PackageDeclaration__PackageNameAssignment_1_in_rule__PackageDeclaration__Group__1__Impl14953 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__PackageDeclaration__Group__2__Impl_in_rule__PackageDeclaration__Group__214983 = new BitSet(new long[]{0x0000000000000000L,0x0000003AB5C54070L,0x0000000000141800L});
public static final BitSet FOLLOW_rule__PackageDeclaration__Group__3_in_rule__PackageDeclaration__Group__214986 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_67_in_rule__PackageDeclaration__Group__2__Impl15014 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__PackageDeclaration__Group__3__Impl_in_rule__PackageDeclaration__Group__315045 = new BitSet(new long[]{0x0000000000000000L,0x0000003AB5C54070L,0x0000000000141800L});
public static final BitSet FOLLOW_rule__PackageDeclaration__Group__4_in_rule__PackageDeclaration__Group__315048 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__PackageDeclaration__ElementsAssignment_3_in_rule__PackageDeclaration__Group__3__Impl15075 = new BitSet(new long[]{0x0000000000000002L,0x0000003AB5C54060L,0x0000000000141800L});
public static final BitSet FOLLOW_rule__PackageDeclaration__Group__4__Impl_in_rule__PackageDeclaration__Group__415106 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_68_in_rule__PackageDeclaration__Group__4__Impl15134 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Enumeration__Group__0__Impl_in_rule__Enumeration__Group__015175 = new BitSet(new long[]{0x0000000000000010L});
public static final BitSet FOLLOW_rule__Enumeration__Group__1_in_rule__Enumeration__Group__015178 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_70_in_rule__Enumeration__Group__0__Impl15206 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Enumeration__Group__1__Impl_in_rule__Enumeration__Group__115237 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000008L});
public static final BitSet FOLLOW_rule__Enumeration__Group__2_in_rule__Enumeration__Group__115240 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Enumeration__NameAssignment_1_in_rule__Enumeration__Group__1__Impl15267 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Enumeration__Group__2__Impl_in_rule__Enumeration__Group__215297 = new BitSet(new long[]{0x0000000000000010L,0x0000000000000010L});
public static final BitSet FOLLOW_rule__Enumeration__Group__3_in_rule__Enumeration__Group__215300 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_67_in_rule__Enumeration__Group__2__Impl15328 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Enumeration__Group__3__Impl_in_rule__Enumeration__Group__315359 = new BitSet(new long[]{0x0000000000000010L,0x0000000000000010L});
public static final BitSet FOLLOW_rule__Enumeration__Group__4_in_rule__Enumeration__Group__315362 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Enumeration__EnumerationValuesAssignment_3_in_rule__Enumeration__Group__3__Impl15389 = new BitSet(new long[]{0x0000000000000012L});
public static final BitSet FOLLOW_rule__Enumeration__Group__4__Impl_in_rule__Enumeration__Group__415420 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_68_in_rule__Enumeration__Group__4__Impl15448 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__EnumerationValue__Group__0__Impl_in_rule__EnumerationValue__Group__015489 = new BitSet(new long[]{0x0000000080000000L});
public static final BitSet FOLLOW_rule__EnumerationValue__Group__1_in_rule__EnumerationValue__Group__015492 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__EnumerationValue__NameAssignment_0_in_rule__EnumerationValue__Group__0__Impl15519 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__EnumerationValue__Group__1__Impl_in_rule__EnumerationValue__Group__115549 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__EnumerationValue__Group_1__0_in_rule__EnumerationValue__Group__1__Impl15576 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__EnumerationValue__Group_1__0__Impl_in_rule__EnumerationValue__Group_1__015611 = new BitSet(new long[]{0x0000000000000100L});
public static final BitSet FOLLOW_rule__EnumerationValue__Group_1__1_in_rule__EnumerationValue__Group_1__015614 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_31_in_rule__EnumerationValue__Group_1__0__Impl15642 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__EnumerationValue__Group_1__1__Impl_in_rule__EnumerationValue__Group_1__115673 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__EnumerationValue__ValueAssignment_1_1_in_rule__EnumerationValue__Group_1__1__Impl15700 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__EntityNaturalKeyFields__Group__0__Impl_in_rule__EntityNaturalKeyFields__Group__015734 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000080L});
public static final BitSet FOLLOW_rule__EntityNaturalKeyFields__Group__1_in_rule__EntityNaturalKeyFields__Group__015737 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__EntityNaturalKeyFields__Group__1__Impl_in_rule__EntityNaturalKeyFields__Group__115795 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000008L});
public static final BitSet FOLLOW_rule__EntityNaturalKeyFields__Group__2_in_rule__EntityNaturalKeyFields__Group__115798 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_71_in_rule__EntityNaturalKeyFields__Group__1__Impl15826 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__EntityNaturalKeyFields__Group__2__Impl_in_rule__EntityNaturalKeyFields__Group__215857 = new BitSet(new long[]{0x0000000000000010L});
public static final BitSet FOLLOW_rule__EntityNaturalKeyFields__Group__3_in_rule__EntityNaturalKeyFields__Group__215860 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_67_in_rule__EntityNaturalKeyFields__Group__2__Impl15888 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__EntityNaturalKeyFields__Group__3__Impl_in_rule__EntityNaturalKeyFields__Group__315919 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000110L});
public static final BitSet FOLLOW_rule__EntityNaturalKeyFields__Group__4_in_rule__EntityNaturalKeyFields__Group__315922 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__EntityNaturalKeyFields__NaturalKeyAttributesAssignment_3_in_rule__EntityNaturalKeyFields__Group__3__Impl15949 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__EntityNaturalKeyFields__Group__4__Impl_in_rule__EntityNaturalKeyFields__Group__415979 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000110L});
public static final BitSet FOLLOW_rule__EntityNaturalKeyFields__Group__5_in_rule__EntityNaturalKeyFields__Group__415982 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__EntityNaturalKeyFields__Group_4__0_in_rule__EntityNaturalKeyFields__Group__4__Impl16009 = new BitSet(new long[]{0x0000000000000002L,0x0000000000000100L});
public static final BitSet FOLLOW_rule__EntityNaturalKeyFields__Group__5__Impl_in_rule__EntityNaturalKeyFields__Group__516040 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_68_in_rule__EntityNaturalKeyFields__Group__5__Impl16068 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__EntityNaturalKeyFields__Group_4__0__Impl_in_rule__EntityNaturalKeyFields__Group_4__016111 = new BitSet(new long[]{0x0000000000000010L});
public static final BitSet FOLLOW_rule__EntityNaturalKeyFields__Group_4__1_in_rule__EntityNaturalKeyFields__Group_4__016114 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_72_in_rule__EntityNaturalKeyFields__Group_4__0__Impl16142 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__EntityNaturalKeyFields__Group_4__1__Impl_in_rule__EntityNaturalKeyFields__Group_4__116173 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__EntityNaturalKeyFields__NaturalKeyAttributesAssignment_4_1_in_rule__EntityNaturalKeyFields__Group_4__1__Impl16200 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__EntityHierarchical__Group__0__Impl_in_rule__EntityHierarchical__Group__016234 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000200L});
public static final BitSet FOLLOW_rule__EntityHierarchical__Group__1_in_rule__EntityHierarchical__Group__016237 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__EntityHierarchical__Group__1__Impl_in_rule__EntityHierarchical__Group__116295 = new BitSet(new long[]{0x0004000000000000L,0x0000000000000000L,0x0200000000000000L});
public static final BitSet FOLLOW_rule__EntityHierarchical__Group__2_in_rule__EntityHierarchical__Group__116298 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_73_in_rule__EntityHierarchical__Group__1__Impl16326 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__EntityHierarchical__Group__2__Impl_in_rule__EntityHierarchical__Group__216357 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__EntityHierarchical__HierarchicalAssignment_2_in_rule__EntityHierarchical__Group__2__Impl16384 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__EntityDisableIdField__Group__0__Impl_in_rule__EntityDisableIdField__Group__016420 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000400L});
public static final BitSet FOLLOW_rule__EntityDisableIdField__Group__1_in_rule__EntityDisableIdField__Group__016423 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__EntityDisableIdField__Group__1__Impl_in_rule__EntityDisableIdField__Group__116481 = new BitSet(new long[]{0x0004000000000000L,0x0000000000000000L,0x0200000000000000L});
public static final BitSet FOLLOW_rule__EntityDisableIdField__Group__2_in_rule__EntityDisableIdField__Group__116484 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_74_in_rule__EntityDisableIdField__Group__1__Impl16512 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__EntityDisableIdField__Group__2__Impl_in_rule__EntityDisableIdField__Group__216543 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__EntityDisableIdField__DisableIdFieldAssignment_2_in_rule__EntityDisableIdField__Group__2__Impl16570 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__EntityLabelField__Group__0__Impl_in_rule__EntityLabelField__Group__016606 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000800L});
public static final BitSet FOLLOW_rule__EntityLabelField__Group__1_in_rule__EntityLabelField__Group__016609 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__EntityLabelField__Group__1__Impl_in_rule__EntityLabelField__Group__116667 = new BitSet(new long[]{0x0000000000000100L});
public static final BitSet FOLLOW_rule__EntityLabelField__Group__2_in_rule__EntityLabelField__Group__116670 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_75_in_rule__EntityLabelField__Group__1__Impl16698 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__EntityLabelField__Group__2__Impl_in_rule__EntityLabelField__Group__216729 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__EntityLabelField__LabelAssignment_2_in_rule__EntityLabelField__Group__2__Impl16756 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__EntityPluralLabelField__Group__0__Impl_in_rule__EntityPluralLabelField__Group__016793 = new BitSet(new long[]{0x0000000000000000L,0x0000000000001000L});
public static final BitSet FOLLOW_rule__EntityPluralLabelField__Group__1_in_rule__EntityPluralLabelField__Group__016796 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__EntityPluralLabelField__Group__1__Impl_in_rule__EntityPluralLabelField__Group__116854 = new BitSet(new long[]{0x0000000000000100L});
public static final BitSet FOLLOW_rule__EntityPluralLabelField__Group__2_in_rule__EntityPluralLabelField__Group__116857 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_76_in_rule__EntityPluralLabelField__Group__1__Impl16885 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__EntityPluralLabelField__Group__2__Impl_in_rule__EntityPluralLabelField__Group__216916 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__EntityPluralLabelField__PluralLabelAssignment_2_in_rule__EntityPluralLabelField__Group__2__Impl16943 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__EntityOptionsContainer__Group__0__Impl_in_rule__EntityOptionsContainer__Group__016981 = new BitSet(new long[]{0x0000000000000000L,0x0000000000002000L});
public static final BitSet FOLLOW_rule__EntityOptionsContainer__Group__1_in_rule__EntityOptionsContainer__Group__016984 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__EntityOptionsContainer__Group__1__Impl_in_rule__EntityOptionsContainer__Group__117042 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000008L});
public static final BitSet FOLLOW_rule__EntityOptionsContainer__Group__2_in_rule__EntityOptionsContainer__Group__117045 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_77_in_rule__EntityOptionsContainer__Group__1__Impl17073 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__EntityOptionsContainer__Group__2__Impl_in_rule__EntityOptionsContainer__Group__217104 = new BitSet(new long[]{0x0000000000000000L,0x0000000000001E90L});
public static final BitSet FOLLOW_rule__EntityOptionsContainer__Group__3_in_rule__EntityOptionsContainer__Group__217107 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_67_in_rule__EntityOptionsContainer__Group__2__Impl17135 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__EntityOptionsContainer__Group__3__Impl_in_rule__EntityOptionsContainer__Group__317166 = new BitSet(new long[]{0x0000000000000000L,0x0000000000001E90L});
public static final BitSet FOLLOW_rule__EntityOptionsContainer__Group__4_in_rule__EntityOptionsContainer__Group__317169 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__EntityOptionsContainer__OptionsAssignment_3_in_rule__EntityOptionsContainer__Group__3__Impl17196 = new BitSet(new long[]{0x0000000000000002L,0x0000000000001E80L});
public static final BitSet FOLLOW_rule__EntityOptionsContainer__Group__4__Impl_in_rule__EntityOptionsContainer__Group__417227 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_68_in_rule__EntityOptionsContainer__Group__4__Impl17255 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Entity__Group__0__Impl_in_rule__Entity__Group__017296 = new BitSet(new long[]{0x0000000000000010L});
public static final BitSet FOLLOW_rule__Entity__Group__1_in_rule__Entity__Group__017299 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_78_in_rule__Entity__Group__0__Impl17327 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Entity__Group__1__Impl_in_rule__Entity__Group__117358 = new BitSet(new long[]{0x0000200000000000L,0x0000000000008008L});
public static final BitSet FOLLOW_rule__Entity__Group__2_in_rule__Entity__Group__117361 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Entity__NameAssignment_1_in_rule__Entity__Group__1__Impl17388 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Entity__Group__2__Impl_in_rule__Entity__Group__217418 = new BitSet(new long[]{0x0000200000000000L,0x0000000000008008L});
public static final BitSet FOLLOW_rule__Entity__Group__3_in_rule__Entity__Group__217421 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Entity__Group_2__0_in_rule__Entity__Group__2__Impl17448 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Entity__Group__3__Impl_in_rule__Entity__Group__317479 = new BitSet(new long[]{0x0000200000000000L,0x0000000000008008L});
public static final BitSet FOLLOW_rule__Entity__Group__4_in_rule__Entity__Group__317482 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Entity__Group_3__0_in_rule__Entity__Group__3__Impl17509 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Entity__Group__4__Impl_in_rule__Entity__Group__417540 = new BitSet(new long[]{0x1B00000000000000L,0x000000054A216050L});
public static final BitSet FOLLOW_rule__Entity__Group__5_in_rule__Entity__Group__417543 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_67_in_rule__Entity__Group__4__Impl17571 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Entity__Group__5__Impl_in_rule__Entity__Group__517602 = new BitSet(new long[]{0x1B00000000000000L,0x000000054A216050L});
public static final BitSet FOLLOW_rule__Entity__Group__6_in_rule__Entity__Group__517605 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Entity__EntityOptionsAssignment_5_in_rule__Entity__Group__5__Impl17632 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Entity__Group__6__Impl_in_rule__Entity__Group__617663 = new BitSet(new long[]{0x1B00000000000000L,0x000000054A216050L});
public static final BitSet FOLLOW_rule__Entity__Group__7_in_rule__Entity__Group__617666 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Entity__AttributesAssignment_6_in_rule__Entity__Group__6__Impl17693 = new BitSet(new long[]{0x1B00000000000002L,0x000000054A214040L});
public static final BitSet FOLLOW_rule__Entity__Group__7__Impl_in_rule__Entity__Group__717724 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_68_in_rule__Entity__Group__7__Impl17752 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Entity__Group_2__0__Impl_in_rule__Entity__Group_2__017799 = new BitSet(new long[]{0x0000000000000010L});
public static final BitSet FOLLOW_rule__Entity__Group_2__1_in_rule__Entity__Group_2__017802 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_45_in_rule__Entity__Group_2__0__Impl17830 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Entity__Group_2__1__Impl_in_rule__Entity__Group_2__117861 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Entity__ExtendsAssignment_2_1_in_rule__Entity__Group_2__1__Impl17888 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Entity__Group_3__0__Impl_in_rule__Entity__Group_3__017922 = new BitSet(new long[]{0x0000000080000010L,0x0000000000000000L,0x0000000000010000L});
public static final BitSet FOLLOW_rule__Entity__Group_3__1_in_rule__Entity__Group_3__017925 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_79_in_rule__Entity__Group_3__0__Impl17953 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Entity__Group_3__1__Impl_in_rule__Entity__Group_3__117984 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Entity__JvmtypeAssignment_3_1_in_rule__Entity__Group_3__1__Impl18011 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__ValueObject__Group__0__Impl_in_rule__ValueObject__Group__018045 = new BitSet(new long[]{0x0000000000000010L});
public static final BitSet FOLLOW_rule__ValueObject__Group__1_in_rule__ValueObject__Group__018048 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_80_in_rule__ValueObject__Group__0__Impl18076 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__ValueObject__Group__1__Impl_in_rule__ValueObject__Group__118107 = new BitSet(new long[]{0x0000200000000000L,0x0000000000008008L});
public static final BitSet FOLLOW_rule__ValueObject__Group__2_in_rule__ValueObject__Group__118110 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__ValueObject__NameAssignment_1_in_rule__ValueObject__Group__1__Impl18137 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__ValueObject__Group__2__Impl_in_rule__ValueObject__Group__218167 = new BitSet(new long[]{0x0000200000000000L,0x0000000000008008L});
public static final BitSet FOLLOW_rule__ValueObject__Group__3_in_rule__ValueObject__Group__218170 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__ValueObject__Group_2__0_in_rule__ValueObject__Group__2__Impl18197 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__ValueObject__Group__3__Impl_in_rule__ValueObject__Group__318228 = new BitSet(new long[]{0x0000200000000000L,0x0000000000008008L});
public static final BitSet FOLLOW_rule__ValueObject__Group__4_in_rule__ValueObject__Group__318231 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__ValueObject__Group_3__0_in_rule__ValueObject__Group__3__Impl18258 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__ValueObject__Group__4__Impl_in_rule__ValueObject__Group__418289 = new BitSet(new long[]{0x1B00000000000000L,0x000000054A214050L});
public static final BitSet FOLLOW_rule__ValueObject__Group__5_in_rule__ValueObject__Group__418292 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_67_in_rule__ValueObject__Group__4__Impl18320 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__ValueObject__Group__5__Impl_in_rule__ValueObject__Group__518351 = new BitSet(new long[]{0x1B00000000000000L,0x000000054A214050L});
public static final BitSet FOLLOW_rule__ValueObject__Group__6_in_rule__ValueObject__Group__518354 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__ValueObject__AttributesAssignment_5_in_rule__ValueObject__Group__5__Impl18381 = new BitSet(new long[]{0x1B00000000000002L,0x000000054A214040L});
public static final BitSet FOLLOW_rule__ValueObject__Group__6__Impl_in_rule__ValueObject__Group__618412 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_68_in_rule__ValueObject__Group__6__Impl18440 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__ValueObject__Group_2__0__Impl_in_rule__ValueObject__Group_2__018485 = new BitSet(new long[]{0x0000000000000010L});
public static final BitSet FOLLOW_rule__ValueObject__Group_2__1_in_rule__ValueObject__Group_2__018488 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_45_in_rule__ValueObject__Group_2__0__Impl18516 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__ValueObject__Group_2__1__Impl_in_rule__ValueObject__Group_2__118547 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__ValueObject__ExtendsAssignment_2_1_in_rule__ValueObject__Group_2__1__Impl18574 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__ValueObject__Group_3__0__Impl_in_rule__ValueObject__Group_3__018608 = new BitSet(new long[]{0x0000000080000010L,0x0000000000000000L,0x0000000000010000L});
public static final BitSet FOLLOW_rule__ValueObject__Group_3__1_in_rule__ValueObject__Group_3__018611 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_79_in_rule__ValueObject__Group_3__0__Impl18639 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__ValueObject__Group_3__1__Impl_in_rule__ValueObject__Group_3__118670 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__ValueObject__JvmtypeAssignment_3_1_in_rule__ValueObject__Group_3__1__Impl18697 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__BaseDataType__Group__0__Impl_in_rule__BaseDataType__Group__018733 = new BitSet(new long[]{0x0000000000000000L,0x0000000000020800L});
public static final BitSet FOLLOW_rule__BaseDataType__Group__1_in_rule__BaseDataType__Group__018736 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__BaseDataType__Group__1__Impl_in_rule__BaseDataType__Group__118794 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__BaseDataType__BaseDatatypePropertiesAssignment_1_in_rule__BaseDataType__Group__1__Impl18821 = new BitSet(new long[]{0x0000000000000002L,0x0000000000020800L});
public static final BitSet FOLLOW_rule__BaseDataTypeWidth__Group__0__Impl_in_rule__BaseDataTypeWidth__Group__018856 = new BitSet(new long[]{0x0000000000000000L,0x0000000000020000L});
public static final BitSet FOLLOW_rule__BaseDataTypeWidth__Group__1_in_rule__BaseDataTypeWidth__Group__018859 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__BaseDataTypeWidth__Group__1__Impl_in_rule__BaseDataTypeWidth__Group__118917 = new BitSet(new long[]{0x0000000000000040L});
public static final BitSet FOLLOW_rule__BaseDataTypeWidth__Group__2_in_rule__BaseDataTypeWidth__Group__118920 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_81_in_rule__BaseDataTypeWidth__Group__1__Impl18948 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__BaseDataTypeWidth__Group__2__Impl_in_rule__BaseDataTypeWidth__Group__218979 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__BaseDataTypeWidth__WidthAssignment_2_in_rule__BaseDataTypeWidth__Group__2__Impl19006 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__BaseDataTypeLabel__Group__0__Impl_in_rule__BaseDataTypeLabel__Group__019043 = new BitSet(new long[]{0x0000000000000000L,0x0000000000020800L});
public static final BitSet FOLLOW_rule__BaseDataTypeLabel__Group__1_in_rule__BaseDataTypeLabel__Group__019046 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__BaseDataTypeLabel__Group__1__Impl_in_rule__BaseDataTypeLabel__Group__119104 = new BitSet(new long[]{0x0000000000000100L});
public static final BitSet FOLLOW_rule__BaseDataTypeLabel__Group__2_in_rule__BaseDataTypeLabel__Group__119107 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_75_in_rule__BaseDataTypeLabel__Group__1__Impl19135 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__BaseDataTypeLabel__Group__2__Impl_in_rule__BaseDataTypeLabel__Group__219166 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__BaseDataTypeLabel__LabelAssignment_2_in_rule__BaseDataTypeLabel__Group__2__Impl19193 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__StringDataType__Group__0__Impl_in_rule__StringDataType__Group__019229 = new BitSet(new long[]{0x0000000000000010L});
public static final BitSet FOLLOW_rule__StringDataType__Group__1_in_rule__StringDataType__Group__019232 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_82_in_rule__StringDataType__Group__0__Impl19260 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__StringDataType__Group__1__Impl_in_rule__StringDataType__Group__119291 = new BitSet(new long[]{0x0000200000000000L,0x0000000000000008L});
public static final BitSet FOLLOW_rule__StringDataType__Group__2_in_rule__StringDataType__Group__119294 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__StringDataType__NameAssignment_1_in_rule__StringDataType__Group__1__Impl19321 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__StringDataType__Group__2__Impl_in_rule__StringDataType__Group__219351 = new BitSet(new long[]{0x0000200000000000L,0x0000000000000008L});
public static final BitSet FOLLOW_rule__StringDataType__Group__3_in_rule__StringDataType__Group__219354 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__StringDataType__Group_2__0_in_rule__StringDataType__Group__2__Impl19381 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__StringDataType__Group__3__Impl_in_rule__StringDataType__Group__319412 = new BitSet(new long[]{0x0000000000000000L,0x0000000000020800L});
public static final BitSet FOLLOW_rule__StringDataType__Group__4_in_rule__StringDataType__Group__319415 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_67_in_rule__StringDataType__Group__3__Impl19443 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__StringDataType__Group__4__Impl_in_rule__StringDataType__Group__419474 = new BitSet(new long[]{0x0000000000000000L,0x0000000000180010L});
public static final BitSet FOLLOW_rule__StringDataType__Group__5_in_rule__StringDataType__Group__419477 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__StringDataType__BaseDataTypeAssignment_4_in_rule__StringDataType__Group__4__Impl19504 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__StringDataType__Group__5__Impl_in_rule__StringDataType__Group__519534 = new BitSet(new long[]{0x0000000000000000L,0x0000000000180010L});
public static final BitSet FOLLOW_rule__StringDataType__Group__6_in_rule__StringDataType__Group__519537 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__StringDataType__Group_5__0_in_rule__StringDataType__Group__5__Impl19564 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__StringDataType__Group__6__Impl_in_rule__StringDataType__Group__619595 = new BitSet(new long[]{0x0000000000000000L,0x0000000000180010L});
public static final BitSet FOLLOW_rule__StringDataType__Group__7_in_rule__StringDataType__Group__619598 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__StringDataType__Group_6__0_in_rule__StringDataType__Group__6__Impl19625 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__StringDataType__Group__7__Impl_in_rule__StringDataType__Group__719656 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_68_in_rule__StringDataType__Group__7__Impl19684 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__StringDataType__Group_2__0__Impl_in_rule__StringDataType__Group_2__019731 = new BitSet(new long[]{0x0000000000000010L});
public static final BitSet FOLLOW_rule__StringDataType__Group_2__1_in_rule__StringDataType__Group_2__019734 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_45_in_rule__StringDataType__Group_2__0__Impl19762 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__StringDataType__Group_2__1__Impl_in_rule__StringDataType__Group_2__119793 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__StringDataType__RefAssignment_2_1_in_rule__StringDataType__Group_2__1__Impl19820 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__StringDataType__Group_5__0__Impl_in_rule__StringDataType__Group_5__019854 = new BitSet(new long[]{0x0000000000000040L});
public static final BitSet FOLLOW_rule__StringDataType__Group_5__1_in_rule__StringDataType__Group_5__019857 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_83_in_rule__StringDataType__Group_5__0__Impl19885 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__StringDataType__Group_5__1__Impl_in_rule__StringDataType__Group_5__119916 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__StringDataType__MaxLengthAssignment_5_1_in_rule__StringDataType__Group_5__1__Impl19943 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__StringDataType__Group_6__0__Impl_in_rule__StringDataType__Group_6__019977 = new BitSet(new long[]{0x0000000000000040L});
public static final BitSet FOLLOW_rule__StringDataType__Group_6__1_in_rule__StringDataType__Group_6__019980 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_84_in_rule__StringDataType__Group_6__0__Impl20008 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__StringDataType__Group_6__1__Impl_in_rule__StringDataType__Group_6__120039 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__StringDataType__MinLengthAssignment_6_1_in_rule__StringDataType__Group_6__1__Impl20066 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__StringEntityAttribute__Group__0__Impl_in_rule__StringEntityAttribute__Group__020100 = new BitSet(new long[]{0x00E0000000000010L});
public static final BitSet FOLLOW_rule__StringEntityAttribute__Group__1_in_rule__StringEntityAttribute__Group__020103 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_59_in_rule__StringEntityAttribute__Group__0__Impl20131 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__StringEntityAttribute__Group__1__Impl_in_rule__StringEntityAttribute__Group__120162 = new BitSet(new long[]{0x00E0000000000010L});
public static final BitSet FOLLOW_rule__StringEntityAttribute__Group__2_in_rule__StringEntityAttribute__Group__120165 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__StringEntityAttribute__TypeAssignment_1_in_rule__StringEntityAttribute__Group__1__Impl20192 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__StringEntityAttribute__Group__2__Impl_in_rule__StringEntityAttribute__Group__220223 = new BitSet(new long[]{0x00E0000000000010L});
public static final BitSet FOLLOW_rule__StringEntityAttribute__Group__3_in_rule__StringEntityAttribute__Group__220226 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__StringEntityAttribute__CardinalityAssignment_2_in_rule__StringEntityAttribute__Group__2__Impl20253 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__StringEntityAttribute__Group__3__Impl_in_rule__StringEntityAttribute__Group__320284 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__StringEntityAttribute__NameAssignment_3_in_rule__StringEntityAttribute__Group__3__Impl20311 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__MapEntityAttribute__Group__0__Impl_in_rule__MapEntityAttribute__Group__020351 = new BitSet(new long[]{0x3F00000000000010L});
public static final BitSet FOLLOW_rule__MapEntityAttribute__Group__1_in_rule__MapEntityAttribute__Group__020354 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_85_in_rule__MapEntityAttribute__Group__0__Impl20382 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__MapEntityAttribute__Group__1__Impl_in_rule__MapEntityAttribute__Group__120413 = new BitSet(new long[]{0x3F00000000000010L});
public static final BitSet FOLLOW_rule__MapEntityAttribute__Group__2_in_rule__MapEntityAttribute__Group__120416 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__MapEntityAttribute__TypeAssignment_1_in_rule__MapEntityAttribute__Group__1__Impl20443 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__MapEntityAttribute__Group__2__Impl_in_rule__MapEntityAttribute__Group__220474 = new BitSet(new long[]{0x3F00000000000010L});
public static final BitSet FOLLOW_rule__MapEntityAttribute__Group__3_in_rule__MapEntityAttribute__Group__220477 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__MapEntityAttribute__Group_2__0_in_rule__MapEntityAttribute__Group__2__Impl20504 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__MapEntityAttribute__Group__3__Impl_in_rule__MapEntityAttribute__Group__320535 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__MapEntityAttribute__NameAssignment_3_in_rule__MapEntityAttribute__Group__3__Impl20562 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__MapEntityAttribute__Group_2__0__Impl_in_rule__MapEntityAttribute__Group_2__020600 = new BitSet(new long[]{0x3F00000000000000L});
public static final BitSet FOLLOW_rule__MapEntityAttribute__Group_2__1_in_rule__MapEntityAttribute__Group_2__020603 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__MapEntityAttribute__KeyTypeAssignment_2_0_in_rule__MapEntityAttribute__Group_2__0__Impl20630 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__MapEntityAttribute__Group_2__1__Impl_in_rule__MapEntityAttribute__Group_2__120660 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__MapEntityAttribute__ValueTypeAssignment_2_1_in_rule__MapEntityAttribute__Group_2__1__Impl20687 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__BooleanDataType__Group__0__Impl_in_rule__BooleanDataType__Group__020721 = new BitSet(new long[]{0x0000000000000010L});
public static final BitSet FOLLOW_rule__BooleanDataType__Group__1_in_rule__BooleanDataType__Group__020724 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_86_in_rule__BooleanDataType__Group__0__Impl20752 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__BooleanDataType__Group__1__Impl_in_rule__BooleanDataType__Group__120783 = new BitSet(new long[]{0x0000200000000000L,0x0000000000000008L});
public static final BitSet FOLLOW_rule__BooleanDataType__Group__2_in_rule__BooleanDataType__Group__120786 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__BooleanDataType__NameAssignment_1_in_rule__BooleanDataType__Group__1__Impl20813 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__BooleanDataType__Group__2__Impl_in_rule__BooleanDataType__Group__220843 = new BitSet(new long[]{0x0000200000000000L,0x0000000000000008L});
public static final BitSet FOLLOW_rule__BooleanDataType__Group__3_in_rule__BooleanDataType__Group__220846 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__BooleanDataType__Group_2__0_in_rule__BooleanDataType__Group__2__Impl20873 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__BooleanDataType__Group__3__Impl_in_rule__BooleanDataType__Group__320904 = new BitSet(new long[]{0x0000000000000000L,0x0000000000020800L});
public static final BitSet FOLLOW_rule__BooleanDataType__Group__4_in_rule__BooleanDataType__Group__320907 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_67_in_rule__BooleanDataType__Group__3__Impl20935 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__BooleanDataType__Group__4__Impl_in_rule__BooleanDataType__Group__420966 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000010L});
public static final BitSet FOLLOW_rule__BooleanDataType__Group__5_in_rule__BooleanDataType__Group__420969 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__BooleanDataType__BaseDataTypeAssignment_4_in_rule__BooleanDataType__Group__4__Impl20996 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__BooleanDataType__Group__5__Impl_in_rule__BooleanDataType__Group__521026 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_68_in_rule__BooleanDataType__Group__5__Impl21054 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__BooleanDataType__Group_2__0__Impl_in_rule__BooleanDataType__Group_2__021097 = new BitSet(new long[]{0x0000000000000010L});
public static final BitSet FOLLOW_rule__BooleanDataType__Group_2__1_in_rule__BooleanDataType__Group_2__021100 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_45_in_rule__BooleanDataType__Group_2__0__Impl21128 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__BooleanDataType__Group_2__1__Impl_in_rule__BooleanDataType__Group_2__121159 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__BooleanDataType__RefAssignment_2_1_in_rule__BooleanDataType__Group_2__1__Impl21186 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__BooleanEntityAttribute__Group__0__Impl_in_rule__BooleanEntityAttribute__Group__021220 = new BitSet(new long[]{0x0000000000000010L});
public static final BitSet FOLLOW_rule__BooleanEntityAttribute__Group__1_in_rule__BooleanEntityAttribute__Group__021223 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_60_in_rule__BooleanEntityAttribute__Group__0__Impl21251 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__BooleanEntityAttribute__Group__1__Impl_in_rule__BooleanEntityAttribute__Group__121282 = new BitSet(new long[]{0x0000000000000010L});
public static final BitSet FOLLOW_rule__BooleanEntityAttribute__Group__2_in_rule__BooleanEntityAttribute__Group__121285 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__BooleanEntityAttribute__TypeAssignment_1_in_rule__BooleanEntityAttribute__Group__1__Impl21312 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__BooleanEntityAttribute__Group__2__Impl_in_rule__BooleanEntityAttribute__Group__221343 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__BooleanEntityAttribute__NameAssignment_2_in_rule__BooleanEntityAttribute__Group__2__Impl21370 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__IntegerDataType__Group__0__Impl_in_rule__IntegerDataType__Group__021406 = new BitSet(new long[]{0x0000000000000010L});
public static final BitSet FOLLOW_rule__IntegerDataType__Group__1_in_rule__IntegerDataType__Group__021409 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_87_in_rule__IntegerDataType__Group__0__Impl21437 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__IntegerDataType__Group__1__Impl_in_rule__IntegerDataType__Group__121468 = new BitSet(new long[]{0x0000200000000000L,0x0000000000000008L});
public static final BitSet FOLLOW_rule__IntegerDataType__Group__2_in_rule__IntegerDataType__Group__121471 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__IntegerDataType__NameAssignment_1_in_rule__IntegerDataType__Group__1__Impl21498 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__IntegerDataType__Group__2__Impl_in_rule__IntegerDataType__Group__221528 = new BitSet(new long[]{0x0000200000000000L,0x0000000000000008L});
public static final BitSet FOLLOW_rule__IntegerDataType__Group__3_in_rule__IntegerDataType__Group__221531 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__IntegerDataType__Group_2__0_in_rule__IntegerDataType__Group__2__Impl21558 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__IntegerDataType__Group__3__Impl_in_rule__IntegerDataType__Group__321589 = new BitSet(new long[]{0x0000000000000000L,0x0000000000020800L});
public static final BitSet FOLLOW_rule__IntegerDataType__Group__4_in_rule__IntegerDataType__Group__321592 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_67_in_rule__IntegerDataType__Group__3__Impl21620 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__IntegerDataType__Group__4__Impl_in_rule__IntegerDataType__Group__421651 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000010L});
public static final BitSet FOLLOW_rule__IntegerDataType__Group__5_in_rule__IntegerDataType__Group__421654 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__IntegerDataType__BaseDataTypeAssignment_4_in_rule__IntegerDataType__Group__4__Impl21681 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__IntegerDataType__Group__5__Impl_in_rule__IntegerDataType__Group__521711 = new BitSet(new long[]{0x0000000000000002L});
}
protected static class FollowSets001 {
public static final BitSet FOLLOW_68_in_rule__IntegerDataType__Group__5__Impl21739 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__IntegerDataType__Group_2__0__Impl_in_rule__IntegerDataType__Group_2__021782 = new BitSet(new long[]{0x0000000000000010L});
public static final BitSet FOLLOW_rule__IntegerDataType__Group_2__1_in_rule__IntegerDataType__Group_2__021785 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_45_in_rule__IntegerDataType__Group_2__0__Impl21813 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__IntegerDataType__Group_2__1__Impl_in_rule__IntegerDataType__Group_2__121844 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__IntegerDataType__RefAssignment_2_1_in_rule__IntegerDataType__Group_2__1__Impl21871 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__IntegerEntityAttribute__Group__0__Impl_in_rule__IntegerEntityAttribute__Group__021905 = new BitSet(new long[]{0x0000000000000010L});
public static final BitSet FOLLOW_rule__IntegerEntityAttribute__Group__1_in_rule__IntegerEntityAttribute__Group__021908 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_57_in_rule__IntegerEntityAttribute__Group__0__Impl21936 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__IntegerEntityAttribute__Group__1__Impl_in_rule__IntegerEntityAttribute__Group__121967 = new BitSet(new long[]{0x0000000000000010L});
public static final BitSet FOLLOW_rule__IntegerEntityAttribute__Group__2_in_rule__IntegerEntityAttribute__Group__121970 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__IntegerEntityAttribute__TypeAssignment_1_in_rule__IntegerEntityAttribute__Group__1__Impl21997 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__IntegerEntityAttribute__Group__2__Impl_in_rule__IntegerEntityAttribute__Group__222028 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__IntegerEntityAttribute__NameAssignment_2_in_rule__IntegerEntityAttribute__Group__2__Impl22055 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DateDataType__Group__0__Impl_in_rule__DateDataType__Group__022091 = new BitSet(new long[]{0x0000000000000010L});
public static final BitSet FOLLOW_rule__DateDataType__Group__1_in_rule__DateDataType__Group__022094 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_88_in_rule__DateDataType__Group__0__Impl22122 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DateDataType__Group__1__Impl_in_rule__DateDataType__Group__122153 = new BitSet(new long[]{0x0000200000000000L,0x0000000000000008L});
public static final BitSet FOLLOW_rule__DateDataType__Group__2_in_rule__DateDataType__Group__122156 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DateDataType__NameAssignment_1_in_rule__DateDataType__Group__1__Impl22183 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DateDataType__Group__2__Impl_in_rule__DateDataType__Group__222213 = new BitSet(new long[]{0x0000200000000000L,0x0000000000000008L});
public static final BitSet FOLLOW_rule__DateDataType__Group__3_in_rule__DateDataType__Group__222216 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DateDataType__Group_2__0_in_rule__DateDataType__Group__2__Impl22243 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DateDataType__Group__3__Impl_in_rule__DateDataType__Group__322274 = new BitSet(new long[]{0x0000000000000000L,0x0000000000020800L});
public static final BitSet FOLLOW_rule__DateDataType__Group__4_in_rule__DateDataType__Group__322277 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_67_in_rule__DateDataType__Group__3__Impl22305 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DateDataType__Group__4__Impl_in_rule__DateDataType__Group__422336 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000010L});
public static final BitSet FOLLOW_rule__DateDataType__Group__5_in_rule__DateDataType__Group__422339 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DateDataType__BaseDataTypeAssignment_4_in_rule__DateDataType__Group__4__Impl22366 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DateDataType__Group__5__Impl_in_rule__DateDataType__Group__522396 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_68_in_rule__DateDataType__Group__5__Impl22424 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DateDataType__Group_2__0__Impl_in_rule__DateDataType__Group_2__022467 = new BitSet(new long[]{0x0000000000000010L});
public static final BitSet FOLLOW_rule__DateDataType__Group_2__1_in_rule__DateDataType__Group_2__022470 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_45_in_rule__DateDataType__Group_2__0__Impl22498 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DateDataType__Group_2__1__Impl_in_rule__DateDataType__Group_2__122529 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DateDataType__RefAssignment_2_1_in_rule__DateDataType__Group_2__1__Impl22556 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DateEntityAttribute__Group__0__Impl_in_rule__DateEntityAttribute__Group__022590 = new BitSet(new long[]{0x0000000000000010L});
public static final BitSet FOLLOW_rule__DateEntityAttribute__Group__1_in_rule__DateEntityAttribute__Group__022593 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_89_in_rule__DateEntityAttribute__Group__0__Impl22621 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DateEntityAttribute__Group__1__Impl_in_rule__DateEntityAttribute__Group__122652 = new BitSet(new long[]{0x0000000000000010L});
public static final BitSet FOLLOW_rule__DateEntityAttribute__Group__2_in_rule__DateEntityAttribute__Group__122655 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DateEntityAttribute__TypeAssignment_1_in_rule__DateEntityAttribute__Group__1__Impl22682 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DateEntityAttribute__Group__2__Impl_in_rule__DateEntityAttribute__Group__222713 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DateEntityAttribute__NameAssignment_2_in_rule__DateEntityAttribute__Group__2__Impl22740 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DecimalDataType__Group__0__Impl_in_rule__DecimalDataType__Group__022776 = new BitSet(new long[]{0x0000000000000010L});
public static final BitSet FOLLOW_rule__DecimalDataType__Group__1_in_rule__DecimalDataType__Group__022779 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_90_in_rule__DecimalDataType__Group__0__Impl22807 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DecimalDataType__Group__1__Impl_in_rule__DecimalDataType__Group__122838 = new BitSet(new long[]{0x0000200000000000L,0x0000000000000008L});
public static final BitSet FOLLOW_rule__DecimalDataType__Group__2_in_rule__DecimalDataType__Group__122841 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DecimalDataType__NameAssignment_1_in_rule__DecimalDataType__Group__1__Impl22868 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DecimalDataType__Group__2__Impl_in_rule__DecimalDataType__Group__222898 = new BitSet(new long[]{0x0000200000000000L,0x0000000000000008L});
public static final BitSet FOLLOW_rule__DecimalDataType__Group__3_in_rule__DecimalDataType__Group__222901 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DecimalDataType__Group_2__0_in_rule__DecimalDataType__Group__2__Impl22928 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DecimalDataType__Group__3__Impl_in_rule__DecimalDataType__Group__322959 = new BitSet(new long[]{0x0000000000000000L,0x0000000000020800L});
public static final BitSet FOLLOW_rule__DecimalDataType__Group__4_in_rule__DecimalDataType__Group__322962 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_67_in_rule__DecimalDataType__Group__3__Impl22990 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DecimalDataType__Group__4__Impl_in_rule__DecimalDataType__Group__423021 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000010L});
public static final BitSet FOLLOW_rule__DecimalDataType__Group__5_in_rule__DecimalDataType__Group__423024 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DecimalDataType__BaseDataTypeAssignment_4_in_rule__DecimalDataType__Group__4__Impl23051 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DecimalDataType__Group__5__Impl_in_rule__DecimalDataType__Group__523081 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_68_in_rule__DecimalDataType__Group__5__Impl23109 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DecimalDataType__Group_2__0__Impl_in_rule__DecimalDataType__Group_2__023152 = new BitSet(new long[]{0x0000000000000010L});
public static final BitSet FOLLOW_rule__DecimalDataType__Group_2__1_in_rule__DecimalDataType__Group_2__023155 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_45_in_rule__DecimalDataType__Group_2__0__Impl23183 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DecimalDataType__Group_2__1__Impl_in_rule__DecimalDataType__Group_2__123214 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DecimalDataType__RefAssignment_2_1_in_rule__DecimalDataType__Group_2__1__Impl23241 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DecimalEntityAttribute__Group__0__Impl_in_rule__DecimalEntityAttribute__Group__023275 = new BitSet(new long[]{0x0000000000000010L});
public static final BitSet FOLLOW_rule__DecimalEntityAttribute__Group__1_in_rule__DecimalEntityAttribute__Group__023278 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_91_in_rule__DecimalEntityAttribute__Group__0__Impl23306 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DecimalEntityAttribute__Group__1__Impl_in_rule__DecimalEntityAttribute__Group__123337 = new BitSet(new long[]{0x0000000000000010L});
public static final BitSet FOLLOW_rule__DecimalEntityAttribute__Group__2_in_rule__DecimalEntityAttribute__Group__123340 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DecimalEntityAttribute__TypeAssignment_1_in_rule__DecimalEntityAttribute__Group__1__Impl23367 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DecimalEntityAttribute__Group__2__Impl_in_rule__DecimalEntityAttribute__Group__223398 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DecimalEntityAttribute__NameAssignment_2_in_rule__DecimalEntityAttribute__Group__2__Impl23425 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__LongDataType__Group__0__Impl_in_rule__LongDataType__Group__023461 = new BitSet(new long[]{0x0000000000000010L});
public static final BitSet FOLLOW_rule__LongDataType__Group__1_in_rule__LongDataType__Group__023464 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_92_in_rule__LongDataType__Group__0__Impl23492 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__LongDataType__Group__1__Impl_in_rule__LongDataType__Group__123523 = new BitSet(new long[]{0x0000200000000000L,0x0000000000000008L});
public static final BitSet FOLLOW_rule__LongDataType__Group__2_in_rule__LongDataType__Group__123526 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__LongDataType__NameAssignment_1_in_rule__LongDataType__Group__1__Impl23553 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__LongDataType__Group__2__Impl_in_rule__LongDataType__Group__223583 = new BitSet(new long[]{0x0000200000000000L,0x0000000000000008L});
public static final BitSet FOLLOW_rule__LongDataType__Group__3_in_rule__LongDataType__Group__223586 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__LongDataType__Group_2__0_in_rule__LongDataType__Group__2__Impl23613 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__LongDataType__Group__3__Impl_in_rule__LongDataType__Group__323644 = new BitSet(new long[]{0x0000000000000000L,0x0000000000020800L});
public static final BitSet FOLLOW_rule__LongDataType__Group__4_in_rule__LongDataType__Group__323647 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_67_in_rule__LongDataType__Group__3__Impl23675 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__LongDataType__Group__4__Impl_in_rule__LongDataType__Group__423706 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000010L});
public static final BitSet FOLLOW_rule__LongDataType__Group__5_in_rule__LongDataType__Group__423709 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__LongDataType__BaseDataTypeAssignment_4_in_rule__LongDataType__Group__4__Impl23736 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__LongDataType__Group__5__Impl_in_rule__LongDataType__Group__523766 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_68_in_rule__LongDataType__Group__5__Impl23794 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__LongDataType__Group_2__0__Impl_in_rule__LongDataType__Group_2__023837 = new BitSet(new long[]{0x0000000000000010L});
public static final BitSet FOLLOW_rule__LongDataType__Group_2__1_in_rule__LongDataType__Group_2__023840 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_45_in_rule__LongDataType__Group_2__0__Impl23868 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__LongDataType__Group_2__1__Impl_in_rule__LongDataType__Group_2__123899 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__LongDataType__RefAssignment_2_1_in_rule__LongDataType__Group_2__1__Impl23926 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__LongEntityAttribute__Group__0__Impl_in_rule__LongEntityAttribute__Group__023960 = new BitSet(new long[]{0x0000000000000010L});
public static final BitSet FOLLOW_rule__LongEntityAttribute__Group__1_in_rule__LongEntityAttribute__Group__023963 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_56_in_rule__LongEntityAttribute__Group__0__Impl23991 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__LongEntityAttribute__Group__1__Impl_in_rule__LongEntityAttribute__Group__124022 = new BitSet(new long[]{0x0000000000000010L});
public static final BitSet FOLLOW_rule__LongEntityAttribute__Group__2_in_rule__LongEntityAttribute__Group__124025 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__LongEntityAttribute__TypeAssignment_1_in_rule__LongEntityAttribute__Group__1__Impl24052 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__LongEntityAttribute__Group__2__Impl_in_rule__LongEntityAttribute__Group__224083 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__LongEntityAttribute__NameAssignment_2_in_rule__LongEntityAttribute__Group__2__Impl24110 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__FloatDataType__Group__0__Impl_in_rule__FloatDataType__Group__024146 = new BitSet(new long[]{0x0000000000000010L});
public static final BitSet FOLLOW_rule__FloatDataType__Group__1_in_rule__FloatDataType__Group__024149 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_93_in_rule__FloatDataType__Group__0__Impl24177 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__FloatDataType__Group__1__Impl_in_rule__FloatDataType__Group__124208 = new BitSet(new long[]{0x0000200000000000L,0x0000000000000008L});
public static final BitSet FOLLOW_rule__FloatDataType__Group__2_in_rule__FloatDataType__Group__124211 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__FloatDataType__NameAssignment_1_in_rule__FloatDataType__Group__1__Impl24238 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__FloatDataType__Group__2__Impl_in_rule__FloatDataType__Group__224268 = new BitSet(new long[]{0x0000200000000000L,0x0000000000000008L});
public static final BitSet FOLLOW_rule__FloatDataType__Group__3_in_rule__FloatDataType__Group__224271 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__FloatDataType__Group_2__0_in_rule__FloatDataType__Group__2__Impl24298 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__FloatDataType__Group__3__Impl_in_rule__FloatDataType__Group__324329 = new BitSet(new long[]{0x0000000000000000L,0x0000000000020800L});
public static final BitSet FOLLOW_rule__FloatDataType__Group__4_in_rule__FloatDataType__Group__324332 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_67_in_rule__FloatDataType__Group__3__Impl24360 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__FloatDataType__Group__4__Impl_in_rule__FloatDataType__Group__424391 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000010L});
public static final BitSet FOLLOW_rule__FloatDataType__Group__5_in_rule__FloatDataType__Group__424394 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__FloatDataType__BaseDataTypeAssignment_4_in_rule__FloatDataType__Group__4__Impl24421 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__FloatDataType__Group__5__Impl_in_rule__FloatDataType__Group__524451 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_68_in_rule__FloatDataType__Group__5__Impl24479 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__FloatDataType__Group_2__0__Impl_in_rule__FloatDataType__Group_2__024522 = new BitSet(new long[]{0x0000000000000010L});
public static final BitSet FOLLOW_rule__FloatDataType__Group_2__1_in_rule__FloatDataType__Group_2__024525 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_45_in_rule__FloatDataType__Group_2__0__Impl24553 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__FloatDataType__Group_2__1__Impl_in_rule__FloatDataType__Group_2__124584 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__FloatDataType__RefAssignment_2_1_in_rule__FloatDataType__Group_2__1__Impl24611 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__FloatEntityAttribute__Group__0__Impl_in_rule__FloatEntityAttribute__Group__024645 = new BitSet(new long[]{0x0000000000000010L});
public static final BitSet FOLLOW_rule__FloatEntityAttribute__Group__1_in_rule__FloatEntityAttribute__Group__024648 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_94_in_rule__FloatEntityAttribute__Group__0__Impl24676 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__FloatEntityAttribute__Group__1__Impl_in_rule__FloatEntityAttribute__Group__124707 = new BitSet(new long[]{0x0000000000000010L});
public static final BitSet FOLLOW_rule__FloatEntityAttribute__Group__2_in_rule__FloatEntityAttribute__Group__124710 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__FloatEntityAttribute__TypeAssignment_1_in_rule__FloatEntityAttribute__Group__1__Impl24737 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__FloatEntityAttribute__Group__2__Impl_in_rule__FloatEntityAttribute__Group__224768 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__FloatEntityAttribute__NameAssignment_2_in_rule__FloatEntityAttribute__Group__2__Impl24795 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DoubleDataType__Group__0__Impl_in_rule__DoubleDataType__Group__024831 = new BitSet(new long[]{0x0000000000000010L});
public static final BitSet FOLLOW_rule__DoubleDataType__Group__1_in_rule__DoubleDataType__Group__024834 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_95_in_rule__DoubleDataType__Group__0__Impl24862 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DoubleDataType__Group__1__Impl_in_rule__DoubleDataType__Group__124893 = new BitSet(new long[]{0x0000200000000000L,0x0000000000000008L});
public static final BitSet FOLLOW_rule__DoubleDataType__Group__2_in_rule__DoubleDataType__Group__124896 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DoubleDataType__NameAssignment_1_in_rule__DoubleDataType__Group__1__Impl24923 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DoubleDataType__Group__2__Impl_in_rule__DoubleDataType__Group__224953 = new BitSet(new long[]{0x0000200000000000L,0x0000000000000008L});
public static final BitSet FOLLOW_rule__DoubleDataType__Group__3_in_rule__DoubleDataType__Group__224956 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DoubleDataType__Group_2__0_in_rule__DoubleDataType__Group__2__Impl24983 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DoubleDataType__Group__3__Impl_in_rule__DoubleDataType__Group__325014 = new BitSet(new long[]{0x0000000000000000L,0x0000000000020800L});
public static final BitSet FOLLOW_rule__DoubleDataType__Group__4_in_rule__DoubleDataType__Group__325017 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_67_in_rule__DoubleDataType__Group__3__Impl25045 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DoubleDataType__Group__4__Impl_in_rule__DoubleDataType__Group__425076 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000010L});
public static final BitSet FOLLOW_rule__DoubleDataType__Group__5_in_rule__DoubleDataType__Group__425079 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DoubleDataType__BaseDataTypeAssignment_4_in_rule__DoubleDataType__Group__4__Impl25106 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DoubleDataType__Group__5__Impl_in_rule__DoubleDataType__Group__525136 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_68_in_rule__DoubleDataType__Group__5__Impl25164 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DoubleDataType__Group_2__0__Impl_in_rule__DoubleDataType__Group_2__025207 = new BitSet(new long[]{0x0000000000000010L});
public static final BitSet FOLLOW_rule__DoubleDataType__Group_2__1_in_rule__DoubleDataType__Group_2__025210 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_45_in_rule__DoubleDataType__Group_2__0__Impl25238 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DoubleDataType__Group_2__1__Impl_in_rule__DoubleDataType__Group_2__125269 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DoubleDataType__RefAssignment_2_1_in_rule__DoubleDataType__Group_2__1__Impl25296 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DoubleEntityAttribute__Group__0__Impl_in_rule__DoubleEntityAttribute__Group__025330 = new BitSet(new long[]{0x0000000000000010L});
public static final BitSet FOLLOW_rule__DoubleEntityAttribute__Group__1_in_rule__DoubleEntityAttribute__Group__025333 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_96_in_rule__DoubleEntityAttribute__Group__0__Impl25361 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DoubleEntityAttribute__Group__1__Impl_in_rule__DoubleEntityAttribute__Group__125392 = new BitSet(new long[]{0x0000000000000010L});
public static final BitSet FOLLOW_rule__DoubleEntityAttribute__Group__2_in_rule__DoubleEntityAttribute__Group__125395 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DoubleEntityAttribute__TypeAssignment_1_in_rule__DoubleEntityAttribute__Group__1__Impl25422 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DoubleEntityAttribute__Group__2__Impl_in_rule__DoubleEntityAttribute__Group__225453 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DoubleEntityAttribute__NameAssignment_2_in_rule__DoubleEntityAttribute__Group__2__Impl25480 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__BinaryDataType__Group__0__Impl_in_rule__BinaryDataType__Group__025516 = new BitSet(new long[]{0x0000000000000010L});
public static final BitSet FOLLOW_rule__BinaryDataType__Group__1_in_rule__BinaryDataType__Group__025519 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_97_in_rule__BinaryDataType__Group__0__Impl25547 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__BinaryDataType__Group__1__Impl_in_rule__BinaryDataType__Group__125578 = new BitSet(new long[]{0x0000200000000000L,0x0000000000000008L});
public static final BitSet FOLLOW_rule__BinaryDataType__Group__2_in_rule__BinaryDataType__Group__125581 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__BinaryDataType__NameAssignment_1_in_rule__BinaryDataType__Group__1__Impl25608 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__BinaryDataType__Group__2__Impl_in_rule__BinaryDataType__Group__225638 = new BitSet(new long[]{0x0000200000000000L,0x0000000000000008L});
public static final BitSet FOLLOW_rule__BinaryDataType__Group__3_in_rule__BinaryDataType__Group__225641 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__BinaryDataType__Group_2__0_in_rule__BinaryDataType__Group__2__Impl25668 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__BinaryDataType__Group__3__Impl_in_rule__BinaryDataType__Group__325699 = new BitSet(new long[]{0x0000000000000000L,0x0000000000020800L});
public static final BitSet FOLLOW_rule__BinaryDataType__Group__4_in_rule__BinaryDataType__Group__325702 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_67_in_rule__BinaryDataType__Group__3__Impl25730 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__BinaryDataType__Group__4__Impl_in_rule__BinaryDataType__Group__425761 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000010L});
public static final BitSet FOLLOW_rule__BinaryDataType__Group__5_in_rule__BinaryDataType__Group__425764 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__BinaryDataType__BaseDataTypeAssignment_4_in_rule__BinaryDataType__Group__4__Impl25791 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__BinaryDataType__Group__5__Impl_in_rule__BinaryDataType__Group__525821 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_68_in_rule__BinaryDataType__Group__5__Impl25849 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__BinaryDataType__Group_2__0__Impl_in_rule__BinaryDataType__Group_2__025892 = new BitSet(new long[]{0x0000000000000010L});
public static final BitSet FOLLOW_rule__BinaryDataType__Group_2__1_in_rule__BinaryDataType__Group_2__025895 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_45_in_rule__BinaryDataType__Group_2__0__Impl25923 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__BinaryDataType__Group_2__1__Impl_in_rule__BinaryDataType__Group_2__125954 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__BinaryDataType__RefAssignment_2_1_in_rule__BinaryDataType__Group_2__1__Impl25981 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__BinaryEntityAttribute__Group__0__Impl_in_rule__BinaryEntityAttribute__Group__026015 = new BitSet(new long[]{0x0000000000000010L});
public static final BitSet FOLLOW_rule__BinaryEntityAttribute__Group__1_in_rule__BinaryEntityAttribute__Group__026018 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_98_in_rule__BinaryEntityAttribute__Group__0__Impl26046 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__BinaryEntityAttribute__Group__1__Impl_in_rule__BinaryEntityAttribute__Group__126077 = new BitSet(new long[]{0x0000000000000010L});
public static final BitSet FOLLOW_rule__BinaryEntityAttribute__Group__2_in_rule__BinaryEntityAttribute__Group__126080 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__BinaryEntityAttribute__TypeAssignment_1_in_rule__BinaryEntityAttribute__Group__1__Impl26107 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__BinaryEntityAttribute__Group__2__Impl_in_rule__BinaryEntityAttribute__Group__226138 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__BinaryEntityAttribute__NameAssignment_2_in_rule__BinaryEntityAttribute__Group__2__Impl26165 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__EntityDataType__Group__0__Impl_in_rule__EntityDataType__Group__026201 = new BitSet(new long[]{0x0000000000000010L});
public static final BitSet FOLLOW_rule__EntityDataType__Group__1_in_rule__EntityDataType__Group__026204 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_99_in_rule__EntityDataType__Group__0__Impl26232 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__EntityDataType__Group__1__Impl_in_rule__EntityDataType__Group__126263 = new BitSet(new long[]{0x0000200000000000L,0x0000000000000008L});
public static final BitSet FOLLOW_rule__EntityDataType__Group__2_in_rule__EntityDataType__Group__126266 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__EntityDataType__NameAssignment_1_in_rule__EntityDataType__Group__1__Impl26293 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__EntityDataType__Group__2__Impl_in_rule__EntityDataType__Group__226323 = new BitSet(new long[]{0x0000200000000000L,0x0000000000000008L});
public static final BitSet FOLLOW_rule__EntityDataType__Group__3_in_rule__EntityDataType__Group__226326 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__EntityDataType__Group_2__0_in_rule__EntityDataType__Group__2__Impl26353 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__EntityDataType__Group__3__Impl_in_rule__EntityDataType__Group__326384 = new BitSet(new long[]{0x0000000000000000L,0x0000000000020800L});
public static final BitSet FOLLOW_rule__EntityDataType__Group__4_in_rule__EntityDataType__Group__326387 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_67_in_rule__EntityDataType__Group__3__Impl26415 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__EntityDataType__Group__4__Impl_in_rule__EntityDataType__Group__426446 = new BitSet(new long[]{0x0000000000000000L,0x0000000000004000L});
public static final BitSet FOLLOW_rule__EntityDataType__Group__5_in_rule__EntityDataType__Group__426449 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__EntityDataType__BaseDataTypeAssignment_4_in_rule__EntityDataType__Group__4__Impl26476 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__EntityDataType__Group__5__Impl_in_rule__EntityDataType__Group__526506 = new BitSet(new long[]{0x0000000000000010L});
public static final BitSet FOLLOW_rule__EntityDataType__Group__6_in_rule__EntityDataType__Group__526509 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_78_in_rule__EntityDataType__Group__5__Impl26537 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__EntityDataType__Group__6__Impl_in_rule__EntityDataType__Group__626568 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000010L});
public static final BitSet FOLLOW_rule__EntityDataType__Group__7_in_rule__EntityDataType__Group__626571 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__EntityDataType__EntityAssignment_6_in_rule__EntityDataType__Group__6__Impl26598 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__EntityDataType__Group__7__Impl_in_rule__EntityDataType__Group__726628 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_68_in_rule__EntityDataType__Group__7__Impl26656 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__EntityDataType__Group_2__0__Impl_in_rule__EntityDataType__Group_2__026703 = new BitSet(new long[]{0x0000000000000010L});
public static final BitSet FOLLOW_rule__EntityDataType__Group_2__1_in_rule__EntityDataType__Group_2__026706 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_45_in_rule__EntityDataType__Group_2__0__Impl26734 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__EntityDataType__Group_2__1__Impl_in_rule__EntityDataType__Group_2__126765 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__EntityDataType__RefAssignment_2_1_in_rule__EntityDataType__Group_2__1__Impl26792 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__EntityEntityAttribute__Group__0__Impl_in_rule__EntityEntityAttribute__Group__026826 = new BitSet(new long[]{0x0000000000000010L});
public static final BitSet FOLLOW_rule__EntityEntityAttribute__Group__1_in_rule__EntityEntityAttribute__Group__026829 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_78_in_rule__EntityEntityAttribute__Group__0__Impl26857 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__EntityEntityAttribute__Group__1__Impl_in_rule__EntityEntityAttribute__Group__126888 = new BitSet(new long[]{0x00E0000000000010L});
public static final BitSet FOLLOW_rule__EntityEntityAttribute__Group__2_in_rule__EntityEntityAttribute__Group__126891 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__EntityEntityAttribute__TypeAssignment_1_in_rule__EntityEntityAttribute__Group__1__Impl26918 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__EntityEntityAttribute__Group__2__Impl_in_rule__EntityEntityAttribute__Group__226948 = new BitSet(new long[]{0x00E0000000000010L});
public static final BitSet FOLLOW_rule__EntityEntityAttribute__Group__3_in_rule__EntityEntityAttribute__Group__226951 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__EntityEntityAttribute__CardinalityAssignment_2_in_rule__EntityEntityAttribute__Group__2__Impl26978 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__EntityEntityAttribute__Group__3__Impl_in_rule__EntityEntityAttribute__Group__327009 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__EntityEntityAttribute__NameAssignment_3_in_rule__EntityEntityAttribute__Group__3__Impl27036 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__ValueObjectEntityAttribute__Group__0__Impl_in_rule__ValueObjectEntityAttribute__Group__027074 = new BitSet(new long[]{0x0000000000000010L});
public static final BitSet FOLLOW_rule__ValueObjectEntityAttribute__Group__1_in_rule__ValueObjectEntityAttribute__Group__027077 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_80_in_rule__ValueObjectEntityAttribute__Group__0__Impl27105 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__ValueObjectEntityAttribute__Group__1__Impl_in_rule__ValueObjectEntityAttribute__Group__127136 = new BitSet(new long[]{0x00E0000000000010L});
public static final BitSet FOLLOW_rule__ValueObjectEntityAttribute__Group__2_in_rule__ValueObjectEntityAttribute__Group__127139 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__ValueObjectEntityAttribute__TypeAssignment_1_in_rule__ValueObjectEntityAttribute__Group__1__Impl27166 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__ValueObjectEntityAttribute__Group__2__Impl_in_rule__ValueObjectEntityAttribute__Group__227196 = new BitSet(new long[]{0x00E0000000000010L});
public static final BitSet FOLLOW_rule__ValueObjectEntityAttribute__Group__3_in_rule__ValueObjectEntityAttribute__Group__227199 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__ValueObjectEntityAttribute__CardinalityAssignment_2_in_rule__ValueObjectEntityAttribute__Group__2__Impl27226 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__ValueObjectEntityAttribute__Group__3__Impl_in_rule__ValueObjectEntityAttribute__Group__327257 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__ValueObjectEntityAttribute__NameAssignment_3_in_rule__ValueObjectEntityAttribute__Group__3__Impl27284 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__EnumerationDataType__Group__0__Impl_in_rule__EnumerationDataType__Group__027322 = new BitSet(new long[]{0x0000000000000010L});
public static final BitSet FOLLOW_rule__EnumerationDataType__Group__1_in_rule__EnumerationDataType__Group__027325 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_100_in_rule__EnumerationDataType__Group__0__Impl27353 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__EnumerationDataType__Group__1__Impl_in_rule__EnumerationDataType__Group__127384 = new BitSet(new long[]{0x0000200000000000L,0x0000000000000008L});
public static final BitSet FOLLOW_rule__EnumerationDataType__Group__2_in_rule__EnumerationDataType__Group__127387 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__EnumerationDataType__NameAssignment_1_in_rule__EnumerationDataType__Group__1__Impl27414 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__EnumerationDataType__Group__2__Impl_in_rule__EnumerationDataType__Group__227444 = new BitSet(new long[]{0x0000200000000000L,0x0000000000000008L});
public static final BitSet FOLLOW_rule__EnumerationDataType__Group__3_in_rule__EnumerationDataType__Group__227447 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__EnumerationDataType__Group_2__0_in_rule__EnumerationDataType__Group__2__Impl27474 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__EnumerationDataType__Group__3__Impl_in_rule__EnumerationDataType__Group__327505 = new BitSet(new long[]{0x0000000000000000L,0x0000000000020800L});
public static final BitSet FOLLOW_rule__EnumerationDataType__Group__4_in_rule__EnumerationDataType__Group__327508 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_67_in_rule__EnumerationDataType__Group__3__Impl27536 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__EnumerationDataType__Group__4__Impl_in_rule__EnumerationDataType__Group__427567 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000040L});
public static final BitSet FOLLOW_rule__EnumerationDataType__Group__5_in_rule__EnumerationDataType__Group__427570 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__EnumerationDataType__BaseDataTypeAssignment_4_in_rule__EnumerationDataType__Group__4__Impl27597 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__EnumerationDataType__Group__5__Impl_in_rule__EnumerationDataType__Group__527627 = new BitSet(new long[]{0x0000000000000010L});
public static final BitSet FOLLOW_rule__EnumerationDataType__Group__6_in_rule__EnumerationDataType__Group__527630 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_70_in_rule__EnumerationDataType__Group__5__Impl27658 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__EnumerationDataType__Group__6__Impl_in_rule__EnumerationDataType__Group__627689 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000010L});
public static final BitSet FOLLOW_rule__EnumerationDataType__Group__7_in_rule__EnumerationDataType__Group__627692 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__EnumerationDataType__EnumerationAssignment_6_in_rule__EnumerationDataType__Group__6__Impl27719 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__EnumerationDataType__Group__7__Impl_in_rule__EnumerationDataType__Group__727749 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_68_in_rule__EnumerationDataType__Group__7__Impl27777 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__EnumerationDataType__Group_2__0__Impl_in_rule__EnumerationDataType__Group_2__027824 = new BitSet(new long[]{0x0000000000000010L});
public static final BitSet FOLLOW_rule__EnumerationDataType__Group_2__1_in_rule__EnumerationDataType__Group_2__027827 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_45_in_rule__EnumerationDataType__Group_2__0__Impl27855 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__EnumerationDataType__Group_2__1__Impl_in_rule__EnumerationDataType__Group_2__127886 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__EnumerationDataType__RefAssignment_2_1_in_rule__EnumerationDataType__Group_2__1__Impl27913 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__EnumerationEntityAttribute__Group__0__Impl_in_rule__EnumerationEntityAttribute__Group__027947 = new BitSet(new long[]{0x0000000000000010L});
public static final BitSet FOLLOW_rule__EnumerationEntityAttribute__Group__1_in_rule__EnumerationEntityAttribute__Group__027950 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_70_in_rule__EnumerationEntityAttribute__Group__0__Impl27978 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__EnumerationEntityAttribute__Group__1__Impl_in_rule__EnumerationEntityAttribute__Group__128009 = new BitSet(new long[]{0x00E0000000000010L});
public static final BitSet FOLLOW_rule__EnumerationEntityAttribute__Group__2_in_rule__EnumerationEntityAttribute__Group__128012 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__EnumerationEntityAttribute__TypeAssignment_1_in_rule__EnumerationEntityAttribute__Group__1__Impl28039 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__EnumerationEntityAttribute__Group__2__Impl_in_rule__EnumerationEntityAttribute__Group__228069 = new BitSet(new long[]{0x00E0000000000010L});
public static final BitSet FOLLOW_rule__EnumerationEntityAttribute__Group__3_in_rule__EnumerationEntityAttribute__Group__228072 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__EnumerationEntityAttribute__CardinalityAssignment_2_in_rule__EnumerationEntityAttribute__Group__2__Impl28099 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__EnumerationEntityAttribute__Group__3__Impl_in_rule__EnumerationEntityAttribute__Group__328130 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__EnumerationEntityAttribute__NameAssignment_3_in_rule__EnumerationEntityAttribute__Group__3__Impl28157 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Dictionary__Group__0__Impl_in_rule__Dictionary__Group__028195 = new BitSet(new long[]{0x0000000000000010L});
public static final BitSet FOLLOW_rule__Dictionary__Group__1_in_rule__Dictionary__Group__028198 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_101_in_rule__Dictionary__Group__0__Impl28226 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Dictionary__Group__1__Impl_in_rule__Dictionary__Group__128257 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000008L});
public static final BitSet FOLLOW_rule__Dictionary__Group__2_in_rule__Dictionary__Group__128260 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Dictionary__NameAssignment_1_in_rule__Dictionary__Group__1__Impl28287 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Dictionary__Group__2__Impl_in_rule__Dictionary__Group__228317 = new BitSet(new long[]{0x0000000000000000L,0x0000000000004000L});
public static final BitSet FOLLOW_rule__Dictionary__Group__3_in_rule__Dictionary__Group__228320 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_67_in_rule__Dictionary__Group__2__Impl28348 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Dictionary__Group__3__Impl_in_rule__Dictionary__Group__328379 = new BitSet(new long[]{0x0000000000000010L});
public static final BitSet FOLLOW_rule__Dictionary__Group__4_in_rule__Dictionary__Group__328382 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_78_in_rule__Dictionary__Group__3__Impl28410 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Dictionary__Group__4__Impl_in_rule__Dictionary__Group__428441 = new BitSet(new long[]{0x0000000000000000L,0x000003C000001810L});
public static final BitSet FOLLOW_rule__Dictionary__Group__5_in_rule__Dictionary__Group__428444 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Dictionary__EntityAssignment_4_in_rule__Dictionary__Group__4__Impl28471 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Dictionary__Group__5__Impl_in_rule__Dictionary__Group__528501 = new BitSet(new long[]{0x0000000000000000L,0x000003C000001810L});
public static final BitSet FOLLOW_rule__Dictionary__Group__6_in_rule__Dictionary__Group__528504 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Dictionary__Group_5__0_in_rule__Dictionary__Group__5__Impl28531 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Dictionary__Group__6__Impl_in_rule__Dictionary__Group__628562 = new BitSet(new long[]{0x0000000000000000L,0x000003C000001810L});
public static final BitSet FOLLOW_rule__Dictionary__Group__7_in_rule__Dictionary__Group__628565 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Dictionary__Group_6__0_in_rule__Dictionary__Group__6__Impl28592 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Dictionary__Group__7__Impl_in_rule__Dictionary__Group__728623 = new BitSet(new long[]{0x0000000000000000L,0x000003C000001810L});
public static final BitSet FOLLOW_rule__Dictionary__Group__8_in_rule__Dictionary__Group__728626 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Dictionary__Group_7__0_in_rule__Dictionary__Group__7__Impl28653 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Dictionary__Group__8__Impl_in_rule__Dictionary__Group__828684 = new BitSet(new long[]{0x0000000000000000L,0x000003C000001810L});
public static final BitSet FOLLOW_rule__Dictionary__Group__9_in_rule__Dictionary__Group__828687 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Dictionary__Group_8__0_in_rule__Dictionary__Group__8__Impl28714 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Dictionary__Group__9__Impl_in_rule__Dictionary__Group__928745 = new BitSet(new long[]{0x0000000000000000L,0x000003C000001810L});
public static final BitSet FOLLOW_rule__Dictionary__Group__10_in_rule__Dictionary__Group__928748 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Dictionary__DictionarysearchAssignment_9_in_rule__Dictionary__Group__9__Impl28775 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Dictionary__Group__10__Impl_in_rule__Dictionary__Group__1028806 = new BitSet(new long[]{0x0000000000000000L,0x000003C000001810L});
public static final BitSet FOLLOW_rule__Dictionary__Group__11_in_rule__Dictionary__Group__1028809 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Dictionary__DictionaryeditorAssignment_10_in_rule__Dictionary__Group__10__Impl28836 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Dictionary__Group__11__Impl_in_rule__Dictionary__Group__1128867 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_68_in_rule__Dictionary__Group__11__Impl28895 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Dictionary__Group_5__0__Impl_in_rule__Dictionary__Group_5__028950 = new BitSet(new long[]{0x0000000000000100L});
public static final BitSet FOLLOW_rule__Dictionary__Group_5__1_in_rule__Dictionary__Group_5__028953 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_75_in_rule__Dictionary__Group_5__0__Impl28981 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Dictionary__Group_5__1__Impl_in_rule__Dictionary__Group_5__129012 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Dictionary__LabelAssignment_5_1_in_rule__Dictionary__Group_5__1__Impl29039 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Dictionary__Group_6__0__Impl_in_rule__Dictionary__Group_6__029073 = new BitSet(new long[]{0x0000000000000100L});
public static final BitSet FOLLOW_rule__Dictionary__Group_6__1_in_rule__Dictionary__Group_6__029076 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_76_in_rule__Dictionary__Group_6__0__Impl29104 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Dictionary__Group_6__1__Impl_in_rule__Dictionary__Group_6__129135 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Dictionary__PluralLabelAssignment_6_1_in_rule__Dictionary__Group_6__1__Impl29162 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Dictionary__Group_7__0__Impl_in_rule__Dictionary__Group_7__029196 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000008L});
public static final BitSet FOLLOW_rule__Dictionary__Group_7__1_in_rule__Dictionary__Group_7__029199 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_102_in_rule__Dictionary__Group_7__0__Impl29227 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Dictionary__Group_7__1__Impl_in_rule__Dictionary__Group_7__129258 = new BitSet(new long[]{0x0000000000000000L,0xA000000000000010L,0x00000000000005FAL});
public static final BitSet FOLLOW_rule__Dictionary__Group_7__2_in_rule__Dictionary__Group_7__129261 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_67_in_rule__Dictionary__Group_7__1__Impl29289 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Dictionary__Group_7__2__Impl_in_rule__Dictionary__Group_7__229320 = new BitSet(new long[]{0x0000000000000000L,0xA000000000000010L,0x00000000000005FAL});
public static final BitSet FOLLOW_rule__Dictionary__Group_7__3_in_rule__Dictionary__Group_7__229323 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Dictionary__DictionarycontrolsAssignment_7_2_in_rule__Dictionary__Group_7__2__Impl29350 = new BitSet(new long[]{0x0000000000000002L,0xA000000000000000L,0x00000000000005FAL});
public static final BitSet FOLLOW_rule__Dictionary__Group_7__3__Impl_in_rule__Dictionary__Group_7__329381 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_68_in_rule__Dictionary__Group_7__3__Impl29409 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Dictionary__Group_8__0__Impl_in_rule__Dictionary__Group_8__029448 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000008L});
public static final BitSet FOLLOW_rule__Dictionary__Group_8__1_in_rule__Dictionary__Group_8__029451 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_103_in_rule__Dictionary__Group_8__0__Impl29479 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Dictionary__Group_8__1__Impl_in_rule__Dictionary__Group_8__129510 = new BitSet(new long[]{0x0000000000000000L,0xA000000000000010L,0x00000000000005FAL});
public static final BitSet FOLLOW_rule__Dictionary__Group_8__2_in_rule__Dictionary__Group_8__129513 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_67_in_rule__Dictionary__Group_8__1__Impl29541 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Dictionary__Group_8__2__Impl_in_rule__Dictionary__Group_8__229572 = new BitSet(new long[]{0x0000000000000000L,0xA000000000000010L,0x00000000000005FAL});
public static final BitSet FOLLOW_rule__Dictionary__Group_8__3_in_rule__Dictionary__Group_8__229575 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Dictionary__LabelcontrolsAssignment_8_2_in_rule__Dictionary__Group_8__2__Impl29602 = new BitSet(new long[]{0x0000000000000002L,0xA000000000000000L,0x00000000000005FAL});
public static final BitSet FOLLOW_rule__Dictionary__Group_8__3__Impl_in_rule__Dictionary__Group_8__329633 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_68_in_rule__Dictionary__Group_8__3__Impl29661 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionarySearch__Group__0__Impl_in_rule__DictionarySearch__Group__029700 = new BitSet(new long[]{0x0000000000000010L});
public static final BitSet FOLLOW_rule__DictionarySearch__Group__1_in_rule__DictionarySearch__Group__029703 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_104_in_rule__DictionarySearch__Group__0__Impl29731 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionarySearch__Group__1__Impl_in_rule__DictionarySearch__Group__129762 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000008L});
public static final BitSet FOLLOW_rule__DictionarySearch__Group__2_in_rule__DictionarySearch__Group__129765 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionarySearch__NameAssignment_1_in_rule__DictionarySearch__Group__1__Impl29792 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionarySearch__Group__2__Impl_in_rule__DictionarySearch__Group__229822 = new BitSet(new long[]{0x0000000000000000L,0x00000C0000000800L});
public static final BitSet FOLLOW_rule__DictionarySearch__Group__3_in_rule__DictionarySearch__Group__229825 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_67_in_rule__DictionarySearch__Group__2__Impl29853 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionarySearch__Group__3__Impl_in_rule__DictionarySearch__Group__329884 = new BitSet(new long[]{0x0000000000000000L,0x00000C0000000800L});
public static final BitSet FOLLOW_rule__DictionarySearch__Group__4_in_rule__DictionarySearch__Group__329887 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionarySearch__Group_3__0_in_rule__DictionarySearch__Group__3__Impl29914 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionarySearch__Group__4__Impl_in_rule__DictionarySearch__Group__429945 = new BitSet(new long[]{0x0000000000000000L,0x00000C0000000800L});
public static final BitSet FOLLOW_rule__DictionarySearch__Group__5_in_rule__DictionarySearch__Group__429948 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionarySearch__DictionaryfiltersAssignment_4_in_rule__DictionarySearch__Group__4__Impl29975 = new BitSet(new long[]{0x0000000000000002L,0x0000040000000000L});
public static final BitSet FOLLOW_rule__DictionarySearch__Group__5__Impl_in_rule__DictionarySearch__Group__530006 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000010L});
public static final BitSet FOLLOW_rule__DictionarySearch__Group__6_in_rule__DictionarySearch__Group__530009 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionarySearch__DictionaryresultAssignment_5_in_rule__DictionarySearch__Group__5__Impl30036 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionarySearch__Group__6__Impl_in_rule__DictionarySearch__Group__630066 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_68_in_rule__DictionarySearch__Group__6__Impl30094 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionarySearch__Group_3__0__Impl_in_rule__DictionarySearch__Group_3__030139 = new BitSet(new long[]{0x0000000000000100L});
public static final BitSet FOLLOW_rule__DictionarySearch__Group_3__1_in_rule__DictionarySearch__Group_3__030142 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_75_in_rule__DictionarySearch__Group_3__0__Impl30170 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionarySearch__Group_3__1__Impl_in_rule__DictionarySearch__Group_3__130201 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionarySearch__LabelAssignment_3_1_in_rule__DictionarySearch__Group_3__1__Impl30228 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryEditor__Group__0__Impl_in_rule__DictionaryEditor__Group__030262 = new BitSet(new long[]{0x0000000000000010L});
public static final BitSet FOLLOW_rule__DictionaryEditor__Group__1_in_rule__DictionaryEditor__Group__030265 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_105_in_rule__DictionaryEditor__Group__0__Impl30293 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryEditor__Group__1__Impl_in_rule__DictionaryEditor__Group__130324 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000008L});
public static final BitSet FOLLOW_rule__DictionaryEditor__Group__2_in_rule__DictionaryEditor__Group__130327 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryEditor__NameAssignment_1_in_rule__DictionaryEditor__Group__1__Impl30354 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryEditor__Group__2__Impl_in_rule__DictionaryEditor__Group__230384 = new BitSet(new long[]{0x0000000000000000L,0xA013500000000810L,0x00000000000005FAL});
public static final BitSet FOLLOW_rule__DictionaryEditor__Group__3_in_rule__DictionaryEditor__Group__230387 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_67_in_rule__DictionaryEditor__Group__2__Impl30415 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryEditor__Group__3__Impl_in_rule__DictionaryEditor__Group__330446 = new BitSet(new long[]{0x0000000000000000L,0xA013500000000810L,0x00000000000005FAL});
public static final BitSet FOLLOW_rule__DictionaryEditor__Group__4_in_rule__DictionaryEditor__Group__330449 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryEditor__Group_3__0_in_rule__DictionaryEditor__Group__3__Impl30476 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryEditor__Group__4__Impl_in_rule__DictionaryEditor__Group__430507 = new BitSet(new long[]{0x0000000000000000L,0xA013500000000810L,0x00000000000005FAL});
public static final BitSet FOLLOW_rule__DictionaryEditor__Group__5_in_rule__DictionaryEditor__Group__430510 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryEditor__LayoutdataAssignment_4_in_rule__DictionaryEditor__Group__4__Impl30537 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryEditor__Group__5__Impl_in_rule__DictionaryEditor__Group__530568 = new BitSet(new long[]{0x0000000000000000L,0xA013500000000810L,0x00000000000005FAL});
public static final BitSet FOLLOW_rule__DictionaryEditor__Group__6_in_rule__DictionaryEditor__Group__530571 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryEditor__LayoutAssignment_5_in_rule__DictionaryEditor__Group__5__Impl30598 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryEditor__Group__6__Impl_in_rule__DictionaryEditor__Group__630629 = new BitSet(new long[]{0x0000000000000000L,0xA013500000000810L,0x00000000000005FAL});
public static final BitSet FOLLOW_rule__DictionaryEditor__Group__7_in_rule__DictionaryEditor__Group__630632 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryEditor__ContainercontentsAssignment_6_in_rule__DictionaryEditor__Group__6__Impl30659 = new BitSet(new long[]{0x0000000000000002L,0xA013000000000000L,0x00000000000005FAL});
public static final BitSet FOLLOW_rule__DictionaryEditor__Group__7__Impl_in_rule__DictionaryEditor__Group__730690 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_68_in_rule__DictionaryEditor__Group__7__Impl30718 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryEditor__Group_3__0__Impl_in_rule__DictionaryEditor__Group_3__030765 = new BitSet(new long[]{0x0000000000000100L});
public static final BitSet FOLLOW_rule__DictionaryEditor__Group_3__1_in_rule__DictionaryEditor__Group_3__030768 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_75_in_rule__DictionaryEditor__Group_3__0__Impl30796 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryEditor__Group_3__1__Impl_in_rule__DictionaryEditor__Group_3__130827 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryEditor__LabelAssignment_3_1_in_rule__DictionaryEditor__Group_3__1__Impl30854 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryFilter__Group__0__Impl_in_rule__DictionaryFilter__Group__030888 = new BitSet(new long[]{0x0000000000000010L});
public static final BitSet FOLLOW_rule__DictionaryFilter__Group__1_in_rule__DictionaryFilter__Group__030891 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_106_in_rule__DictionaryFilter__Group__0__Impl30919 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryFilter__Group__1__Impl_in_rule__DictionaryFilter__Group__130950 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000008L});
public static final BitSet FOLLOW_rule__DictionaryFilter__Group__2_in_rule__DictionaryFilter__Group__130953 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryFilter__NameAssignment_1_in_rule__DictionaryFilter__Group__1__Impl30980 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryFilter__Group__2__Impl_in_rule__DictionaryFilter__Group__231010 = new BitSet(new long[]{0x0000000000000000L,0xA013500000000010L,0x00000000000005FAL});
public static final BitSet FOLLOW_rule__DictionaryFilter__Group__3_in_rule__DictionaryFilter__Group__231013 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_67_in_rule__DictionaryFilter__Group__2__Impl31041 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryFilter__Group__3__Impl_in_rule__DictionaryFilter__Group__331072 = new BitSet(new long[]{0x0000000000000000L,0xA013500000000010L,0x00000000000005FAL});
public static final BitSet FOLLOW_rule__DictionaryFilter__Group__4_in_rule__DictionaryFilter__Group__331075 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryFilter__LayoutdataAssignment_3_in_rule__DictionaryFilter__Group__3__Impl31102 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryFilter__Group__4__Impl_in_rule__DictionaryFilter__Group__431133 = new BitSet(new long[]{0x0000000000000000L,0xA013500000000010L,0x00000000000005FAL});
public static final BitSet FOLLOW_rule__DictionaryFilter__Group__5_in_rule__DictionaryFilter__Group__431136 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryFilter__LayoutAssignment_4_in_rule__DictionaryFilter__Group__4__Impl31163 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryFilter__Group__5__Impl_in_rule__DictionaryFilter__Group__531194 = new BitSet(new long[]{0x0000000000000000L,0xA013500000000010L,0x00000000000005FAL});
public static final BitSet FOLLOW_rule__DictionaryFilter__Group__6_in_rule__DictionaryFilter__Group__531197 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryFilter__ContainercontentsAssignment_5_in_rule__DictionaryFilter__Group__5__Impl31224 = new BitSet(new long[]{0x0000000000000002L,0xA013000000000000L,0x00000000000005FAL});
public static final BitSet FOLLOW_rule__DictionaryFilter__Group__6__Impl_in_rule__DictionaryFilter__Group__631255 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_68_in_rule__DictionaryFilter__Group__6__Impl31283 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryResult__Group__0__Impl_in_rule__DictionaryResult__Group__031328 = new BitSet(new long[]{0x0000000000000010L});
public static final BitSet FOLLOW_rule__DictionaryResult__Group__1_in_rule__DictionaryResult__Group__031331 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_107_in_rule__DictionaryResult__Group__0__Impl31359 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryResult__Group__1__Impl_in_rule__DictionaryResult__Group__131390 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000008L});
public static final BitSet FOLLOW_rule__DictionaryResult__Group__2_in_rule__DictionaryResult__Group__131393 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryResult__NameAssignment_1_in_rule__DictionaryResult__Group__1__Impl31420 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryResult__Group__2__Impl_in_rule__DictionaryResult__Group__231450 = new BitSet(new long[]{0x0000000000000000L,0xA000000000000010L,0x00000000000005FAL});
public static final BitSet FOLLOW_rule__DictionaryResult__Group__3_in_rule__DictionaryResult__Group__231453 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_67_in_rule__DictionaryResult__Group__2__Impl31481 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryResult__Group__3__Impl_in_rule__DictionaryResult__Group__331512 = new BitSet(new long[]{0x0000000000000000L,0xA000000000000010L,0x00000000000005FAL});
public static final BitSet FOLLOW_rule__DictionaryResult__Group__4_in_rule__DictionaryResult__Group__331515 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryResult__ResultcolumnsAssignment_3_in_rule__DictionaryResult__Group__3__Impl31542 = new BitSet(new long[]{0x0000000000000002L,0xA000000000000000L,0x00000000000005FAL});
public static final BitSet FOLLOW_rule__DictionaryResult__Group__4__Impl_in_rule__DictionaryResult__Group__431573 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_68_in_rule__DictionaryResult__Group__4__Impl31601 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__ColumnLayout__Group__0__Impl_in_rule__ColumnLayout__Group__031642 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000008L});
public static final BitSet FOLLOW_rule__ColumnLayout__Group__1_in_rule__ColumnLayout__Group__031645 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_108_in_rule__ColumnLayout__Group__0__Impl31673 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__ColumnLayout__Group__1__Impl_in_rule__ColumnLayout__Group__131704 = new BitSet(new long[]{0x0000000000000000L,0x0000200000000000L});
public static final BitSet FOLLOW_rule__ColumnLayout__Group__2_in_rule__ColumnLayout__Group__131707 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_67_in_rule__ColumnLayout__Group__1__Impl31735 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__ColumnLayout__Group__2__Impl_in_rule__ColumnLayout__Group__231766 = new BitSet(new long[]{0x0000000000000040L});
public static final BitSet FOLLOW_rule__ColumnLayout__Group__3_in_rule__ColumnLayout__Group__231769 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_109_in_rule__ColumnLayout__Group__2__Impl31797 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__ColumnLayout__Group__3__Impl_in_rule__ColumnLayout__Group__331828 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000010L});
public static final BitSet FOLLOW_rule__ColumnLayout__Group__4_in_rule__ColumnLayout__Group__331831 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__ColumnLayout__ColumnsAssignment_3_in_rule__ColumnLayout__Group__3__Impl31858 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__ColumnLayout__Group__4__Impl_in_rule__ColumnLayout__Group__431888 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_68_in_rule__ColumnLayout__Group__4__Impl31916 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__ColumnLayoutData__Group__0__Impl_in_rule__ColumnLayoutData__Group__031957 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000008L});
public static final BitSet FOLLOW_rule__ColumnLayoutData__Group__1_in_rule__ColumnLayoutData__Group__031960 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_110_in_rule__ColumnLayoutData__Group__0__Impl31988 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__ColumnLayoutData__Group__1__Impl_in_rule__ColumnLayoutData__Group__132019 = new BitSet(new long[]{0x0000000000000000L,0x0000800000000000L});
public static final BitSet FOLLOW_rule__ColumnLayoutData__Group__2_in_rule__ColumnLayoutData__Group__132022 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_67_in_rule__ColumnLayoutData__Group__1__Impl32050 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__ColumnLayoutData__Group__2__Impl_in_rule__ColumnLayoutData__Group__232081 = new BitSet(new long[]{0x0000000000000040L});
public static final BitSet FOLLOW_rule__ColumnLayoutData__Group__3_in_rule__ColumnLayoutData__Group__232084 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_111_in_rule__ColumnLayoutData__Group__2__Impl32112 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__ColumnLayoutData__Group__3__Impl_in_rule__ColumnLayoutData__Group__332143 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000010L});
public static final BitSet FOLLOW_rule__ColumnLayoutData__Group__4_in_rule__ColumnLayoutData__Group__332146 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__ColumnLayoutData__ColumnspanAssignment_3_in_rule__ColumnLayoutData__Group__3__Impl32173 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__ColumnLayoutData__Group__4__Impl_in_rule__ColumnLayoutData__Group__432203 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_68_in_rule__ColumnLayoutData__Group__4__Impl32231 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryComposite__Group__0__Impl_in_rule__DictionaryComposite__Group__032272 = new BitSet(new long[]{0x0000000000000010L});
public static final BitSet FOLLOW_rule__DictionaryComposite__Group__1_in_rule__DictionaryComposite__Group__032275 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_112_in_rule__DictionaryComposite__Group__0__Impl32303 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryComposite__Group__1__Impl_in_rule__DictionaryComposite__Group__132334 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000008L});
public static final BitSet FOLLOW_rule__DictionaryComposite__Group__2_in_rule__DictionaryComposite__Group__132337 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryComposite__NameAssignment_1_in_rule__DictionaryComposite__Group__1__Impl32364 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryComposite__Group__2__Impl_in_rule__DictionaryComposite__Group__232394 = new BitSet(new long[]{0x0000000000000000L,0xA013500000000010L,0x00000000000005FAL});
public static final BitSet FOLLOW_rule__DictionaryComposite__Group__3_in_rule__DictionaryComposite__Group__232397 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_67_in_rule__DictionaryComposite__Group__2__Impl32425 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryComposite__Group__3__Impl_in_rule__DictionaryComposite__Group__332456 = new BitSet(new long[]{0x0000000000000000L,0xA013500000000010L,0x00000000000005FAL});
public static final BitSet FOLLOW_rule__DictionaryComposite__Group__4_in_rule__DictionaryComposite__Group__332459 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryComposite__LayoutdataAssignment_3_in_rule__DictionaryComposite__Group__3__Impl32486 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryComposite__Group__4__Impl_in_rule__DictionaryComposite__Group__432517 = new BitSet(new long[]{0x0000000000000000L,0xA013500000000010L,0x00000000000005FAL});
public static final BitSet FOLLOW_rule__DictionaryComposite__Group__5_in_rule__DictionaryComposite__Group__432520 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryComposite__LayoutAssignment_4_in_rule__DictionaryComposite__Group__4__Impl32547 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryComposite__Group__5__Impl_in_rule__DictionaryComposite__Group__532578 = new BitSet(new long[]{0x0000000000000000L,0xA013500000000010L,0x00000000000005FAL});
public static final BitSet FOLLOW_rule__DictionaryComposite__Group__6_in_rule__DictionaryComposite__Group__532581 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryComposite__ContainercontentsAssignment_5_in_rule__DictionaryComposite__Group__5__Impl32608 = new BitSet(new long[]{0x0000000000000002L,0xA013000000000000L,0x00000000000005FAL});
public static final BitSet FOLLOW_rule__DictionaryComposite__Group__6__Impl_in_rule__DictionaryComposite__Group__632639 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_68_in_rule__DictionaryComposite__Group__6__Impl32667 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryEditableTable__Group__0__Impl_in_rule__DictionaryEditableTable__Group__032712 = new BitSet(new long[]{0x0000000000000010L});
public static final BitSet FOLLOW_rule__DictionaryEditableTable__Group__1_in_rule__DictionaryEditableTable__Group__032715 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_113_in_rule__DictionaryEditableTable__Group__0__Impl32743 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryEditableTable__Group__1__Impl_in_rule__DictionaryEditableTable__Group__132774 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000008L});
public static final BitSet FOLLOW_rule__DictionaryEditableTable__Group__2_in_rule__DictionaryEditableTable__Group__132777 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryEditableTable__NameAssignment_1_in_rule__DictionaryEditableTable__Group__1__Impl32804 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryEditableTable__Group__2__Impl_in_rule__DictionaryEditableTable__Group__232834 = new BitSet(new long[]{0x0000000000000000L,0xA017500000000000L,0x00000000000005FAL});
public static final BitSet FOLLOW_rule__DictionaryEditableTable__Group__3_in_rule__DictionaryEditableTable__Group__232837 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_67_in_rule__DictionaryEditableTable__Group__2__Impl32865 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryEditableTable__Group__3__Impl_in_rule__DictionaryEditableTable__Group__332896 = new BitSet(new long[]{0x0000000000000000L,0xA017500000000000L,0x00000000000005FAL});
public static final BitSet FOLLOW_rule__DictionaryEditableTable__Group__4_in_rule__DictionaryEditableTable__Group__332899 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryEditableTable__Group_3__0_in_rule__DictionaryEditableTable__Group__3__Impl32926 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryEditableTable__Group__4__Impl_in_rule__DictionaryEditableTable__Group__432957 = new BitSet(new long[]{0x0000000000000000L,0xA017500000000000L,0x00000000000005FAL});
public static final BitSet FOLLOW_rule__DictionaryEditableTable__Group__5_in_rule__DictionaryEditableTable__Group__432960 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryEditableTable__Group_4__0_in_rule__DictionaryEditableTable__Group__4__Impl32987 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryEditableTable__Group__5__Impl_in_rule__DictionaryEditableTable__Group__533018 = new BitSet(new long[]{0x0000000000000000L,0xA017500000000000L,0x00000000000005FAL});
public static final BitSet FOLLOW_rule__DictionaryEditableTable__Group__6_in_rule__DictionaryEditableTable__Group__533021 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryEditableTable__ContainercontentsAssignment_5_in_rule__DictionaryEditableTable__Group__5__Impl33048 = new BitSet(new long[]{0x0000000000000002L,0xA013000000000000L,0x00000000000005FAL});
public static final BitSet FOLLOW_rule__DictionaryEditableTable__Group__6__Impl_in_rule__DictionaryEditableTable__Group__633079 = new BitSet(new long[]{0x0000000000000010L});
public static final BitSet FOLLOW_rule__DictionaryEditableTable__Group__7_in_rule__DictionaryEditableTable__Group__633082 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_114_in_rule__DictionaryEditableTable__Group__6__Impl33110 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryEditableTable__Group__7__Impl_in_rule__DictionaryEditableTable__Group__733141 = new BitSet(new long[]{0x0000000000000000L,0x0008000000000000L});
public static final BitSet FOLLOW_rule__DictionaryEditableTable__Group__8_in_rule__DictionaryEditableTable__Group__733144 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryEditableTable__EntityattributeAssignment_7_in_rule__DictionaryEditableTable__Group__7__Impl33171 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryEditableTable__Group__8__Impl_in_rule__DictionaryEditableTable__Group__833201 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000008L});
public static final BitSet FOLLOW_rule__DictionaryEditableTable__Group__9_in_rule__DictionaryEditableTable__Group__833204 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_115_in_rule__DictionaryEditableTable__Group__8__Impl33232 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryEditableTable__Group__9__Impl_in_rule__DictionaryEditableTable__Group__933263 = new BitSet(new long[]{0x0000000000000000L,0xA000000000000010L,0x00000000000005FAL});
public static final BitSet FOLLOW_rule__DictionaryEditableTable__Group__10_in_rule__DictionaryEditableTable__Group__933266 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_67_in_rule__DictionaryEditableTable__Group__9__Impl33294 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryEditableTable__Group__10__Impl_in_rule__DictionaryEditableTable__Group__1033325 = new BitSet(new long[]{0x0000000000000000L,0xA000000000000010L,0x00000000000005FAL});
public static final BitSet FOLLOW_rule__DictionaryEditableTable__Group__11_in_rule__DictionaryEditableTable__Group__1033328 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryEditableTable__ColumncontrolsAssignment_10_in_rule__DictionaryEditableTable__Group__10__Impl33355 = new BitSet(new long[]{0x0000000000000002L,0xA000000000000000L,0x00000000000005FAL});
public static final BitSet FOLLOW_rule__DictionaryEditableTable__Group__11__Impl_in_rule__DictionaryEditableTable__Group__1133386 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000010L});
public static final BitSet FOLLOW_rule__DictionaryEditableTable__Group__12_in_rule__DictionaryEditableTable__Group__1133389 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_68_in_rule__DictionaryEditableTable__Group__11__Impl33417 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryEditableTable__Group__12__Impl_in_rule__DictionaryEditableTable__Group__1233448 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_68_in_rule__DictionaryEditableTable__Group__12__Impl33476 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryEditableTable__Group_3__0__Impl_in_rule__DictionaryEditableTable__Group_3__033533 = new BitSet(new long[]{0x0000000000000000L,0x0000400000000000L});
public static final BitSet FOLLOW_rule__DictionaryEditableTable__Group_3__1_in_rule__DictionaryEditableTable__Group_3__033536 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_110_in_rule__DictionaryEditableTable__Group_3__0__Impl33564 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryEditableTable__Group_3__1__Impl_in_rule__DictionaryEditableTable__Group_3__133595 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryEditableTable__LayoutdataAssignment_3_1_in_rule__DictionaryEditableTable__Group_3__1__Impl33622 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryEditableTable__Group_4__0__Impl_in_rule__DictionaryEditableTable__Group_4__033656 = new BitSet(new long[]{0x0000000000000000L,0x0000100000000000L});
public static final BitSet FOLLOW_rule__DictionaryEditableTable__Group_4__1_in_rule__DictionaryEditableTable__Group_4__033659 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_108_in_rule__DictionaryEditableTable__Group_4__0__Impl33687 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryEditableTable__Group_4__1__Impl_in_rule__DictionaryEditableTable__Group_4__133718 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryEditableTable__LayoutAssignment_4_1_in_rule__DictionaryEditableTable__Group_4__1__Impl33745 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryAssignmentTable__Group__0__Impl_in_rule__DictionaryAssignmentTable__Group__033779 = new BitSet(new long[]{0x0000000000000010L});
public static final BitSet FOLLOW_rule__DictionaryAssignmentTable__Group__1_in_rule__DictionaryAssignmentTable__Group__033782 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_116_in_rule__DictionaryAssignmentTable__Group__0__Impl33810 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryAssignmentTable__Group__1__Impl_in_rule__DictionaryAssignmentTable__Group__133841 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000008L});
public static final BitSet FOLLOW_rule__DictionaryAssignmentTable__Group__2_in_rule__DictionaryAssignmentTable__Group__133844 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryAssignmentTable__NameAssignment_1_in_rule__DictionaryAssignmentTable__Group__1__Impl33871 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryAssignmentTable__Group__2__Impl_in_rule__DictionaryAssignmentTable__Group__233901 = new BitSet(new long[]{0x0000000000000000L,0xA017500000000000L,0x00000000000005FAL});
public static final BitSet FOLLOW_rule__DictionaryAssignmentTable__Group__3_in_rule__DictionaryAssignmentTable__Group__233904 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_67_in_rule__DictionaryAssignmentTable__Group__2__Impl33932 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryAssignmentTable__Group__3__Impl_in_rule__DictionaryAssignmentTable__Group__333963 = new BitSet(new long[]{0x0000000000000000L,0xA017500000000000L,0x00000000000005FAL});
public static final BitSet FOLLOW_rule__DictionaryAssignmentTable__Group__4_in_rule__DictionaryAssignmentTable__Group__333966 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryAssignmentTable__Group_3__0_in_rule__DictionaryAssignmentTable__Group__3__Impl33993 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryAssignmentTable__Group__4__Impl_in_rule__DictionaryAssignmentTable__Group__434024 = new BitSet(new long[]{0x0000000000000000L,0xA017500000000000L,0x00000000000005FAL});
public static final BitSet FOLLOW_rule__DictionaryAssignmentTable__Group__5_in_rule__DictionaryAssignmentTable__Group__434027 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryAssignmentTable__Group_4__0_in_rule__DictionaryAssignmentTable__Group__4__Impl34054 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryAssignmentTable__Group__5__Impl_in_rule__DictionaryAssignmentTable__Group__534085 = new BitSet(new long[]{0x0000000000000000L,0xA017500000000000L,0x00000000000005FAL});
public static final BitSet FOLLOW_rule__DictionaryAssignmentTable__Group__6_in_rule__DictionaryAssignmentTable__Group__534088 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryAssignmentTable__ContainercontentsAssignment_5_in_rule__DictionaryAssignmentTable__Group__5__Impl34115 = new BitSet(new long[]{0x0000000000000002L,0xA013000000000000L,0x00000000000005FAL});
public static final BitSet FOLLOW_rule__DictionaryAssignmentTable__Group__6__Impl_in_rule__DictionaryAssignmentTable__Group__634146 = new BitSet(new long[]{0x0000000000000010L});
public static final BitSet FOLLOW_rule__DictionaryAssignmentTable__Group__7_in_rule__DictionaryAssignmentTable__Group__634149 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_114_in_rule__DictionaryAssignmentTable__Group__6__Impl34177 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryAssignmentTable__Group__7__Impl_in_rule__DictionaryAssignmentTable__Group__734208 = new BitSet(new long[]{0x0000000000000000L,0x0000002000000000L});
public static final BitSet FOLLOW_rule__DictionaryAssignmentTable__Group__8_in_rule__DictionaryAssignmentTable__Group__734211 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryAssignmentTable__EntityattributeAssignment_7_in_rule__DictionaryAssignmentTable__Group__7__Impl34238 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryAssignmentTable__Group__8__Impl_in_rule__DictionaryAssignmentTable__Group__834268 = new BitSet(new long[]{0x0000000000000010L});
public static final BitSet FOLLOW_rule__DictionaryAssignmentTable__Group__9_in_rule__DictionaryAssignmentTable__Group__834271 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_101_in_rule__DictionaryAssignmentTable__Group__8__Impl34299 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryAssignmentTable__Group__9__Impl_in_rule__DictionaryAssignmentTable__Group__934330 = new BitSet(new long[]{0x0000000000000000L,0x0008000000000000L});
public static final BitSet FOLLOW_rule__DictionaryAssignmentTable__Group__10_in_rule__DictionaryAssignmentTable__Group__934333 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryAssignmentTable__DictionaryAssignment_9_in_rule__DictionaryAssignmentTable__Group__9__Impl34360 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryAssignmentTable__Group__10__Impl_in_rule__DictionaryAssignmentTable__Group__1034390 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000008L});
public static final BitSet FOLLOW_rule__DictionaryAssignmentTable__Group__11_in_rule__DictionaryAssignmentTable__Group__1034393 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_115_in_rule__DictionaryAssignmentTable__Group__10__Impl34421 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryAssignmentTable__Group__11__Impl_in_rule__DictionaryAssignmentTable__Group__1134452 = new BitSet(new long[]{0x0000000000000000L,0xA000000000000010L,0x00000000000005FAL});
public static final BitSet FOLLOW_rule__DictionaryAssignmentTable__Group__12_in_rule__DictionaryAssignmentTable__Group__1134455 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_67_in_rule__DictionaryAssignmentTable__Group__11__Impl34483 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryAssignmentTable__Group__12__Impl_in_rule__DictionaryAssignmentTable__Group__1234514 = new BitSet(new long[]{0x0000000000000000L,0xA000000000000010L,0x00000000000005FAL});
public static final BitSet FOLLOW_rule__DictionaryAssignmentTable__Group__13_in_rule__DictionaryAssignmentTable__Group__1234517 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryAssignmentTable__ColumncontrolsAssignment_12_in_rule__DictionaryAssignmentTable__Group__12__Impl34544 = new BitSet(new long[]{0x0000000000000002L,0xA000000000000000L,0x00000000000005FAL});
public static final BitSet FOLLOW_rule__DictionaryAssignmentTable__Group__13__Impl_in_rule__DictionaryAssignmentTable__Group__1334575 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000010L});
public static final BitSet FOLLOW_rule__DictionaryAssignmentTable__Group__14_in_rule__DictionaryAssignmentTable__Group__1334578 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_68_in_rule__DictionaryAssignmentTable__Group__13__Impl34606 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryAssignmentTable__Group__14__Impl_in_rule__DictionaryAssignmentTable__Group__1434637 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_68_in_rule__DictionaryAssignmentTable__Group__14__Impl34665 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryAssignmentTable__Group_3__0__Impl_in_rule__DictionaryAssignmentTable__Group_3__034726 = new BitSet(new long[]{0x0000000000000000L,0x0000400000000000L});
public static final BitSet FOLLOW_rule__DictionaryAssignmentTable__Group_3__1_in_rule__DictionaryAssignmentTable__Group_3__034729 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_110_in_rule__DictionaryAssignmentTable__Group_3__0__Impl34757 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryAssignmentTable__Group_3__1__Impl_in_rule__DictionaryAssignmentTable__Group_3__134788 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryAssignmentTable__LayoutdataAssignment_3_1_in_rule__DictionaryAssignmentTable__Group_3__1__Impl34815 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryAssignmentTable__Group_4__0__Impl_in_rule__DictionaryAssignmentTable__Group_4__034849 = new BitSet(new long[]{0x0000000000000000L,0x0000100000000000L});
public static final BitSet FOLLOW_rule__DictionaryAssignmentTable__Group_4__1_in_rule__DictionaryAssignmentTable__Group_4__034852 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_108_in_rule__DictionaryAssignmentTable__Group_4__0__Impl34880 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryAssignmentTable__Group_4__1__Impl_in_rule__DictionaryAssignmentTable__Group_4__134911 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryAssignmentTable__LayoutAssignment_4_1_in_rule__DictionaryAssignmentTable__Group_4__1__Impl34938 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Labels__Group__0__Impl_in_rule__Labels__Group__034972 = new BitSet(new long[]{0x0000000000000000L,0x01E0000000000800L});
public static final BitSet FOLLOW_rule__Labels__Group__1_in_rule__Labels__Group__034975 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Labels__Group__1__Impl_in_rule__Labels__Group__135033 = new BitSet(new long[]{0x0000000000000000L,0x01E0000000000800L});
public static final BitSet FOLLOW_rule__Labels__Group__2_in_rule__Labels__Group__135036 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Labels__Group_1__0_in_rule__Labels__Group__1__Impl35063 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Labels__Group__2__Impl_in_rule__Labels__Group__235094 = new BitSet(new long[]{0x0000000000000000L,0x01E0000000000800L});
public static final BitSet FOLLOW_rule__Labels__Group__3_in_rule__Labels__Group__235097 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Labels__Group_2__0_in_rule__Labels__Group__2__Impl35124 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Labels__Group__3__Impl_in_rule__Labels__Group__335155 = new BitSet(new long[]{0x0000000000000000L,0x01E0000000000800L});
public static final BitSet FOLLOW_rule__Labels__Group__4_in_rule__Labels__Group__335158 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Labels__Group_3__0_in_rule__Labels__Group__3__Impl35185 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Labels__Group__4__Impl_in_rule__Labels__Group__435216 = new BitSet(new long[]{0x0000000000000000L,0x01E0000000000800L});
public static final BitSet FOLLOW_rule__Labels__Group__5_in_rule__Labels__Group__435219 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Labels__Group_4__0_in_rule__Labels__Group__4__Impl35246 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Labels__Group__5__Impl_in_rule__Labels__Group__535277 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Labels__Group_5__0_in_rule__Labels__Group__5__Impl35304 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Labels__Group_1__0__Impl_in_rule__Labels__Group_1__035347 = new BitSet(new long[]{0x0000000000000100L});
public static final BitSet FOLLOW_rule__Labels__Group_1__1_in_rule__Labels__Group_1__035350 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_75_in_rule__Labels__Group_1__0__Impl35378 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Labels__Group_1__1__Impl_in_rule__Labels__Group_1__135409 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Labels__LabelAssignment_1_1_in_rule__Labels__Group_1__1__Impl35436 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Labels__Group_2__0__Impl_in_rule__Labels__Group_2__035470 = new BitSet(new long[]{0x0000000000000100L});
public static final BitSet FOLLOW_rule__Labels__Group_2__1_in_rule__Labels__Group_2__035473 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_117_in_rule__Labels__Group_2__0__Impl35501 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Labels__Group_2__1__Impl_in_rule__Labels__Group_2__135532 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Labels__FilterLabelAssignment_2_1_in_rule__Labels__Group_2__1__Impl35559 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Labels__Group_3__0__Impl_in_rule__Labels__Group_3__035593 = new BitSet(new long[]{0x0000000000000100L});
public static final BitSet FOLLOW_rule__Labels__Group_3__1_in_rule__Labels__Group_3__035596 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_118_in_rule__Labels__Group_3__0__Impl35624 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Labels__Group_3__1__Impl_in_rule__Labels__Group_3__135655 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Labels__ColumnLabelAssignment_3_1_in_rule__Labels__Group_3__1__Impl35682 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Labels__Group_4__0__Impl_in_rule__Labels__Group_4__035716 = new BitSet(new long[]{0x0000000000000100L});
public static final BitSet FOLLOW_rule__Labels__Group_4__1_in_rule__Labels__Group_4__035719 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_119_in_rule__Labels__Group_4__0__Impl35747 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Labels__Group_4__1__Impl_in_rule__Labels__Group_4__135778 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Labels__EditorLabelAssignment_4_1_in_rule__Labels__Group_4__1__Impl35805 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Labels__Group_5__0__Impl_in_rule__Labels__Group_5__035839 = new BitSet(new long[]{0x0000000000000100L});
public static final BitSet FOLLOW_rule__Labels__Group_5__1_in_rule__Labels__Group_5__035842 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_120_in_rule__Labels__Group_5__0__Impl35870 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Labels__Group_5__1__Impl_in_rule__Labels__Group_5__135901 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Labels__ToolTipAssignment_5_1_in_rule__Labels__Group_5__1__Impl35928 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__BaseDictionaryControl__Group__0__Impl_in_rule__BaseDictionaryControl__Group__035962 = new BitSet(new long[]{0x0000000000000000L,0x03E0000000000800L});
public static final BitSet FOLLOW_rule__BaseDictionaryControl__Group__1_in_rule__BaseDictionaryControl__Group__035965 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__BaseDictionaryControl__Group_0__0_in_rule__BaseDictionaryControl__Group__0__Impl35992 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__BaseDictionaryControl__Group__1__Impl_in_rule__BaseDictionaryControl__Group__136023 = new BitSet(new long[]{0x0000000000000000L,0x03E0000000000800L});
public static final BitSet FOLLOW_rule__BaseDictionaryControl__Group__2_in_rule__BaseDictionaryControl__Group__136026 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__BaseDictionaryControl__Group_1__0_in_rule__BaseDictionaryControl__Group__1__Impl36053 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__BaseDictionaryControl__Group__2__Impl_in_rule__BaseDictionaryControl__Group__236084 = new BitSet(new long[]{0x0000000000000000L,0x0400000000020000L,0x0008000000000000L});
public static final BitSet FOLLOW_rule__BaseDictionaryControl__Group__3_in_rule__BaseDictionaryControl__Group__236087 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__BaseDictionaryControl__LabelsAssignment_2_in_rule__BaseDictionaryControl__Group__2__Impl36114 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__BaseDictionaryControl__Group__3__Impl_in_rule__BaseDictionaryControl__Group__336144 = new BitSet(new long[]{0x0000000000000000L,0x0400000000020000L,0x0008000000000000L});
public static final BitSet FOLLOW_rule__BaseDictionaryControl__Group__4_in_rule__BaseDictionaryControl__Group__336147 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__BaseDictionaryControl__MandatoryAssignment_3_in_rule__BaseDictionaryControl__Group__3__Impl36174 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__BaseDictionaryControl__Group__4__Impl_in_rule__BaseDictionaryControl__Group__436205 = new BitSet(new long[]{0x0000000000000000L,0x0400000000020000L,0x0008000000000000L});
public static final BitSet FOLLOW_rule__BaseDictionaryControl__Group__5_in_rule__BaseDictionaryControl__Group__436208 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__BaseDictionaryControl__Group_4__0_in_rule__BaseDictionaryControl__Group__4__Impl36235 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__BaseDictionaryControl__Group__5__Impl_in_rule__BaseDictionaryControl__Group__536266 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__BaseDictionaryControl__Group_5__0_in_rule__BaseDictionaryControl__Group__5__Impl36293 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__BaseDictionaryControl__Group_0__0__Impl_in_rule__BaseDictionaryControl__Group_0__036336 = new BitSet(new long[]{0x0000000000000010L});
public static final BitSet FOLLOW_rule__BaseDictionaryControl__Group_0__1_in_rule__BaseDictionaryControl__Group_0__036339 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_114_in_rule__BaseDictionaryControl__Group_0__0__Impl36367 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__BaseDictionaryControl__Group_0__1__Impl_in_rule__BaseDictionaryControl__Group_0__136398 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__BaseDictionaryControl__EntityattributeAssignment_0_1_in_rule__BaseDictionaryControl__Group_0__1__Impl36425 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__BaseDictionaryControl__Group_1__0__Impl_in_rule__BaseDictionaryControl__Group_1__036459 = new BitSet(new long[]{0x0000000000000010L});
public static final BitSet FOLLOW_rule__BaseDictionaryControl__Group_1__1_in_rule__BaseDictionaryControl__Group_1__036462 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_121_in_rule__BaseDictionaryControl__Group_1__0__Impl36490 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__BaseDictionaryControl__Group_1__1__Impl_in_rule__BaseDictionaryControl__Group_1__136521 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__BaseDictionaryControl__TypeAssignment_1_1_in_rule__BaseDictionaryControl__Group_1__1__Impl36548 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__BaseDictionaryControl__Group_4__0__Impl_in_rule__BaseDictionaryControl__Group_4__036582 = new BitSet(new long[]{0x0000000000000040L});
public static final BitSet FOLLOW_rule__BaseDictionaryControl__Group_4__1_in_rule__BaseDictionaryControl__Group_4__036585 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_81_in_rule__BaseDictionaryControl__Group_4__0__Impl36613 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__BaseDictionaryControl__Group_4__1__Impl_in_rule__BaseDictionaryControl__Group_4__136644 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__BaseDictionaryControl__WidthAssignment_4_1_in_rule__BaseDictionaryControl__Group_4__1__Impl36671 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__BaseDictionaryControl__Group_5__0__Impl_in_rule__BaseDictionaryControl__Group_5__036705 = new BitSet(new long[]{0x0004000000000000L,0x0000000000000000L,0x0200000000000000L});
public static final BitSet FOLLOW_rule__BaseDictionaryControl__Group_5__1_in_rule__BaseDictionaryControl__Group_5__036708 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_122_in_rule__BaseDictionaryControl__Group_5__0__Impl36736 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__BaseDictionaryControl__Group_5__1__Impl_in_rule__BaseDictionaryControl__Group_5__136767 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__BaseDictionaryControl__ReadonlyAssignment_5_1_in_rule__BaseDictionaryControl__Group_5__1__Impl36794 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryControlGroupOptionMultiFilterField__Group__0__Impl_in_rule__DictionaryControlGroupOptionMultiFilterField__Group__036828 = new BitSet(new long[]{0x0000000000000000L,0x0800000000000000L});
public static final BitSet FOLLOW_rule__DictionaryControlGroupOptionMultiFilterField__Group__1_in_rule__DictionaryControlGroupOptionMultiFilterField__Group__036831 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryControlGroupOptionMultiFilterField__Group__1__Impl_in_rule__DictionaryControlGroupOptionMultiFilterField__Group__136889 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryControlGroupOptionMultiFilterField__Group_1__0_in_rule__DictionaryControlGroupOptionMultiFilterField__Group__1__Impl36916 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryControlGroupOptionMultiFilterField__Group_1__0__Impl_in_rule__DictionaryControlGroupOptionMultiFilterField__Group_1__036951 = new BitSet(new long[]{0x0004000000000000L,0x0000000000000000L,0x0200000000000000L});
public static final BitSet FOLLOW_rule__DictionaryControlGroupOptionMultiFilterField__Group_1__1_in_rule__DictionaryControlGroupOptionMultiFilterField__Group_1__036954 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_123_in_rule__DictionaryControlGroupOptionMultiFilterField__Group_1__0__Impl36982 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryControlGroupOptionMultiFilterField__Group_1__1__Impl_in_rule__DictionaryControlGroupOptionMultiFilterField__Group_1__137013 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryControlGroupOptionMultiFilterField__MultiFilterFieldAssignment_1_1_in_rule__DictionaryControlGroupOptionMultiFilterField__Group_1__1__Impl37040 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryControlGroupOptionsContainer__Group__0__Impl_in_rule__DictionaryControlGroupOptionsContainer__Group__037074 = new BitSet(new long[]{0x0000000000000000L,0x1000000000000000L});
public static final BitSet FOLLOW_rule__DictionaryControlGroupOptionsContainer__Group__1_in_rule__DictionaryControlGroupOptionsContainer__Group__037077 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryControlGroupOptionsContainer__Group__1__Impl_in_rule__DictionaryControlGroupOptionsContainer__Group__137135 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000008L});
public static final BitSet FOLLOW_rule__DictionaryControlGroupOptionsContainer__Group__2_in_rule__DictionaryControlGroupOptionsContainer__Group__137138 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_124_in_rule__DictionaryControlGroupOptionsContainer__Group__1__Impl37166 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryControlGroupOptionsContainer__Group__2__Impl_in_rule__DictionaryControlGroupOptionsContainer__Group__237197 = new BitSet(new long[]{0x0000000000000000L,0x0800000000000000L});
public static final BitSet FOLLOW_rule__DictionaryControlGroupOptionsContainer__Group__3_in_rule__DictionaryControlGroupOptionsContainer__Group__237200 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_67_in_rule__DictionaryControlGroupOptionsContainer__Group__2__Impl37228 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryControlGroupOptionsContainer__Group__3__Impl_in_rule__DictionaryControlGroupOptionsContainer__Group__337259 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000010L});
public static final BitSet FOLLOW_rule__DictionaryControlGroupOptionsContainer__Group__4_in_rule__DictionaryControlGroupOptionsContainer__Group__337262 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryControlGroupOptionsContainer__OptionsAssignment_3_in_rule__DictionaryControlGroupOptionsContainer__Group__3__Impl37289 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryControlGroupOptionsContainer__Group__4__Impl_in_rule__DictionaryControlGroupOptionsContainer__Group__437319 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_68_in_rule__DictionaryControlGroupOptionsContainer__Group__4__Impl37347 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryControlGroup__Group__0__Impl_in_rule__DictionaryControlGroup__Group__037388 = new BitSet(new long[]{0x0000000000000000L,0x2000000000000000L});
public static final BitSet FOLLOW_rule__DictionaryControlGroup__Group__1_in_rule__DictionaryControlGroup__Group__037391 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryControlGroup__Group__1__Impl_in_rule__DictionaryControlGroup__Group__137449 = new BitSet(new long[]{0x0000000000000010L,0x4000000000000008L});
public static final BitSet FOLLOW_rule__DictionaryControlGroup__Group__2_in_rule__DictionaryControlGroup__Group__137452 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_125_in_rule__DictionaryControlGroup__Group__1__Impl37480 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryControlGroup__Group__2__Impl_in_rule__DictionaryControlGroup__Group__237511 = new BitSet(new long[]{0x0000000000000010L,0x4000000000000008L});
public static final BitSet FOLLOW_rule__DictionaryControlGroup__Group__3_in_rule__DictionaryControlGroup__Group__237514 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryControlGroup__NameAssignment_2_in_rule__DictionaryControlGroup__Group__2__Impl37541 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryControlGroup__Group__3__Impl_in_rule__DictionaryControlGroup__Group__337572 = new BitSet(new long[]{0x0000000000000010L,0x4000000000000008L});
public static final BitSet FOLLOW_rule__DictionaryControlGroup__Group__4_in_rule__DictionaryControlGroup__Group__337575 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryControlGroup__Group_3__0_in_rule__DictionaryControlGroup__Group__3__Impl37602 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryControlGroup__Group__4__Impl_in_rule__DictionaryControlGroup__Group__437633 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryControlGroup__Group_4__0_in_rule__DictionaryControlGroup__Group__4__Impl37660 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryControlGroup__Group_3__0__Impl_in_rule__DictionaryControlGroup__Group_3__037701 = new BitSet(new long[]{0x0000000000000010L});
public static final BitSet FOLLOW_rule__DictionaryControlGroup__Group_3__1_in_rule__DictionaryControlGroup__Group_3__037704 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_126_in_rule__DictionaryControlGroup__Group_3__0__Impl37732 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryControlGroup__Group_3__1__Impl_in_rule__DictionaryControlGroup__Group_3__137763 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryControlGroup__RefAssignment_3_1_in_rule__DictionaryControlGroup__Group_3__1__Impl37790 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryControlGroup__Group_4__0__Impl_in_rule__DictionaryControlGroup__Group_4__037824 = new BitSet(new long[]{0x0000000000000000L,0x13E4000000000800L});
public static final BitSet FOLLOW_rule__DictionaryControlGroup__Group_4__1_in_rule__DictionaryControlGroup__Group_4__037827 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_67_in_rule__DictionaryControlGroup__Group_4__0__Impl37855 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryControlGroup__Group_4__1__Impl_in_rule__DictionaryControlGroup__Group_4__137886 = new BitSet(new long[]{0x0000000000000000L,0x13E4000000000800L});
public static final BitSet FOLLOW_rule__DictionaryControlGroup__Group_4__2_in_rule__DictionaryControlGroup__Group_4__137889 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryControlGroup__ControlGroupOptionsAssignment_4_1_in_rule__DictionaryControlGroup__Group_4__1__Impl37916 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryControlGroup__Group_4__2__Impl_in_rule__DictionaryControlGroup__Group_4__237947 = new BitSet(new long[]{0x0000000000000000L,0xA000000000000010L,0x00000000000005FAL});
public static final BitSet FOLLOW_rule__DictionaryControlGroup__Group_4__3_in_rule__DictionaryControlGroup__Group_4__237950 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryControlGroup__BaseControlAssignment_4_2_in_rule__DictionaryControlGroup__Group_4__2__Impl37977 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryControlGroup__Group_4__3__Impl_in_rule__DictionaryControlGroup__Group_4__338007 = new BitSet(new long[]{0x0000000000000000L,0xA000000000000010L,0x00000000000005FAL});
public static final BitSet FOLLOW_rule__DictionaryControlGroup__Group_4__4_in_rule__DictionaryControlGroup__Group_4__338010 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryControlGroup__GroupcontrolsAssignment_4_3_in_rule__DictionaryControlGroup__Group_4__3__Impl38037 = new BitSet(new long[]{0x0000000000000002L,0xA000000000000000L,0x00000000000005FAL});
public static final BitSet FOLLOW_rule__DictionaryControlGroup__Group_4__4__Impl_in_rule__DictionaryControlGroup__Group_4__438068 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_68_in_rule__DictionaryControlGroup__Group_4__4__Impl38096 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryHierarchicalControl__Group__0__Impl_in_rule__DictionaryHierarchicalControl__Group__038137 = new BitSet(new long[]{0x0000000000000000L,0x8000000000000000L});
public static final BitSet FOLLOW_rule__DictionaryHierarchicalControl__Group__1_in_rule__DictionaryHierarchicalControl__Group__038140 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryHierarchicalControl__Group__1__Impl_in_rule__DictionaryHierarchicalControl__Group__138198 = new BitSet(new long[]{0x0000000000000010L,0x4000000000000008L});
public static final BitSet FOLLOW_rule__DictionaryHierarchicalControl__Group__2_in_rule__DictionaryHierarchicalControl__Group__138201 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_127_in_rule__DictionaryHierarchicalControl__Group__1__Impl38229 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryHierarchicalControl__Group__2__Impl_in_rule__DictionaryHierarchicalControl__Group__238260 = new BitSet(new long[]{0x0000000000000010L,0x4000000000000008L});
public static final BitSet FOLLOW_rule__DictionaryHierarchicalControl__Group__3_in_rule__DictionaryHierarchicalControl__Group__238263 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryHierarchicalControl__NameAssignment_2_in_rule__DictionaryHierarchicalControl__Group__2__Impl38290 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryHierarchicalControl__Group__3__Impl_in_rule__DictionaryHierarchicalControl__Group__338321 = new BitSet(new long[]{0x0000000000000010L,0x4000000000000008L});
public static final BitSet FOLLOW_rule__DictionaryHierarchicalControl__Group__4_in_rule__DictionaryHierarchicalControl__Group__338324 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryHierarchicalControl__Group_3__0_in_rule__DictionaryHierarchicalControl__Group__3__Impl38351 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryHierarchicalControl__Group__4__Impl_in_rule__DictionaryHierarchicalControl__Group__438382 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryHierarchicalControl__Group_4__0_in_rule__DictionaryHierarchicalControl__Group__4__Impl38409 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryHierarchicalControl__Group_3__0__Impl_in_rule__DictionaryHierarchicalControl__Group_3__038450 = new BitSet(new long[]{0x0000000000000010L});
public static final BitSet FOLLOW_rule__DictionaryHierarchicalControl__Group_3__1_in_rule__DictionaryHierarchicalControl__Group_3__038453 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_126_in_rule__DictionaryHierarchicalControl__Group_3__0__Impl38481 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryHierarchicalControl__Group_3__1__Impl_in_rule__DictionaryHierarchicalControl__Group_3__138512 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryHierarchicalControl__RefAssignment_3_1_in_rule__DictionaryHierarchicalControl__Group_3__1__Impl38539 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryHierarchicalControl__Group_4__0__Impl_in_rule__DictionaryHierarchicalControl__Group_4__038573 = new BitSet(new long[]{0x0000000000000000L,0x13E4000000000800L});
public static final BitSet FOLLOW_rule__DictionaryHierarchicalControl__Group_4__1_in_rule__DictionaryHierarchicalControl__Group_4__038576 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_67_in_rule__DictionaryHierarchicalControl__Group_4__0__Impl38604 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryHierarchicalControl__Group_4__1__Impl_in_rule__DictionaryHierarchicalControl__Group_4__138635 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000000L,0x0000000000000001L});
public static final BitSet FOLLOW_rule__DictionaryHierarchicalControl__Group_4__2_in_rule__DictionaryHierarchicalControl__Group_4__138638 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryHierarchicalControl__BaseControlAssignment_4_1_in_rule__DictionaryHierarchicalControl__Group_4__1__Impl38665 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryHierarchicalControl__Group_4__2__Impl_in_rule__DictionaryHierarchicalControl__Group_4__238695 = new BitSet(new long[]{0x0000000000000100L});
public static final BitSet FOLLOW_rule__DictionaryHierarchicalControl__Group_4__3_in_rule__DictionaryHierarchicalControl__Group_4__238698 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_128_in_rule__DictionaryHierarchicalControl__Group_4__2__Impl38726 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryHierarchicalControl__Group_4__3__Impl_in_rule__DictionaryHierarchicalControl__Group_4__338757 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000010L});
public static final BitSet FOLLOW_rule__DictionaryHierarchicalControl__Group_4__4_in_rule__DictionaryHierarchicalControl__Group_4__338760 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryHierarchicalControl__HierarchicalIdAssignment_4_3_in_rule__DictionaryHierarchicalControl__Group_4__3__Impl38787 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryHierarchicalControl__Group_4__4__Impl_in_rule__DictionaryHierarchicalControl__Group_4__438817 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_68_in_rule__DictionaryHierarchicalControl__Group_4__4__Impl38845 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryTextControl__Group__0__Impl_in_rule__DictionaryTextControl__Group__038886 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000000L,0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryTextControl__Group__1_in_rule__DictionaryTextControl__Group__038889 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryTextControl__Group__1__Impl_in_rule__DictionaryTextControl__Group__138947 = new BitSet(new long[]{0x0000000000000010L,0x4000000000000008L});
public static final BitSet FOLLOW_rule__DictionaryTextControl__Group__2_in_rule__DictionaryTextControl__Group__138950 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_129_in_rule__DictionaryTextControl__Group__1__Impl38978 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryTextControl__Group__2__Impl_in_rule__DictionaryTextControl__Group__239009 = new BitSet(new long[]{0x0000000000000010L,0x4000000000000008L});
public static final BitSet FOLLOW_rule__DictionaryTextControl__Group__3_in_rule__DictionaryTextControl__Group__239012 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryTextControl__NameAssignment_2_in_rule__DictionaryTextControl__Group__2__Impl39039 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryTextControl__Group__3__Impl_in_rule__DictionaryTextControl__Group__339070 = new BitSet(new long[]{0x0000000000000010L,0x4000000000000008L});
public static final BitSet FOLLOW_rule__DictionaryTextControl__Group__4_in_rule__DictionaryTextControl__Group__339073 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryTextControl__Group_3__0_in_rule__DictionaryTextControl__Group__3__Impl39100 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryTextControl__Group__4__Impl_in_rule__DictionaryTextControl__Group__439131 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryTextControl__Group_4__0_in_rule__DictionaryTextControl__Group__4__Impl39158 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryTextControl__Group_3__0__Impl_in_rule__DictionaryTextControl__Group_3__039199 = new BitSet(new long[]{0x0000000000000010L});
public static final BitSet FOLLOW_rule__DictionaryTextControl__Group_3__1_in_rule__DictionaryTextControl__Group_3__039202 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_126_in_rule__DictionaryTextControl__Group_3__0__Impl39230 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryTextControl__Group_3__1__Impl_in_rule__DictionaryTextControl__Group_3__139261 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryTextControl__RefAssignment_3_1_in_rule__DictionaryTextControl__Group_3__1__Impl39288 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryTextControl__Group_4__0__Impl_in_rule__DictionaryTextControl__Group_4__039322 = new BitSet(new long[]{0x0000000000000000L,0x13E4000000000800L});
public static final BitSet FOLLOW_rule__DictionaryTextControl__Group_4__1_in_rule__DictionaryTextControl__Group_4__039325 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_67_in_rule__DictionaryTextControl__Group_4__0__Impl39353 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryTextControl__Group_4__1__Impl_in_rule__DictionaryTextControl__Group_4__139384 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000010L});
public static final BitSet FOLLOW_rule__DictionaryTextControl__Group_4__2_in_rule__DictionaryTextControl__Group_4__139387 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryTextControl__BaseControlAssignment_4_1_in_rule__DictionaryTextControl__Group_4__1__Impl39414 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryTextControl__Group_4__2__Impl_in_rule__DictionaryTextControl__Group_4__239444 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_68_in_rule__DictionaryTextControl__Group_4__2__Impl39472 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryIntegerControlInputType__Group__0__Impl_in_rule__DictionaryIntegerControlInputType__Group__039509 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000000L,0x0000000000000004L});
public static final BitSet FOLLOW_rule__DictionaryIntegerControlInputType__Group__1_in_rule__DictionaryIntegerControlInputType__Group__039512 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryIntegerControlInputType__Group__1__Impl_in_rule__DictionaryIntegerControlInputType__Group__139570 = new BitSet(new long[]{0xC000000000000000L});
public static final BitSet FOLLOW_rule__DictionaryIntegerControlInputType__Group__2_in_rule__DictionaryIntegerControlInputType__Group__139573 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_130_in_rule__DictionaryIntegerControlInputType__Group__1__Impl39601 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryIntegerControlInputType__Group__2__Impl_in_rule__DictionaryIntegerControlInputType__Group__239632 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryIntegerControlInputType__InputtypeAssignment_2_in_rule__DictionaryIntegerControlInputType__Group__2__Impl39659 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryIntegerControl__Group__0__Impl_in_rule__DictionaryIntegerControl__Group__039695 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000000L,0x0000000000000008L});
public static final BitSet FOLLOW_rule__DictionaryIntegerControl__Group__1_in_rule__DictionaryIntegerControl__Group__039698 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryIntegerControl__Group__1__Impl_in_rule__DictionaryIntegerControl__Group__139756 = new BitSet(new long[]{0x0000000000000010L,0x4000000000000008L});
public static final BitSet FOLLOW_rule__DictionaryIntegerControl__Group__2_in_rule__DictionaryIntegerControl__Group__139759 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_131_in_rule__DictionaryIntegerControl__Group__1__Impl39787 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryIntegerControl__Group__2__Impl_in_rule__DictionaryIntegerControl__Group__239818 = new BitSet(new long[]{0x0000000000000010L,0x4000000000000008L});
public static final BitSet FOLLOW_rule__DictionaryIntegerControl__Group__3_in_rule__DictionaryIntegerControl__Group__239821 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryIntegerControl__NameAssignment_2_in_rule__DictionaryIntegerControl__Group__2__Impl39848 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryIntegerControl__Group__3__Impl_in_rule__DictionaryIntegerControl__Group__339879 = new BitSet(new long[]{0x0000000000000010L,0x4000000000000008L});
public static final BitSet FOLLOW_rule__DictionaryIntegerControl__Group__4_in_rule__DictionaryIntegerControl__Group__339882 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryIntegerControl__Group_3__0_in_rule__DictionaryIntegerControl__Group__3__Impl39909 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryIntegerControl__Group__4__Impl_in_rule__DictionaryIntegerControl__Group__439940 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryIntegerControl__Group_4__0_in_rule__DictionaryIntegerControl__Group__4__Impl39967 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryIntegerControl__Group_3__0__Impl_in_rule__DictionaryIntegerControl__Group_3__040008 = new BitSet(new long[]{0x0000000000000010L});
public static final BitSet FOLLOW_rule__DictionaryIntegerControl__Group_3__1_in_rule__DictionaryIntegerControl__Group_3__040011 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_126_in_rule__DictionaryIntegerControl__Group_3__0__Impl40039 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryIntegerControl__Group_3__1__Impl_in_rule__DictionaryIntegerControl__Group_3__140070 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryIntegerControl__RefAssignment_3_1_in_rule__DictionaryIntegerControl__Group_3__1__Impl40097 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryIntegerControl__Group_4__0__Impl_in_rule__DictionaryIntegerControl__Group_4__040131 = new BitSet(new long[]{0x0000000000000000L,0x13E4000000000800L});
public static final BitSet FOLLOW_rule__DictionaryIntegerControl__Group_4__1_in_rule__DictionaryIntegerControl__Group_4__040134 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_67_in_rule__DictionaryIntegerControl__Group_4__0__Impl40162 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryIntegerControl__Group_4__1__Impl_in_rule__DictionaryIntegerControl__Group_4__140193 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000010L,0x0000000000000004L});
public static final BitSet FOLLOW_rule__DictionaryIntegerControl__Group_4__2_in_rule__DictionaryIntegerControl__Group_4__140196 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryIntegerControl__BaseControlAssignment_4_1_in_rule__DictionaryIntegerControl__Group_4__1__Impl40223 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryIntegerControl__Group_4__2__Impl_in_rule__DictionaryIntegerControl__Group_4__240253 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000010L,0x0000000000000004L});
public static final BitSet FOLLOW_rule__DictionaryIntegerControl__Group_4__3_in_rule__DictionaryIntegerControl__Group_4__240256 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryIntegerControl__OptionsAssignment_4_2_in_rule__DictionaryIntegerControl__Group_4__2__Impl40283 = new BitSet(new long[]{0x0000000000000002L,0x0000000000000000L,0x0000000000000004L});
public static final BitSet FOLLOW_rule__DictionaryIntegerControl__Group_4__3__Impl_in_rule__DictionaryIntegerControl__Group_4__340314 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_68_in_rule__DictionaryIntegerControl__Group_4__3__Impl40342 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryBigDecimalControl__Group__0__Impl_in_rule__DictionaryBigDecimalControl__Group__040381 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000000L,0x0000000000000010L});
public static final BitSet FOLLOW_rule__DictionaryBigDecimalControl__Group__1_in_rule__DictionaryBigDecimalControl__Group__040384 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryBigDecimalControl__Group__1__Impl_in_rule__DictionaryBigDecimalControl__Group__140442 = new BitSet(new long[]{0x0000000000000010L,0x4000000000000008L});
public static final BitSet FOLLOW_rule__DictionaryBigDecimalControl__Group__2_in_rule__DictionaryBigDecimalControl__Group__140445 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_132_in_rule__DictionaryBigDecimalControl__Group__1__Impl40473 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryBigDecimalControl__Group__2__Impl_in_rule__DictionaryBigDecimalControl__Group__240504 = new BitSet(new long[]{0x0000000000000010L,0x4000000000000008L});
public static final BitSet FOLLOW_rule__DictionaryBigDecimalControl__Group__3_in_rule__DictionaryBigDecimalControl__Group__240507 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryBigDecimalControl__NameAssignment_2_in_rule__DictionaryBigDecimalControl__Group__2__Impl40534 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryBigDecimalControl__Group__3__Impl_in_rule__DictionaryBigDecimalControl__Group__340565 = new BitSet(new long[]{0x0000000000000010L,0x4000000000000008L});
public static final BitSet FOLLOW_rule__DictionaryBigDecimalControl__Group__4_in_rule__DictionaryBigDecimalControl__Group__340568 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryBigDecimalControl__Group_3__0_in_rule__DictionaryBigDecimalControl__Group__3__Impl40595 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryBigDecimalControl__Group__4__Impl_in_rule__DictionaryBigDecimalControl__Group__440626 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryBigDecimalControl__Group_4__0_in_rule__DictionaryBigDecimalControl__Group__4__Impl40653 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryBigDecimalControl__Group_3__0__Impl_in_rule__DictionaryBigDecimalControl__Group_3__040694 = new BitSet(new long[]{0x0000000000000010L});
public static final BitSet FOLLOW_rule__DictionaryBigDecimalControl__Group_3__1_in_rule__DictionaryBigDecimalControl__Group_3__040697 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_126_in_rule__DictionaryBigDecimalControl__Group_3__0__Impl40725 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryBigDecimalControl__Group_3__1__Impl_in_rule__DictionaryBigDecimalControl__Group_3__140756 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryBigDecimalControl__RefAssignment_3_1_in_rule__DictionaryBigDecimalControl__Group_3__1__Impl40783 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryBigDecimalControl__Group_4__0__Impl_in_rule__DictionaryBigDecimalControl__Group_4__040817 = new BitSet(new long[]{0x0000000000000000L,0x13E4000000000800L});
public static final BitSet FOLLOW_rule__DictionaryBigDecimalControl__Group_4__1_in_rule__DictionaryBigDecimalControl__Group_4__040820 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_67_in_rule__DictionaryBigDecimalControl__Group_4__0__Impl40848 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryBigDecimalControl__Group_4__1__Impl_in_rule__DictionaryBigDecimalControl__Group_4__140879 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000010L});
public static final BitSet FOLLOW_rule__DictionaryBigDecimalControl__Group_4__2_in_rule__DictionaryBigDecimalControl__Group_4__140882 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryBigDecimalControl__BaseControlAssignment_4_1_in_rule__DictionaryBigDecimalControl__Group_4__1__Impl40909 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryBigDecimalControl__Group_4__2__Impl_in_rule__DictionaryBigDecimalControl__Group_4__240939 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_68_in_rule__DictionaryBigDecimalControl__Group_4__2__Impl40967 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryBooleanControl__Group__0__Impl_in_rule__DictionaryBooleanControl__Group__041004 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000000L,0x0000000000000020L});
public static final BitSet FOLLOW_rule__DictionaryBooleanControl__Group__1_in_rule__DictionaryBooleanControl__Group__041007 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryBooleanControl__Group__1__Impl_in_rule__DictionaryBooleanControl__Group__141065 = new BitSet(new long[]{0x0000000000000010L,0x4000000000000008L});
public static final BitSet FOLLOW_rule__DictionaryBooleanControl__Group__2_in_rule__DictionaryBooleanControl__Group__141068 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_133_in_rule__DictionaryBooleanControl__Group__1__Impl41096 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryBooleanControl__Group__2__Impl_in_rule__DictionaryBooleanControl__Group__241127 = new BitSet(new long[]{0x0000000000000010L,0x4000000000000008L});
public static final BitSet FOLLOW_rule__DictionaryBooleanControl__Group__3_in_rule__DictionaryBooleanControl__Group__241130 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryBooleanControl__NameAssignment_2_in_rule__DictionaryBooleanControl__Group__2__Impl41157 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryBooleanControl__Group__3__Impl_in_rule__DictionaryBooleanControl__Group__341188 = new BitSet(new long[]{0x0000000000000010L,0x4000000000000008L});
public static final BitSet FOLLOW_rule__DictionaryBooleanControl__Group__4_in_rule__DictionaryBooleanControl__Group__341191 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryBooleanControl__Group_3__0_in_rule__DictionaryBooleanControl__Group__3__Impl41218 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryBooleanControl__Group__4__Impl_in_rule__DictionaryBooleanControl__Group__441249 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryBooleanControl__Group_4__0_in_rule__DictionaryBooleanControl__Group__4__Impl41276 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryBooleanControl__Group_3__0__Impl_in_rule__DictionaryBooleanControl__Group_3__041317 = new BitSet(new long[]{0x0000000000000010L});
public static final BitSet FOLLOW_rule__DictionaryBooleanControl__Group_3__1_in_rule__DictionaryBooleanControl__Group_3__041320 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_126_in_rule__DictionaryBooleanControl__Group_3__0__Impl41348 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryBooleanControl__Group_3__1__Impl_in_rule__DictionaryBooleanControl__Group_3__141379 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryBooleanControl__RefAssignment_3_1_in_rule__DictionaryBooleanControl__Group_3__1__Impl41406 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryBooleanControl__Group_4__0__Impl_in_rule__DictionaryBooleanControl__Group_4__041440 = new BitSet(new long[]{0x0000000000000000L,0x13E4000000000800L});
public static final BitSet FOLLOW_rule__DictionaryBooleanControl__Group_4__1_in_rule__DictionaryBooleanControl__Group_4__041443 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_67_in_rule__DictionaryBooleanControl__Group_4__0__Impl41471 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryBooleanControl__Group_4__1__Impl_in_rule__DictionaryBooleanControl__Group_4__141502 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000010L});
public static final BitSet FOLLOW_rule__DictionaryBooleanControl__Group_4__2_in_rule__DictionaryBooleanControl__Group_4__141505 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryBooleanControl__BaseControlAssignment_4_1_in_rule__DictionaryBooleanControl__Group_4__1__Impl41532 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryBooleanControl__Group_4__2__Impl_in_rule__DictionaryBooleanControl__Group_4__241562 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_68_in_rule__DictionaryBooleanControl__Group_4__2__Impl41590 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryDateControl__Group__0__Impl_in_rule__DictionaryDateControl__Group__041627 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000000L,0x0000000000000040L});
public static final BitSet FOLLOW_rule__DictionaryDateControl__Group__1_in_rule__DictionaryDateControl__Group__041630 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryDateControl__Group__1__Impl_in_rule__DictionaryDateControl__Group__141688 = new BitSet(new long[]{0x0000000000000010L,0x4000000000000008L});
public static final BitSet FOLLOW_rule__DictionaryDateControl__Group__2_in_rule__DictionaryDateControl__Group__141691 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_134_in_rule__DictionaryDateControl__Group__1__Impl41719 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryDateControl__Group__2__Impl_in_rule__DictionaryDateControl__Group__241750 = new BitSet(new long[]{0x0000000000000010L,0x4000000000000008L});
public static final BitSet FOLLOW_rule__DictionaryDateControl__Group__3_in_rule__DictionaryDateControl__Group__241753 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryDateControl__NameAssignment_2_in_rule__DictionaryDateControl__Group__2__Impl41780 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryDateControl__Group__3__Impl_in_rule__DictionaryDateControl__Group__341811 = new BitSet(new long[]{0x0000000000000010L,0x4000000000000008L});
public static final BitSet FOLLOW_rule__DictionaryDateControl__Group__4_in_rule__DictionaryDateControl__Group__341814 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryDateControl__Group_3__0_in_rule__DictionaryDateControl__Group__3__Impl41841 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryDateControl__Group__4__Impl_in_rule__DictionaryDateControl__Group__441872 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryDateControl__Group_4__0_in_rule__DictionaryDateControl__Group__4__Impl41899 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryDateControl__Group_3__0__Impl_in_rule__DictionaryDateControl__Group_3__041940 = new BitSet(new long[]{0x0000000000000010L});
public static final BitSet FOLLOW_rule__DictionaryDateControl__Group_3__1_in_rule__DictionaryDateControl__Group_3__041943 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_126_in_rule__DictionaryDateControl__Group_3__0__Impl41971 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryDateControl__Group_3__1__Impl_in_rule__DictionaryDateControl__Group_3__142002 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryDateControl__RefAssignment_3_1_in_rule__DictionaryDateControl__Group_3__1__Impl42029 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryDateControl__Group_4__0__Impl_in_rule__DictionaryDateControl__Group_4__042063 = new BitSet(new long[]{0x0000000000000000L,0x13E4000000000800L});
public static final BitSet FOLLOW_rule__DictionaryDateControl__Group_4__1_in_rule__DictionaryDateControl__Group_4__042066 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_67_in_rule__DictionaryDateControl__Group_4__0__Impl42094 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryDateControl__Group_4__1__Impl_in_rule__DictionaryDateControl__Group_4__142125 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000010L});
public static final BitSet FOLLOW_rule__DictionaryDateControl__Group_4__2_in_rule__DictionaryDateControl__Group_4__142128 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryDateControl__BaseControlAssignment_4_1_in_rule__DictionaryDateControl__Group_4__1__Impl42155 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryDateControl__Group_4__2__Impl_in_rule__DictionaryDateControl__Group_4__242185 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_68_in_rule__DictionaryDateControl__Group_4__2__Impl42213 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryEnumerationControl__Group__0__Impl_in_rule__DictionaryEnumerationControl__Group__042250 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000000L,0x0000000000000080L});
public static final BitSet FOLLOW_rule__DictionaryEnumerationControl__Group__1_in_rule__DictionaryEnumerationControl__Group__042253 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryEnumerationControl__Group__1__Impl_in_rule__DictionaryEnumerationControl__Group__142311 = new BitSet(new long[]{0x0000000000000010L,0x4000000000000008L});
public static final BitSet FOLLOW_rule__DictionaryEnumerationControl__Group__2_in_rule__DictionaryEnumerationControl__Group__142314 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_135_in_rule__DictionaryEnumerationControl__Group__1__Impl42342 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryEnumerationControl__Group__2__Impl_in_rule__DictionaryEnumerationControl__Group__242373 = new BitSet(new long[]{0x0000000000000010L,0x4000000000000008L});
public static final BitSet FOLLOW_rule__DictionaryEnumerationControl__Group__3_in_rule__DictionaryEnumerationControl__Group__242376 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryEnumerationControl__NameAssignment_2_in_rule__DictionaryEnumerationControl__Group__2__Impl42403 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryEnumerationControl__Group__3__Impl_in_rule__DictionaryEnumerationControl__Group__342434 = new BitSet(new long[]{0x0000000000000010L,0x4000000000000008L});
public static final BitSet FOLLOW_rule__DictionaryEnumerationControl__Group__4_in_rule__DictionaryEnumerationControl__Group__342437 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryEnumerationControl__Group_3__0_in_rule__DictionaryEnumerationControl__Group__3__Impl42464 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryEnumerationControl__Group__4__Impl_in_rule__DictionaryEnumerationControl__Group__442495 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryEnumerationControl__Group_4__0_in_rule__DictionaryEnumerationControl__Group__4__Impl42522 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryEnumerationControl__Group_3__0__Impl_in_rule__DictionaryEnumerationControl__Group_3__042563 = new BitSet(new long[]{0x0000000000000010L});
public static final BitSet FOLLOW_rule__DictionaryEnumerationControl__Group_3__1_in_rule__DictionaryEnumerationControl__Group_3__042566 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_126_in_rule__DictionaryEnumerationControl__Group_3__0__Impl42594 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryEnumerationControl__Group_3__1__Impl_in_rule__DictionaryEnumerationControl__Group_3__142625 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryEnumerationControl__RefAssignment_3_1_in_rule__DictionaryEnumerationControl__Group_3__1__Impl42652 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryEnumerationControl__Group_4__0__Impl_in_rule__DictionaryEnumerationControl__Group_4__042686 = new BitSet(new long[]{0x0000000000000000L,0x13E4000000000800L});
public static final BitSet FOLLOW_rule__DictionaryEnumerationControl__Group_4__1_in_rule__DictionaryEnumerationControl__Group_4__042689 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_67_in_rule__DictionaryEnumerationControl__Group_4__0__Impl42717 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryEnumerationControl__Group_4__1__Impl_in_rule__DictionaryEnumerationControl__Group_4__142748 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000010L});
public static final BitSet FOLLOW_rule__DictionaryEnumerationControl__Group_4__2_in_rule__DictionaryEnumerationControl__Group_4__142751 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryEnumerationControl__BaseControlAssignment_4_1_in_rule__DictionaryEnumerationControl__Group_4__1__Impl42778 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryEnumerationControl__Group_4__2__Impl_in_rule__DictionaryEnumerationControl__Group_4__242808 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_68_in_rule__DictionaryEnumerationControl__Group_4__2__Impl42836 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryReferenceControl__Group__0__Impl_in_rule__DictionaryReferenceControl__Group__042873 = new BitSet(new long[]{0x0000000000000000L,0xA000000000000000L,0x00000000000005FAL});
public static final BitSet FOLLOW_rule__DictionaryReferenceControl__Group__1_in_rule__DictionaryReferenceControl__Group__042876 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryReferenceControl__Group__1__Impl_in_rule__DictionaryReferenceControl__Group__142934 = new BitSet(new long[]{0x0000000000000010L,0x4000000000000008L});
public static final BitSet FOLLOW_rule__DictionaryReferenceControl__Group__2_in_rule__DictionaryReferenceControl__Group__142937 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_136_in_rule__DictionaryReferenceControl__Group__1__Impl42965 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryReferenceControl__Group__2__Impl_in_rule__DictionaryReferenceControl__Group__242996 = new BitSet(new long[]{0x0000000000000010L,0x4000000000000008L});
public static final BitSet FOLLOW_rule__DictionaryReferenceControl__Group__3_in_rule__DictionaryReferenceControl__Group__242999 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryReferenceControl__NameAssignment_2_in_rule__DictionaryReferenceControl__Group__2__Impl43026 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryReferenceControl__Group__3__Impl_in_rule__DictionaryReferenceControl__Group__343057 = new BitSet(new long[]{0x0000000000000010L,0x4000000000000008L});
public static final BitSet FOLLOW_rule__DictionaryReferenceControl__Group__4_in_rule__DictionaryReferenceControl__Group__343060 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryReferenceControl__Group_3__0_in_rule__DictionaryReferenceControl__Group__3__Impl43087 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryReferenceControl__Group__4__Impl_in_rule__DictionaryReferenceControl__Group__443118 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryReferenceControl__Group_4__0_in_rule__DictionaryReferenceControl__Group__4__Impl43145 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryReferenceControl__Group_3__0__Impl_in_rule__DictionaryReferenceControl__Group_3__043186 = new BitSet(new long[]{0x0000000000000010L});
public static final BitSet FOLLOW_rule__DictionaryReferenceControl__Group_3__1_in_rule__DictionaryReferenceControl__Group_3__043189 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_126_in_rule__DictionaryReferenceControl__Group_3__0__Impl43217 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryReferenceControl__Group_3__1__Impl_in_rule__DictionaryReferenceControl__Group_3__143248 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryReferenceControl__RefAssignment_3_1_in_rule__DictionaryReferenceControl__Group_3__1__Impl43275 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryReferenceControl__Group_4__0__Impl_in_rule__DictionaryReferenceControl__Group_4__043309 = new BitSet(new long[]{0x0000000000000000L,0x13E4000000000800L});
public static final BitSet FOLLOW_rule__DictionaryReferenceControl__Group_4__1_in_rule__DictionaryReferenceControl__Group_4__043312 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_67_in_rule__DictionaryReferenceControl__Group_4__0__Impl43340 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryReferenceControl__Group_4__1__Impl_in_rule__DictionaryReferenceControl__Group_4__143371 = new BitSet(new long[]{0x0000000000000000L,0x000000A000000010L,0x0000000000000200L});
public static final BitSet FOLLOW_rule__DictionaryReferenceControl__Group_4__2_in_rule__DictionaryReferenceControl__Group_4__143374 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryReferenceControl__BaseControlAssignment_4_1_in_rule__DictionaryReferenceControl__Group_4__1__Impl43401 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryReferenceControl__Group_4__2__Impl_in_rule__DictionaryReferenceControl__Group_4__243431 = new BitSet(new long[]{0x0000000000000000L,0x000000A000000010L,0x0000000000000200L});
public static final BitSet FOLLOW_rule__DictionaryReferenceControl__Group_4__3_in_rule__DictionaryReferenceControl__Group_4__243434 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryReferenceControl__Group_4_2__0_in_rule__DictionaryReferenceControl__Group_4__2__Impl43461 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryReferenceControl__Group_4__3__Impl_in_rule__DictionaryReferenceControl__Group_4__343492 = new BitSet(new long[]{0x0000000000000000L,0x000000A000000010L,0x0000000000000200L});
public static final BitSet FOLLOW_rule__DictionaryReferenceControl__Group_4__4_in_rule__DictionaryReferenceControl__Group_4__343495 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryReferenceControl__Group_4_3__0_in_rule__DictionaryReferenceControl__Group_4__3__Impl43522 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryReferenceControl__Group_4__4__Impl_in_rule__DictionaryReferenceControl__Group_4__443553 = new BitSet(new long[]{0x0000000000000000L,0x000000A000000010L,0x0000000000000200L});
public static final BitSet FOLLOW_rule__DictionaryReferenceControl__Group_4__5_in_rule__DictionaryReferenceControl__Group_4__443556 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryReferenceControl__Group_4_4__0_in_rule__DictionaryReferenceControl__Group_4__4__Impl43583 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryReferenceControl__Group_4__5__Impl_in_rule__DictionaryReferenceControl__Group_4__543614 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_68_in_rule__DictionaryReferenceControl__Group_4__5__Impl43642 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryReferenceControl__Group_4_2__0__Impl_in_rule__DictionaryReferenceControl__Group_4_2__043685 = new BitSet(new long[]{0x0000000000000010L});
public static final BitSet FOLLOW_rule__DictionaryReferenceControl__Group_4_2__1_in_rule__DictionaryReferenceControl__Group_4_2__043688 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_101_in_rule__DictionaryReferenceControl__Group_4_2__0__Impl43716 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryReferenceControl__Group_4_2__1__Impl_in_rule__DictionaryReferenceControl__Group_4_2__143747 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryReferenceControl__DictionaryAssignment_4_2_1_in_rule__DictionaryReferenceControl__Group_4_2__1__Impl43774 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryReferenceControl__Group_4_3__0__Impl_in_rule__DictionaryReferenceControl__Group_4_3__043808 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000003L});
public static final BitSet FOLLOW_rule__DictionaryReferenceControl__Group_4_3__1_in_rule__DictionaryReferenceControl__Group_4_3__043811 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_137_in_rule__DictionaryReferenceControl__Group_4_3__0__Impl43839 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryReferenceControl__Group_4_3__1__Impl_in_rule__DictionaryReferenceControl__Group_4_3__143870 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryReferenceControl__ControlTypeAssignment_4_3_1_in_rule__DictionaryReferenceControl__Group_4_3__1__Impl43897 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryReferenceControl__Group_4_4__0__Impl_in_rule__DictionaryReferenceControl__Group_4_4__043931 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000008L});
public static final BitSet FOLLOW_rule__DictionaryReferenceControl__Group_4_4__1_in_rule__DictionaryReferenceControl__Group_4_4__043934 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_103_in_rule__DictionaryReferenceControl__Group_4_4__0__Impl43962 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryReferenceControl__Group_4_4__1__Impl_in_rule__DictionaryReferenceControl__Group_4_4__143993 = new BitSet(new long[]{0x0000000000000000L,0xA000000000000010L,0x00000000000005FAL});
public static final BitSet FOLLOW_rule__DictionaryReferenceControl__Group_4_4__2_in_rule__DictionaryReferenceControl__Group_4_4__143996 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_67_in_rule__DictionaryReferenceControl__Group_4_4__1__Impl44024 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryReferenceControl__Group_4_4__2__Impl_in_rule__DictionaryReferenceControl__Group_4_4__244055 = new BitSet(new long[]{0x0000000000000000L,0xA000000000000010L,0x00000000000005FAL});
public static final BitSet FOLLOW_rule__DictionaryReferenceControl__Group_4_4__3_in_rule__DictionaryReferenceControl__Group_4_4__244058 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryReferenceControl__LabelcontrolsAssignment_4_4_2_in_rule__DictionaryReferenceControl__Group_4_4__2__Impl44085 = new BitSet(new long[]{0x0000000000000002L,0xA000000000000000L,0x00000000000005FAL});
public static final BitSet FOLLOW_rule__DictionaryReferenceControl__Group_4_4__3__Impl_in_rule__DictionaryReferenceControl__Group_4_4__344116 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_68_in_rule__DictionaryReferenceControl__Group_4_4__3__Impl44144 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryFileControl__Group__0__Impl_in_rule__DictionaryFileControl__Group__044183 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000000L,0x0000000000000400L});
public static final BitSet FOLLOW_rule__DictionaryFileControl__Group__1_in_rule__DictionaryFileControl__Group__044186 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryFileControl__Group__1__Impl_in_rule__DictionaryFileControl__Group__144244 = new BitSet(new long[]{0x0000000000000010L,0x4000000000000008L});
public static final BitSet FOLLOW_rule__DictionaryFileControl__Group__2_in_rule__DictionaryFileControl__Group__144247 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_138_in_rule__DictionaryFileControl__Group__1__Impl44275 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryFileControl__Group__2__Impl_in_rule__DictionaryFileControl__Group__244306 = new BitSet(new long[]{0x0000000000000010L,0x4000000000000008L});
public static final BitSet FOLLOW_rule__DictionaryFileControl__Group__3_in_rule__DictionaryFileControl__Group__244309 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryFileControl__NameAssignment_2_in_rule__DictionaryFileControl__Group__2__Impl44336 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryFileControl__Group__3__Impl_in_rule__DictionaryFileControl__Group__344367 = new BitSet(new long[]{0x0000000000000010L,0x4000000000000008L});
public static final BitSet FOLLOW_rule__DictionaryFileControl__Group__4_in_rule__DictionaryFileControl__Group__344370 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryFileControl__Group_3__0_in_rule__DictionaryFileControl__Group__3__Impl44397 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryFileControl__Group__4__Impl_in_rule__DictionaryFileControl__Group__444428 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryFileControl__Group_4__0_in_rule__DictionaryFileControl__Group__4__Impl44455 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryFileControl__Group_3__0__Impl_in_rule__DictionaryFileControl__Group_3__044496 = new BitSet(new long[]{0x0000000000000010L});
public static final BitSet FOLLOW_rule__DictionaryFileControl__Group_3__1_in_rule__DictionaryFileControl__Group_3__044499 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_126_in_rule__DictionaryFileControl__Group_3__0__Impl44527 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryFileControl__Group_3__1__Impl_in_rule__DictionaryFileControl__Group_3__144558 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryFileControl__RefAssignment_3_1_in_rule__DictionaryFileControl__Group_3__1__Impl44585 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryFileControl__Group_4__0__Impl_in_rule__DictionaryFileControl__Group_4__044619 = new BitSet(new long[]{0x0000000000000000L,0x13E4000000000800L});
public static final BitSet FOLLOW_rule__DictionaryFileControl__Group_4__1_in_rule__DictionaryFileControl__Group_4__044622 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_67_in_rule__DictionaryFileControl__Group_4__0__Impl44650 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryFileControl__Group_4__1__Impl_in_rule__DictionaryFileControl__Group_4__144681 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000010L});
public static final BitSet FOLLOW_rule__DictionaryFileControl__Group_4__2_in_rule__DictionaryFileControl__Group_4__144684 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryFileControl__BaseControlAssignment_4_1_in_rule__DictionaryFileControl__Group_4__1__Impl44711 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__DictionaryFileControl__Group_4__2__Impl_in_rule__DictionaryFileControl__Group_4__244741 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_68_in_rule__DictionaryFileControl__Group_4__2__Impl44769 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Module__Group__0__Impl_in_rule__Module__Group__044806 = new BitSet(new long[]{0x0000000000000010L});
}
protected static class FollowSets002 {
public static final BitSet FOLLOW_rule__Module__Group__1_in_rule__Module__Group__044809 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_139_in_rule__Module__Group__0__Impl44837 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Module__Group__1__Impl_in_rule__Module__Group__144868 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000008L});
public static final BitSet FOLLOW_rule__Module__Group__2_in_rule__Module__Group__144871 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Module__NameAssignment_1_in_rule__Module__Group__1__Impl44898 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Module__Group__2__Impl_in_rule__Module__Group__244928 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000000L,0x0000000000001000L});
public static final BitSet FOLLOW_rule__Module__Group__3_in_rule__Module__Group__244931 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_67_in_rule__Module__Group__2__Impl44959 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Module__Group__3__Impl_in_rule__Module__Group__344990 = new BitSet(new long[]{0x0000000000000010L});
public static final BitSet FOLLOW_rule__Module__Group__4_in_rule__Module__Group__344993 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_140_in_rule__Module__Group__3__Impl45021 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Module__Group__4__Impl_in_rule__Module__Group__445052 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000010L,0x0000000000002000L});
public static final BitSet FOLLOW_rule__Module__Group__5_in_rule__Module__Group__445055 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Module__ModuledefinitionAssignment_4_in_rule__Module__Group__4__Impl45082 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Module__Group__5__Impl_in_rule__Module__Group__545112 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000010L,0x0000000000002000L});
public static final BitSet FOLLOW_rule__Module__Group__6_in_rule__Module__Group__545115 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Module__Group_5__0_in_rule__Module__Group__5__Impl45142 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Module__Group__6__Impl_in_rule__Module__Group__645173 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_68_in_rule__Module__Group__6__Impl45201 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Module__Group_5__0__Impl_in_rule__Module__Group_5__045246 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000008L});
public static final BitSet FOLLOW_rule__Module__Group_5__1_in_rule__Module__Group_5__045249 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_141_in_rule__Module__Group_5__0__Impl45277 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Module__Group_5__1__Impl_in_rule__Module__Group_5__145308 = new BitSet(new long[]{0x0000000000000010L,0x0000000000000010L});
public static final BitSet FOLLOW_rule__Module__Group_5__2_in_rule__Module__Group_5__145311 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_67_in_rule__Module__Group_5__1__Impl45339 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Module__Group_5__2__Impl_in_rule__Module__Group_5__245370 = new BitSet(new long[]{0x0000000000000010L,0x0000000000000010L});
public static final BitSet FOLLOW_rule__Module__Group_5__3_in_rule__Module__Group_5__245373 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Module__ModuleParametersAssignment_5_2_in_rule__Module__Group_5__2__Impl45400 = new BitSet(new long[]{0x0000000000000012L});
public static final BitSet FOLLOW_rule__Module__Group_5__3__Impl_in_rule__Module__Group_5__345431 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_68_in_rule__Module__Group_5__3__Impl45459 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__ModuleParameter__Group__0__Impl_in_rule__ModuleParameter__Group__045498 = new BitSet(new long[]{0x0000000000002000L});
public static final BitSet FOLLOW_rule__ModuleParameter__Group__1_in_rule__ModuleParameter__Group__045501 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__ModuleParameter__ModuleDefinitionParameterAssignment_0_in_rule__ModuleParameter__Group__0__Impl45528 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__ModuleParameter__Group__1__Impl_in_rule__ModuleParameter__Group__145558 = new BitSet(new long[]{0x0000000000000100L});
public static final BitSet FOLLOW_rule__ModuleParameter__Group__2_in_rule__ModuleParameter__Group__145561 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_13_in_rule__ModuleParameter__Group__1__Impl45589 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__ModuleParameter__Group__2__Impl_in_rule__ModuleParameter__Group__245620 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__ModuleParameter__ValueAssignment_2_in_rule__ModuleParameter__Group__2__Impl45647 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__ModuleDefinition__Group__0__Impl_in_rule__ModuleDefinition__Group__045683 = new BitSet(new long[]{0x0000000000000010L});
public static final BitSet FOLLOW_rule__ModuleDefinition__Group__1_in_rule__ModuleDefinition__Group__045686 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_140_in_rule__ModuleDefinition__Group__0__Impl45714 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__ModuleDefinition__Group__1__Impl_in_rule__ModuleDefinition__Group__145745 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000008L});
public static final BitSet FOLLOW_rule__ModuleDefinition__Group__2_in_rule__ModuleDefinition__Group__145748 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__ModuleDefinition__NameAssignment_1_in_rule__ModuleDefinition__Group__1__Impl45775 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__ModuleDefinition__Group__2__Impl_in_rule__ModuleDefinition__Group__245805 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000010L,0x0000000000002000L});
public static final BitSet FOLLOW_rule__ModuleDefinition__Group__3_in_rule__ModuleDefinition__Group__245808 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_67_in_rule__ModuleDefinition__Group__2__Impl45836 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__ModuleDefinition__Group__3__Impl_in_rule__ModuleDefinition__Group__345867 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000010L,0x0000000000002000L});
public static final BitSet FOLLOW_rule__ModuleDefinition__Group__4_in_rule__ModuleDefinition__Group__345870 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__ModuleDefinition__Group_3__0_in_rule__ModuleDefinition__Group__3__Impl45897 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__ModuleDefinition__Group__4__Impl_in_rule__ModuleDefinition__Group__445928 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_68_in_rule__ModuleDefinition__Group__4__Impl45956 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__ModuleDefinition__Group_3__0__Impl_in_rule__ModuleDefinition__Group_3__045997 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000008L});
public static final BitSet FOLLOW_rule__ModuleDefinition__Group_3__1_in_rule__ModuleDefinition__Group_3__046000 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_141_in_rule__ModuleDefinition__Group_3__0__Impl46028 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__ModuleDefinition__Group_3__1__Impl_in_rule__ModuleDefinition__Group_3__146059 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000010L,0x0000000000004000L});
public static final BitSet FOLLOW_rule__ModuleDefinition__Group_3__2_in_rule__ModuleDefinition__Group_3__146062 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_67_in_rule__ModuleDefinition__Group_3__1__Impl46090 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__ModuleDefinition__Group_3__2__Impl_in_rule__ModuleDefinition__Group_3__246121 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000010L,0x0000000000004000L});
public static final BitSet FOLLOW_rule__ModuleDefinition__Group_3__3_in_rule__ModuleDefinition__Group_3__246124 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__ModuleDefinition__ModuleDefinitionParametersAssignment_3_2_in_rule__ModuleDefinition__Group_3__2__Impl46151 = new BitSet(new long[]{0x0000000000000002L,0x0000000000000000L,0x0000000000004000L});
public static final BitSet FOLLOW_rule__ModuleDefinition__Group_3__3__Impl_in_rule__ModuleDefinition__Group_3__346182 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_68_in_rule__ModuleDefinition__Group_3__3__Impl46210 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__ModuleDefinitionParameter__Group__0__Impl_in_rule__ModuleDefinitionParameter__Group__046249 = new BitSet(new long[]{0x0000000000000010L});
public static final BitSet FOLLOW_rule__ModuleDefinitionParameter__Group__1_in_rule__ModuleDefinitionParameter__Group__046252 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_142_in_rule__ModuleDefinitionParameter__Group__0__Impl46280 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__ModuleDefinitionParameter__Group__1__Impl_in_rule__ModuleDefinitionParameter__Group__146311 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000008L});
public static final BitSet FOLLOW_rule__ModuleDefinitionParameter__Group__2_in_rule__ModuleDefinitionParameter__Group__146314 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__ModuleDefinitionParameter__NameAssignment_1_in_rule__ModuleDefinitionParameter__Group__1__Impl46341 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__ModuleDefinitionParameter__Group__2__Impl_in_rule__ModuleDefinitionParameter__Group__246371 = new BitSet(new long[]{0x0000000000000000L,0x0200000000000000L});
public static final BitSet FOLLOW_rule__ModuleDefinitionParameter__Group__3_in_rule__ModuleDefinitionParameter__Group__246374 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_67_in_rule__ModuleDefinitionParameter__Group__2__Impl46402 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__ModuleDefinitionParameter__Group__3__Impl_in_rule__ModuleDefinitionParameter__Group__346433 = new BitSet(new long[]{0x3F00000000000000L});
public static final BitSet FOLLOW_rule__ModuleDefinitionParameter__Group__4_in_rule__ModuleDefinitionParameter__Group__346436 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_121_in_rule__ModuleDefinitionParameter__Group__3__Impl46464 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__ModuleDefinitionParameter__Group__4__Impl_in_rule__ModuleDefinitionParameter__Group__446495 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000010L});
public static final BitSet FOLLOW_rule__ModuleDefinitionParameter__Group__5_in_rule__ModuleDefinitionParameter__Group__446498 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__ModuleDefinitionParameter__TypeAssignment_4_in_rule__ModuleDefinitionParameter__Group__4__Impl46525 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__ModuleDefinitionParameter__Group__5__Impl_in_rule__ModuleDefinitionParameter__Group__546555 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_68_in_rule__ModuleDefinitionParameter__Group__5__Impl46583 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__ServiceOptions__Group__0__Impl_in_rule__ServiceOptions__Group__046626 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000000L,0x0010000000000000L});
public static final BitSet FOLLOW_rule__ServiceOptions__Group__1_in_rule__ServiceOptions__Group__046629 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__ServiceOptions__Group__1__Impl_in_rule__ServiceOptions__Group__146687 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__ServiceOptions__NonpublicAssignment_1_in_rule__ServiceOptions__Group__1__Impl46714 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__ServiceMethod__Group__0__Impl_in_rule__ServiceMethod__Group__046749 = new BitSet(new long[]{0x0000000088000010L,0x0000000000000000L,0x0000000000010000L});
public static final BitSet FOLLOW_rule__ServiceMethod__Group__1_in_rule__ServiceMethod__Group__046752 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_143_in_rule__ServiceMethod__Group__0__Impl46780 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__ServiceMethod__Group__1__Impl_in_rule__ServiceMethod__Group__146811 = new BitSet(new long[]{0x0000000088000010L,0x0000000000000000L,0x0000000000010000L});
public static final BitSet FOLLOW_rule__ServiceMethod__Group__2_in_rule__ServiceMethod__Group__146814 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__ServiceMethod__Group_1__0_in_rule__ServiceMethod__Group__1__Impl46841 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__ServiceMethod__Group__2__Impl_in_rule__ServiceMethod__Group__246872 = new BitSet(new long[]{0x0000000000000010L});
public static final BitSet FOLLOW_rule__ServiceMethod__Group__3_in_rule__ServiceMethod__Group__246875 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__ServiceMethod__ReturnTypeAssignment_2_in_rule__ServiceMethod__Group__2__Impl46902 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__ServiceMethod__Group__3__Impl_in_rule__ServiceMethod__Group__346932 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000000L,0x0000000000010000L});
public static final BitSet FOLLOW_rule__ServiceMethod__Group__4_in_rule__ServiceMethod__Group__346935 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__ServiceMethod__NameAssignment_3_in_rule__ServiceMethod__Group__3__Impl46962 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__ServiceMethod__Group__4__Impl_in_rule__ServiceMethod__Group__446992 = new BitSet(new long[]{0x0000000080000010L,0x0000000000000000L,0x0000000000030000L});
public static final BitSet FOLLOW_rule__ServiceMethod__Group__5_in_rule__ServiceMethod__Group__446995 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_144_in_rule__ServiceMethod__Group__4__Impl47023 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__ServiceMethod__Group__5__Impl_in_rule__ServiceMethod__Group__547054 = new BitSet(new long[]{0x0000000080000010L,0x0000000000000000L,0x0000000000030000L});
public static final BitSet FOLLOW_rule__ServiceMethod__Group__6_in_rule__ServiceMethod__Group__547057 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__ServiceMethod__Group_5__0_in_rule__ServiceMethod__Group__5__Impl47084 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__ServiceMethod__Group__6__Impl_in_rule__ServiceMethod__Group__647115 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_145_in_rule__ServiceMethod__Group__6__Impl47143 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__ServiceMethod__Group_1__0__Impl_in_rule__ServiceMethod__Group_1__047188 = new BitSet(new long[]{0x0000000000000010L});
public static final BitSet FOLLOW_rule__ServiceMethod__Group_1__1_in_rule__ServiceMethod__Group_1__047191 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_27_in_rule__ServiceMethod__Group_1__0__Impl47219 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__ServiceMethod__Group_1__1__Impl_in_rule__ServiceMethod__Group_1__147250 = new BitSet(new long[]{0x0000000004000000L});
public static final BitSet FOLLOW_rule__ServiceMethod__Group_1__2_in_rule__ServiceMethod__Group_1__147253 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__ServiceMethod__TypeParameterAssignment_1_1_in_rule__ServiceMethod__Group_1__1__Impl47280 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__ServiceMethod__Group_1__2__Impl_in_rule__ServiceMethod__Group_1__247310 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_26_in_rule__ServiceMethod__Group_1__2__Impl47338 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__ServiceMethod__Group_5__0__Impl_in_rule__ServiceMethod__Group_5__047375 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000100L});
public static final BitSet FOLLOW_rule__ServiceMethod__Group_5__1_in_rule__ServiceMethod__Group_5__047378 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__ServiceMethod__ParamsAssignment_5_0_in_rule__ServiceMethod__Group_5__0__Impl47405 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__ServiceMethod__Group_5__1__Impl_in_rule__ServiceMethod__Group_5__147435 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__ServiceMethod__Group_5_1__0_in_rule__ServiceMethod__Group_5__1__Impl47462 = new BitSet(new long[]{0x0000000000000002L,0x0000000000000100L});
public static final BitSet FOLLOW_rule__ServiceMethod__Group_5_1__0__Impl_in_rule__ServiceMethod__Group_5_1__047497 = new BitSet(new long[]{0x0000000080000010L,0x0000000000000000L,0x0000000000010000L});
public static final BitSet FOLLOW_rule__ServiceMethod__Group_5_1__1_in_rule__ServiceMethod__Group_5_1__047500 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_72_in_rule__ServiceMethod__Group_5_1__0__Impl47528 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__ServiceMethod__Group_5_1__1__Impl_in_rule__ServiceMethod__Group_5_1__147559 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__ServiceMethod__ParamsAssignment_5_1_1_in_rule__ServiceMethod__Group_5_1__1__Impl47586 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Service__Group__0__Impl_in_rule__Service__Group__047620 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000000L,0x0000000000040000L});
public static final BitSet FOLLOW_rule__Service__Group__1_in_rule__Service__Group__047623 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Service__Group__1__Impl_in_rule__Service__Group__147681 = new BitSet(new long[]{0x0000000000000010L});
public static final BitSet FOLLOW_rule__Service__Group__2_in_rule__Service__Group__147684 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_146_in_rule__Service__Group__1__Impl47712 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Service__Group__2__Impl_in_rule__Service__Group__247743 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000008L});
public static final BitSet FOLLOW_rule__Service__Group__3_in_rule__Service__Group__247746 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Service__NameAssignment_2_in_rule__Service__Group__2__Impl47773 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Service__Group__3__Impl_in_rule__Service__Group__347803 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000010L,0x0000000000088000L});
public static final BitSet FOLLOW_rule__Service__Group__4_in_rule__Service__Group__347806 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_67_in_rule__Service__Group__3__Impl47834 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Service__Group__4__Impl_in_rule__Service__Group__447865 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000010L,0x0000000000088000L});
public static final BitSet FOLLOW_rule__Service__Group__5_in_rule__Service__Group__447868 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Service__Group_4__0_in_rule__Service__Group__4__Impl47895 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Service__Group__5__Impl_in_rule__Service__Group__547926 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000010L,0x0000000000088000L});
public static final BitSet FOLLOW_rule__Service__Group__6_in_rule__Service__Group__547929 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Service__RemoteMethodsAssignment_5_in_rule__Service__Group__5__Impl47956 = new BitSet(new long[]{0x0000000000000002L,0x0000000000000000L,0x0000000000008000L});
public static final BitSet FOLLOW_rule__Service__Group__6__Impl_in_rule__Service__Group__647987 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_68_in_rule__Service__Group__6__Impl48015 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Service__Group_4__0__Impl_in_rule__Service__Group_4__048060 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000008L});
public static final BitSet FOLLOW_rule__Service__Group_4__1_in_rule__Service__Group_4__048063 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_147_in_rule__Service__Group_4__0__Impl48091 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Service__Group_4__1__Impl_in_rule__Service__Group_4__148122 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000000L,0x0010000000000000L});
public static final BitSet FOLLOW_rule__Service__Group_4__2_in_rule__Service__Group_4__148125 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_67_in_rule__Service__Group_4__1__Impl48153 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Service__Group_4__2__Impl_in_rule__Service__Group_4__248184 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000010L});
public static final BitSet FOLLOW_rule__Service__Group_4__3_in_rule__Service__Group_4__248187 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Service__RemoteServiceOptionsAssignment_4_2_in_rule__Service__Group_4__2__Impl48214 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Service__Group_4__3__Impl_in_rule__Service__Group_4__348244 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_68_in_rule__Service__Group_4__3__Impl48272 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__NavigationNode__Group__0__Impl_in_rule__NavigationNode__Group__048311 = new BitSet(new long[]{0x0000000000000010L});
public static final BitSet FOLLOW_rule__NavigationNode__Group__1_in_rule__NavigationNode__Group__048314 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_148_in_rule__NavigationNode__Group__0__Impl48342 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__NavigationNode__Group__1__Impl_in_rule__NavigationNode__Group__148373 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000008L});
public static final BitSet FOLLOW_rule__NavigationNode__Group__2_in_rule__NavigationNode__Group__148376 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__NavigationNode__NameAssignment_1_in_rule__NavigationNode__Group__1__Impl48403 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__NavigationNode__Group__2__Impl_in_rule__NavigationNode__Group__248433 = new BitSet(new long[]{0x0000000000000000L,0x0000003AB5C54870L,0x0000000000F41800L});
public static final BitSet FOLLOW_rule__NavigationNode__Group__3_in_rule__NavigationNode__Group__248436 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_67_in_rule__NavigationNode__Group__2__Impl48464 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__NavigationNode__Group__3__Impl_in_rule__NavigationNode__Group__348495 = new BitSet(new long[]{0x0000000000000000L,0x0000003AB5C54870L,0x0000000000F41800L});
public static final BitSet FOLLOW_rule__NavigationNode__Group__4_in_rule__NavigationNode__Group__348498 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__NavigationNode__Group_3__0_in_rule__NavigationNode__Group__3__Impl48525 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__NavigationNode__Group__4__Impl_in_rule__NavigationNode__Group__448556 = new BitSet(new long[]{0x0000000000000000L,0x0000003AB5C54870L,0x0000000000F41800L});
public static final BitSet FOLLOW_rule__NavigationNode__Group__5_in_rule__NavigationNode__Group__448559 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__NavigationNode__Group_4__0_in_rule__NavigationNode__Group__4__Impl48586 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__NavigationNode__Group__5__Impl_in_rule__NavigationNode__Group__548617 = new BitSet(new long[]{0x0000000000000000L,0x0000003AB5C54870L,0x0000000000F41800L});
public static final BitSet FOLLOW_rule__NavigationNode__Group__6_in_rule__NavigationNode__Group__548620 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__NavigationNode__Group_5__0_in_rule__NavigationNode__Group__5__Impl48647 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__NavigationNode__Group__6__Impl_in_rule__NavigationNode__Group__648678 = new BitSet(new long[]{0x0000000000000000L,0x0000003AB5C54870L,0x0000000000F41800L});
public static final BitSet FOLLOW_rule__NavigationNode__Group__7_in_rule__NavigationNode__Group__648681 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__NavigationNode__Group_6__0_in_rule__NavigationNode__Group__6__Impl48708 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__NavigationNode__Group__7__Impl_in_rule__NavigationNode__Group__748739 = new BitSet(new long[]{0x0000000000000000L,0x0000003AB5C54870L,0x0000000000F41800L});
public static final BitSet FOLLOW_rule__NavigationNode__Group__8_in_rule__NavigationNode__Group__748742 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__NavigationNode__Group_7__0_in_rule__NavigationNode__Group__7__Impl48769 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__NavigationNode__Group__8__Impl_in_rule__NavigationNode__Group__848800 = new BitSet(new long[]{0x0000000000000000L,0x0000003AB5C54870L,0x0000000000F41800L});
public static final BitSet FOLLOW_rule__NavigationNode__Group__9_in_rule__NavigationNode__Group__848803 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__NavigationNode__NavigationNodesAssignment_8_in_rule__NavigationNode__Group__8__Impl48830 = new BitSet(new long[]{0x0000000000000002L,0x0000003AB5C54060L,0x0000000000141800L});
public static final BitSet FOLLOW_rule__NavigationNode__Group__9__Impl_in_rule__NavigationNode__Group__948861 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_68_in_rule__NavigationNode__Group__9__Impl48889 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__NavigationNode__Group_3__0__Impl_in_rule__NavigationNode__Group_3__048940 = new BitSet(new long[]{0x0000000000000100L});
public static final BitSet FOLLOW_rule__NavigationNode__Group_3__1_in_rule__NavigationNode__Group_3__048943 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_75_in_rule__NavigationNode__Group_3__0__Impl48971 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__NavigationNode__Group_3__1__Impl_in_rule__NavigationNode__Group_3__149002 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__NavigationNode__LabelAssignment_3_1_in_rule__NavigationNode__Group_3__1__Impl49029 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__NavigationNode__Group_4__0__Impl_in_rule__NavigationNode__Group_4__049063 = new BitSet(new long[]{0x0000000000000010L});
public static final BitSet FOLLOW_rule__NavigationNode__Group_4__1_in_rule__NavigationNode__Group_4__049066 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_149_in_rule__NavigationNode__Group_4__0__Impl49094 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__NavigationNode__Group_4__1__Impl_in_rule__NavigationNode__Group_4__149125 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__NavigationNode__ModuleDefinitionAssignment_4_1_in_rule__NavigationNode__Group_4__1__Impl49152 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__NavigationNode__Group_5__0__Impl_in_rule__NavigationNode__Group_5__049186 = new BitSet(new long[]{0x0000000000000010L});
public static final BitSet FOLLOW_rule__NavigationNode__Group_5__1_in_rule__NavigationNode__Group_5__049189 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_139_in_rule__NavigationNode__Group_5__0__Impl49217 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__NavigationNode__Group_5__1__Impl_in_rule__NavigationNode__Group_5__149248 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__NavigationNode__ModuleAssignment_5_1_in_rule__NavigationNode__Group_5__1__Impl49275 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__NavigationNode__Group_6__0__Impl_in_rule__NavigationNode__Group_6__049309 = new BitSet(new long[]{0x0000000000000010L});
public static final BitSet FOLLOW_rule__NavigationNode__Group_6__1_in_rule__NavigationNode__Group_6__049312 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_150_in_rule__NavigationNode__Group_6__0__Impl49340 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__NavigationNode__Group_6__1__Impl_in_rule__NavigationNode__Group_6__149371 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__NavigationNode__DictionaryEditorAssignment_6_1_in_rule__NavigationNode__Group_6__1__Impl49398 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__NavigationNode__Group_7__0__Impl_in_rule__NavigationNode__Group_7__049432 = new BitSet(new long[]{0x0000000000000010L});
public static final BitSet FOLLOW_rule__NavigationNode__Group_7__1_in_rule__NavigationNode__Group_7__049435 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_151_in_rule__NavigationNode__Group_7__0__Impl49463 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__NavigationNode__Group_7__1__Impl_in_rule__NavigationNode__Group_7__149494 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__NavigationNode__DictionarySearchAssignment_7_1_in_rule__NavigationNode__Group_7__1__Impl49521 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XAssignment__Group_0__0__Impl_in_rule__XAssignment__Group_0__049555 = new BitSet(new long[]{0x0001E00000000010L});
public static final BitSet FOLLOW_rule__XAssignment__Group_0__1_in_rule__XAssignment__Group_0__049558 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XAssignment__Group_0__1__Impl_in_rule__XAssignment__Group_0__149616 = new BitSet(new long[]{0x0000000000002000L});
public static final BitSet FOLLOW_rule__XAssignment__Group_0__2_in_rule__XAssignment__Group_0__149619 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XAssignment__FeatureAssignment_0_1_in_rule__XAssignment__Group_0__1__Impl49646 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XAssignment__Group_0__2__Impl_in_rule__XAssignment__Group_0__249676 = new BitSet(new long[]{0x0007E10C080001F0L,0x0000000000000008L,0x0200BFE298010000L});
public static final BitSet FOLLOW_rule__XAssignment__Group_0__3_in_rule__XAssignment__Group_0__249679 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleOpSingleAssign_in_rule__XAssignment__Group_0__2__Impl49706 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XAssignment__Group_0__3__Impl_in_rule__XAssignment__Group_0__349735 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XAssignment__ValueAssignment_0_3_in_rule__XAssignment__Group_0__3__Impl49762 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XAssignment__Group_1__0__Impl_in_rule__XAssignment__Group_1__049800 = new BitSet(new long[]{0x000000000C1F0000L});
public static final BitSet FOLLOW_rule__XAssignment__Group_1__1_in_rule__XAssignment__Group_1__049803 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXOrExpression_in_rule__XAssignment__Group_1__0__Impl49830 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XAssignment__Group_1__1__Impl_in_rule__XAssignment__Group_1__149859 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XAssignment__Group_1_1__0_in_rule__XAssignment__Group_1__1__Impl49886 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XAssignment__Group_1_1__0__Impl_in_rule__XAssignment__Group_1_1__049921 = new BitSet(new long[]{0x0007E10C080001F0L,0x0000000000000008L,0x0200BFE298010000L});
public static final BitSet FOLLOW_rule__XAssignment__Group_1_1__1_in_rule__XAssignment__Group_1_1__049924 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XAssignment__Group_1_1_0__0_in_rule__XAssignment__Group_1_1__0__Impl49951 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XAssignment__Group_1_1__1__Impl_in_rule__XAssignment__Group_1_1__149981 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XAssignment__RightOperandAssignment_1_1_1_in_rule__XAssignment__Group_1_1__1__Impl50008 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XAssignment__Group_1_1_0__0__Impl_in_rule__XAssignment__Group_1_1_0__050042 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XAssignment__Group_1_1_0_0__0_in_rule__XAssignment__Group_1_1_0__0__Impl50069 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XAssignment__Group_1_1_0_0__0__Impl_in_rule__XAssignment__Group_1_1_0_0__050101 = new BitSet(new long[]{0x000000000C1F0000L});
public static final BitSet FOLLOW_rule__XAssignment__Group_1_1_0_0__1_in_rule__XAssignment__Group_1_1_0_0__050104 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XAssignment__Group_1_1_0_0__1__Impl_in_rule__XAssignment__Group_1_1_0_0__150162 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XAssignment__FeatureAssignment_1_1_0_0_1_in_rule__XAssignment__Group_1_1_0_0__1__Impl50189 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__OpMultiAssign__Group_5__0__Impl_in_rule__OpMultiAssign__Group_5__050223 = new BitSet(new long[]{0x0000000008000000L});
public static final BitSet FOLLOW_rule__OpMultiAssign__Group_5__1_in_rule__OpMultiAssign__Group_5__050226 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_27_in_rule__OpMultiAssign__Group_5__0__Impl50254 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__OpMultiAssign__Group_5__1__Impl_in_rule__OpMultiAssign__Group_5__150285 = new BitSet(new long[]{0x0000000000002000L});
public static final BitSet FOLLOW_rule__OpMultiAssign__Group_5__2_in_rule__OpMultiAssign__Group_5__150288 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_27_in_rule__OpMultiAssign__Group_5__1__Impl50316 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__OpMultiAssign__Group_5__2__Impl_in_rule__OpMultiAssign__Group_5__250347 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_13_in_rule__OpMultiAssign__Group_5__2__Impl50375 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__OpMultiAssign__Group_6__0__Impl_in_rule__OpMultiAssign__Group_6__050412 = new BitSet(new long[]{0x0000000006000000L});
public static final BitSet FOLLOW_rule__OpMultiAssign__Group_6__1_in_rule__OpMultiAssign__Group_6__050415 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_26_in_rule__OpMultiAssign__Group_6__0__Impl50443 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__OpMultiAssign__Group_6__1__Impl_in_rule__OpMultiAssign__Group_6__150474 = new BitSet(new long[]{0x0000000006000000L});
public static final BitSet FOLLOW_rule__OpMultiAssign__Group_6__2_in_rule__OpMultiAssign__Group_6__150477 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_26_in_rule__OpMultiAssign__Group_6__1__Impl50506 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__OpMultiAssign__Group_6__2__Impl_in_rule__OpMultiAssign__Group_6__250539 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_25_in_rule__OpMultiAssign__Group_6__2__Impl50567 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XOrExpression__Group__0__Impl_in_rule__XOrExpression__Group__050604 = new BitSet(new long[]{0x0000000000004000L});
public static final BitSet FOLLOW_rule__XOrExpression__Group__1_in_rule__XOrExpression__Group__050607 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXAndExpression_in_rule__XOrExpression__Group__0__Impl50634 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XOrExpression__Group__1__Impl_in_rule__XOrExpression__Group__150663 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XOrExpression__Group_1__0_in_rule__XOrExpression__Group__1__Impl50690 = new BitSet(new long[]{0x0000000000004002L});
public static final BitSet FOLLOW_rule__XOrExpression__Group_1__0__Impl_in_rule__XOrExpression__Group_1__050725 = new BitSet(new long[]{0x0007E10C080001F0L,0x0000000000000008L,0x0200BFE298010000L});
public static final BitSet FOLLOW_rule__XOrExpression__Group_1__1_in_rule__XOrExpression__Group_1__050728 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XOrExpression__Group_1_0__0_in_rule__XOrExpression__Group_1__0__Impl50755 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XOrExpression__Group_1__1__Impl_in_rule__XOrExpression__Group_1__150785 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XOrExpression__RightOperandAssignment_1_1_in_rule__XOrExpression__Group_1__1__Impl50812 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XOrExpression__Group_1_0__0__Impl_in_rule__XOrExpression__Group_1_0__050846 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XOrExpression__Group_1_0_0__0_in_rule__XOrExpression__Group_1_0__0__Impl50873 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XOrExpression__Group_1_0_0__0__Impl_in_rule__XOrExpression__Group_1_0_0__050905 = new BitSet(new long[]{0x0000000000004000L});
public static final BitSet FOLLOW_rule__XOrExpression__Group_1_0_0__1_in_rule__XOrExpression__Group_1_0_0__050908 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XOrExpression__Group_1_0_0__1__Impl_in_rule__XOrExpression__Group_1_0_0__150966 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XOrExpression__FeatureAssignment_1_0_0_1_in_rule__XOrExpression__Group_1_0_0__1__Impl50993 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XAndExpression__Group__0__Impl_in_rule__XAndExpression__Group__051027 = new BitSet(new long[]{0x0000000000008000L});
public static final BitSet FOLLOW_rule__XAndExpression__Group__1_in_rule__XAndExpression__Group__051030 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXEqualityExpression_in_rule__XAndExpression__Group__0__Impl51057 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XAndExpression__Group__1__Impl_in_rule__XAndExpression__Group__151086 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XAndExpression__Group_1__0_in_rule__XAndExpression__Group__1__Impl51113 = new BitSet(new long[]{0x0000000000008002L});
public static final BitSet FOLLOW_rule__XAndExpression__Group_1__0__Impl_in_rule__XAndExpression__Group_1__051148 = new BitSet(new long[]{0x0007E10C080001F0L,0x0000000000000008L,0x0200BFE298010000L});
public static final BitSet FOLLOW_rule__XAndExpression__Group_1__1_in_rule__XAndExpression__Group_1__051151 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XAndExpression__Group_1_0__0_in_rule__XAndExpression__Group_1__0__Impl51178 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XAndExpression__Group_1__1__Impl_in_rule__XAndExpression__Group_1__151208 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XAndExpression__RightOperandAssignment_1_1_in_rule__XAndExpression__Group_1__1__Impl51235 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XAndExpression__Group_1_0__0__Impl_in_rule__XAndExpression__Group_1_0__051269 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XAndExpression__Group_1_0_0__0_in_rule__XAndExpression__Group_1_0__0__Impl51296 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XAndExpression__Group_1_0_0__0__Impl_in_rule__XAndExpression__Group_1_0_0__051328 = new BitSet(new long[]{0x0000000000008000L});
public static final BitSet FOLLOW_rule__XAndExpression__Group_1_0_0__1_in_rule__XAndExpression__Group_1_0_0__051331 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XAndExpression__Group_1_0_0__1__Impl_in_rule__XAndExpression__Group_1_0_0__151389 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XAndExpression__FeatureAssignment_1_0_0_1_in_rule__XAndExpression__Group_1_0_0__1__Impl51416 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XEqualityExpression__Group__0__Impl_in_rule__XEqualityExpression__Group__051450 = new BitSet(new long[]{0x0000000001E00000L});
public static final BitSet FOLLOW_rule__XEqualityExpression__Group__1_in_rule__XEqualityExpression__Group__051453 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXRelationalExpression_in_rule__XEqualityExpression__Group__0__Impl51480 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XEqualityExpression__Group__1__Impl_in_rule__XEqualityExpression__Group__151509 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XEqualityExpression__Group_1__0_in_rule__XEqualityExpression__Group__1__Impl51536 = new BitSet(new long[]{0x0000000001E00002L});
public static final BitSet FOLLOW_rule__XEqualityExpression__Group_1__0__Impl_in_rule__XEqualityExpression__Group_1__051571 = new BitSet(new long[]{0x0007E10C080001F0L,0x0000000000000008L,0x0200BFE298010000L});
public static final BitSet FOLLOW_rule__XEqualityExpression__Group_1__1_in_rule__XEqualityExpression__Group_1__051574 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XEqualityExpression__Group_1_0__0_in_rule__XEqualityExpression__Group_1__0__Impl51601 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XEqualityExpression__Group_1__1__Impl_in_rule__XEqualityExpression__Group_1__151631 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XEqualityExpression__RightOperandAssignment_1_1_in_rule__XEqualityExpression__Group_1__1__Impl51658 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XEqualityExpression__Group_1_0__0__Impl_in_rule__XEqualityExpression__Group_1_0__051692 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XEqualityExpression__Group_1_0_0__0_in_rule__XEqualityExpression__Group_1_0__0__Impl51719 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XEqualityExpression__Group_1_0_0__0__Impl_in_rule__XEqualityExpression__Group_1_0_0__051751 = new BitSet(new long[]{0x0000000001E00000L});
public static final BitSet FOLLOW_rule__XEqualityExpression__Group_1_0_0__1_in_rule__XEqualityExpression__Group_1_0_0__051754 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XEqualityExpression__Group_1_0_0__1__Impl_in_rule__XEqualityExpression__Group_1_0_0__151812 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XEqualityExpression__FeatureAssignment_1_0_0_1_in_rule__XEqualityExpression__Group_1_0_0__1__Impl51839 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XRelationalExpression__Group__0__Impl_in_rule__XRelationalExpression__Group__051873 = new BitSet(new long[]{0x000000000E000000L,0x0000000000000000L,0x0000000001000000L});
public static final BitSet FOLLOW_rule__XRelationalExpression__Group__1_in_rule__XRelationalExpression__Group__051876 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXOtherOperatorExpression_in_rule__XRelationalExpression__Group__0__Impl51903 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XRelationalExpression__Group__1__Impl_in_rule__XRelationalExpression__Group__151932 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XRelationalExpression__Alternatives_1_in_rule__XRelationalExpression__Group__1__Impl51959 = new BitSet(new long[]{0x000000000E000002L,0x0000000000000000L,0x0000000001000000L});
public static final BitSet FOLLOW_rule__XRelationalExpression__Group_1_0__0__Impl_in_rule__XRelationalExpression__Group_1_0__051994 = new BitSet(new long[]{0x0000000080000010L,0x0000000000000000L,0x0000000000010000L});
public static final BitSet FOLLOW_rule__XRelationalExpression__Group_1_0__1_in_rule__XRelationalExpression__Group_1_0__051997 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XRelationalExpression__Group_1_0_0__0_in_rule__XRelationalExpression__Group_1_0__0__Impl52024 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XRelationalExpression__Group_1_0__1__Impl_in_rule__XRelationalExpression__Group_1_0__152054 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XRelationalExpression__TypeAssignment_1_0_1_in_rule__XRelationalExpression__Group_1_0__1__Impl52081 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XRelationalExpression__Group_1_0_0__0__Impl_in_rule__XRelationalExpression__Group_1_0_0__052115 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XRelationalExpression__Group_1_0_0_0__0_in_rule__XRelationalExpression__Group_1_0_0__0__Impl52142 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XRelationalExpression__Group_1_0_0_0__0__Impl_in_rule__XRelationalExpression__Group_1_0_0_0__052174 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000000L,0x0000000001000000L});
public static final BitSet FOLLOW_rule__XRelationalExpression__Group_1_0_0_0__1_in_rule__XRelationalExpression__Group_1_0_0_0__052177 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XRelationalExpression__Group_1_0_0_0__1__Impl_in_rule__XRelationalExpression__Group_1_0_0_0__152235 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_152_in_rule__XRelationalExpression__Group_1_0_0_0__1__Impl52263 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XRelationalExpression__Group_1_1__0__Impl_in_rule__XRelationalExpression__Group_1_1__052298 = new BitSet(new long[]{0x0007E10C080001F0L,0x0000000000000008L,0x0200BFE298010000L});
public static final BitSet FOLLOW_rule__XRelationalExpression__Group_1_1__1_in_rule__XRelationalExpression__Group_1_1__052301 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XRelationalExpression__Group_1_1_0__0_in_rule__XRelationalExpression__Group_1_1__0__Impl52328 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XRelationalExpression__Group_1_1__1__Impl_in_rule__XRelationalExpression__Group_1_1__152358 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XRelationalExpression__RightOperandAssignment_1_1_1_in_rule__XRelationalExpression__Group_1_1__1__Impl52385 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XRelationalExpression__Group_1_1_0__0__Impl_in_rule__XRelationalExpression__Group_1_1_0__052419 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XRelationalExpression__Group_1_1_0_0__0_in_rule__XRelationalExpression__Group_1_1_0__0__Impl52446 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XRelationalExpression__Group_1_1_0_0__0__Impl_in_rule__XRelationalExpression__Group_1_1_0_0__052478 = new BitSet(new long[]{0x000000000E000000L,0x0000000000000000L,0x0000000001000000L});
public static final BitSet FOLLOW_rule__XRelationalExpression__Group_1_1_0_0__1_in_rule__XRelationalExpression__Group_1_1_0_0__052481 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XRelationalExpression__Group_1_1_0_0__1__Impl_in_rule__XRelationalExpression__Group_1_1_0_0__152539 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XRelationalExpression__FeatureAssignment_1_1_0_0_1_in_rule__XRelationalExpression__Group_1_1_0_0__1__Impl52566 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__OpCompare__Group_1__0__Impl_in_rule__OpCompare__Group_1__052600 = new BitSet(new long[]{0x0000000000002000L});
public static final BitSet FOLLOW_rule__OpCompare__Group_1__1_in_rule__OpCompare__Group_1__052603 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_27_in_rule__OpCompare__Group_1__0__Impl52631 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__OpCompare__Group_1__1__Impl_in_rule__OpCompare__Group_1__152662 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_13_in_rule__OpCompare__Group_1__1__Impl52690 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XOtherOperatorExpression__Group__0__Impl_in_rule__XOtherOperatorExpression__Group__052725 = new BitSet(new long[]{0x00000003FC000000L});
public static final BitSet FOLLOW_rule__XOtherOperatorExpression__Group__1_in_rule__XOtherOperatorExpression__Group__052728 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXAdditiveExpression_in_rule__XOtherOperatorExpression__Group__0__Impl52755 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XOtherOperatorExpression__Group__1__Impl_in_rule__XOtherOperatorExpression__Group__152784 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XOtherOperatorExpression__Group_1__0_in_rule__XOtherOperatorExpression__Group__1__Impl52811 = new BitSet(new long[]{0x00000003FC000002L});
public static final BitSet FOLLOW_rule__XOtherOperatorExpression__Group_1__0__Impl_in_rule__XOtherOperatorExpression__Group_1__052846 = new BitSet(new long[]{0x0007E10C080001F0L,0x0000000000000008L,0x0200BFE298010000L});
public static final BitSet FOLLOW_rule__XOtherOperatorExpression__Group_1__1_in_rule__XOtherOperatorExpression__Group_1__052849 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XOtherOperatorExpression__Group_1_0__0_in_rule__XOtherOperatorExpression__Group_1__0__Impl52876 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XOtherOperatorExpression__Group_1__1__Impl_in_rule__XOtherOperatorExpression__Group_1__152906 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XOtherOperatorExpression__RightOperandAssignment_1_1_in_rule__XOtherOperatorExpression__Group_1__1__Impl52933 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XOtherOperatorExpression__Group_1_0__0__Impl_in_rule__XOtherOperatorExpression__Group_1_0__052967 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XOtherOperatorExpression__Group_1_0_0__0_in_rule__XOtherOperatorExpression__Group_1_0__0__Impl52994 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XOtherOperatorExpression__Group_1_0_0__0__Impl_in_rule__XOtherOperatorExpression__Group_1_0_0__053026 = new BitSet(new long[]{0x00000003FC000000L});
public static final BitSet FOLLOW_rule__XOtherOperatorExpression__Group_1_0_0__1_in_rule__XOtherOperatorExpression__Group_1_0_0__053029 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XOtherOperatorExpression__Group_1_0_0__1__Impl_in_rule__XOtherOperatorExpression__Group_1_0_0__153087 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XOtherOperatorExpression__FeatureAssignment_1_0_0_1_in_rule__XOtherOperatorExpression__Group_1_0_0__1__Impl53114 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__OpOther__Group_2__0__Impl_in_rule__OpOther__Group_2__053148 = new BitSet(new long[]{0x0000000040000000L});
public static final BitSet FOLLOW_rule__OpOther__Group_2__1_in_rule__OpOther__Group_2__053151 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_26_in_rule__OpOther__Group_2__0__Impl53179 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__OpOther__Group_2__1__Impl_in_rule__OpOther__Group_2__153210 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_30_in_rule__OpOther__Group_2__1__Impl53238 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__OpOther__Group_5__0__Impl_in_rule__OpOther__Group_5__053273 = new BitSet(new long[]{0x0000000004000000L});
public static final BitSet FOLLOW_rule__OpOther__Group_5__1_in_rule__OpOther__Group_5__053276 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_26_in_rule__OpOther__Group_5__0__Impl53304 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__OpOther__Group_5__1__Impl_in_rule__OpOther__Group_5__153335 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__OpOther__Alternatives_5_1_in_rule__OpOther__Group_5__1__Impl53362 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__OpOther__Group_5_1_0__0__Impl_in_rule__OpOther__Group_5_1_0__053396 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__OpOther__Group_5_1_0_0__0_in_rule__OpOther__Group_5_1_0__0__Impl53423 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__OpOther__Group_5_1_0_0__0__Impl_in_rule__OpOther__Group_5_1_0_0__053455 = new BitSet(new long[]{0x0000000004000000L});
public static final BitSet FOLLOW_rule__OpOther__Group_5_1_0_0__1_in_rule__OpOther__Group_5_1_0_0__053458 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_26_in_rule__OpOther__Group_5_1_0_0__0__Impl53486 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__OpOther__Group_5_1_0_0__1__Impl_in_rule__OpOther__Group_5_1_0_0__153517 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_26_in_rule__OpOther__Group_5_1_0_0__1__Impl53545 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__OpOther__Group_6__0__Impl_in_rule__OpOther__Group_6__053580 = new BitSet(new long[]{0x0000000088000000L});
public static final BitSet FOLLOW_rule__OpOther__Group_6__1_in_rule__OpOther__Group_6__053583 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_27_in_rule__OpOther__Group_6__0__Impl53611 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__OpOther__Group_6__1__Impl_in_rule__OpOther__Group_6__153642 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__OpOther__Alternatives_6_1_in_rule__OpOther__Group_6__1__Impl53669 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__OpOther__Group_6_1_0__0__Impl_in_rule__OpOther__Group_6_1_0__053703 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__OpOther__Group_6_1_0_0__0_in_rule__OpOther__Group_6_1_0__0__Impl53730 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__OpOther__Group_6_1_0_0__0__Impl_in_rule__OpOther__Group_6_1_0_0__053762 = new BitSet(new long[]{0x0000000008000000L});
public static final BitSet FOLLOW_rule__OpOther__Group_6_1_0_0__1_in_rule__OpOther__Group_6_1_0_0__053765 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_27_in_rule__OpOther__Group_6_1_0_0__0__Impl53793 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__OpOther__Group_6_1_0_0__1__Impl_in_rule__OpOther__Group_6_1_0_0__153824 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_27_in_rule__OpOther__Group_6_1_0_0__1__Impl53852 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XAdditiveExpression__Group__0__Impl_in_rule__XAdditiveExpression__Group__053887 = new BitSet(new long[]{0x0000000C00000000L});
public static final BitSet FOLLOW_rule__XAdditiveExpression__Group__1_in_rule__XAdditiveExpression__Group__053890 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXMultiplicativeExpression_in_rule__XAdditiveExpression__Group__0__Impl53917 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XAdditiveExpression__Group__1__Impl_in_rule__XAdditiveExpression__Group__153946 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XAdditiveExpression__Group_1__0_in_rule__XAdditiveExpression__Group__1__Impl53973 = new BitSet(new long[]{0x0000000C00000002L});
public static final BitSet FOLLOW_rule__XAdditiveExpression__Group_1__0__Impl_in_rule__XAdditiveExpression__Group_1__054008 = new BitSet(new long[]{0x0007E10C080001F0L,0x0000000000000008L,0x0200BFE298010000L});
public static final BitSet FOLLOW_rule__XAdditiveExpression__Group_1__1_in_rule__XAdditiveExpression__Group_1__054011 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XAdditiveExpression__Group_1_0__0_in_rule__XAdditiveExpression__Group_1__0__Impl54038 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XAdditiveExpression__Group_1__1__Impl_in_rule__XAdditiveExpression__Group_1__154068 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XAdditiveExpression__RightOperandAssignment_1_1_in_rule__XAdditiveExpression__Group_1__1__Impl54095 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XAdditiveExpression__Group_1_0__0__Impl_in_rule__XAdditiveExpression__Group_1_0__054129 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XAdditiveExpression__Group_1_0_0__0_in_rule__XAdditiveExpression__Group_1_0__0__Impl54156 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XAdditiveExpression__Group_1_0_0__0__Impl_in_rule__XAdditiveExpression__Group_1_0_0__054188 = new BitSet(new long[]{0x0000000C00000000L});
public static final BitSet FOLLOW_rule__XAdditiveExpression__Group_1_0_0__1_in_rule__XAdditiveExpression__Group_1_0_0__054191 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XAdditiveExpression__Group_1_0_0__1__Impl_in_rule__XAdditiveExpression__Group_1_0_0__154249 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XAdditiveExpression__FeatureAssignment_1_0_0_1_in_rule__XAdditiveExpression__Group_1_0_0__1__Impl54276 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XMultiplicativeExpression__Group__0__Impl_in_rule__XMultiplicativeExpression__Group__054310 = new BitSet(new long[]{0x000000F000000000L});
public static final BitSet FOLLOW_rule__XMultiplicativeExpression__Group__1_in_rule__XMultiplicativeExpression__Group__054313 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXUnaryOperation_in_rule__XMultiplicativeExpression__Group__0__Impl54340 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XMultiplicativeExpression__Group__1__Impl_in_rule__XMultiplicativeExpression__Group__154369 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XMultiplicativeExpression__Group_1__0_in_rule__XMultiplicativeExpression__Group__1__Impl54396 = new BitSet(new long[]{0x000000F000000002L});
public static final BitSet FOLLOW_rule__XMultiplicativeExpression__Group_1__0__Impl_in_rule__XMultiplicativeExpression__Group_1__054431 = new BitSet(new long[]{0x0007E10C080001F0L,0x0000000000000008L,0x0200BFE298010000L});
public static final BitSet FOLLOW_rule__XMultiplicativeExpression__Group_1__1_in_rule__XMultiplicativeExpression__Group_1__054434 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XMultiplicativeExpression__Group_1_0__0_in_rule__XMultiplicativeExpression__Group_1__0__Impl54461 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XMultiplicativeExpression__Group_1__1__Impl_in_rule__XMultiplicativeExpression__Group_1__154491 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XMultiplicativeExpression__RightOperandAssignment_1_1_in_rule__XMultiplicativeExpression__Group_1__1__Impl54518 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XMultiplicativeExpression__Group_1_0__0__Impl_in_rule__XMultiplicativeExpression__Group_1_0__054552 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XMultiplicativeExpression__Group_1_0_0__0_in_rule__XMultiplicativeExpression__Group_1_0__0__Impl54579 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XMultiplicativeExpression__Group_1_0_0__0__Impl_in_rule__XMultiplicativeExpression__Group_1_0_0__054611 = new BitSet(new long[]{0x000000F000000000L});
public static final BitSet FOLLOW_rule__XMultiplicativeExpression__Group_1_0_0__1_in_rule__XMultiplicativeExpression__Group_1_0_0__054614 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XMultiplicativeExpression__Group_1_0_0__1__Impl_in_rule__XMultiplicativeExpression__Group_1_0_0__154672 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XMultiplicativeExpression__FeatureAssignment_1_0_0_1_in_rule__XMultiplicativeExpression__Group_1_0_0__1__Impl54699 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XUnaryOperation__Group_0__0__Impl_in_rule__XUnaryOperation__Group_0__054733 = new BitSet(new long[]{0x0000010C00000000L});
public static final BitSet FOLLOW_rule__XUnaryOperation__Group_0__1_in_rule__XUnaryOperation__Group_0__054736 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XUnaryOperation__Group_0__1__Impl_in_rule__XUnaryOperation__Group_0__154794 = new BitSet(new long[]{0x0007E10C080001F0L,0x0000000000000008L,0x0200BFE298010000L});
public static final BitSet FOLLOW_rule__XUnaryOperation__Group_0__2_in_rule__XUnaryOperation__Group_0__154797 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XUnaryOperation__FeatureAssignment_0_1_in_rule__XUnaryOperation__Group_0__1__Impl54824 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XUnaryOperation__Group_0__2__Impl_in_rule__XUnaryOperation__Group_0__254854 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XUnaryOperation__OperandAssignment_0_2_in_rule__XUnaryOperation__Group_0__2__Impl54881 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XCastedExpression__Group__0__Impl_in_rule__XCastedExpression__Group__054917 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000000L,0x0000000002000000L});
public static final BitSet FOLLOW_rule__XCastedExpression__Group__1_in_rule__XCastedExpression__Group__054920 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXPostfixOperation_in_rule__XCastedExpression__Group__0__Impl54947 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XCastedExpression__Group__1__Impl_in_rule__XCastedExpression__Group__154976 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XCastedExpression__Group_1__0_in_rule__XCastedExpression__Group__1__Impl55003 = new BitSet(new long[]{0x0000000000000002L,0x0000000000000000L,0x0000000002000000L});
public static final BitSet FOLLOW_rule__XCastedExpression__Group_1__0__Impl_in_rule__XCastedExpression__Group_1__055038 = new BitSet(new long[]{0x0000000080000010L,0x0000000000000000L,0x0000000000010000L});
public static final BitSet FOLLOW_rule__XCastedExpression__Group_1__1_in_rule__XCastedExpression__Group_1__055041 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XCastedExpression__Group_1_0__0_in_rule__XCastedExpression__Group_1__0__Impl55068 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XCastedExpression__Group_1__1__Impl_in_rule__XCastedExpression__Group_1__155098 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XCastedExpression__TypeAssignment_1_1_in_rule__XCastedExpression__Group_1__1__Impl55125 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XCastedExpression__Group_1_0__0__Impl_in_rule__XCastedExpression__Group_1_0__055159 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XCastedExpression__Group_1_0_0__0_in_rule__XCastedExpression__Group_1_0__0__Impl55186 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XCastedExpression__Group_1_0_0__0__Impl_in_rule__XCastedExpression__Group_1_0_0__055218 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000000L,0x0000000002000000L});
public static final BitSet FOLLOW_rule__XCastedExpression__Group_1_0_0__1_in_rule__XCastedExpression__Group_1_0_0__055221 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XCastedExpression__Group_1_0_0__1__Impl_in_rule__XCastedExpression__Group_1_0_0__155279 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_153_in_rule__XCastedExpression__Group_1_0_0__1__Impl55307 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XPostfixOperation__Group__0__Impl_in_rule__XPostfixOperation__Group__055342 = new BitSet(new long[]{0x0000060000000000L});
public static final BitSet FOLLOW_rule__XPostfixOperation__Group__1_in_rule__XPostfixOperation__Group__055345 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXMemberFeatureCall_in_rule__XPostfixOperation__Group__0__Impl55372 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XPostfixOperation__Group__1__Impl_in_rule__XPostfixOperation__Group__155401 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XPostfixOperation__Group_1__0_in_rule__XPostfixOperation__Group__1__Impl55428 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XPostfixOperation__Group_1__0__Impl_in_rule__XPostfixOperation__Group_1__055463 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XPostfixOperation__Group_1_0__0_in_rule__XPostfixOperation__Group_1__0__Impl55490 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XPostfixOperation__Group_1_0__0__Impl_in_rule__XPostfixOperation__Group_1_0__055522 = new BitSet(new long[]{0x0000060000000000L});
public static final BitSet FOLLOW_rule__XPostfixOperation__Group_1_0__1_in_rule__XPostfixOperation__Group_1_0__055525 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XPostfixOperation__Group_1_0__1__Impl_in_rule__XPostfixOperation__Group_1_0__155583 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XPostfixOperation__FeatureAssignment_1_0_1_in_rule__XPostfixOperation__Group_1_0__1__Impl55610 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XMemberFeatureCall__Group__0__Impl_in_rule__XMemberFeatureCall__Group__055644 = new BitSet(new long[]{0x0000080000000000L,0x0000000000000000L,0x0060000000000000L});
public static final BitSet FOLLOW_rule__XMemberFeatureCall__Group__1_in_rule__XMemberFeatureCall__Group__055647 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXPrimaryExpression_in_rule__XMemberFeatureCall__Group__0__Impl55674 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XMemberFeatureCall__Group__1__Impl_in_rule__XMemberFeatureCall__Group__155703 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XMemberFeatureCall__Alternatives_1_in_rule__XMemberFeatureCall__Group__1__Impl55730 = new BitSet(new long[]{0x0000080000000002L,0x0000000000000000L,0x0060000000000000L});
public static final BitSet FOLLOW_rule__XMemberFeatureCall__Group_1_0__0__Impl_in_rule__XMemberFeatureCall__Group_1_0__055765 = new BitSet(new long[]{0x0007E10C080001F0L,0x0000000000000008L,0x0200BFE298010000L});
public static final BitSet FOLLOW_rule__XMemberFeatureCall__Group_1_0__1_in_rule__XMemberFeatureCall__Group_1_0__055768 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XMemberFeatureCall__Group_1_0_0__0_in_rule__XMemberFeatureCall__Group_1_0__0__Impl55795 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XMemberFeatureCall__Group_1_0__1__Impl_in_rule__XMemberFeatureCall__Group_1_0__155825 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XMemberFeatureCall__ValueAssignment_1_0_1_in_rule__XMemberFeatureCall__Group_1_0__1__Impl55852 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XMemberFeatureCall__Group_1_0_0__0__Impl_in_rule__XMemberFeatureCall__Group_1_0_0__055886 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XMemberFeatureCall__Group_1_0_0_0__0_in_rule__XMemberFeatureCall__Group_1_0_0__0__Impl55913 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XMemberFeatureCall__Group_1_0_0_0__0__Impl_in_rule__XMemberFeatureCall__Group_1_0_0_0__055945 = new BitSet(new long[]{0x0000080000000000L,0x0000000000000000L,0x0020000000000000L});
public static final BitSet FOLLOW_rule__XMemberFeatureCall__Group_1_0_0_0__1_in_rule__XMemberFeatureCall__Group_1_0_0_0__055948 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XMemberFeatureCall__Group_1_0_0_0__1__Impl_in_rule__XMemberFeatureCall__Group_1_0_0_0__156006 = new BitSet(new long[]{0x0001E00000000010L});
public static final BitSet FOLLOW_rule__XMemberFeatureCall__Group_1_0_0_0__2_in_rule__XMemberFeatureCall__Group_1_0_0_0__156009 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XMemberFeatureCall__Alternatives_1_0_0_0_1_in_rule__XMemberFeatureCall__Group_1_0_0_0__1__Impl56036 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XMemberFeatureCall__Group_1_0_0_0__2__Impl_in_rule__XMemberFeatureCall__Group_1_0_0_0__256066 = new BitSet(new long[]{0x0000000000002000L});
public static final BitSet FOLLOW_rule__XMemberFeatureCall__Group_1_0_0_0__3_in_rule__XMemberFeatureCall__Group_1_0_0_0__256069 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XMemberFeatureCall__FeatureAssignment_1_0_0_0_2_in_rule__XMemberFeatureCall__Group_1_0_0_0__2__Impl56096 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XMemberFeatureCall__Group_1_0_0_0__3__Impl_in_rule__XMemberFeatureCall__Group_1_0_0_0__356126 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleOpSingleAssign_in_rule__XMemberFeatureCall__Group_1_0_0_0__3__Impl56153 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XMemberFeatureCall__Group_1_1__0__Impl_in_rule__XMemberFeatureCall__Group_1_1__056190 = new BitSet(new long[]{0x0003E00008000010L});
public static final BitSet FOLLOW_rule__XMemberFeatureCall__Group_1_1__1_in_rule__XMemberFeatureCall__Group_1_1__056193 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XMemberFeatureCall__Group_1_1_0__0_in_rule__XMemberFeatureCall__Group_1_1__0__Impl56220 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XMemberFeatureCall__Group_1_1__1__Impl_in_rule__XMemberFeatureCall__Group_1_1__156250 = new BitSet(new long[]{0x0003E00008000010L});
public static final BitSet FOLLOW_rule__XMemberFeatureCall__Group_1_1__2_in_rule__XMemberFeatureCall__Group_1_1__156253 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XMemberFeatureCall__Group_1_1_1__0_in_rule__XMemberFeatureCall__Group_1_1__1__Impl56280 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XMemberFeatureCall__Group_1_1__2__Impl_in_rule__XMemberFeatureCall__Group_1_1__256311 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000000L,0x0000000010010000L});
public static final BitSet FOLLOW_rule__XMemberFeatureCall__Group_1_1__3_in_rule__XMemberFeatureCall__Group_1_1__256314 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XMemberFeatureCall__FeatureAssignment_1_1_2_in_rule__XMemberFeatureCall__Group_1_1__2__Impl56341 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XMemberFeatureCall__Group_1_1__3__Impl_in_rule__XMemberFeatureCall__Group_1_1__356371 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000000L,0x0000000010010000L});
public static final BitSet FOLLOW_rule__XMemberFeatureCall__Group_1_1__4_in_rule__XMemberFeatureCall__Group_1_1__356374 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XMemberFeatureCall__Group_1_1_3__0_in_rule__XMemberFeatureCall__Group_1_1__3__Impl56401 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XMemberFeatureCall__Group_1_1__4__Impl_in_rule__XMemberFeatureCall__Group_1_1__456432 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XMemberFeatureCall__MemberCallArgumentsAssignment_1_1_4_in_rule__XMemberFeatureCall__Group_1_1__4__Impl56459 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XMemberFeatureCall__Group_1_1_0__0__Impl_in_rule__XMemberFeatureCall__Group_1_1_0__056500 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XMemberFeatureCall__Group_1_1_0_0__0_in_rule__XMemberFeatureCall__Group_1_1_0__0__Impl56527 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XMemberFeatureCall__Group_1_1_0_0__0__Impl_in_rule__XMemberFeatureCall__Group_1_1_0_0__056559 = new BitSet(new long[]{0x0000080000000000L,0x0000000000000000L,0x0060000000000000L});
public static final BitSet FOLLOW_rule__XMemberFeatureCall__Group_1_1_0_0__1_in_rule__XMemberFeatureCall__Group_1_1_0_0__056562 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XMemberFeatureCall__Group_1_1_0_0__1__Impl_in_rule__XMemberFeatureCall__Group_1_1_0_0__156620 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XMemberFeatureCall__Alternatives_1_1_0_0_1_in_rule__XMemberFeatureCall__Group_1_1_0_0__1__Impl56647 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XMemberFeatureCall__Group_1_1_1__0__Impl_in_rule__XMemberFeatureCall__Group_1_1_1__056681 = new BitSet(new long[]{0x0000000080000010L,0x0000000000000000L,0x0002000000010000L});
public static final BitSet FOLLOW_rule__XMemberFeatureCall__Group_1_1_1__1_in_rule__XMemberFeatureCall__Group_1_1_1__056684 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_27_in_rule__XMemberFeatureCall__Group_1_1_1__0__Impl56712 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XMemberFeatureCall__Group_1_1_1__1__Impl_in_rule__XMemberFeatureCall__Group_1_1_1__156743 = new BitSet(new long[]{0x0000000004000000L,0x0000000000000000L,0x0000000004000000L});
public static final BitSet FOLLOW_rule__XMemberFeatureCall__Group_1_1_1__2_in_rule__XMemberFeatureCall__Group_1_1_1__156746 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XMemberFeatureCall__TypeArgumentsAssignment_1_1_1_1_in_rule__XMemberFeatureCall__Group_1_1_1__1__Impl56773 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XMemberFeatureCall__Group_1_1_1__2__Impl_in_rule__XMemberFeatureCall__Group_1_1_1__256803 = new BitSet(new long[]{0x0000000004000000L,0x0000000000000000L,0x0000000004000000L});
public static final BitSet FOLLOW_rule__XMemberFeatureCall__Group_1_1_1__3_in_rule__XMemberFeatureCall__Group_1_1_1__256806 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XMemberFeatureCall__Group_1_1_1_2__0_in_rule__XMemberFeatureCall__Group_1_1_1__2__Impl56833 = new BitSet(new long[]{0x0000000000000002L,0x0000000000000000L,0x0000000004000000L});
public static final BitSet FOLLOW_rule__XMemberFeatureCall__Group_1_1_1__3__Impl_in_rule__XMemberFeatureCall__Group_1_1_1__356864 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_26_in_rule__XMemberFeatureCall__Group_1_1_1__3__Impl56892 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XMemberFeatureCall__Group_1_1_1_2__0__Impl_in_rule__XMemberFeatureCall__Group_1_1_1_2__056931 = new BitSet(new long[]{0x0000000080000010L,0x0000000000000000L,0x0002000000010000L});
public static final BitSet FOLLOW_rule__XMemberFeatureCall__Group_1_1_1_2__1_in_rule__XMemberFeatureCall__Group_1_1_1_2__056934 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_154_in_rule__XMemberFeatureCall__Group_1_1_1_2__0__Impl56962 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XMemberFeatureCall__Group_1_1_1_2__1__Impl_in_rule__XMemberFeatureCall__Group_1_1_1_2__156993 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XMemberFeatureCall__TypeArgumentsAssignment_1_1_1_2_1_in_rule__XMemberFeatureCall__Group_1_1_1_2__1__Impl57020 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XMemberFeatureCall__Group_1_1_3__0__Impl_in_rule__XMemberFeatureCall__Group_1_1_3__057054 = new BitSet(new long[]{0x0007E10C880001F0L,0x0000000000000008L,0x0280BFE298030000L});
public static final BitSet FOLLOW_rule__XMemberFeatureCall__Group_1_1_3__1_in_rule__XMemberFeatureCall__Group_1_1_3__057057 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XMemberFeatureCall__ExplicitOperationCallAssignment_1_1_3_0_in_rule__XMemberFeatureCall__Group_1_1_3__0__Impl57084 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XMemberFeatureCall__Group_1_1_3__1__Impl_in_rule__XMemberFeatureCall__Group_1_1_3__157114 = new BitSet(new long[]{0x0007E10C880001F0L,0x0000000000000008L,0x0280BFE298030000L});
public static final BitSet FOLLOW_rule__XMemberFeatureCall__Group_1_1_3__2_in_rule__XMemberFeatureCall__Group_1_1_3__157117 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XMemberFeatureCall__Alternatives_1_1_3_1_in_rule__XMemberFeatureCall__Group_1_1_3__1__Impl57144 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XMemberFeatureCall__Group_1_1_3__2__Impl_in_rule__XMemberFeatureCall__Group_1_1_3__257175 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_145_in_rule__XMemberFeatureCall__Group_1_1_3__2__Impl57203 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XMemberFeatureCall__Group_1_1_3_1_1__0__Impl_in_rule__XMemberFeatureCall__Group_1_1_3_1_1__057240 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000000L,0x0000000004000000L});
public static final BitSet FOLLOW_rule__XMemberFeatureCall__Group_1_1_3_1_1__1_in_rule__XMemberFeatureCall__Group_1_1_3_1_1__057243 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XMemberFeatureCall__MemberCallArgumentsAssignment_1_1_3_1_1_0_in_rule__XMemberFeatureCall__Group_1_1_3_1_1__0__Impl57270 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XMemberFeatureCall__Group_1_1_3_1_1__1__Impl_in_rule__XMemberFeatureCall__Group_1_1_3_1_1__157300 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XMemberFeatureCall__Group_1_1_3_1_1_1__0_in_rule__XMemberFeatureCall__Group_1_1_3_1_1__1__Impl57327 = new BitSet(new long[]{0x0000000000000002L,0x0000000000000000L,0x0000000004000000L});
public static final BitSet FOLLOW_rule__XMemberFeatureCall__Group_1_1_3_1_1_1__0__Impl_in_rule__XMemberFeatureCall__Group_1_1_3_1_1_1__057362 = new BitSet(new long[]{0x0007E10C880001F0L,0x0000000000000008L,0x0280BFE298010000L});
public static final BitSet FOLLOW_rule__XMemberFeatureCall__Group_1_1_3_1_1_1__1_in_rule__XMemberFeatureCall__Group_1_1_3_1_1_1__057365 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_154_in_rule__XMemberFeatureCall__Group_1_1_3_1_1_1__0__Impl57393 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XMemberFeatureCall__Group_1_1_3_1_1_1__1__Impl_in_rule__XMemberFeatureCall__Group_1_1_3_1_1_1__157424 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XMemberFeatureCall__MemberCallArgumentsAssignment_1_1_3_1_1_1_1_in_rule__XMemberFeatureCall__Group_1_1_3_1_1_1__1__Impl57451 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XSetLiteral__Group__0__Impl_in_rule__XSetLiteral__Group__057485 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000000L,0x0000000008000000L});
public static final BitSet FOLLOW_rule__XSetLiteral__Group__1_in_rule__XSetLiteral__Group__057488 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XSetLiteral__Group__1__Impl_in_rule__XSetLiteral__Group__157546 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000008L});
public static final BitSet FOLLOW_rule__XSetLiteral__Group__2_in_rule__XSetLiteral__Group__157549 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_155_in_rule__XSetLiteral__Group__1__Impl57577 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XSetLiteral__Group__2__Impl_in_rule__XSetLiteral__Group__257608 = new BitSet(new long[]{0x0007E10C880001F0L,0x0000000000000018L,0x0280BFE298010000L});
public static final BitSet FOLLOW_rule__XSetLiteral__Group__3_in_rule__XSetLiteral__Group__257611 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_67_in_rule__XSetLiteral__Group__2__Impl57639 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XSetLiteral__Group__3__Impl_in_rule__XSetLiteral__Group__357670 = new BitSet(new long[]{0x0007E10C880001F0L,0x0000000000000018L,0x0280BFE298010000L});
public static final BitSet FOLLOW_rule__XSetLiteral__Group__4_in_rule__XSetLiteral__Group__357673 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XSetLiteral__Group_3__0_in_rule__XSetLiteral__Group__3__Impl57700 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XSetLiteral__Group__4__Impl_in_rule__XSetLiteral__Group__457731 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_68_in_rule__XSetLiteral__Group__4__Impl57759 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XSetLiteral__Group_3__0__Impl_in_rule__XSetLiteral__Group_3__057800 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000000L,0x0000000004000000L});
public static final BitSet FOLLOW_rule__XSetLiteral__Group_3__1_in_rule__XSetLiteral__Group_3__057803 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XSetLiteral__ElementsAssignment_3_0_in_rule__XSetLiteral__Group_3__0__Impl57830 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XSetLiteral__Group_3__1__Impl_in_rule__XSetLiteral__Group_3__157860 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XSetLiteral__Group_3_1__0_in_rule__XSetLiteral__Group_3__1__Impl57887 = new BitSet(new long[]{0x0000000000000002L,0x0000000000000000L,0x0000000004000000L});
public static final BitSet FOLLOW_rule__XSetLiteral__Group_3_1__0__Impl_in_rule__XSetLiteral__Group_3_1__057922 = new BitSet(new long[]{0x0007E10C880001F0L,0x0000000000000008L,0x0280BFE298010000L});
public static final BitSet FOLLOW_rule__XSetLiteral__Group_3_1__1_in_rule__XSetLiteral__Group_3_1__057925 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_154_in_rule__XSetLiteral__Group_3_1__0__Impl57953 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XSetLiteral__Group_3_1__1__Impl_in_rule__XSetLiteral__Group_3_1__157984 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XSetLiteral__ElementsAssignment_3_1_1_in_rule__XSetLiteral__Group_3_1__1__Impl58011 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XListLiteral__Group__0__Impl_in_rule__XListLiteral__Group__058045 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000000L,0x0000000008000000L});
public static final BitSet FOLLOW_rule__XListLiteral__Group__1_in_rule__XListLiteral__Group__058048 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XListLiteral__Group__1__Impl_in_rule__XListLiteral__Group__158106 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000000L,0x0000000010000000L});
public static final BitSet FOLLOW_rule__XListLiteral__Group__2_in_rule__XListLiteral__Group__158109 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_155_in_rule__XListLiteral__Group__1__Impl58137 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XListLiteral__Group__2__Impl_in_rule__XListLiteral__Group__258168 = new BitSet(new long[]{0x0007E10C880001F0L,0x0000000000000008L,0x0280BFE2B8010000L});
public static final BitSet FOLLOW_rule__XListLiteral__Group__3_in_rule__XListLiteral__Group__258171 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_156_in_rule__XListLiteral__Group__2__Impl58199 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XListLiteral__Group__3__Impl_in_rule__XListLiteral__Group__358230 = new BitSet(new long[]{0x0007E10C880001F0L,0x0000000000000008L,0x0280BFE2B8010000L});
public static final BitSet FOLLOW_rule__XListLiteral__Group__4_in_rule__XListLiteral__Group__358233 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XListLiteral__Group_3__0_in_rule__XListLiteral__Group__3__Impl58260 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XListLiteral__Group__4__Impl_in_rule__XListLiteral__Group__458291 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_157_in_rule__XListLiteral__Group__4__Impl58319 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XListLiteral__Group_3__0__Impl_in_rule__XListLiteral__Group_3__058360 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000000L,0x0000000004000000L});
public static final BitSet FOLLOW_rule__XListLiteral__Group_3__1_in_rule__XListLiteral__Group_3__058363 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XListLiteral__ElementsAssignment_3_0_in_rule__XListLiteral__Group_3__0__Impl58390 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XListLiteral__Group_3__1__Impl_in_rule__XListLiteral__Group_3__158420 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XListLiteral__Group_3_1__0_in_rule__XListLiteral__Group_3__1__Impl58447 = new BitSet(new long[]{0x0000000000000002L,0x0000000000000000L,0x0000000004000000L});
public static final BitSet FOLLOW_rule__XListLiteral__Group_3_1__0__Impl_in_rule__XListLiteral__Group_3_1__058482 = new BitSet(new long[]{0x0007E10C880001F0L,0x0000000000000008L,0x0280BFE298010000L});
public static final BitSet FOLLOW_rule__XListLiteral__Group_3_1__1_in_rule__XListLiteral__Group_3_1__058485 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_154_in_rule__XListLiteral__Group_3_1__0__Impl58513 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XListLiteral__Group_3_1__1__Impl_in_rule__XListLiteral__Group_3_1__158544 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XListLiteral__ElementsAssignment_3_1_1_in_rule__XListLiteral__Group_3_1__1__Impl58571 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XClosure__Group__0__Impl_in_rule__XClosure__Group__058605 = new BitSet(new long[]{0x0007F10C880001F0L,0x0000000000000008L,0x0380BFE298010000L});
public static final BitSet FOLLOW_rule__XClosure__Group__1_in_rule__XClosure__Group__058608 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XClosure__Group_0__0_in_rule__XClosure__Group__0__Impl58635 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XClosure__Group__1__Impl_in_rule__XClosure__Group__158665 = new BitSet(new long[]{0x0007F10C880001F0L,0x0000000000000008L,0x0380BFE298010000L});
public static final BitSet FOLLOW_rule__XClosure__Group__2_in_rule__XClosure__Group__158668 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XClosure__Group_1__0_in_rule__XClosure__Group__1__Impl58695 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XClosure__Group__2__Impl_in_rule__XClosure__Group__258726 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000000L,0x0000000020000000L});
public static final BitSet FOLLOW_rule__XClosure__Group__3_in_rule__XClosure__Group__258729 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XClosure__ExpressionAssignment_2_in_rule__XClosure__Group__2__Impl58756 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XClosure__Group__3__Impl_in_rule__XClosure__Group__358786 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_157_in_rule__XClosure__Group__3__Impl58814 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XClosure__Group_0__0__Impl_in_rule__XClosure__Group_0__058853 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XClosure__Group_0_0__0_in_rule__XClosure__Group_0__0__Impl58880 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XClosure__Group_0_0__0__Impl_in_rule__XClosure__Group_0_0__058912 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000000L,0x0000000010000000L});
public static final BitSet FOLLOW_rule__XClosure__Group_0_0__1_in_rule__XClosure__Group_0_0__058915 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XClosure__Group_0_0__1__Impl_in_rule__XClosure__Group_0_0__158973 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_156_in_rule__XClosure__Group_0_0__1__Impl59001 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XClosure__Group_1__0__Impl_in_rule__XClosure__Group_1__059036 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XClosure__Group_1_0__0_in_rule__XClosure__Group_1__0__Impl59063 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XClosure__Group_1_0__0__Impl_in_rule__XClosure__Group_1_0__059095 = new BitSet(new long[]{0x0000000080000010L,0x0000000000000000L,0x0080000000010000L});
public static final BitSet FOLLOW_rule__XClosure__Group_1_0__1_in_rule__XClosure__Group_1_0__059098 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XClosure__Group_1_0_0__0_in_rule__XClosure__Group_1_0__0__Impl59125 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XClosure__Group_1_0__1__Impl_in_rule__XClosure__Group_1_0__159156 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XClosure__ExplicitSyntaxAssignment_1_0_1_in_rule__XClosure__Group_1_0__1__Impl59183 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XClosure__Group_1_0_0__0__Impl_in_rule__XClosure__Group_1_0_0__059217 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000000L,0x0000000004000000L});
public static final BitSet FOLLOW_rule__XClosure__Group_1_0_0__1_in_rule__XClosure__Group_1_0_0__059220 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XClosure__DeclaredFormalParametersAssignment_1_0_0_0_in_rule__XClosure__Group_1_0_0__0__Impl59247 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XClosure__Group_1_0_0__1__Impl_in_rule__XClosure__Group_1_0_0__159277 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XClosure__Group_1_0_0_1__0_in_rule__XClosure__Group_1_0_0__1__Impl59304 = new BitSet(new long[]{0x0000000000000002L,0x0000000000000000L,0x0000000004000000L});
public static final BitSet FOLLOW_rule__XClosure__Group_1_0_0_1__0__Impl_in_rule__XClosure__Group_1_0_0_1__059339 = new BitSet(new long[]{0x0000000080000010L,0x0000000000000000L,0x0000000000010000L});
public static final BitSet FOLLOW_rule__XClosure__Group_1_0_0_1__1_in_rule__XClosure__Group_1_0_0_1__059342 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_154_in_rule__XClosure__Group_1_0_0_1__0__Impl59370 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XClosure__Group_1_0_0_1__1__Impl_in_rule__XClosure__Group_1_0_0_1__159401 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XClosure__DeclaredFormalParametersAssignment_1_0_0_1_1_in_rule__XClosure__Group_1_0_0_1__1__Impl59428 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XExpressionInClosure__Group__0__Impl_in_rule__XExpressionInClosure__Group__059462 = new BitSet(new long[]{0x0007F10C880001F0L,0x0000000000000008L,0x0380BFE298010000L});
public static final BitSet FOLLOW_rule__XExpressionInClosure__Group__1_in_rule__XExpressionInClosure__Group__059465 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XExpressionInClosure__Group__1__Impl_in_rule__XExpressionInClosure__Group__159523 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XExpressionInClosure__Group_1__0_in_rule__XExpressionInClosure__Group__1__Impl59550 = new BitSet(new long[]{0x0007F10C880001F2L,0x0000000000000008L,0x0380BFE298010000L});
public static final BitSet FOLLOW_rule__XExpressionInClosure__Group_1__0__Impl_in_rule__XExpressionInClosure__Group_1__059585 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000000L,0x0000000040000000L});
public static final BitSet FOLLOW_rule__XExpressionInClosure__Group_1__1_in_rule__XExpressionInClosure__Group_1__059588 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XExpressionInClosure__ExpressionsAssignment_1_0_in_rule__XExpressionInClosure__Group_1__0__Impl59615 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XExpressionInClosure__Group_1__1__Impl_in_rule__XExpressionInClosure__Group_1__159645 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_158_in_rule__XExpressionInClosure__Group_1__1__Impl59674 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XShortClosure__Group__0__Impl_in_rule__XShortClosure__Group__059711 = new BitSet(new long[]{0x0007E10C880001F0L,0x0000000000000008L,0x0280BFE298010000L});
public static final BitSet FOLLOW_rule__XShortClosure__Group__1_in_rule__XShortClosure__Group__059714 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XShortClosure__Group_0__0_in_rule__XShortClosure__Group__0__Impl59741 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XShortClosure__Group__1__Impl_in_rule__XShortClosure__Group__159771 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XShortClosure__ExpressionAssignment_1_in_rule__XShortClosure__Group__1__Impl59798 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XShortClosure__Group_0__0__Impl_in_rule__XShortClosure__Group_0__059832 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XShortClosure__Group_0_0__0_in_rule__XShortClosure__Group_0__0__Impl59859 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XShortClosure__Group_0_0__0__Impl_in_rule__XShortClosure__Group_0_0__059891 = new BitSet(new long[]{0x0000000080000010L,0x0000000000000000L,0x0080000000010000L});
public static final BitSet FOLLOW_rule__XShortClosure__Group_0_0__1_in_rule__XShortClosure__Group_0_0__059894 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XShortClosure__Group_0_0__1__Impl_in_rule__XShortClosure__Group_0_0__159952 = new BitSet(new long[]{0x0000000080000010L,0x0000000000000000L,0x0080000000010000L});
public static final BitSet FOLLOW_rule__XShortClosure__Group_0_0__2_in_rule__XShortClosure__Group_0_0__159955 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XShortClosure__Group_0_0_1__0_in_rule__XShortClosure__Group_0_0__1__Impl59982 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XShortClosure__Group_0_0__2__Impl_in_rule__XShortClosure__Group_0_0__260013 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XShortClosure__ExplicitSyntaxAssignment_0_0_2_in_rule__XShortClosure__Group_0_0__2__Impl60040 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XShortClosure__Group_0_0_1__0__Impl_in_rule__XShortClosure__Group_0_0_1__060076 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000000L,0x0000000004000000L});
public static final BitSet FOLLOW_rule__XShortClosure__Group_0_0_1__1_in_rule__XShortClosure__Group_0_0_1__060079 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XShortClosure__DeclaredFormalParametersAssignment_0_0_1_0_in_rule__XShortClosure__Group_0_0_1__0__Impl60106 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XShortClosure__Group_0_0_1__1__Impl_in_rule__XShortClosure__Group_0_0_1__160136 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XShortClosure__Group_0_0_1_1__0_in_rule__XShortClosure__Group_0_0_1__1__Impl60163 = new BitSet(new long[]{0x0000000000000002L,0x0000000000000000L,0x0000000004000000L});
public static final BitSet FOLLOW_rule__XShortClosure__Group_0_0_1_1__0__Impl_in_rule__XShortClosure__Group_0_0_1_1__060198 = new BitSet(new long[]{0x0000000080000010L,0x0000000000000000L,0x0000000000010000L});
public static final BitSet FOLLOW_rule__XShortClosure__Group_0_0_1_1__1_in_rule__XShortClosure__Group_0_0_1_1__060201 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_154_in_rule__XShortClosure__Group_0_0_1_1__0__Impl60229 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XShortClosure__Group_0_0_1_1__1__Impl_in_rule__XShortClosure__Group_0_0_1_1__160260 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XShortClosure__DeclaredFormalParametersAssignment_0_0_1_1_1_in_rule__XShortClosure__Group_0_0_1_1__1__Impl60287 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XParenthesizedExpression__Group__0__Impl_in_rule__XParenthesizedExpression__Group__060321 = new BitSet(new long[]{0x0007E10C880001F0L,0x0000000000000008L,0x0280BFE298010000L});
public static final BitSet FOLLOW_rule__XParenthesizedExpression__Group__1_in_rule__XParenthesizedExpression__Group__060324 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_144_in_rule__XParenthesizedExpression__Group__0__Impl60352 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XParenthesizedExpression__Group__1__Impl_in_rule__XParenthesizedExpression__Group__160383 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000000L,0x0000000000020000L});
public static final BitSet FOLLOW_rule__XParenthesizedExpression__Group__2_in_rule__XParenthesizedExpression__Group__160386 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXExpression_in_rule__XParenthesizedExpression__Group__1__Impl60413 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XParenthesizedExpression__Group__2__Impl_in_rule__XParenthesizedExpression__Group__260442 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_145_in_rule__XParenthesizedExpression__Group__2__Impl60470 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XIfExpression__Group__0__Impl_in_rule__XIfExpression__Group__060507 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000000L,0x0000000080000000L});
public static final BitSet FOLLOW_rule__XIfExpression__Group__1_in_rule__XIfExpression__Group__060510 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XIfExpression__Group__1__Impl_in_rule__XIfExpression__Group__160568 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000000L,0x0000000000010000L});
public static final BitSet FOLLOW_rule__XIfExpression__Group__2_in_rule__XIfExpression__Group__160571 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_159_in_rule__XIfExpression__Group__1__Impl60599 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XIfExpression__Group__2__Impl_in_rule__XIfExpression__Group__260630 = new BitSet(new long[]{0x0007E10C880001F0L,0x0000000000000008L,0x0280BFE298010000L});
public static final BitSet FOLLOW_rule__XIfExpression__Group__3_in_rule__XIfExpression__Group__260633 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_144_in_rule__XIfExpression__Group__2__Impl60661 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XIfExpression__Group__3__Impl_in_rule__XIfExpression__Group__360692 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000000L,0x0000000000020000L});
public static final BitSet FOLLOW_rule__XIfExpression__Group__4_in_rule__XIfExpression__Group__360695 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XIfExpression__IfAssignment_3_in_rule__XIfExpression__Group__3__Impl60722 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XIfExpression__Group__4__Impl_in_rule__XIfExpression__Group__460752 = new BitSet(new long[]{0x0007E10C880001F0L,0x0000000000000008L,0x0280BFE298010000L});
public static final BitSet FOLLOW_rule__XIfExpression__Group__5_in_rule__XIfExpression__Group__460755 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_145_in_rule__XIfExpression__Group__4__Impl60783 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XIfExpression__Group__5__Impl_in_rule__XIfExpression__Group__560814 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000000L,0x0000000100000000L});
public static final BitSet FOLLOW_rule__XIfExpression__Group__6_in_rule__XIfExpression__Group__560817 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XIfExpression__ThenAssignment_5_in_rule__XIfExpression__Group__5__Impl60844 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XIfExpression__Group__6__Impl_in_rule__XIfExpression__Group__660874 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XIfExpression__Group_6__0_in_rule__XIfExpression__Group__6__Impl60901 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XIfExpression__Group_6__0__Impl_in_rule__XIfExpression__Group_6__060946 = new BitSet(new long[]{0x0007E10C880001F0L,0x0000000000000008L,0x0280BFE298010000L});
public static final BitSet FOLLOW_rule__XIfExpression__Group_6__1_in_rule__XIfExpression__Group_6__060949 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_160_in_rule__XIfExpression__Group_6__0__Impl60978 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XIfExpression__Group_6__1__Impl_in_rule__XIfExpression__Group_6__161010 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XIfExpression__ElseAssignment_6_1_in_rule__XIfExpression__Group_6__1__Impl61037 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XSwitchExpression__Group__0__Impl_in_rule__XSwitchExpression__Group__061071 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000000L,0x0000000200000000L});
public static final BitSet FOLLOW_rule__XSwitchExpression__Group__1_in_rule__XSwitchExpression__Group__061074 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XSwitchExpression__Group__1__Impl_in_rule__XSwitchExpression__Group__161132 = new BitSet(new long[]{0x0007E10C880001F0L,0x0000000000000008L,0x0280BFE298010000L});
public static final BitSet FOLLOW_rule__XSwitchExpression__Group__2_in_rule__XSwitchExpression__Group__161135 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_161_in_rule__XSwitchExpression__Group__1__Impl61163 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XSwitchExpression__Group__2__Impl_in_rule__XSwitchExpression__Group__261194 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000008L});
public static final BitSet FOLLOW_rule__XSwitchExpression__Group__3_in_rule__XSwitchExpression__Group__261197 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XSwitchExpression__Alternatives_2_in_rule__XSwitchExpression__Group__2__Impl61224 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XSwitchExpression__Group__3__Impl_in_rule__XSwitchExpression__Group__361254 = new BitSet(new long[]{0x0000000080000010L,0x0000000000000010L,0x0000001C04010000L});
public static final BitSet FOLLOW_rule__XSwitchExpression__Group__4_in_rule__XSwitchExpression__Group__361257 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_67_in_rule__XSwitchExpression__Group__3__Impl61285 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XSwitchExpression__Group__4__Impl_in_rule__XSwitchExpression__Group__461316 = new BitSet(new long[]{0x0000000080000010L,0x0000000000000010L,0x0000001C04010000L});
public static final BitSet FOLLOW_rule__XSwitchExpression__Group__5_in_rule__XSwitchExpression__Group__461319 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XSwitchExpression__CasesAssignment_4_in_rule__XSwitchExpression__Group__4__Impl61346 = new BitSet(new long[]{0x0000000080000012L,0x0000000000000000L,0x0000001404010000L});
public static final BitSet FOLLOW_rule__XSwitchExpression__Group__5__Impl_in_rule__XSwitchExpression__Group__561377 = new BitSet(new long[]{0x0000000080000010L,0x0000000000000010L,0x0000001C04010000L});
public static final BitSet FOLLOW_rule__XSwitchExpression__Group__6_in_rule__XSwitchExpression__Group__561380 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XSwitchExpression__Group_5__0_in_rule__XSwitchExpression__Group__5__Impl61407 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XSwitchExpression__Group__6__Impl_in_rule__XSwitchExpression__Group__661438 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_68_in_rule__XSwitchExpression__Group__6__Impl61466 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XSwitchExpression__Group_2_0__0__Impl_in_rule__XSwitchExpression__Group_2_0__061511 = new BitSet(new long[]{0x0007E10C880001F0L,0x0000000000000008L,0x0280BFE298010000L});
public static final BitSet FOLLOW_rule__XSwitchExpression__Group_2_0__1_in_rule__XSwitchExpression__Group_2_0__061514 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XSwitchExpression__Group_2_0_0__0_in_rule__XSwitchExpression__Group_2_0__0__Impl61541 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XSwitchExpression__Group_2_0__1__Impl_in_rule__XSwitchExpression__Group_2_0__161571 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000000L,0x0000000000020000L});
public static final BitSet FOLLOW_rule__XSwitchExpression__Group_2_0__2_in_rule__XSwitchExpression__Group_2_0__161574 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XSwitchExpression__SwitchAssignment_2_0_1_in_rule__XSwitchExpression__Group_2_0__1__Impl61601 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XSwitchExpression__Group_2_0__2__Impl_in_rule__XSwitchExpression__Group_2_0__261631 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_145_in_rule__XSwitchExpression__Group_2_0__2__Impl61659 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XSwitchExpression__Group_2_0_0__0__Impl_in_rule__XSwitchExpression__Group_2_0_0__061696 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XSwitchExpression__Group_2_0_0_0__0_in_rule__XSwitchExpression__Group_2_0_0__0__Impl61723 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XSwitchExpression__Group_2_0_0_0__0__Impl_in_rule__XSwitchExpression__Group_2_0_0_0__061755 = new BitSet(new long[]{0x0000000080000010L,0x0000000000000000L,0x0000000000010000L});
public static final BitSet FOLLOW_rule__XSwitchExpression__Group_2_0_0_0__1_in_rule__XSwitchExpression__Group_2_0_0_0__061758 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_144_in_rule__XSwitchExpression__Group_2_0_0_0__0__Impl61786 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XSwitchExpression__Group_2_0_0_0__1__Impl_in_rule__XSwitchExpression__Group_2_0_0_0__161817 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000000L,0x0000000400000000L});
public static final BitSet FOLLOW_rule__XSwitchExpression__Group_2_0_0_0__2_in_rule__XSwitchExpression__Group_2_0_0_0__161820 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XSwitchExpression__DeclaredParamAssignment_2_0_0_0_1_in_rule__XSwitchExpression__Group_2_0_0_0__1__Impl61847 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XSwitchExpression__Group_2_0_0_0__2__Impl_in_rule__XSwitchExpression__Group_2_0_0_0__261877 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_162_in_rule__XSwitchExpression__Group_2_0_0_0__2__Impl61905 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XSwitchExpression__Group_2_1__0__Impl_in_rule__XSwitchExpression__Group_2_1__061942 = new BitSet(new long[]{0x0007E10C880001F0L,0x0000000000000008L,0x0280BFE298010000L});
public static final BitSet FOLLOW_rule__XSwitchExpression__Group_2_1__1_in_rule__XSwitchExpression__Group_2_1__061945 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XSwitchExpression__Group_2_1_0__0_in_rule__XSwitchExpression__Group_2_1__0__Impl61972 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XSwitchExpression__Group_2_1__1__Impl_in_rule__XSwitchExpression__Group_2_1__162003 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XSwitchExpression__SwitchAssignment_2_1_1_in_rule__XSwitchExpression__Group_2_1__1__Impl62030 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XSwitchExpression__Group_2_1_0__0__Impl_in_rule__XSwitchExpression__Group_2_1_0__062064 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XSwitchExpression__Group_2_1_0_0__0_in_rule__XSwitchExpression__Group_2_1_0__0__Impl62091 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XSwitchExpression__Group_2_1_0_0__0__Impl_in_rule__XSwitchExpression__Group_2_1_0_0__062123 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000000L,0x0000000400000000L});
public static final BitSet FOLLOW_rule__XSwitchExpression__Group_2_1_0_0__1_in_rule__XSwitchExpression__Group_2_1_0_0__062126 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XSwitchExpression__DeclaredParamAssignment_2_1_0_0_0_in_rule__XSwitchExpression__Group_2_1_0_0__0__Impl62153 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XSwitchExpression__Group_2_1_0_0__1__Impl_in_rule__XSwitchExpression__Group_2_1_0_0__162183 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_162_in_rule__XSwitchExpression__Group_2_1_0_0__1__Impl62211 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XSwitchExpression__Group_5__0__Impl_in_rule__XSwitchExpression__Group_5__062246 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000000L,0x0000000400000000L});
public static final BitSet FOLLOW_rule__XSwitchExpression__Group_5__1_in_rule__XSwitchExpression__Group_5__062249 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_163_in_rule__XSwitchExpression__Group_5__0__Impl62277 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XSwitchExpression__Group_5__1__Impl_in_rule__XSwitchExpression__Group_5__162308 = new BitSet(new long[]{0x0007E10C880001F0L,0x0000000000000008L,0x0280BFE298010000L});
public static final BitSet FOLLOW_rule__XSwitchExpression__Group_5__2_in_rule__XSwitchExpression__Group_5__162311 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_162_in_rule__XSwitchExpression__Group_5__1__Impl62339 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XSwitchExpression__Group_5__2__Impl_in_rule__XSwitchExpression__Group_5__262370 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XSwitchExpression__DefaultAssignment_5_2_in_rule__XSwitchExpression__Group_5__2__Impl62397 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XCasePart__Group__0__Impl_in_rule__XCasePart__Group__062433 = new BitSet(new long[]{0x0000000080000010L,0x0000000000000000L,0x0000001404010000L});
public static final BitSet FOLLOW_rule__XCasePart__Group__1_in_rule__XCasePart__Group__062436 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XCasePart__Group__1__Impl_in_rule__XCasePart__Group__162494 = new BitSet(new long[]{0x0000000080000010L,0x0000000000000000L,0x0000001404010000L});
public static final BitSet FOLLOW_rule__XCasePart__Group__2_in_rule__XCasePart__Group__162497 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XCasePart__TypeGuardAssignment_1_in_rule__XCasePart__Group__1__Impl62524 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XCasePart__Group__2__Impl_in_rule__XCasePart__Group__262555 = new BitSet(new long[]{0x0000000080000010L,0x0000000000000000L,0x0000001404010000L});
public static final BitSet FOLLOW_rule__XCasePart__Group__3_in_rule__XCasePart__Group__262558 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XCasePart__Group_2__0_in_rule__XCasePart__Group__2__Impl62585 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XCasePart__Group__3__Impl_in_rule__XCasePart__Group__362616 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XCasePart__Alternatives_3_in_rule__XCasePart__Group__3__Impl62643 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XCasePart__Group_2__0__Impl_in_rule__XCasePart__Group_2__062681 = new BitSet(new long[]{0x0007E10C880001F0L,0x0000000000000008L,0x0280BFE298010000L});
public static final BitSet FOLLOW_rule__XCasePart__Group_2__1_in_rule__XCasePart__Group_2__062684 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_164_in_rule__XCasePart__Group_2__0__Impl62712 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XCasePart__Group_2__1__Impl_in_rule__XCasePart__Group_2__162743 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XCasePart__CaseAssignment_2_1_in_rule__XCasePart__Group_2__1__Impl62770 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XCasePart__Group_3_0__0__Impl_in_rule__XCasePart__Group_3_0__062804 = new BitSet(new long[]{0x0007E10C880001F0L,0x0000000000000008L,0x0280BFE298010000L});
public static final BitSet FOLLOW_rule__XCasePart__Group_3_0__1_in_rule__XCasePart__Group_3_0__062807 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_162_in_rule__XCasePart__Group_3_0__0__Impl62835 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XCasePart__Group_3_0__1__Impl_in_rule__XCasePart__Group_3_0__162866 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XCasePart__ThenAssignment_3_0_1_in_rule__XCasePart__Group_3_0__1__Impl62893 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XForLoopExpression__Group__0__Impl_in_rule__XForLoopExpression__Group__062927 = new BitSet(new long[]{0x0007E10C880001F0L,0x0000000000000008L,0x0280BFE298010000L});
public static final BitSet FOLLOW_rule__XForLoopExpression__Group__1_in_rule__XForLoopExpression__Group__062930 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XForLoopExpression__Group_0__0_in_rule__XForLoopExpression__Group__0__Impl62957 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XForLoopExpression__Group__1__Impl_in_rule__XForLoopExpression__Group__162987 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000000L,0x0000000000020000L});
public static final BitSet FOLLOW_rule__XForLoopExpression__Group__2_in_rule__XForLoopExpression__Group__162990 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XForLoopExpression__ForExpressionAssignment_1_in_rule__XForLoopExpression__Group__1__Impl63017 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XForLoopExpression__Group__2__Impl_in_rule__XForLoopExpression__Group__263047 = new BitSet(new long[]{0x0007E10C880001F0L,0x0000000000000008L,0x0280BFE298010000L});
public static final BitSet FOLLOW_rule__XForLoopExpression__Group__3_in_rule__XForLoopExpression__Group__263050 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_145_in_rule__XForLoopExpression__Group__2__Impl63078 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XForLoopExpression__Group__3__Impl_in_rule__XForLoopExpression__Group__363109 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XForLoopExpression__EachExpressionAssignment_3_in_rule__XForLoopExpression__Group__3__Impl63136 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XForLoopExpression__Group_0__0__Impl_in_rule__XForLoopExpression__Group_0__063174 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XForLoopExpression__Group_0_0__0_in_rule__XForLoopExpression__Group_0__0__Impl63201 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XForLoopExpression__Group_0_0__0__Impl_in_rule__XForLoopExpression__Group_0_0__063233 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000000L,0x0000002000000000L});
public static final BitSet FOLLOW_rule__XForLoopExpression__Group_0_0__1_in_rule__XForLoopExpression__Group_0_0__063236 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XForLoopExpression__Group_0_0__1__Impl_in_rule__XForLoopExpression__Group_0_0__163294 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000000L,0x0000000000010000L});
public static final BitSet FOLLOW_rule__XForLoopExpression__Group_0_0__2_in_rule__XForLoopExpression__Group_0_0__163297 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_165_in_rule__XForLoopExpression__Group_0_0__1__Impl63325 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XForLoopExpression__Group_0_0__2__Impl_in_rule__XForLoopExpression__Group_0_0__263356 = new BitSet(new long[]{0x0000000080000010L,0x0000000000000000L,0x0000000000010000L});
public static final BitSet FOLLOW_rule__XForLoopExpression__Group_0_0__3_in_rule__XForLoopExpression__Group_0_0__263359 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_144_in_rule__XForLoopExpression__Group_0_0__2__Impl63387 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XForLoopExpression__Group_0_0__3__Impl_in_rule__XForLoopExpression__Group_0_0__363418 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000000L,0x0000000400000000L});
public static final BitSet FOLLOW_rule__XForLoopExpression__Group_0_0__4_in_rule__XForLoopExpression__Group_0_0__363421 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XForLoopExpression__DeclaredParamAssignment_0_0_3_in_rule__XForLoopExpression__Group_0_0__3__Impl63448 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XForLoopExpression__Group_0_0__4__Impl_in_rule__XForLoopExpression__Group_0_0__463478 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_162_in_rule__XForLoopExpression__Group_0_0__4__Impl63506 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XBasicForLoopExpression__Group__0__Impl_in_rule__XBasicForLoopExpression__Group__063547 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000000L,0x0000002000000000L});
public static final BitSet FOLLOW_rule__XBasicForLoopExpression__Group__1_in_rule__XBasicForLoopExpression__Group__063550 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XBasicForLoopExpression__Group__1__Impl_in_rule__XBasicForLoopExpression__Group__163608 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000000L,0x0000000000010000L});
public static final BitSet FOLLOW_rule__XBasicForLoopExpression__Group__2_in_rule__XBasicForLoopExpression__Group__163611 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_165_in_rule__XBasicForLoopExpression__Group__1__Impl63639 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XBasicForLoopExpression__Group__2__Impl_in_rule__XBasicForLoopExpression__Group__263670 = new BitSet(new long[]{0x0007F10C880001F0L,0x0000000000000008L,0x0380BFE2D8010000L});
public static final BitSet FOLLOW_rule__XBasicForLoopExpression__Group__3_in_rule__XBasicForLoopExpression__Group__263673 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_144_in_rule__XBasicForLoopExpression__Group__2__Impl63701 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XBasicForLoopExpression__Group__3__Impl_in_rule__XBasicForLoopExpression__Group__363732 = new BitSet(new long[]{0x0007F10C880001F0L,0x0000000000000008L,0x0380BFE2D8010000L});
public static final BitSet FOLLOW_rule__XBasicForLoopExpression__Group__4_in_rule__XBasicForLoopExpression__Group__363735 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XBasicForLoopExpression__Group_3__0_in_rule__XBasicForLoopExpression__Group__3__Impl63762 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XBasicForLoopExpression__Group__4__Impl_in_rule__XBasicForLoopExpression__Group__463793 = new BitSet(new long[]{0x0007E10C880001F0L,0x0000000000000008L,0x0280BFE2D8010000L});
public static final BitSet FOLLOW_rule__XBasicForLoopExpression__Group__5_in_rule__XBasicForLoopExpression__Group__463796 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_158_in_rule__XBasicForLoopExpression__Group__4__Impl63824 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XBasicForLoopExpression__Group__5__Impl_in_rule__XBasicForLoopExpression__Group__563855 = new BitSet(new long[]{0x0007E10C880001F0L,0x0000000000000008L,0x0280BFE2D8010000L});
public static final BitSet FOLLOW_rule__XBasicForLoopExpression__Group__6_in_rule__XBasicForLoopExpression__Group__563858 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XBasicForLoopExpression__ExpressionAssignment_5_in_rule__XBasicForLoopExpression__Group__5__Impl63885 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XBasicForLoopExpression__Group__6__Impl_in_rule__XBasicForLoopExpression__Group__663916 = new BitSet(new long[]{0x0007E10C880001F0L,0x0000000000000008L,0x0280BFE298030000L});
public static final BitSet FOLLOW_rule__XBasicForLoopExpression__Group__7_in_rule__XBasicForLoopExpression__Group__663919 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_158_in_rule__XBasicForLoopExpression__Group__6__Impl63947 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XBasicForLoopExpression__Group__7__Impl_in_rule__XBasicForLoopExpression__Group__763978 = new BitSet(new long[]{0x0007E10C880001F0L,0x0000000000000008L,0x0280BFE298030000L});
public static final BitSet FOLLOW_rule__XBasicForLoopExpression__Group__8_in_rule__XBasicForLoopExpression__Group__763981 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XBasicForLoopExpression__Group_7__0_in_rule__XBasicForLoopExpression__Group__7__Impl64008 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XBasicForLoopExpression__Group__8__Impl_in_rule__XBasicForLoopExpression__Group__864039 = new BitSet(new long[]{0x0007E10C880001F0L,0x0000000000000008L,0x0280BFE298010000L});
public static final BitSet FOLLOW_rule__XBasicForLoopExpression__Group__9_in_rule__XBasicForLoopExpression__Group__864042 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_145_in_rule__XBasicForLoopExpression__Group__8__Impl64070 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XBasicForLoopExpression__Group__9__Impl_in_rule__XBasicForLoopExpression__Group__964101 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XBasicForLoopExpression__EachExpressionAssignment_9_in_rule__XBasicForLoopExpression__Group__9__Impl64128 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XBasicForLoopExpression__Group_3__0__Impl_in_rule__XBasicForLoopExpression__Group_3__064178 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000000L,0x0000000004000000L});
public static final BitSet FOLLOW_rule__XBasicForLoopExpression__Group_3__1_in_rule__XBasicForLoopExpression__Group_3__064181 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XBasicForLoopExpression__InitExpressionsAssignment_3_0_in_rule__XBasicForLoopExpression__Group_3__0__Impl64208 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XBasicForLoopExpression__Group_3__1__Impl_in_rule__XBasicForLoopExpression__Group_3__164238 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XBasicForLoopExpression__Group_3_1__0_in_rule__XBasicForLoopExpression__Group_3__1__Impl64265 = new BitSet(new long[]{0x0000000000000002L,0x0000000000000000L,0x0000000004000000L});
public static final BitSet FOLLOW_rule__XBasicForLoopExpression__Group_3_1__0__Impl_in_rule__XBasicForLoopExpression__Group_3_1__064300 = new BitSet(new long[]{0x0007F10C880001F0L,0x0000000000000008L,0x0380BFE298010000L});
public static final BitSet FOLLOW_rule__XBasicForLoopExpression__Group_3_1__1_in_rule__XBasicForLoopExpression__Group_3_1__064303 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_154_in_rule__XBasicForLoopExpression__Group_3_1__0__Impl64331 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XBasicForLoopExpression__Group_3_1__1__Impl_in_rule__XBasicForLoopExpression__Group_3_1__164362 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XBasicForLoopExpression__InitExpressionsAssignment_3_1_1_in_rule__XBasicForLoopExpression__Group_3_1__1__Impl64389 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XBasicForLoopExpression__Group_7__0__Impl_in_rule__XBasicForLoopExpression__Group_7__064423 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000000L,0x0000000004000000L});
public static final BitSet FOLLOW_rule__XBasicForLoopExpression__Group_7__1_in_rule__XBasicForLoopExpression__Group_7__064426 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XBasicForLoopExpression__UpdateExpressionsAssignment_7_0_in_rule__XBasicForLoopExpression__Group_7__0__Impl64453 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XBasicForLoopExpression__Group_7__1__Impl_in_rule__XBasicForLoopExpression__Group_7__164483 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XBasicForLoopExpression__Group_7_1__0_in_rule__XBasicForLoopExpression__Group_7__1__Impl64510 = new BitSet(new long[]{0x0000000000000002L,0x0000000000000000L,0x0000000004000000L});
public static final BitSet FOLLOW_rule__XBasicForLoopExpression__Group_7_1__0__Impl_in_rule__XBasicForLoopExpression__Group_7_1__064545 = new BitSet(new long[]{0x0007E10C880001F0L,0x0000000000000008L,0x0280BFE298010000L});
public static final BitSet FOLLOW_rule__XBasicForLoopExpression__Group_7_1__1_in_rule__XBasicForLoopExpression__Group_7_1__064548 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_154_in_rule__XBasicForLoopExpression__Group_7_1__0__Impl64576 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XBasicForLoopExpression__Group_7_1__1__Impl_in_rule__XBasicForLoopExpression__Group_7_1__164607 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XBasicForLoopExpression__UpdateExpressionsAssignment_7_1_1_in_rule__XBasicForLoopExpression__Group_7_1__1__Impl64634 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XWhileExpression__Group__0__Impl_in_rule__XWhileExpression__Group__064668 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000000L,0x0000004000000000L});
public static final BitSet FOLLOW_rule__XWhileExpression__Group__1_in_rule__XWhileExpression__Group__064671 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XWhileExpression__Group__1__Impl_in_rule__XWhileExpression__Group__164729 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000000L,0x0000000000010000L});
public static final BitSet FOLLOW_rule__XWhileExpression__Group__2_in_rule__XWhileExpression__Group__164732 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_166_in_rule__XWhileExpression__Group__1__Impl64760 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XWhileExpression__Group__2__Impl_in_rule__XWhileExpression__Group__264791 = new BitSet(new long[]{0x0007E10C880001F0L,0x0000000000000008L,0x0280BFE298010000L});
public static final BitSet FOLLOW_rule__XWhileExpression__Group__3_in_rule__XWhileExpression__Group__264794 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_144_in_rule__XWhileExpression__Group__2__Impl64822 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XWhileExpression__Group__3__Impl_in_rule__XWhileExpression__Group__364853 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000000L,0x0000000000020000L});
public static final BitSet FOLLOW_rule__XWhileExpression__Group__4_in_rule__XWhileExpression__Group__364856 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XWhileExpression__PredicateAssignment_3_in_rule__XWhileExpression__Group__3__Impl64883 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XWhileExpression__Group__4__Impl_in_rule__XWhileExpression__Group__464913 = new BitSet(new long[]{0x0007E10C880001F0L,0x0000000000000008L,0x0280BFE298010000L});
public static final BitSet FOLLOW_rule__XWhileExpression__Group__5_in_rule__XWhileExpression__Group__464916 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_145_in_rule__XWhileExpression__Group__4__Impl64944 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XWhileExpression__Group__5__Impl_in_rule__XWhileExpression__Group__564975 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XWhileExpression__BodyAssignment_5_in_rule__XWhileExpression__Group__5__Impl65002 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XDoWhileExpression__Group__0__Impl_in_rule__XDoWhileExpression__Group__065044 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000000L,0x0000008000000000L});
public static final BitSet FOLLOW_rule__XDoWhileExpression__Group__1_in_rule__XDoWhileExpression__Group__065047 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XDoWhileExpression__Group__1__Impl_in_rule__XDoWhileExpression__Group__165105 = new BitSet(new long[]{0x0007E10C880001F0L,0x0000000000000008L,0x0280BFE298010000L});
public static final BitSet FOLLOW_rule__XDoWhileExpression__Group__2_in_rule__XDoWhileExpression__Group__165108 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_167_in_rule__XDoWhileExpression__Group__1__Impl65136 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XDoWhileExpression__Group__2__Impl_in_rule__XDoWhileExpression__Group__265167 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000000L,0x0000004000000000L});
public static final BitSet FOLLOW_rule__XDoWhileExpression__Group__3_in_rule__XDoWhileExpression__Group__265170 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XDoWhileExpression__BodyAssignment_2_in_rule__XDoWhileExpression__Group__2__Impl65197 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XDoWhileExpression__Group__3__Impl_in_rule__XDoWhileExpression__Group__365227 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000000L,0x0000000000010000L});
public static final BitSet FOLLOW_rule__XDoWhileExpression__Group__4_in_rule__XDoWhileExpression__Group__365230 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_166_in_rule__XDoWhileExpression__Group__3__Impl65258 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XDoWhileExpression__Group__4__Impl_in_rule__XDoWhileExpression__Group__465289 = new BitSet(new long[]{0x0007E10C880001F0L,0x0000000000000008L,0x0280BFE298010000L});
public static final BitSet FOLLOW_rule__XDoWhileExpression__Group__5_in_rule__XDoWhileExpression__Group__465292 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_144_in_rule__XDoWhileExpression__Group__4__Impl65320 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XDoWhileExpression__Group__5__Impl_in_rule__XDoWhileExpression__Group__565351 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000000L,0x0000000000020000L});
public static final BitSet FOLLOW_rule__XDoWhileExpression__Group__6_in_rule__XDoWhileExpression__Group__565354 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XDoWhileExpression__PredicateAssignment_5_in_rule__XDoWhileExpression__Group__5__Impl65381 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XDoWhileExpression__Group__6__Impl_in_rule__XDoWhileExpression__Group__665411 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_145_in_rule__XDoWhileExpression__Group__6__Impl65439 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XBlockExpression__Group__0__Impl_in_rule__XBlockExpression__Group__065484 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000008L});
public static final BitSet FOLLOW_rule__XBlockExpression__Group__1_in_rule__XBlockExpression__Group__065487 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XBlockExpression__Group__1__Impl_in_rule__XBlockExpression__Group__165545 = new BitSet(new long[]{0x0007F10C880001F0L,0x0000000000000018L,0x0380BFE298010000L});
public static final BitSet FOLLOW_rule__XBlockExpression__Group__2_in_rule__XBlockExpression__Group__165548 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_67_in_rule__XBlockExpression__Group__1__Impl65576 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XBlockExpression__Group__2__Impl_in_rule__XBlockExpression__Group__265607 = new BitSet(new long[]{0x0007F10C880001F0L,0x0000000000000018L,0x0380BFE298010000L});
public static final BitSet FOLLOW_rule__XBlockExpression__Group__3_in_rule__XBlockExpression__Group__265610 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XBlockExpression__Group_2__0_in_rule__XBlockExpression__Group__2__Impl65637 = new BitSet(new long[]{0x0007F10C880001F2L,0x0000000000000008L,0x0380BFE298010000L});
public static final BitSet FOLLOW_rule__XBlockExpression__Group__3__Impl_in_rule__XBlockExpression__Group__365668 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_68_in_rule__XBlockExpression__Group__3__Impl65696 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XBlockExpression__Group_2__0__Impl_in_rule__XBlockExpression__Group_2__065735 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000000L,0x0000000040000000L});
public static final BitSet FOLLOW_rule__XBlockExpression__Group_2__1_in_rule__XBlockExpression__Group_2__065738 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XBlockExpression__ExpressionsAssignment_2_0_in_rule__XBlockExpression__Group_2__0__Impl65765 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XBlockExpression__Group_2__1__Impl_in_rule__XBlockExpression__Group_2__165795 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_158_in_rule__XBlockExpression__Group_2__1__Impl65824 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XVariableDeclaration__Group__0__Impl_in_rule__XVariableDeclaration__Group__065861 = new BitSet(new long[]{0x0000100000000000L,0x0000000000000000L,0x0100000000000000L});
public static final BitSet FOLLOW_rule__XVariableDeclaration__Group__1_in_rule__XVariableDeclaration__Group__065864 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XVariableDeclaration__Group__1__Impl_in_rule__XVariableDeclaration__Group__165922 = new BitSet(new long[]{0x0000000080000010L,0x0000000000000000L,0x0000000000010000L});
public static final BitSet FOLLOW_rule__XVariableDeclaration__Group__2_in_rule__XVariableDeclaration__Group__165925 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XVariableDeclaration__Alternatives_1_in_rule__XVariableDeclaration__Group__1__Impl65952 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XVariableDeclaration__Group__2__Impl_in_rule__XVariableDeclaration__Group__265982 = new BitSet(new long[]{0x0000000000002000L});
public static final BitSet FOLLOW_rule__XVariableDeclaration__Group__3_in_rule__XVariableDeclaration__Group__265985 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XVariableDeclaration__Alternatives_2_in_rule__XVariableDeclaration__Group__2__Impl66012 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XVariableDeclaration__Group__3__Impl_in_rule__XVariableDeclaration__Group__366042 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XVariableDeclaration__Group_3__0_in_rule__XVariableDeclaration__Group__3__Impl66069 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XVariableDeclaration__Group_2_0__0__Impl_in_rule__XVariableDeclaration__Group_2_0__066108 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XVariableDeclaration__Group_2_0_0__0_in_rule__XVariableDeclaration__Group_2_0__0__Impl66135 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XVariableDeclaration__Group_2_0_0__0__Impl_in_rule__XVariableDeclaration__Group_2_0_0__066167 = new BitSet(new long[]{0x0000000000000010L});
public static final BitSet FOLLOW_rule__XVariableDeclaration__Group_2_0_0__1_in_rule__XVariableDeclaration__Group_2_0_0__066170 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XVariableDeclaration__TypeAssignment_2_0_0_0_in_rule__XVariableDeclaration__Group_2_0_0__0__Impl66197 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XVariableDeclaration__Group_2_0_0__1__Impl_in_rule__XVariableDeclaration__Group_2_0_0__166227 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XVariableDeclaration__NameAssignment_2_0_0_1_in_rule__XVariableDeclaration__Group_2_0_0__1__Impl66254 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XVariableDeclaration__Group_3__0__Impl_in_rule__XVariableDeclaration__Group_3__066288 = new BitSet(new long[]{0x0007E10C880001F0L,0x0000000000000008L,0x0280BFE298010000L});
public static final BitSet FOLLOW_rule__XVariableDeclaration__Group_3__1_in_rule__XVariableDeclaration__Group_3__066291 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_13_in_rule__XVariableDeclaration__Group_3__0__Impl66319 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XVariableDeclaration__Group_3__1__Impl_in_rule__XVariableDeclaration__Group_3__166350 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XVariableDeclaration__RightAssignment_3_1_in_rule__XVariableDeclaration__Group_3__1__Impl66377 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__JvmFormalParameter__Group__0__Impl_in_rule__JvmFormalParameter__Group__066411 = new BitSet(new long[]{0x0000000080000010L,0x0000000000000000L,0x0000000000010000L});
public static final BitSet FOLLOW_rule__JvmFormalParameter__Group__1_in_rule__JvmFormalParameter__Group__066414 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__JvmFormalParameter__ParameterTypeAssignment_0_in_rule__JvmFormalParameter__Group__0__Impl66441 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__JvmFormalParameter__Group__1__Impl_in_rule__JvmFormalParameter__Group__166472 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__JvmFormalParameter__NameAssignment_1_in_rule__JvmFormalParameter__Group__1__Impl66499 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__FullJvmFormalParameter__Group__0__Impl_in_rule__FullJvmFormalParameter__Group__066533 = new BitSet(new long[]{0x0000000000000010L});
public static final BitSet FOLLOW_rule__FullJvmFormalParameter__Group__1_in_rule__FullJvmFormalParameter__Group__066536 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__FullJvmFormalParameter__ParameterTypeAssignment_0_in_rule__FullJvmFormalParameter__Group__0__Impl66563 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__FullJvmFormalParameter__Group__1__Impl_in_rule__FullJvmFormalParameter__Group__166593 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__FullJvmFormalParameter__NameAssignment_1_in_rule__FullJvmFormalParameter__Group__1__Impl66620 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XFeatureCall__Group__0__Impl_in_rule__XFeatureCall__Group__066654 = new BitSet(new long[]{0x0003E00008000010L});
public static final BitSet FOLLOW_rule__XFeatureCall__Group__1_in_rule__XFeatureCall__Group__066657 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XFeatureCall__Group__1__Impl_in_rule__XFeatureCall__Group__166715 = new BitSet(new long[]{0x0003E00008000010L});
public static final BitSet FOLLOW_rule__XFeatureCall__Group__2_in_rule__XFeatureCall__Group__166718 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XFeatureCall__Group_1__0_in_rule__XFeatureCall__Group__1__Impl66745 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XFeatureCall__Group__2__Impl_in_rule__XFeatureCall__Group__266776 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000000L,0x0000000010010000L});
public static final BitSet FOLLOW_rule__XFeatureCall__Group__3_in_rule__XFeatureCall__Group__266779 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XFeatureCall__FeatureAssignment_2_in_rule__XFeatureCall__Group__2__Impl66806 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XFeatureCall__Group__3__Impl_in_rule__XFeatureCall__Group__366836 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000000L,0x0000000010010000L});
public static final BitSet FOLLOW_rule__XFeatureCall__Group__4_in_rule__XFeatureCall__Group__366839 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XFeatureCall__Group_3__0_in_rule__XFeatureCall__Group__3__Impl66866 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XFeatureCall__Group__4__Impl_in_rule__XFeatureCall__Group__466897 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XFeatureCall__FeatureCallArgumentsAssignment_4_in_rule__XFeatureCall__Group__4__Impl66924 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XFeatureCall__Group_1__0__Impl_in_rule__XFeatureCall__Group_1__066965 = new BitSet(new long[]{0x0000000080000010L,0x0000000000000000L,0x0002000000010000L});
public static final BitSet FOLLOW_rule__XFeatureCall__Group_1__1_in_rule__XFeatureCall__Group_1__066968 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_27_in_rule__XFeatureCall__Group_1__0__Impl66996 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XFeatureCall__Group_1__1__Impl_in_rule__XFeatureCall__Group_1__167027 = new BitSet(new long[]{0x0000000004000000L,0x0000000000000000L,0x0000000004000000L});
public static final BitSet FOLLOW_rule__XFeatureCall__Group_1__2_in_rule__XFeatureCall__Group_1__167030 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XFeatureCall__TypeArgumentsAssignment_1_1_in_rule__XFeatureCall__Group_1__1__Impl67057 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XFeatureCall__Group_1__2__Impl_in_rule__XFeatureCall__Group_1__267087 = new BitSet(new long[]{0x0000000004000000L,0x0000000000000000L,0x0000000004000000L});
public static final BitSet FOLLOW_rule__XFeatureCall__Group_1__3_in_rule__XFeatureCall__Group_1__267090 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XFeatureCall__Group_1_2__0_in_rule__XFeatureCall__Group_1__2__Impl67117 = new BitSet(new long[]{0x0000000000000002L,0x0000000000000000L,0x0000000004000000L});
public static final BitSet FOLLOW_rule__XFeatureCall__Group_1__3__Impl_in_rule__XFeatureCall__Group_1__367148 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_26_in_rule__XFeatureCall__Group_1__3__Impl67176 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XFeatureCall__Group_1_2__0__Impl_in_rule__XFeatureCall__Group_1_2__067215 = new BitSet(new long[]{0x0000000080000010L,0x0000000000000000L,0x0002000000010000L});
public static final BitSet FOLLOW_rule__XFeatureCall__Group_1_2__1_in_rule__XFeatureCall__Group_1_2__067218 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_154_in_rule__XFeatureCall__Group_1_2__0__Impl67246 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XFeatureCall__Group_1_2__1__Impl_in_rule__XFeatureCall__Group_1_2__167277 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XFeatureCall__TypeArgumentsAssignment_1_2_1_in_rule__XFeatureCall__Group_1_2__1__Impl67304 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XFeatureCall__Group_3__0__Impl_in_rule__XFeatureCall__Group_3__067338 = new BitSet(new long[]{0x0007E10C880001F0L,0x0000000000000008L,0x0280BFE298030000L});
public static final BitSet FOLLOW_rule__XFeatureCall__Group_3__1_in_rule__XFeatureCall__Group_3__067341 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XFeatureCall__ExplicitOperationCallAssignment_3_0_in_rule__XFeatureCall__Group_3__0__Impl67368 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XFeatureCall__Group_3__1__Impl_in_rule__XFeatureCall__Group_3__167398 = new BitSet(new long[]{0x0007E10C880001F0L,0x0000000000000008L,0x0280BFE298030000L});
public static final BitSet FOLLOW_rule__XFeatureCall__Group_3__2_in_rule__XFeatureCall__Group_3__167401 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XFeatureCall__Alternatives_3_1_in_rule__XFeatureCall__Group_3__1__Impl67428 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XFeatureCall__Group_3__2__Impl_in_rule__XFeatureCall__Group_3__267459 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_145_in_rule__XFeatureCall__Group_3__2__Impl67487 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XFeatureCall__Group_3_1_1__0__Impl_in_rule__XFeatureCall__Group_3_1_1__067524 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000000L,0x0000000004000000L});
public static final BitSet FOLLOW_rule__XFeatureCall__Group_3_1_1__1_in_rule__XFeatureCall__Group_3_1_1__067527 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XFeatureCall__FeatureCallArgumentsAssignment_3_1_1_0_in_rule__XFeatureCall__Group_3_1_1__0__Impl67554 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XFeatureCall__Group_3_1_1__1__Impl_in_rule__XFeatureCall__Group_3_1_1__167584 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XFeatureCall__Group_3_1_1_1__0_in_rule__XFeatureCall__Group_3_1_1__1__Impl67611 = new BitSet(new long[]{0x0000000000000002L,0x0000000000000000L,0x0000000004000000L});
public static final BitSet FOLLOW_rule__XFeatureCall__Group_3_1_1_1__0__Impl_in_rule__XFeatureCall__Group_3_1_1_1__067646 = new BitSet(new long[]{0x0007E10C880001F0L,0x0000000000000008L,0x0280BFE298010000L});
public static final BitSet FOLLOW_rule__XFeatureCall__Group_3_1_1_1__1_in_rule__XFeatureCall__Group_3_1_1_1__067649 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_154_in_rule__XFeatureCall__Group_3_1_1_1__0__Impl67677 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XFeatureCall__Group_3_1_1_1__1__Impl_in_rule__XFeatureCall__Group_3_1_1_1__167708 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XFeatureCall__FeatureCallArgumentsAssignment_3_1_1_1_1_in_rule__XFeatureCall__Group_3_1_1_1__1__Impl67735 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XConstructorCall__Group__0__Impl_in_rule__XConstructorCall__Group__067769 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000000L,0x0000010000000000L});
public static final BitSet FOLLOW_rule__XConstructorCall__Group__1_in_rule__XConstructorCall__Group__067772 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XConstructorCall__Group__1__Impl_in_rule__XConstructorCall__Group__167830 = new BitSet(new long[]{0x0000000000000010L});
public static final BitSet FOLLOW_rule__XConstructorCall__Group__2_in_rule__XConstructorCall__Group__167833 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_168_in_rule__XConstructorCall__Group__1__Impl67861 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XConstructorCall__Group__2__Impl_in_rule__XConstructorCall__Group__267892 = new BitSet(new long[]{0x0000000008000000L,0x0000000000000000L,0x0000000010010000L});
public static final BitSet FOLLOW_rule__XConstructorCall__Group__3_in_rule__XConstructorCall__Group__267895 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XConstructorCall__ConstructorAssignment_2_in_rule__XConstructorCall__Group__2__Impl67922 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XConstructorCall__Group__3__Impl_in_rule__XConstructorCall__Group__367952 = new BitSet(new long[]{0x0000000008000000L,0x0000000000000000L,0x0000000010010000L});
public static final BitSet FOLLOW_rule__XConstructorCall__Group__4_in_rule__XConstructorCall__Group__367955 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XConstructorCall__Group_3__0_in_rule__XConstructorCall__Group__3__Impl67982 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XConstructorCall__Group__4__Impl_in_rule__XConstructorCall__Group__468013 = new BitSet(new long[]{0x0000000008000000L,0x0000000000000000L,0x0000000010010000L});
public static final BitSet FOLLOW_rule__XConstructorCall__Group__5_in_rule__XConstructorCall__Group__468016 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XConstructorCall__Group_4__0_in_rule__XConstructorCall__Group__4__Impl68043 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XConstructorCall__Group__5__Impl_in_rule__XConstructorCall__Group__568074 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XConstructorCall__ArgumentsAssignment_5_in_rule__XConstructorCall__Group__5__Impl68101 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XConstructorCall__Group_3__0__Impl_in_rule__XConstructorCall__Group_3__068144 = new BitSet(new long[]{0x0000000080000010L,0x0000000000000000L,0x0002000000010000L});
public static final BitSet FOLLOW_rule__XConstructorCall__Group_3__1_in_rule__XConstructorCall__Group_3__068147 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_27_in_rule__XConstructorCall__Group_3__0__Impl68176 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XConstructorCall__Group_3__1__Impl_in_rule__XConstructorCall__Group_3__168208 = new BitSet(new long[]{0x0000000004000000L,0x0000000000000000L,0x0000000004000000L});
public static final BitSet FOLLOW_rule__XConstructorCall__Group_3__2_in_rule__XConstructorCall__Group_3__168211 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XConstructorCall__TypeArgumentsAssignment_3_1_in_rule__XConstructorCall__Group_3__1__Impl68238 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XConstructorCall__Group_3__2__Impl_in_rule__XConstructorCall__Group_3__268268 = new BitSet(new long[]{0x0000000004000000L,0x0000000000000000L,0x0000000004000000L});
public static final BitSet FOLLOW_rule__XConstructorCall__Group_3__3_in_rule__XConstructorCall__Group_3__268271 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XConstructorCall__Group_3_2__0_in_rule__XConstructorCall__Group_3__2__Impl68298 = new BitSet(new long[]{0x0000000000000002L,0x0000000000000000L,0x0000000004000000L});
public static final BitSet FOLLOW_rule__XConstructorCall__Group_3__3__Impl_in_rule__XConstructorCall__Group_3__368329 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_26_in_rule__XConstructorCall__Group_3__3__Impl68357 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XConstructorCall__Group_3_2__0__Impl_in_rule__XConstructorCall__Group_3_2__068396 = new BitSet(new long[]{0x0000000080000010L,0x0000000000000000L,0x0002000000010000L});
public static final BitSet FOLLOW_rule__XConstructorCall__Group_3_2__1_in_rule__XConstructorCall__Group_3_2__068399 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_154_in_rule__XConstructorCall__Group_3_2__0__Impl68427 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XConstructorCall__Group_3_2__1__Impl_in_rule__XConstructorCall__Group_3_2__168458 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XConstructorCall__TypeArgumentsAssignment_3_2_1_in_rule__XConstructorCall__Group_3_2__1__Impl68485 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XConstructorCall__Group_4__0__Impl_in_rule__XConstructorCall__Group_4__068519 = new BitSet(new long[]{0x0007E10C880001F0L,0x0000000000000008L,0x0280BFE298030000L});
public static final BitSet FOLLOW_rule__XConstructorCall__Group_4__1_in_rule__XConstructorCall__Group_4__068522 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XConstructorCall__ExplicitConstructorCallAssignment_4_0_in_rule__XConstructorCall__Group_4__0__Impl68549 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XConstructorCall__Group_4__1__Impl_in_rule__XConstructorCall__Group_4__168579 = new BitSet(new long[]{0x0007E10C880001F0L,0x0000000000000008L,0x0280BFE298030000L});
public static final BitSet FOLLOW_rule__XConstructorCall__Group_4__2_in_rule__XConstructorCall__Group_4__168582 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XConstructorCall__Alternatives_4_1_in_rule__XConstructorCall__Group_4__1__Impl68609 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XConstructorCall__Group_4__2__Impl_in_rule__XConstructorCall__Group_4__268640 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_145_in_rule__XConstructorCall__Group_4__2__Impl68668 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XConstructorCall__Group_4_1_1__0__Impl_in_rule__XConstructorCall__Group_4_1_1__068705 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000000L,0x0000000004000000L});
public static final BitSet FOLLOW_rule__XConstructorCall__Group_4_1_1__1_in_rule__XConstructorCall__Group_4_1_1__068708 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XConstructorCall__ArgumentsAssignment_4_1_1_0_in_rule__XConstructorCall__Group_4_1_1__0__Impl68735 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XConstructorCall__Group_4_1_1__1__Impl_in_rule__XConstructorCall__Group_4_1_1__168765 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XConstructorCall__Group_4_1_1_1__0_in_rule__XConstructorCall__Group_4_1_1__1__Impl68792 = new BitSet(new long[]{0x0000000000000002L,0x0000000000000000L,0x0000000004000000L});
public static final BitSet FOLLOW_rule__XConstructorCall__Group_4_1_1_1__0__Impl_in_rule__XConstructorCall__Group_4_1_1_1__068827 = new BitSet(new long[]{0x0007E10C880001F0L,0x0000000000000008L,0x0280BFE298010000L});
public static final BitSet FOLLOW_rule__XConstructorCall__Group_4_1_1_1__1_in_rule__XConstructorCall__Group_4_1_1_1__068830 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_154_in_rule__XConstructorCall__Group_4_1_1_1__0__Impl68858 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XConstructorCall__Group_4_1_1_1__1__Impl_in_rule__XConstructorCall__Group_4_1_1_1__168889 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XConstructorCall__ArgumentsAssignment_4_1_1_1_1_in_rule__XConstructorCall__Group_4_1_1_1__1__Impl68916 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XBooleanLiteral__Group__0__Impl_in_rule__XBooleanLiteral__Group__068950 = new BitSet(new long[]{0x0004000000000000L,0x0000000000000000L,0x0200000000000000L});
public static final BitSet FOLLOW_rule__XBooleanLiteral__Group__1_in_rule__XBooleanLiteral__Group__068953 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XBooleanLiteral__Group__1__Impl_in_rule__XBooleanLiteral__Group__169011 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XBooleanLiteral__Alternatives_1_in_rule__XBooleanLiteral__Group__1__Impl69038 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XNullLiteral__Group__0__Impl_in_rule__XNullLiteral__Group__069072 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000000L,0x0000020000000000L});
public static final BitSet FOLLOW_rule__XNullLiteral__Group__1_in_rule__XNullLiteral__Group__069075 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XNullLiteral__Group__1__Impl_in_rule__XNullLiteral__Group__169133 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_169_in_rule__XNullLiteral__Group__1__Impl69161 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XNumberLiteral__Group__0__Impl_in_rule__XNumberLiteral__Group__069196 = new BitSet(new long[]{0x00000000000000E0L});
public static final BitSet FOLLOW_rule__XNumberLiteral__Group__1_in_rule__XNumberLiteral__Group__069199 = new BitSet(new long[]{0x0000000000000002L});
}
protected static class FollowSets003 {
public static final BitSet FOLLOW_rule__XNumberLiteral__Group__1__Impl_in_rule__XNumberLiteral__Group__169257 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XNumberLiteral__ValueAssignment_1_in_rule__XNumberLiteral__Group__1__Impl69284 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XStringLiteral__Group__0__Impl_in_rule__XStringLiteral__Group__069318 = new BitSet(new long[]{0x0000000000000100L});
public static final BitSet FOLLOW_rule__XStringLiteral__Group__1_in_rule__XStringLiteral__Group__069321 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XStringLiteral__Group__1__Impl_in_rule__XStringLiteral__Group__169379 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XStringLiteral__ValueAssignment_1_in_rule__XStringLiteral__Group__1__Impl69406 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XTypeLiteral__Group__0__Impl_in_rule__XTypeLiteral__Group__069440 = new BitSet(new long[]{0x00040000000001E0L,0x0000000000000000L,0x0200060018000000L});
public static final BitSet FOLLOW_rule__XTypeLiteral__Group__1_in_rule__XTypeLiteral__Group__069443 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XTypeLiteral__Group__1__Impl_in_rule__XTypeLiteral__Group__169501 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000000L,0x0000000000010000L});
public static final BitSet FOLLOW_rule__XTypeLiteral__Group__2_in_rule__XTypeLiteral__Group__169504 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_170_in_rule__XTypeLiteral__Group__1__Impl69532 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XTypeLiteral__Group__2__Impl_in_rule__XTypeLiteral__Group__269563 = new BitSet(new long[]{0x0000000000000010L});
public static final BitSet FOLLOW_rule__XTypeLiteral__Group__3_in_rule__XTypeLiteral__Group__269566 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_144_in_rule__XTypeLiteral__Group__2__Impl69594 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XTypeLiteral__Group__3__Impl_in_rule__XTypeLiteral__Group__369625 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000000L,0x0000000010020000L});
public static final BitSet FOLLOW_rule__XTypeLiteral__Group__4_in_rule__XTypeLiteral__Group__369628 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XTypeLiteral__TypeAssignment_3_in_rule__XTypeLiteral__Group__3__Impl69655 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XTypeLiteral__Group__4__Impl_in_rule__XTypeLiteral__Group__469685 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000000L,0x0000000010020000L});
public static final BitSet FOLLOW_rule__XTypeLiteral__Group__5_in_rule__XTypeLiteral__Group__469688 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XTypeLiteral__ArrayDimensionsAssignment_4_in_rule__XTypeLiteral__Group__4__Impl69715 = new BitSet(new long[]{0x0000000000000002L,0x0000000000000000L,0x0000000010000000L});
public static final BitSet FOLLOW_rule__XTypeLiteral__Group__5__Impl_in_rule__XTypeLiteral__Group__569746 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_145_in_rule__XTypeLiteral__Group__5__Impl69774 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XThrowExpression__Group__0__Impl_in_rule__XThrowExpression__Group__069817 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000000L,0x0000080000000000L});
public static final BitSet FOLLOW_rule__XThrowExpression__Group__1_in_rule__XThrowExpression__Group__069820 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XThrowExpression__Group__1__Impl_in_rule__XThrowExpression__Group__169878 = new BitSet(new long[]{0x0007E10C880001F0L,0x0000000000000008L,0x0280BFE298010000L});
public static final BitSet FOLLOW_rule__XThrowExpression__Group__2_in_rule__XThrowExpression__Group__169881 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_171_in_rule__XThrowExpression__Group__1__Impl69909 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XThrowExpression__Group__2__Impl_in_rule__XThrowExpression__Group__269940 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XThrowExpression__ExpressionAssignment_2_in_rule__XThrowExpression__Group__2__Impl69967 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XReturnExpression__Group__0__Impl_in_rule__XReturnExpression__Group__070003 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000000L,0x0000100000000000L});
public static final BitSet FOLLOW_rule__XReturnExpression__Group__1_in_rule__XReturnExpression__Group__070006 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XReturnExpression__Group__1__Impl_in_rule__XReturnExpression__Group__170064 = new BitSet(new long[]{0x0007E10C880001F0L,0x0000000000000008L,0x0280BFE298010000L});
public static final BitSet FOLLOW_rule__XReturnExpression__Group__2_in_rule__XReturnExpression__Group__170067 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_172_in_rule__XReturnExpression__Group__1__Impl70095 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XReturnExpression__Group__2__Impl_in_rule__XReturnExpression__Group__270126 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XReturnExpression__ExpressionAssignment_2_in_rule__XReturnExpression__Group__2__Impl70153 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XTryCatchFinallyExpression__Group__0__Impl_in_rule__XTryCatchFinallyExpression__Group__070190 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000000L,0x0000200000000000L});
public static final BitSet FOLLOW_rule__XTryCatchFinallyExpression__Group__1_in_rule__XTryCatchFinallyExpression__Group__070193 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XTryCatchFinallyExpression__Group__1__Impl_in_rule__XTryCatchFinallyExpression__Group__170251 = new BitSet(new long[]{0x0007E10C880001F0L,0x0000000000000008L,0x0280BFE298010000L});
public static final BitSet FOLLOW_rule__XTryCatchFinallyExpression__Group__2_in_rule__XTryCatchFinallyExpression__Group__170254 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_173_in_rule__XTryCatchFinallyExpression__Group__1__Impl70282 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XTryCatchFinallyExpression__Group__2__Impl_in_rule__XTryCatchFinallyExpression__Group__270313 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000000L,0x0001400000000000L});
public static final BitSet FOLLOW_rule__XTryCatchFinallyExpression__Group__3_in_rule__XTryCatchFinallyExpression__Group__270316 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XTryCatchFinallyExpression__ExpressionAssignment_2_in_rule__XTryCatchFinallyExpression__Group__2__Impl70343 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XTryCatchFinallyExpression__Group__3__Impl_in_rule__XTryCatchFinallyExpression__Group__370373 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XTryCatchFinallyExpression__Alternatives_3_in_rule__XTryCatchFinallyExpression__Group__3__Impl70400 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XTryCatchFinallyExpression__Group_3_0__0__Impl_in_rule__XTryCatchFinallyExpression__Group_3_0__070438 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000000L,0x0000400000000000L});
public static final BitSet FOLLOW_rule__XTryCatchFinallyExpression__Group_3_0__1_in_rule__XTryCatchFinallyExpression__Group_3_0__070441 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XTryCatchFinallyExpression__CatchClausesAssignment_3_0_0_in_rule__XTryCatchFinallyExpression__Group_3_0__0__Impl70470 = new BitSet(new long[]{0x0000000000000002L,0x0000000000000000L,0x0001000000000000L});
public static final BitSet FOLLOW_rule__XTryCatchFinallyExpression__CatchClausesAssignment_3_0_0_in_rule__XTryCatchFinallyExpression__Group_3_0__0__Impl70482 = new BitSet(new long[]{0x0000000000000002L,0x0000000000000000L,0x0001000000000000L});
public static final BitSet FOLLOW_rule__XTryCatchFinallyExpression__Group_3_0__1__Impl_in_rule__XTryCatchFinallyExpression__Group_3_0__170515 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XTryCatchFinallyExpression__Group_3_0_1__0_in_rule__XTryCatchFinallyExpression__Group_3_0__1__Impl70542 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XTryCatchFinallyExpression__Group_3_0_1__0__Impl_in_rule__XTryCatchFinallyExpression__Group_3_0_1__070577 = new BitSet(new long[]{0x0007E10C880001F0L,0x0000000000000008L,0x0280BFE298010000L});
public static final BitSet FOLLOW_rule__XTryCatchFinallyExpression__Group_3_0_1__1_in_rule__XTryCatchFinallyExpression__Group_3_0_1__070580 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_174_in_rule__XTryCatchFinallyExpression__Group_3_0_1__0__Impl70609 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XTryCatchFinallyExpression__Group_3_0_1__1__Impl_in_rule__XTryCatchFinallyExpression__Group_3_0_1__170641 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XTryCatchFinallyExpression__FinallyExpressionAssignment_3_0_1_1_in_rule__XTryCatchFinallyExpression__Group_3_0_1__1__Impl70668 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XTryCatchFinallyExpression__Group_3_1__0__Impl_in_rule__XTryCatchFinallyExpression__Group_3_1__070702 = new BitSet(new long[]{0x0007E10C880001F0L,0x0000000000000008L,0x0280BFE298010000L});
public static final BitSet FOLLOW_rule__XTryCatchFinallyExpression__Group_3_1__1_in_rule__XTryCatchFinallyExpression__Group_3_1__070705 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_174_in_rule__XTryCatchFinallyExpression__Group_3_1__0__Impl70733 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XTryCatchFinallyExpression__Group_3_1__1__Impl_in_rule__XTryCatchFinallyExpression__Group_3_1__170764 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XTryCatchFinallyExpression__FinallyExpressionAssignment_3_1_1_in_rule__XTryCatchFinallyExpression__Group_3_1__1__Impl70791 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XSynchronizedExpression__Group__0__Impl_in_rule__XSynchronizedExpression__Group__070825 = new BitSet(new long[]{0x0007E10C880001F0L,0x0000000000000008L,0x0280BFE298010000L});
public static final BitSet FOLLOW_rule__XSynchronizedExpression__Group__1_in_rule__XSynchronizedExpression__Group__070828 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XSynchronizedExpression__Group_0__0_in_rule__XSynchronizedExpression__Group__0__Impl70855 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XSynchronizedExpression__Group__1__Impl_in_rule__XSynchronizedExpression__Group__170885 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000000L,0x0000000000020000L});
public static final BitSet FOLLOW_rule__XSynchronizedExpression__Group__2_in_rule__XSynchronizedExpression__Group__170888 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XSynchronizedExpression__ParamAssignment_1_in_rule__XSynchronizedExpression__Group__1__Impl70915 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XSynchronizedExpression__Group__2__Impl_in_rule__XSynchronizedExpression__Group__270945 = new BitSet(new long[]{0x0007E10C880001F0L,0x0000000000000008L,0x0280BFE298010000L});
public static final BitSet FOLLOW_rule__XSynchronizedExpression__Group__3_in_rule__XSynchronizedExpression__Group__270948 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_145_in_rule__XSynchronizedExpression__Group__2__Impl70976 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XSynchronizedExpression__Group__3__Impl_in_rule__XSynchronizedExpression__Group__371007 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XSynchronizedExpression__ExpressionAssignment_3_in_rule__XSynchronizedExpression__Group__3__Impl71034 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XSynchronizedExpression__Group_0__0__Impl_in_rule__XSynchronizedExpression__Group_0__071072 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XSynchronizedExpression__Group_0_0__0_in_rule__XSynchronizedExpression__Group_0__0__Impl71099 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XSynchronizedExpression__Group_0_0__0__Impl_in_rule__XSynchronizedExpression__Group_0_0__071131 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000000L,0x0000800000000000L});
public static final BitSet FOLLOW_rule__XSynchronizedExpression__Group_0_0__1_in_rule__XSynchronizedExpression__Group_0_0__071134 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XSynchronizedExpression__Group_0_0__1__Impl_in_rule__XSynchronizedExpression__Group_0_0__171192 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000000L,0x0000000000010000L});
public static final BitSet FOLLOW_rule__XSynchronizedExpression__Group_0_0__2_in_rule__XSynchronizedExpression__Group_0_0__171195 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_175_in_rule__XSynchronizedExpression__Group_0_0__1__Impl71223 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XSynchronizedExpression__Group_0_0__2__Impl_in_rule__XSynchronizedExpression__Group_0_0__271254 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_144_in_rule__XSynchronizedExpression__Group_0_0__2__Impl71282 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XCatchClause__Group__0__Impl_in_rule__XCatchClause__Group__071319 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000000L,0x0000000000010000L});
public static final BitSet FOLLOW_rule__XCatchClause__Group__1_in_rule__XCatchClause__Group__071322 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_176_in_rule__XCatchClause__Group__0__Impl71351 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XCatchClause__Group__1__Impl_in_rule__XCatchClause__Group__171383 = new BitSet(new long[]{0x0000000080000010L,0x0000000000000000L,0x0000000000010000L});
public static final BitSet FOLLOW_rule__XCatchClause__Group__2_in_rule__XCatchClause__Group__171386 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_144_in_rule__XCatchClause__Group__1__Impl71414 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XCatchClause__Group__2__Impl_in_rule__XCatchClause__Group__271445 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000000L,0x0000000000020000L});
public static final BitSet FOLLOW_rule__XCatchClause__Group__3_in_rule__XCatchClause__Group__271448 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XCatchClause__DeclaredParamAssignment_2_in_rule__XCatchClause__Group__2__Impl71475 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XCatchClause__Group__3__Impl_in_rule__XCatchClause__Group__371505 = new BitSet(new long[]{0x0007E10C880001F0L,0x0000000000000008L,0x0280BFE298010000L});
public static final BitSet FOLLOW_rule__XCatchClause__Group__4_in_rule__XCatchClause__Group__371508 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_145_in_rule__XCatchClause__Group__3__Impl71536 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XCatchClause__Group__4__Impl_in_rule__XCatchClause__Group__471567 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XCatchClause__ExpressionAssignment_4_in_rule__XCatchClause__Group__4__Impl71594 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__QualifiedName__Group__0__Impl_in_rule__QualifiedName__Group__071634 = new BitSet(new long[]{0x0000080000000000L});
public static final BitSet FOLLOW_rule__QualifiedName__Group__1_in_rule__QualifiedName__Group__071637 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleValidID_in_rule__QualifiedName__Group__0__Impl71664 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__QualifiedName__Group__1__Impl_in_rule__QualifiedName__Group__171693 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__QualifiedName__Group_1__0_in_rule__QualifiedName__Group__1__Impl71720 = new BitSet(new long[]{0x0000080000000002L});
public static final BitSet FOLLOW_rule__QualifiedName__Group_1__0__Impl_in_rule__QualifiedName__Group_1__071755 = new BitSet(new long[]{0x0000000000000010L});
public static final BitSet FOLLOW_rule__QualifiedName__Group_1__1_in_rule__QualifiedName__Group_1__071758 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_43_in_rule__QualifiedName__Group_1__0__Impl71787 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__QualifiedName__Group_1__1__Impl_in_rule__QualifiedName__Group_1__171819 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleValidID_in_rule__QualifiedName__Group_1__1__Impl71846 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Number__Group_1__0__Impl_in_rule__Number__Group_1__071879 = new BitSet(new long[]{0x0000080000000000L});
public static final BitSet FOLLOW_rule__Number__Group_1__1_in_rule__Number__Group_1__071882 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Number__Alternatives_1_0_in_rule__Number__Group_1__0__Impl71909 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Number__Group_1__1__Impl_in_rule__Number__Group_1__171939 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Number__Group_1_1__0_in_rule__Number__Group_1__1__Impl71966 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Number__Group_1_1__0__Impl_in_rule__Number__Group_1_1__072001 = new BitSet(new long[]{0x00000000000000C0L});
public static final BitSet FOLLOW_rule__Number__Group_1_1__1_in_rule__Number__Group_1_1__072004 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_43_in_rule__Number__Group_1_1__0__Impl72032 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Number__Group_1_1__1__Impl_in_rule__Number__Group_1_1__172063 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__Number__Alternatives_1_1_1_in_rule__Number__Group_1_1__1__Impl72090 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__JvmTypeReference__Group_0__0__Impl_in_rule__JvmTypeReference__Group_0__072125 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000000L,0x0000000010000000L});
public static final BitSet FOLLOW_rule__JvmTypeReference__Group_0__1_in_rule__JvmTypeReference__Group_0__072128 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleJvmParameterizedTypeReference_in_rule__JvmTypeReference__Group_0__0__Impl72155 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__JvmTypeReference__Group_0__1__Impl_in_rule__JvmTypeReference__Group_0__172184 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__JvmTypeReference__Group_0_1__0_in_rule__JvmTypeReference__Group_0__1__Impl72211 = new BitSet(new long[]{0x0000000000000002L,0x0000000000000000L,0x0000000010000000L});
public static final BitSet FOLLOW_rule__JvmTypeReference__Group_0_1__0__Impl_in_rule__JvmTypeReference__Group_0_1__072246 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__JvmTypeReference__Group_0_1_0__0_in_rule__JvmTypeReference__Group_0_1__0__Impl72273 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__JvmTypeReference__Group_0_1_0__0__Impl_in_rule__JvmTypeReference__Group_0_1_0__072305 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000000L,0x0000000010000000L});
public static final BitSet FOLLOW_rule__JvmTypeReference__Group_0_1_0__1_in_rule__JvmTypeReference__Group_0_1_0__072308 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__JvmTypeReference__Group_0_1_0__1__Impl_in_rule__JvmTypeReference__Group_0_1_0__172366 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleArrayBrackets_in_rule__JvmTypeReference__Group_0_1_0__1__Impl72393 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__ArrayBrackets__Group__0__Impl_in_rule__ArrayBrackets__Group__072426 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000000L,0x0000000020000000L});
public static final BitSet FOLLOW_rule__ArrayBrackets__Group__1_in_rule__ArrayBrackets__Group__072429 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_156_in_rule__ArrayBrackets__Group__0__Impl72457 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__ArrayBrackets__Group__1__Impl_in_rule__ArrayBrackets__Group__172488 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_157_in_rule__ArrayBrackets__Group__1__Impl72516 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XFunctionTypeRef__Group__0__Impl_in_rule__XFunctionTypeRef__Group__072551 = new BitSet(new long[]{0x0000000080000010L,0x0000000000000000L,0x0000000000010000L});
public static final BitSet FOLLOW_rule__XFunctionTypeRef__Group__1_in_rule__XFunctionTypeRef__Group__072554 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XFunctionTypeRef__Group_0__0_in_rule__XFunctionTypeRef__Group__0__Impl72581 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XFunctionTypeRef__Group__1__Impl_in_rule__XFunctionTypeRef__Group__172612 = new BitSet(new long[]{0x0000000080000010L,0x0000000000000000L,0x0000000000010000L});
public static final BitSet FOLLOW_rule__XFunctionTypeRef__Group__2_in_rule__XFunctionTypeRef__Group__172615 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_31_in_rule__XFunctionTypeRef__Group__1__Impl72643 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XFunctionTypeRef__Group__2__Impl_in_rule__XFunctionTypeRef__Group__272674 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XFunctionTypeRef__ReturnTypeAssignment_2_in_rule__XFunctionTypeRef__Group__2__Impl72701 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XFunctionTypeRef__Group_0__0__Impl_in_rule__XFunctionTypeRef__Group_0__072737 = new BitSet(new long[]{0x0000000080000010L,0x0000000000000000L,0x0000000000030000L});
public static final BitSet FOLLOW_rule__XFunctionTypeRef__Group_0__1_in_rule__XFunctionTypeRef__Group_0__072740 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_144_in_rule__XFunctionTypeRef__Group_0__0__Impl72768 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XFunctionTypeRef__Group_0__1__Impl_in_rule__XFunctionTypeRef__Group_0__172799 = new BitSet(new long[]{0x0000000080000010L,0x0000000000000000L,0x0000000000030000L});
public static final BitSet FOLLOW_rule__XFunctionTypeRef__Group_0__2_in_rule__XFunctionTypeRef__Group_0__172802 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XFunctionTypeRef__Group_0_1__0_in_rule__XFunctionTypeRef__Group_0__1__Impl72829 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XFunctionTypeRef__Group_0__2__Impl_in_rule__XFunctionTypeRef__Group_0__272860 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_145_in_rule__XFunctionTypeRef__Group_0__2__Impl72888 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XFunctionTypeRef__Group_0_1__0__Impl_in_rule__XFunctionTypeRef__Group_0_1__072925 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000000L,0x0000000004000000L});
public static final BitSet FOLLOW_rule__XFunctionTypeRef__Group_0_1__1_in_rule__XFunctionTypeRef__Group_0_1__072928 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XFunctionTypeRef__ParamTypesAssignment_0_1_0_in_rule__XFunctionTypeRef__Group_0_1__0__Impl72955 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XFunctionTypeRef__Group_0_1__1__Impl_in_rule__XFunctionTypeRef__Group_0_1__172985 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XFunctionTypeRef__Group_0_1_1__0_in_rule__XFunctionTypeRef__Group_0_1__1__Impl73012 = new BitSet(new long[]{0x0000000000000002L,0x0000000000000000L,0x0000000004000000L});
public static final BitSet FOLLOW_rule__XFunctionTypeRef__Group_0_1_1__0__Impl_in_rule__XFunctionTypeRef__Group_0_1_1__073047 = new BitSet(new long[]{0x0000000080000010L,0x0000000000000000L,0x0000000000010000L});
public static final BitSet FOLLOW_rule__XFunctionTypeRef__Group_0_1_1__1_in_rule__XFunctionTypeRef__Group_0_1_1__073050 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_154_in_rule__XFunctionTypeRef__Group_0_1_1__0__Impl73078 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XFunctionTypeRef__Group_0_1_1__1__Impl_in_rule__XFunctionTypeRef__Group_0_1_1__173109 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XFunctionTypeRef__ParamTypesAssignment_0_1_1_1_in_rule__XFunctionTypeRef__Group_0_1_1__1__Impl73136 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__JvmParameterizedTypeReference__Group__0__Impl_in_rule__JvmParameterizedTypeReference__Group__073170 = new BitSet(new long[]{0x0000000008000000L});
public static final BitSet FOLLOW_rule__JvmParameterizedTypeReference__Group__1_in_rule__JvmParameterizedTypeReference__Group__073173 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__JvmParameterizedTypeReference__TypeAssignment_0_in_rule__JvmParameterizedTypeReference__Group__0__Impl73200 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__JvmParameterizedTypeReference__Group__1__Impl_in_rule__JvmParameterizedTypeReference__Group__173230 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__JvmParameterizedTypeReference__Group_1__0_in_rule__JvmParameterizedTypeReference__Group__1__Impl73257 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__JvmParameterizedTypeReference__Group_1__0__Impl_in_rule__JvmParameterizedTypeReference__Group_1__073292 = new BitSet(new long[]{0x0000000080000010L,0x0000000000000000L,0x0002000000010000L});
public static final BitSet FOLLOW_rule__JvmParameterizedTypeReference__Group_1__1_in_rule__JvmParameterizedTypeReference__Group_1__073295 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_27_in_rule__JvmParameterizedTypeReference__Group_1__0__Impl73324 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__JvmParameterizedTypeReference__Group_1__1__Impl_in_rule__JvmParameterizedTypeReference__Group_1__173356 = new BitSet(new long[]{0x0000000004000000L,0x0000000000000000L,0x0000000004000000L});
public static final BitSet FOLLOW_rule__JvmParameterizedTypeReference__Group_1__2_in_rule__JvmParameterizedTypeReference__Group_1__173359 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__JvmParameterizedTypeReference__ArgumentsAssignment_1_1_in_rule__JvmParameterizedTypeReference__Group_1__1__Impl73386 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__JvmParameterizedTypeReference__Group_1__2__Impl_in_rule__JvmParameterizedTypeReference__Group_1__273416 = new BitSet(new long[]{0x0000000004000000L,0x0000000000000000L,0x0000000004000000L});
public static final BitSet FOLLOW_rule__JvmParameterizedTypeReference__Group_1__3_in_rule__JvmParameterizedTypeReference__Group_1__273419 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__JvmParameterizedTypeReference__Group_1_2__0_in_rule__JvmParameterizedTypeReference__Group_1__2__Impl73446 = new BitSet(new long[]{0x0000000000000002L,0x0000000000000000L,0x0000000004000000L});
public static final BitSet FOLLOW_rule__JvmParameterizedTypeReference__Group_1__3__Impl_in_rule__JvmParameterizedTypeReference__Group_1__373477 = new BitSet(new long[]{0x0000080000000000L});
public static final BitSet FOLLOW_rule__JvmParameterizedTypeReference__Group_1__4_in_rule__JvmParameterizedTypeReference__Group_1__373480 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_26_in_rule__JvmParameterizedTypeReference__Group_1__3__Impl73508 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__JvmParameterizedTypeReference__Group_1__4__Impl_in_rule__JvmParameterizedTypeReference__Group_1__473539 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__JvmParameterizedTypeReference__Group_1_4__0_in_rule__JvmParameterizedTypeReference__Group_1__4__Impl73566 = new BitSet(new long[]{0x0000080000000002L});
public static final BitSet FOLLOW_rule__JvmParameterizedTypeReference__Group_1_2__0__Impl_in_rule__JvmParameterizedTypeReference__Group_1_2__073607 = new BitSet(new long[]{0x0000000080000010L,0x0000000000000000L,0x0002000000010000L});
public static final BitSet FOLLOW_rule__JvmParameterizedTypeReference__Group_1_2__1_in_rule__JvmParameterizedTypeReference__Group_1_2__073610 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_154_in_rule__JvmParameterizedTypeReference__Group_1_2__0__Impl73638 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__JvmParameterizedTypeReference__Group_1_2__1__Impl_in_rule__JvmParameterizedTypeReference__Group_1_2__173669 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__JvmParameterizedTypeReference__ArgumentsAssignment_1_2_1_in_rule__JvmParameterizedTypeReference__Group_1_2__1__Impl73696 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__JvmParameterizedTypeReference__Group_1_4__0__Impl_in_rule__JvmParameterizedTypeReference__Group_1_4__073730 = new BitSet(new long[]{0x0000000000000010L});
public static final BitSet FOLLOW_rule__JvmParameterizedTypeReference__Group_1_4__1_in_rule__JvmParameterizedTypeReference__Group_1_4__073733 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__JvmParameterizedTypeReference__Group_1_4_0__0_in_rule__JvmParameterizedTypeReference__Group_1_4__0__Impl73760 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__JvmParameterizedTypeReference__Group_1_4__1__Impl_in_rule__JvmParameterizedTypeReference__Group_1_4__173790 = new BitSet(new long[]{0x0000000008000000L});
public static final BitSet FOLLOW_rule__JvmParameterizedTypeReference__Group_1_4__2_in_rule__JvmParameterizedTypeReference__Group_1_4__173793 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__JvmParameterizedTypeReference__TypeAssignment_1_4_1_in_rule__JvmParameterizedTypeReference__Group_1_4__1__Impl73820 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__JvmParameterizedTypeReference__Group_1_4__2__Impl_in_rule__JvmParameterizedTypeReference__Group_1_4__273850 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__JvmParameterizedTypeReference__Group_1_4_2__0_in_rule__JvmParameterizedTypeReference__Group_1_4__2__Impl73877 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__JvmParameterizedTypeReference__Group_1_4_0__0__Impl_in_rule__JvmParameterizedTypeReference__Group_1_4_0__073914 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__JvmParameterizedTypeReference__Group_1_4_0_0__0_in_rule__JvmParameterizedTypeReference__Group_1_4_0__0__Impl73941 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__JvmParameterizedTypeReference__Group_1_4_0_0__0__Impl_in_rule__JvmParameterizedTypeReference__Group_1_4_0_0__073973 = new BitSet(new long[]{0x0000080000000000L});
public static final BitSet FOLLOW_rule__JvmParameterizedTypeReference__Group_1_4_0_0__1_in_rule__JvmParameterizedTypeReference__Group_1_4_0_0__073976 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__JvmParameterizedTypeReference__Group_1_4_0_0__1__Impl_in_rule__JvmParameterizedTypeReference__Group_1_4_0_0__174034 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_43_in_rule__JvmParameterizedTypeReference__Group_1_4_0_0__1__Impl74062 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__JvmParameterizedTypeReference__Group_1_4_2__0__Impl_in_rule__JvmParameterizedTypeReference__Group_1_4_2__074097 = new BitSet(new long[]{0x0000000080000010L,0x0000000000000000L,0x0002000000010000L});
public static final BitSet FOLLOW_rule__JvmParameterizedTypeReference__Group_1_4_2__1_in_rule__JvmParameterizedTypeReference__Group_1_4_2__074100 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_27_in_rule__JvmParameterizedTypeReference__Group_1_4_2__0__Impl74129 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__JvmParameterizedTypeReference__Group_1_4_2__1__Impl_in_rule__JvmParameterizedTypeReference__Group_1_4_2__174161 = new BitSet(new long[]{0x0000000004000000L,0x0000000000000000L,0x0000000004000000L});
public static final BitSet FOLLOW_rule__JvmParameterizedTypeReference__Group_1_4_2__2_in_rule__JvmParameterizedTypeReference__Group_1_4_2__174164 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__JvmParameterizedTypeReference__ArgumentsAssignment_1_4_2_1_in_rule__JvmParameterizedTypeReference__Group_1_4_2__1__Impl74191 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__JvmParameterizedTypeReference__Group_1_4_2__2__Impl_in_rule__JvmParameterizedTypeReference__Group_1_4_2__274221 = new BitSet(new long[]{0x0000000004000000L,0x0000000000000000L,0x0000000004000000L});
public static final BitSet FOLLOW_rule__JvmParameterizedTypeReference__Group_1_4_2__3_in_rule__JvmParameterizedTypeReference__Group_1_4_2__274224 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__JvmParameterizedTypeReference__Group_1_4_2_2__0_in_rule__JvmParameterizedTypeReference__Group_1_4_2__2__Impl74251 = new BitSet(new long[]{0x0000000000000002L,0x0000000000000000L,0x0000000004000000L});
public static final BitSet FOLLOW_rule__JvmParameterizedTypeReference__Group_1_4_2__3__Impl_in_rule__JvmParameterizedTypeReference__Group_1_4_2__374282 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_26_in_rule__JvmParameterizedTypeReference__Group_1_4_2__3__Impl74310 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__JvmParameterizedTypeReference__Group_1_4_2_2__0__Impl_in_rule__JvmParameterizedTypeReference__Group_1_4_2_2__074349 = new BitSet(new long[]{0x0000000080000010L,0x0000000000000000L,0x0002000000010000L});
public static final BitSet FOLLOW_rule__JvmParameterizedTypeReference__Group_1_4_2_2__1_in_rule__JvmParameterizedTypeReference__Group_1_4_2_2__074352 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_154_in_rule__JvmParameterizedTypeReference__Group_1_4_2_2__0__Impl74380 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__JvmParameterizedTypeReference__Group_1_4_2_2__1__Impl_in_rule__JvmParameterizedTypeReference__Group_1_4_2_2__174411 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__JvmParameterizedTypeReference__ArgumentsAssignment_1_4_2_2_1_in_rule__JvmParameterizedTypeReference__Group_1_4_2_2__1__Impl74438 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__JvmWildcardTypeReference__Group__0__Impl_in_rule__JvmWildcardTypeReference__Group__074472 = new BitSet(new long[]{0x0000000080000010L,0x0000000000000000L,0x0002000000010000L});
public static final BitSet FOLLOW_rule__JvmWildcardTypeReference__Group__1_in_rule__JvmWildcardTypeReference__Group__074475 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__JvmWildcardTypeReference__Group__1__Impl_in_rule__JvmWildcardTypeReference__Group__174533 = new BitSet(new long[]{0x0002200000000000L});
public static final BitSet FOLLOW_rule__JvmWildcardTypeReference__Group__2_in_rule__JvmWildcardTypeReference__Group__174536 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_177_in_rule__JvmWildcardTypeReference__Group__1__Impl74564 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__JvmWildcardTypeReference__Group__2__Impl_in_rule__JvmWildcardTypeReference__Group__274595 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__JvmWildcardTypeReference__Alternatives_2_in_rule__JvmWildcardTypeReference__Group__2__Impl74622 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__JvmWildcardTypeReference__Group_2_0__0__Impl_in_rule__JvmWildcardTypeReference__Group_2_0__074659 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000000L,0x0004000000000000L});
public static final BitSet FOLLOW_rule__JvmWildcardTypeReference__Group_2_0__1_in_rule__JvmWildcardTypeReference__Group_2_0__074662 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__JvmWildcardTypeReference__ConstraintsAssignment_2_0_0_in_rule__JvmWildcardTypeReference__Group_2_0__0__Impl74689 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__JvmWildcardTypeReference__Group_2_0__1__Impl_in_rule__JvmWildcardTypeReference__Group_2_0__174719 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__JvmWildcardTypeReference__ConstraintsAssignment_2_0_1_in_rule__JvmWildcardTypeReference__Group_2_0__1__Impl74746 = new BitSet(new long[]{0x0000000000000002L,0x0000000000000000L,0x0004000000000000L});
public static final BitSet FOLLOW_rule__JvmWildcardTypeReference__Group_2_1__0__Impl_in_rule__JvmWildcardTypeReference__Group_2_1__074781 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000000L,0x0004000000000000L});
public static final BitSet FOLLOW_rule__JvmWildcardTypeReference__Group_2_1__1_in_rule__JvmWildcardTypeReference__Group_2_1__074784 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__JvmWildcardTypeReference__ConstraintsAssignment_2_1_0_in_rule__JvmWildcardTypeReference__Group_2_1__0__Impl74811 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__JvmWildcardTypeReference__Group_2_1__1__Impl_in_rule__JvmWildcardTypeReference__Group_2_1__174841 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__JvmWildcardTypeReference__ConstraintsAssignment_2_1_1_in_rule__JvmWildcardTypeReference__Group_2_1__1__Impl74868 = new BitSet(new long[]{0x0000000000000002L,0x0000000000000000L,0x0004000000000000L});
public static final BitSet FOLLOW_rule__JvmUpperBound__Group__0__Impl_in_rule__JvmUpperBound__Group__074903 = new BitSet(new long[]{0x0000000080000010L,0x0000000000000000L,0x0000000000010000L});
public static final BitSet FOLLOW_rule__JvmUpperBound__Group__1_in_rule__JvmUpperBound__Group__074906 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_45_in_rule__JvmUpperBound__Group__0__Impl74934 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__JvmUpperBound__Group__1__Impl_in_rule__JvmUpperBound__Group__174965 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__JvmUpperBound__TypeReferenceAssignment_1_in_rule__JvmUpperBound__Group__1__Impl74992 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__JvmUpperBoundAnded__Group__0__Impl_in_rule__JvmUpperBoundAnded__Group__075026 = new BitSet(new long[]{0x0000000080000010L,0x0000000000000000L,0x0000000000010000L});
public static final BitSet FOLLOW_rule__JvmUpperBoundAnded__Group__1_in_rule__JvmUpperBoundAnded__Group__075029 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_178_in_rule__JvmUpperBoundAnded__Group__0__Impl75057 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__JvmUpperBoundAnded__Group__1__Impl_in_rule__JvmUpperBoundAnded__Group__175088 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__JvmUpperBoundAnded__TypeReferenceAssignment_1_in_rule__JvmUpperBoundAnded__Group__1__Impl75115 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__JvmLowerBound__Group__0__Impl_in_rule__JvmLowerBound__Group__075149 = new BitSet(new long[]{0x0000000080000010L,0x0000000000000000L,0x0000000000010000L});
public static final BitSet FOLLOW_rule__JvmLowerBound__Group__1_in_rule__JvmLowerBound__Group__075152 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_49_in_rule__JvmLowerBound__Group__0__Impl75180 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__JvmLowerBound__Group__1__Impl_in_rule__JvmLowerBound__Group__175211 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__JvmLowerBound__TypeReferenceAssignment_1_in_rule__JvmLowerBound__Group__1__Impl75238 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__JvmLowerBoundAnded__Group__0__Impl_in_rule__JvmLowerBoundAnded__Group__075272 = new BitSet(new long[]{0x0000000080000010L,0x0000000000000000L,0x0000000000010000L});
public static final BitSet FOLLOW_rule__JvmLowerBoundAnded__Group__1_in_rule__JvmLowerBoundAnded__Group__075275 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_178_in_rule__JvmLowerBoundAnded__Group__0__Impl75303 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__JvmLowerBoundAnded__Group__1__Impl_in_rule__JvmLowerBoundAnded__Group__175334 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__JvmLowerBoundAnded__TypeReferenceAssignment_1_in_rule__JvmLowerBoundAnded__Group__1__Impl75361 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__JvmTypeParameter__Group__0__Impl_in_rule__JvmTypeParameter__Group__075395 = new BitSet(new long[]{0x0000200000000000L});
public static final BitSet FOLLOW_rule__JvmTypeParameter__Group__1_in_rule__JvmTypeParameter__Group__075398 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__JvmTypeParameter__NameAssignment_0_in_rule__JvmTypeParameter__Group__0__Impl75425 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__JvmTypeParameter__Group__1__Impl_in_rule__JvmTypeParameter__Group__175455 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__JvmTypeParameter__Group_1__0_in_rule__JvmTypeParameter__Group__1__Impl75482 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__JvmTypeParameter__Group_1__0__Impl_in_rule__JvmTypeParameter__Group_1__075517 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000000L,0x0004000000000000L});
public static final BitSet FOLLOW_rule__JvmTypeParameter__Group_1__1_in_rule__JvmTypeParameter__Group_1__075520 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__JvmTypeParameter__ConstraintsAssignment_1_0_in_rule__JvmTypeParameter__Group_1__0__Impl75547 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__JvmTypeParameter__Group_1__1__Impl_in_rule__JvmTypeParameter__Group_1__175577 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__JvmTypeParameter__ConstraintsAssignment_1_1_in_rule__JvmTypeParameter__Group_1__1__Impl75604 = new BitSet(new long[]{0x0000000000000002L,0x0000000000000000L,0x0004000000000000L});
public static final BitSet FOLLOW_rule__QualifiedNameWithWildcard__Group__0__Impl_in_rule__QualifiedNameWithWildcard__Group__075639 = new BitSet(new long[]{0x0000080000000000L});
public static final BitSet FOLLOW_rule__QualifiedNameWithWildcard__Group__1_in_rule__QualifiedNameWithWildcard__Group__075642 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleQualifiedName_in_rule__QualifiedNameWithWildcard__Group__0__Impl75669 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__QualifiedNameWithWildcard__Group__1__Impl_in_rule__QualifiedNameWithWildcard__Group__175698 = new BitSet(new long[]{0x0000001000000000L});
public static final BitSet FOLLOW_rule__QualifiedNameWithWildcard__Group__2_in_rule__QualifiedNameWithWildcard__Group__175701 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_43_in_rule__QualifiedNameWithWildcard__Group__1__Impl75729 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__QualifiedNameWithWildcard__Group__2__Impl_in_rule__QualifiedNameWithWildcard__Group__275760 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_36_in_rule__QualifiedNameWithWildcard__Group__2__Impl75788 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XImportDeclaration__Group__0__Impl_in_rule__XImportDeclaration__Group__075825 = new BitSet(new long[]{0x0000400000000010L});
public static final BitSet FOLLOW_rule__XImportDeclaration__Group__1_in_rule__XImportDeclaration__Group__075828 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_47_in_rule__XImportDeclaration__Group__0__Impl75856 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XImportDeclaration__Group__1__Impl_in_rule__XImportDeclaration__Group__175887 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000000L,0x0000000040000000L});
public static final BitSet FOLLOW_rule__XImportDeclaration__Group__2_in_rule__XImportDeclaration__Group__175890 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XImportDeclaration__Alternatives_1_in_rule__XImportDeclaration__Group__1__Impl75917 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XImportDeclaration__Group__2__Impl_in_rule__XImportDeclaration__Group__275947 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_158_in_rule__XImportDeclaration__Group__2__Impl75976 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XImportDeclaration__Group_1_0__0__Impl_in_rule__XImportDeclaration__Group_1_0__076015 = new BitSet(new long[]{0x0001000000000010L});
public static final BitSet FOLLOW_rule__XImportDeclaration__Group_1_0__1_in_rule__XImportDeclaration__Group_1_0__076018 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XImportDeclaration__StaticAssignment_1_0_0_in_rule__XImportDeclaration__Group_1_0__0__Impl76045 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XImportDeclaration__Group_1_0__1__Impl_in_rule__XImportDeclaration__Group_1_0__176075 = new BitSet(new long[]{0x0001000000000010L});
public static final BitSet FOLLOW_rule__XImportDeclaration__Group_1_0__2_in_rule__XImportDeclaration__Group_1_0__176078 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XImportDeclaration__ExtensionAssignment_1_0_1_in_rule__XImportDeclaration__Group_1_0__1__Impl76105 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XImportDeclaration__Group_1_0__2__Impl_in_rule__XImportDeclaration__Group_1_0__276136 = new BitSet(new long[]{0x0000001000000010L});
public static final BitSet FOLLOW_rule__XImportDeclaration__Group_1_0__3_in_rule__XImportDeclaration__Group_1_0__276139 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XImportDeclaration__ImportedTypeAssignment_1_0_2_in_rule__XImportDeclaration__Group_1_0__2__Impl76166 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XImportDeclaration__Group_1_0__3__Impl_in_rule__XImportDeclaration__Group_1_0__376196 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XImportDeclaration__Alternatives_1_0_3_in_rule__XImportDeclaration__Group_1_0__3__Impl76223 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__QualifiedNameInStaticImport__Group__0__Impl_in_rule__QualifiedNameInStaticImport__Group__076261 = new BitSet(new long[]{0x0000080000000000L});
public static final BitSet FOLLOW_rule__QualifiedNameInStaticImport__Group__1_in_rule__QualifiedNameInStaticImport__Group__076264 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleValidID_in_rule__QualifiedNameInStaticImport__Group__0__Impl76291 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__QualifiedNameInStaticImport__Group__1__Impl_in_rule__QualifiedNameInStaticImport__Group__176320 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_43_in_rule__QualifiedNameInStaticImport__Group__1__Impl76348 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXImportSection_in_rule__ModelRoot__ImportSectionAssignment_0_076388 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleModel_in_rule__ModelRoot__ModelRootAssignment_0_176419 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleValidID_in_rule__Model__ModelNameAssignment_176450 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rulePackageDeclaration_in_rule__Model__ElementsAssignment_376481 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleQualifiedName_in_rule__PackageDeclaration__PackageNameAssignment_176512 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleAbstractElement_in_rule__PackageDeclaration__ElementsAssignment_376543 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_RULE_ID_in_rule__Enumeration__NameAssignment_176574 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleEnumerationValue_in_rule__Enumeration__EnumerationValuesAssignment_376605 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_RULE_ID_in_rule__EnumerationValue__NameAssignment_076636 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_RULE_STRING_in_rule__EnumerationValue__ValueAssignment_1_176667 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleQualifiedName_in_rule__EntityNaturalKeyFields__NaturalKeyAttributesAssignment_376702 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleQualifiedName_in_rule__EntityNaturalKeyFields__NaturalKeyAttributesAssignment_4_176741 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXBooleanLiteral_in_rule__EntityHierarchical__HierarchicalAssignment_276776 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXBooleanLiteral_in_rule__EntityDisableIdField__DisableIdFieldAssignment_276807 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_RULE_STRING_in_rule__EntityLabelField__LabelAssignment_276838 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_RULE_STRING_in_rule__EntityPluralLabelField__PluralLabelAssignment_276869 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleEntityOptions_in_rule__EntityOptionsContainer__OptionsAssignment_376901 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_RULE_ID_in_rule__Entity__NameAssignment_176932 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleQualifiedName_in_rule__Entity__ExtendsAssignment_2_176967 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleJvmTypeReference_in_rule__Entity__JvmtypeAssignment_3_177002 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleEntityOptionsContainer_in_rule__Entity__EntityOptionsAssignment_577033 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleEntityAttribute_in_rule__Entity__AttributesAssignment_677064 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_RULE_ID_in_rule__ValueObject__NameAssignment_177095 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleQualifiedName_in_rule__ValueObject__ExtendsAssignment_2_177130 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleJvmTypeReference_in_rule__ValueObject__JvmtypeAssignment_3_177165 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleEntityAttribute_in_rule__ValueObject__AttributesAssignment_577196 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleBaseDataTypeProperties_in_rule__BaseDataType__BaseDatatypePropertiesAssignment_177231 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_RULE_INT_in_rule__BaseDataTypeWidth__WidthAssignment_277262 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_RULE_STRING_in_rule__BaseDataTypeLabel__LabelAssignment_277293 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_RULE_ID_in_rule__StringDataType__NameAssignment_177324 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleQualifiedName_in_rule__StringDataType__RefAssignment_2_177359 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleBaseDataType_in_rule__StringDataType__BaseDataTypeAssignment_477394 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_RULE_INT_in_rule__StringDataType__MaxLengthAssignment_5_177425 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_RULE_INT_in_rule__StringDataType__MinLengthAssignment_6_177456 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleQualifiedName_in_rule__StringEntityAttribute__TypeAssignment_177491 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleCardinality_in_rule__StringEntityAttribute__CardinalityAssignment_277526 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_RULE_ID_in_rule__StringEntityAttribute__NameAssignment_377557 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleQualifiedName_in_rule__MapEntityAttribute__TypeAssignment_177597 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleSimpleTypes_in_rule__MapEntityAttribute__KeyTypeAssignment_2_077632 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleSimpleTypes_in_rule__MapEntityAttribute__ValueTypeAssignment_2_177663 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_RULE_ID_in_rule__MapEntityAttribute__NameAssignment_377694 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_RULE_ID_in_rule__BooleanDataType__NameAssignment_177725 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleQualifiedName_in_rule__BooleanDataType__RefAssignment_2_177760 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleBaseDataType_in_rule__BooleanDataType__BaseDataTypeAssignment_477795 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleQualifiedName_in_rule__BooleanEntityAttribute__TypeAssignment_177830 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_RULE_ID_in_rule__BooleanEntityAttribute__NameAssignment_277865 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_RULE_ID_in_rule__IntegerDataType__NameAssignment_177896 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleQualifiedName_in_rule__IntegerDataType__RefAssignment_2_177931 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleBaseDataType_in_rule__IntegerDataType__BaseDataTypeAssignment_477966 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleQualifiedName_in_rule__IntegerEntityAttribute__TypeAssignment_178001 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_RULE_ID_in_rule__IntegerEntityAttribute__NameAssignment_278036 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_RULE_ID_in_rule__DateDataType__NameAssignment_178067 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleQualifiedName_in_rule__DateDataType__RefAssignment_2_178102 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleBaseDataType_in_rule__DateDataType__BaseDataTypeAssignment_478137 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleQualifiedName_in_rule__DateEntityAttribute__TypeAssignment_178172 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_RULE_ID_in_rule__DateEntityAttribute__NameAssignment_278207 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_RULE_ID_in_rule__DecimalDataType__NameAssignment_178238 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleQualifiedName_in_rule__DecimalDataType__RefAssignment_2_178273 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleBaseDataType_in_rule__DecimalDataType__BaseDataTypeAssignment_478308 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleQualifiedName_in_rule__DecimalEntityAttribute__TypeAssignment_178343 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_RULE_ID_in_rule__DecimalEntityAttribute__NameAssignment_278378 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_RULE_ID_in_rule__LongDataType__NameAssignment_178409 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleQualifiedName_in_rule__LongDataType__RefAssignment_2_178444 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleBaseDataType_in_rule__LongDataType__BaseDataTypeAssignment_478479 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleQualifiedName_in_rule__LongEntityAttribute__TypeAssignment_178514 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_RULE_ID_in_rule__LongEntityAttribute__NameAssignment_278549 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_RULE_ID_in_rule__FloatDataType__NameAssignment_178580 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleQualifiedName_in_rule__FloatDataType__RefAssignment_2_178615 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleBaseDataType_in_rule__FloatDataType__BaseDataTypeAssignment_478650 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleQualifiedName_in_rule__FloatEntityAttribute__TypeAssignment_178685 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_RULE_ID_in_rule__FloatEntityAttribute__NameAssignment_278720 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_RULE_ID_in_rule__DoubleDataType__NameAssignment_178751 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleQualifiedName_in_rule__DoubleDataType__RefAssignment_2_178786 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleBaseDataType_in_rule__DoubleDataType__BaseDataTypeAssignment_478821 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleQualifiedName_in_rule__DoubleEntityAttribute__TypeAssignment_178856 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_RULE_ID_in_rule__DoubleEntityAttribute__NameAssignment_278891 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_RULE_ID_in_rule__BinaryDataType__NameAssignment_178922 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleQualifiedName_in_rule__BinaryDataType__RefAssignment_2_178957 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleBaseDataType_in_rule__BinaryDataType__BaseDataTypeAssignment_478992 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleQualifiedName_in_rule__BinaryEntityAttribute__TypeAssignment_179027 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_RULE_ID_in_rule__BinaryEntityAttribute__NameAssignment_279062 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_RULE_ID_in_rule__EntityDataType__NameAssignment_179093 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleQualifiedName_in_rule__EntityDataType__RefAssignment_2_179128 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleBaseDataType_in_rule__EntityDataType__BaseDataTypeAssignment_479163 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleQualifiedName_in_rule__EntityDataType__EntityAssignment_679198 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleQualifiedName_in_rule__EntityEntityAttribute__TypeAssignment_179237 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleCardinality_in_rule__EntityEntityAttribute__CardinalityAssignment_279272 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_RULE_ID_in_rule__EntityEntityAttribute__NameAssignment_379303 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleQualifiedName_in_rule__ValueObjectEntityAttribute__TypeAssignment_179338 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleCardinality_in_rule__ValueObjectEntityAttribute__CardinalityAssignment_279373 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_RULE_ID_in_rule__ValueObjectEntityAttribute__NameAssignment_379404 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_RULE_ID_in_rule__EnumerationDataType__NameAssignment_179435 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleQualifiedName_in_rule__EnumerationDataType__RefAssignment_2_179470 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleBaseDataType_in_rule__EnumerationDataType__BaseDataTypeAssignment_479505 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleQualifiedName_in_rule__EnumerationDataType__EnumerationAssignment_679540 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleQualifiedName_in_rule__EnumerationEntityAttribute__TypeAssignment_179579 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleCardinality_in_rule__EnumerationEntityAttribute__CardinalityAssignment_279614 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_RULE_ID_in_rule__EnumerationEntityAttribute__NameAssignment_379645 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_RULE_ID_in_rule__Dictionary__NameAssignment_179676 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleQualifiedName_in_rule__Dictionary__EntityAssignment_479711 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_RULE_STRING_in_rule__Dictionary__LabelAssignment_5_179746 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_RULE_STRING_in_rule__Dictionary__PluralLabelAssignment_6_179777 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleDictionaryControl_in_rule__Dictionary__DictionarycontrolsAssignment_7_279808 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleDictionaryControl_in_rule__Dictionary__LabelcontrolsAssignment_8_279839 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleDictionarySearch_in_rule__Dictionary__DictionarysearchAssignment_979870 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleDictionaryEditor_in_rule__Dictionary__DictionaryeditorAssignment_1079901 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_RULE_ID_in_rule__DictionarySearch__NameAssignment_179932 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_RULE_STRING_in_rule__DictionarySearch__LabelAssignment_3_179963 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleDictionaryFilter_in_rule__DictionarySearch__DictionaryfiltersAssignment_479994 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleDictionaryResult_in_rule__DictionarySearch__DictionaryresultAssignment_580025 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_RULE_ID_in_rule__DictionaryEditor__NameAssignment_180056 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_RULE_STRING_in_rule__DictionaryEditor__LabelAssignment_3_180087 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleColumnLayoutData_in_rule__DictionaryEditor__LayoutdataAssignment_480118 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleColumnLayout_in_rule__DictionaryEditor__LayoutAssignment_580149 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleDictionaryContainerContent_in_rule__DictionaryEditor__ContainercontentsAssignment_680180 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_RULE_ID_in_rule__DictionaryFilter__NameAssignment_180211 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleColumnLayoutData_in_rule__DictionaryFilter__LayoutdataAssignment_380242 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleColumnLayout_in_rule__DictionaryFilter__LayoutAssignment_480273 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleDictionaryContainerContent_in_rule__DictionaryFilter__ContainercontentsAssignment_580304 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_RULE_ID_in_rule__DictionaryResult__NameAssignment_180335 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleDictionaryControl_in_rule__DictionaryResult__ResultcolumnsAssignment_380366 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_RULE_INT_in_rule__ColumnLayout__ColumnsAssignment_380397 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_RULE_INT_in_rule__ColumnLayoutData__ColumnspanAssignment_380428 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_RULE_ID_in_rule__DictionaryComposite__NameAssignment_180459 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleColumnLayoutData_in_rule__DictionaryComposite__LayoutdataAssignment_380490 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleColumnLayout_in_rule__DictionaryComposite__LayoutAssignment_480521 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleDictionaryContainerContent_in_rule__DictionaryComposite__ContainercontentsAssignment_580552 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_RULE_ID_in_rule__DictionaryEditableTable__NameAssignment_180583 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleColumnLayoutData_in_rule__DictionaryEditableTable__LayoutdataAssignment_3_180614 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleColumnLayout_in_rule__DictionaryEditableTable__LayoutAssignment_4_180645 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleDictionaryContainerContent_in_rule__DictionaryEditableTable__ContainercontentsAssignment_580676 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleQualifiedName_in_rule__DictionaryEditableTable__EntityattributeAssignment_780711 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleDictionaryControl_in_rule__DictionaryEditableTable__ColumncontrolsAssignment_1080746 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_RULE_ID_in_rule__DictionaryAssignmentTable__NameAssignment_180777 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleColumnLayoutData_in_rule__DictionaryAssignmentTable__LayoutdataAssignment_3_180808 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleColumnLayout_in_rule__DictionaryAssignmentTable__LayoutAssignment_4_180839 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleDictionaryContainerContent_in_rule__DictionaryAssignmentTable__ContainercontentsAssignment_580870 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleQualifiedName_in_rule__DictionaryAssignmentTable__EntityattributeAssignment_780905 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleQualifiedName_in_rule__DictionaryAssignmentTable__DictionaryAssignment_980944 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleDictionaryControl_in_rule__DictionaryAssignmentTable__ColumncontrolsAssignment_1280979 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_RULE_STRING_in_rule__Labels__LabelAssignment_1_181010 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_RULE_STRING_in_rule__Labels__FilterLabelAssignment_2_181041 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_RULE_STRING_in_rule__Labels__ColumnLabelAssignment_3_181072 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_RULE_STRING_in_rule__Labels__EditorLabelAssignment_4_181103 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_RULE_STRING_in_rule__Labels__ToolTipAssignment_5_181134 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleQualifiedName_in_rule__BaseDictionaryControl__EntityattributeAssignment_0_181169 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleQualifiedName_in_rule__BaseDictionaryControl__TypeAssignment_1_181208 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleLabels_in_rule__BaseDictionaryControl__LabelsAssignment_281243 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_179_in_rule__BaseDictionaryControl__MandatoryAssignment_381279 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_RULE_INT_in_rule__BaseDictionaryControl__WidthAssignment_4_181318 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXBooleanLiteral_in_rule__BaseDictionaryControl__ReadonlyAssignment_5_181349 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXBooleanLiteral_in_rule__DictionaryControlGroupOptionMultiFilterField__MultiFilterFieldAssignment_1_181380 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleDictionaryControlGroupOptions_in_rule__DictionaryControlGroupOptionsContainer__OptionsAssignment_381411 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_RULE_ID_in_rule__DictionaryControlGroup__NameAssignment_281442 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleQualifiedName_in_rule__DictionaryControlGroup__RefAssignment_3_181477 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleDictionaryControlGroupOptionsContainer_in_rule__DictionaryControlGroup__ControlGroupOptionsAssignment_4_181512 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleBaseDictionaryControl_in_rule__DictionaryControlGroup__BaseControlAssignment_4_281543 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleDictionaryControl_in_rule__DictionaryControlGroup__GroupcontrolsAssignment_4_381574 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_RULE_ID_in_rule__DictionaryHierarchicalControl__NameAssignment_281605 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleQualifiedName_in_rule__DictionaryHierarchicalControl__RefAssignment_3_181640 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleBaseDictionaryControl_in_rule__DictionaryHierarchicalControl__BaseControlAssignment_4_181675 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_RULE_STRING_in_rule__DictionaryHierarchicalControl__HierarchicalIdAssignment_4_381706 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_RULE_ID_in_rule__DictionaryTextControl__NameAssignment_281737 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleQualifiedName_in_rule__DictionaryTextControl__RefAssignment_3_181772 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleBaseDictionaryControl_in_rule__DictionaryTextControl__BaseControlAssignment_4_181807 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleIntegerControlInputType_in_rule__DictionaryIntegerControlInputType__InputtypeAssignment_281838 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_RULE_ID_in_rule__DictionaryIntegerControl__NameAssignment_281869 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleQualifiedName_in_rule__DictionaryIntegerControl__RefAssignment_3_181904 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleBaseDictionaryControl_in_rule__DictionaryIntegerControl__BaseControlAssignment_4_181939 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleDictionaryIntegerControlOptions_in_rule__DictionaryIntegerControl__OptionsAssignment_4_281970 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_RULE_ID_in_rule__DictionaryBigDecimalControl__NameAssignment_282001 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleQualifiedName_in_rule__DictionaryBigDecimalControl__RefAssignment_3_182036 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleBaseDictionaryControl_in_rule__DictionaryBigDecimalControl__BaseControlAssignment_4_182071 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_RULE_ID_in_rule__DictionaryBooleanControl__NameAssignment_282102 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleQualifiedName_in_rule__DictionaryBooleanControl__RefAssignment_3_182137 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleBaseDictionaryControl_in_rule__DictionaryBooleanControl__BaseControlAssignment_4_182172 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_RULE_ID_in_rule__DictionaryDateControl__NameAssignment_282203 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleQualifiedName_in_rule__DictionaryDateControl__RefAssignment_3_182238 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleBaseDictionaryControl_in_rule__DictionaryDateControl__BaseControlAssignment_4_182273 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_RULE_ID_in_rule__DictionaryEnumerationControl__NameAssignment_282304 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleQualifiedName_in_rule__DictionaryEnumerationControl__RefAssignment_3_182339 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleBaseDictionaryControl_in_rule__DictionaryEnumerationControl__BaseControlAssignment_4_182374 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_RULE_ID_in_rule__DictionaryReferenceControl__NameAssignment_282405 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleQualifiedName_in_rule__DictionaryReferenceControl__RefAssignment_3_182440 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleBaseDictionaryControl_in_rule__DictionaryReferenceControl__BaseControlAssignment_4_182475 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleQualifiedName_in_rule__DictionaryReferenceControl__DictionaryAssignment_4_2_182510 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleReferenceControlType_in_rule__DictionaryReferenceControl__ControlTypeAssignment_4_3_182545 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleDictionaryControl_in_rule__DictionaryReferenceControl__LabelcontrolsAssignment_4_4_282576 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_RULE_ID_in_rule__DictionaryFileControl__NameAssignment_282607 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleQualifiedName_in_rule__DictionaryFileControl__RefAssignment_3_182642 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleBaseDictionaryControl_in_rule__DictionaryFileControl__BaseControlAssignment_4_182677 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_RULE_ID_in_rule__Module__NameAssignment_182708 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleQualifiedName_in_rule__Module__ModuledefinitionAssignment_482743 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleModuleParameter_in_rule__Module__ModuleParametersAssignment_5_282778 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleQualifiedName_in_rule__ModuleParameter__ModuleDefinitionParameterAssignment_082813 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_RULE_STRING_in_rule__ModuleParameter__ValueAssignment_282848 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_RULE_ID_in_rule__ModuleDefinition__NameAssignment_182879 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleModuleDefinitionParameter_in_rule__ModuleDefinition__ModuleDefinitionParametersAssignment_3_282910 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_RULE_ID_in_rule__ModuleDefinitionParameter__NameAssignment_182941 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleSimpleTypes_in_rule__ModuleDefinitionParameter__TypeAssignment_482972 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_180_in_rule__ServiceOptions__NonpublicAssignment_183008 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleJvmTypeParameter_in_rule__ServiceMethod__TypeParameterAssignment_1_183047 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleJvmTypeReference_in_rule__ServiceMethod__ReturnTypeAssignment_283078 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleValidID_in_rule__ServiceMethod__NameAssignment_383109 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleFullJvmFormalParameter_in_rule__ServiceMethod__ParamsAssignment_5_083140 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleFullJvmFormalParameter_in_rule__ServiceMethod__ParamsAssignment_5_1_183171 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_RULE_ID_in_rule__Service__NameAssignment_283202 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleServiceOptions_in_rule__Service__RemoteServiceOptionsAssignment_4_283233 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleServiceMethod_in_rule__Service__RemoteMethodsAssignment_583264 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_RULE_ID_in_rule__NavigationNode__NameAssignment_183295 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_RULE_STRING_in_rule__NavigationNode__LabelAssignment_3_183326 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleQualifiedName_in_rule__NavigationNode__ModuleDefinitionAssignment_4_183361 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleQualifiedName_in_rule__NavigationNode__ModuleAssignment_5_183400 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleQualifiedName_in_rule__NavigationNode__DictionaryEditorAssignment_6_183439 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleQualifiedName_in_rule__NavigationNode__DictionarySearchAssignment_7_183478 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleNavigationNode_in_rule__NavigationNode__NavigationNodesAssignment_883513 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleFeatureCallID_in_rule__XAssignment__FeatureAssignment_0_183548 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXAssignment_in_rule__XAssignment__ValueAssignment_0_383583 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleOpMultiAssign_in_rule__XAssignment__FeatureAssignment_1_1_0_0_183618 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXAssignment_in_rule__XAssignment__RightOperandAssignment_1_1_183653 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleOpOr_in_rule__XOrExpression__FeatureAssignment_1_0_0_183688 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXAndExpression_in_rule__XOrExpression__RightOperandAssignment_1_183723 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleOpAnd_in_rule__XAndExpression__FeatureAssignment_1_0_0_183758 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXEqualityExpression_in_rule__XAndExpression__RightOperandAssignment_1_183793 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleOpEquality_in_rule__XEqualityExpression__FeatureAssignment_1_0_0_183828 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXRelationalExpression_in_rule__XEqualityExpression__RightOperandAssignment_1_183863 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleJvmTypeReference_in_rule__XRelationalExpression__TypeAssignment_1_0_183894 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleOpCompare_in_rule__XRelationalExpression__FeatureAssignment_1_1_0_0_183929 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXOtherOperatorExpression_in_rule__XRelationalExpression__RightOperandAssignment_1_1_183964 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleOpOther_in_rule__XOtherOperatorExpression__FeatureAssignment_1_0_0_183999 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXAdditiveExpression_in_rule__XOtherOperatorExpression__RightOperandAssignment_1_184034 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleOpAdd_in_rule__XAdditiveExpression__FeatureAssignment_1_0_0_184069 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXMultiplicativeExpression_in_rule__XAdditiveExpression__RightOperandAssignment_1_184104 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleOpMulti_in_rule__XMultiplicativeExpression__FeatureAssignment_1_0_0_184139 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXUnaryOperation_in_rule__XMultiplicativeExpression__RightOperandAssignment_1_184174 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleOpUnary_in_rule__XUnaryOperation__FeatureAssignment_0_184209 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXUnaryOperation_in_rule__XUnaryOperation__OperandAssignment_0_284244 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleJvmTypeReference_in_rule__XCastedExpression__TypeAssignment_1_184275 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleOpPostfix_in_rule__XPostfixOperation__FeatureAssignment_1_0_184310 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_181_in_rule__XMemberFeatureCall__ExplicitStaticAssignment_1_0_0_0_1_184350 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleFeatureCallID_in_rule__XMemberFeatureCall__FeatureAssignment_1_0_0_0_284393 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXAssignment_in_rule__XMemberFeatureCall__ValueAssignment_1_0_184428 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_182_in_rule__XMemberFeatureCall__NullSafeAssignment_1_1_0_0_1_184464 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_181_in_rule__XMemberFeatureCall__ExplicitStaticAssignment_1_1_0_0_1_284508 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleJvmArgumentTypeReference_in_rule__XMemberFeatureCall__TypeArgumentsAssignment_1_1_1_184547 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleJvmArgumentTypeReference_in_rule__XMemberFeatureCall__TypeArgumentsAssignment_1_1_1_2_184578 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleIdOrSuper_in_rule__XMemberFeatureCall__FeatureAssignment_1_1_284613 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_144_in_rule__XMemberFeatureCall__ExplicitOperationCallAssignment_1_1_3_084653 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXShortClosure_in_rule__XMemberFeatureCall__MemberCallArgumentsAssignment_1_1_3_1_084692 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXExpression_in_rule__XMemberFeatureCall__MemberCallArgumentsAssignment_1_1_3_1_1_084723 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXExpression_in_rule__XMemberFeatureCall__MemberCallArgumentsAssignment_1_1_3_1_1_1_184754 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXClosure_in_rule__XMemberFeatureCall__MemberCallArgumentsAssignment_1_1_484785 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXExpression_in_rule__XSetLiteral__ElementsAssignment_3_084816 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXExpression_in_rule__XSetLiteral__ElementsAssignment_3_1_184847 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXExpression_in_rule__XListLiteral__ElementsAssignment_3_084878 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXExpression_in_rule__XListLiteral__ElementsAssignment_3_1_184909 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleJvmFormalParameter_in_rule__XClosure__DeclaredFormalParametersAssignment_1_0_0_084940 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleJvmFormalParameter_in_rule__XClosure__DeclaredFormalParametersAssignment_1_0_0_1_184971 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_183_in_rule__XClosure__ExplicitSyntaxAssignment_1_0_185007 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXExpressionInClosure_in_rule__XClosure__ExpressionAssignment_285046 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXExpressionOrVarDeclaration_in_rule__XExpressionInClosure__ExpressionsAssignment_1_085077 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleJvmFormalParameter_in_rule__XShortClosure__DeclaredFormalParametersAssignment_0_0_1_085108 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleJvmFormalParameter_in_rule__XShortClosure__DeclaredFormalParametersAssignment_0_0_1_1_185139 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_183_in_rule__XShortClosure__ExplicitSyntaxAssignment_0_0_285175 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXExpression_in_rule__XShortClosure__ExpressionAssignment_185214 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXExpression_in_rule__XIfExpression__IfAssignment_385245 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXExpression_in_rule__XIfExpression__ThenAssignment_585276 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXExpression_in_rule__XIfExpression__ElseAssignment_6_185307 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleJvmFormalParameter_in_rule__XSwitchExpression__DeclaredParamAssignment_2_0_0_0_185338 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXExpression_in_rule__XSwitchExpression__SwitchAssignment_2_0_185369 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleJvmFormalParameter_in_rule__XSwitchExpression__DeclaredParamAssignment_2_1_0_0_085400 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXExpression_in_rule__XSwitchExpression__SwitchAssignment_2_1_185431 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXCasePart_in_rule__XSwitchExpression__CasesAssignment_485462 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXExpression_in_rule__XSwitchExpression__DefaultAssignment_5_285493 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleJvmTypeReference_in_rule__XCasePart__TypeGuardAssignment_185524 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXExpression_in_rule__XCasePart__CaseAssignment_2_185555 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXExpression_in_rule__XCasePart__ThenAssignment_3_0_185586 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_154_in_rule__XCasePart__FallThroughAssignment_3_185622 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleJvmFormalParameter_in_rule__XForLoopExpression__DeclaredParamAssignment_0_0_385661 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXExpression_in_rule__XForLoopExpression__ForExpressionAssignment_185692 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXExpression_in_rule__XForLoopExpression__EachExpressionAssignment_385723 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXExpressionOrVarDeclaration_in_rule__XBasicForLoopExpression__InitExpressionsAssignment_3_085754 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXExpressionOrVarDeclaration_in_rule__XBasicForLoopExpression__InitExpressionsAssignment_3_1_185785 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXExpression_in_rule__XBasicForLoopExpression__ExpressionAssignment_585816 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXExpression_in_rule__XBasicForLoopExpression__UpdateExpressionsAssignment_7_085847 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXExpression_in_rule__XBasicForLoopExpression__UpdateExpressionsAssignment_7_1_185878 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXExpression_in_rule__XBasicForLoopExpression__EachExpressionAssignment_985909 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXExpression_in_rule__XWhileExpression__PredicateAssignment_385940 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXExpression_in_rule__XWhileExpression__BodyAssignment_585971 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXExpression_in_rule__XDoWhileExpression__BodyAssignment_286002 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXExpression_in_rule__XDoWhileExpression__PredicateAssignment_586033 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXExpressionOrVarDeclaration_in_rule__XBlockExpression__ExpressionsAssignment_2_086064 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_184_in_rule__XVariableDeclaration__WriteableAssignment_1_086100 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleJvmTypeReference_in_rule__XVariableDeclaration__TypeAssignment_2_0_0_086139 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleValidID_in_rule__XVariableDeclaration__NameAssignment_2_0_0_186170 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleValidID_in_rule__XVariableDeclaration__NameAssignment_2_186201 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXExpression_in_rule__XVariableDeclaration__RightAssignment_3_186232 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleJvmTypeReference_in_rule__JvmFormalParameter__ParameterTypeAssignment_086263 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleValidID_in_rule__JvmFormalParameter__NameAssignment_186294 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleJvmTypeReference_in_rule__FullJvmFormalParameter__ParameterTypeAssignment_086325 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleValidID_in_rule__FullJvmFormalParameter__NameAssignment_186356 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleJvmArgumentTypeReference_in_rule__XFeatureCall__TypeArgumentsAssignment_1_186387 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleJvmArgumentTypeReference_in_rule__XFeatureCall__TypeArgumentsAssignment_1_2_186418 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleIdOrSuper_in_rule__XFeatureCall__FeatureAssignment_286453 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_144_in_rule__XFeatureCall__ExplicitOperationCallAssignment_3_086493 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXShortClosure_in_rule__XFeatureCall__FeatureCallArgumentsAssignment_3_1_086532 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXExpression_in_rule__XFeatureCall__FeatureCallArgumentsAssignment_3_1_1_086563 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXExpression_in_rule__XFeatureCall__FeatureCallArgumentsAssignment_3_1_1_1_186594 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXClosure_in_rule__XFeatureCall__FeatureCallArgumentsAssignment_486625 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleQualifiedName_in_rule__XConstructorCall__ConstructorAssignment_286660 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleJvmArgumentTypeReference_in_rule__XConstructorCall__TypeArgumentsAssignment_3_186695 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleJvmArgumentTypeReference_in_rule__XConstructorCall__TypeArgumentsAssignment_3_2_186726 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_144_in_rule__XConstructorCall__ExplicitConstructorCallAssignment_4_086762 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXShortClosure_in_rule__XConstructorCall__ArgumentsAssignment_4_1_086801 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXExpression_in_rule__XConstructorCall__ArgumentsAssignment_4_1_1_086832 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXExpression_in_rule__XConstructorCall__ArgumentsAssignment_4_1_1_1_186863 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXClosure_in_rule__XConstructorCall__ArgumentsAssignment_586894 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_185_in_rule__XBooleanLiteral__IsTrueAssignment_1_186930 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleNumber_in_rule__XNumberLiteral__ValueAssignment_186969 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_RULE_STRING_in_rule__XStringLiteral__ValueAssignment_187000 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleQualifiedName_in_rule__XTypeLiteral__TypeAssignment_387035 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleArrayBrackets_in_rule__XTypeLiteral__ArrayDimensionsAssignment_487070 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXExpression_in_rule__XThrowExpression__ExpressionAssignment_287101 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXExpression_in_rule__XReturnExpression__ExpressionAssignment_287132 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXExpression_in_rule__XTryCatchFinallyExpression__ExpressionAssignment_287163 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXCatchClause_in_rule__XTryCatchFinallyExpression__CatchClausesAssignment_3_0_087194 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXExpression_in_rule__XTryCatchFinallyExpression__FinallyExpressionAssignment_3_0_1_187225 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXExpression_in_rule__XTryCatchFinallyExpression__FinallyExpressionAssignment_3_1_187256 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXExpression_in_rule__XSynchronizedExpression__ParamAssignment_187287 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXExpression_in_rule__XSynchronizedExpression__ExpressionAssignment_387318 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleFullJvmFormalParameter_in_rule__XCatchClause__DeclaredParamAssignment_287349 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXExpression_in_rule__XCatchClause__ExpressionAssignment_487380 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleJvmTypeReference_in_rule__XFunctionTypeRef__ParamTypesAssignment_0_1_087411 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleJvmTypeReference_in_rule__XFunctionTypeRef__ParamTypesAssignment_0_1_1_187442 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleJvmTypeReference_in_rule__XFunctionTypeRef__ReturnTypeAssignment_287473 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleQualifiedName_in_rule__JvmParameterizedTypeReference__TypeAssignment_087508 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleJvmArgumentTypeReference_in_rule__JvmParameterizedTypeReference__ArgumentsAssignment_1_187543 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleJvmArgumentTypeReference_in_rule__JvmParameterizedTypeReference__ArgumentsAssignment_1_2_187574 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleValidID_in_rule__JvmParameterizedTypeReference__TypeAssignment_1_4_187609 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleJvmArgumentTypeReference_in_rule__JvmParameterizedTypeReference__ArgumentsAssignment_1_4_2_187644 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleJvmArgumentTypeReference_in_rule__JvmParameterizedTypeReference__ArgumentsAssignment_1_4_2_2_187675 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleJvmUpperBound_in_rule__JvmWildcardTypeReference__ConstraintsAssignment_2_0_087706 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleJvmUpperBoundAnded_in_rule__JvmWildcardTypeReference__ConstraintsAssignment_2_0_187737 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleJvmLowerBound_in_rule__JvmWildcardTypeReference__ConstraintsAssignment_2_1_087768 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleJvmLowerBoundAnded_in_rule__JvmWildcardTypeReference__ConstraintsAssignment_2_1_187799 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleJvmTypeReference_in_rule__JvmUpperBound__TypeReferenceAssignment_187830 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleJvmTypeReference_in_rule__JvmUpperBoundAnded__TypeReferenceAssignment_187861 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleJvmTypeReference_in_rule__JvmLowerBound__TypeReferenceAssignment_187892 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleJvmTypeReference_in_rule__JvmLowerBoundAnded__TypeReferenceAssignment_187923 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleValidID_in_rule__JvmTypeParameter__NameAssignment_087954 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleJvmUpperBound_in_rule__JvmTypeParameter__ConstraintsAssignment_1_087985 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleJvmUpperBoundAnded_in_rule__JvmTypeParameter__ConstraintsAssignment_1_188016 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXImportDeclaration_in_rule__XImportSection__ImportDeclarationsAssignment88047 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_46_in_rule__XImportDeclaration__StaticAssignment_1_0_088083 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_48_in_rule__XImportDeclaration__ExtensionAssignment_1_0_188127 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleQualifiedNameInStaticImport_in_rule__XImportDeclaration__ImportedTypeAssignment_1_0_288170 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_36_in_rule__XImportDeclaration__WildcardAssignment_1_0_3_088210 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleValidID_in_rule__XImportDeclaration__MemberNameAssignment_1_0_3_188249 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleQualifiedName_in_rule__XImportDeclaration__ImportedTypeAssignment_1_188284 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleQualifiedNameWithWildcard_in_rule__XImportDeclaration__ImportedNamespaceAssignment_1_288319 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__OpOther__Group_6_1_0__0_in_synpred75_InternalMango11906 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_27_in_synpred76_InternalMango11925 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XMemberFeatureCall__MemberCallArgumentsAssignment_1_1_3_1_0_in_synpred89_InternalMango12484 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXForLoopExpression_in_synpred97_InternalMango12657 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ruleXBasicForLoopExpression_in_synpred98_InternalMango12675 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XSwitchExpression__Group_2_0__0_in_synpred111_InternalMango12994 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XVariableDeclaration__Group_2_0__0_in_synpred115_InternalMango13198 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XFeatureCall__FeatureCallArgumentsAssignment_3_1_0_in_synpred116_InternalMango13249 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XConstructorCall__ArgumentsAssignment_4_1_0_in_synpred122_InternalMango13464 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XAssignment__Group_1_1__0_in_synpred282_InternalMango49886 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XOrExpression__Group_1__0_in_synpred284_InternalMango50690 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XAndExpression__Group_1__0_in_synpred285_InternalMango51113 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XEqualityExpression__Group_1__0_in_synpred286_InternalMango51536 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XRelationalExpression__Alternatives_1_in_synpred287_InternalMango51959 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XOtherOperatorExpression__Group_1__0_in_synpred288_InternalMango52811 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XAdditiveExpression__Group_1__0_in_synpred289_InternalMango53973 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XMultiplicativeExpression__Group_1__0_in_synpred290_InternalMango54396 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XCastedExpression__Group_1__0_in_synpred291_InternalMango55003 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XPostfixOperation__Group_1__0_in_synpred292_InternalMango55428 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XMemberFeatureCall__Alternatives_1_in_synpred293_InternalMango55730 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XMemberFeatureCall__Group_1_1_3__0_in_synpred295_InternalMango56401 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XMemberFeatureCall__MemberCallArgumentsAssignment_1_1_4_in_synpred296_InternalMango56459 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XClosure__Group_1__0_in_synpred304_InternalMango58695 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XIfExpression__Group_6__0_in_synpred311_InternalMango60901 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XSwitchExpression__Group_2_1_0__0_in_synpred314_InternalMango61972 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XFeatureCall__Group_3__0_in_synpred327_InternalMango66866 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XFeatureCall__FeatureCallArgumentsAssignment_4_in_synpred328_InternalMango66924 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XConstructorCall__Group_3__0_in_synpred332_InternalMango67982 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XConstructorCall__Group_4__0_in_synpred333_InternalMango68043 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XConstructorCall__ArgumentsAssignment_5_in_synpred334_InternalMango68101 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XReturnExpression__ExpressionAssignment_2_in_synpred339_InternalMango70153 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XTryCatchFinallyExpression__CatchClausesAssignment_3_0_0_in_synpred340_InternalMango70482 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__XTryCatchFinallyExpression__Group_3_0_1__0_in_synpred341_InternalMango70542 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__QualifiedName__Group_1__0_in_synpred342_InternalMango71720 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__JvmTypeReference__Group_0_1__0_in_synpred344_InternalMango72211 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__JvmParameterizedTypeReference__Group_1__0_in_synpred348_InternalMango73257 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__JvmParameterizedTypeReference__Group_1_4__0_in_synpred350_InternalMango73566 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_rule__JvmParameterizedTypeReference__Group_1_4_2__0_in_synpred351_InternalMango73877 = new BitSet(new long[]{0x0000000000000002L});
}
}
@SuppressWarnings("all")
abstract class InternalMangoParser2 extends InternalMangoParser1 {
InternalMangoParser2(TokenStream input) {
this(input, new RecognizerSharedState());
}
InternalMangoParser2(TokenStream input, RecognizerSharedState state) {
super(input, state);
}
// $ANTLR start "entryRuleModelRoot"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:61:1: entryRuleModelRoot : ruleModelRoot EOF ;
public final void entryRuleModelRoot() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:62:1: ( ruleModelRoot EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:63:1: ruleModelRoot EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getModelRootRule());
}
pushFollow(FollowSets000.FOLLOW_ruleModelRoot_in_entryRuleModelRoot67);
ruleModelRoot();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getModelRootRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleModelRoot74); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleModelRoot"
// $ANTLR start "ruleModelRoot"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:70:1: ruleModelRoot : ( ( rule__ModelRoot__Alternatives ) ) ;
public final void ruleModelRoot() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:74:2: ( ( ( rule__ModelRoot__Alternatives ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:75:1: ( ( rule__ModelRoot__Alternatives ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:75:1: ( ( rule__ModelRoot__Alternatives ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:76:1: ( rule__ModelRoot__Alternatives )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getModelRootAccess().getAlternatives());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:77:1: ( rule__ModelRoot__Alternatives )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:77:2: rule__ModelRoot__Alternatives
{
pushFollow(FollowSets000.FOLLOW_rule__ModelRoot__Alternatives_in_ruleModelRoot100);
rule__ModelRoot__Alternatives();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getModelRootAccess().getAlternatives());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleModelRoot"
// $ANTLR start "entryRuleModel"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:89:1: entryRuleModel : ruleModel EOF ;
public final void entryRuleModel() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:90:1: ( ruleModel EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:91:1: ruleModel EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getModelRule());
}
pushFollow(FollowSets000.FOLLOW_ruleModel_in_entryRuleModel127);
ruleModel();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getModelRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleModel134); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleModel"
// $ANTLR start "ruleModel"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:98:1: ruleModel : ( ( rule__Model__Group__0 ) ) ;
public final void ruleModel() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:102:2: ( ( ( rule__Model__Group__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:103:1: ( ( rule__Model__Group__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:103:1: ( ( rule__Model__Group__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:104:1: ( rule__Model__Group__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getModelAccess().getGroup());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:105:1: ( rule__Model__Group__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:105:2: rule__Model__Group__0
{
pushFollow(FollowSets000.FOLLOW_rule__Model__Group__0_in_ruleModel160);
rule__Model__Group__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getModelAccess().getGroup());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleModel"
// $ANTLR start "entryRulePackageDeclaration"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:117:1: entryRulePackageDeclaration : rulePackageDeclaration EOF ;
public final void entryRulePackageDeclaration() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:118:1: ( rulePackageDeclaration EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:119:1: rulePackageDeclaration EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getPackageDeclarationRule());
}
pushFollow(FollowSets000.FOLLOW_rulePackageDeclaration_in_entryRulePackageDeclaration187);
rulePackageDeclaration();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getPackageDeclarationRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRulePackageDeclaration194); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRulePackageDeclaration"
// $ANTLR start "rulePackageDeclaration"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:126:1: rulePackageDeclaration : ( ( rule__PackageDeclaration__Group__0 ) ) ;
public final void rulePackageDeclaration() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:130:2: ( ( ( rule__PackageDeclaration__Group__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:131:1: ( ( rule__PackageDeclaration__Group__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:131:1: ( ( rule__PackageDeclaration__Group__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:132:1: ( rule__PackageDeclaration__Group__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getPackageDeclarationAccess().getGroup());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:133:1: ( rule__PackageDeclaration__Group__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:133:2: rule__PackageDeclaration__Group__0
{
pushFollow(FollowSets000.FOLLOW_rule__PackageDeclaration__Group__0_in_rulePackageDeclaration220);
rule__PackageDeclaration__Group__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getPackageDeclarationAccess().getGroup());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rulePackageDeclaration"
// $ANTLR start "entryRuleAbstractElement"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:145:1: entryRuleAbstractElement : ruleAbstractElement EOF ;
public final void entryRuleAbstractElement() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:146:1: ( ruleAbstractElement EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:147:1: ruleAbstractElement EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getAbstractElementRule());
}
pushFollow(FollowSets000.FOLLOW_ruleAbstractElement_in_entryRuleAbstractElement247);
ruleAbstractElement();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getAbstractElementRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleAbstractElement254); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleAbstractElement"
// $ANTLR start "ruleAbstractElement"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:154:1: ruleAbstractElement : ( ( rule__AbstractElement__Alternatives ) ) ;
public final void ruleAbstractElement() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:158:2: ( ( ( rule__AbstractElement__Alternatives ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:159:1: ( ( rule__AbstractElement__Alternatives ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:159:1: ( ( rule__AbstractElement__Alternatives ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:160:1: ( rule__AbstractElement__Alternatives )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getAbstractElementAccess().getAlternatives());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:161:1: ( rule__AbstractElement__Alternatives )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:161:2: rule__AbstractElement__Alternatives
{
pushFollow(FollowSets000.FOLLOW_rule__AbstractElement__Alternatives_in_ruleAbstractElement280);
rule__AbstractElement__Alternatives();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getAbstractElementAccess().getAlternatives());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleAbstractElement"
// $ANTLR start "entryRuleEnumeration"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:173:1: entryRuleEnumeration : ruleEnumeration EOF ;
public final void entryRuleEnumeration() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:174:1: ( ruleEnumeration EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:175:1: ruleEnumeration EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEnumerationRule());
}
pushFollow(FollowSets000.FOLLOW_ruleEnumeration_in_entryRuleEnumeration307);
ruleEnumeration();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getEnumerationRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleEnumeration314); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleEnumeration"
// $ANTLR start "ruleEnumeration"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:182:1: ruleEnumeration : ( ( rule__Enumeration__Group__0 ) ) ;
public final void ruleEnumeration() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:186:2: ( ( ( rule__Enumeration__Group__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:187:1: ( ( rule__Enumeration__Group__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:187:1: ( ( rule__Enumeration__Group__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:188:1: ( rule__Enumeration__Group__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEnumerationAccess().getGroup());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:189:1: ( rule__Enumeration__Group__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:189:2: rule__Enumeration__Group__0
{
pushFollow(FollowSets000.FOLLOW_rule__Enumeration__Group__0_in_ruleEnumeration340);
rule__Enumeration__Group__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getEnumerationAccess().getGroup());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleEnumeration"
// $ANTLR start "entryRuleEnumerationValue"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:201:1: entryRuleEnumerationValue : ruleEnumerationValue EOF ;
public final void entryRuleEnumerationValue() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:202:1: ( ruleEnumerationValue EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:203:1: ruleEnumerationValue EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEnumerationValueRule());
}
pushFollow(FollowSets000.FOLLOW_ruleEnumerationValue_in_entryRuleEnumerationValue367);
ruleEnumerationValue();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getEnumerationValueRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleEnumerationValue374); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleEnumerationValue"
// $ANTLR start "ruleEnumerationValue"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:210:1: ruleEnumerationValue : ( ( rule__EnumerationValue__Group__0 ) ) ;
public final void ruleEnumerationValue() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:214:2: ( ( ( rule__EnumerationValue__Group__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:215:1: ( ( rule__EnumerationValue__Group__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:215:1: ( ( rule__EnumerationValue__Group__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:216:1: ( rule__EnumerationValue__Group__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEnumerationValueAccess().getGroup());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:217:1: ( rule__EnumerationValue__Group__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:217:2: rule__EnumerationValue__Group__0
{
pushFollow(FollowSets000.FOLLOW_rule__EnumerationValue__Group__0_in_ruleEnumerationValue400);
rule__EnumerationValue__Group__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getEnumerationValueAccess().getGroup());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleEnumerationValue"
// $ANTLR start "entryRuleEntityOptions"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:229:1: entryRuleEntityOptions : ruleEntityOptions EOF ;
public final void entryRuleEntityOptions() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:230:1: ( ruleEntityOptions EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:231:1: ruleEntityOptions EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEntityOptionsRule());
}
pushFollow(FollowSets000.FOLLOW_ruleEntityOptions_in_entryRuleEntityOptions427);
ruleEntityOptions();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getEntityOptionsRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleEntityOptions434); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleEntityOptions"
// $ANTLR start "ruleEntityOptions"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:238:1: ruleEntityOptions : ( ( rule__EntityOptions__Alternatives ) ) ;
public final void ruleEntityOptions() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:242:2: ( ( ( rule__EntityOptions__Alternatives ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:243:1: ( ( rule__EntityOptions__Alternatives ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:243:1: ( ( rule__EntityOptions__Alternatives ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:244:1: ( rule__EntityOptions__Alternatives )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEntityOptionsAccess().getAlternatives());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:245:1: ( rule__EntityOptions__Alternatives )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:245:2: rule__EntityOptions__Alternatives
{
pushFollow(FollowSets000.FOLLOW_rule__EntityOptions__Alternatives_in_ruleEntityOptions460);
rule__EntityOptions__Alternatives();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getEntityOptionsAccess().getAlternatives());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleEntityOptions"
// $ANTLR start "entryRuleEntityNaturalKeyFields"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:257:1: entryRuleEntityNaturalKeyFields : ruleEntityNaturalKeyFields EOF ;
public final void entryRuleEntityNaturalKeyFields() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:258:1: ( ruleEntityNaturalKeyFields EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:259:1: ruleEntityNaturalKeyFields EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEntityNaturalKeyFieldsRule());
}
pushFollow(FollowSets000.FOLLOW_ruleEntityNaturalKeyFields_in_entryRuleEntityNaturalKeyFields487);
ruleEntityNaturalKeyFields();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getEntityNaturalKeyFieldsRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleEntityNaturalKeyFields494); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleEntityNaturalKeyFields"
// $ANTLR start "ruleEntityNaturalKeyFields"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:266:1: ruleEntityNaturalKeyFields : ( ( rule__EntityNaturalKeyFields__Group__0 ) ) ;
public final void ruleEntityNaturalKeyFields() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:270:2: ( ( ( rule__EntityNaturalKeyFields__Group__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:271:1: ( ( rule__EntityNaturalKeyFields__Group__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:271:1: ( ( rule__EntityNaturalKeyFields__Group__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:272:1: ( rule__EntityNaturalKeyFields__Group__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEntityNaturalKeyFieldsAccess().getGroup());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:273:1: ( rule__EntityNaturalKeyFields__Group__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:273:2: rule__EntityNaturalKeyFields__Group__0
{
pushFollow(FollowSets000.FOLLOW_rule__EntityNaturalKeyFields__Group__0_in_ruleEntityNaturalKeyFields520);
rule__EntityNaturalKeyFields__Group__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getEntityNaturalKeyFieldsAccess().getGroup());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleEntityNaturalKeyFields"
// $ANTLR start "entryRuleEntityHierarchical"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:285:1: entryRuleEntityHierarchical : ruleEntityHierarchical EOF ;
public final void entryRuleEntityHierarchical() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:286:1: ( ruleEntityHierarchical EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:287:1: ruleEntityHierarchical EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEntityHierarchicalRule());
}
pushFollow(FollowSets000.FOLLOW_ruleEntityHierarchical_in_entryRuleEntityHierarchical547);
ruleEntityHierarchical();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getEntityHierarchicalRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleEntityHierarchical554); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleEntityHierarchical"
// $ANTLR start "ruleEntityHierarchical"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:294:1: ruleEntityHierarchical : ( ( rule__EntityHierarchical__Group__0 ) ) ;
public final void ruleEntityHierarchical() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:298:2: ( ( ( rule__EntityHierarchical__Group__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:299:1: ( ( rule__EntityHierarchical__Group__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:299:1: ( ( rule__EntityHierarchical__Group__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:300:1: ( rule__EntityHierarchical__Group__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEntityHierarchicalAccess().getGroup());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:301:1: ( rule__EntityHierarchical__Group__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:301:2: rule__EntityHierarchical__Group__0
{
pushFollow(FollowSets000.FOLLOW_rule__EntityHierarchical__Group__0_in_ruleEntityHierarchical580);
rule__EntityHierarchical__Group__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getEntityHierarchicalAccess().getGroup());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleEntityHierarchical"
// $ANTLR start "entryRuleEntityDisableIdField"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:313:1: entryRuleEntityDisableIdField : ruleEntityDisableIdField EOF ;
public final void entryRuleEntityDisableIdField() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:314:1: ( ruleEntityDisableIdField EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:315:1: ruleEntityDisableIdField EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEntityDisableIdFieldRule());
}
pushFollow(FollowSets000.FOLLOW_ruleEntityDisableIdField_in_entryRuleEntityDisableIdField607);
ruleEntityDisableIdField();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getEntityDisableIdFieldRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleEntityDisableIdField614); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleEntityDisableIdField"
// $ANTLR start "ruleEntityDisableIdField"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:322:1: ruleEntityDisableIdField : ( ( rule__EntityDisableIdField__Group__0 ) ) ;
public final void ruleEntityDisableIdField() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:326:2: ( ( ( rule__EntityDisableIdField__Group__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:327:1: ( ( rule__EntityDisableIdField__Group__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:327:1: ( ( rule__EntityDisableIdField__Group__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:328:1: ( rule__EntityDisableIdField__Group__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEntityDisableIdFieldAccess().getGroup());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:329:1: ( rule__EntityDisableIdField__Group__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:329:2: rule__EntityDisableIdField__Group__0
{
pushFollow(FollowSets000.FOLLOW_rule__EntityDisableIdField__Group__0_in_ruleEntityDisableIdField640);
rule__EntityDisableIdField__Group__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getEntityDisableIdFieldAccess().getGroup());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleEntityDisableIdField"
// $ANTLR start "entryRuleEntityLabelField"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:341:1: entryRuleEntityLabelField : ruleEntityLabelField EOF ;
public final void entryRuleEntityLabelField() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:342:1: ( ruleEntityLabelField EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:343:1: ruleEntityLabelField EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEntityLabelFieldRule());
}
pushFollow(FollowSets000.FOLLOW_ruleEntityLabelField_in_entryRuleEntityLabelField667);
ruleEntityLabelField();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getEntityLabelFieldRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleEntityLabelField674); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleEntityLabelField"
// $ANTLR start "ruleEntityLabelField"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:350:1: ruleEntityLabelField : ( ( rule__EntityLabelField__Group__0 ) ) ;
public final void ruleEntityLabelField() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:354:2: ( ( ( rule__EntityLabelField__Group__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:355:1: ( ( rule__EntityLabelField__Group__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:355:1: ( ( rule__EntityLabelField__Group__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:356:1: ( rule__EntityLabelField__Group__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEntityLabelFieldAccess().getGroup());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:357:1: ( rule__EntityLabelField__Group__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:357:2: rule__EntityLabelField__Group__0
{
pushFollow(FollowSets000.FOLLOW_rule__EntityLabelField__Group__0_in_ruleEntityLabelField700);
rule__EntityLabelField__Group__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getEntityLabelFieldAccess().getGroup());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleEntityLabelField"
// $ANTLR start "entryRuleEntityPluralLabelField"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:369:1: entryRuleEntityPluralLabelField : ruleEntityPluralLabelField EOF ;
public final void entryRuleEntityPluralLabelField() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:370:1: ( ruleEntityPluralLabelField EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:371:1: ruleEntityPluralLabelField EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEntityPluralLabelFieldRule());
}
pushFollow(FollowSets000.FOLLOW_ruleEntityPluralLabelField_in_entryRuleEntityPluralLabelField727);
ruleEntityPluralLabelField();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getEntityPluralLabelFieldRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleEntityPluralLabelField734); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleEntityPluralLabelField"
// $ANTLR start "ruleEntityPluralLabelField"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:378:1: ruleEntityPluralLabelField : ( ( rule__EntityPluralLabelField__Group__0 ) ) ;
public final void ruleEntityPluralLabelField() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:382:2: ( ( ( rule__EntityPluralLabelField__Group__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:383:1: ( ( rule__EntityPluralLabelField__Group__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:383:1: ( ( rule__EntityPluralLabelField__Group__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:384:1: ( rule__EntityPluralLabelField__Group__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEntityPluralLabelFieldAccess().getGroup());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:385:1: ( rule__EntityPluralLabelField__Group__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:385:2: rule__EntityPluralLabelField__Group__0
{
pushFollow(FollowSets000.FOLLOW_rule__EntityPluralLabelField__Group__0_in_ruleEntityPluralLabelField760);
rule__EntityPluralLabelField__Group__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getEntityPluralLabelFieldAccess().getGroup());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleEntityPluralLabelField"
// $ANTLR start "entryRuleEntityOptionsContainer"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:399:1: entryRuleEntityOptionsContainer : ruleEntityOptionsContainer EOF ;
public final void entryRuleEntityOptionsContainer() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:400:1: ( ruleEntityOptionsContainer EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:401:1: ruleEntityOptionsContainer EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEntityOptionsContainerRule());
}
pushFollow(FollowSets000.FOLLOW_ruleEntityOptionsContainer_in_entryRuleEntityOptionsContainer789);
ruleEntityOptionsContainer();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getEntityOptionsContainerRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleEntityOptionsContainer796); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleEntityOptionsContainer"
// $ANTLR start "ruleEntityOptionsContainer"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:408:1: ruleEntityOptionsContainer : ( ( rule__EntityOptionsContainer__Group__0 ) ) ;
public final void ruleEntityOptionsContainer() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:412:2: ( ( ( rule__EntityOptionsContainer__Group__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:413:1: ( ( rule__EntityOptionsContainer__Group__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:413:1: ( ( rule__EntityOptionsContainer__Group__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:414:1: ( rule__EntityOptionsContainer__Group__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEntityOptionsContainerAccess().getGroup());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:415:1: ( rule__EntityOptionsContainer__Group__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:415:2: rule__EntityOptionsContainer__Group__0
{
pushFollow(FollowSets000.FOLLOW_rule__EntityOptionsContainer__Group__0_in_ruleEntityOptionsContainer822);
rule__EntityOptionsContainer__Group__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getEntityOptionsContainerAccess().getGroup());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleEntityOptionsContainer"
// $ANTLR start "entryRuleEntity"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:427:1: entryRuleEntity : ruleEntity EOF ;
public final void entryRuleEntity() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:428:1: ( ruleEntity EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:429:1: ruleEntity EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEntityRule());
}
pushFollow(FollowSets000.FOLLOW_ruleEntity_in_entryRuleEntity849);
ruleEntity();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getEntityRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleEntity856); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleEntity"
// $ANTLR start "ruleEntity"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:436:1: ruleEntity : ( ( rule__Entity__Group__0 ) ) ;
public final void ruleEntity() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:440:2: ( ( ( rule__Entity__Group__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:441:1: ( ( rule__Entity__Group__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:441:1: ( ( rule__Entity__Group__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:442:1: ( rule__Entity__Group__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEntityAccess().getGroup());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:443:1: ( rule__Entity__Group__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:443:2: rule__Entity__Group__0
{
pushFollow(FollowSets000.FOLLOW_rule__Entity__Group__0_in_ruleEntity882);
rule__Entity__Group__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getEntityAccess().getGroup());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleEntity"
// $ANTLR start "entryRuleValueObject"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:455:1: entryRuleValueObject : ruleValueObject EOF ;
public final void entryRuleValueObject() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:456:1: ( ruleValueObject EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:457:1: ruleValueObject EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getValueObjectRule());
}
pushFollow(FollowSets000.FOLLOW_ruleValueObject_in_entryRuleValueObject909);
ruleValueObject();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getValueObjectRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleValueObject916); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleValueObject"
// $ANTLR start "ruleValueObject"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:464:1: ruleValueObject : ( ( rule__ValueObject__Group__0 ) ) ;
public final void ruleValueObject() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:468:2: ( ( ( rule__ValueObject__Group__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:469:1: ( ( rule__ValueObject__Group__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:469:1: ( ( rule__ValueObject__Group__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:470:1: ( rule__ValueObject__Group__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getValueObjectAccess().getGroup());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:471:1: ( rule__ValueObject__Group__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:471:2: rule__ValueObject__Group__0
{
pushFollow(FollowSets000.FOLLOW_rule__ValueObject__Group__0_in_ruleValueObject942);
rule__ValueObject__Group__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getValueObjectAccess().getGroup());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleValueObject"
// $ANTLR start "entryRuleDatatype"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:485:1: entryRuleDatatype : ruleDatatype EOF ;
public final void entryRuleDatatype() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:486:1: ( ruleDatatype EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:487:1: ruleDatatype EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDatatypeRule());
}
pushFollow(FollowSets000.FOLLOW_ruleDatatype_in_entryRuleDatatype971);
ruleDatatype();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDatatypeRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleDatatype978); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleDatatype"
// $ANTLR start "ruleDatatype"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:494:1: ruleDatatype : ( ( rule__Datatype__Alternatives ) ) ;
public final void ruleDatatype() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:498:2: ( ( ( rule__Datatype__Alternatives ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:499:1: ( ( rule__Datatype__Alternatives ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:499:1: ( ( rule__Datatype__Alternatives ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:500:1: ( rule__Datatype__Alternatives )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDatatypeAccess().getAlternatives());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:501:1: ( rule__Datatype__Alternatives )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:501:2: rule__Datatype__Alternatives
{
pushFollow(FollowSets000.FOLLOW_rule__Datatype__Alternatives_in_ruleDatatype1004);
rule__Datatype__Alternatives();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDatatypeAccess().getAlternatives());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleDatatype"
// $ANTLR start "entryRuleSimpleDataType"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:513:1: entryRuleSimpleDataType : ruleSimpleDataType EOF ;
public final void entryRuleSimpleDataType() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:514:1: ( ruleSimpleDataType EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:515:1: ruleSimpleDataType EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getSimpleDataTypeRule());
}
pushFollow(FollowSets000.FOLLOW_ruleSimpleDataType_in_entryRuleSimpleDataType1031);
ruleSimpleDataType();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getSimpleDataTypeRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleSimpleDataType1038); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleSimpleDataType"
// $ANTLR start "ruleSimpleDataType"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:522:1: ruleSimpleDataType : ( ( rule__SimpleDataType__Alternatives ) ) ;
public final void ruleSimpleDataType() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:526:2: ( ( ( rule__SimpleDataType__Alternatives ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:527:1: ( ( rule__SimpleDataType__Alternatives ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:527:1: ( ( rule__SimpleDataType__Alternatives ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:528:1: ( rule__SimpleDataType__Alternatives )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getSimpleDataTypeAccess().getAlternatives());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:529:1: ( rule__SimpleDataType__Alternatives )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:529:2: rule__SimpleDataType__Alternatives
{
pushFollow(FollowSets000.FOLLOW_rule__SimpleDataType__Alternatives_in_ruleSimpleDataType1064);
rule__SimpleDataType__Alternatives();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getSimpleDataTypeAccess().getAlternatives());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleSimpleDataType"
// $ANTLR start "entryRuleEntityAttribute"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:541:1: entryRuleEntityAttribute : ruleEntityAttribute EOF ;
public final void entryRuleEntityAttribute() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:542:1: ( ruleEntityAttribute EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:543:1: ruleEntityAttribute EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEntityAttributeRule());
}
pushFollow(FollowSets000.FOLLOW_ruleEntityAttribute_in_entryRuleEntityAttribute1091);
ruleEntityAttribute();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getEntityAttributeRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleEntityAttribute1098); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleEntityAttribute"
// $ANTLR start "ruleEntityAttribute"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:550:1: ruleEntityAttribute : ( ( rule__EntityAttribute__Alternatives ) ) ;
public final void ruleEntityAttribute() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:554:2: ( ( ( rule__EntityAttribute__Alternatives ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:555:1: ( ( rule__EntityAttribute__Alternatives ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:555:1: ( ( rule__EntityAttribute__Alternatives ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:556:1: ( rule__EntityAttribute__Alternatives )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEntityAttributeAccess().getAlternatives());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:557:1: ( rule__EntityAttribute__Alternatives )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:557:2: rule__EntityAttribute__Alternatives
{
pushFollow(FollowSets000.FOLLOW_rule__EntityAttribute__Alternatives_in_ruleEntityAttribute1124);
rule__EntityAttribute__Alternatives();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getEntityAttributeAccess().getAlternatives());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleEntityAttribute"
// $ANTLR start "entryRuleSimpleDatatypeEntityAttribute"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:569:1: entryRuleSimpleDatatypeEntityAttribute : ruleSimpleDatatypeEntityAttribute EOF ;
public final void entryRuleSimpleDatatypeEntityAttribute() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:570:1: ( ruleSimpleDatatypeEntityAttribute EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:571:1: ruleSimpleDatatypeEntityAttribute EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getSimpleDatatypeEntityAttributeRule());
}
pushFollow(FollowSets000.FOLLOW_ruleSimpleDatatypeEntityAttribute_in_entryRuleSimpleDatatypeEntityAttribute1151);
ruleSimpleDatatypeEntityAttribute();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getSimpleDatatypeEntityAttributeRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleSimpleDatatypeEntityAttribute1158); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleSimpleDatatypeEntityAttribute"
// $ANTLR start "ruleSimpleDatatypeEntityAttribute"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:578:1: ruleSimpleDatatypeEntityAttribute : ( ( rule__SimpleDatatypeEntityAttribute__Alternatives ) ) ;
public final void ruleSimpleDatatypeEntityAttribute() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:582:2: ( ( ( rule__SimpleDatatypeEntityAttribute__Alternatives ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:583:1: ( ( rule__SimpleDatatypeEntityAttribute__Alternatives ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:583:1: ( ( rule__SimpleDatatypeEntityAttribute__Alternatives ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:584:1: ( rule__SimpleDatatypeEntityAttribute__Alternatives )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getSimpleDatatypeEntityAttributeAccess().getAlternatives());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:585:1: ( rule__SimpleDatatypeEntityAttribute__Alternatives )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:585:2: rule__SimpleDatatypeEntityAttribute__Alternatives
{
pushFollow(FollowSets000.FOLLOW_rule__SimpleDatatypeEntityAttribute__Alternatives_in_ruleSimpleDatatypeEntityAttribute1184);
rule__SimpleDatatypeEntityAttribute__Alternatives();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getSimpleDatatypeEntityAttributeAccess().getAlternatives());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleSimpleDatatypeEntityAttribute"
// $ANTLR start "entryRuleBaseDataType"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:599:1: entryRuleBaseDataType : ruleBaseDataType EOF ;
public final void entryRuleBaseDataType() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:600:1: ( ruleBaseDataType EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:601:1: ruleBaseDataType EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getBaseDataTypeRule());
}
pushFollow(FollowSets000.FOLLOW_ruleBaseDataType_in_entryRuleBaseDataType1213);
ruleBaseDataType();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getBaseDataTypeRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleBaseDataType1220); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleBaseDataType"
// $ANTLR start "ruleBaseDataType"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:608:1: ruleBaseDataType : ( ( rule__BaseDataType__Group__0 ) ) ;
public final void ruleBaseDataType() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:612:2: ( ( ( rule__BaseDataType__Group__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:613:1: ( ( rule__BaseDataType__Group__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:613:1: ( ( rule__BaseDataType__Group__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:614:1: ( rule__BaseDataType__Group__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getBaseDataTypeAccess().getGroup());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:615:1: ( rule__BaseDataType__Group__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:615:2: rule__BaseDataType__Group__0
{
pushFollow(FollowSets000.FOLLOW_rule__BaseDataType__Group__0_in_ruleBaseDataType1246);
rule__BaseDataType__Group__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getBaseDataTypeAccess().getGroup());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleBaseDataType"
// $ANTLR start "entryRuleBaseDataTypeProperties"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:627:1: entryRuleBaseDataTypeProperties : ruleBaseDataTypeProperties EOF ;
public final void entryRuleBaseDataTypeProperties() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:628:1: ( ruleBaseDataTypeProperties EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:629:1: ruleBaseDataTypeProperties EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getBaseDataTypePropertiesRule());
}
pushFollow(FollowSets000.FOLLOW_ruleBaseDataTypeProperties_in_entryRuleBaseDataTypeProperties1273);
ruleBaseDataTypeProperties();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getBaseDataTypePropertiesRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleBaseDataTypeProperties1280); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleBaseDataTypeProperties"
// $ANTLR start "ruleBaseDataTypeProperties"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:636:1: ruleBaseDataTypeProperties : ( ( rule__BaseDataTypeProperties__Alternatives ) ) ;
public final void ruleBaseDataTypeProperties() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:640:2: ( ( ( rule__BaseDataTypeProperties__Alternatives ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:641:1: ( ( rule__BaseDataTypeProperties__Alternatives ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:641:1: ( ( rule__BaseDataTypeProperties__Alternatives ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:642:1: ( rule__BaseDataTypeProperties__Alternatives )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getBaseDataTypePropertiesAccess().getAlternatives());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:643:1: ( rule__BaseDataTypeProperties__Alternatives )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:643:2: rule__BaseDataTypeProperties__Alternatives
{
pushFollow(FollowSets000.FOLLOW_rule__BaseDataTypeProperties__Alternatives_in_ruleBaseDataTypeProperties1306);
rule__BaseDataTypeProperties__Alternatives();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getBaseDataTypePropertiesAccess().getAlternatives());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleBaseDataTypeProperties"
// $ANTLR start "entryRuleBaseDataTypeWidth"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:655:1: entryRuleBaseDataTypeWidth : ruleBaseDataTypeWidth EOF ;
public final void entryRuleBaseDataTypeWidth() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:656:1: ( ruleBaseDataTypeWidth EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:657:1: ruleBaseDataTypeWidth EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getBaseDataTypeWidthRule());
}
pushFollow(FollowSets000.FOLLOW_ruleBaseDataTypeWidth_in_entryRuleBaseDataTypeWidth1333);
ruleBaseDataTypeWidth();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getBaseDataTypeWidthRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleBaseDataTypeWidth1340); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleBaseDataTypeWidth"
// $ANTLR start "ruleBaseDataTypeWidth"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:664:1: ruleBaseDataTypeWidth : ( ( rule__BaseDataTypeWidth__Group__0 ) ) ;
public final void ruleBaseDataTypeWidth() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:668:2: ( ( ( rule__BaseDataTypeWidth__Group__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:669:1: ( ( rule__BaseDataTypeWidth__Group__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:669:1: ( ( rule__BaseDataTypeWidth__Group__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:670:1: ( rule__BaseDataTypeWidth__Group__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getBaseDataTypeWidthAccess().getGroup());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:671:1: ( rule__BaseDataTypeWidth__Group__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:671:2: rule__BaseDataTypeWidth__Group__0
{
pushFollow(FollowSets000.FOLLOW_rule__BaseDataTypeWidth__Group__0_in_ruleBaseDataTypeWidth1366);
rule__BaseDataTypeWidth__Group__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getBaseDataTypeWidthAccess().getGroup());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleBaseDataTypeWidth"
// $ANTLR start "entryRuleBaseDataTypeLabel"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:683:1: entryRuleBaseDataTypeLabel : ruleBaseDataTypeLabel EOF ;
public final void entryRuleBaseDataTypeLabel() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:684:1: ( ruleBaseDataTypeLabel EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:685:1: ruleBaseDataTypeLabel EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getBaseDataTypeLabelRule());
}
pushFollow(FollowSets000.FOLLOW_ruleBaseDataTypeLabel_in_entryRuleBaseDataTypeLabel1393);
ruleBaseDataTypeLabel();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getBaseDataTypeLabelRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleBaseDataTypeLabel1400); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleBaseDataTypeLabel"
// $ANTLR start "ruleBaseDataTypeLabel"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:692:1: ruleBaseDataTypeLabel : ( ( rule__BaseDataTypeLabel__Group__0 ) ) ;
public final void ruleBaseDataTypeLabel() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:696:2: ( ( ( rule__BaseDataTypeLabel__Group__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:697:1: ( ( rule__BaseDataTypeLabel__Group__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:697:1: ( ( rule__BaseDataTypeLabel__Group__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:698:1: ( rule__BaseDataTypeLabel__Group__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getBaseDataTypeLabelAccess().getGroup());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:699:1: ( rule__BaseDataTypeLabel__Group__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:699:2: rule__BaseDataTypeLabel__Group__0
{
pushFollow(FollowSets000.FOLLOW_rule__BaseDataTypeLabel__Group__0_in_ruleBaseDataTypeLabel1426);
rule__BaseDataTypeLabel__Group__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getBaseDataTypeLabelAccess().getGroup());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleBaseDataTypeLabel"
// $ANTLR start "entryRuleStringDataType"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:711:1: entryRuleStringDataType : ruleStringDataType EOF ;
public final void entryRuleStringDataType() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:712:1: ( ruleStringDataType EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:713:1: ruleStringDataType EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getStringDataTypeRule());
}
pushFollow(FollowSets000.FOLLOW_ruleStringDataType_in_entryRuleStringDataType1453);
ruleStringDataType();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getStringDataTypeRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleStringDataType1460); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleStringDataType"
// $ANTLR start "ruleStringDataType"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:720:1: ruleStringDataType : ( ( rule__StringDataType__Group__0 ) ) ;
public final void ruleStringDataType() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:724:2: ( ( ( rule__StringDataType__Group__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:725:1: ( ( rule__StringDataType__Group__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:725:1: ( ( rule__StringDataType__Group__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:726:1: ( rule__StringDataType__Group__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getStringDataTypeAccess().getGroup());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:727:1: ( rule__StringDataType__Group__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:727:2: rule__StringDataType__Group__0
{
pushFollow(FollowSets000.FOLLOW_rule__StringDataType__Group__0_in_ruleStringDataType1486);
rule__StringDataType__Group__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getStringDataTypeAccess().getGroup());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleStringDataType"
// $ANTLR start "entryRuleStringEntityAttribute"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:739:1: entryRuleStringEntityAttribute : ruleStringEntityAttribute EOF ;
public final void entryRuleStringEntityAttribute() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:740:1: ( ruleStringEntityAttribute EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:741:1: ruleStringEntityAttribute EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getStringEntityAttributeRule());
}
pushFollow(FollowSets000.FOLLOW_ruleStringEntityAttribute_in_entryRuleStringEntityAttribute1513);
ruleStringEntityAttribute();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getStringEntityAttributeRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleStringEntityAttribute1520); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleStringEntityAttribute"
// $ANTLR start "ruleStringEntityAttribute"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:748:1: ruleStringEntityAttribute : ( ( rule__StringEntityAttribute__Group__0 ) ) ;
public final void ruleStringEntityAttribute() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:752:2: ( ( ( rule__StringEntityAttribute__Group__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:753:1: ( ( rule__StringEntityAttribute__Group__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:753:1: ( ( rule__StringEntityAttribute__Group__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:754:1: ( rule__StringEntityAttribute__Group__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getStringEntityAttributeAccess().getGroup());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:755:1: ( rule__StringEntityAttribute__Group__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:755:2: rule__StringEntityAttribute__Group__0
{
pushFollow(FollowSets000.FOLLOW_rule__StringEntityAttribute__Group__0_in_ruleStringEntityAttribute1546);
rule__StringEntityAttribute__Group__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getStringEntityAttributeAccess().getGroup());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleStringEntityAttribute"
// $ANTLR start "entryRuleMapEntityAttribute"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:769:1: entryRuleMapEntityAttribute : ruleMapEntityAttribute EOF ;
public final void entryRuleMapEntityAttribute() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:770:1: ( ruleMapEntityAttribute EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:771:1: ruleMapEntityAttribute EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getMapEntityAttributeRule());
}
pushFollow(FollowSets000.FOLLOW_ruleMapEntityAttribute_in_entryRuleMapEntityAttribute1575);
ruleMapEntityAttribute();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getMapEntityAttributeRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleMapEntityAttribute1582); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleMapEntityAttribute"
// $ANTLR start "ruleMapEntityAttribute"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:778:1: ruleMapEntityAttribute : ( ( rule__MapEntityAttribute__Group__0 ) ) ;
public final void ruleMapEntityAttribute() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:782:2: ( ( ( rule__MapEntityAttribute__Group__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:783:1: ( ( rule__MapEntityAttribute__Group__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:783:1: ( ( rule__MapEntityAttribute__Group__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:784:1: ( rule__MapEntityAttribute__Group__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getMapEntityAttributeAccess().getGroup());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:785:1: ( rule__MapEntityAttribute__Group__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:785:2: rule__MapEntityAttribute__Group__0
{
pushFollow(FollowSets000.FOLLOW_rule__MapEntityAttribute__Group__0_in_ruleMapEntityAttribute1608);
rule__MapEntityAttribute__Group__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getMapEntityAttributeAccess().getGroup());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleMapEntityAttribute"
// $ANTLR start "entryRuleBooleanDataType"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:797:1: entryRuleBooleanDataType : ruleBooleanDataType EOF ;
public final void entryRuleBooleanDataType() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:798:1: ( ruleBooleanDataType EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:799:1: ruleBooleanDataType EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getBooleanDataTypeRule());
}
pushFollow(FollowSets000.FOLLOW_ruleBooleanDataType_in_entryRuleBooleanDataType1635);
ruleBooleanDataType();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getBooleanDataTypeRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleBooleanDataType1642); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleBooleanDataType"
// $ANTLR start "ruleBooleanDataType"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:806:1: ruleBooleanDataType : ( ( rule__BooleanDataType__Group__0 ) ) ;
public final void ruleBooleanDataType() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:810:2: ( ( ( rule__BooleanDataType__Group__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:811:1: ( ( rule__BooleanDataType__Group__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:811:1: ( ( rule__BooleanDataType__Group__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:812:1: ( rule__BooleanDataType__Group__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getBooleanDataTypeAccess().getGroup());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:813:1: ( rule__BooleanDataType__Group__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:813:2: rule__BooleanDataType__Group__0
{
pushFollow(FollowSets000.FOLLOW_rule__BooleanDataType__Group__0_in_ruleBooleanDataType1668);
rule__BooleanDataType__Group__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getBooleanDataTypeAccess().getGroup());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleBooleanDataType"
// $ANTLR start "entryRuleBooleanEntityAttribute"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:825:1: entryRuleBooleanEntityAttribute : ruleBooleanEntityAttribute EOF ;
public final void entryRuleBooleanEntityAttribute() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:826:1: ( ruleBooleanEntityAttribute EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:827:1: ruleBooleanEntityAttribute EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getBooleanEntityAttributeRule());
}
pushFollow(FollowSets000.FOLLOW_ruleBooleanEntityAttribute_in_entryRuleBooleanEntityAttribute1695);
ruleBooleanEntityAttribute();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getBooleanEntityAttributeRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleBooleanEntityAttribute1702); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleBooleanEntityAttribute"
// $ANTLR start "ruleBooleanEntityAttribute"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:834:1: ruleBooleanEntityAttribute : ( ( rule__BooleanEntityAttribute__Group__0 ) ) ;
public final void ruleBooleanEntityAttribute() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:838:2: ( ( ( rule__BooleanEntityAttribute__Group__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:839:1: ( ( rule__BooleanEntityAttribute__Group__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:839:1: ( ( rule__BooleanEntityAttribute__Group__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:840:1: ( rule__BooleanEntityAttribute__Group__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getBooleanEntityAttributeAccess().getGroup());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:841:1: ( rule__BooleanEntityAttribute__Group__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:841:2: rule__BooleanEntityAttribute__Group__0
{
pushFollow(FollowSets000.FOLLOW_rule__BooleanEntityAttribute__Group__0_in_ruleBooleanEntityAttribute1728);
rule__BooleanEntityAttribute__Group__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getBooleanEntityAttributeAccess().getGroup());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleBooleanEntityAttribute"
// $ANTLR start "entryRuleIntegerDataType"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:853:1: entryRuleIntegerDataType : ruleIntegerDataType EOF ;
public final void entryRuleIntegerDataType() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:854:1: ( ruleIntegerDataType EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:855:1: ruleIntegerDataType EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getIntegerDataTypeRule());
}
pushFollow(FollowSets000.FOLLOW_ruleIntegerDataType_in_entryRuleIntegerDataType1755);
ruleIntegerDataType();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getIntegerDataTypeRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleIntegerDataType1762); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleIntegerDataType"
// $ANTLR start "ruleIntegerDataType"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:862:1: ruleIntegerDataType : ( ( rule__IntegerDataType__Group__0 ) ) ;
public final void ruleIntegerDataType() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:866:2: ( ( ( rule__IntegerDataType__Group__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:867:1: ( ( rule__IntegerDataType__Group__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:867:1: ( ( rule__IntegerDataType__Group__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:868:1: ( rule__IntegerDataType__Group__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getIntegerDataTypeAccess().getGroup());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:869:1: ( rule__IntegerDataType__Group__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:869:2: rule__IntegerDataType__Group__0
{
pushFollow(FollowSets000.FOLLOW_rule__IntegerDataType__Group__0_in_ruleIntegerDataType1788);
rule__IntegerDataType__Group__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getIntegerDataTypeAccess().getGroup());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleIntegerDataType"
// $ANTLR start "entryRuleIntegerEntityAttribute"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:881:1: entryRuleIntegerEntityAttribute : ruleIntegerEntityAttribute EOF ;
public final void entryRuleIntegerEntityAttribute() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:882:1: ( ruleIntegerEntityAttribute EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:883:1: ruleIntegerEntityAttribute EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getIntegerEntityAttributeRule());
}
pushFollow(FollowSets000.FOLLOW_ruleIntegerEntityAttribute_in_entryRuleIntegerEntityAttribute1815);
ruleIntegerEntityAttribute();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getIntegerEntityAttributeRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleIntegerEntityAttribute1822); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleIntegerEntityAttribute"
// $ANTLR start "ruleIntegerEntityAttribute"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:890:1: ruleIntegerEntityAttribute : ( ( rule__IntegerEntityAttribute__Group__0 ) ) ;
public final void ruleIntegerEntityAttribute() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:894:2: ( ( ( rule__IntegerEntityAttribute__Group__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:895:1: ( ( rule__IntegerEntityAttribute__Group__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:895:1: ( ( rule__IntegerEntityAttribute__Group__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:896:1: ( rule__IntegerEntityAttribute__Group__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getIntegerEntityAttributeAccess().getGroup());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:897:1: ( rule__IntegerEntityAttribute__Group__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:897:2: rule__IntegerEntityAttribute__Group__0
{
pushFollow(FollowSets000.FOLLOW_rule__IntegerEntityAttribute__Group__0_in_ruleIntegerEntityAttribute1848);
rule__IntegerEntityAttribute__Group__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getIntegerEntityAttributeAccess().getGroup());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleIntegerEntityAttribute"
// $ANTLR start "entryRuleDateDataType"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:909:1: entryRuleDateDataType : ruleDateDataType EOF ;
public final void entryRuleDateDataType() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:910:1: ( ruleDateDataType EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:911:1: ruleDateDataType EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDateDataTypeRule());
}
pushFollow(FollowSets000.FOLLOW_ruleDateDataType_in_entryRuleDateDataType1875);
ruleDateDataType();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDateDataTypeRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleDateDataType1882); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleDateDataType"
// $ANTLR start "ruleDateDataType"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:918:1: ruleDateDataType : ( ( rule__DateDataType__Group__0 ) ) ;
public final void ruleDateDataType() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:922:2: ( ( ( rule__DateDataType__Group__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:923:1: ( ( rule__DateDataType__Group__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:923:1: ( ( rule__DateDataType__Group__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:924:1: ( rule__DateDataType__Group__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDateDataTypeAccess().getGroup());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:925:1: ( rule__DateDataType__Group__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:925:2: rule__DateDataType__Group__0
{
pushFollow(FollowSets000.FOLLOW_rule__DateDataType__Group__0_in_ruleDateDataType1908);
rule__DateDataType__Group__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDateDataTypeAccess().getGroup());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleDateDataType"
// $ANTLR start "entryRuleDateEntityAttribute"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:937:1: entryRuleDateEntityAttribute : ruleDateEntityAttribute EOF ;
public final void entryRuleDateEntityAttribute() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:938:1: ( ruleDateEntityAttribute EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:939:1: ruleDateEntityAttribute EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDateEntityAttributeRule());
}
pushFollow(FollowSets000.FOLLOW_ruleDateEntityAttribute_in_entryRuleDateEntityAttribute1935);
ruleDateEntityAttribute();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDateEntityAttributeRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleDateEntityAttribute1942); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleDateEntityAttribute"
// $ANTLR start "ruleDateEntityAttribute"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:946:1: ruleDateEntityAttribute : ( ( rule__DateEntityAttribute__Group__0 ) ) ;
public final void ruleDateEntityAttribute() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:950:2: ( ( ( rule__DateEntityAttribute__Group__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:951:1: ( ( rule__DateEntityAttribute__Group__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:951:1: ( ( rule__DateEntityAttribute__Group__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:952:1: ( rule__DateEntityAttribute__Group__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDateEntityAttributeAccess().getGroup());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:953:1: ( rule__DateEntityAttribute__Group__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:953:2: rule__DateEntityAttribute__Group__0
{
pushFollow(FollowSets000.FOLLOW_rule__DateEntityAttribute__Group__0_in_ruleDateEntityAttribute1968);
rule__DateEntityAttribute__Group__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDateEntityAttributeAccess().getGroup());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleDateEntityAttribute"
// $ANTLR start "entryRuleDecimalDataType"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:965:1: entryRuleDecimalDataType : ruleDecimalDataType EOF ;
public final void entryRuleDecimalDataType() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:966:1: ( ruleDecimalDataType EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:967:1: ruleDecimalDataType EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDecimalDataTypeRule());
}
pushFollow(FollowSets000.FOLLOW_ruleDecimalDataType_in_entryRuleDecimalDataType1995);
ruleDecimalDataType();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDecimalDataTypeRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleDecimalDataType2002); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleDecimalDataType"
// $ANTLR start "ruleDecimalDataType"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:974:1: ruleDecimalDataType : ( ( rule__DecimalDataType__Group__0 ) ) ;
public final void ruleDecimalDataType() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:978:2: ( ( ( rule__DecimalDataType__Group__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:979:1: ( ( rule__DecimalDataType__Group__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:979:1: ( ( rule__DecimalDataType__Group__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:980:1: ( rule__DecimalDataType__Group__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDecimalDataTypeAccess().getGroup());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:981:1: ( rule__DecimalDataType__Group__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:981:2: rule__DecimalDataType__Group__0
{
pushFollow(FollowSets000.FOLLOW_rule__DecimalDataType__Group__0_in_ruleDecimalDataType2028);
rule__DecimalDataType__Group__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDecimalDataTypeAccess().getGroup());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleDecimalDataType"
// $ANTLR start "entryRuleDecimalEntityAttribute"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:993:1: entryRuleDecimalEntityAttribute : ruleDecimalEntityAttribute EOF ;
public final void entryRuleDecimalEntityAttribute() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:994:1: ( ruleDecimalEntityAttribute EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:995:1: ruleDecimalEntityAttribute EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDecimalEntityAttributeRule());
}
pushFollow(FollowSets000.FOLLOW_ruleDecimalEntityAttribute_in_entryRuleDecimalEntityAttribute2055);
ruleDecimalEntityAttribute();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDecimalEntityAttributeRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleDecimalEntityAttribute2062); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleDecimalEntityAttribute"
// $ANTLR start "ruleDecimalEntityAttribute"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1002:1: ruleDecimalEntityAttribute : ( ( rule__DecimalEntityAttribute__Group__0 ) ) ;
public final void ruleDecimalEntityAttribute() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1006:2: ( ( ( rule__DecimalEntityAttribute__Group__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1007:1: ( ( rule__DecimalEntityAttribute__Group__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1007:1: ( ( rule__DecimalEntityAttribute__Group__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1008:1: ( rule__DecimalEntityAttribute__Group__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDecimalEntityAttributeAccess().getGroup());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1009:1: ( rule__DecimalEntityAttribute__Group__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1009:2: rule__DecimalEntityAttribute__Group__0
{
pushFollow(FollowSets000.FOLLOW_rule__DecimalEntityAttribute__Group__0_in_ruleDecimalEntityAttribute2088);
rule__DecimalEntityAttribute__Group__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDecimalEntityAttributeAccess().getGroup());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleDecimalEntityAttribute"
// $ANTLR start "entryRuleLongDataType"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1021:1: entryRuleLongDataType : ruleLongDataType EOF ;
public final void entryRuleLongDataType() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1022:1: ( ruleLongDataType EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1023:1: ruleLongDataType EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getLongDataTypeRule());
}
pushFollow(FollowSets000.FOLLOW_ruleLongDataType_in_entryRuleLongDataType2115);
ruleLongDataType();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getLongDataTypeRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleLongDataType2122); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleLongDataType"
// $ANTLR start "ruleLongDataType"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1030:1: ruleLongDataType : ( ( rule__LongDataType__Group__0 ) ) ;
public final void ruleLongDataType() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1034:2: ( ( ( rule__LongDataType__Group__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1035:1: ( ( rule__LongDataType__Group__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1035:1: ( ( rule__LongDataType__Group__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1036:1: ( rule__LongDataType__Group__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getLongDataTypeAccess().getGroup());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1037:1: ( rule__LongDataType__Group__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1037:2: rule__LongDataType__Group__0
{
pushFollow(FollowSets000.FOLLOW_rule__LongDataType__Group__0_in_ruleLongDataType2148);
rule__LongDataType__Group__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getLongDataTypeAccess().getGroup());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleLongDataType"
// $ANTLR start "entryRuleLongEntityAttribute"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1049:1: entryRuleLongEntityAttribute : ruleLongEntityAttribute EOF ;
public final void entryRuleLongEntityAttribute() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1050:1: ( ruleLongEntityAttribute EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1051:1: ruleLongEntityAttribute EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getLongEntityAttributeRule());
}
pushFollow(FollowSets000.FOLLOW_ruleLongEntityAttribute_in_entryRuleLongEntityAttribute2175);
ruleLongEntityAttribute();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getLongEntityAttributeRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleLongEntityAttribute2182); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleLongEntityAttribute"
// $ANTLR start "ruleLongEntityAttribute"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1058:1: ruleLongEntityAttribute : ( ( rule__LongEntityAttribute__Group__0 ) ) ;
public final void ruleLongEntityAttribute() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1062:2: ( ( ( rule__LongEntityAttribute__Group__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1063:1: ( ( rule__LongEntityAttribute__Group__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1063:1: ( ( rule__LongEntityAttribute__Group__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1064:1: ( rule__LongEntityAttribute__Group__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getLongEntityAttributeAccess().getGroup());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1065:1: ( rule__LongEntityAttribute__Group__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1065:2: rule__LongEntityAttribute__Group__0
{
pushFollow(FollowSets000.FOLLOW_rule__LongEntityAttribute__Group__0_in_ruleLongEntityAttribute2208);
rule__LongEntityAttribute__Group__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getLongEntityAttributeAccess().getGroup());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleLongEntityAttribute"
// $ANTLR start "entryRuleFloatDataType"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1077:1: entryRuleFloatDataType : ruleFloatDataType EOF ;
public final void entryRuleFloatDataType() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1078:1: ( ruleFloatDataType EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1079:1: ruleFloatDataType EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getFloatDataTypeRule());
}
pushFollow(FollowSets000.FOLLOW_ruleFloatDataType_in_entryRuleFloatDataType2235);
ruleFloatDataType();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getFloatDataTypeRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleFloatDataType2242); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleFloatDataType"
// $ANTLR start "ruleFloatDataType"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1086:1: ruleFloatDataType : ( ( rule__FloatDataType__Group__0 ) ) ;
public final void ruleFloatDataType() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1090:2: ( ( ( rule__FloatDataType__Group__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1091:1: ( ( rule__FloatDataType__Group__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1091:1: ( ( rule__FloatDataType__Group__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1092:1: ( rule__FloatDataType__Group__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getFloatDataTypeAccess().getGroup());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1093:1: ( rule__FloatDataType__Group__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1093:2: rule__FloatDataType__Group__0
{
pushFollow(FollowSets000.FOLLOW_rule__FloatDataType__Group__0_in_ruleFloatDataType2268);
rule__FloatDataType__Group__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getFloatDataTypeAccess().getGroup());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleFloatDataType"
// $ANTLR start "entryRuleFloatEntityAttribute"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1105:1: entryRuleFloatEntityAttribute : ruleFloatEntityAttribute EOF ;
public final void entryRuleFloatEntityAttribute() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1106:1: ( ruleFloatEntityAttribute EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1107:1: ruleFloatEntityAttribute EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getFloatEntityAttributeRule());
}
pushFollow(FollowSets000.FOLLOW_ruleFloatEntityAttribute_in_entryRuleFloatEntityAttribute2295);
ruleFloatEntityAttribute();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getFloatEntityAttributeRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleFloatEntityAttribute2302); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleFloatEntityAttribute"
// $ANTLR start "ruleFloatEntityAttribute"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1114:1: ruleFloatEntityAttribute : ( ( rule__FloatEntityAttribute__Group__0 ) ) ;
public final void ruleFloatEntityAttribute() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1118:2: ( ( ( rule__FloatEntityAttribute__Group__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1119:1: ( ( rule__FloatEntityAttribute__Group__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1119:1: ( ( rule__FloatEntityAttribute__Group__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1120:1: ( rule__FloatEntityAttribute__Group__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getFloatEntityAttributeAccess().getGroup());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1121:1: ( rule__FloatEntityAttribute__Group__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1121:2: rule__FloatEntityAttribute__Group__0
{
pushFollow(FollowSets000.FOLLOW_rule__FloatEntityAttribute__Group__0_in_ruleFloatEntityAttribute2328);
rule__FloatEntityAttribute__Group__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getFloatEntityAttributeAccess().getGroup());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleFloatEntityAttribute"
// $ANTLR start "entryRuleDoubleDataType"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1133:1: entryRuleDoubleDataType : ruleDoubleDataType EOF ;
public final void entryRuleDoubleDataType() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1134:1: ( ruleDoubleDataType EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1135:1: ruleDoubleDataType EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDoubleDataTypeRule());
}
pushFollow(FollowSets000.FOLLOW_ruleDoubleDataType_in_entryRuleDoubleDataType2355);
ruleDoubleDataType();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDoubleDataTypeRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleDoubleDataType2362); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleDoubleDataType"
// $ANTLR start "ruleDoubleDataType"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1142:1: ruleDoubleDataType : ( ( rule__DoubleDataType__Group__0 ) ) ;
public final void ruleDoubleDataType() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1146:2: ( ( ( rule__DoubleDataType__Group__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1147:1: ( ( rule__DoubleDataType__Group__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1147:1: ( ( rule__DoubleDataType__Group__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1148:1: ( rule__DoubleDataType__Group__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDoubleDataTypeAccess().getGroup());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1149:1: ( rule__DoubleDataType__Group__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1149:2: rule__DoubleDataType__Group__0
{
pushFollow(FollowSets000.FOLLOW_rule__DoubleDataType__Group__0_in_ruleDoubleDataType2388);
rule__DoubleDataType__Group__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDoubleDataTypeAccess().getGroup());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleDoubleDataType"
// $ANTLR start "entryRuleDoubleEntityAttribute"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1161:1: entryRuleDoubleEntityAttribute : ruleDoubleEntityAttribute EOF ;
public final void entryRuleDoubleEntityAttribute() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1162:1: ( ruleDoubleEntityAttribute EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1163:1: ruleDoubleEntityAttribute EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDoubleEntityAttributeRule());
}
pushFollow(FollowSets000.FOLLOW_ruleDoubleEntityAttribute_in_entryRuleDoubleEntityAttribute2415);
ruleDoubleEntityAttribute();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDoubleEntityAttributeRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleDoubleEntityAttribute2422); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleDoubleEntityAttribute"
// $ANTLR start "ruleDoubleEntityAttribute"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1170:1: ruleDoubleEntityAttribute : ( ( rule__DoubleEntityAttribute__Group__0 ) ) ;
public final void ruleDoubleEntityAttribute() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1174:2: ( ( ( rule__DoubleEntityAttribute__Group__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1175:1: ( ( rule__DoubleEntityAttribute__Group__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1175:1: ( ( rule__DoubleEntityAttribute__Group__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1176:1: ( rule__DoubleEntityAttribute__Group__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDoubleEntityAttributeAccess().getGroup());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1177:1: ( rule__DoubleEntityAttribute__Group__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1177:2: rule__DoubleEntityAttribute__Group__0
{
pushFollow(FollowSets000.FOLLOW_rule__DoubleEntityAttribute__Group__0_in_ruleDoubleEntityAttribute2448);
rule__DoubleEntityAttribute__Group__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDoubleEntityAttributeAccess().getGroup());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleDoubleEntityAttribute"
// $ANTLR start "entryRuleBinaryDataType"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1189:1: entryRuleBinaryDataType : ruleBinaryDataType EOF ;
public final void entryRuleBinaryDataType() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1190:1: ( ruleBinaryDataType EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1191:1: ruleBinaryDataType EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getBinaryDataTypeRule());
}
pushFollow(FollowSets000.FOLLOW_ruleBinaryDataType_in_entryRuleBinaryDataType2475);
ruleBinaryDataType();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getBinaryDataTypeRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleBinaryDataType2482); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleBinaryDataType"
// $ANTLR start "ruleBinaryDataType"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1198:1: ruleBinaryDataType : ( ( rule__BinaryDataType__Group__0 ) ) ;
public final void ruleBinaryDataType() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1202:2: ( ( ( rule__BinaryDataType__Group__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1203:1: ( ( rule__BinaryDataType__Group__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1203:1: ( ( rule__BinaryDataType__Group__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1204:1: ( rule__BinaryDataType__Group__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getBinaryDataTypeAccess().getGroup());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1205:1: ( rule__BinaryDataType__Group__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1205:2: rule__BinaryDataType__Group__0
{
pushFollow(FollowSets000.FOLLOW_rule__BinaryDataType__Group__0_in_ruleBinaryDataType2508);
rule__BinaryDataType__Group__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getBinaryDataTypeAccess().getGroup());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleBinaryDataType"
// $ANTLR start "entryRuleBinaryEntityAttribute"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1217:1: entryRuleBinaryEntityAttribute : ruleBinaryEntityAttribute EOF ;
public final void entryRuleBinaryEntityAttribute() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1218:1: ( ruleBinaryEntityAttribute EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1219:1: ruleBinaryEntityAttribute EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getBinaryEntityAttributeRule());
}
pushFollow(FollowSets000.FOLLOW_ruleBinaryEntityAttribute_in_entryRuleBinaryEntityAttribute2535);
ruleBinaryEntityAttribute();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getBinaryEntityAttributeRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleBinaryEntityAttribute2542); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleBinaryEntityAttribute"
// $ANTLR start "ruleBinaryEntityAttribute"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1226:1: ruleBinaryEntityAttribute : ( ( rule__BinaryEntityAttribute__Group__0 ) ) ;
public final void ruleBinaryEntityAttribute() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1230:2: ( ( ( rule__BinaryEntityAttribute__Group__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1231:1: ( ( rule__BinaryEntityAttribute__Group__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1231:1: ( ( rule__BinaryEntityAttribute__Group__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1232:1: ( rule__BinaryEntityAttribute__Group__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getBinaryEntityAttributeAccess().getGroup());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1233:1: ( rule__BinaryEntityAttribute__Group__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1233:2: rule__BinaryEntityAttribute__Group__0
{
pushFollow(FollowSets000.FOLLOW_rule__BinaryEntityAttribute__Group__0_in_ruleBinaryEntityAttribute2568);
rule__BinaryEntityAttribute__Group__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getBinaryEntityAttributeAccess().getGroup());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleBinaryEntityAttribute"
// $ANTLR start "entryRuleEntityDataType"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1245:1: entryRuleEntityDataType : ruleEntityDataType EOF ;
public final void entryRuleEntityDataType() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1246:1: ( ruleEntityDataType EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1247:1: ruleEntityDataType EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEntityDataTypeRule());
}
pushFollow(FollowSets000.FOLLOW_ruleEntityDataType_in_entryRuleEntityDataType2595);
ruleEntityDataType();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getEntityDataTypeRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleEntityDataType2602); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleEntityDataType"
// $ANTLR start "ruleEntityDataType"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1254:1: ruleEntityDataType : ( ( rule__EntityDataType__Group__0 ) ) ;
public final void ruleEntityDataType() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1258:2: ( ( ( rule__EntityDataType__Group__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1259:1: ( ( rule__EntityDataType__Group__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1259:1: ( ( rule__EntityDataType__Group__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1260:1: ( rule__EntityDataType__Group__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEntityDataTypeAccess().getGroup());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1261:1: ( rule__EntityDataType__Group__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1261:2: rule__EntityDataType__Group__0
{
pushFollow(FollowSets000.FOLLOW_rule__EntityDataType__Group__0_in_ruleEntityDataType2628);
rule__EntityDataType__Group__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getEntityDataTypeAccess().getGroup());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleEntityDataType"
// $ANTLR start "entryRuleEntityEntityAttribute"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1275:1: entryRuleEntityEntityAttribute : ruleEntityEntityAttribute EOF ;
public final void entryRuleEntityEntityAttribute() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1276:1: ( ruleEntityEntityAttribute EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1277:1: ruleEntityEntityAttribute EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEntityEntityAttributeRule());
}
pushFollow(FollowSets000.FOLLOW_ruleEntityEntityAttribute_in_entryRuleEntityEntityAttribute2657);
ruleEntityEntityAttribute();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getEntityEntityAttributeRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleEntityEntityAttribute2664); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleEntityEntityAttribute"
// $ANTLR start "ruleEntityEntityAttribute"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1284:1: ruleEntityEntityAttribute : ( ( rule__EntityEntityAttribute__Group__0 ) ) ;
public final void ruleEntityEntityAttribute() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1288:2: ( ( ( rule__EntityEntityAttribute__Group__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1289:1: ( ( rule__EntityEntityAttribute__Group__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1289:1: ( ( rule__EntityEntityAttribute__Group__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1290:1: ( rule__EntityEntityAttribute__Group__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEntityEntityAttributeAccess().getGroup());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1291:1: ( rule__EntityEntityAttribute__Group__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1291:2: rule__EntityEntityAttribute__Group__0
{
pushFollow(FollowSets000.FOLLOW_rule__EntityEntityAttribute__Group__0_in_ruleEntityEntityAttribute2690);
rule__EntityEntityAttribute__Group__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getEntityEntityAttributeAccess().getGroup());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleEntityEntityAttribute"
// $ANTLR start "entryRuleValueObjectEntityAttribute"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1303:1: entryRuleValueObjectEntityAttribute : ruleValueObjectEntityAttribute EOF ;
public final void entryRuleValueObjectEntityAttribute() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1304:1: ( ruleValueObjectEntityAttribute EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1305:1: ruleValueObjectEntityAttribute EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getValueObjectEntityAttributeRule());
}
pushFollow(FollowSets000.FOLLOW_ruleValueObjectEntityAttribute_in_entryRuleValueObjectEntityAttribute2717);
ruleValueObjectEntityAttribute();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getValueObjectEntityAttributeRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleValueObjectEntityAttribute2724); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleValueObjectEntityAttribute"
// $ANTLR start "ruleValueObjectEntityAttribute"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1312:1: ruleValueObjectEntityAttribute : ( ( rule__ValueObjectEntityAttribute__Group__0 ) ) ;
public final void ruleValueObjectEntityAttribute() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1316:2: ( ( ( rule__ValueObjectEntityAttribute__Group__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1317:1: ( ( rule__ValueObjectEntityAttribute__Group__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1317:1: ( ( rule__ValueObjectEntityAttribute__Group__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1318:1: ( rule__ValueObjectEntityAttribute__Group__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getValueObjectEntityAttributeAccess().getGroup());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1319:1: ( rule__ValueObjectEntityAttribute__Group__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1319:2: rule__ValueObjectEntityAttribute__Group__0
{
pushFollow(FollowSets000.FOLLOW_rule__ValueObjectEntityAttribute__Group__0_in_ruleValueObjectEntityAttribute2750);
rule__ValueObjectEntityAttribute__Group__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getValueObjectEntityAttributeAccess().getGroup());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleValueObjectEntityAttribute"
// $ANTLR start "entryRuleEnumerationDataType"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1331:1: entryRuleEnumerationDataType : ruleEnumerationDataType EOF ;
public final void entryRuleEnumerationDataType() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1332:1: ( ruleEnumerationDataType EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1333:1: ruleEnumerationDataType EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEnumerationDataTypeRule());
}
pushFollow(FollowSets000.FOLLOW_ruleEnumerationDataType_in_entryRuleEnumerationDataType2777);
ruleEnumerationDataType();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getEnumerationDataTypeRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleEnumerationDataType2784); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleEnumerationDataType"
// $ANTLR start "ruleEnumerationDataType"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1340:1: ruleEnumerationDataType : ( ( rule__EnumerationDataType__Group__0 ) ) ;
public final void ruleEnumerationDataType() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1344:2: ( ( ( rule__EnumerationDataType__Group__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1345:1: ( ( rule__EnumerationDataType__Group__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1345:1: ( ( rule__EnumerationDataType__Group__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1346:1: ( rule__EnumerationDataType__Group__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEnumerationDataTypeAccess().getGroup());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1347:1: ( rule__EnumerationDataType__Group__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1347:2: rule__EnumerationDataType__Group__0
{
pushFollow(FollowSets000.FOLLOW_rule__EnumerationDataType__Group__0_in_ruleEnumerationDataType2810);
rule__EnumerationDataType__Group__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getEnumerationDataTypeAccess().getGroup());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleEnumerationDataType"
// $ANTLR start "entryRuleEnumerationEntityAttribute"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1361:1: entryRuleEnumerationEntityAttribute : ruleEnumerationEntityAttribute EOF ;
public final void entryRuleEnumerationEntityAttribute() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1362:1: ( ruleEnumerationEntityAttribute EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1363:1: ruleEnumerationEntityAttribute EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEnumerationEntityAttributeRule());
}
pushFollow(FollowSets000.FOLLOW_ruleEnumerationEntityAttribute_in_entryRuleEnumerationEntityAttribute2839);
ruleEnumerationEntityAttribute();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getEnumerationEntityAttributeRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleEnumerationEntityAttribute2846); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleEnumerationEntityAttribute"
// $ANTLR start "ruleEnumerationEntityAttribute"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1370:1: ruleEnumerationEntityAttribute : ( ( rule__EnumerationEntityAttribute__Group__0 ) ) ;
public final void ruleEnumerationEntityAttribute() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1374:2: ( ( ( rule__EnumerationEntityAttribute__Group__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1375:1: ( ( rule__EnumerationEntityAttribute__Group__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1375:1: ( ( rule__EnumerationEntityAttribute__Group__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1376:1: ( rule__EnumerationEntityAttribute__Group__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEnumerationEntityAttributeAccess().getGroup());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1377:1: ( rule__EnumerationEntityAttribute__Group__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1377:2: rule__EnumerationEntityAttribute__Group__0
{
pushFollow(FollowSets000.FOLLOW_rule__EnumerationEntityAttribute__Group__0_in_ruleEnumerationEntityAttribute2872);
rule__EnumerationEntityAttribute__Group__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getEnumerationEntityAttributeAccess().getGroup());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleEnumerationEntityAttribute"
// $ANTLR start "entryRuleDictionary"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1389:1: entryRuleDictionary : ruleDictionary EOF ;
public final void entryRuleDictionary() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1390:1: ( ruleDictionary EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1391:1: ruleDictionary EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryRule());
}
pushFollow(FollowSets000.FOLLOW_ruleDictionary_in_entryRuleDictionary2899);
ruleDictionary();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleDictionary2906); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleDictionary"
// $ANTLR start "ruleDictionary"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1398:1: ruleDictionary : ( ( rule__Dictionary__Group__0 ) ) ;
public final void ruleDictionary() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1402:2: ( ( ( rule__Dictionary__Group__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1403:1: ( ( rule__Dictionary__Group__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1403:1: ( ( rule__Dictionary__Group__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1404:1: ( rule__Dictionary__Group__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryAccess().getGroup());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1405:1: ( rule__Dictionary__Group__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1405:2: rule__Dictionary__Group__0
{
pushFollow(FollowSets000.FOLLOW_rule__Dictionary__Group__0_in_ruleDictionary2932);
rule__Dictionary__Group__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryAccess().getGroup());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleDictionary"
// $ANTLR start "entryRuleDictionarySearch"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1417:1: entryRuleDictionarySearch : ruleDictionarySearch EOF ;
public final void entryRuleDictionarySearch() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1418:1: ( ruleDictionarySearch EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1419:1: ruleDictionarySearch EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionarySearchRule());
}
pushFollow(FollowSets000.FOLLOW_ruleDictionarySearch_in_entryRuleDictionarySearch2959);
ruleDictionarySearch();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionarySearchRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleDictionarySearch2966); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleDictionarySearch"
// $ANTLR start "ruleDictionarySearch"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1426:1: ruleDictionarySearch : ( ( rule__DictionarySearch__Group__0 ) ) ;
public final void ruleDictionarySearch() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1430:2: ( ( ( rule__DictionarySearch__Group__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1431:1: ( ( rule__DictionarySearch__Group__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1431:1: ( ( rule__DictionarySearch__Group__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1432:1: ( rule__DictionarySearch__Group__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionarySearchAccess().getGroup());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1433:1: ( rule__DictionarySearch__Group__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1433:2: rule__DictionarySearch__Group__0
{
pushFollow(FollowSets000.FOLLOW_rule__DictionarySearch__Group__0_in_ruleDictionarySearch2992);
rule__DictionarySearch__Group__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionarySearchAccess().getGroup());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleDictionarySearch"
// $ANTLR start "entryRuleDictionaryEditor"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1445:1: entryRuleDictionaryEditor : ruleDictionaryEditor EOF ;
public final void entryRuleDictionaryEditor() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1446:1: ( ruleDictionaryEditor EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1447:1: ruleDictionaryEditor EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryEditorRule());
}
pushFollow(FollowSets000.FOLLOW_ruleDictionaryEditor_in_entryRuleDictionaryEditor3019);
ruleDictionaryEditor();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryEditorRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleDictionaryEditor3026); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleDictionaryEditor"
// $ANTLR start "ruleDictionaryEditor"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1454:1: ruleDictionaryEditor : ( ( rule__DictionaryEditor__Group__0 ) ) ;
public final void ruleDictionaryEditor() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1458:2: ( ( ( rule__DictionaryEditor__Group__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1459:1: ( ( rule__DictionaryEditor__Group__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1459:1: ( ( rule__DictionaryEditor__Group__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1460:1: ( rule__DictionaryEditor__Group__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryEditorAccess().getGroup());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1461:1: ( rule__DictionaryEditor__Group__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1461:2: rule__DictionaryEditor__Group__0
{
pushFollow(FollowSets000.FOLLOW_rule__DictionaryEditor__Group__0_in_ruleDictionaryEditor3052);
rule__DictionaryEditor__Group__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryEditorAccess().getGroup());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleDictionaryEditor"
// $ANTLR start "entryRuleDictionaryFilter"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1473:1: entryRuleDictionaryFilter : ruleDictionaryFilter EOF ;
public final void entryRuleDictionaryFilter() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1474:1: ( ruleDictionaryFilter EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1475:1: ruleDictionaryFilter EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryFilterRule());
}
pushFollow(FollowSets000.FOLLOW_ruleDictionaryFilter_in_entryRuleDictionaryFilter3079);
ruleDictionaryFilter();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryFilterRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleDictionaryFilter3086); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleDictionaryFilter"
// $ANTLR start "ruleDictionaryFilter"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1482:1: ruleDictionaryFilter : ( ( rule__DictionaryFilter__Group__0 ) ) ;
public final void ruleDictionaryFilter() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1486:2: ( ( ( rule__DictionaryFilter__Group__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1487:1: ( ( rule__DictionaryFilter__Group__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1487:1: ( ( rule__DictionaryFilter__Group__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1488:1: ( rule__DictionaryFilter__Group__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryFilterAccess().getGroup());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1489:1: ( rule__DictionaryFilter__Group__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1489:2: rule__DictionaryFilter__Group__0
{
pushFollow(FollowSets000.FOLLOW_rule__DictionaryFilter__Group__0_in_ruleDictionaryFilter3112);
rule__DictionaryFilter__Group__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryFilterAccess().getGroup());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleDictionaryFilter"
// $ANTLR start "entryRuleDictionaryResult"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1501:1: entryRuleDictionaryResult : ruleDictionaryResult EOF ;
public final void entryRuleDictionaryResult() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1502:1: ( ruleDictionaryResult EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1503:1: ruleDictionaryResult EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryResultRule());
}
pushFollow(FollowSets000.FOLLOW_ruleDictionaryResult_in_entryRuleDictionaryResult3139);
ruleDictionaryResult();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryResultRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleDictionaryResult3146); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleDictionaryResult"
// $ANTLR start "ruleDictionaryResult"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1510:1: ruleDictionaryResult : ( ( rule__DictionaryResult__Group__0 ) ) ;
public final void ruleDictionaryResult() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1514:2: ( ( ( rule__DictionaryResult__Group__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1515:1: ( ( rule__DictionaryResult__Group__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1515:1: ( ( rule__DictionaryResult__Group__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1516:1: ( rule__DictionaryResult__Group__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryResultAccess().getGroup());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1517:1: ( rule__DictionaryResult__Group__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1517:2: rule__DictionaryResult__Group__0
{
pushFollow(FollowSets000.FOLLOW_rule__DictionaryResult__Group__0_in_ruleDictionaryResult3172);
rule__DictionaryResult__Group__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryResultAccess().getGroup());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleDictionaryResult"
// $ANTLR start "entryRuleColumnLayout"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1529:1: entryRuleColumnLayout : ruleColumnLayout EOF ;
public final void entryRuleColumnLayout() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1530:1: ( ruleColumnLayout EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1531:1: ruleColumnLayout EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getColumnLayoutRule());
}
pushFollow(FollowSets000.FOLLOW_ruleColumnLayout_in_entryRuleColumnLayout3199);
ruleColumnLayout();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getColumnLayoutRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleColumnLayout3206); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleColumnLayout"
// $ANTLR start "ruleColumnLayout"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1538:1: ruleColumnLayout : ( ( rule__ColumnLayout__Group__0 ) ) ;
public final void ruleColumnLayout() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1542:2: ( ( ( rule__ColumnLayout__Group__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1543:1: ( ( rule__ColumnLayout__Group__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1543:1: ( ( rule__ColumnLayout__Group__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1544:1: ( rule__ColumnLayout__Group__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getColumnLayoutAccess().getGroup());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1545:1: ( rule__ColumnLayout__Group__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1545:2: rule__ColumnLayout__Group__0
{
pushFollow(FollowSets000.FOLLOW_rule__ColumnLayout__Group__0_in_ruleColumnLayout3232);
rule__ColumnLayout__Group__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getColumnLayoutAccess().getGroup());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleColumnLayout"
// $ANTLR start "entryRuleColumnLayoutData"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1557:1: entryRuleColumnLayoutData : ruleColumnLayoutData EOF ;
public final void entryRuleColumnLayoutData() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1558:1: ( ruleColumnLayoutData EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1559:1: ruleColumnLayoutData EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getColumnLayoutDataRule());
}
pushFollow(FollowSets000.FOLLOW_ruleColumnLayoutData_in_entryRuleColumnLayoutData3259);
ruleColumnLayoutData();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getColumnLayoutDataRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleColumnLayoutData3266); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleColumnLayoutData"
// $ANTLR start "ruleColumnLayoutData"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1566:1: ruleColumnLayoutData : ( ( rule__ColumnLayoutData__Group__0 ) ) ;
public final void ruleColumnLayoutData() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1570:2: ( ( ( rule__ColumnLayoutData__Group__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1571:1: ( ( rule__ColumnLayoutData__Group__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1571:1: ( ( rule__ColumnLayoutData__Group__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1572:1: ( rule__ColumnLayoutData__Group__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getColumnLayoutDataAccess().getGroup());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1573:1: ( rule__ColumnLayoutData__Group__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1573:2: rule__ColumnLayoutData__Group__0
{
pushFollow(FollowSets000.FOLLOW_rule__ColumnLayoutData__Group__0_in_ruleColumnLayoutData3292);
rule__ColumnLayoutData__Group__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getColumnLayoutDataAccess().getGroup());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleColumnLayoutData"
// $ANTLR start "entryRuleDictionaryContainer"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1585:1: entryRuleDictionaryContainer : ruleDictionaryContainer EOF ;
public final void entryRuleDictionaryContainer() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1586:1: ( ruleDictionaryContainer EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1587:1: ruleDictionaryContainer EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryContainerRule());
}
pushFollow(FollowSets000.FOLLOW_ruleDictionaryContainer_in_entryRuleDictionaryContainer3319);
ruleDictionaryContainer();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryContainerRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleDictionaryContainer3326); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleDictionaryContainer"
// $ANTLR start "ruleDictionaryContainer"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1594:1: ruleDictionaryContainer : ( ( rule__DictionaryContainer__Alternatives ) ) ;
public final void ruleDictionaryContainer() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1598:2: ( ( ( rule__DictionaryContainer__Alternatives ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1599:1: ( ( rule__DictionaryContainer__Alternatives ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1599:1: ( ( rule__DictionaryContainer__Alternatives ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1600:1: ( rule__DictionaryContainer__Alternatives )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryContainerAccess().getAlternatives());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1601:1: ( rule__DictionaryContainer__Alternatives )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1601:2: rule__DictionaryContainer__Alternatives
{
pushFollow(FollowSets000.FOLLOW_rule__DictionaryContainer__Alternatives_in_ruleDictionaryContainer3352);
rule__DictionaryContainer__Alternatives();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryContainerAccess().getAlternatives());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleDictionaryContainer"
// $ANTLR start "entryRuleDictionaryComposite"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1613:1: entryRuleDictionaryComposite : ruleDictionaryComposite EOF ;
public final void entryRuleDictionaryComposite() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1614:1: ( ruleDictionaryComposite EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1615:1: ruleDictionaryComposite EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryCompositeRule());
}
pushFollow(FollowSets000.FOLLOW_ruleDictionaryComposite_in_entryRuleDictionaryComposite3379);
ruleDictionaryComposite();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryCompositeRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleDictionaryComposite3386); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleDictionaryComposite"
// $ANTLR start "ruleDictionaryComposite"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1622:1: ruleDictionaryComposite : ( ( rule__DictionaryComposite__Group__0 ) ) ;
public final void ruleDictionaryComposite() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1626:2: ( ( ( rule__DictionaryComposite__Group__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1627:1: ( ( rule__DictionaryComposite__Group__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1627:1: ( ( rule__DictionaryComposite__Group__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1628:1: ( rule__DictionaryComposite__Group__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryCompositeAccess().getGroup());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1629:1: ( rule__DictionaryComposite__Group__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1629:2: rule__DictionaryComposite__Group__0
{
pushFollow(FollowSets000.FOLLOW_rule__DictionaryComposite__Group__0_in_ruleDictionaryComposite3412);
rule__DictionaryComposite__Group__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryCompositeAccess().getGroup());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleDictionaryComposite"
// $ANTLR start "entryRuleDictionaryEditableTable"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1641:1: entryRuleDictionaryEditableTable : ruleDictionaryEditableTable EOF ;
public final void entryRuleDictionaryEditableTable() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1642:1: ( ruleDictionaryEditableTable EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1643:1: ruleDictionaryEditableTable EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryEditableTableRule());
}
pushFollow(FollowSets000.FOLLOW_ruleDictionaryEditableTable_in_entryRuleDictionaryEditableTable3439);
ruleDictionaryEditableTable();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryEditableTableRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleDictionaryEditableTable3446); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleDictionaryEditableTable"
// $ANTLR start "ruleDictionaryEditableTable"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1650:1: ruleDictionaryEditableTable : ( ( rule__DictionaryEditableTable__Group__0 ) ) ;
public final void ruleDictionaryEditableTable() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1654:2: ( ( ( rule__DictionaryEditableTable__Group__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1655:1: ( ( rule__DictionaryEditableTable__Group__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1655:1: ( ( rule__DictionaryEditableTable__Group__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1656:1: ( rule__DictionaryEditableTable__Group__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryEditableTableAccess().getGroup());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1657:1: ( rule__DictionaryEditableTable__Group__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1657:2: rule__DictionaryEditableTable__Group__0
{
pushFollow(FollowSets000.FOLLOW_rule__DictionaryEditableTable__Group__0_in_ruleDictionaryEditableTable3472);
rule__DictionaryEditableTable__Group__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryEditableTableAccess().getGroup());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleDictionaryEditableTable"
// $ANTLR start "entryRuleDictionaryAssignmentTable"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1669:1: entryRuleDictionaryAssignmentTable : ruleDictionaryAssignmentTable EOF ;
public final void entryRuleDictionaryAssignmentTable() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1670:1: ( ruleDictionaryAssignmentTable EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1671:1: ruleDictionaryAssignmentTable EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryAssignmentTableRule());
}
pushFollow(FollowSets000.FOLLOW_ruleDictionaryAssignmentTable_in_entryRuleDictionaryAssignmentTable3499);
ruleDictionaryAssignmentTable();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryAssignmentTableRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleDictionaryAssignmentTable3506); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleDictionaryAssignmentTable"
// $ANTLR start "ruleDictionaryAssignmentTable"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1678:1: ruleDictionaryAssignmentTable : ( ( rule__DictionaryAssignmentTable__Group__0 ) ) ;
public final void ruleDictionaryAssignmentTable() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1682:2: ( ( ( rule__DictionaryAssignmentTable__Group__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1683:1: ( ( rule__DictionaryAssignmentTable__Group__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1683:1: ( ( rule__DictionaryAssignmentTable__Group__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1684:1: ( rule__DictionaryAssignmentTable__Group__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryAssignmentTableAccess().getGroup());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1685:1: ( rule__DictionaryAssignmentTable__Group__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1685:2: rule__DictionaryAssignmentTable__Group__0
{
pushFollow(FollowSets000.FOLLOW_rule__DictionaryAssignmentTable__Group__0_in_ruleDictionaryAssignmentTable3532);
rule__DictionaryAssignmentTable__Group__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryAssignmentTableAccess().getGroup());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleDictionaryAssignmentTable"
// $ANTLR start "entryRuleDictionaryContainerContent"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1697:1: entryRuleDictionaryContainerContent : ruleDictionaryContainerContent EOF ;
public final void entryRuleDictionaryContainerContent() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1698:1: ( ruleDictionaryContainerContent EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1699:1: ruleDictionaryContainerContent EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryContainerContentRule());
}
pushFollow(FollowSets000.FOLLOW_ruleDictionaryContainerContent_in_entryRuleDictionaryContainerContent3559);
ruleDictionaryContainerContent();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryContainerContentRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleDictionaryContainerContent3566); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleDictionaryContainerContent"
// $ANTLR start "ruleDictionaryContainerContent"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1706:1: ruleDictionaryContainerContent : ( ( rule__DictionaryContainerContent__Alternatives ) ) ;
public final void ruleDictionaryContainerContent() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1710:2: ( ( ( rule__DictionaryContainerContent__Alternatives ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1711:1: ( ( rule__DictionaryContainerContent__Alternatives ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1711:1: ( ( rule__DictionaryContainerContent__Alternatives ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1712:1: ( rule__DictionaryContainerContent__Alternatives )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryContainerContentAccess().getAlternatives());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1713:1: ( rule__DictionaryContainerContent__Alternatives )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1713:2: rule__DictionaryContainerContent__Alternatives
{
pushFollow(FollowSets000.FOLLOW_rule__DictionaryContainerContent__Alternatives_in_ruleDictionaryContainerContent3592);
rule__DictionaryContainerContent__Alternatives();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryContainerContentAccess().getAlternatives());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleDictionaryContainerContent"
// $ANTLR start "entryRuleLabels"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1725:1: entryRuleLabels : ruleLabels EOF ;
public final void entryRuleLabels() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1726:1: ( ruleLabels EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1727:1: ruleLabels EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getLabelsRule());
}
pushFollow(FollowSets000.FOLLOW_ruleLabels_in_entryRuleLabels3619);
ruleLabels();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getLabelsRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleLabels3626); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleLabels"
// $ANTLR start "ruleLabels"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1734:1: ruleLabels : ( ( rule__Labels__Group__0 ) ) ;
public final void ruleLabels() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1738:2: ( ( ( rule__Labels__Group__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1739:1: ( ( rule__Labels__Group__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1739:1: ( ( rule__Labels__Group__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1740:1: ( rule__Labels__Group__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getLabelsAccess().getGroup());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1741:1: ( rule__Labels__Group__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1741:2: rule__Labels__Group__0
{
pushFollow(FollowSets000.FOLLOW_rule__Labels__Group__0_in_ruleLabels3652);
rule__Labels__Group__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getLabelsAccess().getGroup());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleLabels"
// $ANTLR start "entryRuleBaseDictionaryControl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1753:1: entryRuleBaseDictionaryControl : ruleBaseDictionaryControl EOF ;
public final void entryRuleBaseDictionaryControl() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1754:1: ( ruleBaseDictionaryControl EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1755:1: ruleBaseDictionaryControl EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getBaseDictionaryControlRule());
}
pushFollow(FollowSets000.FOLLOW_ruleBaseDictionaryControl_in_entryRuleBaseDictionaryControl3679);
ruleBaseDictionaryControl();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getBaseDictionaryControlRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleBaseDictionaryControl3686); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleBaseDictionaryControl"
// $ANTLR start "ruleBaseDictionaryControl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1762:1: ruleBaseDictionaryControl : ( ( rule__BaseDictionaryControl__Group__0 ) ) ;
public final void ruleBaseDictionaryControl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1766:2: ( ( ( rule__BaseDictionaryControl__Group__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1767:1: ( ( rule__BaseDictionaryControl__Group__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1767:1: ( ( rule__BaseDictionaryControl__Group__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1768:1: ( rule__BaseDictionaryControl__Group__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getBaseDictionaryControlAccess().getGroup());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1769:1: ( rule__BaseDictionaryControl__Group__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1769:2: rule__BaseDictionaryControl__Group__0
{
pushFollow(FollowSets000.FOLLOW_rule__BaseDictionaryControl__Group__0_in_ruleBaseDictionaryControl3712);
rule__BaseDictionaryControl__Group__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getBaseDictionaryControlAccess().getGroup());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleBaseDictionaryControl"
// $ANTLR start "entryRuleDictionaryControl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1781:1: entryRuleDictionaryControl : ruleDictionaryControl EOF ;
public final void entryRuleDictionaryControl() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1782:1: ( ruleDictionaryControl EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1783:1: ruleDictionaryControl EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryControlRule());
}
pushFollow(FollowSets000.FOLLOW_ruleDictionaryControl_in_entryRuleDictionaryControl3739);
ruleDictionaryControl();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryControlRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleDictionaryControl3746); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleDictionaryControl"
// $ANTLR start "ruleDictionaryControl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1790:1: ruleDictionaryControl : ( ( rule__DictionaryControl__Alternatives ) ) ;
public final void ruleDictionaryControl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1794:2: ( ( ( rule__DictionaryControl__Alternatives ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1795:1: ( ( rule__DictionaryControl__Alternatives ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1795:1: ( ( rule__DictionaryControl__Alternatives ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1796:1: ( rule__DictionaryControl__Alternatives )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryControlAccess().getAlternatives());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1797:1: ( rule__DictionaryControl__Alternatives )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1797:2: rule__DictionaryControl__Alternatives
{
pushFollow(FollowSets000.FOLLOW_rule__DictionaryControl__Alternatives_in_ruleDictionaryControl3772);
rule__DictionaryControl__Alternatives();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryControlAccess().getAlternatives());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleDictionaryControl"
// $ANTLR start "entryRuleDictionaryControlGroupOptions"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1809:1: entryRuleDictionaryControlGroupOptions : ruleDictionaryControlGroupOptions EOF ;
public final void entryRuleDictionaryControlGroupOptions() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1810:1: ( ruleDictionaryControlGroupOptions EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1811:1: ruleDictionaryControlGroupOptions EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryControlGroupOptionsRule());
}
pushFollow(FollowSets000.FOLLOW_ruleDictionaryControlGroupOptions_in_entryRuleDictionaryControlGroupOptions3799);
ruleDictionaryControlGroupOptions();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryControlGroupOptionsRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleDictionaryControlGroupOptions3806); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleDictionaryControlGroupOptions"
// $ANTLR start "ruleDictionaryControlGroupOptions"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1818:1: ruleDictionaryControlGroupOptions : ( ruleDictionaryControlGroupOptionMultiFilterField ) ;
public final void ruleDictionaryControlGroupOptions() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1822:2: ( ( ruleDictionaryControlGroupOptionMultiFilterField ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1823:1: ( ruleDictionaryControlGroupOptionMultiFilterField )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1823:1: ( ruleDictionaryControlGroupOptionMultiFilterField )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1824:1: ruleDictionaryControlGroupOptionMultiFilterField
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryControlGroupOptionsAccess().getDictionaryControlGroupOptionMultiFilterFieldParserRuleCall());
}
pushFollow(FollowSets000.FOLLOW_ruleDictionaryControlGroupOptionMultiFilterField_in_ruleDictionaryControlGroupOptions3832);
ruleDictionaryControlGroupOptionMultiFilterField();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryControlGroupOptionsAccess().getDictionaryControlGroupOptionMultiFilterFieldParserRuleCall());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleDictionaryControlGroupOptions"
// $ANTLR start "entryRuleDictionaryControlGroupOptionMultiFilterField"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1837:1: entryRuleDictionaryControlGroupOptionMultiFilterField : ruleDictionaryControlGroupOptionMultiFilterField EOF ;
public final void entryRuleDictionaryControlGroupOptionMultiFilterField() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1838:1: ( ruleDictionaryControlGroupOptionMultiFilterField EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1839:1: ruleDictionaryControlGroupOptionMultiFilterField EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryControlGroupOptionMultiFilterFieldRule());
}
pushFollow(FollowSets000.FOLLOW_ruleDictionaryControlGroupOptionMultiFilterField_in_entryRuleDictionaryControlGroupOptionMultiFilterField3858);
ruleDictionaryControlGroupOptionMultiFilterField();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryControlGroupOptionMultiFilterFieldRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleDictionaryControlGroupOptionMultiFilterField3865); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleDictionaryControlGroupOptionMultiFilterField"
// $ANTLR start "ruleDictionaryControlGroupOptionMultiFilterField"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1846:1: ruleDictionaryControlGroupOptionMultiFilterField : ( ( rule__DictionaryControlGroupOptionMultiFilterField__Group__0 ) ) ;
public final void ruleDictionaryControlGroupOptionMultiFilterField() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1850:2: ( ( ( rule__DictionaryControlGroupOptionMultiFilterField__Group__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1851:1: ( ( rule__DictionaryControlGroupOptionMultiFilterField__Group__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1851:1: ( ( rule__DictionaryControlGroupOptionMultiFilterField__Group__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1852:1: ( rule__DictionaryControlGroupOptionMultiFilterField__Group__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryControlGroupOptionMultiFilterFieldAccess().getGroup());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1853:1: ( rule__DictionaryControlGroupOptionMultiFilterField__Group__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1853:2: rule__DictionaryControlGroupOptionMultiFilterField__Group__0
{
pushFollow(FollowSets000.FOLLOW_rule__DictionaryControlGroupOptionMultiFilterField__Group__0_in_ruleDictionaryControlGroupOptionMultiFilterField3891);
rule__DictionaryControlGroupOptionMultiFilterField__Group__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryControlGroupOptionMultiFilterFieldAccess().getGroup());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleDictionaryControlGroupOptionMultiFilterField"
// $ANTLR start "entryRuleDictionaryControlGroupOptionsContainer"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1865:1: entryRuleDictionaryControlGroupOptionsContainer : ruleDictionaryControlGroupOptionsContainer EOF ;
public final void entryRuleDictionaryControlGroupOptionsContainer() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1866:1: ( ruleDictionaryControlGroupOptionsContainer EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1867:1: ruleDictionaryControlGroupOptionsContainer EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryControlGroupOptionsContainerRule());
}
pushFollow(FollowSets000.FOLLOW_ruleDictionaryControlGroupOptionsContainer_in_entryRuleDictionaryControlGroupOptionsContainer3918);
ruleDictionaryControlGroupOptionsContainer();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryControlGroupOptionsContainerRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleDictionaryControlGroupOptionsContainer3925); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleDictionaryControlGroupOptionsContainer"
// $ANTLR start "ruleDictionaryControlGroupOptionsContainer"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1874:1: ruleDictionaryControlGroupOptionsContainer : ( ( rule__DictionaryControlGroupOptionsContainer__Group__0 ) ) ;
public final void ruleDictionaryControlGroupOptionsContainer() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1878:2: ( ( ( rule__DictionaryControlGroupOptionsContainer__Group__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1879:1: ( ( rule__DictionaryControlGroupOptionsContainer__Group__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1879:1: ( ( rule__DictionaryControlGroupOptionsContainer__Group__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1880:1: ( rule__DictionaryControlGroupOptionsContainer__Group__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryControlGroupOptionsContainerAccess().getGroup());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1881:1: ( rule__DictionaryControlGroupOptionsContainer__Group__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1881:2: rule__DictionaryControlGroupOptionsContainer__Group__0
{
pushFollow(FollowSets000.FOLLOW_rule__DictionaryControlGroupOptionsContainer__Group__0_in_ruleDictionaryControlGroupOptionsContainer3951);
rule__DictionaryControlGroupOptionsContainer__Group__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryControlGroupOptionsContainerAccess().getGroup());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleDictionaryControlGroupOptionsContainer"
// $ANTLR start "entryRuleDictionaryControlGroup"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1893:1: entryRuleDictionaryControlGroup : ruleDictionaryControlGroup EOF ;
public final void entryRuleDictionaryControlGroup() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1894:1: ( ruleDictionaryControlGroup EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1895:1: ruleDictionaryControlGroup EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryControlGroupRule());
}
pushFollow(FollowSets000.FOLLOW_ruleDictionaryControlGroup_in_entryRuleDictionaryControlGroup3978);
ruleDictionaryControlGroup();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryControlGroupRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleDictionaryControlGroup3985); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleDictionaryControlGroup"
// $ANTLR start "ruleDictionaryControlGroup"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1902:1: ruleDictionaryControlGroup : ( ( rule__DictionaryControlGroup__Group__0 ) ) ;
public final void ruleDictionaryControlGroup() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1906:2: ( ( ( rule__DictionaryControlGroup__Group__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1907:1: ( ( rule__DictionaryControlGroup__Group__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1907:1: ( ( rule__DictionaryControlGroup__Group__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1908:1: ( rule__DictionaryControlGroup__Group__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryControlGroupAccess().getGroup());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1909:1: ( rule__DictionaryControlGroup__Group__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1909:2: rule__DictionaryControlGroup__Group__0
{
pushFollow(FollowSets000.FOLLOW_rule__DictionaryControlGroup__Group__0_in_ruleDictionaryControlGroup4011);
rule__DictionaryControlGroup__Group__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryControlGroupAccess().getGroup());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleDictionaryControlGroup"
// $ANTLR start "entryRuleDictionaryHierarchicalControl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1921:1: entryRuleDictionaryHierarchicalControl : ruleDictionaryHierarchicalControl EOF ;
public final void entryRuleDictionaryHierarchicalControl() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1922:1: ( ruleDictionaryHierarchicalControl EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1923:1: ruleDictionaryHierarchicalControl EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryHierarchicalControlRule());
}
pushFollow(FollowSets000.FOLLOW_ruleDictionaryHierarchicalControl_in_entryRuleDictionaryHierarchicalControl4038);
ruleDictionaryHierarchicalControl();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryHierarchicalControlRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleDictionaryHierarchicalControl4045); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleDictionaryHierarchicalControl"
// $ANTLR start "ruleDictionaryHierarchicalControl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1930:1: ruleDictionaryHierarchicalControl : ( ( rule__DictionaryHierarchicalControl__Group__0 ) ) ;
public final void ruleDictionaryHierarchicalControl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1934:2: ( ( ( rule__DictionaryHierarchicalControl__Group__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1935:1: ( ( rule__DictionaryHierarchicalControl__Group__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1935:1: ( ( rule__DictionaryHierarchicalControl__Group__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1936:1: ( rule__DictionaryHierarchicalControl__Group__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryHierarchicalControlAccess().getGroup());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1937:1: ( rule__DictionaryHierarchicalControl__Group__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1937:2: rule__DictionaryHierarchicalControl__Group__0
{
pushFollow(FollowSets000.FOLLOW_rule__DictionaryHierarchicalControl__Group__0_in_ruleDictionaryHierarchicalControl4071);
rule__DictionaryHierarchicalControl__Group__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryHierarchicalControlAccess().getGroup());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleDictionaryHierarchicalControl"
// $ANTLR start "entryRuleDictionaryTextControl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1949:1: entryRuleDictionaryTextControl : ruleDictionaryTextControl EOF ;
public final void entryRuleDictionaryTextControl() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1950:1: ( ruleDictionaryTextControl EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1951:1: ruleDictionaryTextControl EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryTextControlRule());
}
pushFollow(FollowSets000.FOLLOW_ruleDictionaryTextControl_in_entryRuleDictionaryTextControl4098);
ruleDictionaryTextControl();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryTextControlRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleDictionaryTextControl4105); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleDictionaryTextControl"
// $ANTLR start "ruleDictionaryTextControl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1958:1: ruleDictionaryTextControl : ( ( rule__DictionaryTextControl__Group__0 ) ) ;
public final void ruleDictionaryTextControl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1962:2: ( ( ( rule__DictionaryTextControl__Group__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1963:1: ( ( rule__DictionaryTextControl__Group__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1963:1: ( ( rule__DictionaryTextControl__Group__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1964:1: ( rule__DictionaryTextControl__Group__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryTextControlAccess().getGroup());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1965:1: ( rule__DictionaryTextControl__Group__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1965:2: rule__DictionaryTextControl__Group__0
{
pushFollow(FollowSets000.FOLLOW_rule__DictionaryTextControl__Group__0_in_ruleDictionaryTextControl4131);
rule__DictionaryTextControl__Group__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryTextControlAccess().getGroup());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleDictionaryTextControl"
// $ANTLR start "entryRuleDictionaryIntegerControlInputType"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1977:1: entryRuleDictionaryIntegerControlInputType : ruleDictionaryIntegerControlInputType EOF ;
public final void entryRuleDictionaryIntegerControlInputType() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1978:1: ( ruleDictionaryIntegerControlInputType EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1979:1: ruleDictionaryIntegerControlInputType EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryIntegerControlInputTypeRule());
}
pushFollow(FollowSets000.FOLLOW_ruleDictionaryIntegerControlInputType_in_entryRuleDictionaryIntegerControlInputType4158);
ruleDictionaryIntegerControlInputType();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryIntegerControlInputTypeRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleDictionaryIntegerControlInputType4165); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleDictionaryIntegerControlInputType"
// $ANTLR start "ruleDictionaryIntegerControlInputType"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1986:1: ruleDictionaryIntegerControlInputType : ( ( rule__DictionaryIntegerControlInputType__Group__0 ) ) ;
public final void ruleDictionaryIntegerControlInputType() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1990:2: ( ( ( rule__DictionaryIntegerControlInputType__Group__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1991:1: ( ( rule__DictionaryIntegerControlInputType__Group__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1991:1: ( ( rule__DictionaryIntegerControlInputType__Group__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1992:1: ( rule__DictionaryIntegerControlInputType__Group__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryIntegerControlInputTypeAccess().getGroup());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1993:1: ( rule__DictionaryIntegerControlInputType__Group__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:1993:2: rule__DictionaryIntegerControlInputType__Group__0
{
pushFollow(FollowSets000.FOLLOW_rule__DictionaryIntegerControlInputType__Group__0_in_ruleDictionaryIntegerControlInputType4191);
rule__DictionaryIntegerControlInputType__Group__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryIntegerControlInputTypeAccess().getGroup());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleDictionaryIntegerControlInputType"
// $ANTLR start "entryRuleDictionaryIntegerControlOptions"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2005:1: entryRuleDictionaryIntegerControlOptions : ruleDictionaryIntegerControlOptions EOF ;
public final void entryRuleDictionaryIntegerControlOptions() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2006:1: ( ruleDictionaryIntegerControlOptions EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2007:1: ruleDictionaryIntegerControlOptions EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryIntegerControlOptionsRule());
}
pushFollow(FollowSets000.FOLLOW_ruleDictionaryIntegerControlOptions_in_entryRuleDictionaryIntegerControlOptions4218);
ruleDictionaryIntegerControlOptions();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryIntegerControlOptionsRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleDictionaryIntegerControlOptions4225); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleDictionaryIntegerControlOptions"
// $ANTLR start "ruleDictionaryIntegerControlOptions"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2014:1: ruleDictionaryIntegerControlOptions : ( ruleDictionaryIntegerControlInputType ) ;
public final void ruleDictionaryIntegerControlOptions() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2018:2: ( ( ruleDictionaryIntegerControlInputType ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2019:1: ( ruleDictionaryIntegerControlInputType )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2019:1: ( ruleDictionaryIntegerControlInputType )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2020:1: ruleDictionaryIntegerControlInputType
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryIntegerControlOptionsAccess().getDictionaryIntegerControlInputTypeParserRuleCall());
}
pushFollow(FollowSets000.FOLLOW_ruleDictionaryIntegerControlInputType_in_ruleDictionaryIntegerControlOptions4251);
ruleDictionaryIntegerControlInputType();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryIntegerControlOptionsAccess().getDictionaryIntegerControlInputTypeParserRuleCall());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleDictionaryIntegerControlOptions"
// $ANTLR start "entryRuleDictionaryIntegerControl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2033:1: entryRuleDictionaryIntegerControl : ruleDictionaryIntegerControl EOF ;
public final void entryRuleDictionaryIntegerControl() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2034:1: ( ruleDictionaryIntegerControl EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2035:1: ruleDictionaryIntegerControl EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryIntegerControlRule());
}
pushFollow(FollowSets000.FOLLOW_ruleDictionaryIntegerControl_in_entryRuleDictionaryIntegerControl4277);
ruleDictionaryIntegerControl();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryIntegerControlRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleDictionaryIntegerControl4284); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleDictionaryIntegerControl"
// $ANTLR start "ruleDictionaryIntegerControl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2042:1: ruleDictionaryIntegerControl : ( ( rule__DictionaryIntegerControl__Group__0 ) ) ;
public final void ruleDictionaryIntegerControl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2046:2: ( ( ( rule__DictionaryIntegerControl__Group__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2047:1: ( ( rule__DictionaryIntegerControl__Group__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2047:1: ( ( rule__DictionaryIntegerControl__Group__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2048:1: ( rule__DictionaryIntegerControl__Group__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryIntegerControlAccess().getGroup());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2049:1: ( rule__DictionaryIntegerControl__Group__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2049:2: rule__DictionaryIntegerControl__Group__0
{
pushFollow(FollowSets000.FOLLOW_rule__DictionaryIntegerControl__Group__0_in_ruleDictionaryIntegerControl4310);
rule__DictionaryIntegerControl__Group__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryIntegerControlAccess().getGroup());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleDictionaryIntegerControl"
// $ANTLR start "entryRuleDictionaryBigDecimalControl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2061:1: entryRuleDictionaryBigDecimalControl : ruleDictionaryBigDecimalControl EOF ;
public final void entryRuleDictionaryBigDecimalControl() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2062:1: ( ruleDictionaryBigDecimalControl EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2063:1: ruleDictionaryBigDecimalControl EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryBigDecimalControlRule());
}
pushFollow(FollowSets000.FOLLOW_ruleDictionaryBigDecimalControl_in_entryRuleDictionaryBigDecimalControl4337);
ruleDictionaryBigDecimalControl();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryBigDecimalControlRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleDictionaryBigDecimalControl4344); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleDictionaryBigDecimalControl"
// $ANTLR start "ruleDictionaryBigDecimalControl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2070:1: ruleDictionaryBigDecimalControl : ( ( rule__DictionaryBigDecimalControl__Group__0 ) ) ;
public final void ruleDictionaryBigDecimalControl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2074:2: ( ( ( rule__DictionaryBigDecimalControl__Group__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2075:1: ( ( rule__DictionaryBigDecimalControl__Group__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2075:1: ( ( rule__DictionaryBigDecimalControl__Group__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2076:1: ( rule__DictionaryBigDecimalControl__Group__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryBigDecimalControlAccess().getGroup());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2077:1: ( rule__DictionaryBigDecimalControl__Group__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2077:2: rule__DictionaryBigDecimalControl__Group__0
{
pushFollow(FollowSets000.FOLLOW_rule__DictionaryBigDecimalControl__Group__0_in_ruleDictionaryBigDecimalControl4370);
rule__DictionaryBigDecimalControl__Group__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryBigDecimalControlAccess().getGroup());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleDictionaryBigDecimalControl"
// $ANTLR start "entryRuleDictionaryBooleanControl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2089:1: entryRuleDictionaryBooleanControl : ruleDictionaryBooleanControl EOF ;
public final void entryRuleDictionaryBooleanControl() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2090:1: ( ruleDictionaryBooleanControl EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2091:1: ruleDictionaryBooleanControl EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryBooleanControlRule());
}
pushFollow(FollowSets000.FOLLOW_ruleDictionaryBooleanControl_in_entryRuleDictionaryBooleanControl4397);
ruleDictionaryBooleanControl();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryBooleanControlRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleDictionaryBooleanControl4404); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleDictionaryBooleanControl"
// $ANTLR start "ruleDictionaryBooleanControl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2098:1: ruleDictionaryBooleanControl : ( ( rule__DictionaryBooleanControl__Group__0 ) ) ;
public final void ruleDictionaryBooleanControl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2102:2: ( ( ( rule__DictionaryBooleanControl__Group__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2103:1: ( ( rule__DictionaryBooleanControl__Group__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2103:1: ( ( rule__DictionaryBooleanControl__Group__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2104:1: ( rule__DictionaryBooleanControl__Group__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryBooleanControlAccess().getGroup());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2105:1: ( rule__DictionaryBooleanControl__Group__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2105:2: rule__DictionaryBooleanControl__Group__0
{
pushFollow(FollowSets000.FOLLOW_rule__DictionaryBooleanControl__Group__0_in_ruleDictionaryBooleanControl4430);
rule__DictionaryBooleanControl__Group__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryBooleanControlAccess().getGroup());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleDictionaryBooleanControl"
// $ANTLR start "entryRuleDictionaryDateControl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2117:1: entryRuleDictionaryDateControl : ruleDictionaryDateControl EOF ;
public final void entryRuleDictionaryDateControl() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2118:1: ( ruleDictionaryDateControl EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2119:1: ruleDictionaryDateControl EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryDateControlRule());
}
pushFollow(FollowSets000.FOLLOW_ruleDictionaryDateControl_in_entryRuleDictionaryDateControl4457);
ruleDictionaryDateControl();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryDateControlRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleDictionaryDateControl4464); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleDictionaryDateControl"
// $ANTLR start "ruleDictionaryDateControl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2126:1: ruleDictionaryDateControl : ( ( rule__DictionaryDateControl__Group__0 ) ) ;
public final void ruleDictionaryDateControl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2130:2: ( ( ( rule__DictionaryDateControl__Group__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2131:1: ( ( rule__DictionaryDateControl__Group__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2131:1: ( ( rule__DictionaryDateControl__Group__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2132:1: ( rule__DictionaryDateControl__Group__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryDateControlAccess().getGroup());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2133:1: ( rule__DictionaryDateControl__Group__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2133:2: rule__DictionaryDateControl__Group__0
{
pushFollow(FollowSets000.FOLLOW_rule__DictionaryDateControl__Group__0_in_ruleDictionaryDateControl4490);
rule__DictionaryDateControl__Group__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryDateControlAccess().getGroup());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleDictionaryDateControl"
// $ANTLR start "entryRuleDictionaryEnumerationControl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2145:1: entryRuleDictionaryEnumerationControl : ruleDictionaryEnumerationControl EOF ;
public final void entryRuleDictionaryEnumerationControl() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2146:1: ( ruleDictionaryEnumerationControl EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2147:1: ruleDictionaryEnumerationControl EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryEnumerationControlRule());
}
pushFollow(FollowSets000.FOLLOW_ruleDictionaryEnumerationControl_in_entryRuleDictionaryEnumerationControl4517);
ruleDictionaryEnumerationControl();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryEnumerationControlRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleDictionaryEnumerationControl4524); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleDictionaryEnumerationControl"
// $ANTLR start "ruleDictionaryEnumerationControl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2154:1: ruleDictionaryEnumerationControl : ( ( rule__DictionaryEnumerationControl__Group__0 ) ) ;
public final void ruleDictionaryEnumerationControl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2158:2: ( ( ( rule__DictionaryEnumerationControl__Group__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2159:1: ( ( rule__DictionaryEnumerationControl__Group__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2159:1: ( ( rule__DictionaryEnumerationControl__Group__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2160:1: ( rule__DictionaryEnumerationControl__Group__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryEnumerationControlAccess().getGroup());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2161:1: ( rule__DictionaryEnumerationControl__Group__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2161:2: rule__DictionaryEnumerationControl__Group__0
{
pushFollow(FollowSets000.FOLLOW_rule__DictionaryEnumerationControl__Group__0_in_ruleDictionaryEnumerationControl4550);
rule__DictionaryEnumerationControl__Group__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryEnumerationControlAccess().getGroup());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleDictionaryEnumerationControl"
// $ANTLR start "entryRuleDictionaryReferenceControl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2173:1: entryRuleDictionaryReferenceControl : ruleDictionaryReferenceControl EOF ;
public final void entryRuleDictionaryReferenceControl() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2174:1: ( ruleDictionaryReferenceControl EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2175:1: ruleDictionaryReferenceControl EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryReferenceControlRule());
}
pushFollow(FollowSets000.FOLLOW_ruleDictionaryReferenceControl_in_entryRuleDictionaryReferenceControl4577);
ruleDictionaryReferenceControl();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryReferenceControlRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleDictionaryReferenceControl4584); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleDictionaryReferenceControl"
// $ANTLR start "ruleDictionaryReferenceControl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2182:1: ruleDictionaryReferenceControl : ( ( rule__DictionaryReferenceControl__Group__0 ) ) ;
public final void ruleDictionaryReferenceControl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2186:2: ( ( ( rule__DictionaryReferenceControl__Group__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2187:1: ( ( rule__DictionaryReferenceControl__Group__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2187:1: ( ( rule__DictionaryReferenceControl__Group__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2188:1: ( rule__DictionaryReferenceControl__Group__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryReferenceControlAccess().getGroup());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2189:1: ( rule__DictionaryReferenceControl__Group__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2189:2: rule__DictionaryReferenceControl__Group__0
{
pushFollow(FollowSets000.FOLLOW_rule__DictionaryReferenceControl__Group__0_in_ruleDictionaryReferenceControl4610);
rule__DictionaryReferenceControl__Group__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryReferenceControlAccess().getGroup());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleDictionaryReferenceControl"
// $ANTLR start "entryRuleDictionaryFileControl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2201:1: entryRuleDictionaryFileControl : ruleDictionaryFileControl EOF ;
public final void entryRuleDictionaryFileControl() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2202:1: ( ruleDictionaryFileControl EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2203:1: ruleDictionaryFileControl EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryFileControlRule());
}
pushFollow(FollowSets000.FOLLOW_ruleDictionaryFileControl_in_entryRuleDictionaryFileControl4637);
ruleDictionaryFileControl();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryFileControlRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleDictionaryFileControl4644); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleDictionaryFileControl"
// $ANTLR start "ruleDictionaryFileControl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2210:1: ruleDictionaryFileControl : ( ( rule__DictionaryFileControl__Group__0 ) ) ;
public final void ruleDictionaryFileControl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2214:2: ( ( ( rule__DictionaryFileControl__Group__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2215:1: ( ( rule__DictionaryFileControl__Group__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2215:1: ( ( rule__DictionaryFileControl__Group__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2216:1: ( rule__DictionaryFileControl__Group__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryFileControlAccess().getGroup());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2217:1: ( rule__DictionaryFileControl__Group__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2217:2: rule__DictionaryFileControl__Group__0
{
pushFollow(FollowSets000.FOLLOW_rule__DictionaryFileControl__Group__0_in_ruleDictionaryFileControl4670);
rule__DictionaryFileControl__Group__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryFileControlAccess().getGroup());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleDictionaryFileControl"
// $ANTLR start "entryRuleModule"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2229:1: entryRuleModule : ruleModule EOF ;
public final void entryRuleModule() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2230:1: ( ruleModule EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2231:1: ruleModule EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getModuleRule());
}
pushFollow(FollowSets000.FOLLOW_ruleModule_in_entryRuleModule4697);
ruleModule();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getModuleRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleModule4704); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleModule"
// $ANTLR start "ruleModule"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2238:1: ruleModule : ( ( rule__Module__Group__0 ) ) ;
public final void ruleModule() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2242:2: ( ( ( rule__Module__Group__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2243:1: ( ( rule__Module__Group__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2243:1: ( ( rule__Module__Group__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2244:1: ( rule__Module__Group__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getModuleAccess().getGroup());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2245:1: ( rule__Module__Group__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2245:2: rule__Module__Group__0
{
pushFollow(FollowSets000.FOLLOW_rule__Module__Group__0_in_ruleModule4730);
rule__Module__Group__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getModuleAccess().getGroup());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleModule"
// $ANTLR start "entryRuleModuleParameter"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2257:1: entryRuleModuleParameter : ruleModuleParameter EOF ;
public final void entryRuleModuleParameter() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2258:1: ( ruleModuleParameter EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2259:1: ruleModuleParameter EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getModuleParameterRule());
}
pushFollow(FollowSets000.FOLLOW_ruleModuleParameter_in_entryRuleModuleParameter4757);
ruleModuleParameter();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getModuleParameterRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleModuleParameter4764); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleModuleParameter"
// $ANTLR start "ruleModuleParameter"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2266:1: ruleModuleParameter : ( ( rule__ModuleParameter__Group__0 ) ) ;
public final void ruleModuleParameter() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2270:2: ( ( ( rule__ModuleParameter__Group__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2271:1: ( ( rule__ModuleParameter__Group__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2271:1: ( ( rule__ModuleParameter__Group__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2272:1: ( rule__ModuleParameter__Group__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getModuleParameterAccess().getGroup());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2273:1: ( rule__ModuleParameter__Group__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2273:2: rule__ModuleParameter__Group__0
{
pushFollow(FollowSets000.FOLLOW_rule__ModuleParameter__Group__0_in_ruleModuleParameter4790);
rule__ModuleParameter__Group__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getModuleParameterAccess().getGroup());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleModuleParameter"
// $ANTLR start "entryRuleModuleDefinition"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2285:1: entryRuleModuleDefinition : ruleModuleDefinition EOF ;
public final void entryRuleModuleDefinition() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2286:1: ( ruleModuleDefinition EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2287:1: ruleModuleDefinition EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getModuleDefinitionRule());
}
pushFollow(FollowSets000.FOLLOW_ruleModuleDefinition_in_entryRuleModuleDefinition4817);
ruleModuleDefinition();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getModuleDefinitionRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleModuleDefinition4824); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleModuleDefinition"
// $ANTLR start "ruleModuleDefinition"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2294:1: ruleModuleDefinition : ( ( rule__ModuleDefinition__Group__0 ) ) ;
public final void ruleModuleDefinition() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2298:2: ( ( ( rule__ModuleDefinition__Group__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2299:1: ( ( rule__ModuleDefinition__Group__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2299:1: ( ( rule__ModuleDefinition__Group__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2300:1: ( rule__ModuleDefinition__Group__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getModuleDefinitionAccess().getGroup());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2301:1: ( rule__ModuleDefinition__Group__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2301:2: rule__ModuleDefinition__Group__0
{
pushFollow(FollowSets000.FOLLOW_rule__ModuleDefinition__Group__0_in_ruleModuleDefinition4850);
rule__ModuleDefinition__Group__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getModuleDefinitionAccess().getGroup());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleModuleDefinition"
// $ANTLR start "entryRuleModuleDefinitionParameter"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2313:1: entryRuleModuleDefinitionParameter : ruleModuleDefinitionParameter EOF ;
public final void entryRuleModuleDefinitionParameter() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2314:1: ( ruleModuleDefinitionParameter EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2315:1: ruleModuleDefinitionParameter EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getModuleDefinitionParameterRule());
}
pushFollow(FollowSets000.FOLLOW_ruleModuleDefinitionParameter_in_entryRuleModuleDefinitionParameter4877);
ruleModuleDefinitionParameter();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getModuleDefinitionParameterRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleModuleDefinitionParameter4884); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleModuleDefinitionParameter"
// $ANTLR start "ruleModuleDefinitionParameter"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2322:1: ruleModuleDefinitionParameter : ( ( rule__ModuleDefinitionParameter__Group__0 ) ) ;
public final void ruleModuleDefinitionParameter() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2326:2: ( ( ( rule__ModuleDefinitionParameter__Group__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2327:1: ( ( rule__ModuleDefinitionParameter__Group__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2327:1: ( ( rule__ModuleDefinitionParameter__Group__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2328:1: ( rule__ModuleDefinitionParameter__Group__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getModuleDefinitionParameterAccess().getGroup());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2329:1: ( rule__ModuleDefinitionParameter__Group__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2329:2: rule__ModuleDefinitionParameter__Group__0
{
pushFollow(FollowSets000.FOLLOW_rule__ModuleDefinitionParameter__Group__0_in_ruleModuleDefinitionParameter4910);
rule__ModuleDefinitionParameter__Group__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getModuleDefinitionParameterAccess().getGroup());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleModuleDefinitionParameter"
// $ANTLR start "entryRuleServiceOptions"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2341:1: entryRuleServiceOptions : ruleServiceOptions EOF ;
public final void entryRuleServiceOptions() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2342:1: ( ruleServiceOptions EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2343:1: ruleServiceOptions EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getServiceOptionsRule());
}
pushFollow(FollowSets000.FOLLOW_ruleServiceOptions_in_entryRuleServiceOptions4937);
ruleServiceOptions();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getServiceOptionsRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleServiceOptions4944); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleServiceOptions"
// $ANTLR start "ruleServiceOptions"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2350:1: ruleServiceOptions : ( ( rule__ServiceOptions__Group__0 ) ) ;
public final void ruleServiceOptions() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2354:2: ( ( ( rule__ServiceOptions__Group__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2355:1: ( ( rule__ServiceOptions__Group__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2355:1: ( ( rule__ServiceOptions__Group__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2356:1: ( rule__ServiceOptions__Group__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getServiceOptionsAccess().getGroup());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2357:1: ( rule__ServiceOptions__Group__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2357:2: rule__ServiceOptions__Group__0
{
pushFollow(FollowSets000.FOLLOW_rule__ServiceOptions__Group__0_in_ruleServiceOptions4970);
rule__ServiceOptions__Group__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getServiceOptionsAccess().getGroup());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleServiceOptions"
// $ANTLR start "entryRuleServiceMethod"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2369:1: entryRuleServiceMethod : ruleServiceMethod EOF ;
public final void entryRuleServiceMethod() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2370:1: ( ruleServiceMethod EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2371:1: ruleServiceMethod EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getServiceMethodRule());
}
pushFollow(FollowSets000.FOLLOW_ruleServiceMethod_in_entryRuleServiceMethod4997);
ruleServiceMethod();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getServiceMethodRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleServiceMethod5004); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleServiceMethod"
// $ANTLR start "ruleServiceMethod"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2378:1: ruleServiceMethod : ( ( rule__ServiceMethod__Group__0 ) ) ;
public final void ruleServiceMethod() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2382:2: ( ( ( rule__ServiceMethod__Group__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2383:1: ( ( rule__ServiceMethod__Group__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2383:1: ( ( rule__ServiceMethod__Group__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2384:1: ( rule__ServiceMethod__Group__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getServiceMethodAccess().getGroup());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2385:1: ( rule__ServiceMethod__Group__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2385:2: rule__ServiceMethod__Group__0
{
pushFollow(FollowSets000.FOLLOW_rule__ServiceMethod__Group__0_in_ruleServiceMethod5030);
rule__ServiceMethod__Group__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getServiceMethodAccess().getGroup());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleServiceMethod"
// $ANTLR start "entryRuleService"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2397:1: entryRuleService : ruleService EOF ;
public final void entryRuleService() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2398:1: ( ruleService EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2399:1: ruleService EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getServiceRule());
}
pushFollow(FollowSets000.FOLLOW_ruleService_in_entryRuleService5057);
ruleService();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getServiceRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleService5064); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleService"
// $ANTLR start "ruleService"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2406:1: ruleService : ( ( rule__Service__Group__0 ) ) ;
public final void ruleService() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2410:2: ( ( ( rule__Service__Group__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2411:1: ( ( rule__Service__Group__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2411:1: ( ( rule__Service__Group__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2412:1: ( rule__Service__Group__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getServiceAccess().getGroup());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2413:1: ( rule__Service__Group__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2413:2: rule__Service__Group__0
{
pushFollow(FollowSets000.FOLLOW_rule__Service__Group__0_in_ruleService5090);
rule__Service__Group__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getServiceAccess().getGroup());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleService"
// $ANTLR start "entryRuleNavigationNode"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2425:1: entryRuleNavigationNode : ruleNavigationNode EOF ;
public final void entryRuleNavigationNode() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2426:1: ( ruleNavigationNode EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2427:1: ruleNavigationNode EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getNavigationNodeRule());
}
pushFollow(FollowSets000.FOLLOW_ruleNavigationNode_in_entryRuleNavigationNode5117);
ruleNavigationNode();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getNavigationNodeRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleNavigationNode5124); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleNavigationNode"
// $ANTLR start "ruleNavigationNode"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2434:1: ruleNavigationNode : ( ( rule__NavigationNode__Group__0 ) ) ;
public final void ruleNavigationNode() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2438:2: ( ( ( rule__NavigationNode__Group__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2439:1: ( ( rule__NavigationNode__Group__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2439:1: ( ( rule__NavigationNode__Group__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2440:1: ( rule__NavigationNode__Group__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getNavigationNodeAccess().getGroup());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2441:1: ( rule__NavigationNode__Group__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2441:2: rule__NavigationNode__Group__0
{
pushFollow(FollowSets000.FOLLOW_rule__NavigationNode__Group__0_in_ruleNavigationNode5150);
rule__NavigationNode__Group__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getNavigationNodeAccess().getGroup());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleNavigationNode"
// $ANTLR start "entryRuleXExpression"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2453:1: entryRuleXExpression : ruleXExpression EOF ;
public final void entryRuleXExpression() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2454:1: ( ruleXExpression EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2455:1: ruleXExpression EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXExpressionRule());
}
pushFollow(FollowSets000.FOLLOW_ruleXExpression_in_entryRuleXExpression5177);
ruleXExpression();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXExpressionRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleXExpression5184); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleXExpression"
// $ANTLR start "ruleXExpression"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2462:1: ruleXExpression : ( ruleXAssignment ) ;
public final void ruleXExpression() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2466:2: ( ( ruleXAssignment ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2467:1: ( ruleXAssignment )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2467:1: ( ruleXAssignment )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2468:1: ruleXAssignment
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXExpressionAccess().getXAssignmentParserRuleCall());
}
pushFollow(FollowSets000.FOLLOW_ruleXAssignment_in_ruleXExpression5210);
ruleXAssignment();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXExpressionAccess().getXAssignmentParserRuleCall());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleXExpression"
// $ANTLR start "entryRuleXAssignment"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2481:1: entryRuleXAssignment : ruleXAssignment EOF ;
public final void entryRuleXAssignment() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2482:1: ( ruleXAssignment EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2483:1: ruleXAssignment EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXAssignmentRule());
}
pushFollow(FollowSets000.FOLLOW_ruleXAssignment_in_entryRuleXAssignment5236);
ruleXAssignment();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXAssignmentRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleXAssignment5243); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleXAssignment"
// $ANTLR start "ruleXAssignment"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2490:1: ruleXAssignment : ( ( rule__XAssignment__Alternatives ) ) ;
public final void ruleXAssignment() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2494:2: ( ( ( rule__XAssignment__Alternatives ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2495:1: ( ( rule__XAssignment__Alternatives ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2495:1: ( ( rule__XAssignment__Alternatives ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2496:1: ( rule__XAssignment__Alternatives )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXAssignmentAccess().getAlternatives());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2497:1: ( rule__XAssignment__Alternatives )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2497:2: rule__XAssignment__Alternatives
{
pushFollow(FollowSets000.FOLLOW_rule__XAssignment__Alternatives_in_ruleXAssignment5269);
rule__XAssignment__Alternatives();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXAssignmentAccess().getAlternatives());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleXAssignment"
// $ANTLR start "entryRuleOpSingleAssign"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2509:1: entryRuleOpSingleAssign : ruleOpSingleAssign EOF ;
public final void entryRuleOpSingleAssign() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2510:1: ( ruleOpSingleAssign EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2511:1: ruleOpSingleAssign EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getOpSingleAssignRule());
}
pushFollow(FollowSets000.FOLLOW_ruleOpSingleAssign_in_entryRuleOpSingleAssign5296);
ruleOpSingleAssign();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getOpSingleAssignRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleOpSingleAssign5303); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleOpSingleAssign"
// $ANTLR start "ruleOpSingleAssign"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2518:1: ruleOpSingleAssign : ( '=' ) ;
public final void ruleOpSingleAssign() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2522:2: ( ( '=' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2523:1: ( '=' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2523:1: ( '=' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2524:1: '='
{
if ( state.backtracking==0 ) {
before(grammarAccess.getOpSingleAssignAccess().getEqualsSignKeyword());
}
match(input,13,FollowSets000.FOLLOW_13_in_ruleOpSingleAssign5330); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getOpSingleAssignAccess().getEqualsSignKeyword());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleOpSingleAssign"
// $ANTLR start "entryRuleOpMultiAssign"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2539:1: entryRuleOpMultiAssign : ruleOpMultiAssign EOF ;
public final void entryRuleOpMultiAssign() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2540:1: ( ruleOpMultiAssign EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2541:1: ruleOpMultiAssign EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getOpMultiAssignRule());
}
pushFollow(FollowSets000.FOLLOW_ruleOpMultiAssign_in_entryRuleOpMultiAssign5358);
ruleOpMultiAssign();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getOpMultiAssignRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleOpMultiAssign5365); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleOpMultiAssign"
// $ANTLR start "ruleOpMultiAssign"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2548:1: ruleOpMultiAssign : ( ( rule__OpMultiAssign__Alternatives ) ) ;
public final void ruleOpMultiAssign() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2552:2: ( ( ( rule__OpMultiAssign__Alternatives ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2553:1: ( ( rule__OpMultiAssign__Alternatives ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2553:1: ( ( rule__OpMultiAssign__Alternatives ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2554:1: ( rule__OpMultiAssign__Alternatives )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getOpMultiAssignAccess().getAlternatives());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2555:1: ( rule__OpMultiAssign__Alternatives )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2555:2: rule__OpMultiAssign__Alternatives
{
pushFollow(FollowSets000.FOLLOW_rule__OpMultiAssign__Alternatives_in_ruleOpMultiAssign5391);
rule__OpMultiAssign__Alternatives();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getOpMultiAssignAccess().getAlternatives());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleOpMultiAssign"
// $ANTLR start "entryRuleXOrExpression"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2567:1: entryRuleXOrExpression : ruleXOrExpression EOF ;
public final void entryRuleXOrExpression() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2568:1: ( ruleXOrExpression EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2569:1: ruleXOrExpression EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXOrExpressionRule());
}
pushFollow(FollowSets000.FOLLOW_ruleXOrExpression_in_entryRuleXOrExpression5418);
ruleXOrExpression();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXOrExpressionRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleXOrExpression5425); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleXOrExpression"
// $ANTLR start "ruleXOrExpression"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2576:1: ruleXOrExpression : ( ( rule__XOrExpression__Group__0 ) ) ;
public final void ruleXOrExpression() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2580:2: ( ( ( rule__XOrExpression__Group__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2581:1: ( ( rule__XOrExpression__Group__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2581:1: ( ( rule__XOrExpression__Group__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2582:1: ( rule__XOrExpression__Group__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXOrExpressionAccess().getGroup());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2583:1: ( rule__XOrExpression__Group__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2583:2: rule__XOrExpression__Group__0
{
pushFollow(FollowSets000.FOLLOW_rule__XOrExpression__Group__0_in_ruleXOrExpression5451);
rule__XOrExpression__Group__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXOrExpressionAccess().getGroup());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleXOrExpression"
// $ANTLR start "entryRuleOpOr"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2595:1: entryRuleOpOr : ruleOpOr EOF ;
public final void entryRuleOpOr() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2596:1: ( ruleOpOr EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2597:1: ruleOpOr EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getOpOrRule());
}
pushFollow(FollowSets000.FOLLOW_ruleOpOr_in_entryRuleOpOr5478);
ruleOpOr();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getOpOrRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleOpOr5485); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleOpOr"
// $ANTLR start "ruleOpOr"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2604:1: ruleOpOr : ( '||' ) ;
public final void ruleOpOr() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2608:2: ( ( '||' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2609:1: ( '||' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2609:1: ( '||' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2610:1: '||'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getOpOrAccess().getVerticalLineVerticalLineKeyword());
}
match(input,14,FollowSets000.FOLLOW_14_in_ruleOpOr5512); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getOpOrAccess().getVerticalLineVerticalLineKeyword());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleOpOr"
// $ANTLR start "entryRuleXAndExpression"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2625:1: entryRuleXAndExpression : ruleXAndExpression EOF ;
public final void entryRuleXAndExpression() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2626:1: ( ruleXAndExpression EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2627:1: ruleXAndExpression EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXAndExpressionRule());
}
pushFollow(FollowSets000.FOLLOW_ruleXAndExpression_in_entryRuleXAndExpression5540);
ruleXAndExpression();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXAndExpressionRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleXAndExpression5547); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleXAndExpression"
// $ANTLR start "ruleXAndExpression"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2634:1: ruleXAndExpression : ( ( rule__XAndExpression__Group__0 ) ) ;
public final void ruleXAndExpression() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2638:2: ( ( ( rule__XAndExpression__Group__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2639:1: ( ( rule__XAndExpression__Group__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2639:1: ( ( rule__XAndExpression__Group__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2640:1: ( rule__XAndExpression__Group__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXAndExpressionAccess().getGroup());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2641:1: ( rule__XAndExpression__Group__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2641:2: rule__XAndExpression__Group__0
{
pushFollow(FollowSets000.FOLLOW_rule__XAndExpression__Group__0_in_ruleXAndExpression5573);
rule__XAndExpression__Group__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXAndExpressionAccess().getGroup());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleXAndExpression"
// $ANTLR start "entryRuleOpAnd"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2653:1: entryRuleOpAnd : ruleOpAnd EOF ;
public final void entryRuleOpAnd() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2654:1: ( ruleOpAnd EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2655:1: ruleOpAnd EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getOpAndRule());
}
pushFollow(FollowSets000.FOLLOW_ruleOpAnd_in_entryRuleOpAnd5600);
ruleOpAnd();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getOpAndRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleOpAnd5607); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleOpAnd"
// $ANTLR start "ruleOpAnd"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2662:1: ruleOpAnd : ( '&&' ) ;
public final void ruleOpAnd() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2666:2: ( ( '&&' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2667:1: ( '&&' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2667:1: ( '&&' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2668:1: '&&'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getOpAndAccess().getAmpersandAmpersandKeyword());
}
match(input,15,FollowSets000.FOLLOW_15_in_ruleOpAnd5634); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getOpAndAccess().getAmpersandAmpersandKeyword());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleOpAnd"
// $ANTLR start "entryRuleXEqualityExpression"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2683:1: entryRuleXEqualityExpression : ruleXEqualityExpression EOF ;
public final void entryRuleXEqualityExpression() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2684:1: ( ruleXEqualityExpression EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2685:1: ruleXEqualityExpression EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXEqualityExpressionRule());
}
pushFollow(FollowSets000.FOLLOW_ruleXEqualityExpression_in_entryRuleXEqualityExpression5662);
ruleXEqualityExpression();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXEqualityExpressionRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleXEqualityExpression5669); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleXEqualityExpression"
// $ANTLR start "ruleXEqualityExpression"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2692:1: ruleXEqualityExpression : ( ( rule__XEqualityExpression__Group__0 ) ) ;
public final void ruleXEqualityExpression() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2696:2: ( ( ( rule__XEqualityExpression__Group__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2697:1: ( ( rule__XEqualityExpression__Group__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2697:1: ( ( rule__XEqualityExpression__Group__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2698:1: ( rule__XEqualityExpression__Group__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXEqualityExpressionAccess().getGroup());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2699:1: ( rule__XEqualityExpression__Group__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2699:2: rule__XEqualityExpression__Group__0
{
pushFollow(FollowSets000.FOLLOW_rule__XEqualityExpression__Group__0_in_ruleXEqualityExpression5695);
rule__XEqualityExpression__Group__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXEqualityExpressionAccess().getGroup());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleXEqualityExpression"
// $ANTLR start "entryRuleOpEquality"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2711:1: entryRuleOpEquality : ruleOpEquality EOF ;
public final void entryRuleOpEquality() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2712:1: ( ruleOpEquality EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2713:1: ruleOpEquality EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getOpEqualityRule());
}
pushFollow(FollowSets000.FOLLOW_ruleOpEquality_in_entryRuleOpEquality5722);
ruleOpEquality();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getOpEqualityRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleOpEquality5729); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleOpEquality"
// $ANTLR start "ruleOpEquality"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2720:1: ruleOpEquality : ( ( rule__OpEquality__Alternatives ) ) ;
public final void ruleOpEquality() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2724:2: ( ( ( rule__OpEquality__Alternatives ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2725:1: ( ( rule__OpEquality__Alternatives ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2725:1: ( ( rule__OpEquality__Alternatives ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2726:1: ( rule__OpEquality__Alternatives )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getOpEqualityAccess().getAlternatives());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2727:1: ( rule__OpEquality__Alternatives )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2727:2: rule__OpEquality__Alternatives
{
pushFollow(FollowSets000.FOLLOW_rule__OpEquality__Alternatives_in_ruleOpEquality5755);
rule__OpEquality__Alternatives();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getOpEqualityAccess().getAlternatives());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleOpEquality"
// $ANTLR start "entryRuleXRelationalExpression"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2739:1: entryRuleXRelationalExpression : ruleXRelationalExpression EOF ;
public final void entryRuleXRelationalExpression() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2740:1: ( ruleXRelationalExpression EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2741:1: ruleXRelationalExpression EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXRelationalExpressionRule());
}
pushFollow(FollowSets000.FOLLOW_ruleXRelationalExpression_in_entryRuleXRelationalExpression5782);
ruleXRelationalExpression();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXRelationalExpressionRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleXRelationalExpression5789); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleXRelationalExpression"
// $ANTLR start "ruleXRelationalExpression"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2748:1: ruleXRelationalExpression : ( ( rule__XRelationalExpression__Group__0 ) ) ;
public final void ruleXRelationalExpression() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2752:2: ( ( ( rule__XRelationalExpression__Group__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2753:1: ( ( rule__XRelationalExpression__Group__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2753:1: ( ( rule__XRelationalExpression__Group__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2754:1: ( rule__XRelationalExpression__Group__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXRelationalExpressionAccess().getGroup());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2755:1: ( rule__XRelationalExpression__Group__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2755:2: rule__XRelationalExpression__Group__0
{
pushFollow(FollowSets000.FOLLOW_rule__XRelationalExpression__Group__0_in_ruleXRelationalExpression5815);
rule__XRelationalExpression__Group__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXRelationalExpressionAccess().getGroup());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleXRelationalExpression"
// $ANTLR start "entryRuleOpCompare"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2767:1: entryRuleOpCompare : ruleOpCompare EOF ;
public final void entryRuleOpCompare() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2768:1: ( ruleOpCompare EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2769:1: ruleOpCompare EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getOpCompareRule());
}
pushFollow(FollowSets000.FOLLOW_ruleOpCompare_in_entryRuleOpCompare5842);
ruleOpCompare();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getOpCompareRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleOpCompare5849); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleOpCompare"
// $ANTLR start "ruleOpCompare"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2776:1: ruleOpCompare : ( ( rule__OpCompare__Alternatives ) ) ;
public final void ruleOpCompare() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2780:2: ( ( ( rule__OpCompare__Alternatives ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2781:1: ( ( rule__OpCompare__Alternatives ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2781:1: ( ( rule__OpCompare__Alternatives ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2782:1: ( rule__OpCompare__Alternatives )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getOpCompareAccess().getAlternatives());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2783:1: ( rule__OpCompare__Alternatives )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2783:2: rule__OpCompare__Alternatives
{
pushFollow(FollowSets000.FOLLOW_rule__OpCompare__Alternatives_in_ruleOpCompare5875);
rule__OpCompare__Alternatives();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getOpCompareAccess().getAlternatives());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleOpCompare"
// $ANTLR start "entryRuleXOtherOperatorExpression"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2795:1: entryRuleXOtherOperatorExpression : ruleXOtherOperatorExpression EOF ;
public final void entryRuleXOtherOperatorExpression() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2796:1: ( ruleXOtherOperatorExpression EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2797:1: ruleXOtherOperatorExpression EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXOtherOperatorExpressionRule());
}
pushFollow(FollowSets000.FOLLOW_ruleXOtherOperatorExpression_in_entryRuleXOtherOperatorExpression5902);
ruleXOtherOperatorExpression();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXOtherOperatorExpressionRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleXOtherOperatorExpression5909); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleXOtherOperatorExpression"
// $ANTLR start "ruleXOtherOperatorExpression"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2804:1: ruleXOtherOperatorExpression : ( ( rule__XOtherOperatorExpression__Group__0 ) ) ;
public final void ruleXOtherOperatorExpression() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2808:2: ( ( ( rule__XOtherOperatorExpression__Group__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2809:1: ( ( rule__XOtherOperatorExpression__Group__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2809:1: ( ( rule__XOtherOperatorExpression__Group__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2810:1: ( rule__XOtherOperatorExpression__Group__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXOtherOperatorExpressionAccess().getGroup());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2811:1: ( rule__XOtherOperatorExpression__Group__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2811:2: rule__XOtherOperatorExpression__Group__0
{
pushFollow(FollowSets000.FOLLOW_rule__XOtherOperatorExpression__Group__0_in_ruleXOtherOperatorExpression5935);
rule__XOtherOperatorExpression__Group__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXOtherOperatorExpressionAccess().getGroup());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleXOtherOperatorExpression"
// $ANTLR start "entryRuleOpOther"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2823:1: entryRuleOpOther : ruleOpOther EOF ;
public final void entryRuleOpOther() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2824:1: ( ruleOpOther EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2825:1: ruleOpOther EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getOpOtherRule());
}
pushFollow(FollowSets000.FOLLOW_ruleOpOther_in_entryRuleOpOther5962);
ruleOpOther();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getOpOtherRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleOpOther5969); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleOpOther"
// $ANTLR start "ruleOpOther"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2832:1: ruleOpOther : ( ( rule__OpOther__Alternatives ) ) ;
public final void ruleOpOther() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2836:2: ( ( ( rule__OpOther__Alternatives ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2837:1: ( ( rule__OpOther__Alternatives ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2837:1: ( ( rule__OpOther__Alternatives ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2838:1: ( rule__OpOther__Alternatives )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getOpOtherAccess().getAlternatives());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2839:1: ( rule__OpOther__Alternatives )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2839:2: rule__OpOther__Alternatives
{
pushFollow(FollowSets000.FOLLOW_rule__OpOther__Alternatives_in_ruleOpOther5995);
rule__OpOther__Alternatives();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getOpOtherAccess().getAlternatives());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleOpOther"
// $ANTLR start "entryRuleXAdditiveExpression"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2851:1: entryRuleXAdditiveExpression : ruleXAdditiveExpression EOF ;
public final void entryRuleXAdditiveExpression() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2852:1: ( ruleXAdditiveExpression EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2853:1: ruleXAdditiveExpression EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXAdditiveExpressionRule());
}
pushFollow(FollowSets000.FOLLOW_ruleXAdditiveExpression_in_entryRuleXAdditiveExpression6022);
ruleXAdditiveExpression();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXAdditiveExpressionRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleXAdditiveExpression6029); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleXAdditiveExpression"
// $ANTLR start "ruleXAdditiveExpression"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2860:1: ruleXAdditiveExpression : ( ( rule__XAdditiveExpression__Group__0 ) ) ;
public final void ruleXAdditiveExpression() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2864:2: ( ( ( rule__XAdditiveExpression__Group__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2865:1: ( ( rule__XAdditiveExpression__Group__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2865:1: ( ( rule__XAdditiveExpression__Group__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2866:1: ( rule__XAdditiveExpression__Group__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXAdditiveExpressionAccess().getGroup());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2867:1: ( rule__XAdditiveExpression__Group__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2867:2: rule__XAdditiveExpression__Group__0
{
pushFollow(FollowSets000.FOLLOW_rule__XAdditiveExpression__Group__0_in_ruleXAdditiveExpression6055);
rule__XAdditiveExpression__Group__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXAdditiveExpressionAccess().getGroup());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleXAdditiveExpression"
// $ANTLR start "entryRuleOpAdd"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2879:1: entryRuleOpAdd : ruleOpAdd EOF ;
public final void entryRuleOpAdd() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2880:1: ( ruleOpAdd EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2881:1: ruleOpAdd EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getOpAddRule());
}
pushFollow(FollowSets000.FOLLOW_ruleOpAdd_in_entryRuleOpAdd6082);
ruleOpAdd();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getOpAddRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleOpAdd6089); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleOpAdd"
// $ANTLR start "ruleOpAdd"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2888:1: ruleOpAdd : ( ( rule__OpAdd__Alternatives ) ) ;
public final void ruleOpAdd() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2892:2: ( ( ( rule__OpAdd__Alternatives ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2893:1: ( ( rule__OpAdd__Alternatives ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2893:1: ( ( rule__OpAdd__Alternatives ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2894:1: ( rule__OpAdd__Alternatives )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getOpAddAccess().getAlternatives());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2895:1: ( rule__OpAdd__Alternatives )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2895:2: rule__OpAdd__Alternatives
{
pushFollow(FollowSets000.FOLLOW_rule__OpAdd__Alternatives_in_ruleOpAdd6115);
rule__OpAdd__Alternatives();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getOpAddAccess().getAlternatives());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleOpAdd"
// $ANTLR start "entryRuleXMultiplicativeExpression"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2907:1: entryRuleXMultiplicativeExpression : ruleXMultiplicativeExpression EOF ;
public final void entryRuleXMultiplicativeExpression() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2908:1: ( ruleXMultiplicativeExpression EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2909:1: ruleXMultiplicativeExpression EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXMultiplicativeExpressionRule());
}
pushFollow(FollowSets000.FOLLOW_ruleXMultiplicativeExpression_in_entryRuleXMultiplicativeExpression6142);
ruleXMultiplicativeExpression();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXMultiplicativeExpressionRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleXMultiplicativeExpression6149); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleXMultiplicativeExpression"
// $ANTLR start "ruleXMultiplicativeExpression"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2916:1: ruleXMultiplicativeExpression : ( ( rule__XMultiplicativeExpression__Group__0 ) ) ;
public final void ruleXMultiplicativeExpression() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2920:2: ( ( ( rule__XMultiplicativeExpression__Group__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2921:1: ( ( rule__XMultiplicativeExpression__Group__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2921:1: ( ( rule__XMultiplicativeExpression__Group__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2922:1: ( rule__XMultiplicativeExpression__Group__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXMultiplicativeExpressionAccess().getGroup());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2923:1: ( rule__XMultiplicativeExpression__Group__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2923:2: rule__XMultiplicativeExpression__Group__0
{
pushFollow(FollowSets000.FOLLOW_rule__XMultiplicativeExpression__Group__0_in_ruleXMultiplicativeExpression6175);
rule__XMultiplicativeExpression__Group__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXMultiplicativeExpressionAccess().getGroup());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleXMultiplicativeExpression"
// $ANTLR start "entryRuleOpMulti"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2935:1: entryRuleOpMulti : ruleOpMulti EOF ;
public final void entryRuleOpMulti() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2936:1: ( ruleOpMulti EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2937:1: ruleOpMulti EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getOpMultiRule());
}
pushFollow(FollowSets000.FOLLOW_ruleOpMulti_in_entryRuleOpMulti6202);
ruleOpMulti();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getOpMultiRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleOpMulti6209); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleOpMulti"
// $ANTLR start "ruleOpMulti"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2944:1: ruleOpMulti : ( ( rule__OpMulti__Alternatives ) ) ;
public final void ruleOpMulti() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2948:2: ( ( ( rule__OpMulti__Alternatives ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2949:1: ( ( rule__OpMulti__Alternatives ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2949:1: ( ( rule__OpMulti__Alternatives ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2950:1: ( rule__OpMulti__Alternatives )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getOpMultiAccess().getAlternatives());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2951:1: ( rule__OpMulti__Alternatives )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2951:2: rule__OpMulti__Alternatives
{
pushFollow(FollowSets000.FOLLOW_rule__OpMulti__Alternatives_in_ruleOpMulti6235);
rule__OpMulti__Alternatives();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getOpMultiAccess().getAlternatives());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleOpMulti"
// $ANTLR start "entryRuleXUnaryOperation"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2963:1: entryRuleXUnaryOperation : ruleXUnaryOperation EOF ;
public final void entryRuleXUnaryOperation() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2964:1: ( ruleXUnaryOperation EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2965:1: ruleXUnaryOperation EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXUnaryOperationRule());
}
pushFollow(FollowSets000.FOLLOW_ruleXUnaryOperation_in_entryRuleXUnaryOperation6262);
ruleXUnaryOperation();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXUnaryOperationRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleXUnaryOperation6269); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleXUnaryOperation"
// $ANTLR start "ruleXUnaryOperation"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2972:1: ruleXUnaryOperation : ( ( rule__XUnaryOperation__Alternatives ) ) ;
public final void ruleXUnaryOperation() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2976:2: ( ( ( rule__XUnaryOperation__Alternatives ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2977:1: ( ( rule__XUnaryOperation__Alternatives ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2977:1: ( ( rule__XUnaryOperation__Alternatives ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2978:1: ( rule__XUnaryOperation__Alternatives )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXUnaryOperationAccess().getAlternatives());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2979:1: ( rule__XUnaryOperation__Alternatives )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2979:2: rule__XUnaryOperation__Alternatives
{
pushFollow(FollowSets000.FOLLOW_rule__XUnaryOperation__Alternatives_in_ruleXUnaryOperation6295);
rule__XUnaryOperation__Alternatives();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXUnaryOperationAccess().getAlternatives());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleXUnaryOperation"
// $ANTLR start "entryRuleOpUnary"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2991:1: entryRuleOpUnary : ruleOpUnary EOF ;
public final void entryRuleOpUnary() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2992:1: ( ruleOpUnary EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:2993:1: ruleOpUnary EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getOpUnaryRule());
}
pushFollow(FollowSets000.FOLLOW_ruleOpUnary_in_entryRuleOpUnary6322);
ruleOpUnary();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getOpUnaryRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleOpUnary6329); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleOpUnary"
// $ANTLR start "ruleOpUnary"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3000:1: ruleOpUnary : ( ( rule__OpUnary__Alternatives ) ) ;
public final void ruleOpUnary() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3004:2: ( ( ( rule__OpUnary__Alternatives ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3005:1: ( ( rule__OpUnary__Alternatives ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3005:1: ( ( rule__OpUnary__Alternatives ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3006:1: ( rule__OpUnary__Alternatives )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getOpUnaryAccess().getAlternatives());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3007:1: ( rule__OpUnary__Alternatives )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3007:2: rule__OpUnary__Alternatives
{
pushFollow(FollowSets000.FOLLOW_rule__OpUnary__Alternatives_in_ruleOpUnary6355);
rule__OpUnary__Alternatives();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getOpUnaryAccess().getAlternatives());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleOpUnary"
// $ANTLR start "entryRuleXCastedExpression"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3019:1: entryRuleXCastedExpression : ruleXCastedExpression EOF ;
public final void entryRuleXCastedExpression() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3020:1: ( ruleXCastedExpression EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3021:1: ruleXCastedExpression EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXCastedExpressionRule());
}
pushFollow(FollowSets000.FOLLOW_ruleXCastedExpression_in_entryRuleXCastedExpression6382);
ruleXCastedExpression();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXCastedExpressionRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleXCastedExpression6389); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleXCastedExpression"
// $ANTLR start "ruleXCastedExpression"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3028:1: ruleXCastedExpression : ( ( rule__XCastedExpression__Group__0 ) ) ;
public final void ruleXCastedExpression() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3032:2: ( ( ( rule__XCastedExpression__Group__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3033:1: ( ( rule__XCastedExpression__Group__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3033:1: ( ( rule__XCastedExpression__Group__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3034:1: ( rule__XCastedExpression__Group__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXCastedExpressionAccess().getGroup());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3035:1: ( rule__XCastedExpression__Group__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3035:2: rule__XCastedExpression__Group__0
{
pushFollow(FollowSets000.FOLLOW_rule__XCastedExpression__Group__0_in_ruleXCastedExpression6415);
rule__XCastedExpression__Group__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXCastedExpressionAccess().getGroup());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleXCastedExpression"
// $ANTLR start "entryRuleXPostfixOperation"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3047:1: entryRuleXPostfixOperation : ruleXPostfixOperation EOF ;
public final void entryRuleXPostfixOperation() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3048:1: ( ruleXPostfixOperation EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3049:1: ruleXPostfixOperation EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXPostfixOperationRule());
}
pushFollow(FollowSets000.FOLLOW_ruleXPostfixOperation_in_entryRuleXPostfixOperation6442);
ruleXPostfixOperation();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXPostfixOperationRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleXPostfixOperation6449); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleXPostfixOperation"
// $ANTLR start "ruleXPostfixOperation"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3056:1: ruleXPostfixOperation : ( ( rule__XPostfixOperation__Group__0 ) ) ;
public final void ruleXPostfixOperation() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3060:2: ( ( ( rule__XPostfixOperation__Group__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3061:1: ( ( rule__XPostfixOperation__Group__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3061:1: ( ( rule__XPostfixOperation__Group__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3062:1: ( rule__XPostfixOperation__Group__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXPostfixOperationAccess().getGroup());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3063:1: ( rule__XPostfixOperation__Group__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3063:2: rule__XPostfixOperation__Group__0
{
pushFollow(FollowSets000.FOLLOW_rule__XPostfixOperation__Group__0_in_ruleXPostfixOperation6475);
rule__XPostfixOperation__Group__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXPostfixOperationAccess().getGroup());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleXPostfixOperation"
// $ANTLR start "entryRuleOpPostfix"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3075:1: entryRuleOpPostfix : ruleOpPostfix EOF ;
public final void entryRuleOpPostfix() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3076:1: ( ruleOpPostfix EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3077:1: ruleOpPostfix EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getOpPostfixRule());
}
pushFollow(FollowSets000.FOLLOW_ruleOpPostfix_in_entryRuleOpPostfix6502);
ruleOpPostfix();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getOpPostfixRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleOpPostfix6509); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleOpPostfix"
// $ANTLR start "ruleOpPostfix"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3084:1: ruleOpPostfix : ( ( rule__OpPostfix__Alternatives ) ) ;
public final void ruleOpPostfix() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3088:2: ( ( ( rule__OpPostfix__Alternatives ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3089:1: ( ( rule__OpPostfix__Alternatives ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3089:1: ( ( rule__OpPostfix__Alternatives ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3090:1: ( rule__OpPostfix__Alternatives )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getOpPostfixAccess().getAlternatives());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3091:1: ( rule__OpPostfix__Alternatives )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3091:2: rule__OpPostfix__Alternatives
{
pushFollow(FollowSets000.FOLLOW_rule__OpPostfix__Alternatives_in_ruleOpPostfix6535);
rule__OpPostfix__Alternatives();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getOpPostfixAccess().getAlternatives());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleOpPostfix"
// $ANTLR start "entryRuleXMemberFeatureCall"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3103:1: entryRuleXMemberFeatureCall : ruleXMemberFeatureCall EOF ;
public final void entryRuleXMemberFeatureCall() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3104:1: ( ruleXMemberFeatureCall EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3105:1: ruleXMemberFeatureCall EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXMemberFeatureCallRule());
}
pushFollow(FollowSets000.FOLLOW_ruleXMemberFeatureCall_in_entryRuleXMemberFeatureCall6562);
ruleXMemberFeatureCall();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXMemberFeatureCallRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleXMemberFeatureCall6569); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleXMemberFeatureCall"
// $ANTLR start "ruleXMemberFeatureCall"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3112:1: ruleXMemberFeatureCall : ( ( rule__XMemberFeatureCall__Group__0 ) ) ;
public final void ruleXMemberFeatureCall() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3116:2: ( ( ( rule__XMemberFeatureCall__Group__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3117:1: ( ( rule__XMemberFeatureCall__Group__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3117:1: ( ( rule__XMemberFeatureCall__Group__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3118:1: ( rule__XMemberFeatureCall__Group__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXMemberFeatureCallAccess().getGroup());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3119:1: ( rule__XMemberFeatureCall__Group__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3119:2: rule__XMemberFeatureCall__Group__0
{
pushFollow(FollowSets000.FOLLOW_rule__XMemberFeatureCall__Group__0_in_ruleXMemberFeatureCall6595);
rule__XMemberFeatureCall__Group__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXMemberFeatureCallAccess().getGroup());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleXMemberFeatureCall"
// $ANTLR start "entryRuleXPrimaryExpression"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3131:1: entryRuleXPrimaryExpression : ruleXPrimaryExpression EOF ;
public final void entryRuleXPrimaryExpression() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3132:1: ( ruleXPrimaryExpression EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3133:1: ruleXPrimaryExpression EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXPrimaryExpressionRule());
}
pushFollow(FollowSets000.FOLLOW_ruleXPrimaryExpression_in_entryRuleXPrimaryExpression6622);
ruleXPrimaryExpression();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXPrimaryExpressionRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleXPrimaryExpression6629); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleXPrimaryExpression"
// $ANTLR start "ruleXPrimaryExpression"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3140:1: ruleXPrimaryExpression : ( ( rule__XPrimaryExpression__Alternatives ) ) ;
public final void ruleXPrimaryExpression() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3144:2: ( ( ( rule__XPrimaryExpression__Alternatives ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3145:1: ( ( rule__XPrimaryExpression__Alternatives ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3145:1: ( ( rule__XPrimaryExpression__Alternatives ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3146:1: ( rule__XPrimaryExpression__Alternatives )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXPrimaryExpressionAccess().getAlternatives());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3147:1: ( rule__XPrimaryExpression__Alternatives )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3147:2: rule__XPrimaryExpression__Alternatives
{
pushFollow(FollowSets000.FOLLOW_rule__XPrimaryExpression__Alternatives_in_ruleXPrimaryExpression6655);
rule__XPrimaryExpression__Alternatives();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXPrimaryExpressionAccess().getAlternatives());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleXPrimaryExpression"
// $ANTLR start "entryRuleXLiteral"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3159:1: entryRuleXLiteral : ruleXLiteral EOF ;
public final void entryRuleXLiteral() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3160:1: ( ruleXLiteral EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3161:1: ruleXLiteral EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXLiteralRule());
}
pushFollow(FollowSets000.FOLLOW_ruleXLiteral_in_entryRuleXLiteral6682);
ruleXLiteral();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXLiteralRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleXLiteral6689); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleXLiteral"
// $ANTLR start "ruleXLiteral"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3168:1: ruleXLiteral : ( ( rule__XLiteral__Alternatives ) ) ;
public final void ruleXLiteral() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3172:2: ( ( ( rule__XLiteral__Alternatives ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3173:1: ( ( rule__XLiteral__Alternatives ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3173:1: ( ( rule__XLiteral__Alternatives ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3174:1: ( rule__XLiteral__Alternatives )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXLiteralAccess().getAlternatives());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3175:1: ( rule__XLiteral__Alternatives )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3175:2: rule__XLiteral__Alternatives
{
pushFollow(FollowSets000.FOLLOW_rule__XLiteral__Alternatives_in_ruleXLiteral6715);
rule__XLiteral__Alternatives();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXLiteralAccess().getAlternatives());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleXLiteral"
// $ANTLR start "entryRuleXCollectionLiteral"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3187:1: entryRuleXCollectionLiteral : ruleXCollectionLiteral EOF ;
public final void entryRuleXCollectionLiteral() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3188:1: ( ruleXCollectionLiteral EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3189:1: ruleXCollectionLiteral EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXCollectionLiteralRule());
}
pushFollow(FollowSets000.FOLLOW_ruleXCollectionLiteral_in_entryRuleXCollectionLiteral6742);
ruleXCollectionLiteral();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXCollectionLiteralRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleXCollectionLiteral6749); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleXCollectionLiteral"
// $ANTLR start "ruleXCollectionLiteral"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3196:1: ruleXCollectionLiteral : ( ( rule__XCollectionLiteral__Alternatives ) ) ;
public final void ruleXCollectionLiteral() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3200:2: ( ( ( rule__XCollectionLiteral__Alternatives ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3201:1: ( ( rule__XCollectionLiteral__Alternatives ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3201:1: ( ( rule__XCollectionLiteral__Alternatives ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3202:1: ( rule__XCollectionLiteral__Alternatives )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXCollectionLiteralAccess().getAlternatives());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3203:1: ( rule__XCollectionLiteral__Alternatives )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3203:2: rule__XCollectionLiteral__Alternatives
{
pushFollow(FollowSets000.FOLLOW_rule__XCollectionLiteral__Alternatives_in_ruleXCollectionLiteral6775);
rule__XCollectionLiteral__Alternatives();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXCollectionLiteralAccess().getAlternatives());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleXCollectionLiteral"
// $ANTLR start "entryRuleXSetLiteral"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3215:1: entryRuleXSetLiteral : ruleXSetLiteral EOF ;
public final void entryRuleXSetLiteral() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3216:1: ( ruleXSetLiteral EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3217:1: ruleXSetLiteral EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXSetLiteralRule());
}
pushFollow(FollowSets000.FOLLOW_ruleXSetLiteral_in_entryRuleXSetLiteral6802);
ruleXSetLiteral();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXSetLiteralRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleXSetLiteral6809); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleXSetLiteral"
// $ANTLR start "ruleXSetLiteral"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3224:1: ruleXSetLiteral : ( ( rule__XSetLiteral__Group__0 ) ) ;
public final void ruleXSetLiteral() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3228:2: ( ( ( rule__XSetLiteral__Group__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3229:1: ( ( rule__XSetLiteral__Group__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3229:1: ( ( rule__XSetLiteral__Group__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3230:1: ( rule__XSetLiteral__Group__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXSetLiteralAccess().getGroup());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3231:1: ( rule__XSetLiteral__Group__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3231:2: rule__XSetLiteral__Group__0
{
pushFollow(FollowSets000.FOLLOW_rule__XSetLiteral__Group__0_in_ruleXSetLiteral6835);
rule__XSetLiteral__Group__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXSetLiteralAccess().getGroup());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleXSetLiteral"
// $ANTLR start "entryRuleXListLiteral"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3243:1: entryRuleXListLiteral : ruleXListLiteral EOF ;
public final void entryRuleXListLiteral() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3244:1: ( ruleXListLiteral EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3245:1: ruleXListLiteral EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXListLiteralRule());
}
pushFollow(FollowSets000.FOLLOW_ruleXListLiteral_in_entryRuleXListLiteral6862);
ruleXListLiteral();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXListLiteralRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleXListLiteral6869); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleXListLiteral"
// $ANTLR start "ruleXListLiteral"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3252:1: ruleXListLiteral : ( ( rule__XListLiteral__Group__0 ) ) ;
public final void ruleXListLiteral() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3256:2: ( ( ( rule__XListLiteral__Group__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3257:1: ( ( rule__XListLiteral__Group__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3257:1: ( ( rule__XListLiteral__Group__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3258:1: ( rule__XListLiteral__Group__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXListLiteralAccess().getGroup());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3259:1: ( rule__XListLiteral__Group__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3259:2: rule__XListLiteral__Group__0
{
pushFollow(FollowSets000.FOLLOW_rule__XListLiteral__Group__0_in_ruleXListLiteral6895);
rule__XListLiteral__Group__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXListLiteralAccess().getGroup());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleXListLiteral"
// $ANTLR start "entryRuleXClosure"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3271:1: entryRuleXClosure : ruleXClosure EOF ;
public final void entryRuleXClosure() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3272:1: ( ruleXClosure EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3273:1: ruleXClosure EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXClosureRule());
}
pushFollow(FollowSets000.FOLLOW_ruleXClosure_in_entryRuleXClosure6922);
ruleXClosure();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXClosureRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleXClosure6929); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleXClosure"
// $ANTLR start "ruleXClosure"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3280:1: ruleXClosure : ( ( rule__XClosure__Group__0 ) ) ;
public final void ruleXClosure() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3284:2: ( ( ( rule__XClosure__Group__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3285:1: ( ( rule__XClosure__Group__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3285:1: ( ( rule__XClosure__Group__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3286:1: ( rule__XClosure__Group__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXClosureAccess().getGroup());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3287:1: ( rule__XClosure__Group__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3287:2: rule__XClosure__Group__0
{
pushFollow(FollowSets000.FOLLOW_rule__XClosure__Group__0_in_ruleXClosure6955);
rule__XClosure__Group__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXClosureAccess().getGroup());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleXClosure"
// $ANTLR start "entryRuleXExpressionInClosure"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3299:1: entryRuleXExpressionInClosure : ruleXExpressionInClosure EOF ;
public final void entryRuleXExpressionInClosure() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3300:1: ( ruleXExpressionInClosure EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3301:1: ruleXExpressionInClosure EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXExpressionInClosureRule());
}
pushFollow(FollowSets000.FOLLOW_ruleXExpressionInClosure_in_entryRuleXExpressionInClosure6982);
ruleXExpressionInClosure();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXExpressionInClosureRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleXExpressionInClosure6989); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleXExpressionInClosure"
// $ANTLR start "ruleXExpressionInClosure"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3308:1: ruleXExpressionInClosure : ( ( rule__XExpressionInClosure__Group__0 ) ) ;
public final void ruleXExpressionInClosure() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3312:2: ( ( ( rule__XExpressionInClosure__Group__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3313:1: ( ( rule__XExpressionInClosure__Group__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3313:1: ( ( rule__XExpressionInClosure__Group__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3314:1: ( rule__XExpressionInClosure__Group__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXExpressionInClosureAccess().getGroup());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3315:1: ( rule__XExpressionInClosure__Group__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3315:2: rule__XExpressionInClosure__Group__0
{
pushFollow(FollowSets000.FOLLOW_rule__XExpressionInClosure__Group__0_in_ruleXExpressionInClosure7015);
rule__XExpressionInClosure__Group__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXExpressionInClosureAccess().getGroup());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleXExpressionInClosure"
// $ANTLR start "entryRuleXShortClosure"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3327:1: entryRuleXShortClosure : ruleXShortClosure EOF ;
public final void entryRuleXShortClosure() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3328:1: ( ruleXShortClosure EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3329:1: ruleXShortClosure EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXShortClosureRule());
}
pushFollow(FollowSets000.FOLLOW_ruleXShortClosure_in_entryRuleXShortClosure7042);
ruleXShortClosure();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXShortClosureRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleXShortClosure7049); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleXShortClosure"
// $ANTLR start "ruleXShortClosure"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3336:1: ruleXShortClosure : ( ( rule__XShortClosure__Group__0 ) ) ;
public final void ruleXShortClosure() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3340:2: ( ( ( rule__XShortClosure__Group__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3341:1: ( ( rule__XShortClosure__Group__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3341:1: ( ( rule__XShortClosure__Group__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3342:1: ( rule__XShortClosure__Group__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXShortClosureAccess().getGroup());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3343:1: ( rule__XShortClosure__Group__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3343:2: rule__XShortClosure__Group__0
{
pushFollow(FollowSets000.FOLLOW_rule__XShortClosure__Group__0_in_ruleXShortClosure7075);
rule__XShortClosure__Group__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXShortClosureAccess().getGroup());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleXShortClosure"
// $ANTLR start "entryRuleXParenthesizedExpression"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3355:1: entryRuleXParenthesizedExpression : ruleXParenthesizedExpression EOF ;
public final void entryRuleXParenthesizedExpression() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3356:1: ( ruleXParenthesizedExpression EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3357:1: ruleXParenthesizedExpression EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXParenthesizedExpressionRule());
}
pushFollow(FollowSets000.FOLLOW_ruleXParenthesizedExpression_in_entryRuleXParenthesizedExpression7102);
ruleXParenthesizedExpression();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXParenthesizedExpressionRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleXParenthesizedExpression7109); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleXParenthesizedExpression"
// $ANTLR start "ruleXParenthesizedExpression"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3364:1: ruleXParenthesizedExpression : ( ( rule__XParenthesizedExpression__Group__0 ) ) ;
public final void ruleXParenthesizedExpression() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3368:2: ( ( ( rule__XParenthesizedExpression__Group__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3369:1: ( ( rule__XParenthesizedExpression__Group__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3369:1: ( ( rule__XParenthesizedExpression__Group__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3370:1: ( rule__XParenthesizedExpression__Group__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXParenthesizedExpressionAccess().getGroup());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3371:1: ( rule__XParenthesizedExpression__Group__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3371:2: rule__XParenthesizedExpression__Group__0
{
pushFollow(FollowSets000.FOLLOW_rule__XParenthesizedExpression__Group__0_in_ruleXParenthesizedExpression7135);
rule__XParenthesizedExpression__Group__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXParenthesizedExpressionAccess().getGroup());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleXParenthesizedExpression"
// $ANTLR start "entryRuleXIfExpression"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3383:1: entryRuleXIfExpression : ruleXIfExpression EOF ;
public final void entryRuleXIfExpression() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3384:1: ( ruleXIfExpression EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3385:1: ruleXIfExpression EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXIfExpressionRule());
}
pushFollow(FollowSets000.FOLLOW_ruleXIfExpression_in_entryRuleXIfExpression7162);
ruleXIfExpression();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXIfExpressionRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleXIfExpression7169); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleXIfExpression"
// $ANTLR start "ruleXIfExpression"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3392:1: ruleXIfExpression : ( ( rule__XIfExpression__Group__0 ) ) ;
public final void ruleXIfExpression() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3396:2: ( ( ( rule__XIfExpression__Group__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3397:1: ( ( rule__XIfExpression__Group__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3397:1: ( ( rule__XIfExpression__Group__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3398:1: ( rule__XIfExpression__Group__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXIfExpressionAccess().getGroup());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3399:1: ( rule__XIfExpression__Group__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3399:2: rule__XIfExpression__Group__0
{
pushFollow(FollowSets000.FOLLOW_rule__XIfExpression__Group__0_in_ruleXIfExpression7195);
rule__XIfExpression__Group__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXIfExpressionAccess().getGroup());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleXIfExpression"
// $ANTLR start "entryRuleXSwitchExpression"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3411:1: entryRuleXSwitchExpression : ruleXSwitchExpression EOF ;
public final void entryRuleXSwitchExpression() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3412:1: ( ruleXSwitchExpression EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3413:1: ruleXSwitchExpression EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXSwitchExpressionRule());
}
pushFollow(FollowSets000.FOLLOW_ruleXSwitchExpression_in_entryRuleXSwitchExpression7222);
ruleXSwitchExpression();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXSwitchExpressionRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleXSwitchExpression7229); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleXSwitchExpression"
// $ANTLR start "ruleXSwitchExpression"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3420:1: ruleXSwitchExpression : ( ( rule__XSwitchExpression__Group__0 ) ) ;
public final void ruleXSwitchExpression() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3424:2: ( ( ( rule__XSwitchExpression__Group__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3425:1: ( ( rule__XSwitchExpression__Group__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3425:1: ( ( rule__XSwitchExpression__Group__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3426:1: ( rule__XSwitchExpression__Group__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXSwitchExpressionAccess().getGroup());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3427:1: ( rule__XSwitchExpression__Group__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3427:2: rule__XSwitchExpression__Group__0
{
pushFollow(FollowSets000.FOLLOW_rule__XSwitchExpression__Group__0_in_ruleXSwitchExpression7255);
rule__XSwitchExpression__Group__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXSwitchExpressionAccess().getGroup());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleXSwitchExpression"
// $ANTLR start "entryRuleXCasePart"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3439:1: entryRuleXCasePart : ruleXCasePart EOF ;
public final void entryRuleXCasePart() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3440:1: ( ruleXCasePart EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3441:1: ruleXCasePart EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXCasePartRule());
}
pushFollow(FollowSets000.FOLLOW_ruleXCasePart_in_entryRuleXCasePart7282);
ruleXCasePart();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXCasePartRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleXCasePart7289); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleXCasePart"
// $ANTLR start "ruleXCasePart"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3448:1: ruleXCasePart : ( ( rule__XCasePart__Group__0 ) ) ;
public final void ruleXCasePart() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3452:2: ( ( ( rule__XCasePart__Group__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3453:1: ( ( rule__XCasePart__Group__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3453:1: ( ( rule__XCasePart__Group__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3454:1: ( rule__XCasePart__Group__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXCasePartAccess().getGroup());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3455:1: ( rule__XCasePart__Group__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3455:2: rule__XCasePart__Group__0
{
pushFollow(FollowSets000.FOLLOW_rule__XCasePart__Group__0_in_ruleXCasePart7315);
rule__XCasePart__Group__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXCasePartAccess().getGroup());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleXCasePart"
// $ANTLR start "entryRuleXForLoopExpression"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3467:1: entryRuleXForLoopExpression : ruleXForLoopExpression EOF ;
public final void entryRuleXForLoopExpression() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3468:1: ( ruleXForLoopExpression EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3469:1: ruleXForLoopExpression EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXForLoopExpressionRule());
}
pushFollow(FollowSets000.FOLLOW_ruleXForLoopExpression_in_entryRuleXForLoopExpression7342);
ruleXForLoopExpression();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXForLoopExpressionRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleXForLoopExpression7349); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleXForLoopExpression"
// $ANTLR start "ruleXForLoopExpression"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3476:1: ruleXForLoopExpression : ( ( rule__XForLoopExpression__Group__0 ) ) ;
public final void ruleXForLoopExpression() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3480:2: ( ( ( rule__XForLoopExpression__Group__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3481:1: ( ( rule__XForLoopExpression__Group__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3481:1: ( ( rule__XForLoopExpression__Group__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3482:1: ( rule__XForLoopExpression__Group__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXForLoopExpressionAccess().getGroup());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3483:1: ( rule__XForLoopExpression__Group__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3483:2: rule__XForLoopExpression__Group__0
{
pushFollow(FollowSets000.FOLLOW_rule__XForLoopExpression__Group__0_in_ruleXForLoopExpression7375);
rule__XForLoopExpression__Group__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXForLoopExpressionAccess().getGroup());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleXForLoopExpression"
// $ANTLR start "entryRuleXBasicForLoopExpression"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3495:1: entryRuleXBasicForLoopExpression : ruleXBasicForLoopExpression EOF ;
public final void entryRuleXBasicForLoopExpression() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3496:1: ( ruleXBasicForLoopExpression EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3497:1: ruleXBasicForLoopExpression EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXBasicForLoopExpressionRule());
}
pushFollow(FollowSets000.FOLLOW_ruleXBasicForLoopExpression_in_entryRuleXBasicForLoopExpression7402);
ruleXBasicForLoopExpression();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXBasicForLoopExpressionRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleXBasicForLoopExpression7409); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleXBasicForLoopExpression"
// $ANTLR start "ruleXBasicForLoopExpression"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3504:1: ruleXBasicForLoopExpression : ( ( rule__XBasicForLoopExpression__Group__0 ) ) ;
public final void ruleXBasicForLoopExpression() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3508:2: ( ( ( rule__XBasicForLoopExpression__Group__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3509:1: ( ( rule__XBasicForLoopExpression__Group__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3509:1: ( ( rule__XBasicForLoopExpression__Group__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3510:1: ( rule__XBasicForLoopExpression__Group__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXBasicForLoopExpressionAccess().getGroup());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3511:1: ( rule__XBasicForLoopExpression__Group__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3511:2: rule__XBasicForLoopExpression__Group__0
{
pushFollow(FollowSets000.FOLLOW_rule__XBasicForLoopExpression__Group__0_in_ruleXBasicForLoopExpression7435);
rule__XBasicForLoopExpression__Group__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXBasicForLoopExpressionAccess().getGroup());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleXBasicForLoopExpression"
// $ANTLR start "entryRuleXWhileExpression"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3523:1: entryRuleXWhileExpression : ruleXWhileExpression EOF ;
public final void entryRuleXWhileExpression() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3524:1: ( ruleXWhileExpression EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3525:1: ruleXWhileExpression EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXWhileExpressionRule());
}
pushFollow(FollowSets000.FOLLOW_ruleXWhileExpression_in_entryRuleXWhileExpression7462);
ruleXWhileExpression();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXWhileExpressionRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleXWhileExpression7469); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleXWhileExpression"
// $ANTLR start "ruleXWhileExpression"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3532:1: ruleXWhileExpression : ( ( rule__XWhileExpression__Group__0 ) ) ;
public final void ruleXWhileExpression() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3536:2: ( ( ( rule__XWhileExpression__Group__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3537:1: ( ( rule__XWhileExpression__Group__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3537:1: ( ( rule__XWhileExpression__Group__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3538:1: ( rule__XWhileExpression__Group__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXWhileExpressionAccess().getGroup());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3539:1: ( rule__XWhileExpression__Group__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3539:2: rule__XWhileExpression__Group__0
{
pushFollow(FollowSets000.FOLLOW_rule__XWhileExpression__Group__0_in_ruleXWhileExpression7495);
rule__XWhileExpression__Group__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXWhileExpressionAccess().getGroup());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleXWhileExpression"
// $ANTLR start "entryRuleXDoWhileExpression"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3551:1: entryRuleXDoWhileExpression : ruleXDoWhileExpression EOF ;
public final void entryRuleXDoWhileExpression() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3552:1: ( ruleXDoWhileExpression EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3553:1: ruleXDoWhileExpression EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXDoWhileExpressionRule());
}
pushFollow(FollowSets000.FOLLOW_ruleXDoWhileExpression_in_entryRuleXDoWhileExpression7522);
ruleXDoWhileExpression();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXDoWhileExpressionRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleXDoWhileExpression7529); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleXDoWhileExpression"
// $ANTLR start "ruleXDoWhileExpression"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3560:1: ruleXDoWhileExpression : ( ( rule__XDoWhileExpression__Group__0 ) ) ;
public final void ruleXDoWhileExpression() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3564:2: ( ( ( rule__XDoWhileExpression__Group__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3565:1: ( ( rule__XDoWhileExpression__Group__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3565:1: ( ( rule__XDoWhileExpression__Group__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3566:1: ( rule__XDoWhileExpression__Group__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXDoWhileExpressionAccess().getGroup());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3567:1: ( rule__XDoWhileExpression__Group__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3567:2: rule__XDoWhileExpression__Group__0
{
pushFollow(FollowSets000.FOLLOW_rule__XDoWhileExpression__Group__0_in_ruleXDoWhileExpression7555);
rule__XDoWhileExpression__Group__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXDoWhileExpressionAccess().getGroup());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleXDoWhileExpression"
// $ANTLR start "entryRuleXBlockExpression"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3579:1: entryRuleXBlockExpression : ruleXBlockExpression EOF ;
public final void entryRuleXBlockExpression() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3580:1: ( ruleXBlockExpression EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3581:1: ruleXBlockExpression EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXBlockExpressionRule());
}
pushFollow(FollowSets000.FOLLOW_ruleXBlockExpression_in_entryRuleXBlockExpression7582);
ruleXBlockExpression();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXBlockExpressionRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleXBlockExpression7589); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleXBlockExpression"
// $ANTLR start "ruleXBlockExpression"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3588:1: ruleXBlockExpression : ( ( rule__XBlockExpression__Group__0 ) ) ;
public final void ruleXBlockExpression() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3592:2: ( ( ( rule__XBlockExpression__Group__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3593:1: ( ( rule__XBlockExpression__Group__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3593:1: ( ( rule__XBlockExpression__Group__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3594:1: ( rule__XBlockExpression__Group__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXBlockExpressionAccess().getGroup());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3595:1: ( rule__XBlockExpression__Group__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3595:2: rule__XBlockExpression__Group__0
{
pushFollow(FollowSets000.FOLLOW_rule__XBlockExpression__Group__0_in_ruleXBlockExpression7615);
rule__XBlockExpression__Group__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXBlockExpressionAccess().getGroup());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleXBlockExpression"
// $ANTLR start "entryRuleXExpressionOrVarDeclaration"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3607:1: entryRuleXExpressionOrVarDeclaration : ruleXExpressionOrVarDeclaration EOF ;
public final void entryRuleXExpressionOrVarDeclaration() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3608:1: ( ruleXExpressionOrVarDeclaration EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3609:1: ruleXExpressionOrVarDeclaration EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXExpressionOrVarDeclarationRule());
}
pushFollow(FollowSets000.FOLLOW_ruleXExpressionOrVarDeclaration_in_entryRuleXExpressionOrVarDeclaration7642);
ruleXExpressionOrVarDeclaration();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXExpressionOrVarDeclarationRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleXExpressionOrVarDeclaration7649); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleXExpressionOrVarDeclaration"
// $ANTLR start "ruleXExpressionOrVarDeclaration"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3616:1: ruleXExpressionOrVarDeclaration : ( ( rule__XExpressionOrVarDeclaration__Alternatives ) ) ;
public final void ruleXExpressionOrVarDeclaration() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3620:2: ( ( ( rule__XExpressionOrVarDeclaration__Alternatives ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3621:1: ( ( rule__XExpressionOrVarDeclaration__Alternatives ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3621:1: ( ( rule__XExpressionOrVarDeclaration__Alternatives ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3622:1: ( rule__XExpressionOrVarDeclaration__Alternatives )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXExpressionOrVarDeclarationAccess().getAlternatives());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3623:1: ( rule__XExpressionOrVarDeclaration__Alternatives )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3623:2: rule__XExpressionOrVarDeclaration__Alternatives
{
pushFollow(FollowSets000.FOLLOW_rule__XExpressionOrVarDeclaration__Alternatives_in_ruleXExpressionOrVarDeclaration7675);
rule__XExpressionOrVarDeclaration__Alternatives();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXExpressionOrVarDeclarationAccess().getAlternatives());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleXExpressionOrVarDeclaration"
// $ANTLR start "entryRuleXVariableDeclaration"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3635:1: entryRuleXVariableDeclaration : ruleXVariableDeclaration EOF ;
public final void entryRuleXVariableDeclaration() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3636:1: ( ruleXVariableDeclaration EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3637:1: ruleXVariableDeclaration EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXVariableDeclarationRule());
}
pushFollow(FollowSets000.FOLLOW_ruleXVariableDeclaration_in_entryRuleXVariableDeclaration7702);
ruleXVariableDeclaration();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXVariableDeclarationRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleXVariableDeclaration7709); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleXVariableDeclaration"
// $ANTLR start "ruleXVariableDeclaration"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3644:1: ruleXVariableDeclaration : ( ( rule__XVariableDeclaration__Group__0 ) ) ;
public final void ruleXVariableDeclaration() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3648:2: ( ( ( rule__XVariableDeclaration__Group__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3649:1: ( ( rule__XVariableDeclaration__Group__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3649:1: ( ( rule__XVariableDeclaration__Group__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3650:1: ( rule__XVariableDeclaration__Group__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXVariableDeclarationAccess().getGroup());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3651:1: ( rule__XVariableDeclaration__Group__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3651:2: rule__XVariableDeclaration__Group__0
{
pushFollow(FollowSets000.FOLLOW_rule__XVariableDeclaration__Group__0_in_ruleXVariableDeclaration7735);
rule__XVariableDeclaration__Group__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXVariableDeclarationAccess().getGroup());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleXVariableDeclaration"
// $ANTLR start "entryRuleJvmFormalParameter"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3663:1: entryRuleJvmFormalParameter : ruleJvmFormalParameter EOF ;
public final void entryRuleJvmFormalParameter() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3664:1: ( ruleJvmFormalParameter EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3665:1: ruleJvmFormalParameter EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getJvmFormalParameterRule());
}
pushFollow(FollowSets000.FOLLOW_ruleJvmFormalParameter_in_entryRuleJvmFormalParameter7762);
ruleJvmFormalParameter();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getJvmFormalParameterRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleJvmFormalParameter7769); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleJvmFormalParameter"
// $ANTLR start "ruleJvmFormalParameter"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3672:1: ruleJvmFormalParameter : ( ( rule__JvmFormalParameter__Group__0 ) ) ;
public final void ruleJvmFormalParameter() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3676:2: ( ( ( rule__JvmFormalParameter__Group__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3677:1: ( ( rule__JvmFormalParameter__Group__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3677:1: ( ( rule__JvmFormalParameter__Group__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3678:1: ( rule__JvmFormalParameter__Group__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getJvmFormalParameterAccess().getGroup());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3679:1: ( rule__JvmFormalParameter__Group__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3679:2: rule__JvmFormalParameter__Group__0
{
pushFollow(FollowSets000.FOLLOW_rule__JvmFormalParameter__Group__0_in_ruleJvmFormalParameter7795);
rule__JvmFormalParameter__Group__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getJvmFormalParameterAccess().getGroup());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleJvmFormalParameter"
// $ANTLR start "entryRuleFullJvmFormalParameter"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3691:1: entryRuleFullJvmFormalParameter : ruleFullJvmFormalParameter EOF ;
public final void entryRuleFullJvmFormalParameter() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3692:1: ( ruleFullJvmFormalParameter EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3693:1: ruleFullJvmFormalParameter EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getFullJvmFormalParameterRule());
}
pushFollow(FollowSets000.FOLLOW_ruleFullJvmFormalParameter_in_entryRuleFullJvmFormalParameter7822);
ruleFullJvmFormalParameter();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getFullJvmFormalParameterRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleFullJvmFormalParameter7829); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleFullJvmFormalParameter"
// $ANTLR start "ruleFullJvmFormalParameter"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3700:1: ruleFullJvmFormalParameter : ( ( rule__FullJvmFormalParameter__Group__0 ) ) ;
public final void ruleFullJvmFormalParameter() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3704:2: ( ( ( rule__FullJvmFormalParameter__Group__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3705:1: ( ( rule__FullJvmFormalParameter__Group__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3705:1: ( ( rule__FullJvmFormalParameter__Group__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3706:1: ( rule__FullJvmFormalParameter__Group__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getFullJvmFormalParameterAccess().getGroup());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3707:1: ( rule__FullJvmFormalParameter__Group__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3707:2: rule__FullJvmFormalParameter__Group__0
{
pushFollow(FollowSets000.FOLLOW_rule__FullJvmFormalParameter__Group__0_in_ruleFullJvmFormalParameter7855);
rule__FullJvmFormalParameter__Group__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getFullJvmFormalParameterAccess().getGroup());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleFullJvmFormalParameter"
// $ANTLR start "entryRuleXFeatureCall"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3719:1: entryRuleXFeatureCall : ruleXFeatureCall EOF ;
public final void entryRuleXFeatureCall() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3720:1: ( ruleXFeatureCall EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3721:1: ruleXFeatureCall EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXFeatureCallRule());
}
pushFollow(FollowSets000.FOLLOW_ruleXFeatureCall_in_entryRuleXFeatureCall7882);
ruleXFeatureCall();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXFeatureCallRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleXFeatureCall7889); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleXFeatureCall"
// $ANTLR start "ruleXFeatureCall"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3728:1: ruleXFeatureCall : ( ( rule__XFeatureCall__Group__0 ) ) ;
public final void ruleXFeatureCall() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3732:2: ( ( ( rule__XFeatureCall__Group__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3733:1: ( ( rule__XFeatureCall__Group__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3733:1: ( ( rule__XFeatureCall__Group__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3734:1: ( rule__XFeatureCall__Group__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXFeatureCallAccess().getGroup());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3735:1: ( rule__XFeatureCall__Group__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3735:2: rule__XFeatureCall__Group__0
{
pushFollow(FollowSets000.FOLLOW_rule__XFeatureCall__Group__0_in_ruleXFeatureCall7915);
rule__XFeatureCall__Group__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXFeatureCallAccess().getGroup());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleXFeatureCall"
// $ANTLR start "entryRuleFeatureCallID"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3747:1: entryRuleFeatureCallID : ruleFeatureCallID EOF ;
public final void entryRuleFeatureCallID() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3748:1: ( ruleFeatureCallID EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3749:1: ruleFeatureCallID EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getFeatureCallIDRule());
}
pushFollow(FollowSets000.FOLLOW_ruleFeatureCallID_in_entryRuleFeatureCallID7942);
ruleFeatureCallID();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getFeatureCallIDRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleFeatureCallID7949); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleFeatureCallID"
// $ANTLR start "ruleFeatureCallID"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3756:1: ruleFeatureCallID : ( ( rule__FeatureCallID__Alternatives ) ) ;
public final void ruleFeatureCallID() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3760:2: ( ( ( rule__FeatureCallID__Alternatives ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3761:1: ( ( rule__FeatureCallID__Alternatives ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3761:1: ( ( rule__FeatureCallID__Alternatives ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3762:1: ( rule__FeatureCallID__Alternatives )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getFeatureCallIDAccess().getAlternatives());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3763:1: ( rule__FeatureCallID__Alternatives )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3763:2: rule__FeatureCallID__Alternatives
{
pushFollow(FollowSets000.FOLLOW_rule__FeatureCallID__Alternatives_in_ruleFeatureCallID7975);
rule__FeatureCallID__Alternatives();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getFeatureCallIDAccess().getAlternatives());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleFeatureCallID"
// $ANTLR start "entryRuleIdOrSuper"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3775:1: entryRuleIdOrSuper : ruleIdOrSuper EOF ;
public final void entryRuleIdOrSuper() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3776:1: ( ruleIdOrSuper EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3777:1: ruleIdOrSuper EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getIdOrSuperRule());
}
pushFollow(FollowSets000.FOLLOW_ruleIdOrSuper_in_entryRuleIdOrSuper8002);
ruleIdOrSuper();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getIdOrSuperRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleIdOrSuper8009); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleIdOrSuper"
// $ANTLR start "ruleIdOrSuper"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3784:1: ruleIdOrSuper : ( ( rule__IdOrSuper__Alternatives ) ) ;
public final void ruleIdOrSuper() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3788:2: ( ( ( rule__IdOrSuper__Alternatives ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3789:1: ( ( rule__IdOrSuper__Alternatives ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3789:1: ( ( rule__IdOrSuper__Alternatives ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3790:1: ( rule__IdOrSuper__Alternatives )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getIdOrSuperAccess().getAlternatives());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3791:1: ( rule__IdOrSuper__Alternatives )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3791:2: rule__IdOrSuper__Alternatives
{
pushFollow(FollowSets000.FOLLOW_rule__IdOrSuper__Alternatives_in_ruleIdOrSuper8035);
rule__IdOrSuper__Alternatives();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getIdOrSuperAccess().getAlternatives());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleIdOrSuper"
// $ANTLR start "entryRuleXConstructorCall"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3803:1: entryRuleXConstructorCall : ruleXConstructorCall EOF ;
public final void entryRuleXConstructorCall() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3804:1: ( ruleXConstructorCall EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3805:1: ruleXConstructorCall EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXConstructorCallRule());
}
pushFollow(FollowSets000.FOLLOW_ruleXConstructorCall_in_entryRuleXConstructorCall8062);
ruleXConstructorCall();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXConstructorCallRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleXConstructorCall8069); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleXConstructorCall"
// $ANTLR start "ruleXConstructorCall"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3812:1: ruleXConstructorCall : ( ( rule__XConstructorCall__Group__0 ) ) ;
public final void ruleXConstructorCall() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3816:2: ( ( ( rule__XConstructorCall__Group__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3817:1: ( ( rule__XConstructorCall__Group__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3817:1: ( ( rule__XConstructorCall__Group__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3818:1: ( rule__XConstructorCall__Group__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXConstructorCallAccess().getGroup());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3819:1: ( rule__XConstructorCall__Group__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3819:2: rule__XConstructorCall__Group__0
{
pushFollow(FollowSets000.FOLLOW_rule__XConstructorCall__Group__0_in_ruleXConstructorCall8095);
rule__XConstructorCall__Group__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXConstructorCallAccess().getGroup());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleXConstructorCall"
// $ANTLR start "entryRuleXBooleanLiteral"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3831:1: entryRuleXBooleanLiteral : ruleXBooleanLiteral EOF ;
public final void entryRuleXBooleanLiteral() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3832:1: ( ruleXBooleanLiteral EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3833:1: ruleXBooleanLiteral EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXBooleanLiteralRule());
}
pushFollow(FollowSets000.FOLLOW_ruleXBooleanLiteral_in_entryRuleXBooleanLiteral8122);
ruleXBooleanLiteral();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXBooleanLiteralRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleXBooleanLiteral8129); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleXBooleanLiteral"
// $ANTLR start "ruleXBooleanLiteral"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3840:1: ruleXBooleanLiteral : ( ( rule__XBooleanLiteral__Group__0 ) ) ;
public final void ruleXBooleanLiteral() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3844:2: ( ( ( rule__XBooleanLiteral__Group__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3845:1: ( ( rule__XBooleanLiteral__Group__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3845:1: ( ( rule__XBooleanLiteral__Group__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3846:1: ( rule__XBooleanLiteral__Group__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXBooleanLiteralAccess().getGroup());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3847:1: ( rule__XBooleanLiteral__Group__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3847:2: rule__XBooleanLiteral__Group__0
{
pushFollow(FollowSets000.FOLLOW_rule__XBooleanLiteral__Group__0_in_ruleXBooleanLiteral8155);
rule__XBooleanLiteral__Group__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXBooleanLiteralAccess().getGroup());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleXBooleanLiteral"
// $ANTLR start "entryRuleXNullLiteral"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3859:1: entryRuleXNullLiteral : ruleXNullLiteral EOF ;
public final void entryRuleXNullLiteral() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3860:1: ( ruleXNullLiteral EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3861:1: ruleXNullLiteral EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXNullLiteralRule());
}
pushFollow(FollowSets000.FOLLOW_ruleXNullLiteral_in_entryRuleXNullLiteral8182);
ruleXNullLiteral();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXNullLiteralRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleXNullLiteral8189); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleXNullLiteral"
// $ANTLR start "ruleXNullLiteral"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3868:1: ruleXNullLiteral : ( ( rule__XNullLiteral__Group__0 ) ) ;
public final void ruleXNullLiteral() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3872:2: ( ( ( rule__XNullLiteral__Group__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3873:1: ( ( rule__XNullLiteral__Group__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3873:1: ( ( rule__XNullLiteral__Group__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3874:1: ( rule__XNullLiteral__Group__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXNullLiteralAccess().getGroup());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3875:1: ( rule__XNullLiteral__Group__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3875:2: rule__XNullLiteral__Group__0
{
pushFollow(FollowSets000.FOLLOW_rule__XNullLiteral__Group__0_in_ruleXNullLiteral8215);
rule__XNullLiteral__Group__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXNullLiteralAccess().getGroup());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleXNullLiteral"
// $ANTLR start "entryRuleXNumberLiteral"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3887:1: entryRuleXNumberLiteral : ruleXNumberLiteral EOF ;
public final void entryRuleXNumberLiteral() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3888:1: ( ruleXNumberLiteral EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3889:1: ruleXNumberLiteral EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXNumberLiteralRule());
}
pushFollow(FollowSets000.FOLLOW_ruleXNumberLiteral_in_entryRuleXNumberLiteral8242);
ruleXNumberLiteral();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXNumberLiteralRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleXNumberLiteral8249); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleXNumberLiteral"
// $ANTLR start "ruleXNumberLiteral"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3896:1: ruleXNumberLiteral : ( ( rule__XNumberLiteral__Group__0 ) ) ;
public final void ruleXNumberLiteral() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3900:2: ( ( ( rule__XNumberLiteral__Group__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3901:1: ( ( rule__XNumberLiteral__Group__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3901:1: ( ( rule__XNumberLiteral__Group__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3902:1: ( rule__XNumberLiteral__Group__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXNumberLiteralAccess().getGroup());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3903:1: ( rule__XNumberLiteral__Group__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3903:2: rule__XNumberLiteral__Group__0
{
pushFollow(FollowSets000.FOLLOW_rule__XNumberLiteral__Group__0_in_ruleXNumberLiteral8275);
rule__XNumberLiteral__Group__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXNumberLiteralAccess().getGroup());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleXNumberLiteral"
// $ANTLR start "entryRuleXStringLiteral"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3915:1: entryRuleXStringLiteral : ruleXStringLiteral EOF ;
public final void entryRuleXStringLiteral() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3916:1: ( ruleXStringLiteral EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3917:1: ruleXStringLiteral EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXStringLiteralRule());
}
pushFollow(FollowSets000.FOLLOW_ruleXStringLiteral_in_entryRuleXStringLiteral8302);
ruleXStringLiteral();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXStringLiteralRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleXStringLiteral8309); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleXStringLiteral"
// $ANTLR start "ruleXStringLiteral"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3924:1: ruleXStringLiteral : ( ( rule__XStringLiteral__Group__0 ) ) ;
public final void ruleXStringLiteral() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3928:2: ( ( ( rule__XStringLiteral__Group__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3929:1: ( ( rule__XStringLiteral__Group__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3929:1: ( ( rule__XStringLiteral__Group__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3930:1: ( rule__XStringLiteral__Group__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXStringLiteralAccess().getGroup());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3931:1: ( rule__XStringLiteral__Group__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3931:2: rule__XStringLiteral__Group__0
{
pushFollow(FollowSets000.FOLLOW_rule__XStringLiteral__Group__0_in_ruleXStringLiteral8335);
rule__XStringLiteral__Group__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXStringLiteralAccess().getGroup());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleXStringLiteral"
// $ANTLR start "entryRuleXTypeLiteral"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3943:1: entryRuleXTypeLiteral : ruleXTypeLiteral EOF ;
public final void entryRuleXTypeLiteral() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3944:1: ( ruleXTypeLiteral EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3945:1: ruleXTypeLiteral EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXTypeLiteralRule());
}
pushFollow(FollowSets000.FOLLOW_ruleXTypeLiteral_in_entryRuleXTypeLiteral8362);
ruleXTypeLiteral();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXTypeLiteralRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleXTypeLiteral8369); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleXTypeLiteral"
// $ANTLR start "ruleXTypeLiteral"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3952:1: ruleXTypeLiteral : ( ( rule__XTypeLiteral__Group__0 ) ) ;
public final void ruleXTypeLiteral() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3956:2: ( ( ( rule__XTypeLiteral__Group__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3957:1: ( ( rule__XTypeLiteral__Group__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3957:1: ( ( rule__XTypeLiteral__Group__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3958:1: ( rule__XTypeLiteral__Group__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXTypeLiteralAccess().getGroup());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3959:1: ( rule__XTypeLiteral__Group__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3959:2: rule__XTypeLiteral__Group__0
{
pushFollow(FollowSets000.FOLLOW_rule__XTypeLiteral__Group__0_in_ruleXTypeLiteral8395);
rule__XTypeLiteral__Group__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXTypeLiteralAccess().getGroup());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleXTypeLiteral"
// $ANTLR start "entryRuleXThrowExpression"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3971:1: entryRuleXThrowExpression : ruleXThrowExpression EOF ;
public final void entryRuleXThrowExpression() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3972:1: ( ruleXThrowExpression EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3973:1: ruleXThrowExpression EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXThrowExpressionRule());
}
pushFollow(FollowSets000.FOLLOW_ruleXThrowExpression_in_entryRuleXThrowExpression8422);
ruleXThrowExpression();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXThrowExpressionRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleXThrowExpression8429); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleXThrowExpression"
// $ANTLR start "ruleXThrowExpression"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3980:1: ruleXThrowExpression : ( ( rule__XThrowExpression__Group__0 ) ) ;
public final void ruleXThrowExpression() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3984:2: ( ( ( rule__XThrowExpression__Group__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3985:1: ( ( rule__XThrowExpression__Group__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3985:1: ( ( rule__XThrowExpression__Group__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3986:1: ( rule__XThrowExpression__Group__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXThrowExpressionAccess().getGroup());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3987:1: ( rule__XThrowExpression__Group__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3987:2: rule__XThrowExpression__Group__0
{
pushFollow(FollowSets000.FOLLOW_rule__XThrowExpression__Group__0_in_ruleXThrowExpression8455);
rule__XThrowExpression__Group__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXThrowExpressionAccess().getGroup());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleXThrowExpression"
// $ANTLR start "entryRuleXReturnExpression"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:3999:1: entryRuleXReturnExpression : ruleXReturnExpression EOF ;
public final void entryRuleXReturnExpression() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4000:1: ( ruleXReturnExpression EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4001:1: ruleXReturnExpression EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXReturnExpressionRule());
}
pushFollow(FollowSets000.FOLLOW_ruleXReturnExpression_in_entryRuleXReturnExpression8482);
ruleXReturnExpression();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXReturnExpressionRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleXReturnExpression8489); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleXReturnExpression"
// $ANTLR start "ruleXReturnExpression"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4008:1: ruleXReturnExpression : ( ( rule__XReturnExpression__Group__0 ) ) ;
public final void ruleXReturnExpression() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4012:2: ( ( ( rule__XReturnExpression__Group__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4013:1: ( ( rule__XReturnExpression__Group__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4013:1: ( ( rule__XReturnExpression__Group__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4014:1: ( rule__XReturnExpression__Group__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXReturnExpressionAccess().getGroup());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4015:1: ( rule__XReturnExpression__Group__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4015:2: rule__XReturnExpression__Group__0
{
pushFollow(FollowSets000.FOLLOW_rule__XReturnExpression__Group__0_in_ruleXReturnExpression8515);
rule__XReturnExpression__Group__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXReturnExpressionAccess().getGroup());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleXReturnExpression"
// $ANTLR start "entryRuleXTryCatchFinallyExpression"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4027:1: entryRuleXTryCatchFinallyExpression : ruleXTryCatchFinallyExpression EOF ;
public final void entryRuleXTryCatchFinallyExpression() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4028:1: ( ruleXTryCatchFinallyExpression EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4029:1: ruleXTryCatchFinallyExpression EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXTryCatchFinallyExpressionRule());
}
pushFollow(FollowSets000.FOLLOW_ruleXTryCatchFinallyExpression_in_entryRuleXTryCatchFinallyExpression8542);
ruleXTryCatchFinallyExpression();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXTryCatchFinallyExpressionRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleXTryCatchFinallyExpression8549); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleXTryCatchFinallyExpression"
// $ANTLR start "ruleXTryCatchFinallyExpression"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4036:1: ruleXTryCatchFinallyExpression : ( ( rule__XTryCatchFinallyExpression__Group__0 ) ) ;
public final void ruleXTryCatchFinallyExpression() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4040:2: ( ( ( rule__XTryCatchFinallyExpression__Group__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4041:1: ( ( rule__XTryCatchFinallyExpression__Group__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4041:1: ( ( rule__XTryCatchFinallyExpression__Group__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4042:1: ( rule__XTryCatchFinallyExpression__Group__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXTryCatchFinallyExpressionAccess().getGroup());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4043:1: ( rule__XTryCatchFinallyExpression__Group__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4043:2: rule__XTryCatchFinallyExpression__Group__0
{
pushFollow(FollowSets000.FOLLOW_rule__XTryCatchFinallyExpression__Group__0_in_ruleXTryCatchFinallyExpression8575);
rule__XTryCatchFinallyExpression__Group__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXTryCatchFinallyExpressionAccess().getGroup());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleXTryCatchFinallyExpression"
// $ANTLR start "entryRuleXSynchronizedExpression"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4055:1: entryRuleXSynchronizedExpression : ruleXSynchronizedExpression EOF ;
public final void entryRuleXSynchronizedExpression() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4056:1: ( ruleXSynchronizedExpression EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4057:1: ruleXSynchronizedExpression EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXSynchronizedExpressionRule());
}
pushFollow(FollowSets000.FOLLOW_ruleXSynchronizedExpression_in_entryRuleXSynchronizedExpression8602);
ruleXSynchronizedExpression();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXSynchronizedExpressionRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleXSynchronizedExpression8609); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleXSynchronizedExpression"
// $ANTLR start "ruleXSynchronizedExpression"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4064:1: ruleXSynchronizedExpression : ( ( rule__XSynchronizedExpression__Group__0 ) ) ;
public final void ruleXSynchronizedExpression() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4068:2: ( ( ( rule__XSynchronizedExpression__Group__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4069:1: ( ( rule__XSynchronizedExpression__Group__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4069:1: ( ( rule__XSynchronizedExpression__Group__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4070:1: ( rule__XSynchronizedExpression__Group__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXSynchronizedExpressionAccess().getGroup());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4071:1: ( rule__XSynchronizedExpression__Group__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4071:2: rule__XSynchronizedExpression__Group__0
{
pushFollow(FollowSets000.FOLLOW_rule__XSynchronizedExpression__Group__0_in_ruleXSynchronizedExpression8635);
rule__XSynchronizedExpression__Group__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXSynchronizedExpressionAccess().getGroup());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleXSynchronizedExpression"
// $ANTLR start "entryRuleXCatchClause"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4083:1: entryRuleXCatchClause : ruleXCatchClause EOF ;
public final void entryRuleXCatchClause() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4084:1: ( ruleXCatchClause EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4085:1: ruleXCatchClause EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXCatchClauseRule());
}
pushFollow(FollowSets000.FOLLOW_ruleXCatchClause_in_entryRuleXCatchClause8662);
ruleXCatchClause();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXCatchClauseRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleXCatchClause8669); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleXCatchClause"
// $ANTLR start "ruleXCatchClause"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4092:1: ruleXCatchClause : ( ( rule__XCatchClause__Group__0 ) ) ;
public final void ruleXCatchClause() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4096:2: ( ( ( rule__XCatchClause__Group__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4097:1: ( ( rule__XCatchClause__Group__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4097:1: ( ( rule__XCatchClause__Group__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4098:1: ( rule__XCatchClause__Group__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXCatchClauseAccess().getGroup());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4099:1: ( rule__XCatchClause__Group__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4099:2: rule__XCatchClause__Group__0
{
pushFollow(FollowSets000.FOLLOW_rule__XCatchClause__Group__0_in_ruleXCatchClause8695);
rule__XCatchClause__Group__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXCatchClauseAccess().getGroup());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleXCatchClause"
// $ANTLR start "entryRuleQualifiedName"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4111:1: entryRuleQualifiedName : ruleQualifiedName EOF ;
public final void entryRuleQualifiedName() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4112:1: ( ruleQualifiedName EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4113:1: ruleQualifiedName EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getQualifiedNameRule());
}
pushFollow(FollowSets000.FOLLOW_ruleQualifiedName_in_entryRuleQualifiedName8722);
ruleQualifiedName();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getQualifiedNameRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleQualifiedName8729); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleQualifiedName"
// $ANTLR start "ruleQualifiedName"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4120:1: ruleQualifiedName : ( ( rule__QualifiedName__Group__0 ) ) ;
public final void ruleQualifiedName() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4124:2: ( ( ( rule__QualifiedName__Group__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4125:1: ( ( rule__QualifiedName__Group__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4125:1: ( ( rule__QualifiedName__Group__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4126:1: ( rule__QualifiedName__Group__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getQualifiedNameAccess().getGroup());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4127:1: ( rule__QualifiedName__Group__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4127:2: rule__QualifiedName__Group__0
{
pushFollow(FollowSets000.FOLLOW_rule__QualifiedName__Group__0_in_ruleQualifiedName8755);
rule__QualifiedName__Group__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getQualifiedNameAccess().getGroup());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleQualifiedName"
// $ANTLR start "entryRuleNumber"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4139:1: entryRuleNumber : ruleNumber EOF ;
public final void entryRuleNumber() throws RecognitionException {
HiddenTokens myHiddenTokenState = ((XtextTokenStream)input).setHiddenTokens();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4143:1: ( ruleNumber EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4144:1: ruleNumber EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getNumberRule());
}
pushFollow(FollowSets000.FOLLOW_ruleNumber_in_entryRuleNumber8787);
ruleNumber();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getNumberRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleNumber8794); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
myHiddenTokenState.restore();
}
return ;
}
// $ANTLR end "entryRuleNumber"
// $ANTLR start "ruleNumber"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4154:1: ruleNumber : ( ( rule__Number__Alternatives ) ) ;
public final void ruleNumber() throws RecognitionException {
HiddenTokens myHiddenTokenState = ((XtextTokenStream)input).setHiddenTokens();
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4159:2: ( ( ( rule__Number__Alternatives ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4160:1: ( ( rule__Number__Alternatives ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4160:1: ( ( rule__Number__Alternatives ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4161:1: ( rule__Number__Alternatives )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getNumberAccess().getAlternatives());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4162:1: ( rule__Number__Alternatives )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4162:2: rule__Number__Alternatives
{
pushFollow(FollowSets000.FOLLOW_rule__Number__Alternatives_in_ruleNumber8824);
rule__Number__Alternatives();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getNumberAccess().getAlternatives());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
myHiddenTokenState.restore();
}
return ;
}
// $ANTLR end "ruleNumber"
// $ANTLR start "entryRuleJvmTypeReference"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4177:1: entryRuleJvmTypeReference : ruleJvmTypeReference EOF ;
public final void entryRuleJvmTypeReference() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4178:1: ( ruleJvmTypeReference EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4179:1: ruleJvmTypeReference EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getJvmTypeReferenceRule());
}
pushFollow(FollowSets000.FOLLOW_ruleJvmTypeReference_in_entryRuleJvmTypeReference8853);
ruleJvmTypeReference();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getJvmTypeReferenceRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleJvmTypeReference8860); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleJvmTypeReference"
// $ANTLR start "ruleJvmTypeReference"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4186:1: ruleJvmTypeReference : ( ( rule__JvmTypeReference__Alternatives ) ) ;
public final void ruleJvmTypeReference() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4190:2: ( ( ( rule__JvmTypeReference__Alternatives ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4191:1: ( ( rule__JvmTypeReference__Alternatives ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4191:1: ( ( rule__JvmTypeReference__Alternatives ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4192:1: ( rule__JvmTypeReference__Alternatives )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getJvmTypeReferenceAccess().getAlternatives());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4193:1: ( rule__JvmTypeReference__Alternatives )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4193:2: rule__JvmTypeReference__Alternatives
{
pushFollow(FollowSets000.FOLLOW_rule__JvmTypeReference__Alternatives_in_ruleJvmTypeReference8886);
rule__JvmTypeReference__Alternatives();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getJvmTypeReferenceAccess().getAlternatives());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleJvmTypeReference"
// $ANTLR start "entryRuleArrayBrackets"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4205:1: entryRuleArrayBrackets : ruleArrayBrackets EOF ;
public final void entryRuleArrayBrackets() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4206:1: ( ruleArrayBrackets EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4207:1: ruleArrayBrackets EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getArrayBracketsRule());
}
pushFollow(FollowSets000.FOLLOW_ruleArrayBrackets_in_entryRuleArrayBrackets8913);
ruleArrayBrackets();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getArrayBracketsRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleArrayBrackets8920); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleArrayBrackets"
// $ANTLR start "ruleArrayBrackets"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4214:1: ruleArrayBrackets : ( ( rule__ArrayBrackets__Group__0 ) ) ;
public final void ruleArrayBrackets() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4218:2: ( ( ( rule__ArrayBrackets__Group__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4219:1: ( ( rule__ArrayBrackets__Group__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4219:1: ( ( rule__ArrayBrackets__Group__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4220:1: ( rule__ArrayBrackets__Group__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getArrayBracketsAccess().getGroup());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4221:1: ( rule__ArrayBrackets__Group__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4221:2: rule__ArrayBrackets__Group__0
{
pushFollow(FollowSets000.FOLLOW_rule__ArrayBrackets__Group__0_in_ruleArrayBrackets8946);
rule__ArrayBrackets__Group__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getArrayBracketsAccess().getGroup());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleArrayBrackets"
// $ANTLR start "entryRuleXFunctionTypeRef"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4233:1: entryRuleXFunctionTypeRef : ruleXFunctionTypeRef EOF ;
public final void entryRuleXFunctionTypeRef() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4234:1: ( ruleXFunctionTypeRef EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4235:1: ruleXFunctionTypeRef EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXFunctionTypeRefRule());
}
pushFollow(FollowSets000.FOLLOW_ruleXFunctionTypeRef_in_entryRuleXFunctionTypeRef8973);
ruleXFunctionTypeRef();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXFunctionTypeRefRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleXFunctionTypeRef8980); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleXFunctionTypeRef"
// $ANTLR start "ruleXFunctionTypeRef"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4242:1: ruleXFunctionTypeRef : ( ( rule__XFunctionTypeRef__Group__0 ) ) ;
public final void ruleXFunctionTypeRef() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4246:2: ( ( ( rule__XFunctionTypeRef__Group__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4247:1: ( ( rule__XFunctionTypeRef__Group__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4247:1: ( ( rule__XFunctionTypeRef__Group__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4248:1: ( rule__XFunctionTypeRef__Group__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXFunctionTypeRefAccess().getGroup());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4249:1: ( rule__XFunctionTypeRef__Group__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4249:2: rule__XFunctionTypeRef__Group__0
{
pushFollow(FollowSets000.FOLLOW_rule__XFunctionTypeRef__Group__0_in_ruleXFunctionTypeRef9006);
rule__XFunctionTypeRef__Group__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXFunctionTypeRefAccess().getGroup());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleXFunctionTypeRef"
// $ANTLR start "entryRuleJvmParameterizedTypeReference"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4261:1: entryRuleJvmParameterizedTypeReference : ruleJvmParameterizedTypeReference EOF ;
public final void entryRuleJvmParameterizedTypeReference() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4262:1: ( ruleJvmParameterizedTypeReference EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4263:1: ruleJvmParameterizedTypeReference EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getJvmParameterizedTypeReferenceRule());
}
pushFollow(FollowSets000.FOLLOW_ruleJvmParameterizedTypeReference_in_entryRuleJvmParameterizedTypeReference9033);
ruleJvmParameterizedTypeReference();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getJvmParameterizedTypeReferenceRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleJvmParameterizedTypeReference9040); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleJvmParameterizedTypeReference"
// $ANTLR start "ruleJvmParameterizedTypeReference"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4270:1: ruleJvmParameterizedTypeReference : ( ( rule__JvmParameterizedTypeReference__Group__0 ) ) ;
public final void ruleJvmParameterizedTypeReference() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4274:2: ( ( ( rule__JvmParameterizedTypeReference__Group__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4275:1: ( ( rule__JvmParameterizedTypeReference__Group__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4275:1: ( ( rule__JvmParameterizedTypeReference__Group__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4276:1: ( rule__JvmParameterizedTypeReference__Group__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getJvmParameterizedTypeReferenceAccess().getGroup());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4277:1: ( rule__JvmParameterizedTypeReference__Group__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4277:2: rule__JvmParameterizedTypeReference__Group__0
{
pushFollow(FollowSets000.FOLLOW_rule__JvmParameterizedTypeReference__Group__0_in_ruleJvmParameterizedTypeReference9066);
rule__JvmParameterizedTypeReference__Group__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getJvmParameterizedTypeReferenceAccess().getGroup());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleJvmParameterizedTypeReference"
// $ANTLR start "entryRuleJvmArgumentTypeReference"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4289:1: entryRuleJvmArgumentTypeReference : ruleJvmArgumentTypeReference EOF ;
public final void entryRuleJvmArgumentTypeReference() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4290:1: ( ruleJvmArgumentTypeReference EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4291:1: ruleJvmArgumentTypeReference EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getJvmArgumentTypeReferenceRule());
}
pushFollow(FollowSets000.FOLLOW_ruleJvmArgumentTypeReference_in_entryRuleJvmArgumentTypeReference9093);
ruleJvmArgumentTypeReference();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getJvmArgumentTypeReferenceRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleJvmArgumentTypeReference9100); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleJvmArgumentTypeReference"
// $ANTLR start "ruleJvmArgumentTypeReference"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4298:1: ruleJvmArgumentTypeReference : ( ( rule__JvmArgumentTypeReference__Alternatives ) ) ;
public final void ruleJvmArgumentTypeReference() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4302:2: ( ( ( rule__JvmArgumentTypeReference__Alternatives ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4303:1: ( ( rule__JvmArgumentTypeReference__Alternatives ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4303:1: ( ( rule__JvmArgumentTypeReference__Alternatives ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4304:1: ( rule__JvmArgumentTypeReference__Alternatives )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getJvmArgumentTypeReferenceAccess().getAlternatives());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4305:1: ( rule__JvmArgumentTypeReference__Alternatives )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4305:2: rule__JvmArgumentTypeReference__Alternatives
{
pushFollow(FollowSets000.FOLLOW_rule__JvmArgumentTypeReference__Alternatives_in_ruleJvmArgumentTypeReference9126);
rule__JvmArgumentTypeReference__Alternatives();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getJvmArgumentTypeReferenceAccess().getAlternatives());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleJvmArgumentTypeReference"
// $ANTLR start "entryRuleJvmWildcardTypeReference"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4317:1: entryRuleJvmWildcardTypeReference : ruleJvmWildcardTypeReference EOF ;
public final void entryRuleJvmWildcardTypeReference() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4318:1: ( ruleJvmWildcardTypeReference EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4319:1: ruleJvmWildcardTypeReference EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getJvmWildcardTypeReferenceRule());
}
pushFollow(FollowSets000.FOLLOW_ruleJvmWildcardTypeReference_in_entryRuleJvmWildcardTypeReference9153);
ruleJvmWildcardTypeReference();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getJvmWildcardTypeReferenceRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleJvmWildcardTypeReference9160); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleJvmWildcardTypeReference"
// $ANTLR start "ruleJvmWildcardTypeReference"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4326:1: ruleJvmWildcardTypeReference : ( ( rule__JvmWildcardTypeReference__Group__0 ) ) ;
public final void ruleJvmWildcardTypeReference() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4330:2: ( ( ( rule__JvmWildcardTypeReference__Group__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4331:1: ( ( rule__JvmWildcardTypeReference__Group__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4331:1: ( ( rule__JvmWildcardTypeReference__Group__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4332:1: ( rule__JvmWildcardTypeReference__Group__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getJvmWildcardTypeReferenceAccess().getGroup());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4333:1: ( rule__JvmWildcardTypeReference__Group__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4333:2: rule__JvmWildcardTypeReference__Group__0
{
pushFollow(FollowSets000.FOLLOW_rule__JvmWildcardTypeReference__Group__0_in_ruleJvmWildcardTypeReference9186);
rule__JvmWildcardTypeReference__Group__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getJvmWildcardTypeReferenceAccess().getGroup());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleJvmWildcardTypeReference"
// $ANTLR start "entryRuleJvmUpperBound"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4345:1: entryRuleJvmUpperBound : ruleJvmUpperBound EOF ;
public final void entryRuleJvmUpperBound() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4346:1: ( ruleJvmUpperBound EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4347:1: ruleJvmUpperBound EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getJvmUpperBoundRule());
}
pushFollow(FollowSets000.FOLLOW_ruleJvmUpperBound_in_entryRuleJvmUpperBound9213);
ruleJvmUpperBound();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getJvmUpperBoundRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleJvmUpperBound9220); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleJvmUpperBound"
// $ANTLR start "ruleJvmUpperBound"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4354:1: ruleJvmUpperBound : ( ( rule__JvmUpperBound__Group__0 ) ) ;
public final void ruleJvmUpperBound() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4358:2: ( ( ( rule__JvmUpperBound__Group__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4359:1: ( ( rule__JvmUpperBound__Group__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4359:1: ( ( rule__JvmUpperBound__Group__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4360:1: ( rule__JvmUpperBound__Group__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getJvmUpperBoundAccess().getGroup());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4361:1: ( rule__JvmUpperBound__Group__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4361:2: rule__JvmUpperBound__Group__0
{
pushFollow(FollowSets000.FOLLOW_rule__JvmUpperBound__Group__0_in_ruleJvmUpperBound9246);
rule__JvmUpperBound__Group__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getJvmUpperBoundAccess().getGroup());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleJvmUpperBound"
// $ANTLR start "entryRuleJvmUpperBoundAnded"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4373:1: entryRuleJvmUpperBoundAnded : ruleJvmUpperBoundAnded EOF ;
public final void entryRuleJvmUpperBoundAnded() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4374:1: ( ruleJvmUpperBoundAnded EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4375:1: ruleJvmUpperBoundAnded EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getJvmUpperBoundAndedRule());
}
pushFollow(FollowSets000.FOLLOW_ruleJvmUpperBoundAnded_in_entryRuleJvmUpperBoundAnded9273);
ruleJvmUpperBoundAnded();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getJvmUpperBoundAndedRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleJvmUpperBoundAnded9280); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleJvmUpperBoundAnded"
// $ANTLR start "ruleJvmUpperBoundAnded"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4382:1: ruleJvmUpperBoundAnded : ( ( rule__JvmUpperBoundAnded__Group__0 ) ) ;
public final void ruleJvmUpperBoundAnded() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4386:2: ( ( ( rule__JvmUpperBoundAnded__Group__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4387:1: ( ( rule__JvmUpperBoundAnded__Group__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4387:1: ( ( rule__JvmUpperBoundAnded__Group__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4388:1: ( rule__JvmUpperBoundAnded__Group__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getJvmUpperBoundAndedAccess().getGroup());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4389:1: ( rule__JvmUpperBoundAnded__Group__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4389:2: rule__JvmUpperBoundAnded__Group__0
{
pushFollow(FollowSets000.FOLLOW_rule__JvmUpperBoundAnded__Group__0_in_ruleJvmUpperBoundAnded9306);
rule__JvmUpperBoundAnded__Group__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getJvmUpperBoundAndedAccess().getGroup());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleJvmUpperBoundAnded"
// $ANTLR start "entryRuleJvmLowerBound"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4401:1: entryRuleJvmLowerBound : ruleJvmLowerBound EOF ;
public final void entryRuleJvmLowerBound() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4402:1: ( ruleJvmLowerBound EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4403:1: ruleJvmLowerBound EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getJvmLowerBoundRule());
}
pushFollow(FollowSets000.FOLLOW_ruleJvmLowerBound_in_entryRuleJvmLowerBound9333);
ruleJvmLowerBound();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getJvmLowerBoundRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleJvmLowerBound9340); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleJvmLowerBound"
// $ANTLR start "ruleJvmLowerBound"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4410:1: ruleJvmLowerBound : ( ( rule__JvmLowerBound__Group__0 ) ) ;
public final void ruleJvmLowerBound() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4414:2: ( ( ( rule__JvmLowerBound__Group__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4415:1: ( ( rule__JvmLowerBound__Group__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4415:1: ( ( rule__JvmLowerBound__Group__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4416:1: ( rule__JvmLowerBound__Group__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getJvmLowerBoundAccess().getGroup());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4417:1: ( rule__JvmLowerBound__Group__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4417:2: rule__JvmLowerBound__Group__0
{
pushFollow(FollowSets000.FOLLOW_rule__JvmLowerBound__Group__0_in_ruleJvmLowerBound9366);
rule__JvmLowerBound__Group__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getJvmLowerBoundAccess().getGroup());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleJvmLowerBound"
// $ANTLR start "entryRuleJvmLowerBoundAnded"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4429:1: entryRuleJvmLowerBoundAnded : ruleJvmLowerBoundAnded EOF ;
public final void entryRuleJvmLowerBoundAnded() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4430:1: ( ruleJvmLowerBoundAnded EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4431:1: ruleJvmLowerBoundAnded EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getJvmLowerBoundAndedRule());
}
pushFollow(FollowSets000.FOLLOW_ruleJvmLowerBoundAnded_in_entryRuleJvmLowerBoundAnded9393);
ruleJvmLowerBoundAnded();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getJvmLowerBoundAndedRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleJvmLowerBoundAnded9400); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleJvmLowerBoundAnded"
// $ANTLR start "ruleJvmLowerBoundAnded"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4438:1: ruleJvmLowerBoundAnded : ( ( rule__JvmLowerBoundAnded__Group__0 ) ) ;
public final void ruleJvmLowerBoundAnded() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4442:2: ( ( ( rule__JvmLowerBoundAnded__Group__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4443:1: ( ( rule__JvmLowerBoundAnded__Group__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4443:1: ( ( rule__JvmLowerBoundAnded__Group__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4444:1: ( rule__JvmLowerBoundAnded__Group__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getJvmLowerBoundAndedAccess().getGroup());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4445:1: ( rule__JvmLowerBoundAnded__Group__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4445:2: rule__JvmLowerBoundAnded__Group__0
{
pushFollow(FollowSets000.FOLLOW_rule__JvmLowerBoundAnded__Group__0_in_ruleJvmLowerBoundAnded9426);
rule__JvmLowerBoundAnded__Group__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getJvmLowerBoundAndedAccess().getGroup());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleJvmLowerBoundAnded"
// $ANTLR start "entryRuleJvmTypeParameter"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4457:1: entryRuleJvmTypeParameter : ruleJvmTypeParameter EOF ;
public final void entryRuleJvmTypeParameter() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4458:1: ( ruleJvmTypeParameter EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4459:1: ruleJvmTypeParameter EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getJvmTypeParameterRule());
}
pushFollow(FollowSets000.FOLLOW_ruleJvmTypeParameter_in_entryRuleJvmTypeParameter9453);
ruleJvmTypeParameter();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getJvmTypeParameterRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleJvmTypeParameter9460); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleJvmTypeParameter"
// $ANTLR start "ruleJvmTypeParameter"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4466:1: ruleJvmTypeParameter : ( ( rule__JvmTypeParameter__Group__0 ) ) ;
public final void ruleJvmTypeParameter() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4470:2: ( ( ( rule__JvmTypeParameter__Group__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4471:1: ( ( rule__JvmTypeParameter__Group__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4471:1: ( ( rule__JvmTypeParameter__Group__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4472:1: ( rule__JvmTypeParameter__Group__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getJvmTypeParameterAccess().getGroup());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4473:1: ( rule__JvmTypeParameter__Group__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4473:2: rule__JvmTypeParameter__Group__0
{
pushFollow(FollowSets000.FOLLOW_rule__JvmTypeParameter__Group__0_in_ruleJvmTypeParameter9486);
rule__JvmTypeParameter__Group__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getJvmTypeParameterAccess().getGroup());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleJvmTypeParameter"
// $ANTLR start "entryRuleQualifiedNameWithWildcard"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4485:1: entryRuleQualifiedNameWithWildcard : ruleQualifiedNameWithWildcard EOF ;
public final void entryRuleQualifiedNameWithWildcard() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4486:1: ( ruleQualifiedNameWithWildcard EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4487:1: ruleQualifiedNameWithWildcard EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getQualifiedNameWithWildcardRule());
}
pushFollow(FollowSets000.FOLLOW_ruleQualifiedNameWithWildcard_in_entryRuleQualifiedNameWithWildcard9513);
ruleQualifiedNameWithWildcard();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getQualifiedNameWithWildcardRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleQualifiedNameWithWildcard9520); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleQualifiedNameWithWildcard"
// $ANTLR start "ruleQualifiedNameWithWildcard"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4494:1: ruleQualifiedNameWithWildcard : ( ( rule__QualifiedNameWithWildcard__Group__0 ) ) ;
public final void ruleQualifiedNameWithWildcard() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4498:2: ( ( ( rule__QualifiedNameWithWildcard__Group__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4499:1: ( ( rule__QualifiedNameWithWildcard__Group__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4499:1: ( ( rule__QualifiedNameWithWildcard__Group__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4500:1: ( rule__QualifiedNameWithWildcard__Group__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getQualifiedNameWithWildcardAccess().getGroup());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4501:1: ( rule__QualifiedNameWithWildcard__Group__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4501:2: rule__QualifiedNameWithWildcard__Group__0
{
pushFollow(FollowSets000.FOLLOW_rule__QualifiedNameWithWildcard__Group__0_in_ruleQualifiedNameWithWildcard9546);
rule__QualifiedNameWithWildcard__Group__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getQualifiedNameWithWildcardAccess().getGroup());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleQualifiedNameWithWildcard"
// $ANTLR start "entryRuleValidID"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4513:1: entryRuleValidID : ruleValidID EOF ;
public final void entryRuleValidID() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4514:1: ( ruleValidID EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4515:1: ruleValidID EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getValidIDRule());
}
pushFollow(FollowSets000.FOLLOW_ruleValidID_in_entryRuleValidID9573);
ruleValidID();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getValidIDRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleValidID9580); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleValidID"
// $ANTLR start "ruleValidID"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4522:1: ruleValidID : ( RULE_ID ) ;
public final void ruleValidID() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4526:2: ( ( RULE_ID ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4527:1: ( RULE_ID )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4527:1: ( RULE_ID )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4528:1: RULE_ID
{
if ( state.backtracking==0 ) {
before(grammarAccess.getValidIDAccess().getIDTerminalRuleCall());
}
match(input,RULE_ID,FollowSets000.FOLLOW_RULE_ID_in_ruleValidID9606); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getValidIDAccess().getIDTerminalRuleCall());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleValidID"
// $ANTLR start "entryRuleXImportSection"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4541:1: entryRuleXImportSection : ruleXImportSection EOF ;
public final void entryRuleXImportSection() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4542:1: ( ruleXImportSection EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4543:1: ruleXImportSection EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXImportSectionRule());
}
pushFollow(FollowSets000.FOLLOW_ruleXImportSection_in_entryRuleXImportSection9632);
ruleXImportSection();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXImportSectionRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleXImportSection9639); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleXImportSection"
// $ANTLR start "ruleXImportSection"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4550:1: ruleXImportSection : ( ( ( rule__XImportSection__ImportDeclarationsAssignment ) ) ( ( rule__XImportSection__ImportDeclarationsAssignment )* ) ) ;
public final void ruleXImportSection() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4554:2: ( ( ( ( rule__XImportSection__ImportDeclarationsAssignment ) ) ( ( rule__XImportSection__ImportDeclarationsAssignment )* ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4555:1: ( ( ( rule__XImportSection__ImportDeclarationsAssignment ) ) ( ( rule__XImportSection__ImportDeclarationsAssignment )* ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4555:1: ( ( ( rule__XImportSection__ImportDeclarationsAssignment ) ) ( ( rule__XImportSection__ImportDeclarationsAssignment )* ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4556:1: ( ( rule__XImportSection__ImportDeclarationsAssignment ) ) ( ( rule__XImportSection__ImportDeclarationsAssignment )* )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4556:1: ( ( rule__XImportSection__ImportDeclarationsAssignment ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4557:1: ( rule__XImportSection__ImportDeclarationsAssignment )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXImportSectionAccess().getImportDeclarationsAssignment());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4558:1: ( rule__XImportSection__ImportDeclarationsAssignment )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4558:2: rule__XImportSection__ImportDeclarationsAssignment
{
pushFollow(FollowSets000.FOLLOW_rule__XImportSection__ImportDeclarationsAssignment_in_ruleXImportSection9667);
rule__XImportSection__ImportDeclarationsAssignment();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXImportSectionAccess().getImportDeclarationsAssignment());
}
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4561:1: ( ( rule__XImportSection__ImportDeclarationsAssignment )* )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4562:1: ( rule__XImportSection__ImportDeclarationsAssignment )*
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXImportSectionAccess().getImportDeclarationsAssignment());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4563:1: ( rule__XImportSection__ImportDeclarationsAssignment )*
loop1:
do {
int alt1=2;
int LA1_0 = input.LA(1);
if ( (LA1_0==47) ) {
alt1=1;
}
switch (alt1) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4563:2: rule__XImportSection__ImportDeclarationsAssignment
{
pushFollow(FollowSets000.FOLLOW_rule__XImportSection__ImportDeclarationsAssignment_in_ruleXImportSection9679);
rule__XImportSection__ImportDeclarationsAssignment();
state._fsp--;
if (state.failed) return ;
}
break;
default :
break loop1;
}
} while (true);
if ( state.backtracking==0 ) {
after(grammarAccess.getXImportSectionAccess().getImportDeclarationsAssignment());
}
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleXImportSection"
// $ANTLR start "entryRuleXImportDeclaration"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4576:1: entryRuleXImportDeclaration : ruleXImportDeclaration EOF ;
public final void entryRuleXImportDeclaration() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4577:1: ( ruleXImportDeclaration EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4578:1: ruleXImportDeclaration EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXImportDeclarationRule());
}
pushFollow(FollowSets000.FOLLOW_ruleXImportDeclaration_in_entryRuleXImportDeclaration9709);
ruleXImportDeclaration();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXImportDeclarationRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleXImportDeclaration9716); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleXImportDeclaration"
// $ANTLR start "ruleXImportDeclaration"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4585:1: ruleXImportDeclaration : ( ( rule__XImportDeclaration__Group__0 ) ) ;
public final void ruleXImportDeclaration() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4589:2: ( ( ( rule__XImportDeclaration__Group__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4590:1: ( ( rule__XImportDeclaration__Group__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4590:1: ( ( rule__XImportDeclaration__Group__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4591:1: ( rule__XImportDeclaration__Group__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXImportDeclarationAccess().getGroup());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4592:1: ( rule__XImportDeclaration__Group__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4592:2: rule__XImportDeclaration__Group__0
{
pushFollow(FollowSets000.FOLLOW_rule__XImportDeclaration__Group__0_in_ruleXImportDeclaration9742);
rule__XImportDeclaration__Group__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXImportDeclarationAccess().getGroup());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleXImportDeclaration"
// $ANTLR start "entryRuleQualifiedNameInStaticImport"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4604:1: entryRuleQualifiedNameInStaticImport : ruleQualifiedNameInStaticImport EOF ;
public final void entryRuleQualifiedNameInStaticImport() throws RecognitionException {
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4605:1: ( ruleQualifiedNameInStaticImport EOF )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4606:1: ruleQualifiedNameInStaticImport EOF
{
if ( state.backtracking==0 ) {
before(grammarAccess.getQualifiedNameInStaticImportRule());
}
pushFollow(FollowSets000.FOLLOW_ruleQualifiedNameInStaticImport_in_entryRuleQualifiedNameInStaticImport9769);
ruleQualifiedNameInStaticImport();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getQualifiedNameInStaticImportRule());
}
match(input,EOF,FollowSets000.FOLLOW_EOF_in_entryRuleQualifiedNameInStaticImport9776); if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "entryRuleQualifiedNameInStaticImport"
// $ANTLR start "ruleQualifiedNameInStaticImport"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4613:1: ruleQualifiedNameInStaticImport : ( ( ( rule__QualifiedNameInStaticImport__Group__0 ) ) ( ( rule__QualifiedNameInStaticImport__Group__0 )* ) ) ;
public final void ruleQualifiedNameInStaticImport() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4617:2: ( ( ( ( rule__QualifiedNameInStaticImport__Group__0 ) ) ( ( rule__QualifiedNameInStaticImport__Group__0 )* ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4618:1: ( ( ( rule__QualifiedNameInStaticImport__Group__0 ) ) ( ( rule__QualifiedNameInStaticImport__Group__0 )* ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4618:1: ( ( ( rule__QualifiedNameInStaticImport__Group__0 ) ) ( ( rule__QualifiedNameInStaticImport__Group__0 )* ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4619:1: ( ( rule__QualifiedNameInStaticImport__Group__0 ) ) ( ( rule__QualifiedNameInStaticImport__Group__0 )* )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4619:1: ( ( rule__QualifiedNameInStaticImport__Group__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4620:1: ( rule__QualifiedNameInStaticImport__Group__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getQualifiedNameInStaticImportAccess().getGroup());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4621:1: ( rule__QualifiedNameInStaticImport__Group__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4621:2: rule__QualifiedNameInStaticImport__Group__0
{
pushFollow(FollowSets000.FOLLOW_rule__QualifiedNameInStaticImport__Group__0_in_ruleQualifiedNameInStaticImport9804);
rule__QualifiedNameInStaticImport__Group__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getQualifiedNameInStaticImportAccess().getGroup());
}
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4624:1: ( ( rule__QualifiedNameInStaticImport__Group__0 )* )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4625:1: ( rule__QualifiedNameInStaticImport__Group__0 )*
{
if ( state.backtracking==0 ) {
before(grammarAccess.getQualifiedNameInStaticImportAccess().getGroup());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4626:1: ( rule__QualifiedNameInStaticImport__Group__0 )*
loop2:
do {
int alt2=2;
int LA2_0 = input.LA(1);
if ( (LA2_0==RULE_ID) ) {
int LA2_2 = input.LA(2);
if ( (LA2_2==43) ) {
alt2=1;
}
}
switch (alt2) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4626:2: rule__QualifiedNameInStaticImport__Group__0
{
pushFollow(FollowSets000.FOLLOW_rule__QualifiedNameInStaticImport__Group__0_in_ruleQualifiedNameInStaticImport9816);
rule__QualifiedNameInStaticImport__Group__0();
state._fsp--;
if (state.failed) return ;
}
break;
default :
break loop2;
}
} while (true);
if ( state.backtracking==0 ) {
after(grammarAccess.getQualifiedNameInStaticImportAccess().getGroup());
}
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleQualifiedNameInStaticImport"
// $ANTLR start "ruleIdGeneratorStrategy"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4640:1: ruleIdGeneratorStrategy : ( ( rule__IdGeneratorStrategy__Alternatives ) ) ;
public final void ruleIdGeneratorStrategy() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4644:1: ( ( ( rule__IdGeneratorStrategy__Alternatives ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4645:1: ( ( rule__IdGeneratorStrategy__Alternatives ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4645:1: ( ( rule__IdGeneratorStrategy__Alternatives ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4646:1: ( rule__IdGeneratorStrategy__Alternatives )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getIdGeneratorStrategyAccess().getAlternatives());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4647:1: ( rule__IdGeneratorStrategy__Alternatives )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4647:2: rule__IdGeneratorStrategy__Alternatives
{
pushFollow(FollowSets000.FOLLOW_rule__IdGeneratorStrategy__Alternatives_in_ruleIdGeneratorStrategy9856);
rule__IdGeneratorStrategy__Alternatives();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getIdGeneratorStrategyAccess().getAlternatives());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleIdGeneratorStrategy"
// $ANTLR start "ruleCardinality"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4659:1: ruleCardinality : ( ( rule__Cardinality__Alternatives ) ) ;
public final void ruleCardinality() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4663:1: ( ( ( rule__Cardinality__Alternatives ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4664:1: ( ( rule__Cardinality__Alternatives ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4664:1: ( ( rule__Cardinality__Alternatives ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4665:1: ( rule__Cardinality__Alternatives )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getCardinalityAccess().getAlternatives());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4666:1: ( rule__Cardinality__Alternatives )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4666:2: rule__Cardinality__Alternatives
{
pushFollow(FollowSets000.FOLLOW_rule__Cardinality__Alternatives_in_ruleCardinality9892);
rule__Cardinality__Alternatives();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getCardinalityAccess().getAlternatives());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleCardinality"
// $ANTLR start "ruleSimpleTypes"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4678:1: ruleSimpleTypes : ( ( rule__SimpleTypes__Alternatives ) ) ;
public final void ruleSimpleTypes() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4682:1: ( ( ( rule__SimpleTypes__Alternatives ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4683:1: ( ( rule__SimpleTypes__Alternatives ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4683:1: ( ( rule__SimpleTypes__Alternatives ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4684:1: ( rule__SimpleTypes__Alternatives )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getSimpleTypesAccess().getAlternatives());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4685:1: ( rule__SimpleTypes__Alternatives )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4685:2: rule__SimpleTypes__Alternatives
{
pushFollow(FollowSets000.FOLLOW_rule__SimpleTypes__Alternatives_in_ruleSimpleTypes9928);
rule__SimpleTypes__Alternatives();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getSimpleTypesAccess().getAlternatives());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleSimpleTypes"
// $ANTLR start "ruleIntegerControlInputType"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4697:1: ruleIntegerControlInputType : ( ( rule__IntegerControlInputType__Alternatives ) ) ;
public final void ruleIntegerControlInputType() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4701:1: ( ( ( rule__IntegerControlInputType__Alternatives ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4702:1: ( ( rule__IntegerControlInputType__Alternatives ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4702:1: ( ( rule__IntegerControlInputType__Alternatives ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4703:1: ( rule__IntegerControlInputType__Alternatives )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getIntegerControlInputTypeAccess().getAlternatives());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4704:1: ( rule__IntegerControlInputType__Alternatives )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4704:2: rule__IntegerControlInputType__Alternatives
{
pushFollow(FollowSets000.FOLLOW_rule__IntegerControlInputType__Alternatives_in_ruleIntegerControlInputType9964);
rule__IntegerControlInputType__Alternatives();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getIntegerControlInputTypeAccess().getAlternatives());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleIntegerControlInputType"
// $ANTLR start "ruleReferenceControlType"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4716:1: ruleReferenceControlType : ( ( rule__ReferenceControlType__Alternatives ) ) ;
public final void ruleReferenceControlType() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4720:1: ( ( ( rule__ReferenceControlType__Alternatives ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4721:1: ( ( rule__ReferenceControlType__Alternatives ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4721:1: ( ( rule__ReferenceControlType__Alternatives ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4722:1: ( rule__ReferenceControlType__Alternatives )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getReferenceControlTypeAccess().getAlternatives());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4723:1: ( rule__ReferenceControlType__Alternatives )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4723:2: rule__ReferenceControlType__Alternatives
{
pushFollow(FollowSets000.FOLLOW_rule__ReferenceControlType__Alternatives_in_ruleReferenceControlType10000);
rule__ReferenceControlType__Alternatives();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getReferenceControlTypeAccess().getAlternatives());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "ruleReferenceControlType"
// $ANTLR start "rule__ModelRoot__Alternatives"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4734:1: rule__ModelRoot__Alternatives : ( ( ( rule__ModelRoot__Group_0__0 ) ) | ( rulePackageDeclaration ) );
public final void rule__ModelRoot__Alternatives() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4738:1: ( ( ( rule__ModelRoot__Group_0__0 ) ) | ( rulePackageDeclaration ) )
int alt3=2;
int LA3_0 = input.LA(1);
if ( (LA3_0==47||LA3_0==66) ) {
alt3=1;
}
else if ( (LA3_0==69) ) {
alt3=2;
}
else {
if (state.backtracking>0) {state.failed=true; return ;}
NoViableAltException nvae =
new NoViableAltException("", 3, 0, input);
throw nvae;
}
switch (alt3) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4739:1: ( ( rule__ModelRoot__Group_0__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4739:1: ( ( rule__ModelRoot__Group_0__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4740:1: ( rule__ModelRoot__Group_0__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getModelRootAccess().getGroup_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4741:1: ( rule__ModelRoot__Group_0__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4741:2: rule__ModelRoot__Group_0__0
{
pushFollow(FollowSets000.FOLLOW_rule__ModelRoot__Group_0__0_in_rule__ModelRoot__Alternatives10035);
rule__ModelRoot__Group_0__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getModelRootAccess().getGroup_0());
}
}
}
break;
case 2 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4745:6: ( rulePackageDeclaration )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4745:6: ( rulePackageDeclaration )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4746:1: rulePackageDeclaration
{
if ( state.backtracking==0 ) {
before(grammarAccess.getModelRootAccess().getPackageDeclarationParserRuleCall_1());
}
pushFollow(FollowSets000.FOLLOW_rulePackageDeclaration_in_rule__ModelRoot__Alternatives10053);
rulePackageDeclaration();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getModelRootAccess().getPackageDeclarationParserRuleCall_1());
}
}
}
break;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ModelRoot__Alternatives"
// $ANTLR start "rule__AbstractElement__Alternatives"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4756:1: rule__AbstractElement__Alternatives : ( ( rulePackageDeclaration ) | ( ruleValueObject ) | ( ruleDatatype ) | ( ruleEntity ) | ( ruleEnumeration ) | ( ruleModuleDefinition ) | ( ruleModule ) | ( ruleService ) | ( ruleDictionary ) | ( ruleNavigationNode ) );
public final void rule__AbstractElement__Alternatives() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4760:1: ( ( rulePackageDeclaration ) | ( ruleValueObject ) | ( ruleDatatype ) | ( ruleEntity ) | ( ruleEnumeration ) | ( ruleModuleDefinition ) | ( ruleModule ) | ( ruleService ) | ( ruleDictionary ) | ( ruleNavigationNode ) )
int alt4=10;
switch ( input.LA(1) ) {
case 69:
{
alt4=1;
}
break;
case 80:
{
alt4=2;
}
break;
case 82:
case 86:
case 87:
case 88:
case 90:
case 92:
case 93:
case 95:
case 97:
case 99:
case 100:
{
alt4=3;
}
break;
case 78:
{
alt4=4;
}
break;
case 70:
{
alt4=5;
}
break;
case 140:
{
alt4=6;
}
break;
case 139:
{
alt4=7;
}
break;
case 146:
{
alt4=8;
}
break;
case 101:
{
alt4=9;
}
break;
case 148:
{
alt4=10;
}
break;
default:
if (state.backtracking>0) {state.failed=true; return ;}
NoViableAltException nvae =
new NoViableAltException("", 4, 0, input);
throw nvae;
}
switch (alt4) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4761:1: ( rulePackageDeclaration )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4761:1: ( rulePackageDeclaration )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4762:1: rulePackageDeclaration
{
if ( state.backtracking==0 ) {
before(grammarAccess.getAbstractElementAccess().getPackageDeclarationParserRuleCall_0());
}
pushFollow(FollowSets000.FOLLOW_rulePackageDeclaration_in_rule__AbstractElement__Alternatives10085);
rulePackageDeclaration();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getAbstractElementAccess().getPackageDeclarationParserRuleCall_0());
}
}
}
break;
case 2 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4767:6: ( ruleValueObject )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4767:6: ( ruleValueObject )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4768:1: ruleValueObject
{
if ( state.backtracking==0 ) {
before(grammarAccess.getAbstractElementAccess().getValueObjectParserRuleCall_1());
}
pushFollow(FollowSets000.FOLLOW_ruleValueObject_in_rule__AbstractElement__Alternatives10102);
ruleValueObject();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getAbstractElementAccess().getValueObjectParserRuleCall_1());
}
}
}
break;
case 3 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4773:6: ( ruleDatatype )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4773:6: ( ruleDatatype )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4774:1: ruleDatatype
{
if ( state.backtracking==0 ) {
before(grammarAccess.getAbstractElementAccess().getDatatypeParserRuleCall_2());
}
pushFollow(FollowSets000.FOLLOW_ruleDatatype_in_rule__AbstractElement__Alternatives10119);
ruleDatatype();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getAbstractElementAccess().getDatatypeParserRuleCall_2());
}
}
}
break;
case 4 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4779:6: ( ruleEntity )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4779:6: ( ruleEntity )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4780:1: ruleEntity
{
if ( state.backtracking==0 ) {
before(grammarAccess.getAbstractElementAccess().getEntityParserRuleCall_3());
}
pushFollow(FollowSets000.FOLLOW_ruleEntity_in_rule__AbstractElement__Alternatives10136);
ruleEntity();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getAbstractElementAccess().getEntityParserRuleCall_3());
}
}
}
break;
case 5 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4785:6: ( ruleEnumeration )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4785:6: ( ruleEnumeration )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4786:1: ruleEnumeration
{
if ( state.backtracking==0 ) {
before(grammarAccess.getAbstractElementAccess().getEnumerationParserRuleCall_4());
}
pushFollow(FollowSets000.FOLLOW_ruleEnumeration_in_rule__AbstractElement__Alternatives10153);
ruleEnumeration();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getAbstractElementAccess().getEnumerationParserRuleCall_4());
}
}
}
break;
case 6 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4791:6: ( ruleModuleDefinition )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4791:6: ( ruleModuleDefinition )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4792:1: ruleModuleDefinition
{
if ( state.backtracking==0 ) {
before(grammarAccess.getAbstractElementAccess().getModuleDefinitionParserRuleCall_5());
}
pushFollow(FollowSets000.FOLLOW_ruleModuleDefinition_in_rule__AbstractElement__Alternatives10170);
ruleModuleDefinition();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getAbstractElementAccess().getModuleDefinitionParserRuleCall_5());
}
}
}
break;
case 7 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4797:6: ( ruleModule )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4797:6: ( ruleModule )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4798:1: ruleModule
{
if ( state.backtracking==0 ) {
before(grammarAccess.getAbstractElementAccess().getModuleParserRuleCall_6());
}
pushFollow(FollowSets000.FOLLOW_ruleModule_in_rule__AbstractElement__Alternatives10187);
ruleModule();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getAbstractElementAccess().getModuleParserRuleCall_6());
}
}
}
break;
case 8 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4803:6: ( ruleService )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4803:6: ( ruleService )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4804:1: ruleService
{
if ( state.backtracking==0 ) {
before(grammarAccess.getAbstractElementAccess().getServiceParserRuleCall_7());
}
pushFollow(FollowSets000.FOLLOW_ruleService_in_rule__AbstractElement__Alternatives10204);
ruleService();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getAbstractElementAccess().getServiceParserRuleCall_7());
}
}
}
break;
case 9 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4809:6: ( ruleDictionary )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4809:6: ( ruleDictionary )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4810:1: ruleDictionary
{
if ( state.backtracking==0 ) {
before(grammarAccess.getAbstractElementAccess().getDictionaryParserRuleCall_8());
}
pushFollow(FollowSets000.FOLLOW_ruleDictionary_in_rule__AbstractElement__Alternatives10221);
ruleDictionary();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getAbstractElementAccess().getDictionaryParserRuleCall_8());
}
}
}
break;
case 10 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4815:6: ( ruleNavigationNode )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4815:6: ( ruleNavigationNode )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4816:1: ruleNavigationNode
{
if ( state.backtracking==0 ) {
before(grammarAccess.getAbstractElementAccess().getNavigationNodeParserRuleCall_9());
}
pushFollow(FollowSets000.FOLLOW_ruleNavigationNode_in_rule__AbstractElement__Alternatives10238);
ruleNavigationNode();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getAbstractElementAccess().getNavigationNodeParserRuleCall_9());
}
}
}
break;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__AbstractElement__Alternatives"
// $ANTLR start "rule__EntityOptions__Alternatives"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4826:1: rule__EntityOptions__Alternatives : ( ( ruleEntityDisableIdField ) | ( ruleEntityLabelField ) | ( ruleEntityPluralLabelField ) | ( ruleEntityNaturalKeyFields ) | ( ruleEntityHierarchical ) );
public final void rule__EntityOptions__Alternatives() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4830:1: ( ( ruleEntityDisableIdField ) | ( ruleEntityLabelField ) | ( ruleEntityPluralLabelField ) | ( ruleEntityNaturalKeyFields ) | ( ruleEntityHierarchical ) )
int alt5=5;
switch ( input.LA(1) ) {
case 74:
{
alt5=1;
}
break;
case 75:
{
alt5=2;
}
break;
case 76:
{
alt5=3;
}
break;
case 71:
{
alt5=4;
}
break;
case 73:
{
alt5=5;
}
break;
default:
if (state.backtracking>0) {state.failed=true; return ;}
NoViableAltException nvae =
new NoViableAltException("", 5, 0, input);
throw nvae;
}
switch (alt5) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4831:1: ( ruleEntityDisableIdField )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4831:1: ( ruleEntityDisableIdField )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4832:1: ruleEntityDisableIdField
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEntityOptionsAccess().getEntityDisableIdFieldParserRuleCall_0());
}
pushFollow(FollowSets000.FOLLOW_ruleEntityDisableIdField_in_rule__EntityOptions__Alternatives10270);
ruleEntityDisableIdField();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getEntityOptionsAccess().getEntityDisableIdFieldParserRuleCall_0());
}
}
}
break;
case 2 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4837:6: ( ruleEntityLabelField )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4837:6: ( ruleEntityLabelField )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4838:1: ruleEntityLabelField
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEntityOptionsAccess().getEntityLabelFieldParserRuleCall_1());
}
pushFollow(FollowSets000.FOLLOW_ruleEntityLabelField_in_rule__EntityOptions__Alternatives10287);
ruleEntityLabelField();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getEntityOptionsAccess().getEntityLabelFieldParserRuleCall_1());
}
}
}
break;
case 3 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4843:6: ( ruleEntityPluralLabelField )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4843:6: ( ruleEntityPluralLabelField )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4844:1: ruleEntityPluralLabelField
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEntityOptionsAccess().getEntityPluralLabelFieldParserRuleCall_2());
}
pushFollow(FollowSets000.FOLLOW_ruleEntityPluralLabelField_in_rule__EntityOptions__Alternatives10304);
ruleEntityPluralLabelField();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getEntityOptionsAccess().getEntityPluralLabelFieldParserRuleCall_2());
}
}
}
break;
case 4 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4849:6: ( ruleEntityNaturalKeyFields )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4849:6: ( ruleEntityNaturalKeyFields )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4850:1: ruleEntityNaturalKeyFields
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEntityOptionsAccess().getEntityNaturalKeyFieldsParserRuleCall_3());
}
pushFollow(FollowSets000.FOLLOW_ruleEntityNaturalKeyFields_in_rule__EntityOptions__Alternatives10321);
ruleEntityNaturalKeyFields();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getEntityOptionsAccess().getEntityNaturalKeyFieldsParserRuleCall_3());
}
}
}
break;
case 5 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4855:6: ( ruleEntityHierarchical )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4855:6: ( ruleEntityHierarchical )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4856:1: ruleEntityHierarchical
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEntityOptionsAccess().getEntityHierarchicalParserRuleCall_4());
}
pushFollow(FollowSets000.FOLLOW_ruleEntityHierarchical_in_rule__EntityOptions__Alternatives10338);
ruleEntityHierarchical();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getEntityOptionsAccess().getEntityHierarchicalParserRuleCall_4());
}
}
}
break;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EntityOptions__Alternatives"
// $ANTLR start "rule__Datatype__Alternatives"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4866:1: rule__Datatype__Alternatives : ( ( ruleSimpleDataType ) | ( ruleEntityDataType ) );
public final void rule__Datatype__Alternatives() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4870:1: ( ( ruleSimpleDataType ) | ( ruleEntityDataType ) )
int alt6=2;
int LA6_0 = input.LA(1);
if ( (LA6_0==82||(LA6_0>=86 && LA6_0<=88)||LA6_0==90||(LA6_0>=92 && LA6_0<=93)||LA6_0==95||LA6_0==97||LA6_0==100) ) {
alt6=1;
}
else if ( (LA6_0==99) ) {
alt6=2;
}
else {
if (state.backtracking>0) {state.failed=true; return ;}
NoViableAltException nvae =
new NoViableAltException("", 6, 0, input);
throw nvae;
}
switch (alt6) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4871:1: ( ruleSimpleDataType )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4871:1: ( ruleSimpleDataType )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4872:1: ruleSimpleDataType
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDatatypeAccess().getSimpleDataTypeParserRuleCall_0());
}
pushFollow(FollowSets000.FOLLOW_ruleSimpleDataType_in_rule__Datatype__Alternatives10370);
ruleSimpleDataType();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDatatypeAccess().getSimpleDataTypeParserRuleCall_0());
}
}
}
break;
case 2 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4877:6: ( ruleEntityDataType )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4877:6: ( ruleEntityDataType )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4878:1: ruleEntityDataType
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDatatypeAccess().getEntityDataTypeParserRuleCall_1());
}
pushFollow(FollowSets000.FOLLOW_ruleEntityDataType_in_rule__Datatype__Alternatives10387);
ruleEntityDataType();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDatatypeAccess().getEntityDataTypeParserRuleCall_1());
}
}
}
break;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Datatype__Alternatives"
// $ANTLR start "rule__SimpleDataType__Alternatives"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4888:1: rule__SimpleDataType__Alternatives : ( ( ruleStringDataType ) | ( ruleDecimalDataType ) | ( ruleDateDataType ) | ( ruleIntegerDataType ) | ( ruleBinaryDataType ) | ( ruleEnumerationDataType ) | ( ruleBooleanDataType ) | ( ruleDoubleDataType ) | ( ruleFloatDataType ) | ( ruleLongDataType ) );
public final void rule__SimpleDataType__Alternatives() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4892:1: ( ( ruleStringDataType ) | ( ruleDecimalDataType ) | ( ruleDateDataType ) | ( ruleIntegerDataType ) | ( ruleBinaryDataType ) | ( ruleEnumerationDataType ) | ( ruleBooleanDataType ) | ( ruleDoubleDataType ) | ( ruleFloatDataType ) | ( ruleLongDataType ) )
int alt7=10;
switch ( input.LA(1) ) {
case 82:
{
alt7=1;
}
break;
case 90:
{
alt7=2;
}
break;
case 88:
{
alt7=3;
}
break;
case 87:
{
alt7=4;
}
break;
case 97:
{
alt7=5;
}
break;
case 100:
{
alt7=6;
}
break;
case 86:
{
alt7=7;
}
break;
case 95:
{
alt7=8;
}
break;
case 93:
{
alt7=9;
}
break;
case 92:
{
alt7=10;
}
break;
default:
if (state.backtracking>0) {state.failed=true; return ;}
NoViableAltException nvae =
new NoViableAltException("", 7, 0, input);
throw nvae;
}
switch (alt7) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4893:1: ( ruleStringDataType )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4893:1: ( ruleStringDataType )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4894:1: ruleStringDataType
{
if ( state.backtracking==0 ) {
before(grammarAccess.getSimpleDataTypeAccess().getStringDataTypeParserRuleCall_0());
}
pushFollow(FollowSets000.FOLLOW_ruleStringDataType_in_rule__SimpleDataType__Alternatives10419);
ruleStringDataType();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getSimpleDataTypeAccess().getStringDataTypeParserRuleCall_0());
}
}
}
break;
case 2 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4899:6: ( ruleDecimalDataType )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4899:6: ( ruleDecimalDataType )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4900:1: ruleDecimalDataType
{
if ( state.backtracking==0 ) {
before(grammarAccess.getSimpleDataTypeAccess().getDecimalDataTypeParserRuleCall_1());
}
pushFollow(FollowSets000.FOLLOW_ruleDecimalDataType_in_rule__SimpleDataType__Alternatives10436);
ruleDecimalDataType();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getSimpleDataTypeAccess().getDecimalDataTypeParserRuleCall_1());
}
}
}
break;
case 3 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4905:6: ( ruleDateDataType )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4905:6: ( ruleDateDataType )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4906:1: ruleDateDataType
{
if ( state.backtracking==0 ) {
before(grammarAccess.getSimpleDataTypeAccess().getDateDataTypeParserRuleCall_2());
}
pushFollow(FollowSets000.FOLLOW_ruleDateDataType_in_rule__SimpleDataType__Alternatives10453);
ruleDateDataType();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getSimpleDataTypeAccess().getDateDataTypeParserRuleCall_2());
}
}
}
break;
case 4 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4911:6: ( ruleIntegerDataType )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4911:6: ( ruleIntegerDataType )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4912:1: ruleIntegerDataType
{
if ( state.backtracking==0 ) {
before(grammarAccess.getSimpleDataTypeAccess().getIntegerDataTypeParserRuleCall_3());
}
pushFollow(FollowSets000.FOLLOW_ruleIntegerDataType_in_rule__SimpleDataType__Alternatives10470);
ruleIntegerDataType();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getSimpleDataTypeAccess().getIntegerDataTypeParserRuleCall_3());
}
}
}
break;
case 5 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4917:6: ( ruleBinaryDataType )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4917:6: ( ruleBinaryDataType )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4918:1: ruleBinaryDataType
{
if ( state.backtracking==0 ) {
before(grammarAccess.getSimpleDataTypeAccess().getBinaryDataTypeParserRuleCall_4());
}
pushFollow(FollowSets000.FOLLOW_ruleBinaryDataType_in_rule__SimpleDataType__Alternatives10487);
ruleBinaryDataType();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getSimpleDataTypeAccess().getBinaryDataTypeParserRuleCall_4());
}
}
}
break;
case 6 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4923:6: ( ruleEnumerationDataType )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4923:6: ( ruleEnumerationDataType )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4924:1: ruleEnumerationDataType
{
if ( state.backtracking==0 ) {
before(grammarAccess.getSimpleDataTypeAccess().getEnumerationDataTypeParserRuleCall_5());
}
pushFollow(FollowSets000.FOLLOW_ruleEnumerationDataType_in_rule__SimpleDataType__Alternatives10504);
ruleEnumerationDataType();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getSimpleDataTypeAccess().getEnumerationDataTypeParserRuleCall_5());
}
}
}
break;
case 7 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4929:6: ( ruleBooleanDataType )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4929:6: ( ruleBooleanDataType )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4930:1: ruleBooleanDataType
{
if ( state.backtracking==0 ) {
before(grammarAccess.getSimpleDataTypeAccess().getBooleanDataTypeParserRuleCall_6());
}
pushFollow(FollowSets000.FOLLOW_ruleBooleanDataType_in_rule__SimpleDataType__Alternatives10521);
ruleBooleanDataType();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getSimpleDataTypeAccess().getBooleanDataTypeParserRuleCall_6());
}
}
}
break;
case 8 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4935:6: ( ruleDoubleDataType )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4935:6: ( ruleDoubleDataType )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4936:1: ruleDoubleDataType
{
if ( state.backtracking==0 ) {
before(grammarAccess.getSimpleDataTypeAccess().getDoubleDataTypeParserRuleCall_7());
}
pushFollow(FollowSets000.FOLLOW_ruleDoubleDataType_in_rule__SimpleDataType__Alternatives10538);
ruleDoubleDataType();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getSimpleDataTypeAccess().getDoubleDataTypeParserRuleCall_7());
}
}
}
break;
case 9 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4941:6: ( ruleFloatDataType )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4941:6: ( ruleFloatDataType )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4942:1: ruleFloatDataType
{
if ( state.backtracking==0 ) {
before(grammarAccess.getSimpleDataTypeAccess().getFloatDataTypeParserRuleCall_8());
}
pushFollow(FollowSets000.FOLLOW_ruleFloatDataType_in_rule__SimpleDataType__Alternatives10555);
ruleFloatDataType();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getSimpleDataTypeAccess().getFloatDataTypeParserRuleCall_8());
}
}
}
break;
case 10 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4947:6: ( ruleLongDataType )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4947:6: ( ruleLongDataType )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4948:1: ruleLongDataType
{
if ( state.backtracking==0 ) {
before(grammarAccess.getSimpleDataTypeAccess().getLongDataTypeParserRuleCall_9());
}
pushFollow(FollowSets000.FOLLOW_ruleLongDataType_in_rule__SimpleDataType__Alternatives10572);
ruleLongDataType();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getSimpleDataTypeAccess().getLongDataTypeParserRuleCall_9());
}
}
}
break;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__SimpleDataType__Alternatives"
// $ANTLR start "rule__EntityAttribute__Alternatives"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4958:1: rule__EntityAttribute__Alternatives : ( ( ruleSimpleDatatypeEntityAttribute ) | ( ruleEntityEntityAttribute ) | ( ruleValueObjectEntityAttribute ) );
public final void rule__EntityAttribute__Alternatives() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4962:1: ( ( ruleSimpleDatatypeEntityAttribute ) | ( ruleEntityEntityAttribute ) | ( ruleValueObjectEntityAttribute ) )
int alt8=3;
switch ( input.LA(1) ) {
case 56:
case 57:
case 59:
case 60:
case 70:
case 85:
case 89:
case 91:
case 94:
case 96:
case 98:
{
alt8=1;
}
break;
case 78:
{
alt8=2;
}
break;
case 80:
{
alt8=3;
}
break;
default:
if (state.backtracking>0) {state.failed=true; return ;}
NoViableAltException nvae =
new NoViableAltException("", 8, 0, input);
throw nvae;
}
switch (alt8) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4963:1: ( ruleSimpleDatatypeEntityAttribute )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4963:1: ( ruleSimpleDatatypeEntityAttribute )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4964:1: ruleSimpleDatatypeEntityAttribute
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEntityAttributeAccess().getSimpleDatatypeEntityAttributeParserRuleCall_0());
}
pushFollow(FollowSets000.FOLLOW_ruleSimpleDatatypeEntityAttribute_in_rule__EntityAttribute__Alternatives10604);
ruleSimpleDatatypeEntityAttribute();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getEntityAttributeAccess().getSimpleDatatypeEntityAttributeParserRuleCall_0());
}
}
}
break;
case 2 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4969:6: ( ruleEntityEntityAttribute )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4969:6: ( ruleEntityEntityAttribute )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4970:1: ruleEntityEntityAttribute
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEntityAttributeAccess().getEntityEntityAttributeParserRuleCall_1());
}
pushFollow(FollowSets000.FOLLOW_ruleEntityEntityAttribute_in_rule__EntityAttribute__Alternatives10621);
ruleEntityEntityAttribute();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getEntityAttributeAccess().getEntityEntityAttributeParserRuleCall_1());
}
}
}
break;
case 3 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4975:6: ( ruleValueObjectEntityAttribute )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4975:6: ( ruleValueObjectEntityAttribute )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4976:1: ruleValueObjectEntityAttribute
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEntityAttributeAccess().getValueObjectEntityAttributeParserRuleCall_2());
}
pushFollow(FollowSets000.FOLLOW_ruleValueObjectEntityAttribute_in_rule__EntityAttribute__Alternatives10638);
ruleValueObjectEntityAttribute();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getEntityAttributeAccess().getValueObjectEntityAttributeParserRuleCall_2());
}
}
}
break;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EntityAttribute__Alternatives"
// $ANTLR start "rule__SimpleDatatypeEntityAttribute__Alternatives"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4986:1: rule__SimpleDatatypeEntityAttribute__Alternatives : ( ( ruleMapEntityAttribute ) | ( ruleLongEntityAttribute ) | ( ruleDateEntityAttribute ) | ( ruleDecimalEntityAttribute ) | ( ruleDoubleEntityAttribute ) | ( ruleFloatEntityAttribute ) | ( ruleStringEntityAttribute ) | ( ruleBooleanEntityAttribute ) | ( ruleIntegerEntityAttribute ) | ( ruleBinaryEntityAttribute ) | ( ruleEnumerationEntityAttribute ) );
public final void rule__SimpleDatatypeEntityAttribute__Alternatives() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4990:1: ( ( ruleMapEntityAttribute ) | ( ruleLongEntityAttribute ) | ( ruleDateEntityAttribute ) | ( ruleDecimalEntityAttribute ) | ( ruleDoubleEntityAttribute ) | ( ruleFloatEntityAttribute ) | ( ruleStringEntityAttribute ) | ( ruleBooleanEntityAttribute ) | ( ruleIntegerEntityAttribute ) | ( ruleBinaryEntityAttribute ) | ( ruleEnumerationEntityAttribute ) )
int alt9=11;
switch ( input.LA(1) ) {
case 85:
{
alt9=1;
}
break;
case 56:
{
alt9=2;
}
break;
case 89:
{
alt9=3;
}
break;
case 91:
{
alt9=4;
}
break;
case 96:
{
alt9=5;
}
break;
case 94:
{
alt9=6;
}
break;
case 59:
{
alt9=7;
}
break;
case 60:
{
alt9=8;
}
break;
case 57:
{
alt9=9;
}
break;
case 98:
{
alt9=10;
}
break;
case 70:
{
alt9=11;
}
break;
default:
if (state.backtracking>0) {state.failed=true; return ;}
NoViableAltException nvae =
new NoViableAltException("", 9, 0, input);
throw nvae;
}
switch (alt9) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4991:1: ( ruleMapEntityAttribute )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4991:1: ( ruleMapEntityAttribute )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4992:1: ruleMapEntityAttribute
{
if ( state.backtracking==0 ) {
before(grammarAccess.getSimpleDatatypeEntityAttributeAccess().getMapEntityAttributeParserRuleCall_0());
}
pushFollow(FollowSets000.FOLLOW_ruleMapEntityAttribute_in_rule__SimpleDatatypeEntityAttribute__Alternatives10670);
ruleMapEntityAttribute();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getSimpleDatatypeEntityAttributeAccess().getMapEntityAttributeParserRuleCall_0());
}
}
}
break;
case 2 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4997:6: ( ruleLongEntityAttribute )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4997:6: ( ruleLongEntityAttribute )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:4998:1: ruleLongEntityAttribute
{
if ( state.backtracking==0 ) {
before(grammarAccess.getSimpleDatatypeEntityAttributeAccess().getLongEntityAttributeParserRuleCall_1());
}
pushFollow(FollowSets000.FOLLOW_ruleLongEntityAttribute_in_rule__SimpleDatatypeEntityAttribute__Alternatives10687);
ruleLongEntityAttribute();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getSimpleDatatypeEntityAttributeAccess().getLongEntityAttributeParserRuleCall_1());
}
}
}
break;
case 3 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5003:6: ( ruleDateEntityAttribute )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5003:6: ( ruleDateEntityAttribute )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5004:1: ruleDateEntityAttribute
{
if ( state.backtracking==0 ) {
before(grammarAccess.getSimpleDatatypeEntityAttributeAccess().getDateEntityAttributeParserRuleCall_2());
}
pushFollow(FollowSets000.FOLLOW_ruleDateEntityAttribute_in_rule__SimpleDatatypeEntityAttribute__Alternatives10704);
ruleDateEntityAttribute();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getSimpleDatatypeEntityAttributeAccess().getDateEntityAttributeParserRuleCall_2());
}
}
}
break;
case 4 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5009:6: ( ruleDecimalEntityAttribute )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5009:6: ( ruleDecimalEntityAttribute )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5010:1: ruleDecimalEntityAttribute
{
if ( state.backtracking==0 ) {
before(grammarAccess.getSimpleDatatypeEntityAttributeAccess().getDecimalEntityAttributeParserRuleCall_3());
}
pushFollow(FollowSets000.FOLLOW_ruleDecimalEntityAttribute_in_rule__SimpleDatatypeEntityAttribute__Alternatives10721);
ruleDecimalEntityAttribute();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getSimpleDatatypeEntityAttributeAccess().getDecimalEntityAttributeParserRuleCall_3());
}
}
}
break;
case 5 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5015:6: ( ruleDoubleEntityAttribute )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5015:6: ( ruleDoubleEntityAttribute )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5016:1: ruleDoubleEntityAttribute
{
if ( state.backtracking==0 ) {
before(grammarAccess.getSimpleDatatypeEntityAttributeAccess().getDoubleEntityAttributeParserRuleCall_4());
}
pushFollow(FollowSets000.FOLLOW_ruleDoubleEntityAttribute_in_rule__SimpleDatatypeEntityAttribute__Alternatives10738);
ruleDoubleEntityAttribute();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getSimpleDatatypeEntityAttributeAccess().getDoubleEntityAttributeParserRuleCall_4());
}
}
}
break;
case 6 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5021:6: ( ruleFloatEntityAttribute )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5021:6: ( ruleFloatEntityAttribute )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5022:1: ruleFloatEntityAttribute
{
if ( state.backtracking==0 ) {
before(grammarAccess.getSimpleDatatypeEntityAttributeAccess().getFloatEntityAttributeParserRuleCall_5());
}
pushFollow(FollowSets000.FOLLOW_ruleFloatEntityAttribute_in_rule__SimpleDatatypeEntityAttribute__Alternatives10755);
ruleFloatEntityAttribute();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getSimpleDatatypeEntityAttributeAccess().getFloatEntityAttributeParserRuleCall_5());
}
}
}
break;
case 7 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5027:6: ( ruleStringEntityAttribute )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5027:6: ( ruleStringEntityAttribute )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5028:1: ruleStringEntityAttribute
{
if ( state.backtracking==0 ) {
before(grammarAccess.getSimpleDatatypeEntityAttributeAccess().getStringEntityAttributeParserRuleCall_6());
}
pushFollow(FollowSets000.FOLLOW_ruleStringEntityAttribute_in_rule__SimpleDatatypeEntityAttribute__Alternatives10772);
ruleStringEntityAttribute();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getSimpleDatatypeEntityAttributeAccess().getStringEntityAttributeParserRuleCall_6());
}
}
}
break;
case 8 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5033:6: ( ruleBooleanEntityAttribute )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5033:6: ( ruleBooleanEntityAttribute )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5034:1: ruleBooleanEntityAttribute
{
if ( state.backtracking==0 ) {
before(grammarAccess.getSimpleDatatypeEntityAttributeAccess().getBooleanEntityAttributeParserRuleCall_7());
}
pushFollow(FollowSets000.FOLLOW_ruleBooleanEntityAttribute_in_rule__SimpleDatatypeEntityAttribute__Alternatives10789);
ruleBooleanEntityAttribute();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getSimpleDatatypeEntityAttributeAccess().getBooleanEntityAttributeParserRuleCall_7());
}
}
}
break;
case 9 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5039:6: ( ruleIntegerEntityAttribute )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5039:6: ( ruleIntegerEntityAttribute )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5040:1: ruleIntegerEntityAttribute
{
if ( state.backtracking==0 ) {
before(grammarAccess.getSimpleDatatypeEntityAttributeAccess().getIntegerEntityAttributeParserRuleCall_8());
}
pushFollow(FollowSets000.FOLLOW_ruleIntegerEntityAttribute_in_rule__SimpleDatatypeEntityAttribute__Alternatives10806);
ruleIntegerEntityAttribute();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getSimpleDatatypeEntityAttributeAccess().getIntegerEntityAttributeParserRuleCall_8());
}
}
}
break;
case 10 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5045:6: ( ruleBinaryEntityAttribute )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5045:6: ( ruleBinaryEntityAttribute )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5046:1: ruleBinaryEntityAttribute
{
if ( state.backtracking==0 ) {
before(grammarAccess.getSimpleDatatypeEntityAttributeAccess().getBinaryEntityAttributeParserRuleCall_9());
}
pushFollow(FollowSets000.FOLLOW_ruleBinaryEntityAttribute_in_rule__SimpleDatatypeEntityAttribute__Alternatives10823);
ruleBinaryEntityAttribute();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getSimpleDatatypeEntityAttributeAccess().getBinaryEntityAttributeParserRuleCall_9());
}
}
}
break;
case 11 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5051:6: ( ruleEnumerationEntityAttribute )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5051:6: ( ruleEnumerationEntityAttribute )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5052:1: ruleEnumerationEntityAttribute
{
if ( state.backtracking==0 ) {
before(grammarAccess.getSimpleDatatypeEntityAttributeAccess().getEnumerationEntityAttributeParserRuleCall_10());
}
pushFollow(FollowSets000.FOLLOW_ruleEnumerationEntityAttribute_in_rule__SimpleDatatypeEntityAttribute__Alternatives10840);
ruleEnumerationEntityAttribute();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getSimpleDatatypeEntityAttributeAccess().getEnumerationEntityAttributeParserRuleCall_10());
}
}
}
break;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__SimpleDatatypeEntityAttribute__Alternatives"
// $ANTLR start "rule__BaseDataTypeProperties__Alternatives"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5062:1: rule__BaseDataTypeProperties__Alternatives : ( ( ruleBaseDataTypeWidth ) | ( ruleBaseDataTypeLabel ) );
public final void rule__BaseDataTypeProperties__Alternatives() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5066:1: ( ( ruleBaseDataTypeWidth ) | ( ruleBaseDataTypeLabel ) )
int alt10=2;
int LA10_0 = input.LA(1);
if ( (LA10_0==81) ) {
alt10=1;
}
else if ( (LA10_0==75) ) {
alt10=2;
}
else {
if (state.backtracking>0) {state.failed=true; return ;}
NoViableAltException nvae =
new NoViableAltException("", 10, 0, input);
throw nvae;
}
switch (alt10) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5067:1: ( ruleBaseDataTypeWidth )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5067:1: ( ruleBaseDataTypeWidth )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5068:1: ruleBaseDataTypeWidth
{
if ( state.backtracking==0 ) {
before(grammarAccess.getBaseDataTypePropertiesAccess().getBaseDataTypeWidthParserRuleCall_0());
}
pushFollow(FollowSets000.FOLLOW_ruleBaseDataTypeWidth_in_rule__BaseDataTypeProperties__Alternatives10872);
ruleBaseDataTypeWidth();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getBaseDataTypePropertiesAccess().getBaseDataTypeWidthParserRuleCall_0());
}
}
}
break;
case 2 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5073:6: ( ruleBaseDataTypeLabel )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5073:6: ( ruleBaseDataTypeLabel )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5074:1: ruleBaseDataTypeLabel
{
if ( state.backtracking==0 ) {
before(grammarAccess.getBaseDataTypePropertiesAccess().getBaseDataTypeLabelParserRuleCall_1());
}
pushFollow(FollowSets000.FOLLOW_ruleBaseDataTypeLabel_in_rule__BaseDataTypeProperties__Alternatives10889);
ruleBaseDataTypeLabel();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getBaseDataTypePropertiesAccess().getBaseDataTypeLabelParserRuleCall_1());
}
}
}
break;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__BaseDataTypeProperties__Alternatives"
// $ANTLR start "rule__DictionaryContainer__Alternatives"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5086:1: rule__DictionaryContainer__Alternatives : ( ( ruleDictionaryComposite ) | ( ruleDictionaryEditableTable ) | ( ruleDictionaryAssignmentTable ) );
public final void rule__DictionaryContainer__Alternatives() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5090:1: ( ( ruleDictionaryComposite ) | ( ruleDictionaryEditableTable ) | ( ruleDictionaryAssignmentTable ) )
int alt11=3;
switch ( input.LA(1) ) {
case 112:
{
alt11=1;
}
break;
case 113:
{
alt11=2;
}
break;
case 116:
{
alt11=3;
}
break;
default:
if (state.backtracking>0) {state.failed=true; return ;}
NoViableAltException nvae =
new NoViableAltException("", 11, 0, input);
throw nvae;
}
switch (alt11) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5091:1: ( ruleDictionaryComposite )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5091:1: ( ruleDictionaryComposite )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5092:1: ruleDictionaryComposite
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryContainerAccess().getDictionaryCompositeParserRuleCall_0());
}
pushFollow(FollowSets000.FOLLOW_ruleDictionaryComposite_in_rule__DictionaryContainer__Alternatives10923);
ruleDictionaryComposite();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryContainerAccess().getDictionaryCompositeParserRuleCall_0());
}
}
}
break;
case 2 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5097:6: ( ruleDictionaryEditableTable )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5097:6: ( ruleDictionaryEditableTable )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5098:1: ruleDictionaryEditableTable
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryContainerAccess().getDictionaryEditableTableParserRuleCall_1());
}
pushFollow(FollowSets000.FOLLOW_ruleDictionaryEditableTable_in_rule__DictionaryContainer__Alternatives10940);
ruleDictionaryEditableTable();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryContainerAccess().getDictionaryEditableTableParserRuleCall_1());
}
}
}
break;
case 3 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5103:6: ( ruleDictionaryAssignmentTable )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5103:6: ( ruleDictionaryAssignmentTable )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5104:1: ruleDictionaryAssignmentTable
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryContainerAccess().getDictionaryAssignmentTableParserRuleCall_2());
}
pushFollow(FollowSets000.FOLLOW_ruleDictionaryAssignmentTable_in_rule__DictionaryContainer__Alternatives10957);
ruleDictionaryAssignmentTable();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryContainerAccess().getDictionaryAssignmentTableParserRuleCall_2());
}
}
}
break;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryContainer__Alternatives"
// $ANTLR start "rule__DictionaryContainerContent__Alternatives"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5114:1: rule__DictionaryContainerContent__Alternatives : ( ( ruleDictionaryContainer ) | ( ruleDictionaryControl ) );
public final void rule__DictionaryContainerContent__Alternatives() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5118:1: ( ( ruleDictionaryContainer ) | ( ruleDictionaryControl ) )
int alt12=2;
int LA12_0 = input.LA(1);
if ( ((LA12_0>=112 && LA12_0<=113)||LA12_0==116) ) {
alt12=1;
}
else if ( (LA12_0==125||LA12_0==127||LA12_0==129||(LA12_0>=131 && LA12_0<=136)||LA12_0==138) ) {
alt12=2;
}
else {
if (state.backtracking>0) {state.failed=true; return ;}
NoViableAltException nvae =
new NoViableAltException("", 12, 0, input);
throw nvae;
}
switch (alt12) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5119:1: ( ruleDictionaryContainer )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5119:1: ( ruleDictionaryContainer )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5120:1: ruleDictionaryContainer
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryContainerContentAccess().getDictionaryContainerParserRuleCall_0());
}
pushFollow(FollowSets000.FOLLOW_ruleDictionaryContainer_in_rule__DictionaryContainerContent__Alternatives10989);
ruleDictionaryContainer();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryContainerContentAccess().getDictionaryContainerParserRuleCall_0());
}
}
}
break;
case 2 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5125:6: ( ruleDictionaryControl )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5125:6: ( ruleDictionaryControl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5126:1: ruleDictionaryControl
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryContainerContentAccess().getDictionaryControlParserRuleCall_1());
}
pushFollow(FollowSets000.FOLLOW_ruleDictionaryControl_in_rule__DictionaryContainerContent__Alternatives11006);
ruleDictionaryControl();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryContainerContentAccess().getDictionaryControlParserRuleCall_1());
}
}
}
break;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryContainerContent__Alternatives"
// $ANTLR start "rule__DictionaryControl__Alternatives"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5136:1: rule__DictionaryControl__Alternatives : ( ( ruleDictionaryControlGroup ) | ( ruleDictionaryTextControl ) | ( ruleDictionaryIntegerControl ) | ( ruleDictionaryHierarchicalControl ) | ( ruleDictionaryBigDecimalControl ) | ( ruleDictionaryBooleanControl ) | ( ruleDictionaryDateControl ) | ( ruleDictionaryEnumerationControl ) | ( ruleDictionaryFileControl ) | ( ruleDictionaryReferenceControl ) );
public final void rule__DictionaryControl__Alternatives() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5140:1: ( ( ruleDictionaryControlGroup ) | ( ruleDictionaryTextControl ) | ( ruleDictionaryIntegerControl ) | ( ruleDictionaryHierarchicalControl ) | ( ruleDictionaryBigDecimalControl ) | ( ruleDictionaryBooleanControl ) | ( ruleDictionaryDateControl ) | ( ruleDictionaryEnumerationControl ) | ( ruleDictionaryFileControl ) | ( ruleDictionaryReferenceControl ) )
int alt13=10;
switch ( input.LA(1) ) {
case 125:
{
alt13=1;
}
break;
case 129:
{
alt13=2;
}
break;
case 131:
{
alt13=3;
}
break;
case 127:
{
alt13=4;
}
break;
case 132:
{
alt13=5;
}
break;
case 133:
{
alt13=6;
}
break;
case 134:
{
alt13=7;
}
break;
case 135:
{
alt13=8;
}
break;
case 138:
{
alt13=9;
}
break;
case 136:
{
alt13=10;
}
break;
default:
if (state.backtracking>0) {state.failed=true; return ;}
NoViableAltException nvae =
new NoViableAltException("", 13, 0, input);
throw nvae;
}
switch (alt13) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5141:1: ( ruleDictionaryControlGroup )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5141:1: ( ruleDictionaryControlGroup )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5142:1: ruleDictionaryControlGroup
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryControlAccess().getDictionaryControlGroupParserRuleCall_0());
}
pushFollow(FollowSets000.FOLLOW_ruleDictionaryControlGroup_in_rule__DictionaryControl__Alternatives11038);
ruleDictionaryControlGroup();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryControlAccess().getDictionaryControlGroupParserRuleCall_0());
}
}
}
break;
case 2 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5147:6: ( ruleDictionaryTextControl )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5147:6: ( ruleDictionaryTextControl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5148:1: ruleDictionaryTextControl
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryControlAccess().getDictionaryTextControlParserRuleCall_1());
}
pushFollow(FollowSets000.FOLLOW_ruleDictionaryTextControl_in_rule__DictionaryControl__Alternatives11055);
ruleDictionaryTextControl();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryControlAccess().getDictionaryTextControlParserRuleCall_1());
}
}
}
break;
case 3 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5153:6: ( ruleDictionaryIntegerControl )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5153:6: ( ruleDictionaryIntegerControl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5154:1: ruleDictionaryIntegerControl
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryControlAccess().getDictionaryIntegerControlParserRuleCall_2());
}
pushFollow(FollowSets000.FOLLOW_ruleDictionaryIntegerControl_in_rule__DictionaryControl__Alternatives11072);
ruleDictionaryIntegerControl();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryControlAccess().getDictionaryIntegerControlParserRuleCall_2());
}
}
}
break;
case 4 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5159:6: ( ruleDictionaryHierarchicalControl )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5159:6: ( ruleDictionaryHierarchicalControl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5160:1: ruleDictionaryHierarchicalControl
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryControlAccess().getDictionaryHierarchicalControlParserRuleCall_3());
}
pushFollow(FollowSets000.FOLLOW_ruleDictionaryHierarchicalControl_in_rule__DictionaryControl__Alternatives11089);
ruleDictionaryHierarchicalControl();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryControlAccess().getDictionaryHierarchicalControlParserRuleCall_3());
}
}
}
break;
case 5 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5165:6: ( ruleDictionaryBigDecimalControl )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5165:6: ( ruleDictionaryBigDecimalControl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5166:1: ruleDictionaryBigDecimalControl
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryControlAccess().getDictionaryBigDecimalControlParserRuleCall_4());
}
pushFollow(FollowSets000.FOLLOW_ruleDictionaryBigDecimalControl_in_rule__DictionaryControl__Alternatives11106);
ruleDictionaryBigDecimalControl();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryControlAccess().getDictionaryBigDecimalControlParserRuleCall_4());
}
}
}
break;
case 6 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5171:6: ( ruleDictionaryBooleanControl )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5171:6: ( ruleDictionaryBooleanControl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5172:1: ruleDictionaryBooleanControl
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryControlAccess().getDictionaryBooleanControlParserRuleCall_5());
}
pushFollow(FollowSets000.FOLLOW_ruleDictionaryBooleanControl_in_rule__DictionaryControl__Alternatives11123);
ruleDictionaryBooleanControl();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryControlAccess().getDictionaryBooleanControlParserRuleCall_5());
}
}
}
break;
case 7 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5177:6: ( ruleDictionaryDateControl )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5177:6: ( ruleDictionaryDateControl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5178:1: ruleDictionaryDateControl
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryControlAccess().getDictionaryDateControlParserRuleCall_6());
}
pushFollow(FollowSets000.FOLLOW_ruleDictionaryDateControl_in_rule__DictionaryControl__Alternatives11140);
ruleDictionaryDateControl();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryControlAccess().getDictionaryDateControlParserRuleCall_6());
}
}
}
break;
case 8 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5183:6: ( ruleDictionaryEnumerationControl )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5183:6: ( ruleDictionaryEnumerationControl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5184:1: ruleDictionaryEnumerationControl
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryControlAccess().getDictionaryEnumerationControlParserRuleCall_7());
}
pushFollow(FollowSets000.FOLLOW_ruleDictionaryEnumerationControl_in_rule__DictionaryControl__Alternatives11157);
ruleDictionaryEnumerationControl();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryControlAccess().getDictionaryEnumerationControlParserRuleCall_7());
}
}
}
break;
case 9 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5189:6: ( ruleDictionaryFileControl )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5189:6: ( ruleDictionaryFileControl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5190:1: ruleDictionaryFileControl
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryControlAccess().getDictionaryFileControlParserRuleCall_8());
}
pushFollow(FollowSets000.FOLLOW_ruleDictionaryFileControl_in_rule__DictionaryControl__Alternatives11174);
ruleDictionaryFileControl();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryControlAccess().getDictionaryFileControlParserRuleCall_8());
}
}
}
break;
case 10 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5195:6: ( ruleDictionaryReferenceControl )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5195:6: ( ruleDictionaryReferenceControl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5196:1: ruleDictionaryReferenceControl
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryControlAccess().getDictionaryReferenceControlParserRuleCall_9());
}
pushFollow(FollowSets000.FOLLOW_ruleDictionaryReferenceControl_in_rule__DictionaryControl__Alternatives11191);
ruleDictionaryReferenceControl();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryControlAccess().getDictionaryReferenceControlParserRuleCall_9());
}
}
}
break;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryControl__Alternatives"
// $ANTLR start "rule__XAssignment__Alternatives"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5206:1: rule__XAssignment__Alternatives : ( ( ( rule__XAssignment__Group_0__0 ) ) | ( ( rule__XAssignment__Group_1__0 ) ) );
public final void rule__XAssignment__Alternatives() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5210:1: ( ( ( rule__XAssignment__Group_0__0 ) ) | ( ( rule__XAssignment__Group_1__0 ) ) )
int alt14=2;
switch ( input.LA(1) ) {
case RULE_ID:
{
int LA14_1 = input.LA(2);
if ( (LA14_1==EOF||(LA14_1>=RULE_ID && LA14_1<=RULE_STRING)||(LA14_1>=14 && LA14_1<=50)||(LA14_1>=67 && LA14_1<=68)||(LA14_1>=144 && LA14_1<=145)||(LA14_1>=152 && LA14_1<=176)||(LA14_1>=181 && LA14_1<=182)||(LA14_1>=184 && LA14_1<=185)) ) {
alt14=2;
}
else if ( (LA14_1==13) ) {
alt14=1;
}
else {
if (state.backtracking>0) {state.failed=true; return ;}
NoViableAltException nvae =
new NoViableAltException("", 14, 1, input);
throw nvae;
}
}
break;
case 45:
{
int LA14_2 = input.LA(2);
if ( (LA14_2==EOF||(LA14_2>=RULE_ID && LA14_2<=RULE_STRING)||(LA14_2>=14 && LA14_2<=50)||(LA14_2>=67 && LA14_2<=68)||(LA14_2>=144 && LA14_2<=145)||(LA14_2>=152 && LA14_2<=176)||(LA14_2>=181 && LA14_2<=182)||(LA14_2>=184 && LA14_2<=185)) ) {
alt14=2;
}
else if ( (LA14_2==13) ) {
alt14=1;
}
else {
if (state.backtracking>0) {state.failed=true; return ;}
NoViableAltException nvae =
new NoViableAltException("", 14, 2, input);
throw nvae;
}
}
break;
case 46:
{
int LA14_3 = input.LA(2);
if ( (LA14_3==13) ) {
alt14=1;
}
else if ( (LA14_3==EOF||(LA14_3>=RULE_ID && LA14_3<=RULE_STRING)||(LA14_3>=14 && LA14_3<=50)||(LA14_3>=67 && LA14_3<=68)||(LA14_3>=144 && LA14_3<=145)||(LA14_3>=152 && LA14_3<=176)||(LA14_3>=181 && LA14_3<=182)||(LA14_3>=184 && LA14_3<=185)) ) {
alt14=2;
}
else {
if (state.backtracking>0) {state.failed=true; return ;}
NoViableAltException nvae =
new NoViableAltException("", 14, 3, input);
throw nvae;
}
}
break;
case 47:
{
int LA14_4 = input.LA(2);
if ( (LA14_4==13) ) {
alt14=1;
}
else if ( (LA14_4==EOF||(LA14_4>=RULE_ID && LA14_4<=RULE_STRING)||(LA14_4>=14 && LA14_4<=50)||(LA14_4>=67 && LA14_4<=68)||(LA14_4>=144 && LA14_4<=145)||(LA14_4>=152 && LA14_4<=176)||(LA14_4>=181 && LA14_4<=182)||(LA14_4>=184 && LA14_4<=185)) ) {
alt14=2;
}
else {
if (state.backtracking>0) {state.failed=true; return ;}
NoViableAltException nvae =
new NoViableAltException("", 14, 4, input);
throw nvae;
}
}
break;
case 48:
{
int LA14_5 = input.LA(2);
if ( (LA14_5==13) ) {
alt14=1;
}
else if ( (LA14_5==EOF||(LA14_5>=RULE_ID && LA14_5<=RULE_STRING)||(LA14_5>=14 && LA14_5<=50)||(LA14_5>=67 && LA14_5<=68)||(LA14_5>=144 && LA14_5<=145)||(LA14_5>=152 && LA14_5<=176)||(LA14_5>=181 && LA14_5<=182)||(LA14_5>=184 && LA14_5<=185)) ) {
alt14=2;
}
else {
if (state.backtracking>0) {state.failed=true; return ;}
NoViableAltException nvae =
new NoViableAltException("", 14, 5, input);
throw nvae;
}
}
break;
case RULE_HEX:
case RULE_INT:
case RULE_DECIMAL:
case RULE_STRING:
case 27:
case 34:
case 35:
case 40:
case 49:
case 50:
case 67:
case 144:
case 155:
case 156:
case 159:
case 161:
case 165:
case 166:
case 167:
case 168:
case 169:
case 170:
case 171:
case 172:
case 173:
case 175:
case 185:
{
alt14=2;
}
break;
default:
if (state.backtracking>0) {state.failed=true; return ;}
NoViableAltException nvae =
new NoViableAltException("", 14, 0, input);
throw nvae;
}
switch (alt14) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5211:1: ( ( rule__XAssignment__Group_0__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5211:1: ( ( rule__XAssignment__Group_0__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5212:1: ( rule__XAssignment__Group_0__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXAssignmentAccess().getGroup_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5213:1: ( rule__XAssignment__Group_0__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5213:2: rule__XAssignment__Group_0__0
{
pushFollow(FollowSets000.FOLLOW_rule__XAssignment__Group_0__0_in_rule__XAssignment__Alternatives11223);
rule__XAssignment__Group_0__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXAssignmentAccess().getGroup_0());
}
}
}
break;
case 2 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5217:6: ( ( rule__XAssignment__Group_1__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5217:6: ( ( rule__XAssignment__Group_1__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5218:1: ( rule__XAssignment__Group_1__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXAssignmentAccess().getGroup_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5219:1: ( rule__XAssignment__Group_1__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5219:2: rule__XAssignment__Group_1__0
{
pushFollow(FollowSets000.FOLLOW_rule__XAssignment__Group_1__0_in_rule__XAssignment__Alternatives11241);
rule__XAssignment__Group_1__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXAssignmentAccess().getGroup_1());
}
}
}
break;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XAssignment__Alternatives"
// $ANTLR start "rule__OpMultiAssign__Alternatives"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5228:1: rule__OpMultiAssign__Alternatives : ( ( '+=' ) | ( '-=' ) | ( '*=' ) | ( '/=' ) | ( '%=' ) | ( ( rule__OpMultiAssign__Group_5__0 ) ) | ( ( rule__OpMultiAssign__Group_6__0 ) ) );
public final void rule__OpMultiAssign__Alternatives() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5232:1: ( ( '+=' ) | ( '-=' ) | ( '*=' ) | ( '/=' ) | ( '%=' ) | ( ( rule__OpMultiAssign__Group_5__0 ) ) | ( ( rule__OpMultiAssign__Group_6__0 ) ) )
int alt15=7;
switch ( input.LA(1) ) {
case 16:
{
alt15=1;
}
break;
case 17:
{
alt15=2;
}
break;
case 18:
{
alt15=3;
}
break;
case 19:
{
alt15=4;
}
break;
case 20:
{
alt15=5;
}
break;
case 27:
{
alt15=6;
}
break;
case 26:
{
alt15=7;
}
break;
default:
if (state.backtracking>0) {state.failed=true; return ;}
NoViableAltException nvae =
new NoViableAltException("", 15, 0, input);
throw nvae;
}
switch (alt15) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5233:1: ( '+=' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5233:1: ( '+=' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5234:1: '+='
{
if ( state.backtracking==0 ) {
before(grammarAccess.getOpMultiAssignAccess().getPlusSignEqualsSignKeyword_0());
}
match(input,16,FollowSets000.FOLLOW_16_in_rule__OpMultiAssign__Alternatives11275); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getOpMultiAssignAccess().getPlusSignEqualsSignKeyword_0());
}
}
}
break;
case 2 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5241:6: ( '-=' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5241:6: ( '-=' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5242:1: '-='
{
if ( state.backtracking==0 ) {
before(grammarAccess.getOpMultiAssignAccess().getHyphenMinusEqualsSignKeyword_1());
}
match(input,17,FollowSets000.FOLLOW_17_in_rule__OpMultiAssign__Alternatives11295); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getOpMultiAssignAccess().getHyphenMinusEqualsSignKeyword_1());
}
}
}
break;
case 3 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5249:6: ( '*=' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5249:6: ( '*=' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5250:1: '*='
{
if ( state.backtracking==0 ) {
before(grammarAccess.getOpMultiAssignAccess().getAsteriskEqualsSignKeyword_2());
}
match(input,18,FollowSets000.FOLLOW_18_in_rule__OpMultiAssign__Alternatives11315); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getOpMultiAssignAccess().getAsteriskEqualsSignKeyword_2());
}
}
}
break;
case 4 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5257:6: ( '/=' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5257:6: ( '/=' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5258:1: '/='
{
if ( state.backtracking==0 ) {
before(grammarAccess.getOpMultiAssignAccess().getSolidusEqualsSignKeyword_3());
}
match(input,19,FollowSets000.FOLLOW_19_in_rule__OpMultiAssign__Alternatives11335); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getOpMultiAssignAccess().getSolidusEqualsSignKeyword_3());
}
}
}
break;
case 5 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5265:6: ( '%=' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5265:6: ( '%=' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5266:1: '%='
{
if ( state.backtracking==0 ) {
before(grammarAccess.getOpMultiAssignAccess().getPercentSignEqualsSignKeyword_4());
}
match(input,20,FollowSets000.FOLLOW_20_in_rule__OpMultiAssign__Alternatives11355); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getOpMultiAssignAccess().getPercentSignEqualsSignKeyword_4());
}
}
}
break;
case 6 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5273:6: ( ( rule__OpMultiAssign__Group_5__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5273:6: ( ( rule__OpMultiAssign__Group_5__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5274:1: ( rule__OpMultiAssign__Group_5__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getOpMultiAssignAccess().getGroup_5());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5275:1: ( rule__OpMultiAssign__Group_5__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5275:2: rule__OpMultiAssign__Group_5__0
{
pushFollow(FollowSets000.FOLLOW_rule__OpMultiAssign__Group_5__0_in_rule__OpMultiAssign__Alternatives11374);
rule__OpMultiAssign__Group_5__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getOpMultiAssignAccess().getGroup_5());
}
}
}
break;
case 7 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5279:6: ( ( rule__OpMultiAssign__Group_6__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5279:6: ( ( rule__OpMultiAssign__Group_6__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5280:1: ( rule__OpMultiAssign__Group_6__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getOpMultiAssignAccess().getGroup_6());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5281:1: ( rule__OpMultiAssign__Group_6__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5281:2: rule__OpMultiAssign__Group_6__0
{
pushFollow(FollowSets000.FOLLOW_rule__OpMultiAssign__Group_6__0_in_rule__OpMultiAssign__Alternatives11392);
rule__OpMultiAssign__Group_6__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getOpMultiAssignAccess().getGroup_6());
}
}
}
break;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__OpMultiAssign__Alternatives"
// $ANTLR start "rule__OpEquality__Alternatives"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5290:1: rule__OpEquality__Alternatives : ( ( '==' ) | ( '!=' ) | ( '===' ) | ( '!==' ) );
public final void rule__OpEquality__Alternatives() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5294:1: ( ( '==' ) | ( '!=' ) | ( '===' ) | ( '!==' ) )
int alt16=4;
switch ( input.LA(1) ) {
case 21:
{
alt16=1;
}
break;
case 22:
{
alt16=2;
}
break;
case 23:
{
alt16=3;
}
break;
case 24:
{
alt16=4;
}
break;
default:
if (state.backtracking>0) {state.failed=true; return ;}
NoViableAltException nvae =
new NoViableAltException("", 16, 0, input);
throw nvae;
}
switch (alt16) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5295:1: ( '==' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5295:1: ( '==' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5296:1: '=='
{
if ( state.backtracking==0 ) {
before(grammarAccess.getOpEqualityAccess().getEqualsSignEqualsSignKeyword_0());
}
match(input,21,FollowSets000.FOLLOW_21_in_rule__OpEquality__Alternatives11426); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getOpEqualityAccess().getEqualsSignEqualsSignKeyword_0());
}
}
}
break;
case 2 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5303:6: ( '!=' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5303:6: ( '!=' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5304:1: '!='
{
if ( state.backtracking==0 ) {
before(grammarAccess.getOpEqualityAccess().getExclamationMarkEqualsSignKeyword_1());
}
match(input,22,FollowSets000.FOLLOW_22_in_rule__OpEquality__Alternatives11446); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getOpEqualityAccess().getExclamationMarkEqualsSignKeyword_1());
}
}
}
break;
case 3 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5311:6: ( '===' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5311:6: ( '===' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5312:1: '==='
{
if ( state.backtracking==0 ) {
before(grammarAccess.getOpEqualityAccess().getEqualsSignEqualsSignEqualsSignKeyword_2());
}
match(input,23,FollowSets000.FOLLOW_23_in_rule__OpEquality__Alternatives11466); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getOpEqualityAccess().getEqualsSignEqualsSignEqualsSignKeyword_2());
}
}
}
break;
case 4 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5319:6: ( '!==' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5319:6: ( '!==' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5320:1: '!=='
{
if ( state.backtracking==0 ) {
before(grammarAccess.getOpEqualityAccess().getExclamationMarkEqualsSignEqualsSignKeyword_3());
}
match(input,24,FollowSets000.FOLLOW_24_in_rule__OpEquality__Alternatives11486); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getOpEqualityAccess().getExclamationMarkEqualsSignEqualsSignKeyword_3());
}
}
}
break;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__OpEquality__Alternatives"
// $ANTLR start "rule__XRelationalExpression__Alternatives_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5332:1: rule__XRelationalExpression__Alternatives_1 : ( ( ( rule__XRelationalExpression__Group_1_0__0 ) ) | ( ( rule__XRelationalExpression__Group_1_1__0 ) ) );
public final void rule__XRelationalExpression__Alternatives_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5336:1: ( ( ( rule__XRelationalExpression__Group_1_0__0 ) ) | ( ( rule__XRelationalExpression__Group_1_1__0 ) ) )
int alt17=2;
int LA17_0 = input.LA(1);
if ( (LA17_0==152) ) {
alt17=1;
}
else if ( ((LA17_0>=25 && LA17_0<=27)) ) {
alt17=2;
}
else {
if (state.backtracking>0) {state.failed=true; return ;}
NoViableAltException nvae =
new NoViableAltException("", 17, 0, input);
throw nvae;
}
switch (alt17) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5337:1: ( ( rule__XRelationalExpression__Group_1_0__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5337:1: ( ( rule__XRelationalExpression__Group_1_0__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5338:1: ( rule__XRelationalExpression__Group_1_0__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXRelationalExpressionAccess().getGroup_1_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5339:1: ( rule__XRelationalExpression__Group_1_0__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5339:2: rule__XRelationalExpression__Group_1_0__0
{
pushFollow(FollowSets000.FOLLOW_rule__XRelationalExpression__Group_1_0__0_in_rule__XRelationalExpression__Alternatives_111520);
rule__XRelationalExpression__Group_1_0__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXRelationalExpressionAccess().getGroup_1_0());
}
}
}
break;
case 2 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5343:6: ( ( rule__XRelationalExpression__Group_1_1__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5343:6: ( ( rule__XRelationalExpression__Group_1_1__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5344:1: ( rule__XRelationalExpression__Group_1_1__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXRelationalExpressionAccess().getGroup_1_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5345:1: ( rule__XRelationalExpression__Group_1_1__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5345:2: rule__XRelationalExpression__Group_1_1__0
{
pushFollow(FollowSets000.FOLLOW_rule__XRelationalExpression__Group_1_1__0_in_rule__XRelationalExpression__Alternatives_111538);
rule__XRelationalExpression__Group_1_1__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXRelationalExpressionAccess().getGroup_1_1());
}
}
}
break;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XRelationalExpression__Alternatives_1"
// $ANTLR start "rule__OpCompare__Alternatives"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5354:1: rule__OpCompare__Alternatives : ( ( '>=' ) | ( ( rule__OpCompare__Group_1__0 ) ) | ( '>' ) | ( '<' ) );
public final void rule__OpCompare__Alternatives() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5358:1: ( ( '>=' ) | ( ( rule__OpCompare__Group_1__0 ) ) | ( '>' ) | ( '<' ) )
int alt18=4;
switch ( input.LA(1) ) {
case 25:
{
alt18=1;
}
break;
case 27:
{
int LA18_2 = input.LA(2);
if ( (LA18_2==13) ) {
alt18=2;
}
else if ( (LA18_2==EOF||(LA18_2>=RULE_ID && LA18_2<=RULE_STRING)||LA18_2==27||(LA18_2>=34 && LA18_2<=35)||LA18_2==40||(LA18_2>=45 && LA18_2<=50)||LA18_2==67||LA18_2==144||(LA18_2>=155 && LA18_2<=156)||LA18_2==159||LA18_2==161||(LA18_2>=165 && LA18_2<=173)||LA18_2==175||LA18_2==185) ) {
alt18=4;
}
else {
if (state.backtracking>0) {state.failed=true; return ;}
NoViableAltException nvae =
new NoViableAltException("", 18, 2, input);
throw nvae;
}
}
break;
case 26:
{
alt18=3;
}
break;
default:
if (state.backtracking>0) {state.failed=true; return ;}
NoViableAltException nvae =
new NoViableAltException("", 18, 0, input);
throw nvae;
}
switch (alt18) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5359:1: ( '>=' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5359:1: ( '>=' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5360:1: '>='
{
if ( state.backtracking==0 ) {
before(grammarAccess.getOpCompareAccess().getGreaterThanSignEqualsSignKeyword_0());
}
match(input,25,FollowSets000.FOLLOW_25_in_rule__OpCompare__Alternatives11572); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getOpCompareAccess().getGreaterThanSignEqualsSignKeyword_0());
}
}
}
break;
case 2 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5367:6: ( ( rule__OpCompare__Group_1__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5367:6: ( ( rule__OpCompare__Group_1__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5368:1: ( rule__OpCompare__Group_1__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getOpCompareAccess().getGroup_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5369:1: ( rule__OpCompare__Group_1__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5369:2: rule__OpCompare__Group_1__0
{
pushFollow(FollowSets000.FOLLOW_rule__OpCompare__Group_1__0_in_rule__OpCompare__Alternatives11591);
rule__OpCompare__Group_1__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getOpCompareAccess().getGroup_1());
}
}
}
break;
case 3 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5373:6: ( '>' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5373:6: ( '>' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5374:1: '>'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getOpCompareAccess().getGreaterThanSignKeyword_2());
}
match(input,26,FollowSets000.FOLLOW_26_in_rule__OpCompare__Alternatives11610); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getOpCompareAccess().getGreaterThanSignKeyword_2());
}
}
}
break;
case 4 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5381:6: ( '<' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5381:6: ( '<' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5382:1: '<'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getOpCompareAccess().getLessThanSignKeyword_3());
}
match(input,27,FollowSets000.FOLLOW_27_in_rule__OpCompare__Alternatives11630); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getOpCompareAccess().getLessThanSignKeyword_3());
}
}
}
break;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__OpCompare__Alternatives"
// $ANTLR start "rule__OpOther__Alternatives"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5394:1: rule__OpOther__Alternatives : ( ( '->' ) | ( '..<' ) | ( ( rule__OpOther__Group_2__0 ) ) | ( '..' ) | ( '=>' ) | ( ( rule__OpOther__Group_5__0 ) ) | ( ( rule__OpOther__Group_6__0 ) ) | ( '<>' ) | ( '?:' ) );
public final void rule__OpOther__Alternatives() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5398:1: ( ( '->' ) | ( '..<' ) | ( ( rule__OpOther__Group_2__0 ) ) | ( '..' ) | ( '=>' ) | ( ( rule__OpOther__Group_5__0 ) ) | ( ( rule__OpOther__Group_6__0 ) ) | ( '<>' ) | ( '?:' ) )
int alt19=9;
alt19 = dfa19.predict(input);
switch (alt19) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5399:1: ( '->' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5399:1: ( '->' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5400:1: '->'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getOpOtherAccess().getHyphenMinusGreaterThanSignKeyword_0());
}
match(input,28,FollowSets000.FOLLOW_28_in_rule__OpOther__Alternatives11665); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getOpOtherAccess().getHyphenMinusGreaterThanSignKeyword_0());
}
}
}
break;
case 2 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5407:6: ( '..<' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5407:6: ( '..<' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5408:1: '..<'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getOpOtherAccess().getFullStopFullStopLessThanSignKeyword_1());
}
match(input,29,FollowSets000.FOLLOW_29_in_rule__OpOther__Alternatives11685); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getOpOtherAccess().getFullStopFullStopLessThanSignKeyword_1());
}
}
}
break;
case 3 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5415:6: ( ( rule__OpOther__Group_2__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5415:6: ( ( rule__OpOther__Group_2__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5416:1: ( rule__OpOther__Group_2__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getOpOtherAccess().getGroup_2());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5417:1: ( rule__OpOther__Group_2__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5417:2: rule__OpOther__Group_2__0
{
pushFollow(FollowSets000.FOLLOW_rule__OpOther__Group_2__0_in_rule__OpOther__Alternatives11704);
rule__OpOther__Group_2__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getOpOtherAccess().getGroup_2());
}
}
}
break;
case 4 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5421:6: ( '..' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5421:6: ( '..' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5422:1: '..'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getOpOtherAccess().getFullStopFullStopKeyword_3());
}
match(input,30,FollowSets000.FOLLOW_30_in_rule__OpOther__Alternatives11723); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getOpOtherAccess().getFullStopFullStopKeyword_3());
}
}
}
break;
case 5 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5429:6: ( '=>' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5429:6: ( '=>' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5430:1: '=>'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getOpOtherAccess().getEqualsSignGreaterThanSignKeyword_4());
}
match(input,31,FollowSets000.FOLLOW_31_in_rule__OpOther__Alternatives11743); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getOpOtherAccess().getEqualsSignGreaterThanSignKeyword_4());
}
}
}
break;
case 6 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5437:6: ( ( rule__OpOther__Group_5__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5437:6: ( ( rule__OpOther__Group_5__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5438:1: ( rule__OpOther__Group_5__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getOpOtherAccess().getGroup_5());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5439:1: ( rule__OpOther__Group_5__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5439:2: rule__OpOther__Group_5__0
{
pushFollow(FollowSets000.FOLLOW_rule__OpOther__Group_5__0_in_rule__OpOther__Alternatives11762);
rule__OpOther__Group_5__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getOpOtherAccess().getGroup_5());
}
}
}
break;
case 7 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5443:6: ( ( rule__OpOther__Group_6__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5443:6: ( ( rule__OpOther__Group_6__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5444:1: ( rule__OpOther__Group_6__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getOpOtherAccess().getGroup_6());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5445:1: ( rule__OpOther__Group_6__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5445:2: rule__OpOther__Group_6__0
{
pushFollow(FollowSets000.FOLLOW_rule__OpOther__Group_6__0_in_rule__OpOther__Alternatives11780);
rule__OpOther__Group_6__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getOpOtherAccess().getGroup_6());
}
}
}
break;
case 8 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5449:6: ( '<>' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5449:6: ( '<>' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5450:1: '<>'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getOpOtherAccess().getLessThanSignGreaterThanSignKeyword_7());
}
match(input,32,FollowSets000.FOLLOW_32_in_rule__OpOther__Alternatives11799); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getOpOtherAccess().getLessThanSignGreaterThanSignKeyword_7());
}
}
}
break;
case 9 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5457:6: ( '?:' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5457:6: ( '?:' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5458:1: '?:'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getOpOtherAccess().getQuestionMarkColonKeyword_8());
}
match(input,33,FollowSets000.FOLLOW_33_in_rule__OpOther__Alternatives11819); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getOpOtherAccess().getQuestionMarkColonKeyword_8());
}
}
}
break;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__OpOther__Alternatives"
// $ANTLR start "rule__OpOther__Alternatives_5_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5470:1: rule__OpOther__Alternatives_5_1 : ( ( ( rule__OpOther__Group_5_1_0__0 ) ) | ( '>' ) );
public final void rule__OpOther__Alternatives_5_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5474:1: ( ( ( rule__OpOther__Group_5_1_0__0 ) ) | ( '>' ) )
int alt20=2;
int LA20_0 = input.LA(1);
if ( (LA20_0==26) ) {
int LA20_1 = input.LA(2);
if ( (LA20_1==EOF||(LA20_1>=RULE_ID && LA20_1<=RULE_STRING)||LA20_1==27||(LA20_1>=34 && LA20_1<=35)||LA20_1==40||(LA20_1>=45 && LA20_1<=50)||LA20_1==67||LA20_1==144||(LA20_1>=155 && LA20_1<=156)||LA20_1==159||LA20_1==161||(LA20_1>=165 && LA20_1<=173)||LA20_1==175||LA20_1==185) ) {
alt20=2;
}
else if ( (LA20_1==26) ) {
alt20=1;
}
else {
if (state.backtracking>0) {state.failed=true; return ;}
NoViableAltException nvae =
new NoViableAltException("", 20, 1, input);
throw nvae;
}
}
else {
if (state.backtracking>0) {state.failed=true; return ;}
NoViableAltException nvae =
new NoViableAltException("", 20, 0, input);
throw nvae;
}
switch (alt20) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5475:1: ( ( rule__OpOther__Group_5_1_0__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5475:1: ( ( rule__OpOther__Group_5_1_0__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5476:1: ( rule__OpOther__Group_5_1_0__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getOpOtherAccess().getGroup_5_1_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5477:1: ( rule__OpOther__Group_5_1_0__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5477:2: rule__OpOther__Group_5_1_0__0
{
pushFollow(FollowSets000.FOLLOW_rule__OpOther__Group_5_1_0__0_in_rule__OpOther__Alternatives_5_111853);
rule__OpOther__Group_5_1_0__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getOpOtherAccess().getGroup_5_1_0());
}
}
}
break;
case 2 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5481:6: ( '>' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5481:6: ( '>' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5482:1: '>'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getOpOtherAccess().getGreaterThanSignKeyword_5_1_1());
}
match(input,26,FollowSets000.FOLLOW_26_in_rule__OpOther__Alternatives_5_111872); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getOpOtherAccess().getGreaterThanSignKeyword_5_1_1());
}
}
}
break;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__OpOther__Alternatives_5_1"
// $ANTLR start "rule__OpOther__Alternatives_6_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5494:1: rule__OpOther__Alternatives_6_1 : ( ( ( rule__OpOther__Group_6_1_0__0 ) ) | ( '<' ) | ( '=>' ) );
public final void rule__OpOther__Alternatives_6_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5498:1: ( ( ( rule__OpOther__Group_6_1_0__0 ) ) | ( '<' ) | ( '=>' ) )
int alt21=3;
int LA21_0 = input.LA(1);
if ( (LA21_0==27) ) {
int LA21_1 = input.LA(2);
if ( (synpred75_InternalMango()) ) {
alt21=1;
}
else if ( (synpred76_InternalMango()) ) {
alt21=2;
}
else {
if (state.backtracking>0) {state.failed=true; return ;}
NoViableAltException nvae =
new NoViableAltException("", 21, 1, input);
throw nvae;
}
}
else if ( (LA21_0==31) ) {
alt21=3;
}
else {
if (state.backtracking>0) {state.failed=true; return ;}
NoViableAltException nvae =
new NoViableAltException("", 21, 0, input);
throw nvae;
}
switch (alt21) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5499:1: ( ( rule__OpOther__Group_6_1_0__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5499:1: ( ( rule__OpOther__Group_6_1_0__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5500:1: ( rule__OpOther__Group_6_1_0__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getOpOtherAccess().getGroup_6_1_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5501:1: ( rule__OpOther__Group_6_1_0__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5501:2: rule__OpOther__Group_6_1_0__0
{
pushFollow(FollowSets000.FOLLOW_rule__OpOther__Group_6_1_0__0_in_rule__OpOther__Alternatives_6_111906);
rule__OpOther__Group_6_1_0__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getOpOtherAccess().getGroup_6_1_0());
}
}
}
break;
case 2 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5505:6: ( '<' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5505:6: ( '<' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5506:1: '<'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getOpOtherAccess().getLessThanSignKeyword_6_1_1());
}
match(input,27,FollowSets000.FOLLOW_27_in_rule__OpOther__Alternatives_6_111925); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getOpOtherAccess().getLessThanSignKeyword_6_1_1());
}
}
}
break;
case 3 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5513:6: ( '=>' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5513:6: ( '=>' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5514:1: '=>'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getOpOtherAccess().getEqualsSignGreaterThanSignKeyword_6_1_2());
}
match(input,31,FollowSets000.FOLLOW_31_in_rule__OpOther__Alternatives_6_111945); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getOpOtherAccess().getEqualsSignGreaterThanSignKeyword_6_1_2());
}
}
}
break;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__OpOther__Alternatives_6_1"
// $ANTLR start "rule__OpAdd__Alternatives"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5526:1: rule__OpAdd__Alternatives : ( ( '+' ) | ( '-' ) );
public final void rule__OpAdd__Alternatives() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5530:1: ( ( '+' ) | ( '-' ) )
int alt22=2;
int LA22_0 = input.LA(1);
if ( (LA22_0==34) ) {
alt22=1;
}
else if ( (LA22_0==35) ) {
alt22=2;
}
else {
if (state.backtracking>0) {state.failed=true; return ;}
NoViableAltException nvae =
new NoViableAltException("", 22, 0, input);
throw nvae;
}
switch (alt22) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5531:1: ( '+' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5531:1: ( '+' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5532:1: '+'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getOpAddAccess().getPlusSignKeyword_0());
}
match(input,34,FollowSets000.FOLLOW_34_in_rule__OpAdd__Alternatives11980); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getOpAddAccess().getPlusSignKeyword_0());
}
}
}
break;
case 2 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5539:6: ( '-' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5539:6: ( '-' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5540:1: '-'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getOpAddAccess().getHyphenMinusKeyword_1());
}
match(input,35,FollowSets000.FOLLOW_35_in_rule__OpAdd__Alternatives12000); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getOpAddAccess().getHyphenMinusKeyword_1());
}
}
}
break;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__OpAdd__Alternatives"
// $ANTLR start "rule__OpMulti__Alternatives"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5552:1: rule__OpMulti__Alternatives : ( ( '*' ) | ( '**' ) | ( '/' ) | ( '%' ) );
public final void rule__OpMulti__Alternatives() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5556:1: ( ( '*' ) | ( '**' ) | ( '/' ) | ( '%' ) )
int alt23=4;
switch ( input.LA(1) ) {
case 36:
{
alt23=1;
}
break;
case 37:
{
alt23=2;
}
break;
case 38:
{
alt23=3;
}
break;
case 39:
{
alt23=4;
}
break;
default:
if (state.backtracking>0) {state.failed=true; return ;}
NoViableAltException nvae =
new NoViableAltException("", 23, 0, input);
throw nvae;
}
switch (alt23) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5557:1: ( '*' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5557:1: ( '*' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5558:1: '*'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getOpMultiAccess().getAsteriskKeyword_0());
}
match(input,36,FollowSets000.FOLLOW_36_in_rule__OpMulti__Alternatives12035); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getOpMultiAccess().getAsteriskKeyword_0());
}
}
}
break;
case 2 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5565:6: ( '**' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5565:6: ( '**' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5566:1: '**'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getOpMultiAccess().getAsteriskAsteriskKeyword_1());
}
match(input,37,FollowSets000.FOLLOW_37_in_rule__OpMulti__Alternatives12055); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getOpMultiAccess().getAsteriskAsteriskKeyword_1());
}
}
}
break;
case 3 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5573:6: ( '/' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5573:6: ( '/' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5574:1: '/'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getOpMultiAccess().getSolidusKeyword_2());
}
match(input,38,FollowSets000.FOLLOW_38_in_rule__OpMulti__Alternatives12075); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getOpMultiAccess().getSolidusKeyword_2());
}
}
}
break;
case 4 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5581:6: ( '%' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5581:6: ( '%' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5582:1: '%'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getOpMultiAccess().getPercentSignKeyword_3());
}
match(input,39,FollowSets000.FOLLOW_39_in_rule__OpMulti__Alternatives12095); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getOpMultiAccess().getPercentSignKeyword_3());
}
}
}
break;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__OpMulti__Alternatives"
// $ANTLR start "rule__XUnaryOperation__Alternatives"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5594:1: rule__XUnaryOperation__Alternatives : ( ( ( rule__XUnaryOperation__Group_0__0 ) ) | ( ruleXCastedExpression ) );
public final void rule__XUnaryOperation__Alternatives() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5598:1: ( ( ( rule__XUnaryOperation__Group_0__0 ) ) | ( ruleXCastedExpression ) )
int alt24=2;
int LA24_0 = input.LA(1);
if ( ((LA24_0>=34 && LA24_0<=35)||LA24_0==40) ) {
alt24=1;
}
else if ( ((LA24_0>=RULE_ID && LA24_0<=RULE_STRING)||LA24_0==27||(LA24_0>=45 && LA24_0<=50)||LA24_0==67||LA24_0==144||(LA24_0>=155 && LA24_0<=156)||LA24_0==159||LA24_0==161||(LA24_0>=165 && LA24_0<=173)||LA24_0==175||LA24_0==185) ) {
alt24=2;
}
else {
if (state.backtracking>0) {state.failed=true; return ;}
NoViableAltException nvae =
new NoViableAltException("", 24, 0, input);
throw nvae;
}
switch (alt24) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5599:1: ( ( rule__XUnaryOperation__Group_0__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5599:1: ( ( rule__XUnaryOperation__Group_0__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5600:1: ( rule__XUnaryOperation__Group_0__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXUnaryOperationAccess().getGroup_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5601:1: ( rule__XUnaryOperation__Group_0__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5601:2: rule__XUnaryOperation__Group_0__0
{
pushFollow(FollowSets000.FOLLOW_rule__XUnaryOperation__Group_0__0_in_rule__XUnaryOperation__Alternatives12129);
rule__XUnaryOperation__Group_0__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXUnaryOperationAccess().getGroup_0());
}
}
}
break;
case 2 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5605:6: ( ruleXCastedExpression )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5605:6: ( ruleXCastedExpression )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5606:1: ruleXCastedExpression
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXUnaryOperationAccess().getXCastedExpressionParserRuleCall_1());
}
pushFollow(FollowSets000.FOLLOW_ruleXCastedExpression_in_rule__XUnaryOperation__Alternatives12147);
ruleXCastedExpression();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXUnaryOperationAccess().getXCastedExpressionParserRuleCall_1());
}
}
}
break;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XUnaryOperation__Alternatives"
// $ANTLR start "rule__OpUnary__Alternatives"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5616:1: rule__OpUnary__Alternatives : ( ( '!' ) | ( '-' ) | ( '+' ) );
public final void rule__OpUnary__Alternatives() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5620:1: ( ( '!' ) | ( '-' ) | ( '+' ) )
int alt25=3;
switch ( input.LA(1) ) {
case 40:
{
alt25=1;
}
break;
case 35:
{
alt25=2;
}
break;
case 34:
{
alt25=3;
}
break;
default:
if (state.backtracking>0) {state.failed=true; return ;}
NoViableAltException nvae =
new NoViableAltException("", 25, 0, input);
throw nvae;
}
switch (alt25) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5621:1: ( '!' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5621:1: ( '!' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5622:1: '!'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getOpUnaryAccess().getExclamationMarkKeyword_0());
}
match(input,40,FollowSets000.FOLLOW_40_in_rule__OpUnary__Alternatives12180); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getOpUnaryAccess().getExclamationMarkKeyword_0());
}
}
}
break;
case 2 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5629:6: ( '-' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5629:6: ( '-' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5630:1: '-'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getOpUnaryAccess().getHyphenMinusKeyword_1());
}
match(input,35,FollowSets000.FOLLOW_35_in_rule__OpUnary__Alternatives12200); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getOpUnaryAccess().getHyphenMinusKeyword_1());
}
}
}
break;
case 3 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5637:6: ( '+' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5637:6: ( '+' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5638:1: '+'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getOpUnaryAccess().getPlusSignKeyword_2());
}
match(input,34,FollowSets000.FOLLOW_34_in_rule__OpUnary__Alternatives12220); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getOpUnaryAccess().getPlusSignKeyword_2());
}
}
}
break;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__OpUnary__Alternatives"
// $ANTLR start "rule__OpPostfix__Alternatives"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5650:1: rule__OpPostfix__Alternatives : ( ( '++' ) | ( '--' ) );
public final void rule__OpPostfix__Alternatives() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5654:1: ( ( '++' ) | ( '--' ) )
int alt26=2;
int LA26_0 = input.LA(1);
if ( (LA26_0==41) ) {
alt26=1;
}
else if ( (LA26_0==42) ) {
alt26=2;
}
else {
if (state.backtracking>0) {state.failed=true; return ;}
NoViableAltException nvae =
new NoViableAltException("", 26, 0, input);
throw nvae;
}
switch (alt26) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5655:1: ( '++' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5655:1: ( '++' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5656:1: '++'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getOpPostfixAccess().getPlusSignPlusSignKeyword_0());
}
match(input,41,FollowSets000.FOLLOW_41_in_rule__OpPostfix__Alternatives12255); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getOpPostfixAccess().getPlusSignPlusSignKeyword_0());
}
}
}
break;
case 2 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5663:6: ( '--' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5663:6: ( '--' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5664:1: '--'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getOpPostfixAccess().getHyphenMinusHyphenMinusKeyword_1());
}
match(input,42,FollowSets000.FOLLOW_42_in_rule__OpPostfix__Alternatives12275); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getOpPostfixAccess().getHyphenMinusHyphenMinusKeyword_1());
}
}
}
break;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__OpPostfix__Alternatives"
// $ANTLR start "rule__XMemberFeatureCall__Alternatives_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5676:1: rule__XMemberFeatureCall__Alternatives_1 : ( ( ( rule__XMemberFeatureCall__Group_1_0__0 ) ) | ( ( rule__XMemberFeatureCall__Group_1_1__0 ) ) );
public final void rule__XMemberFeatureCall__Alternatives_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5680:1: ( ( ( rule__XMemberFeatureCall__Group_1_0__0 ) ) | ( ( rule__XMemberFeatureCall__Group_1_1__0 ) ) )
int alt27=2;
alt27 = dfa27.predict(input);
switch (alt27) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5681:1: ( ( rule__XMemberFeatureCall__Group_1_0__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5681:1: ( ( rule__XMemberFeatureCall__Group_1_0__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5682:1: ( rule__XMemberFeatureCall__Group_1_0__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXMemberFeatureCallAccess().getGroup_1_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5683:1: ( rule__XMemberFeatureCall__Group_1_0__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5683:2: rule__XMemberFeatureCall__Group_1_0__0
{
pushFollow(FollowSets000.FOLLOW_rule__XMemberFeatureCall__Group_1_0__0_in_rule__XMemberFeatureCall__Alternatives_112309);
rule__XMemberFeatureCall__Group_1_0__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXMemberFeatureCallAccess().getGroup_1_0());
}
}
}
break;
case 2 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5687:6: ( ( rule__XMemberFeatureCall__Group_1_1__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5687:6: ( ( rule__XMemberFeatureCall__Group_1_1__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5688:1: ( rule__XMemberFeatureCall__Group_1_1__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXMemberFeatureCallAccess().getGroup_1_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5689:1: ( rule__XMemberFeatureCall__Group_1_1__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5689:2: rule__XMemberFeatureCall__Group_1_1__0
{
pushFollow(FollowSets000.FOLLOW_rule__XMemberFeatureCall__Group_1_1__0_in_rule__XMemberFeatureCall__Alternatives_112327);
rule__XMemberFeatureCall__Group_1_1__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXMemberFeatureCallAccess().getGroup_1_1());
}
}
}
break;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XMemberFeatureCall__Alternatives_1"
// $ANTLR start "rule__XMemberFeatureCall__Alternatives_1_0_0_0_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5698:1: rule__XMemberFeatureCall__Alternatives_1_0_0_0_1 : ( ( '.' ) | ( ( rule__XMemberFeatureCall__ExplicitStaticAssignment_1_0_0_0_1_1 ) ) );
public final void rule__XMemberFeatureCall__Alternatives_1_0_0_0_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5702:1: ( ( '.' ) | ( ( rule__XMemberFeatureCall__ExplicitStaticAssignment_1_0_0_0_1_1 ) ) )
int alt28=2;
int LA28_0 = input.LA(1);
if ( (LA28_0==43) ) {
alt28=1;
}
else if ( (LA28_0==181) ) {
alt28=2;
}
else {
if (state.backtracking>0) {state.failed=true; return ;}
NoViableAltException nvae =
new NoViableAltException("", 28, 0, input);
throw nvae;
}
switch (alt28) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5703:1: ( '.' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5703:1: ( '.' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5704:1: '.'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXMemberFeatureCallAccess().getFullStopKeyword_1_0_0_0_1_0());
}
match(input,43,FollowSets000.FOLLOW_43_in_rule__XMemberFeatureCall__Alternatives_1_0_0_0_112361); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXMemberFeatureCallAccess().getFullStopKeyword_1_0_0_0_1_0());
}
}
}
break;
case 2 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5711:6: ( ( rule__XMemberFeatureCall__ExplicitStaticAssignment_1_0_0_0_1_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5711:6: ( ( rule__XMemberFeatureCall__ExplicitStaticAssignment_1_0_0_0_1_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5712:1: ( rule__XMemberFeatureCall__ExplicitStaticAssignment_1_0_0_0_1_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXMemberFeatureCallAccess().getExplicitStaticAssignment_1_0_0_0_1_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5713:1: ( rule__XMemberFeatureCall__ExplicitStaticAssignment_1_0_0_0_1_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5713:2: rule__XMemberFeatureCall__ExplicitStaticAssignment_1_0_0_0_1_1
{
pushFollow(FollowSets000.FOLLOW_rule__XMemberFeatureCall__ExplicitStaticAssignment_1_0_0_0_1_1_in_rule__XMemberFeatureCall__Alternatives_1_0_0_0_112380);
rule__XMemberFeatureCall__ExplicitStaticAssignment_1_0_0_0_1_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXMemberFeatureCallAccess().getExplicitStaticAssignment_1_0_0_0_1_1());
}
}
}
break;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XMemberFeatureCall__Alternatives_1_0_0_0_1"
// $ANTLR start "rule__XMemberFeatureCall__Alternatives_1_1_0_0_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5722:1: rule__XMemberFeatureCall__Alternatives_1_1_0_0_1 : ( ( '.' ) | ( ( rule__XMemberFeatureCall__NullSafeAssignment_1_1_0_0_1_1 ) ) | ( ( rule__XMemberFeatureCall__ExplicitStaticAssignment_1_1_0_0_1_2 ) ) );
public final void rule__XMemberFeatureCall__Alternatives_1_1_0_0_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5726:1: ( ( '.' ) | ( ( rule__XMemberFeatureCall__NullSafeAssignment_1_1_0_0_1_1 ) ) | ( ( rule__XMemberFeatureCall__ExplicitStaticAssignment_1_1_0_0_1_2 ) ) )
int alt29=3;
switch ( input.LA(1) ) {
case 43:
{
alt29=1;
}
break;
case 182:
{
alt29=2;
}
break;
case 181:
{
alt29=3;
}
break;
default:
if (state.backtracking>0) {state.failed=true; return ;}
NoViableAltException nvae =
new NoViableAltException("", 29, 0, input);
throw nvae;
}
switch (alt29) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5727:1: ( '.' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5727:1: ( '.' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5728:1: '.'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXMemberFeatureCallAccess().getFullStopKeyword_1_1_0_0_1_0());
}
match(input,43,FollowSets000.FOLLOW_43_in_rule__XMemberFeatureCall__Alternatives_1_1_0_0_112414); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXMemberFeatureCallAccess().getFullStopKeyword_1_1_0_0_1_0());
}
}
}
break;
case 2 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5735:6: ( ( rule__XMemberFeatureCall__NullSafeAssignment_1_1_0_0_1_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5735:6: ( ( rule__XMemberFeatureCall__NullSafeAssignment_1_1_0_0_1_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5736:1: ( rule__XMemberFeatureCall__NullSafeAssignment_1_1_0_0_1_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXMemberFeatureCallAccess().getNullSafeAssignment_1_1_0_0_1_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5737:1: ( rule__XMemberFeatureCall__NullSafeAssignment_1_1_0_0_1_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5737:2: rule__XMemberFeatureCall__NullSafeAssignment_1_1_0_0_1_1
{
pushFollow(FollowSets000.FOLLOW_rule__XMemberFeatureCall__NullSafeAssignment_1_1_0_0_1_1_in_rule__XMemberFeatureCall__Alternatives_1_1_0_0_112433);
rule__XMemberFeatureCall__NullSafeAssignment_1_1_0_0_1_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXMemberFeatureCallAccess().getNullSafeAssignment_1_1_0_0_1_1());
}
}
}
break;
case 3 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5741:6: ( ( rule__XMemberFeatureCall__ExplicitStaticAssignment_1_1_0_0_1_2 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5741:6: ( ( rule__XMemberFeatureCall__ExplicitStaticAssignment_1_1_0_0_1_2 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5742:1: ( rule__XMemberFeatureCall__ExplicitStaticAssignment_1_1_0_0_1_2 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXMemberFeatureCallAccess().getExplicitStaticAssignment_1_1_0_0_1_2());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5743:1: ( rule__XMemberFeatureCall__ExplicitStaticAssignment_1_1_0_0_1_2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5743:2: rule__XMemberFeatureCall__ExplicitStaticAssignment_1_1_0_0_1_2
{
pushFollow(FollowSets000.FOLLOW_rule__XMemberFeatureCall__ExplicitStaticAssignment_1_1_0_0_1_2_in_rule__XMemberFeatureCall__Alternatives_1_1_0_0_112451);
rule__XMemberFeatureCall__ExplicitStaticAssignment_1_1_0_0_1_2();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXMemberFeatureCallAccess().getExplicitStaticAssignment_1_1_0_0_1_2());
}
}
}
break;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XMemberFeatureCall__Alternatives_1_1_0_0_1"
// $ANTLR start "rule__XMemberFeatureCall__Alternatives_1_1_3_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5752:1: rule__XMemberFeatureCall__Alternatives_1_1_3_1 : ( ( ( rule__XMemberFeatureCall__MemberCallArgumentsAssignment_1_1_3_1_0 ) ) | ( ( rule__XMemberFeatureCall__Group_1_1_3_1_1__0 ) ) );
public final void rule__XMemberFeatureCall__Alternatives_1_1_3_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5756:1: ( ( ( rule__XMemberFeatureCall__MemberCallArgumentsAssignment_1_1_3_1_0 ) ) | ( ( rule__XMemberFeatureCall__Group_1_1_3_1_1__0 ) ) )
int alt30=2;
alt30 = dfa30.predict(input);
switch (alt30) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5757:1: ( ( rule__XMemberFeatureCall__MemberCallArgumentsAssignment_1_1_3_1_0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5757:1: ( ( rule__XMemberFeatureCall__MemberCallArgumentsAssignment_1_1_3_1_0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5758:1: ( rule__XMemberFeatureCall__MemberCallArgumentsAssignment_1_1_3_1_0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXMemberFeatureCallAccess().getMemberCallArgumentsAssignment_1_1_3_1_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5759:1: ( rule__XMemberFeatureCall__MemberCallArgumentsAssignment_1_1_3_1_0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5759:2: rule__XMemberFeatureCall__MemberCallArgumentsAssignment_1_1_3_1_0
{
pushFollow(FollowSets000.FOLLOW_rule__XMemberFeatureCall__MemberCallArgumentsAssignment_1_1_3_1_0_in_rule__XMemberFeatureCall__Alternatives_1_1_3_112484);
rule__XMemberFeatureCall__MemberCallArgumentsAssignment_1_1_3_1_0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXMemberFeatureCallAccess().getMemberCallArgumentsAssignment_1_1_3_1_0());
}
}
}
break;
case 2 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5763:6: ( ( rule__XMemberFeatureCall__Group_1_1_3_1_1__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5763:6: ( ( rule__XMemberFeatureCall__Group_1_1_3_1_1__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5764:1: ( rule__XMemberFeatureCall__Group_1_1_3_1_1__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXMemberFeatureCallAccess().getGroup_1_1_3_1_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5765:1: ( rule__XMemberFeatureCall__Group_1_1_3_1_1__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5765:2: rule__XMemberFeatureCall__Group_1_1_3_1_1__0
{
pushFollow(FollowSets000.FOLLOW_rule__XMemberFeatureCall__Group_1_1_3_1_1__0_in_rule__XMemberFeatureCall__Alternatives_1_1_3_112502);
rule__XMemberFeatureCall__Group_1_1_3_1_1__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXMemberFeatureCallAccess().getGroup_1_1_3_1_1());
}
}
}
break;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XMemberFeatureCall__Alternatives_1_1_3_1"
// $ANTLR start "rule__XPrimaryExpression__Alternatives"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5774:1: rule__XPrimaryExpression__Alternatives : ( ( ruleXConstructorCall ) | ( ruleXBlockExpression ) | ( ruleXSwitchExpression ) | ( ( ruleXSynchronizedExpression ) ) | ( ruleXFeatureCall ) | ( ruleXLiteral ) | ( ruleXIfExpression ) | ( ( ruleXForLoopExpression ) ) | ( ruleXBasicForLoopExpression ) | ( ruleXWhileExpression ) | ( ruleXDoWhileExpression ) | ( ruleXThrowExpression ) | ( ruleXReturnExpression ) | ( ruleXTryCatchFinallyExpression ) | ( ruleXParenthesizedExpression ) );
public final void rule__XPrimaryExpression__Alternatives() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5778:1: ( ( ruleXConstructorCall ) | ( ruleXBlockExpression ) | ( ruleXSwitchExpression ) | ( ( ruleXSynchronizedExpression ) ) | ( ruleXFeatureCall ) | ( ruleXLiteral ) | ( ruleXIfExpression ) | ( ( ruleXForLoopExpression ) ) | ( ruleXBasicForLoopExpression ) | ( ruleXWhileExpression ) | ( ruleXDoWhileExpression ) | ( ruleXThrowExpression ) | ( ruleXReturnExpression ) | ( ruleXTryCatchFinallyExpression ) | ( ruleXParenthesizedExpression ) )
int alt31=15;
alt31 = dfa31.predict(input);
switch (alt31) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5779:1: ( ruleXConstructorCall )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5779:1: ( ruleXConstructorCall )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5780:1: ruleXConstructorCall
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXPrimaryExpressionAccess().getXConstructorCallParserRuleCall_0());
}
pushFollow(FollowSets000.FOLLOW_ruleXConstructorCall_in_rule__XPrimaryExpression__Alternatives12535);
ruleXConstructorCall();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXPrimaryExpressionAccess().getXConstructorCallParserRuleCall_0());
}
}
}
break;
case 2 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5785:6: ( ruleXBlockExpression )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5785:6: ( ruleXBlockExpression )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5786:1: ruleXBlockExpression
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXPrimaryExpressionAccess().getXBlockExpressionParserRuleCall_1());
}
pushFollow(FollowSets000.FOLLOW_ruleXBlockExpression_in_rule__XPrimaryExpression__Alternatives12552);
ruleXBlockExpression();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXPrimaryExpressionAccess().getXBlockExpressionParserRuleCall_1());
}
}
}
break;
case 3 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5791:6: ( ruleXSwitchExpression )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5791:6: ( ruleXSwitchExpression )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5792:1: ruleXSwitchExpression
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXPrimaryExpressionAccess().getXSwitchExpressionParserRuleCall_2());
}
pushFollow(FollowSets000.FOLLOW_ruleXSwitchExpression_in_rule__XPrimaryExpression__Alternatives12569);
ruleXSwitchExpression();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXPrimaryExpressionAccess().getXSwitchExpressionParserRuleCall_2());
}
}
}
break;
case 4 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5797:6: ( ( ruleXSynchronizedExpression ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5797:6: ( ( ruleXSynchronizedExpression ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5798:1: ( ruleXSynchronizedExpression )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXPrimaryExpressionAccess().getXSynchronizedExpressionParserRuleCall_3());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5799:1: ( ruleXSynchronizedExpression )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5799:3: ruleXSynchronizedExpression
{
pushFollow(FollowSets000.FOLLOW_ruleXSynchronizedExpression_in_rule__XPrimaryExpression__Alternatives12587);
ruleXSynchronizedExpression();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXPrimaryExpressionAccess().getXSynchronizedExpressionParserRuleCall_3());
}
}
}
break;
case 5 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5803:6: ( ruleXFeatureCall )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5803:6: ( ruleXFeatureCall )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5804:1: ruleXFeatureCall
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXPrimaryExpressionAccess().getXFeatureCallParserRuleCall_4());
}
pushFollow(FollowSets000.FOLLOW_ruleXFeatureCall_in_rule__XPrimaryExpression__Alternatives12605);
ruleXFeatureCall();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXPrimaryExpressionAccess().getXFeatureCallParserRuleCall_4());
}
}
}
break;
case 6 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5809:6: ( ruleXLiteral )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5809:6: ( ruleXLiteral )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5810:1: ruleXLiteral
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXPrimaryExpressionAccess().getXLiteralParserRuleCall_5());
}
pushFollow(FollowSets000.FOLLOW_ruleXLiteral_in_rule__XPrimaryExpression__Alternatives12622);
ruleXLiteral();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXPrimaryExpressionAccess().getXLiteralParserRuleCall_5());
}
}
}
break;
case 7 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5815:6: ( ruleXIfExpression )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5815:6: ( ruleXIfExpression )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5816:1: ruleXIfExpression
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXPrimaryExpressionAccess().getXIfExpressionParserRuleCall_6());
}
pushFollow(FollowSets000.FOLLOW_ruleXIfExpression_in_rule__XPrimaryExpression__Alternatives12639);
ruleXIfExpression();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXPrimaryExpressionAccess().getXIfExpressionParserRuleCall_6());
}
}
}
break;
case 8 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5821:6: ( ( ruleXForLoopExpression ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5821:6: ( ( ruleXForLoopExpression ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5822:1: ( ruleXForLoopExpression )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXPrimaryExpressionAccess().getXForLoopExpressionParserRuleCall_7());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5823:1: ( ruleXForLoopExpression )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5823:3: ruleXForLoopExpression
{
pushFollow(FollowSets000.FOLLOW_ruleXForLoopExpression_in_rule__XPrimaryExpression__Alternatives12657);
ruleXForLoopExpression();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXPrimaryExpressionAccess().getXForLoopExpressionParserRuleCall_7());
}
}
}
break;
case 9 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5827:6: ( ruleXBasicForLoopExpression )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5827:6: ( ruleXBasicForLoopExpression )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5828:1: ruleXBasicForLoopExpression
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXPrimaryExpressionAccess().getXBasicForLoopExpressionParserRuleCall_8());
}
pushFollow(FollowSets000.FOLLOW_ruleXBasicForLoopExpression_in_rule__XPrimaryExpression__Alternatives12675);
ruleXBasicForLoopExpression();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXPrimaryExpressionAccess().getXBasicForLoopExpressionParserRuleCall_8());
}
}
}
break;
case 10 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5833:6: ( ruleXWhileExpression )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5833:6: ( ruleXWhileExpression )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5834:1: ruleXWhileExpression
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXPrimaryExpressionAccess().getXWhileExpressionParserRuleCall_9());
}
pushFollow(FollowSets000.FOLLOW_ruleXWhileExpression_in_rule__XPrimaryExpression__Alternatives12692);
ruleXWhileExpression();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXPrimaryExpressionAccess().getXWhileExpressionParserRuleCall_9());
}
}
}
break;
case 11 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5839:6: ( ruleXDoWhileExpression )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5839:6: ( ruleXDoWhileExpression )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5840:1: ruleXDoWhileExpression
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXPrimaryExpressionAccess().getXDoWhileExpressionParserRuleCall_10());
}
pushFollow(FollowSets000.FOLLOW_ruleXDoWhileExpression_in_rule__XPrimaryExpression__Alternatives12709);
ruleXDoWhileExpression();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXPrimaryExpressionAccess().getXDoWhileExpressionParserRuleCall_10());
}
}
}
break;
case 12 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5845:6: ( ruleXThrowExpression )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5845:6: ( ruleXThrowExpression )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5846:1: ruleXThrowExpression
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXPrimaryExpressionAccess().getXThrowExpressionParserRuleCall_11());
}
pushFollow(FollowSets000.FOLLOW_ruleXThrowExpression_in_rule__XPrimaryExpression__Alternatives12726);
ruleXThrowExpression();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXPrimaryExpressionAccess().getXThrowExpressionParserRuleCall_11());
}
}
}
break;
case 13 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5851:6: ( ruleXReturnExpression )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5851:6: ( ruleXReturnExpression )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5852:1: ruleXReturnExpression
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXPrimaryExpressionAccess().getXReturnExpressionParserRuleCall_12());
}
pushFollow(FollowSets000.FOLLOW_ruleXReturnExpression_in_rule__XPrimaryExpression__Alternatives12743);
ruleXReturnExpression();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXPrimaryExpressionAccess().getXReturnExpressionParserRuleCall_12());
}
}
}
break;
case 14 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5857:6: ( ruleXTryCatchFinallyExpression )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5857:6: ( ruleXTryCatchFinallyExpression )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5858:1: ruleXTryCatchFinallyExpression
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXPrimaryExpressionAccess().getXTryCatchFinallyExpressionParserRuleCall_13());
}
pushFollow(FollowSets000.FOLLOW_ruleXTryCatchFinallyExpression_in_rule__XPrimaryExpression__Alternatives12760);
ruleXTryCatchFinallyExpression();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXPrimaryExpressionAccess().getXTryCatchFinallyExpressionParserRuleCall_13());
}
}
}
break;
case 15 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5863:6: ( ruleXParenthesizedExpression )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5863:6: ( ruleXParenthesizedExpression )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5864:1: ruleXParenthesizedExpression
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXPrimaryExpressionAccess().getXParenthesizedExpressionParserRuleCall_14());
}
pushFollow(FollowSets000.FOLLOW_ruleXParenthesizedExpression_in_rule__XPrimaryExpression__Alternatives12777);
ruleXParenthesizedExpression();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXPrimaryExpressionAccess().getXParenthesizedExpressionParserRuleCall_14());
}
}
}
break;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XPrimaryExpression__Alternatives"
// $ANTLR start "rule__XLiteral__Alternatives"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5874:1: rule__XLiteral__Alternatives : ( ( ruleXCollectionLiteral ) | ( ( ruleXClosure ) ) | ( ruleXBooleanLiteral ) | ( ruleXNumberLiteral ) | ( ruleXNullLiteral ) | ( ruleXStringLiteral ) | ( ruleXTypeLiteral ) );
public final void rule__XLiteral__Alternatives() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5878:1: ( ( ruleXCollectionLiteral ) | ( ( ruleXClosure ) ) | ( ruleXBooleanLiteral ) | ( ruleXNumberLiteral ) | ( ruleXNullLiteral ) | ( ruleXStringLiteral ) | ( ruleXTypeLiteral ) )
int alt32=7;
switch ( input.LA(1) ) {
case 155:
{
alt32=1;
}
break;
case 156:
{
alt32=2;
}
break;
case 50:
case 185:
{
alt32=3;
}
break;
case RULE_HEX:
case RULE_INT:
case RULE_DECIMAL:
{
alt32=4;
}
break;
case 169:
{
alt32=5;
}
break;
case RULE_STRING:
{
alt32=6;
}
break;
case 170:
{
alt32=7;
}
break;
default:
if (state.backtracking>0) {state.failed=true; return ;}
NoViableAltException nvae =
new NoViableAltException("", 32, 0, input);
throw nvae;
}
switch (alt32) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5879:1: ( ruleXCollectionLiteral )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5879:1: ( ruleXCollectionLiteral )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5880:1: ruleXCollectionLiteral
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXLiteralAccess().getXCollectionLiteralParserRuleCall_0());
}
pushFollow(FollowSets000.FOLLOW_ruleXCollectionLiteral_in_rule__XLiteral__Alternatives12809);
ruleXCollectionLiteral();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXLiteralAccess().getXCollectionLiteralParserRuleCall_0());
}
}
}
break;
case 2 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5885:6: ( ( ruleXClosure ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5885:6: ( ( ruleXClosure ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5886:1: ( ruleXClosure )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXLiteralAccess().getXClosureParserRuleCall_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5887:1: ( ruleXClosure )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5887:3: ruleXClosure
{
pushFollow(FollowSets000.FOLLOW_ruleXClosure_in_rule__XLiteral__Alternatives12827);
ruleXClosure();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXLiteralAccess().getXClosureParserRuleCall_1());
}
}
}
break;
case 3 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5891:6: ( ruleXBooleanLiteral )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5891:6: ( ruleXBooleanLiteral )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5892:1: ruleXBooleanLiteral
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXLiteralAccess().getXBooleanLiteralParserRuleCall_2());
}
pushFollow(FollowSets000.FOLLOW_ruleXBooleanLiteral_in_rule__XLiteral__Alternatives12845);
ruleXBooleanLiteral();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXLiteralAccess().getXBooleanLiteralParserRuleCall_2());
}
}
}
break;
case 4 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5897:6: ( ruleXNumberLiteral )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5897:6: ( ruleXNumberLiteral )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5898:1: ruleXNumberLiteral
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXLiteralAccess().getXNumberLiteralParserRuleCall_3());
}
pushFollow(FollowSets000.FOLLOW_ruleXNumberLiteral_in_rule__XLiteral__Alternatives12862);
ruleXNumberLiteral();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXLiteralAccess().getXNumberLiteralParserRuleCall_3());
}
}
}
break;
case 5 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5903:6: ( ruleXNullLiteral )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5903:6: ( ruleXNullLiteral )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5904:1: ruleXNullLiteral
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXLiteralAccess().getXNullLiteralParserRuleCall_4());
}
pushFollow(FollowSets000.FOLLOW_ruleXNullLiteral_in_rule__XLiteral__Alternatives12879);
ruleXNullLiteral();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXLiteralAccess().getXNullLiteralParserRuleCall_4());
}
}
}
break;
case 6 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5909:6: ( ruleXStringLiteral )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5909:6: ( ruleXStringLiteral )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5910:1: ruleXStringLiteral
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXLiteralAccess().getXStringLiteralParserRuleCall_5());
}
pushFollow(FollowSets000.FOLLOW_ruleXStringLiteral_in_rule__XLiteral__Alternatives12896);
ruleXStringLiteral();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXLiteralAccess().getXStringLiteralParserRuleCall_5());
}
}
}
break;
case 7 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5915:6: ( ruleXTypeLiteral )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5915:6: ( ruleXTypeLiteral )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5916:1: ruleXTypeLiteral
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXLiteralAccess().getXTypeLiteralParserRuleCall_6());
}
pushFollow(FollowSets000.FOLLOW_ruleXTypeLiteral_in_rule__XLiteral__Alternatives12913);
ruleXTypeLiteral();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXLiteralAccess().getXTypeLiteralParserRuleCall_6());
}
}
}
break;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XLiteral__Alternatives"
// $ANTLR start "rule__XCollectionLiteral__Alternatives"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5926:1: rule__XCollectionLiteral__Alternatives : ( ( ruleXSetLiteral ) | ( ruleXListLiteral ) );
public final void rule__XCollectionLiteral__Alternatives() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5930:1: ( ( ruleXSetLiteral ) | ( ruleXListLiteral ) )
int alt33=2;
int LA33_0 = input.LA(1);
if ( (LA33_0==155) ) {
int LA33_1 = input.LA(2);
if ( (LA33_1==67) ) {
alt33=1;
}
else if ( (LA33_1==156) ) {
alt33=2;
}
else {
if (state.backtracking>0) {state.failed=true; return ;}
NoViableAltException nvae =
new NoViableAltException("", 33, 1, input);
throw nvae;
}
}
else {
if (state.backtracking>0) {state.failed=true; return ;}
NoViableAltException nvae =
new NoViableAltException("", 33, 0, input);
throw nvae;
}
switch (alt33) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5931:1: ( ruleXSetLiteral )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5931:1: ( ruleXSetLiteral )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5932:1: ruleXSetLiteral
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXCollectionLiteralAccess().getXSetLiteralParserRuleCall_0());
}
pushFollow(FollowSets000.FOLLOW_ruleXSetLiteral_in_rule__XCollectionLiteral__Alternatives12945);
ruleXSetLiteral();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXCollectionLiteralAccess().getXSetLiteralParserRuleCall_0());
}
}
}
break;
case 2 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5937:6: ( ruleXListLiteral )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5937:6: ( ruleXListLiteral )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5938:1: ruleXListLiteral
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXCollectionLiteralAccess().getXListLiteralParserRuleCall_1());
}
pushFollow(FollowSets000.FOLLOW_ruleXListLiteral_in_rule__XCollectionLiteral__Alternatives12962);
ruleXListLiteral();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXCollectionLiteralAccess().getXListLiteralParserRuleCall_1());
}
}
}
break;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XCollectionLiteral__Alternatives"
// $ANTLR start "rule__XSwitchExpression__Alternatives_2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5948:1: rule__XSwitchExpression__Alternatives_2 : ( ( ( rule__XSwitchExpression__Group_2_0__0 ) ) | ( ( rule__XSwitchExpression__Group_2_1__0 ) ) );
public final void rule__XSwitchExpression__Alternatives_2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5952:1: ( ( ( rule__XSwitchExpression__Group_2_0__0 ) ) | ( ( rule__XSwitchExpression__Group_2_1__0 ) ) )
int alt34=2;
alt34 = dfa34.predict(input);
switch (alt34) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5953:1: ( ( rule__XSwitchExpression__Group_2_0__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5953:1: ( ( rule__XSwitchExpression__Group_2_0__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5954:1: ( rule__XSwitchExpression__Group_2_0__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXSwitchExpressionAccess().getGroup_2_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5955:1: ( rule__XSwitchExpression__Group_2_0__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5955:2: rule__XSwitchExpression__Group_2_0__0
{
pushFollow(FollowSets000.FOLLOW_rule__XSwitchExpression__Group_2_0__0_in_rule__XSwitchExpression__Alternatives_212994);
rule__XSwitchExpression__Group_2_0__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXSwitchExpressionAccess().getGroup_2_0());
}
}
}
break;
case 2 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5959:6: ( ( rule__XSwitchExpression__Group_2_1__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5959:6: ( ( rule__XSwitchExpression__Group_2_1__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5960:1: ( rule__XSwitchExpression__Group_2_1__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXSwitchExpressionAccess().getGroup_2_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5961:1: ( rule__XSwitchExpression__Group_2_1__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5961:2: rule__XSwitchExpression__Group_2_1__0
{
pushFollow(FollowSets000.FOLLOW_rule__XSwitchExpression__Group_2_1__0_in_rule__XSwitchExpression__Alternatives_213012);
rule__XSwitchExpression__Group_2_1__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXSwitchExpressionAccess().getGroup_2_1());
}
}
}
break;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XSwitchExpression__Alternatives_2"
// $ANTLR start "rule__XCasePart__Alternatives_3"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5970:1: rule__XCasePart__Alternatives_3 : ( ( ( rule__XCasePart__Group_3_0__0 ) ) | ( ( rule__XCasePart__FallThroughAssignment_3_1 ) ) );
public final void rule__XCasePart__Alternatives_3() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5974:1: ( ( ( rule__XCasePart__Group_3_0__0 ) ) | ( ( rule__XCasePart__FallThroughAssignment_3_1 ) ) )
int alt35=2;
int LA35_0 = input.LA(1);
if ( (LA35_0==162) ) {
alt35=1;
}
else if ( (LA35_0==154) ) {
alt35=2;
}
else {
if (state.backtracking>0) {state.failed=true; return ;}
NoViableAltException nvae =
new NoViableAltException("", 35, 0, input);
throw nvae;
}
switch (alt35) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5975:1: ( ( rule__XCasePart__Group_3_0__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5975:1: ( ( rule__XCasePart__Group_3_0__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5976:1: ( rule__XCasePart__Group_3_0__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXCasePartAccess().getGroup_3_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5977:1: ( rule__XCasePart__Group_3_0__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5977:2: rule__XCasePart__Group_3_0__0
{
pushFollow(FollowSets000.FOLLOW_rule__XCasePart__Group_3_0__0_in_rule__XCasePart__Alternatives_313045);
rule__XCasePart__Group_3_0__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXCasePartAccess().getGroup_3_0());
}
}
}
break;
case 2 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5981:6: ( ( rule__XCasePart__FallThroughAssignment_3_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5981:6: ( ( rule__XCasePart__FallThroughAssignment_3_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5982:1: ( rule__XCasePart__FallThroughAssignment_3_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXCasePartAccess().getFallThroughAssignment_3_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5983:1: ( rule__XCasePart__FallThroughAssignment_3_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5983:2: rule__XCasePart__FallThroughAssignment_3_1
{
pushFollow(FollowSets000.FOLLOW_rule__XCasePart__FallThroughAssignment_3_1_in_rule__XCasePart__Alternatives_313063);
rule__XCasePart__FallThroughAssignment_3_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXCasePartAccess().getFallThroughAssignment_3_1());
}
}
}
break;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XCasePart__Alternatives_3"
// $ANTLR start "rule__XExpressionOrVarDeclaration__Alternatives"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5992:1: rule__XExpressionOrVarDeclaration__Alternatives : ( ( ruleXVariableDeclaration ) | ( ruleXExpression ) );
public final void rule__XExpressionOrVarDeclaration__Alternatives() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5996:1: ( ( ruleXVariableDeclaration ) | ( ruleXExpression ) )
int alt36=2;
int LA36_0 = input.LA(1);
if ( (LA36_0==44||LA36_0==184) ) {
alt36=1;
}
else if ( ((LA36_0>=RULE_ID && LA36_0<=RULE_STRING)||LA36_0==27||(LA36_0>=34 && LA36_0<=35)||LA36_0==40||(LA36_0>=45 && LA36_0<=50)||LA36_0==67||LA36_0==144||(LA36_0>=155 && LA36_0<=156)||LA36_0==159||LA36_0==161||(LA36_0>=165 && LA36_0<=173)||LA36_0==175||LA36_0==185) ) {
alt36=2;
}
else {
if (state.backtracking>0) {state.failed=true; return ;}
NoViableAltException nvae =
new NoViableAltException("", 36, 0, input);
throw nvae;
}
switch (alt36) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5997:1: ( ruleXVariableDeclaration )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5997:1: ( ruleXVariableDeclaration )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5998:1: ruleXVariableDeclaration
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXExpressionOrVarDeclarationAccess().getXVariableDeclarationParserRuleCall_0());
}
pushFollow(FollowSets000.FOLLOW_ruleXVariableDeclaration_in_rule__XExpressionOrVarDeclaration__Alternatives13096);
ruleXVariableDeclaration();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXExpressionOrVarDeclarationAccess().getXVariableDeclarationParserRuleCall_0());
}
}
}
break;
case 2 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6003:6: ( ruleXExpression )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6003:6: ( ruleXExpression )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6004:1: ruleXExpression
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXExpressionOrVarDeclarationAccess().getXExpressionParserRuleCall_1());
}
pushFollow(FollowSets000.FOLLOW_ruleXExpression_in_rule__XExpressionOrVarDeclaration__Alternatives13113);
ruleXExpression();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXExpressionOrVarDeclarationAccess().getXExpressionParserRuleCall_1());
}
}
}
break;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XExpressionOrVarDeclaration__Alternatives"
// $ANTLR start "rule__XVariableDeclaration__Alternatives_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6014:1: rule__XVariableDeclaration__Alternatives_1 : ( ( ( rule__XVariableDeclaration__WriteableAssignment_1_0 ) ) | ( 'val' ) );
public final void rule__XVariableDeclaration__Alternatives_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6018:1: ( ( ( rule__XVariableDeclaration__WriteableAssignment_1_0 ) ) | ( 'val' ) )
int alt37=2;
int LA37_0 = input.LA(1);
if ( (LA37_0==184) ) {
alt37=1;
}
else if ( (LA37_0==44) ) {
alt37=2;
}
else {
if (state.backtracking>0) {state.failed=true; return ;}
NoViableAltException nvae =
new NoViableAltException("", 37, 0, input);
throw nvae;
}
switch (alt37) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6019:1: ( ( rule__XVariableDeclaration__WriteableAssignment_1_0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6019:1: ( ( rule__XVariableDeclaration__WriteableAssignment_1_0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6020:1: ( rule__XVariableDeclaration__WriteableAssignment_1_0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXVariableDeclarationAccess().getWriteableAssignment_1_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6021:1: ( rule__XVariableDeclaration__WriteableAssignment_1_0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6021:2: rule__XVariableDeclaration__WriteableAssignment_1_0
{
pushFollow(FollowSets000.FOLLOW_rule__XVariableDeclaration__WriteableAssignment_1_0_in_rule__XVariableDeclaration__Alternatives_113145);
rule__XVariableDeclaration__WriteableAssignment_1_0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXVariableDeclarationAccess().getWriteableAssignment_1_0());
}
}
}
break;
case 2 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6025:6: ( 'val' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6025:6: ( 'val' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6026:1: 'val'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXVariableDeclarationAccess().getValKeyword_1_1());
}
match(input,44,FollowSets000.FOLLOW_44_in_rule__XVariableDeclaration__Alternatives_113164); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXVariableDeclarationAccess().getValKeyword_1_1());
}
}
}
break;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XVariableDeclaration__Alternatives_1"
// $ANTLR start "rule__XVariableDeclaration__Alternatives_2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6038:1: rule__XVariableDeclaration__Alternatives_2 : ( ( ( rule__XVariableDeclaration__Group_2_0__0 ) ) | ( ( rule__XVariableDeclaration__NameAssignment_2_1 ) ) );
public final void rule__XVariableDeclaration__Alternatives_2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6042:1: ( ( ( rule__XVariableDeclaration__Group_2_0__0 ) ) | ( ( rule__XVariableDeclaration__NameAssignment_2_1 ) ) )
int alt38=2;
int LA38_0 = input.LA(1);
if ( (LA38_0==RULE_ID) ) {
int LA38_1 = input.LA(2);
if ( (synpred115_InternalMango()) ) {
alt38=1;
}
else if ( (true) ) {
alt38=2;
}
else {
if (state.backtracking>0) {state.failed=true; return ;}
NoViableAltException nvae =
new NoViableAltException("", 38, 1, input);
throw nvae;
}
}
else if ( (LA38_0==31||LA38_0==144) ) {
alt38=1;
}
else {
if (state.backtracking>0) {state.failed=true; return ;}
NoViableAltException nvae =
new NoViableAltException("", 38, 0, input);
throw nvae;
}
switch (alt38) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6043:1: ( ( rule__XVariableDeclaration__Group_2_0__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6043:1: ( ( rule__XVariableDeclaration__Group_2_0__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6044:1: ( rule__XVariableDeclaration__Group_2_0__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXVariableDeclarationAccess().getGroup_2_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6045:1: ( rule__XVariableDeclaration__Group_2_0__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6045:2: rule__XVariableDeclaration__Group_2_0__0
{
pushFollow(FollowSets000.FOLLOW_rule__XVariableDeclaration__Group_2_0__0_in_rule__XVariableDeclaration__Alternatives_213198);
rule__XVariableDeclaration__Group_2_0__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXVariableDeclarationAccess().getGroup_2_0());
}
}
}
break;
case 2 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6049:6: ( ( rule__XVariableDeclaration__NameAssignment_2_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6049:6: ( ( rule__XVariableDeclaration__NameAssignment_2_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6050:1: ( rule__XVariableDeclaration__NameAssignment_2_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXVariableDeclarationAccess().getNameAssignment_2_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6051:1: ( rule__XVariableDeclaration__NameAssignment_2_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6051:2: rule__XVariableDeclaration__NameAssignment_2_1
{
pushFollow(FollowSets000.FOLLOW_rule__XVariableDeclaration__NameAssignment_2_1_in_rule__XVariableDeclaration__Alternatives_213216);
rule__XVariableDeclaration__NameAssignment_2_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXVariableDeclarationAccess().getNameAssignment_2_1());
}
}
}
break;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XVariableDeclaration__Alternatives_2"
// $ANTLR start "rule__XFeatureCall__Alternatives_3_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6060:1: rule__XFeatureCall__Alternatives_3_1 : ( ( ( rule__XFeatureCall__FeatureCallArgumentsAssignment_3_1_0 ) ) | ( ( rule__XFeatureCall__Group_3_1_1__0 ) ) );
public final void rule__XFeatureCall__Alternatives_3_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6064:1: ( ( ( rule__XFeatureCall__FeatureCallArgumentsAssignment_3_1_0 ) ) | ( ( rule__XFeatureCall__Group_3_1_1__0 ) ) )
int alt39=2;
alt39 = dfa39.predict(input);
switch (alt39) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6065:1: ( ( rule__XFeatureCall__FeatureCallArgumentsAssignment_3_1_0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6065:1: ( ( rule__XFeatureCall__FeatureCallArgumentsAssignment_3_1_0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6066:1: ( rule__XFeatureCall__FeatureCallArgumentsAssignment_3_1_0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXFeatureCallAccess().getFeatureCallArgumentsAssignment_3_1_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6067:1: ( rule__XFeatureCall__FeatureCallArgumentsAssignment_3_1_0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6067:2: rule__XFeatureCall__FeatureCallArgumentsAssignment_3_1_0
{
pushFollow(FollowSets000.FOLLOW_rule__XFeatureCall__FeatureCallArgumentsAssignment_3_1_0_in_rule__XFeatureCall__Alternatives_3_113249);
rule__XFeatureCall__FeatureCallArgumentsAssignment_3_1_0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXFeatureCallAccess().getFeatureCallArgumentsAssignment_3_1_0());
}
}
}
break;
case 2 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6071:6: ( ( rule__XFeatureCall__Group_3_1_1__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6071:6: ( ( rule__XFeatureCall__Group_3_1_1__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6072:1: ( rule__XFeatureCall__Group_3_1_1__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXFeatureCallAccess().getGroup_3_1_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6073:1: ( rule__XFeatureCall__Group_3_1_1__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6073:2: rule__XFeatureCall__Group_3_1_1__0
{
pushFollow(FollowSets000.FOLLOW_rule__XFeatureCall__Group_3_1_1__0_in_rule__XFeatureCall__Alternatives_3_113267);
rule__XFeatureCall__Group_3_1_1__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXFeatureCallAccess().getGroup_3_1_1());
}
}
}
break;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XFeatureCall__Alternatives_3_1"
// $ANTLR start "rule__FeatureCallID__Alternatives"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6082:1: rule__FeatureCallID__Alternatives : ( ( ruleValidID ) | ( 'extends' ) | ( 'static' ) | ( 'import' ) | ( 'extension' ) );
public final void rule__FeatureCallID__Alternatives() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6086:1: ( ( ruleValidID ) | ( 'extends' ) | ( 'static' ) | ( 'import' ) | ( 'extension' ) )
int alt40=5;
switch ( input.LA(1) ) {
case RULE_ID:
{
alt40=1;
}
break;
case 45:
{
alt40=2;
}
break;
case 46:
{
alt40=3;
}
break;
case 47:
{
alt40=4;
}
break;
case 48:
{
alt40=5;
}
break;
default:
if (state.backtracking>0) {state.failed=true; return ;}
NoViableAltException nvae =
new NoViableAltException("", 40, 0, input);
throw nvae;
}
switch (alt40) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6087:1: ( ruleValidID )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6087:1: ( ruleValidID )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6088:1: ruleValidID
{
if ( state.backtracking==0 ) {
before(grammarAccess.getFeatureCallIDAccess().getValidIDParserRuleCall_0());
}
pushFollow(FollowSets000.FOLLOW_ruleValidID_in_rule__FeatureCallID__Alternatives13300);
ruleValidID();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getFeatureCallIDAccess().getValidIDParserRuleCall_0());
}
}
}
break;
case 2 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6093:6: ( 'extends' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6093:6: ( 'extends' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6094:1: 'extends'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getFeatureCallIDAccess().getExtendsKeyword_1());
}
match(input,45,FollowSets000.FOLLOW_45_in_rule__FeatureCallID__Alternatives13318); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getFeatureCallIDAccess().getExtendsKeyword_1());
}
}
}
break;
case 3 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6101:6: ( 'static' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6101:6: ( 'static' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6102:1: 'static'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getFeatureCallIDAccess().getStaticKeyword_2());
}
match(input,46,FollowSets000.FOLLOW_46_in_rule__FeatureCallID__Alternatives13338); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getFeatureCallIDAccess().getStaticKeyword_2());
}
}
}
break;
case 4 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6109:6: ( 'import' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6109:6: ( 'import' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6110:1: 'import'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getFeatureCallIDAccess().getImportKeyword_3());
}
match(input,47,FollowSets000.FOLLOW_47_in_rule__FeatureCallID__Alternatives13358); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getFeatureCallIDAccess().getImportKeyword_3());
}
}
}
break;
case 5 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6117:6: ( 'extension' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6117:6: ( 'extension' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6118:1: 'extension'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getFeatureCallIDAccess().getExtensionKeyword_4());
}
match(input,48,FollowSets000.FOLLOW_48_in_rule__FeatureCallID__Alternatives13378); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getFeatureCallIDAccess().getExtensionKeyword_4());
}
}
}
break;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__FeatureCallID__Alternatives"
// $ANTLR start "rule__IdOrSuper__Alternatives"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6130:1: rule__IdOrSuper__Alternatives : ( ( ruleFeatureCallID ) | ( 'super' ) );
public final void rule__IdOrSuper__Alternatives() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6134:1: ( ( ruleFeatureCallID ) | ( 'super' ) )
int alt41=2;
int LA41_0 = input.LA(1);
if ( (LA41_0==RULE_ID||(LA41_0>=45 && LA41_0<=48)) ) {
alt41=1;
}
else if ( (LA41_0==49) ) {
alt41=2;
}
else {
if (state.backtracking>0) {state.failed=true; return ;}
NoViableAltException nvae =
new NoViableAltException("", 41, 0, input);
throw nvae;
}
switch (alt41) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6135:1: ( ruleFeatureCallID )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6135:1: ( ruleFeatureCallID )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6136:1: ruleFeatureCallID
{
if ( state.backtracking==0 ) {
before(grammarAccess.getIdOrSuperAccess().getFeatureCallIDParserRuleCall_0());
}
pushFollow(FollowSets000.FOLLOW_ruleFeatureCallID_in_rule__IdOrSuper__Alternatives13412);
ruleFeatureCallID();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getIdOrSuperAccess().getFeatureCallIDParserRuleCall_0());
}
}
}
break;
case 2 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6141:6: ( 'super' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6141:6: ( 'super' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6142:1: 'super'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getIdOrSuperAccess().getSuperKeyword_1());
}
match(input,49,FollowSets000.FOLLOW_49_in_rule__IdOrSuper__Alternatives13430); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getIdOrSuperAccess().getSuperKeyword_1());
}
}
}
break;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__IdOrSuper__Alternatives"
// $ANTLR start "rule__XConstructorCall__Alternatives_4_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6154:1: rule__XConstructorCall__Alternatives_4_1 : ( ( ( rule__XConstructorCall__ArgumentsAssignment_4_1_0 ) ) | ( ( rule__XConstructorCall__Group_4_1_1__0 ) ) );
public final void rule__XConstructorCall__Alternatives_4_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6158:1: ( ( ( rule__XConstructorCall__ArgumentsAssignment_4_1_0 ) ) | ( ( rule__XConstructorCall__Group_4_1_1__0 ) ) )
int alt42=2;
alt42 = dfa42.predict(input);
switch (alt42) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6159:1: ( ( rule__XConstructorCall__ArgumentsAssignment_4_1_0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6159:1: ( ( rule__XConstructorCall__ArgumentsAssignment_4_1_0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6160:1: ( rule__XConstructorCall__ArgumentsAssignment_4_1_0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXConstructorCallAccess().getArgumentsAssignment_4_1_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6161:1: ( rule__XConstructorCall__ArgumentsAssignment_4_1_0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6161:2: rule__XConstructorCall__ArgumentsAssignment_4_1_0
{
pushFollow(FollowSets000.FOLLOW_rule__XConstructorCall__ArgumentsAssignment_4_1_0_in_rule__XConstructorCall__Alternatives_4_113464);
rule__XConstructorCall__ArgumentsAssignment_4_1_0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXConstructorCallAccess().getArgumentsAssignment_4_1_0());
}
}
}
break;
case 2 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6165:6: ( ( rule__XConstructorCall__Group_4_1_1__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6165:6: ( ( rule__XConstructorCall__Group_4_1_1__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6166:1: ( rule__XConstructorCall__Group_4_1_1__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXConstructorCallAccess().getGroup_4_1_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6167:1: ( rule__XConstructorCall__Group_4_1_1__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6167:2: rule__XConstructorCall__Group_4_1_1__0
{
pushFollow(FollowSets000.FOLLOW_rule__XConstructorCall__Group_4_1_1__0_in_rule__XConstructorCall__Alternatives_4_113482);
rule__XConstructorCall__Group_4_1_1__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXConstructorCallAccess().getGroup_4_1_1());
}
}
}
break;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XConstructorCall__Alternatives_4_1"
// $ANTLR start "rule__XBooleanLiteral__Alternatives_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6176:1: rule__XBooleanLiteral__Alternatives_1 : ( ( 'false' ) | ( ( rule__XBooleanLiteral__IsTrueAssignment_1_1 ) ) );
public final void rule__XBooleanLiteral__Alternatives_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6180:1: ( ( 'false' ) | ( ( rule__XBooleanLiteral__IsTrueAssignment_1_1 ) ) )
int alt43=2;
int LA43_0 = input.LA(1);
if ( (LA43_0==50) ) {
alt43=1;
}
else if ( (LA43_0==185) ) {
alt43=2;
}
else {
if (state.backtracking>0) {state.failed=true; return ;}
NoViableAltException nvae =
new NoViableAltException("", 43, 0, input);
throw nvae;
}
switch (alt43) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6181:1: ( 'false' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6181:1: ( 'false' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6182:1: 'false'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXBooleanLiteralAccess().getFalseKeyword_1_0());
}
match(input,50,FollowSets000.FOLLOW_50_in_rule__XBooleanLiteral__Alternatives_113516); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXBooleanLiteralAccess().getFalseKeyword_1_0());
}
}
}
break;
case 2 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6189:6: ( ( rule__XBooleanLiteral__IsTrueAssignment_1_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6189:6: ( ( rule__XBooleanLiteral__IsTrueAssignment_1_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6190:1: ( rule__XBooleanLiteral__IsTrueAssignment_1_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXBooleanLiteralAccess().getIsTrueAssignment_1_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6191:1: ( rule__XBooleanLiteral__IsTrueAssignment_1_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6191:2: rule__XBooleanLiteral__IsTrueAssignment_1_1
{
pushFollow(FollowSets000.FOLLOW_rule__XBooleanLiteral__IsTrueAssignment_1_1_in_rule__XBooleanLiteral__Alternatives_113535);
rule__XBooleanLiteral__IsTrueAssignment_1_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXBooleanLiteralAccess().getIsTrueAssignment_1_1());
}
}
}
break;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XBooleanLiteral__Alternatives_1"
// $ANTLR start "rule__XTryCatchFinallyExpression__Alternatives_3"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6200:1: rule__XTryCatchFinallyExpression__Alternatives_3 : ( ( ( rule__XTryCatchFinallyExpression__Group_3_0__0 ) ) | ( ( rule__XTryCatchFinallyExpression__Group_3_1__0 ) ) );
public final void rule__XTryCatchFinallyExpression__Alternatives_3() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6204:1: ( ( ( rule__XTryCatchFinallyExpression__Group_3_0__0 ) ) | ( ( rule__XTryCatchFinallyExpression__Group_3_1__0 ) ) )
int alt44=2;
int LA44_0 = input.LA(1);
if ( (LA44_0==176) ) {
alt44=1;
}
else if ( (LA44_0==174) ) {
alt44=2;
}
else {
if (state.backtracking>0) {state.failed=true; return ;}
NoViableAltException nvae =
new NoViableAltException("", 44, 0, input);
throw nvae;
}
switch (alt44) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6205:1: ( ( rule__XTryCatchFinallyExpression__Group_3_0__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6205:1: ( ( rule__XTryCatchFinallyExpression__Group_3_0__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6206:1: ( rule__XTryCatchFinallyExpression__Group_3_0__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXTryCatchFinallyExpressionAccess().getGroup_3_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6207:1: ( rule__XTryCatchFinallyExpression__Group_3_0__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6207:2: rule__XTryCatchFinallyExpression__Group_3_0__0
{
pushFollow(FollowSets000.FOLLOW_rule__XTryCatchFinallyExpression__Group_3_0__0_in_rule__XTryCatchFinallyExpression__Alternatives_313568);
rule__XTryCatchFinallyExpression__Group_3_0__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXTryCatchFinallyExpressionAccess().getGroup_3_0());
}
}
}
break;
case 2 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6211:6: ( ( rule__XTryCatchFinallyExpression__Group_3_1__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6211:6: ( ( rule__XTryCatchFinallyExpression__Group_3_1__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6212:1: ( rule__XTryCatchFinallyExpression__Group_3_1__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXTryCatchFinallyExpressionAccess().getGroup_3_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6213:1: ( rule__XTryCatchFinallyExpression__Group_3_1__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6213:2: rule__XTryCatchFinallyExpression__Group_3_1__0
{
pushFollow(FollowSets000.FOLLOW_rule__XTryCatchFinallyExpression__Group_3_1__0_in_rule__XTryCatchFinallyExpression__Alternatives_313586);
rule__XTryCatchFinallyExpression__Group_3_1__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXTryCatchFinallyExpressionAccess().getGroup_3_1());
}
}
}
break;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XTryCatchFinallyExpression__Alternatives_3"
// $ANTLR start "rule__Number__Alternatives"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6222:1: rule__Number__Alternatives : ( ( RULE_HEX ) | ( ( rule__Number__Group_1__0 ) ) );
public final void rule__Number__Alternatives() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6226:1: ( ( RULE_HEX ) | ( ( rule__Number__Group_1__0 ) ) )
int alt45=2;
int LA45_0 = input.LA(1);
if ( (LA45_0==RULE_HEX) ) {
alt45=1;
}
else if ( ((LA45_0>=RULE_INT && LA45_0<=RULE_DECIMAL)) ) {
alt45=2;
}
else {
if (state.backtracking>0) {state.failed=true; return ;}
NoViableAltException nvae =
new NoViableAltException("", 45, 0, input);
throw nvae;
}
switch (alt45) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6227:1: ( RULE_HEX )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6227:1: ( RULE_HEX )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6228:1: RULE_HEX
{
if ( state.backtracking==0 ) {
before(grammarAccess.getNumberAccess().getHEXTerminalRuleCall_0());
}
match(input,RULE_HEX,FollowSets000.FOLLOW_RULE_HEX_in_rule__Number__Alternatives13619); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getNumberAccess().getHEXTerminalRuleCall_0());
}
}
}
break;
case 2 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6233:6: ( ( rule__Number__Group_1__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6233:6: ( ( rule__Number__Group_1__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6234:1: ( rule__Number__Group_1__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getNumberAccess().getGroup_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6235:1: ( rule__Number__Group_1__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6235:2: rule__Number__Group_1__0
{
pushFollow(FollowSets000.FOLLOW_rule__Number__Group_1__0_in_rule__Number__Alternatives13636);
rule__Number__Group_1__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getNumberAccess().getGroup_1());
}
}
}
break;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Number__Alternatives"
// $ANTLR start "rule__Number__Alternatives_1_0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6244:1: rule__Number__Alternatives_1_0 : ( ( RULE_INT ) | ( RULE_DECIMAL ) );
public final void rule__Number__Alternatives_1_0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6248:1: ( ( RULE_INT ) | ( RULE_DECIMAL ) )
int alt46=2;
int LA46_0 = input.LA(1);
if ( (LA46_0==RULE_INT) ) {
alt46=1;
}
else if ( (LA46_0==RULE_DECIMAL) ) {
alt46=2;
}
else {
if (state.backtracking>0) {state.failed=true; return ;}
NoViableAltException nvae =
new NoViableAltException("", 46, 0, input);
throw nvae;
}
switch (alt46) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6249:1: ( RULE_INT )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6249:1: ( RULE_INT )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6250:1: RULE_INT
{
if ( state.backtracking==0 ) {
before(grammarAccess.getNumberAccess().getINTTerminalRuleCall_1_0_0());
}
match(input,RULE_INT,FollowSets000.FOLLOW_RULE_INT_in_rule__Number__Alternatives_1_013669); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getNumberAccess().getINTTerminalRuleCall_1_0_0());
}
}
}
break;
case 2 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6255:6: ( RULE_DECIMAL )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6255:6: ( RULE_DECIMAL )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6256:1: RULE_DECIMAL
{
if ( state.backtracking==0 ) {
before(grammarAccess.getNumberAccess().getDECIMALTerminalRuleCall_1_0_1());
}
match(input,RULE_DECIMAL,FollowSets000.FOLLOW_RULE_DECIMAL_in_rule__Number__Alternatives_1_013686); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getNumberAccess().getDECIMALTerminalRuleCall_1_0_1());
}
}
}
break;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Number__Alternatives_1_0"
// $ANTLR start "rule__Number__Alternatives_1_1_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6266:1: rule__Number__Alternatives_1_1_1 : ( ( RULE_INT ) | ( RULE_DECIMAL ) );
public final void rule__Number__Alternatives_1_1_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6270:1: ( ( RULE_INT ) | ( RULE_DECIMAL ) )
int alt47=2;
int LA47_0 = input.LA(1);
if ( (LA47_0==RULE_INT) ) {
alt47=1;
}
else if ( (LA47_0==RULE_DECIMAL) ) {
alt47=2;
}
else {
if (state.backtracking>0) {state.failed=true; return ;}
NoViableAltException nvae =
new NoViableAltException("", 47, 0, input);
throw nvae;
}
switch (alt47) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6271:1: ( RULE_INT )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6271:1: ( RULE_INT )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6272:1: RULE_INT
{
if ( state.backtracking==0 ) {
before(grammarAccess.getNumberAccess().getINTTerminalRuleCall_1_1_1_0());
}
match(input,RULE_INT,FollowSets000.FOLLOW_RULE_INT_in_rule__Number__Alternatives_1_1_113718); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getNumberAccess().getINTTerminalRuleCall_1_1_1_0());
}
}
}
break;
case 2 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6277:6: ( RULE_DECIMAL )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6277:6: ( RULE_DECIMAL )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6278:1: RULE_DECIMAL
{
if ( state.backtracking==0 ) {
before(grammarAccess.getNumberAccess().getDECIMALTerminalRuleCall_1_1_1_1());
}
match(input,RULE_DECIMAL,FollowSets000.FOLLOW_RULE_DECIMAL_in_rule__Number__Alternatives_1_1_113735); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getNumberAccess().getDECIMALTerminalRuleCall_1_1_1_1());
}
}
}
break;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Number__Alternatives_1_1_1"
// $ANTLR start "rule__JvmTypeReference__Alternatives"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6288:1: rule__JvmTypeReference__Alternatives : ( ( ( rule__JvmTypeReference__Group_0__0 ) ) | ( ruleXFunctionTypeRef ) );
public final void rule__JvmTypeReference__Alternatives() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6292:1: ( ( ( rule__JvmTypeReference__Group_0__0 ) ) | ( ruleXFunctionTypeRef ) )
int alt48=2;
int LA48_0 = input.LA(1);
if ( (LA48_0==RULE_ID) ) {
alt48=1;
}
else if ( (LA48_0==31||LA48_0==144) ) {
alt48=2;
}
else {
if (state.backtracking>0) {state.failed=true; return ;}
NoViableAltException nvae =
new NoViableAltException("", 48, 0, input);
throw nvae;
}
switch (alt48) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6293:1: ( ( rule__JvmTypeReference__Group_0__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6293:1: ( ( rule__JvmTypeReference__Group_0__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6294:1: ( rule__JvmTypeReference__Group_0__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getJvmTypeReferenceAccess().getGroup_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6295:1: ( rule__JvmTypeReference__Group_0__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6295:2: rule__JvmTypeReference__Group_0__0
{
pushFollow(FollowSets000.FOLLOW_rule__JvmTypeReference__Group_0__0_in_rule__JvmTypeReference__Alternatives13767);
rule__JvmTypeReference__Group_0__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getJvmTypeReferenceAccess().getGroup_0());
}
}
}
break;
case 2 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6299:6: ( ruleXFunctionTypeRef )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6299:6: ( ruleXFunctionTypeRef )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6300:1: ruleXFunctionTypeRef
{
if ( state.backtracking==0 ) {
before(grammarAccess.getJvmTypeReferenceAccess().getXFunctionTypeRefParserRuleCall_1());
}
pushFollow(FollowSets000.FOLLOW_ruleXFunctionTypeRef_in_rule__JvmTypeReference__Alternatives13785);
ruleXFunctionTypeRef();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getJvmTypeReferenceAccess().getXFunctionTypeRefParserRuleCall_1());
}
}
}
break;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__JvmTypeReference__Alternatives"
// $ANTLR start "rule__JvmArgumentTypeReference__Alternatives"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6310:1: rule__JvmArgumentTypeReference__Alternatives : ( ( ruleJvmTypeReference ) | ( ruleJvmWildcardTypeReference ) );
public final void rule__JvmArgumentTypeReference__Alternatives() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6314:1: ( ( ruleJvmTypeReference ) | ( ruleJvmWildcardTypeReference ) )
int alt49=2;
int LA49_0 = input.LA(1);
if ( (LA49_0==RULE_ID||LA49_0==31||LA49_0==144) ) {
alt49=1;
}
else if ( (LA49_0==177) ) {
alt49=2;
}
else {
if (state.backtracking>0) {state.failed=true; return ;}
NoViableAltException nvae =
new NoViableAltException("", 49, 0, input);
throw nvae;
}
switch (alt49) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6315:1: ( ruleJvmTypeReference )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6315:1: ( ruleJvmTypeReference )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6316:1: ruleJvmTypeReference
{
if ( state.backtracking==0 ) {
before(grammarAccess.getJvmArgumentTypeReferenceAccess().getJvmTypeReferenceParserRuleCall_0());
}
pushFollow(FollowSets000.FOLLOW_ruleJvmTypeReference_in_rule__JvmArgumentTypeReference__Alternatives13817);
ruleJvmTypeReference();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getJvmArgumentTypeReferenceAccess().getJvmTypeReferenceParserRuleCall_0());
}
}
}
break;
case 2 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6321:6: ( ruleJvmWildcardTypeReference )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6321:6: ( ruleJvmWildcardTypeReference )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6322:1: ruleJvmWildcardTypeReference
{
if ( state.backtracking==0 ) {
before(grammarAccess.getJvmArgumentTypeReferenceAccess().getJvmWildcardTypeReferenceParserRuleCall_1());
}
pushFollow(FollowSets000.FOLLOW_ruleJvmWildcardTypeReference_in_rule__JvmArgumentTypeReference__Alternatives13834);
ruleJvmWildcardTypeReference();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getJvmArgumentTypeReferenceAccess().getJvmWildcardTypeReferenceParserRuleCall_1());
}
}
}
break;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__JvmArgumentTypeReference__Alternatives"
// $ANTLR start "rule__JvmWildcardTypeReference__Alternatives_2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6332:1: rule__JvmWildcardTypeReference__Alternatives_2 : ( ( ( rule__JvmWildcardTypeReference__Group_2_0__0 ) ) | ( ( rule__JvmWildcardTypeReference__Group_2_1__0 ) ) );
public final void rule__JvmWildcardTypeReference__Alternatives_2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6336:1: ( ( ( rule__JvmWildcardTypeReference__Group_2_0__0 ) ) | ( ( rule__JvmWildcardTypeReference__Group_2_1__0 ) ) )
int alt50=2;
int LA50_0 = input.LA(1);
if ( (LA50_0==45) ) {
alt50=1;
}
else if ( (LA50_0==49) ) {
alt50=2;
}
else {
if (state.backtracking>0) {state.failed=true; return ;}
NoViableAltException nvae =
new NoViableAltException("", 50, 0, input);
throw nvae;
}
switch (alt50) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6337:1: ( ( rule__JvmWildcardTypeReference__Group_2_0__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6337:1: ( ( rule__JvmWildcardTypeReference__Group_2_0__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6338:1: ( rule__JvmWildcardTypeReference__Group_2_0__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getJvmWildcardTypeReferenceAccess().getGroup_2_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6339:1: ( rule__JvmWildcardTypeReference__Group_2_0__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6339:2: rule__JvmWildcardTypeReference__Group_2_0__0
{
pushFollow(FollowSets000.FOLLOW_rule__JvmWildcardTypeReference__Group_2_0__0_in_rule__JvmWildcardTypeReference__Alternatives_213866);
rule__JvmWildcardTypeReference__Group_2_0__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getJvmWildcardTypeReferenceAccess().getGroup_2_0());
}
}
}
break;
case 2 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6343:6: ( ( rule__JvmWildcardTypeReference__Group_2_1__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6343:6: ( ( rule__JvmWildcardTypeReference__Group_2_1__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6344:1: ( rule__JvmWildcardTypeReference__Group_2_1__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getJvmWildcardTypeReferenceAccess().getGroup_2_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6345:1: ( rule__JvmWildcardTypeReference__Group_2_1__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6345:2: rule__JvmWildcardTypeReference__Group_2_1__0
{
pushFollow(FollowSets000.FOLLOW_rule__JvmWildcardTypeReference__Group_2_1__0_in_rule__JvmWildcardTypeReference__Alternatives_213884);
rule__JvmWildcardTypeReference__Group_2_1__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getJvmWildcardTypeReferenceAccess().getGroup_2_1());
}
}
}
break;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__JvmWildcardTypeReference__Alternatives_2"
// $ANTLR start "rule__XImportDeclaration__Alternatives_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6354:1: rule__XImportDeclaration__Alternatives_1 : ( ( ( rule__XImportDeclaration__Group_1_0__0 ) ) | ( ( rule__XImportDeclaration__ImportedTypeAssignment_1_1 ) ) | ( ( rule__XImportDeclaration__ImportedNamespaceAssignment_1_2 ) ) );
public final void rule__XImportDeclaration__Alternatives_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6358:1: ( ( ( rule__XImportDeclaration__Group_1_0__0 ) ) | ( ( rule__XImportDeclaration__ImportedTypeAssignment_1_1 ) ) | ( ( rule__XImportDeclaration__ImportedNamespaceAssignment_1_2 ) ) )
int alt51=3;
alt51 = dfa51.predict(input);
switch (alt51) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6359:1: ( ( rule__XImportDeclaration__Group_1_0__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6359:1: ( ( rule__XImportDeclaration__Group_1_0__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6360:1: ( rule__XImportDeclaration__Group_1_0__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXImportDeclarationAccess().getGroup_1_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6361:1: ( rule__XImportDeclaration__Group_1_0__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6361:2: rule__XImportDeclaration__Group_1_0__0
{
pushFollow(FollowSets000.FOLLOW_rule__XImportDeclaration__Group_1_0__0_in_rule__XImportDeclaration__Alternatives_113917);
rule__XImportDeclaration__Group_1_0__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXImportDeclarationAccess().getGroup_1_0());
}
}
}
break;
case 2 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6365:6: ( ( rule__XImportDeclaration__ImportedTypeAssignment_1_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6365:6: ( ( rule__XImportDeclaration__ImportedTypeAssignment_1_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6366:1: ( rule__XImportDeclaration__ImportedTypeAssignment_1_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXImportDeclarationAccess().getImportedTypeAssignment_1_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6367:1: ( rule__XImportDeclaration__ImportedTypeAssignment_1_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6367:2: rule__XImportDeclaration__ImportedTypeAssignment_1_1
{
pushFollow(FollowSets000.FOLLOW_rule__XImportDeclaration__ImportedTypeAssignment_1_1_in_rule__XImportDeclaration__Alternatives_113935);
rule__XImportDeclaration__ImportedTypeAssignment_1_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXImportDeclarationAccess().getImportedTypeAssignment_1_1());
}
}
}
break;
case 3 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6371:6: ( ( rule__XImportDeclaration__ImportedNamespaceAssignment_1_2 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6371:6: ( ( rule__XImportDeclaration__ImportedNamespaceAssignment_1_2 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6372:1: ( rule__XImportDeclaration__ImportedNamespaceAssignment_1_2 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXImportDeclarationAccess().getImportedNamespaceAssignment_1_2());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6373:1: ( rule__XImportDeclaration__ImportedNamespaceAssignment_1_2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6373:2: rule__XImportDeclaration__ImportedNamespaceAssignment_1_2
{
pushFollow(FollowSets000.FOLLOW_rule__XImportDeclaration__ImportedNamespaceAssignment_1_2_in_rule__XImportDeclaration__Alternatives_113953);
rule__XImportDeclaration__ImportedNamespaceAssignment_1_2();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXImportDeclarationAccess().getImportedNamespaceAssignment_1_2());
}
}
}
break;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XImportDeclaration__Alternatives_1"
// $ANTLR start "rule__XImportDeclaration__Alternatives_1_0_3"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6382:1: rule__XImportDeclaration__Alternatives_1_0_3 : ( ( ( rule__XImportDeclaration__WildcardAssignment_1_0_3_0 ) ) | ( ( rule__XImportDeclaration__MemberNameAssignment_1_0_3_1 ) ) );
public final void rule__XImportDeclaration__Alternatives_1_0_3() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6386:1: ( ( ( rule__XImportDeclaration__WildcardAssignment_1_0_3_0 ) ) | ( ( rule__XImportDeclaration__MemberNameAssignment_1_0_3_1 ) ) )
int alt52=2;
int LA52_0 = input.LA(1);
if ( (LA52_0==36) ) {
alt52=1;
}
else if ( (LA52_0==RULE_ID) ) {
alt52=2;
}
else {
if (state.backtracking>0) {state.failed=true; return ;}
NoViableAltException nvae =
new NoViableAltException("", 52, 0, input);
throw nvae;
}
switch (alt52) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6387:1: ( ( rule__XImportDeclaration__WildcardAssignment_1_0_3_0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6387:1: ( ( rule__XImportDeclaration__WildcardAssignment_1_0_3_0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6388:1: ( rule__XImportDeclaration__WildcardAssignment_1_0_3_0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXImportDeclarationAccess().getWildcardAssignment_1_0_3_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6389:1: ( rule__XImportDeclaration__WildcardAssignment_1_0_3_0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6389:2: rule__XImportDeclaration__WildcardAssignment_1_0_3_0
{
pushFollow(FollowSets000.FOLLOW_rule__XImportDeclaration__WildcardAssignment_1_0_3_0_in_rule__XImportDeclaration__Alternatives_1_0_313986);
rule__XImportDeclaration__WildcardAssignment_1_0_3_0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXImportDeclarationAccess().getWildcardAssignment_1_0_3_0());
}
}
}
break;
case 2 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6393:6: ( ( rule__XImportDeclaration__MemberNameAssignment_1_0_3_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6393:6: ( ( rule__XImportDeclaration__MemberNameAssignment_1_0_3_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6394:1: ( rule__XImportDeclaration__MemberNameAssignment_1_0_3_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXImportDeclarationAccess().getMemberNameAssignment_1_0_3_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6395:1: ( rule__XImportDeclaration__MemberNameAssignment_1_0_3_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6395:2: rule__XImportDeclaration__MemberNameAssignment_1_0_3_1
{
pushFollow(FollowSets000.FOLLOW_rule__XImportDeclaration__MemberNameAssignment_1_0_3_1_in_rule__XImportDeclaration__Alternatives_1_0_314004);
rule__XImportDeclaration__MemberNameAssignment_1_0_3_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXImportDeclarationAccess().getMemberNameAssignment_1_0_3_1());
}
}
}
break;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XImportDeclaration__Alternatives_1_0_3"
// $ANTLR start "rule__IdGeneratorStrategy__Alternatives"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6404:1: rule__IdGeneratorStrategy__Alternatives : ( ( ( 'TABLE' ) ) | ( ( 'SEQUENCE' ) ) );
public final void rule__IdGeneratorStrategy__Alternatives() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6408:1: ( ( ( 'TABLE' ) ) | ( ( 'SEQUENCE' ) ) )
int alt53=2;
int LA53_0 = input.LA(1);
if ( (LA53_0==51) ) {
alt53=1;
}
else if ( (LA53_0==52) ) {
alt53=2;
}
else {
if (state.backtracking>0) {state.failed=true; return ;}
NoViableAltException nvae =
new NoViableAltException("", 53, 0, input);
throw nvae;
}
switch (alt53) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6409:1: ( ( 'TABLE' ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6409:1: ( ( 'TABLE' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6410:1: ( 'TABLE' )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getIdGeneratorStrategyAccess().getTABLEEnumLiteralDeclaration_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6411:1: ( 'TABLE' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6411:3: 'TABLE'
{
match(input,51,FollowSets000.FOLLOW_51_in_rule__IdGeneratorStrategy__Alternatives14038); if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getIdGeneratorStrategyAccess().getTABLEEnumLiteralDeclaration_0());
}
}
}
break;
case 2 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6416:6: ( ( 'SEQUENCE' ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6416:6: ( ( 'SEQUENCE' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6417:1: ( 'SEQUENCE' )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getIdGeneratorStrategyAccess().getSEQUENCEEnumLiteralDeclaration_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6418:1: ( 'SEQUENCE' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6418:3: 'SEQUENCE'
{
match(input,52,FollowSets000.FOLLOW_52_in_rule__IdGeneratorStrategy__Alternatives14059); if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getIdGeneratorStrategyAccess().getSEQUENCEEnumLiteralDeclaration_1());
}
}
}
break;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__IdGeneratorStrategy__Alternatives"
// $ANTLR start "rule__Cardinality__Alternatives"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6428:1: rule__Cardinality__Alternatives : ( ( ( '0..1' ) ) | ( ( '0..n' ) ) | ( ( 'n..n' ) ) );
public final void rule__Cardinality__Alternatives() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6432:1: ( ( ( '0..1' ) ) | ( ( '0..n' ) ) | ( ( 'n..n' ) ) )
int alt54=3;
switch ( input.LA(1) ) {
case 53:
{
alt54=1;
}
break;
case 54:
{
alt54=2;
}
break;
case 55:
{
alt54=3;
}
break;
default:
if (state.backtracking>0) {state.failed=true; return ;}
NoViableAltException nvae =
new NoViableAltException("", 54, 0, input);
throw nvae;
}
switch (alt54) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6433:1: ( ( '0..1' ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6433:1: ( ( '0..1' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6434:1: ( '0..1' )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getCardinalityAccess().getOnetooneEnumLiteralDeclaration_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6435:1: ( '0..1' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6435:3: '0..1'
{
match(input,53,FollowSets000.FOLLOW_53_in_rule__Cardinality__Alternatives14095); if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getCardinalityAccess().getOnetooneEnumLiteralDeclaration_0());
}
}
}
break;
case 2 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6440:6: ( ( '0..n' ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6440:6: ( ( '0..n' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6441:1: ( '0..n' )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getCardinalityAccess().getOnetomanyEnumLiteralDeclaration_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6442:1: ( '0..n' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6442:3: '0..n'
{
match(input,54,FollowSets000.FOLLOW_54_in_rule__Cardinality__Alternatives14116); if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getCardinalityAccess().getOnetomanyEnumLiteralDeclaration_1());
}
}
}
break;
case 3 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6447:6: ( ( 'n..n' ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6447:6: ( ( 'n..n' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6448:1: ( 'n..n' )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getCardinalityAccess().getManytomanyEnumLiteralDeclaration_2());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6449:1: ( 'n..n' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6449:3: 'n..n'
{
match(input,55,FollowSets000.FOLLOW_55_in_rule__Cardinality__Alternatives14137); if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getCardinalityAccess().getManytomanyEnumLiteralDeclaration_2());
}
}
}
break;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Cardinality__Alternatives"
// $ANTLR start "rule__SimpleTypes__Alternatives"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6459:1: rule__SimpleTypes__Alternatives : ( ( ( 'long' ) ) | ( ( 'integer' ) ) | ( ( 'bigdecimal' ) ) | ( ( 'string' ) ) | ( ( 'boolean' ) ) | ( ( 'reference' ) ) );
public final void rule__SimpleTypes__Alternatives() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6463:1: ( ( ( 'long' ) ) | ( ( 'integer' ) ) | ( ( 'bigdecimal' ) ) | ( ( 'string' ) ) | ( ( 'boolean' ) ) | ( ( 'reference' ) ) )
int alt55=6;
switch ( input.LA(1) ) {
case 56:
{
alt55=1;
}
break;
case 57:
{
alt55=2;
}
break;
case 58:
{
alt55=3;
}
break;
case 59:
{
alt55=4;
}
break;
case 60:
{
alt55=5;
}
break;
case 61:
{
alt55=6;
}
break;
default:
if (state.backtracking>0) {state.failed=true; return ;}
NoViableAltException nvae =
new NoViableAltException("", 55, 0, input);
throw nvae;
}
switch (alt55) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6464:1: ( ( 'long' ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6464:1: ( ( 'long' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6465:1: ( 'long' )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getSimpleTypesAccess().getLongEnumLiteralDeclaration_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6466:1: ( 'long' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6466:3: 'long'
{
match(input,56,FollowSets000.FOLLOW_56_in_rule__SimpleTypes__Alternatives14173); if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getSimpleTypesAccess().getLongEnumLiteralDeclaration_0());
}
}
}
break;
case 2 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6471:6: ( ( 'integer' ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6471:6: ( ( 'integer' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6472:1: ( 'integer' )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getSimpleTypesAccess().getIntegerEnumLiteralDeclaration_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6473:1: ( 'integer' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6473:3: 'integer'
{
match(input,57,FollowSets000.FOLLOW_57_in_rule__SimpleTypes__Alternatives14194); if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getSimpleTypesAccess().getIntegerEnumLiteralDeclaration_1());
}
}
}
break;
case 3 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6478:6: ( ( 'bigdecimal' ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6478:6: ( ( 'bigdecimal' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6479:1: ( 'bigdecimal' )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getSimpleTypesAccess().getBigdecimalEnumLiteralDeclaration_2());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6480:1: ( 'bigdecimal' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6480:3: 'bigdecimal'
{
match(input,58,FollowSets000.FOLLOW_58_in_rule__SimpleTypes__Alternatives14215); if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getSimpleTypesAccess().getBigdecimalEnumLiteralDeclaration_2());
}
}
}
break;
case 4 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6485:6: ( ( 'string' ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6485:6: ( ( 'string' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6486:1: ( 'string' )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getSimpleTypesAccess().getStringEnumLiteralDeclaration_3());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6487:1: ( 'string' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6487:3: 'string'
{
match(input,59,FollowSets000.FOLLOW_59_in_rule__SimpleTypes__Alternatives14236); if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getSimpleTypesAccess().getStringEnumLiteralDeclaration_3());
}
}
}
break;
case 5 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6492:6: ( ( 'boolean' ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6492:6: ( ( 'boolean' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6493:1: ( 'boolean' )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getSimpleTypesAccess().getBooleanEnumLiteralDeclaration_4());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6494:1: ( 'boolean' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6494:3: 'boolean'
{
match(input,60,FollowSets000.FOLLOW_60_in_rule__SimpleTypes__Alternatives14257); if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getSimpleTypesAccess().getBooleanEnumLiteralDeclaration_4());
}
}
}
break;
case 6 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6499:6: ( ( 'reference' ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6499:6: ( ( 'reference' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6500:1: ( 'reference' )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getSimpleTypesAccess().getReferenceEnumLiteralDeclaration_5());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6501:1: ( 'reference' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6501:3: 'reference'
{
match(input,61,FollowSets000.FOLLOW_61_in_rule__SimpleTypes__Alternatives14278); if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getSimpleTypesAccess().getReferenceEnumLiteralDeclaration_5());
}
}
}
break;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__SimpleTypes__Alternatives"
// $ANTLR start "rule__IntegerControlInputType__Alternatives"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6511:1: rule__IntegerControlInputType__Alternatives : ( ( ( 'textbox' ) ) | ( ( 'rating' ) ) );
public final void rule__IntegerControlInputType__Alternatives() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6515:1: ( ( ( 'textbox' ) ) | ( ( 'rating' ) ) )
int alt56=2;
int LA56_0 = input.LA(1);
if ( (LA56_0==62) ) {
alt56=1;
}
else if ( (LA56_0==63) ) {
alt56=2;
}
else {
if (state.backtracking>0) {state.failed=true; return ;}
NoViableAltException nvae =
new NoViableAltException("", 56, 0, input);
throw nvae;
}
switch (alt56) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6516:1: ( ( 'textbox' ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6516:1: ( ( 'textbox' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6517:1: ( 'textbox' )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getIntegerControlInputTypeAccess().getTextEnumLiteralDeclaration_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6518:1: ( 'textbox' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6518:3: 'textbox'
{
match(input,62,FollowSets000.FOLLOW_62_in_rule__IntegerControlInputType__Alternatives14314); if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getIntegerControlInputTypeAccess().getTextEnumLiteralDeclaration_0());
}
}
}
break;
case 2 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6523:6: ( ( 'rating' ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6523:6: ( ( 'rating' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6524:1: ( 'rating' )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getIntegerControlInputTypeAccess().getRatingEnumLiteralDeclaration_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6525:1: ( 'rating' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6525:3: 'rating'
{
match(input,63,FollowSets000.FOLLOW_63_in_rule__IntegerControlInputType__Alternatives14335); if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getIntegerControlInputTypeAccess().getRatingEnumLiteralDeclaration_1());
}
}
}
break;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__IntegerControlInputType__Alternatives"
// $ANTLR start "rule__ReferenceControlType__Alternatives"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6535:1: rule__ReferenceControlType__Alternatives : ( ( ( 'text' ) ) | ( ( 'dropdown' ) ) );
public final void rule__ReferenceControlType__Alternatives() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6539:1: ( ( ( 'text' ) ) | ( ( 'dropdown' ) ) )
int alt57=2;
int LA57_0 = input.LA(1);
if ( (LA57_0==64) ) {
alt57=1;
}
else if ( (LA57_0==65) ) {
alt57=2;
}
else {
if (state.backtracking>0) {state.failed=true; return ;}
NoViableAltException nvae =
new NoViableAltException("", 57, 0, input);
throw nvae;
}
switch (alt57) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6540:1: ( ( 'text' ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6540:1: ( ( 'text' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6541:1: ( 'text' )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getReferenceControlTypeAccess().getTextEnumLiteralDeclaration_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6542:1: ( 'text' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6542:3: 'text'
{
match(input,64,FollowSets000.FOLLOW_64_in_rule__ReferenceControlType__Alternatives14371); if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getReferenceControlTypeAccess().getTextEnumLiteralDeclaration_0());
}
}
}
break;
case 2 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6547:6: ( ( 'dropdown' ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6547:6: ( ( 'dropdown' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6548:1: ( 'dropdown' )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getReferenceControlTypeAccess().getDropdownEnumLiteralDeclaration_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6549:1: ( 'dropdown' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6549:3: 'dropdown'
{
match(input,65,FollowSets000.FOLLOW_65_in_rule__ReferenceControlType__Alternatives14392); if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getReferenceControlTypeAccess().getDropdownEnumLiteralDeclaration_1());
}
}
}
break;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ReferenceControlType__Alternatives"
// $ANTLR start "rule__ModelRoot__Group_0__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6561:1: rule__ModelRoot__Group_0__0 : rule__ModelRoot__Group_0__0__Impl rule__ModelRoot__Group_0__1 ;
public final void rule__ModelRoot__Group_0__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6565:1: ( rule__ModelRoot__Group_0__0__Impl rule__ModelRoot__Group_0__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6566:2: rule__ModelRoot__Group_0__0__Impl rule__ModelRoot__Group_0__1
{
pushFollow(FollowSets000.FOLLOW_rule__ModelRoot__Group_0__0__Impl_in_rule__ModelRoot__Group_0__014425);
rule__ModelRoot__Group_0__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets000.FOLLOW_rule__ModelRoot__Group_0__1_in_rule__ModelRoot__Group_0__014428);
rule__ModelRoot__Group_0__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ModelRoot__Group_0__0"
// $ANTLR start "rule__ModelRoot__Group_0__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6573:1: rule__ModelRoot__Group_0__0__Impl : ( ( rule__ModelRoot__ImportSectionAssignment_0_0 )? ) ;
public final void rule__ModelRoot__Group_0__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6577:1: ( ( ( rule__ModelRoot__ImportSectionAssignment_0_0 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6578:1: ( ( rule__ModelRoot__ImportSectionAssignment_0_0 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6578:1: ( ( rule__ModelRoot__ImportSectionAssignment_0_0 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6579:1: ( rule__ModelRoot__ImportSectionAssignment_0_0 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getModelRootAccess().getImportSectionAssignment_0_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6580:1: ( rule__ModelRoot__ImportSectionAssignment_0_0 )?
int alt58=2;
int LA58_0 = input.LA(1);
if ( (LA58_0==47) ) {
alt58=1;
}
switch (alt58) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6580:2: rule__ModelRoot__ImportSectionAssignment_0_0
{
pushFollow(FollowSets000.FOLLOW_rule__ModelRoot__ImportSectionAssignment_0_0_in_rule__ModelRoot__Group_0__0__Impl14455);
rule__ModelRoot__ImportSectionAssignment_0_0();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getModelRootAccess().getImportSectionAssignment_0_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ModelRoot__Group_0__0__Impl"
// $ANTLR start "rule__ModelRoot__Group_0__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6590:1: rule__ModelRoot__Group_0__1 : rule__ModelRoot__Group_0__1__Impl ;
public final void rule__ModelRoot__Group_0__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6594:1: ( rule__ModelRoot__Group_0__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6595:2: rule__ModelRoot__Group_0__1__Impl
{
pushFollow(FollowSets000.FOLLOW_rule__ModelRoot__Group_0__1__Impl_in_rule__ModelRoot__Group_0__114486);
rule__ModelRoot__Group_0__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ModelRoot__Group_0__1"
// $ANTLR start "rule__ModelRoot__Group_0__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6601:1: rule__ModelRoot__Group_0__1__Impl : ( ( rule__ModelRoot__ModelRootAssignment_0_1 ) ) ;
public final void rule__ModelRoot__Group_0__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6605:1: ( ( ( rule__ModelRoot__ModelRootAssignment_0_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6606:1: ( ( rule__ModelRoot__ModelRootAssignment_0_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6606:1: ( ( rule__ModelRoot__ModelRootAssignment_0_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6607:1: ( rule__ModelRoot__ModelRootAssignment_0_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getModelRootAccess().getModelRootAssignment_0_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6608:1: ( rule__ModelRoot__ModelRootAssignment_0_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6608:2: rule__ModelRoot__ModelRootAssignment_0_1
{
pushFollow(FollowSets000.FOLLOW_rule__ModelRoot__ModelRootAssignment_0_1_in_rule__ModelRoot__Group_0__1__Impl14513);
rule__ModelRoot__ModelRootAssignment_0_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getModelRootAccess().getModelRootAssignment_0_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ModelRoot__Group_0__1__Impl"
// $ANTLR start "rule__Model__Group__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6622:1: rule__Model__Group__0 : rule__Model__Group__0__Impl rule__Model__Group__1 ;
public final void rule__Model__Group__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6626:1: ( rule__Model__Group__0__Impl rule__Model__Group__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6627:2: rule__Model__Group__0__Impl rule__Model__Group__1
{
pushFollow(FollowSets000.FOLLOW_rule__Model__Group__0__Impl_in_rule__Model__Group__014547);
rule__Model__Group__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets000.FOLLOW_rule__Model__Group__1_in_rule__Model__Group__014550);
rule__Model__Group__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Model__Group__0"
// $ANTLR start "rule__Model__Group__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6634:1: rule__Model__Group__0__Impl : ( 'project' ) ;
public final void rule__Model__Group__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6638:1: ( ( 'project' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6639:1: ( 'project' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6639:1: ( 'project' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6640:1: 'project'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getModelAccess().getProjectKeyword_0());
}
match(input,66,FollowSets000.FOLLOW_66_in_rule__Model__Group__0__Impl14578); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getModelAccess().getProjectKeyword_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Model__Group__0__Impl"
// $ANTLR start "rule__Model__Group__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6653:1: rule__Model__Group__1 : rule__Model__Group__1__Impl rule__Model__Group__2 ;
public final void rule__Model__Group__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6657:1: ( rule__Model__Group__1__Impl rule__Model__Group__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6658:2: rule__Model__Group__1__Impl rule__Model__Group__2
{
pushFollow(FollowSets000.FOLLOW_rule__Model__Group__1__Impl_in_rule__Model__Group__114609);
rule__Model__Group__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets000.FOLLOW_rule__Model__Group__2_in_rule__Model__Group__114612);
rule__Model__Group__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Model__Group__1"
// $ANTLR start "rule__Model__Group__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6665:1: rule__Model__Group__1__Impl : ( ( rule__Model__ModelNameAssignment_1 ) ) ;
public final void rule__Model__Group__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6669:1: ( ( ( rule__Model__ModelNameAssignment_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6670:1: ( ( rule__Model__ModelNameAssignment_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6670:1: ( ( rule__Model__ModelNameAssignment_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6671:1: ( rule__Model__ModelNameAssignment_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getModelAccess().getModelNameAssignment_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6672:1: ( rule__Model__ModelNameAssignment_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6672:2: rule__Model__ModelNameAssignment_1
{
pushFollow(FollowSets000.FOLLOW_rule__Model__ModelNameAssignment_1_in_rule__Model__Group__1__Impl14639);
rule__Model__ModelNameAssignment_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getModelAccess().getModelNameAssignment_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Model__Group__1__Impl"
// $ANTLR start "rule__Model__Group__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6682:1: rule__Model__Group__2 : rule__Model__Group__2__Impl rule__Model__Group__3 ;
public final void rule__Model__Group__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6686:1: ( rule__Model__Group__2__Impl rule__Model__Group__3 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6687:2: rule__Model__Group__2__Impl rule__Model__Group__3
{
pushFollow(FollowSets000.FOLLOW_rule__Model__Group__2__Impl_in_rule__Model__Group__214669);
rule__Model__Group__2__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets000.FOLLOW_rule__Model__Group__3_in_rule__Model__Group__214672);
rule__Model__Group__3();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Model__Group__2"
// $ANTLR start "rule__Model__Group__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6694:1: rule__Model__Group__2__Impl : ( '{' ) ;
public final void rule__Model__Group__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6698:1: ( ( '{' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6699:1: ( '{' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6699:1: ( '{' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6700:1: '{'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getModelAccess().getLeftCurlyBracketKeyword_2());
}
match(input,67,FollowSets000.FOLLOW_67_in_rule__Model__Group__2__Impl14700); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getModelAccess().getLeftCurlyBracketKeyword_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Model__Group__2__Impl"
// $ANTLR start "rule__Model__Group__3"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6713:1: rule__Model__Group__3 : rule__Model__Group__3__Impl rule__Model__Group__4 ;
public final void rule__Model__Group__3() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6717:1: ( rule__Model__Group__3__Impl rule__Model__Group__4 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6718:2: rule__Model__Group__3__Impl rule__Model__Group__4
{
pushFollow(FollowSets000.FOLLOW_rule__Model__Group__3__Impl_in_rule__Model__Group__314731);
rule__Model__Group__3__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets000.FOLLOW_rule__Model__Group__4_in_rule__Model__Group__314734);
rule__Model__Group__4();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Model__Group__3"
// $ANTLR start "rule__Model__Group__3__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6725:1: rule__Model__Group__3__Impl : ( ( rule__Model__ElementsAssignment_3 )* ) ;
public final void rule__Model__Group__3__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6729:1: ( ( ( rule__Model__ElementsAssignment_3 )* ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6730:1: ( ( rule__Model__ElementsAssignment_3 )* )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6730:1: ( ( rule__Model__ElementsAssignment_3 )* )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6731:1: ( rule__Model__ElementsAssignment_3 )*
{
if ( state.backtracking==0 ) {
before(grammarAccess.getModelAccess().getElementsAssignment_3());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6732:1: ( rule__Model__ElementsAssignment_3 )*
loop59:
do {
int alt59=2;
int LA59_0 = input.LA(1);
if ( (LA59_0==69) ) {
alt59=1;
}
switch (alt59) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6732:2: rule__Model__ElementsAssignment_3
{
pushFollow(FollowSets000.FOLLOW_rule__Model__ElementsAssignment_3_in_rule__Model__Group__3__Impl14761);
rule__Model__ElementsAssignment_3();
state._fsp--;
if (state.failed) return ;
}
break;
default :
break loop59;
}
} while (true);
if ( state.backtracking==0 ) {
after(grammarAccess.getModelAccess().getElementsAssignment_3());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Model__Group__3__Impl"
// $ANTLR start "rule__Model__Group__4"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6742:1: rule__Model__Group__4 : rule__Model__Group__4__Impl ;
public final void rule__Model__Group__4() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6746:1: ( rule__Model__Group__4__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6747:2: rule__Model__Group__4__Impl
{
pushFollow(FollowSets000.FOLLOW_rule__Model__Group__4__Impl_in_rule__Model__Group__414792);
rule__Model__Group__4__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Model__Group__4"
// $ANTLR start "rule__Model__Group__4__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6753:1: rule__Model__Group__4__Impl : ( '}' ) ;
public final void rule__Model__Group__4__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6757:1: ( ( '}' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6758:1: ( '}' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6758:1: ( '}' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6759:1: '}'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getModelAccess().getRightCurlyBracketKeyword_4());
}
match(input,68,FollowSets000.FOLLOW_68_in_rule__Model__Group__4__Impl14820); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getModelAccess().getRightCurlyBracketKeyword_4());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Model__Group__4__Impl"
// $ANTLR start "rule__PackageDeclaration__Group__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6782:1: rule__PackageDeclaration__Group__0 : rule__PackageDeclaration__Group__0__Impl rule__PackageDeclaration__Group__1 ;
public final void rule__PackageDeclaration__Group__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6786:1: ( rule__PackageDeclaration__Group__0__Impl rule__PackageDeclaration__Group__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6787:2: rule__PackageDeclaration__Group__0__Impl rule__PackageDeclaration__Group__1
{
pushFollow(FollowSets000.FOLLOW_rule__PackageDeclaration__Group__0__Impl_in_rule__PackageDeclaration__Group__014861);
rule__PackageDeclaration__Group__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets000.FOLLOW_rule__PackageDeclaration__Group__1_in_rule__PackageDeclaration__Group__014864);
rule__PackageDeclaration__Group__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__PackageDeclaration__Group__0"
// $ANTLR start "rule__PackageDeclaration__Group__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6794:1: rule__PackageDeclaration__Group__0__Impl : ( 'package' ) ;
public final void rule__PackageDeclaration__Group__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6798:1: ( ( 'package' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6799:1: ( 'package' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6799:1: ( 'package' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6800:1: 'package'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getPackageDeclarationAccess().getPackageKeyword_0());
}
match(input,69,FollowSets000.FOLLOW_69_in_rule__PackageDeclaration__Group__0__Impl14892); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getPackageDeclarationAccess().getPackageKeyword_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__PackageDeclaration__Group__0__Impl"
// $ANTLR start "rule__PackageDeclaration__Group__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6813:1: rule__PackageDeclaration__Group__1 : rule__PackageDeclaration__Group__1__Impl rule__PackageDeclaration__Group__2 ;
public final void rule__PackageDeclaration__Group__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6817:1: ( rule__PackageDeclaration__Group__1__Impl rule__PackageDeclaration__Group__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6818:2: rule__PackageDeclaration__Group__1__Impl rule__PackageDeclaration__Group__2
{
pushFollow(FollowSets000.FOLLOW_rule__PackageDeclaration__Group__1__Impl_in_rule__PackageDeclaration__Group__114923);
rule__PackageDeclaration__Group__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets000.FOLLOW_rule__PackageDeclaration__Group__2_in_rule__PackageDeclaration__Group__114926);
rule__PackageDeclaration__Group__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__PackageDeclaration__Group__1"
// $ANTLR start "rule__PackageDeclaration__Group__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6825:1: rule__PackageDeclaration__Group__1__Impl : ( ( rule__PackageDeclaration__PackageNameAssignment_1 ) ) ;
public final void rule__PackageDeclaration__Group__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6829:1: ( ( ( rule__PackageDeclaration__PackageNameAssignment_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6830:1: ( ( rule__PackageDeclaration__PackageNameAssignment_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6830:1: ( ( rule__PackageDeclaration__PackageNameAssignment_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6831:1: ( rule__PackageDeclaration__PackageNameAssignment_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getPackageDeclarationAccess().getPackageNameAssignment_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6832:1: ( rule__PackageDeclaration__PackageNameAssignment_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6832:2: rule__PackageDeclaration__PackageNameAssignment_1
{
pushFollow(FollowSets000.FOLLOW_rule__PackageDeclaration__PackageNameAssignment_1_in_rule__PackageDeclaration__Group__1__Impl14953);
rule__PackageDeclaration__PackageNameAssignment_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getPackageDeclarationAccess().getPackageNameAssignment_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__PackageDeclaration__Group__1__Impl"
// $ANTLR start "rule__PackageDeclaration__Group__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6842:1: rule__PackageDeclaration__Group__2 : rule__PackageDeclaration__Group__2__Impl rule__PackageDeclaration__Group__3 ;
public final void rule__PackageDeclaration__Group__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6846:1: ( rule__PackageDeclaration__Group__2__Impl rule__PackageDeclaration__Group__3 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6847:2: rule__PackageDeclaration__Group__2__Impl rule__PackageDeclaration__Group__3
{
pushFollow(FollowSets000.FOLLOW_rule__PackageDeclaration__Group__2__Impl_in_rule__PackageDeclaration__Group__214983);
rule__PackageDeclaration__Group__2__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets000.FOLLOW_rule__PackageDeclaration__Group__3_in_rule__PackageDeclaration__Group__214986);
rule__PackageDeclaration__Group__3();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__PackageDeclaration__Group__2"
// $ANTLR start "rule__PackageDeclaration__Group__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6854:1: rule__PackageDeclaration__Group__2__Impl : ( '{' ) ;
public final void rule__PackageDeclaration__Group__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6858:1: ( ( '{' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6859:1: ( '{' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6859:1: ( '{' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6860:1: '{'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getPackageDeclarationAccess().getLeftCurlyBracketKeyword_2());
}
match(input,67,FollowSets000.FOLLOW_67_in_rule__PackageDeclaration__Group__2__Impl15014); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getPackageDeclarationAccess().getLeftCurlyBracketKeyword_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__PackageDeclaration__Group__2__Impl"
// $ANTLR start "rule__PackageDeclaration__Group__3"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6873:1: rule__PackageDeclaration__Group__3 : rule__PackageDeclaration__Group__3__Impl rule__PackageDeclaration__Group__4 ;
public final void rule__PackageDeclaration__Group__3() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6877:1: ( rule__PackageDeclaration__Group__3__Impl rule__PackageDeclaration__Group__4 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6878:2: rule__PackageDeclaration__Group__3__Impl rule__PackageDeclaration__Group__4
{
pushFollow(FollowSets000.FOLLOW_rule__PackageDeclaration__Group__3__Impl_in_rule__PackageDeclaration__Group__315045);
rule__PackageDeclaration__Group__3__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets000.FOLLOW_rule__PackageDeclaration__Group__4_in_rule__PackageDeclaration__Group__315048);
rule__PackageDeclaration__Group__4();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__PackageDeclaration__Group__3"
// $ANTLR start "rule__PackageDeclaration__Group__3__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6885:1: rule__PackageDeclaration__Group__3__Impl : ( ( rule__PackageDeclaration__ElementsAssignment_3 )* ) ;
public final void rule__PackageDeclaration__Group__3__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6889:1: ( ( ( rule__PackageDeclaration__ElementsAssignment_3 )* ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6890:1: ( ( rule__PackageDeclaration__ElementsAssignment_3 )* )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6890:1: ( ( rule__PackageDeclaration__ElementsAssignment_3 )* )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6891:1: ( rule__PackageDeclaration__ElementsAssignment_3 )*
{
if ( state.backtracking==0 ) {
before(grammarAccess.getPackageDeclarationAccess().getElementsAssignment_3());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6892:1: ( rule__PackageDeclaration__ElementsAssignment_3 )*
loop60:
do {
int alt60=2;
int LA60_0 = input.LA(1);
if ( ((LA60_0>=69 && LA60_0<=70)||LA60_0==78||LA60_0==80||LA60_0==82||(LA60_0>=86 && LA60_0<=88)||LA60_0==90||(LA60_0>=92 && LA60_0<=93)||LA60_0==95||LA60_0==97||(LA60_0>=99 && LA60_0<=101)||(LA60_0>=139 && LA60_0<=140)||LA60_0==146||LA60_0==148) ) {
alt60=1;
}
switch (alt60) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6892:2: rule__PackageDeclaration__ElementsAssignment_3
{
pushFollow(FollowSets000.FOLLOW_rule__PackageDeclaration__ElementsAssignment_3_in_rule__PackageDeclaration__Group__3__Impl15075);
rule__PackageDeclaration__ElementsAssignment_3();
state._fsp--;
if (state.failed) return ;
}
break;
default :
break loop60;
}
} while (true);
if ( state.backtracking==0 ) {
after(grammarAccess.getPackageDeclarationAccess().getElementsAssignment_3());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__PackageDeclaration__Group__3__Impl"
// $ANTLR start "rule__PackageDeclaration__Group__4"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6902:1: rule__PackageDeclaration__Group__4 : rule__PackageDeclaration__Group__4__Impl ;
public final void rule__PackageDeclaration__Group__4() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6906:1: ( rule__PackageDeclaration__Group__4__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6907:2: rule__PackageDeclaration__Group__4__Impl
{
pushFollow(FollowSets000.FOLLOW_rule__PackageDeclaration__Group__4__Impl_in_rule__PackageDeclaration__Group__415106);
rule__PackageDeclaration__Group__4__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__PackageDeclaration__Group__4"
// $ANTLR start "rule__PackageDeclaration__Group__4__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6913:1: rule__PackageDeclaration__Group__4__Impl : ( '}' ) ;
public final void rule__PackageDeclaration__Group__4__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6917:1: ( ( '}' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6918:1: ( '}' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6918:1: ( '}' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6919:1: '}'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getPackageDeclarationAccess().getRightCurlyBracketKeyword_4());
}
match(input,68,FollowSets000.FOLLOW_68_in_rule__PackageDeclaration__Group__4__Impl15134); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getPackageDeclarationAccess().getRightCurlyBracketKeyword_4());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__PackageDeclaration__Group__4__Impl"
// $ANTLR start "rule__Enumeration__Group__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6942:1: rule__Enumeration__Group__0 : rule__Enumeration__Group__0__Impl rule__Enumeration__Group__1 ;
public final void rule__Enumeration__Group__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6946:1: ( rule__Enumeration__Group__0__Impl rule__Enumeration__Group__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6947:2: rule__Enumeration__Group__0__Impl rule__Enumeration__Group__1
{
pushFollow(FollowSets000.FOLLOW_rule__Enumeration__Group__0__Impl_in_rule__Enumeration__Group__015175);
rule__Enumeration__Group__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets000.FOLLOW_rule__Enumeration__Group__1_in_rule__Enumeration__Group__015178);
rule__Enumeration__Group__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Enumeration__Group__0"
// $ANTLR start "rule__Enumeration__Group__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6954:1: rule__Enumeration__Group__0__Impl : ( 'enumeration' ) ;
public final void rule__Enumeration__Group__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6958:1: ( ( 'enumeration' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6959:1: ( 'enumeration' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6959:1: ( 'enumeration' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6960:1: 'enumeration'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEnumerationAccess().getEnumerationKeyword_0());
}
match(input,70,FollowSets000.FOLLOW_70_in_rule__Enumeration__Group__0__Impl15206); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getEnumerationAccess().getEnumerationKeyword_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Enumeration__Group__0__Impl"
// $ANTLR start "rule__Enumeration__Group__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6973:1: rule__Enumeration__Group__1 : rule__Enumeration__Group__1__Impl rule__Enumeration__Group__2 ;
public final void rule__Enumeration__Group__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6977:1: ( rule__Enumeration__Group__1__Impl rule__Enumeration__Group__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6978:2: rule__Enumeration__Group__1__Impl rule__Enumeration__Group__2
{
pushFollow(FollowSets000.FOLLOW_rule__Enumeration__Group__1__Impl_in_rule__Enumeration__Group__115237);
rule__Enumeration__Group__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets000.FOLLOW_rule__Enumeration__Group__2_in_rule__Enumeration__Group__115240);
rule__Enumeration__Group__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Enumeration__Group__1"
// $ANTLR start "rule__Enumeration__Group__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6985:1: rule__Enumeration__Group__1__Impl : ( ( rule__Enumeration__NameAssignment_1 ) ) ;
public final void rule__Enumeration__Group__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6989:1: ( ( ( rule__Enumeration__NameAssignment_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6990:1: ( ( rule__Enumeration__NameAssignment_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6990:1: ( ( rule__Enumeration__NameAssignment_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6991:1: ( rule__Enumeration__NameAssignment_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEnumerationAccess().getNameAssignment_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6992:1: ( rule__Enumeration__NameAssignment_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6992:2: rule__Enumeration__NameAssignment_1
{
pushFollow(FollowSets000.FOLLOW_rule__Enumeration__NameAssignment_1_in_rule__Enumeration__Group__1__Impl15267);
rule__Enumeration__NameAssignment_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getEnumerationAccess().getNameAssignment_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Enumeration__Group__1__Impl"
// $ANTLR start "rule__Enumeration__Group__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7002:1: rule__Enumeration__Group__2 : rule__Enumeration__Group__2__Impl rule__Enumeration__Group__3 ;
public final void rule__Enumeration__Group__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7006:1: ( rule__Enumeration__Group__2__Impl rule__Enumeration__Group__3 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7007:2: rule__Enumeration__Group__2__Impl rule__Enumeration__Group__3
{
pushFollow(FollowSets000.FOLLOW_rule__Enumeration__Group__2__Impl_in_rule__Enumeration__Group__215297);
rule__Enumeration__Group__2__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets000.FOLLOW_rule__Enumeration__Group__3_in_rule__Enumeration__Group__215300);
rule__Enumeration__Group__3();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Enumeration__Group__2"
// $ANTLR start "rule__Enumeration__Group__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7014:1: rule__Enumeration__Group__2__Impl : ( '{' ) ;
public final void rule__Enumeration__Group__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7018:1: ( ( '{' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7019:1: ( '{' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7019:1: ( '{' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7020:1: '{'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEnumerationAccess().getLeftCurlyBracketKeyword_2());
}
match(input,67,FollowSets000.FOLLOW_67_in_rule__Enumeration__Group__2__Impl15328); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getEnumerationAccess().getLeftCurlyBracketKeyword_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Enumeration__Group__2__Impl"
// $ANTLR start "rule__Enumeration__Group__3"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7033:1: rule__Enumeration__Group__3 : rule__Enumeration__Group__3__Impl rule__Enumeration__Group__4 ;
public final void rule__Enumeration__Group__3() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7037:1: ( rule__Enumeration__Group__3__Impl rule__Enumeration__Group__4 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7038:2: rule__Enumeration__Group__3__Impl rule__Enumeration__Group__4
{
pushFollow(FollowSets000.FOLLOW_rule__Enumeration__Group__3__Impl_in_rule__Enumeration__Group__315359);
rule__Enumeration__Group__3__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets000.FOLLOW_rule__Enumeration__Group__4_in_rule__Enumeration__Group__315362);
rule__Enumeration__Group__4();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Enumeration__Group__3"
// $ANTLR start "rule__Enumeration__Group__3__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7045:1: rule__Enumeration__Group__3__Impl : ( ( rule__Enumeration__EnumerationValuesAssignment_3 )* ) ;
public final void rule__Enumeration__Group__3__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7049:1: ( ( ( rule__Enumeration__EnumerationValuesAssignment_3 )* ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7050:1: ( ( rule__Enumeration__EnumerationValuesAssignment_3 )* )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7050:1: ( ( rule__Enumeration__EnumerationValuesAssignment_3 )* )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7051:1: ( rule__Enumeration__EnumerationValuesAssignment_3 )*
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEnumerationAccess().getEnumerationValuesAssignment_3());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7052:1: ( rule__Enumeration__EnumerationValuesAssignment_3 )*
loop61:
do {
int alt61=2;
int LA61_0 = input.LA(1);
if ( (LA61_0==RULE_ID) ) {
alt61=1;
}
switch (alt61) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7052:2: rule__Enumeration__EnumerationValuesAssignment_3
{
pushFollow(FollowSets000.FOLLOW_rule__Enumeration__EnumerationValuesAssignment_3_in_rule__Enumeration__Group__3__Impl15389);
rule__Enumeration__EnumerationValuesAssignment_3();
state._fsp--;
if (state.failed) return ;
}
break;
default :
break loop61;
}
} while (true);
if ( state.backtracking==0 ) {
after(grammarAccess.getEnumerationAccess().getEnumerationValuesAssignment_3());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Enumeration__Group__3__Impl"
// $ANTLR start "rule__Enumeration__Group__4"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7062:1: rule__Enumeration__Group__4 : rule__Enumeration__Group__4__Impl ;
public final void rule__Enumeration__Group__4() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7066:1: ( rule__Enumeration__Group__4__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7067:2: rule__Enumeration__Group__4__Impl
{
pushFollow(FollowSets000.FOLLOW_rule__Enumeration__Group__4__Impl_in_rule__Enumeration__Group__415420);
rule__Enumeration__Group__4__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Enumeration__Group__4"
// $ANTLR start "rule__Enumeration__Group__4__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7073:1: rule__Enumeration__Group__4__Impl : ( '}' ) ;
public final void rule__Enumeration__Group__4__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7077:1: ( ( '}' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7078:1: ( '}' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7078:1: ( '}' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7079:1: '}'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEnumerationAccess().getRightCurlyBracketKeyword_4());
}
match(input,68,FollowSets000.FOLLOW_68_in_rule__Enumeration__Group__4__Impl15448); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getEnumerationAccess().getRightCurlyBracketKeyword_4());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Enumeration__Group__4__Impl"
// $ANTLR start "rule__EnumerationValue__Group__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7102:1: rule__EnumerationValue__Group__0 : rule__EnumerationValue__Group__0__Impl rule__EnumerationValue__Group__1 ;
public final void rule__EnumerationValue__Group__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7106:1: ( rule__EnumerationValue__Group__0__Impl rule__EnumerationValue__Group__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7107:2: rule__EnumerationValue__Group__0__Impl rule__EnumerationValue__Group__1
{
pushFollow(FollowSets000.FOLLOW_rule__EnumerationValue__Group__0__Impl_in_rule__EnumerationValue__Group__015489);
rule__EnumerationValue__Group__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets000.FOLLOW_rule__EnumerationValue__Group__1_in_rule__EnumerationValue__Group__015492);
rule__EnumerationValue__Group__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EnumerationValue__Group__0"
// $ANTLR start "rule__EnumerationValue__Group__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7114:1: rule__EnumerationValue__Group__0__Impl : ( ( rule__EnumerationValue__NameAssignment_0 ) ) ;
public final void rule__EnumerationValue__Group__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7118:1: ( ( ( rule__EnumerationValue__NameAssignment_0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7119:1: ( ( rule__EnumerationValue__NameAssignment_0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7119:1: ( ( rule__EnumerationValue__NameAssignment_0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7120:1: ( rule__EnumerationValue__NameAssignment_0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEnumerationValueAccess().getNameAssignment_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7121:1: ( rule__EnumerationValue__NameAssignment_0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7121:2: rule__EnumerationValue__NameAssignment_0
{
pushFollow(FollowSets000.FOLLOW_rule__EnumerationValue__NameAssignment_0_in_rule__EnumerationValue__Group__0__Impl15519);
rule__EnumerationValue__NameAssignment_0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getEnumerationValueAccess().getNameAssignment_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EnumerationValue__Group__0__Impl"
// $ANTLR start "rule__EnumerationValue__Group__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7131:1: rule__EnumerationValue__Group__1 : rule__EnumerationValue__Group__1__Impl ;
public final void rule__EnumerationValue__Group__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7135:1: ( rule__EnumerationValue__Group__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7136:2: rule__EnumerationValue__Group__1__Impl
{
pushFollow(FollowSets000.FOLLOW_rule__EnumerationValue__Group__1__Impl_in_rule__EnumerationValue__Group__115549);
rule__EnumerationValue__Group__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EnumerationValue__Group__1"
// $ANTLR start "rule__EnumerationValue__Group__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7142:1: rule__EnumerationValue__Group__1__Impl : ( ( rule__EnumerationValue__Group_1__0 )? ) ;
public final void rule__EnumerationValue__Group__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7146:1: ( ( ( rule__EnumerationValue__Group_1__0 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7147:1: ( ( rule__EnumerationValue__Group_1__0 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7147:1: ( ( rule__EnumerationValue__Group_1__0 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7148:1: ( rule__EnumerationValue__Group_1__0 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEnumerationValueAccess().getGroup_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7149:1: ( rule__EnumerationValue__Group_1__0 )?
int alt62=2;
int LA62_0 = input.LA(1);
if ( (LA62_0==31) ) {
alt62=1;
}
switch (alt62) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7149:2: rule__EnumerationValue__Group_1__0
{
pushFollow(FollowSets000.FOLLOW_rule__EnumerationValue__Group_1__0_in_rule__EnumerationValue__Group__1__Impl15576);
rule__EnumerationValue__Group_1__0();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getEnumerationValueAccess().getGroup_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EnumerationValue__Group__1__Impl"
// $ANTLR start "rule__EnumerationValue__Group_1__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7163:1: rule__EnumerationValue__Group_1__0 : rule__EnumerationValue__Group_1__0__Impl rule__EnumerationValue__Group_1__1 ;
public final void rule__EnumerationValue__Group_1__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7167:1: ( rule__EnumerationValue__Group_1__0__Impl rule__EnumerationValue__Group_1__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7168:2: rule__EnumerationValue__Group_1__0__Impl rule__EnumerationValue__Group_1__1
{
pushFollow(FollowSets000.FOLLOW_rule__EnumerationValue__Group_1__0__Impl_in_rule__EnumerationValue__Group_1__015611);
rule__EnumerationValue__Group_1__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets000.FOLLOW_rule__EnumerationValue__Group_1__1_in_rule__EnumerationValue__Group_1__015614);
rule__EnumerationValue__Group_1__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EnumerationValue__Group_1__0"
// $ANTLR start "rule__EnumerationValue__Group_1__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7175:1: rule__EnumerationValue__Group_1__0__Impl : ( '=>' ) ;
public final void rule__EnumerationValue__Group_1__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7179:1: ( ( '=>' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7180:1: ( '=>' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7180:1: ( '=>' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7181:1: '=>'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEnumerationValueAccess().getEqualsSignGreaterThanSignKeyword_1_0());
}
match(input,31,FollowSets000.FOLLOW_31_in_rule__EnumerationValue__Group_1__0__Impl15642); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getEnumerationValueAccess().getEqualsSignGreaterThanSignKeyword_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EnumerationValue__Group_1__0__Impl"
// $ANTLR start "rule__EnumerationValue__Group_1__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7194:1: rule__EnumerationValue__Group_1__1 : rule__EnumerationValue__Group_1__1__Impl ;
public final void rule__EnumerationValue__Group_1__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7198:1: ( rule__EnumerationValue__Group_1__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7199:2: rule__EnumerationValue__Group_1__1__Impl
{
pushFollow(FollowSets000.FOLLOW_rule__EnumerationValue__Group_1__1__Impl_in_rule__EnumerationValue__Group_1__115673);
rule__EnumerationValue__Group_1__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EnumerationValue__Group_1__1"
// $ANTLR start "rule__EnumerationValue__Group_1__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7205:1: rule__EnumerationValue__Group_1__1__Impl : ( ( rule__EnumerationValue__ValueAssignment_1_1 ) ) ;
public final void rule__EnumerationValue__Group_1__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7209:1: ( ( ( rule__EnumerationValue__ValueAssignment_1_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7210:1: ( ( rule__EnumerationValue__ValueAssignment_1_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7210:1: ( ( rule__EnumerationValue__ValueAssignment_1_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7211:1: ( rule__EnumerationValue__ValueAssignment_1_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEnumerationValueAccess().getValueAssignment_1_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7212:1: ( rule__EnumerationValue__ValueAssignment_1_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7212:2: rule__EnumerationValue__ValueAssignment_1_1
{
pushFollow(FollowSets000.FOLLOW_rule__EnumerationValue__ValueAssignment_1_1_in_rule__EnumerationValue__Group_1__1__Impl15700);
rule__EnumerationValue__ValueAssignment_1_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getEnumerationValueAccess().getValueAssignment_1_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EnumerationValue__Group_1__1__Impl"
// $ANTLR start "rule__EntityNaturalKeyFields__Group__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7226:1: rule__EntityNaturalKeyFields__Group__0 : rule__EntityNaturalKeyFields__Group__0__Impl rule__EntityNaturalKeyFields__Group__1 ;
public final void rule__EntityNaturalKeyFields__Group__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7230:1: ( rule__EntityNaturalKeyFields__Group__0__Impl rule__EntityNaturalKeyFields__Group__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7231:2: rule__EntityNaturalKeyFields__Group__0__Impl rule__EntityNaturalKeyFields__Group__1
{
pushFollow(FollowSets000.FOLLOW_rule__EntityNaturalKeyFields__Group__0__Impl_in_rule__EntityNaturalKeyFields__Group__015734);
rule__EntityNaturalKeyFields__Group__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets000.FOLLOW_rule__EntityNaturalKeyFields__Group__1_in_rule__EntityNaturalKeyFields__Group__015737);
rule__EntityNaturalKeyFields__Group__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EntityNaturalKeyFields__Group__0"
// $ANTLR start "rule__EntityNaturalKeyFields__Group__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7238:1: rule__EntityNaturalKeyFields__Group__0__Impl : ( () ) ;
public final void rule__EntityNaturalKeyFields__Group__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7242:1: ( ( () ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7243:1: ( () )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7243:1: ( () )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7244:1: ()
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEntityNaturalKeyFieldsAccess().getEntityNaturalKeyFieldsAction_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7245:1: ()
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7247:1:
{
}
if ( state.backtracking==0 ) {
after(grammarAccess.getEntityNaturalKeyFieldsAccess().getEntityNaturalKeyFieldsAction_0());
}
}
}
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EntityNaturalKeyFields__Group__0__Impl"
// $ANTLR start "rule__EntityNaturalKeyFields__Group__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7257:1: rule__EntityNaturalKeyFields__Group__1 : rule__EntityNaturalKeyFields__Group__1__Impl rule__EntityNaturalKeyFields__Group__2 ;
public final void rule__EntityNaturalKeyFields__Group__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7261:1: ( rule__EntityNaturalKeyFields__Group__1__Impl rule__EntityNaturalKeyFields__Group__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7262:2: rule__EntityNaturalKeyFields__Group__1__Impl rule__EntityNaturalKeyFields__Group__2
{
pushFollow(FollowSets000.FOLLOW_rule__EntityNaturalKeyFields__Group__1__Impl_in_rule__EntityNaturalKeyFields__Group__115795);
rule__EntityNaturalKeyFields__Group__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets000.FOLLOW_rule__EntityNaturalKeyFields__Group__2_in_rule__EntityNaturalKeyFields__Group__115798);
rule__EntityNaturalKeyFields__Group__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EntityNaturalKeyFields__Group__1"
// $ANTLR start "rule__EntityNaturalKeyFields__Group__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7269:1: rule__EntityNaturalKeyFields__Group__1__Impl : ( 'naturalkey' ) ;
public final void rule__EntityNaturalKeyFields__Group__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7273:1: ( ( 'naturalkey' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7274:1: ( 'naturalkey' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7274:1: ( 'naturalkey' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7275:1: 'naturalkey'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEntityNaturalKeyFieldsAccess().getNaturalkeyKeyword_1());
}
match(input,71,FollowSets000.FOLLOW_71_in_rule__EntityNaturalKeyFields__Group__1__Impl15826); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getEntityNaturalKeyFieldsAccess().getNaturalkeyKeyword_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EntityNaturalKeyFields__Group__1__Impl"
// $ANTLR start "rule__EntityNaturalKeyFields__Group__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7288:1: rule__EntityNaturalKeyFields__Group__2 : rule__EntityNaturalKeyFields__Group__2__Impl rule__EntityNaturalKeyFields__Group__3 ;
public final void rule__EntityNaturalKeyFields__Group__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7292:1: ( rule__EntityNaturalKeyFields__Group__2__Impl rule__EntityNaturalKeyFields__Group__3 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7293:2: rule__EntityNaturalKeyFields__Group__2__Impl rule__EntityNaturalKeyFields__Group__3
{
pushFollow(FollowSets000.FOLLOW_rule__EntityNaturalKeyFields__Group__2__Impl_in_rule__EntityNaturalKeyFields__Group__215857);
rule__EntityNaturalKeyFields__Group__2__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets000.FOLLOW_rule__EntityNaturalKeyFields__Group__3_in_rule__EntityNaturalKeyFields__Group__215860);
rule__EntityNaturalKeyFields__Group__3();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EntityNaturalKeyFields__Group__2"
// $ANTLR start "rule__EntityNaturalKeyFields__Group__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7300:1: rule__EntityNaturalKeyFields__Group__2__Impl : ( '{' ) ;
public final void rule__EntityNaturalKeyFields__Group__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7304:1: ( ( '{' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7305:1: ( '{' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7305:1: ( '{' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7306:1: '{'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEntityNaturalKeyFieldsAccess().getLeftCurlyBracketKeyword_2());
}
match(input,67,FollowSets000.FOLLOW_67_in_rule__EntityNaturalKeyFields__Group__2__Impl15888); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getEntityNaturalKeyFieldsAccess().getLeftCurlyBracketKeyword_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EntityNaturalKeyFields__Group__2__Impl"
// $ANTLR start "rule__EntityNaturalKeyFields__Group__3"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7319:1: rule__EntityNaturalKeyFields__Group__3 : rule__EntityNaturalKeyFields__Group__3__Impl rule__EntityNaturalKeyFields__Group__4 ;
public final void rule__EntityNaturalKeyFields__Group__3() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7323:1: ( rule__EntityNaturalKeyFields__Group__3__Impl rule__EntityNaturalKeyFields__Group__4 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7324:2: rule__EntityNaturalKeyFields__Group__3__Impl rule__EntityNaturalKeyFields__Group__4
{
pushFollow(FollowSets000.FOLLOW_rule__EntityNaturalKeyFields__Group__3__Impl_in_rule__EntityNaturalKeyFields__Group__315919);
rule__EntityNaturalKeyFields__Group__3__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets000.FOLLOW_rule__EntityNaturalKeyFields__Group__4_in_rule__EntityNaturalKeyFields__Group__315922);
rule__EntityNaturalKeyFields__Group__4();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EntityNaturalKeyFields__Group__3"
// $ANTLR start "rule__EntityNaturalKeyFields__Group__3__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7331:1: rule__EntityNaturalKeyFields__Group__3__Impl : ( ( rule__EntityNaturalKeyFields__NaturalKeyAttributesAssignment_3 ) ) ;
public final void rule__EntityNaturalKeyFields__Group__3__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7335:1: ( ( ( rule__EntityNaturalKeyFields__NaturalKeyAttributesAssignment_3 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7336:1: ( ( rule__EntityNaturalKeyFields__NaturalKeyAttributesAssignment_3 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7336:1: ( ( rule__EntityNaturalKeyFields__NaturalKeyAttributesAssignment_3 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7337:1: ( rule__EntityNaturalKeyFields__NaturalKeyAttributesAssignment_3 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEntityNaturalKeyFieldsAccess().getNaturalKeyAttributesAssignment_3());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7338:1: ( rule__EntityNaturalKeyFields__NaturalKeyAttributesAssignment_3 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7338:2: rule__EntityNaturalKeyFields__NaturalKeyAttributesAssignment_3
{
pushFollow(FollowSets000.FOLLOW_rule__EntityNaturalKeyFields__NaturalKeyAttributesAssignment_3_in_rule__EntityNaturalKeyFields__Group__3__Impl15949);
rule__EntityNaturalKeyFields__NaturalKeyAttributesAssignment_3();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getEntityNaturalKeyFieldsAccess().getNaturalKeyAttributesAssignment_3());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EntityNaturalKeyFields__Group__3__Impl"
// $ANTLR start "rule__EntityNaturalKeyFields__Group__4"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7348:1: rule__EntityNaturalKeyFields__Group__4 : rule__EntityNaturalKeyFields__Group__4__Impl rule__EntityNaturalKeyFields__Group__5 ;
public final void rule__EntityNaturalKeyFields__Group__4() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7352:1: ( rule__EntityNaturalKeyFields__Group__4__Impl rule__EntityNaturalKeyFields__Group__5 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7353:2: rule__EntityNaturalKeyFields__Group__4__Impl rule__EntityNaturalKeyFields__Group__5
{
pushFollow(FollowSets000.FOLLOW_rule__EntityNaturalKeyFields__Group__4__Impl_in_rule__EntityNaturalKeyFields__Group__415979);
rule__EntityNaturalKeyFields__Group__4__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets000.FOLLOW_rule__EntityNaturalKeyFields__Group__5_in_rule__EntityNaturalKeyFields__Group__415982);
rule__EntityNaturalKeyFields__Group__5();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EntityNaturalKeyFields__Group__4"
// $ANTLR start "rule__EntityNaturalKeyFields__Group__4__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7360:1: rule__EntityNaturalKeyFields__Group__4__Impl : ( ( rule__EntityNaturalKeyFields__Group_4__0 )* ) ;
public final void rule__EntityNaturalKeyFields__Group__4__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7364:1: ( ( ( rule__EntityNaturalKeyFields__Group_4__0 )* ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7365:1: ( ( rule__EntityNaturalKeyFields__Group_4__0 )* )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7365:1: ( ( rule__EntityNaturalKeyFields__Group_4__0 )* )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7366:1: ( rule__EntityNaturalKeyFields__Group_4__0 )*
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEntityNaturalKeyFieldsAccess().getGroup_4());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7367:1: ( rule__EntityNaturalKeyFields__Group_4__0 )*
loop63:
do {
int alt63=2;
int LA63_0 = input.LA(1);
if ( (LA63_0==72) ) {
alt63=1;
}
switch (alt63) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7367:2: rule__EntityNaturalKeyFields__Group_4__0
{
pushFollow(FollowSets000.FOLLOW_rule__EntityNaturalKeyFields__Group_4__0_in_rule__EntityNaturalKeyFields__Group__4__Impl16009);
rule__EntityNaturalKeyFields__Group_4__0();
state._fsp--;
if (state.failed) return ;
}
break;
default :
break loop63;
}
} while (true);
if ( state.backtracking==0 ) {
after(grammarAccess.getEntityNaturalKeyFieldsAccess().getGroup_4());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EntityNaturalKeyFields__Group__4__Impl"
// $ANTLR start "rule__EntityNaturalKeyFields__Group__5"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7377:1: rule__EntityNaturalKeyFields__Group__5 : rule__EntityNaturalKeyFields__Group__5__Impl ;
public final void rule__EntityNaturalKeyFields__Group__5() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7381:1: ( rule__EntityNaturalKeyFields__Group__5__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7382:2: rule__EntityNaturalKeyFields__Group__5__Impl
{
pushFollow(FollowSets000.FOLLOW_rule__EntityNaturalKeyFields__Group__5__Impl_in_rule__EntityNaturalKeyFields__Group__516040);
rule__EntityNaturalKeyFields__Group__5__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EntityNaturalKeyFields__Group__5"
// $ANTLR start "rule__EntityNaturalKeyFields__Group__5__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7388:1: rule__EntityNaturalKeyFields__Group__5__Impl : ( '}' ) ;
public final void rule__EntityNaturalKeyFields__Group__5__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7392:1: ( ( '}' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7393:1: ( '}' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7393:1: ( '}' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7394:1: '}'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEntityNaturalKeyFieldsAccess().getRightCurlyBracketKeyword_5());
}
match(input,68,FollowSets000.FOLLOW_68_in_rule__EntityNaturalKeyFields__Group__5__Impl16068); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getEntityNaturalKeyFieldsAccess().getRightCurlyBracketKeyword_5());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EntityNaturalKeyFields__Group__5__Impl"
// $ANTLR start "rule__EntityNaturalKeyFields__Group_4__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7419:1: rule__EntityNaturalKeyFields__Group_4__0 : rule__EntityNaturalKeyFields__Group_4__0__Impl rule__EntityNaturalKeyFields__Group_4__1 ;
public final void rule__EntityNaturalKeyFields__Group_4__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7423:1: ( rule__EntityNaturalKeyFields__Group_4__0__Impl rule__EntityNaturalKeyFields__Group_4__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7424:2: rule__EntityNaturalKeyFields__Group_4__0__Impl rule__EntityNaturalKeyFields__Group_4__1
{
pushFollow(FollowSets000.FOLLOW_rule__EntityNaturalKeyFields__Group_4__0__Impl_in_rule__EntityNaturalKeyFields__Group_4__016111);
rule__EntityNaturalKeyFields__Group_4__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets000.FOLLOW_rule__EntityNaturalKeyFields__Group_4__1_in_rule__EntityNaturalKeyFields__Group_4__016114);
rule__EntityNaturalKeyFields__Group_4__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EntityNaturalKeyFields__Group_4__0"
// $ANTLR start "rule__EntityNaturalKeyFields__Group_4__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7431:1: rule__EntityNaturalKeyFields__Group_4__0__Impl : ( ', ' ) ;
public final void rule__EntityNaturalKeyFields__Group_4__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7435:1: ( ( ', ' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7436:1: ( ', ' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7436:1: ( ', ' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7437:1: ', '
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEntityNaturalKeyFieldsAccess().getCommaSpaceKeyword_4_0());
}
match(input,72,FollowSets000.FOLLOW_72_in_rule__EntityNaturalKeyFields__Group_4__0__Impl16142); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getEntityNaturalKeyFieldsAccess().getCommaSpaceKeyword_4_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EntityNaturalKeyFields__Group_4__0__Impl"
// $ANTLR start "rule__EntityNaturalKeyFields__Group_4__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7450:1: rule__EntityNaturalKeyFields__Group_4__1 : rule__EntityNaturalKeyFields__Group_4__1__Impl ;
public final void rule__EntityNaturalKeyFields__Group_4__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7454:1: ( rule__EntityNaturalKeyFields__Group_4__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7455:2: rule__EntityNaturalKeyFields__Group_4__1__Impl
{
pushFollow(FollowSets000.FOLLOW_rule__EntityNaturalKeyFields__Group_4__1__Impl_in_rule__EntityNaturalKeyFields__Group_4__116173);
rule__EntityNaturalKeyFields__Group_4__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EntityNaturalKeyFields__Group_4__1"
// $ANTLR start "rule__EntityNaturalKeyFields__Group_4__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7461:1: rule__EntityNaturalKeyFields__Group_4__1__Impl : ( ( rule__EntityNaturalKeyFields__NaturalKeyAttributesAssignment_4_1 ) ) ;
public final void rule__EntityNaturalKeyFields__Group_4__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7465:1: ( ( ( rule__EntityNaturalKeyFields__NaturalKeyAttributesAssignment_4_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7466:1: ( ( rule__EntityNaturalKeyFields__NaturalKeyAttributesAssignment_4_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7466:1: ( ( rule__EntityNaturalKeyFields__NaturalKeyAttributesAssignment_4_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7467:1: ( rule__EntityNaturalKeyFields__NaturalKeyAttributesAssignment_4_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEntityNaturalKeyFieldsAccess().getNaturalKeyAttributesAssignment_4_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7468:1: ( rule__EntityNaturalKeyFields__NaturalKeyAttributesAssignment_4_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7468:2: rule__EntityNaturalKeyFields__NaturalKeyAttributesAssignment_4_1
{
pushFollow(FollowSets000.FOLLOW_rule__EntityNaturalKeyFields__NaturalKeyAttributesAssignment_4_1_in_rule__EntityNaturalKeyFields__Group_4__1__Impl16200);
rule__EntityNaturalKeyFields__NaturalKeyAttributesAssignment_4_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getEntityNaturalKeyFieldsAccess().getNaturalKeyAttributesAssignment_4_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EntityNaturalKeyFields__Group_4__1__Impl"
// $ANTLR start "rule__EntityHierarchical__Group__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7482:1: rule__EntityHierarchical__Group__0 : rule__EntityHierarchical__Group__0__Impl rule__EntityHierarchical__Group__1 ;
public final void rule__EntityHierarchical__Group__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7486:1: ( rule__EntityHierarchical__Group__0__Impl rule__EntityHierarchical__Group__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7487:2: rule__EntityHierarchical__Group__0__Impl rule__EntityHierarchical__Group__1
{
pushFollow(FollowSets000.FOLLOW_rule__EntityHierarchical__Group__0__Impl_in_rule__EntityHierarchical__Group__016234);
rule__EntityHierarchical__Group__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets000.FOLLOW_rule__EntityHierarchical__Group__1_in_rule__EntityHierarchical__Group__016237);
rule__EntityHierarchical__Group__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EntityHierarchical__Group__0"
// $ANTLR start "rule__EntityHierarchical__Group__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7494:1: rule__EntityHierarchical__Group__0__Impl : ( () ) ;
public final void rule__EntityHierarchical__Group__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7498:1: ( ( () ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7499:1: ( () )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7499:1: ( () )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7500:1: ()
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEntityHierarchicalAccess().getEntityHierarchicalAction_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7501:1: ()
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7503:1:
{
}
if ( state.backtracking==0 ) {
after(grammarAccess.getEntityHierarchicalAccess().getEntityHierarchicalAction_0());
}
}
}
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EntityHierarchical__Group__0__Impl"
// $ANTLR start "rule__EntityHierarchical__Group__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7513:1: rule__EntityHierarchical__Group__1 : rule__EntityHierarchical__Group__1__Impl rule__EntityHierarchical__Group__2 ;
public final void rule__EntityHierarchical__Group__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7517:1: ( rule__EntityHierarchical__Group__1__Impl rule__EntityHierarchical__Group__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7518:2: rule__EntityHierarchical__Group__1__Impl rule__EntityHierarchical__Group__2
{
pushFollow(FollowSets000.FOLLOW_rule__EntityHierarchical__Group__1__Impl_in_rule__EntityHierarchical__Group__116295);
rule__EntityHierarchical__Group__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets000.FOLLOW_rule__EntityHierarchical__Group__2_in_rule__EntityHierarchical__Group__116298);
rule__EntityHierarchical__Group__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EntityHierarchical__Group__1"
// $ANTLR start "rule__EntityHierarchical__Group__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7525:1: rule__EntityHierarchical__Group__1__Impl : ( 'hierarchicalEntity' ) ;
public final void rule__EntityHierarchical__Group__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7529:1: ( ( 'hierarchicalEntity' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7530:1: ( 'hierarchicalEntity' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7530:1: ( 'hierarchicalEntity' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7531:1: 'hierarchicalEntity'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEntityHierarchicalAccess().getHierarchicalEntityKeyword_1());
}
match(input,73,FollowSets000.FOLLOW_73_in_rule__EntityHierarchical__Group__1__Impl16326); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getEntityHierarchicalAccess().getHierarchicalEntityKeyword_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EntityHierarchical__Group__1__Impl"
// $ANTLR start "rule__EntityHierarchical__Group__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7544:1: rule__EntityHierarchical__Group__2 : rule__EntityHierarchical__Group__2__Impl ;
public final void rule__EntityHierarchical__Group__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7548:1: ( rule__EntityHierarchical__Group__2__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7549:2: rule__EntityHierarchical__Group__2__Impl
{
pushFollow(FollowSets000.FOLLOW_rule__EntityHierarchical__Group__2__Impl_in_rule__EntityHierarchical__Group__216357);
rule__EntityHierarchical__Group__2__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EntityHierarchical__Group__2"
// $ANTLR start "rule__EntityHierarchical__Group__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7555:1: rule__EntityHierarchical__Group__2__Impl : ( ( rule__EntityHierarchical__HierarchicalAssignment_2 ) ) ;
public final void rule__EntityHierarchical__Group__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7559:1: ( ( ( rule__EntityHierarchical__HierarchicalAssignment_2 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7560:1: ( ( rule__EntityHierarchical__HierarchicalAssignment_2 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7560:1: ( ( rule__EntityHierarchical__HierarchicalAssignment_2 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7561:1: ( rule__EntityHierarchical__HierarchicalAssignment_2 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEntityHierarchicalAccess().getHierarchicalAssignment_2());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7562:1: ( rule__EntityHierarchical__HierarchicalAssignment_2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7562:2: rule__EntityHierarchical__HierarchicalAssignment_2
{
pushFollow(FollowSets000.FOLLOW_rule__EntityHierarchical__HierarchicalAssignment_2_in_rule__EntityHierarchical__Group__2__Impl16384);
rule__EntityHierarchical__HierarchicalAssignment_2();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getEntityHierarchicalAccess().getHierarchicalAssignment_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EntityHierarchical__Group__2__Impl"
// $ANTLR start "rule__EntityDisableIdField__Group__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7578:1: rule__EntityDisableIdField__Group__0 : rule__EntityDisableIdField__Group__0__Impl rule__EntityDisableIdField__Group__1 ;
public final void rule__EntityDisableIdField__Group__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7582:1: ( rule__EntityDisableIdField__Group__0__Impl rule__EntityDisableIdField__Group__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7583:2: rule__EntityDisableIdField__Group__0__Impl rule__EntityDisableIdField__Group__1
{
pushFollow(FollowSets000.FOLLOW_rule__EntityDisableIdField__Group__0__Impl_in_rule__EntityDisableIdField__Group__016420);
rule__EntityDisableIdField__Group__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets000.FOLLOW_rule__EntityDisableIdField__Group__1_in_rule__EntityDisableIdField__Group__016423);
rule__EntityDisableIdField__Group__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EntityDisableIdField__Group__0"
// $ANTLR start "rule__EntityDisableIdField__Group__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7590:1: rule__EntityDisableIdField__Group__0__Impl : ( () ) ;
public final void rule__EntityDisableIdField__Group__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7594:1: ( ( () ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7595:1: ( () )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7595:1: ( () )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7596:1: ()
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEntityDisableIdFieldAccess().getEntityDisableIdFieldAction_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7597:1: ()
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7599:1:
{
}
if ( state.backtracking==0 ) {
after(grammarAccess.getEntityDisableIdFieldAccess().getEntityDisableIdFieldAction_0());
}
}
}
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EntityDisableIdField__Group__0__Impl"
// $ANTLR start "rule__EntityDisableIdField__Group__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7609:1: rule__EntityDisableIdField__Group__1 : rule__EntityDisableIdField__Group__1__Impl rule__EntityDisableIdField__Group__2 ;
public final void rule__EntityDisableIdField__Group__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7613:1: ( rule__EntityDisableIdField__Group__1__Impl rule__EntityDisableIdField__Group__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7614:2: rule__EntityDisableIdField__Group__1__Impl rule__EntityDisableIdField__Group__2
{
pushFollow(FollowSets000.FOLLOW_rule__EntityDisableIdField__Group__1__Impl_in_rule__EntityDisableIdField__Group__116481);
rule__EntityDisableIdField__Group__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets000.FOLLOW_rule__EntityDisableIdField__Group__2_in_rule__EntityDisableIdField__Group__116484);
rule__EntityDisableIdField__Group__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EntityDisableIdField__Group__1"
// $ANTLR start "rule__EntityDisableIdField__Group__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7621:1: rule__EntityDisableIdField__Group__1__Impl : ( 'disableIdField' ) ;
public final void rule__EntityDisableIdField__Group__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7625:1: ( ( 'disableIdField' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7626:1: ( 'disableIdField' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7626:1: ( 'disableIdField' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7627:1: 'disableIdField'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEntityDisableIdFieldAccess().getDisableIdFieldKeyword_1());
}
match(input,74,FollowSets000.FOLLOW_74_in_rule__EntityDisableIdField__Group__1__Impl16512); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getEntityDisableIdFieldAccess().getDisableIdFieldKeyword_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EntityDisableIdField__Group__1__Impl"
// $ANTLR start "rule__EntityDisableIdField__Group__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7640:1: rule__EntityDisableIdField__Group__2 : rule__EntityDisableIdField__Group__2__Impl ;
public final void rule__EntityDisableIdField__Group__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7644:1: ( rule__EntityDisableIdField__Group__2__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7645:2: rule__EntityDisableIdField__Group__2__Impl
{
pushFollow(FollowSets000.FOLLOW_rule__EntityDisableIdField__Group__2__Impl_in_rule__EntityDisableIdField__Group__216543);
rule__EntityDisableIdField__Group__2__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EntityDisableIdField__Group__2"
// $ANTLR start "rule__EntityDisableIdField__Group__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7651:1: rule__EntityDisableIdField__Group__2__Impl : ( ( rule__EntityDisableIdField__DisableIdFieldAssignment_2 ) ) ;
public final void rule__EntityDisableIdField__Group__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7655:1: ( ( ( rule__EntityDisableIdField__DisableIdFieldAssignment_2 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7656:1: ( ( rule__EntityDisableIdField__DisableIdFieldAssignment_2 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7656:1: ( ( rule__EntityDisableIdField__DisableIdFieldAssignment_2 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7657:1: ( rule__EntityDisableIdField__DisableIdFieldAssignment_2 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEntityDisableIdFieldAccess().getDisableIdFieldAssignment_2());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7658:1: ( rule__EntityDisableIdField__DisableIdFieldAssignment_2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7658:2: rule__EntityDisableIdField__DisableIdFieldAssignment_2
{
pushFollow(FollowSets000.FOLLOW_rule__EntityDisableIdField__DisableIdFieldAssignment_2_in_rule__EntityDisableIdField__Group__2__Impl16570);
rule__EntityDisableIdField__DisableIdFieldAssignment_2();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getEntityDisableIdFieldAccess().getDisableIdFieldAssignment_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EntityDisableIdField__Group__2__Impl"
// $ANTLR start "rule__EntityLabelField__Group__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7674:1: rule__EntityLabelField__Group__0 : rule__EntityLabelField__Group__0__Impl rule__EntityLabelField__Group__1 ;
public final void rule__EntityLabelField__Group__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7678:1: ( rule__EntityLabelField__Group__0__Impl rule__EntityLabelField__Group__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7679:2: rule__EntityLabelField__Group__0__Impl rule__EntityLabelField__Group__1
{
pushFollow(FollowSets000.FOLLOW_rule__EntityLabelField__Group__0__Impl_in_rule__EntityLabelField__Group__016606);
rule__EntityLabelField__Group__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets000.FOLLOW_rule__EntityLabelField__Group__1_in_rule__EntityLabelField__Group__016609);
rule__EntityLabelField__Group__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EntityLabelField__Group__0"
// $ANTLR start "rule__EntityLabelField__Group__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7686:1: rule__EntityLabelField__Group__0__Impl : ( () ) ;
public final void rule__EntityLabelField__Group__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7690:1: ( ( () ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7691:1: ( () )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7691:1: ( () )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7692:1: ()
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEntityLabelFieldAccess().getEntityLabelFieldAction_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7693:1: ()
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7695:1:
{
}
if ( state.backtracking==0 ) {
after(grammarAccess.getEntityLabelFieldAccess().getEntityLabelFieldAction_0());
}
}
}
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EntityLabelField__Group__0__Impl"
// $ANTLR start "rule__EntityLabelField__Group__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7705:1: rule__EntityLabelField__Group__1 : rule__EntityLabelField__Group__1__Impl rule__EntityLabelField__Group__2 ;
public final void rule__EntityLabelField__Group__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7709:1: ( rule__EntityLabelField__Group__1__Impl rule__EntityLabelField__Group__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7710:2: rule__EntityLabelField__Group__1__Impl rule__EntityLabelField__Group__2
{
pushFollow(FollowSets000.FOLLOW_rule__EntityLabelField__Group__1__Impl_in_rule__EntityLabelField__Group__116667);
rule__EntityLabelField__Group__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets000.FOLLOW_rule__EntityLabelField__Group__2_in_rule__EntityLabelField__Group__116670);
rule__EntityLabelField__Group__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EntityLabelField__Group__1"
// $ANTLR start "rule__EntityLabelField__Group__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7717:1: rule__EntityLabelField__Group__1__Impl : ( 'label' ) ;
public final void rule__EntityLabelField__Group__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7721:1: ( ( 'label' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7722:1: ( 'label' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7722:1: ( 'label' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7723:1: 'label'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEntityLabelFieldAccess().getLabelKeyword_1());
}
match(input,75,FollowSets000.FOLLOW_75_in_rule__EntityLabelField__Group__1__Impl16698); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getEntityLabelFieldAccess().getLabelKeyword_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EntityLabelField__Group__1__Impl"
// $ANTLR start "rule__EntityLabelField__Group__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7736:1: rule__EntityLabelField__Group__2 : rule__EntityLabelField__Group__2__Impl ;
public final void rule__EntityLabelField__Group__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7740:1: ( rule__EntityLabelField__Group__2__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7741:2: rule__EntityLabelField__Group__2__Impl
{
pushFollow(FollowSets000.FOLLOW_rule__EntityLabelField__Group__2__Impl_in_rule__EntityLabelField__Group__216729);
rule__EntityLabelField__Group__2__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EntityLabelField__Group__2"
// $ANTLR start "rule__EntityLabelField__Group__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7747:1: rule__EntityLabelField__Group__2__Impl : ( ( rule__EntityLabelField__LabelAssignment_2 )? ) ;
public final void rule__EntityLabelField__Group__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7751:1: ( ( ( rule__EntityLabelField__LabelAssignment_2 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7752:1: ( ( rule__EntityLabelField__LabelAssignment_2 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7752:1: ( ( rule__EntityLabelField__LabelAssignment_2 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7753:1: ( rule__EntityLabelField__LabelAssignment_2 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEntityLabelFieldAccess().getLabelAssignment_2());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7754:1: ( rule__EntityLabelField__LabelAssignment_2 )?
int alt64=2;
int LA64_0 = input.LA(1);
if ( (LA64_0==RULE_STRING) ) {
alt64=1;
}
switch (alt64) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7754:2: rule__EntityLabelField__LabelAssignment_2
{
pushFollow(FollowSets000.FOLLOW_rule__EntityLabelField__LabelAssignment_2_in_rule__EntityLabelField__Group__2__Impl16756);
rule__EntityLabelField__LabelAssignment_2();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getEntityLabelFieldAccess().getLabelAssignment_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EntityLabelField__Group__2__Impl"
// $ANTLR start "rule__EntityPluralLabelField__Group__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7770:1: rule__EntityPluralLabelField__Group__0 : rule__EntityPluralLabelField__Group__0__Impl rule__EntityPluralLabelField__Group__1 ;
public final void rule__EntityPluralLabelField__Group__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7774:1: ( rule__EntityPluralLabelField__Group__0__Impl rule__EntityPluralLabelField__Group__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7775:2: rule__EntityPluralLabelField__Group__0__Impl rule__EntityPluralLabelField__Group__1
{
pushFollow(FollowSets000.FOLLOW_rule__EntityPluralLabelField__Group__0__Impl_in_rule__EntityPluralLabelField__Group__016793);
rule__EntityPluralLabelField__Group__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets000.FOLLOW_rule__EntityPluralLabelField__Group__1_in_rule__EntityPluralLabelField__Group__016796);
rule__EntityPluralLabelField__Group__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EntityPluralLabelField__Group__0"
// $ANTLR start "rule__EntityPluralLabelField__Group__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7782:1: rule__EntityPluralLabelField__Group__0__Impl : ( () ) ;
public final void rule__EntityPluralLabelField__Group__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7786:1: ( ( () ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7787:1: ( () )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7787:1: ( () )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7788:1: ()
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEntityPluralLabelFieldAccess().getEntityPluralLabelFieldAction_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7789:1: ()
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7791:1:
{
}
if ( state.backtracking==0 ) {
after(grammarAccess.getEntityPluralLabelFieldAccess().getEntityPluralLabelFieldAction_0());
}
}
}
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EntityPluralLabelField__Group__0__Impl"
// $ANTLR start "rule__EntityPluralLabelField__Group__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7801:1: rule__EntityPluralLabelField__Group__1 : rule__EntityPluralLabelField__Group__1__Impl rule__EntityPluralLabelField__Group__2 ;
public final void rule__EntityPluralLabelField__Group__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7805:1: ( rule__EntityPluralLabelField__Group__1__Impl rule__EntityPluralLabelField__Group__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7806:2: rule__EntityPluralLabelField__Group__1__Impl rule__EntityPluralLabelField__Group__2
{
pushFollow(FollowSets000.FOLLOW_rule__EntityPluralLabelField__Group__1__Impl_in_rule__EntityPluralLabelField__Group__116854);
rule__EntityPluralLabelField__Group__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets000.FOLLOW_rule__EntityPluralLabelField__Group__2_in_rule__EntityPluralLabelField__Group__116857);
rule__EntityPluralLabelField__Group__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EntityPluralLabelField__Group__1"
// $ANTLR start "rule__EntityPluralLabelField__Group__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7813:1: rule__EntityPluralLabelField__Group__1__Impl : ( 'pluralLabel' ) ;
public final void rule__EntityPluralLabelField__Group__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7817:1: ( ( 'pluralLabel' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7818:1: ( 'pluralLabel' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7818:1: ( 'pluralLabel' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7819:1: 'pluralLabel'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEntityPluralLabelFieldAccess().getPluralLabelKeyword_1());
}
match(input,76,FollowSets000.FOLLOW_76_in_rule__EntityPluralLabelField__Group__1__Impl16885); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getEntityPluralLabelFieldAccess().getPluralLabelKeyword_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EntityPluralLabelField__Group__1__Impl"
// $ANTLR start "rule__EntityPluralLabelField__Group__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7832:1: rule__EntityPluralLabelField__Group__2 : rule__EntityPluralLabelField__Group__2__Impl ;
public final void rule__EntityPluralLabelField__Group__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7836:1: ( rule__EntityPluralLabelField__Group__2__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7837:2: rule__EntityPluralLabelField__Group__2__Impl
{
pushFollow(FollowSets000.FOLLOW_rule__EntityPluralLabelField__Group__2__Impl_in_rule__EntityPluralLabelField__Group__216916);
rule__EntityPluralLabelField__Group__2__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EntityPluralLabelField__Group__2"
// $ANTLR start "rule__EntityPluralLabelField__Group__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7843:1: rule__EntityPluralLabelField__Group__2__Impl : ( ( rule__EntityPluralLabelField__PluralLabelAssignment_2 ) ) ;
public final void rule__EntityPluralLabelField__Group__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7847:1: ( ( ( rule__EntityPluralLabelField__PluralLabelAssignment_2 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7848:1: ( ( rule__EntityPluralLabelField__PluralLabelAssignment_2 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7848:1: ( ( rule__EntityPluralLabelField__PluralLabelAssignment_2 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7849:1: ( rule__EntityPluralLabelField__PluralLabelAssignment_2 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEntityPluralLabelFieldAccess().getPluralLabelAssignment_2());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7850:1: ( rule__EntityPluralLabelField__PluralLabelAssignment_2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7850:2: rule__EntityPluralLabelField__PluralLabelAssignment_2
{
pushFollow(FollowSets000.FOLLOW_rule__EntityPluralLabelField__PluralLabelAssignment_2_in_rule__EntityPluralLabelField__Group__2__Impl16943);
rule__EntityPluralLabelField__PluralLabelAssignment_2();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getEntityPluralLabelFieldAccess().getPluralLabelAssignment_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EntityPluralLabelField__Group__2__Impl"
// $ANTLR start "rule__EntityOptionsContainer__Group__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7868:1: rule__EntityOptionsContainer__Group__0 : rule__EntityOptionsContainer__Group__0__Impl rule__EntityOptionsContainer__Group__1 ;
public final void rule__EntityOptionsContainer__Group__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7872:1: ( rule__EntityOptionsContainer__Group__0__Impl rule__EntityOptionsContainer__Group__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7873:2: rule__EntityOptionsContainer__Group__0__Impl rule__EntityOptionsContainer__Group__1
{
pushFollow(FollowSets000.FOLLOW_rule__EntityOptionsContainer__Group__0__Impl_in_rule__EntityOptionsContainer__Group__016981);
rule__EntityOptionsContainer__Group__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets000.FOLLOW_rule__EntityOptionsContainer__Group__1_in_rule__EntityOptionsContainer__Group__016984);
rule__EntityOptionsContainer__Group__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EntityOptionsContainer__Group__0"
// $ANTLR start "rule__EntityOptionsContainer__Group__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7880:1: rule__EntityOptionsContainer__Group__0__Impl : ( () ) ;
public final void rule__EntityOptionsContainer__Group__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7884:1: ( ( () ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7885:1: ( () )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7885:1: ( () )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7886:1: ()
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEntityOptionsContainerAccess().getEntityOptionsContainerAction_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7887:1: ()
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7889:1:
{
}
if ( state.backtracking==0 ) {
after(grammarAccess.getEntityOptionsContainerAccess().getEntityOptionsContainerAction_0());
}
}
}
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EntityOptionsContainer__Group__0__Impl"
// $ANTLR start "rule__EntityOptionsContainer__Group__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7899:1: rule__EntityOptionsContainer__Group__1 : rule__EntityOptionsContainer__Group__1__Impl rule__EntityOptionsContainer__Group__2 ;
public final void rule__EntityOptionsContainer__Group__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7903:1: ( rule__EntityOptionsContainer__Group__1__Impl rule__EntityOptionsContainer__Group__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7904:2: rule__EntityOptionsContainer__Group__1__Impl rule__EntityOptionsContainer__Group__2
{
pushFollow(FollowSets000.FOLLOW_rule__EntityOptionsContainer__Group__1__Impl_in_rule__EntityOptionsContainer__Group__117042);
rule__EntityOptionsContainer__Group__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets000.FOLLOW_rule__EntityOptionsContainer__Group__2_in_rule__EntityOptionsContainer__Group__117045);
rule__EntityOptionsContainer__Group__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EntityOptionsContainer__Group__1"
// $ANTLR start "rule__EntityOptionsContainer__Group__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7911:1: rule__EntityOptionsContainer__Group__1__Impl : ( 'entityoptions' ) ;
public final void rule__EntityOptionsContainer__Group__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7915:1: ( ( 'entityoptions' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7916:1: ( 'entityoptions' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7916:1: ( 'entityoptions' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7917:1: 'entityoptions'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEntityOptionsContainerAccess().getEntityoptionsKeyword_1());
}
match(input,77,FollowSets000.FOLLOW_77_in_rule__EntityOptionsContainer__Group__1__Impl17073); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getEntityOptionsContainerAccess().getEntityoptionsKeyword_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EntityOptionsContainer__Group__1__Impl"
// $ANTLR start "rule__EntityOptionsContainer__Group__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7930:1: rule__EntityOptionsContainer__Group__2 : rule__EntityOptionsContainer__Group__2__Impl rule__EntityOptionsContainer__Group__3 ;
public final void rule__EntityOptionsContainer__Group__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7934:1: ( rule__EntityOptionsContainer__Group__2__Impl rule__EntityOptionsContainer__Group__3 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7935:2: rule__EntityOptionsContainer__Group__2__Impl rule__EntityOptionsContainer__Group__3
{
pushFollow(FollowSets000.FOLLOW_rule__EntityOptionsContainer__Group__2__Impl_in_rule__EntityOptionsContainer__Group__217104);
rule__EntityOptionsContainer__Group__2__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets000.FOLLOW_rule__EntityOptionsContainer__Group__3_in_rule__EntityOptionsContainer__Group__217107);
rule__EntityOptionsContainer__Group__3();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EntityOptionsContainer__Group__2"
// $ANTLR start "rule__EntityOptionsContainer__Group__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7942:1: rule__EntityOptionsContainer__Group__2__Impl : ( '{' ) ;
public final void rule__EntityOptionsContainer__Group__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7946:1: ( ( '{' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7947:1: ( '{' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7947:1: ( '{' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7948:1: '{'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEntityOptionsContainerAccess().getLeftCurlyBracketKeyword_2());
}
match(input,67,FollowSets000.FOLLOW_67_in_rule__EntityOptionsContainer__Group__2__Impl17135); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getEntityOptionsContainerAccess().getLeftCurlyBracketKeyword_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EntityOptionsContainer__Group__2__Impl"
// $ANTLR start "rule__EntityOptionsContainer__Group__3"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7961:1: rule__EntityOptionsContainer__Group__3 : rule__EntityOptionsContainer__Group__3__Impl rule__EntityOptionsContainer__Group__4 ;
public final void rule__EntityOptionsContainer__Group__3() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7965:1: ( rule__EntityOptionsContainer__Group__3__Impl rule__EntityOptionsContainer__Group__4 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7966:2: rule__EntityOptionsContainer__Group__3__Impl rule__EntityOptionsContainer__Group__4
{
pushFollow(FollowSets000.FOLLOW_rule__EntityOptionsContainer__Group__3__Impl_in_rule__EntityOptionsContainer__Group__317166);
rule__EntityOptionsContainer__Group__3__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets000.FOLLOW_rule__EntityOptionsContainer__Group__4_in_rule__EntityOptionsContainer__Group__317169);
rule__EntityOptionsContainer__Group__4();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EntityOptionsContainer__Group__3"
// $ANTLR start "rule__EntityOptionsContainer__Group__3__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7973:1: rule__EntityOptionsContainer__Group__3__Impl : ( ( rule__EntityOptionsContainer__OptionsAssignment_3 )* ) ;
public final void rule__EntityOptionsContainer__Group__3__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7977:1: ( ( ( rule__EntityOptionsContainer__OptionsAssignment_3 )* ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7978:1: ( ( rule__EntityOptionsContainer__OptionsAssignment_3 )* )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7978:1: ( ( rule__EntityOptionsContainer__OptionsAssignment_3 )* )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7979:1: ( rule__EntityOptionsContainer__OptionsAssignment_3 )*
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEntityOptionsContainerAccess().getOptionsAssignment_3());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7980:1: ( rule__EntityOptionsContainer__OptionsAssignment_3 )*
loop65:
do {
int alt65=2;
int LA65_0 = input.LA(1);
if ( (LA65_0==71||(LA65_0>=73 && LA65_0<=76)) ) {
alt65=1;
}
switch (alt65) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7980:2: rule__EntityOptionsContainer__OptionsAssignment_3
{
pushFollow(FollowSets000.FOLLOW_rule__EntityOptionsContainer__OptionsAssignment_3_in_rule__EntityOptionsContainer__Group__3__Impl17196);
rule__EntityOptionsContainer__OptionsAssignment_3();
state._fsp--;
if (state.failed) return ;
}
break;
default :
break loop65;
}
} while (true);
if ( state.backtracking==0 ) {
after(grammarAccess.getEntityOptionsContainerAccess().getOptionsAssignment_3());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EntityOptionsContainer__Group__3__Impl"
// $ANTLR start "rule__EntityOptionsContainer__Group__4"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7990:1: rule__EntityOptionsContainer__Group__4 : rule__EntityOptionsContainer__Group__4__Impl ;
public final void rule__EntityOptionsContainer__Group__4() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7994:1: ( rule__EntityOptionsContainer__Group__4__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:7995:2: rule__EntityOptionsContainer__Group__4__Impl
{
pushFollow(FollowSets000.FOLLOW_rule__EntityOptionsContainer__Group__4__Impl_in_rule__EntityOptionsContainer__Group__417227);
rule__EntityOptionsContainer__Group__4__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EntityOptionsContainer__Group__4"
// $ANTLR start "rule__EntityOptionsContainer__Group__4__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8001:1: rule__EntityOptionsContainer__Group__4__Impl : ( '}' ) ;
public final void rule__EntityOptionsContainer__Group__4__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8005:1: ( ( '}' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8006:1: ( '}' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8006:1: ( '}' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8007:1: '}'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEntityOptionsContainerAccess().getRightCurlyBracketKeyword_4());
}
match(input,68,FollowSets000.FOLLOW_68_in_rule__EntityOptionsContainer__Group__4__Impl17255); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getEntityOptionsContainerAccess().getRightCurlyBracketKeyword_4());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EntityOptionsContainer__Group__4__Impl"
// $ANTLR start "rule__Entity__Group__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8030:1: rule__Entity__Group__0 : rule__Entity__Group__0__Impl rule__Entity__Group__1 ;
public final void rule__Entity__Group__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8034:1: ( rule__Entity__Group__0__Impl rule__Entity__Group__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8035:2: rule__Entity__Group__0__Impl rule__Entity__Group__1
{
pushFollow(FollowSets000.FOLLOW_rule__Entity__Group__0__Impl_in_rule__Entity__Group__017296);
rule__Entity__Group__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets000.FOLLOW_rule__Entity__Group__1_in_rule__Entity__Group__017299);
rule__Entity__Group__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Entity__Group__0"
// $ANTLR start "rule__Entity__Group__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8042:1: rule__Entity__Group__0__Impl : ( 'entity' ) ;
public final void rule__Entity__Group__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8046:1: ( ( 'entity' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8047:1: ( 'entity' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8047:1: ( 'entity' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8048:1: 'entity'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEntityAccess().getEntityKeyword_0());
}
match(input,78,FollowSets000.FOLLOW_78_in_rule__Entity__Group__0__Impl17327); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getEntityAccess().getEntityKeyword_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Entity__Group__0__Impl"
// $ANTLR start "rule__Entity__Group__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8061:1: rule__Entity__Group__1 : rule__Entity__Group__1__Impl rule__Entity__Group__2 ;
public final void rule__Entity__Group__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8065:1: ( rule__Entity__Group__1__Impl rule__Entity__Group__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8066:2: rule__Entity__Group__1__Impl rule__Entity__Group__2
{
pushFollow(FollowSets000.FOLLOW_rule__Entity__Group__1__Impl_in_rule__Entity__Group__117358);
rule__Entity__Group__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets000.FOLLOW_rule__Entity__Group__2_in_rule__Entity__Group__117361);
rule__Entity__Group__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Entity__Group__1"
// $ANTLR start "rule__Entity__Group__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8073:1: rule__Entity__Group__1__Impl : ( ( rule__Entity__NameAssignment_1 ) ) ;
public final void rule__Entity__Group__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8077:1: ( ( ( rule__Entity__NameAssignment_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8078:1: ( ( rule__Entity__NameAssignment_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8078:1: ( ( rule__Entity__NameAssignment_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8079:1: ( rule__Entity__NameAssignment_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEntityAccess().getNameAssignment_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8080:1: ( rule__Entity__NameAssignment_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8080:2: rule__Entity__NameAssignment_1
{
pushFollow(FollowSets000.FOLLOW_rule__Entity__NameAssignment_1_in_rule__Entity__Group__1__Impl17388);
rule__Entity__NameAssignment_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getEntityAccess().getNameAssignment_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Entity__Group__1__Impl"
// $ANTLR start "rule__Entity__Group__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8090:1: rule__Entity__Group__2 : rule__Entity__Group__2__Impl rule__Entity__Group__3 ;
public final void rule__Entity__Group__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8094:1: ( rule__Entity__Group__2__Impl rule__Entity__Group__3 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8095:2: rule__Entity__Group__2__Impl rule__Entity__Group__3
{
pushFollow(FollowSets000.FOLLOW_rule__Entity__Group__2__Impl_in_rule__Entity__Group__217418);
rule__Entity__Group__2__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets000.FOLLOW_rule__Entity__Group__3_in_rule__Entity__Group__217421);
rule__Entity__Group__3();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Entity__Group__2"
// $ANTLR start "rule__Entity__Group__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8102:1: rule__Entity__Group__2__Impl : ( ( rule__Entity__Group_2__0 )? ) ;
public final void rule__Entity__Group__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8106:1: ( ( ( rule__Entity__Group_2__0 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8107:1: ( ( rule__Entity__Group_2__0 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8107:1: ( ( rule__Entity__Group_2__0 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8108:1: ( rule__Entity__Group_2__0 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEntityAccess().getGroup_2());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8109:1: ( rule__Entity__Group_2__0 )?
int alt66=2;
int LA66_0 = input.LA(1);
if ( (LA66_0==45) ) {
alt66=1;
}
switch (alt66) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8109:2: rule__Entity__Group_2__0
{
pushFollow(FollowSets000.FOLLOW_rule__Entity__Group_2__0_in_rule__Entity__Group__2__Impl17448);
rule__Entity__Group_2__0();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getEntityAccess().getGroup_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Entity__Group__2__Impl"
// $ANTLR start "rule__Entity__Group__3"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8119:1: rule__Entity__Group__3 : rule__Entity__Group__3__Impl rule__Entity__Group__4 ;
public final void rule__Entity__Group__3() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8123:1: ( rule__Entity__Group__3__Impl rule__Entity__Group__4 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8124:2: rule__Entity__Group__3__Impl rule__Entity__Group__4
{
pushFollow(FollowSets000.FOLLOW_rule__Entity__Group__3__Impl_in_rule__Entity__Group__317479);
rule__Entity__Group__3__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets000.FOLLOW_rule__Entity__Group__4_in_rule__Entity__Group__317482);
rule__Entity__Group__4();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Entity__Group__3"
// $ANTLR start "rule__Entity__Group__3__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8131:1: rule__Entity__Group__3__Impl : ( ( rule__Entity__Group_3__0 )? ) ;
public final void rule__Entity__Group__3__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8135:1: ( ( ( rule__Entity__Group_3__0 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8136:1: ( ( rule__Entity__Group_3__0 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8136:1: ( ( rule__Entity__Group_3__0 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8137:1: ( rule__Entity__Group_3__0 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEntityAccess().getGroup_3());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8138:1: ( rule__Entity__Group_3__0 )?
int alt67=2;
int LA67_0 = input.LA(1);
if ( (LA67_0==79) ) {
alt67=1;
}
switch (alt67) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8138:2: rule__Entity__Group_3__0
{
pushFollow(FollowSets000.FOLLOW_rule__Entity__Group_3__0_in_rule__Entity__Group__3__Impl17509);
rule__Entity__Group_3__0();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getEntityAccess().getGroup_3());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Entity__Group__3__Impl"
// $ANTLR start "rule__Entity__Group__4"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8148:1: rule__Entity__Group__4 : rule__Entity__Group__4__Impl rule__Entity__Group__5 ;
public final void rule__Entity__Group__4() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8152:1: ( rule__Entity__Group__4__Impl rule__Entity__Group__5 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8153:2: rule__Entity__Group__4__Impl rule__Entity__Group__5
{
pushFollow(FollowSets000.FOLLOW_rule__Entity__Group__4__Impl_in_rule__Entity__Group__417540);
rule__Entity__Group__4__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets000.FOLLOW_rule__Entity__Group__5_in_rule__Entity__Group__417543);
rule__Entity__Group__5();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Entity__Group__4"
// $ANTLR start "rule__Entity__Group__4__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8160:1: rule__Entity__Group__4__Impl : ( '{' ) ;
public final void rule__Entity__Group__4__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8164:1: ( ( '{' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8165:1: ( '{' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8165:1: ( '{' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8166:1: '{'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEntityAccess().getLeftCurlyBracketKeyword_4());
}
match(input,67,FollowSets000.FOLLOW_67_in_rule__Entity__Group__4__Impl17571); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getEntityAccess().getLeftCurlyBracketKeyword_4());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Entity__Group__4__Impl"
// $ANTLR start "rule__Entity__Group__5"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8179:1: rule__Entity__Group__5 : rule__Entity__Group__5__Impl rule__Entity__Group__6 ;
public final void rule__Entity__Group__5() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8183:1: ( rule__Entity__Group__5__Impl rule__Entity__Group__6 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8184:2: rule__Entity__Group__5__Impl rule__Entity__Group__6
{
pushFollow(FollowSets000.FOLLOW_rule__Entity__Group__5__Impl_in_rule__Entity__Group__517602);
rule__Entity__Group__5__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets000.FOLLOW_rule__Entity__Group__6_in_rule__Entity__Group__517605);
rule__Entity__Group__6();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Entity__Group__5"
// $ANTLR start "rule__Entity__Group__5__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8191:1: rule__Entity__Group__5__Impl : ( ( rule__Entity__EntityOptionsAssignment_5 )? ) ;
public final void rule__Entity__Group__5__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8195:1: ( ( ( rule__Entity__EntityOptionsAssignment_5 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8196:1: ( ( rule__Entity__EntityOptionsAssignment_5 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8196:1: ( ( rule__Entity__EntityOptionsAssignment_5 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8197:1: ( rule__Entity__EntityOptionsAssignment_5 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEntityAccess().getEntityOptionsAssignment_5());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8198:1: ( rule__Entity__EntityOptionsAssignment_5 )?
int alt68=2;
int LA68_0 = input.LA(1);
if ( (LA68_0==77) ) {
alt68=1;
}
switch (alt68) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8198:2: rule__Entity__EntityOptionsAssignment_5
{
pushFollow(FollowSets000.FOLLOW_rule__Entity__EntityOptionsAssignment_5_in_rule__Entity__Group__5__Impl17632);
rule__Entity__EntityOptionsAssignment_5();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getEntityAccess().getEntityOptionsAssignment_5());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Entity__Group__5__Impl"
// $ANTLR start "rule__Entity__Group__6"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8208:1: rule__Entity__Group__6 : rule__Entity__Group__6__Impl rule__Entity__Group__7 ;
public final void rule__Entity__Group__6() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8212:1: ( rule__Entity__Group__6__Impl rule__Entity__Group__7 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8213:2: rule__Entity__Group__6__Impl rule__Entity__Group__7
{
pushFollow(FollowSets000.FOLLOW_rule__Entity__Group__6__Impl_in_rule__Entity__Group__617663);
rule__Entity__Group__6__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets000.FOLLOW_rule__Entity__Group__7_in_rule__Entity__Group__617666);
rule__Entity__Group__7();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Entity__Group__6"
// $ANTLR start "rule__Entity__Group__6__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8220:1: rule__Entity__Group__6__Impl : ( ( rule__Entity__AttributesAssignment_6 )* ) ;
public final void rule__Entity__Group__6__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8224:1: ( ( ( rule__Entity__AttributesAssignment_6 )* ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8225:1: ( ( rule__Entity__AttributesAssignment_6 )* )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8225:1: ( ( rule__Entity__AttributesAssignment_6 )* )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8226:1: ( rule__Entity__AttributesAssignment_6 )*
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEntityAccess().getAttributesAssignment_6());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8227:1: ( rule__Entity__AttributesAssignment_6 )*
loop69:
do {
int alt69=2;
int LA69_0 = input.LA(1);
if ( ((LA69_0>=56 && LA69_0<=57)||(LA69_0>=59 && LA69_0<=60)||LA69_0==70||LA69_0==78||LA69_0==80||LA69_0==85||LA69_0==89||LA69_0==91||LA69_0==94||LA69_0==96||LA69_0==98) ) {
alt69=1;
}
switch (alt69) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8227:2: rule__Entity__AttributesAssignment_6
{
pushFollow(FollowSets000.FOLLOW_rule__Entity__AttributesAssignment_6_in_rule__Entity__Group__6__Impl17693);
rule__Entity__AttributesAssignment_6();
state._fsp--;
if (state.failed) return ;
}
break;
default :
break loop69;
}
} while (true);
if ( state.backtracking==0 ) {
after(grammarAccess.getEntityAccess().getAttributesAssignment_6());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Entity__Group__6__Impl"
// $ANTLR start "rule__Entity__Group__7"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8237:1: rule__Entity__Group__7 : rule__Entity__Group__7__Impl ;
public final void rule__Entity__Group__7() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8241:1: ( rule__Entity__Group__7__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8242:2: rule__Entity__Group__7__Impl
{
pushFollow(FollowSets000.FOLLOW_rule__Entity__Group__7__Impl_in_rule__Entity__Group__717724);
rule__Entity__Group__7__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Entity__Group__7"
// $ANTLR start "rule__Entity__Group__7__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8248:1: rule__Entity__Group__7__Impl : ( '}' ) ;
public final void rule__Entity__Group__7__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8252:1: ( ( '}' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8253:1: ( '}' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8253:1: ( '}' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8254:1: '}'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEntityAccess().getRightCurlyBracketKeyword_7());
}
match(input,68,FollowSets000.FOLLOW_68_in_rule__Entity__Group__7__Impl17752); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getEntityAccess().getRightCurlyBracketKeyword_7());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Entity__Group__7__Impl"
// $ANTLR start "rule__Entity__Group_2__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8283:1: rule__Entity__Group_2__0 : rule__Entity__Group_2__0__Impl rule__Entity__Group_2__1 ;
public final void rule__Entity__Group_2__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8287:1: ( rule__Entity__Group_2__0__Impl rule__Entity__Group_2__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8288:2: rule__Entity__Group_2__0__Impl rule__Entity__Group_2__1
{
pushFollow(FollowSets000.FOLLOW_rule__Entity__Group_2__0__Impl_in_rule__Entity__Group_2__017799);
rule__Entity__Group_2__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets000.FOLLOW_rule__Entity__Group_2__1_in_rule__Entity__Group_2__017802);
rule__Entity__Group_2__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Entity__Group_2__0"
// $ANTLR start "rule__Entity__Group_2__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8295:1: rule__Entity__Group_2__0__Impl : ( 'extends' ) ;
public final void rule__Entity__Group_2__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8299:1: ( ( 'extends' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8300:1: ( 'extends' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8300:1: ( 'extends' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8301:1: 'extends'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEntityAccess().getExtendsKeyword_2_0());
}
match(input,45,FollowSets000.FOLLOW_45_in_rule__Entity__Group_2__0__Impl17830); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getEntityAccess().getExtendsKeyword_2_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Entity__Group_2__0__Impl"
// $ANTLR start "rule__Entity__Group_2__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8314:1: rule__Entity__Group_2__1 : rule__Entity__Group_2__1__Impl ;
public final void rule__Entity__Group_2__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8318:1: ( rule__Entity__Group_2__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8319:2: rule__Entity__Group_2__1__Impl
{
pushFollow(FollowSets000.FOLLOW_rule__Entity__Group_2__1__Impl_in_rule__Entity__Group_2__117861);
rule__Entity__Group_2__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Entity__Group_2__1"
// $ANTLR start "rule__Entity__Group_2__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8325:1: rule__Entity__Group_2__1__Impl : ( ( rule__Entity__ExtendsAssignment_2_1 ) ) ;
public final void rule__Entity__Group_2__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8329:1: ( ( ( rule__Entity__ExtendsAssignment_2_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8330:1: ( ( rule__Entity__ExtendsAssignment_2_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8330:1: ( ( rule__Entity__ExtendsAssignment_2_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8331:1: ( rule__Entity__ExtendsAssignment_2_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEntityAccess().getExtendsAssignment_2_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8332:1: ( rule__Entity__ExtendsAssignment_2_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8332:2: rule__Entity__ExtendsAssignment_2_1
{
pushFollow(FollowSets000.FOLLOW_rule__Entity__ExtendsAssignment_2_1_in_rule__Entity__Group_2__1__Impl17888);
rule__Entity__ExtendsAssignment_2_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getEntityAccess().getExtendsAssignment_2_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Entity__Group_2__1__Impl"
// $ANTLR start "rule__Entity__Group_3__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8346:1: rule__Entity__Group_3__0 : rule__Entity__Group_3__0__Impl rule__Entity__Group_3__1 ;
public final void rule__Entity__Group_3__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8350:1: ( rule__Entity__Group_3__0__Impl rule__Entity__Group_3__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8351:2: rule__Entity__Group_3__0__Impl rule__Entity__Group_3__1
{
pushFollow(FollowSets000.FOLLOW_rule__Entity__Group_3__0__Impl_in_rule__Entity__Group_3__017922);
rule__Entity__Group_3__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets000.FOLLOW_rule__Entity__Group_3__1_in_rule__Entity__Group_3__017925);
rule__Entity__Group_3__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Entity__Group_3__0"
// $ANTLR start "rule__Entity__Group_3__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8358:1: rule__Entity__Group_3__0__Impl : ( 'jvmtype' ) ;
public final void rule__Entity__Group_3__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8362:1: ( ( 'jvmtype' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8363:1: ( 'jvmtype' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8363:1: ( 'jvmtype' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8364:1: 'jvmtype'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEntityAccess().getJvmtypeKeyword_3_0());
}
match(input,79,FollowSets000.FOLLOW_79_in_rule__Entity__Group_3__0__Impl17953); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getEntityAccess().getJvmtypeKeyword_3_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Entity__Group_3__0__Impl"
// $ANTLR start "rule__Entity__Group_3__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8377:1: rule__Entity__Group_3__1 : rule__Entity__Group_3__1__Impl ;
public final void rule__Entity__Group_3__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8381:1: ( rule__Entity__Group_3__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8382:2: rule__Entity__Group_3__1__Impl
{
pushFollow(FollowSets000.FOLLOW_rule__Entity__Group_3__1__Impl_in_rule__Entity__Group_3__117984);
rule__Entity__Group_3__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Entity__Group_3__1"
// $ANTLR start "rule__Entity__Group_3__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8388:1: rule__Entity__Group_3__1__Impl : ( ( rule__Entity__JvmtypeAssignment_3_1 ) ) ;
public final void rule__Entity__Group_3__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8392:1: ( ( ( rule__Entity__JvmtypeAssignment_3_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8393:1: ( ( rule__Entity__JvmtypeAssignment_3_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8393:1: ( ( rule__Entity__JvmtypeAssignment_3_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8394:1: ( rule__Entity__JvmtypeAssignment_3_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEntityAccess().getJvmtypeAssignment_3_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8395:1: ( rule__Entity__JvmtypeAssignment_3_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8395:2: rule__Entity__JvmtypeAssignment_3_1
{
pushFollow(FollowSets000.FOLLOW_rule__Entity__JvmtypeAssignment_3_1_in_rule__Entity__Group_3__1__Impl18011);
rule__Entity__JvmtypeAssignment_3_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getEntityAccess().getJvmtypeAssignment_3_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Entity__Group_3__1__Impl"
// $ANTLR start "rule__ValueObject__Group__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8409:1: rule__ValueObject__Group__0 : rule__ValueObject__Group__0__Impl rule__ValueObject__Group__1 ;
public final void rule__ValueObject__Group__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8413:1: ( rule__ValueObject__Group__0__Impl rule__ValueObject__Group__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8414:2: rule__ValueObject__Group__0__Impl rule__ValueObject__Group__1
{
pushFollow(FollowSets000.FOLLOW_rule__ValueObject__Group__0__Impl_in_rule__ValueObject__Group__018045);
rule__ValueObject__Group__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets000.FOLLOW_rule__ValueObject__Group__1_in_rule__ValueObject__Group__018048);
rule__ValueObject__Group__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ValueObject__Group__0"
// $ANTLR start "rule__ValueObject__Group__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8421:1: rule__ValueObject__Group__0__Impl : ( 'valueobject' ) ;
public final void rule__ValueObject__Group__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8425:1: ( ( 'valueobject' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8426:1: ( 'valueobject' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8426:1: ( 'valueobject' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8427:1: 'valueobject'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getValueObjectAccess().getValueobjectKeyword_0());
}
match(input,80,FollowSets000.FOLLOW_80_in_rule__ValueObject__Group__0__Impl18076); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getValueObjectAccess().getValueobjectKeyword_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ValueObject__Group__0__Impl"
// $ANTLR start "rule__ValueObject__Group__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8440:1: rule__ValueObject__Group__1 : rule__ValueObject__Group__1__Impl rule__ValueObject__Group__2 ;
public final void rule__ValueObject__Group__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8444:1: ( rule__ValueObject__Group__1__Impl rule__ValueObject__Group__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8445:2: rule__ValueObject__Group__1__Impl rule__ValueObject__Group__2
{
pushFollow(FollowSets000.FOLLOW_rule__ValueObject__Group__1__Impl_in_rule__ValueObject__Group__118107);
rule__ValueObject__Group__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets000.FOLLOW_rule__ValueObject__Group__2_in_rule__ValueObject__Group__118110);
rule__ValueObject__Group__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ValueObject__Group__1"
// $ANTLR start "rule__ValueObject__Group__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8452:1: rule__ValueObject__Group__1__Impl : ( ( rule__ValueObject__NameAssignment_1 ) ) ;
public final void rule__ValueObject__Group__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8456:1: ( ( ( rule__ValueObject__NameAssignment_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8457:1: ( ( rule__ValueObject__NameAssignment_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8457:1: ( ( rule__ValueObject__NameAssignment_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8458:1: ( rule__ValueObject__NameAssignment_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getValueObjectAccess().getNameAssignment_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8459:1: ( rule__ValueObject__NameAssignment_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8459:2: rule__ValueObject__NameAssignment_1
{
pushFollow(FollowSets000.FOLLOW_rule__ValueObject__NameAssignment_1_in_rule__ValueObject__Group__1__Impl18137);
rule__ValueObject__NameAssignment_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getValueObjectAccess().getNameAssignment_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ValueObject__Group__1__Impl"
// $ANTLR start "rule__ValueObject__Group__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8469:1: rule__ValueObject__Group__2 : rule__ValueObject__Group__2__Impl rule__ValueObject__Group__3 ;
public final void rule__ValueObject__Group__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8473:1: ( rule__ValueObject__Group__2__Impl rule__ValueObject__Group__3 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8474:2: rule__ValueObject__Group__2__Impl rule__ValueObject__Group__3
{
pushFollow(FollowSets000.FOLLOW_rule__ValueObject__Group__2__Impl_in_rule__ValueObject__Group__218167);
rule__ValueObject__Group__2__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets000.FOLLOW_rule__ValueObject__Group__3_in_rule__ValueObject__Group__218170);
rule__ValueObject__Group__3();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ValueObject__Group__2"
// $ANTLR start "rule__ValueObject__Group__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8481:1: rule__ValueObject__Group__2__Impl : ( ( rule__ValueObject__Group_2__0 )? ) ;
public final void rule__ValueObject__Group__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8485:1: ( ( ( rule__ValueObject__Group_2__0 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8486:1: ( ( rule__ValueObject__Group_2__0 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8486:1: ( ( rule__ValueObject__Group_2__0 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8487:1: ( rule__ValueObject__Group_2__0 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getValueObjectAccess().getGroup_2());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8488:1: ( rule__ValueObject__Group_2__0 )?
int alt70=2;
int LA70_0 = input.LA(1);
if ( (LA70_0==45) ) {
alt70=1;
}
switch (alt70) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8488:2: rule__ValueObject__Group_2__0
{
pushFollow(FollowSets000.FOLLOW_rule__ValueObject__Group_2__0_in_rule__ValueObject__Group__2__Impl18197);
rule__ValueObject__Group_2__0();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getValueObjectAccess().getGroup_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ValueObject__Group__2__Impl"
// $ANTLR start "rule__ValueObject__Group__3"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8498:1: rule__ValueObject__Group__3 : rule__ValueObject__Group__3__Impl rule__ValueObject__Group__4 ;
public final void rule__ValueObject__Group__3() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8502:1: ( rule__ValueObject__Group__3__Impl rule__ValueObject__Group__4 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8503:2: rule__ValueObject__Group__3__Impl rule__ValueObject__Group__4
{
pushFollow(FollowSets000.FOLLOW_rule__ValueObject__Group__3__Impl_in_rule__ValueObject__Group__318228);
rule__ValueObject__Group__3__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets000.FOLLOW_rule__ValueObject__Group__4_in_rule__ValueObject__Group__318231);
rule__ValueObject__Group__4();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ValueObject__Group__3"
// $ANTLR start "rule__ValueObject__Group__3__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8510:1: rule__ValueObject__Group__3__Impl : ( ( rule__ValueObject__Group_3__0 )? ) ;
public final void rule__ValueObject__Group__3__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8514:1: ( ( ( rule__ValueObject__Group_3__0 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8515:1: ( ( rule__ValueObject__Group_3__0 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8515:1: ( ( rule__ValueObject__Group_3__0 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8516:1: ( rule__ValueObject__Group_3__0 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getValueObjectAccess().getGroup_3());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8517:1: ( rule__ValueObject__Group_3__0 )?
int alt71=2;
int LA71_0 = input.LA(1);
if ( (LA71_0==79) ) {
alt71=1;
}
switch (alt71) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8517:2: rule__ValueObject__Group_3__0
{
pushFollow(FollowSets000.FOLLOW_rule__ValueObject__Group_3__0_in_rule__ValueObject__Group__3__Impl18258);
rule__ValueObject__Group_3__0();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getValueObjectAccess().getGroup_3());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ValueObject__Group__3__Impl"
// $ANTLR start "rule__ValueObject__Group__4"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8527:1: rule__ValueObject__Group__4 : rule__ValueObject__Group__4__Impl rule__ValueObject__Group__5 ;
public final void rule__ValueObject__Group__4() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8531:1: ( rule__ValueObject__Group__4__Impl rule__ValueObject__Group__5 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8532:2: rule__ValueObject__Group__4__Impl rule__ValueObject__Group__5
{
pushFollow(FollowSets000.FOLLOW_rule__ValueObject__Group__4__Impl_in_rule__ValueObject__Group__418289);
rule__ValueObject__Group__4__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets000.FOLLOW_rule__ValueObject__Group__5_in_rule__ValueObject__Group__418292);
rule__ValueObject__Group__5();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ValueObject__Group__4"
// $ANTLR start "rule__ValueObject__Group__4__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8539:1: rule__ValueObject__Group__4__Impl : ( '{' ) ;
public final void rule__ValueObject__Group__4__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8543:1: ( ( '{' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8544:1: ( '{' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8544:1: ( '{' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8545:1: '{'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getValueObjectAccess().getLeftCurlyBracketKeyword_4());
}
match(input,67,FollowSets000.FOLLOW_67_in_rule__ValueObject__Group__4__Impl18320); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getValueObjectAccess().getLeftCurlyBracketKeyword_4());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ValueObject__Group__4__Impl"
// $ANTLR start "rule__ValueObject__Group__5"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8558:1: rule__ValueObject__Group__5 : rule__ValueObject__Group__5__Impl rule__ValueObject__Group__6 ;
public final void rule__ValueObject__Group__5() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8562:1: ( rule__ValueObject__Group__5__Impl rule__ValueObject__Group__6 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8563:2: rule__ValueObject__Group__5__Impl rule__ValueObject__Group__6
{
pushFollow(FollowSets000.FOLLOW_rule__ValueObject__Group__5__Impl_in_rule__ValueObject__Group__518351);
rule__ValueObject__Group__5__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets000.FOLLOW_rule__ValueObject__Group__6_in_rule__ValueObject__Group__518354);
rule__ValueObject__Group__6();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ValueObject__Group__5"
// $ANTLR start "rule__ValueObject__Group__5__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8570:1: rule__ValueObject__Group__5__Impl : ( ( rule__ValueObject__AttributesAssignment_5 )* ) ;
public final void rule__ValueObject__Group__5__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8574:1: ( ( ( rule__ValueObject__AttributesAssignment_5 )* ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8575:1: ( ( rule__ValueObject__AttributesAssignment_5 )* )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8575:1: ( ( rule__ValueObject__AttributesAssignment_5 )* )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8576:1: ( rule__ValueObject__AttributesAssignment_5 )*
{
if ( state.backtracking==0 ) {
before(grammarAccess.getValueObjectAccess().getAttributesAssignment_5());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8577:1: ( rule__ValueObject__AttributesAssignment_5 )*
loop72:
do {
int alt72=2;
int LA72_0 = input.LA(1);
if ( ((LA72_0>=56 && LA72_0<=57)||(LA72_0>=59 && LA72_0<=60)||LA72_0==70||LA72_0==78||LA72_0==80||LA72_0==85||LA72_0==89||LA72_0==91||LA72_0==94||LA72_0==96||LA72_0==98) ) {
alt72=1;
}
switch (alt72) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8577:2: rule__ValueObject__AttributesAssignment_5
{
pushFollow(FollowSets000.FOLLOW_rule__ValueObject__AttributesAssignment_5_in_rule__ValueObject__Group__5__Impl18381);
rule__ValueObject__AttributesAssignment_5();
state._fsp--;
if (state.failed) return ;
}
break;
default :
break loop72;
}
} while (true);
if ( state.backtracking==0 ) {
after(grammarAccess.getValueObjectAccess().getAttributesAssignment_5());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ValueObject__Group__5__Impl"
// $ANTLR start "rule__ValueObject__Group__6"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8587:1: rule__ValueObject__Group__6 : rule__ValueObject__Group__6__Impl ;
public final void rule__ValueObject__Group__6() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8591:1: ( rule__ValueObject__Group__6__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8592:2: rule__ValueObject__Group__6__Impl
{
pushFollow(FollowSets000.FOLLOW_rule__ValueObject__Group__6__Impl_in_rule__ValueObject__Group__618412);
rule__ValueObject__Group__6__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ValueObject__Group__6"
// $ANTLR start "rule__ValueObject__Group__6__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8598:1: rule__ValueObject__Group__6__Impl : ( '}' ) ;
public final void rule__ValueObject__Group__6__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8602:1: ( ( '}' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8603:1: ( '}' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8603:1: ( '}' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8604:1: '}'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getValueObjectAccess().getRightCurlyBracketKeyword_6());
}
match(input,68,FollowSets000.FOLLOW_68_in_rule__ValueObject__Group__6__Impl18440); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getValueObjectAccess().getRightCurlyBracketKeyword_6());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ValueObject__Group__6__Impl"
// $ANTLR start "rule__ValueObject__Group_2__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8631:1: rule__ValueObject__Group_2__0 : rule__ValueObject__Group_2__0__Impl rule__ValueObject__Group_2__1 ;
public final void rule__ValueObject__Group_2__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8635:1: ( rule__ValueObject__Group_2__0__Impl rule__ValueObject__Group_2__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8636:2: rule__ValueObject__Group_2__0__Impl rule__ValueObject__Group_2__1
{
pushFollow(FollowSets000.FOLLOW_rule__ValueObject__Group_2__0__Impl_in_rule__ValueObject__Group_2__018485);
rule__ValueObject__Group_2__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets000.FOLLOW_rule__ValueObject__Group_2__1_in_rule__ValueObject__Group_2__018488);
rule__ValueObject__Group_2__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ValueObject__Group_2__0"
// $ANTLR start "rule__ValueObject__Group_2__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8643:1: rule__ValueObject__Group_2__0__Impl : ( 'extends' ) ;
public final void rule__ValueObject__Group_2__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8647:1: ( ( 'extends' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8648:1: ( 'extends' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8648:1: ( 'extends' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8649:1: 'extends'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getValueObjectAccess().getExtendsKeyword_2_0());
}
match(input,45,FollowSets000.FOLLOW_45_in_rule__ValueObject__Group_2__0__Impl18516); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getValueObjectAccess().getExtendsKeyword_2_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ValueObject__Group_2__0__Impl"
// $ANTLR start "rule__ValueObject__Group_2__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8662:1: rule__ValueObject__Group_2__1 : rule__ValueObject__Group_2__1__Impl ;
public final void rule__ValueObject__Group_2__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8666:1: ( rule__ValueObject__Group_2__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8667:2: rule__ValueObject__Group_2__1__Impl
{
pushFollow(FollowSets000.FOLLOW_rule__ValueObject__Group_2__1__Impl_in_rule__ValueObject__Group_2__118547);
rule__ValueObject__Group_2__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ValueObject__Group_2__1"
// $ANTLR start "rule__ValueObject__Group_2__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8673:1: rule__ValueObject__Group_2__1__Impl : ( ( rule__ValueObject__ExtendsAssignment_2_1 ) ) ;
public final void rule__ValueObject__Group_2__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8677:1: ( ( ( rule__ValueObject__ExtendsAssignment_2_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8678:1: ( ( rule__ValueObject__ExtendsAssignment_2_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8678:1: ( ( rule__ValueObject__ExtendsAssignment_2_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8679:1: ( rule__ValueObject__ExtendsAssignment_2_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getValueObjectAccess().getExtendsAssignment_2_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8680:1: ( rule__ValueObject__ExtendsAssignment_2_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8680:2: rule__ValueObject__ExtendsAssignment_2_1
{
pushFollow(FollowSets000.FOLLOW_rule__ValueObject__ExtendsAssignment_2_1_in_rule__ValueObject__Group_2__1__Impl18574);
rule__ValueObject__ExtendsAssignment_2_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getValueObjectAccess().getExtendsAssignment_2_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ValueObject__Group_2__1__Impl"
// $ANTLR start "rule__ValueObject__Group_3__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8694:1: rule__ValueObject__Group_3__0 : rule__ValueObject__Group_3__0__Impl rule__ValueObject__Group_3__1 ;
public final void rule__ValueObject__Group_3__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8698:1: ( rule__ValueObject__Group_3__0__Impl rule__ValueObject__Group_3__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8699:2: rule__ValueObject__Group_3__0__Impl rule__ValueObject__Group_3__1
{
pushFollow(FollowSets000.FOLLOW_rule__ValueObject__Group_3__0__Impl_in_rule__ValueObject__Group_3__018608);
rule__ValueObject__Group_3__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets000.FOLLOW_rule__ValueObject__Group_3__1_in_rule__ValueObject__Group_3__018611);
rule__ValueObject__Group_3__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ValueObject__Group_3__0"
// $ANTLR start "rule__ValueObject__Group_3__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8706:1: rule__ValueObject__Group_3__0__Impl : ( 'jvmtype' ) ;
public final void rule__ValueObject__Group_3__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8710:1: ( ( 'jvmtype' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8711:1: ( 'jvmtype' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8711:1: ( 'jvmtype' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8712:1: 'jvmtype'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getValueObjectAccess().getJvmtypeKeyword_3_0());
}
match(input,79,FollowSets000.FOLLOW_79_in_rule__ValueObject__Group_3__0__Impl18639); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getValueObjectAccess().getJvmtypeKeyword_3_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ValueObject__Group_3__0__Impl"
// $ANTLR start "rule__ValueObject__Group_3__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8725:1: rule__ValueObject__Group_3__1 : rule__ValueObject__Group_3__1__Impl ;
public final void rule__ValueObject__Group_3__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8729:1: ( rule__ValueObject__Group_3__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8730:2: rule__ValueObject__Group_3__1__Impl
{
pushFollow(FollowSets000.FOLLOW_rule__ValueObject__Group_3__1__Impl_in_rule__ValueObject__Group_3__118670);
rule__ValueObject__Group_3__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ValueObject__Group_3__1"
// $ANTLR start "rule__ValueObject__Group_3__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8736:1: rule__ValueObject__Group_3__1__Impl : ( ( rule__ValueObject__JvmtypeAssignment_3_1 ) ) ;
public final void rule__ValueObject__Group_3__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8740:1: ( ( ( rule__ValueObject__JvmtypeAssignment_3_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8741:1: ( ( rule__ValueObject__JvmtypeAssignment_3_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8741:1: ( ( rule__ValueObject__JvmtypeAssignment_3_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8742:1: ( rule__ValueObject__JvmtypeAssignment_3_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getValueObjectAccess().getJvmtypeAssignment_3_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8743:1: ( rule__ValueObject__JvmtypeAssignment_3_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8743:2: rule__ValueObject__JvmtypeAssignment_3_1
{
pushFollow(FollowSets000.FOLLOW_rule__ValueObject__JvmtypeAssignment_3_1_in_rule__ValueObject__Group_3__1__Impl18697);
rule__ValueObject__JvmtypeAssignment_3_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getValueObjectAccess().getJvmtypeAssignment_3_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ValueObject__Group_3__1__Impl"
// $ANTLR start "rule__BaseDataType__Group__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8759:1: rule__BaseDataType__Group__0 : rule__BaseDataType__Group__0__Impl rule__BaseDataType__Group__1 ;
public final void rule__BaseDataType__Group__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8763:1: ( rule__BaseDataType__Group__0__Impl rule__BaseDataType__Group__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8764:2: rule__BaseDataType__Group__0__Impl rule__BaseDataType__Group__1
{
pushFollow(FollowSets000.FOLLOW_rule__BaseDataType__Group__0__Impl_in_rule__BaseDataType__Group__018733);
rule__BaseDataType__Group__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets000.FOLLOW_rule__BaseDataType__Group__1_in_rule__BaseDataType__Group__018736);
rule__BaseDataType__Group__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__BaseDataType__Group__0"
// $ANTLR start "rule__BaseDataType__Group__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8771:1: rule__BaseDataType__Group__0__Impl : ( () ) ;
public final void rule__BaseDataType__Group__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8775:1: ( ( () ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8776:1: ( () )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8776:1: ( () )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8777:1: ()
{
if ( state.backtracking==0 ) {
before(grammarAccess.getBaseDataTypeAccess().getBaseDataTypeAction_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8778:1: ()
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8780:1:
{
}
if ( state.backtracking==0 ) {
after(grammarAccess.getBaseDataTypeAccess().getBaseDataTypeAction_0());
}
}
}
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__BaseDataType__Group__0__Impl"
// $ANTLR start "rule__BaseDataType__Group__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8790:1: rule__BaseDataType__Group__1 : rule__BaseDataType__Group__1__Impl ;
public final void rule__BaseDataType__Group__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8794:1: ( rule__BaseDataType__Group__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8795:2: rule__BaseDataType__Group__1__Impl
{
pushFollow(FollowSets000.FOLLOW_rule__BaseDataType__Group__1__Impl_in_rule__BaseDataType__Group__118794);
rule__BaseDataType__Group__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__BaseDataType__Group__1"
// $ANTLR start "rule__BaseDataType__Group__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8801:1: rule__BaseDataType__Group__1__Impl : ( ( rule__BaseDataType__BaseDatatypePropertiesAssignment_1 )* ) ;
public final void rule__BaseDataType__Group__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8805:1: ( ( ( rule__BaseDataType__BaseDatatypePropertiesAssignment_1 )* ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8806:1: ( ( rule__BaseDataType__BaseDatatypePropertiesAssignment_1 )* )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8806:1: ( ( rule__BaseDataType__BaseDatatypePropertiesAssignment_1 )* )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8807:1: ( rule__BaseDataType__BaseDatatypePropertiesAssignment_1 )*
{
if ( state.backtracking==0 ) {
before(grammarAccess.getBaseDataTypeAccess().getBaseDatatypePropertiesAssignment_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8808:1: ( rule__BaseDataType__BaseDatatypePropertiesAssignment_1 )*
loop73:
do {
int alt73=2;
int LA73_0 = input.LA(1);
if ( (LA73_0==75||LA73_0==81) ) {
alt73=1;
}
switch (alt73) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8808:2: rule__BaseDataType__BaseDatatypePropertiesAssignment_1
{
pushFollow(FollowSets000.FOLLOW_rule__BaseDataType__BaseDatatypePropertiesAssignment_1_in_rule__BaseDataType__Group__1__Impl18821);
rule__BaseDataType__BaseDatatypePropertiesAssignment_1();
state._fsp--;
if (state.failed) return ;
}
break;
default :
break loop73;
}
} while (true);
if ( state.backtracking==0 ) {
after(grammarAccess.getBaseDataTypeAccess().getBaseDatatypePropertiesAssignment_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__BaseDataType__Group__1__Impl"
// $ANTLR start "rule__BaseDataTypeWidth__Group__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8822:1: rule__BaseDataTypeWidth__Group__0 : rule__BaseDataTypeWidth__Group__0__Impl rule__BaseDataTypeWidth__Group__1 ;
public final void rule__BaseDataTypeWidth__Group__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8826:1: ( rule__BaseDataTypeWidth__Group__0__Impl rule__BaseDataTypeWidth__Group__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8827:2: rule__BaseDataTypeWidth__Group__0__Impl rule__BaseDataTypeWidth__Group__1
{
pushFollow(FollowSets000.FOLLOW_rule__BaseDataTypeWidth__Group__0__Impl_in_rule__BaseDataTypeWidth__Group__018856);
rule__BaseDataTypeWidth__Group__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets000.FOLLOW_rule__BaseDataTypeWidth__Group__1_in_rule__BaseDataTypeWidth__Group__018859);
rule__BaseDataTypeWidth__Group__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__BaseDataTypeWidth__Group__0"
// $ANTLR start "rule__BaseDataTypeWidth__Group__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8834:1: rule__BaseDataTypeWidth__Group__0__Impl : ( () ) ;
public final void rule__BaseDataTypeWidth__Group__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8838:1: ( ( () ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8839:1: ( () )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8839:1: ( () )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8840:1: ()
{
if ( state.backtracking==0 ) {
before(grammarAccess.getBaseDataTypeWidthAccess().getBaseDataTypeWidthAction_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8841:1: ()
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8843:1:
{
}
if ( state.backtracking==0 ) {
after(grammarAccess.getBaseDataTypeWidthAccess().getBaseDataTypeWidthAction_0());
}
}
}
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__BaseDataTypeWidth__Group__0__Impl"
// $ANTLR start "rule__BaseDataTypeWidth__Group__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8853:1: rule__BaseDataTypeWidth__Group__1 : rule__BaseDataTypeWidth__Group__1__Impl rule__BaseDataTypeWidth__Group__2 ;
public final void rule__BaseDataTypeWidth__Group__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8857:1: ( rule__BaseDataTypeWidth__Group__1__Impl rule__BaseDataTypeWidth__Group__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8858:2: rule__BaseDataTypeWidth__Group__1__Impl rule__BaseDataTypeWidth__Group__2
{
pushFollow(FollowSets000.FOLLOW_rule__BaseDataTypeWidth__Group__1__Impl_in_rule__BaseDataTypeWidth__Group__118917);
rule__BaseDataTypeWidth__Group__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets000.FOLLOW_rule__BaseDataTypeWidth__Group__2_in_rule__BaseDataTypeWidth__Group__118920);
rule__BaseDataTypeWidth__Group__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__BaseDataTypeWidth__Group__1"
// $ANTLR start "rule__BaseDataTypeWidth__Group__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8865:1: rule__BaseDataTypeWidth__Group__1__Impl : ( 'width' ) ;
public final void rule__BaseDataTypeWidth__Group__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8869:1: ( ( 'width' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8870:1: ( 'width' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8870:1: ( 'width' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8871:1: 'width'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getBaseDataTypeWidthAccess().getWidthKeyword_1());
}
match(input,81,FollowSets000.FOLLOW_81_in_rule__BaseDataTypeWidth__Group__1__Impl18948); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getBaseDataTypeWidthAccess().getWidthKeyword_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__BaseDataTypeWidth__Group__1__Impl"
// $ANTLR start "rule__BaseDataTypeWidth__Group__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8884:1: rule__BaseDataTypeWidth__Group__2 : rule__BaseDataTypeWidth__Group__2__Impl ;
public final void rule__BaseDataTypeWidth__Group__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8888:1: ( rule__BaseDataTypeWidth__Group__2__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8889:2: rule__BaseDataTypeWidth__Group__2__Impl
{
pushFollow(FollowSets000.FOLLOW_rule__BaseDataTypeWidth__Group__2__Impl_in_rule__BaseDataTypeWidth__Group__218979);
rule__BaseDataTypeWidth__Group__2__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__BaseDataTypeWidth__Group__2"
// $ANTLR start "rule__BaseDataTypeWidth__Group__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8895:1: rule__BaseDataTypeWidth__Group__2__Impl : ( ( rule__BaseDataTypeWidth__WidthAssignment_2 )? ) ;
public final void rule__BaseDataTypeWidth__Group__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8899:1: ( ( ( rule__BaseDataTypeWidth__WidthAssignment_2 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8900:1: ( ( rule__BaseDataTypeWidth__WidthAssignment_2 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8900:1: ( ( rule__BaseDataTypeWidth__WidthAssignment_2 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8901:1: ( rule__BaseDataTypeWidth__WidthAssignment_2 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getBaseDataTypeWidthAccess().getWidthAssignment_2());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8902:1: ( rule__BaseDataTypeWidth__WidthAssignment_2 )?
int alt74=2;
int LA74_0 = input.LA(1);
if ( (LA74_0==RULE_INT) ) {
alt74=1;
}
switch (alt74) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8902:2: rule__BaseDataTypeWidth__WidthAssignment_2
{
pushFollow(FollowSets000.FOLLOW_rule__BaseDataTypeWidth__WidthAssignment_2_in_rule__BaseDataTypeWidth__Group__2__Impl19006);
rule__BaseDataTypeWidth__WidthAssignment_2();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getBaseDataTypeWidthAccess().getWidthAssignment_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__BaseDataTypeWidth__Group__2__Impl"
// $ANTLR start "rule__BaseDataTypeLabel__Group__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8918:1: rule__BaseDataTypeLabel__Group__0 : rule__BaseDataTypeLabel__Group__0__Impl rule__BaseDataTypeLabel__Group__1 ;
public final void rule__BaseDataTypeLabel__Group__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8922:1: ( rule__BaseDataTypeLabel__Group__0__Impl rule__BaseDataTypeLabel__Group__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8923:2: rule__BaseDataTypeLabel__Group__0__Impl rule__BaseDataTypeLabel__Group__1
{
pushFollow(FollowSets000.FOLLOW_rule__BaseDataTypeLabel__Group__0__Impl_in_rule__BaseDataTypeLabel__Group__019043);
rule__BaseDataTypeLabel__Group__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets000.FOLLOW_rule__BaseDataTypeLabel__Group__1_in_rule__BaseDataTypeLabel__Group__019046);
rule__BaseDataTypeLabel__Group__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__BaseDataTypeLabel__Group__0"
// $ANTLR start "rule__BaseDataTypeLabel__Group__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8930:1: rule__BaseDataTypeLabel__Group__0__Impl : ( () ) ;
public final void rule__BaseDataTypeLabel__Group__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8934:1: ( ( () ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8935:1: ( () )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8935:1: ( () )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8936:1: ()
{
if ( state.backtracking==0 ) {
before(grammarAccess.getBaseDataTypeLabelAccess().getBaseDataTypeLabelAction_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8937:1: ()
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8939:1:
{
}
if ( state.backtracking==0 ) {
after(grammarAccess.getBaseDataTypeLabelAccess().getBaseDataTypeLabelAction_0());
}
}
}
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__BaseDataTypeLabel__Group__0__Impl"
// $ANTLR start "rule__BaseDataTypeLabel__Group__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8949:1: rule__BaseDataTypeLabel__Group__1 : rule__BaseDataTypeLabel__Group__1__Impl rule__BaseDataTypeLabel__Group__2 ;
public final void rule__BaseDataTypeLabel__Group__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8953:1: ( rule__BaseDataTypeLabel__Group__1__Impl rule__BaseDataTypeLabel__Group__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8954:2: rule__BaseDataTypeLabel__Group__1__Impl rule__BaseDataTypeLabel__Group__2
{
pushFollow(FollowSets000.FOLLOW_rule__BaseDataTypeLabel__Group__1__Impl_in_rule__BaseDataTypeLabel__Group__119104);
rule__BaseDataTypeLabel__Group__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets000.FOLLOW_rule__BaseDataTypeLabel__Group__2_in_rule__BaseDataTypeLabel__Group__119107);
rule__BaseDataTypeLabel__Group__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__BaseDataTypeLabel__Group__1"
// $ANTLR start "rule__BaseDataTypeLabel__Group__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8961:1: rule__BaseDataTypeLabel__Group__1__Impl : ( 'label' ) ;
public final void rule__BaseDataTypeLabel__Group__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8965:1: ( ( 'label' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8966:1: ( 'label' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8966:1: ( 'label' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8967:1: 'label'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getBaseDataTypeLabelAccess().getLabelKeyword_1());
}
match(input,75,FollowSets000.FOLLOW_75_in_rule__BaseDataTypeLabel__Group__1__Impl19135); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getBaseDataTypeLabelAccess().getLabelKeyword_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__BaseDataTypeLabel__Group__1__Impl"
// $ANTLR start "rule__BaseDataTypeLabel__Group__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8980:1: rule__BaseDataTypeLabel__Group__2 : rule__BaseDataTypeLabel__Group__2__Impl ;
public final void rule__BaseDataTypeLabel__Group__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8984:1: ( rule__BaseDataTypeLabel__Group__2__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8985:2: rule__BaseDataTypeLabel__Group__2__Impl
{
pushFollow(FollowSets000.FOLLOW_rule__BaseDataTypeLabel__Group__2__Impl_in_rule__BaseDataTypeLabel__Group__219166);
rule__BaseDataTypeLabel__Group__2__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__BaseDataTypeLabel__Group__2"
// $ANTLR start "rule__BaseDataTypeLabel__Group__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8991:1: rule__BaseDataTypeLabel__Group__2__Impl : ( ( rule__BaseDataTypeLabel__LabelAssignment_2 ) ) ;
public final void rule__BaseDataTypeLabel__Group__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8995:1: ( ( ( rule__BaseDataTypeLabel__LabelAssignment_2 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8996:1: ( ( rule__BaseDataTypeLabel__LabelAssignment_2 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8996:1: ( ( rule__BaseDataTypeLabel__LabelAssignment_2 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8997:1: ( rule__BaseDataTypeLabel__LabelAssignment_2 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getBaseDataTypeLabelAccess().getLabelAssignment_2());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8998:1: ( rule__BaseDataTypeLabel__LabelAssignment_2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:8998:2: rule__BaseDataTypeLabel__LabelAssignment_2
{
pushFollow(FollowSets000.FOLLOW_rule__BaseDataTypeLabel__LabelAssignment_2_in_rule__BaseDataTypeLabel__Group__2__Impl19193);
rule__BaseDataTypeLabel__LabelAssignment_2();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getBaseDataTypeLabelAccess().getLabelAssignment_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__BaseDataTypeLabel__Group__2__Impl"
// $ANTLR start "rule__StringDataType__Group__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9014:1: rule__StringDataType__Group__0 : rule__StringDataType__Group__0__Impl rule__StringDataType__Group__1 ;
public final void rule__StringDataType__Group__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9018:1: ( rule__StringDataType__Group__0__Impl rule__StringDataType__Group__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9019:2: rule__StringDataType__Group__0__Impl rule__StringDataType__Group__1
{
pushFollow(FollowSets000.FOLLOW_rule__StringDataType__Group__0__Impl_in_rule__StringDataType__Group__019229);
rule__StringDataType__Group__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets000.FOLLOW_rule__StringDataType__Group__1_in_rule__StringDataType__Group__019232);
rule__StringDataType__Group__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__StringDataType__Group__0"
// $ANTLR start "rule__StringDataType__Group__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9026:1: rule__StringDataType__Group__0__Impl : ( 'stringdatatype' ) ;
public final void rule__StringDataType__Group__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9030:1: ( ( 'stringdatatype' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9031:1: ( 'stringdatatype' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9031:1: ( 'stringdatatype' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9032:1: 'stringdatatype'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getStringDataTypeAccess().getStringdatatypeKeyword_0());
}
match(input,82,FollowSets000.FOLLOW_82_in_rule__StringDataType__Group__0__Impl19260); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getStringDataTypeAccess().getStringdatatypeKeyword_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__StringDataType__Group__0__Impl"
// $ANTLR start "rule__StringDataType__Group__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9045:1: rule__StringDataType__Group__1 : rule__StringDataType__Group__1__Impl rule__StringDataType__Group__2 ;
public final void rule__StringDataType__Group__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9049:1: ( rule__StringDataType__Group__1__Impl rule__StringDataType__Group__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9050:2: rule__StringDataType__Group__1__Impl rule__StringDataType__Group__2
{
pushFollow(FollowSets000.FOLLOW_rule__StringDataType__Group__1__Impl_in_rule__StringDataType__Group__119291);
rule__StringDataType__Group__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets000.FOLLOW_rule__StringDataType__Group__2_in_rule__StringDataType__Group__119294);
rule__StringDataType__Group__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__StringDataType__Group__1"
// $ANTLR start "rule__StringDataType__Group__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9057:1: rule__StringDataType__Group__1__Impl : ( ( rule__StringDataType__NameAssignment_1 ) ) ;
public final void rule__StringDataType__Group__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9061:1: ( ( ( rule__StringDataType__NameAssignment_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9062:1: ( ( rule__StringDataType__NameAssignment_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9062:1: ( ( rule__StringDataType__NameAssignment_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9063:1: ( rule__StringDataType__NameAssignment_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getStringDataTypeAccess().getNameAssignment_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9064:1: ( rule__StringDataType__NameAssignment_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9064:2: rule__StringDataType__NameAssignment_1
{
pushFollow(FollowSets000.FOLLOW_rule__StringDataType__NameAssignment_1_in_rule__StringDataType__Group__1__Impl19321);
rule__StringDataType__NameAssignment_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getStringDataTypeAccess().getNameAssignment_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__StringDataType__Group__1__Impl"
// $ANTLR start "rule__StringDataType__Group__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9074:1: rule__StringDataType__Group__2 : rule__StringDataType__Group__2__Impl rule__StringDataType__Group__3 ;
public final void rule__StringDataType__Group__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9078:1: ( rule__StringDataType__Group__2__Impl rule__StringDataType__Group__3 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9079:2: rule__StringDataType__Group__2__Impl rule__StringDataType__Group__3
{
pushFollow(FollowSets000.FOLLOW_rule__StringDataType__Group__2__Impl_in_rule__StringDataType__Group__219351);
rule__StringDataType__Group__2__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets000.FOLLOW_rule__StringDataType__Group__3_in_rule__StringDataType__Group__219354);
rule__StringDataType__Group__3();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__StringDataType__Group__2"
// $ANTLR start "rule__StringDataType__Group__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9086:1: rule__StringDataType__Group__2__Impl : ( ( rule__StringDataType__Group_2__0 )? ) ;
public final void rule__StringDataType__Group__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9090:1: ( ( ( rule__StringDataType__Group_2__0 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9091:1: ( ( rule__StringDataType__Group_2__0 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9091:1: ( ( rule__StringDataType__Group_2__0 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9092:1: ( rule__StringDataType__Group_2__0 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getStringDataTypeAccess().getGroup_2());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9093:1: ( rule__StringDataType__Group_2__0 )?
int alt75=2;
int LA75_0 = input.LA(1);
if ( (LA75_0==45) ) {
alt75=1;
}
switch (alt75) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9093:2: rule__StringDataType__Group_2__0
{
pushFollow(FollowSets000.FOLLOW_rule__StringDataType__Group_2__0_in_rule__StringDataType__Group__2__Impl19381);
rule__StringDataType__Group_2__0();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getStringDataTypeAccess().getGroup_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__StringDataType__Group__2__Impl"
// $ANTLR start "rule__StringDataType__Group__3"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9103:1: rule__StringDataType__Group__3 : rule__StringDataType__Group__3__Impl rule__StringDataType__Group__4 ;
public final void rule__StringDataType__Group__3() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9107:1: ( rule__StringDataType__Group__3__Impl rule__StringDataType__Group__4 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9108:2: rule__StringDataType__Group__3__Impl rule__StringDataType__Group__4
{
pushFollow(FollowSets000.FOLLOW_rule__StringDataType__Group__3__Impl_in_rule__StringDataType__Group__319412);
rule__StringDataType__Group__3__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets000.FOLLOW_rule__StringDataType__Group__4_in_rule__StringDataType__Group__319415);
rule__StringDataType__Group__4();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__StringDataType__Group__3"
// $ANTLR start "rule__StringDataType__Group__3__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9115:1: rule__StringDataType__Group__3__Impl : ( '{' ) ;
public final void rule__StringDataType__Group__3__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9119:1: ( ( '{' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9120:1: ( '{' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9120:1: ( '{' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9121:1: '{'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getStringDataTypeAccess().getLeftCurlyBracketKeyword_3());
}
match(input,67,FollowSets000.FOLLOW_67_in_rule__StringDataType__Group__3__Impl19443); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getStringDataTypeAccess().getLeftCurlyBracketKeyword_3());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__StringDataType__Group__3__Impl"
// $ANTLR start "rule__StringDataType__Group__4"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9134:1: rule__StringDataType__Group__4 : rule__StringDataType__Group__4__Impl rule__StringDataType__Group__5 ;
public final void rule__StringDataType__Group__4() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9138:1: ( rule__StringDataType__Group__4__Impl rule__StringDataType__Group__5 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9139:2: rule__StringDataType__Group__4__Impl rule__StringDataType__Group__5
{
pushFollow(FollowSets000.FOLLOW_rule__StringDataType__Group__4__Impl_in_rule__StringDataType__Group__419474);
rule__StringDataType__Group__4__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets000.FOLLOW_rule__StringDataType__Group__5_in_rule__StringDataType__Group__419477);
rule__StringDataType__Group__5();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__StringDataType__Group__4"
// $ANTLR start "rule__StringDataType__Group__4__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9146:1: rule__StringDataType__Group__4__Impl : ( ( rule__StringDataType__BaseDataTypeAssignment_4 ) ) ;
public final void rule__StringDataType__Group__4__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9150:1: ( ( ( rule__StringDataType__BaseDataTypeAssignment_4 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9151:1: ( ( rule__StringDataType__BaseDataTypeAssignment_4 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9151:1: ( ( rule__StringDataType__BaseDataTypeAssignment_4 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9152:1: ( rule__StringDataType__BaseDataTypeAssignment_4 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getStringDataTypeAccess().getBaseDataTypeAssignment_4());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9153:1: ( rule__StringDataType__BaseDataTypeAssignment_4 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9153:2: rule__StringDataType__BaseDataTypeAssignment_4
{
pushFollow(FollowSets000.FOLLOW_rule__StringDataType__BaseDataTypeAssignment_4_in_rule__StringDataType__Group__4__Impl19504);
rule__StringDataType__BaseDataTypeAssignment_4();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getStringDataTypeAccess().getBaseDataTypeAssignment_4());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__StringDataType__Group__4__Impl"
// $ANTLR start "rule__StringDataType__Group__5"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9163:1: rule__StringDataType__Group__5 : rule__StringDataType__Group__5__Impl rule__StringDataType__Group__6 ;
public final void rule__StringDataType__Group__5() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9167:1: ( rule__StringDataType__Group__5__Impl rule__StringDataType__Group__6 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9168:2: rule__StringDataType__Group__5__Impl rule__StringDataType__Group__6
{
pushFollow(FollowSets000.FOLLOW_rule__StringDataType__Group__5__Impl_in_rule__StringDataType__Group__519534);
rule__StringDataType__Group__5__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets000.FOLLOW_rule__StringDataType__Group__6_in_rule__StringDataType__Group__519537);
rule__StringDataType__Group__6();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__StringDataType__Group__5"
// $ANTLR start "rule__StringDataType__Group__5__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9175:1: rule__StringDataType__Group__5__Impl : ( ( rule__StringDataType__Group_5__0 )? ) ;
public final void rule__StringDataType__Group__5__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9179:1: ( ( ( rule__StringDataType__Group_5__0 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9180:1: ( ( rule__StringDataType__Group_5__0 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9180:1: ( ( rule__StringDataType__Group_5__0 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9181:1: ( rule__StringDataType__Group_5__0 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getStringDataTypeAccess().getGroup_5());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9182:1: ( rule__StringDataType__Group_5__0 )?
int alt76=2;
int LA76_0 = input.LA(1);
if ( (LA76_0==83) ) {
alt76=1;
}
switch (alt76) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9182:2: rule__StringDataType__Group_5__0
{
pushFollow(FollowSets000.FOLLOW_rule__StringDataType__Group_5__0_in_rule__StringDataType__Group__5__Impl19564);
rule__StringDataType__Group_5__0();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getStringDataTypeAccess().getGroup_5());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__StringDataType__Group__5__Impl"
// $ANTLR start "rule__StringDataType__Group__6"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9192:1: rule__StringDataType__Group__6 : rule__StringDataType__Group__6__Impl rule__StringDataType__Group__7 ;
public final void rule__StringDataType__Group__6() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9196:1: ( rule__StringDataType__Group__6__Impl rule__StringDataType__Group__7 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9197:2: rule__StringDataType__Group__6__Impl rule__StringDataType__Group__7
{
pushFollow(FollowSets000.FOLLOW_rule__StringDataType__Group__6__Impl_in_rule__StringDataType__Group__619595);
rule__StringDataType__Group__6__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets000.FOLLOW_rule__StringDataType__Group__7_in_rule__StringDataType__Group__619598);
rule__StringDataType__Group__7();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__StringDataType__Group__6"
// $ANTLR start "rule__StringDataType__Group__6__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9204:1: rule__StringDataType__Group__6__Impl : ( ( rule__StringDataType__Group_6__0 )? ) ;
public final void rule__StringDataType__Group__6__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9208:1: ( ( ( rule__StringDataType__Group_6__0 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9209:1: ( ( rule__StringDataType__Group_6__0 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9209:1: ( ( rule__StringDataType__Group_6__0 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9210:1: ( rule__StringDataType__Group_6__0 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getStringDataTypeAccess().getGroup_6());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9211:1: ( rule__StringDataType__Group_6__0 )?
int alt77=2;
int LA77_0 = input.LA(1);
if ( (LA77_0==84) ) {
alt77=1;
}
switch (alt77) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9211:2: rule__StringDataType__Group_6__0
{
pushFollow(FollowSets000.FOLLOW_rule__StringDataType__Group_6__0_in_rule__StringDataType__Group__6__Impl19625);
rule__StringDataType__Group_6__0();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getStringDataTypeAccess().getGroup_6());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__StringDataType__Group__6__Impl"
// $ANTLR start "rule__StringDataType__Group__7"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9221:1: rule__StringDataType__Group__7 : rule__StringDataType__Group__7__Impl ;
public final void rule__StringDataType__Group__7() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9225:1: ( rule__StringDataType__Group__7__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9226:2: rule__StringDataType__Group__7__Impl
{
pushFollow(FollowSets000.FOLLOW_rule__StringDataType__Group__7__Impl_in_rule__StringDataType__Group__719656);
rule__StringDataType__Group__7__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__StringDataType__Group__7"
// $ANTLR start "rule__StringDataType__Group__7__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9232:1: rule__StringDataType__Group__7__Impl : ( '}' ) ;
public final void rule__StringDataType__Group__7__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9236:1: ( ( '}' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9237:1: ( '}' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9237:1: ( '}' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9238:1: '}'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getStringDataTypeAccess().getRightCurlyBracketKeyword_7());
}
match(input,68,FollowSets000.FOLLOW_68_in_rule__StringDataType__Group__7__Impl19684); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getStringDataTypeAccess().getRightCurlyBracketKeyword_7());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__StringDataType__Group__7__Impl"
// $ANTLR start "rule__StringDataType__Group_2__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9267:1: rule__StringDataType__Group_2__0 : rule__StringDataType__Group_2__0__Impl rule__StringDataType__Group_2__1 ;
public final void rule__StringDataType__Group_2__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9271:1: ( rule__StringDataType__Group_2__0__Impl rule__StringDataType__Group_2__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9272:2: rule__StringDataType__Group_2__0__Impl rule__StringDataType__Group_2__1
{
pushFollow(FollowSets000.FOLLOW_rule__StringDataType__Group_2__0__Impl_in_rule__StringDataType__Group_2__019731);
rule__StringDataType__Group_2__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets000.FOLLOW_rule__StringDataType__Group_2__1_in_rule__StringDataType__Group_2__019734);
rule__StringDataType__Group_2__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__StringDataType__Group_2__0"
// $ANTLR start "rule__StringDataType__Group_2__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9279:1: rule__StringDataType__Group_2__0__Impl : ( 'extends' ) ;
public final void rule__StringDataType__Group_2__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9283:1: ( ( 'extends' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9284:1: ( 'extends' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9284:1: ( 'extends' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9285:1: 'extends'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getStringDataTypeAccess().getExtendsKeyword_2_0());
}
match(input,45,FollowSets000.FOLLOW_45_in_rule__StringDataType__Group_2__0__Impl19762); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getStringDataTypeAccess().getExtendsKeyword_2_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__StringDataType__Group_2__0__Impl"
// $ANTLR start "rule__StringDataType__Group_2__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9298:1: rule__StringDataType__Group_2__1 : rule__StringDataType__Group_2__1__Impl ;
public final void rule__StringDataType__Group_2__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9302:1: ( rule__StringDataType__Group_2__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9303:2: rule__StringDataType__Group_2__1__Impl
{
pushFollow(FollowSets000.FOLLOW_rule__StringDataType__Group_2__1__Impl_in_rule__StringDataType__Group_2__119793);
rule__StringDataType__Group_2__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__StringDataType__Group_2__1"
// $ANTLR start "rule__StringDataType__Group_2__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9309:1: rule__StringDataType__Group_2__1__Impl : ( ( rule__StringDataType__RefAssignment_2_1 ) ) ;
public final void rule__StringDataType__Group_2__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9313:1: ( ( ( rule__StringDataType__RefAssignment_2_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9314:1: ( ( rule__StringDataType__RefAssignment_2_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9314:1: ( ( rule__StringDataType__RefAssignment_2_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9315:1: ( rule__StringDataType__RefAssignment_2_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getStringDataTypeAccess().getRefAssignment_2_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9316:1: ( rule__StringDataType__RefAssignment_2_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9316:2: rule__StringDataType__RefAssignment_2_1
{
pushFollow(FollowSets000.FOLLOW_rule__StringDataType__RefAssignment_2_1_in_rule__StringDataType__Group_2__1__Impl19820);
rule__StringDataType__RefAssignment_2_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getStringDataTypeAccess().getRefAssignment_2_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__StringDataType__Group_2__1__Impl"
// $ANTLR start "rule__StringDataType__Group_5__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9330:1: rule__StringDataType__Group_5__0 : rule__StringDataType__Group_5__0__Impl rule__StringDataType__Group_5__1 ;
public final void rule__StringDataType__Group_5__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9334:1: ( rule__StringDataType__Group_5__0__Impl rule__StringDataType__Group_5__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9335:2: rule__StringDataType__Group_5__0__Impl rule__StringDataType__Group_5__1
{
pushFollow(FollowSets000.FOLLOW_rule__StringDataType__Group_5__0__Impl_in_rule__StringDataType__Group_5__019854);
rule__StringDataType__Group_5__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets000.FOLLOW_rule__StringDataType__Group_5__1_in_rule__StringDataType__Group_5__019857);
rule__StringDataType__Group_5__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__StringDataType__Group_5__0"
// $ANTLR start "rule__StringDataType__Group_5__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9342:1: rule__StringDataType__Group_5__0__Impl : ( 'maxLength' ) ;
public final void rule__StringDataType__Group_5__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9346:1: ( ( 'maxLength' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9347:1: ( 'maxLength' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9347:1: ( 'maxLength' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9348:1: 'maxLength'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getStringDataTypeAccess().getMaxLengthKeyword_5_0());
}
match(input,83,FollowSets000.FOLLOW_83_in_rule__StringDataType__Group_5__0__Impl19885); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getStringDataTypeAccess().getMaxLengthKeyword_5_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__StringDataType__Group_5__0__Impl"
// $ANTLR start "rule__StringDataType__Group_5__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9361:1: rule__StringDataType__Group_5__1 : rule__StringDataType__Group_5__1__Impl ;
public final void rule__StringDataType__Group_5__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9365:1: ( rule__StringDataType__Group_5__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9366:2: rule__StringDataType__Group_5__1__Impl
{
pushFollow(FollowSets000.FOLLOW_rule__StringDataType__Group_5__1__Impl_in_rule__StringDataType__Group_5__119916);
rule__StringDataType__Group_5__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__StringDataType__Group_5__1"
// $ANTLR start "rule__StringDataType__Group_5__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9372:1: rule__StringDataType__Group_5__1__Impl : ( ( rule__StringDataType__MaxLengthAssignment_5_1 ) ) ;
public final void rule__StringDataType__Group_5__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9376:1: ( ( ( rule__StringDataType__MaxLengthAssignment_5_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9377:1: ( ( rule__StringDataType__MaxLengthAssignment_5_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9377:1: ( ( rule__StringDataType__MaxLengthAssignment_5_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9378:1: ( rule__StringDataType__MaxLengthAssignment_5_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getStringDataTypeAccess().getMaxLengthAssignment_5_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9379:1: ( rule__StringDataType__MaxLengthAssignment_5_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9379:2: rule__StringDataType__MaxLengthAssignment_5_1
{
pushFollow(FollowSets000.FOLLOW_rule__StringDataType__MaxLengthAssignment_5_1_in_rule__StringDataType__Group_5__1__Impl19943);
rule__StringDataType__MaxLengthAssignment_5_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getStringDataTypeAccess().getMaxLengthAssignment_5_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__StringDataType__Group_5__1__Impl"
// $ANTLR start "rule__StringDataType__Group_6__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9393:1: rule__StringDataType__Group_6__0 : rule__StringDataType__Group_6__0__Impl rule__StringDataType__Group_6__1 ;
public final void rule__StringDataType__Group_6__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9397:1: ( rule__StringDataType__Group_6__0__Impl rule__StringDataType__Group_6__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9398:2: rule__StringDataType__Group_6__0__Impl rule__StringDataType__Group_6__1
{
pushFollow(FollowSets000.FOLLOW_rule__StringDataType__Group_6__0__Impl_in_rule__StringDataType__Group_6__019977);
rule__StringDataType__Group_6__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets000.FOLLOW_rule__StringDataType__Group_6__1_in_rule__StringDataType__Group_6__019980);
rule__StringDataType__Group_6__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__StringDataType__Group_6__0"
// $ANTLR start "rule__StringDataType__Group_6__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9405:1: rule__StringDataType__Group_6__0__Impl : ( 'minLength' ) ;
public final void rule__StringDataType__Group_6__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9409:1: ( ( 'minLength' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9410:1: ( 'minLength' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9410:1: ( 'minLength' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9411:1: 'minLength'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getStringDataTypeAccess().getMinLengthKeyword_6_0());
}
match(input,84,FollowSets000.FOLLOW_84_in_rule__StringDataType__Group_6__0__Impl20008); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getStringDataTypeAccess().getMinLengthKeyword_6_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__StringDataType__Group_6__0__Impl"
// $ANTLR start "rule__StringDataType__Group_6__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9424:1: rule__StringDataType__Group_6__1 : rule__StringDataType__Group_6__1__Impl ;
public final void rule__StringDataType__Group_6__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9428:1: ( rule__StringDataType__Group_6__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9429:2: rule__StringDataType__Group_6__1__Impl
{
pushFollow(FollowSets000.FOLLOW_rule__StringDataType__Group_6__1__Impl_in_rule__StringDataType__Group_6__120039);
rule__StringDataType__Group_6__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__StringDataType__Group_6__1"
// $ANTLR start "rule__StringDataType__Group_6__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9435:1: rule__StringDataType__Group_6__1__Impl : ( ( rule__StringDataType__MinLengthAssignment_6_1 ) ) ;
public final void rule__StringDataType__Group_6__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9439:1: ( ( ( rule__StringDataType__MinLengthAssignment_6_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9440:1: ( ( rule__StringDataType__MinLengthAssignment_6_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9440:1: ( ( rule__StringDataType__MinLengthAssignment_6_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9441:1: ( rule__StringDataType__MinLengthAssignment_6_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getStringDataTypeAccess().getMinLengthAssignment_6_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9442:1: ( rule__StringDataType__MinLengthAssignment_6_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9442:2: rule__StringDataType__MinLengthAssignment_6_1
{
pushFollow(FollowSets000.FOLLOW_rule__StringDataType__MinLengthAssignment_6_1_in_rule__StringDataType__Group_6__1__Impl20066);
rule__StringDataType__MinLengthAssignment_6_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getStringDataTypeAccess().getMinLengthAssignment_6_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__StringDataType__Group_6__1__Impl"
// $ANTLR start "rule__StringEntityAttribute__Group__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9456:1: rule__StringEntityAttribute__Group__0 : rule__StringEntityAttribute__Group__0__Impl rule__StringEntityAttribute__Group__1 ;
public final void rule__StringEntityAttribute__Group__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9460:1: ( rule__StringEntityAttribute__Group__0__Impl rule__StringEntityAttribute__Group__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9461:2: rule__StringEntityAttribute__Group__0__Impl rule__StringEntityAttribute__Group__1
{
pushFollow(FollowSets000.FOLLOW_rule__StringEntityAttribute__Group__0__Impl_in_rule__StringEntityAttribute__Group__020100);
rule__StringEntityAttribute__Group__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets000.FOLLOW_rule__StringEntityAttribute__Group__1_in_rule__StringEntityAttribute__Group__020103);
rule__StringEntityAttribute__Group__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__StringEntityAttribute__Group__0"
// $ANTLR start "rule__StringEntityAttribute__Group__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9468:1: rule__StringEntityAttribute__Group__0__Impl : ( 'string' ) ;
public final void rule__StringEntityAttribute__Group__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9472:1: ( ( 'string' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9473:1: ( 'string' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9473:1: ( 'string' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9474:1: 'string'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getStringEntityAttributeAccess().getStringKeyword_0());
}
match(input,59,FollowSets000.FOLLOW_59_in_rule__StringEntityAttribute__Group__0__Impl20131); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getStringEntityAttributeAccess().getStringKeyword_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__StringEntityAttribute__Group__0__Impl"
// $ANTLR start "rule__StringEntityAttribute__Group__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9487:1: rule__StringEntityAttribute__Group__1 : rule__StringEntityAttribute__Group__1__Impl rule__StringEntityAttribute__Group__2 ;
public final void rule__StringEntityAttribute__Group__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9491:1: ( rule__StringEntityAttribute__Group__1__Impl rule__StringEntityAttribute__Group__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9492:2: rule__StringEntityAttribute__Group__1__Impl rule__StringEntityAttribute__Group__2
{
pushFollow(FollowSets000.FOLLOW_rule__StringEntityAttribute__Group__1__Impl_in_rule__StringEntityAttribute__Group__120162);
rule__StringEntityAttribute__Group__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets000.FOLLOW_rule__StringEntityAttribute__Group__2_in_rule__StringEntityAttribute__Group__120165);
rule__StringEntityAttribute__Group__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__StringEntityAttribute__Group__1"
// $ANTLR start "rule__StringEntityAttribute__Group__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9499:1: rule__StringEntityAttribute__Group__1__Impl : ( ( rule__StringEntityAttribute__TypeAssignment_1 )? ) ;
public final void rule__StringEntityAttribute__Group__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9503:1: ( ( ( rule__StringEntityAttribute__TypeAssignment_1 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9504:1: ( ( rule__StringEntityAttribute__TypeAssignment_1 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9504:1: ( ( rule__StringEntityAttribute__TypeAssignment_1 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9505:1: ( rule__StringEntityAttribute__TypeAssignment_1 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getStringEntityAttributeAccess().getTypeAssignment_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9506:1: ( rule__StringEntityAttribute__TypeAssignment_1 )?
int alt78=2;
int LA78_0 = input.LA(1);
if ( (LA78_0==RULE_ID) ) {
int LA78_1 = input.LA(2);
if ( (LA78_1==RULE_ID||LA78_1==43||(LA78_1>=53 && LA78_1<=55)) ) {
alt78=1;
}
}
switch (alt78) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9506:2: rule__StringEntityAttribute__TypeAssignment_1
{
pushFollow(FollowSets000.FOLLOW_rule__StringEntityAttribute__TypeAssignment_1_in_rule__StringEntityAttribute__Group__1__Impl20192);
rule__StringEntityAttribute__TypeAssignment_1();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getStringEntityAttributeAccess().getTypeAssignment_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__StringEntityAttribute__Group__1__Impl"
// $ANTLR start "rule__StringEntityAttribute__Group__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9516:1: rule__StringEntityAttribute__Group__2 : rule__StringEntityAttribute__Group__2__Impl rule__StringEntityAttribute__Group__3 ;
public final void rule__StringEntityAttribute__Group__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9520:1: ( rule__StringEntityAttribute__Group__2__Impl rule__StringEntityAttribute__Group__3 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9521:2: rule__StringEntityAttribute__Group__2__Impl rule__StringEntityAttribute__Group__3
{
pushFollow(FollowSets000.FOLLOW_rule__StringEntityAttribute__Group__2__Impl_in_rule__StringEntityAttribute__Group__220223);
rule__StringEntityAttribute__Group__2__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets000.FOLLOW_rule__StringEntityAttribute__Group__3_in_rule__StringEntityAttribute__Group__220226);
rule__StringEntityAttribute__Group__3();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__StringEntityAttribute__Group__2"
// $ANTLR start "rule__StringEntityAttribute__Group__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9528:1: rule__StringEntityAttribute__Group__2__Impl : ( ( rule__StringEntityAttribute__CardinalityAssignment_2 )? ) ;
public final void rule__StringEntityAttribute__Group__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9532:1: ( ( ( rule__StringEntityAttribute__CardinalityAssignment_2 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9533:1: ( ( rule__StringEntityAttribute__CardinalityAssignment_2 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9533:1: ( ( rule__StringEntityAttribute__CardinalityAssignment_2 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9534:1: ( rule__StringEntityAttribute__CardinalityAssignment_2 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getStringEntityAttributeAccess().getCardinalityAssignment_2());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9535:1: ( rule__StringEntityAttribute__CardinalityAssignment_2 )?
int alt79=2;
int LA79_0 = input.LA(1);
if ( ((LA79_0>=53 && LA79_0<=55)) ) {
alt79=1;
}
switch (alt79) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9535:2: rule__StringEntityAttribute__CardinalityAssignment_2
{
pushFollow(FollowSets000.FOLLOW_rule__StringEntityAttribute__CardinalityAssignment_2_in_rule__StringEntityAttribute__Group__2__Impl20253);
rule__StringEntityAttribute__CardinalityAssignment_2();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getStringEntityAttributeAccess().getCardinalityAssignment_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__StringEntityAttribute__Group__2__Impl"
// $ANTLR start "rule__StringEntityAttribute__Group__3"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9545:1: rule__StringEntityAttribute__Group__3 : rule__StringEntityAttribute__Group__3__Impl ;
public final void rule__StringEntityAttribute__Group__3() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9549:1: ( rule__StringEntityAttribute__Group__3__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9550:2: rule__StringEntityAttribute__Group__3__Impl
{
pushFollow(FollowSets000.FOLLOW_rule__StringEntityAttribute__Group__3__Impl_in_rule__StringEntityAttribute__Group__320284);
rule__StringEntityAttribute__Group__3__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__StringEntityAttribute__Group__3"
// $ANTLR start "rule__StringEntityAttribute__Group__3__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9556:1: rule__StringEntityAttribute__Group__3__Impl : ( ( rule__StringEntityAttribute__NameAssignment_3 ) ) ;
public final void rule__StringEntityAttribute__Group__3__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9560:1: ( ( ( rule__StringEntityAttribute__NameAssignment_3 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9561:1: ( ( rule__StringEntityAttribute__NameAssignment_3 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9561:1: ( ( rule__StringEntityAttribute__NameAssignment_3 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9562:1: ( rule__StringEntityAttribute__NameAssignment_3 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getStringEntityAttributeAccess().getNameAssignment_3());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9563:1: ( rule__StringEntityAttribute__NameAssignment_3 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9563:2: rule__StringEntityAttribute__NameAssignment_3
{
pushFollow(FollowSets000.FOLLOW_rule__StringEntityAttribute__NameAssignment_3_in_rule__StringEntityAttribute__Group__3__Impl20311);
rule__StringEntityAttribute__NameAssignment_3();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getStringEntityAttributeAccess().getNameAssignment_3());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__StringEntityAttribute__Group__3__Impl"
// $ANTLR start "rule__MapEntityAttribute__Group__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9583:1: rule__MapEntityAttribute__Group__0 : rule__MapEntityAttribute__Group__0__Impl rule__MapEntityAttribute__Group__1 ;
public final void rule__MapEntityAttribute__Group__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9587:1: ( rule__MapEntityAttribute__Group__0__Impl rule__MapEntityAttribute__Group__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9588:2: rule__MapEntityAttribute__Group__0__Impl rule__MapEntityAttribute__Group__1
{
pushFollow(FollowSets000.FOLLOW_rule__MapEntityAttribute__Group__0__Impl_in_rule__MapEntityAttribute__Group__020351);
rule__MapEntityAttribute__Group__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets000.FOLLOW_rule__MapEntityAttribute__Group__1_in_rule__MapEntityAttribute__Group__020354);
rule__MapEntityAttribute__Group__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__MapEntityAttribute__Group__0"
// $ANTLR start "rule__MapEntityAttribute__Group__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9595:1: rule__MapEntityAttribute__Group__0__Impl : ( 'map' ) ;
public final void rule__MapEntityAttribute__Group__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9599:1: ( ( 'map' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9600:1: ( 'map' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9600:1: ( 'map' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9601:1: 'map'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getMapEntityAttributeAccess().getMapKeyword_0());
}
match(input,85,FollowSets000.FOLLOW_85_in_rule__MapEntityAttribute__Group__0__Impl20382); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getMapEntityAttributeAccess().getMapKeyword_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__MapEntityAttribute__Group__0__Impl"
// $ANTLR start "rule__MapEntityAttribute__Group__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9614:1: rule__MapEntityAttribute__Group__1 : rule__MapEntityAttribute__Group__1__Impl rule__MapEntityAttribute__Group__2 ;
public final void rule__MapEntityAttribute__Group__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9618:1: ( rule__MapEntityAttribute__Group__1__Impl rule__MapEntityAttribute__Group__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9619:2: rule__MapEntityAttribute__Group__1__Impl rule__MapEntityAttribute__Group__2
{
pushFollow(FollowSets000.FOLLOW_rule__MapEntityAttribute__Group__1__Impl_in_rule__MapEntityAttribute__Group__120413);
rule__MapEntityAttribute__Group__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets000.FOLLOW_rule__MapEntityAttribute__Group__2_in_rule__MapEntityAttribute__Group__120416);
rule__MapEntityAttribute__Group__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__MapEntityAttribute__Group__1"
// $ANTLR start "rule__MapEntityAttribute__Group__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9626:1: rule__MapEntityAttribute__Group__1__Impl : ( ( rule__MapEntityAttribute__TypeAssignment_1 )? ) ;
public final void rule__MapEntityAttribute__Group__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9630:1: ( ( ( rule__MapEntityAttribute__TypeAssignment_1 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9631:1: ( ( rule__MapEntityAttribute__TypeAssignment_1 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9631:1: ( ( rule__MapEntityAttribute__TypeAssignment_1 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9632:1: ( rule__MapEntityAttribute__TypeAssignment_1 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getMapEntityAttributeAccess().getTypeAssignment_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9633:1: ( rule__MapEntityAttribute__TypeAssignment_1 )?
int alt80=2;
int LA80_0 = input.LA(1);
if ( (LA80_0==RULE_ID) ) {
switch ( input.LA(2) ) {
case RULE_ID:
case 43:
case 58:
case 61:
{
alt80=1;
}
break;
case 56:
{
int LA80_4 = input.LA(3);
if ( ((LA80_4>=56 && LA80_4<=61)) ) {
alt80=1;
}
}
break;
case 57:
{
int LA80_5 = input.LA(3);
if ( ((LA80_5>=56 && LA80_5<=61)) ) {
alt80=1;
}
}
break;
case 59:
{
int LA80_6 = input.LA(3);
if ( ((LA80_6>=56 && LA80_6<=61)) ) {
alt80=1;
}
}
break;
case 60:
{
int LA80_7 = input.LA(3);
if ( ((LA80_7>=56 && LA80_7<=61)) ) {
alt80=1;
}
}
break;
}
}
switch (alt80) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9633:2: rule__MapEntityAttribute__TypeAssignment_1
{
pushFollow(FollowSets000.FOLLOW_rule__MapEntityAttribute__TypeAssignment_1_in_rule__MapEntityAttribute__Group__1__Impl20443);
rule__MapEntityAttribute__TypeAssignment_1();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getMapEntityAttributeAccess().getTypeAssignment_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__MapEntityAttribute__Group__1__Impl"
// $ANTLR start "rule__MapEntityAttribute__Group__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9643:1: rule__MapEntityAttribute__Group__2 : rule__MapEntityAttribute__Group__2__Impl rule__MapEntityAttribute__Group__3 ;
public final void rule__MapEntityAttribute__Group__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9647:1: ( rule__MapEntityAttribute__Group__2__Impl rule__MapEntityAttribute__Group__3 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9648:2: rule__MapEntityAttribute__Group__2__Impl rule__MapEntityAttribute__Group__3
{
pushFollow(FollowSets000.FOLLOW_rule__MapEntityAttribute__Group__2__Impl_in_rule__MapEntityAttribute__Group__220474);
rule__MapEntityAttribute__Group__2__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets000.FOLLOW_rule__MapEntityAttribute__Group__3_in_rule__MapEntityAttribute__Group__220477);
rule__MapEntityAttribute__Group__3();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__MapEntityAttribute__Group__2"
// $ANTLR start "rule__MapEntityAttribute__Group__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9655:1: rule__MapEntityAttribute__Group__2__Impl : ( ( rule__MapEntityAttribute__Group_2__0 )? ) ;
public final void rule__MapEntityAttribute__Group__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9659:1: ( ( ( rule__MapEntityAttribute__Group_2__0 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9660:1: ( ( rule__MapEntityAttribute__Group_2__0 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9660:1: ( ( rule__MapEntityAttribute__Group_2__0 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9661:1: ( rule__MapEntityAttribute__Group_2__0 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getMapEntityAttributeAccess().getGroup_2());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9662:1: ( rule__MapEntityAttribute__Group_2__0 )?
int alt81=2;
int LA81_0 = input.LA(1);
if ( ((LA81_0>=56 && LA81_0<=61)) ) {
alt81=1;
}
switch (alt81) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9662:2: rule__MapEntityAttribute__Group_2__0
{
pushFollow(FollowSets000.FOLLOW_rule__MapEntityAttribute__Group_2__0_in_rule__MapEntityAttribute__Group__2__Impl20504);
rule__MapEntityAttribute__Group_2__0();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getMapEntityAttributeAccess().getGroup_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__MapEntityAttribute__Group__2__Impl"
// $ANTLR start "rule__MapEntityAttribute__Group__3"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9672:1: rule__MapEntityAttribute__Group__3 : rule__MapEntityAttribute__Group__3__Impl ;
public final void rule__MapEntityAttribute__Group__3() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9676:1: ( rule__MapEntityAttribute__Group__3__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9677:2: rule__MapEntityAttribute__Group__3__Impl
{
pushFollow(FollowSets000.FOLLOW_rule__MapEntityAttribute__Group__3__Impl_in_rule__MapEntityAttribute__Group__320535);
rule__MapEntityAttribute__Group__3__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__MapEntityAttribute__Group__3"
// $ANTLR start "rule__MapEntityAttribute__Group__3__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9683:1: rule__MapEntityAttribute__Group__3__Impl : ( ( rule__MapEntityAttribute__NameAssignment_3 ) ) ;
public final void rule__MapEntityAttribute__Group__3__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9687:1: ( ( ( rule__MapEntityAttribute__NameAssignment_3 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9688:1: ( ( rule__MapEntityAttribute__NameAssignment_3 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9688:1: ( ( rule__MapEntityAttribute__NameAssignment_3 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9689:1: ( rule__MapEntityAttribute__NameAssignment_3 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getMapEntityAttributeAccess().getNameAssignment_3());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9690:1: ( rule__MapEntityAttribute__NameAssignment_3 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9690:2: rule__MapEntityAttribute__NameAssignment_3
{
pushFollow(FollowSets000.FOLLOW_rule__MapEntityAttribute__NameAssignment_3_in_rule__MapEntityAttribute__Group__3__Impl20562);
rule__MapEntityAttribute__NameAssignment_3();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getMapEntityAttributeAccess().getNameAssignment_3());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__MapEntityAttribute__Group__3__Impl"
// $ANTLR start "rule__MapEntityAttribute__Group_2__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9708:1: rule__MapEntityAttribute__Group_2__0 : rule__MapEntityAttribute__Group_2__0__Impl rule__MapEntityAttribute__Group_2__1 ;
public final void rule__MapEntityAttribute__Group_2__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9712:1: ( rule__MapEntityAttribute__Group_2__0__Impl rule__MapEntityAttribute__Group_2__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9713:2: rule__MapEntityAttribute__Group_2__0__Impl rule__MapEntityAttribute__Group_2__1
{
pushFollow(FollowSets000.FOLLOW_rule__MapEntityAttribute__Group_2__0__Impl_in_rule__MapEntityAttribute__Group_2__020600);
rule__MapEntityAttribute__Group_2__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets000.FOLLOW_rule__MapEntityAttribute__Group_2__1_in_rule__MapEntityAttribute__Group_2__020603);
rule__MapEntityAttribute__Group_2__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__MapEntityAttribute__Group_2__0"
// $ANTLR start "rule__MapEntityAttribute__Group_2__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9720:1: rule__MapEntityAttribute__Group_2__0__Impl : ( ( rule__MapEntityAttribute__KeyTypeAssignment_2_0 ) ) ;
public final void rule__MapEntityAttribute__Group_2__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9724:1: ( ( ( rule__MapEntityAttribute__KeyTypeAssignment_2_0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9725:1: ( ( rule__MapEntityAttribute__KeyTypeAssignment_2_0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9725:1: ( ( rule__MapEntityAttribute__KeyTypeAssignment_2_0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9726:1: ( rule__MapEntityAttribute__KeyTypeAssignment_2_0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getMapEntityAttributeAccess().getKeyTypeAssignment_2_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9727:1: ( rule__MapEntityAttribute__KeyTypeAssignment_2_0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9727:2: rule__MapEntityAttribute__KeyTypeAssignment_2_0
{
pushFollow(FollowSets000.FOLLOW_rule__MapEntityAttribute__KeyTypeAssignment_2_0_in_rule__MapEntityAttribute__Group_2__0__Impl20630);
rule__MapEntityAttribute__KeyTypeAssignment_2_0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getMapEntityAttributeAccess().getKeyTypeAssignment_2_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__MapEntityAttribute__Group_2__0__Impl"
// $ANTLR start "rule__MapEntityAttribute__Group_2__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9737:1: rule__MapEntityAttribute__Group_2__1 : rule__MapEntityAttribute__Group_2__1__Impl ;
public final void rule__MapEntityAttribute__Group_2__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9741:1: ( rule__MapEntityAttribute__Group_2__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9742:2: rule__MapEntityAttribute__Group_2__1__Impl
{
pushFollow(FollowSets000.FOLLOW_rule__MapEntityAttribute__Group_2__1__Impl_in_rule__MapEntityAttribute__Group_2__120660);
rule__MapEntityAttribute__Group_2__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__MapEntityAttribute__Group_2__1"
// $ANTLR start "rule__MapEntityAttribute__Group_2__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9748:1: rule__MapEntityAttribute__Group_2__1__Impl : ( ( rule__MapEntityAttribute__ValueTypeAssignment_2_1 ) ) ;
public final void rule__MapEntityAttribute__Group_2__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9752:1: ( ( ( rule__MapEntityAttribute__ValueTypeAssignment_2_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9753:1: ( ( rule__MapEntityAttribute__ValueTypeAssignment_2_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9753:1: ( ( rule__MapEntityAttribute__ValueTypeAssignment_2_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9754:1: ( rule__MapEntityAttribute__ValueTypeAssignment_2_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getMapEntityAttributeAccess().getValueTypeAssignment_2_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9755:1: ( rule__MapEntityAttribute__ValueTypeAssignment_2_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9755:2: rule__MapEntityAttribute__ValueTypeAssignment_2_1
{
pushFollow(FollowSets000.FOLLOW_rule__MapEntityAttribute__ValueTypeAssignment_2_1_in_rule__MapEntityAttribute__Group_2__1__Impl20687);
rule__MapEntityAttribute__ValueTypeAssignment_2_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getMapEntityAttributeAccess().getValueTypeAssignment_2_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__MapEntityAttribute__Group_2__1__Impl"
// $ANTLR start "rule__BooleanDataType__Group__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9769:1: rule__BooleanDataType__Group__0 : rule__BooleanDataType__Group__0__Impl rule__BooleanDataType__Group__1 ;
public final void rule__BooleanDataType__Group__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9773:1: ( rule__BooleanDataType__Group__0__Impl rule__BooleanDataType__Group__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9774:2: rule__BooleanDataType__Group__0__Impl rule__BooleanDataType__Group__1
{
pushFollow(FollowSets000.FOLLOW_rule__BooleanDataType__Group__0__Impl_in_rule__BooleanDataType__Group__020721);
rule__BooleanDataType__Group__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets000.FOLLOW_rule__BooleanDataType__Group__1_in_rule__BooleanDataType__Group__020724);
rule__BooleanDataType__Group__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__BooleanDataType__Group__0"
// $ANTLR start "rule__BooleanDataType__Group__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9781:1: rule__BooleanDataType__Group__0__Impl : ( 'booleandatatype' ) ;
public final void rule__BooleanDataType__Group__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9785:1: ( ( 'booleandatatype' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9786:1: ( 'booleandatatype' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9786:1: ( 'booleandatatype' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9787:1: 'booleandatatype'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getBooleanDataTypeAccess().getBooleandatatypeKeyword_0());
}
match(input,86,FollowSets000.FOLLOW_86_in_rule__BooleanDataType__Group__0__Impl20752); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getBooleanDataTypeAccess().getBooleandatatypeKeyword_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__BooleanDataType__Group__0__Impl"
// $ANTLR start "rule__BooleanDataType__Group__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9800:1: rule__BooleanDataType__Group__1 : rule__BooleanDataType__Group__1__Impl rule__BooleanDataType__Group__2 ;
public final void rule__BooleanDataType__Group__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9804:1: ( rule__BooleanDataType__Group__1__Impl rule__BooleanDataType__Group__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9805:2: rule__BooleanDataType__Group__1__Impl rule__BooleanDataType__Group__2
{
pushFollow(FollowSets000.FOLLOW_rule__BooleanDataType__Group__1__Impl_in_rule__BooleanDataType__Group__120783);
rule__BooleanDataType__Group__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets000.FOLLOW_rule__BooleanDataType__Group__2_in_rule__BooleanDataType__Group__120786);
rule__BooleanDataType__Group__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__BooleanDataType__Group__1"
// $ANTLR start "rule__BooleanDataType__Group__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9812:1: rule__BooleanDataType__Group__1__Impl : ( ( rule__BooleanDataType__NameAssignment_1 ) ) ;
public final void rule__BooleanDataType__Group__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9816:1: ( ( ( rule__BooleanDataType__NameAssignment_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9817:1: ( ( rule__BooleanDataType__NameAssignment_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9817:1: ( ( rule__BooleanDataType__NameAssignment_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9818:1: ( rule__BooleanDataType__NameAssignment_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getBooleanDataTypeAccess().getNameAssignment_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9819:1: ( rule__BooleanDataType__NameAssignment_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9819:2: rule__BooleanDataType__NameAssignment_1
{
pushFollow(FollowSets000.FOLLOW_rule__BooleanDataType__NameAssignment_1_in_rule__BooleanDataType__Group__1__Impl20813);
rule__BooleanDataType__NameAssignment_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getBooleanDataTypeAccess().getNameAssignment_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__BooleanDataType__Group__1__Impl"
// $ANTLR start "rule__BooleanDataType__Group__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9829:1: rule__BooleanDataType__Group__2 : rule__BooleanDataType__Group__2__Impl rule__BooleanDataType__Group__3 ;
public final void rule__BooleanDataType__Group__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9833:1: ( rule__BooleanDataType__Group__2__Impl rule__BooleanDataType__Group__3 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9834:2: rule__BooleanDataType__Group__2__Impl rule__BooleanDataType__Group__3
{
pushFollow(FollowSets000.FOLLOW_rule__BooleanDataType__Group__2__Impl_in_rule__BooleanDataType__Group__220843);
rule__BooleanDataType__Group__2__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets000.FOLLOW_rule__BooleanDataType__Group__3_in_rule__BooleanDataType__Group__220846);
rule__BooleanDataType__Group__3();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__BooleanDataType__Group__2"
// $ANTLR start "rule__BooleanDataType__Group__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9841:1: rule__BooleanDataType__Group__2__Impl : ( ( rule__BooleanDataType__Group_2__0 )? ) ;
public final void rule__BooleanDataType__Group__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9845:1: ( ( ( rule__BooleanDataType__Group_2__0 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9846:1: ( ( rule__BooleanDataType__Group_2__0 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9846:1: ( ( rule__BooleanDataType__Group_2__0 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9847:1: ( rule__BooleanDataType__Group_2__0 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getBooleanDataTypeAccess().getGroup_2());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9848:1: ( rule__BooleanDataType__Group_2__0 )?
int alt82=2;
int LA82_0 = input.LA(1);
if ( (LA82_0==45) ) {
alt82=1;
}
switch (alt82) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9848:2: rule__BooleanDataType__Group_2__0
{
pushFollow(FollowSets000.FOLLOW_rule__BooleanDataType__Group_2__0_in_rule__BooleanDataType__Group__2__Impl20873);
rule__BooleanDataType__Group_2__0();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getBooleanDataTypeAccess().getGroup_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__BooleanDataType__Group__2__Impl"
// $ANTLR start "rule__BooleanDataType__Group__3"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9858:1: rule__BooleanDataType__Group__3 : rule__BooleanDataType__Group__3__Impl rule__BooleanDataType__Group__4 ;
public final void rule__BooleanDataType__Group__3() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9862:1: ( rule__BooleanDataType__Group__3__Impl rule__BooleanDataType__Group__4 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9863:2: rule__BooleanDataType__Group__3__Impl rule__BooleanDataType__Group__4
{
pushFollow(FollowSets000.FOLLOW_rule__BooleanDataType__Group__3__Impl_in_rule__BooleanDataType__Group__320904);
rule__BooleanDataType__Group__3__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets000.FOLLOW_rule__BooleanDataType__Group__4_in_rule__BooleanDataType__Group__320907);
rule__BooleanDataType__Group__4();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__BooleanDataType__Group__3"
// $ANTLR start "rule__BooleanDataType__Group__3__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9870:1: rule__BooleanDataType__Group__3__Impl : ( '{' ) ;
public final void rule__BooleanDataType__Group__3__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9874:1: ( ( '{' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9875:1: ( '{' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9875:1: ( '{' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9876:1: '{'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getBooleanDataTypeAccess().getLeftCurlyBracketKeyword_3());
}
match(input,67,FollowSets000.FOLLOW_67_in_rule__BooleanDataType__Group__3__Impl20935); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getBooleanDataTypeAccess().getLeftCurlyBracketKeyword_3());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__BooleanDataType__Group__3__Impl"
// $ANTLR start "rule__BooleanDataType__Group__4"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9889:1: rule__BooleanDataType__Group__4 : rule__BooleanDataType__Group__4__Impl rule__BooleanDataType__Group__5 ;
public final void rule__BooleanDataType__Group__4() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9893:1: ( rule__BooleanDataType__Group__4__Impl rule__BooleanDataType__Group__5 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9894:2: rule__BooleanDataType__Group__4__Impl rule__BooleanDataType__Group__5
{
pushFollow(FollowSets000.FOLLOW_rule__BooleanDataType__Group__4__Impl_in_rule__BooleanDataType__Group__420966);
rule__BooleanDataType__Group__4__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets000.FOLLOW_rule__BooleanDataType__Group__5_in_rule__BooleanDataType__Group__420969);
rule__BooleanDataType__Group__5();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__BooleanDataType__Group__4"
// $ANTLR start "rule__BooleanDataType__Group__4__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9901:1: rule__BooleanDataType__Group__4__Impl : ( ( rule__BooleanDataType__BaseDataTypeAssignment_4 ) ) ;
public final void rule__BooleanDataType__Group__4__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9905:1: ( ( ( rule__BooleanDataType__BaseDataTypeAssignment_4 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9906:1: ( ( rule__BooleanDataType__BaseDataTypeAssignment_4 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9906:1: ( ( rule__BooleanDataType__BaseDataTypeAssignment_4 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9907:1: ( rule__BooleanDataType__BaseDataTypeAssignment_4 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getBooleanDataTypeAccess().getBaseDataTypeAssignment_4());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9908:1: ( rule__BooleanDataType__BaseDataTypeAssignment_4 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9908:2: rule__BooleanDataType__BaseDataTypeAssignment_4
{
pushFollow(FollowSets000.FOLLOW_rule__BooleanDataType__BaseDataTypeAssignment_4_in_rule__BooleanDataType__Group__4__Impl20996);
rule__BooleanDataType__BaseDataTypeAssignment_4();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getBooleanDataTypeAccess().getBaseDataTypeAssignment_4());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__BooleanDataType__Group__4__Impl"
// $ANTLR start "rule__BooleanDataType__Group__5"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9918:1: rule__BooleanDataType__Group__5 : rule__BooleanDataType__Group__5__Impl ;
public final void rule__BooleanDataType__Group__5() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9922:1: ( rule__BooleanDataType__Group__5__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9923:2: rule__BooleanDataType__Group__5__Impl
{
pushFollow(FollowSets000.FOLLOW_rule__BooleanDataType__Group__5__Impl_in_rule__BooleanDataType__Group__521026);
rule__BooleanDataType__Group__5__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__BooleanDataType__Group__5"
// $ANTLR start "rule__BooleanDataType__Group__5__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9929:1: rule__BooleanDataType__Group__5__Impl : ( '}' ) ;
public final void rule__BooleanDataType__Group__5__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9933:1: ( ( '}' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9934:1: ( '}' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9934:1: ( '}' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9935:1: '}'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getBooleanDataTypeAccess().getRightCurlyBracketKeyword_5());
}
match(input,68,FollowSets000.FOLLOW_68_in_rule__BooleanDataType__Group__5__Impl21054); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getBooleanDataTypeAccess().getRightCurlyBracketKeyword_5());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__BooleanDataType__Group__5__Impl"
// $ANTLR start "rule__BooleanDataType__Group_2__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9960:1: rule__BooleanDataType__Group_2__0 : rule__BooleanDataType__Group_2__0__Impl rule__BooleanDataType__Group_2__1 ;
public final void rule__BooleanDataType__Group_2__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9964:1: ( rule__BooleanDataType__Group_2__0__Impl rule__BooleanDataType__Group_2__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9965:2: rule__BooleanDataType__Group_2__0__Impl rule__BooleanDataType__Group_2__1
{
pushFollow(FollowSets000.FOLLOW_rule__BooleanDataType__Group_2__0__Impl_in_rule__BooleanDataType__Group_2__021097);
rule__BooleanDataType__Group_2__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets000.FOLLOW_rule__BooleanDataType__Group_2__1_in_rule__BooleanDataType__Group_2__021100);
rule__BooleanDataType__Group_2__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__BooleanDataType__Group_2__0"
// $ANTLR start "rule__BooleanDataType__Group_2__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9972:1: rule__BooleanDataType__Group_2__0__Impl : ( 'extends' ) ;
public final void rule__BooleanDataType__Group_2__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9976:1: ( ( 'extends' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9977:1: ( 'extends' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9977:1: ( 'extends' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9978:1: 'extends'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getBooleanDataTypeAccess().getExtendsKeyword_2_0());
}
match(input,45,FollowSets000.FOLLOW_45_in_rule__BooleanDataType__Group_2__0__Impl21128); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getBooleanDataTypeAccess().getExtendsKeyword_2_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__BooleanDataType__Group_2__0__Impl"
// $ANTLR start "rule__BooleanDataType__Group_2__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9991:1: rule__BooleanDataType__Group_2__1 : rule__BooleanDataType__Group_2__1__Impl ;
public final void rule__BooleanDataType__Group_2__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9995:1: ( rule__BooleanDataType__Group_2__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:9996:2: rule__BooleanDataType__Group_2__1__Impl
{
pushFollow(FollowSets000.FOLLOW_rule__BooleanDataType__Group_2__1__Impl_in_rule__BooleanDataType__Group_2__121159);
rule__BooleanDataType__Group_2__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__BooleanDataType__Group_2__1"
// $ANTLR start "rule__BooleanDataType__Group_2__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10002:1: rule__BooleanDataType__Group_2__1__Impl : ( ( rule__BooleanDataType__RefAssignment_2_1 ) ) ;
public final void rule__BooleanDataType__Group_2__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10006:1: ( ( ( rule__BooleanDataType__RefAssignment_2_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10007:1: ( ( rule__BooleanDataType__RefAssignment_2_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10007:1: ( ( rule__BooleanDataType__RefAssignment_2_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10008:1: ( rule__BooleanDataType__RefAssignment_2_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getBooleanDataTypeAccess().getRefAssignment_2_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10009:1: ( rule__BooleanDataType__RefAssignment_2_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10009:2: rule__BooleanDataType__RefAssignment_2_1
{
pushFollow(FollowSets000.FOLLOW_rule__BooleanDataType__RefAssignment_2_1_in_rule__BooleanDataType__Group_2__1__Impl21186);
rule__BooleanDataType__RefAssignment_2_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getBooleanDataTypeAccess().getRefAssignment_2_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__BooleanDataType__Group_2__1__Impl"
// $ANTLR start "rule__BooleanEntityAttribute__Group__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10023:1: rule__BooleanEntityAttribute__Group__0 : rule__BooleanEntityAttribute__Group__0__Impl rule__BooleanEntityAttribute__Group__1 ;
public final void rule__BooleanEntityAttribute__Group__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10027:1: ( rule__BooleanEntityAttribute__Group__0__Impl rule__BooleanEntityAttribute__Group__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10028:2: rule__BooleanEntityAttribute__Group__0__Impl rule__BooleanEntityAttribute__Group__1
{
pushFollow(FollowSets000.FOLLOW_rule__BooleanEntityAttribute__Group__0__Impl_in_rule__BooleanEntityAttribute__Group__021220);
rule__BooleanEntityAttribute__Group__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets000.FOLLOW_rule__BooleanEntityAttribute__Group__1_in_rule__BooleanEntityAttribute__Group__021223);
rule__BooleanEntityAttribute__Group__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__BooleanEntityAttribute__Group__0"
// $ANTLR start "rule__BooleanEntityAttribute__Group__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10035:1: rule__BooleanEntityAttribute__Group__0__Impl : ( 'boolean' ) ;
public final void rule__BooleanEntityAttribute__Group__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10039:1: ( ( 'boolean' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10040:1: ( 'boolean' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10040:1: ( 'boolean' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10041:1: 'boolean'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getBooleanEntityAttributeAccess().getBooleanKeyword_0());
}
match(input,60,FollowSets000.FOLLOW_60_in_rule__BooleanEntityAttribute__Group__0__Impl21251); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getBooleanEntityAttributeAccess().getBooleanKeyword_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__BooleanEntityAttribute__Group__0__Impl"
// $ANTLR start "rule__BooleanEntityAttribute__Group__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10054:1: rule__BooleanEntityAttribute__Group__1 : rule__BooleanEntityAttribute__Group__1__Impl rule__BooleanEntityAttribute__Group__2 ;
public final void rule__BooleanEntityAttribute__Group__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10058:1: ( rule__BooleanEntityAttribute__Group__1__Impl rule__BooleanEntityAttribute__Group__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10059:2: rule__BooleanEntityAttribute__Group__1__Impl rule__BooleanEntityAttribute__Group__2
{
pushFollow(FollowSets000.FOLLOW_rule__BooleanEntityAttribute__Group__1__Impl_in_rule__BooleanEntityAttribute__Group__121282);
rule__BooleanEntityAttribute__Group__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets000.FOLLOW_rule__BooleanEntityAttribute__Group__2_in_rule__BooleanEntityAttribute__Group__121285);
rule__BooleanEntityAttribute__Group__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__BooleanEntityAttribute__Group__1"
// $ANTLR start "rule__BooleanEntityAttribute__Group__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10066:1: rule__BooleanEntityAttribute__Group__1__Impl : ( ( rule__BooleanEntityAttribute__TypeAssignment_1 )? ) ;
public final void rule__BooleanEntityAttribute__Group__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10070:1: ( ( ( rule__BooleanEntityAttribute__TypeAssignment_1 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10071:1: ( ( rule__BooleanEntityAttribute__TypeAssignment_1 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10071:1: ( ( rule__BooleanEntityAttribute__TypeAssignment_1 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10072:1: ( rule__BooleanEntityAttribute__TypeAssignment_1 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getBooleanEntityAttributeAccess().getTypeAssignment_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10073:1: ( rule__BooleanEntityAttribute__TypeAssignment_1 )?
int alt83=2;
int LA83_0 = input.LA(1);
if ( (LA83_0==RULE_ID) ) {
int LA83_1 = input.LA(2);
if ( (LA83_1==RULE_ID||LA83_1==43) ) {
alt83=1;
}
}
switch (alt83) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10073:2: rule__BooleanEntityAttribute__TypeAssignment_1
{
pushFollow(FollowSets000.FOLLOW_rule__BooleanEntityAttribute__TypeAssignment_1_in_rule__BooleanEntityAttribute__Group__1__Impl21312);
rule__BooleanEntityAttribute__TypeAssignment_1();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getBooleanEntityAttributeAccess().getTypeAssignment_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__BooleanEntityAttribute__Group__1__Impl"
// $ANTLR start "rule__BooleanEntityAttribute__Group__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10083:1: rule__BooleanEntityAttribute__Group__2 : rule__BooleanEntityAttribute__Group__2__Impl ;
public final void rule__BooleanEntityAttribute__Group__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10087:1: ( rule__BooleanEntityAttribute__Group__2__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10088:2: rule__BooleanEntityAttribute__Group__2__Impl
{
pushFollow(FollowSets000.FOLLOW_rule__BooleanEntityAttribute__Group__2__Impl_in_rule__BooleanEntityAttribute__Group__221343);
rule__BooleanEntityAttribute__Group__2__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__BooleanEntityAttribute__Group__2"
// $ANTLR start "rule__BooleanEntityAttribute__Group__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10094:1: rule__BooleanEntityAttribute__Group__2__Impl : ( ( rule__BooleanEntityAttribute__NameAssignment_2 ) ) ;
public final void rule__BooleanEntityAttribute__Group__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10098:1: ( ( ( rule__BooleanEntityAttribute__NameAssignment_2 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10099:1: ( ( rule__BooleanEntityAttribute__NameAssignment_2 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10099:1: ( ( rule__BooleanEntityAttribute__NameAssignment_2 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10100:1: ( rule__BooleanEntityAttribute__NameAssignment_2 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getBooleanEntityAttributeAccess().getNameAssignment_2());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10101:1: ( rule__BooleanEntityAttribute__NameAssignment_2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10101:2: rule__BooleanEntityAttribute__NameAssignment_2
{
pushFollow(FollowSets000.FOLLOW_rule__BooleanEntityAttribute__NameAssignment_2_in_rule__BooleanEntityAttribute__Group__2__Impl21370);
rule__BooleanEntityAttribute__NameAssignment_2();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getBooleanEntityAttributeAccess().getNameAssignment_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__BooleanEntityAttribute__Group__2__Impl"
// $ANTLR start "rule__IntegerDataType__Group__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10117:1: rule__IntegerDataType__Group__0 : rule__IntegerDataType__Group__0__Impl rule__IntegerDataType__Group__1 ;
public final void rule__IntegerDataType__Group__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10121:1: ( rule__IntegerDataType__Group__0__Impl rule__IntegerDataType__Group__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10122:2: rule__IntegerDataType__Group__0__Impl rule__IntegerDataType__Group__1
{
pushFollow(FollowSets000.FOLLOW_rule__IntegerDataType__Group__0__Impl_in_rule__IntegerDataType__Group__021406);
rule__IntegerDataType__Group__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets000.FOLLOW_rule__IntegerDataType__Group__1_in_rule__IntegerDataType__Group__021409);
rule__IntegerDataType__Group__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__IntegerDataType__Group__0"
// $ANTLR start "rule__IntegerDataType__Group__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10129:1: rule__IntegerDataType__Group__0__Impl : ( 'integerdatatype' ) ;
public final void rule__IntegerDataType__Group__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10133:1: ( ( 'integerdatatype' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10134:1: ( 'integerdatatype' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10134:1: ( 'integerdatatype' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10135:1: 'integerdatatype'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getIntegerDataTypeAccess().getIntegerdatatypeKeyword_0());
}
match(input,87,FollowSets000.FOLLOW_87_in_rule__IntegerDataType__Group__0__Impl21437); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getIntegerDataTypeAccess().getIntegerdatatypeKeyword_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__IntegerDataType__Group__0__Impl"
// $ANTLR start "rule__IntegerDataType__Group__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10148:1: rule__IntegerDataType__Group__1 : rule__IntegerDataType__Group__1__Impl rule__IntegerDataType__Group__2 ;
public final void rule__IntegerDataType__Group__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10152:1: ( rule__IntegerDataType__Group__1__Impl rule__IntegerDataType__Group__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10153:2: rule__IntegerDataType__Group__1__Impl rule__IntegerDataType__Group__2
{
pushFollow(FollowSets000.FOLLOW_rule__IntegerDataType__Group__1__Impl_in_rule__IntegerDataType__Group__121468);
rule__IntegerDataType__Group__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets000.FOLLOW_rule__IntegerDataType__Group__2_in_rule__IntegerDataType__Group__121471);
rule__IntegerDataType__Group__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__IntegerDataType__Group__1"
// $ANTLR start "rule__IntegerDataType__Group__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10160:1: rule__IntegerDataType__Group__1__Impl : ( ( rule__IntegerDataType__NameAssignment_1 ) ) ;
public final void rule__IntegerDataType__Group__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10164:1: ( ( ( rule__IntegerDataType__NameAssignment_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10165:1: ( ( rule__IntegerDataType__NameAssignment_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10165:1: ( ( rule__IntegerDataType__NameAssignment_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10166:1: ( rule__IntegerDataType__NameAssignment_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getIntegerDataTypeAccess().getNameAssignment_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10167:1: ( rule__IntegerDataType__NameAssignment_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10167:2: rule__IntegerDataType__NameAssignment_1
{
pushFollow(FollowSets000.FOLLOW_rule__IntegerDataType__NameAssignment_1_in_rule__IntegerDataType__Group__1__Impl21498);
rule__IntegerDataType__NameAssignment_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getIntegerDataTypeAccess().getNameAssignment_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__IntegerDataType__Group__1__Impl"
// $ANTLR start "rule__IntegerDataType__Group__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10177:1: rule__IntegerDataType__Group__2 : rule__IntegerDataType__Group__2__Impl rule__IntegerDataType__Group__3 ;
public final void rule__IntegerDataType__Group__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10181:1: ( rule__IntegerDataType__Group__2__Impl rule__IntegerDataType__Group__3 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10182:2: rule__IntegerDataType__Group__2__Impl rule__IntegerDataType__Group__3
{
pushFollow(FollowSets000.FOLLOW_rule__IntegerDataType__Group__2__Impl_in_rule__IntegerDataType__Group__221528);
rule__IntegerDataType__Group__2__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets000.FOLLOW_rule__IntegerDataType__Group__3_in_rule__IntegerDataType__Group__221531);
rule__IntegerDataType__Group__3();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__IntegerDataType__Group__2"
// $ANTLR start "rule__IntegerDataType__Group__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10189:1: rule__IntegerDataType__Group__2__Impl : ( ( rule__IntegerDataType__Group_2__0 )? ) ;
public final void rule__IntegerDataType__Group__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10193:1: ( ( ( rule__IntegerDataType__Group_2__0 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10194:1: ( ( rule__IntegerDataType__Group_2__0 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10194:1: ( ( rule__IntegerDataType__Group_2__0 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10195:1: ( rule__IntegerDataType__Group_2__0 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getIntegerDataTypeAccess().getGroup_2());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10196:1: ( rule__IntegerDataType__Group_2__0 )?
int alt84=2;
int LA84_0 = input.LA(1);
if ( (LA84_0==45) ) {
alt84=1;
}
switch (alt84) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10196:2: rule__IntegerDataType__Group_2__0
{
pushFollow(FollowSets000.FOLLOW_rule__IntegerDataType__Group_2__0_in_rule__IntegerDataType__Group__2__Impl21558);
rule__IntegerDataType__Group_2__0();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getIntegerDataTypeAccess().getGroup_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__IntegerDataType__Group__2__Impl"
// $ANTLR start "rule__IntegerDataType__Group__3"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10206:1: rule__IntegerDataType__Group__3 : rule__IntegerDataType__Group__3__Impl rule__IntegerDataType__Group__4 ;
public final void rule__IntegerDataType__Group__3() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10210:1: ( rule__IntegerDataType__Group__3__Impl rule__IntegerDataType__Group__4 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10211:2: rule__IntegerDataType__Group__3__Impl rule__IntegerDataType__Group__4
{
pushFollow(FollowSets000.FOLLOW_rule__IntegerDataType__Group__3__Impl_in_rule__IntegerDataType__Group__321589);
rule__IntegerDataType__Group__3__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets000.FOLLOW_rule__IntegerDataType__Group__4_in_rule__IntegerDataType__Group__321592);
rule__IntegerDataType__Group__4();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__IntegerDataType__Group__3"
// $ANTLR start "rule__IntegerDataType__Group__3__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10218:1: rule__IntegerDataType__Group__3__Impl : ( '{' ) ;
public final void rule__IntegerDataType__Group__3__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10222:1: ( ( '{' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10223:1: ( '{' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10223:1: ( '{' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10224:1: '{'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getIntegerDataTypeAccess().getLeftCurlyBracketKeyword_3());
}
match(input,67,FollowSets000.FOLLOW_67_in_rule__IntegerDataType__Group__3__Impl21620); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getIntegerDataTypeAccess().getLeftCurlyBracketKeyword_3());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__IntegerDataType__Group__3__Impl"
// $ANTLR start "rule__IntegerDataType__Group__4"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10237:1: rule__IntegerDataType__Group__4 : rule__IntegerDataType__Group__4__Impl rule__IntegerDataType__Group__5 ;
public final void rule__IntegerDataType__Group__4() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10241:1: ( rule__IntegerDataType__Group__4__Impl rule__IntegerDataType__Group__5 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10242:2: rule__IntegerDataType__Group__4__Impl rule__IntegerDataType__Group__5
{
pushFollow(FollowSets000.FOLLOW_rule__IntegerDataType__Group__4__Impl_in_rule__IntegerDataType__Group__421651);
rule__IntegerDataType__Group__4__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets000.FOLLOW_rule__IntegerDataType__Group__5_in_rule__IntegerDataType__Group__421654);
rule__IntegerDataType__Group__5();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__IntegerDataType__Group__4"
// $ANTLR start "rule__IntegerDataType__Group__4__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10249:1: rule__IntegerDataType__Group__4__Impl : ( ( rule__IntegerDataType__BaseDataTypeAssignment_4 ) ) ;
public final void rule__IntegerDataType__Group__4__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10253:1: ( ( ( rule__IntegerDataType__BaseDataTypeAssignment_4 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10254:1: ( ( rule__IntegerDataType__BaseDataTypeAssignment_4 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10254:1: ( ( rule__IntegerDataType__BaseDataTypeAssignment_4 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10255:1: ( rule__IntegerDataType__BaseDataTypeAssignment_4 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getIntegerDataTypeAccess().getBaseDataTypeAssignment_4());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10256:1: ( rule__IntegerDataType__BaseDataTypeAssignment_4 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10256:2: rule__IntegerDataType__BaseDataTypeAssignment_4
{
pushFollow(FollowSets000.FOLLOW_rule__IntegerDataType__BaseDataTypeAssignment_4_in_rule__IntegerDataType__Group__4__Impl21681);
rule__IntegerDataType__BaseDataTypeAssignment_4();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getIntegerDataTypeAccess().getBaseDataTypeAssignment_4());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__IntegerDataType__Group__4__Impl"
// $ANTLR start "rule__IntegerDataType__Group__5"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10266:1: rule__IntegerDataType__Group__5 : rule__IntegerDataType__Group__5__Impl ;
public final void rule__IntegerDataType__Group__5() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10270:1: ( rule__IntegerDataType__Group__5__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10271:2: rule__IntegerDataType__Group__5__Impl
{
pushFollow(FollowSets000.FOLLOW_rule__IntegerDataType__Group__5__Impl_in_rule__IntegerDataType__Group__521711);
rule__IntegerDataType__Group__5__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__IntegerDataType__Group__5"
// $ANTLR start "rule__IntegerDataType__Group__5__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10277:1: rule__IntegerDataType__Group__5__Impl : ( '}' ) ;
public final void rule__IntegerDataType__Group__5__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10281:1: ( ( '}' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10282:1: ( '}' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10282:1: ( '}' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10283:1: '}'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getIntegerDataTypeAccess().getRightCurlyBracketKeyword_5());
}
match(input,68,FollowSets001.FOLLOW_68_in_rule__IntegerDataType__Group__5__Impl21739); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getIntegerDataTypeAccess().getRightCurlyBracketKeyword_5());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__IntegerDataType__Group__5__Impl"
// $ANTLR start "rule__IntegerDataType__Group_2__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10308:1: rule__IntegerDataType__Group_2__0 : rule__IntegerDataType__Group_2__0__Impl rule__IntegerDataType__Group_2__1 ;
public final void rule__IntegerDataType__Group_2__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10312:1: ( rule__IntegerDataType__Group_2__0__Impl rule__IntegerDataType__Group_2__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10313:2: rule__IntegerDataType__Group_2__0__Impl rule__IntegerDataType__Group_2__1
{
pushFollow(FollowSets001.FOLLOW_rule__IntegerDataType__Group_2__0__Impl_in_rule__IntegerDataType__Group_2__021782);
rule__IntegerDataType__Group_2__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__IntegerDataType__Group_2__1_in_rule__IntegerDataType__Group_2__021785);
rule__IntegerDataType__Group_2__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__IntegerDataType__Group_2__0"
// $ANTLR start "rule__IntegerDataType__Group_2__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10320:1: rule__IntegerDataType__Group_2__0__Impl : ( 'extends' ) ;
public final void rule__IntegerDataType__Group_2__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10324:1: ( ( 'extends' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10325:1: ( 'extends' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10325:1: ( 'extends' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10326:1: 'extends'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getIntegerDataTypeAccess().getExtendsKeyword_2_0());
}
match(input,45,FollowSets001.FOLLOW_45_in_rule__IntegerDataType__Group_2__0__Impl21813); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getIntegerDataTypeAccess().getExtendsKeyword_2_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__IntegerDataType__Group_2__0__Impl"
// $ANTLR start "rule__IntegerDataType__Group_2__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10339:1: rule__IntegerDataType__Group_2__1 : rule__IntegerDataType__Group_2__1__Impl ;
public final void rule__IntegerDataType__Group_2__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10343:1: ( rule__IntegerDataType__Group_2__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10344:2: rule__IntegerDataType__Group_2__1__Impl
{
pushFollow(FollowSets001.FOLLOW_rule__IntegerDataType__Group_2__1__Impl_in_rule__IntegerDataType__Group_2__121844);
rule__IntegerDataType__Group_2__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__IntegerDataType__Group_2__1"
// $ANTLR start "rule__IntegerDataType__Group_2__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10350:1: rule__IntegerDataType__Group_2__1__Impl : ( ( rule__IntegerDataType__RefAssignment_2_1 ) ) ;
public final void rule__IntegerDataType__Group_2__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10354:1: ( ( ( rule__IntegerDataType__RefAssignment_2_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10355:1: ( ( rule__IntegerDataType__RefAssignment_2_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10355:1: ( ( rule__IntegerDataType__RefAssignment_2_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10356:1: ( rule__IntegerDataType__RefAssignment_2_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getIntegerDataTypeAccess().getRefAssignment_2_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10357:1: ( rule__IntegerDataType__RefAssignment_2_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10357:2: rule__IntegerDataType__RefAssignment_2_1
{
pushFollow(FollowSets001.FOLLOW_rule__IntegerDataType__RefAssignment_2_1_in_rule__IntegerDataType__Group_2__1__Impl21871);
rule__IntegerDataType__RefAssignment_2_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getIntegerDataTypeAccess().getRefAssignment_2_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__IntegerDataType__Group_2__1__Impl"
// $ANTLR start "rule__IntegerEntityAttribute__Group__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10371:1: rule__IntegerEntityAttribute__Group__0 : rule__IntegerEntityAttribute__Group__0__Impl rule__IntegerEntityAttribute__Group__1 ;
public final void rule__IntegerEntityAttribute__Group__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10375:1: ( rule__IntegerEntityAttribute__Group__0__Impl rule__IntegerEntityAttribute__Group__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10376:2: rule__IntegerEntityAttribute__Group__0__Impl rule__IntegerEntityAttribute__Group__1
{
pushFollow(FollowSets001.FOLLOW_rule__IntegerEntityAttribute__Group__0__Impl_in_rule__IntegerEntityAttribute__Group__021905);
rule__IntegerEntityAttribute__Group__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__IntegerEntityAttribute__Group__1_in_rule__IntegerEntityAttribute__Group__021908);
rule__IntegerEntityAttribute__Group__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__IntegerEntityAttribute__Group__0"
// $ANTLR start "rule__IntegerEntityAttribute__Group__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10383:1: rule__IntegerEntityAttribute__Group__0__Impl : ( 'integer' ) ;
public final void rule__IntegerEntityAttribute__Group__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10387:1: ( ( 'integer' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10388:1: ( 'integer' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10388:1: ( 'integer' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10389:1: 'integer'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getIntegerEntityAttributeAccess().getIntegerKeyword_0());
}
match(input,57,FollowSets001.FOLLOW_57_in_rule__IntegerEntityAttribute__Group__0__Impl21936); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getIntegerEntityAttributeAccess().getIntegerKeyword_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__IntegerEntityAttribute__Group__0__Impl"
// $ANTLR start "rule__IntegerEntityAttribute__Group__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10402:1: rule__IntegerEntityAttribute__Group__1 : rule__IntegerEntityAttribute__Group__1__Impl rule__IntegerEntityAttribute__Group__2 ;
public final void rule__IntegerEntityAttribute__Group__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10406:1: ( rule__IntegerEntityAttribute__Group__1__Impl rule__IntegerEntityAttribute__Group__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10407:2: rule__IntegerEntityAttribute__Group__1__Impl rule__IntegerEntityAttribute__Group__2
{
pushFollow(FollowSets001.FOLLOW_rule__IntegerEntityAttribute__Group__1__Impl_in_rule__IntegerEntityAttribute__Group__121967);
rule__IntegerEntityAttribute__Group__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__IntegerEntityAttribute__Group__2_in_rule__IntegerEntityAttribute__Group__121970);
rule__IntegerEntityAttribute__Group__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__IntegerEntityAttribute__Group__1"
// $ANTLR start "rule__IntegerEntityAttribute__Group__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10414:1: rule__IntegerEntityAttribute__Group__1__Impl : ( ( rule__IntegerEntityAttribute__TypeAssignment_1 )? ) ;
public final void rule__IntegerEntityAttribute__Group__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10418:1: ( ( ( rule__IntegerEntityAttribute__TypeAssignment_1 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10419:1: ( ( rule__IntegerEntityAttribute__TypeAssignment_1 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10419:1: ( ( rule__IntegerEntityAttribute__TypeAssignment_1 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10420:1: ( rule__IntegerEntityAttribute__TypeAssignment_1 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getIntegerEntityAttributeAccess().getTypeAssignment_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10421:1: ( rule__IntegerEntityAttribute__TypeAssignment_1 )?
int alt85=2;
int LA85_0 = input.LA(1);
if ( (LA85_0==RULE_ID) ) {
int LA85_1 = input.LA(2);
if ( (LA85_1==RULE_ID||LA85_1==43) ) {
alt85=1;
}
}
switch (alt85) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10421:2: rule__IntegerEntityAttribute__TypeAssignment_1
{
pushFollow(FollowSets001.FOLLOW_rule__IntegerEntityAttribute__TypeAssignment_1_in_rule__IntegerEntityAttribute__Group__1__Impl21997);
rule__IntegerEntityAttribute__TypeAssignment_1();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getIntegerEntityAttributeAccess().getTypeAssignment_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__IntegerEntityAttribute__Group__1__Impl"
// $ANTLR start "rule__IntegerEntityAttribute__Group__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10431:1: rule__IntegerEntityAttribute__Group__2 : rule__IntegerEntityAttribute__Group__2__Impl ;
public final void rule__IntegerEntityAttribute__Group__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10435:1: ( rule__IntegerEntityAttribute__Group__2__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10436:2: rule__IntegerEntityAttribute__Group__2__Impl
{
pushFollow(FollowSets001.FOLLOW_rule__IntegerEntityAttribute__Group__2__Impl_in_rule__IntegerEntityAttribute__Group__222028);
rule__IntegerEntityAttribute__Group__2__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__IntegerEntityAttribute__Group__2"
// $ANTLR start "rule__IntegerEntityAttribute__Group__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10442:1: rule__IntegerEntityAttribute__Group__2__Impl : ( ( rule__IntegerEntityAttribute__NameAssignment_2 ) ) ;
public final void rule__IntegerEntityAttribute__Group__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10446:1: ( ( ( rule__IntegerEntityAttribute__NameAssignment_2 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10447:1: ( ( rule__IntegerEntityAttribute__NameAssignment_2 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10447:1: ( ( rule__IntegerEntityAttribute__NameAssignment_2 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10448:1: ( rule__IntegerEntityAttribute__NameAssignment_2 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getIntegerEntityAttributeAccess().getNameAssignment_2());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10449:1: ( rule__IntegerEntityAttribute__NameAssignment_2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10449:2: rule__IntegerEntityAttribute__NameAssignment_2
{
pushFollow(FollowSets001.FOLLOW_rule__IntegerEntityAttribute__NameAssignment_2_in_rule__IntegerEntityAttribute__Group__2__Impl22055);
rule__IntegerEntityAttribute__NameAssignment_2();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getIntegerEntityAttributeAccess().getNameAssignment_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__IntegerEntityAttribute__Group__2__Impl"
// $ANTLR start "rule__DateDataType__Group__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10465:1: rule__DateDataType__Group__0 : rule__DateDataType__Group__0__Impl rule__DateDataType__Group__1 ;
public final void rule__DateDataType__Group__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10469:1: ( rule__DateDataType__Group__0__Impl rule__DateDataType__Group__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10470:2: rule__DateDataType__Group__0__Impl rule__DateDataType__Group__1
{
pushFollow(FollowSets001.FOLLOW_rule__DateDataType__Group__0__Impl_in_rule__DateDataType__Group__022091);
rule__DateDataType__Group__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DateDataType__Group__1_in_rule__DateDataType__Group__022094);
rule__DateDataType__Group__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DateDataType__Group__0"
// $ANTLR start "rule__DateDataType__Group__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10477:1: rule__DateDataType__Group__0__Impl : ( 'datedatatype' ) ;
public final void rule__DateDataType__Group__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10481:1: ( ( 'datedatatype' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10482:1: ( 'datedatatype' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10482:1: ( 'datedatatype' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10483:1: 'datedatatype'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDateDataTypeAccess().getDatedatatypeKeyword_0());
}
match(input,88,FollowSets001.FOLLOW_88_in_rule__DateDataType__Group__0__Impl22122); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDateDataTypeAccess().getDatedatatypeKeyword_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DateDataType__Group__0__Impl"
// $ANTLR start "rule__DateDataType__Group__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10496:1: rule__DateDataType__Group__1 : rule__DateDataType__Group__1__Impl rule__DateDataType__Group__2 ;
public final void rule__DateDataType__Group__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10500:1: ( rule__DateDataType__Group__1__Impl rule__DateDataType__Group__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10501:2: rule__DateDataType__Group__1__Impl rule__DateDataType__Group__2
{
pushFollow(FollowSets001.FOLLOW_rule__DateDataType__Group__1__Impl_in_rule__DateDataType__Group__122153);
rule__DateDataType__Group__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DateDataType__Group__2_in_rule__DateDataType__Group__122156);
rule__DateDataType__Group__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DateDataType__Group__1"
// $ANTLR start "rule__DateDataType__Group__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10508:1: rule__DateDataType__Group__1__Impl : ( ( rule__DateDataType__NameAssignment_1 ) ) ;
public final void rule__DateDataType__Group__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10512:1: ( ( ( rule__DateDataType__NameAssignment_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10513:1: ( ( rule__DateDataType__NameAssignment_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10513:1: ( ( rule__DateDataType__NameAssignment_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10514:1: ( rule__DateDataType__NameAssignment_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDateDataTypeAccess().getNameAssignment_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10515:1: ( rule__DateDataType__NameAssignment_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10515:2: rule__DateDataType__NameAssignment_1
{
pushFollow(FollowSets001.FOLLOW_rule__DateDataType__NameAssignment_1_in_rule__DateDataType__Group__1__Impl22183);
rule__DateDataType__NameAssignment_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDateDataTypeAccess().getNameAssignment_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DateDataType__Group__1__Impl"
// $ANTLR start "rule__DateDataType__Group__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10525:1: rule__DateDataType__Group__2 : rule__DateDataType__Group__2__Impl rule__DateDataType__Group__3 ;
public final void rule__DateDataType__Group__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10529:1: ( rule__DateDataType__Group__2__Impl rule__DateDataType__Group__3 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10530:2: rule__DateDataType__Group__2__Impl rule__DateDataType__Group__3
{
pushFollow(FollowSets001.FOLLOW_rule__DateDataType__Group__2__Impl_in_rule__DateDataType__Group__222213);
rule__DateDataType__Group__2__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DateDataType__Group__3_in_rule__DateDataType__Group__222216);
rule__DateDataType__Group__3();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DateDataType__Group__2"
// $ANTLR start "rule__DateDataType__Group__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10537:1: rule__DateDataType__Group__2__Impl : ( ( rule__DateDataType__Group_2__0 )? ) ;
public final void rule__DateDataType__Group__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10541:1: ( ( ( rule__DateDataType__Group_2__0 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10542:1: ( ( rule__DateDataType__Group_2__0 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10542:1: ( ( rule__DateDataType__Group_2__0 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10543:1: ( rule__DateDataType__Group_2__0 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDateDataTypeAccess().getGroup_2());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10544:1: ( rule__DateDataType__Group_2__0 )?
int alt86=2;
int LA86_0 = input.LA(1);
if ( (LA86_0==45) ) {
alt86=1;
}
switch (alt86) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10544:2: rule__DateDataType__Group_2__0
{
pushFollow(FollowSets001.FOLLOW_rule__DateDataType__Group_2__0_in_rule__DateDataType__Group__2__Impl22243);
rule__DateDataType__Group_2__0();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDateDataTypeAccess().getGroup_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DateDataType__Group__2__Impl"
// $ANTLR start "rule__DateDataType__Group__3"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10554:1: rule__DateDataType__Group__3 : rule__DateDataType__Group__3__Impl rule__DateDataType__Group__4 ;
public final void rule__DateDataType__Group__3() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10558:1: ( rule__DateDataType__Group__3__Impl rule__DateDataType__Group__4 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10559:2: rule__DateDataType__Group__3__Impl rule__DateDataType__Group__4
{
pushFollow(FollowSets001.FOLLOW_rule__DateDataType__Group__3__Impl_in_rule__DateDataType__Group__322274);
rule__DateDataType__Group__3__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DateDataType__Group__4_in_rule__DateDataType__Group__322277);
rule__DateDataType__Group__4();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DateDataType__Group__3"
// $ANTLR start "rule__DateDataType__Group__3__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10566:1: rule__DateDataType__Group__3__Impl : ( '{' ) ;
public final void rule__DateDataType__Group__3__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10570:1: ( ( '{' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10571:1: ( '{' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10571:1: ( '{' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10572:1: '{'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDateDataTypeAccess().getLeftCurlyBracketKeyword_3());
}
match(input,67,FollowSets001.FOLLOW_67_in_rule__DateDataType__Group__3__Impl22305); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDateDataTypeAccess().getLeftCurlyBracketKeyword_3());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DateDataType__Group__3__Impl"
// $ANTLR start "rule__DateDataType__Group__4"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10585:1: rule__DateDataType__Group__4 : rule__DateDataType__Group__4__Impl rule__DateDataType__Group__5 ;
public final void rule__DateDataType__Group__4() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10589:1: ( rule__DateDataType__Group__4__Impl rule__DateDataType__Group__5 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10590:2: rule__DateDataType__Group__4__Impl rule__DateDataType__Group__5
{
pushFollow(FollowSets001.FOLLOW_rule__DateDataType__Group__4__Impl_in_rule__DateDataType__Group__422336);
rule__DateDataType__Group__4__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DateDataType__Group__5_in_rule__DateDataType__Group__422339);
rule__DateDataType__Group__5();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DateDataType__Group__4"
// $ANTLR start "rule__DateDataType__Group__4__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10597:1: rule__DateDataType__Group__4__Impl : ( ( rule__DateDataType__BaseDataTypeAssignment_4 ) ) ;
public final void rule__DateDataType__Group__4__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10601:1: ( ( ( rule__DateDataType__BaseDataTypeAssignment_4 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10602:1: ( ( rule__DateDataType__BaseDataTypeAssignment_4 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10602:1: ( ( rule__DateDataType__BaseDataTypeAssignment_4 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10603:1: ( rule__DateDataType__BaseDataTypeAssignment_4 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDateDataTypeAccess().getBaseDataTypeAssignment_4());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10604:1: ( rule__DateDataType__BaseDataTypeAssignment_4 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10604:2: rule__DateDataType__BaseDataTypeAssignment_4
{
pushFollow(FollowSets001.FOLLOW_rule__DateDataType__BaseDataTypeAssignment_4_in_rule__DateDataType__Group__4__Impl22366);
rule__DateDataType__BaseDataTypeAssignment_4();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDateDataTypeAccess().getBaseDataTypeAssignment_4());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DateDataType__Group__4__Impl"
// $ANTLR start "rule__DateDataType__Group__5"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10614:1: rule__DateDataType__Group__5 : rule__DateDataType__Group__5__Impl ;
public final void rule__DateDataType__Group__5() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10618:1: ( rule__DateDataType__Group__5__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10619:2: rule__DateDataType__Group__5__Impl
{
pushFollow(FollowSets001.FOLLOW_rule__DateDataType__Group__5__Impl_in_rule__DateDataType__Group__522396);
rule__DateDataType__Group__5__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DateDataType__Group__5"
// $ANTLR start "rule__DateDataType__Group__5__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10625:1: rule__DateDataType__Group__5__Impl : ( '}' ) ;
public final void rule__DateDataType__Group__5__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10629:1: ( ( '}' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10630:1: ( '}' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10630:1: ( '}' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10631:1: '}'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDateDataTypeAccess().getRightCurlyBracketKeyword_5());
}
match(input,68,FollowSets001.FOLLOW_68_in_rule__DateDataType__Group__5__Impl22424); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDateDataTypeAccess().getRightCurlyBracketKeyword_5());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DateDataType__Group__5__Impl"
// $ANTLR start "rule__DateDataType__Group_2__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10656:1: rule__DateDataType__Group_2__0 : rule__DateDataType__Group_2__0__Impl rule__DateDataType__Group_2__1 ;
public final void rule__DateDataType__Group_2__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10660:1: ( rule__DateDataType__Group_2__0__Impl rule__DateDataType__Group_2__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10661:2: rule__DateDataType__Group_2__0__Impl rule__DateDataType__Group_2__1
{
pushFollow(FollowSets001.FOLLOW_rule__DateDataType__Group_2__0__Impl_in_rule__DateDataType__Group_2__022467);
rule__DateDataType__Group_2__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DateDataType__Group_2__1_in_rule__DateDataType__Group_2__022470);
rule__DateDataType__Group_2__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DateDataType__Group_2__0"
// $ANTLR start "rule__DateDataType__Group_2__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10668:1: rule__DateDataType__Group_2__0__Impl : ( 'extends' ) ;
public final void rule__DateDataType__Group_2__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10672:1: ( ( 'extends' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10673:1: ( 'extends' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10673:1: ( 'extends' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10674:1: 'extends'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDateDataTypeAccess().getExtendsKeyword_2_0());
}
match(input,45,FollowSets001.FOLLOW_45_in_rule__DateDataType__Group_2__0__Impl22498); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDateDataTypeAccess().getExtendsKeyword_2_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DateDataType__Group_2__0__Impl"
// $ANTLR start "rule__DateDataType__Group_2__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10687:1: rule__DateDataType__Group_2__1 : rule__DateDataType__Group_2__1__Impl ;
public final void rule__DateDataType__Group_2__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10691:1: ( rule__DateDataType__Group_2__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10692:2: rule__DateDataType__Group_2__1__Impl
{
pushFollow(FollowSets001.FOLLOW_rule__DateDataType__Group_2__1__Impl_in_rule__DateDataType__Group_2__122529);
rule__DateDataType__Group_2__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DateDataType__Group_2__1"
// $ANTLR start "rule__DateDataType__Group_2__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10698:1: rule__DateDataType__Group_2__1__Impl : ( ( rule__DateDataType__RefAssignment_2_1 ) ) ;
public final void rule__DateDataType__Group_2__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10702:1: ( ( ( rule__DateDataType__RefAssignment_2_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10703:1: ( ( rule__DateDataType__RefAssignment_2_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10703:1: ( ( rule__DateDataType__RefAssignment_2_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10704:1: ( rule__DateDataType__RefAssignment_2_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDateDataTypeAccess().getRefAssignment_2_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10705:1: ( rule__DateDataType__RefAssignment_2_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10705:2: rule__DateDataType__RefAssignment_2_1
{
pushFollow(FollowSets001.FOLLOW_rule__DateDataType__RefAssignment_2_1_in_rule__DateDataType__Group_2__1__Impl22556);
rule__DateDataType__RefAssignment_2_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDateDataTypeAccess().getRefAssignment_2_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DateDataType__Group_2__1__Impl"
// $ANTLR start "rule__DateEntityAttribute__Group__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10719:1: rule__DateEntityAttribute__Group__0 : rule__DateEntityAttribute__Group__0__Impl rule__DateEntityAttribute__Group__1 ;
public final void rule__DateEntityAttribute__Group__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10723:1: ( rule__DateEntityAttribute__Group__0__Impl rule__DateEntityAttribute__Group__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10724:2: rule__DateEntityAttribute__Group__0__Impl rule__DateEntityAttribute__Group__1
{
pushFollow(FollowSets001.FOLLOW_rule__DateEntityAttribute__Group__0__Impl_in_rule__DateEntityAttribute__Group__022590);
rule__DateEntityAttribute__Group__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DateEntityAttribute__Group__1_in_rule__DateEntityAttribute__Group__022593);
rule__DateEntityAttribute__Group__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DateEntityAttribute__Group__0"
// $ANTLR start "rule__DateEntityAttribute__Group__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10731:1: rule__DateEntityAttribute__Group__0__Impl : ( 'date' ) ;
public final void rule__DateEntityAttribute__Group__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10735:1: ( ( 'date' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10736:1: ( 'date' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10736:1: ( 'date' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10737:1: 'date'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDateEntityAttributeAccess().getDateKeyword_0());
}
match(input,89,FollowSets001.FOLLOW_89_in_rule__DateEntityAttribute__Group__0__Impl22621); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDateEntityAttributeAccess().getDateKeyword_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DateEntityAttribute__Group__0__Impl"
// $ANTLR start "rule__DateEntityAttribute__Group__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10750:1: rule__DateEntityAttribute__Group__1 : rule__DateEntityAttribute__Group__1__Impl rule__DateEntityAttribute__Group__2 ;
public final void rule__DateEntityAttribute__Group__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10754:1: ( rule__DateEntityAttribute__Group__1__Impl rule__DateEntityAttribute__Group__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10755:2: rule__DateEntityAttribute__Group__1__Impl rule__DateEntityAttribute__Group__2
{
pushFollow(FollowSets001.FOLLOW_rule__DateEntityAttribute__Group__1__Impl_in_rule__DateEntityAttribute__Group__122652);
rule__DateEntityAttribute__Group__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DateEntityAttribute__Group__2_in_rule__DateEntityAttribute__Group__122655);
rule__DateEntityAttribute__Group__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DateEntityAttribute__Group__1"
// $ANTLR start "rule__DateEntityAttribute__Group__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10762:1: rule__DateEntityAttribute__Group__1__Impl : ( ( rule__DateEntityAttribute__TypeAssignment_1 )? ) ;
public final void rule__DateEntityAttribute__Group__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10766:1: ( ( ( rule__DateEntityAttribute__TypeAssignment_1 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10767:1: ( ( rule__DateEntityAttribute__TypeAssignment_1 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10767:1: ( ( rule__DateEntityAttribute__TypeAssignment_1 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10768:1: ( rule__DateEntityAttribute__TypeAssignment_1 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDateEntityAttributeAccess().getTypeAssignment_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10769:1: ( rule__DateEntityAttribute__TypeAssignment_1 )?
int alt87=2;
int LA87_0 = input.LA(1);
if ( (LA87_0==RULE_ID) ) {
int LA87_1 = input.LA(2);
if ( (LA87_1==RULE_ID||LA87_1==43) ) {
alt87=1;
}
}
switch (alt87) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10769:2: rule__DateEntityAttribute__TypeAssignment_1
{
pushFollow(FollowSets001.FOLLOW_rule__DateEntityAttribute__TypeAssignment_1_in_rule__DateEntityAttribute__Group__1__Impl22682);
rule__DateEntityAttribute__TypeAssignment_1();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDateEntityAttributeAccess().getTypeAssignment_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DateEntityAttribute__Group__1__Impl"
// $ANTLR start "rule__DateEntityAttribute__Group__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10779:1: rule__DateEntityAttribute__Group__2 : rule__DateEntityAttribute__Group__2__Impl ;
public final void rule__DateEntityAttribute__Group__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10783:1: ( rule__DateEntityAttribute__Group__2__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10784:2: rule__DateEntityAttribute__Group__2__Impl
{
pushFollow(FollowSets001.FOLLOW_rule__DateEntityAttribute__Group__2__Impl_in_rule__DateEntityAttribute__Group__222713);
rule__DateEntityAttribute__Group__2__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DateEntityAttribute__Group__2"
// $ANTLR start "rule__DateEntityAttribute__Group__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10790:1: rule__DateEntityAttribute__Group__2__Impl : ( ( rule__DateEntityAttribute__NameAssignment_2 ) ) ;
public final void rule__DateEntityAttribute__Group__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10794:1: ( ( ( rule__DateEntityAttribute__NameAssignment_2 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10795:1: ( ( rule__DateEntityAttribute__NameAssignment_2 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10795:1: ( ( rule__DateEntityAttribute__NameAssignment_2 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10796:1: ( rule__DateEntityAttribute__NameAssignment_2 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDateEntityAttributeAccess().getNameAssignment_2());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10797:1: ( rule__DateEntityAttribute__NameAssignment_2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10797:2: rule__DateEntityAttribute__NameAssignment_2
{
pushFollow(FollowSets001.FOLLOW_rule__DateEntityAttribute__NameAssignment_2_in_rule__DateEntityAttribute__Group__2__Impl22740);
rule__DateEntityAttribute__NameAssignment_2();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDateEntityAttributeAccess().getNameAssignment_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DateEntityAttribute__Group__2__Impl"
// $ANTLR start "rule__DecimalDataType__Group__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10813:1: rule__DecimalDataType__Group__0 : rule__DecimalDataType__Group__0__Impl rule__DecimalDataType__Group__1 ;
public final void rule__DecimalDataType__Group__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10817:1: ( rule__DecimalDataType__Group__0__Impl rule__DecimalDataType__Group__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10818:2: rule__DecimalDataType__Group__0__Impl rule__DecimalDataType__Group__1
{
pushFollow(FollowSets001.FOLLOW_rule__DecimalDataType__Group__0__Impl_in_rule__DecimalDataType__Group__022776);
rule__DecimalDataType__Group__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DecimalDataType__Group__1_in_rule__DecimalDataType__Group__022779);
rule__DecimalDataType__Group__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DecimalDataType__Group__0"
// $ANTLR start "rule__DecimalDataType__Group__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10825:1: rule__DecimalDataType__Group__0__Impl : ( 'decimaldatatype' ) ;
public final void rule__DecimalDataType__Group__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10829:1: ( ( 'decimaldatatype' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10830:1: ( 'decimaldatatype' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10830:1: ( 'decimaldatatype' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10831:1: 'decimaldatatype'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDecimalDataTypeAccess().getDecimaldatatypeKeyword_0());
}
match(input,90,FollowSets001.FOLLOW_90_in_rule__DecimalDataType__Group__0__Impl22807); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDecimalDataTypeAccess().getDecimaldatatypeKeyword_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DecimalDataType__Group__0__Impl"
// $ANTLR start "rule__DecimalDataType__Group__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10844:1: rule__DecimalDataType__Group__1 : rule__DecimalDataType__Group__1__Impl rule__DecimalDataType__Group__2 ;
public final void rule__DecimalDataType__Group__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10848:1: ( rule__DecimalDataType__Group__1__Impl rule__DecimalDataType__Group__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10849:2: rule__DecimalDataType__Group__1__Impl rule__DecimalDataType__Group__2
{
pushFollow(FollowSets001.FOLLOW_rule__DecimalDataType__Group__1__Impl_in_rule__DecimalDataType__Group__122838);
rule__DecimalDataType__Group__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DecimalDataType__Group__2_in_rule__DecimalDataType__Group__122841);
rule__DecimalDataType__Group__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DecimalDataType__Group__1"
// $ANTLR start "rule__DecimalDataType__Group__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10856:1: rule__DecimalDataType__Group__1__Impl : ( ( rule__DecimalDataType__NameAssignment_1 ) ) ;
public final void rule__DecimalDataType__Group__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10860:1: ( ( ( rule__DecimalDataType__NameAssignment_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10861:1: ( ( rule__DecimalDataType__NameAssignment_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10861:1: ( ( rule__DecimalDataType__NameAssignment_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10862:1: ( rule__DecimalDataType__NameAssignment_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDecimalDataTypeAccess().getNameAssignment_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10863:1: ( rule__DecimalDataType__NameAssignment_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10863:2: rule__DecimalDataType__NameAssignment_1
{
pushFollow(FollowSets001.FOLLOW_rule__DecimalDataType__NameAssignment_1_in_rule__DecimalDataType__Group__1__Impl22868);
rule__DecimalDataType__NameAssignment_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDecimalDataTypeAccess().getNameAssignment_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DecimalDataType__Group__1__Impl"
// $ANTLR start "rule__DecimalDataType__Group__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10873:1: rule__DecimalDataType__Group__2 : rule__DecimalDataType__Group__2__Impl rule__DecimalDataType__Group__3 ;
public final void rule__DecimalDataType__Group__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10877:1: ( rule__DecimalDataType__Group__2__Impl rule__DecimalDataType__Group__3 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10878:2: rule__DecimalDataType__Group__2__Impl rule__DecimalDataType__Group__3
{
pushFollow(FollowSets001.FOLLOW_rule__DecimalDataType__Group__2__Impl_in_rule__DecimalDataType__Group__222898);
rule__DecimalDataType__Group__2__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DecimalDataType__Group__3_in_rule__DecimalDataType__Group__222901);
rule__DecimalDataType__Group__3();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DecimalDataType__Group__2"
// $ANTLR start "rule__DecimalDataType__Group__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10885:1: rule__DecimalDataType__Group__2__Impl : ( ( rule__DecimalDataType__Group_2__0 )? ) ;
public final void rule__DecimalDataType__Group__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10889:1: ( ( ( rule__DecimalDataType__Group_2__0 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10890:1: ( ( rule__DecimalDataType__Group_2__0 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10890:1: ( ( rule__DecimalDataType__Group_2__0 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10891:1: ( rule__DecimalDataType__Group_2__0 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDecimalDataTypeAccess().getGroup_2());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10892:1: ( rule__DecimalDataType__Group_2__0 )?
int alt88=2;
int LA88_0 = input.LA(1);
if ( (LA88_0==45) ) {
alt88=1;
}
switch (alt88) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10892:2: rule__DecimalDataType__Group_2__0
{
pushFollow(FollowSets001.FOLLOW_rule__DecimalDataType__Group_2__0_in_rule__DecimalDataType__Group__2__Impl22928);
rule__DecimalDataType__Group_2__0();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDecimalDataTypeAccess().getGroup_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DecimalDataType__Group__2__Impl"
// $ANTLR start "rule__DecimalDataType__Group__3"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10902:1: rule__DecimalDataType__Group__3 : rule__DecimalDataType__Group__3__Impl rule__DecimalDataType__Group__4 ;
public final void rule__DecimalDataType__Group__3() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10906:1: ( rule__DecimalDataType__Group__3__Impl rule__DecimalDataType__Group__4 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10907:2: rule__DecimalDataType__Group__3__Impl rule__DecimalDataType__Group__4
{
pushFollow(FollowSets001.FOLLOW_rule__DecimalDataType__Group__3__Impl_in_rule__DecimalDataType__Group__322959);
rule__DecimalDataType__Group__3__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DecimalDataType__Group__4_in_rule__DecimalDataType__Group__322962);
rule__DecimalDataType__Group__4();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DecimalDataType__Group__3"
// $ANTLR start "rule__DecimalDataType__Group__3__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10914:1: rule__DecimalDataType__Group__3__Impl : ( '{' ) ;
public final void rule__DecimalDataType__Group__3__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10918:1: ( ( '{' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10919:1: ( '{' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10919:1: ( '{' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10920:1: '{'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDecimalDataTypeAccess().getLeftCurlyBracketKeyword_3());
}
match(input,67,FollowSets001.FOLLOW_67_in_rule__DecimalDataType__Group__3__Impl22990); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDecimalDataTypeAccess().getLeftCurlyBracketKeyword_3());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DecimalDataType__Group__3__Impl"
// $ANTLR start "rule__DecimalDataType__Group__4"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10933:1: rule__DecimalDataType__Group__4 : rule__DecimalDataType__Group__4__Impl rule__DecimalDataType__Group__5 ;
public final void rule__DecimalDataType__Group__4() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10937:1: ( rule__DecimalDataType__Group__4__Impl rule__DecimalDataType__Group__5 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10938:2: rule__DecimalDataType__Group__4__Impl rule__DecimalDataType__Group__5
{
pushFollow(FollowSets001.FOLLOW_rule__DecimalDataType__Group__4__Impl_in_rule__DecimalDataType__Group__423021);
rule__DecimalDataType__Group__4__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DecimalDataType__Group__5_in_rule__DecimalDataType__Group__423024);
rule__DecimalDataType__Group__5();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DecimalDataType__Group__4"
// $ANTLR start "rule__DecimalDataType__Group__4__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10945:1: rule__DecimalDataType__Group__4__Impl : ( ( rule__DecimalDataType__BaseDataTypeAssignment_4 ) ) ;
public final void rule__DecimalDataType__Group__4__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10949:1: ( ( ( rule__DecimalDataType__BaseDataTypeAssignment_4 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10950:1: ( ( rule__DecimalDataType__BaseDataTypeAssignment_4 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10950:1: ( ( rule__DecimalDataType__BaseDataTypeAssignment_4 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10951:1: ( rule__DecimalDataType__BaseDataTypeAssignment_4 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDecimalDataTypeAccess().getBaseDataTypeAssignment_4());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10952:1: ( rule__DecimalDataType__BaseDataTypeAssignment_4 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10952:2: rule__DecimalDataType__BaseDataTypeAssignment_4
{
pushFollow(FollowSets001.FOLLOW_rule__DecimalDataType__BaseDataTypeAssignment_4_in_rule__DecimalDataType__Group__4__Impl23051);
rule__DecimalDataType__BaseDataTypeAssignment_4();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDecimalDataTypeAccess().getBaseDataTypeAssignment_4());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DecimalDataType__Group__4__Impl"
// $ANTLR start "rule__DecimalDataType__Group__5"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10962:1: rule__DecimalDataType__Group__5 : rule__DecimalDataType__Group__5__Impl ;
public final void rule__DecimalDataType__Group__5() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10966:1: ( rule__DecimalDataType__Group__5__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10967:2: rule__DecimalDataType__Group__5__Impl
{
pushFollow(FollowSets001.FOLLOW_rule__DecimalDataType__Group__5__Impl_in_rule__DecimalDataType__Group__523081);
rule__DecimalDataType__Group__5__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DecimalDataType__Group__5"
// $ANTLR start "rule__DecimalDataType__Group__5__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10973:1: rule__DecimalDataType__Group__5__Impl : ( '}' ) ;
public final void rule__DecimalDataType__Group__5__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10977:1: ( ( '}' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10978:1: ( '}' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10978:1: ( '}' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:10979:1: '}'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDecimalDataTypeAccess().getRightCurlyBracketKeyword_5());
}
match(input,68,FollowSets001.FOLLOW_68_in_rule__DecimalDataType__Group__5__Impl23109); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDecimalDataTypeAccess().getRightCurlyBracketKeyword_5());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DecimalDataType__Group__5__Impl"
// $ANTLR start "rule__DecimalDataType__Group_2__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11004:1: rule__DecimalDataType__Group_2__0 : rule__DecimalDataType__Group_2__0__Impl rule__DecimalDataType__Group_2__1 ;
public final void rule__DecimalDataType__Group_2__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11008:1: ( rule__DecimalDataType__Group_2__0__Impl rule__DecimalDataType__Group_2__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11009:2: rule__DecimalDataType__Group_2__0__Impl rule__DecimalDataType__Group_2__1
{
pushFollow(FollowSets001.FOLLOW_rule__DecimalDataType__Group_2__0__Impl_in_rule__DecimalDataType__Group_2__023152);
rule__DecimalDataType__Group_2__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DecimalDataType__Group_2__1_in_rule__DecimalDataType__Group_2__023155);
rule__DecimalDataType__Group_2__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DecimalDataType__Group_2__0"
// $ANTLR start "rule__DecimalDataType__Group_2__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11016:1: rule__DecimalDataType__Group_2__0__Impl : ( 'extends' ) ;
public final void rule__DecimalDataType__Group_2__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11020:1: ( ( 'extends' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11021:1: ( 'extends' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11021:1: ( 'extends' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11022:1: 'extends'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDecimalDataTypeAccess().getExtendsKeyword_2_0());
}
match(input,45,FollowSets001.FOLLOW_45_in_rule__DecimalDataType__Group_2__0__Impl23183); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDecimalDataTypeAccess().getExtendsKeyword_2_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DecimalDataType__Group_2__0__Impl"
// $ANTLR start "rule__DecimalDataType__Group_2__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11035:1: rule__DecimalDataType__Group_2__1 : rule__DecimalDataType__Group_2__1__Impl ;
public final void rule__DecimalDataType__Group_2__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11039:1: ( rule__DecimalDataType__Group_2__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11040:2: rule__DecimalDataType__Group_2__1__Impl
{
pushFollow(FollowSets001.FOLLOW_rule__DecimalDataType__Group_2__1__Impl_in_rule__DecimalDataType__Group_2__123214);
rule__DecimalDataType__Group_2__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DecimalDataType__Group_2__1"
// $ANTLR start "rule__DecimalDataType__Group_2__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11046:1: rule__DecimalDataType__Group_2__1__Impl : ( ( rule__DecimalDataType__RefAssignment_2_1 ) ) ;
public final void rule__DecimalDataType__Group_2__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11050:1: ( ( ( rule__DecimalDataType__RefAssignment_2_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11051:1: ( ( rule__DecimalDataType__RefAssignment_2_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11051:1: ( ( rule__DecimalDataType__RefAssignment_2_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11052:1: ( rule__DecimalDataType__RefAssignment_2_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDecimalDataTypeAccess().getRefAssignment_2_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11053:1: ( rule__DecimalDataType__RefAssignment_2_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11053:2: rule__DecimalDataType__RefAssignment_2_1
{
pushFollow(FollowSets001.FOLLOW_rule__DecimalDataType__RefAssignment_2_1_in_rule__DecimalDataType__Group_2__1__Impl23241);
rule__DecimalDataType__RefAssignment_2_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDecimalDataTypeAccess().getRefAssignment_2_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DecimalDataType__Group_2__1__Impl"
// $ANTLR start "rule__DecimalEntityAttribute__Group__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11067:1: rule__DecimalEntityAttribute__Group__0 : rule__DecimalEntityAttribute__Group__0__Impl rule__DecimalEntityAttribute__Group__1 ;
public final void rule__DecimalEntityAttribute__Group__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11071:1: ( rule__DecimalEntityAttribute__Group__0__Impl rule__DecimalEntityAttribute__Group__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11072:2: rule__DecimalEntityAttribute__Group__0__Impl rule__DecimalEntityAttribute__Group__1
{
pushFollow(FollowSets001.FOLLOW_rule__DecimalEntityAttribute__Group__0__Impl_in_rule__DecimalEntityAttribute__Group__023275);
rule__DecimalEntityAttribute__Group__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DecimalEntityAttribute__Group__1_in_rule__DecimalEntityAttribute__Group__023278);
rule__DecimalEntityAttribute__Group__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DecimalEntityAttribute__Group__0"
// $ANTLR start "rule__DecimalEntityAttribute__Group__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11079:1: rule__DecimalEntityAttribute__Group__0__Impl : ( 'decimal' ) ;
public final void rule__DecimalEntityAttribute__Group__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11083:1: ( ( 'decimal' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11084:1: ( 'decimal' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11084:1: ( 'decimal' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11085:1: 'decimal'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDecimalEntityAttributeAccess().getDecimalKeyword_0());
}
match(input,91,FollowSets001.FOLLOW_91_in_rule__DecimalEntityAttribute__Group__0__Impl23306); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDecimalEntityAttributeAccess().getDecimalKeyword_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DecimalEntityAttribute__Group__0__Impl"
// $ANTLR start "rule__DecimalEntityAttribute__Group__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11098:1: rule__DecimalEntityAttribute__Group__1 : rule__DecimalEntityAttribute__Group__1__Impl rule__DecimalEntityAttribute__Group__2 ;
public final void rule__DecimalEntityAttribute__Group__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11102:1: ( rule__DecimalEntityAttribute__Group__1__Impl rule__DecimalEntityAttribute__Group__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11103:2: rule__DecimalEntityAttribute__Group__1__Impl rule__DecimalEntityAttribute__Group__2
{
pushFollow(FollowSets001.FOLLOW_rule__DecimalEntityAttribute__Group__1__Impl_in_rule__DecimalEntityAttribute__Group__123337);
rule__DecimalEntityAttribute__Group__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DecimalEntityAttribute__Group__2_in_rule__DecimalEntityAttribute__Group__123340);
rule__DecimalEntityAttribute__Group__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DecimalEntityAttribute__Group__1"
// $ANTLR start "rule__DecimalEntityAttribute__Group__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11110:1: rule__DecimalEntityAttribute__Group__1__Impl : ( ( rule__DecimalEntityAttribute__TypeAssignment_1 )? ) ;
public final void rule__DecimalEntityAttribute__Group__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11114:1: ( ( ( rule__DecimalEntityAttribute__TypeAssignment_1 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11115:1: ( ( rule__DecimalEntityAttribute__TypeAssignment_1 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11115:1: ( ( rule__DecimalEntityAttribute__TypeAssignment_1 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11116:1: ( rule__DecimalEntityAttribute__TypeAssignment_1 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDecimalEntityAttributeAccess().getTypeAssignment_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11117:1: ( rule__DecimalEntityAttribute__TypeAssignment_1 )?
int alt89=2;
int LA89_0 = input.LA(1);
if ( (LA89_0==RULE_ID) ) {
int LA89_1 = input.LA(2);
if ( (LA89_1==RULE_ID||LA89_1==43) ) {
alt89=1;
}
}
switch (alt89) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11117:2: rule__DecimalEntityAttribute__TypeAssignment_1
{
pushFollow(FollowSets001.FOLLOW_rule__DecimalEntityAttribute__TypeAssignment_1_in_rule__DecimalEntityAttribute__Group__1__Impl23367);
rule__DecimalEntityAttribute__TypeAssignment_1();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDecimalEntityAttributeAccess().getTypeAssignment_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DecimalEntityAttribute__Group__1__Impl"
// $ANTLR start "rule__DecimalEntityAttribute__Group__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11127:1: rule__DecimalEntityAttribute__Group__2 : rule__DecimalEntityAttribute__Group__2__Impl ;
public final void rule__DecimalEntityAttribute__Group__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11131:1: ( rule__DecimalEntityAttribute__Group__2__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11132:2: rule__DecimalEntityAttribute__Group__2__Impl
{
pushFollow(FollowSets001.FOLLOW_rule__DecimalEntityAttribute__Group__2__Impl_in_rule__DecimalEntityAttribute__Group__223398);
rule__DecimalEntityAttribute__Group__2__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DecimalEntityAttribute__Group__2"
// $ANTLR start "rule__DecimalEntityAttribute__Group__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11138:1: rule__DecimalEntityAttribute__Group__2__Impl : ( ( rule__DecimalEntityAttribute__NameAssignment_2 ) ) ;
public final void rule__DecimalEntityAttribute__Group__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11142:1: ( ( ( rule__DecimalEntityAttribute__NameAssignment_2 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11143:1: ( ( rule__DecimalEntityAttribute__NameAssignment_2 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11143:1: ( ( rule__DecimalEntityAttribute__NameAssignment_2 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11144:1: ( rule__DecimalEntityAttribute__NameAssignment_2 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDecimalEntityAttributeAccess().getNameAssignment_2());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11145:1: ( rule__DecimalEntityAttribute__NameAssignment_2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11145:2: rule__DecimalEntityAttribute__NameAssignment_2
{
pushFollow(FollowSets001.FOLLOW_rule__DecimalEntityAttribute__NameAssignment_2_in_rule__DecimalEntityAttribute__Group__2__Impl23425);
rule__DecimalEntityAttribute__NameAssignment_2();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDecimalEntityAttributeAccess().getNameAssignment_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DecimalEntityAttribute__Group__2__Impl"
// $ANTLR start "rule__LongDataType__Group__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11161:1: rule__LongDataType__Group__0 : rule__LongDataType__Group__0__Impl rule__LongDataType__Group__1 ;
public final void rule__LongDataType__Group__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11165:1: ( rule__LongDataType__Group__0__Impl rule__LongDataType__Group__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11166:2: rule__LongDataType__Group__0__Impl rule__LongDataType__Group__1
{
pushFollow(FollowSets001.FOLLOW_rule__LongDataType__Group__0__Impl_in_rule__LongDataType__Group__023461);
rule__LongDataType__Group__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__LongDataType__Group__1_in_rule__LongDataType__Group__023464);
rule__LongDataType__Group__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__LongDataType__Group__0"
// $ANTLR start "rule__LongDataType__Group__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11173:1: rule__LongDataType__Group__0__Impl : ( 'longdatatype' ) ;
public final void rule__LongDataType__Group__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11177:1: ( ( 'longdatatype' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11178:1: ( 'longdatatype' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11178:1: ( 'longdatatype' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11179:1: 'longdatatype'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getLongDataTypeAccess().getLongdatatypeKeyword_0());
}
match(input,92,FollowSets001.FOLLOW_92_in_rule__LongDataType__Group__0__Impl23492); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getLongDataTypeAccess().getLongdatatypeKeyword_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__LongDataType__Group__0__Impl"
// $ANTLR start "rule__LongDataType__Group__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11192:1: rule__LongDataType__Group__1 : rule__LongDataType__Group__1__Impl rule__LongDataType__Group__2 ;
public final void rule__LongDataType__Group__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11196:1: ( rule__LongDataType__Group__1__Impl rule__LongDataType__Group__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11197:2: rule__LongDataType__Group__1__Impl rule__LongDataType__Group__2
{
pushFollow(FollowSets001.FOLLOW_rule__LongDataType__Group__1__Impl_in_rule__LongDataType__Group__123523);
rule__LongDataType__Group__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__LongDataType__Group__2_in_rule__LongDataType__Group__123526);
rule__LongDataType__Group__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__LongDataType__Group__1"
// $ANTLR start "rule__LongDataType__Group__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11204:1: rule__LongDataType__Group__1__Impl : ( ( rule__LongDataType__NameAssignment_1 ) ) ;
public final void rule__LongDataType__Group__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11208:1: ( ( ( rule__LongDataType__NameAssignment_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11209:1: ( ( rule__LongDataType__NameAssignment_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11209:1: ( ( rule__LongDataType__NameAssignment_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11210:1: ( rule__LongDataType__NameAssignment_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getLongDataTypeAccess().getNameAssignment_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11211:1: ( rule__LongDataType__NameAssignment_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11211:2: rule__LongDataType__NameAssignment_1
{
pushFollow(FollowSets001.FOLLOW_rule__LongDataType__NameAssignment_1_in_rule__LongDataType__Group__1__Impl23553);
rule__LongDataType__NameAssignment_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getLongDataTypeAccess().getNameAssignment_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__LongDataType__Group__1__Impl"
// $ANTLR start "rule__LongDataType__Group__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11221:1: rule__LongDataType__Group__2 : rule__LongDataType__Group__2__Impl rule__LongDataType__Group__3 ;
public final void rule__LongDataType__Group__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11225:1: ( rule__LongDataType__Group__2__Impl rule__LongDataType__Group__3 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11226:2: rule__LongDataType__Group__2__Impl rule__LongDataType__Group__3
{
pushFollow(FollowSets001.FOLLOW_rule__LongDataType__Group__2__Impl_in_rule__LongDataType__Group__223583);
rule__LongDataType__Group__2__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__LongDataType__Group__3_in_rule__LongDataType__Group__223586);
rule__LongDataType__Group__3();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__LongDataType__Group__2"
// $ANTLR start "rule__LongDataType__Group__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11233:1: rule__LongDataType__Group__2__Impl : ( ( rule__LongDataType__Group_2__0 )? ) ;
public final void rule__LongDataType__Group__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11237:1: ( ( ( rule__LongDataType__Group_2__0 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11238:1: ( ( rule__LongDataType__Group_2__0 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11238:1: ( ( rule__LongDataType__Group_2__0 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11239:1: ( rule__LongDataType__Group_2__0 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getLongDataTypeAccess().getGroup_2());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11240:1: ( rule__LongDataType__Group_2__0 )?
int alt90=2;
int LA90_0 = input.LA(1);
if ( (LA90_0==45) ) {
alt90=1;
}
switch (alt90) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11240:2: rule__LongDataType__Group_2__0
{
pushFollow(FollowSets001.FOLLOW_rule__LongDataType__Group_2__0_in_rule__LongDataType__Group__2__Impl23613);
rule__LongDataType__Group_2__0();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getLongDataTypeAccess().getGroup_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__LongDataType__Group__2__Impl"
// $ANTLR start "rule__LongDataType__Group__3"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11250:1: rule__LongDataType__Group__3 : rule__LongDataType__Group__3__Impl rule__LongDataType__Group__4 ;
public final void rule__LongDataType__Group__3() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11254:1: ( rule__LongDataType__Group__3__Impl rule__LongDataType__Group__4 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11255:2: rule__LongDataType__Group__3__Impl rule__LongDataType__Group__4
{
pushFollow(FollowSets001.FOLLOW_rule__LongDataType__Group__3__Impl_in_rule__LongDataType__Group__323644);
rule__LongDataType__Group__3__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__LongDataType__Group__4_in_rule__LongDataType__Group__323647);
rule__LongDataType__Group__4();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__LongDataType__Group__3"
// $ANTLR start "rule__LongDataType__Group__3__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11262:1: rule__LongDataType__Group__3__Impl : ( '{' ) ;
public final void rule__LongDataType__Group__3__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11266:1: ( ( '{' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11267:1: ( '{' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11267:1: ( '{' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11268:1: '{'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getLongDataTypeAccess().getLeftCurlyBracketKeyword_3());
}
match(input,67,FollowSets001.FOLLOW_67_in_rule__LongDataType__Group__3__Impl23675); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getLongDataTypeAccess().getLeftCurlyBracketKeyword_3());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__LongDataType__Group__3__Impl"
// $ANTLR start "rule__LongDataType__Group__4"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11281:1: rule__LongDataType__Group__4 : rule__LongDataType__Group__4__Impl rule__LongDataType__Group__5 ;
public final void rule__LongDataType__Group__4() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11285:1: ( rule__LongDataType__Group__4__Impl rule__LongDataType__Group__5 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11286:2: rule__LongDataType__Group__4__Impl rule__LongDataType__Group__5
{
pushFollow(FollowSets001.FOLLOW_rule__LongDataType__Group__4__Impl_in_rule__LongDataType__Group__423706);
rule__LongDataType__Group__4__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__LongDataType__Group__5_in_rule__LongDataType__Group__423709);
rule__LongDataType__Group__5();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__LongDataType__Group__4"
// $ANTLR start "rule__LongDataType__Group__4__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11293:1: rule__LongDataType__Group__4__Impl : ( ( rule__LongDataType__BaseDataTypeAssignment_4 ) ) ;
public final void rule__LongDataType__Group__4__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11297:1: ( ( ( rule__LongDataType__BaseDataTypeAssignment_4 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11298:1: ( ( rule__LongDataType__BaseDataTypeAssignment_4 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11298:1: ( ( rule__LongDataType__BaseDataTypeAssignment_4 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11299:1: ( rule__LongDataType__BaseDataTypeAssignment_4 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getLongDataTypeAccess().getBaseDataTypeAssignment_4());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11300:1: ( rule__LongDataType__BaseDataTypeAssignment_4 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11300:2: rule__LongDataType__BaseDataTypeAssignment_4
{
pushFollow(FollowSets001.FOLLOW_rule__LongDataType__BaseDataTypeAssignment_4_in_rule__LongDataType__Group__4__Impl23736);
rule__LongDataType__BaseDataTypeAssignment_4();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getLongDataTypeAccess().getBaseDataTypeAssignment_4());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__LongDataType__Group__4__Impl"
// $ANTLR start "rule__LongDataType__Group__5"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11310:1: rule__LongDataType__Group__5 : rule__LongDataType__Group__5__Impl ;
public final void rule__LongDataType__Group__5() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11314:1: ( rule__LongDataType__Group__5__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11315:2: rule__LongDataType__Group__5__Impl
{
pushFollow(FollowSets001.FOLLOW_rule__LongDataType__Group__5__Impl_in_rule__LongDataType__Group__523766);
rule__LongDataType__Group__5__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__LongDataType__Group__5"
// $ANTLR start "rule__LongDataType__Group__5__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11321:1: rule__LongDataType__Group__5__Impl : ( '}' ) ;
public final void rule__LongDataType__Group__5__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11325:1: ( ( '}' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11326:1: ( '}' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11326:1: ( '}' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11327:1: '}'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getLongDataTypeAccess().getRightCurlyBracketKeyword_5());
}
match(input,68,FollowSets001.FOLLOW_68_in_rule__LongDataType__Group__5__Impl23794); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getLongDataTypeAccess().getRightCurlyBracketKeyword_5());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__LongDataType__Group__5__Impl"
// $ANTLR start "rule__LongDataType__Group_2__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11352:1: rule__LongDataType__Group_2__0 : rule__LongDataType__Group_2__0__Impl rule__LongDataType__Group_2__1 ;
public final void rule__LongDataType__Group_2__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11356:1: ( rule__LongDataType__Group_2__0__Impl rule__LongDataType__Group_2__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11357:2: rule__LongDataType__Group_2__0__Impl rule__LongDataType__Group_2__1
{
pushFollow(FollowSets001.FOLLOW_rule__LongDataType__Group_2__0__Impl_in_rule__LongDataType__Group_2__023837);
rule__LongDataType__Group_2__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__LongDataType__Group_2__1_in_rule__LongDataType__Group_2__023840);
rule__LongDataType__Group_2__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__LongDataType__Group_2__0"
// $ANTLR start "rule__LongDataType__Group_2__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11364:1: rule__LongDataType__Group_2__0__Impl : ( 'extends' ) ;
public final void rule__LongDataType__Group_2__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11368:1: ( ( 'extends' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11369:1: ( 'extends' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11369:1: ( 'extends' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11370:1: 'extends'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getLongDataTypeAccess().getExtendsKeyword_2_0());
}
match(input,45,FollowSets001.FOLLOW_45_in_rule__LongDataType__Group_2__0__Impl23868); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getLongDataTypeAccess().getExtendsKeyword_2_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__LongDataType__Group_2__0__Impl"
// $ANTLR start "rule__LongDataType__Group_2__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11383:1: rule__LongDataType__Group_2__1 : rule__LongDataType__Group_2__1__Impl ;
public final void rule__LongDataType__Group_2__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11387:1: ( rule__LongDataType__Group_2__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11388:2: rule__LongDataType__Group_2__1__Impl
{
pushFollow(FollowSets001.FOLLOW_rule__LongDataType__Group_2__1__Impl_in_rule__LongDataType__Group_2__123899);
rule__LongDataType__Group_2__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__LongDataType__Group_2__1"
// $ANTLR start "rule__LongDataType__Group_2__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11394:1: rule__LongDataType__Group_2__1__Impl : ( ( rule__LongDataType__RefAssignment_2_1 ) ) ;
public final void rule__LongDataType__Group_2__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11398:1: ( ( ( rule__LongDataType__RefAssignment_2_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11399:1: ( ( rule__LongDataType__RefAssignment_2_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11399:1: ( ( rule__LongDataType__RefAssignment_2_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11400:1: ( rule__LongDataType__RefAssignment_2_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getLongDataTypeAccess().getRefAssignment_2_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11401:1: ( rule__LongDataType__RefAssignment_2_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11401:2: rule__LongDataType__RefAssignment_2_1
{
pushFollow(FollowSets001.FOLLOW_rule__LongDataType__RefAssignment_2_1_in_rule__LongDataType__Group_2__1__Impl23926);
rule__LongDataType__RefAssignment_2_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getLongDataTypeAccess().getRefAssignment_2_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__LongDataType__Group_2__1__Impl"
// $ANTLR start "rule__LongEntityAttribute__Group__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11415:1: rule__LongEntityAttribute__Group__0 : rule__LongEntityAttribute__Group__0__Impl rule__LongEntityAttribute__Group__1 ;
public final void rule__LongEntityAttribute__Group__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11419:1: ( rule__LongEntityAttribute__Group__0__Impl rule__LongEntityAttribute__Group__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11420:2: rule__LongEntityAttribute__Group__0__Impl rule__LongEntityAttribute__Group__1
{
pushFollow(FollowSets001.FOLLOW_rule__LongEntityAttribute__Group__0__Impl_in_rule__LongEntityAttribute__Group__023960);
rule__LongEntityAttribute__Group__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__LongEntityAttribute__Group__1_in_rule__LongEntityAttribute__Group__023963);
rule__LongEntityAttribute__Group__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__LongEntityAttribute__Group__0"
// $ANTLR start "rule__LongEntityAttribute__Group__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11427:1: rule__LongEntityAttribute__Group__0__Impl : ( 'long' ) ;
public final void rule__LongEntityAttribute__Group__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11431:1: ( ( 'long' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11432:1: ( 'long' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11432:1: ( 'long' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11433:1: 'long'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getLongEntityAttributeAccess().getLongKeyword_0());
}
match(input,56,FollowSets001.FOLLOW_56_in_rule__LongEntityAttribute__Group__0__Impl23991); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getLongEntityAttributeAccess().getLongKeyword_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__LongEntityAttribute__Group__0__Impl"
// $ANTLR start "rule__LongEntityAttribute__Group__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11446:1: rule__LongEntityAttribute__Group__1 : rule__LongEntityAttribute__Group__1__Impl rule__LongEntityAttribute__Group__2 ;
public final void rule__LongEntityAttribute__Group__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11450:1: ( rule__LongEntityAttribute__Group__1__Impl rule__LongEntityAttribute__Group__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11451:2: rule__LongEntityAttribute__Group__1__Impl rule__LongEntityAttribute__Group__2
{
pushFollow(FollowSets001.FOLLOW_rule__LongEntityAttribute__Group__1__Impl_in_rule__LongEntityAttribute__Group__124022);
rule__LongEntityAttribute__Group__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__LongEntityAttribute__Group__2_in_rule__LongEntityAttribute__Group__124025);
rule__LongEntityAttribute__Group__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__LongEntityAttribute__Group__1"
// $ANTLR start "rule__LongEntityAttribute__Group__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11458:1: rule__LongEntityAttribute__Group__1__Impl : ( ( rule__LongEntityAttribute__TypeAssignment_1 )? ) ;
public final void rule__LongEntityAttribute__Group__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11462:1: ( ( ( rule__LongEntityAttribute__TypeAssignment_1 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11463:1: ( ( rule__LongEntityAttribute__TypeAssignment_1 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11463:1: ( ( rule__LongEntityAttribute__TypeAssignment_1 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11464:1: ( rule__LongEntityAttribute__TypeAssignment_1 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getLongEntityAttributeAccess().getTypeAssignment_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11465:1: ( rule__LongEntityAttribute__TypeAssignment_1 )?
int alt91=2;
int LA91_0 = input.LA(1);
if ( (LA91_0==RULE_ID) ) {
int LA91_1 = input.LA(2);
if ( (LA91_1==RULE_ID||LA91_1==43) ) {
alt91=1;
}
}
switch (alt91) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11465:2: rule__LongEntityAttribute__TypeAssignment_1
{
pushFollow(FollowSets001.FOLLOW_rule__LongEntityAttribute__TypeAssignment_1_in_rule__LongEntityAttribute__Group__1__Impl24052);
rule__LongEntityAttribute__TypeAssignment_1();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getLongEntityAttributeAccess().getTypeAssignment_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__LongEntityAttribute__Group__1__Impl"
// $ANTLR start "rule__LongEntityAttribute__Group__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11475:1: rule__LongEntityAttribute__Group__2 : rule__LongEntityAttribute__Group__2__Impl ;
public final void rule__LongEntityAttribute__Group__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11479:1: ( rule__LongEntityAttribute__Group__2__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11480:2: rule__LongEntityAttribute__Group__2__Impl
{
pushFollow(FollowSets001.FOLLOW_rule__LongEntityAttribute__Group__2__Impl_in_rule__LongEntityAttribute__Group__224083);
rule__LongEntityAttribute__Group__2__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__LongEntityAttribute__Group__2"
// $ANTLR start "rule__LongEntityAttribute__Group__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11486:1: rule__LongEntityAttribute__Group__2__Impl : ( ( rule__LongEntityAttribute__NameAssignment_2 ) ) ;
public final void rule__LongEntityAttribute__Group__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11490:1: ( ( ( rule__LongEntityAttribute__NameAssignment_2 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11491:1: ( ( rule__LongEntityAttribute__NameAssignment_2 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11491:1: ( ( rule__LongEntityAttribute__NameAssignment_2 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11492:1: ( rule__LongEntityAttribute__NameAssignment_2 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getLongEntityAttributeAccess().getNameAssignment_2());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11493:1: ( rule__LongEntityAttribute__NameAssignment_2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11493:2: rule__LongEntityAttribute__NameAssignment_2
{
pushFollow(FollowSets001.FOLLOW_rule__LongEntityAttribute__NameAssignment_2_in_rule__LongEntityAttribute__Group__2__Impl24110);
rule__LongEntityAttribute__NameAssignment_2();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getLongEntityAttributeAccess().getNameAssignment_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__LongEntityAttribute__Group__2__Impl"
// $ANTLR start "rule__FloatDataType__Group__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11509:1: rule__FloatDataType__Group__0 : rule__FloatDataType__Group__0__Impl rule__FloatDataType__Group__1 ;
public final void rule__FloatDataType__Group__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11513:1: ( rule__FloatDataType__Group__0__Impl rule__FloatDataType__Group__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11514:2: rule__FloatDataType__Group__0__Impl rule__FloatDataType__Group__1
{
pushFollow(FollowSets001.FOLLOW_rule__FloatDataType__Group__0__Impl_in_rule__FloatDataType__Group__024146);
rule__FloatDataType__Group__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__FloatDataType__Group__1_in_rule__FloatDataType__Group__024149);
rule__FloatDataType__Group__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__FloatDataType__Group__0"
// $ANTLR start "rule__FloatDataType__Group__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11521:1: rule__FloatDataType__Group__0__Impl : ( 'floatdatatype' ) ;
public final void rule__FloatDataType__Group__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11525:1: ( ( 'floatdatatype' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11526:1: ( 'floatdatatype' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11526:1: ( 'floatdatatype' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11527:1: 'floatdatatype'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getFloatDataTypeAccess().getFloatdatatypeKeyword_0());
}
match(input,93,FollowSets001.FOLLOW_93_in_rule__FloatDataType__Group__0__Impl24177); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getFloatDataTypeAccess().getFloatdatatypeKeyword_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__FloatDataType__Group__0__Impl"
// $ANTLR start "rule__FloatDataType__Group__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11540:1: rule__FloatDataType__Group__1 : rule__FloatDataType__Group__1__Impl rule__FloatDataType__Group__2 ;
public final void rule__FloatDataType__Group__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11544:1: ( rule__FloatDataType__Group__1__Impl rule__FloatDataType__Group__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11545:2: rule__FloatDataType__Group__1__Impl rule__FloatDataType__Group__2
{
pushFollow(FollowSets001.FOLLOW_rule__FloatDataType__Group__1__Impl_in_rule__FloatDataType__Group__124208);
rule__FloatDataType__Group__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__FloatDataType__Group__2_in_rule__FloatDataType__Group__124211);
rule__FloatDataType__Group__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__FloatDataType__Group__1"
// $ANTLR start "rule__FloatDataType__Group__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11552:1: rule__FloatDataType__Group__1__Impl : ( ( rule__FloatDataType__NameAssignment_1 ) ) ;
public final void rule__FloatDataType__Group__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11556:1: ( ( ( rule__FloatDataType__NameAssignment_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11557:1: ( ( rule__FloatDataType__NameAssignment_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11557:1: ( ( rule__FloatDataType__NameAssignment_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11558:1: ( rule__FloatDataType__NameAssignment_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getFloatDataTypeAccess().getNameAssignment_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11559:1: ( rule__FloatDataType__NameAssignment_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11559:2: rule__FloatDataType__NameAssignment_1
{
pushFollow(FollowSets001.FOLLOW_rule__FloatDataType__NameAssignment_1_in_rule__FloatDataType__Group__1__Impl24238);
rule__FloatDataType__NameAssignment_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getFloatDataTypeAccess().getNameAssignment_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__FloatDataType__Group__1__Impl"
// $ANTLR start "rule__FloatDataType__Group__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11569:1: rule__FloatDataType__Group__2 : rule__FloatDataType__Group__2__Impl rule__FloatDataType__Group__3 ;
public final void rule__FloatDataType__Group__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11573:1: ( rule__FloatDataType__Group__2__Impl rule__FloatDataType__Group__3 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11574:2: rule__FloatDataType__Group__2__Impl rule__FloatDataType__Group__3
{
pushFollow(FollowSets001.FOLLOW_rule__FloatDataType__Group__2__Impl_in_rule__FloatDataType__Group__224268);
rule__FloatDataType__Group__2__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__FloatDataType__Group__3_in_rule__FloatDataType__Group__224271);
rule__FloatDataType__Group__3();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__FloatDataType__Group__2"
// $ANTLR start "rule__FloatDataType__Group__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11581:1: rule__FloatDataType__Group__2__Impl : ( ( rule__FloatDataType__Group_2__0 )? ) ;
public final void rule__FloatDataType__Group__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11585:1: ( ( ( rule__FloatDataType__Group_2__0 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11586:1: ( ( rule__FloatDataType__Group_2__0 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11586:1: ( ( rule__FloatDataType__Group_2__0 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11587:1: ( rule__FloatDataType__Group_2__0 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getFloatDataTypeAccess().getGroup_2());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11588:1: ( rule__FloatDataType__Group_2__0 )?
int alt92=2;
int LA92_0 = input.LA(1);
if ( (LA92_0==45) ) {
alt92=1;
}
switch (alt92) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11588:2: rule__FloatDataType__Group_2__0
{
pushFollow(FollowSets001.FOLLOW_rule__FloatDataType__Group_2__0_in_rule__FloatDataType__Group__2__Impl24298);
rule__FloatDataType__Group_2__0();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getFloatDataTypeAccess().getGroup_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__FloatDataType__Group__2__Impl"
// $ANTLR start "rule__FloatDataType__Group__3"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11598:1: rule__FloatDataType__Group__3 : rule__FloatDataType__Group__3__Impl rule__FloatDataType__Group__4 ;
public final void rule__FloatDataType__Group__3() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11602:1: ( rule__FloatDataType__Group__3__Impl rule__FloatDataType__Group__4 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11603:2: rule__FloatDataType__Group__3__Impl rule__FloatDataType__Group__4
{
pushFollow(FollowSets001.FOLLOW_rule__FloatDataType__Group__3__Impl_in_rule__FloatDataType__Group__324329);
rule__FloatDataType__Group__3__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__FloatDataType__Group__4_in_rule__FloatDataType__Group__324332);
rule__FloatDataType__Group__4();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__FloatDataType__Group__3"
// $ANTLR start "rule__FloatDataType__Group__3__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11610:1: rule__FloatDataType__Group__3__Impl : ( '{' ) ;
public final void rule__FloatDataType__Group__3__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11614:1: ( ( '{' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11615:1: ( '{' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11615:1: ( '{' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11616:1: '{'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getFloatDataTypeAccess().getLeftCurlyBracketKeyword_3());
}
match(input,67,FollowSets001.FOLLOW_67_in_rule__FloatDataType__Group__3__Impl24360); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getFloatDataTypeAccess().getLeftCurlyBracketKeyword_3());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__FloatDataType__Group__3__Impl"
// $ANTLR start "rule__FloatDataType__Group__4"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11629:1: rule__FloatDataType__Group__4 : rule__FloatDataType__Group__4__Impl rule__FloatDataType__Group__5 ;
public final void rule__FloatDataType__Group__4() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11633:1: ( rule__FloatDataType__Group__4__Impl rule__FloatDataType__Group__5 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11634:2: rule__FloatDataType__Group__4__Impl rule__FloatDataType__Group__5
{
pushFollow(FollowSets001.FOLLOW_rule__FloatDataType__Group__4__Impl_in_rule__FloatDataType__Group__424391);
rule__FloatDataType__Group__4__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__FloatDataType__Group__5_in_rule__FloatDataType__Group__424394);
rule__FloatDataType__Group__5();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__FloatDataType__Group__4"
// $ANTLR start "rule__FloatDataType__Group__4__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11641:1: rule__FloatDataType__Group__4__Impl : ( ( rule__FloatDataType__BaseDataTypeAssignment_4 ) ) ;
public final void rule__FloatDataType__Group__4__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11645:1: ( ( ( rule__FloatDataType__BaseDataTypeAssignment_4 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11646:1: ( ( rule__FloatDataType__BaseDataTypeAssignment_4 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11646:1: ( ( rule__FloatDataType__BaseDataTypeAssignment_4 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11647:1: ( rule__FloatDataType__BaseDataTypeAssignment_4 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getFloatDataTypeAccess().getBaseDataTypeAssignment_4());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11648:1: ( rule__FloatDataType__BaseDataTypeAssignment_4 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11648:2: rule__FloatDataType__BaseDataTypeAssignment_4
{
pushFollow(FollowSets001.FOLLOW_rule__FloatDataType__BaseDataTypeAssignment_4_in_rule__FloatDataType__Group__4__Impl24421);
rule__FloatDataType__BaseDataTypeAssignment_4();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getFloatDataTypeAccess().getBaseDataTypeAssignment_4());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__FloatDataType__Group__4__Impl"
// $ANTLR start "rule__FloatDataType__Group__5"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11658:1: rule__FloatDataType__Group__5 : rule__FloatDataType__Group__5__Impl ;
public final void rule__FloatDataType__Group__5() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11662:1: ( rule__FloatDataType__Group__5__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11663:2: rule__FloatDataType__Group__5__Impl
{
pushFollow(FollowSets001.FOLLOW_rule__FloatDataType__Group__5__Impl_in_rule__FloatDataType__Group__524451);
rule__FloatDataType__Group__5__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__FloatDataType__Group__5"
// $ANTLR start "rule__FloatDataType__Group__5__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11669:1: rule__FloatDataType__Group__5__Impl : ( '}' ) ;
public final void rule__FloatDataType__Group__5__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11673:1: ( ( '}' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11674:1: ( '}' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11674:1: ( '}' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11675:1: '}'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getFloatDataTypeAccess().getRightCurlyBracketKeyword_5());
}
match(input,68,FollowSets001.FOLLOW_68_in_rule__FloatDataType__Group__5__Impl24479); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getFloatDataTypeAccess().getRightCurlyBracketKeyword_5());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__FloatDataType__Group__5__Impl"
// $ANTLR start "rule__FloatDataType__Group_2__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11700:1: rule__FloatDataType__Group_2__0 : rule__FloatDataType__Group_2__0__Impl rule__FloatDataType__Group_2__1 ;
public final void rule__FloatDataType__Group_2__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11704:1: ( rule__FloatDataType__Group_2__0__Impl rule__FloatDataType__Group_2__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11705:2: rule__FloatDataType__Group_2__0__Impl rule__FloatDataType__Group_2__1
{
pushFollow(FollowSets001.FOLLOW_rule__FloatDataType__Group_2__0__Impl_in_rule__FloatDataType__Group_2__024522);
rule__FloatDataType__Group_2__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__FloatDataType__Group_2__1_in_rule__FloatDataType__Group_2__024525);
rule__FloatDataType__Group_2__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__FloatDataType__Group_2__0"
// $ANTLR start "rule__FloatDataType__Group_2__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11712:1: rule__FloatDataType__Group_2__0__Impl : ( 'extends' ) ;
public final void rule__FloatDataType__Group_2__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11716:1: ( ( 'extends' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11717:1: ( 'extends' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11717:1: ( 'extends' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11718:1: 'extends'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getFloatDataTypeAccess().getExtendsKeyword_2_0());
}
match(input,45,FollowSets001.FOLLOW_45_in_rule__FloatDataType__Group_2__0__Impl24553); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getFloatDataTypeAccess().getExtendsKeyword_2_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__FloatDataType__Group_2__0__Impl"
// $ANTLR start "rule__FloatDataType__Group_2__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11731:1: rule__FloatDataType__Group_2__1 : rule__FloatDataType__Group_2__1__Impl ;
public final void rule__FloatDataType__Group_2__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11735:1: ( rule__FloatDataType__Group_2__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11736:2: rule__FloatDataType__Group_2__1__Impl
{
pushFollow(FollowSets001.FOLLOW_rule__FloatDataType__Group_2__1__Impl_in_rule__FloatDataType__Group_2__124584);
rule__FloatDataType__Group_2__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__FloatDataType__Group_2__1"
// $ANTLR start "rule__FloatDataType__Group_2__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11742:1: rule__FloatDataType__Group_2__1__Impl : ( ( rule__FloatDataType__RefAssignment_2_1 ) ) ;
public final void rule__FloatDataType__Group_2__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11746:1: ( ( ( rule__FloatDataType__RefAssignment_2_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11747:1: ( ( rule__FloatDataType__RefAssignment_2_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11747:1: ( ( rule__FloatDataType__RefAssignment_2_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11748:1: ( rule__FloatDataType__RefAssignment_2_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getFloatDataTypeAccess().getRefAssignment_2_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11749:1: ( rule__FloatDataType__RefAssignment_2_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11749:2: rule__FloatDataType__RefAssignment_2_1
{
pushFollow(FollowSets001.FOLLOW_rule__FloatDataType__RefAssignment_2_1_in_rule__FloatDataType__Group_2__1__Impl24611);
rule__FloatDataType__RefAssignment_2_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getFloatDataTypeAccess().getRefAssignment_2_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__FloatDataType__Group_2__1__Impl"
// $ANTLR start "rule__FloatEntityAttribute__Group__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11763:1: rule__FloatEntityAttribute__Group__0 : rule__FloatEntityAttribute__Group__0__Impl rule__FloatEntityAttribute__Group__1 ;
public final void rule__FloatEntityAttribute__Group__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11767:1: ( rule__FloatEntityAttribute__Group__0__Impl rule__FloatEntityAttribute__Group__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11768:2: rule__FloatEntityAttribute__Group__0__Impl rule__FloatEntityAttribute__Group__1
{
pushFollow(FollowSets001.FOLLOW_rule__FloatEntityAttribute__Group__0__Impl_in_rule__FloatEntityAttribute__Group__024645);
rule__FloatEntityAttribute__Group__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__FloatEntityAttribute__Group__1_in_rule__FloatEntityAttribute__Group__024648);
rule__FloatEntityAttribute__Group__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__FloatEntityAttribute__Group__0"
// $ANTLR start "rule__FloatEntityAttribute__Group__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11775:1: rule__FloatEntityAttribute__Group__0__Impl : ( 'float' ) ;
public final void rule__FloatEntityAttribute__Group__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11779:1: ( ( 'float' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11780:1: ( 'float' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11780:1: ( 'float' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11781:1: 'float'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getFloatEntityAttributeAccess().getFloatKeyword_0());
}
match(input,94,FollowSets001.FOLLOW_94_in_rule__FloatEntityAttribute__Group__0__Impl24676); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getFloatEntityAttributeAccess().getFloatKeyword_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__FloatEntityAttribute__Group__0__Impl"
// $ANTLR start "rule__FloatEntityAttribute__Group__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11794:1: rule__FloatEntityAttribute__Group__1 : rule__FloatEntityAttribute__Group__1__Impl rule__FloatEntityAttribute__Group__2 ;
public final void rule__FloatEntityAttribute__Group__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11798:1: ( rule__FloatEntityAttribute__Group__1__Impl rule__FloatEntityAttribute__Group__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11799:2: rule__FloatEntityAttribute__Group__1__Impl rule__FloatEntityAttribute__Group__2
{
pushFollow(FollowSets001.FOLLOW_rule__FloatEntityAttribute__Group__1__Impl_in_rule__FloatEntityAttribute__Group__124707);
rule__FloatEntityAttribute__Group__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__FloatEntityAttribute__Group__2_in_rule__FloatEntityAttribute__Group__124710);
rule__FloatEntityAttribute__Group__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__FloatEntityAttribute__Group__1"
// $ANTLR start "rule__FloatEntityAttribute__Group__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11806:1: rule__FloatEntityAttribute__Group__1__Impl : ( ( rule__FloatEntityAttribute__TypeAssignment_1 )? ) ;
public final void rule__FloatEntityAttribute__Group__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11810:1: ( ( ( rule__FloatEntityAttribute__TypeAssignment_1 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11811:1: ( ( rule__FloatEntityAttribute__TypeAssignment_1 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11811:1: ( ( rule__FloatEntityAttribute__TypeAssignment_1 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11812:1: ( rule__FloatEntityAttribute__TypeAssignment_1 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getFloatEntityAttributeAccess().getTypeAssignment_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11813:1: ( rule__FloatEntityAttribute__TypeAssignment_1 )?
int alt93=2;
int LA93_0 = input.LA(1);
if ( (LA93_0==RULE_ID) ) {
int LA93_1 = input.LA(2);
if ( (LA93_1==RULE_ID||LA93_1==43) ) {
alt93=1;
}
}
switch (alt93) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11813:2: rule__FloatEntityAttribute__TypeAssignment_1
{
pushFollow(FollowSets001.FOLLOW_rule__FloatEntityAttribute__TypeAssignment_1_in_rule__FloatEntityAttribute__Group__1__Impl24737);
rule__FloatEntityAttribute__TypeAssignment_1();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getFloatEntityAttributeAccess().getTypeAssignment_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__FloatEntityAttribute__Group__1__Impl"
// $ANTLR start "rule__FloatEntityAttribute__Group__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11823:1: rule__FloatEntityAttribute__Group__2 : rule__FloatEntityAttribute__Group__2__Impl ;
public final void rule__FloatEntityAttribute__Group__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11827:1: ( rule__FloatEntityAttribute__Group__2__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11828:2: rule__FloatEntityAttribute__Group__2__Impl
{
pushFollow(FollowSets001.FOLLOW_rule__FloatEntityAttribute__Group__2__Impl_in_rule__FloatEntityAttribute__Group__224768);
rule__FloatEntityAttribute__Group__2__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__FloatEntityAttribute__Group__2"
// $ANTLR start "rule__FloatEntityAttribute__Group__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11834:1: rule__FloatEntityAttribute__Group__2__Impl : ( ( rule__FloatEntityAttribute__NameAssignment_2 ) ) ;
public final void rule__FloatEntityAttribute__Group__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11838:1: ( ( ( rule__FloatEntityAttribute__NameAssignment_2 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11839:1: ( ( rule__FloatEntityAttribute__NameAssignment_2 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11839:1: ( ( rule__FloatEntityAttribute__NameAssignment_2 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11840:1: ( rule__FloatEntityAttribute__NameAssignment_2 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getFloatEntityAttributeAccess().getNameAssignment_2());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11841:1: ( rule__FloatEntityAttribute__NameAssignment_2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11841:2: rule__FloatEntityAttribute__NameAssignment_2
{
pushFollow(FollowSets001.FOLLOW_rule__FloatEntityAttribute__NameAssignment_2_in_rule__FloatEntityAttribute__Group__2__Impl24795);
rule__FloatEntityAttribute__NameAssignment_2();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getFloatEntityAttributeAccess().getNameAssignment_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__FloatEntityAttribute__Group__2__Impl"
// $ANTLR start "rule__DoubleDataType__Group__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11857:1: rule__DoubleDataType__Group__0 : rule__DoubleDataType__Group__0__Impl rule__DoubleDataType__Group__1 ;
public final void rule__DoubleDataType__Group__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11861:1: ( rule__DoubleDataType__Group__0__Impl rule__DoubleDataType__Group__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11862:2: rule__DoubleDataType__Group__0__Impl rule__DoubleDataType__Group__1
{
pushFollow(FollowSets001.FOLLOW_rule__DoubleDataType__Group__0__Impl_in_rule__DoubleDataType__Group__024831);
rule__DoubleDataType__Group__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DoubleDataType__Group__1_in_rule__DoubleDataType__Group__024834);
rule__DoubleDataType__Group__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DoubleDataType__Group__0"
// $ANTLR start "rule__DoubleDataType__Group__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11869:1: rule__DoubleDataType__Group__0__Impl : ( 'doubledatatype' ) ;
public final void rule__DoubleDataType__Group__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11873:1: ( ( 'doubledatatype' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11874:1: ( 'doubledatatype' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11874:1: ( 'doubledatatype' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11875:1: 'doubledatatype'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDoubleDataTypeAccess().getDoubledatatypeKeyword_0());
}
match(input,95,FollowSets001.FOLLOW_95_in_rule__DoubleDataType__Group__0__Impl24862); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDoubleDataTypeAccess().getDoubledatatypeKeyword_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DoubleDataType__Group__0__Impl"
// $ANTLR start "rule__DoubleDataType__Group__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11888:1: rule__DoubleDataType__Group__1 : rule__DoubleDataType__Group__1__Impl rule__DoubleDataType__Group__2 ;
public final void rule__DoubleDataType__Group__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11892:1: ( rule__DoubleDataType__Group__1__Impl rule__DoubleDataType__Group__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11893:2: rule__DoubleDataType__Group__1__Impl rule__DoubleDataType__Group__2
{
pushFollow(FollowSets001.FOLLOW_rule__DoubleDataType__Group__1__Impl_in_rule__DoubleDataType__Group__124893);
rule__DoubleDataType__Group__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DoubleDataType__Group__2_in_rule__DoubleDataType__Group__124896);
rule__DoubleDataType__Group__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DoubleDataType__Group__1"
// $ANTLR start "rule__DoubleDataType__Group__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11900:1: rule__DoubleDataType__Group__1__Impl : ( ( rule__DoubleDataType__NameAssignment_1 ) ) ;
public final void rule__DoubleDataType__Group__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11904:1: ( ( ( rule__DoubleDataType__NameAssignment_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11905:1: ( ( rule__DoubleDataType__NameAssignment_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11905:1: ( ( rule__DoubleDataType__NameAssignment_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11906:1: ( rule__DoubleDataType__NameAssignment_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDoubleDataTypeAccess().getNameAssignment_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11907:1: ( rule__DoubleDataType__NameAssignment_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11907:2: rule__DoubleDataType__NameAssignment_1
{
pushFollow(FollowSets001.FOLLOW_rule__DoubleDataType__NameAssignment_1_in_rule__DoubleDataType__Group__1__Impl24923);
rule__DoubleDataType__NameAssignment_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDoubleDataTypeAccess().getNameAssignment_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DoubleDataType__Group__1__Impl"
// $ANTLR start "rule__DoubleDataType__Group__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11917:1: rule__DoubleDataType__Group__2 : rule__DoubleDataType__Group__2__Impl rule__DoubleDataType__Group__3 ;
public final void rule__DoubleDataType__Group__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11921:1: ( rule__DoubleDataType__Group__2__Impl rule__DoubleDataType__Group__3 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11922:2: rule__DoubleDataType__Group__2__Impl rule__DoubleDataType__Group__3
{
pushFollow(FollowSets001.FOLLOW_rule__DoubleDataType__Group__2__Impl_in_rule__DoubleDataType__Group__224953);
rule__DoubleDataType__Group__2__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DoubleDataType__Group__3_in_rule__DoubleDataType__Group__224956);
rule__DoubleDataType__Group__3();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DoubleDataType__Group__2"
// $ANTLR start "rule__DoubleDataType__Group__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11929:1: rule__DoubleDataType__Group__2__Impl : ( ( rule__DoubleDataType__Group_2__0 )? ) ;
public final void rule__DoubleDataType__Group__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11933:1: ( ( ( rule__DoubleDataType__Group_2__0 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11934:1: ( ( rule__DoubleDataType__Group_2__0 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11934:1: ( ( rule__DoubleDataType__Group_2__0 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11935:1: ( rule__DoubleDataType__Group_2__0 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDoubleDataTypeAccess().getGroup_2());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11936:1: ( rule__DoubleDataType__Group_2__0 )?
int alt94=2;
int LA94_0 = input.LA(1);
if ( (LA94_0==45) ) {
alt94=1;
}
switch (alt94) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11936:2: rule__DoubleDataType__Group_2__0
{
pushFollow(FollowSets001.FOLLOW_rule__DoubleDataType__Group_2__0_in_rule__DoubleDataType__Group__2__Impl24983);
rule__DoubleDataType__Group_2__0();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDoubleDataTypeAccess().getGroup_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DoubleDataType__Group__2__Impl"
// $ANTLR start "rule__DoubleDataType__Group__3"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11946:1: rule__DoubleDataType__Group__3 : rule__DoubleDataType__Group__3__Impl rule__DoubleDataType__Group__4 ;
public final void rule__DoubleDataType__Group__3() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11950:1: ( rule__DoubleDataType__Group__3__Impl rule__DoubleDataType__Group__4 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11951:2: rule__DoubleDataType__Group__3__Impl rule__DoubleDataType__Group__4
{
pushFollow(FollowSets001.FOLLOW_rule__DoubleDataType__Group__3__Impl_in_rule__DoubleDataType__Group__325014);
rule__DoubleDataType__Group__3__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DoubleDataType__Group__4_in_rule__DoubleDataType__Group__325017);
rule__DoubleDataType__Group__4();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DoubleDataType__Group__3"
// $ANTLR start "rule__DoubleDataType__Group__3__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11958:1: rule__DoubleDataType__Group__3__Impl : ( '{' ) ;
public final void rule__DoubleDataType__Group__3__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11962:1: ( ( '{' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11963:1: ( '{' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11963:1: ( '{' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11964:1: '{'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDoubleDataTypeAccess().getLeftCurlyBracketKeyword_3());
}
match(input,67,FollowSets001.FOLLOW_67_in_rule__DoubleDataType__Group__3__Impl25045); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDoubleDataTypeAccess().getLeftCurlyBracketKeyword_3());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DoubleDataType__Group__3__Impl"
// $ANTLR start "rule__DoubleDataType__Group__4"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11977:1: rule__DoubleDataType__Group__4 : rule__DoubleDataType__Group__4__Impl rule__DoubleDataType__Group__5 ;
public final void rule__DoubleDataType__Group__4() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11981:1: ( rule__DoubleDataType__Group__4__Impl rule__DoubleDataType__Group__5 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11982:2: rule__DoubleDataType__Group__4__Impl rule__DoubleDataType__Group__5
{
pushFollow(FollowSets001.FOLLOW_rule__DoubleDataType__Group__4__Impl_in_rule__DoubleDataType__Group__425076);
rule__DoubleDataType__Group__4__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DoubleDataType__Group__5_in_rule__DoubleDataType__Group__425079);
rule__DoubleDataType__Group__5();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DoubleDataType__Group__4"
// $ANTLR start "rule__DoubleDataType__Group__4__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11989:1: rule__DoubleDataType__Group__4__Impl : ( ( rule__DoubleDataType__BaseDataTypeAssignment_4 ) ) ;
public final void rule__DoubleDataType__Group__4__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11993:1: ( ( ( rule__DoubleDataType__BaseDataTypeAssignment_4 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11994:1: ( ( rule__DoubleDataType__BaseDataTypeAssignment_4 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11994:1: ( ( rule__DoubleDataType__BaseDataTypeAssignment_4 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11995:1: ( rule__DoubleDataType__BaseDataTypeAssignment_4 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDoubleDataTypeAccess().getBaseDataTypeAssignment_4());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11996:1: ( rule__DoubleDataType__BaseDataTypeAssignment_4 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:11996:2: rule__DoubleDataType__BaseDataTypeAssignment_4
{
pushFollow(FollowSets001.FOLLOW_rule__DoubleDataType__BaseDataTypeAssignment_4_in_rule__DoubleDataType__Group__4__Impl25106);
rule__DoubleDataType__BaseDataTypeAssignment_4();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDoubleDataTypeAccess().getBaseDataTypeAssignment_4());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DoubleDataType__Group__4__Impl"
// $ANTLR start "rule__DoubleDataType__Group__5"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12006:1: rule__DoubleDataType__Group__5 : rule__DoubleDataType__Group__5__Impl ;
public final void rule__DoubleDataType__Group__5() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12010:1: ( rule__DoubleDataType__Group__5__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12011:2: rule__DoubleDataType__Group__5__Impl
{
pushFollow(FollowSets001.FOLLOW_rule__DoubleDataType__Group__5__Impl_in_rule__DoubleDataType__Group__525136);
rule__DoubleDataType__Group__5__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DoubleDataType__Group__5"
// $ANTLR start "rule__DoubleDataType__Group__5__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12017:1: rule__DoubleDataType__Group__5__Impl : ( '}' ) ;
public final void rule__DoubleDataType__Group__5__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12021:1: ( ( '}' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12022:1: ( '}' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12022:1: ( '}' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12023:1: '}'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDoubleDataTypeAccess().getRightCurlyBracketKeyword_5());
}
match(input,68,FollowSets001.FOLLOW_68_in_rule__DoubleDataType__Group__5__Impl25164); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDoubleDataTypeAccess().getRightCurlyBracketKeyword_5());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DoubleDataType__Group__5__Impl"
// $ANTLR start "rule__DoubleDataType__Group_2__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12048:1: rule__DoubleDataType__Group_2__0 : rule__DoubleDataType__Group_2__0__Impl rule__DoubleDataType__Group_2__1 ;
public final void rule__DoubleDataType__Group_2__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12052:1: ( rule__DoubleDataType__Group_2__0__Impl rule__DoubleDataType__Group_2__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12053:2: rule__DoubleDataType__Group_2__0__Impl rule__DoubleDataType__Group_2__1
{
pushFollow(FollowSets001.FOLLOW_rule__DoubleDataType__Group_2__0__Impl_in_rule__DoubleDataType__Group_2__025207);
rule__DoubleDataType__Group_2__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DoubleDataType__Group_2__1_in_rule__DoubleDataType__Group_2__025210);
rule__DoubleDataType__Group_2__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DoubleDataType__Group_2__0"
// $ANTLR start "rule__DoubleDataType__Group_2__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12060:1: rule__DoubleDataType__Group_2__0__Impl : ( 'extends' ) ;
public final void rule__DoubleDataType__Group_2__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12064:1: ( ( 'extends' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12065:1: ( 'extends' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12065:1: ( 'extends' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12066:1: 'extends'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDoubleDataTypeAccess().getExtendsKeyword_2_0());
}
match(input,45,FollowSets001.FOLLOW_45_in_rule__DoubleDataType__Group_2__0__Impl25238); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDoubleDataTypeAccess().getExtendsKeyword_2_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DoubleDataType__Group_2__0__Impl"
// $ANTLR start "rule__DoubleDataType__Group_2__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12079:1: rule__DoubleDataType__Group_2__1 : rule__DoubleDataType__Group_2__1__Impl ;
public final void rule__DoubleDataType__Group_2__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12083:1: ( rule__DoubleDataType__Group_2__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12084:2: rule__DoubleDataType__Group_2__1__Impl
{
pushFollow(FollowSets001.FOLLOW_rule__DoubleDataType__Group_2__1__Impl_in_rule__DoubleDataType__Group_2__125269);
rule__DoubleDataType__Group_2__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DoubleDataType__Group_2__1"
// $ANTLR start "rule__DoubleDataType__Group_2__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12090:1: rule__DoubleDataType__Group_2__1__Impl : ( ( rule__DoubleDataType__RefAssignment_2_1 ) ) ;
public final void rule__DoubleDataType__Group_2__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12094:1: ( ( ( rule__DoubleDataType__RefAssignment_2_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12095:1: ( ( rule__DoubleDataType__RefAssignment_2_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12095:1: ( ( rule__DoubleDataType__RefAssignment_2_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12096:1: ( rule__DoubleDataType__RefAssignment_2_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDoubleDataTypeAccess().getRefAssignment_2_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12097:1: ( rule__DoubleDataType__RefAssignment_2_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12097:2: rule__DoubleDataType__RefAssignment_2_1
{
pushFollow(FollowSets001.FOLLOW_rule__DoubleDataType__RefAssignment_2_1_in_rule__DoubleDataType__Group_2__1__Impl25296);
rule__DoubleDataType__RefAssignment_2_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDoubleDataTypeAccess().getRefAssignment_2_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DoubleDataType__Group_2__1__Impl"
// $ANTLR start "rule__DoubleEntityAttribute__Group__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12111:1: rule__DoubleEntityAttribute__Group__0 : rule__DoubleEntityAttribute__Group__0__Impl rule__DoubleEntityAttribute__Group__1 ;
public final void rule__DoubleEntityAttribute__Group__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12115:1: ( rule__DoubleEntityAttribute__Group__0__Impl rule__DoubleEntityAttribute__Group__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12116:2: rule__DoubleEntityAttribute__Group__0__Impl rule__DoubleEntityAttribute__Group__1
{
pushFollow(FollowSets001.FOLLOW_rule__DoubleEntityAttribute__Group__0__Impl_in_rule__DoubleEntityAttribute__Group__025330);
rule__DoubleEntityAttribute__Group__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DoubleEntityAttribute__Group__1_in_rule__DoubleEntityAttribute__Group__025333);
rule__DoubleEntityAttribute__Group__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DoubleEntityAttribute__Group__0"
// $ANTLR start "rule__DoubleEntityAttribute__Group__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12123:1: rule__DoubleEntityAttribute__Group__0__Impl : ( 'double' ) ;
public final void rule__DoubleEntityAttribute__Group__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12127:1: ( ( 'double' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12128:1: ( 'double' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12128:1: ( 'double' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12129:1: 'double'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDoubleEntityAttributeAccess().getDoubleKeyword_0());
}
match(input,96,FollowSets001.FOLLOW_96_in_rule__DoubleEntityAttribute__Group__0__Impl25361); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDoubleEntityAttributeAccess().getDoubleKeyword_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DoubleEntityAttribute__Group__0__Impl"
// $ANTLR start "rule__DoubleEntityAttribute__Group__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12142:1: rule__DoubleEntityAttribute__Group__1 : rule__DoubleEntityAttribute__Group__1__Impl rule__DoubleEntityAttribute__Group__2 ;
public final void rule__DoubleEntityAttribute__Group__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12146:1: ( rule__DoubleEntityAttribute__Group__1__Impl rule__DoubleEntityAttribute__Group__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12147:2: rule__DoubleEntityAttribute__Group__1__Impl rule__DoubleEntityAttribute__Group__2
{
pushFollow(FollowSets001.FOLLOW_rule__DoubleEntityAttribute__Group__1__Impl_in_rule__DoubleEntityAttribute__Group__125392);
rule__DoubleEntityAttribute__Group__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DoubleEntityAttribute__Group__2_in_rule__DoubleEntityAttribute__Group__125395);
rule__DoubleEntityAttribute__Group__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DoubleEntityAttribute__Group__1"
// $ANTLR start "rule__DoubleEntityAttribute__Group__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12154:1: rule__DoubleEntityAttribute__Group__1__Impl : ( ( rule__DoubleEntityAttribute__TypeAssignment_1 )? ) ;
public final void rule__DoubleEntityAttribute__Group__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12158:1: ( ( ( rule__DoubleEntityAttribute__TypeAssignment_1 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12159:1: ( ( rule__DoubleEntityAttribute__TypeAssignment_1 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12159:1: ( ( rule__DoubleEntityAttribute__TypeAssignment_1 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12160:1: ( rule__DoubleEntityAttribute__TypeAssignment_1 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDoubleEntityAttributeAccess().getTypeAssignment_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12161:1: ( rule__DoubleEntityAttribute__TypeAssignment_1 )?
int alt95=2;
int LA95_0 = input.LA(1);
if ( (LA95_0==RULE_ID) ) {
int LA95_1 = input.LA(2);
if ( (LA95_1==RULE_ID||LA95_1==43) ) {
alt95=1;
}
}
switch (alt95) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12161:2: rule__DoubleEntityAttribute__TypeAssignment_1
{
pushFollow(FollowSets001.FOLLOW_rule__DoubleEntityAttribute__TypeAssignment_1_in_rule__DoubleEntityAttribute__Group__1__Impl25422);
rule__DoubleEntityAttribute__TypeAssignment_1();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDoubleEntityAttributeAccess().getTypeAssignment_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DoubleEntityAttribute__Group__1__Impl"
// $ANTLR start "rule__DoubleEntityAttribute__Group__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12171:1: rule__DoubleEntityAttribute__Group__2 : rule__DoubleEntityAttribute__Group__2__Impl ;
public final void rule__DoubleEntityAttribute__Group__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12175:1: ( rule__DoubleEntityAttribute__Group__2__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12176:2: rule__DoubleEntityAttribute__Group__2__Impl
{
pushFollow(FollowSets001.FOLLOW_rule__DoubleEntityAttribute__Group__2__Impl_in_rule__DoubleEntityAttribute__Group__225453);
rule__DoubleEntityAttribute__Group__2__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DoubleEntityAttribute__Group__2"
// $ANTLR start "rule__DoubleEntityAttribute__Group__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12182:1: rule__DoubleEntityAttribute__Group__2__Impl : ( ( rule__DoubleEntityAttribute__NameAssignment_2 ) ) ;
public final void rule__DoubleEntityAttribute__Group__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12186:1: ( ( ( rule__DoubleEntityAttribute__NameAssignment_2 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12187:1: ( ( rule__DoubleEntityAttribute__NameAssignment_2 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12187:1: ( ( rule__DoubleEntityAttribute__NameAssignment_2 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12188:1: ( rule__DoubleEntityAttribute__NameAssignment_2 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDoubleEntityAttributeAccess().getNameAssignment_2());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12189:1: ( rule__DoubleEntityAttribute__NameAssignment_2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12189:2: rule__DoubleEntityAttribute__NameAssignment_2
{
pushFollow(FollowSets001.FOLLOW_rule__DoubleEntityAttribute__NameAssignment_2_in_rule__DoubleEntityAttribute__Group__2__Impl25480);
rule__DoubleEntityAttribute__NameAssignment_2();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDoubleEntityAttributeAccess().getNameAssignment_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DoubleEntityAttribute__Group__2__Impl"
// $ANTLR start "rule__BinaryDataType__Group__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12205:1: rule__BinaryDataType__Group__0 : rule__BinaryDataType__Group__0__Impl rule__BinaryDataType__Group__1 ;
public final void rule__BinaryDataType__Group__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12209:1: ( rule__BinaryDataType__Group__0__Impl rule__BinaryDataType__Group__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12210:2: rule__BinaryDataType__Group__0__Impl rule__BinaryDataType__Group__1
{
pushFollow(FollowSets001.FOLLOW_rule__BinaryDataType__Group__0__Impl_in_rule__BinaryDataType__Group__025516);
rule__BinaryDataType__Group__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__BinaryDataType__Group__1_in_rule__BinaryDataType__Group__025519);
rule__BinaryDataType__Group__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__BinaryDataType__Group__0"
// $ANTLR start "rule__BinaryDataType__Group__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12217:1: rule__BinaryDataType__Group__0__Impl : ( 'binarydatatype' ) ;
public final void rule__BinaryDataType__Group__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12221:1: ( ( 'binarydatatype' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12222:1: ( 'binarydatatype' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12222:1: ( 'binarydatatype' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12223:1: 'binarydatatype'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getBinaryDataTypeAccess().getBinarydatatypeKeyword_0());
}
match(input,97,FollowSets001.FOLLOW_97_in_rule__BinaryDataType__Group__0__Impl25547); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getBinaryDataTypeAccess().getBinarydatatypeKeyword_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__BinaryDataType__Group__0__Impl"
// $ANTLR start "rule__BinaryDataType__Group__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12236:1: rule__BinaryDataType__Group__1 : rule__BinaryDataType__Group__1__Impl rule__BinaryDataType__Group__2 ;
public final void rule__BinaryDataType__Group__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12240:1: ( rule__BinaryDataType__Group__1__Impl rule__BinaryDataType__Group__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12241:2: rule__BinaryDataType__Group__1__Impl rule__BinaryDataType__Group__2
{
pushFollow(FollowSets001.FOLLOW_rule__BinaryDataType__Group__1__Impl_in_rule__BinaryDataType__Group__125578);
rule__BinaryDataType__Group__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__BinaryDataType__Group__2_in_rule__BinaryDataType__Group__125581);
rule__BinaryDataType__Group__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__BinaryDataType__Group__1"
// $ANTLR start "rule__BinaryDataType__Group__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12248:1: rule__BinaryDataType__Group__1__Impl : ( ( rule__BinaryDataType__NameAssignment_1 ) ) ;
public final void rule__BinaryDataType__Group__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12252:1: ( ( ( rule__BinaryDataType__NameAssignment_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12253:1: ( ( rule__BinaryDataType__NameAssignment_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12253:1: ( ( rule__BinaryDataType__NameAssignment_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12254:1: ( rule__BinaryDataType__NameAssignment_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getBinaryDataTypeAccess().getNameAssignment_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12255:1: ( rule__BinaryDataType__NameAssignment_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12255:2: rule__BinaryDataType__NameAssignment_1
{
pushFollow(FollowSets001.FOLLOW_rule__BinaryDataType__NameAssignment_1_in_rule__BinaryDataType__Group__1__Impl25608);
rule__BinaryDataType__NameAssignment_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getBinaryDataTypeAccess().getNameAssignment_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__BinaryDataType__Group__1__Impl"
// $ANTLR start "rule__BinaryDataType__Group__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12265:1: rule__BinaryDataType__Group__2 : rule__BinaryDataType__Group__2__Impl rule__BinaryDataType__Group__3 ;
public final void rule__BinaryDataType__Group__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12269:1: ( rule__BinaryDataType__Group__2__Impl rule__BinaryDataType__Group__3 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12270:2: rule__BinaryDataType__Group__2__Impl rule__BinaryDataType__Group__3
{
pushFollow(FollowSets001.FOLLOW_rule__BinaryDataType__Group__2__Impl_in_rule__BinaryDataType__Group__225638);
rule__BinaryDataType__Group__2__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__BinaryDataType__Group__3_in_rule__BinaryDataType__Group__225641);
rule__BinaryDataType__Group__3();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__BinaryDataType__Group__2"
// $ANTLR start "rule__BinaryDataType__Group__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12277:1: rule__BinaryDataType__Group__2__Impl : ( ( rule__BinaryDataType__Group_2__0 )? ) ;
public final void rule__BinaryDataType__Group__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12281:1: ( ( ( rule__BinaryDataType__Group_2__0 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12282:1: ( ( rule__BinaryDataType__Group_2__0 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12282:1: ( ( rule__BinaryDataType__Group_2__0 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12283:1: ( rule__BinaryDataType__Group_2__0 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getBinaryDataTypeAccess().getGroup_2());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12284:1: ( rule__BinaryDataType__Group_2__0 )?
int alt96=2;
int LA96_0 = input.LA(1);
if ( (LA96_0==45) ) {
alt96=1;
}
switch (alt96) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12284:2: rule__BinaryDataType__Group_2__0
{
pushFollow(FollowSets001.FOLLOW_rule__BinaryDataType__Group_2__0_in_rule__BinaryDataType__Group__2__Impl25668);
rule__BinaryDataType__Group_2__0();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getBinaryDataTypeAccess().getGroup_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__BinaryDataType__Group__2__Impl"
// $ANTLR start "rule__BinaryDataType__Group__3"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12294:1: rule__BinaryDataType__Group__3 : rule__BinaryDataType__Group__3__Impl rule__BinaryDataType__Group__4 ;
public final void rule__BinaryDataType__Group__3() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12298:1: ( rule__BinaryDataType__Group__3__Impl rule__BinaryDataType__Group__4 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12299:2: rule__BinaryDataType__Group__3__Impl rule__BinaryDataType__Group__4
{
pushFollow(FollowSets001.FOLLOW_rule__BinaryDataType__Group__3__Impl_in_rule__BinaryDataType__Group__325699);
rule__BinaryDataType__Group__3__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__BinaryDataType__Group__4_in_rule__BinaryDataType__Group__325702);
rule__BinaryDataType__Group__4();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__BinaryDataType__Group__3"
// $ANTLR start "rule__BinaryDataType__Group__3__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12306:1: rule__BinaryDataType__Group__3__Impl : ( '{' ) ;
public final void rule__BinaryDataType__Group__3__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12310:1: ( ( '{' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12311:1: ( '{' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12311:1: ( '{' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12312:1: '{'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getBinaryDataTypeAccess().getLeftCurlyBracketKeyword_3());
}
match(input,67,FollowSets001.FOLLOW_67_in_rule__BinaryDataType__Group__3__Impl25730); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getBinaryDataTypeAccess().getLeftCurlyBracketKeyword_3());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__BinaryDataType__Group__3__Impl"
// $ANTLR start "rule__BinaryDataType__Group__4"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12325:1: rule__BinaryDataType__Group__4 : rule__BinaryDataType__Group__4__Impl rule__BinaryDataType__Group__5 ;
public final void rule__BinaryDataType__Group__4() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12329:1: ( rule__BinaryDataType__Group__4__Impl rule__BinaryDataType__Group__5 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12330:2: rule__BinaryDataType__Group__4__Impl rule__BinaryDataType__Group__5
{
pushFollow(FollowSets001.FOLLOW_rule__BinaryDataType__Group__4__Impl_in_rule__BinaryDataType__Group__425761);
rule__BinaryDataType__Group__4__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__BinaryDataType__Group__5_in_rule__BinaryDataType__Group__425764);
rule__BinaryDataType__Group__5();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__BinaryDataType__Group__4"
// $ANTLR start "rule__BinaryDataType__Group__4__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12337:1: rule__BinaryDataType__Group__4__Impl : ( ( rule__BinaryDataType__BaseDataTypeAssignment_4 ) ) ;
public final void rule__BinaryDataType__Group__4__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12341:1: ( ( ( rule__BinaryDataType__BaseDataTypeAssignment_4 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12342:1: ( ( rule__BinaryDataType__BaseDataTypeAssignment_4 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12342:1: ( ( rule__BinaryDataType__BaseDataTypeAssignment_4 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12343:1: ( rule__BinaryDataType__BaseDataTypeAssignment_4 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getBinaryDataTypeAccess().getBaseDataTypeAssignment_4());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12344:1: ( rule__BinaryDataType__BaseDataTypeAssignment_4 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12344:2: rule__BinaryDataType__BaseDataTypeAssignment_4
{
pushFollow(FollowSets001.FOLLOW_rule__BinaryDataType__BaseDataTypeAssignment_4_in_rule__BinaryDataType__Group__4__Impl25791);
rule__BinaryDataType__BaseDataTypeAssignment_4();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getBinaryDataTypeAccess().getBaseDataTypeAssignment_4());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__BinaryDataType__Group__4__Impl"
// $ANTLR start "rule__BinaryDataType__Group__5"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12354:1: rule__BinaryDataType__Group__5 : rule__BinaryDataType__Group__5__Impl ;
public final void rule__BinaryDataType__Group__5() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12358:1: ( rule__BinaryDataType__Group__5__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12359:2: rule__BinaryDataType__Group__5__Impl
{
pushFollow(FollowSets001.FOLLOW_rule__BinaryDataType__Group__5__Impl_in_rule__BinaryDataType__Group__525821);
rule__BinaryDataType__Group__5__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__BinaryDataType__Group__5"
// $ANTLR start "rule__BinaryDataType__Group__5__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12365:1: rule__BinaryDataType__Group__5__Impl : ( '}' ) ;
public final void rule__BinaryDataType__Group__5__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12369:1: ( ( '}' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12370:1: ( '}' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12370:1: ( '}' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12371:1: '}'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getBinaryDataTypeAccess().getRightCurlyBracketKeyword_5());
}
match(input,68,FollowSets001.FOLLOW_68_in_rule__BinaryDataType__Group__5__Impl25849); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getBinaryDataTypeAccess().getRightCurlyBracketKeyword_5());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__BinaryDataType__Group__5__Impl"
// $ANTLR start "rule__BinaryDataType__Group_2__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12396:1: rule__BinaryDataType__Group_2__0 : rule__BinaryDataType__Group_2__0__Impl rule__BinaryDataType__Group_2__1 ;
public final void rule__BinaryDataType__Group_2__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12400:1: ( rule__BinaryDataType__Group_2__0__Impl rule__BinaryDataType__Group_2__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12401:2: rule__BinaryDataType__Group_2__0__Impl rule__BinaryDataType__Group_2__1
{
pushFollow(FollowSets001.FOLLOW_rule__BinaryDataType__Group_2__0__Impl_in_rule__BinaryDataType__Group_2__025892);
rule__BinaryDataType__Group_2__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__BinaryDataType__Group_2__1_in_rule__BinaryDataType__Group_2__025895);
rule__BinaryDataType__Group_2__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__BinaryDataType__Group_2__0"
// $ANTLR start "rule__BinaryDataType__Group_2__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12408:1: rule__BinaryDataType__Group_2__0__Impl : ( 'extends' ) ;
public final void rule__BinaryDataType__Group_2__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12412:1: ( ( 'extends' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12413:1: ( 'extends' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12413:1: ( 'extends' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12414:1: 'extends'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getBinaryDataTypeAccess().getExtendsKeyword_2_0());
}
match(input,45,FollowSets001.FOLLOW_45_in_rule__BinaryDataType__Group_2__0__Impl25923); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getBinaryDataTypeAccess().getExtendsKeyword_2_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__BinaryDataType__Group_2__0__Impl"
// $ANTLR start "rule__BinaryDataType__Group_2__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12427:1: rule__BinaryDataType__Group_2__1 : rule__BinaryDataType__Group_2__1__Impl ;
public final void rule__BinaryDataType__Group_2__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12431:1: ( rule__BinaryDataType__Group_2__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12432:2: rule__BinaryDataType__Group_2__1__Impl
{
pushFollow(FollowSets001.FOLLOW_rule__BinaryDataType__Group_2__1__Impl_in_rule__BinaryDataType__Group_2__125954);
rule__BinaryDataType__Group_2__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__BinaryDataType__Group_2__1"
// $ANTLR start "rule__BinaryDataType__Group_2__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12438:1: rule__BinaryDataType__Group_2__1__Impl : ( ( rule__BinaryDataType__RefAssignment_2_1 ) ) ;
public final void rule__BinaryDataType__Group_2__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12442:1: ( ( ( rule__BinaryDataType__RefAssignment_2_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12443:1: ( ( rule__BinaryDataType__RefAssignment_2_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12443:1: ( ( rule__BinaryDataType__RefAssignment_2_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12444:1: ( rule__BinaryDataType__RefAssignment_2_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getBinaryDataTypeAccess().getRefAssignment_2_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12445:1: ( rule__BinaryDataType__RefAssignment_2_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12445:2: rule__BinaryDataType__RefAssignment_2_1
{
pushFollow(FollowSets001.FOLLOW_rule__BinaryDataType__RefAssignment_2_1_in_rule__BinaryDataType__Group_2__1__Impl25981);
rule__BinaryDataType__RefAssignment_2_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getBinaryDataTypeAccess().getRefAssignment_2_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__BinaryDataType__Group_2__1__Impl"
// $ANTLR start "rule__BinaryEntityAttribute__Group__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12459:1: rule__BinaryEntityAttribute__Group__0 : rule__BinaryEntityAttribute__Group__0__Impl rule__BinaryEntityAttribute__Group__1 ;
public final void rule__BinaryEntityAttribute__Group__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12463:1: ( rule__BinaryEntityAttribute__Group__0__Impl rule__BinaryEntityAttribute__Group__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12464:2: rule__BinaryEntityAttribute__Group__0__Impl rule__BinaryEntityAttribute__Group__1
{
pushFollow(FollowSets001.FOLLOW_rule__BinaryEntityAttribute__Group__0__Impl_in_rule__BinaryEntityAttribute__Group__026015);
rule__BinaryEntityAttribute__Group__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__BinaryEntityAttribute__Group__1_in_rule__BinaryEntityAttribute__Group__026018);
rule__BinaryEntityAttribute__Group__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__BinaryEntityAttribute__Group__0"
// $ANTLR start "rule__BinaryEntityAttribute__Group__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12471:1: rule__BinaryEntityAttribute__Group__0__Impl : ( 'binary' ) ;
public final void rule__BinaryEntityAttribute__Group__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12475:1: ( ( 'binary' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12476:1: ( 'binary' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12476:1: ( 'binary' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12477:1: 'binary'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getBinaryEntityAttributeAccess().getBinaryKeyword_0());
}
match(input,98,FollowSets001.FOLLOW_98_in_rule__BinaryEntityAttribute__Group__0__Impl26046); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getBinaryEntityAttributeAccess().getBinaryKeyword_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__BinaryEntityAttribute__Group__0__Impl"
// $ANTLR start "rule__BinaryEntityAttribute__Group__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12490:1: rule__BinaryEntityAttribute__Group__1 : rule__BinaryEntityAttribute__Group__1__Impl rule__BinaryEntityAttribute__Group__2 ;
public final void rule__BinaryEntityAttribute__Group__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12494:1: ( rule__BinaryEntityAttribute__Group__1__Impl rule__BinaryEntityAttribute__Group__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12495:2: rule__BinaryEntityAttribute__Group__1__Impl rule__BinaryEntityAttribute__Group__2
{
pushFollow(FollowSets001.FOLLOW_rule__BinaryEntityAttribute__Group__1__Impl_in_rule__BinaryEntityAttribute__Group__126077);
rule__BinaryEntityAttribute__Group__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__BinaryEntityAttribute__Group__2_in_rule__BinaryEntityAttribute__Group__126080);
rule__BinaryEntityAttribute__Group__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__BinaryEntityAttribute__Group__1"
// $ANTLR start "rule__BinaryEntityAttribute__Group__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12502:1: rule__BinaryEntityAttribute__Group__1__Impl : ( ( rule__BinaryEntityAttribute__TypeAssignment_1 )? ) ;
public final void rule__BinaryEntityAttribute__Group__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12506:1: ( ( ( rule__BinaryEntityAttribute__TypeAssignment_1 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12507:1: ( ( rule__BinaryEntityAttribute__TypeAssignment_1 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12507:1: ( ( rule__BinaryEntityAttribute__TypeAssignment_1 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12508:1: ( rule__BinaryEntityAttribute__TypeAssignment_1 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getBinaryEntityAttributeAccess().getTypeAssignment_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12509:1: ( rule__BinaryEntityAttribute__TypeAssignment_1 )?
int alt97=2;
int LA97_0 = input.LA(1);
if ( (LA97_0==RULE_ID) ) {
int LA97_1 = input.LA(2);
if ( (LA97_1==RULE_ID||LA97_1==43) ) {
alt97=1;
}
}
switch (alt97) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12509:2: rule__BinaryEntityAttribute__TypeAssignment_1
{
pushFollow(FollowSets001.FOLLOW_rule__BinaryEntityAttribute__TypeAssignment_1_in_rule__BinaryEntityAttribute__Group__1__Impl26107);
rule__BinaryEntityAttribute__TypeAssignment_1();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getBinaryEntityAttributeAccess().getTypeAssignment_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__BinaryEntityAttribute__Group__1__Impl"
// $ANTLR start "rule__BinaryEntityAttribute__Group__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12519:1: rule__BinaryEntityAttribute__Group__2 : rule__BinaryEntityAttribute__Group__2__Impl ;
public final void rule__BinaryEntityAttribute__Group__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12523:1: ( rule__BinaryEntityAttribute__Group__2__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12524:2: rule__BinaryEntityAttribute__Group__2__Impl
{
pushFollow(FollowSets001.FOLLOW_rule__BinaryEntityAttribute__Group__2__Impl_in_rule__BinaryEntityAttribute__Group__226138);
rule__BinaryEntityAttribute__Group__2__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__BinaryEntityAttribute__Group__2"
// $ANTLR start "rule__BinaryEntityAttribute__Group__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12530:1: rule__BinaryEntityAttribute__Group__2__Impl : ( ( rule__BinaryEntityAttribute__NameAssignment_2 ) ) ;
public final void rule__BinaryEntityAttribute__Group__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12534:1: ( ( ( rule__BinaryEntityAttribute__NameAssignment_2 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12535:1: ( ( rule__BinaryEntityAttribute__NameAssignment_2 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12535:1: ( ( rule__BinaryEntityAttribute__NameAssignment_2 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12536:1: ( rule__BinaryEntityAttribute__NameAssignment_2 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getBinaryEntityAttributeAccess().getNameAssignment_2());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12537:1: ( rule__BinaryEntityAttribute__NameAssignment_2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12537:2: rule__BinaryEntityAttribute__NameAssignment_2
{
pushFollow(FollowSets001.FOLLOW_rule__BinaryEntityAttribute__NameAssignment_2_in_rule__BinaryEntityAttribute__Group__2__Impl26165);
rule__BinaryEntityAttribute__NameAssignment_2();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getBinaryEntityAttributeAccess().getNameAssignment_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__BinaryEntityAttribute__Group__2__Impl"
// $ANTLR start "rule__EntityDataType__Group__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12553:1: rule__EntityDataType__Group__0 : rule__EntityDataType__Group__0__Impl rule__EntityDataType__Group__1 ;
public final void rule__EntityDataType__Group__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12557:1: ( rule__EntityDataType__Group__0__Impl rule__EntityDataType__Group__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12558:2: rule__EntityDataType__Group__0__Impl rule__EntityDataType__Group__1
{
pushFollow(FollowSets001.FOLLOW_rule__EntityDataType__Group__0__Impl_in_rule__EntityDataType__Group__026201);
rule__EntityDataType__Group__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__EntityDataType__Group__1_in_rule__EntityDataType__Group__026204);
rule__EntityDataType__Group__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EntityDataType__Group__0"
// $ANTLR start "rule__EntityDataType__Group__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12565:1: rule__EntityDataType__Group__0__Impl : ( 'entitydatatype' ) ;
public final void rule__EntityDataType__Group__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12569:1: ( ( 'entitydatatype' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12570:1: ( 'entitydatatype' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12570:1: ( 'entitydatatype' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12571:1: 'entitydatatype'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEntityDataTypeAccess().getEntitydatatypeKeyword_0());
}
match(input,99,FollowSets001.FOLLOW_99_in_rule__EntityDataType__Group__0__Impl26232); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getEntityDataTypeAccess().getEntitydatatypeKeyword_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EntityDataType__Group__0__Impl"
// $ANTLR start "rule__EntityDataType__Group__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12584:1: rule__EntityDataType__Group__1 : rule__EntityDataType__Group__1__Impl rule__EntityDataType__Group__2 ;
public final void rule__EntityDataType__Group__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12588:1: ( rule__EntityDataType__Group__1__Impl rule__EntityDataType__Group__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12589:2: rule__EntityDataType__Group__1__Impl rule__EntityDataType__Group__2
{
pushFollow(FollowSets001.FOLLOW_rule__EntityDataType__Group__1__Impl_in_rule__EntityDataType__Group__126263);
rule__EntityDataType__Group__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__EntityDataType__Group__2_in_rule__EntityDataType__Group__126266);
rule__EntityDataType__Group__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EntityDataType__Group__1"
// $ANTLR start "rule__EntityDataType__Group__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12596:1: rule__EntityDataType__Group__1__Impl : ( ( rule__EntityDataType__NameAssignment_1 ) ) ;
public final void rule__EntityDataType__Group__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12600:1: ( ( ( rule__EntityDataType__NameAssignment_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12601:1: ( ( rule__EntityDataType__NameAssignment_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12601:1: ( ( rule__EntityDataType__NameAssignment_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12602:1: ( rule__EntityDataType__NameAssignment_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEntityDataTypeAccess().getNameAssignment_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12603:1: ( rule__EntityDataType__NameAssignment_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12603:2: rule__EntityDataType__NameAssignment_1
{
pushFollow(FollowSets001.FOLLOW_rule__EntityDataType__NameAssignment_1_in_rule__EntityDataType__Group__1__Impl26293);
rule__EntityDataType__NameAssignment_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getEntityDataTypeAccess().getNameAssignment_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EntityDataType__Group__1__Impl"
// $ANTLR start "rule__EntityDataType__Group__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12613:1: rule__EntityDataType__Group__2 : rule__EntityDataType__Group__2__Impl rule__EntityDataType__Group__3 ;
public final void rule__EntityDataType__Group__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12617:1: ( rule__EntityDataType__Group__2__Impl rule__EntityDataType__Group__3 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12618:2: rule__EntityDataType__Group__2__Impl rule__EntityDataType__Group__3
{
pushFollow(FollowSets001.FOLLOW_rule__EntityDataType__Group__2__Impl_in_rule__EntityDataType__Group__226323);
rule__EntityDataType__Group__2__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__EntityDataType__Group__3_in_rule__EntityDataType__Group__226326);
rule__EntityDataType__Group__3();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EntityDataType__Group__2"
// $ANTLR start "rule__EntityDataType__Group__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12625:1: rule__EntityDataType__Group__2__Impl : ( ( rule__EntityDataType__Group_2__0 )? ) ;
public final void rule__EntityDataType__Group__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12629:1: ( ( ( rule__EntityDataType__Group_2__0 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12630:1: ( ( rule__EntityDataType__Group_2__0 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12630:1: ( ( rule__EntityDataType__Group_2__0 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12631:1: ( rule__EntityDataType__Group_2__0 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEntityDataTypeAccess().getGroup_2());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12632:1: ( rule__EntityDataType__Group_2__0 )?
int alt98=2;
int LA98_0 = input.LA(1);
if ( (LA98_0==45) ) {
alt98=1;
}
switch (alt98) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12632:2: rule__EntityDataType__Group_2__0
{
pushFollow(FollowSets001.FOLLOW_rule__EntityDataType__Group_2__0_in_rule__EntityDataType__Group__2__Impl26353);
rule__EntityDataType__Group_2__0();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getEntityDataTypeAccess().getGroup_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EntityDataType__Group__2__Impl"
// $ANTLR start "rule__EntityDataType__Group__3"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12642:1: rule__EntityDataType__Group__3 : rule__EntityDataType__Group__3__Impl rule__EntityDataType__Group__4 ;
public final void rule__EntityDataType__Group__3() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12646:1: ( rule__EntityDataType__Group__3__Impl rule__EntityDataType__Group__4 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12647:2: rule__EntityDataType__Group__3__Impl rule__EntityDataType__Group__4
{
pushFollow(FollowSets001.FOLLOW_rule__EntityDataType__Group__3__Impl_in_rule__EntityDataType__Group__326384);
rule__EntityDataType__Group__3__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__EntityDataType__Group__4_in_rule__EntityDataType__Group__326387);
rule__EntityDataType__Group__4();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EntityDataType__Group__3"
// $ANTLR start "rule__EntityDataType__Group__3__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12654:1: rule__EntityDataType__Group__3__Impl : ( '{' ) ;
public final void rule__EntityDataType__Group__3__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12658:1: ( ( '{' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12659:1: ( '{' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12659:1: ( '{' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12660:1: '{'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEntityDataTypeAccess().getLeftCurlyBracketKeyword_3());
}
match(input,67,FollowSets001.FOLLOW_67_in_rule__EntityDataType__Group__3__Impl26415); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getEntityDataTypeAccess().getLeftCurlyBracketKeyword_3());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EntityDataType__Group__3__Impl"
// $ANTLR start "rule__EntityDataType__Group__4"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12673:1: rule__EntityDataType__Group__4 : rule__EntityDataType__Group__4__Impl rule__EntityDataType__Group__5 ;
public final void rule__EntityDataType__Group__4() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12677:1: ( rule__EntityDataType__Group__4__Impl rule__EntityDataType__Group__5 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12678:2: rule__EntityDataType__Group__4__Impl rule__EntityDataType__Group__5
{
pushFollow(FollowSets001.FOLLOW_rule__EntityDataType__Group__4__Impl_in_rule__EntityDataType__Group__426446);
rule__EntityDataType__Group__4__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__EntityDataType__Group__5_in_rule__EntityDataType__Group__426449);
rule__EntityDataType__Group__5();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EntityDataType__Group__4"
// $ANTLR start "rule__EntityDataType__Group__4__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12685:1: rule__EntityDataType__Group__4__Impl : ( ( rule__EntityDataType__BaseDataTypeAssignment_4 ) ) ;
public final void rule__EntityDataType__Group__4__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12689:1: ( ( ( rule__EntityDataType__BaseDataTypeAssignment_4 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12690:1: ( ( rule__EntityDataType__BaseDataTypeAssignment_4 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12690:1: ( ( rule__EntityDataType__BaseDataTypeAssignment_4 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12691:1: ( rule__EntityDataType__BaseDataTypeAssignment_4 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEntityDataTypeAccess().getBaseDataTypeAssignment_4());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12692:1: ( rule__EntityDataType__BaseDataTypeAssignment_4 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12692:2: rule__EntityDataType__BaseDataTypeAssignment_4
{
pushFollow(FollowSets001.FOLLOW_rule__EntityDataType__BaseDataTypeAssignment_4_in_rule__EntityDataType__Group__4__Impl26476);
rule__EntityDataType__BaseDataTypeAssignment_4();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getEntityDataTypeAccess().getBaseDataTypeAssignment_4());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EntityDataType__Group__4__Impl"
// $ANTLR start "rule__EntityDataType__Group__5"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12702:1: rule__EntityDataType__Group__5 : rule__EntityDataType__Group__5__Impl rule__EntityDataType__Group__6 ;
public final void rule__EntityDataType__Group__5() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12706:1: ( rule__EntityDataType__Group__5__Impl rule__EntityDataType__Group__6 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12707:2: rule__EntityDataType__Group__5__Impl rule__EntityDataType__Group__6
{
pushFollow(FollowSets001.FOLLOW_rule__EntityDataType__Group__5__Impl_in_rule__EntityDataType__Group__526506);
rule__EntityDataType__Group__5__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__EntityDataType__Group__6_in_rule__EntityDataType__Group__526509);
rule__EntityDataType__Group__6();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EntityDataType__Group__5"
// $ANTLR start "rule__EntityDataType__Group__5__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12714:1: rule__EntityDataType__Group__5__Impl : ( 'entity' ) ;
public final void rule__EntityDataType__Group__5__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12718:1: ( ( 'entity' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12719:1: ( 'entity' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12719:1: ( 'entity' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12720:1: 'entity'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEntityDataTypeAccess().getEntityKeyword_5());
}
match(input,78,FollowSets001.FOLLOW_78_in_rule__EntityDataType__Group__5__Impl26537); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getEntityDataTypeAccess().getEntityKeyword_5());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EntityDataType__Group__5__Impl"
// $ANTLR start "rule__EntityDataType__Group__6"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12733:1: rule__EntityDataType__Group__6 : rule__EntityDataType__Group__6__Impl rule__EntityDataType__Group__7 ;
public final void rule__EntityDataType__Group__6() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12737:1: ( rule__EntityDataType__Group__6__Impl rule__EntityDataType__Group__7 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12738:2: rule__EntityDataType__Group__6__Impl rule__EntityDataType__Group__7
{
pushFollow(FollowSets001.FOLLOW_rule__EntityDataType__Group__6__Impl_in_rule__EntityDataType__Group__626568);
rule__EntityDataType__Group__6__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__EntityDataType__Group__7_in_rule__EntityDataType__Group__626571);
rule__EntityDataType__Group__7();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EntityDataType__Group__6"
// $ANTLR start "rule__EntityDataType__Group__6__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12745:1: rule__EntityDataType__Group__6__Impl : ( ( rule__EntityDataType__EntityAssignment_6 ) ) ;
public final void rule__EntityDataType__Group__6__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12749:1: ( ( ( rule__EntityDataType__EntityAssignment_6 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12750:1: ( ( rule__EntityDataType__EntityAssignment_6 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12750:1: ( ( rule__EntityDataType__EntityAssignment_6 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12751:1: ( rule__EntityDataType__EntityAssignment_6 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEntityDataTypeAccess().getEntityAssignment_6());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12752:1: ( rule__EntityDataType__EntityAssignment_6 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12752:2: rule__EntityDataType__EntityAssignment_6
{
pushFollow(FollowSets001.FOLLOW_rule__EntityDataType__EntityAssignment_6_in_rule__EntityDataType__Group__6__Impl26598);
rule__EntityDataType__EntityAssignment_6();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getEntityDataTypeAccess().getEntityAssignment_6());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EntityDataType__Group__6__Impl"
// $ANTLR start "rule__EntityDataType__Group__7"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12762:1: rule__EntityDataType__Group__7 : rule__EntityDataType__Group__7__Impl ;
public final void rule__EntityDataType__Group__7() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12766:1: ( rule__EntityDataType__Group__7__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12767:2: rule__EntityDataType__Group__7__Impl
{
pushFollow(FollowSets001.FOLLOW_rule__EntityDataType__Group__7__Impl_in_rule__EntityDataType__Group__726628);
rule__EntityDataType__Group__7__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EntityDataType__Group__7"
// $ANTLR start "rule__EntityDataType__Group__7__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12773:1: rule__EntityDataType__Group__7__Impl : ( '}' ) ;
public final void rule__EntityDataType__Group__7__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12777:1: ( ( '}' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12778:1: ( '}' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12778:1: ( '}' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12779:1: '}'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEntityDataTypeAccess().getRightCurlyBracketKeyword_7());
}
match(input,68,FollowSets001.FOLLOW_68_in_rule__EntityDataType__Group__7__Impl26656); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getEntityDataTypeAccess().getRightCurlyBracketKeyword_7());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EntityDataType__Group__7__Impl"
// $ANTLR start "rule__EntityDataType__Group_2__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12808:1: rule__EntityDataType__Group_2__0 : rule__EntityDataType__Group_2__0__Impl rule__EntityDataType__Group_2__1 ;
public final void rule__EntityDataType__Group_2__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12812:1: ( rule__EntityDataType__Group_2__0__Impl rule__EntityDataType__Group_2__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12813:2: rule__EntityDataType__Group_2__0__Impl rule__EntityDataType__Group_2__1
{
pushFollow(FollowSets001.FOLLOW_rule__EntityDataType__Group_2__0__Impl_in_rule__EntityDataType__Group_2__026703);
rule__EntityDataType__Group_2__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__EntityDataType__Group_2__1_in_rule__EntityDataType__Group_2__026706);
rule__EntityDataType__Group_2__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EntityDataType__Group_2__0"
// $ANTLR start "rule__EntityDataType__Group_2__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12820:1: rule__EntityDataType__Group_2__0__Impl : ( 'extends' ) ;
public final void rule__EntityDataType__Group_2__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12824:1: ( ( 'extends' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12825:1: ( 'extends' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12825:1: ( 'extends' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12826:1: 'extends'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEntityDataTypeAccess().getExtendsKeyword_2_0());
}
match(input,45,FollowSets001.FOLLOW_45_in_rule__EntityDataType__Group_2__0__Impl26734); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getEntityDataTypeAccess().getExtendsKeyword_2_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EntityDataType__Group_2__0__Impl"
// $ANTLR start "rule__EntityDataType__Group_2__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12839:1: rule__EntityDataType__Group_2__1 : rule__EntityDataType__Group_2__1__Impl ;
public final void rule__EntityDataType__Group_2__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12843:1: ( rule__EntityDataType__Group_2__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12844:2: rule__EntityDataType__Group_2__1__Impl
{
pushFollow(FollowSets001.FOLLOW_rule__EntityDataType__Group_2__1__Impl_in_rule__EntityDataType__Group_2__126765);
rule__EntityDataType__Group_2__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EntityDataType__Group_2__1"
// $ANTLR start "rule__EntityDataType__Group_2__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12850:1: rule__EntityDataType__Group_2__1__Impl : ( ( rule__EntityDataType__RefAssignment_2_1 ) ) ;
public final void rule__EntityDataType__Group_2__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12854:1: ( ( ( rule__EntityDataType__RefAssignment_2_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12855:1: ( ( rule__EntityDataType__RefAssignment_2_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12855:1: ( ( rule__EntityDataType__RefAssignment_2_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12856:1: ( rule__EntityDataType__RefAssignment_2_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEntityDataTypeAccess().getRefAssignment_2_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12857:1: ( rule__EntityDataType__RefAssignment_2_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12857:2: rule__EntityDataType__RefAssignment_2_1
{
pushFollow(FollowSets001.FOLLOW_rule__EntityDataType__RefAssignment_2_1_in_rule__EntityDataType__Group_2__1__Impl26792);
rule__EntityDataType__RefAssignment_2_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getEntityDataTypeAccess().getRefAssignment_2_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EntityDataType__Group_2__1__Impl"
// $ANTLR start "rule__EntityEntityAttribute__Group__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12871:1: rule__EntityEntityAttribute__Group__0 : rule__EntityEntityAttribute__Group__0__Impl rule__EntityEntityAttribute__Group__1 ;
public final void rule__EntityEntityAttribute__Group__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12875:1: ( rule__EntityEntityAttribute__Group__0__Impl rule__EntityEntityAttribute__Group__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12876:2: rule__EntityEntityAttribute__Group__0__Impl rule__EntityEntityAttribute__Group__1
{
pushFollow(FollowSets001.FOLLOW_rule__EntityEntityAttribute__Group__0__Impl_in_rule__EntityEntityAttribute__Group__026826);
rule__EntityEntityAttribute__Group__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__EntityEntityAttribute__Group__1_in_rule__EntityEntityAttribute__Group__026829);
rule__EntityEntityAttribute__Group__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EntityEntityAttribute__Group__0"
// $ANTLR start "rule__EntityEntityAttribute__Group__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12883:1: rule__EntityEntityAttribute__Group__0__Impl : ( 'entity' ) ;
public final void rule__EntityEntityAttribute__Group__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12887:1: ( ( 'entity' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12888:1: ( 'entity' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12888:1: ( 'entity' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12889:1: 'entity'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEntityEntityAttributeAccess().getEntityKeyword_0());
}
match(input,78,FollowSets001.FOLLOW_78_in_rule__EntityEntityAttribute__Group__0__Impl26857); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getEntityEntityAttributeAccess().getEntityKeyword_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EntityEntityAttribute__Group__0__Impl"
// $ANTLR start "rule__EntityEntityAttribute__Group__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12902:1: rule__EntityEntityAttribute__Group__1 : rule__EntityEntityAttribute__Group__1__Impl rule__EntityEntityAttribute__Group__2 ;
public final void rule__EntityEntityAttribute__Group__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12906:1: ( rule__EntityEntityAttribute__Group__1__Impl rule__EntityEntityAttribute__Group__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12907:2: rule__EntityEntityAttribute__Group__1__Impl rule__EntityEntityAttribute__Group__2
{
pushFollow(FollowSets001.FOLLOW_rule__EntityEntityAttribute__Group__1__Impl_in_rule__EntityEntityAttribute__Group__126888);
rule__EntityEntityAttribute__Group__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__EntityEntityAttribute__Group__2_in_rule__EntityEntityAttribute__Group__126891);
rule__EntityEntityAttribute__Group__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EntityEntityAttribute__Group__1"
// $ANTLR start "rule__EntityEntityAttribute__Group__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12914:1: rule__EntityEntityAttribute__Group__1__Impl : ( ( rule__EntityEntityAttribute__TypeAssignment_1 ) ) ;
public final void rule__EntityEntityAttribute__Group__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12918:1: ( ( ( rule__EntityEntityAttribute__TypeAssignment_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12919:1: ( ( rule__EntityEntityAttribute__TypeAssignment_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12919:1: ( ( rule__EntityEntityAttribute__TypeAssignment_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12920:1: ( rule__EntityEntityAttribute__TypeAssignment_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEntityEntityAttributeAccess().getTypeAssignment_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12921:1: ( rule__EntityEntityAttribute__TypeAssignment_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12921:2: rule__EntityEntityAttribute__TypeAssignment_1
{
pushFollow(FollowSets001.FOLLOW_rule__EntityEntityAttribute__TypeAssignment_1_in_rule__EntityEntityAttribute__Group__1__Impl26918);
rule__EntityEntityAttribute__TypeAssignment_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getEntityEntityAttributeAccess().getTypeAssignment_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EntityEntityAttribute__Group__1__Impl"
// $ANTLR start "rule__EntityEntityAttribute__Group__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12931:1: rule__EntityEntityAttribute__Group__2 : rule__EntityEntityAttribute__Group__2__Impl rule__EntityEntityAttribute__Group__3 ;
public final void rule__EntityEntityAttribute__Group__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12935:1: ( rule__EntityEntityAttribute__Group__2__Impl rule__EntityEntityAttribute__Group__3 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12936:2: rule__EntityEntityAttribute__Group__2__Impl rule__EntityEntityAttribute__Group__3
{
pushFollow(FollowSets001.FOLLOW_rule__EntityEntityAttribute__Group__2__Impl_in_rule__EntityEntityAttribute__Group__226948);
rule__EntityEntityAttribute__Group__2__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__EntityEntityAttribute__Group__3_in_rule__EntityEntityAttribute__Group__226951);
rule__EntityEntityAttribute__Group__3();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EntityEntityAttribute__Group__2"
// $ANTLR start "rule__EntityEntityAttribute__Group__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12943:1: rule__EntityEntityAttribute__Group__2__Impl : ( ( rule__EntityEntityAttribute__CardinalityAssignment_2 )? ) ;
public final void rule__EntityEntityAttribute__Group__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12947:1: ( ( ( rule__EntityEntityAttribute__CardinalityAssignment_2 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12948:1: ( ( rule__EntityEntityAttribute__CardinalityAssignment_2 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12948:1: ( ( rule__EntityEntityAttribute__CardinalityAssignment_2 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12949:1: ( rule__EntityEntityAttribute__CardinalityAssignment_2 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEntityEntityAttributeAccess().getCardinalityAssignment_2());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12950:1: ( rule__EntityEntityAttribute__CardinalityAssignment_2 )?
int alt99=2;
int LA99_0 = input.LA(1);
if ( ((LA99_0>=53 && LA99_0<=55)) ) {
alt99=1;
}
switch (alt99) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12950:2: rule__EntityEntityAttribute__CardinalityAssignment_2
{
pushFollow(FollowSets001.FOLLOW_rule__EntityEntityAttribute__CardinalityAssignment_2_in_rule__EntityEntityAttribute__Group__2__Impl26978);
rule__EntityEntityAttribute__CardinalityAssignment_2();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getEntityEntityAttributeAccess().getCardinalityAssignment_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EntityEntityAttribute__Group__2__Impl"
// $ANTLR start "rule__EntityEntityAttribute__Group__3"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12960:1: rule__EntityEntityAttribute__Group__3 : rule__EntityEntityAttribute__Group__3__Impl ;
public final void rule__EntityEntityAttribute__Group__3() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12964:1: ( rule__EntityEntityAttribute__Group__3__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12965:2: rule__EntityEntityAttribute__Group__3__Impl
{
pushFollow(FollowSets001.FOLLOW_rule__EntityEntityAttribute__Group__3__Impl_in_rule__EntityEntityAttribute__Group__327009);
rule__EntityEntityAttribute__Group__3__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EntityEntityAttribute__Group__3"
// $ANTLR start "rule__EntityEntityAttribute__Group__3__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12971:1: rule__EntityEntityAttribute__Group__3__Impl : ( ( rule__EntityEntityAttribute__NameAssignment_3 ) ) ;
public final void rule__EntityEntityAttribute__Group__3__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12975:1: ( ( ( rule__EntityEntityAttribute__NameAssignment_3 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12976:1: ( ( rule__EntityEntityAttribute__NameAssignment_3 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12976:1: ( ( rule__EntityEntityAttribute__NameAssignment_3 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12977:1: ( rule__EntityEntityAttribute__NameAssignment_3 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEntityEntityAttributeAccess().getNameAssignment_3());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12978:1: ( rule__EntityEntityAttribute__NameAssignment_3 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12978:2: rule__EntityEntityAttribute__NameAssignment_3
{
pushFollow(FollowSets001.FOLLOW_rule__EntityEntityAttribute__NameAssignment_3_in_rule__EntityEntityAttribute__Group__3__Impl27036);
rule__EntityEntityAttribute__NameAssignment_3();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getEntityEntityAttributeAccess().getNameAssignment_3());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EntityEntityAttribute__Group__3__Impl"
// $ANTLR start "rule__ValueObjectEntityAttribute__Group__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:12996:1: rule__ValueObjectEntityAttribute__Group__0 : rule__ValueObjectEntityAttribute__Group__0__Impl rule__ValueObjectEntityAttribute__Group__1 ;
public final void rule__ValueObjectEntityAttribute__Group__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13000:1: ( rule__ValueObjectEntityAttribute__Group__0__Impl rule__ValueObjectEntityAttribute__Group__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13001:2: rule__ValueObjectEntityAttribute__Group__0__Impl rule__ValueObjectEntityAttribute__Group__1
{
pushFollow(FollowSets001.FOLLOW_rule__ValueObjectEntityAttribute__Group__0__Impl_in_rule__ValueObjectEntityAttribute__Group__027074);
rule__ValueObjectEntityAttribute__Group__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__ValueObjectEntityAttribute__Group__1_in_rule__ValueObjectEntityAttribute__Group__027077);
rule__ValueObjectEntityAttribute__Group__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ValueObjectEntityAttribute__Group__0"
// $ANTLR start "rule__ValueObjectEntityAttribute__Group__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13008:1: rule__ValueObjectEntityAttribute__Group__0__Impl : ( 'valueobject' ) ;
public final void rule__ValueObjectEntityAttribute__Group__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13012:1: ( ( 'valueobject' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13013:1: ( 'valueobject' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13013:1: ( 'valueobject' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13014:1: 'valueobject'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getValueObjectEntityAttributeAccess().getValueobjectKeyword_0());
}
match(input,80,FollowSets001.FOLLOW_80_in_rule__ValueObjectEntityAttribute__Group__0__Impl27105); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getValueObjectEntityAttributeAccess().getValueobjectKeyword_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ValueObjectEntityAttribute__Group__0__Impl"
// $ANTLR start "rule__ValueObjectEntityAttribute__Group__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13027:1: rule__ValueObjectEntityAttribute__Group__1 : rule__ValueObjectEntityAttribute__Group__1__Impl rule__ValueObjectEntityAttribute__Group__2 ;
public final void rule__ValueObjectEntityAttribute__Group__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13031:1: ( rule__ValueObjectEntityAttribute__Group__1__Impl rule__ValueObjectEntityAttribute__Group__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13032:2: rule__ValueObjectEntityAttribute__Group__1__Impl rule__ValueObjectEntityAttribute__Group__2
{
pushFollow(FollowSets001.FOLLOW_rule__ValueObjectEntityAttribute__Group__1__Impl_in_rule__ValueObjectEntityAttribute__Group__127136);
rule__ValueObjectEntityAttribute__Group__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__ValueObjectEntityAttribute__Group__2_in_rule__ValueObjectEntityAttribute__Group__127139);
rule__ValueObjectEntityAttribute__Group__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ValueObjectEntityAttribute__Group__1"
// $ANTLR start "rule__ValueObjectEntityAttribute__Group__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13039:1: rule__ValueObjectEntityAttribute__Group__1__Impl : ( ( rule__ValueObjectEntityAttribute__TypeAssignment_1 ) ) ;
public final void rule__ValueObjectEntityAttribute__Group__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13043:1: ( ( ( rule__ValueObjectEntityAttribute__TypeAssignment_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13044:1: ( ( rule__ValueObjectEntityAttribute__TypeAssignment_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13044:1: ( ( rule__ValueObjectEntityAttribute__TypeAssignment_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13045:1: ( rule__ValueObjectEntityAttribute__TypeAssignment_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getValueObjectEntityAttributeAccess().getTypeAssignment_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13046:1: ( rule__ValueObjectEntityAttribute__TypeAssignment_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13046:2: rule__ValueObjectEntityAttribute__TypeAssignment_1
{
pushFollow(FollowSets001.FOLLOW_rule__ValueObjectEntityAttribute__TypeAssignment_1_in_rule__ValueObjectEntityAttribute__Group__1__Impl27166);
rule__ValueObjectEntityAttribute__TypeAssignment_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getValueObjectEntityAttributeAccess().getTypeAssignment_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ValueObjectEntityAttribute__Group__1__Impl"
// $ANTLR start "rule__ValueObjectEntityAttribute__Group__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13056:1: rule__ValueObjectEntityAttribute__Group__2 : rule__ValueObjectEntityAttribute__Group__2__Impl rule__ValueObjectEntityAttribute__Group__3 ;
public final void rule__ValueObjectEntityAttribute__Group__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13060:1: ( rule__ValueObjectEntityAttribute__Group__2__Impl rule__ValueObjectEntityAttribute__Group__3 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13061:2: rule__ValueObjectEntityAttribute__Group__2__Impl rule__ValueObjectEntityAttribute__Group__3
{
pushFollow(FollowSets001.FOLLOW_rule__ValueObjectEntityAttribute__Group__2__Impl_in_rule__ValueObjectEntityAttribute__Group__227196);
rule__ValueObjectEntityAttribute__Group__2__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__ValueObjectEntityAttribute__Group__3_in_rule__ValueObjectEntityAttribute__Group__227199);
rule__ValueObjectEntityAttribute__Group__3();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ValueObjectEntityAttribute__Group__2"
// $ANTLR start "rule__ValueObjectEntityAttribute__Group__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13068:1: rule__ValueObjectEntityAttribute__Group__2__Impl : ( ( rule__ValueObjectEntityAttribute__CardinalityAssignment_2 )? ) ;
public final void rule__ValueObjectEntityAttribute__Group__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13072:1: ( ( ( rule__ValueObjectEntityAttribute__CardinalityAssignment_2 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13073:1: ( ( rule__ValueObjectEntityAttribute__CardinalityAssignment_2 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13073:1: ( ( rule__ValueObjectEntityAttribute__CardinalityAssignment_2 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13074:1: ( rule__ValueObjectEntityAttribute__CardinalityAssignment_2 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getValueObjectEntityAttributeAccess().getCardinalityAssignment_2());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13075:1: ( rule__ValueObjectEntityAttribute__CardinalityAssignment_2 )?
int alt100=2;
int LA100_0 = input.LA(1);
if ( ((LA100_0>=53 && LA100_0<=55)) ) {
alt100=1;
}
switch (alt100) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13075:2: rule__ValueObjectEntityAttribute__CardinalityAssignment_2
{
pushFollow(FollowSets001.FOLLOW_rule__ValueObjectEntityAttribute__CardinalityAssignment_2_in_rule__ValueObjectEntityAttribute__Group__2__Impl27226);
rule__ValueObjectEntityAttribute__CardinalityAssignment_2();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getValueObjectEntityAttributeAccess().getCardinalityAssignment_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ValueObjectEntityAttribute__Group__2__Impl"
// $ANTLR start "rule__ValueObjectEntityAttribute__Group__3"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13085:1: rule__ValueObjectEntityAttribute__Group__3 : rule__ValueObjectEntityAttribute__Group__3__Impl ;
public final void rule__ValueObjectEntityAttribute__Group__3() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13089:1: ( rule__ValueObjectEntityAttribute__Group__3__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13090:2: rule__ValueObjectEntityAttribute__Group__3__Impl
{
pushFollow(FollowSets001.FOLLOW_rule__ValueObjectEntityAttribute__Group__3__Impl_in_rule__ValueObjectEntityAttribute__Group__327257);
rule__ValueObjectEntityAttribute__Group__3__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ValueObjectEntityAttribute__Group__3"
// $ANTLR start "rule__ValueObjectEntityAttribute__Group__3__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13096:1: rule__ValueObjectEntityAttribute__Group__3__Impl : ( ( rule__ValueObjectEntityAttribute__NameAssignment_3 ) ) ;
public final void rule__ValueObjectEntityAttribute__Group__3__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13100:1: ( ( ( rule__ValueObjectEntityAttribute__NameAssignment_3 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13101:1: ( ( rule__ValueObjectEntityAttribute__NameAssignment_3 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13101:1: ( ( rule__ValueObjectEntityAttribute__NameAssignment_3 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13102:1: ( rule__ValueObjectEntityAttribute__NameAssignment_3 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getValueObjectEntityAttributeAccess().getNameAssignment_3());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13103:1: ( rule__ValueObjectEntityAttribute__NameAssignment_3 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13103:2: rule__ValueObjectEntityAttribute__NameAssignment_3
{
pushFollow(FollowSets001.FOLLOW_rule__ValueObjectEntityAttribute__NameAssignment_3_in_rule__ValueObjectEntityAttribute__Group__3__Impl27284);
rule__ValueObjectEntityAttribute__NameAssignment_3();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getValueObjectEntityAttributeAccess().getNameAssignment_3());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ValueObjectEntityAttribute__Group__3__Impl"
// $ANTLR start "rule__EnumerationDataType__Group__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13121:1: rule__EnumerationDataType__Group__0 : rule__EnumerationDataType__Group__0__Impl rule__EnumerationDataType__Group__1 ;
public final void rule__EnumerationDataType__Group__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13125:1: ( rule__EnumerationDataType__Group__0__Impl rule__EnumerationDataType__Group__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13126:2: rule__EnumerationDataType__Group__0__Impl rule__EnumerationDataType__Group__1
{
pushFollow(FollowSets001.FOLLOW_rule__EnumerationDataType__Group__0__Impl_in_rule__EnumerationDataType__Group__027322);
rule__EnumerationDataType__Group__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__EnumerationDataType__Group__1_in_rule__EnumerationDataType__Group__027325);
rule__EnumerationDataType__Group__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EnumerationDataType__Group__0"
// $ANTLR start "rule__EnumerationDataType__Group__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13133:1: rule__EnumerationDataType__Group__0__Impl : ( 'enumerationdatatype' ) ;
public final void rule__EnumerationDataType__Group__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13137:1: ( ( 'enumerationdatatype' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13138:1: ( 'enumerationdatatype' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13138:1: ( 'enumerationdatatype' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13139:1: 'enumerationdatatype'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEnumerationDataTypeAccess().getEnumerationdatatypeKeyword_0());
}
match(input,100,FollowSets001.FOLLOW_100_in_rule__EnumerationDataType__Group__0__Impl27353); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getEnumerationDataTypeAccess().getEnumerationdatatypeKeyword_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EnumerationDataType__Group__0__Impl"
// $ANTLR start "rule__EnumerationDataType__Group__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13152:1: rule__EnumerationDataType__Group__1 : rule__EnumerationDataType__Group__1__Impl rule__EnumerationDataType__Group__2 ;
public final void rule__EnumerationDataType__Group__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13156:1: ( rule__EnumerationDataType__Group__1__Impl rule__EnumerationDataType__Group__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13157:2: rule__EnumerationDataType__Group__1__Impl rule__EnumerationDataType__Group__2
{
pushFollow(FollowSets001.FOLLOW_rule__EnumerationDataType__Group__1__Impl_in_rule__EnumerationDataType__Group__127384);
rule__EnumerationDataType__Group__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__EnumerationDataType__Group__2_in_rule__EnumerationDataType__Group__127387);
rule__EnumerationDataType__Group__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EnumerationDataType__Group__1"
// $ANTLR start "rule__EnumerationDataType__Group__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13164:1: rule__EnumerationDataType__Group__1__Impl : ( ( rule__EnumerationDataType__NameAssignment_1 ) ) ;
public final void rule__EnumerationDataType__Group__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13168:1: ( ( ( rule__EnumerationDataType__NameAssignment_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13169:1: ( ( rule__EnumerationDataType__NameAssignment_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13169:1: ( ( rule__EnumerationDataType__NameAssignment_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13170:1: ( rule__EnumerationDataType__NameAssignment_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEnumerationDataTypeAccess().getNameAssignment_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13171:1: ( rule__EnumerationDataType__NameAssignment_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13171:2: rule__EnumerationDataType__NameAssignment_1
{
pushFollow(FollowSets001.FOLLOW_rule__EnumerationDataType__NameAssignment_1_in_rule__EnumerationDataType__Group__1__Impl27414);
rule__EnumerationDataType__NameAssignment_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getEnumerationDataTypeAccess().getNameAssignment_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EnumerationDataType__Group__1__Impl"
// $ANTLR start "rule__EnumerationDataType__Group__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13181:1: rule__EnumerationDataType__Group__2 : rule__EnumerationDataType__Group__2__Impl rule__EnumerationDataType__Group__3 ;
public final void rule__EnumerationDataType__Group__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13185:1: ( rule__EnumerationDataType__Group__2__Impl rule__EnumerationDataType__Group__3 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13186:2: rule__EnumerationDataType__Group__2__Impl rule__EnumerationDataType__Group__3
{
pushFollow(FollowSets001.FOLLOW_rule__EnumerationDataType__Group__2__Impl_in_rule__EnumerationDataType__Group__227444);
rule__EnumerationDataType__Group__2__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__EnumerationDataType__Group__3_in_rule__EnumerationDataType__Group__227447);
rule__EnumerationDataType__Group__3();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EnumerationDataType__Group__2"
// $ANTLR start "rule__EnumerationDataType__Group__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13193:1: rule__EnumerationDataType__Group__2__Impl : ( ( rule__EnumerationDataType__Group_2__0 )? ) ;
public final void rule__EnumerationDataType__Group__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13197:1: ( ( ( rule__EnumerationDataType__Group_2__0 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13198:1: ( ( rule__EnumerationDataType__Group_2__0 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13198:1: ( ( rule__EnumerationDataType__Group_2__0 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13199:1: ( rule__EnumerationDataType__Group_2__0 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEnumerationDataTypeAccess().getGroup_2());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13200:1: ( rule__EnumerationDataType__Group_2__0 )?
int alt101=2;
int LA101_0 = input.LA(1);
if ( (LA101_0==45) ) {
alt101=1;
}
switch (alt101) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13200:2: rule__EnumerationDataType__Group_2__0
{
pushFollow(FollowSets001.FOLLOW_rule__EnumerationDataType__Group_2__0_in_rule__EnumerationDataType__Group__2__Impl27474);
rule__EnumerationDataType__Group_2__0();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getEnumerationDataTypeAccess().getGroup_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EnumerationDataType__Group__2__Impl"
// $ANTLR start "rule__EnumerationDataType__Group__3"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13210:1: rule__EnumerationDataType__Group__3 : rule__EnumerationDataType__Group__3__Impl rule__EnumerationDataType__Group__4 ;
public final void rule__EnumerationDataType__Group__3() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13214:1: ( rule__EnumerationDataType__Group__3__Impl rule__EnumerationDataType__Group__4 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13215:2: rule__EnumerationDataType__Group__3__Impl rule__EnumerationDataType__Group__4
{
pushFollow(FollowSets001.FOLLOW_rule__EnumerationDataType__Group__3__Impl_in_rule__EnumerationDataType__Group__327505);
rule__EnumerationDataType__Group__3__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__EnumerationDataType__Group__4_in_rule__EnumerationDataType__Group__327508);
rule__EnumerationDataType__Group__4();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EnumerationDataType__Group__3"
// $ANTLR start "rule__EnumerationDataType__Group__3__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13222:1: rule__EnumerationDataType__Group__3__Impl : ( '{' ) ;
public final void rule__EnumerationDataType__Group__3__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13226:1: ( ( '{' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13227:1: ( '{' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13227:1: ( '{' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13228:1: '{'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEnumerationDataTypeAccess().getLeftCurlyBracketKeyword_3());
}
match(input,67,FollowSets001.FOLLOW_67_in_rule__EnumerationDataType__Group__3__Impl27536); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getEnumerationDataTypeAccess().getLeftCurlyBracketKeyword_3());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EnumerationDataType__Group__3__Impl"
// $ANTLR start "rule__EnumerationDataType__Group__4"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13241:1: rule__EnumerationDataType__Group__4 : rule__EnumerationDataType__Group__4__Impl rule__EnumerationDataType__Group__5 ;
public final void rule__EnumerationDataType__Group__4() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13245:1: ( rule__EnumerationDataType__Group__4__Impl rule__EnumerationDataType__Group__5 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13246:2: rule__EnumerationDataType__Group__4__Impl rule__EnumerationDataType__Group__5
{
pushFollow(FollowSets001.FOLLOW_rule__EnumerationDataType__Group__4__Impl_in_rule__EnumerationDataType__Group__427567);
rule__EnumerationDataType__Group__4__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__EnumerationDataType__Group__5_in_rule__EnumerationDataType__Group__427570);
rule__EnumerationDataType__Group__5();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EnumerationDataType__Group__4"
// $ANTLR start "rule__EnumerationDataType__Group__4__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13253:1: rule__EnumerationDataType__Group__4__Impl : ( ( rule__EnumerationDataType__BaseDataTypeAssignment_4 ) ) ;
public final void rule__EnumerationDataType__Group__4__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13257:1: ( ( ( rule__EnumerationDataType__BaseDataTypeAssignment_4 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13258:1: ( ( rule__EnumerationDataType__BaseDataTypeAssignment_4 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13258:1: ( ( rule__EnumerationDataType__BaseDataTypeAssignment_4 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13259:1: ( rule__EnumerationDataType__BaseDataTypeAssignment_4 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEnumerationDataTypeAccess().getBaseDataTypeAssignment_4());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13260:1: ( rule__EnumerationDataType__BaseDataTypeAssignment_4 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13260:2: rule__EnumerationDataType__BaseDataTypeAssignment_4
{
pushFollow(FollowSets001.FOLLOW_rule__EnumerationDataType__BaseDataTypeAssignment_4_in_rule__EnumerationDataType__Group__4__Impl27597);
rule__EnumerationDataType__BaseDataTypeAssignment_4();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getEnumerationDataTypeAccess().getBaseDataTypeAssignment_4());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EnumerationDataType__Group__4__Impl"
// $ANTLR start "rule__EnumerationDataType__Group__5"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13270:1: rule__EnumerationDataType__Group__5 : rule__EnumerationDataType__Group__5__Impl rule__EnumerationDataType__Group__6 ;
public final void rule__EnumerationDataType__Group__5() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13274:1: ( rule__EnumerationDataType__Group__5__Impl rule__EnumerationDataType__Group__6 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13275:2: rule__EnumerationDataType__Group__5__Impl rule__EnumerationDataType__Group__6
{
pushFollow(FollowSets001.FOLLOW_rule__EnumerationDataType__Group__5__Impl_in_rule__EnumerationDataType__Group__527627);
rule__EnumerationDataType__Group__5__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__EnumerationDataType__Group__6_in_rule__EnumerationDataType__Group__527630);
rule__EnumerationDataType__Group__6();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EnumerationDataType__Group__5"
// $ANTLR start "rule__EnumerationDataType__Group__5__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13282:1: rule__EnumerationDataType__Group__5__Impl : ( 'enumeration' ) ;
public final void rule__EnumerationDataType__Group__5__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13286:1: ( ( 'enumeration' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13287:1: ( 'enumeration' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13287:1: ( 'enumeration' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13288:1: 'enumeration'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEnumerationDataTypeAccess().getEnumerationKeyword_5());
}
match(input,70,FollowSets001.FOLLOW_70_in_rule__EnumerationDataType__Group__5__Impl27658); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getEnumerationDataTypeAccess().getEnumerationKeyword_5());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EnumerationDataType__Group__5__Impl"
// $ANTLR start "rule__EnumerationDataType__Group__6"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13301:1: rule__EnumerationDataType__Group__6 : rule__EnumerationDataType__Group__6__Impl rule__EnumerationDataType__Group__7 ;
public final void rule__EnumerationDataType__Group__6() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13305:1: ( rule__EnumerationDataType__Group__6__Impl rule__EnumerationDataType__Group__7 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13306:2: rule__EnumerationDataType__Group__6__Impl rule__EnumerationDataType__Group__7
{
pushFollow(FollowSets001.FOLLOW_rule__EnumerationDataType__Group__6__Impl_in_rule__EnumerationDataType__Group__627689);
rule__EnumerationDataType__Group__6__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__EnumerationDataType__Group__7_in_rule__EnumerationDataType__Group__627692);
rule__EnumerationDataType__Group__7();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EnumerationDataType__Group__6"
// $ANTLR start "rule__EnumerationDataType__Group__6__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13313:1: rule__EnumerationDataType__Group__6__Impl : ( ( rule__EnumerationDataType__EnumerationAssignment_6 ) ) ;
public final void rule__EnumerationDataType__Group__6__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13317:1: ( ( ( rule__EnumerationDataType__EnumerationAssignment_6 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13318:1: ( ( rule__EnumerationDataType__EnumerationAssignment_6 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13318:1: ( ( rule__EnumerationDataType__EnumerationAssignment_6 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13319:1: ( rule__EnumerationDataType__EnumerationAssignment_6 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEnumerationDataTypeAccess().getEnumerationAssignment_6());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13320:1: ( rule__EnumerationDataType__EnumerationAssignment_6 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13320:2: rule__EnumerationDataType__EnumerationAssignment_6
{
pushFollow(FollowSets001.FOLLOW_rule__EnumerationDataType__EnumerationAssignment_6_in_rule__EnumerationDataType__Group__6__Impl27719);
rule__EnumerationDataType__EnumerationAssignment_6();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getEnumerationDataTypeAccess().getEnumerationAssignment_6());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EnumerationDataType__Group__6__Impl"
// $ANTLR start "rule__EnumerationDataType__Group__7"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13330:1: rule__EnumerationDataType__Group__7 : rule__EnumerationDataType__Group__7__Impl ;
public final void rule__EnumerationDataType__Group__7() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13334:1: ( rule__EnumerationDataType__Group__7__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13335:2: rule__EnumerationDataType__Group__7__Impl
{
pushFollow(FollowSets001.FOLLOW_rule__EnumerationDataType__Group__7__Impl_in_rule__EnumerationDataType__Group__727749);
rule__EnumerationDataType__Group__7__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EnumerationDataType__Group__7"
// $ANTLR start "rule__EnumerationDataType__Group__7__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13341:1: rule__EnumerationDataType__Group__7__Impl : ( '}' ) ;
public final void rule__EnumerationDataType__Group__7__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13345:1: ( ( '}' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13346:1: ( '}' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13346:1: ( '}' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13347:1: '}'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEnumerationDataTypeAccess().getRightCurlyBracketKeyword_7());
}
match(input,68,FollowSets001.FOLLOW_68_in_rule__EnumerationDataType__Group__7__Impl27777); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getEnumerationDataTypeAccess().getRightCurlyBracketKeyword_7());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EnumerationDataType__Group__7__Impl"
// $ANTLR start "rule__EnumerationDataType__Group_2__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13376:1: rule__EnumerationDataType__Group_2__0 : rule__EnumerationDataType__Group_2__0__Impl rule__EnumerationDataType__Group_2__1 ;
public final void rule__EnumerationDataType__Group_2__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13380:1: ( rule__EnumerationDataType__Group_2__0__Impl rule__EnumerationDataType__Group_2__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13381:2: rule__EnumerationDataType__Group_2__0__Impl rule__EnumerationDataType__Group_2__1
{
pushFollow(FollowSets001.FOLLOW_rule__EnumerationDataType__Group_2__0__Impl_in_rule__EnumerationDataType__Group_2__027824);
rule__EnumerationDataType__Group_2__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__EnumerationDataType__Group_2__1_in_rule__EnumerationDataType__Group_2__027827);
rule__EnumerationDataType__Group_2__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EnumerationDataType__Group_2__0"
// $ANTLR start "rule__EnumerationDataType__Group_2__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13388:1: rule__EnumerationDataType__Group_2__0__Impl : ( 'extends' ) ;
public final void rule__EnumerationDataType__Group_2__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13392:1: ( ( 'extends' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13393:1: ( 'extends' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13393:1: ( 'extends' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13394:1: 'extends'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEnumerationDataTypeAccess().getExtendsKeyword_2_0());
}
match(input,45,FollowSets001.FOLLOW_45_in_rule__EnumerationDataType__Group_2__0__Impl27855); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getEnumerationDataTypeAccess().getExtendsKeyword_2_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EnumerationDataType__Group_2__0__Impl"
// $ANTLR start "rule__EnumerationDataType__Group_2__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13407:1: rule__EnumerationDataType__Group_2__1 : rule__EnumerationDataType__Group_2__1__Impl ;
public final void rule__EnumerationDataType__Group_2__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13411:1: ( rule__EnumerationDataType__Group_2__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13412:2: rule__EnumerationDataType__Group_2__1__Impl
{
pushFollow(FollowSets001.FOLLOW_rule__EnumerationDataType__Group_2__1__Impl_in_rule__EnumerationDataType__Group_2__127886);
rule__EnumerationDataType__Group_2__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EnumerationDataType__Group_2__1"
// $ANTLR start "rule__EnumerationDataType__Group_2__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13418:1: rule__EnumerationDataType__Group_2__1__Impl : ( ( rule__EnumerationDataType__RefAssignment_2_1 ) ) ;
public final void rule__EnumerationDataType__Group_2__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13422:1: ( ( ( rule__EnumerationDataType__RefAssignment_2_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13423:1: ( ( rule__EnumerationDataType__RefAssignment_2_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13423:1: ( ( rule__EnumerationDataType__RefAssignment_2_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13424:1: ( rule__EnumerationDataType__RefAssignment_2_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEnumerationDataTypeAccess().getRefAssignment_2_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13425:1: ( rule__EnumerationDataType__RefAssignment_2_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13425:2: rule__EnumerationDataType__RefAssignment_2_1
{
pushFollow(FollowSets001.FOLLOW_rule__EnumerationDataType__RefAssignment_2_1_in_rule__EnumerationDataType__Group_2__1__Impl27913);
rule__EnumerationDataType__RefAssignment_2_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getEnumerationDataTypeAccess().getRefAssignment_2_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EnumerationDataType__Group_2__1__Impl"
// $ANTLR start "rule__EnumerationEntityAttribute__Group__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13439:1: rule__EnumerationEntityAttribute__Group__0 : rule__EnumerationEntityAttribute__Group__0__Impl rule__EnumerationEntityAttribute__Group__1 ;
public final void rule__EnumerationEntityAttribute__Group__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13443:1: ( rule__EnumerationEntityAttribute__Group__0__Impl rule__EnumerationEntityAttribute__Group__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13444:2: rule__EnumerationEntityAttribute__Group__0__Impl rule__EnumerationEntityAttribute__Group__1
{
pushFollow(FollowSets001.FOLLOW_rule__EnumerationEntityAttribute__Group__0__Impl_in_rule__EnumerationEntityAttribute__Group__027947);
rule__EnumerationEntityAttribute__Group__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__EnumerationEntityAttribute__Group__1_in_rule__EnumerationEntityAttribute__Group__027950);
rule__EnumerationEntityAttribute__Group__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EnumerationEntityAttribute__Group__0"
// $ANTLR start "rule__EnumerationEntityAttribute__Group__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13451:1: rule__EnumerationEntityAttribute__Group__0__Impl : ( 'enumeration' ) ;
public final void rule__EnumerationEntityAttribute__Group__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13455:1: ( ( 'enumeration' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13456:1: ( 'enumeration' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13456:1: ( 'enumeration' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13457:1: 'enumeration'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEnumerationEntityAttributeAccess().getEnumerationKeyword_0());
}
match(input,70,FollowSets001.FOLLOW_70_in_rule__EnumerationEntityAttribute__Group__0__Impl27978); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getEnumerationEntityAttributeAccess().getEnumerationKeyword_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EnumerationEntityAttribute__Group__0__Impl"
// $ANTLR start "rule__EnumerationEntityAttribute__Group__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13470:1: rule__EnumerationEntityAttribute__Group__1 : rule__EnumerationEntityAttribute__Group__1__Impl rule__EnumerationEntityAttribute__Group__2 ;
public final void rule__EnumerationEntityAttribute__Group__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13474:1: ( rule__EnumerationEntityAttribute__Group__1__Impl rule__EnumerationEntityAttribute__Group__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13475:2: rule__EnumerationEntityAttribute__Group__1__Impl rule__EnumerationEntityAttribute__Group__2
{
pushFollow(FollowSets001.FOLLOW_rule__EnumerationEntityAttribute__Group__1__Impl_in_rule__EnumerationEntityAttribute__Group__128009);
rule__EnumerationEntityAttribute__Group__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__EnumerationEntityAttribute__Group__2_in_rule__EnumerationEntityAttribute__Group__128012);
rule__EnumerationEntityAttribute__Group__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EnumerationEntityAttribute__Group__1"
// $ANTLR start "rule__EnumerationEntityAttribute__Group__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13482:1: rule__EnumerationEntityAttribute__Group__1__Impl : ( ( rule__EnumerationEntityAttribute__TypeAssignment_1 ) ) ;
public final void rule__EnumerationEntityAttribute__Group__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13486:1: ( ( ( rule__EnumerationEntityAttribute__TypeAssignment_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13487:1: ( ( rule__EnumerationEntityAttribute__TypeAssignment_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13487:1: ( ( rule__EnumerationEntityAttribute__TypeAssignment_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13488:1: ( rule__EnumerationEntityAttribute__TypeAssignment_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEnumerationEntityAttributeAccess().getTypeAssignment_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13489:1: ( rule__EnumerationEntityAttribute__TypeAssignment_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13489:2: rule__EnumerationEntityAttribute__TypeAssignment_1
{
pushFollow(FollowSets001.FOLLOW_rule__EnumerationEntityAttribute__TypeAssignment_1_in_rule__EnumerationEntityAttribute__Group__1__Impl28039);
rule__EnumerationEntityAttribute__TypeAssignment_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getEnumerationEntityAttributeAccess().getTypeAssignment_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EnumerationEntityAttribute__Group__1__Impl"
// $ANTLR start "rule__EnumerationEntityAttribute__Group__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13499:1: rule__EnumerationEntityAttribute__Group__2 : rule__EnumerationEntityAttribute__Group__2__Impl rule__EnumerationEntityAttribute__Group__3 ;
public final void rule__EnumerationEntityAttribute__Group__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13503:1: ( rule__EnumerationEntityAttribute__Group__2__Impl rule__EnumerationEntityAttribute__Group__3 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13504:2: rule__EnumerationEntityAttribute__Group__2__Impl rule__EnumerationEntityAttribute__Group__3
{
pushFollow(FollowSets001.FOLLOW_rule__EnumerationEntityAttribute__Group__2__Impl_in_rule__EnumerationEntityAttribute__Group__228069);
rule__EnumerationEntityAttribute__Group__2__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__EnumerationEntityAttribute__Group__3_in_rule__EnumerationEntityAttribute__Group__228072);
rule__EnumerationEntityAttribute__Group__3();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EnumerationEntityAttribute__Group__2"
// $ANTLR start "rule__EnumerationEntityAttribute__Group__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13511:1: rule__EnumerationEntityAttribute__Group__2__Impl : ( ( rule__EnumerationEntityAttribute__CardinalityAssignment_2 )? ) ;
public final void rule__EnumerationEntityAttribute__Group__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13515:1: ( ( ( rule__EnumerationEntityAttribute__CardinalityAssignment_2 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13516:1: ( ( rule__EnumerationEntityAttribute__CardinalityAssignment_2 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13516:1: ( ( rule__EnumerationEntityAttribute__CardinalityAssignment_2 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13517:1: ( rule__EnumerationEntityAttribute__CardinalityAssignment_2 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEnumerationEntityAttributeAccess().getCardinalityAssignment_2());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13518:1: ( rule__EnumerationEntityAttribute__CardinalityAssignment_2 )?
int alt102=2;
int LA102_0 = input.LA(1);
if ( ((LA102_0>=53 && LA102_0<=55)) ) {
alt102=1;
}
switch (alt102) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13518:2: rule__EnumerationEntityAttribute__CardinalityAssignment_2
{
pushFollow(FollowSets001.FOLLOW_rule__EnumerationEntityAttribute__CardinalityAssignment_2_in_rule__EnumerationEntityAttribute__Group__2__Impl28099);
rule__EnumerationEntityAttribute__CardinalityAssignment_2();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getEnumerationEntityAttributeAccess().getCardinalityAssignment_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EnumerationEntityAttribute__Group__2__Impl"
// $ANTLR start "rule__EnumerationEntityAttribute__Group__3"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13528:1: rule__EnumerationEntityAttribute__Group__3 : rule__EnumerationEntityAttribute__Group__3__Impl ;
public final void rule__EnumerationEntityAttribute__Group__3() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13532:1: ( rule__EnumerationEntityAttribute__Group__3__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13533:2: rule__EnumerationEntityAttribute__Group__3__Impl
{
pushFollow(FollowSets001.FOLLOW_rule__EnumerationEntityAttribute__Group__3__Impl_in_rule__EnumerationEntityAttribute__Group__328130);
rule__EnumerationEntityAttribute__Group__3__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EnumerationEntityAttribute__Group__3"
// $ANTLR start "rule__EnumerationEntityAttribute__Group__3__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13539:1: rule__EnumerationEntityAttribute__Group__3__Impl : ( ( rule__EnumerationEntityAttribute__NameAssignment_3 ) ) ;
public final void rule__EnumerationEntityAttribute__Group__3__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13543:1: ( ( ( rule__EnumerationEntityAttribute__NameAssignment_3 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13544:1: ( ( rule__EnumerationEntityAttribute__NameAssignment_3 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13544:1: ( ( rule__EnumerationEntityAttribute__NameAssignment_3 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13545:1: ( rule__EnumerationEntityAttribute__NameAssignment_3 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEnumerationEntityAttributeAccess().getNameAssignment_3());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13546:1: ( rule__EnumerationEntityAttribute__NameAssignment_3 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13546:2: rule__EnumerationEntityAttribute__NameAssignment_3
{
pushFollow(FollowSets001.FOLLOW_rule__EnumerationEntityAttribute__NameAssignment_3_in_rule__EnumerationEntityAttribute__Group__3__Impl28157);
rule__EnumerationEntityAttribute__NameAssignment_3();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getEnumerationEntityAttributeAccess().getNameAssignment_3());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EnumerationEntityAttribute__Group__3__Impl"
// $ANTLR start "rule__Dictionary__Group__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13564:1: rule__Dictionary__Group__0 : rule__Dictionary__Group__0__Impl rule__Dictionary__Group__1 ;
public final void rule__Dictionary__Group__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13568:1: ( rule__Dictionary__Group__0__Impl rule__Dictionary__Group__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13569:2: rule__Dictionary__Group__0__Impl rule__Dictionary__Group__1
{
pushFollow(FollowSets001.FOLLOW_rule__Dictionary__Group__0__Impl_in_rule__Dictionary__Group__028195);
rule__Dictionary__Group__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__Dictionary__Group__1_in_rule__Dictionary__Group__028198);
rule__Dictionary__Group__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Dictionary__Group__0"
// $ANTLR start "rule__Dictionary__Group__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13576:1: rule__Dictionary__Group__0__Impl : ( 'dictionary' ) ;
public final void rule__Dictionary__Group__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13580:1: ( ( 'dictionary' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13581:1: ( 'dictionary' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13581:1: ( 'dictionary' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13582:1: 'dictionary'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryAccess().getDictionaryKeyword_0());
}
match(input,101,FollowSets001.FOLLOW_101_in_rule__Dictionary__Group__0__Impl28226); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryAccess().getDictionaryKeyword_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Dictionary__Group__0__Impl"
// $ANTLR start "rule__Dictionary__Group__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13595:1: rule__Dictionary__Group__1 : rule__Dictionary__Group__1__Impl rule__Dictionary__Group__2 ;
public final void rule__Dictionary__Group__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13599:1: ( rule__Dictionary__Group__1__Impl rule__Dictionary__Group__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13600:2: rule__Dictionary__Group__1__Impl rule__Dictionary__Group__2
{
pushFollow(FollowSets001.FOLLOW_rule__Dictionary__Group__1__Impl_in_rule__Dictionary__Group__128257);
rule__Dictionary__Group__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__Dictionary__Group__2_in_rule__Dictionary__Group__128260);
rule__Dictionary__Group__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Dictionary__Group__1"
// $ANTLR start "rule__Dictionary__Group__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13607:1: rule__Dictionary__Group__1__Impl : ( ( rule__Dictionary__NameAssignment_1 ) ) ;
public final void rule__Dictionary__Group__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13611:1: ( ( ( rule__Dictionary__NameAssignment_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13612:1: ( ( rule__Dictionary__NameAssignment_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13612:1: ( ( rule__Dictionary__NameAssignment_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13613:1: ( rule__Dictionary__NameAssignment_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryAccess().getNameAssignment_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13614:1: ( rule__Dictionary__NameAssignment_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13614:2: rule__Dictionary__NameAssignment_1
{
pushFollow(FollowSets001.FOLLOW_rule__Dictionary__NameAssignment_1_in_rule__Dictionary__Group__1__Impl28287);
rule__Dictionary__NameAssignment_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryAccess().getNameAssignment_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Dictionary__Group__1__Impl"
// $ANTLR start "rule__Dictionary__Group__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13624:1: rule__Dictionary__Group__2 : rule__Dictionary__Group__2__Impl rule__Dictionary__Group__3 ;
public final void rule__Dictionary__Group__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13628:1: ( rule__Dictionary__Group__2__Impl rule__Dictionary__Group__3 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13629:2: rule__Dictionary__Group__2__Impl rule__Dictionary__Group__3
{
pushFollow(FollowSets001.FOLLOW_rule__Dictionary__Group__2__Impl_in_rule__Dictionary__Group__228317);
rule__Dictionary__Group__2__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__Dictionary__Group__3_in_rule__Dictionary__Group__228320);
rule__Dictionary__Group__3();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Dictionary__Group__2"
// $ANTLR start "rule__Dictionary__Group__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13636:1: rule__Dictionary__Group__2__Impl : ( '{' ) ;
public final void rule__Dictionary__Group__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13640:1: ( ( '{' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13641:1: ( '{' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13641:1: ( '{' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13642:1: '{'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryAccess().getLeftCurlyBracketKeyword_2());
}
match(input,67,FollowSets001.FOLLOW_67_in_rule__Dictionary__Group__2__Impl28348); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryAccess().getLeftCurlyBracketKeyword_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Dictionary__Group__2__Impl"
// $ANTLR start "rule__Dictionary__Group__3"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13655:1: rule__Dictionary__Group__3 : rule__Dictionary__Group__3__Impl rule__Dictionary__Group__4 ;
public final void rule__Dictionary__Group__3() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13659:1: ( rule__Dictionary__Group__3__Impl rule__Dictionary__Group__4 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13660:2: rule__Dictionary__Group__3__Impl rule__Dictionary__Group__4
{
pushFollow(FollowSets001.FOLLOW_rule__Dictionary__Group__3__Impl_in_rule__Dictionary__Group__328379);
rule__Dictionary__Group__3__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__Dictionary__Group__4_in_rule__Dictionary__Group__328382);
rule__Dictionary__Group__4();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Dictionary__Group__3"
// $ANTLR start "rule__Dictionary__Group__3__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13667:1: rule__Dictionary__Group__3__Impl : ( 'entity' ) ;
public final void rule__Dictionary__Group__3__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13671:1: ( ( 'entity' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13672:1: ( 'entity' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13672:1: ( 'entity' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13673:1: 'entity'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryAccess().getEntityKeyword_3());
}
match(input,78,FollowSets001.FOLLOW_78_in_rule__Dictionary__Group__3__Impl28410); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryAccess().getEntityKeyword_3());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Dictionary__Group__3__Impl"
// $ANTLR start "rule__Dictionary__Group__4"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13686:1: rule__Dictionary__Group__4 : rule__Dictionary__Group__4__Impl rule__Dictionary__Group__5 ;
public final void rule__Dictionary__Group__4() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13690:1: ( rule__Dictionary__Group__4__Impl rule__Dictionary__Group__5 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13691:2: rule__Dictionary__Group__4__Impl rule__Dictionary__Group__5
{
pushFollow(FollowSets001.FOLLOW_rule__Dictionary__Group__4__Impl_in_rule__Dictionary__Group__428441);
rule__Dictionary__Group__4__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__Dictionary__Group__5_in_rule__Dictionary__Group__428444);
rule__Dictionary__Group__5();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Dictionary__Group__4"
// $ANTLR start "rule__Dictionary__Group__4__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13698:1: rule__Dictionary__Group__4__Impl : ( ( rule__Dictionary__EntityAssignment_4 ) ) ;
public final void rule__Dictionary__Group__4__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13702:1: ( ( ( rule__Dictionary__EntityAssignment_4 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13703:1: ( ( rule__Dictionary__EntityAssignment_4 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13703:1: ( ( rule__Dictionary__EntityAssignment_4 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13704:1: ( rule__Dictionary__EntityAssignment_4 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryAccess().getEntityAssignment_4());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13705:1: ( rule__Dictionary__EntityAssignment_4 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13705:2: rule__Dictionary__EntityAssignment_4
{
pushFollow(FollowSets001.FOLLOW_rule__Dictionary__EntityAssignment_4_in_rule__Dictionary__Group__4__Impl28471);
rule__Dictionary__EntityAssignment_4();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryAccess().getEntityAssignment_4());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Dictionary__Group__4__Impl"
// $ANTLR start "rule__Dictionary__Group__5"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13715:1: rule__Dictionary__Group__5 : rule__Dictionary__Group__5__Impl rule__Dictionary__Group__6 ;
public final void rule__Dictionary__Group__5() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13719:1: ( rule__Dictionary__Group__5__Impl rule__Dictionary__Group__6 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13720:2: rule__Dictionary__Group__5__Impl rule__Dictionary__Group__6
{
pushFollow(FollowSets001.FOLLOW_rule__Dictionary__Group__5__Impl_in_rule__Dictionary__Group__528501);
rule__Dictionary__Group__5__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__Dictionary__Group__6_in_rule__Dictionary__Group__528504);
rule__Dictionary__Group__6();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Dictionary__Group__5"
// $ANTLR start "rule__Dictionary__Group__5__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13727:1: rule__Dictionary__Group__5__Impl : ( ( rule__Dictionary__Group_5__0 )? ) ;
public final void rule__Dictionary__Group__5__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13731:1: ( ( ( rule__Dictionary__Group_5__0 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13732:1: ( ( rule__Dictionary__Group_5__0 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13732:1: ( ( rule__Dictionary__Group_5__0 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13733:1: ( rule__Dictionary__Group_5__0 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryAccess().getGroup_5());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13734:1: ( rule__Dictionary__Group_5__0 )?
int alt103=2;
int LA103_0 = input.LA(1);
if ( (LA103_0==75) ) {
alt103=1;
}
switch (alt103) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13734:2: rule__Dictionary__Group_5__0
{
pushFollow(FollowSets001.FOLLOW_rule__Dictionary__Group_5__0_in_rule__Dictionary__Group__5__Impl28531);
rule__Dictionary__Group_5__0();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryAccess().getGroup_5());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Dictionary__Group__5__Impl"
// $ANTLR start "rule__Dictionary__Group__6"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13744:1: rule__Dictionary__Group__6 : rule__Dictionary__Group__6__Impl rule__Dictionary__Group__7 ;
public final void rule__Dictionary__Group__6() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13748:1: ( rule__Dictionary__Group__6__Impl rule__Dictionary__Group__7 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13749:2: rule__Dictionary__Group__6__Impl rule__Dictionary__Group__7
{
pushFollow(FollowSets001.FOLLOW_rule__Dictionary__Group__6__Impl_in_rule__Dictionary__Group__628562);
rule__Dictionary__Group__6__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__Dictionary__Group__7_in_rule__Dictionary__Group__628565);
rule__Dictionary__Group__7();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Dictionary__Group__6"
// $ANTLR start "rule__Dictionary__Group__6__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13756:1: rule__Dictionary__Group__6__Impl : ( ( rule__Dictionary__Group_6__0 )? ) ;
public final void rule__Dictionary__Group__6__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13760:1: ( ( ( rule__Dictionary__Group_6__0 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13761:1: ( ( rule__Dictionary__Group_6__0 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13761:1: ( ( rule__Dictionary__Group_6__0 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13762:1: ( rule__Dictionary__Group_6__0 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryAccess().getGroup_6());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13763:1: ( rule__Dictionary__Group_6__0 )?
int alt104=2;
int LA104_0 = input.LA(1);
if ( (LA104_0==76) ) {
alt104=1;
}
switch (alt104) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13763:2: rule__Dictionary__Group_6__0
{
pushFollow(FollowSets001.FOLLOW_rule__Dictionary__Group_6__0_in_rule__Dictionary__Group__6__Impl28592);
rule__Dictionary__Group_6__0();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryAccess().getGroup_6());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Dictionary__Group__6__Impl"
// $ANTLR start "rule__Dictionary__Group__7"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13773:1: rule__Dictionary__Group__7 : rule__Dictionary__Group__7__Impl rule__Dictionary__Group__8 ;
public final void rule__Dictionary__Group__7() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13777:1: ( rule__Dictionary__Group__7__Impl rule__Dictionary__Group__8 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13778:2: rule__Dictionary__Group__7__Impl rule__Dictionary__Group__8
{
pushFollow(FollowSets001.FOLLOW_rule__Dictionary__Group__7__Impl_in_rule__Dictionary__Group__728623);
rule__Dictionary__Group__7__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__Dictionary__Group__8_in_rule__Dictionary__Group__728626);
rule__Dictionary__Group__8();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Dictionary__Group__7"
// $ANTLR start "rule__Dictionary__Group__7__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13785:1: rule__Dictionary__Group__7__Impl : ( ( rule__Dictionary__Group_7__0 )? ) ;
public final void rule__Dictionary__Group__7__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13789:1: ( ( ( rule__Dictionary__Group_7__0 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13790:1: ( ( rule__Dictionary__Group_7__0 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13790:1: ( ( rule__Dictionary__Group_7__0 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13791:1: ( rule__Dictionary__Group_7__0 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryAccess().getGroup_7());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13792:1: ( rule__Dictionary__Group_7__0 )?
int alt105=2;
int LA105_0 = input.LA(1);
if ( (LA105_0==102) ) {
alt105=1;
}
switch (alt105) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13792:2: rule__Dictionary__Group_7__0
{
pushFollow(FollowSets001.FOLLOW_rule__Dictionary__Group_7__0_in_rule__Dictionary__Group__7__Impl28653);
rule__Dictionary__Group_7__0();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryAccess().getGroup_7());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Dictionary__Group__7__Impl"
// $ANTLR start "rule__Dictionary__Group__8"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13802:1: rule__Dictionary__Group__8 : rule__Dictionary__Group__8__Impl rule__Dictionary__Group__9 ;
public final void rule__Dictionary__Group__8() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13806:1: ( rule__Dictionary__Group__8__Impl rule__Dictionary__Group__9 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13807:2: rule__Dictionary__Group__8__Impl rule__Dictionary__Group__9
{
pushFollow(FollowSets001.FOLLOW_rule__Dictionary__Group__8__Impl_in_rule__Dictionary__Group__828684);
rule__Dictionary__Group__8__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__Dictionary__Group__9_in_rule__Dictionary__Group__828687);
rule__Dictionary__Group__9();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Dictionary__Group__8"
// $ANTLR start "rule__Dictionary__Group__8__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13814:1: rule__Dictionary__Group__8__Impl : ( ( rule__Dictionary__Group_8__0 )? ) ;
public final void rule__Dictionary__Group__8__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13818:1: ( ( ( rule__Dictionary__Group_8__0 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13819:1: ( ( rule__Dictionary__Group_8__0 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13819:1: ( ( rule__Dictionary__Group_8__0 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13820:1: ( rule__Dictionary__Group_8__0 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryAccess().getGroup_8());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13821:1: ( rule__Dictionary__Group_8__0 )?
int alt106=2;
int LA106_0 = input.LA(1);
if ( (LA106_0==103) ) {
alt106=1;
}
switch (alt106) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13821:2: rule__Dictionary__Group_8__0
{
pushFollow(FollowSets001.FOLLOW_rule__Dictionary__Group_8__0_in_rule__Dictionary__Group__8__Impl28714);
rule__Dictionary__Group_8__0();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryAccess().getGroup_8());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Dictionary__Group__8__Impl"
// $ANTLR start "rule__Dictionary__Group__9"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13831:1: rule__Dictionary__Group__9 : rule__Dictionary__Group__9__Impl rule__Dictionary__Group__10 ;
public final void rule__Dictionary__Group__9() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13835:1: ( rule__Dictionary__Group__9__Impl rule__Dictionary__Group__10 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13836:2: rule__Dictionary__Group__9__Impl rule__Dictionary__Group__10
{
pushFollow(FollowSets001.FOLLOW_rule__Dictionary__Group__9__Impl_in_rule__Dictionary__Group__928745);
rule__Dictionary__Group__9__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__Dictionary__Group__10_in_rule__Dictionary__Group__928748);
rule__Dictionary__Group__10();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Dictionary__Group__9"
// $ANTLR start "rule__Dictionary__Group__9__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13843:1: rule__Dictionary__Group__9__Impl : ( ( rule__Dictionary__DictionarysearchAssignment_9 )? ) ;
public final void rule__Dictionary__Group__9__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13847:1: ( ( ( rule__Dictionary__DictionarysearchAssignment_9 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13848:1: ( ( rule__Dictionary__DictionarysearchAssignment_9 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13848:1: ( ( rule__Dictionary__DictionarysearchAssignment_9 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13849:1: ( rule__Dictionary__DictionarysearchAssignment_9 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryAccess().getDictionarysearchAssignment_9());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13850:1: ( rule__Dictionary__DictionarysearchAssignment_9 )?
int alt107=2;
int LA107_0 = input.LA(1);
if ( (LA107_0==104) ) {
alt107=1;
}
switch (alt107) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13850:2: rule__Dictionary__DictionarysearchAssignment_9
{
pushFollow(FollowSets001.FOLLOW_rule__Dictionary__DictionarysearchAssignment_9_in_rule__Dictionary__Group__9__Impl28775);
rule__Dictionary__DictionarysearchAssignment_9();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryAccess().getDictionarysearchAssignment_9());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Dictionary__Group__9__Impl"
// $ANTLR start "rule__Dictionary__Group__10"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13860:1: rule__Dictionary__Group__10 : rule__Dictionary__Group__10__Impl rule__Dictionary__Group__11 ;
public final void rule__Dictionary__Group__10() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13864:1: ( rule__Dictionary__Group__10__Impl rule__Dictionary__Group__11 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13865:2: rule__Dictionary__Group__10__Impl rule__Dictionary__Group__11
{
pushFollow(FollowSets001.FOLLOW_rule__Dictionary__Group__10__Impl_in_rule__Dictionary__Group__1028806);
rule__Dictionary__Group__10__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__Dictionary__Group__11_in_rule__Dictionary__Group__1028809);
rule__Dictionary__Group__11();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Dictionary__Group__10"
// $ANTLR start "rule__Dictionary__Group__10__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13872:1: rule__Dictionary__Group__10__Impl : ( ( rule__Dictionary__DictionaryeditorAssignment_10 )? ) ;
public final void rule__Dictionary__Group__10__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13876:1: ( ( ( rule__Dictionary__DictionaryeditorAssignment_10 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13877:1: ( ( rule__Dictionary__DictionaryeditorAssignment_10 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13877:1: ( ( rule__Dictionary__DictionaryeditorAssignment_10 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13878:1: ( rule__Dictionary__DictionaryeditorAssignment_10 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryAccess().getDictionaryeditorAssignment_10());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13879:1: ( rule__Dictionary__DictionaryeditorAssignment_10 )?
int alt108=2;
int LA108_0 = input.LA(1);
if ( (LA108_0==105) ) {
alt108=1;
}
switch (alt108) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13879:2: rule__Dictionary__DictionaryeditorAssignment_10
{
pushFollow(FollowSets001.FOLLOW_rule__Dictionary__DictionaryeditorAssignment_10_in_rule__Dictionary__Group__10__Impl28836);
rule__Dictionary__DictionaryeditorAssignment_10();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryAccess().getDictionaryeditorAssignment_10());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Dictionary__Group__10__Impl"
// $ANTLR start "rule__Dictionary__Group__11"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13889:1: rule__Dictionary__Group__11 : rule__Dictionary__Group__11__Impl ;
public final void rule__Dictionary__Group__11() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13893:1: ( rule__Dictionary__Group__11__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13894:2: rule__Dictionary__Group__11__Impl
{
pushFollow(FollowSets001.FOLLOW_rule__Dictionary__Group__11__Impl_in_rule__Dictionary__Group__1128867);
rule__Dictionary__Group__11__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Dictionary__Group__11"
// $ANTLR start "rule__Dictionary__Group__11__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13900:1: rule__Dictionary__Group__11__Impl : ( '}' ) ;
public final void rule__Dictionary__Group__11__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13904:1: ( ( '}' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13905:1: ( '}' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13905:1: ( '}' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13906:1: '}'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryAccess().getRightCurlyBracketKeyword_11());
}
match(input,68,FollowSets001.FOLLOW_68_in_rule__Dictionary__Group__11__Impl28895); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryAccess().getRightCurlyBracketKeyword_11());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Dictionary__Group__11__Impl"
// $ANTLR start "rule__Dictionary__Group_5__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13943:1: rule__Dictionary__Group_5__0 : rule__Dictionary__Group_5__0__Impl rule__Dictionary__Group_5__1 ;
public final void rule__Dictionary__Group_5__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13947:1: ( rule__Dictionary__Group_5__0__Impl rule__Dictionary__Group_5__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13948:2: rule__Dictionary__Group_5__0__Impl rule__Dictionary__Group_5__1
{
pushFollow(FollowSets001.FOLLOW_rule__Dictionary__Group_5__0__Impl_in_rule__Dictionary__Group_5__028950);
rule__Dictionary__Group_5__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__Dictionary__Group_5__1_in_rule__Dictionary__Group_5__028953);
rule__Dictionary__Group_5__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Dictionary__Group_5__0"
// $ANTLR start "rule__Dictionary__Group_5__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13955:1: rule__Dictionary__Group_5__0__Impl : ( 'label' ) ;
public final void rule__Dictionary__Group_5__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13959:1: ( ( 'label' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13960:1: ( 'label' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13960:1: ( 'label' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13961:1: 'label'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryAccess().getLabelKeyword_5_0());
}
match(input,75,FollowSets001.FOLLOW_75_in_rule__Dictionary__Group_5__0__Impl28981); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryAccess().getLabelKeyword_5_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Dictionary__Group_5__0__Impl"
// $ANTLR start "rule__Dictionary__Group_5__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13974:1: rule__Dictionary__Group_5__1 : rule__Dictionary__Group_5__1__Impl ;
public final void rule__Dictionary__Group_5__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13978:1: ( rule__Dictionary__Group_5__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13979:2: rule__Dictionary__Group_5__1__Impl
{
pushFollow(FollowSets001.FOLLOW_rule__Dictionary__Group_5__1__Impl_in_rule__Dictionary__Group_5__129012);
rule__Dictionary__Group_5__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Dictionary__Group_5__1"
// $ANTLR start "rule__Dictionary__Group_5__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13985:1: rule__Dictionary__Group_5__1__Impl : ( ( rule__Dictionary__LabelAssignment_5_1 ) ) ;
public final void rule__Dictionary__Group_5__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13989:1: ( ( ( rule__Dictionary__LabelAssignment_5_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13990:1: ( ( rule__Dictionary__LabelAssignment_5_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13990:1: ( ( rule__Dictionary__LabelAssignment_5_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13991:1: ( rule__Dictionary__LabelAssignment_5_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryAccess().getLabelAssignment_5_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13992:1: ( rule__Dictionary__LabelAssignment_5_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:13992:2: rule__Dictionary__LabelAssignment_5_1
{
pushFollow(FollowSets001.FOLLOW_rule__Dictionary__LabelAssignment_5_1_in_rule__Dictionary__Group_5__1__Impl29039);
rule__Dictionary__LabelAssignment_5_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryAccess().getLabelAssignment_5_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Dictionary__Group_5__1__Impl"
// $ANTLR start "rule__Dictionary__Group_6__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14006:1: rule__Dictionary__Group_6__0 : rule__Dictionary__Group_6__0__Impl rule__Dictionary__Group_6__1 ;
public final void rule__Dictionary__Group_6__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14010:1: ( rule__Dictionary__Group_6__0__Impl rule__Dictionary__Group_6__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14011:2: rule__Dictionary__Group_6__0__Impl rule__Dictionary__Group_6__1
{
pushFollow(FollowSets001.FOLLOW_rule__Dictionary__Group_6__0__Impl_in_rule__Dictionary__Group_6__029073);
rule__Dictionary__Group_6__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__Dictionary__Group_6__1_in_rule__Dictionary__Group_6__029076);
rule__Dictionary__Group_6__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Dictionary__Group_6__0"
// $ANTLR start "rule__Dictionary__Group_6__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14018:1: rule__Dictionary__Group_6__0__Impl : ( 'pluralLabel' ) ;
public final void rule__Dictionary__Group_6__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14022:1: ( ( 'pluralLabel' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14023:1: ( 'pluralLabel' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14023:1: ( 'pluralLabel' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14024:1: 'pluralLabel'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryAccess().getPluralLabelKeyword_6_0());
}
match(input,76,FollowSets001.FOLLOW_76_in_rule__Dictionary__Group_6__0__Impl29104); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryAccess().getPluralLabelKeyword_6_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Dictionary__Group_6__0__Impl"
// $ANTLR start "rule__Dictionary__Group_6__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14037:1: rule__Dictionary__Group_6__1 : rule__Dictionary__Group_6__1__Impl ;
public final void rule__Dictionary__Group_6__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14041:1: ( rule__Dictionary__Group_6__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14042:2: rule__Dictionary__Group_6__1__Impl
{
pushFollow(FollowSets001.FOLLOW_rule__Dictionary__Group_6__1__Impl_in_rule__Dictionary__Group_6__129135);
rule__Dictionary__Group_6__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Dictionary__Group_6__1"
// $ANTLR start "rule__Dictionary__Group_6__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14048:1: rule__Dictionary__Group_6__1__Impl : ( ( rule__Dictionary__PluralLabelAssignment_6_1 ) ) ;
public final void rule__Dictionary__Group_6__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14052:1: ( ( ( rule__Dictionary__PluralLabelAssignment_6_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14053:1: ( ( rule__Dictionary__PluralLabelAssignment_6_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14053:1: ( ( rule__Dictionary__PluralLabelAssignment_6_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14054:1: ( rule__Dictionary__PluralLabelAssignment_6_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryAccess().getPluralLabelAssignment_6_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14055:1: ( rule__Dictionary__PluralLabelAssignment_6_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14055:2: rule__Dictionary__PluralLabelAssignment_6_1
{
pushFollow(FollowSets001.FOLLOW_rule__Dictionary__PluralLabelAssignment_6_1_in_rule__Dictionary__Group_6__1__Impl29162);
rule__Dictionary__PluralLabelAssignment_6_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryAccess().getPluralLabelAssignment_6_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Dictionary__Group_6__1__Impl"
// $ANTLR start "rule__Dictionary__Group_7__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14069:1: rule__Dictionary__Group_7__0 : rule__Dictionary__Group_7__0__Impl rule__Dictionary__Group_7__1 ;
public final void rule__Dictionary__Group_7__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14073:1: ( rule__Dictionary__Group_7__0__Impl rule__Dictionary__Group_7__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14074:2: rule__Dictionary__Group_7__0__Impl rule__Dictionary__Group_7__1
{
pushFollow(FollowSets001.FOLLOW_rule__Dictionary__Group_7__0__Impl_in_rule__Dictionary__Group_7__029196);
rule__Dictionary__Group_7__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__Dictionary__Group_7__1_in_rule__Dictionary__Group_7__029199);
rule__Dictionary__Group_7__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Dictionary__Group_7__0"
// $ANTLR start "rule__Dictionary__Group_7__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14081:1: rule__Dictionary__Group_7__0__Impl : ( 'dictionarycontrols' ) ;
public final void rule__Dictionary__Group_7__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14085:1: ( ( 'dictionarycontrols' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14086:1: ( 'dictionarycontrols' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14086:1: ( 'dictionarycontrols' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14087:1: 'dictionarycontrols'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryAccess().getDictionarycontrolsKeyword_7_0());
}
match(input,102,FollowSets001.FOLLOW_102_in_rule__Dictionary__Group_7__0__Impl29227); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryAccess().getDictionarycontrolsKeyword_7_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Dictionary__Group_7__0__Impl"
// $ANTLR start "rule__Dictionary__Group_7__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14100:1: rule__Dictionary__Group_7__1 : rule__Dictionary__Group_7__1__Impl rule__Dictionary__Group_7__2 ;
public final void rule__Dictionary__Group_7__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14104:1: ( rule__Dictionary__Group_7__1__Impl rule__Dictionary__Group_7__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14105:2: rule__Dictionary__Group_7__1__Impl rule__Dictionary__Group_7__2
{
pushFollow(FollowSets001.FOLLOW_rule__Dictionary__Group_7__1__Impl_in_rule__Dictionary__Group_7__129258);
rule__Dictionary__Group_7__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__Dictionary__Group_7__2_in_rule__Dictionary__Group_7__129261);
rule__Dictionary__Group_7__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Dictionary__Group_7__1"
// $ANTLR start "rule__Dictionary__Group_7__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14112:1: rule__Dictionary__Group_7__1__Impl : ( '{' ) ;
public final void rule__Dictionary__Group_7__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14116:1: ( ( '{' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14117:1: ( '{' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14117:1: ( '{' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14118:1: '{'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryAccess().getLeftCurlyBracketKeyword_7_1());
}
match(input,67,FollowSets001.FOLLOW_67_in_rule__Dictionary__Group_7__1__Impl29289); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryAccess().getLeftCurlyBracketKeyword_7_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Dictionary__Group_7__1__Impl"
// $ANTLR start "rule__Dictionary__Group_7__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14131:1: rule__Dictionary__Group_7__2 : rule__Dictionary__Group_7__2__Impl rule__Dictionary__Group_7__3 ;
public final void rule__Dictionary__Group_7__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14135:1: ( rule__Dictionary__Group_7__2__Impl rule__Dictionary__Group_7__3 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14136:2: rule__Dictionary__Group_7__2__Impl rule__Dictionary__Group_7__3
{
pushFollow(FollowSets001.FOLLOW_rule__Dictionary__Group_7__2__Impl_in_rule__Dictionary__Group_7__229320);
rule__Dictionary__Group_7__2__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__Dictionary__Group_7__3_in_rule__Dictionary__Group_7__229323);
rule__Dictionary__Group_7__3();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Dictionary__Group_7__2"
// $ANTLR start "rule__Dictionary__Group_7__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14143:1: rule__Dictionary__Group_7__2__Impl : ( ( rule__Dictionary__DictionarycontrolsAssignment_7_2 )* ) ;
public final void rule__Dictionary__Group_7__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14147:1: ( ( ( rule__Dictionary__DictionarycontrolsAssignment_7_2 )* ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14148:1: ( ( rule__Dictionary__DictionarycontrolsAssignment_7_2 )* )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14148:1: ( ( rule__Dictionary__DictionarycontrolsAssignment_7_2 )* )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14149:1: ( rule__Dictionary__DictionarycontrolsAssignment_7_2 )*
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryAccess().getDictionarycontrolsAssignment_7_2());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14150:1: ( rule__Dictionary__DictionarycontrolsAssignment_7_2 )*
loop109:
do {
int alt109=2;
int LA109_0 = input.LA(1);
if ( (LA109_0==125||LA109_0==127||LA109_0==129||(LA109_0>=131 && LA109_0<=136)||LA109_0==138) ) {
alt109=1;
}
switch (alt109) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14150:2: rule__Dictionary__DictionarycontrolsAssignment_7_2
{
pushFollow(FollowSets001.FOLLOW_rule__Dictionary__DictionarycontrolsAssignment_7_2_in_rule__Dictionary__Group_7__2__Impl29350);
rule__Dictionary__DictionarycontrolsAssignment_7_2();
state._fsp--;
if (state.failed) return ;
}
break;
default :
break loop109;
}
} while (true);
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryAccess().getDictionarycontrolsAssignment_7_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Dictionary__Group_7__2__Impl"
// $ANTLR start "rule__Dictionary__Group_7__3"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14160:1: rule__Dictionary__Group_7__3 : rule__Dictionary__Group_7__3__Impl ;
public final void rule__Dictionary__Group_7__3() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14164:1: ( rule__Dictionary__Group_7__3__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14165:2: rule__Dictionary__Group_7__3__Impl
{
pushFollow(FollowSets001.FOLLOW_rule__Dictionary__Group_7__3__Impl_in_rule__Dictionary__Group_7__329381);
rule__Dictionary__Group_7__3__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Dictionary__Group_7__3"
// $ANTLR start "rule__Dictionary__Group_7__3__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14171:1: rule__Dictionary__Group_7__3__Impl : ( '}' ) ;
public final void rule__Dictionary__Group_7__3__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14175:1: ( ( '}' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14176:1: ( '}' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14176:1: ( '}' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14177:1: '}'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryAccess().getRightCurlyBracketKeyword_7_3());
}
match(input,68,FollowSets001.FOLLOW_68_in_rule__Dictionary__Group_7__3__Impl29409); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryAccess().getRightCurlyBracketKeyword_7_3());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Dictionary__Group_7__3__Impl"
// $ANTLR start "rule__Dictionary__Group_8__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14198:1: rule__Dictionary__Group_8__0 : rule__Dictionary__Group_8__0__Impl rule__Dictionary__Group_8__1 ;
public final void rule__Dictionary__Group_8__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14202:1: ( rule__Dictionary__Group_8__0__Impl rule__Dictionary__Group_8__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14203:2: rule__Dictionary__Group_8__0__Impl rule__Dictionary__Group_8__1
{
pushFollow(FollowSets001.FOLLOW_rule__Dictionary__Group_8__0__Impl_in_rule__Dictionary__Group_8__029448);
rule__Dictionary__Group_8__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__Dictionary__Group_8__1_in_rule__Dictionary__Group_8__029451);
rule__Dictionary__Group_8__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Dictionary__Group_8__0"
// $ANTLR start "rule__Dictionary__Group_8__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14210:1: rule__Dictionary__Group_8__0__Impl : ( 'labelcontrols' ) ;
public final void rule__Dictionary__Group_8__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14214:1: ( ( 'labelcontrols' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14215:1: ( 'labelcontrols' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14215:1: ( 'labelcontrols' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14216:1: 'labelcontrols'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryAccess().getLabelcontrolsKeyword_8_0());
}
match(input,103,FollowSets001.FOLLOW_103_in_rule__Dictionary__Group_8__0__Impl29479); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryAccess().getLabelcontrolsKeyword_8_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Dictionary__Group_8__0__Impl"
// $ANTLR start "rule__Dictionary__Group_8__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14229:1: rule__Dictionary__Group_8__1 : rule__Dictionary__Group_8__1__Impl rule__Dictionary__Group_8__2 ;
public final void rule__Dictionary__Group_8__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14233:1: ( rule__Dictionary__Group_8__1__Impl rule__Dictionary__Group_8__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14234:2: rule__Dictionary__Group_8__1__Impl rule__Dictionary__Group_8__2
{
pushFollow(FollowSets001.FOLLOW_rule__Dictionary__Group_8__1__Impl_in_rule__Dictionary__Group_8__129510);
rule__Dictionary__Group_8__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__Dictionary__Group_8__2_in_rule__Dictionary__Group_8__129513);
rule__Dictionary__Group_8__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Dictionary__Group_8__1"
// $ANTLR start "rule__Dictionary__Group_8__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14241:1: rule__Dictionary__Group_8__1__Impl : ( '{' ) ;
public final void rule__Dictionary__Group_8__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14245:1: ( ( '{' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14246:1: ( '{' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14246:1: ( '{' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14247:1: '{'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryAccess().getLeftCurlyBracketKeyword_8_1());
}
match(input,67,FollowSets001.FOLLOW_67_in_rule__Dictionary__Group_8__1__Impl29541); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryAccess().getLeftCurlyBracketKeyword_8_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Dictionary__Group_8__1__Impl"
// $ANTLR start "rule__Dictionary__Group_8__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14260:1: rule__Dictionary__Group_8__2 : rule__Dictionary__Group_8__2__Impl rule__Dictionary__Group_8__3 ;
public final void rule__Dictionary__Group_8__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14264:1: ( rule__Dictionary__Group_8__2__Impl rule__Dictionary__Group_8__3 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14265:2: rule__Dictionary__Group_8__2__Impl rule__Dictionary__Group_8__3
{
pushFollow(FollowSets001.FOLLOW_rule__Dictionary__Group_8__2__Impl_in_rule__Dictionary__Group_8__229572);
rule__Dictionary__Group_8__2__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__Dictionary__Group_8__3_in_rule__Dictionary__Group_8__229575);
rule__Dictionary__Group_8__3();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Dictionary__Group_8__2"
// $ANTLR start "rule__Dictionary__Group_8__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14272:1: rule__Dictionary__Group_8__2__Impl : ( ( rule__Dictionary__LabelcontrolsAssignment_8_2 )* ) ;
public final void rule__Dictionary__Group_8__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14276:1: ( ( ( rule__Dictionary__LabelcontrolsAssignment_8_2 )* ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14277:1: ( ( rule__Dictionary__LabelcontrolsAssignment_8_2 )* )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14277:1: ( ( rule__Dictionary__LabelcontrolsAssignment_8_2 )* )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14278:1: ( rule__Dictionary__LabelcontrolsAssignment_8_2 )*
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryAccess().getLabelcontrolsAssignment_8_2());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14279:1: ( rule__Dictionary__LabelcontrolsAssignment_8_2 )*
loop110:
do {
int alt110=2;
int LA110_0 = input.LA(1);
if ( (LA110_0==125||LA110_0==127||LA110_0==129||(LA110_0>=131 && LA110_0<=136)||LA110_0==138) ) {
alt110=1;
}
switch (alt110) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14279:2: rule__Dictionary__LabelcontrolsAssignment_8_2
{
pushFollow(FollowSets001.FOLLOW_rule__Dictionary__LabelcontrolsAssignment_8_2_in_rule__Dictionary__Group_8__2__Impl29602);
rule__Dictionary__LabelcontrolsAssignment_8_2();
state._fsp--;
if (state.failed) return ;
}
break;
default :
break loop110;
}
} while (true);
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryAccess().getLabelcontrolsAssignment_8_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Dictionary__Group_8__2__Impl"
// $ANTLR start "rule__Dictionary__Group_8__3"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14289:1: rule__Dictionary__Group_8__3 : rule__Dictionary__Group_8__3__Impl ;
public final void rule__Dictionary__Group_8__3() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14293:1: ( rule__Dictionary__Group_8__3__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14294:2: rule__Dictionary__Group_8__3__Impl
{
pushFollow(FollowSets001.FOLLOW_rule__Dictionary__Group_8__3__Impl_in_rule__Dictionary__Group_8__329633);
rule__Dictionary__Group_8__3__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Dictionary__Group_8__3"
// $ANTLR start "rule__Dictionary__Group_8__3__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14300:1: rule__Dictionary__Group_8__3__Impl : ( '}' ) ;
public final void rule__Dictionary__Group_8__3__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14304:1: ( ( '}' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14305:1: ( '}' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14305:1: ( '}' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14306:1: '}'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryAccess().getRightCurlyBracketKeyword_8_3());
}
match(input,68,FollowSets001.FOLLOW_68_in_rule__Dictionary__Group_8__3__Impl29661); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryAccess().getRightCurlyBracketKeyword_8_3());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Dictionary__Group_8__3__Impl"
// $ANTLR start "rule__DictionarySearch__Group__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14327:1: rule__DictionarySearch__Group__0 : rule__DictionarySearch__Group__0__Impl rule__DictionarySearch__Group__1 ;
public final void rule__DictionarySearch__Group__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14331:1: ( rule__DictionarySearch__Group__0__Impl rule__DictionarySearch__Group__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14332:2: rule__DictionarySearch__Group__0__Impl rule__DictionarySearch__Group__1
{
pushFollow(FollowSets001.FOLLOW_rule__DictionarySearch__Group__0__Impl_in_rule__DictionarySearch__Group__029700);
rule__DictionarySearch__Group__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionarySearch__Group__1_in_rule__DictionarySearch__Group__029703);
rule__DictionarySearch__Group__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionarySearch__Group__0"
// $ANTLR start "rule__DictionarySearch__Group__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14339:1: rule__DictionarySearch__Group__0__Impl : ( 'dictionarysearch' ) ;
public final void rule__DictionarySearch__Group__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14343:1: ( ( 'dictionarysearch' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14344:1: ( 'dictionarysearch' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14344:1: ( 'dictionarysearch' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14345:1: 'dictionarysearch'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionarySearchAccess().getDictionarysearchKeyword_0());
}
match(input,104,FollowSets001.FOLLOW_104_in_rule__DictionarySearch__Group__0__Impl29731); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionarySearchAccess().getDictionarysearchKeyword_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionarySearch__Group__0__Impl"
// $ANTLR start "rule__DictionarySearch__Group__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14358:1: rule__DictionarySearch__Group__1 : rule__DictionarySearch__Group__1__Impl rule__DictionarySearch__Group__2 ;
public final void rule__DictionarySearch__Group__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14362:1: ( rule__DictionarySearch__Group__1__Impl rule__DictionarySearch__Group__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14363:2: rule__DictionarySearch__Group__1__Impl rule__DictionarySearch__Group__2
{
pushFollow(FollowSets001.FOLLOW_rule__DictionarySearch__Group__1__Impl_in_rule__DictionarySearch__Group__129762);
rule__DictionarySearch__Group__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionarySearch__Group__2_in_rule__DictionarySearch__Group__129765);
rule__DictionarySearch__Group__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionarySearch__Group__1"
// $ANTLR start "rule__DictionarySearch__Group__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14370:1: rule__DictionarySearch__Group__1__Impl : ( ( rule__DictionarySearch__NameAssignment_1 ) ) ;
public final void rule__DictionarySearch__Group__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14374:1: ( ( ( rule__DictionarySearch__NameAssignment_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14375:1: ( ( rule__DictionarySearch__NameAssignment_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14375:1: ( ( rule__DictionarySearch__NameAssignment_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14376:1: ( rule__DictionarySearch__NameAssignment_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionarySearchAccess().getNameAssignment_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14377:1: ( rule__DictionarySearch__NameAssignment_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14377:2: rule__DictionarySearch__NameAssignment_1
{
pushFollow(FollowSets001.FOLLOW_rule__DictionarySearch__NameAssignment_1_in_rule__DictionarySearch__Group__1__Impl29792);
rule__DictionarySearch__NameAssignment_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionarySearchAccess().getNameAssignment_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionarySearch__Group__1__Impl"
// $ANTLR start "rule__DictionarySearch__Group__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14387:1: rule__DictionarySearch__Group__2 : rule__DictionarySearch__Group__2__Impl rule__DictionarySearch__Group__3 ;
public final void rule__DictionarySearch__Group__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14391:1: ( rule__DictionarySearch__Group__2__Impl rule__DictionarySearch__Group__3 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14392:2: rule__DictionarySearch__Group__2__Impl rule__DictionarySearch__Group__3
{
pushFollow(FollowSets001.FOLLOW_rule__DictionarySearch__Group__2__Impl_in_rule__DictionarySearch__Group__229822);
rule__DictionarySearch__Group__2__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionarySearch__Group__3_in_rule__DictionarySearch__Group__229825);
rule__DictionarySearch__Group__3();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionarySearch__Group__2"
// $ANTLR start "rule__DictionarySearch__Group__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14399:1: rule__DictionarySearch__Group__2__Impl : ( '{' ) ;
public final void rule__DictionarySearch__Group__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14403:1: ( ( '{' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14404:1: ( '{' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14404:1: ( '{' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14405:1: '{'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionarySearchAccess().getLeftCurlyBracketKeyword_2());
}
match(input,67,FollowSets001.FOLLOW_67_in_rule__DictionarySearch__Group__2__Impl29853); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionarySearchAccess().getLeftCurlyBracketKeyword_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionarySearch__Group__2__Impl"
// $ANTLR start "rule__DictionarySearch__Group__3"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14418:1: rule__DictionarySearch__Group__3 : rule__DictionarySearch__Group__3__Impl rule__DictionarySearch__Group__4 ;
public final void rule__DictionarySearch__Group__3() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14422:1: ( rule__DictionarySearch__Group__3__Impl rule__DictionarySearch__Group__4 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14423:2: rule__DictionarySearch__Group__3__Impl rule__DictionarySearch__Group__4
{
pushFollow(FollowSets001.FOLLOW_rule__DictionarySearch__Group__3__Impl_in_rule__DictionarySearch__Group__329884);
rule__DictionarySearch__Group__3__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionarySearch__Group__4_in_rule__DictionarySearch__Group__329887);
rule__DictionarySearch__Group__4();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionarySearch__Group__3"
// $ANTLR start "rule__DictionarySearch__Group__3__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14430:1: rule__DictionarySearch__Group__3__Impl : ( ( rule__DictionarySearch__Group_3__0 )? ) ;
public final void rule__DictionarySearch__Group__3__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14434:1: ( ( ( rule__DictionarySearch__Group_3__0 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14435:1: ( ( rule__DictionarySearch__Group_3__0 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14435:1: ( ( rule__DictionarySearch__Group_3__0 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14436:1: ( rule__DictionarySearch__Group_3__0 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionarySearchAccess().getGroup_3());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14437:1: ( rule__DictionarySearch__Group_3__0 )?
int alt111=2;
int LA111_0 = input.LA(1);
if ( (LA111_0==75) ) {
alt111=1;
}
switch (alt111) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14437:2: rule__DictionarySearch__Group_3__0
{
pushFollow(FollowSets001.FOLLOW_rule__DictionarySearch__Group_3__0_in_rule__DictionarySearch__Group__3__Impl29914);
rule__DictionarySearch__Group_3__0();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionarySearchAccess().getGroup_3());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionarySearch__Group__3__Impl"
// $ANTLR start "rule__DictionarySearch__Group__4"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14447:1: rule__DictionarySearch__Group__4 : rule__DictionarySearch__Group__4__Impl rule__DictionarySearch__Group__5 ;
public final void rule__DictionarySearch__Group__4() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14451:1: ( rule__DictionarySearch__Group__4__Impl rule__DictionarySearch__Group__5 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14452:2: rule__DictionarySearch__Group__4__Impl rule__DictionarySearch__Group__5
{
pushFollow(FollowSets001.FOLLOW_rule__DictionarySearch__Group__4__Impl_in_rule__DictionarySearch__Group__429945);
rule__DictionarySearch__Group__4__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionarySearch__Group__5_in_rule__DictionarySearch__Group__429948);
rule__DictionarySearch__Group__5();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionarySearch__Group__4"
// $ANTLR start "rule__DictionarySearch__Group__4__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14459:1: rule__DictionarySearch__Group__4__Impl : ( ( rule__DictionarySearch__DictionaryfiltersAssignment_4 )* ) ;
public final void rule__DictionarySearch__Group__4__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14463:1: ( ( ( rule__DictionarySearch__DictionaryfiltersAssignment_4 )* ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14464:1: ( ( rule__DictionarySearch__DictionaryfiltersAssignment_4 )* )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14464:1: ( ( rule__DictionarySearch__DictionaryfiltersAssignment_4 )* )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14465:1: ( rule__DictionarySearch__DictionaryfiltersAssignment_4 )*
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionarySearchAccess().getDictionaryfiltersAssignment_4());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14466:1: ( rule__DictionarySearch__DictionaryfiltersAssignment_4 )*
loop112:
do {
int alt112=2;
int LA112_0 = input.LA(1);
if ( (LA112_0==106) ) {
alt112=1;
}
switch (alt112) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14466:2: rule__DictionarySearch__DictionaryfiltersAssignment_4
{
pushFollow(FollowSets001.FOLLOW_rule__DictionarySearch__DictionaryfiltersAssignment_4_in_rule__DictionarySearch__Group__4__Impl29975);
rule__DictionarySearch__DictionaryfiltersAssignment_4();
state._fsp--;
if (state.failed) return ;
}
break;
default :
break loop112;
}
} while (true);
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionarySearchAccess().getDictionaryfiltersAssignment_4());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionarySearch__Group__4__Impl"
// $ANTLR start "rule__DictionarySearch__Group__5"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14476:1: rule__DictionarySearch__Group__5 : rule__DictionarySearch__Group__5__Impl rule__DictionarySearch__Group__6 ;
public final void rule__DictionarySearch__Group__5() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14480:1: ( rule__DictionarySearch__Group__5__Impl rule__DictionarySearch__Group__6 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14481:2: rule__DictionarySearch__Group__5__Impl rule__DictionarySearch__Group__6
{
pushFollow(FollowSets001.FOLLOW_rule__DictionarySearch__Group__5__Impl_in_rule__DictionarySearch__Group__530006);
rule__DictionarySearch__Group__5__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionarySearch__Group__6_in_rule__DictionarySearch__Group__530009);
rule__DictionarySearch__Group__6();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionarySearch__Group__5"
// $ANTLR start "rule__DictionarySearch__Group__5__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14488:1: rule__DictionarySearch__Group__5__Impl : ( ( rule__DictionarySearch__DictionaryresultAssignment_5 ) ) ;
public final void rule__DictionarySearch__Group__5__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14492:1: ( ( ( rule__DictionarySearch__DictionaryresultAssignment_5 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14493:1: ( ( rule__DictionarySearch__DictionaryresultAssignment_5 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14493:1: ( ( rule__DictionarySearch__DictionaryresultAssignment_5 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14494:1: ( rule__DictionarySearch__DictionaryresultAssignment_5 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionarySearchAccess().getDictionaryresultAssignment_5());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14495:1: ( rule__DictionarySearch__DictionaryresultAssignment_5 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14495:2: rule__DictionarySearch__DictionaryresultAssignment_5
{
pushFollow(FollowSets001.FOLLOW_rule__DictionarySearch__DictionaryresultAssignment_5_in_rule__DictionarySearch__Group__5__Impl30036);
rule__DictionarySearch__DictionaryresultAssignment_5();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionarySearchAccess().getDictionaryresultAssignment_5());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionarySearch__Group__5__Impl"
// $ANTLR start "rule__DictionarySearch__Group__6"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14505:1: rule__DictionarySearch__Group__6 : rule__DictionarySearch__Group__6__Impl ;
public final void rule__DictionarySearch__Group__6() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14509:1: ( rule__DictionarySearch__Group__6__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14510:2: rule__DictionarySearch__Group__6__Impl
{
pushFollow(FollowSets001.FOLLOW_rule__DictionarySearch__Group__6__Impl_in_rule__DictionarySearch__Group__630066);
rule__DictionarySearch__Group__6__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionarySearch__Group__6"
// $ANTLR start "rule__DictionarySearch__Group__6__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14516:1: rule__DictionarySearch__Group__6__Impl : ( '}' ) ;
public final void rule__DictionarySearch__Group__6__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14520:1: ( ( '}' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14521:1: ( '}' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14521:1: ( '}' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14522:1: '}'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionarySearchAccess().getRightCurlyBracketKeyword_6());
}
match(input,68,FollowSets001.FOLLOW_68_in_rule__DictionarySearch__Group__6__Impl30094); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionarySearchAccess().getRightCurlyBracketKeyword_6());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionarySearch__Group__6__Impl"
// $ANTLR start "rule__DictionarySearch__Group_3__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14549:1: rule__DictionarySearch__Group_3__0 : rule__DictionarySearch__Group_3__0__Impl rule__DictionarySearch__Group_3__1 ;
public final void rule__DictionarySearch__Group_3__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14553:1: ( rule__DictionarySearch__Group_3__0__Impl rule__DictionarySearch__Group_3__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14554:2: rule__DictionarySearch__Group_3__0__Impl rule__DictionarySearch__Group_3__1
{
pushFollow(FollowSets001.FOLLOW_rule__DictionarySearch__Group_3__0__Impl_in_rule__DictionarySearch__Group_3__030139);
rule__DictionarySearch__Group_3__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionarySearch__Group_3__1_in_rule__DictionarySearch__Group_3__030142);
rule__DictionarySearch__Group_3__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionarySearch__Group_3__0"
// $ANTLR start "rule__DictionarySearch__Group_3__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14561:1: rule__DictionarySearch__Group_3__0__Impl : ( 'label' ) ;
public final void rule__DictionarySearch__Group_3__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14565:1: ( ( 'label' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14566:1: ( 'label' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14566:1: ( 'label' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14567:1: 'label'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionarySearchAccess().getLabelKeyword_3_0());
}
match(input,75,FollowSets001.FOLLOW_75_in_rule__DictionarySearch__Group_3__0__Impl30170); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionarySearchAccess().getLabelKeyword_3_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionarySearch__Group_3__0__Impl"
// $ANTLR start "rule__DictionarySearch__Group_3__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14580:1: rule__DictionarySearch__Group_3__1 : rule__DictionarySearch__Group_3__1__Impl ;
public final void rule__DictionarySearch__Group_3__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14584:1: ( rule__DictionarySearch__Group_3__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14585:2: rule__DictionarySearch__Group_3__1__Impl
{
pushFollow(FollowSets001.FOLLOW_rule__DictionarySearch__Group_3__1__Impl_in_rule__DictionarySearch__Group_3__130201);
rule__DictionarySearch__Group_3__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionarySearch__Group_3__1"
// $ANTLR start "rule__DictionarySearch__Group_3__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14591:1: rule__DictionarySearch__Group_3__1__Impl : ( ( rule__DictionarySearch__LabelAssignment_3_1 ) ) ;
public final void rule__DictionarySearch__Group_3__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14595:1: ( ( ( rule__DictionarySearch__LabelAssignment_3_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14596:1: ( ( rule__DictionarySearch__LabelAssignment_3_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14596:1: ( ( rule__DictionarySearch__LabelAssignment_3_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14597:1: ( rule__DictionarySearch__LabelAssignment_3_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionarySearchAccess().getLabelAssignment_3_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14598:1: ( rule__DictionarySearch__LabelAssignment_3_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14598:2: rule__DictionarySearch__LabelAssignment_3_1
{
pushFollow(FollowSets001.FOLLOW_rule__DictionarySearch__LabelAssignment_3_1_in_rule__DictionarySearch__Group_3__1__Impl30228);
rule__DictionarySearch__LabelAssignment_3_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionarySearchAccess().getLabelAssignment_3_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionarySearch__Group_3__1__Impl"
// $ANTLR start "rule__DictionaryEditor__Group__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14612:1: rule__DictionaryEditor__Group__0 : rule__DictionaryEditor__Group__0__Impl rule__DictionaryEditor__Group__1 ;
public final void rule__DictionaryEditor__Group__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14616:1: ( rule__DictionaryEditor__Group__0__Impl rule__DictionaryEditor__Group__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14617:2: rule__DictionaryEditor__Group__0__Impl rule__DictionaryEditor__Group__1
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryEditor__Group__0__Impl_in_rule__DictionaryEditor__Group__030262);
rule__DictionaryEditor__Group__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryEditor__Group__1_in_rule__DictionaryEditor__Group__030265);
rule__DictionaryEditor__Group__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryEditor__Group__0"
// $ANTLR start "rule__DictionaryEditor__Group__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14624:1: rule__DictionaryEditor__Group__0__Impl : ( 'dictionaryeditor' ) ;
public final void rule__DictionaryEditor__Group__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14628:1: ( ( 'dictionaryeditor' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14629:1: ( 'dictionaryeditor' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14629:1: ( 'dictionaryeditor' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14630:1: 'dictionaryeditor'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryEditorAccess().getDictionaryeditorKeyword_0());
}
match(input,105,FollowSets001.FOLLOW_105_in_rule__DictionaryEditor__Group__0__Impl30293); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryEditorAccess().getDictionaryeditorKeyword_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryEditor__Group__0__Impl"
// $ANTLR start "rule__DictionaryEditor__Group__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14643:1: rule__DictionaryEditor__Group__1 : rule__DictionaryEditor__Group__1__Impl rule__DictionaryEditor__Group__2 ;
public final void rule__DictionaryEditor__Group__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14647:1: ( rule__DictionaryEditor__Group__1__Impl rule__DictionaryEditor__Group__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14648:2: rule__DictionaryEditor__Group__1__Impl rule__DictionaryEditor__Group__2
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryEditor__Group__1__Impl_in_rule__DictionaryEditor__Group__130324);
rule__DictionaryEditor__Group__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryEditor__Group__2_in_rule__DictionaryEditor__Group__130327);
rule__DictionaryEditor__Group__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryEditor__Group__1"
// $ANTLR start "rule__DictionaryEditor__Group__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14655:1: rule__DictionaryEditor__Group__1__Impl : ( ( rule__DictionaryEditor__NameAssignment_1 ) ) ;
public final void rule__DictionaryEditor__Group__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14659:1: ( ( ( rule__DictionaryEditor__NameAssignment_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14660:1: ( ( rule__DictionaryEditor__NameAssignment_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14660:1: ( ( rule__DictionaryEditor__NameAssignment_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14661:1: ( rule__DictionaryEditor__NameAssignment_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryEditorAccess().getNameAssignment_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14662:1: ( rule__DictionaryEditor__NameAssignment_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14662:2: rule__DictionaryEditor__NameAssignment_1
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryEditor__NameAssignment_1_in_rule__DictionaryEditor__Group__1__Impl30354);
rule__DictionaryEditor__NameAssignment_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryEditorAccess().getNameAssignment_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryEditor__Group__1__Impl"
// $ANTLR start "rule__DictionaryEditor__Group__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14672:1: rule__DictionaryEditor__Group__2 : rule__DictionaryEditor__Group__2__Impl rule__DictionaryEditor__Group__3 ;
public final void rule__DictionaryEditor__Group__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14676:1: ( rule__DictionaryEditor__Group__2__Impl rule__DictionaryEditor__Group__3 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14677:2: rule__DictionaryEditor__Group__2__Impl rule__DictionaryEditor__Group__3
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryEditor__Group__2__Impl_in_rule__DictionaryEditor__Group__230384);
rule__DictionaryEditor__Group__2__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryEditor__Group__3_in_rule__DictionaryEditor__Group__230387);
rule__DictionaryEditor__Group__3();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryEditor__Group__2"
// $ANTLR start "rule__DictionaryEditor__Group__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14684:1: rule__DictionaryEditor__Group__2__Impl : ( '{' ) ;
public final void rule__DictionaryEditor__Group__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14688:1: ( ( '{' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14689:1: ( '{' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14689:1: ( '{' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14690:1: '{'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryEditorAccess().getLeftCurlyBracketKeyword_2());
}
match(input,67,FollowSets001.FOLLOW_67_in_rule__DictionaryEditor__Group__2__Impl30415); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryEditorAccess().getLeftCurlyBracketKeyword_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryEditor__Group__2__Impl"
// $ANTLR start "rule__DictionaryEditor__Group__3"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14703:1: rule__DictionaryEditor__Group__3 : rule__DictionaryEditor__Group__3__Impl rule__DictionaryEditor__Group__4 ;
public final void rule__DictionaryEditor__Group__3() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14707:1: ( rule__DictionaryEditor__Group__3__Impl rule__DictionaryEditor__Group__4 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14708:2: rule__DictionaryEditor__Group__3__Impl rule__DictionaryEditor__Group__4
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryEditor__Group__3__Impl_in_rule__DictionaryEditor__Group__330446);
rule__DictionaryEditor__Group__3__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryEditor__Group__4_in_rule__DictionaryEditor__Group__330449);
rule__DictionaryEditor__Group__4();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryEditor__Group__3"
// $ANTLR start "rule__DictionaryEditor__Group__3__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14715:1: rule__DictionaryEditor__Group__3__Impl : ( ( rule__DictionaryEditor__Group_3__0 )? ) ;
public final void rule__DictionaryEditor__Group__3__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14719:1: ( ( ( rule__DictionaryEditor__Group_3__0 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14720:1: ( ( rule__DictionaryEditor__Group_3__0 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14720:1: ( ( rule__DictionaryEditor__Group_3__0 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14721:1: ( rule__DictionaryEditor__Group_3__0 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryEditorAccess().getGroup_3());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14722:1: ( rule__DictionaryEditor__Group_3__0 )?
int alt113=2;
int LA113_0 = input.LA(1);
if ( (LA113_0==75) ) {
alt113=1;
}
switch (alt113) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14722:2: rule__DictionaryEditor__Group_3__0
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryEditor__Group_3__0_in_rule__DictionaryEditor__Group__3__Impl30476);
rule__DictionaryEditor__Group_3__0();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryEditorAccess().getGroup_3());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryEditor__Group__3__Impl"
// $ANTLR start "rule__DictionaryEditor__Group__4"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14732:1: rule__DictionaryEditor__Group__4 : rule__DictionaryEditor__Group__4__Impl rule__DictionaryEditor__Group__5 ;
public final void rule__DictionaryEditor__Group__4() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14736:1: ( rule__DictionaryEditor__Group__4__Impl rule__DictionaryEditor__Group__5 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14737:2: rule__DictionaryEditor__Group__4__Impl rule__DictionaryEditor__Group__5
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryEditor__Group__4__Impl_in_rule__DictionaryEditor__Group__430507);
rule__DictionaryEditor__Group__4__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryEditor__Group__5_in_rule__DictionaryEditor__Group__430510);
rule__DictionaryEditor__Group__5();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryEditor__Group__4"
// $ANTLR start "rule__DictionaryEditor__Group__4__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14744:1: rule__DictionaryEditor__Group__4__Impl : ( ( rule__DictionaryEditor__LayoutdataAssignment_4 )? ) ;
public final void rule__DictionaryEditor__Group__4__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14748:1: ( ( ( rule__DictionaryEditor__LayoutdataAssignment_4 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14749:1: ( ( rule__DictionaryEditor__LayoutdataAssignment_4 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14749:1: ( ( rule__DictionaryEditor__LayoutdataAssignment_4 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14750:1: ( rule__DictionaryEditor__LayoutdataAssignment_4 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryEditorAccess().getLayoutdataAssignment_4());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14751:1: ( rule__DictionaryEditor__LayoutdataAssignment_4 )?
int alt114=2;
int LA114_0 = input.LA(1);
if ( (LA114_0==110) ) {
alt114=1;
}
switch (alt114) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14751:2: rule__DictionaryEditor__LayoutdataAssignment_4
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryEditor__LayoutdataAssignment_4_in_rule__DictionaryEditor__Group__4__Impl30537);
rule__DictionaryEditor__LayoutdataAssignment_4();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryEditorAccess().getLayoutdataAssignment_4());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryEditor__Group__4__Impl"
// $ANTLR start "rule__DictionaryEditor__Group__5"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14761:1: rule__DictionaryEditor__Group__5 : rule__DictionaryEditor__Group__5__Impl rule__DictionaryEditor__Group__6 ;
public final void rule__DictionaryEditor__Group__5() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14765:1: ( rule__DictionaryEditor__Group__5__Impl rule__DictionaryEditor__Group__6 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14766:2: rule__DictionaryEditor__Group__5__Impl rule__DictionaryEditor__Group__6
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryEditor__Group__5__Impl_in_rule__DictionaryEditor__Group__530568);
rule__DictionaryEditor__Group__5__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryEditor__Group__6_in_rule__DictionaryEditor__Group__530571);
rule__DictionaryEditor__Group__6();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryEditor__Group__5"
// $ANTLR start "rule__DictionaryEditor__Group__5__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14773:1: rule__DictionaryEditor__Group__5__Impl : ( ( rule__DictionaryEditor__LayoutAssignment_5 )? ) ;
public final void rule__DictionaryEditor__Group__5__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14777:1: ( ( ( rule__DictionaryEditor__LayoutAssignment_5 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14778:1: ( ( rule__DictionaryEditor__LayoutAssignment_5 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14778:1: ( ( rule__DictionaryEditor__LayoutAssignment_5 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14779:1: ( rule__DictionaryEditor__LayoutAssignment_5 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryEditorAccess().getLayoutAssignment_5());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14780:1: ( rule__DictionaryEditor__LayoutAssignment_5 )?
int alt115=2;
int LA115_0 = input.LA(1);
if ( (LA115_0==108) ) {
alt115=1;
}
switch (alt115) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14780:2: rule__DictionaryEditor__LayoutAssignment_5
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryEditor__LayoutAssignment_5_in_rule__DictionaryEditor__Group__5__Impl30598);
rule__DictionaryEditor__LayoutAssignment_5();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryEditorAccess().getLayoutAssignment_5());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryEditor__Group__5__Impl"
// $ANTLR start "rule__DictionaryEditor__Group__6"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14790:1: rule__DictionaryEditor__Group__6 : rule__DictionaryEditor__Group__6__Impl rule__DictionaryEditor__Group__7 ;
public final void rule__DictionaryEditor__Group__6() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14794:1: ( rule__DictionaryEditor__Group__6__Impl rule__DictionaryEditor__Group__7 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14795:2: rule__DictionaryEditor__Group__6__Impl rule__DictionaryEditor__Group__7
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryEditor__Group__6__Impl_in_rule__DictionaryEditor__Group__630629);
rule__DictionaryEditor__Group__6__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryEditor__Group__7_in_rule__DictionaryEditor__Group__630632);
rule__DictionaryEditor__Group__7();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryEditor__Group__6"
// $ANTLR start "rule__DictionaryEditor__Group__6__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14802:1: rule__DictionaryEditor__Group__6__Impl : ( ( rule__DictionaryEditor__ContainercontentsAssignment_6 )* ) ;
public final void rule__DictionaryEditor__Group__6__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14806:1: ( ( ( rule__DictionaryEditor__ContainercontentsAssignment_6 )* ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14807:1: ( ( rule__DictionaryEditor__ContainercontentsAssignment_6 )* )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14807:1: ( ( rule__DictionaryEditor__ContainercontentsAssignment_6 )* )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14808:1: ( rule__DictionaryEditor__ContainercontentsAssignment_6 )*
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryEditorAccess().getContainercontentsAssignment_6());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14809:1: ( rule__DictionaryEditor__ContainercontentsAssignment_6 )*
loop116:
do {
int alt116=2;
int LA116_0 = input.LA(1);
if ( ((LA116_0>=112 && LA116_0<=113)||LA116_0==116||LA116_0==125||LA116_0==127||LA116_0==129||(LA116_0>=131 && LA116_0<=136)||LA116_0==138) ) {
alt116=1;
}
switch (alt116) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14809:2: rule__DictionaryEditor__ContainercontentsAssignment_6
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryEditor__ContainercontentsAssignment_6_in_rule__DictionaryEditor__Group__6__Impl30659);
rule__DictionaryEditor__ContainercontentsAssignment_6();
state._fsp--;
if (state.failed) return ;
}
break;
default :
break loop116;
}
} while (true);
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryEditorAccess().getContainercontentsAssignment_6());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryEditor__Group__6__Impl"
// $ANTLR start "rule__DictionaryEditor__Group__7"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14819:1: rule__DictionaryEditor__Group__7 : rule__DictionaryEditor__Group__7__Impl ;
public final void rule__DictionaryEditor__Group__7() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14823:1: ( rule__DictionaryEditor__Group__7__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14824:2: rule__DictionaryEditor__Group__7__Impl
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryEditor__Group__7__Impl_in_rule__DictionaryEditor__Group__730690);
rule__DictionaryEditor__Group__7__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryEditor__Group__7"
// $ANTLR start "rule__DictionaryEditor__Group__7__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14830:1: rule__DictionaryEditor__Group__7__Impl : ( '}' ) ;
public final void rule__DictionaryEditor__Group__7__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14834:1: ( ( '}' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14835:1: ( '}' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14835:1: ( '}' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14836:1: '}'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryEditorAccess().getRightCurlyBracketKeyword_7());
}
match(input,68,FollowSets001.FOLLOW_68_in_rule__DictionaryEditor__Group__7__Impl30718); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryEditorAccess().getRightCurlyBracketKeyword_7());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryEditor__Group__7__Impl"
// $ANTLR start "rule__DictionaryEditor__Group_3__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14865:1: rule__DictionaryEditor__Group_3__0 : rule__DictionaryEditor__Group_3__0__Impl rule__DictionaryEditor__Group_3__1 ;
public final void rule__DictionaryEditor__Group_3__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14869:1: ( rule__DictionaryEditor__Group_3__0__Impl rule__DictionaryEditor__Group_3__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14870:2: rule__DictionaryEditor__Group_3__0__Impl rule__DictionaryEditor__Group_3__1
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryEditor__Group_3__0__Impl_in_rule__DictionaryEditor__Group_3__030765);
rule__DictionaryEditor__Group_3__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryEditor__Group_3__1_in_rule__DictionaryEditor__Group_3__030768);
rule__DictionaryEditor__Group_3__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryEditor__Group_3__0"
// $ANTLR start "rule__DictionaryEditor__Group_3__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14877:1: rule__DictionaryEditor__Group_3__0__Impl : ( 'label' ) ;
public final void rule__DictionaryEditor__Group_3__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14881:1: ( ( 'label' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14882:1: ( 'label' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14882:1: ( 'label' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14883:1: 'label'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryEditorAccess().getLabelKeyword_3_0());
}
match(input,75,FollowSets001.FOLLOW_75_in_rule__DictionaryEditor__Group_3__0__Impl30796); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryEditorAccess().getLabelKeyword_3_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryEditor__Group_3__0__Impl"
// $ANTLR start "rule__DictionaryEditor__Group_3__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14896:1: rule__DictionaryEditor__Group_3__1 : rule__DictionaryEditor__Group_3__1__Impl ;
public final void rule__DictionaryEditor__Group_3__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14900:1: ( rule__DictionaryEditor__Group_3__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14901:2: rule__DictionaryEditor__Group_3__1__Impl
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryEditor__Group_3__1__Impl_in_rule__DictionaryEditor__Group_3__130827);
rule__DictionaryEditor__Group_3__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryEditor__Group_3__1"
// $ANTLR start "rule__DictionaryEditor__Group_3__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14907:1: rule__DictionaryEditor__Group_3__1__Impl : ( ( rule__DictionaryEditor__LabelAssignment_3_1 ) ) ;
public final void rule__DictionaryEditor__Group_3__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14911:1: ( ( ( rule__DictionaryEditor__LabelAssignment_3_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14912:1: ( ( rule__DictionaryEditor__LabelAssignment_3_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14912:1: ( ( rule__DictionaryEditor__LabelAssignment_3_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14913:1: ( rule__DictionaryEditor__LabelAssignment_3_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryEditorAccess().getLabelAssignment_3_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14914:1: ( rule__DictionaryEditor__LabelAssignment_3_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14914:2: rule__DictionaryEditor__LabelAssignment_3_1
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryEditor__LabelAssignment_3_1_in_rule__DictionaryEditor__Group_3__1__Impl30854);
rule__DictionaryEditor__LabelAssignment_3_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryEditorAccess().getLabelAssignment_3_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryEditor__Group_3__1__Impl"
// $ANTLR start "rule__DictionaryFilter__Group__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14928:1: rule__DictionaryFilter__Group__0 : rule__DictionaryFilter__Group__0__Impl rule__DictionaryFilter__Group__1 ;
public final void rule__DictionaryFilter__Group__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14932:1: ( rule__DictionaryFilter__Group__0__Impl rule__DictionaryFilter__Group__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14933:2: rule__DictionaryFilter__Group__0__Impl rule__DictionaryFilter__Group__1
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryFilter__Group__0__Impl_in_rule__DictionaryFilter__Group__030888);
rule__DictionaryFilter__Group__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryFilter__Group__1_in_rule__DictionaryFilter__Group__030891);
rule__DictionaryFilter__Group__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryFilter__Group__0"
// $ANTLR start "rule__DictionaryFilter__Group__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14940:1: rule__DictionaryFilter__Group__0__Impl : ( 'dictionaryfilter' ) ;
public final void rule__DictionaryFilter__Group__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14944:1: ( ( 'dictionaryfilter' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14945:1: ( 'dictionaryfilter' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14945:1: ( 'dictionaryfilter' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14946:1: 'dictionaryfilter'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryFilterAccess().getDictionaryfilterKeyword_0());
}
match(input,106,FollowSets001.FOLLOW_106_in_rule__DictionaryFilter__Group__0__Impl30919); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryFilterAccess().getDictionaryfilterKeyword_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryFilter__Group__0__Impl"
// $ANTLR start "rule__DictionaryFilter__Group__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14959:1: rule__DictionaryFilter__Group__1 : rule__DictionaryFilter__Group__1__Impl rule__DictionaryFilter__Group__2 ;
public final void rule__DictionaryFilter__Group__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14963:1: ( rule__DictionaryFilter__Group__1__Impl rule__DictionaryFilter__Group__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14964:2: rule__DictionaryFilter__Group__1__Impl rule__DictionaryFilter__Group__2
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryFilter__Group__1__Impl_in_rule__DictionaryFilter__Group__130950);
rule__DictionaryFilter__Group__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryFilter__Group__2_in_rule__DictionaryFilter__Group__130953);
rule__DictionaryFilter__Group__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryFilter__Group__1"
// $ANTLR start "rule__DictionaryFilter__Group__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14971:1: rule__DictionaryFilter__Group__1__Impl : ( ( rule__DictionaryFilter__NameAssignment_1 ) ) ;
public final void rule__DictionaryFilter__Group__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14975:1: ( ( ( rule__DictionaryFilter__NameAssignment_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14976:1: ( ( rule__DictionaryFilter__NameAssignment_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14976:1: ( ( rule__DictionaryFilter__NameAssignment_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14977:1: ( rule__DictionaryFilter__NameAssignment_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryFilterAccess().getNameAssignment_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14978:1: ( rule__DictionaryFilter__NameAssignment_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14978:2: rule__DictionaryFilter__NameAssignment_1
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryFilter__NameAssignment_1_in_rule__DictionaryFilter__Group__1__Impl30980);
rule__DictionaryFilter__NameAssignment_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryFilterAccess().getNameAssignment_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryFilter__Group__1__Impl"
// $ANTLR start "rule__DictionaryFilter__Group__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14988:1: rule__DictionaryFilter__Group__2 : rule__DictionaryFilter__Group__2__Impl rule__DictionaryFilter__Group__3 ;
public final void rule__DictionaryFilter__Group__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14992:1: ( rule__DictionaryFilter__Group__2__Impl rule__DictionaryFilter__Group__3 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:14993:2: rule__DictionaryFilter__Group__2__Impl rule__DictionaryFilter__Group__3
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryFilter__Group__2__Impl_in_rule__DictionaryFilter__Group__231010);
rule__DictionaryFilter__Group__2__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryFilter__Group__3_in_rule__DictionaryFilter__Group__231013);
rule__DictionaryFilter__Group__3();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryFilter__Group__2"
// $ANTLR start "rule__DictionaryFilter__Group__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15000:1: rule__DictionaryFilter__Group__2__Impl : ( '{' ) ;
public final void rule__DictionaryFilter__Group__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15004:1: ( ( '{' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15005:1: ( '{' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15005:1: ( '{' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15006:1: '{'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryFilterAccess().getLeftCurlyBracketKeyword_2());
}
match(input,67,FollowSets001.FOLLOW_67_in_rule__DictionaryFilter__Group__2__Impl31041); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryFilterAccess().getLeftCurlyBracketKeyword_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryFilter__Group__2__Impl"
// $ANTLR start "rule__DictionaryFilter__Group__3"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15019:1: rule__DictionaryFilter__Group__3 : rule__DictionaryFilter__Group__3__Impl rule__DictionaryFilter__Group__4 ;
public final void rule__DictionaryFilter__Group__3() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15023:1: ( rule__DictionaryFilter__Group__3__Impl rule__DictionaryFilter__Group__4 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15024:2: rule__DictionaryFilter__Group__3__Impl rule__DictionaryFilter__Group__4
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryFilter__Group__3__Impl_in_rule__DictionaryFilter__Group__331072);
rule__DictionaryFilter__Group__3__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryFilter__Group__4_in_rule__DictionaryFilter__Group__331075);
rule__DictionaryFilter__Group__4();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryFilter__Group__3"
// $ANTLR start "rule__DictionaryFilter__Group__3__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15031:1: rule__DictionaryFilter__Group__3__Impl : ( ( rule__DictionaryFilter__LayoutdataAssignment_3 )? ) ;
public final void rule__DictionaryFilter__Group__3__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15035:1: ( ( ( rule__DictionaryFilter__LayoutdataAssignment_3 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15036:1: ( ( rule__DictionaryFilter__LayoutdataAssignment_3 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15036:1: ( ( rule__DictionaryFilter__LayoutdataAssignment_3 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15037:1: ( rule__DictionaryFilter__LayoutdataAssignment_3 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryFilterAccess().getLayoutdataAssignment_3());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15038:1: ( rule__DictionaryFilter__LayoutdataAssignment_3 )?
int alt117=2;
int LA117_0 = input.LA(1);
if ( (LA117_0==110) ) {
alt117=1;
}
switch (alt117) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15038:2: rule__DictionaryFilter__LayoutdataAssignment_3
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryFilter__LayoutdataAssignment_3_in_rule__DictionaryFilter__Group__3__Impl31102);
rule__DictionaryFilter__LayoutdataAssignment_3();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryFilterAccess().getLayoutdataAssignment_3());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryFilter__Group__3__Impl"
// $ANTLR start "rule__DictionaryFilter__Group__4"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15048:1: rule__DictionaryFilter__Group__4 : rule__DictionaryFilter__Group__4__Impl rule__DictionaryFilter__Group__5 ;
public final void rule__DictionaryFilter__Group__4() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15052:1: ( rule__DictionaryFilter__Group__4__Impl rule__DictionaryFilter__Group__5 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15053:2: rule__DictionaryFilter__Group__4__Impl rule__DictionaryFilter__Group__5
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryFilter__Group__4__Impl_in_rule__DictionaryFilter__Group__431133);
rule__DictionaryFilter__Group__4__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryFilter__Group__5_in_rule__DictionaryFilter__Group__431136);
rule__DictionaryFilter__Group__5();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryFilter__Group__4"
// $ANTLR start "rule__DictionaryFilter__Group__4__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15060:1: rule__DictionaryFilter__Group__4__Impl : ( ( rule__DictionaryFilter__LayoutAssignment_4 )? ) ;
public final void rule__DictionaryFilter__Group__4__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15064:1: ( ( ( rule__DictionaryFilter__LayoutAssignment_4 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15065:1: ( ( rule__DictionaryFilter__LayoutAssignment_4 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15065:1: ( ( rule__DictionaryFilter__LayoutAssignment_4 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15066:1: ( rule__DictionaryFilter__LayoutAssignment_4 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryFilterAccess().getLayoutAssignment_4());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15067:1: ( rule__DictionaryFilter__LayoutAssignment_4 )?
int alt118=2;
int LA118_0 = input.LA(1);
if ( (LA118_0==108) ) {
alt118=1;
}
switch (alt118) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15067:2: rule__DictionaryFilter__LayoutAssignment_4
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryFilter__LayoutAssignment_4_in_rule__DictionaryFilter__Group__4__Impl31163);
rule__DictionaryFilter__LayoutAssignment_4();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryFilterAccess().getLayoutAssignment_4());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryFilter__Group__4__Impl"
// $ANTLR start "rule__DictionaryFilter__Group__5"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15077:1: rule__DictionaryFilter__Group__5 : rule__DictionaryFilter__Group__5__Impl rule__DictionaryFilter__Group__6 ;
public final void rule__DictionaryFilter__Group__5() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15081:1: ( rule__DictionaryFilter__Group__5__Impl rule__DictionaryFilter__Group__6 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15082:2: rule__DictionaryFilter__Group__5__Impl rule__DictionaryFilter__Group__6
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryFilter__Group__5__Impl_in_rule__DictionaryFilter__Group__531194);
rule__DictionaryFilter__Group__5__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryFilter__Group__6_in_rule__DictionaryFilter__Group__531197);
rule__DictionaryFilter__Group__6();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryFilter__Group__5"
// $ANTLR start "rule__DictionaryFilter__Group__5__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15089:1: rule__DictionaryFilter__Group__5__Impl : ( ( rule__DictionaryFilter__ContainercontentsAssignment_5 )* ) ;
public final void rule__DictionaryFilter__Group__5__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15093:1: ( ( ( rule__DictionaryFilter__ContainercontentsAssignment_5 )* ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15094:1: ( ( rule__DictionaryFilter__ContainercontentsAssignment_5 )* )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15094:1: ( ( rule__DictionaryFilter__ContainercontentsAssignment_5 )* )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15095:1: ( rule__DictionaryFilter__ContainercontentsAssignment_5 )*
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryFilterAccess().getContainercontentsAssignment_5());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15096:1: ( rule__DictionaryFilter__ContainercontentsAssignment_5 )*
loop119:
do {
int alt119=2;
int LA119_0 = input.LA(1);
if ( ((LA119_0>=112 && LA119_0<=113)||LA119_0==116||LA119_0==125||LA119_0==127||LA119_0==129||(LA119_0>=131 && LA119_0<=136)||LA119_0==138) ) {
alt119=1;
}
switch (alt119) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15096:2: rule__DictionaryFilter__ContainercontentsAssignment_5
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryFilter__ContainercontentsAssignment_5_in_rule__DictionaryFilter__Group__5__Impl31224);
rule__DictionaryFilter__ContainercontentsAssignment_5();
state._fsp--;
if (state.failed) return ;
}
break;
default :
break loop119;
}
} while (true);
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryFilterAccess().getContainercontentsAssignment_5());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryFilter__Group__5__Impl"
// $ANTLR start "rule__DictionaryFilter__Group__6"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15106:1: rule__DictionaryFilter__Group__6 : rule__DictionaryFilter__Group__6__Impl ;
public final void rule__DictionaryFilter__Group__6() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15110:1: ( rule__DictionaryFilter__Group__6__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15111:2: rule__DictionaryFilter__Group__6__Impl
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryFilter__Group__6__Impl_in_rule__DictionaryFilter__Group__631255);
rule__DictionaryFilter__Group__6__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryFilter__Group__6"
// $ANTLR start "rule__DictionaryFilter__Group__6__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15117:1: rule__DictionaryFilter__Group__6__Impl : ( '}' ) ;
public final void rule__DictionaryFilter__Group__6__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15121:1: ( ( '}' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15122:1: ( '}' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15122:1: ( '}' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15123:1: '}'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryFilterAccess().getRightCurlyBracketKeyword_6());
}
match(input,68,FollowSets001.FOLLOW_68_in_rule__DictionaryFilter__Group__6__Impl31283); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryFilterAccess().getRightCurlyBracketKeyword_6());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryFilter__Group__6__Impl"
// $ANTLR start "rule__DictionaryResult__Group__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15150:1: rule__DictionaryResult__Group__0 : rule__DictionaryResult__Group__0__Impl rule__DictionaryResult__Group__1 ;
public final void rule__DictionaryResult__Group__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15154:1: ( rule__DictionaryResult__Group__0__Impl rule__DictionaryResult__Group__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15155:2: rule__DictionaryResult__Group__0__Impl rule__DictionaryResult__Group__1
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryResult__Group__0__Impl_in_rule__DictionaryResult__Group__031328);
rule__DictionaryResult__Group__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryResult__Group__1_in_rule__DictionaryResult__Group__031331);
rule__DictionaryResult__Group__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryResult__Group__0"
// $ANTLR start "rule__DictionaryResult__Group__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15162:1: rule__DictionaryResult__Group__0__Impl : ( 'dictionaryresult' ) ;
public final void rule__DictionaryResult__Group__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15166:1: ( ( 'dictionaryresult' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15167:1: ( 'dictionaryresult' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15167:1: ( 'dictionaryresult' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15168:1: 'dictionaryresult'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryResultAccess().getDictionaryresultKeyword_0());
}
match(input,107,FollowSets001.FOLLOW_107_in_rule__DictionaryResult__Group__0__Impl31359); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryResultAccess().getDictionaryresultKeyword_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryResult__Group__0__Impl"
// $ANTLR start "rule__DictionaryResult__Group__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15181:1: rule__DictionaryResult__Group__1 : rule__DictionaryResult__Group__1__Impl rule__DictionaryResult__Group__2 ;
public final void rule__DictionaryResult__Group__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15185:1: ( rule__DictionaryResult__Group__1__Impl rule__DictionaryResult__Group__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15186:2: rule__DictionaryResult__Group__1__Impl rule__DictionaryResult__Group__2
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryResult__Group__1__Impl_in_rule__DictionaryResult__Group__131390);
rule__DictionaryResult__Group__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryResult__Group__2_in_rule__DictionaryResult__Group__131393);
rule__DictionaryResult__Group__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryResult__Group__1"
// $ANTLR start "rule__DictionaryResult__Group__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15193:1: rule__DictionaryResult__Group__1__Impl : ( ( rule__DictionaryResult__NameAssignment_1 ) ) ;
public final void rule__DictionaryResult__Group__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15197:1: ( ( ( rule__DictionaryResult__NameAssignment_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15198:1: ( ( rule__DictionaryResult__NameAssignment_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15198:1: ( ( rule__DictionaryResult__NameAssignment_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15199:1: ( rule__DictionaryResult__NameAssignment_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryResultAccess().getNameAssignment_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15200:1: ( rule__DictionaryResult__NameAssignment_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15200:2: rule__DictionaryResult__NameAssignment_1
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryResult__NameAssignment_1_in_rule__DictionaryResult__Group__1__Impl31420);
rule__DictionaryResult__NameAssignment_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryResultAccess().getNameAssignment_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryResult__Group__1__Impl"
// $ANTLR start "rule__DictionaryResult__Group__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15210:1: rule__DictionaryResult__Group__2 : rule__DictionaryResult__Group__2__Impl rule__DictionaryResult__Group__3 ;
public final void rule__DictionaryResult__Group__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15214:1: ( rule__DictionaryResult__Group__2__Impl rule__DictionaryResult__Group__3 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15215:2: rule__DictionaryResult__Group__2__Impl rule__DictionaryResult__Group__3
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryResult__Group__2__Impl_in_rule__DictionaryResult__Group__231450);
rule__DictionaryResult__Group__2__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryResult__Group__3_in_rule__DictionaryResult__Group__231453);
rule__DictionaryResult__Group__3();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryResult__Group__2"
// $ANTLR start "rule__DictionaryResult__Group__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15222:1: rule__DictionaryResult__Group__2__Impl : ( '{' ) ;
public final void rule__DictionaryResult__Group__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15226:1: ( ( '{' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15227:1: ( '{' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15227:1: ( '{' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15228:1: '{'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryResultAccess().getLeftCurlyBracketKeyword_2());
}
match(input,67,FollowSets001.FOLLOW_67_in_rule__DictionaryResult__Group__2__Impl31481); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryResultAccess().getLeftCurlyBracketKeyword_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryResult__Group__2__Impl"
// $ANTLR start "rule__DictionaryResult__Group__3"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15241:1: rule__DictionaryResult__Group__3 : rule__DictionaryResult__Group__3__Impl rule__DictionaryResult__Group__4 ;
public final void rule__DictionaryResult__Group__3() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15245:1: ( rule__DictionaryResult__Group__3__Impl rule__DictionaryResult__Group__4 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15246:2: rule__DictionaryResult__Group__3__Impl rule__DictionaryResult__Group__4
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryResult__Group__3__Impl_in_rule__DictionaryResult__Group__331512);
rule__DictionaryResult__Group__3__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryResult__Group__4_in_rule__DictionaryResult__Group__331515);
rule__DictionaryResult__Group__4();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryResult__Group__3"
// $ANTLR start "rule__DictionaryResult__Group__3__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15253:1: rule__DictionaryResult__Group__3__Impl : ( ( rule__DictionaryResult__ResultcolumnsAssignment_3 )* ) ;
public final void rule__DictionaryResult__Group__3__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15257:1: ( ( ( rule__DictionaryResult__ResultcolumnsAssignment_3 )* ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15258:1: ( ( rule__DictionaryResult__ResultcolumnsAssignment_3 )* )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15258:1: ( ( rule__DictionaryResult__ResultcolumnsAssignment_3 )* )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15259:1: ( rule__DictionaryResult__ResultcolumnsAssignment_3 )*
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryResultAccess().getResultcolumnsAssignment_3());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15260:1: ( rule__DictionaryResult__ResultcolumnsAssignment_3 )*
loop120:
do {
int alt120=2;
int LA120_0 = input.LA(1);
if ( (LA120_0==125||LA120_0==127||LA120_0==129||(LA120_0>=131 && LA120_0<=136)||LA120_0==138) ) {
alt120=1;
}
switch (alt120) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15260:2: rule__DictionaryResult__ResultcolumnsAssignment_3
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryResult__ResultcolumnsAssignment_3_in_rule__DictionaryResult__Group__3__Impl31542);
rule__DictionaryResult__ResultcolumnsAssignment_3();
state._fsp--;
if (state.failed) return ;
}
break;
default :
break loop120;
}
} while (true);
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryResultAccess().getResultcolumnsAssignment_3());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryResult__Group__3__Impl"
// $ANTLR start "rule__DictionaryResult__Group__4"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15270:1: rule__DictionaryResult__Group__4 : rule__DictionaryResult__Group__4__Impl ;
public final void rule__DictionaryResult__Group__4() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15274:1: ( rule__DictionaryResult__Group__4__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15275:2: rule__DictionaryResult__Group__4__Impl
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryResult__Group__4__Impl_in_rule__DictionaryResult__Group__431573);
rule__DictionaryResult__Group__4__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryResult__Group__4"
// $ANTLR start "rule__DictionaryResult__Group__4__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15281:1: rule__DictionaryResult__Group__4__Impl : ( '}' ) ;
public final void rule__DictionaryResult__Group__4__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15285:1: ( ( '}' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15286:1: ( '}' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15286:1: ( '}' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15287:1: '}'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryResultAccess().getRightCurlyBracketKeyword_4());
}
match(input,68,FollowSets001.FOLLOW_68_in_rule__DictionaryResult__Group__4__Impl31601); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryResultAccess().getRightCurlyBracketKeyword_4());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryResult__Group__4__Impl"
// $ANTLR start "rule__ColumnLayout__Group__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15310:1: rule__ColumnLayout__Group__0 : rule__ColumnLayout__Group__0__Impl rule__ColumnLayout__Group__1 ;
public final void rule__ColumnLayout__Group__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15314:1: ( rule__ColumnLayout__Group__0__Impl rule__ColumnLayout__Group__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15315:2: rule__ColumnLayout__Group__0__Impl rule__ColumnLayout__Group__1
{
pushFollow(FollowSets001.FOLLOW_rule__ColumnLayout__Group__0__Impl_in_rule__ColumnLayout__Group__031642);
rule__ColumnLayout__Group__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__ColumnLayout__Group__1_in_rule__ColumnLayout__Group__031645);
rule__ColumnLayout__Group__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ColumnLayout__Group__0"
// $ANTLR start "rule__ColumnLayout__Group__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15322:1: rule__ColumnLayout__Group__0__Impl : ( 'layout' ) ;
public final void rule__ColumnLayout__Group__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15326:1: ( ( 'layout' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15327:1: ( 'layout' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15327:1: ( 'layout' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15328:1: 'layout'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getColumnLayoutAccess().getLayoutKeyword_0());
}
match(input,108,FollowSets001.FOLLOW_108_in_rule__ColumnLayout__Group__0__Impl31673); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getColumnLayoutAccess().getLayoutKeyword_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ColumnLayout__Group__0__Impl"
// $ANTLR start "rule__ColumnLayout__Group__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15341:1: rule__ColumnLayout__Group__1 : rule__ColumnLayout__Group__1__Impl rule__ColumnLayout__Group__2 ;
public final void rule__ColumnLayout__Group__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15345:1: ( rule__ColumnLayout__Group__1__Impl rule__ColumnLayout__Group__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15346:2: rule__ColumnLayout__Group__1__Impl rule__ColumnLayout__Group__2
{
pushFollow(FollowSets001.FOLLOW_rule__ColumnLayout__Group__1__Impl_in_rule__ColumnLayout__Group__131704);
rule__ColumnLayout__Group__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__ColumnLayout__Group__2_in_rule__ColumnLayout__Group__131707);
rule__ColumnLayout__Group__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ColumnLayout__Group__1"
// $ANTLR start "rule__ColumnLayout__Group__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15353:1: rule__ColumnLayout__Group__1__Impl : ( '{' ) ;
public final void rule__ColumnLayout__Group__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15357:1: ( ( '{' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15358:1: ( '{' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15358:1: ( '{' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15359:1: '{'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getColumnLayoutAccess().getLeftCurlyBracketKeyword_1());
}
match(input,67,FollowSets001.FOLLOW_67_in_rule__ColumnLayout__Group__1__Impl31735); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getColumnLayoutAccess().getLeftCurlyBracketKeyword_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ColumnLayout__Group__1__Impl"
// $ANTLR start "rule__ColumnLayout__Group__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15372:1: rule__ColumnLayout__Group__2 : rule__ColumnLayout__Group__2__Impl rule__ColumnLayout__Group__3 ;
public final void rule__ColumnLayout__Group__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15376:1: ( rule__ColumnLayout__Group__2__Impl rule__ColumnLayout__Group__3 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15377:2: rule__ColumnLayout__Group__2__Impl rule__ColumnLayout__Group__3
{
pushFollow(FollowSets001.FOLLOW_rule__ColumnLayout__Group__2__Impl_in_rule__ColumnLayout__Group__231766);
rule__ColumnLayout__Group__2__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__ColumnLayout__Group__3_in_rule__ColumnLayout__Group__231769);
rule__ColumnLayout__Group__3();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ColumnLayout__Group__2"
// $ANTLR start "rule__ColumnLayout__Group__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15384:1: rule__ColumnLayout__Group__2__Impl : ( 'columns' ) ;
public final void rule__ColumnLayout__Group__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15388:1: ( ( 'columns' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15389:1: ( 'columns' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15389:1: ( 'columns' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15390:1: 'columns'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getColumnLayoutAccess().getColumnsKeyword_2());
}
match(input,109,FollowSets001.FOLLOW_109_in_rule__ColumnLayout__Group__2__Impl31797); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getColumnLayoutAccess().getColumnsKeyword_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ColumnLayout__Group__2__Impl"
// $ANTLR start "rule__ColumnLayout__Group__3"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15403:1: rule__ColumnLayout__Group__3 : rule__ColumnLayout__Group__3__Impl rule__ColumnLayout__Group__4 ;
public final void rule__ColumnLayout__Group__3() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15407:1: ( rule__ColumnLayout__Group__3__Impl rule__ColumnLayout__Group__4 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15408:2: rule__ColumnLayout__Group__3__Impl rule__ColumnLayout__Group__4
{
pushFollow(FollowSets001.FOLLOW_rule__ColumnLayout__Group__3__Impl_in_rule__ColumnLayout__Group__331828);
rule__ColumnLayout__Group__3__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__ColumnLayout__Group__4_in_rule__ColumnLayout__Group__331831);
rule__ColumnLayout__Group__4();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ColumnLayout__Group__3"
// $ANTLR start "rule__ColumnLayout__Group__3__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15415:1: rule__ColumnLayout__Group__3__Impl : ( ( rule__ColumnLayout__ColumnsAssignment_3 ) ) ;
public final void rule__ColumnLayout__Group__3__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15419:1: ( ( ( rule__ColumnLayout__ColumnsAssignment_3 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15420:1: ( ( rule__ColumnLayout__ColumnsAssignment_3 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15420:1: ( ( rule__ColumnLayout__ColumnsAssignment_3 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15421:1: ( rule__ColumnLayout__ColumnsAssignment_3 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getColumnLayoutAccess().getColumnsAssignment_3());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15422:1: ( rule__ColumnLayout__ColumnsAssignment_3 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15422:2: rule__ColumnLayout__ColumnsAssignment_3
{
pushFollow(FollowSets001.FOLLOW_rule__ColumnLayout__ColumnsAssignment_3_in_rule__ColumnLayout__Group__3__Impl31858);
rule__ColumnLayout__ColumnsAssignment_3();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getColumnLayoutAccess().getColumnsAssignment_3());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ColumnLayout__Group__3__Impl"
// $ANTLR start "rule__ColumnLayout__Group__4"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15432:1: rule__ColumnLayout__Group__4 : rule__ColumnLayout__Group__4__Impl ;
public final void rule__ColumnLayout__Group__4() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15436:1: ( rule__ColumnLayout__Group__4__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15437:2: rule__ColumnLayout__Group__4__Impl
{
pushFollow(FollowSets001.FOLLOW_rule__ColumnLayout__Group__4__Impl_in_rule__ColumnLayout__Group__431888);
rule__ColumnLayout__Group__4__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ColumnLayout__Group__4"
// $ANTLR start "rule__ColumnLayout__Group__4__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15443:1: rule__ColumnLayout__Group__4__Impl : ( '}' ) ;
public final void rule__ColumnLayout__Group__4__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15447:1: ( ( '}' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15448:1: ( '}' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15448:1: ( '}' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15449:1: '}'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getColumnLayoutAccess().getRightCurlyBracketKeyword_4());
}
match(input,68,FollowSets001.FOLLOW_68_in_rule__ColumnLayout__Group__4__Impl31916); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getColumnLayoutAccess().getRightCurlyBracketKeyword_4());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ColumnLayout__Group__4__Impl"
// $ANTLR start "rule__ColumnLayoutData__Group__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15472:1: rule__ColumnLayoutData__Group__0 : rule__ColumnLayoutData__Group__0__Impl rule__ColumnLayoutData__Group__1 ;
public final void rule__ColumnLayoutData__Group__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15476:1: ( rule__ColumnLayoutData__Group__0__Impl rule__ColumnLayoutData__Group__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15477:2: rule__ColumnLayoutData__Group__0__Impl rule__ColumnLayoutData__Group__1
{
pushFollow(FollowSets001.FOLLOW_rule__ColumnLayoutData__Group__0__Impl_in_rule__ColumnLayoutData__Group__031957);
rule__ColumnLayoutData__Group__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__ColumnLayoutData__Group__1_in_rule__ColumnLayoutData__Group__031960);
rule__ColumnLayoutData__Group__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ColumnLayoutData__Group__0"
// $ANTLR start "rule__ColumnLayoutData__Group__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15484:1: rule__ColumnLayoutData__Group__0__Impl : ( 'layoutdata' ) ;
public final void rule__ColumnLayoutData__Group__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15488:1: ( ( 'layoutdata' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15489:1: ( 'layoutdata' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15489:1: ( 'layoutdata' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15490:1: 'layoutdata'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getColumnLayoutDataAccess().getLayoutdataKeyword_0());
}
match(input,110,FollowSets001.FOLLOW_110_in_rule__ColumnLayoutData__Group__0__Impl31988); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getColumnLayoutDataAccess().getLayoutdataKeyword_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ColumnLayoutData__Group__0__Impl"
// $ANTLR start "rule__ColumnLayoutData__Group__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15503:1: rule__ColumnLayoutData__Group__1 : rule__ColumnLayoutData__Group__1__Impl rule__ColumnLayoutData__Group__2 ;
public final void rule__ColumnLayoutData__Group__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15507:1: ( rule__ColumnLayoutData__Group__1__Impl rule__ColumnLayoutData__Group__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15508:2: rule__ColumnLayoutData__Group__1__Impl rule__ColumnLayoutData__Group__2
{
pushFollow(FollowSets001.FOLLOW_rule__ColumnLayoutData__Group__1__Impl_in_rule__ColumnLayoutData__Group__132019);
rule__ColumnLayoutData__Group__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__ColumnLayoutData__Group__2_in_rule__ColumnLayoutData__Group__132022);
rule__ColumnLayoutData__Group__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ColumnLayoutData__Group__1"
// $ANTLR start "rule__ColumnLayoutData__Group__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15515:1: rule__ColumnLayoutData__Group__1__Impl : ( '{' ) ;
public final void rule__ColumnLayoutData__Group__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15519:1: ( ( '{' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15520:1: ( '{' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15520:1: ( '{' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15521:1: '{'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getColumnLayoutDataAccess().getLeftCurlyBracketKeyword_1());
}
match(input,67,FollowSets001.FOLLOW_67_in_rule__ColumnLayoutData__Group__1__Impl32050); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getColumnLayoutDataAccess().getLeftCurlyBracketKeyword_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ColumnLayoutData__Group__1__Impl"
// $ANTLR start "rule__ColumnLayoutData__Group__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15534:1: rule__ColumnLayoutData__Group__2 : rule__ColumnLayoutData__Group__2__Impl rule__ColumnLayoutData__Group__3 ;
public final void rule__ColumnLayoutData__Group__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15538:1: ( rule__ColumnLayoutData__Group__2__Impl rule__ColumnLayoutData__Group__3 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15539:2: rule__ColumnLayoutData__Group__2__Impl rule__ColumnLayoutData__Group__3
{
pushFollow(FollowSets001.FOLLOW_rule__ColumnLayoutData__Group__2__Impl_in_rule__ColumnLayoutData__Group__232081);
rule__ColumnLayoutData__Group__2__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__ColumnLayoutData__Group__3_in_rule__ColumnLayoutData__Group__232084);
rule__ColumnLayoutData__Group__3();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ColumnLayoutData__Group__2"
// $ANTLR start "rule__ColumnLayoutData__Group__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15546:1: rule__ColumnLayoutData__Group__2__Impl : ( 'columnspan' ) ;
public final void rule__ColumnLayoutData__Group__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15550:1: ( ( 'columnspan' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15551:1: ( 'columnspan' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15551:1: ( 'columnspan' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15552:1: 'columnspan'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getColumnLayoutDataAccess().getColumnspanKeyword_2());
}
match(input,111,FollowSets001.FOLLOW_111_in_rule__ColumnLayoutData__Group__2__Impl32112); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getColumnLayoutDataAccess().getColumnspanKeyword_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ColumnLayoutData__Group__2__Impl"
// $ANTLR start "rule__ColumnLayoutData__Group__3"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15565:1: rule__ColumnLayoutData__Group__3 : rule__ColumnLayoutData__Group__3__Impl rule__ColumnLayoutData__Group__4 ;
public final void rule__ColumnLayoutData__Group__3() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15569:1: ( rule__ColumnLayoutData__Group__3__Impl rule__ColumnLayoutData__Group__4 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15570:2: rule__ColumnLayoutData__Group__3__Impl rule__ColumnLayoutData__Group__4
{
pushFollow(FollowSets001.FOLLOW_rule__ColumnLayoutData__Group__3__Impl_in_rule__ColumnLayoutData__Group__332143);
rule__ColumnLayoutData__Group__3__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__ColumnLayoutData__Group__4_in_rule__ColumnLayoutData__Group__332146);
rule__ColumnLayoutData__Group__4();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ColumnLayoutData__Group__3"
// $ANTLR start "rule__ColumnLayoutData__Group__3__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15577:1: rule__ColumnLayoutData__Group__3__Impl : ( ( rule__ColumnLayoutData__ColumnspanAssignment_3 ) ) ;
public final void rule__ColumnLayoutData__Group__3__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15581:1: ( ( ( rule__ColumnLayoutData__ColumnspanAssignment_3 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15582:1: ( ( rule__ColumnLayoutData__ColumnspanAssignment_3 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15582:1: ( ( rule__ColumnLayoutData__ColumnspanAssignment_3 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15583:1: ( rule__ColumnLayoutData__ColumnspanAssignment_3 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getColumnLayoutDataAccess().getColumnspanAssignment_3());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15584:1: ( rule__ColumnLayoutData__ColumnspanAssignment_3 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15584:2: rule__ColumnLayoutData__ColumnspanAssignment_3
{
pushFollow(FollowSets001.FOLLOW_rule__ColumnLayoutData__ColumnspanAssignment_3_in_rule__ColumnLayoutData__Group__3__Impl32173);
rule__ColumnLayoutData__ColumnspanAssignment_3();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getColumnLayoutDataAccess().getColumnspanAssignment_3());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ColumnLayoutData__Group__3__Impl"
// $ANTLR start "rule__ColumnLayoutData__Group__4"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15594:1: rule__ColumnLayoutData__Group__4 : rule__ColumnLayoutData__Group__4__Impl ;
public final void rule__ColumnLayoutData__Group__4() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15598:1: ( rule__ColumnLayoutData__Group__4__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15599:2: rule__ColumnLayoutData__Group__4__Impl
{
pushFollow(FollowSets001.FOLLOW_rule__ColumnLayoutData__Group__4__Impl_in_rule__ColumnLayoutData__Group__432203);
rule__ColumnLayoutData__Group__4__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ColumnLayoutData__Group__4"
// $ANTLR start "rule__ColumnLayoutData__Group__4__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15605:1: rule__ColumnLayoutData__Group__4__Impl : ( '}' ) ;
public final void rule__ColumnLayoutData__Group__4__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15609:1: ( ( '}' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15610:1: ( '}' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15610:1: ( '}' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15611:1: '}'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getColumnLayoutDataAccess().getRightCurlyBracketKeyword_4());
}
match(input,68,FollowSets001.FOLLOW_68_in_rule__ColumnLayoutData__Group__4__Impl32231); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getColumnLayoutDataAccess().getRightCurlyBracketKeyword_4());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ColumnLayoutData__Group__4__Impl"
// $ANTLR start "rule__DictionaryComposite__Group__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15634:1: rule__DictionaryComposite__Group__0 : rule__DictionaryComposite__Group__0__Impl rule__DictionaryComposite__Group__1 ;
public final void rule__DictionaryComposite__Group__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15638:1: ( rule__DictionaryComposite__Group__0__Impl rule__DictionaryComposite__Group__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15639:2: rule__DictionaryComposite__Group__0__Impl rule__DictionaryComposite__Group__1
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryComposite__Group__0__Impl_in_rule__DictionaryComposite__Group__032272);
rule__DictionaryComposite__Group__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryComposite__Group__1_in_rule__DictionaryComposite__Group__032275);
rule__DictionaryComposite__Group__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryComposite__Group__0"
// $ANTLR start "rule__DictionaryComposite__Group__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15646:1: rule__DictionaryComposite__Group__0__Impl : ( 'composite' ) ;
public final void rule__DictionaryComposite__Group__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15650:1: ( ( 'composite' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15651:1: ( 'composite' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15651:1: ( 'composite' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15652:1: 'composite'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryCompositeAccess().getCompositeKeyword_0());
}
match(input,112,FollowSets001.FOLLOW_112_in_rule__DictionaryComposite__Group__0__Impl32303); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryCompositeAccess().getCompositeKeyword_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryComposite__Group__0__Impl"
// $ANTLR start "rule__DictionaryComposite__Group__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15665:1: rule__DictionaryComposite__Group__1 : rule__DictionaryComposite__Group__1__Impl rule__DictionaryComposite__Group__2 ;
public final void rule__DictionaryComposite__Group__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15669:1: ( rule__DictionaryComposite__Group__1__Impl rule__DictionaryComposite__Group__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15670:2: rule__DictionaryComposite__Group__1__Impl rule__DictionaryComposite__Group__2
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryComposite__Group__1__Impl_in_rule__DictionaryComposite__Group__132334);
rule__DictionaryComposite__Group__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryComposite__Group__2_in_rule__DictionaryComposite__Group__132337);
rule__DictionaryComposite__Group__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryComposite__Group__1"
// $ANTLR start "rule__DictionaryComposite__Group__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15677:1: rule__DictionaryComposite__Group__1__Impl : ( ( rule__DictionaryComposite__NameAssignment_1 ) ) ;
public final void rule__DictionaryComposite__Group__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15681:1: ( ( ( rule__DictionaryComposite__NameAssignment_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15682:1: ( ( rule__DictionaryComposite__NameAssignment_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15682:1: ( ( rule__DictionaryComposite__NameAssignment_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15683:1: ( rule__DictionaryComposite__NameAssignment_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryCompositeAccess().getNameAssignment_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15684:1: ( rule__DictionaryComposite__NameAssignment_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15684:2: rule__DictionaryComposite__NameAssignment_1
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryComposite__NameAssignment_1_in_rule__DictionaryComposite__Group__1__Impl32364);
rule__DictionaryComposite__NameAssignment_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryCompositeAccess().getNameAssignment_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryComposite__Group__1__Impl"
// $ANTLR start "rule__DictionaryComposite__Group__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15694:1: rule__DictionaryComposite__Group__2 : rule__DictionaryComposite__Group__2__Impl rule__DictionaryComposite__Group__3 ;
public final void rule__DictionaryComposite__Group__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15698:1: ( rule__DictionaryComposite__Group__2__Impl rule__DictionaryComposite__Group__3 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15699:2: rule__DictionaryComposite__Group__2__Impl rule__DictionaryComposite__Group__3
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryComposite__Group__2__Impl_in_rule__DictionaryComposite__Group__232394);
rule__DictionaryComposite__Group__2__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryComposite__Group__3_in_rule__DictionaryComposite__Group__232397);
rule__DictionaryComposite__Group__3();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryComposite__Group__2"
// $ANTLR start "rule__DictionaryComposite__Group__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15706:1: rule__DictionaryComposite__Group__2__Impl : ( '{' ) ;
public final void rule__DictionaryComposite__Group__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15710:1: ( ( '{' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15711:1: ( '{' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15711:1: ( '{' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15712:1: '{'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryCompositeAccess().getLeftCurlyBracketKeyword_2());
}
match(input,67,FollowSets001.FOLLOW_67_in_rule__DictionaryComposite__Group__2__Impl32425); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryCompositeAccess().getLeftCurlyBracketKeyword_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryComposite__Group__2__Impl"
// $ANTLR start "rule__DictionaryComposite__Group__3"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15725:1: rule__DictionaryComposite__Group__3 : rule__DictionaryComposite__Group__3__Impl rule__DictionaryComposite__Group__4 ;
public final void rule__DictionaryComposite__Group__3() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15729:1: ( rule__DictionaryComposite__Group__3__Impl rule__DictionaryComposite__Group__4 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15730:2: rule__DictionaryComposite__Group__3__Impl rule__DictionaryComposite__Group__4
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryComposite__Group__3__Impl_in_rule__DictionaryComposite__Group__332456);
rule__DictionaryComposite__Group__3__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryComposite__Group__4_in_rule__DictionaryComposite__Group__332459);
rule__DictionaryComposite__Group__4();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryComposite__Group__3"
// $ANTLR start "rule__DictionaryComposite__Group__3__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15737:1: rule__DictionaryComposite__Group__3__Impl : ( ( rule__DictionaryComposite__LayoutdataAssignment_3 )? ) ;
public final void rule__DictionaryComposite__Group__3__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15741:1: ( ( ( rule__DictionaryComposite__LayoutdataAssignment_3 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15742:1: ( ( rule__DictionaryComposite__LayoutdataAssignment_3 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15742:1: ( ( rule__DictionaryComposite__LayoutdataAssignment_3 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15743:1: ( rule__DictionaryComposite__LayoutdataAssignment_3 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryCompositeAccess().getLayoutdataAssignment_3());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15744:1: ( rule__DictionaryComposite__LayoutdataAssignment_3 )?
int alt121=2;
int LA121_0 = input.LA(1);
if ( (LA121_0==110) ) {
alt121=1;
}
switch (alt121) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15744:2: rule__DictionaryComposite__LayoutdataAssignment_3
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryComposite__LayoutdataAssignment_3_in_rule__DictionaryComposite__Group__3__Impl32486);
rule__DictionaryComposite__LayoutdataAssignment_3();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryCompositeAccess().getLayoutdataAssignment_3());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryComposite__Group__3__Impl"
// $ANTLR start "rule__DictionaryComposite__Group__4"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15754:1: rule__DictionaryComposite__Group__4 : rule__DictionaryComposite__Group__4__Impl rule__DictionaryComposite__Group__5 ;
public final void rule__DictionaryComposite__Group__4() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15758:1: ( rule__DictionaryComposite__Group__4__Impl rule__DictionaryComposite__Group__5 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15759:2: rule__DictionaryComposite__Group__4__Impl rule__DictionaryComposite__Group__5
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryComposite__Group__4__Impl_in_rule__DictionaryComposite__Group__432517);
rule__DictionaryComposite__Group__4__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryComposite__Group__5_in_rule__DictionaryComposite__Group__432520);
rule__DictionaryComposite__Group__5();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryComposite__Group__4"
// $ANTLR start "rule__DictionaryComposite__Group__4__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15766:1: rule__DictionaryComposite__Group__4__Impl : ( ( rule__DictionaryComposite__LayoutAssignment_4 )? ) ;
public final void rule__DictionaryComposite__Group__4__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15770:1: ( ( ( rule__DictionaryComposite__LayoutAssignment_4 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15771:1: ( ( rule__DictionaryComposite__LayoutAssignment_4 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15771:1: ( ( rule__DictionaryComposite__LayoutAssignment_4 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15772:1: ( rule__DictionaryComposite__LayoutAssignment_4 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryCompositeAccess().getLayoutAssignment_4());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15773:1: ( rule__DictionaryComposite__LayoutAssignment_4 )?
int alt122=2;
int LA122_0 = input.LA(1);
if ( (LA122_0==108) ) {
alt122=1;
}
switch (alt122) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15773:2: rule__DictionaryComposite__LayoutAssignment_4
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryComposite__LayoutAssignment_4_in_rule__DictionaryComposite__Group__4__Impl32547);
rule__DictionaryComposite__LayoutAssignment_4();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryCompositeAccess().getLayoutAssignment_4());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryComposite__Group__4__Impl"
// $ANTLR start "rule__DictionaryComposite__Group__5"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15783:1: rule__DictionaryComposite__Group__5 : rule__DictionaryComposite__Group__5__Impl rule__DictionaryComposite__Group__6 ;
public final void rule__DictionaryComposite__Group__5() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15787:1: ( rule__DictionaryComposite__Group__5__Impl rule__DictionaryComposite__Group__6 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15788:2: rule__DictionaryComposite__Group__5__Impl rule__DictionaryComposite__Group__6
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryComposite__Group__5__Impl_in_rule__DictionaryComposite__Group__532578);
rule__DictionaryComposite__Group__5__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryComposite__Group__6_in_rule__DictionaryComposite__Group__532581);
rule__DictionaryComposite__Group__6();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryComposite__Group__5"
// $ANTLR start "rule__DictionaryComposite__Group__5__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15795:1: rule__DictionaryComposite__Group__5__Impl : ( ( rule__DictionaryComposite__ContainercontentsAssignment_5 )* ) ;
public final void rule__DictionaryComposite__Group__5__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15799:1: ( ( ( rule__DictionaryComposite__ContainercontentsAssignment_5 )* ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15800:1: ( ( rule__DictionaryComposite__ContainercontentsAssignment_5 )* )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15800:1: ( ( rule__DictionaryComposite__ContainercontentsAssignment_5 )* )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15801:1: ( rule__DictionaryComposite__ContainercontentsAssignment_5 )*
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryCompositeAccess().getContainercontentsAssignment_5());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15802:1: ( rule__DictionaryComposite__ContainercontentsAssignment_5 )*
loop123:
do {
int alt123=2;
int LA123_0 = input.LA(1);
if ( ((LA123_0>=112 && LA123_0<=113)||LA123_0==116||LA123_0==125||LA123_0==127||LA123_0==129||(LA123_0>=131 && LA123_0<=136)||LA123_0==138) ) {
alt123=1;
}
switch (alt123) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15802:2: rule__DictionaryComposite__ContainercontentsAssignment_5
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryComposite__ContainercontentsAssignment_5_in_rule__DictionaryComposite__Group__5__Impl32608);
rule__DictionaryComposite__ContainercontentsAssignment_5();
state._fsp--;
if (state.failed) return ;
}
break;
default :
break loop123;
}
} while (true);
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryCompositeAccess().getContainercontentsAssignment_5());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryComposite__Group__5__Impl"
// $ANTLR start "rule__DictionaryComposite__Group__6"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15812:1: rule__DictionaryComposite__Group__6 : rule__DictionaryComposite__Group__6__Impl ;
public final void rule__DictionaryComposite__Group__6() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15816:1: ( rule__DictionaryComposite__Group__6__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15817:2: rule__DictionaryComposite__Group__6__Impl
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryComposite__Group__6__Impl_in_rule__DictionaryComposite__Group__632639);
rule__DictionaryComposite__Group__6__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryComposite__Group__6"
// $ANTLR start "rule__DictionaryComposite__Group__6__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15823:1: rule__DictionaryComposite__Group__6__Impl : ( '}' ) ;
public final void rule__DictionaryComposite__Group__6__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15827:1: ( ( '}' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15828:1: ( '}' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15828:1: ( '}' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15829:1: '}'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryCompositeAccess().getRightCurlyBracketKeyword_6());
}
match(input,68,FollowSets001.FOLLOW_68_in_rule__DictionaryComposite__Group__6__Impl32667); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryCompositeAccess().getRightCurlyBracketKeyword_6());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryComposite__Group__6__Impl"
// $ANTLR start "rule__DictionaryEditableTable__Group__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15856:1: rule__DictionaryEditableTable__Group__0 : rule__DictionaryEditableTable__Group__0__Impl rule__DictionaryEditableTable__Group__1 ;
public final void rule__DictionaryEditableTable__Group__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15860:1: ( rule__DictionaryEditableTable__Group__0__Impl rule__DictionaryEditableTable__Group__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15861:2: rule__DictionaryEditableTable__Group__0__Impl rule__DictionaryEditableTable__Group__1
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryEditableTable__Group__0__Impl_in_rule__DictionaryEditableTable__Group__032712);
rule__DictionaryEditableTable__Group__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryEditableTable__Group__1_in_rule__DictionaryEditableTable__Group__032715);
rule__DictionaryEditableTable__Group__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryEditableTable__Group__0"
// $ANTLR start "rule__DictionaryEditableTable__Group__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15868:1: rule__DictionaryEditableTable__Group__0__Impl : ( 'editabletable' ) ;
public final void rule__DictionaryEditableTable__Group__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15872:1: ( ( 'editabletable' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15873:1: ( 'editabletable' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15873:1: ( 'editabletable' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15874:1: 'editabletable'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryEditableTableAccess().getEditabletableKeyword_0());
}
match(input,113,FollowSets001.FOLLOW_113_in_rule__DictionaryEditableTable__Group__0__Impl32743); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryEditableTableAccess().getEditabletableKeyword_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryEditableTable__Group__0__Impl"
// $ANTLR start "rule__DictionaryEditableTable__Group__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15887:1: rule__DictionaryEditableTable__Group__1 : rule__DictionaryEditableTable__Group__1__Impl rule__DictionaryEditableTable__Group__2 ;
public final void rule__DictionaryEditableTable__Group__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15891:1: ( rule__DictionaryEditableTable__Group__1__Impl rule__DictionaryEditableTable__Group__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15892:2: rule__DictionaryEditableTable__Group__1__Impl rule__DictionaryEditableTable__Group__2
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryEditableTable__Group__1__Impl_in_rule__DictionaryEditableTable__Group__132774);
rule__DictionaryEditableTable__Group__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryEditableTable__Group__2_in_rule__DictionaryEditableTable__Group__132777);
rule__DictionaryEditableTable__Group__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryEditableTable__Group__1"
// $ANTLR start "rule__DictionaryEditableTable__Group__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15899:1: rule__DictionaryEditableTable__Group__1__Impl : ( ( rule__DictionaryEditableTable__NameAssignment_1 ) ) ;
public final void rule__DictionaryEditableTable__Group__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15903:1: ( ( ( rule__DictionaryEditableTable__NameAssignment_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15904:1: ( ( rule__DictionaryEditableTable__NameAssignment_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15904:1: ( ( rule__DictionaryEditableTable__NameAssignment_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15905:1: ( rule__DictionaryEditableTable__NameAssignment_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryEditableTableAccess().getNameAssignment_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15906:1: ( rule__DictionaryEditableTable__NameAssignment_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15906:2: rule__DictionaryEditableTable__NameAssignment_1
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryEditableTable__NameAssignment_1_in_rule__DictionaryEditableTable__Group__1__Impl32804);
rule__DictionaryEditableTable__NameAssignment_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryEditableTableAccess().getNameAssignment_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryEditableTable__Group__1__Impl"
// $ANTLR start "rule__DictionaryEditableTable__Group__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15916:1: rule__DictionaryEditableTable__Group__2 : rule__DictionaryEditableTable__Group__2__Impl rule__DictionaryEditableTable__Group__3 ;
public final void rule__DictionaryEditableTable__Group__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15920:1: ( rule__DictionaryEditableTable__Group__2__Impl rule__DictionaryEditableTable__Group__3 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15921:2: rule__DictionaryEditableTable__Group__2__Impl rule__DictionaryEditableTable__Group__3
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryEditableTable__Group__2__Impl_in_rule__DictionaryEditableTable__Group__232834);
rule__DictionaryEditableTable__Group__2__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryEditableTable__Group__3_in_rule__DictionaryEditableTable__Group__232837);
rule__DictionaryEditableTable__Group__3();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryEditableTable__Group__2"
// $ANTLR start "rule__DictionaryEditableTable__Group__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15928:1: rule__DictionaryEditableTable__Group__2__Impl : ( '{' ) ;
public final void rule__DictionaryEditableTable__Group__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15932:1: ( ( '{' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15933:1: ( '{' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15933:1: ( '{' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15934:1: '{'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryEditableTableAccess().getLeftCurlyBracketKeyword_2());
}
match(input,67,FollowSets001.FOLLOW_67_in_rule__DictionaryEditableTable__Group__2__Impl32865); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryEditableTableAccess().getLeftCurlyBracketKeyword_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryEditableTable__Group__2__Impl"
// $ANTLR start "rule__DictionaryEditableTable__Group__3"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15947:1: rule__DictionaryEditableTable__Group__3 : rule__DictionaryEditableTable__Group__3__Impl rule__DictionaryEditableTable__Group__4 ;
public final void rule__DictionaryEditableTable__Group__3() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15951:1: ( rule__DictionaryEditableTable__Group__3__Impl rule__DictionaryEditableTable__Group__4 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15952:2: rule__DictionaryEditableTable__Group__3__Impl rule__DictionaryEditableTable__Group__4
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryEditableTable__Group__3__Impl_in_rule__DictionaryEditableTable__Group__332896);
rule__DictionaryEditableTable__Group__3__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryEditableTable__Group__4_in_rule__DictionaryEditableTable__Group__332899);
rule__DictionaryEditableTable__Group__4();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryEditableTable__Group__3"
// $ANTLR start "rule__DictionaryEditableTable__Group__3__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15959:1: rule__DictionaryEditableTable__Group__3__Impl : ( ( rule__DictionaryEditableTable__Group_3__0 )? ) ;
public final void rule__DictionaryEditableTable__Group__3__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15963:1: ( ( ( rule__DictionaryEditableTable__Group_3__0 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15964:1: ( ( rule__DictionaryEditableTable__Group_3__0 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15964:1: ( ( rule__DictionaryEditableTable__Group_3__0 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15965:1: ( rule__DictionaryEditableTable__Group_3__0 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryEditableTableAccess().getGroup_3());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15966:1: ( rule__DictionaryEditableTable__Group_3__0 )?
int alt124=2;
int LA124_0 = input.LA(1);
if ( (LA124_0==110) ) {
alt124=1;
}
switch (alt124) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15966:2: rule__DictionaryEditableTable__Group_3__0
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryEditableTable__Group_3__0_in_rule__DictionaryEditableTable__Group__3__Impl32926);
rule__DictionaryEditableTable__Group_3__0();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryEditableTableAccess().getGroup_3());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryEditableTable__Group__3__Impl"
// $ANTLR start "rule__DictionaryEditableTable__Group__4"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15976:1: rule__DictionaryEditableTable__Group__4 : rule__DictionaryEditableTable__Group__4__Impl rule__DictionaryEditableTable__Group__5 ;
public final void rule__DictionaryEditableTable__Group__4() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15980:1: ( rule__DictionaryEditableTable__Group__4__Impl rule__DictionaryEditableTable__Group__5 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15981:2: rule__DictionaryEditableTable__Group__4__Impl rule__DictionaryEditableTable__Group__5
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryEditableTable__Group__4__Impl_in_rule__DictionaryEditableTable__Group__432957);
rule__DictionaryEditableTable__Group__4__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryEditableTable__Group__5_in_rule__DictionaryEditableTable__Group__432960);
rule__DictionaryEditableTable__Group__5();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryEditableTable__Group__4"
// $ANTLR start "rule__DictionaryEditableTable__Group__4__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15988:1: rule__DictionaryEditableTable__Group__4__Impl : ( ( rule__DictionaryEditableTable__Group_4__0 )? ) ;
public final void rule__DictionaryEditableTable__Group__4__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15992:1: ( ( ( rule__DictionaryEditableTable__Group_4__0 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15993:1: ( ( rule__DictionaryEditableTable__Group_4__0 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15993:1: ( ( rule__DictionaryEditableTable__Group_4__0 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15994:1: ( rule__DictionaryEditableTable__Group_4__0 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryEditableTableAccess().getGroup_4());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15995:1: ( rule__DictionaryEditableTable__Group_4__0 )?
int alt125=2;
int LA125_0 = input.LA(1);
if ( (LA125_0==108) ) {
alt125=1;
}
switch (alt125) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:15995:2: rule__DictionaryEditableTable__Group_4__0
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryEditableTable__Group_4__0_in_rule__DictionaryEditableTable__Group__4__Impl32987);
rule__DictionaryEditableTable__Group_4__0();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryEditableTableAccess().getGroup_4());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryEditableTable__Group__4__Impl"
// $ANTLR start "rule__DictionaryEditableTable__Group__5"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16005:1: rule__DictionaryEditableTable__Group__5 : rule__DictionaryEditableTable__Group__5__Impl rule__DictionaryEditableTable__Group__6 ;
public final void rule__DictionaryEditableTable__Group__5() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16009:1: ( rule__DictionaryEditableTable__Group__5__Impl rule__DictionaryEditableTable__Group__6 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16010:2: rule__DictionaryEditableTable__Group__5__Impl rule__DictionaryEditableTable__Group__6
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryEditableTable__Group__5__Impl_in_rule__DictionaryEditableTable__Group__533018);
rule__DictionaryEditableTable__Group__5__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryEditableTable__Group__6_in_rule__DictionaryEditableTable__Group__533021);
rule__DictionaryEditableTable__Group__6();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryEditableTable__Group__5"
// $ANTLR start "rule__DictionaryEditableTable__Group__5__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16017:1: rule__DictionaryEditableTable__Group__5__Impl : ( ( rule__DictionaryEditableTable__ContainercontentsAssignment_5 )* ) ;
public final void rule__DictionaryEditableTable__Group__5__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16021:1: ( ( ( rule__DictionaryEditableTable__ContainercontentsAssignment_5 )* ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16022:1: ( ( rule__DictionaryEditableTable__ContainercontentsAssignment_5 )* )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16022:1: ( ( rule__DictionaryEditableTable__ContainercontentsAssignment_5 )* )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16023:1: ( rule__DictionaryEditableTable__ContainercontentsAssignment_5 )*
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryEditableTableAccess().getContainercontentsAssignment_5());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16024:1: ( rule__DictionaryEditableTable__ContainercontentsAssignment_5 )*
loop126:
do {
int alt126=2;
int LA126_0 = input.LA(1);
if ( ((LA126_0>=112 && LA126_0<=113)||LA126_0==116||LA126_0==125||LA126_0==127||LA126_0==129||(LA126_0>=131 && LA126_0<=136)||LA126_0==138) ) {
alt126=1;
}
switch (alt126) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16024:2: rule__DictionaryEditableTable__ContainercontentsAssignment_5
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryEditableTable__ContainercontentsAssignment_5_in_rule__DictionaryEditableTable__Group__5__Impl33048);
rule__DictionaryEditableTable__ContainercontentsAssignment_5();
state._fsp--;
if (state.failed) return ;
}
break;
default :
break loop126;
}
} while (true);
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryEditableTableAccess().getContainercontentsAssignment_5());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryEditableTable__Group__5__Impl"
// $ANTLR start "rule__DictionaryEditableTable__Group__6"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16034:1: rule__DictionaryEditableTable__Group__6 : rule__DictionaryEditableTable__Group__6__Impl rule__DictionaryEditableTable__Group__7 ;
public final void rule__DictionaryEditableTable__Group__6() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16038:1: ( rule__DictionaryEditableTable__Group__6__Impl rule__DictionaryEditableTable__Group__7 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16039:2: rule__DictionaryEditableTable__Group__6__Impl rule__DictionaryEditableTable__Group__7
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryEditableTable__Group__6__Impl_in_rule__DictionaryEditableTable__Group__633079);
rule__DictionaryEditableTable__Group__6__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryEditableTable__Group__7_in_rule__DictionaryEditableTable__Group__633082);
rule__DictionaryEditableTable__Group__7();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryEditableTable__Group__6"
// $ANTLR start "rule__DictionaryEditableTable__Group__6__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16046:1: rule__DictionaryEditableTable__Group__6__Impl : ( 'entityattribute' ) ;
public final void rule__DictionaryEditableTable__Group__6__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16050:1: ( ( 'entityattribute' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16051:1: ( 'entityattribute' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16051:1: ( 'entityattribute' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16052:1: 'entityattribute'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryEditableTableAccess().getEntityattributeKeyword_6());
}
match(input,114,FollowSets001.FOLLOW_114_in_rule__DictionaryEditableTable__Group__6__Impl33110); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryEditableTableAccess().getEntityattributeKeyword_6());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryEditableTable__Group__6__Impl"
// $ANTLR start "rule__DictionaryEditableTable__Group__7"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16065:1: rule__DictionaryEditableTable__Group__7 : rule__DictionaryEditableTable__Group__7__Impl rule__DictionaryEditableTable__Group__8 ;
public final void rule__DictionaryEditableTable__Group__7() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16069:1: ( rule__DictionaryEditableTable__Group__7__Impl rule__DictionaryEditableTable__Group__8 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16070:2: rule__DictionaryEditableTable__Group__7__Impl rule__DictionaryEditableTable__Group__8
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryEditableTable__Group__7__Impl_in_rule__DictionaryEditableTable__Group__733141);
rule__DictionaryEditableTable__Group__7__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryEditableTable__Group__8_in_rule__DictionaryEditableTable__Group__733144);
rule__DictionaryEditableTable__Group__8();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryEditableTable__Group__7"
// $ANTLR start "rule__DictionaryEditableTable__Group__7__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16077:1: rule__DictionaryEditableTable__Group__7__Impl : ( ( rule__DictionaryEditableTable__EntityattributeAssignment_7 ) ) ;
public final void rule__DictionaryEditableTable__Group__7__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16081:1: ( ( ( rule__DictionaryEditableTable__EntityattributeAssignment_7 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16082:1: ( ( rule__DictionaryEditableTable__EntityattributeAssignment_7 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16082:1: ( ( rule__DictionaryEditableTable__EntityattributeAssignment_7 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16083:1: ( rule__DictionaryEditableTable__EntityattributeAssignment_7 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryEditableTableAccess().getEntityattributeAssignment_7());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16084:1: ( rule__DictionaryEditableTable__EntityattributeAssignment_7 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16084:2: rule__DictionaryEditableTable__EntityattributeAssignment_7
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryEditableTable__EntityattributeAssignment_7_in_rule__DictionaryEditableTable__Group__7__Impl33171);
rule__DictionaryEditableTable__EntityattributeAssignment_7();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryEditableTableAccess().getEntityattributeAssignment_7());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryEditableTable__Group__7__Impl"
// $ANTLR start "rule__DictionaryEditableTable__Group__8"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16094:1: rule__DictionaryEditableTable__Group__8 : rule__DictionaryEditableTable__Group__8__Impl rule__DictionaryEditableTable__Group__9 ;
public final void rule__DictionaryEditableTable__Group__8() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16098:1: ( rule__DictionaryEditableTable__Group__8__Impl rule__DictionaryEditableTable__Group__9 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16099:2: rule__DictionaryEditableTable__Group__8__Impl rule__DictionaryEditableTable__Group__9
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryEditableTable__Group__8__Impl_in_rule__DictionaryEditableTable__Group__833201);
rule__DictionaryEditableTable__Group__8__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryEditableTable__Group__9_in_rule__DictionaryEditableTable__Group__833204);
rule__DictionaryEditableTable__Group__9();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryEditableTable__Group__8"
// $ANTLR start "rule__DictionaryEditableTable__Group__8__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16106:1: rule__DictionaryEditableTable__Group__8__Impl : ( 'columncontrols' ) ;
public final void rule__DictionaryEditableTable__Group__8__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16110:1: ( ( 'columncontrols' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16111:1: ( 'columncontrols' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16111:1: ( 'columncontrols' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16112:1: 'columncontrols'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryEditableTableAccess().getColumncontrolsKeyword_8());
}
match(input,115,FollowSets001.FOLLOW_115_in_rule__DictionaryEditableTable__Group__8__Impl33232); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryEditableTableAccess().getColumncontrolsKeyword_8());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryEditableTable__Group__8__Impl"
// $ANTLR start "rule__DictionaryEditableTable__Group__9"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16125:1: rule__DictionaryEditableTable__Group__9 : rule__DictionaryEditableTable__Group__9__Impl rule__DictionaryEditableTable__Group__10 ;
public final void rule__DictionaryEditableTable__Group__9() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16129:1: ( rule__DictionaryEditableTable__Group__9__Impl rule__DictionaryEditableTable__Group__10 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16130:2: rule__DictionaryEditableTable__Group__9__Impl rule__DictionaryEditableTable__Group__10
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryEditableTable__Group__9__Impl_in_rule__DictionaryEditableTable__Group__933263);
rule__DictionaryEditableTable__Group__9__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryEditableTable__Group__10_in_rule__DictionaryEditableTable__Group__933266);
rule__DictionaryEditableTable__Group__10();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryEditableTable__Group__9"
// $ANTLR start "rule__DictionaryEditableTable__Group__9__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16137:1: rule__DictionaryEditableTable__Group__9__Impl : ( '{' ) ;
public final void rule__DictionaryEditableTable__Group__9__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16141:1: ( ( '{' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16142:1: ( '{' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16142:1: ( '{' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16143:1: '{'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryEditableTableAccess().getLeftCurlyBracketKeyword_9());
}
match(input,67,FollowSets001.FOLLOW_67_in_rule__DictionaryEditableTable__Group__9__Impl33294); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryEditableTableAccess().getLeftCurlyBracketKeyword_9());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryEditableTable__Group__9__Impl"
// $ANTLR start "rule__DictionaryEditableTable__Group__10"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16156:1: rule__DictionaryEditableTable__Group__10 : rule__DictionaryEditableTable__Group__10__Impl rule__DictionaryEditableTable__Group__11 ;
public final void rule__DictionaryEditableTable__Group__10() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16160:1: ( rule__DictionaryEditableTable__Group__10__Impl rule__DictionaryEditableTable__Group__11 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16161:2: rule__DictionaryEditableTable__Group__10__Impl rule__DictionaryEditableTable__Group__11
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryEditableTable__Group__10__Impl_in_rule__DictionaryEditableTable__Group__1033325);
rule__DictionaryEditableTable__Group__10__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryEditableTable__Group__11_in_rule__DictionaryEditableTable__Group__1033328);
rule__DictionaryEditableTable__Group__11();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryEditableTable__Group__10"
// $ANTLR start "rule__DictionaryEditableTable__Group__10__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16168:1: rule__DictionaryEditableTable__Group__10__Impl : ( ( rule__DictionaryEditableTable__ColumncontrolsAssignment_10 )* ) ;
public final void rule__DictionaryEditableTable__Group__10__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16172:1: ( ( ( rule__DictionaryEditableTable__ColumncontrolsAssignment_10 )* ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16173:1: ( ( rule__DictionaryEditableTable__ColumncontrolsAssignment_10 )* )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16173:1: ( ( rule__DictionaryEditableTable__ColumncontrolsAssignment_10 )* )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16174:1: ( rule__DictionaryEditableTable__ColumncontrolsAssignment_10 )*
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryEditableTableAccess().getColumncontrolsAssignment_10());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16175:1: ( rule__DictionaryEditableTable__ColumncontrolsAssignment_10 )*
loop127:
do {
int alt127=2;
int LA127_0 = input.LA(1);
if ( (LA127_0==125||LA127_0==127||LA127_0==129||(LA127_0>=131 && LA127_0<=136)||LA127_0==138) ) {
alt127=1;
}
switch (alt127) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16175:2: rule__DictionaryEditableTable__ColumncontrolsAssignment_10
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryEditableTable__ColumncontrolsAssignment_10_in_rule__DictionaryEditableTable__Group__10__Impl33355);
rule__DictionaryEditableTable__ColumncontrolsAssignment_10();
state._fsp--;
if (state.failed) return ;
}
break;
default :
break loop127;
}
} while (true);
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryEditableTableAccess().getColumncontrolsAssignment_10());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryEditableTable__Group__10__Impl"
// $ANTLR start "rule__DictionaryEditableTable__Group__11"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16185:1: rule__DictionaryEditableTable__Group__11 : rule__DictionaryEditableTable__Group__11__Impl rule__DictionaryEditableTable__Group__12 ;
public final void rule__DictionaryEditableTable__Group__11() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16189:1: ( rule__DictionaryEditableTable__Group__11__Impl rule__DictionaryEditableTable__Group__12 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16190:2: rule__DictionaryEditableTable__Group__11__Impl rule__DictionaryEditableTable__Group__12
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryEditableTable__Group__11__Impl_in_rule__DictionaryEditableTable__Group__1133386);
rule__DictionaryEditableTable__Group__11__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryEditableTable__Group__12_in_rule__DictionaryEditableTable__Group__1133389);
rule__DictionaryEditableTable__Group__12();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryEditableTable__Group__11"
// $ANTLR start "rule__DictionaryEditableTable__Group__11__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16197:1: rule__DictionaryEditableTable__Group__11__Impl : ( '}' ) ;
public final void rule__DictionaryEditableTable__Group__11__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16201:1: ( ( '}' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16202:1: ( '}' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16202:1: ( '}' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16203:1: '}'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryEditableTableAccess().getRightCurlyBracketKeyword_11());
}
match(input,68,FollowSets001.FOLLOW_68_in_rule__DictionaryEditableTable__Group__11__Impl33417); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryEditableTableAccess().getRightCurlyBracketKeyword_11());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryEditableTable__Group__11__Impl"
// $ANTLR start "rule__DictionaryEditableTable__Group__12"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16216:1: rule__DictionaryEditableTable__Group__12 : rule__DictionaryEditableTable__Group__12__Impl ;
public final void rule__DictionaryEditableTable__Group__12() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16220:1: ( rule__DictionaryEditableTable__Group__12__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16221:2: rule__DictionaryEditableTable__Group__12__Impl
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryEditableTable__Group__12__Impl_in_rule__DictionaryEditableTable__Group__1233448);
rule__DictionaryEditableTable__Group__12__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryEditableTable__Group__12"
// $ANTLR start "rule__DictionaryEditableTable__Group__12__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16227:1: rule__DictionaryEditableTable__Group__12__Impl : ( '}' ) ;
public final void rule__DictionaryEditableTable__Group__12__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16231:1: ( ( '}' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16232:1: ( '}' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16232:1: ( '}' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16233:1: '}'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryEditableTableAccess().getRightCurlyBracketKeyword_12());
}
match(input,68,FollowSets001.FOLLOW_68_in_rule__DictionaryEditableTable__Group__12__Impl33476); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryEditableTableAccess().getRightCurlyBracketKeyword_12());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryEditableTable__Group__12__Impl"
// $ANTLR start "rule__DictionaryEditableTable__Group_3__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16272:1: rule__DictionaryEditableTable__Group_3__0 : rule__DictionaryEditableTable__Group_3__0__Impl rule__DictionaryEditableTable__Group_3__1 ;
public final void rule__DictionaryEditableTable__Group_3__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16276:1: ( rule__DictionaryEditableTable__Group_3__0__Impl rule__DictionaryEditableTable__Group_3__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16277:2: rule__DictionaryEditableTable__Group_3__0__Impl rule__DictionaryEditableTable__Group_3__1
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryEditableTable__Group_3__0__Impl_in_rule__DictionaryEditableTable__Group_3__033533);
rule__DictionaryEditableTable__Group_3__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryEditableTable__Group_3__1_in_rule__DictionaryEditableTable__Group_3__033536);
rule__DictionaryEditableTable__Group_3__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryEditableTable__Group_3__0"
// $ANTLR start "rule__DictionaryEditableTable__Group_3__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16284:1: rule__DictionaryEditableTable__Group_3__0__Impl : ( 'layoutdata' ) ;
public final void rule__DictionaryEditableTable__Group_3__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16288:1: ( ( 'layoutdata' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16289:1: ( 'layoutdata' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16289:1: ( 'layoutdata' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16290:1: 'layoutdata'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryEditableTableAccess().getLayoutdataKeyword_3_0());
}
match(input,110,FollowSets001.FOLLOW_110_in_rule__DictionaryEditableTable__Group_3__0__Impl33564); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryEditableTableAccess().getLayoutdataKeyword_3_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryEditableTable__Group_3__0__Impl"
// $ANTLR start "rule__DictionaryEditableTable__Group_3__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16303:1: rule__DictionaryEditableTable__Group_3__1 : rule__DictionaryEditableTable__Group_3__1__Impl ;
public final void rule__DictionaryEditableTable__Group_3__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16307:1: ( rule__DictionaryEditableTable__Group_3__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16308:2: rule__DictionaryEditableTable__Group_3__1__Impl
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryEditableTable__Group_3__1__Impl_in_rule__DictionaryEditableTable__Group_3__133595);
rule__DictionaryEditableTable__Group_3__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryEditableTable__Group_3__1"
// $ANTLR start "rule__DictionaryEditableTable__Group_3__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16314:1: rule__DictionaryEditableTable__Group_3__1__Impl : ( ( rule__DictionaryEditableTable__LayoutdataAssignment_3_1 ) ) ;
public final void rule__DictionaryEditableTable__Group_3__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16318:1: ( ( ( rule__DictionaryEditableTable__LayoutdataAssignment_3_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16319:1: ( ( rule__DictionaryEditableTable__LayoutdataAssignment_3_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16319:1: ( ( rule__DictionaryEditableTable__LayoutdataAssignment_3_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16320:1: ( rule__DictionaryEditableTable__LayoutdataAssignment_3_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryEditableTableAccess().getLayoutdataAssignment_3_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16321:1: ( rule__DictionaryEditableTable__LayoutdataAssignment_3_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16321:2: rule__DictionaryEditableTable__LayoutdataAssignment_3_1
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryEditableTable__LayoutdataAssignment_3_1_in_rule__DictionaryEditableTable__Group_3__1__Impl33622);
rule__DictionaryEditableTable__LayoutdataAssignment_3_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryEditableTableAccess().getLayoutdataAssignment_3_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryEditableTable__Group_3__1__Impl"
}
@SuppressWarnings("all")
abstract class InternalMangoParser3 extends InternalMangoParser2 {
InternalMangoParser3(TokenStream input) {
this(input, new RecognizerSharedState());
}
InternalMangoParser3(TokenStream input, RecognizerSharedState state) {
super(input, state);
}
// $ANTLR start "rule__DictionaryEditableTable__Group_4__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16335:1: rule__DictionaryEditableTable__Group_4__0 : rule__DictionaryEditableTable__Group_4__0__Impl rule__DictionaryEditableTable__Group_4__1 ;
public final void rule__DictionaryEditableTable__Group_4__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16339:1: ( rule__DictionaryEditableTable__Group_4__0__Impl rule__DictionaryEditableTable__Group_4__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16340:2: rule__DictionaryEditableTable__Group_4__0__Impl rule__DictionaryEditableTable__Group_4__1
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryEditableTable__Group_4__0__Impl_in_rule__DictionaryEditableTable__Group_4__033656);
rule__DictionaryEditableTable__Group_4__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryEditableTable__Group_4__1_in_rule__DictionaryEditableTable__Group_4__033659);
rule__DictionaryEditableTable__Group_4__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryEditableTable__Group_4__0"
// $ANTLR start "rule__DictionaryEditableTable__Group_4__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16347:1: rule__DictionaryEditableTable__Group_4__0__Impl : ( 'layout' ) ;
public final void rule__DictionaryEditableTable__Group_4__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16351:1: ( ( 'layout' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16352:1: ( 'layout' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16352:1: ( 'layout' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16353:1: 'layout'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryEditableTableAccess().getLayoutKeyword_4_0());
}
match(input,108,FollowSets001.FOLLOW_108_in_rule__DictionaryEditableTable__Group_4__0__Impl33687); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryEditableTableAccess().getLayoutKeyword_4_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryEditableTable__Group_4__0__Impl"
// $ANTLR start "rule__DictionaryEditableTable__Group_4__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16366:1: rule__DictionaryEditableTable__Group_4__1 : rule__DictionaryEditableTable__Group_4__1__Impl ;
public final void rule__DictionaryEditableTable__Group_4__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16370:1: ( rule__DictionaryEditableTable__Group_4__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16371:2: rule__DictionaryEditableTable__Group_4__1__Impl
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryEditableTable__Group_4__1__Impl_in_rule__DictionaryEditableTable__Group_4__133718);
rule__DictionaryEditableTable__Group_4__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryEditableTable__Group_4__1"
// $ANTLR start "rule__DictionaryEditableTable__Group_4__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16377:1: rule__DictionaryEditableTable__Group_4__1__Impl : ( ( rule__DictionaryEditableTable__LayoutAssignment_4_1 ) ) ;
public final void rule__DictionaryEditableTable__Group_4__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16381:1: ( ( ( rule__DictionaryEditableTable__LayoutAssignment_4_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16382:1: ( ( rule__DictionaryEditableTable__LayoutAssignment_4_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16382:1: ( ( rule__DictionaryEditableTable__LayoutAssignment_4_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16383:1: ( rule__DictionaryEditableTable__LayoutAssignment_4_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryEditableTableAccess().getLayoutAssignment_4_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16384:1: ( rule__DictionaryEditableTable__LayoutAssignment_4_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16384:2: rule__DictionaryEditableTable__LayoutAssignment_4_1
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryEditableTable__LayoutAssignment_4_1_in_rule__DictionaryEditableTable__Group_4__1__Impl33745);
rule__DictionaryEditableTable__LayoutAssignment_4_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryEditableTableAccess().getLayoutAssignment_4_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryEditableTable__Group_4__1__Impl"
// $ANTLR start "rule__DictionaryAssignmentTable__Group__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16398:1: rule__DictionaryAssignmentTable__Group__0 : rule__DictionaryAssignmentTable__Group__0__Impl rule__DictionaryAssignmentTable__Group__1 ;
public final void rule__DictionaryAssignmentTable__Group__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16402:1: ( rule__DictionaryAssignmentTable__Group__0__Impl rule__DictionaryAssignmentTable__Group__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16403:2: rule__DictionaryAssignmentTable__Group__0__Impl rule__DictionaryAssignmentTable__Group__1
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryAssignmentTable__Group__0__Impl_in_rule__DictionaryAssignmentTable__Group__033779);
rule__DictionaryAssignmentTable__Group__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryAssignmentTable__Group__1_in_rule__DictionaryAssignmentTable__Group__033782);
rule__DictionaryAssignmentTable__Group__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryAssignmentTable__Group__0"
// $ANTLR start "rule__DictionaryAssignmentTable__Group__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16410:1: rule__DictionaryAssignmentTable__Group__0__Impl : ( 'assignmenttable' ) ;
public final void rule__DictionaryAssignmentTable__Group__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16414:1: ( ( 'assignmenttable' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16415:1: ( 'assignmenttable' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16415:1: ( 'assignmenttable' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16416:1: 'assignmenttable'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryAssignmentTableAccess().getAssignmenttableKeyword_0());
}
match(input,116,FollowSets001.FOLLOW_116_in_rule__DictionaryAssignmentTable__Group__0__Impl33810); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryAssignmentTableAccess().getAssignmenttableKeyword_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryAssignmentTable__Group__0__Impl"
// $ANTLR start "rule__DictionaryAssignmentTable__Group__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16429:1: rule__DictionaryAssignmentTable__Group__1 : rule__DictionaryAssignmentTable__Group__1__Impl rule__DictionaryAssignmentTable__Group__2 ;
public final void rule__DictionaryAssignmentTable__Group__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16433:1: ( rule__DictionaryAssignmentTable__Group__1__Impl rule__DictionaryAssignmentTable__Group__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16434:2: rule__DictionaryAssignmentTable__Group__1__Impl rule__DictionaryAssignmentTable__Group__2
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryAssignmentTable__Group__1__Impl_in_rule__DictionaryAssignmentTable__Group__133841);
rule__DictionaryAssignmentTable__Group__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryAssignmentTable__Group__2_in_rule__DictionaryAssignmentTable__Group__133844);
rule__DictionaryAssignmentTable__Group__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryAssignmentTable__Group__1"
// $ANTLR start "rule__DictionaryAssignmentTable__Group__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16441:1: rule__DictionaryAssignmentTable__Group__1__Impl : ( ( rule__DictionaryAssignmentTable__NameAssignment_1 ) ) ;
public final void rule__DictionaryAssignmentTable__Group__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16445:1: ( ( ( rule__DictionaryAssignmentTable__NameAssignment_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16446:1: ( ( rule__DictionaryAssignmentTable__NameAssignment_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16446:1: ( ( rule__DictionaryAssignmentTable__NameAssignment_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16447:1: ( rule__DictionaryAssignmentTable__NameAssignment_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryAssignmentTableAccess().getNameAssignment_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16448:1: ( rule__DictionaryAssignmentTable__NameAssignment_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16448:2: rule__DictionaryAssignmentTable__NameAssignment_1
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryAssignmentTable__NameAssignment_1_in_rule__DictionaryAssignmentTable__Group__1__Impl33871);
rule__DictionaryAssignmentTable__NameAssignment_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryAssignmentTableAccess().getNameAssignment_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryAssignmentTable__Group__1__Impl"
// $ANTLR start "rule__DictionaryAssignmentTable__Group__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16458:1: rule__DictionaryAssignmentTable__Group__2 : rule__DictionaryAssignmentTable__Group__2__Impl rule__DictionaryAssignmentTable__Group__3 ;
public final void rule__DictionaryAssignmentTable__Group__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16462:1: ( rule__DictionaryAssignmentTable__Group__2__Impl rule__DictionaryAssignmentTable__Group__3 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16463:2: rule__DictionaryAssignmentTable__Group__2__Impl rule__DictionaryAssignmentTable__Group__3
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryAssignmentTable__Group__2__Impl_in_rule__DictionaryAssignmentTable__Group__233901);
rule__DictionaryAssignmentTable__Group__2__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryAssignmentTable__Group__3_in_rule__DictionaryAssignmentTable__Group__233904);
rule__DictionaryAssignmentTable__Group__3();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryAssignmentTable__Group__2"
// $ANTLR start "rule__DictionaryAssignmentTable__Group__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16470:1: rule__DictionaryAssignmentTable__Group__2__Impl : ( '{' ) ;
public final void rule__DictionaryAssignmentTable__Group__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16474:1: ( ( '{' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16475:1: ( '{' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16475:1: ( '{' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16476:1: '{'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryAssignmentTableAccess().getLeftCurlyBracketKeyword_2());
}
match(input,67,FollowSets001.FOLLOW_67_in_rule__DictionaryAssignmentTable__Group__2__Impl33932); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryAssignmentTableAccess().getLeftCurlyBracketKeyword_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryAssignmentTable__Group__2__Impl"
// $ANTLR start "rule__DictionaryAssignmentTable__Group__3"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16489:1: rule__DictionaryAssignmentTable__Group__3 : rule__DictionaryAssignmentTable__Group__3__Impl rule__DictionaryAssignmentTable__Group__4 ;
public final void rule__DictionaryAssignmentTable__Group__3() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16493:1: ( rule__DictionaryAssignmentTable__Group__3__Impl rule__DictionaryAssignmentTable__Group__4 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16494:2: rule__DictionaryAssignmentTable__Group__3__Impl rule__DictionaryAssignmentTable__Group__4
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryAssignmentTable__Group__3__Impl_in_rule__DictionaryAssignmentTable__Group__333963);
rule__DictionaryAssignmentTable__Group__3__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryAssignmentTable__Group__4_in_rule__DictionaryAssignmentTable__Group__333966);
rule__DictionaryAssignmentTable__Group__4();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryAssignmentTable__Group__3"
// $ANTLR start "rule__DictionaryAssignmentTable__Group__3__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16501:1: rule__DictionaryAssignmentTable__Group__3__Impl : ( ( rule__DictionaryAssignmentTable__Group_3__0 )? ) ;
public final void rule__DictionaryAssignmentTable__Group__3__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16505:1: ( ( ( rule__DictionaryAssignmentTable__Group_3__0 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16506:1: ( ( rule__DictionaryAssignmentTable__Group_3__0 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16506:1: ( ( rule__DictionaryAssignmentTable__Group_3__0 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16507:1: ( rule__DictionaryAssignmentTable__Group_3__0 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryAssignmentTableAccess().getGroup_3());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16508:1: ( rule__DictionaryAssignmentTable__Group_3__0 )?
int alt128=2;
int LA128_0 = input.LA(1);
if ( (LA128_0==110) ) {
alt128=1;
}
switch (alt128) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16508:2: rule__DictionaryAssignmentTable__Group_3__0
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryAssignmentTable__Group_3__0_in_rule__DictionaryAssignmentTable__Group__3__Impl33993);
rule__DictionaryAssignmentTable__Group_3__0();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryAssignmentTableAccess().getGroup_3());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryAssignmentTable__Group__3__Impl"
// $ANTLR start "rule__DictionaryAssignmentTable__Group__4"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16518:1: rule__DictionaryAssignmentTable__Group__4 : rule__DictionaryAssignmentTable__Group__4__Impl rule__DictionaryAssignmentTable__Group__5 ;
public final void rule__DictionaryAssignmentTable__Group__4() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16522:1: ( rule__DictionaryAssignmentTable__Group__4__Impl rule__DictionaryAssignmentTable__Group__5 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16523:2: rule__DictionaryAssignmentTable__Group__4__Impl rule__DictionaryAssignmentTable__Group__5
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryAssignmentTable__Group__4__Impl_in_rule__DictionaryAssignmentTable__Group__434024);
rule__DictionaryAssignmentTable__Group__4__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryAssignmentTable__Group__5_in_rule__DictionaryAssignmentTable__Group__434027);
rule__DictionaryAssignmentTable__Group__5();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryAssignmentTable__Group__4"
// $ANTLR start "rule__DictionaryAssignmentTable__Group__4__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16530:1: rule__DictionaryAssignmentTable__Group__4__Impl : ( ( rule__DictionaryAssignmentTable__Group_4__0 )? ) ;
public final void rule__DictionaryAssignmentTable__Group__4__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16534:1: ( ( ( rule__DictionaryAssignmentTable__Group_4__0 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16535:1: ( ( rule__DictionaryAssignmentTable__Group_4__0 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16535:1: ( ( rule__DictionaryAssignmentTable__Group_4__0 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16536:1: ( rule__DictionaryAssignmentTable__Group_4__0 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryAssignmentTableAccess().getGroup_4());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16537:1: ( rule__DictionaryAssignmentTable__Group_4__0 )?
int alt129=2;
int LA129_0 = input.LA(1);
if ( (LA129_0==108) ) {
alt129=1;
}
switch (alt129) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16537:2: rule__DictionaryAssignmentTable__Group_4__0
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryAssignmentTable__Group_4__0_in_rule__DictionaryAssignmentTable__Group__4__Impl34054);
rule__DictionaryAssignmentTable__Group_4__0();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryAssignmentTableAccess().getGroup_4());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryAssignmentTable__Group__4__Impl"
// $ANTLR start "rule__DictionaryAssignmentTable__Group__5"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16547:1: rule__DictionaryAssignmentTable__Group__5 : rule__DictionaryAssignmentTable__Group__5__Impl rule__DictionaryAssignmentTable__Group__6 ;
public final void rule__DictionaryAssignmentTable__Group__5() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16551:1: ( rule__DictionaryAssignmentTable__Group__5__Impl rule__DictionaryAssignmentTable__Group__6 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16552:2: rule__DictionaryAssignmentTable__Group__5__Impl rule__DictionaryAssignmentTable__Group__6
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryAssignmentTable__Group__5__Impl_in_rule__DictionaryAssignmentTable__Group__534085);
rule__DictionaryAssignmentTable__Group__5__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryAssignmentTable__Group__6_in_rule__DictionaryAssignmentTable__Group__534088);
rule__DictionaryAssignmentTable__Group__6();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryAssignmentTable__Group__5"
// $ANTLR start "rule__DictionaryAssignmentTable__Group__5__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16559:1: rule__DictionaryAssignmentTable__Group__5__Impl : ( ( rule__DictionaryAssignmentTable__ContainercontentsAssignment_5 )* ) ;
public final void rule__DictionaryAssignmentTable__Group__5__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16563:1: ( ( ( rule__DictionaryAssignmentTable__ContainercontentsAssignment_5 )* ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16564:1: ( ( rule__DictionaryAssignmentTable__ContainercontentsAssignment_5 )* )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16564:1: ( ( rule__DictionaryAssignmentTable__ContainercontentsAssignment_5 )* )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16565:1: ( rule__DictionaryAssignmentTable__ContainercontentsAssignment_5 )*
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryAssignmentTableAccess().getContainercontentsAssignment_5());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16566:1: ( rule__DictionaryAssignmentTable__ContainercontentsAssignment_5 )*
loop130:
do {
int alt130=2;
int LA130_0 = input.LA(1);
if ( ((LA130_0>=112 && LA130_0<=113)||LA130_0==116||LA130_0==125||LA130_0==127||LA130_0==129||(LA130_0>=131 && LA130_0<=136)||LA130_0==138) ) {
alt130=1;
}
switch (alt130) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16566:2: rule__DictionaryAssignmentTable__ContainercontentsAssignment_5
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryAssignmentTable__ContainercontentsAssignment_5_in_rule__DictionaryAssignmentTable__Group__5__Impl34115);
rule__DictionaryAssignmentTable__ContainercontentsAssignment_5();
state._fsp--;
if (state.failed) return ;
}
break;
default :
break loop130;
}
} while (true);
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryAssignmentTableAccess().getContainercontentsAssignment_5());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryAssignmentTable__Group__5__Impl"
// $ANTLR start "rule__DictionaryAssignmentTable__Group__6"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16576:1: rule__DictionaryAssignmentTable__Group__6 : rule__DictionaryAssignmentTable__Group__6__Impl rule__DictionaryAssignmentTable__Group__7 ;
public final void rule__DictionaryAssignmentTable__Group__6() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16580:1: ( rule__DictionaryAssignmentTable__Group__6__Impl rule__DictionaryAssignmentTable__Group__7 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16581:2: rule__DictionaryAssignmentTable__Group__6__Impl rule__DictionaryAssignmentTable__Group__7
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryAssignmentTable__Group__6__Impl_in_rule__DictionaryAssignmentTable__Group__634146);
rule__DictionaryAssignmentTable__Group__6__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryAssignmentTable__Group__7_in_rule__DictionaryAssignmentTable__Group__634149);
rule__DictionaryAssignmentTable__Group__7();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryAssignmentTable__Group__6"
// $ANTLR start "rule__DictionaryAssignmentTable__Group__6__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16588:1: rule__DictionaryAssignmentTable__Group__6__Impl : ( 'entityattribute' ) ;
public final void rule__DictionaryAssignmentTable__Group__6__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16592:1: ( ( 'entityattribute' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16593:1: ( 'entityattribute' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16593:1: ( 'entityattribute' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16594:1: 'entityattribute'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryAssignmentTableAccess().getEntityattributeKeyword_6());
}
match(input,114,FollowSets001.FOLLOW_114_in_rule__DictionaryAssignmentTable__Group__6__Impl34177); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryAssignmentTableAccess().getEntityattributeKeyword_6());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryAssignmentTable__Group__6__Impl"
// $ANTLR start "rule__DictionaryAssignmentTable__Group__7"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16607:1: rule__DictionaryAssignmentTable__Group__7 : rule__DictionaryAssignmentTable__Group__7__Impl rule__DictionaryAssignmentTable__Group__8 ;
public final void rule__DictionaryAssignmentTable__Group__7() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16611:1: ( rule__DictionaryAssignmentTable__Group__7__Impl rule__DictionaryAssignmentTable__Group__8 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16612:2: rule__DictionaryAssignmentTable__Group__7__Impl rule__DictionaryAssignmentTable__Group__8
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryAssignmentTable__Group__7__Impl_in_rule__DictionaryAssignmentTable__Group__734208);
rule__DictionaryAssignmentTable__Group__7__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryAssignmentTable__Group__8_in_rule__DictionaryAssignmentTable__Group__734211);
rule__DictionaryAssignmentTable__Group__8();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryAssignmentTable__Group__7"
// $ANTLR start "rule__DictionaryAssignmentTable__Group__7__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16619:1: rule__DictionaryAssignmentTable__Group__7__Impl : ( ( rule__DictionaryAssignmentTable__EntityattributeAssignment_7 ) ) ;
public final void rule__DictionaryAssignmentTable__Group__7__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16623:1: ( ( ( rule__DictionaryAssignmentTable__EntityattributeAssignment_7 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16624:1: ( ( rule__DictionaryAssignmentTable__EntityattributeAssignment_7 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16624:1: ( ( rule__DictionaryAssignmentTable__EntityattributeAssignment_7 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16625:1: ( rule__DictionaryAssignmentTable__EntityattributeAssignment_7 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryAssignmentTableAccess().getEntityattributeAssignment_7());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16626:1: ( rule__DictionaryAssignmentTable__EntityattributeAssignment_7 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16626:2: rule__DictionaryAssignmentTable__EntityattributeAssignment_7
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryAssignmentTable__EntityattributeAssignment_7_in_rule__DictionaryAssignmentTable__Group__7__Impl34238);
rule__DictionaryAssignmentTable__EntityattributeAssignment_7();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryAssignmentTableAccess().getEntityattributeAssignment_7());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryAssignmentTable__Group__7__Impl"
// $ANTLR start "rule__DictionaryAssignmentTable__Group__8"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16636:1: rule__DictionaryAssignmentTable__Group__8 : rule__DictionaryAssignmentTable__Group__8__Impl rule__DictionaryAssignmentTable__Group__9 ;
public final void rule__DictionaryAssignmentTable__Group__8() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16640:1: ( rule__DictionaryAssignmentTable__Group__8__Impl rule__DictionaryAssignmentTable__Group__9 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16641:2: rule__DictionaryAssignmentTable__Group__8__Impl rule__DictionaryAssignmentTable__Group__9
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryAssignmentTable__Group__8__Impl_in_rule__DictionaryAssignmentTable__Group__834268);
rule__DictionaryAssignmentTable__Group__8__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryAssignmentTable__Group__9_in_rule__DictionaryAssignmentTable__Group__834271);
rule__DictionaryAssignmentTable__Group__9();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryAssignmentTable__Group__8"
// $ANTLR start "rule__DictionaryAssignmentTable__Group__8__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16648:1: rule__DictionaryAssignmentTable__Group__8__Impl : ( 'dictionary' ) ;
public final void rule__DictionaryAssignmentTable__Group__8__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16652:1: ( ( 'dictionary' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16653:1: ( 'dictionary' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16653:1: ( 'dictionary' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16654:1: 'dictionary'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryAssignmentTableAccess().getDictionaryKeyword_8());
}
match(input,101,FollowSets001.FOLLOW_101_in_rule__DictionaryAssignmentTable__Group__8__Impl34299); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryAssignmentTableAccess().getDictionaryKeyword_8());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryAssignmentTable__Group__8__Impl"
// $ANTLR start "rule__DictionaryAssignmentTable__Group__9"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16667:1: rule__DictionaryAssignmentTable__Group__9 : rule__DictionaryAssignmentTable__Group__9__Impl rule__DictionaryAssignmentTable__Group__10 ;
public final void rule__DictionaryAssignmentTable__Group__9() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16671:1: ( rule__DictionaryAssignmentTable__Group__9__Impl rule__DictionaryAssignmentTable__Group__10 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16672:2: rule__DictionaryAssignmentTable__Group__9__Impl rule__DictionaryAssignmentTable__Group__10
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryAssignmentTable__Group__9__Impl_in_rule__DictionaryAssignmentTable__Group__934330);
rule__DictionaryAssignmentTable__Group__9__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryAssignmentTable__Group__10_in_rule__DictionaryAssignmentTable__Group__934333);
rule__DictionaryAssignmentTable__Group__10();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryAssignmentTable__Group__9"
// $ANTLR start "rule__DictionaryAssignmentTable__Group__9__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16679:1: rule__DictionaryAssignmentTable__Group__9__Impl : ( ( rule__DictionaryAssignmentTable__DictionaryAssignment_9 ) ) ;
public final void rule__DictionaryAssignmentTable__Group__9__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16683:1: ( ( ( rule__DictionaryAssignmentTable__DictionaryAssignment_9 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16684:1: ( ( rule__DictionaryAssignmentTable__DictionaryAssignment_9 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16684:1: ( ( rule__DictionaryAssignmentTable__DictionaryAssignment_9 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16685:1: ( rule__DictionaryAssignmentTable__DictionaryAssignment_9 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryAssignmentTableAccess().getDictionaryAssignment_9());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16686:1: ( rule__DictionaryAssignmentTable__DictionaryAssignment_9 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16686:2: rule__DictionaryAssignmentTable__DictionaryAssignment_9
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryAssignmentTable__DictionaryAssignment_9_in_rule__DictionaryAssignmentTable__Group__9__Impl34360);
rule__DictionaryAssignmentTable__DictionaryAssignment_9();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryAssignmentTableAccess().getDictionaryAssignment_9());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryAssignmentTable__Group__9__Impl"
// $ANTLR start "rule__DictionaryAssignmentTable__Group__10"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16696:1: rule__DictionaryAssignmentTable__Group__10 : rule__DictionaryAssignmentTable__Group__10__Impl rule__DictionaryAssignmentTable__Group__11 ;
public final void rule__DictionaryAssignmentTable__Group__10() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16700:1: ( rule__DictionaryAssignmentTable__Group__10__Impl rule__DictionaryAssignmentTable__Group__11 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16701:2: rule__DictionaryAssignmentTable__Group__10__Impl rule__DictionaryAssignmentTable__Group__11
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryAssignmentTable__Group__10__Impl_in_rule__DictionaryAssignmentTable__Group__1034390);
rule__DictionaryAssignmentTable__Group__10__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryAssignmentTable__Group__11_in_rule__DictionaryAssignmentTable__Group__1034393);
rule__DictionaryAssignmentTable__Group__11();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryAssignmentTable__Group__10"
// $ANTLR start "rule__DictionaryAssignmentTable__Group__10__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16708:1: rule__DictionaryAssignmentTable__Group__10__Impl : ( 'columncontrols' ) ;
public final void rule__DictionaryAssignmentTable__Group__10__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16712:1: ( ( 'columncontrols' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16713:1: ( 'columncontrols' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16713:1: ( 'columncontrols' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16714:1: 'columncontrols'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryAssignmentTableAccess().getColumncontrolsKeyword_10());
}
match(input,115,FollowSets001.FOLLOW_115_in_rule__DictionaryAssignmentTable__Group__10__Impl34421); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryAssignmentTableAccess().getColumncontrolsKeyword_10());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryAssignmentTable__Group__10__Impl"
// $ANTLR start "rule__DictionaryAssignmentTable__Group__11"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16727:1: rule__DictionaryAssignmentTable__Group__11 : rule__DictionaryAssignmentTable__Group__11__Impl rule__DictionaryAssignmentTable__Group__12 ;
public final void rule__DictionaryAssignmentTable__Group__11() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16731:1: ( rule__DictionaryAssignmentTable__Group__11__Impl rule__DictionaryAssignmentTable__Group__12 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16732:2: rule__DictionaryAssignmentTable__Group__11__Impl rule__DictionaryAssignmentTable__Group__12
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryAssignmentTable__Group__11__Impl_in_rule__DictionaryAssignmentTable__Group__1134452);
rule__DictionaryAssignmentTable__Group__11__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryAssignmentTable__Group__12_in_rule__DictionaryAssignmentTable__Group__1134455);
rule__DictionaryAssignmentTable__Group__12();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryAssignmentTable__Group__11"
// $ANTLR start "rule__DictionaryAssignmentTable__Group__11__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16739:1: rule__DictionaryAssignmentTable__Group__11__Impl : ( '{' ) ;
public final void rule__DictionaryAssignmentTable__Group__11__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16743:1: ( ( '{' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16744:1: ( '{' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16744:1: ( '{' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16745:1: '{'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryAssignmentTableAccess().getLeftCurlyBracketKeyword_11());
}
match(input,67,FollowSets001.FOLLOW_67_in_rule__DictionaryAssignmentTable__Group__11__Impl34483); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryAssignmentTableAccess().getLeftCurlyBracketKeyword_11());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryAssignmentTable__Group__11__Impl"
// $ANTLR start "rule__DictionaryAssignmentTable__Group__12"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16758:1: rule__DictionaryAssignmentTable__Group__12 : rule__DictionaryAssignmentTable__Group__12__Impl rule__DictionaryAssignmentTable__Group__13 ;
public final void rule__DictionaryAssignmentTable__Group__12() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16762:1: ( rule__DictionaryAssignmentTable__Group__12__Impl rule__DictionaryAssignmentTable__Group__13 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16763:2: rule__DictionaryAssignmentTable__Group__12__Impl rule__DictionaryAssignmentTable__Group__13
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryAssignmentTable__Group__12__Impl_in_rule__DictionaryAssignmentTable__Group__1234514);
rule__DictionaryAssignmentTable__Group__12__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryAssignmentTable__Group__13_in_rule__DictionaryAssignmentTable__Group__1234517);
rule__DictionaryAssignmentTable__Group__13();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryAssignmentTable__Group__12"
// $ANTLR start "rule__DictionaryAssignmentTable__Group__12__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16770:1: rule__DictionaryAssignmentTable__Group__12__Impl : ( ( rule__DictionaryAssignmentTable__ColumncontrolsAssignment_12 )* ) ;
public final void rule__DictionaryAssignmentTable__Group__12__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16774:1: ( ( ( rule__DictionaryAssignmentTable__ColumncontrolsAssignment_12 )* ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16775:1: ( ( rule__DictionaryAssignmentTable__ColumncontrolsAssignment_12 )* )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16775:1: ( ( rule__DictionaryAssignmentTable__ColumncontrolsAssignment_12 )* )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16776:1: ( rule__DictionaryAssignmentTable__ColumncontrolsAssignment_12 )*
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryAssignmentTableAccess().getColumncontrolsAssignment_12());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16777:1: ( rule__DictionaryAssignmentTable__ColumncontrolsAssignment_12 )*
loop131:
do {
int alt131=2;
int LA131_0 = input.LA(1);
if ( (LA131_0==125||LA131_0==127||LA131_0==129||(LA131_0>=131 && LA131_0<=136)||LA131_0==138) ) {
alt131=1;
}
switch (alt131) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16777:2: rule__DictionaryAssignmentTable__ColumncontrolsAssignment_12
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryAssignmentTable__ColumncontrolsAssignment_12_in_rule__DictionaryAssignmentTable__Group__12__Impl34544);
rule__DictionaryAssignmentTable__ColumncontrolsAssignment_12();
state._fsp--;
if (state.failed) return ;
}
break;
default :
break loop131;
}
} while (true);
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryAssignmentTableAccess().getColumncontrolsAssignment_12());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryAssignmentTable__Group__12__Impl"
// $ANTLR start "rule__DictionaryAssignmentTable__Group__13"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16787:1: rule__DictionaryAssignmentTable__Group__13 : rule__DictionaryAssignmentTable__Group__13__Impl rule__DictionaryAssignmentTable__Group__14 ;
public final void rule__DictionaryAssignmentTable__Group__13() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16791:1: ( rule__DictionaryAssignmentTable__Group__13__Impl rule__DictionaryAssignmentTable__Group__14 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16792:2: rule__DictionaryAssignmentTable__Group__13__Impl rule__DictionaryAssignmentTable__Group__14
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryAssignmentTable__Group__13__Impl_in_rule__DictionaryAssignmentTable__Group__1334575);
rule__DictionaryAssignmentTable__Group__13__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryAssignmentTable__Group__14_in_rule__DictionaryAssignmentTable__Group__1334578);
rule__DictionaryAssignmentTable__Group__14();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryAssignmentTable__Group__13"
// $ANTLR start "rule__DictionaryAssignmentTable__Group__13__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16799:1: rule__DictionaryAssignmentTable__Group__13__Impl : ( '}' ) ;
public final void rule__DictionaryAssignmentTable__Group__13__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16803:1: ( ( '}' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16804:1: ( '}' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16804:1: ( '}' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16805:1: '}'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryAssignmentTableAccess().getRightCurlyBracketKeyword_13());
}
match(input,68,FollowSets001.FOLLOW_68_in_rule__DictionaryAssignmentTable__Group__13__Impl34606); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryAssignmentTableAccess().getRightCurlyBracketKeyword_13());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryAssignmentTable__Group__13__Impl"
// $ANTLR start "rule__DictionaryAssignmentTable__Group__14"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16818:1: rule__DictionaryAssignmentTable__Group__14 : rule__DictionaryAssignmentTable__Group__14__Impl ;
public final void rule__DictionaryAssignmentTable__Group__14() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16822:1: ( rule__DictionaryAssignmentTable__Group__14__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16823:2: rule__DictionaryAssignmentTable__Group__14__Impl
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryAssignmentTable__Group__14__Impl_in_rule__DictionaryAssignmentTable__Group__1434637);
rule__DictionaryAssignmentTable__Group__14__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryAssignmentTable__Group__14"
// $ANTLR start "rule__DictionaryAssignmentTable__Group__14__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16829:1: rule__DictionaryAssignmentTable__Group__14__Impl : ( '}' ) ;
public final void rule__DictionaryAssignmentTable__Group__14__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16833:1: ( ( '}' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16834:1: ( '}' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16834:1: ( '}' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16835:1: '}'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryAssignmentTableAccess().getRightCurlyBracketKeyword_14());
}
match(input,68,FollowSets001.FOLLOW_68_in_rule__DictionaryAssignmentTable__Group__14__Impl34665); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryAssignmentTableAccess().getRightCurlyBracketKeyword_14());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryAssignmentTable__Group__14__Impl"
// $ANTLR start "rule__DictionaryAssignmentTable__Group_3__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16878:1: rule__DictionaryAssignmentTable__Group_3__0 : rule__DictionaryAssignmentTable__Group_3__0__Impl rule__DictionaryAssignmentTable__Group_3__1 ;
public final void rule__DictionaryAssignmentTable__Group_3__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16882:1: ( rule__DictionaryAssignmentTable__Group_3__0__Impl rule__DictionaryAssignmentTable__Group_3__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16883:2: rule__DictionaryAssignmentTable__Group_3__0__Impl rule__DictionaryAssignmentTable__Group_3__1
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryAssignmentTable__Group_3__0__Impl_in_rule__DictionaryAssignmentTable__Group_3__034726);
rule__DictionaryAssignmentTable__Group_3__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryAssignmentTable__Group_3__1_in_rule__DictionaryAssignmentTable__Group_3__034729);
rule__DictionaryAssignmentTable__Group_3__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryAssignmentTable__Group_3__0"
// $ANTLR start "rule__DictionaryAssignmentTable__Group_3__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16890:1: rule__DictionaryAssignmentTable__Group_3__0__Impl : ( 'layoutdata' ) ;
public final void rule__DictionaryAssignmentTable__Group_3__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16894:1: ( ( 'layoutdata' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16895:1: ( 'layoutdata' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16895:1: ( 'layoutdata' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16896:1: 'layoutdata'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryAssignmentTableAccess().getLayoutdataKeyword_3_0());
}
match(input,110,FollowSets001.FOLLOW_110_in_rule__DictionaryAssignmentTable__Group_3__0__Impl34757); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryAssignmentTableAccess().getLayoutdataKeyword_3_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryAssignmentTable__Group_3__0__Impl"
// $ANTLR start "rule__DictionaryAssignmentTable__Group_3__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16909:1: rule__DictionaryAssignmentTable__Group_3__1 : rule__DictionaryAssignmentTable__Group_3__1__Impl ;
public final void rule__DictionaryAssignmentTable__Group_3__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16913:1: ( rule__DictionaryAssignmentTable__Group_3__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16914:2: rule__DictionaryAssignmentTable__Group_3__1__Impl
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryAssignmentTable__Group_3__1__Impl_in_rule__DictionaryAssignmentTable__Group_3__134788);
rule__DictionaryAssignmentTable__Group_3__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryAssignmentTable__Group_3__1"
// $ANTLR start "rule__DictionaryAssignmentTable__Group_3__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16920:1: rule__DictionaryAssignmentTable__Group_3__1__Impl : ( ( rule__DictionaryAssignmentTable__LayoutdataAssignment_3_1 ) ) ;
public final void rule__DictionaryAssignmentTable__Group_3__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16924:1: ( ( ( rule__DictionaryAssignmentTable__LayoutdataAssignment_3_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16925:1: ( ( rule__DictionaryAssignmentTable__LayoutdataAssignment_3_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16925:1: ( ( rule__DictionaryAssignmentTable__LayoutdataAssignment_3_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16926:1: ( rule__DictionaryAssignmentTable__LayoutdataAssignment_3_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryAssignmentTableAccess().getLayoutdataAssignment_3_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16927:1: ( rule__DictionaryAssignmentTable__LayoutdataAssignment_3_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16927:2: rule__DictionaryAssignmentTable__LayoutdataAssignment_3_1
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryAssignmentTable__LayoutdataAssignment_3_1_in_rule__DictionaryAssignmentTable__Group_3__1__Impl34815);
rule__DictionaryAssignmentTable__LayoutdataAssignment_3_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryAssignmentTableAccess().getLayoutdataAssignment_3_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryAssignmentTable__Group_3__1__Impl"
// $ANTLR start "rule__DictionaryAssignmentTable__Group_4__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16941:1: rule__DictionaryAssignmentTable__Group_4__0 : rule__DictionaryAssignmentTable__Group_4__0__Impl rule__DictionaryAssignmentTable__Group_4__1 ;
public final void rule__DictionaryAssignmentTable__Group_4__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16945:1: ( rule__DictionaryAssignmentTable__Group_4__0__Impl rule__DictionaryAssignmentTable__Group_4__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16946:2: rule__DictionaryAssignmentTable__Group_4__0__Impl rule__DictionaryAssignmentTable__Group_4__1
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryAssignmentTable__Group_4__0__Impl_in_rule__DictionaryAssignmentTable__Group_4__034849);
rule__DictionaryAssignmentTable__Group_4__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryAssignmentTable__Group_4__1_in_rule__DictionaryAssignmentTable__Group_4__034852);
rule__DictionaryAssignmentTable__Group_4__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryAssignmentTable__Group_4__0"
// $ANTLR start "rule__DictionaryAssignmentTable__Group_4__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16953:1: rule__DictionaryAssignmentTable__Group_4__0__Impl : ( 'layout' ) ;
public final void rule__DictionaryAssignmentTable__Group_4__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16957:1: ( ( 'layout' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16958:1: ( 'layout' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16958:1: ( 'layout' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16959:1: 'layout'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryAssignmentTableAccess().getLayoutKeyword_4_0());
}
match(input,108,FollowSets001.FOLLOW_108_in_rule__DictionaryAssignmentTable__Group_4__0__Impl34880); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryAssignmentTableAccess().getLayoutKeyword_4_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryAssignmentTable__Group_4__0__Impl"
// $ANTLR start "rule__DictionaryAssignmentTable__Group_4__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16972:1: rule__DictionaryAssignmentTable__Group_4__1 : rule__DictionaryAssignmentTable__Group_4__1__Impl ;
public final void rule__DictionaryAssignmentTable__Group_4__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16976:1: ( rule__DictionaryAssignmentTable__Group_4__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16977:2: rule__DictionaryAssignmentTable__Group_4__1__Impl
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryAssignmentTable__Group_4__1__Impl_in_rule__DictionaryAssignmentTable__Group_4__134911);
rule__DictionaryAssignmentTable__Group_4__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryAssignmentTable__Group_4__1"
// $ANTLR start "rule__DictionaryAssignmentTable__Group_4__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16983:1: rule__DictionaryAssignmentTable__Group_4__1__Impl : ( ( rule__DictionaryAssignmentTable__LayoutAssignment_4_1 ) ) ;
public final void rule__DictionaryAssignmentTable__Group_4__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16987:1: ( ( ( rule__DictionaryAssignmentTable__LayoutAssignment_4_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16988:1: ( ( rule__DictionaryAssignmentTable__LayoutAssignment_4_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16988:1: ( ( rule__DictionaryAssignmentTable__LayoutAssignment_4_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16989:1: ( rule__DictionaryAssignmentTable__LayoutAssignment_4_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryAssignmentTableAccess().getLayoutAssignment_4_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16990:1: ( rule__DictionaryAssignmentTable__LayoutAssignment_4_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:16990:2: rule__DictionaryAssignmentTable__LayoutAssignment_4_1
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryAssignmentTable__LayoutAssignment_4_1_in_rule__DictionaryAssignmentTable__Group_4__1__Impl34938);
rule__DictionaryAssignmentTable__LayoutAssignment_4_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryAssignmentTableAccess().getLayoutAssignment_4_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryAssignmentTable__Group_4__1__Impl"
// $ANTLR start "rule__Labels__Group__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17004:1: rule__Labels__Group__0 : rule__Labels__Group__0__Impl rule__Labels__Group__1 ;
public final void rule__Labels__Group__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17008:1: ( rule__Labels__Group__0__Impl rule__Labels__Group__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17009:2: rule__Labels__Group__0__Impl rule__Labels__Group__1
{
pushFollow(FollowSets001.FOLLOW_rule__Labels__Group__0__Impl_in_rule__Labels__Group__034972);
rule__Labels__Group__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__Labels__Group__1_in_rule__Labels__Group__034975);
rule__Labels__Group__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Labels__Group__0"
// $ANTLR start "rule__Labels__Group__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17016:1: rule__Labels__Group__0__Impl : ( () ) ;
public final void rule__Labels__Group__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17020:1: ( ( () ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17021:1: ( () )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17021:1: ( () )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17022:1: ()
{
if ( state.backtracking==0 ) {
before(grammarAccess.getLabelsAccess().getLabelsAction_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17023:1: ()
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17025:1:
{
}
if ( state.backtracking==0 ) {
after(grammarAccess.getLabelsAccess().getLabelsAction_0());
}
}
}
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Labels__Group__0__Impl"
// $ANTLR start "rule__Labels__Group__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17035:1: rule__Labels__Group__1 : rule__Labels__Group__1__Impl rule__Labels__Group__2 ;
public final void rule__Labels__Group__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17039:1: ( rule__Labels__Group__1__Impl rule__Labels__Group__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17040:2: rule__Labels__Group__1__Impl rule__Labels__Group__2
{
pushFollow(FollowSets001.FOLLOW_rule__Labels__Group__1__Impl_in_rule__Labels__Group__135033);
rule__Labels__Group__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__Labels__Group__2_in_rule__Labels__Group__135036);
rule__Labels__Group__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Labels__Group__1"
// $ANTLR start "rule__Labels__Group__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17047:1: rule__Labels__Group__1__Impl : ( ( rule__Labels__Group_1__0 )? ) ;
public final void rule__Labels__Group__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17051:1: ( ( ( rule__Labels__Group_1__0 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17052:1: ( ( rule__Labels__Group_1__0 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17052:1: ( ( rule__Labels__Group_1__0 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17053:1: ( rule__Labels__Group_1__0 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getLabelsAccess().getGroup_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17054:1: ( rule__Labels__Group_1__0 )?
int alt132=2;
int LA132_0 = input.LA(1);
if ( (LA132_0==75) ) {
alt132=1;
}
switch (alt132) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17054:2: rule__Labels__Group_1__0
{
pushFollow(FollowSets001.FOLLOW_rule__Labels__Group_1__0_in_rule__Labels__Group__1__Impl35063);
rule__Labels__Group_1__0();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getLabelsAccess().getGroup_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Labels__Group__1__Impl"
// $ANTLR start "rule__Labels__Group__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17064:1: rule__Labels__Group__2 : rule__Labels__Group__2__Impl rule__Labels__Group__3 ;
public final void rule__Labels__Group__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17068:1: ( rule__Labels__Group__2__Impl rule__Labels__Group__3 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17069:2: rule__Labels__Group__2__Impl rule__Labels__Group__3
{
pushFollow(FollowSets001.FOLLOW_rule__Labels__Group__2__Impl_in_rule__Labels__Group__235094);
rule__Labels__Group__2__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__Labels__Group__3_in_rule__Labels__Group__235097);
rule__Labels__Group__3();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Labels__Group__2"
// $ANTLR start "rule__Labels__Group__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17076:1: rule__Labels__Group__2__Impl : ( ( rule__Labels__Group_2__0 )? ) ;
public final void rule__Labels__Group__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17080:1: ( ( ( rule__Labels__Group_2__0 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17081:1: ( ( rule__Labels__Group_2__0 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17081:1: ( ( rule__Labels__Group_2__0 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17082:1: ( rule__Labels__Group_2__0 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getLabelsAccess().getGroup_2());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17083:1: ( rule__Labels__Group_2__0 )?
int alt133=2;
int LA133_0 = input.LA(1);
if ( (LA133_0==117) ) {
alt133=1;
}
switch (alt133) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17083:2: rule__Labels__Group_2__0
{
pushFollow(FollowSets001.FOLLOW_rule__Labels__Group_2__0_in_rule__Labels__Group__2__Impl35124);
rule__Labels__Group_2__0();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getLabelsAccess().getGroup_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Labels__Group__2__Impl"
// $ANTLR start "rule__Labels__Group__3"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17093:1: rule__Labels__Group__3 : rule__Labels__Group__3__Impl rule__Labels__Group__4 ;
public final void rule__Labels__Group__3() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17097:1: ( rule__Labels__Group__3__Impl rule__Labels__Group__4 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17098:2: rule__Labels__Group__3__Impl rule__Labels__Group__4
{
pushFollow(FollowSets001.FOLLOW_rule__Labels__Group__3__Impl_in_rule__Labels__Group__335155);
rule__Labels__Group__3__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__Labels__Group__4_in_rule__Labels__Group__335158);
rule__Labels__Group__4();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Labels__Group__3"
// $ANTLR start "rule__Labels__Group__3__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17105:1: rule__Labels__Group__3__Impl : ( ( rule__Labels__Group_3__0 )? ) ;
public final void rule__Labels__Group__3__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17109:1: ( ( ( rule__Labels__Group_3__0 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17110:1: ( ( rule__Labels__Group_3__0 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17110:1: ( ( rule__Labels__Group_3__0 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17111:1: ( rule__Labels__Group_3__0 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getLabelsAccess().getGroup_3());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17112:1: ( rule__Labels__Group_3__0 )?
int alt134=2;
int LA134_0 = input.LA(1);
if ( (LA134_0==118) ) {
alt134=1;
}
switch (alt134) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17112:2: rule__Labels__Group_3__0
{
pushFollow(FollowSets001.FOLLOW_rule__Labels__Group_3__0_in_rule__Labels__Group__3__Impl35185);
rule__Labels__Group_3__0();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getLabelsAccess().getGroup_3());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Labels__Group__3__Impl"
// $ANTLR start "rule__Labels__Group__4"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17122:1: rule__Labels__Group__4 : rule__Labels__Group__4__Impl rule__Labels__Group__5 ;
public final void rule__Labels__Group__4() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17126:1: ( rule__Labels__Group__4__Impl rule__Labels__Group__5 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17127:2: rule__Labels__Group__4__Impl rule__Labels__Group__5
{
pushFollow(FollowSets001.FOLLOW_rule__Labels__Group__4__Impl_in_rule__Labels__Group__435216);
rule__Labels__Group__4__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__Labels__Group__5_in_rule__Labels__Group__435219);
rule__Labels__Group__5();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Labels__Group__4"
// $ANTLR start "rule__Labels__Group__4__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17134:1: rule__Labels__Group__4__Impl : ( ( rule__Labels__Group_4__0 )? ) ;
public final void rule__Labels__Group__4__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17138:1: ( ( ( rule__Labels__Group_4__0 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17139:1: ( ( rule__Labels__Group_4__0 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17139:1: ( ( rule__Labels__Group_4__0 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17140:1: ( rule__Labels__Group_4__0 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getLabelsAccess().getGroup_4());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17141:1: ( rule__Labels__Group_4__0 )?
int alt135=2;
int LA135_0 = input.LA(1);
if ( (LA135_0==119) ) {
alt135=1;
}
switch (alt135) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17141:2: rule__Labels__Group_4__0
{
pushFollow(FollowSets001.FOLLOW_rule__Labels__Group_4__0_in_rule__Labels__Group__4__Impl35246);
rule__Labels__Group_4__0();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getLabelsAccess().getGroup_4());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Labels__Group__4__Impl"
// $ANTLR start "rule__Labels__Group__5"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17151:1: rule__Labels__Group__5 : rule__Labels__Group__5__Impl ;
public final void rule__Labels__Group__5() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17155:1: ( rule__Labels__Group__5__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17156:2: rule__Labels__Group__5__Impl
{
pushFollow(FollowSets001.FOLLOW_rule__Labels__Group__5__Impl_in_rule__Labels__Group__535277);
rule__Labels__Group__5__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Labels__Group__5"
// $ANTLR start "rule__Labels__Group__5__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17162:1: rule__Labels__Group__5__Impl : ( ( rule__Labels__Group_5__0 )? ) ;
public final void rule__Labels__Group__5__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17166:1: ( ( ( rule__Labels__Group_5__0 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17167:1: ( ( rule__Labels__Group_5__0 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17167:1: ( ( rule__Labels__Group_5__0 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17168:1: ( rule__Labels__Group_5__0 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getLabelsAccess().getGroup_5());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17169:1: ( rule__Labels__Group_5__0 )?
int alt136=2;
int LA136_0 = input.LA(1);
if ( (LA136_0==120) ) {
alt136=1;
}
switch (alt136) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17169:2: rule__Labels__Group_5__0
{
pushFollow(FollowSets001.FOLLOW_rule__Labels__Group_5__0_in_rule__Labels__Group__5__Impl35304);
rule__Labels__Group_5__0();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getLabelsAccess().getGroup_5());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Labels__Group__5__Impl"
// $ANTLR start "rule__Labels__Group_1__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17191:1: rule__Labels__Group_1__0 : rule__Labels__Group_1__0__Impl rule__Labels__Group_1__1 ;
public final void rule__Labels__Group_1__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17195:1: ( rule__Labels__Group_1__0__Impl rule__Labels__Group_1__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17196:2: rule__Labels__Group_1__0__Impl rule__Labels__Group_1__1
{
pushFollow(FollowSets001.FOLLOW_rule__Labels__Group_1__0__Impl_in_rule__Labels__Group_1__035347);
rule__Labels__Group_1__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__Labels__Group_1__1_in_rule__Labels__Group_1__035350);
rule__Labels__Group_1__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Labels__Group_1__0"
// $ANTLR start "rule__Labels__Group_1__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17203:1: rule__Labels__Group_1__0__Impl : ( 'label' ) ;
public final void rule__Labels__Group_1__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17207:1: ( ( 'label' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17208:1: ( 'label' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17208:1: ( 'label' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17209:1: 'label'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getLabelsAccess().getLabelKeyword_1_0());
}
match(input,75,FollowSets001.FOLLOW_75_in_rule__Labels__Group_1__0__Impl35378); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getLabelsAccess().getLabelKeyword_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Labels__Group_1__0__Impl"
// $ANTLR start "rule__Labels__Group_1__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17222:1: rule__Labels__Group_1__1 : rule__Labels__Group_1__1__Impl ;
public final void rule__Labels__Group_1__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17226:1: ( rule__Labels__Group_1__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17227:2: rule__Labels__Group_1__1__Impl
{
pushFollow(FollowSets001.FOLLOW_rule__Labels__Group_1__1__Impl_in_rule__Labels__Group_1__135409);
rule__Labels__Group_1__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Labels__Group_1__1"
// $ANTLR start "rule__Labels__Group_1__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17233:1: rule__Labels__Group_1__1__Impl : ( ( rule__Labels__LabelAssignment_1_1 ) ) ;
public final void rule__Labels__Group_1__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17237:1: ( ( ( rule__Labels__LabelAssignment_1_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17238:1: ( ( rule__Labels__LabelAssignment_1_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17238:1: ( ( rule__Labels__LabelAssignment_1_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17239:1: ( rule__Labels__LabelAssignment_1_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getLabelsAccess().getLabelAssignment_1_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17240:1: ( rule__Labels__LabelAssignment_1_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17240:2: rule__Labels__LabelAssignment_1_1
{
pushFollow(FollowSets001.FOLLOW_rule__Labels__LabelAssignment_1_1_in_rule__Labels__Group_1__1__Impl35436);
rule__Labels__LabelAssignment_1_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getLabelsAccess().getLabelAssignment_1_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Labels__Group_1__1__Impl"
// $ANTLR start "rule__Labels__Group_2__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17254:1: rule__Labels__Group_2__0 : rule__Labels__Group_2__0__Impl rule__Labels__Group_2__1 ;
public final void rule__Labels__Group_2__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17258:1: ( rule__Labels__Group_2__0__Impl rule__Labels__Group_2__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17259:2: rule__Labels__Group_2__0__Impl rule__Labels__Group_2__1
{
pushFollow(FollowSets001.FOLLOW_rule__Labels__Group_2__0__Impl_in_rule__Labels__Group_2__035470);
rule__Labels__Group_2__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__Labels__Group_2__1_in_rule__Labels__Group_2__035473);
rule__Labels__Group_2__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Labels__Group_2__0"
// $ANTLR start "rule__Labels__Group_2__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17266:1: rule__Labels__Group_2__0__Impl : ( 'filterLabel' ) ;
public final void rule__Labels__Group_2__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17270:1: ( ( 'filterLabel' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17271:1: ( 'filterLabel' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17271:1: ( 'filterLabel' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17272:1: 'filterLabel'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getLabelsAccess().getFilterLabelKeyword_2_0());
}
match(input,117,FollowSets001.FOLLOW_117_in_rule__Labels__Group_2__0__Impl35501); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getLabelsAccess().getFilterLabelKeyword_2_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Labels__Group_2__0__Impl"
// $ANTLR start "rule__Labels__Group_2__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17285:1: rule__Labels__Group_2__1 : rule__Labels__Group_2__1__Impl ;
public final void rule__Labels__Group_2__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17289:1: ( rule__Labels__Group_2__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17290:2: rule__Labels__Group_2__1__Impl
{
pushFollow(FollowSets001.FOLLOW_rule__Labels__Group_2__1__Impl_in_rule__Labels__Group_2__135532);
rule__Labels__Group_2__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Labels__Group_2__1"
// $ANTLR start "rule__Labels__Group_2__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17296:1: rule__Labels__Group_2__1__Impl : ( ( rule__Labels__FilterLabelAssignment_2_1 ) ) ;
public final void rule__Labels__Group_2__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17300:1: ( ( ( rule__Labels__FilterLabelAssignment_2_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17301:1: ( ( rule__Labels__FilterLabelAssignment_2_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17301:1: ( ( rule__Labels__FilterLabelAssignment_2_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17302:1: ( rule__Labels__FilterLabelAssignment_2_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getLabelsAccess().getFilterLabelAssignment_2_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17303:1: ( rule__Labels__FilterLabelAssignment_2_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17303:2: rule__Labels__FilterLabelAssignment_2_1
{
pushFollow(FollowSets001.FOLLOW_rule__Labels__FilterLabelAssignment_2_1_in_rule__Labels__Group_2__1__Impl35559);
rule__Labels__FilterLabelAssignment_2_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getLabelsAccess().getFilterLabelAssignment_2_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Labels__Group_2__1__Impl"
// $ANTLR start "rule__Labels__Group_3__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17317:1: rule__Labels__Group_3__0 : rule__Labels__Group_3__0__Impl rule__Labels__Group_3__1 ;
public final void rule__Labels__Group_3__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17321:1: ( rule__Labels__Group_3__0__Impl rule__Labels__Group_3__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17322:2: rule__Labels__Group_3__0__Impl rule__Labels__Group_3__1
{
pushFollow(FollowSets001.FOLLOW_rule__Labels__Group_3__0__Impl_in_rule__Labels__Group_3__035593);
rule__Labels__Group_3__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__Labels__Group_3__1_in_rule__Labels__Group_3__035596);
rule__Labels__Group_3__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Labels__Group_3__0"
// $ANTLR start "rule__Labels__Group_3__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17329:1: rule__Labels__Group_3__0__Impl : ( 'columnLabel' ) ;
public final void rule__Labels__Group_3__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17333:1: ( ( 'columnLabel' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17334:1: ( 'columnLabel' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17334:1: ( 'columnLabel' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17335:1: 'columnLabel'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getLabelsAccess().getColumnLabelKeyword_3_0());
}
match(input,118,FollowSets001.FOLLOW_118_in_rule__Labels__Group_3__0__Impl35624); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getLabelsAccess().getColumnLabelKeyword_3_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Labels__Group_3__0__Impl"
// $ANTLR start "rule__Labels__Group_3__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17348:1: rule__Labels__Group_3__1 : rule__Labels__Group_3__1__Impl ;
public final void rule__Labels__Group_3__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17352:1: ( rule__Labels__Group_3__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17353:2: rule__Labels__Group_3__1__Impl
{
pushFollow(FollowSets001.FOLLOW_rule__Labels__Group_3__1__Impl_in_rule__Labels__Group_3__135655);
rule__Labels__Group_3__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Labels__Group_3__1"
// $ANTLR start "rule__Labels__Group_3__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17359:1: rule__Labels__Group_3__1__Impl : ( ( rule__Labels__ColumnLabelAssignment_3_1 ) ) ;
public final void rule__Labels__Group_3__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17363:1: ( ( ( rule__Labels__ColumnLabelAssignment_3_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17364:1: ( ( rule__Labels__ColumnLabelAssignment_3_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17364:1: ( ( rule__Labels__ColumnLabelAssignment_3_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17365:1: ( rule__Labels__ColumnLabelAssignment_3_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getLabelsAccess().getColumnLabelAssignment_3_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17366:1: ( rule__Labels__ColumnLabelAssignment_3_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17366:2: rule__Labels__ColumnLabelAssignment_3_1
{
pushFollow(FollowSets001.FOLLOW_rule__Labels__ColumnLabelAssignment_3_1_in_rule__Labels__Group_3__1__Impl35682);
rule__Labels__ColumnLabelAssignment_3_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getLabelsAccess().getColumnLabelAssignment_3_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Labels__Group_3__1__Impl"
// $ANTLR start "rule__Labels__Group_4__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17380:1: rule__Labels__Group_4__0 : rule__Labels__Group_4__0__Impl rule__Labels__Group_4__1 ;
public final void rule__Labels__Group_4__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17384:1: ( rule__Labels__Group_4__0__Impl rule__Labels__Group_4__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17385:2: rule__Labels__Group_4__0__Impl rule__Labels__Group_4__1
{
pushFollow(FollowSets001.FOLLOW_rule__Labels__Group_4__0__Impl_in_rule__Labels__Group_4__035716);
rule__Labels__Group_4__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__Labels__Group_4__1_in_rule__Labels__Group_4__035719);
rule__Labels__Group_4__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Labels__Group_4__0"
// $ANTLR start "rule__Labels__Group_4__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17392:1: rule__Labels__Group_4__0__Impl : ( 'editorLabel' ) ;
public final void rule__Labels__Group_4__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17396:1: ( ( 'editorLabel' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17397:1: ( 'editorLabel' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17397:1: ( 'editorLabel' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17398:1: 'editorLabel'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getLabelsAccess().getEditorLabelKeyword_4_0());
}
match(input,119,FollowSets001.FOLLOW_119_in_rule__Labels__Group_4__0__Impl35747); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getLabelsAccess().getEditorLabelKeyword_4_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Labels__Group_4__0__Impl"
// $ANTLR start "rule__Labels__Group_4__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17411:1: rule__Labels__Group_4__1 : rule__Labels__Group_4__1__Impl ;
public final void rule__Labels__Group_4__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17415:1: ( rule__Labels__Group_4__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17416:2: rule__Labels__Group_4__1__Impl
{
pushFollow(FollowSets001.FOLLOW_rule__Labels__Group_4__1__Impl_in_rule__Labels__Group_4__135778);
rule__Labels__Group_4__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Labels__Group_4__1"
// $ANTLR start "rule__Labels__Group_4__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17422:1: rule__Labels__Group_4__1__Impl : ( ( rule__Labels__EditorLabelAssignment_4_1 ) ) ;
public final void rule__Labels__Group_4__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17426:1: ( ( ( rule__Labels__EditorLabelAssignment_4_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17427:1: ( ( rule__Labels__EditorLabelAssignment_4_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17427:1: ( ( rule__Labels__EditorLabelAssignment_4_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17428:1: ( rule__Labels__EditorLabelAssignment_4_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getLabelsAccess().getEditorLabelAssignment_4_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17429:1: ( rule__Labels__EditorLabelAssignment_4_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17429:2: rule__Labels__EditorLabelAssignment_4_1
{
pushFollow(FollowSets001.FOLLOW_rule__Labels__EditorLabelAssignment_4_1_in_rule__Labels__Group_4__1__Impl35805);
rule__Labels__EditorLabelAssignment_4_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getLabelsAccess().getEditorLabelAssignment_4_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Labels__Group_4__1__Impl"
// $ANTLR start "rule__Labels__Group_5__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17443:1: rule__Labels__Group_5__0 : rule__Labels__Group_5__0__Impl rule__Labels__Group_5__1 ;
public final void rule__Labels__Group_5__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17447:1: ( rule__Labels__Group_5__0__Impl rule__Labels__Group_5__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17448:2: rule__Labels__Group_5__0__Impl rule__Labels__Group_5__1
{
pushFollow(FollowSets001.FOLLOW_rule__Labels__Group_5__0__Impl_in_rule__Labels__Group_5__035839);
rule__Labels__Group_5__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__Labels__Group_5__1_in_rule__Labels__Group_5__035842);
rule__Labels__Group_5__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Labels__Group_5__0"
// $ANTLR start "rule__Labels__Group_5__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17455:1: rule__Labels__Group_5__0__Impl : ( 'toolTip' ) ;
public final void rule__Labels__Group_5__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17459:1: ( ( 'toolTip' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17460:1: ( 'toolTip' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17460:1: ( 'toolTip' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17461:1: 'toolTip'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getLabelsAccess().getToolTipKeyword_5_0());
}
match(input,120,FollowSets001.FOLLOW_120_in_rule__Labels__Group_5__0__Impl35870); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getLabelsAccess().getToolTipKeyword_5_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Labels__Group_5__0__Impl"
// $ANTLR start "rule__Labels__Group_5__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17474:1: rule__Labels__Group_5__1 : rule__Labels__Group_5__1__Impl ;
public final void rule__Labels__Group_5__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17478:1: ( rule__Labels__Group_5__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17479:2: rule__Labels__Group_5__1__Impl
{
pushFollow(FollowSets001.FOLLOW_rule__Labels__Group_5__1__Impl_in_rule__Labels__Group_5__135901);
rule__Labels__Group_5__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Labels__Group_5__1"
// $ANTLR start "rule__Labels__Group_5__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17485:1: rule__Labels__Group_5__1__Impl : ( ( rule__Labels__ToolTipAssignment_5_1 ) ) ;
public final void rule__Labels__Group_5__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17489:1: ( ( ( rule__Labels__ToolTipAssignment_5_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17490:1: ( ( rule__Labels__ToolTipAssignment_5_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17490:1: ( ( rule__Labels__ToolTipAssignment_5_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17491:1: ( rule__Labels__ToolTipAssignment_5_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getLabelsAccess().getToolTipAssignment_5_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17492:1: ( rule__Labels__ToolTipAssignment_5_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17492:2: rule__Labels__ToolTipAssignment_5_1
{
pushFollow(FollowSets001.FOLLOW_rule__Labels__ToolTipAssignment_5_1_in_rule__Labels__Group_5__1__Impl35928);
rule__Labels__ToolTipAssignment_5_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getLabelsAccess().getToolTipAssignment_5_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Labels__Group_5__1__Impl"
// $ANTLR start "rule__BaseDictionaryControl__Group__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17506:1: rule__BaseDictionaryControl__Group__0 : rule__BaseDictionaryControl__Group__0__Impl rule__BaseDictionaryControl__Group__1 ;
public final void rule__BaseDictionaryControl__Group__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17510:1: ( rule__BaseDictionaryControl__Group__0__Impl rule__BaseDictionaryControl__Group__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17511:2: rule__BaseDictionaryControl__Group__0__Impl rule__BaseDictionaryControl__Group__1
{
pushFollow(FollowSets001.FOLLOW_rule__BaseDictionaryControl__Group__0__Impl_in_rule__BaseDictionaryControl__Group__035962);
rule__BaseDictionaryControl__Group__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__BaseDictionaryControl__Group__1_in_rule__BaseDictionaryControl__Group__035965);
rule__BaseDictionaryControl__Group__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__BaseDictionaryControl__Group__0"
// $ANTLR start "rule__BaseDictionaryControl__Group__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17518:1: rule__BaseDictionaryControl__Group__0__Impl : ( ( rule__BaseDictionaryControl__Group_0__0 )? ) ;
public final void rule__BaseDictionaryControl__Group__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17522:1: ( ( ( rule__BaseDictionaryControl__Group_0__0 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17523:1: ( ( rule__BaseDictionaryControl__Group_0__0 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17523:1: ( ( rule__BaseDictionaryControl__Group_0__0 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17524:1: ( rule__BaseDictionaryControl__Group_0__0 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getBaseDictionaryControlAccess().getGroup_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17525:1: ( rule__BaseDictionaryControl__Group_0__0 )?
int alt137=2;
int LA137_0 = input.LA(1);
if ( (LA137_0==114) ) {
alt137=1;
}
switch (alt137) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17525:2: rule__BaseDictionaryControl__Group_0__0
{
pushFollow(FollowSets001.FOLLOW_rule__BaseDictionaryControl__Group_0__0_in_rule__BaseDictionaryControl__Group__0__Impl35992);
rule__BaseDictionaryControl__Group_0__0();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getBaseDictionaryControlAccess().getGroup_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__BaseDictionaryControl__Group__0__Impl"
// $ANTLR start "rule__BaseDictionaryControl__Group__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17535:1: rule__BaseDictionaryControl__Group__1 : rule__BaseDictionaryControl__Group__1__Impl rule__BaseDictionaryControl__Group__2 ;
public final void rule__BaseDictionaryControl__Group__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17539:1: ( rule__BaseDictionaryControl__Group__1__Impl rule__BaseDictionaryControl__Group__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17540:2: rule__BaseDictionaryControl__Group__1__Impl rule__BaseDictionaryControl__Group__2
{
pushFollow(FollowSets001.FOLLOW_rule__BaseDictionaryControl__Group__1__Impl_in_rule__BaseDictionaryControl__Group__136023);
rule__BaseDictionaryControl__Group__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__BaseDictionaryControl__Group__2_in_rule__BaseDictionaryControl__Group__136026);
rule__BaseDictionaryControl__Group__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__BaseDictionaryControl__Group__1"
// $ANTLR start "rule__BaseDictionaryControl__Group__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17547:1: rule__BaseDictionaryControl__Group__1__Impl : ( ( rule__BaseDictionaryControl__Group_1__0 )? ) ;
public final void rule__BaseDictionaryControl__Group__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17551:1: ( ( ( rule__BaseDictionaryControl__Group_1__0 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17552:1: ( ( rule__BaseDictionaryControl__Group_1__0 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17552:1: ( ( rule__BaseDictionaryControl__Group_1__0 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17553:1: ( rule__BaseDictionaryControl__Group_1__0 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getBaseDictionaryControlAccess().getGroup_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17554:1: ( rule__BaseDictionaryControl__Group_1__0 )?
int alt138=2;
int LA138_0 = input.LA(1);
if ( (LA138_0==121) ) {
alt138=1;
}
switch (alt138) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17554:2: rule__BaseDictionaryControl__Group_1__0
{
pushFollow(FollowSets001.FOLLOW_rule__BaseDictionaryControl__Group_1__0_in_rule__BaseDictionaryControl__Group__1__Impl36053);
rule__BaseDictionaryControl__Group_1__0();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getBaseDictionaryControlAccess().getGroup_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__BaseDictionaryControl__Group__1__Impl"
// $ANTLR start "rule__BaseDictionaryControl__Group__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17564:1: rule__BaseDictionaryControl__Group__2 : rule__BaseDictionaryControl__Group__2__Impl rule__BaseDictionaryControl__Group__3 ;
public final void rule__BaseDictionaryControl__Group__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17568:1: ( rule__BaseDictionaryControl__Group__2__Impl rule__BaseDictionaryControl__Group__3 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17569:2: rule__BaseDictionaryControl__Group__2__Impl rule__BaseDictionaryControl__Group__3
{
pushFollow(FollowSets001.FOLLOW_rule__BaseDictionaryControl__Group__2__Impl_in_rule__BaseDictionaryControl__Group__236084);
rule__BaseDictionaryControl__Group__2__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__BaseDictionaryControl__Group__3_in_rule__BaseDictionaryControl__Group__236087);
rule__BaseDictionaryControl__Group__3();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__BaseDictionaryControl__Group__2"
// $ANTLR start "rule__BaseDictionaryControl__Group__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17576:1: rule__BaseDictionaryControl__Group__2__Impl : ( ( rule__BaseDictionaryControl__LabelsAssignment_2 ) ) ;
public final void rule__BaseDictionaryControl__Group__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17580:1: ( ( ( rule__BaseDictionaryControl__LabelsAssignment_2 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17581:1: ( ( rule__BaseDictionaryControl__LabelsAssignment_2 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17581:1: ( ( rule__BaseDictionaryControl__LabelsAssignment_2 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17582:1: ( rule__BaseDictionaryControl__LabelsAssignment_2 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getBaseDictionaryControlAccess().getLabelsAssignment_2());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17583:1: ( rule__BaseDictionaryControl__LabelsAssignment_2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17583:2: rule__BaseDictionaryControl__LabelsAssignment_2
{
pushFollow(FollowSets001.FOLLOW_rule__BaseDictionaryControl__LabelsAssignment_2_in_rule__BaseDictionaryControl__Group__2__Impl36114);
rule__BaseDictionaryControl__LabelsAssignment_2();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getBaseDictionaryControlAccess().getLabelsAssignment_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__BaseDictionaryControl__Group__2__Impl"
// $ANTLR start "rule__BaseDictionaryControl__Group__3"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17593:1: rule__BaseDictionaryControl__Group__3 : rule__BaseDictionaryControl__Group__3__Impl rule__BaseDictionaryControl__Group__4 ;
public final void rule__BaseDictionaryControl__Group__3() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17597:1: ( rule__BaseDictionaryControl__Group__3__Impl rule__BaseDictionaryControl__Group__4 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17598:2: rule__BaseDictionaryControl__Group__3__Impl rule__BaseDictionaryControl__Group__4
{
pushFollow(FollowSets001.FOLLOW_rule__BaseDictionaryControl__Group__3__Impl_in_rule__BaseDictionaryControl__Group__336144);
rule__BaseDictionaryControl__Group__3__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__BaseDictionaryControl__Group__4_in_rule__BaseDictionaryControl__Group__336147);
rule__BaseDictionaryControl__Group__4();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__BaseDictionaryControl__Group__3"
// $ANTLR start "rule__BaseDictionaryControl__Group__3__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17605:1: rule__BaseDictionaryControl__Group__3__Impl : ( ( rule__BaseDictionaryControl__MandatoryAssignment_3 )? ) ;
public final void rule__BaseDictionaryControl__Group__3__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17609:1: ( ( ( rule__BaseDictionaryControl__MandatoryAssignment_3 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17610:1: ( ( rule__BaseDictionaryControl__MandatoryAssignment_3 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17610:1: ( ( rule__BaseDictionaryControl__MandatoryAssignment_3 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17611:1: ( rule__BaseDictionaryControl__MandatoryAssignment_3 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getBaseDictionaryControlAccess().getMandatoryAssignment_3());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17612:1: ( rule__BaseDictionaryControl__MandatoryAssignment_3 )?
int alt139=2;
int LA139_0 = input.LA(1);
if ( (LA139_0==179) ) {
alt139=1;
}
switch (alt139) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17612:2: rule__BaseDictionaryControl__MandatoryAssignment_3
{
pushFollow(FollowSets001.FOLLOW_rule__BaseDictionaryControl__MandatoryAssignment_3_in_rule__BaseDictionaryControl__Group__3__Impl36174);
rule__BaseDictionaryControl__MandatoryAssignment_3();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getBaseDictionaryControlAccess().getMandatoryAssignment_3());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__BaseDictionaryControl__Group__3__Impl"
// $ANTLR start "rule__BaseDictionaryControl__Group__4"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17622:1: rule__BaseDictionaryControl__Group__4 : rule__BaseDictionaryControl__Group__4__Impl rule__BaseDictionaryControl__Group__5 ;
public final void rule__BaseDictionaryControl__Group__4() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17626:1: ( rule__BaseDictionaryControl__Group__4__Impl rule__BaseDictionaryControl__Group__5 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17627:2: rule__BaseDictionaryControl__Group__4__Impl rule__BaseDictionaryControl__Group__5
{
pushFollow(FollowSets001.FOLLOW_rule__BaseDictionaryControl__Group__4__Impl_in_rule__BaseDictionaryControl__Group__436205);
rule__BaseDictionaryControl__Group__4__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__BaseDictionaryControl__Group__5_in_rule__BaseDictionaryControl__Group__436208);
rule__BaseDictionaryControl__Group__5();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__BaseDictionaryControl__Group__4"
// $ANTLR start "rule__BaseDictionaryControl__Group__4__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17634:1: rule__BaseDictionaryControl__Group__4__Impl : ( ( rule__BaseDictionaryControl__Group_4__0 )? ) ;
public final void rule__BaseDictionaryControl__Group__4__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17638:1: ( ( ( rule__BaseDictionaryControl__Group_4__0 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17639:1: ( ( rule__BaseDictionaryControl__Group_4__0 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17639:1: ( ( rule__BaseDictionaryControl__Group_4__0 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17640:1: ( rule__BaseDictionaryControl__Group_4__0 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getBaseDictionaryControlAccess().getGroup_4());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17641:1: ( rule__BaseDictionaryControl__Group_4__0 )?
int alt140=2;
int LA140_0 = input.LA(1);
if ( (LA140_0==81) ) {
alt140=1;
}
switch (alt140) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17641:2: rule__BaseDictionaryControl__Group_4__0
{
pushFollow(FollowSets001.FOLLOW_rule__BaseDictionaryControl__Group_4__0_in_rule__BaseDictionaryControl__Group__4__Impl36235);
rule__BaseDictionaryControl__Group_4__0();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getBaseDictionaryControlAccess().getGroup_4());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__BaseDictionaryControl__Group__4__Impl"
// $ANTLR start "rule__BaseDictionaryControl__Group__5"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17651:1: rule__BaseDictionaryControl__Group__5 : rule__BaseDictionaryControl__Group__5__Impl ;
public final void rule__BaseDictionaryControl__Group__5() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17655:1: ( rule__BaseDictionaryControl__Group__5__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17656:2: rule__BaseDictionaryControl__Group__5__Impl
{
pushFollow(FollowSets001.FOLLOW_rule__BaseDictionaryControl__Group__5__Impl_in_rule__BaseDictionaryControl__Group__536266);
rule__BaseDictionaryControl__Group__5__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__BaseDictionaryControl__Group__5"
// $ANTLR start "rule__BaseDictionaryControl__Group__5__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17662:1: rule__BaseDictionaryControl__Group__5__Impl : ( ( rule__BaseDictionaryControl__Group_5__0 )? ) ;
public final void rule__BaseDictionaryControl__Group__5__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17666:1: ( ( ( rule__BaseDictionaryControl__Group_5__0 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17667:1: ( ( rule__BaseDictionaryControl__Group_5__0 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17667:1: ( ( rule__BaseDictionaryControl__Group_5__0 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17668:1: ( rule__BaseDictionaryControl__Group_5__0 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getBaseDictionaryControlAccess().getGroup_5());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17669:1: ( rule__BaseDictionaryControl__Group_5__0 )?
int alt141=2;
int LA141_0 = input.LA(1);
if ( (LA141_0==122) ) {
alt141=1;
}
switch (alt141) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17669:2: rule__BaseDictionaryControl__Group_5__0
{
pushFollow(FollowSets001.FOLLOW_rule__BaseDictionaryControl__Group_5__0_in_rule__BaseDictionaryControl__Group__5__Impl36293);
rule__BaseDictionaryControl__Group_5__0();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getBaseDictionaryControlAccess().getGroup_5());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__BaseDictionaryControl__Group__5__Impl"
// $ANTLR start "rule__BaseDictionaryControl__Group_0__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17691:1: rule__BaseDictionaryControl__Group_0__0 : rule__BaseDictionaryControl__Group_0__0__Impl rule__BaseDictionaryControl__Group_0__1 ;
public final void rule__BaseDictionaryControl__Group_0__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17695:1: ( rule__BaseDictionaryControl__Group_0__0__Impl rule__BaseDictionaryControl__Group_0__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17696:2: rule__BaseDictionaryControl__Group_0__0__Impl rule__BaseDictionaryControl__Group_0__1
{
pushFollow(FollowSets001.FOLLOW_rule__BaseDictionaryControl__Group_0__0__Impl_in_rule__BaseDictionaryControl__Group_0__036336);
rule__BaseDictionaryControl__Group_0__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__BaseDictionaryControl__Group_0__1_in_rule__BaseDictionaryControl__Group_0__036339);
rule__BaseDictionaryControl__Group_0__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__BaseDictionaryControl__Group_0__0"
// $ANTLR start "rule__BaseDictionaryControl__Group_0__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17703:1: rule__BaseDictionaryControl__Group_0__0__Impl : ( 'entityattribute' ) ;
public final void rule__BaseDictionaryControl__Group_0__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17707:1: ( ( 'entityattribute' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17708:1: ( 'entityattribute' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17708:1: ( 'entityattribute' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17709:1: 'entityattribute'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getBaseDictionaryControlAccess().getEntityattributeKeyword_0_0());
}
match(input,114,FollowSets001.FOLLOW_114_in_rule__BaseDictionaryControl__Group_0__0__Impl36367); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getBaseDictionaryControlAccess().getEntityattributeKeyword_0_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__BaseDictionaryControl__Group_0__0__Impl"
// $ANTLR start "rule__BaseDictionaryControl__Group_0__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17722:1: rule__BaseDictionaryControl__Group_0__1 : rule__BaseDictionaryControl__Group_0__1__Impl ;
public final void rule__BaseDictionaryControl__Group_0__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17726:1: ( rule__BaseDictionaryControl__Group_0__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17727:2: rule__BaseDictionaryControl__Group_0__1__Impl
{
pushFollow(FollowSets001.FOLLOW_rule__BaseDictionaryControl__Group_0__1__Impl_in_rule__BaseDictionaryControl__Group_0__136398);
rule__BaseDictionaryControl__Group_0__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__BaseDictionaryControl__Group_0__1"
// $ANTLR start "rule__BaseDictionaryControl__Group_0__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17733:1: rule__BaseDictionaryControl__Group_0__1__Impl : ( ( rule__BaseDictionaryControl__EntityattributeAssignment_0_1 ) ) ;
public final void rule__BaseDictionaryControl__Group_0__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17737:1: ( ( ( rule__BaseDictionaryControl__EntityattributeAssignment_0_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17738:1: ( ( rule__BaseDictionaryControl__EntityattributeAssignment_0_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17738:1: ( ( rule__BaseDictionaryControl__EntityattributeAssignment_0_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17739:1: ( rule__BaseDictionaryControl__EntityattributeAssignment_0_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getBaseDictionaryControlAccess().getEntityattributeAssignment_0_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17740:1: ( rule__BaseDictionaryControl__EntityattributeAssignment_0_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17740:2: rule__BaseDictionaryControl__EntityattributeAssignment_0_1
{
pushFollow(FollowSets001.FOLLOW_rule__BaseDictionaryControl__EntityattributeAssignment_0_1_in_rule__BaseDictionaryControl__Group_0__1__Impl36425);
rule__BaseDictionaryControl__EntityattributeAssignment_0_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getBaseDictionaryControlAccess().getEntityattributeAssignment_0_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__BaseDictionaryControl__Group_0__1__Impl"
// $ANTLR start "rule__BaseDictionaryControl__Group_1__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17754:1: rule__BaseDictionaryControl__Group_1__0 : rule__BaseDictionaryControl__Group_1__0__Impl rule__BaseDictionaryControl__Group_1__1 ;
public final void rule__BaseDictionaryControl__Group_1__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17758:1: ( rule__BaseDictionaryControl__Group_1__0__Impl rule__BaseDictionaryControl__Group_1__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17759:2: rule__BaseDictionaryControl__Group_1__0__Impl rule__BaseDictionaryControl__Group_1__1
{
pushFollow(FollowSets001.FOLLOW_rule__BaseDictionaryControl__Group_1__0__Impl_in_rule__BaseDictionaryControl__Group_1__036459);
rule__BaseDictionaryControl__Group_1__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__BaseDictionaryControl__Group_1__1_in_rule__BaseDictionaryControl__Group_1__036462);
rule__BaseDictionaryControl__Group_1__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__BaseDictionaryControl__Group_1__0"
// $ANTLR start "rule__BaseDictionaryControl__Group_1__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17766:1: rule__BaseDictionaryControl__Group_1__0__Impl : ( 'type' ) ;
public final void rule__BaseDictionaryControl__Group_1__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17770:1: ( ( 'type' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17771:1: ( 'type' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17771:1: ( 'type' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17772:1: 'type'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getBaseDictionaryControlAccess().getTypeKeyword_1_0());
}
match(input,121,FollowSets001.FOLLOW_121_in_rule__BaseDictionaryControl__Group_1__0__Impl36490); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getBaseDictionaryControlAccess().getTypeKeyword_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__BaseDictionaryControl__Group_1__0__Impl"
// $ANTLR start "rule__BaseDictionaryControl__Group_1__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17785:1: rule__BaseDictionaryControl__Group_1__1 : rule__BaseDictionaryControl__Group_1__1__Impl ;
public final void rule__BaseDictionaryControl__Group_1__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17789:1: ( rule__BaseDictionaryControl__Group_1__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17790:2: rule__BaseDictionaryControl__Group_1__1__Impl
{
pushFollow(FollowSets001.FOLLOW_rule__BaseDictionaryControl__Group_1__1__Impl_in_rule__BaseDictionaryControl__Group_1__136521);
rule__BaseDictionaryControl__Group_1__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__BaseDictionaryControl__Group_1__1"
// $ANTLR start "rule__BaseDictionaryControl__Group_1__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17796:1: rule__BaseDictionaryControl__Group_1__1__Impl : ( ( rule__BaseDictionaryControl__TypeAssignment_1_1 ) ) ;
public final void rule__BaseDictionaryControl__Group_1__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17800:1: ( ( ( rule__BaseDictionaryControl__TypeAssignment_1_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17801:1: ( ( rule__BaseDictionaryControl__TypeAssignment_1_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17801:1: ( ( rule__BaseDictionaryControl__TypeAssignment_1_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17802:1: ( rule__BaseDictionaryControl__TypeAssignment_1_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getBaseDictionaryControlAccess().getTypeAssignment_1_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17803:1: ( rule__BaseDictionaryControl__TypeAssignment_1_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17803:2: rule__BaseDictionaryControl__TypeAssignment_1_1
{
pushFollow(FollowSets001.FOLLOW_rule__BaseDictionaryControl__TypeAssignment_1_1_in_rule__BaseDictionaryControl__Group_1__1__Impl36548);
rule__BaseDictionaryControl__TypeAssignment_1_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getBaseDictionaryControlAccess().getTypeAssignment_1_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__BaseDictionaryControl__Group_1__1__Impl"
// $ANTLR start "rule__BaseDictionaryControl__Group_4__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17817:1: rule__BaseDictionaryControl__Group_4__0 : rule__BaseDictionaryControl__Group_4__0__Impl rule__BaseDictionaryControl__Group_4__1 ;
public final void rule__BaseDictionaryControl__Group_4__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17821:1: ( rule__BaseDictionaryControl__Group_4__0__Impl rule__BaseDictionaryControl__Group_4__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17822:2: rule__BaseDictionaryControl__Group_4__0__Impl rule__BaseDictionaryControl__Group_4__1
{
pushFollow(FollowSets001.FOLLOW_rule__BaseDictionaryControl__Group_4__0__Impl_in_rule__BaseDictionaryControl__Group_4__036582);
rule__BaseDictionaryControl__Group_4__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__BaseDictionaryControl__Group_4__1_in_rule__BaseDictionaryControl__Group_4__036585);
rule__BaseDictionaryControl__Group_4__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__BaseDictionaryControl__Group_4__0"
// $ANTLR start "rule__BaseDictionaryControl__Group_4__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17829:1: rule__BaseDictionaryControl__Group_4__0__Impl : ( 'width' ) ;
public final void rule__BaseDictionaryControl__Group_4__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17833:1: ( ( 'width' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17834:1: ( 'width' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17834:1: ( 'width' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17835:1: 'width'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getBaseDictionaryControlAccess().getWidthKeyword_4_0());
}
match(input,81,FollowSets001.FOLLOW_81_in_rule__BaseDictionaryControl__Group_4__0__Impl36613); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getBaseDictionaryControlAccess().getWidthKeyword_4_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__BaseDictionaryControl__Group_4__0__Impl"
// $ANTLR start "rule__BaseDictionaryControl__Group_4__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17848:1: rule__BaseDictionaryControl__Group_4__1 : rule__BaseDictionaryControl__Group_4__1__Impl ;
public final void rule__BaseDictionaryControl__Group_4__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17852:1: ( rule__BaseDictionaryControl__Group_4__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17853:2: rule__BaseDictionaryControl__Group_4__1__Impl
{
pushFollow(FollowSets001.FOLLOW_rule__BaseDictionaryControl__Group_4__1__Impl_in_rule__BaseDictionaryControl__Group_4__136644);
rule__BaseDictionaryControl__Group_4__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__BaseDictionaryControl__Group_4__1"
// $ANTLR start "rule__BaseDictionaryControl__Group_4__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17859:1: rule__BaseDictionaryControl__Group_4__1__Impl : ( ( rule__BaseDictionaryControl__WidthAssignment_4_1 ) ) ;
public final void rule__BaseDictionaryControl__Group_4__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17863:1: ( ( ( rule__BaseDictionaryControl__WidthAssignment_4_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17864:1: ( ( rule__BaseDictionaryControl__WidthAssignment_4_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17864:1: ( ( rule__BaseDictionaryControl__WidthAssignment_4_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17865:1: ( rule__BaseDictionaryControl__WidthAssignment_4_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getBaseDictionaryControlAccess().getWidthAssignment_4_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17866:1: ( rule__BaseDictionaryControl__WidthAssignment_4_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17866:2: rule__BaseDictionaryControl__WidthAssignment_4_1
{
pushFollow(FollowSets001.FOLLOW_rule__BaseDictionaryControl__WidthAssignment_4_1_in_rule__BaseDictionaryControl__Group_4__1__Impl36671);
rule__BaseDictionaryControl__WidthAssignment_4_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getBaseDictionaryControlAccess().getWidthAssignment_4_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__BaseDictionaryControl__Group_4__1__Impl"
// $ANTLR start "rule__BaseDictionaryControl__Group_5__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17880:1: rule__BaseDictionaryControl__Group_5__0 : rule__BaseDictionaryControl__Group_5__0__Impl rule__BaseDictionaryControl__Group_5__1 ;
public final void rule__BaseDictionaryControl__Group_5__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17884:1: ( rule__BaseDictionaryControl__Group_5__0__Impl rule__BaseDictionaryControl__Group_5__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17885:2: rule__BaseDictionaryControl__Group_5__0__Impl rule__BaseDictionaryControl__Group_5__1
{
pushFollow(FollowSets001.FOLLOW_rule__BaseDictionaryControl__Group_5__0__Impl_in_rule__BaseDictionaryControl__Group_5__036705);
rule__BaseDictionaryControl__Group_5__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__BaseDictionaryControl__Group_5__1_in_rule__BaseDictionaryControl__Group_5__036708);
rule__BaseDictionaryControl__Group_5__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__BaseDictionaryControl__Group_5__0"
// $ANTLR start "rule__BaseDictionaryControl__Group_5__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17892:1: rule__BaseDictionaryControl__Group_5__0__Impl : ( 'readonly' ) ;
public final void rule__BaseDictionaryControl__Group_5__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17896:1: ( ( 'readonly' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17897:1: ( 'readonly' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17897:1: ( 'readonly' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17898:1: 'readonly'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getBaseDictionaryControlAccess().getReadonlyKeyword_5_0());
}
match(input,122,FollowSets001.FOLLOW_122_in_rule__BaseDictionaryControl__Group_5__0__Impl36736); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getBaseDictionaryControlAccess().getReadonlyKeyword_5_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__BaseDictionaryControl__Group_5__0__Impl"
// $ANTLR start "rule__BaseDictionaryControl__Group_5__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17911:1: rule__BaseDictionaryControl__Group_5__1 : rule__BaseDictionaryControl__Group_5__1__Impl ;
public final void rule__BaseDictionaryControl__Group_5__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17915:1: ( rule__BaseDictionaryControl__Group_5__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17916:2: rule__BaseDictionaryControl__Group_5__1__Impl
{
pushFollow(FollowSets001.FOLLOW_rule__BaseDictionaryControl__Group_5__1__Impl_in_rule__BaseDictionaryControl__Group_5__136767);
rule__BaseDictionaryControl__Group_5__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__BaseDictionaryControl__Group_5__1"
// $ANTLR start "rule__BaseDictionaryControl__Group_5__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17922:1: rule__BaseDictionaryControl__Group_5__1__Impl : ( ( rule__BaseDictionaryControl__ReadonlyAssignment_5_1 ) ) ;
public final void rule__BaseDictionaryControl__Group_5__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17926:1: ( ( ( rule__BaseDictionaryControl__ReadonlyAssignment_5_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17927:1: ( ( rule__BaseDictionaryControl__ReadonlyAssignment_5_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17927:1: ( ( rule__BaseDictionaryControl__ReadonlyAssignment_5_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17928:1: ( rule__BaseDictionaryControl__ReadonlyAssignment_5_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getBaseDictionaryControlAccess().getReadonlyAssignment_5_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17929:1: ( rule__BaseDictionaryControl__ReadonlyAssignment_5_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17929:2: rule__BaseDictionaryControl__ReadonlyAssignment_5_1
{
pushFollow(FollowSets001.FOLLOW_rule__BaseDictionaryControl__ReadonlyAssignment_5_1_in_rule__BaseDictionaryControl__Group_5__1__Impl36794);
rule__BaseDictionaryControl__ReadonlyAssignment_5_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getBaseDictionaryControlAccess().getReadonlyAssignment_5_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__BaseDictionaryControl__Group_5__1__Impl"
// $ANTLR start "rule__DictionaryControlGroupOptionMultiFilterField__Group__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17943:1: rule__DictionaryControlGroupOptionMultiFilterField__Group__0 : rule__DictionaryControlGroupOptionMultiFilterField__Group__0__Impl rule__DictionaryControlGroupOptionMultiFilterField__Group__1 ;
public final void rule__DictionaryControlGroupOptionMultiFilterField__Group__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17947:1: ( rule__DictionaryControlGroupOptionMultiFilterField__Group__0__Impl rule__DictionaryControlGroupOptionMultiFilterField__Group__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17948:2: rule__DictionaryControlGroupOptionMultiFilterField__Group__0__Impl rule__DictionaryControlGroupOptionMultiFilterField__Group__1
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryControlGroupOptionMultiFilterField__Group__0__Impl_in_rule__DictionaryControlGroupOptionMultiFilterField__Group__036828);
rule__DictionaryControlGroupOptionMultiFilterField__Group__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryControlGroupOptionMultiFilterField__Group__1_in_rule__DictionaryControlGroupOptionMultiFilterField__Group__036831);
rule__DictionaryControlGroupOptionMultiFilterField__Group__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryControlGroupOptionMultiFilterField__Group__0"
// $ANTLR start "rule__DictionaryControlGroupOptionMultiFilterField__Group__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17955:1: rule__DictionaryControlGroupOptionMultiFilterField__Group__0__Impl : ( () ) ;
public final void rule__DictionaryControlGroupOptionMultiFilterField__Group__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17959:1: ( ( () ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17960:1: ( () )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17960:1: ( () )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17961:1: ()
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryControlGroupOptionMultiFilterFieldAccess().getDictionaryControlGroupOptionMultiFilterFieldAction_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17962:1: ()
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17964:1:
{
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryControlGroupOptionMultiFilterFieldAccess().getDictionaryControlGroupOptionMultiFilterFieldAction_0());
}
}
}
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryControlGroupOptionMultiFilterField__Group__0__Impl"
// $ANTLR start "rule__DictionaryControlGroupOptionMultiFilterField__Group__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17974:1: rule__DictionaryControlGroupOptionMultiFilterField__Group__1 : rule__DictionaryControlGroupOptionMultiFilterField__Group__1__Impl ;
public final void rule__DictionaryControlGroupOptionMultiFilterField__Group__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17978:1: ( rule__DictionaryControlGroupOptionMultiFilterField__Group__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17979:2: rule__DictionaryControlGroupOptionMultiFilterField__Group__1__Impl
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryControlGroupOptionMultiFilterField__Group__1__Impl_in_rule__DictionaryControlGroupOptionMultiFilterField__Group__136889);
rule__DictionaryControlGroupOptionMultiFilterField__Group__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryControlGroupOptionMultiFilterField__Group__1"
// $ANTLR start "rule__DictionaryControlGroupOptionMultiFilterField__Group__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17985:1: rule__DictionaryControlGroupOptionMultiFilterField__Group__1__Impl : ( ( rule__DictionaryControlGroupOptionMultiFilterField__Group_1__0 )? ) ;
public final void rule__DictionaryControlGroupOptionMultiFilterField__Group__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17989:1: ( ( ( rule__DictionaryControlGroupOptionMultiFilterField__Group_1__0 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17990:1: ( ( rule__DictionaryControlGroupOptionMultiFilterField__Group_1__0 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17990:1: ( ( rule__DictionaryControlGroupOptionMultiFilterField__Group_1__0 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17991:1: ( rule__DictionaryControlGroupOptionMultiFilterField__Group_1__0 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryControlGroupOptionMultiFilterFieldAccess().getGroup_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17992:1: ( rule__DictionaryControlGroupOptionMultiFilterField__Group_1__0 )?
int alt142=2;
int LA142_0 = input.LA(1);
if ( (LA142_0==123) ) {
alt142=1;
}
switch (alt142) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:17992:2: rule__DictionaryControlGroupOptionMultiFilterField__Group_1__0
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryControlGroupOptionMultiFilterField__Group_1__0_in_rule__DictionaryControlGroupOptionMultiFilterField__Group__1__Impl36916);
rule__DictionaryControlGroupOptionMultiFilterField__Group_1__0();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryControlGroupOptionMultiFilterFieldAccess().getGroup_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryControlGroupOptionMultiFilterField__Group__1__Impl"
// $ANTLR start "rule__DictionaryControlGroupOptionMultiFilterField__Group_1__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18006:1: rule__DictionaryControlGroupOptionMultiFilterField__Group_1__0 : rule__DictionaryControlGroupOptionMultiFilterField__Group_1__0__Impl rule__DictionaryControlGroupOptionMultiFilterField__Group_1__1 ;
public final void rule__DictionaryControlGroupOptionMultiFilterField__Group_1__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18010:1: ( rule__DictionaryControlGroupOptionMultiFilterField__Group_1__0__Impl rule__DictionaryControlGroupOptionMultiFilterField__Group_1__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18011:2: rule__DictionaryControlGroupOptionMultiFilterField__Group_1__0__Impl rule__DictionaryControlGroupOptionMultiFilterField__Group_1__1
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryControlGroupOptionMultiFilterField__Group_1__0__Impl_in_rule__DictionaryControlGroupOptionMultiFilterField__Group_1__036951);
rule__DictionaryControlGroupOptionMultiFilterField__Group_1__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryControlGroupOptionMultiFilterField__Group_1__1_in_rule__DictionaryControlGroupOptionMultiFilterField__Group_1__036954);
rule__DictionaryControlGroupOptionMultiFilterField__Group_1__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryControlGroupOptionMultiFilterField__Group_1__0"
// $ANTLR start "rule__DictionaryControlGroupOptionMultiFilterField__Group_1__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18018:1: rule__DictionaryControlGroupOptionMultiFilterField__Group_1__0__Impl : ( 'multiFilterField' ) ;
public final void rule__DictionaryControlGroupOptionMultiFilterField__Group_1__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18022:1: ( ( 'multiFilterField' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18023:1: ( 'multiFilterField' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18023:1: ( 'multiFilterField' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18024:1: 'multiFilterField'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryControlGroupOptionMultiFilterFieldAccess().getMultiFilterFieldKeyword_1_0());
}
match(input,123,FollowSets001.FOLLOW_123_in_rule__DictionaryControlGroupOptionMultiFilterField__Group_1__0__Impl36982); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryControlGroupOptionMultiFilterFieldAccess().getMultiFilterFieldKeyword_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryControlGroupOptionMultiFilterField__Group_1__0__Impl"
// $ANTLR start "rule__DictionaryControlGroupOptionMultiFilterField__Group_1__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18037:1: rule__DictionaryControlGroupOptionMultiFilterField__Group_1__1 : rule__DictionaryControlGroupOptionMultiFilterField__Group_1__1__Impl ;
public final void rule__DictionaryControlGroupOptionMultiFilterField__Group_1__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18041:1: ( rule__DictionaryControlGroupOptionMultiFilterField__Group_1__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18042:2: rule__DictionaryControlGroupOptionMultiFilterField__Group_1__1__Impl
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryControlGroupOptionMultiFilterField__Group_1__1__Impl_in_rule__DictionaryControlGroupOptionMultiFilterField__Group_1__137013);
rule__DictionaryControlGroupOptionMultiFilterField__Group_1__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryControlGroupOptionMultiFilterField__Group_1__1"
// $ANTLR start "rule__DictionaryControlGroupOptionMultiFilterField__Group_1__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18048:1: rule__DictionaryControlGroupOptionMultiFilterField__Group_1__1__Impl : ( ( rule__DictionaryControlGroupOptionMultiFilterField__MultiFilterFieldAssignment_1_1 ) ) ;
public final void rule__DictionaryControlGroupOptionMultiFilterField__Group_1__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18052:1: ( ( ( rule__DictionaryControlGroupOptionMultiFilterField__MultiFilterFieldAssignment_1_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18053:1: ( ( rule__DictionaryControlGroupOptionMultiFilterField__MultiFilterFieldAssignment_1_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18053:1: ( ( rule__DictionaryControlGroupOptionMultiFilterField__MultiFilterFieldAssignment_1_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18054:1: ( rule__DictionaryControlGroupOptionMultiFilterField__MultiFilterFieldAssignment_1_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryControlGroupOptionMultiFilterFieldAccess().getMultiFilterFieldAssignment_1_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18055:1: ( rule__DictionaryControlGroupOptionMultiFilterField__MultiFilterFieldAssignment_1_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18055:2: rule__DictionaryControlGroupOptionMultiFilterField__MultiFilterFieldAssignment_1_1
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryControlGroupOptionMultiFilterField__MultiFilterFieldAssignment_1_1_in_rule__DictionaryControlGroupOptionMultiFilterField__Group_1__1__Impl37040);
rule__DictionaryControlGroupOptionMultiFilterField__MultiFilterFieldAssignment_1_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryControlGroupOptionMultiFilterFieldAccess().getMultiFilterFieldAssignment_1_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryControlGroupOptionMultiFilterField__Group_1__1__Impl"
// $ANTLR start "rule__DictionaryControlGroupOptionsContainer__Group__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18069:1: rule__DictionaryControlGroupOptionsContainer__Group__0 : rule__DictionaryControlGroupOptionsContainer__Group__0__Impl rule__DictionaryControlGroupOptionsContainer__Group__1 ;
public final void rule__DictionaryControlGroupOptionsContainer__Group__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18073:1: ( rule__DictionaryControlGroupOptionsContainer__Group__0__Impl rule__DictionaryControlGroupOptionsContainer__Group__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18074:2: rule__DictionaryControlGroupOptionsContainer__Group__0__Impl rule__DictionaryControlGroupOptionsContainer__Group__1
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryControlGroupOptionsContainer__Group__0__Impl_in_rule__DictionaryControlGroupOptionsContainer__Group__037074);
rule__DictionaryControlGroupOptionsContainer__Group__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryControlGroupOptionsContainer__Group__1_in_rule__DictionaryControlGroupOptionsContainer__Group__037077);
rule__DictionaryControlGroupOptionsContainer__Group__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryControlGroupOptionsContainer__Group__0"
// $ANTLR start "rule__DictionaryControlGroupOptionsContainer__Group__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18081:1: rule__DictionaryControlGroupOptionsContainer__Group__0__Impl : ( () ) ;
public final void rule__DictionaryControlGroupOptionsContainer__Group__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18085:1: ( ( () ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18086:1: ( () )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18086:1: ( () )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18087:1: ()
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryControlGroupOptionsContainerAccess().getDictionaryControlGroupOptionsContainerAction_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18088:1: ()
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18090:1:
{
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryControlGroupOptionsContainerAccess().getDictionaryControlGroupOptionsContainerAction_0());
}
}
}
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryControlGroupOptionsContainer__Group__0__Impl"
// $ANTLR start "rule__DictionaryControlGroupOptionsContainer__Group__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18100:1: rule__DictionaryControlGroupOptionsContainer__Group__1 : rule__DictionaryControlGroupOptionsContainer__Group__1__Impl rule__DictionaryControlGroupOptionsContainer__Group__2 ;
public final void rule__DictionaryControlGroupOptionsContainer__Group__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18104:1: ( rule__DictionaryControlGroupOptionsContainer__Group__1__Impl rule__DictionaryControlGroupOptionsContainer__Group__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18105:2: rule__DictionaryControlGroupOptionsContainer__Group__1__Impl rule__DictionaryControlGroupOptionsContainer__Group__2
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryControlGroupOptionsContainer__Group__1__Impl_in_rule__DictionaryControlGroupOptionsContainer__Group__137135);
rule__DictionaryControlGroupOptionsContainer__Group__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryControlGroupOptionsContainer__Group__2_in_rule__DictionaryControlGroupOptionsContainer__Group__137138);
rule__DictionaryControlGroupOptionsContainer__Group__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryControlGroupOptionsContainer__Group__1"
// $ANTLR start "rule__DictionaryControlGroupOptionsContainer__Group__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18112:1: rule__DictionaryControlGroupOptionsContainer__Group__1__Impl : ( 'groupoptions' ) ;
public final void rule__DictionaryControlGroupOptionsContainer__Group__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18116:1: ( ( 'groupoptions' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18117:1: ( 'groupoptions' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18117:1: ( 'groupoptions' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18118:1: 'groupoptions'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryControlGroupOptionsContainerAccess().getGroupoptionsKeyword_1());
}
match(input,124,FollowSets001.FOLLOW_124_in_rule__DictionaryControlGroupOptionsContainer__Group__1__Impl37166); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryControlGroupOptionsContainerAccess().getGroupoptionsKeyword_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryControlGroupOptionsContainer__Group__1__Impl"
// $ANTLR start "rule__DictionaryControlGroupOptionsContainer__Group__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18131:1: rule__DictionaryControlGroupOptionsContainer__Group__2 : rule__DictionaryControlGroupOptionsContainer__Group__2__Impl rule__DictionaryControlGroupOptionsContainer__Group__3 ;
public final void rule__DictionaryControlGroupOptionsContainer__Group__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18135:1: ( rule__DictionaryControlGroupOptionsContainer__Group__2__Impl rule__DictionaryControlGroupOptionsContainer__Group__3 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18136:2: rule__DictionaryControlGroupOptionsContainer__Group__2__Impl rule__DictionaryControlGroupOptionsContainer__Group__3
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryControlGroupOptionsContainer__Group__2__Impl_in_rule__DictionaryControlGroupOptionsContainer__Group__237197);
rule__DictionaryControlGroupOptionsContainer__Group__2__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryControlGroupOptionsContainer__Group__3_in_rule__DictionaryControlGroupOptionsContainer__Group__237200);
rule__DictionaryControlGroupOptionsContainer__Group__3();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryControlGroupOptionsContainer__Group__2"
// $ANTLR start "rule__DictionaryControlGroupOptionsContainer__Group__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18143:1: rule__DictionaryControlGroupOptionsContainer__Group__2__Impl : ( '{' ) ;
public final void rule__DictionaryControlGroupOptionsContainer__Group__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18147:1: ( ( '{' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18148:1: ( '{' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18148:1: ( '{' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18149:1: '{'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryControlGroupOptionsContainerAccess().getLeftCurlyBracketKeyword_2());
}
match(input,67,FollowSets001.FOLLOW_67_in_rule__DictionaryControlGroupOptionsContainer__Group__2__Impl37228); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryControlGroupOptionsContainerAccess().getLeftCurlyBracketKeyword_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryControlGroupOptionsContainer__Group__2__Impl"
// $ANTLR start "rule__DictionaryControlGroupOptionsContainer__Group__3"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18162:1: rule__DictionaryControlGroupOptionsContainer__Group__3 : rule__DictionaryControlGroupOptionsContainer__Group__3__Impl rule__DictionaryControlGroupOptionsContainer__Group__4 ;
public final void rule__DictionaryControlGroupOptionsContainer__Group__3() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18166:1: ( rule__DictionaryControlGroupOptionsContainer__Group__3__Impl rule__DictionaryControlGroupOptionsContainer__Group__4 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18167:2: rule__DictionaryControlGroupOptionsContainer__Group__3__Impl rule__DictionaryControlGroupOptionsContainer__Group__4
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryControlGroupOptionsContainer__Group__3__Impl_in_rule__DictionaryControlGroupOptionsContainer__Group__337259);
rule__DictionaryControlGroupOptionsContainer__Group__3__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryControlGroupOptionsContainer__Group__4_in_rule__DictionaryControlGroupOptionsContainer__Group__337262);
rule__DictionaryControlGroupOptionsContainer__Group__4();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryControlGroupOptionsContainer__Group__3"
// $ANTLR start "rule__DictionaryControlGroupOptionsContainer__Group__3__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18174:1: rule__DictionaryControlGroupOptionsContainer__Group__3__Impl : ( ( rule__DictionaryControlGroupOptionsContainer__OptionsAssignment_3 ) ) ;
public final void rule__DictionaryControlGroupOptionsContainer__Group__3__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18178:1: ( ( ( rule__DictionaryControlGroupOptionsContainer__OptionsAssignment_3 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18179:1: ( ( rule__DictionaryControlGroupOptionsContainer__OptionsAssignment_3 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18179:1: ( ( rule__DictionaryControlGroupOptionsContainer__OptionsAssignment_3 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18180:1: ( rule__DictionaryControlGroupOptionsContainer__OptionsAssignment_3 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryControlGroupOptionsContainerAccess().getOptionsAssignment_3());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18181:1: ( rule__DictionaryControlGroupOptionsContainer__OptionsAssignment_3 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18181:2: rule__DictionaryControlGroupOptionsContainer__OptionsAssignment_3
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryControlGroupOptionsContainer__OptionsAssignment_3_in_rule__DictionaryControlGroupOptionsContainer__Group__3__Impl37289);
rule__DictionaryControlGroupOptionsContainer__OptionsAssignment_3();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryControlGroupOptionsContainerAccess().getOptionsAssignment_3());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryControlGroupOptionsContainer__Group__3__Impl"
// $ANTLR start "rule__DictionaryControlGroupOptionsContainer__Group__4"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18191:1: rule__DictionaryControlGroupOptionsContainer__Group__4 : rule__DictionaryControlGroupOptionsContainer__Group__4__Impl ;
public final void rule__DictionaryControlGroupOptionsContainer__Group__4() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18195:1: ( rule__DictionaryControlGroupOptionsContainer__Group__4__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18196:2: rule__DictionaryControlGroupOptionsContainer__Group__4__Impl
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryControlGroupOptionsContainer__Group__4__Impl_in_rule__DictionaryControlGroupOptionsContainer__Group__437319);
rule__DictionaryControlGroupOptionsContainer__Group__4__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryControlGroupOptionsContainer__Group__4"
// $ANTLR start "rule__DictionaryControlGroupOptionsContainer__Group__4__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18202:1: rule__DictionaryControlGroupOptionsContainer__Group__4__Impl : ( '}' ) ;
public final void rule__DictionaryControlGroupOptionsContainer__Group__4__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18206:1: ( ( '}' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18207:1: ( '}' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18207:1: ( '}' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18208:1: '}'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryControlGroupOptionsContainerAccess().getRightCurlyBracketKeyword_4());
}
match(input,68,FollowSets001.FOLLOW_68_in_rule__DictionaryControlGroupOptionsContainer__Group__4__Impl37347); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryControlGroupOptionsContainerAccess().getRightCurlyBracketKeyword_4());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryControlGroupOptionsContainer__Group__4__Impl"
// $ANTLR start "rule__DictionaryControlGroup__Group__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18231:1: rule__DictionaryControlGroup__Group__0 : rule__DictionaryControlGroup__Group__0__Impl rule__DictionaryControlGroup__Group__1 ;
public final void rule__DictionaryControlGroup__Group__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18235:1: ( rule__DictionaryControlGroup__Group__0__Impl rule__DictionaryControlGroup__Group__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18236:2: rule__DictionaryControlGroup__Group__0__Impl rule__DictionaryControlGroup__Group__1
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryControlGroup__Group__0__Impl_in_rule__DictionaryControlGroup__Group__037388);
rule__DictionaryControlGroup__Group__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryControlGroup__Group__1_in_rule__DictionaryControlGroup__Group__037391);
rule__DictionaryControlGroup__Group__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryControlGroup__Group__0"
// $ANTLR start "rule__DictionaryControlGroup__Group__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18243:1: rule__DictionaryControlGroup__Group__0__Impl : ( () ) ;
public final void rule__DictionaryControlGroup__Group__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18247:1: ( ( () ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18248:1: ( () )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18248:1: ( () )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18249:1: ()
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryControlGroupAccess().getDictionaryControlGroupAction_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18250:1: ()
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18252:1:
{
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryControlGroupAccess().getDictionaryControlGroupAction_0());
}
}
}
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryControlGroup__Group__0__Impl"
// $ANTLR start "rule__DictionaryControlGroup__Group__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18262:1: rule__DictionaryControlGroup__Group__1 : rule__DictionaryControlGroup__Group__1__Impl rule__DictionaryControlGroup__Group__2 ;
public final void rule__DictionaryControlGroup__Group__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18266:1: ( rule__DictionaryControlGroup__Group__1__Impl rule__DictionaryControlGroup__Group__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18267:2: rule__DictionaryControlGroup__Group__1__Impl rule__DictionaryControlGroup__Group__2
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryControlGroup__Group__1__Impl_in_rule__DictionaryControlGroup__Group__137449);
rule__DictionaryControlGroup__Group__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryControlGroup__Group__2_in_rule__DictionaryControlGroup__Group__137452);
rule__DictionaryControlGroup__Group__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryControlGroup__Group__1"
// $ANTLR start "rule__DictionaryControlGroup__Group__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18274:1: rule__DictionaryControlGroup__Group__1__Impl : ( 'controlgroup' ) ;
public final void rule__DictionaryControlGroup__Group__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18278:1: ( ( 'controlgroup' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18279:1: ( 'controlgroup' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18279:1: ( 'controlgroup' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18280:1: 'controlgroup'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryControlGroupAccess().getControlgroupKeyword_1());
}
match(input,125,FollowSets001.FOLLOW_125_in_rule__DictionaryControlGroup__Group__1__Impl37480); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryControlGroupAccess().getControlgroupKeyword_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryControlGroup__Group__1__Impl"
// $ANTLR start "rule__DictionaryControlGroup__Group__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18293:1: rule__DictionaryControlGroup__Group__2 : rule__DictionaryControlGroup__Group__2__Impl rule__DictionaryControlGroup__Group__3 ;
public final void rule__DictionaryControlGroup__Group__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18297:1: ( rule__DictionaryControlGroup__Group__2__Impl rule__DictionaryControlGroup__Group__3 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18298:2: rule__DictionaryControlGroup__Group__2__Impl rule__DictionaryControlGroup__Group__3
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryControlGroup__Group__2__Impl_in_rule__DictionaryControlGroup__Group__237511);
rule__DictionaryControlGroup__Group__2__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryControlGroup__Group__3_in_rule__DictionaryControlGroup__Group__237514);
rule__DictionaryControlGroup__Group__3();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryControlGroup__Group__2"
// $ANTLR start "rule__DictionaryControlGroup__Group__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18305:1: rule__DictionaryControlGroup__Group__2__Impl : ( ( rule__DictionaryControlGroup__NameAssignment_2 )? ) ;
public final void rule__DictionaryControlGroup__Group__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18309:1: ( ( ( rule__DictionaryControlGroup__NameAssignment_2 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18310:1: ( ( rule__DictionaryControlGroup__NameAssignment_2 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18310:1: ( ( rule__DictionaryControlGroup__NameAssignment_2 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18311:1: ( rule__DictionaryControlGroup__NameAssignment_2 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryControlGroupAccess().getNameAssignment_2());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18312:1: ( rule__DictionaryControlGroup__NameAssignment_2 )?
int alt143=2;
int LA143_0 = input.LA(1);
if ( (LA143_0==RULE_ID) ) {
alt143=1;
}
switch (alt143) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18312:2: rule__DictionaryControlGroup__NameAssignment_2
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryControlGroup__NameAssignment_2_in_rule__DictionaryControlGroup__Group__2__Impl37541);
rule__DictionaryControlGroup__NameAssignment_2();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryControlGroupAccess().getNameAssignment_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryControlGroup__Group__2__Impl"
// $ANTLR start "rule__DictionaryControlGroup__Group__3"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18322:1: rule__DictionaryControlGroup__Group__3 : rule__DictionaryControlGroup__Group__3__Impl rule__DictionaryControlGroup__Group__4 ;
public final void rule__DictionaryControlGroup__Group__3() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18326:1: ( rule__DictionaryControlGroup__Group__3__Impl rule__DictionaryControlGroup__Group__4 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18327:2: rule__DictionaryControlGroup__Group__3__Impl rule__DictionaryControlGroup__Group__4
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryControlGroup__Group__3__Impl_in_rule__DictionaryControlGroup__Group__337572);
rule__DictionaryControlGroup__Group__3__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryControlGroup__Group__4_in_rule__DictionaryControlGroup__Group__337575);
rule__DictionaryControlGroup__Group__4();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryControlGroup__Group__3"
// $ANTLR start "rule__DictionaryControlGroup__Group__3__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18334:1: rule__DictionaryControlGroup__Group__3__Impl : ( ( rule__DictionaryControlGroup__Group_3__0 )? ) ;
public final void rule__DictionaryControlGroup__Group__3__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18338:1: ( ( ( rule__DictionaryControlGroup__Group_3__0 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18339:1: ( ( rule__DictionaryControlGroup__Group_3__0 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18339:1: ( ( rule__DictionaryControlGroup__Group_3__0 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18340:1: ( rule__DictionaryControlGroup__Group_3__0 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryControlGroupAccess().getGroup_3());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18341:1: ( rule__DictionaryControlGroup__Group_3__0 )?
int alt144=2;
int LA144_0 = input.LA(1);
if ( (LA144_0==126) ) {
alt144=1;
}
switch (alt144) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18341:2: rule__DictionaryControlGroup__Group_3__0
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryControlGroup__Group_3__0_in_rule__DictionaryControlGroup__Group__3__Impl37602);
rule__DictionaryControlGroup__Group_3__0();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryControlGroupAccess().getGroup_3());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryControlGroup__Group__3__Impl"
// $ANTLR start "rule__DictionaryControlGroup__Group__4"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18351:1: rule__DictionaryControlGroup__Group__4 : rule__DictionaryControlGroup__Group__4__Impl ;
public final void rule__DictionaryControlGroup__Group__4() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18355:1: ( rule__DictionaryControlGroup__Group__4__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18356:2: rule__DictionaryControlGroup__Group__4__Impl
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryControlGroup__Group__4__Impl_in_rule__DictionaryControlGroup__Group__437633);
rule__DictionaryControlGroup__Group__4__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryControlGroup__Group__4"
// $ANTLR start "rule__DictionaryControlGroup__Group__4__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18362:1: rule__DictionaryControlGroup__Group__4__Impl : ( ( rule__DictionaryControlGroup__Group_4__0 )? ) ;
public final void rule__DictionaryControlGroup__Group__4__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18366:1: ( ( ( rule__DictionaryControlGroup__Group_4__0 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18367:1: ( ( rule__DictionaryControlGroup__Group_4__0 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18367:1: ( ( rule__DictionaryControlGroup__Group_4__0 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18368:1: ( rule__DictionaryControlGroup__Group_4__0 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryControlGroupAccess().getGroup_4());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18369:1: ( rule__DictionaryControlGroup__Group_4__0 )?
int alt145=2;
int LA145_0 = input.LA(1);
if ( (LA145_0==67) ) {
alt145=1;
}
switch (alt145) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18369:2: rule__DictionaryControlGroup__Group_4__0
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryControlGroup__Group_4__0_in_rule__DictionaryControlGroup__Group__4__Impl37660);
rule__DictionaryControlGroup__Group_4__0();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryControlGroupAccess().getGroup_4());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryControlGroup__Group__4__Impl"
// $ANTLR start "rule__DictionaryControlGroup__Group_3__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18389:1: rule__DictionaryControlGroup__Group_3__0 : rule__DictionaryControlGroup__Group_3__0__Impl rule__DictionaryControlGroup__Group_3__1 ;
public final void rule__DictionaryControlGroup__Group_3__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18393:1: ( rule__DictionaryControlGroup__Group_3__0__Impl rule__DictionaryControlGroup__Group_3__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18394:2: rule__DictionaryControlGroup__Group_3__0__Impl rule__DictionaryControlGroup__Group_3__1
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryControlGroup__Group_3__0__Impl_in_rule__DictionaryControlGroup__Group_3__037701);
rule__DictionaryControlGroup__Group_3__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryControlGroup__Group_3__1_in_rule__DictionaryControlGroup__Group_3__037704);
rule__DictionaryControlGroup__Group_3__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryControlGroup__Group_3__0"
// $ANTLR start "rule__DictionaryControlGroup__Group_3__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18401:1: rule__DictionaryControlGroup__Group_3__0__Impl : ( 'ref' ) ;
public final void rule__DictionaryControlGroup__Group_3__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18405:1: ( ( 'ref' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18406:1: ( 'ref' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18406:1: ( 'ref' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18407:1: 'ref'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryControlGroupAccess().getRefKeyword_3_0());
}
match(input,126,FollowSets001.FOLLOW_126_in_rule__DictionaryControlGroup__Group_3__0__Impl37732); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryControlGroupAccess().getRefKeyword_3_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryControlGroup__Group_3__0__Impl"
// $ANTLR start "rule__DictionaryControlGroup__Group_3__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18420:1: rule__DictionaryControlGroup__Group_3__1 : rule__DictionaryControlGroup__Group_3__1__Impl ;
public final void rule__DictionaryControlGroup__Group_3__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18424:1: ( rule__DictionaryControlGroup__Group_3__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18425:2: rule__DictionaryControlGroup__Group_3__1__Impl
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryControlGroup__Group_3__1__Impl_in_rule__DictionaryControlGroup__Group_3__137763);
rule__DictionaryControlGroup__Group_3__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryControlGroup__Group_3__1"
// $ANTLR start "rule__DictionaryControlGroup__Group_3__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18431:1: rule__DictionaryControlGroup__Group_3__1__Impl : ( ( rule__DictionaryControlGroup__RefAssignment_3_1 ) ) ;
public final void rule__DictionaryControlGroup__Group_3__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18435:1: ( ( ( rule__DictionaryControlGroup__RefAssignment_3_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18436:1: ( ( rule__DictionaryControlGroup__RefAssignment_3_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18436:1: ( ( rule__DictionaryControlGroup__RefAssignment_3_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18437:1: ( rule__DictionaryControlGroup__RefAssignment_3_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryControlGroupAccess().getRefAssignment_3_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18438:1: ( rule__DictionaryControlGroup__RefAssignment_3_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18438:2: rule__DictionaryControlGroup__RefAssignment_3_1
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryControlGroup__RefAssignment_3_1_in_rule__DictionaryControlGroup__Group_3__1__Impl37790);
rule__DictionaryControlGroup__RefAssignment_3_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryControlGroupAccess().getRefAssignment_3_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryControlGroup__Group_3__1__Impl"
// $ANTLR start "rule__DictionaryControlGroup__Group_4__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18452:1: rule__DictionaryControlGroup__Group_4__0 : rule__DictionaryControlGroup__Group_4__0__Impl rule__DictionaryControlGroup__Group_4__1 ;
public final void rule__DictionaryControlGroup__Group_4__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18456:1: ( rule__DictionaryControlGroup__Group_4__0__Impl rule__DictionaryControlGroup__Group_4__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18457:2: rule__DictionaryControlGroup__Group_4__0__Impl rule__DictionaryControlGroup__Group_4__1
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryControlGroup__Group_4__0__Impl_in_rule__DictionaryControlGroup__Group_4__037824);
rule__DictionaryControlGroup__Group_4__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryControlGroup__Group_4__1_in_rule__DictionaryControlGroup__Group_4__037827);
rule__DictionaryControlGroup__Group_4__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryControlGroup__Group_4__0"
// $ANTLR start "rule__DictionaryControlGroup__Group_4__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18464:1: rule__DictionaryControlGroup__Group_4__0__Impl : ( '{' ) ;
public final void rule__DictionaryControlGroup__Group_4__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18468:1: ( ( '{' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18469:1: ( '{' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18469:1: ( '{' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18470:1: '{'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryControlGroupAccess().getLeftCurlyBracketKeyword_4_0());
}
match(input,67,FollowSets001.FOLLOW_67_in_rule__DictionaryControlGroup__Group_4__0__Impl37855); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryControlGroupAccess().getLeftCurlyBracketKeyword_4_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryControlGroup__Group_4__0__Impl"
// $ANTLR start "rule__DictionaryControlGroup__Group_4__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18483:1: rule__DictionaryControlGroup__Group_4__1 : rule__DictionaryControlGroup__Group_4__1__Impl rule__DictionaryControlGroup__Group_4__2 ;
public final void rule__DictionaryControlGroup__Group_4__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18487:1: ( rule__DictionaryControlGroup__Group_4__1__Impl rule__DictionaryControlGroup__Group_4__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18488:2: rule__DictionaryControlGroup__Group_4__1__Impl rule__DictionaryControlGroup__Group_4__2
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryControlGroup__Group_4__1__Impl_in_rule__DictionaryControlGroup__Group_4__137886);
rule__DictionaryControlGroup__Group_4__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryControlGroup__Group_4__2_in_rule__DictionaryControlGroup__Group_4__137889);
rule__DictionaryControlGroup__Group_4__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryControlGroup__Group_4__1"
// $ANTLR start "rule__DictionaryControlGroup__Group_4__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18495:1: rule__DictionaryControlGroup__Group_4__1__Impl : ( ( rule__DictionaryControlGroup__ControlGroupOptionsAssignment_4_1 )? ) ;
public final void rule__DictionaryControlGroup__Group_4__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18499:1: ( ( ( rule__DictionaryControlGroup__ControlGroupOptionsAssignment_4_1 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18500:1: ( ( rule__DictionaryControlGroup__ControlGroupOptionsAssignment_4_1 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18500:1: ( ( rule__DictionaryControlGroup__ControlGroupOptionsAssignment_4_1 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18501:1: ( rule__DictionaryControlGroup__ControlGroupOptionsAssignment_4_1 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryControlGroupAccess().getControlGroupOptionsAssignment_4_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18502:1: ( rule__DictionaryControlGroup__ControlGroupOptionsAssignment_4_1 )?
int alt146=2;
int LA146_0 = input.LA(1);
if ( (LA146_0==124) ) {
alt146=1;
}
switch (alt146) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18502:2: rule__DictionaryControlGroup__ControlGroupOptionsAssignment_4_1
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryControlGroup__ControlGroupOptionsAssignment_4_1_in_rule__DictionaryControlGroup__Group_4__1__Impl37916);
rule__DictionaryControlGroup__ControlGroupOptionsAssignment_4_1();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryControlGroupAccess().getControlGroupOptionsAssignment_4_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryControlGroup__Group_4__1__Impl"
// $ANTLR start "rule__DictionaryControlGroup__Group_4__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18512:1: rule__DictionaryControlGroup__Group_4__2 : rule__DictionaryControlGroup__Group_4__2__Impl rule__DictionaryControlGroup__Group_4__3 ;
public final void rule__DictionaryControlGroup__Group_4__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18516:1: ( rule__DictionaryControlGroup__Group_4__2__Impl rule__DictionaryControlGroup__Group_4__3 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18517:2: rule__DictionaryControlGroup__Group_4__2__Impl rule__DictionaryControlGroup__Group_4__3
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryControlGroup__Group_4__2__Impl_in_rule__DictionaryControlGroup__Group_4__237947);
rule__DictionaryControlGroup__Group_4__2__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryControlGroup__Group_4__3_in_rule__DictionaryControlGroup__Group_4__237950);
rule__DictionaryControlGroup__Group_4__3();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryControlGroup__Group_4__2"
// $ANTLR start "rule__DictionaryControlGroup__Group_4__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18524:1: rule__DictionaryControlGroup__Group_4__2__Impl : ( ( rule__DictionaryControlGroup__BaseControlAssignment_4_2 ) ) ;
public final void rule__DictionaryControlGroup__Group_4__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18528:1: ( ( ( rule__DictionaryControlGroup__BaseControlAssignment_4_2 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18529:1: ( ( rule__DictionaryControlGroup__BaseControlAssignment_4_2 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18529:1: ( ( rule__DictionaryControlGroup__BaseControlAssignment_4_2 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18530:1: ( rule__DictionaryControlGroup__BaseControlAssignment_4_2 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryControlGroupAccess().getBaseControlAssignment_4_2());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18531:1: ( rule__DictionaryControlGroup__BaseControlAssignment_4_2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18531:2: rule__DictionaryControlGroup__BaseControlAssignment_4_2
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryControlGroup__BaseControlAssignment_4_2_in_rule__DictionaryControlGroup__Group_4__2__Impl37977);
rule__DictionaryControlGroup__BaseControlAssignment_4_2();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryControlGroupAccess().getBaseControlAssignment_4_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryControlGroup__Group_4__2__Impl"
// $ANTLR start "rule__DictionaryControlGroup__Group_4__3"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18541:1: rule__DictionaryControlGroup__Group_4__3 : rule__DictionaryControlGroup__Group_4__3__Impl rule__DictionaryControlGroup__Group_4__4 ;
public final void rule__DictionaryControlGroup__Group_4__3() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18545:1: ( rule__DictionaryControlGroup__Group_4__3__Impl rule__DictionaryControlGroup__Group_4__4 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18546:2: rule__DictionaryControlGroup__Group_4__3__Impl rule__DictionaryControlGroup__Group_4__4
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryControlGroup__Group_4__3__Impl_in_rule__DictionaryControlGroup__Group_4__338007);
rule__DictionaryControlGroup__Group_4__3__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryControlGroup__Group_4__4_in_rule__DictionaryControlGroup__Group_4__338010);
rule__DictionaryControlGroup__Group_4__4();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryControlGroup__Group_4__3"
// $ANTLR start "rule__DictionaryControlGroup__Group_4__3__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18553:1: rule__DictionaryControlGroup__Group_4__3__Impl : ( ( rule__DictionaryControlGroup__GroupcontrolsAssignment_4_3 )* ) ;
public final void rule__DictionaryControlGroup__Group_4__3__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18557:1: ( ( ( rule__DictionaryControlGroup__GroupcontrolsAssignment_4_3 )* ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18558:1: ( ( rule__DictionaryControlGroup__GroupcontrolsAssignment_4_3 )* )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18558:1: ( ( rule__DictionaryControlGroup__GroupcontrolsAssignment_4_3 )* )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18559:1: ( rule__DictionaryControlGroup__GroupcontrolsAssignment_4_3 )*
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryControlGroupAccess().getGroupcontrolsAssignment_4_3());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18560:1: ( rule__DictionaryControlGroup__GroupcontrolsAssignment_4_3 )*
loop147:
do {
int alt147=2;
int LA147_0 = input.LA(1);
if ( (LA147_0==125||LA147_0==127||LA147_0==129||(LA147_0>=131 && LA147_0<=136)||LA147_0==138) ) {
alt147=1;
}
switch (alt147) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18560:2: rule__DictionaryControlGroup__GroupcontrolsAssignment_4_3
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryControlGroup__GroupcontrolsAssignment_4_3_in_rule__DictionaryControlGroup__Group_4__3__Impl38037);
rule__DictionaryControlGroup__GroupcontrolsAssignment_4_3();
state._fsp--;
if (state.failed) return ;
}
break;
default :
break loop147;
}
} while (true);
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryControlGroupAccess().getGroupcontrolsAssignment_4_3());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryControlGroup__Group_4__3__Impl"
// $ANTLR start "rule__DictionaryControlGroup__Group_4__4"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18570:1: rule__DictionaryControlGroup__Group_4__4 : rule__DictionaryControlGroup__Group_4__4__Impl ;
public final void rule__DictionaryControlGroup__Group_4__4() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18574:1: ( rule__DictionaryControlGroup__Group_4__4__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18575:2: rule__DictionaryControlGroup__Group_4__4__Impl
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryControlGroup__Group_4__4__Impl_in_rule__DictionaryControlGroup__Group_4__438068);
rule__DictionaryControlGroup__Group_4__4__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryControlGroup__Group_4__4"
// $ANTLR start "rule__DictionaryControlGroup__Group_4__4__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18581:1: rule__DictionaryControlGroup__Group_4__4__Impl : ( '}' ) ;
public final void rule__DictionaryControlGroup__Group_4__4__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18585:1: ( ( '}' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18586:1: ( '}' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18586:1: ( '}' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18587:1: '}'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryControlGroupAccess().getRightCurlyBracketKeyword_4_4());
}
match(input,68,FollowSets001.FOLLOW_68_in_rule__DictionaryControlGroup__Group_4__4__Impl38096); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryControlGroupAccess().getRightCurlyBracketKeyword_4_4());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryControlGroup__Group_4__4__Impl"
// $ANTLR start "rule__DictionaryHierarchicalControl__Group__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18610:1: rule__DictionaryHierarchicalControl__Group__0 : rule__DictionaryHierarchicalControl__Group__0__Impl rule__DictionaryHierarchicalControl__Group__1 ;
public final void rule__DictionaryHierarchicalControl__Group__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18614:1: ( rule__DictionaryHierarchicalControl__Group__0__Impl rule__DictionaryHierarchicalControl__Group__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18615:2: rule__DictionaryHierarchicalControl__Group__0__Impl rule__DictionaryHierarchicalControl__Group__1
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryHierarchicalControl__Group__0__Impl_in_rule__DictionaryHierarchicalControl__Group__038137);
rule__DictionaryHierarchicalControl__Group__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryHierarchicalControl__Group__1_in_rule__DictionaryHierarchicalControl__Group__038140);
rule__DictionaryHierarchicalControl__Group__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryHierarchicalControl__Group__0"
// $ANTLR start "rule__DictionaryHierarchicalControl__Group__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18622:1: rule__DictionaryHierarchicalControl__Group__0__Impl : ( () ) ;
public final void rule__DictionaryHierarchicalControl__Group__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18626:1: ( ( () ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18627:1: ( () )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18627:1: ( () )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18628:1: ()
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryHierarchicalControlAccess().getDictionaryHierarchicalControlAction_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18629:1: ()
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18631:1:
{
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryHierarchicalControlAccess().getDictionaryHierarchicalControlAction_0());
}
}
}
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryHierarchicalControl__Group__0__Impl"
// $ANTLR start "rule__DictionaryHierarchicalControl__Group__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18641:1: rule__DictionaryHierarchicalControl__Group__1 : rule__DictionaryHierarchicalControl__Group__1__Impl rule__DictionaryHierarchicalControl__Group__2 ;
public final void rule__DictionaryHierarchicalControl__Group__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18645:1: ( rule__DictionaryHierarchicalControl__Group__1__Impl rule__DictionaryHierarchicalControl__Group__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18646:2: rule__DictionaryHierarchicalControl__Group__1__Impl rule__DictionaryHierarchicalControl__Group__2
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryHierarchicalControl__Group__1__Impl_in_rule__DictionaryHierarchicalControl__Group__138198);
rule__DictionaryHierarchicalControl__Group__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryHierarchicalControl__Group__2_in_rule__DictionaryHierarchicalControl__Group__138201);
rule__DictionaryHierarchicalControl__Group__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryHierarchicalControl__Group__1"
// $ANTLR start "rule__DictionaryHierarchicalControl__Group__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18653:1: rule__DictionaryHierarchicalControl__Group__1__Impl : ( 'hierarchicalcontrol' ) ;
public final void rule__DictionaryHierarchicalControl__Group__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18657:1: ( ( 'hierarchicalcontrol' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18658:1: ( 'hierarchicalcontrol' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18658:1: ( 'hierarchicalcontrol' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18659:1: 'hierarchicalcontrol'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryHierarchicalControlAccess().getHierarchicalcontrolKeyword_1());
}
match(input,127,FollowSets001.FOLLOW_127_in_rule__DictionaryHierarchicalControl__Group__1__Impl38229); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryHierarchicalControlAccess().getHierarchicalcontrolKeyword_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryHierarchicalControl__Group__1__Impl"
// $ANTLR start "rule__DictionaryHierarchicalControl__Group__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18672:1: rule__DictionaryHierarchicalControl__Group__2 : rule__DictionaryHierarchicalControl__Group__2__Impl rule__DictionaryHierarchicalControl__Group__3 ;
public final void rule__DictionaryHierarchicalControl__Group__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18676:1: ( rule__DictionaryHierarchicalControl__Group__2__Impl rule__DictionaryHierarchicalControl__Group__3 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18677:2: rule__DictionaryHierarchicalControl__Group__2__Impl rule__DictionaryHierarchicalControl__Group__3
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryHierarchicalControl__Group__2__Impl_in_rule__DictionaryHierarchicalControl__Group__238260);
rule__DictionaryHierarchicalControl__Group__2__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryHierarchicalControl__Group__3_in_rule__DictionaryHierarchicalControl__Group__238263);
rule__DictionaryHierarchicalControl__Group__3();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryHierarchicalControl__Group__2"
// $ANTLR start "rule__DictionaryHierarchicalControl__Group__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18684:1: rule__DictionaryHierarchicalControl__Group__2__Impl : ( ( rule__DictionaryHierarchicalControl__NameAssignment_2 )? ) ;
public final void rule__DictionaryHierarchicalControl__Group__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18688:1: ( ( ( rule__DictionaryHierarchicalControl__NameAssignment_2 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18689:1: ( ( rule__DictionaryHierarchicalControl__NameAssignment_2 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18689:1: ( ( rule__DictionaryHierarchicalControl__NameAssignment_2 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18690:1: ( rule__DictionaryHierarchicalControl__NameAssignment_2 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryHierarchicalControlAccess().getNameAssignment_2());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18691:1: ( rule__DictionaryHierarchicalControl__NameAssignment_2 )?
int alt148=2;
int LA148_0 = input.LA(1);
if ( (LA148_0==RULE_ID) ) {
alt148=1;
}
switch (alt148) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18691:2: rule__DictionaryHierarchicalControl__NameAssignment_2
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryHierarchicalControl__NameAssignment_2_in_rule__DictionaryHierarchicalControl__Group__2__Impl38290);
rule__DictionaryHierarchicalControl__NameAssignment_2();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryHierarchicalControlAccess().getNameAssignment_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryHierarchicalControl__Group__2__Impl"
// $ANTLR start "rule__DictionaryHierarchicalControl__Group__3"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18701:1: rule__DictionaryHierarchicalControl__Group__3 : rule__DictionaryHierarchicalControl__Group__3__Impl rule__DictionaryHierarchicalControl__Group__4 ;
public final void rule__DictionaryHierarchicalControl__Group__3() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18705:1: ( rule__DictionaryHierarchicalControl__Group__3__Impl rule__DictionaryHierarchicalControl__Group__4 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18706:2: rule__DictionaryHierarchicalControl__Group__3__Impl rule__DictionaryHierarchicalControl__Group__4
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryHierarchicalControl__Group__3__Impl_in_rule__DictionaryHierarchicalControl__Group__338321);
rule__DictionaryHierarchicalControl__Group__3__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryHierarchicalControl__Group__4_in_rule__DictionaryHierarchicalControl__Group__338324);
rule__DictionaryHierarchicalControl__Group__4();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryHierarchicalControl__Group__3"
// $ANTLR start "rule__DictionaryHierarchicalControl__Group__3__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18713:1: rule__DictionaryHierarchicalControl__Group__3__Impl : ( ( rule__DictionaryHierarchicalControl__Group_3__0 )? ) ;
public final void rule__DictionaryHierarchicalControl__Group__3__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18717:1: ( ( ( rule__DictionaryHierarchicalControl__Group_3__0 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18718:1: ( ( rule__DictionaryHierarchicalControl__Group_3__0 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18718:1: ( ( rule__DictionaryHierarchicalControl__Group_3__0 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18719:1: ( rule__DictionaryHierarchicalControl__Group_3__0 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryHierarchicalControlAccess().getGroup_3());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18720:1: ( rule__DictionaryHierarchicalControl__Group_3__0 )?
int alt149=2;
int LA149_0 = input.LA(1);
if ( (LA149_0==126) ) {
alt149=1;
}
switch (alt149) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18720:2: rule__DictionaryHierarchicalControl__Group_3__0
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryHierarchicalControl__Group_3__0_in_rule__DictionaryHierarchicalControl__Group__3__Impl38351);
rule__DictionaryHierarchicalControl__Group_3__0();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryHierarchicalControlAccess().getGroup_3());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryHierarchicalControl__Group__3__Impl"
// $ANTLR start "rule__DictionaryHierarchicalControl__Group__4"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18730:1: rule__DictionaryHierarchicalControl__Group__4 : rule__DictionaryHierarchicalControl__Group__4__Impl ;
public final void rule__DictionaryHierarchicalControl__Group__4() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18734:1: ( rule__DictionaryHierarchicalControl__Group__4__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18735:2: rule__DictionaryHierarchicalControl__Group__4__Impl
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryHierarchicalControl__Group__4__Impl_in_rule__DictionaryHierarchicalControl__Group__438382);
rule__DictionaryHierarchicalControl__Group__4__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryHierarchicalControl__Group__4"
// $ANTLR start "rule__DictionaryHierarchicalControl__Group__4__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18741:1: rule__DictionaryHierarchicalControl__Group__4__Impl : ( ( rule__DictionaryHierarchicalControl__Group_4__0 )? ) ;
public final void rule__DictionaryHierarchicalControl__Group__4__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18745:1: ( ( ( rule__DictionaryHierarchicalControl__Group_4__0 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18746:1: ( ( rule__DictionaryHierarchicalControl__Group_4__0 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18746:1: ( ( rule__DictionaryHierarchicalControl__Group_4__0 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18747:1: ( rule__DictionaryHierarchicalControl__Group_4__0 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryHierarchicalControlAccess().getGroup_4());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18748:1: ( rule__DictionaryHierarchicalControl__Group_4__0 )?
int alt150=2;
int LA150_0 = input.LA(1);
if ( (LA150_0==67) ) {
alt150=1;
}
switch (alt150) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18748:2: rule__DictionaryHierarchicalControl__Group_4__0
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryHierarchicalControl__Group_4__0_in_rule__DictionaryHierarchicalControl__Group__4__Impl38409);
rule__DictionaryHierarchicalControl__Group_4__0();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryHierarchicalControlAccess().getGroup_4());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryHierarchicalControl__Group__4__Impl"
// $ANTLR start "rule__DictionaryHierarchicalControl__Group_3__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18768:1: rule__DictionaryHierarchicalControl__Group_3__0 : rule__DictionaryHierarchicalControl__Group_3__0__Impl rule__DictionaryHierarchicalControl__Group_3__1 ;
public final void rule__DictionaryHierarchicalControl__Group_3__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18772:1: ( rule__DictionaryHierarchicalControl__Group_3__0__Impl rule__DictionaryHierarchicalControl__Group_3__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18773:2: rule__DictionaryHierarchicalControl__Group_3__0__Impl rule__DictionaryHierarchicalControl__Group_3__1
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryHierarchicalControl__Group_3__0__Impl_in_rule__DictionaryHierarchicalControl__Group_3__038450);
rule__DictionaryHierarchicalControl__Group_3__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryHierarchicalControl__Group_3__1_in_rule__DictionaryHierarchicalControl__Group_3__038453);
rule__DictionaryHierarchicalControl__Group_3__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryHierarchicalControl__Group_3__0"
// $ANTLR start "rule__DictionaryHierarchicalControl__Group_3__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18780:1: rule__DictionaryHierarchicalControl__Group_3__0__Impl : ( 'ref' ) ;
public final void rule__DictionaryHierarchicalControl__Group_3__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18784:1: ( ( 'ref' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18785:1: ( 'ref' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18785:1: ( 'ref' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18786:1: 'ref'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryHierarchicalControlAccess().getRefKeyword_3_0());
}
match(input,126,FollowSets001.FOLLOW_126_in_rule__DictionaryHierarchicalControl__Group_3__0__Impl38481); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryHierarchicalControlAccess().getRefKeyword_3_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryHierarchicalControl__Group_3__0__Impl"
// $ANTLR start "rule__DictionaryHierarchicalControl__Group_3__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18799:1: rule__DictionaryHierarchicalControl__Group_3__1 : rule__DictionaryHierarchicalControl__Group_3__1__Impl ;
public final void rule__DictionaryHierarchicalControl__Group_3__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18803:1: ( rule__DictionaryHierarchicalControl__Group_3__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18804:2: rule__DictionaryHierarchicalControl__Group_3__1__Impl
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryHierarchicalControl__Group_3__1__Impl_in_rule__DictionaryHierarchicalControl__Group_3__138512);
rule__DictionaryHierarchicalControl__Group_3__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryHierarchicalControl__Group_3__1"
// $ANTLR start "rule__DictionaryHierarchicalControl__Group_3__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18810:1: rule__DictionaryHierarchicalControl__Group_3__1__Impl : ( ( rule__DictionaryHierarchicalControl__RefAssignment_3_1 ) ) ;
public final void rule__DictionaryHierarchicalControl__Group_3__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18814:1: ( ( ( rule__DictionaryHierarchicalControl__RefAssignment_3_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18815:1: ( ( rule__DictionaryHierarchicalControl__RefAssignment_3_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18815:1: ( ( rule__DictionaryHierarchicalControl__RefAssignment_3_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18816:1: ( rule__DictionaryHierarchicalControl__RefAssignment_3_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryHierarchicalControlAccess().getRefAssignment_3_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18817:1: ( rule__DictionaryHierarchicalControl__RefAssignment_3_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18817:2: rule__DictionaryHierarchicalControl__RefAssignment_3_1
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryHierarchicalControl__RefAssignment_3_1_in_rule__DictionaryHierarchicalControl__Group_3__1__Impl38539);
rule__DictionaryHierarchicalControl__RefAssignment_3_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryHierarchicalControlAccess().getRefAssignment_3_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryHierarchicalControl__Group_3__1__Impl"
// $ANTLR start "rule__DictionaryHierarchicalControl__Group_4__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18831:1: rule__DictionaryHierarchicalControl__Group_4__0 : rule__DictionaryHierarchicalControl__Group_4__0__Impl rule__DictionaryHierarchicalControl__Group_4__1 ;
public final void rule__DictionaryHierarchicalControl__Group_4__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18835:1: ( rule__DictionaryHierarchicalControl__Group_4__0__Impl rule__DictionaryHierarchicalControl__Group_4__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18836:2: rule__DictionaryHierarchicalControl__Group_4__0__Impl rule__DictionaryHierarchicalControl__Group_4__1
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryHierarchicalControl__Group_4__0__Impl_in_rule__DictionaryHierarchicalControl__Group_4__038573);
rule__DictionaryHierarchicalControl__Group_4__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryHierarchicalControl__Group_4__1_in_rule__DictionaryHierarchicalControl__Group_4__038576);
rule__DictionaryHierarchicalControl__Group_4__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryHierarchicalControl__Group_4__0"
// $ANTLR start "rule__DictionaryHierarchicalControl__Group_4__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18843:1: rule__DictionaryHierarchicalControl__Group_4__0__Impl : ( '{' ) ;
public final void rule__DictionaryHierarchicalControl__Group_4__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18847:1: ( ( '{' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18848:1: ( '{' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18848:1: ( '{' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18849:1: '{'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryHierarchicalControlAccess().getLeftCurlyBracketKeyword_4_0());
}
match(input,67,FollowSets001.FOLLOW_67_in_rule__DictionaryHierarchicalControl__Group_4__0__Impl38604); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryHierarchicalControlAccess().getLeftCurlyBracketKeyword_4_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryHierarchicalControl__Group_4__0__Impl"
// $ANTLR start "rule__DictionaryHierarchicalControl__Group_4__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18862:1: rule__DictionaryHierarchicalControl__Group_4__1 : rule__DictionaryHierarchicalControl__Group_4__1__Impl rule__DictionaryHierarchicalControl__Group_4__2 ;
public final void rule__DictionaryHierarchicalControl__Group_4__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18866:1: ( rule__DictionaryHierarchicalControl__Group_4__1__Impl rule__DictionaryHierarchicalControl__Group_4__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18867:2: rule__DictionaryHierarchicalControl__Group_4__1__Impl rule__DictionaryHierarchicalControl__Group_4__2
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryHierarchicalControl__Group_4__1__Impl_in_rule__DictionaryHierarchicalControl__Group_4__138635);
rule__DictionaryHierarchicalControl__Group_4__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryHierarchicalControl__Group_4__2_in_rule__DictionaryHierarchicalControl__Group_4__138638);
rule__DictionaryHierarchicalControl__Group_4__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryHierarchicalControl__Group_4__1"
// $ANTLR start "rule__DictionaryHierarchicalControl__Group_4__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18874:1: rule__DictionaryHierarchicalControl__Group_4__1__Impl : ( ( rule__DictionaryHierarchicalControl__BaseControlAssignment_4_1 ) ) ;
public final void rule__DictionaryHierarchicalControl__Group_4__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18878:1: ( ( ( rule__DictionaryHierarchicalControl__BaseControlAssignment_4_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18879:1: ( ( rule__DictionaryHierarchicalControl__BaseControlAssignment_4_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18879:1: ( ( rule__DictionaryHierarchicalControl__BaseControlAssignment_4_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18880:1: ( rule__DictionaryHierarchicalControl__BaseControlAssignment_4_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryHierarchicalControlAccess().getBaseControlAssignment_4_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18881:1: ( rule__DictionaryHierarchicalControl__BaseControlAssignment_4_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18881:2: rule__DictionaryHierarchicalControl__BaseControlAssignment_4_1
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryHierarchicalControl__BaseControlAssignment_4_1_in_rule__DictionaryHierarchicalControl__Group_4__1__Impl38665);
rule__DictionaryHierarchicalControl__BaseControlAssignment_4_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryHierarchicalControlAccess().getBaseControlAssignment_4_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryHierarchicalControl__Group_4__1__Impl"
// $ANTLR start "rule__DictionaryHierarchicalControl__Group_4__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18891:1: rule__DictionaryHierarchicalControl__Group_4__2 : rule__DictionaryHierarchicalControl__Group_4__2__Impl rule__DictionaryHierarchicalControl__Group_4__3 ;
public final void rule__DictionaryHierarchicalControl__Group_4__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18895:1: ( rule__DictionaryHierarchicalControl__Group_4__2__Impl rule__DictionaryHierarchicalControl__Group_4__3 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18896:2: rule__DictionaryHierarchicalControl__Group_4__2__Impl rule__DictionaryHierarchicalControl__Group_4__3
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryHierarchicalControl__Group_4__2__Impl_in_rule__DictionaryHierarchicalControl__Group_4__238695);
rule__DictionaryHierarchicalControl__Group_4__2__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryHierarchicalControl__Group_4__3_in_rule__DictionaryHierarchicalControl__Group_4__238698);
rule__DictionaryHierarchicalControl__Group_4__3();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryHierarchicalControl__Group_4__2"
// $ANTLR start "rule__DictionaryHierarchicalControl__Group_4__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18903:1: rule__DictionaryHierarchicalControl__Group_4__2__Impl : ( 'hierarchicalId' ) ;
public final void rule__DictionaryHierarchicalControl__Group_4__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18907:1: ( ( 'hierarchicalId' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18908:1: ( 'hierarchicalId' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18908:1: ( 'hierarchicalId' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18909:1: 'hierarchicalId'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryHierarchicalControlAccess().getHierarchicalIdKeyword_4_2());
}
match(input,128,FollowSets001.FOLLOW_128_in_rule__DictionaryHierarchicalControl__Group_4__2__Impl38726); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryHierarchicalControlAccess().getHierarchicalIdKeyword_4_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryHierarchicalControl__Group_4__2__Impl"
// $ANTLR start "rule__DictionaryHierarchicalControl__Group_4__3"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18922:1: rule__DictionaryHierarchicalControl__Group_4__3 : rule__DictionaryHierarchicalControl__Group_4__3__Impl rule__DictionaryHierarchicalControl__Group_4__4 ;
public final void rule__DictionaryHierarchicalControl__Group_4__3() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18926:1: ( rule__DictionaryHierarchicalControl__Group_4__3__Impl rule__DictionaryHierarchicalControl__Group_4__4 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18927:2: rule__DictionaryHierarchicalControl__Group_4__3__Impl rule__DictionaryHierarchicalControl__Group_4__4
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryHierarchicalControl__Group_4__3__Impl_in_rule__DictionaryHierarchicalControl__Group_4__338757);
rule__DictionaryHierarchicalControl__Group_4__3__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryHierarchicalControl__Group_4__4_in_rule__DictionaryHierarchicalControl__Group_4__338760);
rule__DictionaryHierarchicalControl__Group_4__4();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryHierarchicalControl__Group_4__3"
// $ANTLR start "rule__DictionaryHierarchicalControl__Group_4__3__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18934:1: rule__DictionaryHierarchicalControl__Group_4__3__Impl : ( ( rule__DictionaryHierarchicalControl__HierarchicalIdAssignment_4_3 ) ) ;
public final void rule__DictionaryHierarchicalControl__Group_4__3__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18938:1: ( ( ( rule__DictionaryHierarchicalControl__HierarchicalIdAssignment_4_3 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18939:1: ( ( rule__DictionaryHierarchicalControl__HierarchicalIdAssignment_4_3 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18939:1: ( ( rule__DictionaryHierarchicalControl__HierarchicalIdAssignment_4_3 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18940:1: ( rule__DictionaryHierarchicalControl__HierarchicalIdAssignment_4_3 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryHierarchicalControlAccess().getHierarchicalIdAssignment_4_3());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18941:1: ( rule__DictionaryHierarchicalControl__HierarchicalIdAssignment_4_3 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18941:2: rule__DictionaryHierarchicalControl__HierarchicalIdAssignment_4_3
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryHierarchicalControl__HierarchicalIdAssignment_4_3_in_rule__DictionaryHierarchicalControl__Group_4__3__Impl38787);
rule__DictionaryHierarchicalControl__HierarchicalIdAssignment_4_3();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryHierarchicalControlAccess().getHierarchicalIdAssignment_4_3());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryHierarchicalControl__Group_4__3__Impl"
// $ANTLR start "rule__DictionaryHierarchicalControl__Group_4__4"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18951:1: rule__DictionaryHierarchicalControl__Group_4__4 : rule__DictionaryHierarchicalControl__Group_4__4__Impl ;
public final void rule__DictionaryHierarchicalControl__Group_4__4() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18955:1: ( rule__DictionaryHierarchicalControl__Group_4__4__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18956:2: rule__DictionaryHierarchicalControl__Group_4__4__Impl
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryHierarchicalControl__Group_4__4__Impl_in_rule__DictionaryHierarchicalControl__Group_4__438817);
rule__DictionaryHierarchicalControl__Group_4__4__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryHierarchicalControl__Group_4__4"
// $ANTLR start "rule__DictionaryHierarchicalControl__Group_4__4__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18962:1: rule__DictionaryHierarchicalControl__Group_4__4__Impl : ( '}' ) ;
public final void rule__DictionaryHierarchicalControl__Group_4__4__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18966:1: ( ( '}' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18967:1: ( '}' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18967:1: ( '}' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18968:1: '}'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryHierarchicalControlAccess().getRightCurlyBracketKeyword_4_4());
}
match(input,68,FollowSets001.FOLLOW_68_in_rule__DictionaryHierarchicalControl__Group_4__4__Impl38845); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryHierarchicalControlAccess().getRightCurlyBracketKeyword_4_4());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryHierarchicalControl__Group_4__4__Impl"
// $ANTLR start "rule__DictionaryTextControl__Group__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18991:1: rule__DictionaryTextControl__Group__0 : rule__DictionaryTextControl__Group__0__Impl rule__DictionaryTextControl__Group__1 ;
public final void rule__DictionaryTextControl__Group__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18995:1: ( rule__DictionaryTextControl__Group__0__Impl rule__DictionaryTextControl__Group__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:18996:2: rule__DictionaryTextControl__Group__0__Impl rule__DictionaryTextControl__Group__1
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryTextControl__Group__0__Impl_in_rule__DictionaryTextControl__Group__038886);
rule__DictionaryTextControl__Group__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryTextControl__Group__1_in_rule__DictionaryTextControl__Group__038889);
rule__DictionaryTextControl__Group__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryTextControl__Group__0"
// $ANTLR start "rule__DictionaryTextControl__Group__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19003:1: rule__DictionaryTextControl__Group__0__Impl : ( () ) ;
public final void rule__DictionaryTextControl__Group__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19007:1: ( ( () ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19008:1: ( () )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19008:1: ( () )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19009:1: ()
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryTextControlAccess().getDictionaryTextControlAction_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19010:1: ()
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19012:1:
{
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryTextControlAccess().getDictionaryTextControlAction_0());
}
}
}
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryTextControl__Group__0__Impl"
// $ANTLR start "rule__DictionaryTextControl__Group__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19022:1: rule__DictionaryTextControl__Group__1 : rule__DictionaryTextControl__Group__1__Impl rule__DictionaryTextControl__Group__2 ;
public final void rule__DictionaryTextControl__Group__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19026:1: ( rule__DictionaryTextControl__Group__1__Impl rule__DictionaryTextControl__Group__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19027:2: rule__DictionaryTextControl__Group__1__Impl rule__DictionaryTextControl__Group__2
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryTextControl__Group__1__Impl_in_rule__DictionaryTextControl__Group__138947);
rule__DictionaryTextControl__Group__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryTextControl__Group__2_in_rule__DictionaryTextControl__Group__138950);
rule__DictionaryTextControl__Group__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryTextControl__Group__1"
// $ANTLR start "rule__DictionaryTextControl__Group__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19034:1: rule__DictionaryTextControl__Group__1__Impl : ( 'textcontrol' ) ;
public final void rule__DictionaryTextControl__Group__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19038:1: ( ( 'textcontrol' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19039:1: ( 'textcontrol' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19039:1: ( 'textcontrol' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19040:1: 'textcontrol'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryTextControlAccess().getTextcontrolKeyword_1());
}
match(input,129,FollowSets001.FOLLOW_129_in_rule__DictionaryTextControl__Group__1__Impl38978); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryTextControlAccess().getTextcontrolKeyword_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryTextControl__Group__1__Impl"
// $ANTLR start "rule__DictionaryTextControl__Group__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19053:1: rule__DictionaryTextControl__Group__2 : rule__DictionaryTextControl__Group__2__Impl rule__DictionaryTextControl__Group__3 ;
public final void rule__DictionaryTextControl__Group__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19057:1: ( rule__DictionaryTextControl__Group__2__Impl rule__DictionaryTextControl__Group__3 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19058:2: rule__DictionaryTextControl__Group__2__Impl rule__DictionaryTextControl__Group__3
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryTextControl__Group__2__Impl_in_rule__DictionaryTextControl__Group__239009);
rule__DictionaryTextControl__Group__2__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryTextControl__Group__3_in_rule__DictionaryTextControl__Group__239012);
rule__DictionaryTextControl__Group__3();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryTextControl__Group__2"
// $ANTLR start "rule__DictionaryTextControl__Group__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19065:1: rule__DictionaryTextControl__Group__2__Impl : ( ( rule__DictionaryTextControl__NameAssignment_2 )? ) ;
public final void rule__DictionaryTextControl__Group__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19069:1: ( ( ( rule__DictionaryTextControl__NameAssignment_2 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19070:1: ( ( rule__DictionaryTextControl__NameAssignment_2 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19070:1: ( ( rule__DictionaryTextControl__NameAssignment_2 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19071:1: ( rule__DictionaryTextControl__NameAssignment_2 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryTextControlAccess().getNameAssignment_2());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19072:1: ( rule__DictionaryTextControl__NameAssignment_2 )?
int alt151=2;
int LA151_0 = input.LA(1);
if ( (LA151_0==RULE_ID) ) {
alt151=1;
}
switch (alt151) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19072:2: rule__DictionaryTextControl__NameAssignment_2
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryTextControl__NameAssignment_2_in_rule__DictionaryTextControl__Group__2__Impl39039);
rule__DictionaryTextControl__NameAssignment_2();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryTextControlAccess().getNameAssignment_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryTextControl__Group__2__Impl"
// $ANTLR start "rule__DictionaryTextControl__Group__3"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19082:1: rule__DictionaryTextControl__Group__3 : rule__DictionaryTextControl__Group__3__Impl rule__DictionaryTextControl__Group__4 ;
public final void rule__DictionaryTextControl__Group__3() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19086:1: ( rule__DictionaryTextControl__Group__3__Impl rule__DictionaryTextControl__Group__4 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19087:2: rule__DictionaryTextControl__Group__3__Impl rule__DictionaryTextControl__Group__4
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryTextControl__Group__3__Impl_in_rule__DictionaryTextControl__Group__339070);
rule__DictionaryTextControl__Group__3__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryTextControl__Group__4_in_rule__DictionaryTextControl__Group__339073);
rule__DictionaryTextControl__Group__4();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryTextControl__Group__3"
// $ANTLR start "rule__DictionaryTextControl__Group__3__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19094:1: rule__DictionaryTextControl__Group__3__Impl : ( ( rule__DictionaryTextControl__Group_3__0 )? ) ;
public final void rule__DictionaryTextControl__Group__3__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19098:1: ( ( ( rule__DictionaryTextControl__Group_3__0 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19099:1: ( ( rule__DictionaryTextControl__Group_3__0 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19099:1: ( ( rule__DictionaryTextControl__Group_3__0 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19100:1: ( rule__DictionaryTextControl__Group_3__0 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryTextControlAccess().getGroup_3());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19101:1: ( rule__DictionaryTextControl__Group_3__0 )?
int alt152=2;
int LA152_0 = input.LA(1);
if ( (LA152_0==126) ) {
alt152=1;
}
switch (alt152) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19101:2: rule__DictionaryTextControl__Group_3__0
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryTextControl__Group_3__0_in_rule__DictionaryTextControl__Group__3__Impl39100);
rule__DictionaryTextControl__Group_3__0();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryTextControlAccess().getGroup_3());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryTextControl__Group__3__Impl"
// $ANTLR start "rule__DictionaryTextControl__Group__4"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19111:1: rule__DictionaryTextControl__Group__4 : rule__DictionaryTextControl__Group__4__Impl ;
public final void rule__DictionaryTextControl__Group__4() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19115:1: ( rule__DictionaryTextControl__Group__4__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19116:2: rule__DictionaryTextControl__Group__4__Impl
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryTextControl__Group__4__Impl_in_rule__DictionaryTextControl__Group__439131);
rule__DictionaryTextControl__Group__4__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryTextControl__Group__4"
// $ANTLR start "rule__DictionaryTextControl__Group__4__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19122:1: rule__DictionaryTextControl__Group__4__Impl : ( ( rule__DictionaryTextControl__Group_4__0 )? ) ;
public final void rule__DictionaryTextControl__Group__4__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19126:1: ( ( ( rule__DictionaryTextControl__Group_4__0 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19127:1: ( ( rule__DictionaryTextControl__Group_4__0 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19127:1: ( ( rule__DictionaryTextControl__Group_4__0 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19128:1: ( rule__DictionaryTextControl__Group_4__0 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryTextControlAccess().getGroup_4());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19129:1: ( rule__DictionaryTextControl__Group_4__0 )?
int alt153=2;
int LA153_0 = input.LA(1);
if ( (LA153_0==67) ) {
alt153=1;
}
switch (alt153) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19129:2: rule__DictionaryTextControl__Group_4__0
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryTextControl__Group_4__0_in_rule__DictionaryTextControl__Group__4__Impl39158);
rule__DictionaryTextControl__Group_4__0();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryTextControlAccess().getGroup_4());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryTextControl__Group__4__Impl"
// $ANTLR start "rule__DictionaryTextControl__Group_3__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19149:1: rule__DictionaryTextControl__Group_3__0 : rule__DictionaryTextControl__Group_3__0__Impl rule__DictionaryTextControl__Group_3__1 ;
public final void rule__DictionaryTextControl__Group_3__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19153:1: ( rule__DictionaryTextControl__Group_3__0__Impl rule__DictionaryTextControl__Group_3__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19154:2: rule__DictionaryTextControl__Group_3__0__Impl rule__DictionaryTextControl__Group_3__1
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryTextControl__Group_3__0__Impl_in_rule__DictionaryTextControl__Group_3__039199);
rule__DictionaryTextControl__Group_3__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryTextControl__Group_3__1_in_rule__DictionaryTextControl__Group_3__039202);
rule__DictionaryTextControl__Group_3__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryTextControl__Group_3__0"
// $ANTLR start "rule__DictionaryTextControl__Group_3__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19161:1: rule__DictionaryTextControl__Group_3__0__Impl : ( 'ref' ) ;
public final void rule__DictionaryTextControl__Group_3__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19165:1: ( ( 'ref' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19166:1: ( 'ref' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19166:1: ( 'ref' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19167:1: 'ref'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryTextControlAccess().getRefKeyword_3_0());
}
match(input,126,FollowSets001.FOLLOW_126_in_rule__DictionaryTextControl__Group_3__0__Impl39230); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryTextControlAccess().getRefKeyword_3_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryTextControl__Group_3__0__Impl"
// $ANTLR start "rule__DictionaryTextControl__Group_3__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19180:1: rule__DictionaryTextControl__Group_3__1 : rule__DictionaryTextControl__Group_3__1__Impl ;
public final void rule__DictionaryTextControl__Group_3__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19184:1: ( rule__DictionaryTextControl__Group_3__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19185:2: rule__DictionaryTextControl__Group_3__1__Impl
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryTextControl__Group_3__1__Impl_in_rule__DictionaryTextControl__Group_3__139261);
rule__DictionaryTextControl__Group_3__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryTextControl__Group_3__1"
// $ANTLR start "rule__DictionaryTextControl__Group_3__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19191:1: rule__DictionaryTextControl__Group_3__1__Impl : ( ( rule__DictionaryTextControl__RefAssignment_3_1 ) ) ;
public final void rule__DictionaryTextControl__Group_3__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19195:1: ( ( ( rule__DictionaryTextControl__RefAssignment_3_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19196:1: ( ( rule__DictionaryTextControl__RefAssignment_3_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19196:1: ( ( rule__DictionaryTextControl__RefAssignment_3_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19197:1: ( rule__DictionaryTextControl__RefAssignment_3_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryTextControlAccess().getRefAssignment_3_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19198:1: ( rule__DictionaryTextControl__RefAssignment_3_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19198:2: rule__DictionaryTextControl__RefAssignment_3_1
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryTextControl__RefAssignment_3_1_in_rule__DictionaryTextControl__Group_3__1__Impl39288);
rule__DictionaryTextControl__RefAssignment_3_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryTextControlAccess().getRefAssignment_3_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryTextControl__Group_3__1__Impl"
// $ANTLR start "rule__DictionaryTextControl__Group_4__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19212:1: rule__DictionaryTextControl__Group_4__0 : rule__DictionaryTextControl__Group_4__0__Impl rule__DictionaryTextControl__Group_4__1 ;
public final void rule__DictionaryTextControl__Group_4__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19216:1: ( rule__DictionaryTextControl__Group_4__0__Impl rule__DictionaryTextControl__Group_4__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19217:2: rule__DictionaryTextControl__Group_4__0__Impl rule__DictionaryTextControl__Group_4__1
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryTextControl__Group_4__0__Impl_in_rule__DictionaryTextControl__Group_4__039322);
rule__DictionaryTextControl__Group_4__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryTextControl__Group_4__1_in_rule__DictionaryTextControl__Group_4__039325);
rule__DictionaryTextControl__Group_4__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryTextControl__Group_4__0"
// $ANTLR start "rule__DictionaryTextControl__Group_4__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19224:1: rule__DictionaryTextControl__Group_4__0__Impl : ( '{' ) ;
public final void rule__DictionaryTextControl__Group_4__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19228:1: ( ( '{' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19229:1: ( '{' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19229:1: ( '{' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19230:1: '{'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryTextControlAccess().getLeftCurlyBracketKeyword_4_0());
}
match(input,67,FollowSets001.FOLLOW_67_in_rule__DictionaryTextControl__Group_4__0__Impl39353); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryTextControlAccess().getLeftCurlyBracketKeyword_4_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryTextControl__Group_4__0__Impl"
// $ANTLR start "rule__DictionaryTextControl__Group_4__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19243:1: rule__DictionaryTextControl__Group_4__1 : rule__DictionaryTextControl__Group_4__1__Impl rule__DictionaryTextControl__Group_4__2 ;
public final void rule__DictionaryTextControl__Group_4__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19247:1: ( rule__DictionaryTextControl__Group_4__1__Impl rule__DictionaryTextControl__Group_4__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19248:2: rule__DictionaryTextControl__Group_4__1__Impl rule__DictionaryTextControl__Group_4__2
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryTextControl__Group_4__1__Impl_in_rule__DictionaryTextControl__Group_4__139384);
rule__DictionaryTextControl__Group_4__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryTextControl__Group_4__2_in_rule__DictionaryTextControl__Group_4__139387);
rule__DictionaryTextControl__Group_4__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryTextControl__Group_4__1"
// $ANTLR start "rule__DictionaryTextControl__Group_4__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19255:1: rule__DictionaryTextControl__Group_4__1__Impl : ( ( rule__DictionaryTextControl__BaseControlAssignment_4_1 ) ) ;
public final void rule__DictionaryTextControl__Group_4__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19259:1: ( ( ( rule__DictionaryTextControl__BaseControlAssignment_4_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19260:1: ( ( rule__DictionaryTextControl__BaseControlAssignment_4_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19260:1: ( ( rule__DictionaryTextControl__BaseControlAssignment_4_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19261:1: ( rule__DictionaryTextControl__BaseControlAssignment_4_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryTextControlAccess().getBaseControlAssignment_4_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19262:1: ( rule__DictionaryTextControl__BaseControlAssignment_4_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19262:2: rule__DictionaryTextControl__BaseControlAssignment_4_1
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryTextControl__BaseControlAssignment_4_1_in_rule__DictionaryTextControl__Group_4__1__Impl39414);
rule__DictionaryTextControl__BaseControlAssignment_4_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryTextControlAccess().getBaseControlAssignment_4_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryTextControl__Group_4__1__Impl"
// $ANTLR start "rule__DictionaryTextControl__Group_4__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19272:1: rule__DictionaryTextControl__Group_4__2 : rule__DictionaryTextControl__Group_4__2__Impl ;
public final void rule__DictionaryTextControl__Group_4__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19276:1: ( rule__DictionaryTextControl__Group_4__2__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19277:2: rule__DictionaryTextControl__Group_4__2__Impl
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryTextControl__Group_4__2__Impl_in_rule__DictionaryTextControl__Group_4__239444);
rule__DictionaryTextControl__Group_4__2__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryTextControl__Group_4__2"
// $ANTLR start "rule__DictionaryTextControl__Group_4__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19283:1: rule__DictionaryTextControl__Group_4__2__Impl : ( '}' ) ;
public final void rule__DictionaryTextControl__Group_4__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19287:1: ( ( '}' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19288:1: ( '}' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19288:1: ( '}' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19289:1: '}'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryTextControlAccess().getRightCurlyBracketKeyword_4_2());
}
match(input,68,FollowSets001.FOLLOW_68_in_rule__DictionaryTextControl__Group_4__2__Impl39472); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryTextControlAccess().getRightCurlyBracketKeyword_4_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryTextControl__Group_4__2__Impl"
// $ANTLR start "rule__DictionaryIntegerControlInputType__Group__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19308:1: rule__DictionaryIntegerControlInputType__Group__0 : rule__DictionaryIntegerControlInputType__Group__0__Impl rule__DictionaryIntegerControlInputType__Group__1 ;
public final void rule__DictionaryIntegerControlInputType__Group__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19312:1: ( rule__DictionaryIntegerControlInputType__Group__0__Impl rule__DictionaryIntegerControlInputType__Group__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19313:2: rule__DictionaryIntegerControlInputType__Group__0__Impl rule__DictionaryIntegerControlInputType__Group__1
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryIntegerControlInputType__Group__0__Impl_in_rule__DictionaryIntegerControlInputType__Group__039509);
rule__DictionaryIntegerControlInputType__Group__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryIntegerControlInputType__Group__1_in_rule__DictionaryIntegerControlInputType__Group__039512);
rule__DictionaryIntegerControlInputType__Group__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryIntegerControlInputType__Group__0"
// $ANTLR start "rule__DictionaryIntegerControlInputType__Group__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19320:1: rule__DictionaryIntegerControlInputType__Group__0__Impl : ( () ) ;
public final void rule__DictionaryIntegerControlInputType__Group__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19324:1: ( ( () ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19325:1: ( () )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19325:1: ( () )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19326:1: ()
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryIntegerControlInputTypeAccess().getDictionaryIntegerControlInputTypeAction_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19327:1: ()
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19329:1:
{
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryIntegerControlInputTypeAccess().getDictionaryIntegerControlInputTypeAction_0());
}
}
}
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryIntegerControlInputType__Group__0__Impl"
// $ANTLR start "rule__DictionaryIntegerControlInputType__Group__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19339:1: rule__DictionaryIntegerControlInputType__Group__1 : rule__DictionaryIntegerControlInputType__Group__1__Impl rule__DictionaryIntegerControlInputType__Group__2 ;
public final void rule__DictionaryIntegerControlInputType__Group__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19343:1: ( rule__DictionaryIntegerControlInputType__Group__1__Impl rule__DictionaryIntegerControlInputType__Group__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19344:2: rule__DictionaryIntegerControlInputType__Group__1__Impl rule__DictionaryIntegerControlInputType__Group__2
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryIntegerControlInputType__Group__1__Impl_in_rule__DictionaryIntegerControlInputType__Group__139570);
rule__DictionaryIntegerControlInputType__Group__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryIntegerControlInputType__Group__2_in_rule__DictionaryIntegerControlInputType__Group__139573);
rule__DictionaryIntegerControlInputType__Group__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryIntegerControlInputType__Group__1"
// $ANTLR start "rule__DictionaryIntegerControlInputType__Group__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19351:1: rule__DictionaryIntegerControlInputType__Group__1__Impl : ( 'inputtype' ) ;
public final void rule__DictionaryIntegerControlInputType__Group__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19355:1: ( ( 'inputtype' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19356:1: ( 'inputtype' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19356:1: ( 'inputtype' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19357:1: 'inputtype'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryIntegerControlInputTypeAccess().getInputtypeKeyword_1());
}
match(input,130,FollowSets001.FOLLOW_130_in_rule__DictionaryIntegerControlInputType__Group__1__Impl39601); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryIntegerControlInputTypeAccess().getInputtypeKeyword_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryIntegerControlInputType__Group__1__Impl"
// $ANTLR start "rule__DictionaryIntegerControlInputType__Group__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19370:1: rule__DictionaryIntegerControlInputType__Group__2 : rule__DictionaryIntegerControlInputType__Group__2__Impl ;
public final void rule__DictionaryIntegerControlInputType__Group__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19374:1: ( rule__DictionaryIntegerControlInputType__Group__2__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19375:2: rule__DictionaryIntegerControlInputType__Group__2__Impl
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryIntegerControlInputType__Group__2__Impl_in_rule__DictionaryIntegerControlInputType__Group__239632);
rule__DictionaryIntegerControlInputType__Group__2__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryIntegerControlInputType__Group__2"
// $ANTLR start "rule__DictionaryIntegerControlInputType__Group__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19381:1: rule__DictionaryIntegerControlInputType__Group__2__Impl : ( ( rule__DictionaryIntegerControlInputType__InputtypeAssignment_2 ) ) ;
public final void rule__DictionaryIntegerControlInputType__Group__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19385:1: ( ( ( rule__DictionaryIntegerControlInputType__InputtypeAssignment_2 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19386:1: ( ( rule__DictionaryIntegerControlInputType__InputtypeAssignment_2 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19386:1: ( ( rule__DictionaryIntegerControlInputType__InputtypeAssignment_2 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19387:1: ( rule__DictionaryIntegerControlInputType__InputtypeAssignment_2 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryIntegerControlInputTypeAccess().getInputtypeAssignment_2());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19388:1: ( rule__DictionaryIntegerControlInputType__InputtypeAssignment_2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19388:2: rule__DictionaryIntegerControlInputType__InputtypeAssignment_2
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryIntegerControlInputType__InputtypeAssignment_2_in_rule__DictionaryIntegerControlInputType__Group__2__Impl39659);
rule__DictionaryIntegerControlInputType__InputtypeAssignment_2();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryIntegerControlInputTypeAccess().getInputtypeAssignment_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryIntegerControlInputType__Group__2__Impl"
// $ANTLR start "rule__DictionaryIntegerControl__Group__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19404:1: rule__DictionaryIntegerControl__Group__0 : rule__DictionaryIntegerControl__Group__0__Impl rule__DictionaryIntegerControl__Group__1 ;
public final void rule__DictionaryIntegerControl__Group__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19408:1: ( rule__DictionaryIntegerControl__Group__0__Impl rule__DictionaryIntegerControl__Group__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19409:2: rule__DictionaryIntegerControl__Group__0__Impl rule__DictionaryIntegerControl__Group__1
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryIntegerControl__Group__0__Impl_in_rule__DictionaryIntegerControl__Group__039695);
rule__DictionaryIntegerControl__Group__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryIntegerControl__Group__1_in_rule__DictionaryIntegerControl__Group__039698);
rule__DictionaryIntegerControl__Group__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryIntegerControl__Group__0"
// $ANTLR start "rule__DictionaryIntegerControl__Group__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19416:1: rule__DictionaryIntegerControl__Group__0__Impl : ( () ) ;
public final void rule__DictionaryIntegerControl__Group__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19420:1: ( ( () ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19421:1: ( () )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19421:1: ( () )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19422:1: ()
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryIntegerControlAccess().getDictionaryIntegerControlAction_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19423:1: ()
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19425:1:
{
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryIntegerControlAccess().getDictionaryIntegerControlAction_0());
}
}
}
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryIntegerControl__Group__0__Impl"
// $ANTLR start "rule__DictionaryIntegerControl__Group__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19435:1: rule__DictionaryIntegerControl__Group__1 : rule__DictionaryIntegerControl__Group__1__Impl rule__DictionaryIntegerControl__Group__2 ;
public final void rule__DictionaryIntegerControl__Group__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19439:1: ( rule__DictionaryIntegerControl__Group__1__Impl rule__DictionaryIntegerControl__Group__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19440:2: rule__DictionaryIntegerControl__Group__1__Impl rule__DictionaryIntegerControl__Group__2
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryIntegerControl__Group__1__Impl_in_rule__DictionaryIntegerControl__Group__139756);
rule__DictionaryIntegerControl__Group__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryIntegerControl__Group__2_in_rule__DictionaryIntegerControl__Group__139759);
rule__DictionaryIntegerControl__Group__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryIntegerControl__Group__1"
// $ANTLR start "rule__DictionaryIntegerControl__Group__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19447:1: rule__DictionaryIntegerControl__Group__1__Impl : ( 'integercontrol' ) ;
public final void rule__DictionaryIntegerControl__Group__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19451:1: ( ( 'integercontrol' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19452:1: ( 'integercontrol' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19452:1: ( 'integercontrol' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19453:1: 'integercontrol'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryIntegerControlAccess().getIntegercontrolKeyword_1());
}
match(input,131,FollowSets001.FOLLOW_131_in_rule__DictionaryIntegerControl__Group__1__Impl39787); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryIntegerControlAccess().getIntegercontrolKeyword_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryIntegerControl__Group__1__Impl"
// $ANTLR start "rule__DictionaryIntegerControl__Group__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19466:1: rule__DictionaryIntegerControl__Group__2 : rule__DictionaryIntegerControl__Group__2__Impl rule__DictionaryIntegerControl__Group__3 ;
public final void rule__DictionaryIntegerControl__Group__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19470:1: ( rule__DictionaryIntegerControl__Group__2__Impl rule__DictionaryIntegerControl__Group__3 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19471:2: rule__DictionaryIntegerControl__Group__2__Impl rule__DictionaryIntegerControl__Group__3
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryIntegerControl__Group__2__Impl_in_rule__DictionaryIntegerControl__Group__239818);
rule__DictionaryIntegerControl__Group__2__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryIntegerControl__Group__3_in_rule__DictionaryIntegerControl__Group__239821);
rule__DictionaryIntegerControl__Group__3();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryIntegerControl__Group__2"
// $ANTLR start "rule__DictionaryIntegerControl__Group__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19478:1: rule__DictionaryIntegerControl__Group__2__Impl : ( ( rule__DictionaryIntegerControl__NameAssignment_2 )? ) ;
public final void rule__DictionaryIntegerControl__Group__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19482:1: ( ( ( rule__DictionaryIntegerControl__NameAssignment_2 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19483:1: ( ( rule__DictionaryIntegerControl__NameAssignment_2 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19483:1: ( ( rule__DictionaryIntegerControl__NameAssignment_2 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19484:1: ( rule__DictionaryIntegerControl__NameAssignment_2 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryIntegerControlAccess().getNameAssignment_2());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19485:1: ( rule__DictionaryIntegerControl__NameAssignment_2 )?
int alt154=2;
int LA154_0 = input.LA(1);
if ( (LA154_0==RULE_ID) ) {
alt154=1;
}
switch (alt154) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19485:2: rule__DictionaryIntegerControl__NameAssignment_2
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryIntegerControl__NameAssignment_2_in_rule__DictionaryIntegerControl__Group__2__Impl39848);
rule__DictionaryIntegerControl__NameAssignment_2();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryIntegerControlAccess().getNameAssignment_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryIntegerControl__Group__2__Impl"
// $ANTLR start "rule__DictionaryIntegerControl__Group__3"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19495:1: rule__DictionaryIntegerControl__Group__3 : rule__DictionaryIntegerControl__Group__3__Impl rule__DictionaryIntegerControl__Group__4 ;
public final void rule__DictionaryIntegerControl__Group__3() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19499:1: ( rule__DictionaryIntegerControl__Group__3__Impl rule__DictionaryIntegerControl__Group__4 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19500:2: rule__DictionaryIntegerControl__Group__3__Impl rule__DictionaryIntegerControl__Group__4
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryIntegerControl__Group__3__Impl_in_rule__DictionaryIntegerControl__Group__339879);
rule__DictionaryIntegerControl__Group__3__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryIntegerControl__Group__4_in_rule__DictionaryIntegerControl__Group__339882);
rule__DictionaryIntegerControl__Group__4();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryIntegerControl__Group__3"
// $ANTLR start "rule__DictionaryIntegerControl__Group__3__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19507:1: rule__DictionaryIntegerControl__Group__3__Impl : ( ( rule__DictionaryIntegerControl__Group_3__0 )? ) ;
public final void rule__DictionaryIntegerControl__Group__3__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19511:1: ( ( ( rule__DictionaryIntegerControl__Group_3__0 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19512:1: ( ( rule__DictionaryIntegerControl__Group_3__0 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19512:1: ( ( rule__DictionaryIntegerControl__Group_3__0 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19513:1: ( rule__DictionaryIntegerControl__Group_3__0 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryIntegerControlAccess().getGroup_3());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19514:1: ( rule__DictionaryIntegerControl__Group_3__0 )?
int alt155=2;
int LA155_0 = input.LA(1);
if ( (LA155_0==126) ) {
alt155=1;
}
switch (alt155) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19514:2: rule__DictionaryIntegerControl__Group_3__0
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryIntegerControl__Group_3__0_in_rule__DictionaryIntegerControl__Group__3__Impl39909);
rule__DictionaryIntegerControl__Group_3__0();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryIntegerControlAccess().getGroup_3());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryIntegerControl__Group__3__Impl"
// $ANTLR start "rule__DictionaryIntegerControl__Group__4"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19524:1: rule__DictionaryIntegerControl__Group__4 : rule__DictionaryIntegerControl__Group__4__Impl ;
public final void rule__DictionaryIntegerControl__Group__4() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19528:1: ( rule__DictionaryIntegerControl__Group__4__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19529:2: rule__DictionaryIntegerControl__Group__4__Impl
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryIntegerControl__Group__4__Impl_in_rule__DictionaryIntegerControl__Group__439940);
rule__DictionaryIntegerControl__Group__4__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryIntegerControl__Group__4"
// $ANTLR start "rule__DictionaryIntegerControl__Group__4__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19535:1: rule__DictionaryIntegerControl__Group__4__Impl : ( ( rule__DictionaryIntegerControl__Group_4__0 )? ) ;
public final void rule__DictionaryIntegerControl__Group__4__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19539:1: ( ( ( rule__DictionaryIntegerControl__Group_4__0 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19540:1: ( ( rule__DictionaryIntegerControl__Group_4__0 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19540:1: ( ( rule__DictionaryIntegerControl__Group_4__0 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19541:1: ( rule__DictionaryIntegerControl__Group_4__0 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryIntegerControlAccess().getGroup_4());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19542:1: ( rule__DictionaryIntegerControl__Group_4__0 )?
int alt156=2;
int LA156_0 = input.LA(1);
if ( (LA156_0==67) ) {
alt156=1;
}
switch (alt156) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19542:2: rule__DictionaryIntegerControl__Group_4__0
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryIntegerControl__Group_4__0_in_rule__DictionaryIntegerControl__Group__4__Impl39967);
rule__DictionaryIntegerControl__Group_4__0();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryIntegerControlAccess().getGroup_4());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryIntegerControl__Group__4__Impl"
// $ANTLR start "rule__DictionaryIntegerControl__Group_3__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19562:1: rule__DictionaryIntegerControl__Group_3__0 : rule__DictionaryIntegerControl__Group_3__0__Impl rule__DictionaryIntegerControl__Group_3__1 ;
public final void rule__DictionaryIntegerControl__Group_3__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19566:1: ( rule__DictionaryIntegerControl__Group_3__0__Impl rule__DictionaryIntegerControl__Group_3__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19567:2: rule__DictionaryIntegerControl__Group_3__0__Impl rule__DictionaryIntegerControl__Group_3__1
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryIntegerControl__Group_3__0__Impl_in_rule__DictionaryIntegerControl__Group_3__040008);
rule__DictionaryIntegerControl__Group_3__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryIntegerControl__Group_3__1_in_rule__DictionaryIntegerControl__Group_3__040011);
rule__DictionaryIntegerControl__Group_3__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryIntegerControl__Group_3__0"
// $ANTLR start "rule__DictionaryIntegerControl__Group_3__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19574:1: rule__DictionaryIntegerControl__Group_3__0__Impl : ( 'ref' ) ;
public final void rule__DictionaryIntegerControl__Group_3__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19578:1: ( ( 'ref' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19579:1: ( 'ref' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19579:1: ( 'ref' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19580:1: 'ref'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryIntegerControlAccess().getRefKeyword_3_0());
}
match(input,126,FollowSets001.FOLLOW_126_in_rule__DictionaryIntegerControl__Group_3__0__Impl40039); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryIntegerControlAccess().getRefKeyword_3_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryIntegerControl__Group_3__0__Impl"
// $ANTLR start "rule__DictionaryIntegerControl__Group_3__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19593:1: rule__DictionaryIntegerControl__Group_3__1 : rule__DictionaryIntegerControl__Group_3__1__Impl ;
public final void rule__DictionaryIntegerControl__Group_3__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19597:1: ( rule__DictionaryIntegerControl__Group_3__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19598:2: rule__DictionaryIntegerControl__Group_3__1__Impl
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryIntegerControl__Group_3__1__Impl_in_rule__DictionaryIntegerControl__Group_3__140070);
rule__DictionaryIntegerControl__Group_3__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryIntegerControl__Group_3__1"
// $ANTLR start "rule__DictionaryIntegerControl__Group_3__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19604:1: rule__DictionaryIntegerControl__Group_3__1__Impl : ( ( rule__DictionaryIntegerControl__RefAssignment_3_1 ) ) ;
public final void rule__DictionaryIntegerControl__Group_3__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19608:1: ( ( ( rule__DictionaryIntegerControl__RefAssignment_3_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19609:1: ( ( rule__DictionaryIntegerControl__RefAssignment_3_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19609:1: ( ( rule__DictionaryIntegerControl__RefAssignment_3_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19610:1: ( rule__DictionaryIntegerControl__RefAssignment_3_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryIntegerControlAccess().getRefAssignment_3_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19611:1: ( rule__DictionaryIntegerControl__RefAssignment_3_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19611:2: rule__DictionaryIntegerControl__RefAssignment_3_1
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryIntegerControl__RefAssignment_3_1_in_rule__DictionaryIntegerControl__Group_3__1__Impl40097);
rule__DictionaryIntegerControl__RefAssignment_3_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryIntegerControlAccess().getRefAssignment_3_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryIntegerControl__Group_3__1__Impl"
// $ANTLR start "rule__DictionaryIntegerControl__Group_4__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19625:1: rule__DictionaryIntegerControl__Group_4__0 : rule__DictionaryIntegerControl__Group_4__0__Impl rule__DictionaryIntegerControl__Group_4__1 ;
public final void rule__DictionaryIntegerControl__Group_4__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19629:1: ( rule__DictionaryIntegerControl__Group_4__0__Impl rule__DictionaryIntegerControl__Group_4__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19630:2: rule__DictionaryIntegerControl__Group_4__0__Impl rule__DictionaryIntegerControl__Group_4__1
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryIntegerControl__Group_4__0__Impl_in_rule__DictionaryIntegerControl__Group_4__040131);
rule__DictionaryIntegerControl__Group_4__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryIntegerControl__Group_4__1_in_rule__DictionaryIntegerControl__Group_4__040134);
rule__DictionaryIntegerControl__Group_4__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryIntegerControl__Group_4__0"
// $ANTLR start "rule__DictionaryIntegerControl__Group_4__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19637:1: rule__DictionaryIntegerControl__Group_4__0__Impl : ( '{' ) ;
public final void rule__DictionaryIntegerControl__Group_4__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19641:1: ( ( '{' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19642:1: ( '{' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19642:1: ( '{' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19643:1: '{'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryIntegerControlAccess().getLeftCurlyBracketKeyword_4_0());
}
match(input,67,FollowSets001.FOLLOW_67_in_rule__DictionaryIntegerControl__Group_4__0__Impl40162); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryIntegerControlAccess().getLeftCurlyBracketKeyword_4_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryIntegerControl__Group_4__0__Impl"
// $ANTLR start "rule__DictionaryIntegerControl__Group_4__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19656:1: rule__DictionaryIntegerControl__Group_4__1 : rule__DictionaryIntegerControl__Group_4__1__Impl rule__DictionaryIntegerControl__Group_4__2 ;
public final void rule__DictionaryIntegerControl__Group_4__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19660:1: ( rule__DictionaryIntegerControl__Group_4__1__Impl rule__DictionaryIntegerControl__Group_4__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19661:2: rule__DictionaryIntegerControl__Group_4__1__Impl rule__DictionaryIntegerControl__Group_4__2
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryIntegerControl__Group_4__1__Impl_in_rule__DictionaryIntegerControl__Group_4__140193);
rule__DictionaryIntegerControl__Group_4__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryIntegerControl__Group_4__2_in_rule__DictionaryIntegerControl__Group_4__140196);
rule__DictionaryIntegerControl__Group_4__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryIntegerControl__Group_4__1"
// $ANTLR start "rule__DictionaryIntegerControl__Group_4__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19668:1: rule__DictionaryIntegerControl__Group_4__1__Impl : ( ( rule__DictionaryIntegerControl__BaseControlAssignment_4_1 ) ) ;
public final void rule__DictionaryIntegerControl__Group_4__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19672:1: ( ( ( rule__DictionaryIntegerControl__BaseControlAssignment_4_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19673:1: ( ( rule__DictionaryIntegerControl__BaseControlAssignment_4_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19673:1: ( ( rule__DictionaryIntegerControl__BaseControlAssignment_4_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19674:1: ( rule__DictionaryIntegerControl__BaseControlAssignment_4_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryIntegerControlAccess().getBaseControlAssignment_4_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19675:1: ( rule__DictionaryIntegerControl__BaseControlAssignment_4_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19675:2: rule__DictionaryIntegerControl__BaseControlAssignment_4_1
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryIntegerControl__BaseControlAssignment_4_1_in_rule__DictionaryIntegerControl__Group_4__1__Impl40223);
rule__DictionaryIntegerControl__BaseControlAssignment_4_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryIntegerControlAccess().getBaseControlAssignment_4_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryIntegerControl__Group_4__1__Impl"
// $ANTLR start "rule__DictionaryIntegerControl__Group_4__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19685:1: rule__DictionaryIntegerControl__Group_4__2 : rule__DictionaryIntegerControl__Group_4__2__Impl rule__DictionaryIntegerControl__Group_4__3 ;
public final void rule__DictionaryIntegerControl__Group_4__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19689:1: ( rule__DictionaryIntegerControl__Group_4__2__Impl rule__DictionaryIntegerControl__Group_4__3 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19690:2: rule__DictionaryIntegerControl__Group_4__2__Impl rule__DictionaryIntegerControl__Group_4__3
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryIntegerControl__Group_4__2__Impl_in_rule__DictionaryIntegerControl__Group_4__240253);
rule__DictionaryIntegerControl__Group_4__2__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryIntegerControl__Group_4__3_in_rule__DictionaryIntegerControl__Group_4__240256);
rule__DictionaryIntegerControl__Group_4__3();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryIntegerControl__Group_4__2"
// $ANTLR start "rule__DictionaryIntegerControl__Group_4__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19697:1: rule__DictionaryIntegerControl__Group_4__2__Impl : ( ( rule__DictionaryIntegerControl__OptionsAssignment_4_2 )* ) ;
public final void rule__DictionaryIntegerControl__Group_4__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19701:1: ( ( ( rule__DictionaryIntegerControl__OptionsAssignment_4_2 )* ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19702:1: ( ( rule__DictionaryIntegerControl__OptionsAssignment_4_2 )* )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19702:1: ( ( rule__DictionaryIntegerControl__OptionsAssignment_4_2 )* )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19703:1: ( rule__DictionaryIntegerControl__OptionsAssignment_4_2 )*
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryIntegerControlAccess().getOptionsAssignment_4_2());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19704:1: ( rule__DictionaryIntegerControl__OptionsAssignment_4_2 )*
loop157:
do {
int alt157=2;
int LA157_0 = input.LA(1);
if ( (LA157_0==130) ) {
alt157=1;
}
switch (alt157) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19704:2: rule__DictionaryIntegerControl__OptionsAssignment_4_2
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryIntegerControl__OptionsAssignment_4_2_in_rule__DictionaryIntegerControl__Group_4__2__Impl40283);
rule__DictionaryIntegerControl__OptionsAssignment_4_2();
state._fsp--;
if (state.failed) return ;
}
break;
default :
break loop157;
}
} while (true);
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryIntegerControlAccess().getOptionsAssignment_4_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryIntegerControl__Group_4__2__Impl"
// $ANTLR start "rule__DictionaryIntegerControl__Group_4__3"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19714:1: rule__DictionaryIntegerControl__Group_4__3 : rule__DictionaryIntegerControl__Group_4__3__Impl ;
public final void rule__DictionaryIntegerControl__Group_4__3() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19718:1: ( rule__DictionaryIntegerControl__Group_4__3__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19719:2: rule__DictionaryIntegerControl__Group_4__3__Impl
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryIntegerControl__Group_4__3__Impl_in_rule__DictionaryIntegerControl__Group_4__340314);
rule__DictionaryIntegerControl__Group_4__3__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryIntegerControl__Group_4__3"
// $ANTLR start "rule__DictionaryIntegerControl__Group_4__3__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19725:1: rule__DictionaryIntegerControl__Group_4__3__Impl : ( '}' ) ;
public final void rule__DictionaryIntegerControl__Group_4__3__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19729:1: ( ( '}' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19730:1: ( '}' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19730:1: ( '}' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19731:1: '}'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryIntegerControlAccess().getRightCurlyBracketKeyword_4_3());
}
match(input,68,FollowSets001.FOLLOW_68_in_rule__DictionaryIntegerControl__Group_4__3__Impl40342); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryIntegerControlAccess().getRightCurlyBracketKeyword_4_3());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryIntegerControl__Group_4__3__Impl"
// $ANTLR start "rule__DictionaryBigDecimalControl__Group__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19752:1: rule__DictionaryBigDecimalControl__Group__0 : rule__DictionaryBigDecimalControl__Group__0__Impl rule__DictionaryBigDecimalControl__Group__1 ;
public final void rule__DictionaryBigDecimalControl__Group__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19756:1: ( rule__DictionaryBigDecimalControl__Group__0__Impl rule__DictionaryBigDecimalControl__Group__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19757:2: rule__DictionaryBigDecimalControl__Group__0__Impl rule__DictionaryBigDecimalControl__Group__1
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryBigDecimalControl__Group__0__Impl_in_rule__DictionaryBigDecimalControl__Group__040381);
rule__DictionaryBigDecimalControl__Group__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryBigDecimalControl__Group__1_in_rule__DictionaryBigDecimalControl__Group__040384);
rule__DictionaryBigDecimalControl__Group__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryBigDecimalControl__Group__0"
// $ANTLR start "rule__DictionaryBigDecimalControl__Group__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19764:1: rule__DictionaryBigDecimalControl__Group__0__Impl : ( () ) ;
public final void rule__DictionaryBigDecimalControl__Group__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19768:1: ( ( () ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19769:1: ( () )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19769:1: ( () )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19770:1: ()
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryBigDecimalControlAccess().getDictionaryBigDecimalControlAction_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19771:1: ()
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19773:1:
{
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryBigDecimalControlAccess().getDictionaryBigDecimalControlAction_0());
}
}
}
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryBigDecimalControl__Group__0__Impl"
// $ANTLR start "rule__DictionaryBigDecimalControl__Group__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19783:1: rule__DictionaryBigDecimalControl__Group__1 : rule__DictionaryBigDecimalControl__Group__1__Impl rule__DictionaryBigDecimalControl__Group__2 ;
public final void rule__DictionaryBigDecimalControl__Group__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19787:1: ( rule__DictionaryBigDecimalControl__Group__1__Impl rule__DictionaryBigDecimalControl__Group__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19788:2: rule__DictionaryBigDecimalControl__Group__1__Impl rule__DictionaryBigDecimalControl__Group__2
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryBigDecimalControl__Group__1__Impl_in_rule__DictionaryBigDecimalControl__Group__140442);
rule__DictionaryBigDecimalControl__Group__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryBigDecimalControl__Group__2_in_rule__DictionaryBigDecimalControl__Group__140445);
rule__DictionaryBigDecimalControl__Group__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryBigDecimalControl__Group__1"
// $ANTLR start "rule__DictionaryBigDecimalControl__Group__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19795:1: rule__DictionaryBigDecimalControl__Group__1__Impl : ( 'bigdecimalcontrol' ) ;
public final void rule__DictionaryBigDecimalControl__Group__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19799:1: ( ( 'bigdecimalcontrol' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19800:1: ( 'bigdecimalcontrol' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19800:1: ( 'bigdecimalcontrol' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19801:1: 'bigdecimalcontrol'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryBigDecimalControlAccess().getBigdecimalcontrolKeyword_1());
}
match(input,132,FollowSets001.FOLLOW_132_in_rule__DictionaryBigDecimalControl__Group__1__Impl40473); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryBigDecimalControlAccess().getBigdecimalcontrolKeyword_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryBigDecimalControl__Group__1__Impl"
// $ANTLR start "rule__DictionaryBigDecimalControl__Group__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19814:1: rule__DictionaryBigDecimalControl__Group__2 : rule__DictionaryBigDecimalControl__Group__2__Impl rule__DictionaryBigDecimalControl__Group__3 ;
public final void rule__DictionaryBigDecimalControl__Group__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19818:1: ( rule__DictionaryBigDecimalControl__Group__2__Impl rule__DictionaryBigDecimalControl__Group__3 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19819:2: rule__DictionaryBigDecimalControl__Group__2__Impl rule__DictionaryBigDecimalControl__Group__3
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryBigDecimalControl__Group__2__Impl_in_rule__DictionaryBigDecimalControl__Group__240504);
rule__DictionaryBigDecimalControl__Group__2__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryBigDecimalControl__Group__3_in_rule__DictionaryBigDecimalControl__Group__240507);
rule__DictionaryBigDecimalControl__Group__3();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryBigDecimalControl__Group__2"
// $ANTLR start "rule__DictionaryBigDecimalControl__Group__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19826:1: rule__DictionaryBigDecimalControl__Group__2__Impl : ( ( rule__DictionaryBigDecimalControl__NameAssignment_2 )? ) ;
public final void rule__DictionaryBigDecimalControl__Group__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19830:1: ( ( ( rule__DictionaryBigDecimalControl__NameAssignment_2 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19831:1: ( ( rule__DictionaryBigDecimalControl__NameAssignment_2 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19831:1: ( ( rule__DictionaryBigDecimalControl__NameAssignment_2 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19832:1: ( rule__DictionaryBigDecimalControl__NameAssignment_2 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryBigDecimalControlAccess().getNameAssignment_2());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19833:1: ( rule__DictionaryBigDecimalControl__NameAssignment_2 )?
int alt158=2;
int LA158_0 = input.LA(1);
if ( (LA158_0==RULE_ID) ) {
alt158=1;
}
switch (alt158) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19833:2: rule__DictionaryBigDecimalControl__NameAssignment_2
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryBigDecimalControl__NameAssignment_2_in_rule__DictionaryBigDecimalControl__Group__2__Impl40534);
rule__DictionaryBigDecimalControl__NameAssignment_2();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryBigDecimalControlAccess().getNameAssignment_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryBigDecimalControl__Group__2__Impl"
// $ANTLR start "rule__DictionaryBigDecimalControl__Group__3"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19843:1: rule__DictionaryBigDecimalControl__Group__3 : rule__DictionaryBigDecimalControl__Group__3__Impl rule__DictionaryBigDecimalControl__Group__4 ;
public final void rule__DictionaryBigDecimalControl__Group__3() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19847:1: ( rule__DictionaryBigDecimalControl__Group__3__Impl rule__DictionaryBigDecimalControl__Group__4 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19848:2: rule__DictionaryBigDecimalControl__Group__3__Impl rule__DictionaryBigDecimalControl__Group__4
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryBigDecimalControl__Group__3__Impl_in_rule__DictionaryBigDecimalControl__Group__340565);
rule__DictionaryBigDecimalControl__Group__3__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryBigDecimalControl__Group__4_in_rule__DictionaryBigDecimalControl__Group__340568);
rule__DictionaryBigDecimalControl__Group__4();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryBigDecimalControl__Group__3"
// $ANTLR start "rule__DictionaryBigDecimalControl__Group__3__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19855:1: rule__DictionaryBigDecimalControl__Group__3__Impl : ( ( rule__DictionaryBigDecimalControl__Group_3__0 )? ) ;
public final void rule__DictionaryBigDecimalControl__Group__3__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19859:1: ( ( ( rule__DictionaryBigDecimalControl__Group_3__0 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19860:1: ( ( rule__DictionaryBigDecimalControl__Group_3__0 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19860:1: ( ( rule__DictionaryBigDecimalControl__Group_3__0 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19861:1: ( rule__DictionaryBigDecimalControl__Group_3__0 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryBigDecimalControlAccess().getGroup_3());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19862:1: ( rule__DictionaryBigDecimalControl__Group_3__0 )?
int alt159=2;
int LA159_0 = input.LA(1);
if ( (LA159_0==126) ) {
alt159=1;
}
switch (alt159) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19862:2: rule__DictionaryBigDecimalControl__Group_3__0
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryBigDecimalControl__Group_3__0_in_rule__DictionaryBigDecimalControl__Group__3__Impl40595);
rule__DictionaryBigDecimalControl__Group_3__0();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryBigDecimalControlAccess().getGroup_3());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryBigDecimalControl__Group__3__Impl"
// $ANTLR start "rule__DictionaryBigDecimalControl__Group__4"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19872:1: rule__DictionaryBigDecimalControl__Group__4 : rule__DictionaryBigDecimalControl__Group__4__Impl ;
public final void rule__DictionaryBigDecimalControl__Group__4() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19876:1: ( rule__DictionaryBigDecimalControl__Group__4__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19877:2: rule__DictionaryBigDecimalControl__Group__4__Impl
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryBigDecimalControl__Group__4__Impl_in_rule__DictionaryBigDecimalControl__Group__440626);
rule__DictionaryBigDecimalControl__Group__4__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryBigDecimalControl__Group__4"
// $ANTLR start "rule__DictionaryBigDecimalControl__Group__4__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19883:1: rule__DictionaryBigDecimalControl__Group__4__Impl : ( ( rule__DictionaryBigDecimalControl__Group_4__0 )? ) ;
public final void rule__DictionaryBigDecimalControl__Group__4__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19887:1: ( ( ( rule__DictionaryBigDecimalControl__Group_4__0 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19888:1: ( ( rule__DictionaryBigDecimalControl__Group_4__0 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19888:1: ( ( rule__DictionaryBigDecimalControl__Group_4__0 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19889:1: ( rule__DictionaryBigDecimalControl__Group_4__0 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryBigDecimalControlAccess().getGroup_4());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19890:1: ( rule__DictionaryBigDecimalControl__Group_4__0 )?
int alt160=2;
int LA160_0 = input.LA(1);
if ( (LA160_0==67) ) {
alt160=1;
}
switch (alt160) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19890:2: rule__DictionaryBigDecimalControl__Group_4__0
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryBigDecimalControl__Group_4__0_in_rule__DictionaryBigDecimalControl__Group__4__Impl40653);
rule__DictionaryBigDecimalControl__Group_4__0();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryBigDecimalControlAccess().getGroup_4());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryBigDecimalControl__Group__4__Impl"
// $ANTLR start "rule__DictionaryBigDecimalControl__Group_3__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19910:1: rule__DictionaryBigDecimalControl__Group_3__0 : rule__DictionaryBigDecimalControl__Group_3__0__Impl rule__DictionaryBigDecimalControl__Group_3__1 ;
public final void rule__DictionaryBigDecimalControl__Group_3__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19914:1: ( rule__DictionaryBigDecimalControl__Group_3__0__Impl rule__DictionaryBigDecimalControl__Group_3__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19915:2: rule__DictionaryBigDecimalControl__Group_3__0__Impl rule__DictionaryBigDecimalControl__Group_3__1
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryBigDecimalControl__Group_3__0__Impl_in_rule__DictionaryBigDecimalControl__Group_3__040694);
rule__DictionaryBigDecimalControl__Group_3__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryBigDecimalControl__Group_3__1_in_rule__DictionaryBigDecimalControl__Group_3__040697);
rule__DictionaryBigDecimalControl__Group_3__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryBigDecimalControl__Group_3__0"
// $ANTLR start "rule__DictionaryBigDecimalControl__Group_3__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19922:1: rule__DictionaryBigDecimalControl__Group_3__0__Impl : ( 'ref' ) ;
public final void rule__DictionaryBigDecimalControl__Group_3__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19926:1: ( ( 'ref' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19927:1: ( 'ref' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19927:1: ( 'ref' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19928:1: 'ref'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryBigDecimalControlAccess().getRefKeyword_3_0());
}
match(input,126,FollowSets001.FOLLOW_126_in_rule__DictionaryBigDecimalControl__Group_3__0__Impl40725); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryBigDecimalControlAccess().getRefKeyword_3_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryBigDecimalControl__Group_3__0__Impl"
// $ANTLR start "rule__DictionaryBigDecimalControl__Group_3__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19941:1: rule__DictionaryBigDecimalControl__Group_3__1 : rule__DictionaryBigDecimalControl__Group_3__1__Impl ;
public final void rule__DictionaryBigDecimalControl__Group_3__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19945:1: ( rule__DictionaryBigDecimalControl__Group_3__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19946:2: rule__DictionaryBigDecimalControl__Group_3__1__Impl
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryBigDecimalControl__Group_3__1__Impl_in_rule__DictionaryBigDecimalControl__Group_3__140756);
rule__DictionaryBigDecimalControl__Group_3__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryBigDecimalControl__Group_3__1"
// $ANTLR start "rule__DictionaryBigDecimalControl__Group_3__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19952:1: rule__DictionaryBigDecimalControl__Group_3__1__Impl : ( ( rule__DictionaryBigDecimalControl__RefAssignment_3_1 ) ) ;
public final void rule__DictionaryBigDecimalControl__Group_3__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19956:1: ( ( ( rule__DictionaryBigDecimalControl__RefAssignment_3_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19957:1: ( ( rule__DictionaryBigDecimalControl__RefAssignment_3_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19957:1: ( ( rule__DictionaryBigDecimalControl__RefAssignment_3_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19958:1: ( rule__DictionaryBigDecimalControl__RefAssignment_3_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryBigDecimalControlAccess().getRefAssignment_3_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19959:1: ( rule__DictionaryBigDecimalControl__RefAssignment_3_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19959:2: rule__DictionaryBigDecimalControl__RefAssignment_3_1
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryBigDecimalControl__RefAssignment_3_1_in_rule__DictionaryBigDecimalControl__Group_3__1__Impl40783);
rule__DictionaryBigDecimalControl__RefAssignment_3_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryBigDecimalControlAccess().getRefAssignment_3_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryBigDecimalControl__Group_3__1__Impl"
// $ANTLR start "rule__DictionaryBigDecimalControl__Group_4__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19973:1: rule__DictionaryBigDecimalControl__Group_4__0 : rule__DictionaryBigDecimalControl__Group_4__0__Impl rule__DictionaryBigDecimalControl__Group_4__1 ;
public final void rule__DictionaryBigDecimalControl__Group_4__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19977:1: ( rule__DictionaryBigDecimalControl__Group_4__0__Impl rule__DictionaryBigDecimalControl__Group_4__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19978:2: rule__DictionaryBigDecimalControl__Group_4__0__Impl rule__DictionaryBigDecimalControl__Group_4__1
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryBigDecimalControl__Group_4__0__Impl_in_rule__DictionaryBigDecimalControl__Group_4__040817);
rule__DictionaryBigDecimalControl__Group_4__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryBigDecimalControl__Group_4__1_in_rule__DictionaryBigDecimalControl__Group_4__040820);
rule__DictionaryBigDecimalControl__Group_4__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryBigDecimalControl__Group_4__0"
// $ANTLR start "rule__DictionaryBigDecimalControl__Group_4__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19985:1: rule__DictionaryBigDecimalControl__Group_4__0__Impl : ( '{' ) ;
public final void rule__DictionaryBigDecimalControl__Group_4__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19989:1: ( ( '{' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19990:1: ( '{' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19990:1: ( '{' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:19991:1: '{'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryBigDecimalControlAccess().getLeftCurlyBracketKeyword_4_0());
}
match(input,67,FollowSets001.FOLLOW_67_in_rule__DictionaryBigDecimalControl__Group_4__0__Impl40848); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryBigDecimalControlAccess().getLeftCurlyBracketKeyword_4_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryBigDecimalControl__Group_4__0__Impl"
// $ANTLR start "rule__DictionaryBigDecimalControl__Group_4__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20004:1: rule__DictionaryBigDecimalControl__Group_4__1 : rule__DictionaryBigDecimalControl__Group_4__1__Impl rule__DictionaryBigDecimalControl__Group_4__2 ;
public final void rule__DictionaryBigDecimalControl__Group_4__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20008:1: ( rule__DictionaryBigDecimalControl__Group_4__1__Impl rule__DictionaryBigDecimalControl__Group_4__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20009:2: rule__DictionaryBigDecimalControl__Group_4__1__Impl rule__DictionaryBigDecimalControl__Group_4__2
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryBigDecimalControl__Group_4__1__Impl_in_rule__DictionaryBigDecimalControl__Group_4__140879);
rule__DictionaryBigDecimalControl__Group_4__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryBigDecimalControl__Group_4__2_in_rule__DictionaryBigDecimalControl__Group_4__140882);
rule__DictionaryBigDecimalControl__Group_4__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryBigDecimalControl__Group_4__1"
// $ANTLR start "rule__DictionaryBigDecimalControl__Group_4__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20016:1: rule__DictionaryBigDecimalControl__Group_4__1__Impl : ( ( rule__DictionaryBigDecimalControl__BaseControlAssignment_4_1 ) ) ;
public final void rule__DictionaryBigDecimalControl__Group_4__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20020:1: ( ( ( rule__DictionaryBigDecimalControl__BaseControlAssignment_4_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20021:1: ( ( rule__DictionaryBigDecimalControl__BaseControlAssignment_4_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20021:1: ( ( rule__DictionaryBigDecimalControl__BaseControlAssignment_4_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20022:1: ( rule__DictionaryBigDecimalControl__BaseControlAssignment_4_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryBigDecimalControlAccess().getBaseControlAssignment_4_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20023:1: ( rule__DictionaryBigDecimalControl__BaseControlAssignment_4_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20023:2: rule__DictionaryBigDecimalControl__BaseControlAssignment_4_1
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryBigDecimalControl__BaseControlAssignment_4_1_in_rule__DictionaryBigDecimalControl__Group_4__1__Impl40909);
rule__DictionaryBigDecimalControl__BaseControlAssignment_4_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryBigDecimalControlAccess().getBaseControlAssignment_4_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryBigDecimalControl__Group_4__1__Impl"
// $ANTLR start "rule__DictionaryBigDecimalControl__Group_4__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20033:1: rule__DictionaryBigDecimalControl__Group_4__2 : rule__DictionaryBigDecimalControl__Group_4__2__Impl ;
public final void rule__DictionaryBigDecimalControl__Group_4__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20037:1: ( rule__DictionaryBigDecimalControl__Group_4__2__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20038:2: rule__DictionaryBigDecimalControl__Group_4__2__Impl
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryBigDecimalControl__Group_4__2__Impl_in_rule__DictionaryBigDecimalControl__Group_4__240939);
rule__DictionaryBigDecimalControl__Group_4__2__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryBigDecimalControl__Group_4__2"
// $ANTLR start "rule__DictionaryBigDecimalControl__Group_4__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20044:1: rule__DictionaryBigDecimalControl__Group_4__2__Impl : ( '}' ) ;
public final void rule__DictionaryBigDecimalControl__Group_4__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20048:1: ( ( '}' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20049:1: ( '}' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20049:1: ( '}' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20050:1: '}'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryBigDecimalControlAccess().getRightCurlyBracketKeyword_4_2());
}
match(input,68,FollowSets001.FOLLOW_68_in_rule__DictionaryBigDecimalControl__Group_4__2__Impl40967); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryBigDecimalControlAccess().getRightCurlyBracketKeyword_4_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryBigDecimalControl__Group_4__2__Impl"
// $ANTLR start "rule__DictionaryBooleanControl__Group__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20069:1: rule__DictionaryBooleanControl__Group__0 : rule__DictionaryBooleanControl__Group__0__Impl rule__DictionaryBooleanControl__Group__1 ;
public final void rule__DictionaryBooleanControl__Group__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20073:1: ( rule__DictionaryBooleanControl__Group__0__Impl rule__DictionaryBooleanControl__Group__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20074:2: rule__DictionaryBooleanControl__Group__0__Impl rule__DictionaryBooleanControl__Group__1
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryBooleanControl__Group__0__Impl_in_rule__DictionaryBooleanControl__Group__041004);
rule__DictionaryBooleanControl__Group__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryBooleanControl__Group__1_in_rule__DictionaryBooleanControl__Group__041007);
rule__DictionaryBooleanControl__Group__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryBooleanControl__Group__0"
// $ANTLR start "rule__DictionaryBooleanControl__Group__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20081:1: rule__DictionaryBooleanControl__Group__0__Impl : ( () ) ;
public final void rule__DictionaryBooleanControl__Group__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20085:1: ( ( () ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20086:1: ( () )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20086:1: ( () )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20087:1: ()
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryBooleanControlAccess().getDictionaryBooleanControlAction_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20088:1: ()
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20090:1:
{
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryBooleanControlAccess().getDictionaryBooleanControlAction_0());
}
}
}
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryBooleanControl__Group__0__Impl"
// $ANTLR start "rule__DictionaryBooleanControl__Group__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20100:1: rule__DictionaryBooleanControl__Group__1 : rule__DictionaryBooleanControl__Group__1__Impl rule__DictionaryBooleanControl__Group__2 ;
public final void rule__DictionaryBooleanControl__Group__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20104:1: ( rule__DictionaryBooleanControl__Group__1__Impl rule__DictionaryBooleanControl__Group__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20105:2: rule__DictionaryBooleanControl__Group__1__Impl rule__DictionaryBooleanControl__Group__2
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryBooleanControl__Group__1__Impl_in_rule__DictionaryBooleanControl__Group__141065);
rule__DictionaryBooleanControl__Group__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryBooleanControl__Group__2_in_rule__DictionaryBooleanControl__Group__141068);
rule__DictionaryBooleanControl__Group__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryBooleanControl__Group__1"
// $ANTLR start "rule__DictionaryBooleanControl__Group__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20112:1: rule__DictionaryBooleanControl__Group__1__Impl : ( 'booleancontrol' ) ;
public final void rule__DictionaryBooleanControl__Group__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20116:1: ( ( 'booleancontrol' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20117:1: ( 'booleancontrol' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20117:1: ( 'booleancontrol' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20118:1: 'booleancontrol'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryBooleanControlAccess().getBooleancontrolKeyword_1());
}
match(input,133,FollowSets001.FOLLOW_133_in_rule__DictionaryBooleanControl__Group__1__Impl41096); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryBooleanControlAccess().getBooleancontrolKeyword_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryBooleanControl__Group__1__Impl"
// $ANTLR start "rule__DictionaryBooleanControl__Group__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20131:1: rule__DictionaryBooleanControl__Group__2 : rule__DictionaryBooleanControl__Group__2__Impl rule__DictionaryBooleanControl__Group__3 ;
public final void rule__DictionaryBooleanControl__Group__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20135:1: ( rule__DictionaryBooleanControl__Group__2__Impl rule__DictionaryBooleanControl__Group__3 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20136:2: rule__DictionaryBooleanControl__Group__2__Impl rule__DictionaryBooleanControl__Group__3
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryBooleanControl__Group__2__Impl_in_rule__DictionaryBooleanControl__Group__241127);
rule__DictionaryBooleanControl__Group__2__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryBooleanControl__Group__3_in_rule__DictionaryBooleanControl__Group__241130);
rule__DictionaryBooleanControl__Group__3();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryBooleanControl__Group__2"
// $ANTLR start "rule__DictionaryBooleanControl__Group__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20143:1: rule__DictionaryBooleanControl__Group__2__Impl : ( ( rule__DictionaryBooleanControl__NameAssignment_2 )? ) ;
public final void rule__DictionaryBooleanControl__Group__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20147:1: ( ( ( rule__DictionaryBooleanControl__NameAssignment_2 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20148:1: ( ( rule__DictionaryBooleanControl__NameAssignment_2 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20148:1: ( ( rule__DictionaryBooleanControl__NameAssignment_2 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20149:1: ( rule__DictionaryBooleanControl__NameAssignment_2 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryBooleanControlAccess().getNameAssignment_2());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20150:1: ( rule__DictionaryBooleanControl__NameAssignment_2 )?
int alt161=2;
int LA161_0 = input.LA(1);
if ( (LA161_0==RULE_ID) ) {
alt161=1;
}
switch (alt161) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20150:2: rule__DictionaryBooleanControl__NameAssignment_2
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryBooleanControl__NameAssignment_2_in_rule__DictionaryBooleanControl__Group__2__Impl41157);
rule__DictionaryBooleanControl__NameAssignment_2();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryBooleanControlAccess().getNameAssignment_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryBooleanControl__Group__2__Impl"
// $ANTLR start "rule__DictionaryBooleanControl__Group__3"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20160:1: rule__DictionaryBooleanControl__Group__3 : rule__DictionaryBooleanControl__Group__3__Impl rule__DictionaryBooleanControl__Group__4 ;
public final void rule__DictionaryBooleanControl__Group__3() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20164:1: ( rule__DictionaryBooleanControl__Group__3__Impl rule__DictionaryBooleanControl__Group__4 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20165:2: rule__DictionaryBooleanControl__Group__3__Impl rule__DictionaryBooleanControl__Group__4
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryBooleanControl__Group__3__Impl_in_rule__DictionaryBooleanControl__Group__341188);
rule__DictionaryBooleanControl__Group__3__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryBooleanControl__Group__4_in_rule__DictionaryBooleanControl__Group__341191);
rule__DictionaryBooleanControl__Group__4();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryBooleanControl__Group__3"
// $ANTLR start "rule__DictionaryBooleanControl__Group__3__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20172:1: rule__DictionaryBooleanControl__Group__3__Impl : ( ( rule__DictionaryBooleanControl__Group_3__0 )? ) ;
public final void rule__DictionaryBooleanControl__Group__3__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20176:1: ( ( ( rule__DictionaryBooleanControl__Group_3__0 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20177:1: ( ( rule__DictionaryBooleanControl__Group_3__0 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20177:1: ( ( rule__DictionaryBooleanControl__Group_3__0 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20178:1: ( rule__DictionaryBooleanControl__Group_3__0 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryBooleanControlAccess().getGroup_3());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20179:1: ( rule__DictionaryBooleanControl__Group_3__0 )?
int alt162=2;
int LA162_0 = input.LA(1);
if ( (LA162_0==126) ) {
alt162=1;
}
switch (alt162) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20179:2: rule__DictionaryBooleanControl__Group_3__0
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryBooleanControl__Group_3__0_in_rule__DictionaryBooleanControl__Group__3__Impl41218);
rule__DictionaryBooleanControl__Group_3__0();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryBooleanControlAccess().getGroup_3());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryBooleanControl__Group__3__Impl"
// $ANTLR start "rule__DictionaryBooleanControl__Group__4"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20189:1: rule__DictionaryBooleanControl__Group__4 : rule__DictionaryBooleanControl__Group__4__Impl ;
public final void rule__DictionaryBooleanControl__Group__4() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20193:1: ( rule__DictionaryBooleanControl__Group__4__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20194:2: rule__DictionaryBooleanControl__Group__4__Impl
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryBooleanControl__Group__4__Impl_in_rule__DictionaryBooleanControl__Group__441249);
rule__DictionaryBooleanControl__Group__4__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryBooleanControl__Group__4"
// $ANTLR start "rule__DictionaryBooleanControl__Group__4__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20200:1: rule__DictionaryBooleanControl__Group__4__Impl : ( ( rule__DictionaryBooleanControl__Group_4__0 )? ) ;
public final void rule__DictionaryBooleanControl__Group__4__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20204:1: ( ( ( rule__DictionaryBooleanControl__Group_4__0 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20205:1: ( ( rule__DictionaryBooleanControl__Group_4__0 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20205:1: ( ( rule__DictionaryBooleanControl__Group_4__0 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20206:1: ( rule__DictionaryBooleanControl__Group_4__0 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryBooleanControlAccess().getGroup_4());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20207:1: ( rule__DictionaryBooleanControl__Group_4__0 )?
int alt163=2;
int LA163_0 = input.LA(1);
if ( (LA163_0==67) ) {
alt163=1;
}
switch (alt163) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20207:2: rule__DictionaryBooleanControl__Group_4__0
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryBooleanControl__Group_4__0_in_rule__DictionaryBooleanControl__Group__4__Impl41276);
rule__DictionaryBooleanControl__Group_4__0();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryBooleanControlAccess().getGroup_4());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryBooleanControl__Group__4__Impl"
// $ANTLR start "rule__DictionaryBooleanControl__Group_3__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20227:1: rule__DictionaryBooleanControl__Group_3__0 : rule__DictionaryBooleanControl__Group_3__0__Impl rule__DictionaryBooleanControl__Group_3__1 ;
public final void rule__DictionaryBooleanControl__Group_3__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20231:1: ( rule__DictionaryBooleanControl__Group_3__0__Impl rule__DictionaryBooleanControl__Group_3__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20232:2: rule__DictionaryBooleanControl__Group_3__0__Impl rule__DictionaryBooleanControl__Group_3__1
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryBooleanControl__Group_3__0__Impl_in_rule__DictionaryBooleanControl__Group_3__041317);
rule__DictionaryBooleanControl__Group_3__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryBooleanControl__Group_3__1_in_rule__DictionaryBooleanControl__Group_3__041320);
rule__DictionaryBooleanControl__Group_3__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryBooleanControl__Group_3__0"
// $ANTLR start "rule__DictionaryBooleanControl__Group_3__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20239:1: rule__DictionaryBooleanControl__Group_3__0__Impl : ( 'ref' ) ;
public final void rule__DictionaryBooleanControl__Group_3__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20243:1: ( ( 'ref' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20244:1: ( 'ref' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20244:1: ( 'ref' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20245:1: 'ref'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryBooleanControlAccess().getRefKeyword_3_0());
}
match(input,126,FollowSets001.FOLLOW_126_in_rule__DictionaryBooleanControl__Group_3__0__Impl41348); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryBooleanControlAccess().getRefKeyword_3_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryBooleanControl__Group_3__0__Impl"
// $ANTLR start "rule__DictionaryBooleanControl__Group_3__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20258:1: rule__DictionaryBooleanControl__Group_3__1 : rule__DictionaryBooleanControl__Group_3__1__Impl ;
public final void rule__DictionaryBooleanControl__Group_3__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20262:1: ( rule__DictionaryBooleanControl__Group_3__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20263:2: rule__DictionaryBooleanControl__Group_3__1__Impl
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryBooleanControl__Group_3__1__Impl_in_rule__DictionaryBooleanControl__Group_3__141379);
rule__DictionaryBooleanControl__Group_3__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryBooleanControl__Group_3__1"
// $ANTLR start "rule__DictionaryBooleanControl__Group_3__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20269:1: rule__DictionaryBooleanControl__Group_3__1__Impl : ( ( rule__DictionaryBooleanControl__RefAssignment_3_1 ) ) ;
public final void rule__DictionaryBooleanControl__Group_3__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20273:1: ( ( ( rule__DictionaryBooleanControl__RefAssignment_3_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20274:1: ( ( rule__DictionaryBooleanControl__RefAssignment_3_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20274:1: ( ( rule__DictionaryBooleanControl__RefAssignment_3_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20275:1: ( rule__DictionaryBooleanControl__RefAssignment_3_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryBooleanControlAccess().getRefAssignment_3_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20276:1: ( rule__DictionaryBooleanControl__RefAssignment_3_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20276:2: rule__DictionaryBooleanControl__RefAssignment_3_1
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryBooleanControl__RefAssignment_3_1_in_rule__DictionaryBooleanControl__Group_3__1__Impl41406);
rule__DictionaryBooleanControl__RefAssignment_3_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryBooleanControlAccess().getRefAssignment_3_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryBooleanControl__Group_3__1__Impl"
// $ANTLR start "rule__DictionaryBooleanControl__Group_4__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20290:1: rule__DictionaryBooleanControl__Group_4__0 : rule__DictionaryBooleanControl__Group_4__0__Impl rule__DictionaryBooleanControl__Group_4__1 ;
public final void rule__DictionaryBooleanControl__Group_4__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20294:1: ( rule__DictionaryBooleanControl__Group_4__0__Impl rule__DictionaryBooleanControl__Group_4__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20295:2: rule__DictionaryBooleanControl__Group_4__0__Impl rule__DictionaryBooleanControl__Group_4__1
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryBooleanControl__Group_4__0__Impl_in_rule__DictionaryBooleanControl__Group_4__041440);
rule__DictionaryBooleanControl__Group_4__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryBooleanControl__Group_4__1_in_rule__DictionaryBooleanControl__Group_4__041443);
rule__DictionaryBooleanControl__Group_4__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryBooleanControl__Group_4__0"
// $ANTLR start "rule__DictionaryBooleanControl__Group_4__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20302:1: rule__DictionaryBooleanControl__Group_4__0__Impl : ( '{' ) ;
public final void rule__DictionaryBooleanControl__Group_4__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20306:1: ( ( '{' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20307:1: ( '{' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20307:1: ( '{' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20308:1: '{'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryBooleanControlAccess().getLeftCurlyBracketKeyword_4_0());
}
match(input,67,FollowSets001.FOLLOW_67_in_rule__DictionaryBooleanControl__Group_4__0__Impl41471); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryBooleanControlAccess().getLeftCurlyBracketKeyword_4_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryBooleanControl__Group_4__0__Impl"
// $ANTLR start "rule__DictionaryBooleanControl__Group_4__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20321:1: rule__DictionaryBooleanControl__Group_4__1 : rule__DictionaryBooleanControl__Group_4__1__Impl rule__DictionaryBooleanControl__Group_4__2 ;
public final void rule__DictionaryBooleanControl__Group_4__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20325:1: ( rule__DictionaryBooleanControl__Group_4__1__Impl rule__DictionaryBooleanControl__Group_4__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20326:2: rule__DictionaryBooleanControl__Group_4__1__Impl rule__DictionaryBooleanControl__Group_4__2
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryBooleanControl__Group_4__1__Impl_in_rule__DictionaryBooleanControl__Group_4__141502);
rule__DictionaryBooleanControl__Group_4__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryBooleanControl__Group_4__2_in_rule__DictionaryBooleanControl__Group_4__141505);
rule__DictionaryBooleanControl__Group_4__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryBooleanControl__Group_4__1"
// $ANTLR start "rule__DictionaryBooleanControl__Group_4__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20333:1: rule__DictionaryBooleanControl__Group_4__1__Impl : ( ( rule__DictionaryBooleanControl__BaseControlAssignment_4_1 ) ) ;
public final void rule__DictionaryBooleanControl__Group_4__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20337:1: ( ( ( rule__DictionaryBooleanControl__BaseControlAssignment_4_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20338:1: ( ( rule__DictionaryBooleanControl__BaseControlAssignment_4_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20338:1: ( ( rule__DictionaryBooleanControl__BaseControlAssignment_4_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20339:1: ( rule__DictionaryBooleanControl__BaseControlAssignment_4_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryBooleanControlAccess().getBaseControlAssignment_4_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20340:1: ( rule__DictionaryBooleanControl__BaseControlAssignment_4_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20340:2: rule__DictionaryBooleanControl__BaseControlAssignment_4_1
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryBooleanControl__BaseControlAssignment_4_1_in_rule__DictionaryBooleanControl__Group_4__1__Impl41532);
rule__DictionaryBooleanControl__BaseControlAssignment_4_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryBooleanControlAccess().getBaseControlAssignment_4_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryBooleanControl__Group_4__1__Impl"
// $ANTLR start "rule__DictionaryBooleanControl__Group_4__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20350:1: rule__DictionaryBooleanControl__Group_4__2 : rule__DictionaryBooleanControl__Group_4__2__Impl ;
public final void rule__DictionaryBooleanControl__Group_4__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20354:1: ( rule__DictionaryBooleanControl__Group_4__2__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20355:2: rule__DictionaryBooleanControl__Group_4__2__Impl
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryBooleanControl__Group_4__2__Impl_in_rule__DictionaryBooleanControl__Group_4__241562);
rule__DictionaryBooleanControl__Group_4__2__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryBooleanControl__Group_4__2"
// $ANTLR start "rule__DictionaryBooleanControl__Group_4__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20361:1: rule__DictionaryBooleanControl__Group_4__2__Impl : ( '}' ) ;
public final void rule__DictionaryBooleanControl__Group_4__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20365:1: ( ( '}' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20366:1: ( '}' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20366:1: ( '}' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20367:1: '}'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryBooleanControlAccess().getRightCurlyBracketKeyword_4_2());
}
match(input,68,FollowSets001.FOLLOW_68_in_rule__DictionaryBooleanControl__Group_4__2__Impl41590); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryBooleanControlAccess().getRightCurlyBracketKeyword_4_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryBooleanControl__Group_4__2__Impl"
// $ANTLR start "rule__DictionaryDateControl__Group__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20386:1: rule__DictionaryDateControl__Group__0 : rule__DictionaryDateControl__Group__0__Impl rule__DictionaryDateControl__Group__1 ;
public final void rule__DictionaryDateControl__Group__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20390:1: ( rule__DictionaryDateControl__Group__0__Impl rule__DictionaryDateControl__Group__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20391:2: rule__DictionaryDateControl__Group__0__Impl rule__DictionaryDateControl__Group__1
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryDateControl__Group__0__Impl_in_rule__DictionaryDateControl__Group__041627);
rule__DictionaryDateControl__Group__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryDateControl__Group__1_in_rule__DictionaryDateControl__Group__041630);
rule__DictionaryDateControl__Group__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryDateControl__Group__0"
// $ANTLR start "rule__DictionaryDateControl__Group__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20398:1: rule__DictionaryDateControl__Group__0__Impl : ( () ) ;
public final void rule__DictionaryDateControl__Group__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20402:1: ( ( () ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20403:1: ( () )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20403:1: ( () )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20404:1: ()
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryDateControlAccess().getDictionaryDateControlAction_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20405:1: ()
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20407:1:
{
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryDateControlAccess().getDictionaryDateControlAction_0());
}
}
}
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryDateControl__Group__0__Impl"
// $ANTLR start "rule__DictionaryDateControl__Group__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20417:1: rule__DictionaryDateControl__Group__1 : rule__DictionaryDateControl__Group__1__Impl rule__DictionaryDateControl__Group__2 ;
public final void rule__DictionaryDateControl__Group__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20421:1: ( rule__DictionaryDateControl__Group__1__Impl rule__DictionaryDateControl__Group__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20422:2: rule__DictionaryDateControl__Group__1__Impl rule__DictionaryDateControl__Group__2
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryDateControl__Group__1__Impl_in_rule__DictionaryDateControl__Group__141688);
rule__DictionaryDateControl__Group__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryDateControl__Group__2_in_rule__DictionaryDateControl__Group__141691);
rule__DictionaryDateControl__Group__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryDateControl__Group__1"
// $ANTLR start "rule__DictionaryDateControl__Group__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20429:1: rule__DictionaryDateControl__Group__1__Impl : ( 'datecontrol' ) ;
public final void rule__DictionaryDateControl__Group__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20433:1: ( ( 'datecontrol' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20434:1: ( 'datecontrol' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20434:1: ( 'datecontrol' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20435:1: 'datecontrol'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryDateControlAccess().getDatecontrolKeyword_1());
}
match(input,134,FollowSets001.FOLLOW_134_in_rule__DictionaryDateControl__Group__1__Impl41719); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryDateControlAccess().getDatecontrolKeyword_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryDateControl__Group__1__Impl"
// $ANTLR start "rule__DictionaryDateControl__Group__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20448:1: rule__DictionaryDateControl__Group__2 : rule__DictionaryDateControl__Group__2__Impl rule__DictionaryDateControl__Group__3 ;
public final void rule__DictionaryDateControl__Group__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20452:1: ( rule__DictionaryDateControl__Group__2__Impl rule__DictionaryDateControl__Group__3 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20453:2: rule__DictionaryDateControl__Group__2__Impl rule__DictionaryDateControl__Group__3
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryDateControl__Group__2__Impl_in_rule__DictionaryDateControl__Group__241750);
rule__DictionaryDateControl__Group__2__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryDateControl__Group__3_in_rule__DictionaryDateControl__Group__241753);
rule__DictionaryDateControl__Group__3();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryDateControl__Group__2"
// $ANTLR start "rule__DictionaryDateControl__Group__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20460:1: rule__DictionaryDateControl__Group__2__Impl : ( ( rule__DictionaryDateControl__NameAssignment_2 )? ) ;
public final void rule__DictionaryDateControl__Group__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20464:1: ( ( ( rule__DictionaryDateControl__NameAssignment_2 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20465:1: ( ( rule__DictionaryDateControl__NameAssignment_2 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20465:1: ( ( rule__DictionaryDateControl__NameAssignment_2 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20466:1: ( rule__DictionaryDateControl__NameAssignment_2 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryDateControlAccess().getNameAssignment_2());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20467:1: ( rule__DictionaryDateControl__NameAssignment_2 )?
int alt164=2;
int LA164_0 = input.LA(1);
if ( (LA164_0==RULE_ID) ) {
alt164=1;
}
switch (alt164) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20467:2: rule__DictionaryDateControl__NameAssignment_2
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryDateControl__NameAssignment_2_in_rule__DictionaryDateControl__Group__2__Impl41780);
rule__DictionaryDateControl__NameAssignment_2();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryDateControlAccess().getNameAssignment_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryDateControl__Group__2__Impl"
// $ANTLR start "rule__DictionaryDateControl__Group__3"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20477:1: rule__DictionaryDateControl__Group__3 : rule__DictionaryDateControl__Group__3__Impl rule__DictionaryDateControl__Group__4 ;
public final void rule__DictionaryDateControl__Group__3() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20481:1: ( rule__DictionaryDateControl__Group__3__Impl rule__DictionaryDateControl__Group__4 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20482:2: rule__DictionaryDateControl__Group__3__Impl rule__DictionaryDateControl__Group__4
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryDateControl__Group__3__Impl_in_rule__DictionaryDateControl__Group__341811);
rule__DictionaryDateControl__Group__3__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryDateControl__Group__4_in_rule__DictionaryDateControl__Group__341814);
rule__DictionaryDateControl__Group__4();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryDateControl__Group__3"
// $ANTLR start "rule__DictionaryDateControl__Group__3__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20489:1: rule__DictionaryDateControl__Group__3__Impl : ( ( rule__DictionaryDateControl__Group_3__0 )? ) ;
public final void rule__DictionaryDateControl__Group__3__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20493:1: ( ( ( rule__DictionaryDateControl__Group_3__0 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20494:1: ( ( rule__DictionaryDateControl__Group_3__0 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20494:1: ( ( rule__DictionaryDateControl__Group_3__0 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20495:1: ( rule__DictionaryDateControl__Group_3__0 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryDateControlAccess().getGroup_3());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20496:1: ( rule__DictionaryDateControl__Group_3__0 )?
int alt165=2;
int LA165_0 = input.LA(1);
if ( (LA165_0==126) ) {
alt165=1;
}
switch (alt165) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20496:2: rule__DictionaryDateControl__Group_3__0
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryDateControl__Group_3__0_in_rule__DictionaryDateControl__Group__3__Impl41841);
rule__DictionaryDateControl__Group_3__0();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryDateControlAccess().getGroup_3());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryDateControl__Group__3__Impl"
// $ANTLR start "rule__DictionaryDateControl__Group__4"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20506:1: rule__DictionaryDateControl__Group__4 : rule__DictionaryDateControl__Group__4__Impl ;
public final void rule__DictionaryDateControl__Group__4() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20510:1: ( rule__DictionaryDateControl__Group__4__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20511:2: rule__DictionaryDateControl__Group__4__Impl
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryDateControl__Group__4__Impl_in_rule__DictionaryDateControl__Group__441872);
rule__DictionaryDateControl__Group__4__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryDateControl__Group__4"
// $ANTLR start "rule__DictionaryDateControl__Group__4__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20517:1: rule__DictionaryDateControl__Group__4__Impl : ( ( rule__DictionaryDateControl__Group_4__0 )? ) ;
public final void rule__DictionaryDateControl__Group__4__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20521:1: ( ( ( rule__DictionaryDateControl__Group_4__0 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20522:1: ( ( rule__DictionaryDateControl__Group_4__0 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20522:1: ( ( rule__DictionaryDateControl__Group_4__0 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20523:1: ( rule__DictionaryDateControl__Group_4__0 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryDateControlAccess().getGroup_4());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20524:1: ( rule__DictionaryDateControl__Group_4__0 )?
int alt166=2;
int LA166_0 = input.LA(1);
if ( (LA166_0==67) ) {
alt166=1;
}
switch (alt166) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20524:2: rule__DictionaryDateControl__Group_4__0
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryDateControl__Group_4__0_in_rule__DictionaryDateControl__Group__4__Impl41899);
rule__DictionaryDateControl__Group_4__0();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryDateControlAccess().getGroup_4());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryDateControl__Group__4__Impl"
// $ANTLR start "rule__DictionaryDateControl__Group_3__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20544:1: rule__DictionaryDateControl__Group_3__0 : rule__DictionaryDateControl__Group_3__0__Impl rule__DictionaryDateControl__Group_3__1 ;
public final void rule__DictionaryDateControl__Group_3__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20548:1: ( rule__DictionaryDateControl__Group_3__0__Impl rule__DictionaryDateControl__Group_3__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20549:2: rule__DictionaryDateControl__Group_3__0__Impl rule__DictionaryDateControl__Group_3__1
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryDateControl__Group_3__0__Impl_in_rule__DictionaryDateControl__Group_3__041940);
rule__DictionaryDateControl__Group_3__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryDateControl__Group_3__1_in_rule__DictionaryDateControl__Group_3__041943);
rule__DictionaryDateControl__Group_3__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryDateControl__Group_3__0"
// $ANTLR start "rule__DictionaryDateControl__Group_3__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20556:1: rule__DictionaryDateControl__Group_3__0__Impl : ( 'ref' ) ;
public final void rule__DictionaryDateControl__Group_3__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20560:1: ( ( 'ref' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20561:1: ( 'ref' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20561:1: ( 'ref' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20562:1: 'ref'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryDateControlAccess().getRefKeyword_3_0());
}
match(input,126,FollowSets001.FOLLOW_126_in_rule__DictionaryDateControl__Group_3__0__Impl41971); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryDateControlAccess().getRefKeyword_3_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryDateControl__Group_3__0__Impl"
// $ANTLR start "rule__DictionaryDateControl__Group_3__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20575:1: rule__DictionaryDateControl__Group_3__1 : rule__DictionaryDateControl__Group_3__1__Impl ;
public final void rule__DictionaryDateControl__Group_3__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20579:1: ( rule__DictionaryDateControl__Group_3__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20580:2: rule__DictionaryDateControl__Group_3__1__Impl
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryDateControl__Group_3__1__Impl_in_rule__DictionaryDateControl__Group_3__142002);
rule__DictionaryDateControl__Group_3__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryDateControl__Group_3__1"
// $ANTLR start "rule__DictionaryDateControl__Group_3__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20586:1: rule__DictionaryDateControl__Group_3__1__Impl : ( ( rule__DictionaryDateControl__RefAssignment_3_1 ) ) ;
public final void rule__DictionaryDateControl__Group_3__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20590:1: ( ( ( rule__DictionaryDateControl__RefAssignment_3_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20591:1: ( ( rule__DictionaryDateControl__RefAssignment_3_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20591:1: ( ( rule__DictionaryDateControl__RefAssignment_3_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20592:1: ( rule__DictionaryDateControl__RefAssignment_3_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryDateControlAccess().getRefAssignment_3_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20593:1: ( rule__DictionaryDateControl__RefAssignment_3_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20593:2: rule__DictionaryDateControl__RefAssignment_3_1
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryDateControl__RefAssignment_3_1_in_rule__DictionaryDateControl__Group_3__1__Impl42029);
rule__DictionaryDateControl__RefAssignment_3_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryDateControlAccess().getRefAssignment_3_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryDateControl__Group_3__1__Impl"
// $ANTLR start "rule__DictionaryDateControl__Group_4__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20607:1: rule__DictionaryDateControl__Group_4__0 : rule__DictionaryDateControl__Group_4__0__Impl rule__DictionaryDateControl__Group_4__1 ;
public final void rule__DictionaryDateControl__Group_4__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20611:1: ( rule__DictionaryDateControl__Group_4__0__Impl rule__DictionaryDateControl__Group_4__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20612:2: rule__DictionaryDateControl__Group_4__0__Impl rule__DictionaryDateControl__Group_4__1
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryDateControl__Group_4__0__Impl_in_rule__DictionaryDateControl__Group_4__042063);
rule__DictionaryDateControl__Group_4__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryDateControl__Group_4__1_in_rule__DictionaryDateControl__Group_4__042066);
rule__DictionaryDateControl__Group_4__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryDateControl__Group_4__0"
// $ANTLR start "rule__DictionaryDateControl__Group_4__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20619:1: rule__DictionaryDateControl__Group_4__0__Impl : ( '{' ) ;
public final void rule__DictionaryDateControl__Group_4__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20623:1: ( ( '{' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20624:1: ( '{' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20624:1: ( '{' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20625:1: '{'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryDateControlAccess().getLeftCurlyBracketKeyword_4_0());
}
match(input,67,FollowSets001.FOLLOW_67_in_rule__DictionaryDateControl__Group_4__0__Impl42094); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryDateControlAccess().getLeftCurlyBracketKeyword_4_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryDateControl__Group_4__0__Impl"
// $ANTLR start "rule__DictionaryDateControl__Group_4__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20638:1: rule__DictionaryDateControl__Group_4__1 : rule__DictionaryDateControl__Group_4__1__Impl rule__DictionaryDateControl__Group_4__2 ;
public final void rule__DictionaryDateControl__Group_4__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20642:1: ( rule__DictionaryDateControl__Group_4__1__Impl rule__DictionaryDateControl__Group_4__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20643:2: rule__DictionaryDateControl__Group_4__1__Impl rule__DictionaryDateControl__Group_4__2
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryDateControl__Group_4__1__Impl_in_rule__DictionaryDateControl__Group_4__142125);
rule__DictionaryDateControl__Group_4__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryDateControl__Group_4__2_in_rule__DictionaryDateControl__Group_4__142128);
rule__DictionaryDateControl__Group_4__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryDateControl__Group_4__1"
// $ANTLR start "rule__DictionaryDateControl__Group_4__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20650:1: rule__DictionaryDateControl__Group_4__1__Impl : ( ( rule__DictionaryDateControl__BaseControlAssignment_4_1 ) ) ;
public final void rule__DictionaryDateControl__Group_4__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20654:1: ( ( ( rule__DictionaryDateControl__BaseControlAssignment_4_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20655:1: ( ( rule__DictionaryDateControl__BaseControlAssignment_4_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20655:1: ( ( rule__DictionaryDateControl__BaseControlAssignment_4_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20656:1: ( rule__DictionaryDateControl__BaseControlAssignment_4_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryDateControlAccess().getBaseControlAssignment_4_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20657:1: ( rule__DictionaryDateControl__BaseControlAssignment_4_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20657:2: rule__DictionaryDateControl__BaseControlAssignment_4_1
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryDateControl__BaseControlAssignment_4_1_in_rule__DictionaryDateControl__Group_4__1__Impl42155);
rule__DictionaryDateControl__BaseControlAssignment_4_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryDateControlAccess().getBaseControlAssignment_4_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryDateControl__Group_4__1__Impl"
// $ANTLR start "rule__DictionaryDateControl__Group_4__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20667:1: rule__DictionaryDateControl__Group_4__2 : rule__DictionaryDateControl__Group_4__2__Impl ;
public final void rule__DictionaryDateControl__Group_4__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20671:1: ( rule__DictionaryDateControl__Group_4__2__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20672:2: rule__DictionaryDateControl__Group_4__2__Impl
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryDateControl__Group_4__2__Impl_in_rule__DictionaryDateControl__Group_4__242185);
rule__DictionaryDateControl__Group_4__2__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryDateControl__Group_4__2"
// $ANTLR start "rule__DictionaryDateControl__Group_4__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20678:1: rule__DictionaryDateControl__Group_4__2__Impl : ( '}' ) ;
public final void rule__DictionaryDateControl__Group_4__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20682:1: ( ( '}' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20683:1: ( '}' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20683:1: ( '}' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20684:1: '}'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryDateControlAccess().getRightCurlyBracketKeyword_4_2());
}
match(input,68,FollowSets001.FOLLOW_68_in_rule__DictionaryDateControl__Group_4__2__Impl42213); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryDateControlAccess().getRightCurlyBracketKeyword_4_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryDateControl__Group_4__2__Impl"
// $ANTLR start "rule__DictionaryEnumerationControl__Group__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20703:1: rule__DictionaryEnumerationControl__Group__0 : rule__DictionaryEnumerationControl__Group__0__Impl rule__DictionaryEnumerationControl__Group__1 ;
public final void rule__DictionaryEnumerationControl__Group__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20707:1: ( rule__DictionaryEnumerationControl__Group__0__Impl rule__DictionaryEnumerationControl__Group__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20708:2: rule__DictionaryEnumerationControl__Group__0__Impl rule__DictionaryEnumerationControl__Group__1
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryEnumerationControl__Group__0__Impl_in_rule__DictionaryEnumerationControl__Group__042250);
rule__DictionaryEnumerationControl__Group__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryEnumerationControl__Group__1_in_rule__DictionaryEnumerationControl__Group__042253);
rule__DictionaryEnumerationControl__Group__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryEnumerationControl__Group__0"
// $ANTLR start "rule__DictionaryEnumerationControl__Group__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20715:1: rule__DictionaryEnumerationControl__Group__0__Impl : ( () ) ;
public final void rule__DictionaryEnumerationControl__Group__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20719:1: ( ( () ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20720:1: ( () )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20720:1: ( () )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20721:1: ()
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryEnumerationControlAccess().getDictionaryEnumerationControlAction_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20722:1: ()
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20724:1:
{
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryEnumerationControlAccess().getDictionaryEnumerationControlAction_0());
}
}
}
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryEnumerationControl__Group__0__Impl"
// $ANTLR start "rule__DictionaryEnumerationControl__Group__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20734:1: rule__DictionaryEnumerationControl__Group__1 : rule__DictionaryEnumerationControl__Group__1__Impl rule__DictionaryEnumerationControl__Group__2 ;
public final void rule__DictionaryEnumerationControl__Group__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20738:1: ( rule__DictionaryEnumerationControl__Group__1__Impl rule__DictionaryEnumerationControl__Group__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20739:2: rule__DictionaryEnumerationControl__Group__1__Impl rule__DictionaryEnumerationControl__Group__2
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryEnumerationControl__Group__1__Impl_in_rule__DictionaryEnumerationControl__Group__142311);
rule__DictionaryEnumerationControl__Group__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryEnumerationControl__Group__2_in_rule__DictionaryEnumerationControl__Group__142314);
rule__DictionaryEnumerationControl__Group__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryEnumerationControl__Group__1"
// $ANTLR start "rule__DictionaryEnumerationControl__Group__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20746:1: rule__DictionaryEnumerationControl__Group__1__Impl : ( 'enumerationcontrol' ) ;
public final void rule__DictionaryEnumerationControl__Group__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20750:1: ( ( 'enumerationcontrol' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20751:1: ( 'enumerationcontrol' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20751:1: ( 'enumerationcontrol' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20752:1: 'enumerationcontrol'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryEnumerationControlAccess().getEnumerationcontrolKeyword_1());
}
match(input,135,FollowSets001.FOLLOW_135_in_rule__DictionaryEnumerationControl__Group__1__Impl42342); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryEnumerationControlAccess().getEnumerationcontrolKeyword_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryEnumerationControl__Group__1__Impl"
// $ANTLR start "rule__DictionaryEnumerationControl__Group__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20765:1: rule__DictionaryEnumerationControl__Group__2 : rule__DictionaryEnumerationControl__Group__2__Impl rule__DictionaryEnumerationControl__Group__3 ;
public final void rule__DictionaryEnumerationControl__Group__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20769:1: ( rule__DictionaryEnumerationControl__Group__2__Impl rule__DictionaryEnumerationControl__Group__3 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20770:2: rule__DictionaryEnumerationControl__Group__2__Impl rule__DictionaryEnumerationControl__Group__3
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryEnumerationControl__Group__2__Impl_in_rule__DictionaryEnumerationControl__Group__242373);
rule__DictionaryEnumerationControl__Group__2__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryEnumerationControl__Group__3_in_rule__DictionaryEnumerationControl__Group__242376);
rule__DictionaryEnumerationControl__Group__3();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryEnumerationControl__Group__2"
// $ANTLR start "rule__DictionaryEnumerationControl__Group__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20777:1: rule__DictionaryEnumerationControl__Group__2__Impl : ( ( rule__DictionaryEnumerationControl__NameAssignment_2 )? ) ;
public final void rule__DictionaryEnumerationControl__Group__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20781:1: ( ( ( rule__DictionaryEnumerationControl__NameAssignment_2 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20782:1: ( ( rule__DictionaryEnumerationControl__NameAssignment_2 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20782:1: ( ( rule__DictionaryEnumerationControl__NameAssignment_2 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20783:1: ( rule__DictionaryEnumerationControl__NameAssignment_2 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryEnumerationControlAccess().getNameAssignment_2());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20784:1: ( rule__DictionaryEnumerationControl__NameAssignment_2 )?
int alt167=2;
int LA167_0 = input.LA(1);
if ( (LA167_0==RULE_ID) ) {
alt167=1;
}
switch (alt167) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20784:2: rule__DictionaryEnumerationControl__NameAssignment_2
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryEnumerationControl__NameAssignment_2_in_rule__DictionaryEnumerationControl__Group__2__Impl42403);
rule__DictionaryEnumerationControl__NameAssignment_2();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryEnumerationControlAccess().getNameAssignment_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryEnumerationControl__Group__2__Impl"
// $ANTLR start "rule__DictionaryEnumerationControl__Group__3"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20794:1: rule__DictionaryEnumerationControl__Group__3 : rule__DictionaryEnumerationControl__Group__3__Impl rule__DictionaryEnumerationControl__Group__4 ;
public final void rule__DictionaryEnumerationControl__Group__3() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20798:1: ( rule__DictionaryEnumerationControl__Group__3__Impl rule__DictionaryEnumerationControl__Group__4 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20799:2: rule__DictionaryEnumerationControl__Group__3__Impl rule__DictionaryEnumerationControl__Group__4
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryEnumerationControl__Group__3__Impl_in_rule__DictionaryEnumerationControl__Group__342434);
rule__DictionaryEnumerationControl__Group__3__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryEnumerationControl__Group__4_in_rule__DictionaryEnumerationControl__Group__342437);
rule__DictionaryEnumerationControl__Group__4();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryEnumerationControl__Group__3"
// $ANTLR start "rule__DictionaryEnumerationControl__Group__3__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20806:1: rule__DictionaryEnumerationControl__Group__3__Impl : ( ( rule__DictionaryEnumerationControl__Group_3__0 )? ) ;
public final void rule__DictionaryEnumerationControl__Group__3__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20810:1: ( ( ( rule__DictionaryEnumerationControl__Group_3__0 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20811:1: ( ( rule__DictionaryEnumerationControl__Group_3__0 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20811:1: ( ( rule__DictionaryEnumerationControl__Group_3__0 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20812:1: ( rule__DictionaryEnumerationControl__Group_3__0 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryEnumerationControlAccess().getGroup_3());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20813:1: ( rule__DictionaryEnumerationControl__Group_3__0 )?
int alt168=2;
int LA168_0 = input.LA(1);
if ( (LA168_0==126) ) {
alt168=1;
}
switch (alt168) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20813:2: rule__DictionaryEnumerationControl__Group_3__0
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryEnumerationControl__Group_3__0_in_rule__DictionaryEnumerationControl__Group__3__Impl42464);
rule__DictionaryEnumerationControl__Group_3__0();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryEnumerationControlAccess().getGroup_3());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryEnumerationControl__Group__3__Impl"
// $ANTLR start "rule__DictionaryEnumerationControl__Group__4"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20823:1: rule__DictionaryEnumerationControl__Group__4 : rule__DictionaryEnumerationControl__Group__4__Impl ;
public final void rule__DictionaryEnumerationControl__Group__4() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20827:1: ( rule__DictionaryEnumerationControl__Group__4__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20828:2: rule__DictionaryEnumerationControl__Group__4__Impl
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryEnumerationControl__Group__4__Impl_in_rule__DictionaryEnumerationControl__Group__442495);
rule__DictionaryEnumerationControl__Group__4__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryEnumerationControl__Group__4"
// $ANTLR start "rule__DictionaryEnumerationControl__Group__4__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20834:1: rule__DictionaryEnumerationControl__Group__4__Impl : ( ( rule__DictionaryEnumerationControl__Group_4__0 )? ) ;
public final void rule__DictionaryEnumerationControl__Group__4__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20838:1: ( ( ( rule__DictionaryEnumerationControl__Group_4__0 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20839:1: ( ( rule__DictionaryEnumerationControl__Group_4__0 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20839:1: ( ( rule__DictionaryEnumerationControl__Group_4__0 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20840:1: ( rule__DictionaryEnumerationControl__Group_4__0 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryEnumerationControlAccess().getGroup_4());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20841:1: ( rule__DictionaryEnumerationControl__Group_4__0 )?
int alt169=2;
int LA169_0 = input.LA(1);
if ( (LA169_0==67) ) {
alt169=1;
}
switch (alt169) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20841:2: rule__DictionaryEnumerationControl__Group_4__0
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryEnumerationControl__Group_4__0_in_rule__DictionaryEnumerationControl__Group__4__Impl42522);
rule__DictionaryEnumerationControl__Group_4__0();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryEnumerationControlAccess().getGroup_4());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryEnumerationControl__Group__4__Impl"
// $ANTLR start "rule__DictionaryEnumerationControl__Group_3__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20861:1: rule__DictionaryEnumerationControl__Group_3__0 : rule__DictionaryEnumerationControl__Group_3__0__Impl rule__DictionaryEnumerationControl__Group_3__1 ;
public final void rule__DictionaryEnumerationControl__Group_3__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20865:1: ( rule__DictionaryEnumerationControl__Group_3__0__Impl rule__DictionaryEnumerationControl__Group_3__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20866:2: rule__DictionaryEnumerationControl__Group_3__0__Impl rule__DictionaryEnumerationControl__Group_3__1
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryEnumerationControl__Group_3__0__Impl_in_rule__DictionaryEnumerationControl__Group_3__042563);
rule__DictionaryEnumerationControl__Group_3__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryEnumerationControl__Group_3__1_in_rule__DictionaryEnumerationControl__Group_3__042566);
rule__DictionaryEnumerationControl__Group_3__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryEnumerationControl__Group_3__0"
// $ANTLR start "rule__DictionaryEnumerationControl__Group_3__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20873:1: rule__DictionaryEnumerationControl__Group_3__0__Impl : ( 'ref' ) ;
public final void rule__DictionaryEnumerationControl__Group_3__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20877:1: ( ( 'ref' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20878:1: ( 'ref' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20878:1: ( 'ref' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20879:1: 'ref'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryEnumerationControlAccess().getRefKeyword_3_0());
}
match(input,126,FollowSets001.FOLLOW_126_in_rule__DictionaryEnumerationControl__Group_3__0__Impl42594); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryEnumerationControlAccess().getRefKeyword_3_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryEnumerationControl__Group_3__0__Impl"
// $ANTLR start "rule__DictionaryEnumerationControl__Group_3__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20892:1: rule__DictionaryEnumerationControl__Group_3__1 : rule__DictionaryEnumerationControl__Group_3__1__Impl ;
public final void rule__DictionaryEnumerationControl__Group_3__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20896:1: ( rule__DictionaryEnumerationControl__Group_3__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20897:2: rule__DictionaryEnumerationControl__Group_3__1__Impl
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryEnumerationControl__Group_3__1__Impl_in_rule__DictionaryEnumerationControl__Group_3__142625);
rule__DictionaryEnumerationControl__Group_3__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryEnumerationControl__Group_3__1"
// $ANTLR start "rule__DictionaryEnumerationControl__Group_3__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20903:1: rule__DictionaryEnumerationControl__Group_3__1__Impl : ( ( rule__DictionaryEnumerationControl__RefAssignment_3_1 ) ) ;
public final void rule__DictionaryEnumerationControl__Group_3__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20907:1: ( ( ( rule__DictionaryEnumerationControl__RefAssignment_3_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20908:1: ( ( rule__DictionaryEnumerationControl__RefAssignment_3_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20908:1: ( ( rule__DictionaryEnumerationControl__RefAssignment_3_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20909:1: ( rule__DictionaryEnumerationControl__RefAssignment_3_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryEnumerationControlAccess().getRefAssignment_3_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20910:1: ( rule__DictionaryEnumerationControl__RefAssignment_3_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20910:2: rule__DictionaryEnumerationControl__RefAssignment_3_1
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryEnumerationControl__RefAssignment_3_1_in_rule__DictionaryEnumerationControl__Group_3__1__Impl42652);
rule__DictionaryEnumerationControl__RefAssignment_3_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryEnumerationControlAccess().getRefAssignment_3_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryEnumerationControl__Group_3__1__Impl"
// $ANTLR start "rule__DictionaryEnumerationControl__Group_4__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20924:1: rule__DictionaryEnumerationControl__Group_4__0 : rule__DictionaryEnumerationControl__Group_4__0__Impl rule__DictionaryEnumerationControl__Group_4__1 ;
public final void rule__DictionaryEnumerationControl__Group_4__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20928:1: ( rule__DictionaryEnumerationControl__Group_4__0__Impl rule__DictionaryEnumerationControl__Group_4__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20929:2: rule__DictionaryEnumerationControl__Group_4__0__Impl rule__DictionaryEnumerationControl__Group_4__1
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryEnumerationControl__Group_4__0__Impl_in_rule__DictionaryEnumerationControl__Group_4__042686);
rule__DictionaryEnumerationControl__Group_4__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryEnumerationControl__Group_4__1_in_rule__DictionaryEnumerationControl__Group_4__042689);
rule__DictionaryEnumerationControl__Group_4__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryEnumerationControl__Group_4__0"
// $ANTLR start "rule__DictionaryEnumerationControl__Group_4__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20936:1: rule__DictionaryEnumerationControl__Group_4__0__Impl : ( '{' ) ;
public final void rule__DictionaryEnumerationControl__Group_4__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20940:1: ( ( '{' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20941:1: ( '{' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20941:1: ( '{' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20942:1: '{'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryEnumerationControlAccess().getLeftCurlyBracketKeyword_4_0());
}
match(input,67,FollowSets001.FOLLOW_67_in_rule__DictionaryEnumerationControl__Group_4__0__Impl42717); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryEnumerationControlAccess().getLeftCurlyBracketKeyword_4_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryEnumerationControl__Group_4__0__Impl"
// $ANTLR start "rule__DictionaryEnumerationControl__Group_4__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20955:1: rule__DictionaryEnumerationControl__Group_4__1 : rule__DictionaryEnumerationControl__Group_4__1__Impl rule__DictionaryEnumerationControl__Group_4__2 ;
public final void rule__DictionaryEnumerationControl__Group_4__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20959:1: ( rule__DictionaryEnumerationControl__Group_4__1__Impl rule__DictionaryEnumerationControl__Group_4__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20960:2: rule__DictionaryEnumerationControl__Group_4__1__Impl rule__DictionaryEnumerationControl__Group_4__2
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryEnumerationControl__Group_4__1__Impl_in_rule__DictionaryEnumerationControl__Group_4__142748);
rule__DictionaryEnumerationControl__Group_4__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryEnumerationControl__Group_4__2_in_rule__DictionaryEnumerationControl__Group_4__142751);
rule__DictionaryEnumerationControl__Group_4__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryEnumerationControl__Group_4__1"
// $ANTLR start "rule__DictionaryEnumerationControl__Group_4__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20967:1: rule__DictionaryEnumerationControl__Group_4__1__Impl : ( ( rule__DictionaryEnumerationControl__BaseControlAssignment_4_1 ) ) ;
public final void rule__DictionaryEnumerationControl__Group_4__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20971:1: ( ( ( rule__DictionaryEnumerationControl__BaseControlAssignment_4_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20972:1: ( ( rule__DictionaryEnumerationControl__BaseControlAssignment_4_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20972:1: ( ( rule__DictionaryEnumerationControl__BaseControlAssignment_4_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20973:1: ( rule__DictionaryEnumerationControl__BaseControlAssignment_4_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryEnumerationControlAccess().getBaseControlAssignment_4_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20974:1: ( rule__DictionaryEnumerationControl__BaseControlAssignment_4_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20974:2: rule__DictionaryEnumerationControl__BaseControlAssignment_4_1
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryEnumerationControl__BaseControlAssignment_4_1_in_rule__DictionaryEnumerationControl__Group_4__1__Impl42778);
rule__DictionaryEnumerationControl__BaseControlAssignment_4_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryEnumerationControlAccess().getBaseControlAssignment_4_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryEnumerationControl__Group_4__1__Impl"
// $ANTLR start "rule__DictionaryEnumerationControl__Group_4__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20984:1: rule__DictionaryEnumerationControl__Group_4__2 : rule__DictionaryEnumerationControl__Group_4__2__Impl ;
public final void rule__DictionaryEnumerationControl__Group_4__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20988:1: ( rule__DictionaryEnumerationControl__Group_4__2__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20989:2: rule__DictionaryEnumerationControl__Group_4__2__Impl
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryEnumerationControl__Group_4__2__Impl_in_rule__DictionaryEnumerationControl__Group_4__242808);
rule__DictionaryEnumerationControl__Group_4__2__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryEnumerationControl__Group_4__2"
// $ANTLR start "rule__DictionaryEnumerationControl__Group_4__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20995:1: rule__DictionaryEnumerationControl__Group_4__2__Impl : ( '}' ) ;
public final void rule__DictionaryEnumerationControl__Group_4__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:20999:1: ( ( '}' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21000:1: ( '}' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21000:1: ( '}' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21001:1: '}'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryEnumerationControlAccess().getRightCurlyBracketKeyword_4_2());
}
match(input,68,FollowSets001.FOLLOW_68_in_rule__DictionaryEnumerationControl__Group_4__2__Impl42836); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryEnumerationControlAccess().getRightCurlyBracketKeyword_4_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryEnumerationControl__Group_4__2__Impl"
// $ANTLR start "rule__DictionaryReferenceControl__Group__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21020:1: rule__DictionaryReferenceControl__Group__0 : rule__DictionaryReferenceControl__Group__0__Impl rule__DictionaryReferenceControl__Group__1 ;
public final void rule__DictionaryReferenceControl__Group__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21024:1: ( rule__DictionaryReferenceControl__Group__0__Impl rule__DictionaryReferenceControl__Group__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21025:2: rule__DictionaryReferenceControl__Group__0__Impl rule__DictionaryReferenceControl__Group__1
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryReferenceControl__Group__0__Impl_in_rule__DictionaryReferenceControl__Group__042873);
rule__DictionaryReferenceControl__Group__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryReferenceControl__Group__1_in_rule__DictionaryReferenceControl__Group__042876);
rule__DictionaryReferenceControl__Group__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryReferenceControl__Group__0"
// $ANTLR start "rule__DictionaryReferenceControl__Group__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21032:1: rule__DictionaryReferenceControl__Group__0__Impl : ( () ) ;
public final void rule__DictionaryReferenceControl__Group__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21036:1: ( ( () ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21037:1: ( () )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21037:1: ( () )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21038:1: ()
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryReferenceControlAccess().getDictionaryReferenceControlAction_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21039:1: ()
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21041:1:
{
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryReferenceControlAccess().getDictionaryReferenceControlAction_0());
}
}
}
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryReferenceControl__Group__0__Impl"
// $ANTLR start "rule__DictionaryReferenceControl__Group__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21051:1: rule__DictionaryReferenceControl__Group__1 : rule__DictionaryReferenceControl__Group__1__Impl rule__DictionaryReferenceControl__Group__2 ;
public final void rule__DictionaryReferenceControl__Group__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21055:1: ( rule__DictionaryReferenceControl__Group__1__Impl rule__DictionaryReferenceControl__Group__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21056:2: rule__DictionaryReferenceControl__Group__1__Impl rule__DictionaryReferenceControl__Group__2
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryReferenceControl__Group__1__Impl_in_rule__DictionaryReferenceControl__Group__142934);
rule__DictionaryReferenceControl__Group__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryReferenceControl__Group__2_in_rule__DictionaryReferenceControl__Group__142937);
rule__DictionaryReferenceControl__Group__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryReferenceControl__Group__1"
// $ANTLR start "rule__DictionaryReferenceControl__Group__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21063:1: rule__DictionaryReferenceControl__Group__1__Impl : ( 'referencecontrol' ) ;
public final void rule__DictionaryReferenceControl__Group__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21067:1: ( ( 'referencecontrol' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21068:1: ( 'referencecontrol' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21068:1: ( 'referencecontrol' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21069:1: 'referencecontrol'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryReferenceControlAccess().getReferencecontrolKeyword_1());
}
match(input,136,FollowSets001.FOLLOW_136_in_rule__DictionaryReferenceControl__Group__1__Impl42965); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryReferenceControlAccess().getReferencecontrolKeyword_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryReferenceControl__Group__1__Impl"
// $ANTLR start "rule__DictionaryReferenceControl__Group__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21082:1: rule__DictionaryReferenceControl__Group__2 : rule__DictionaryReferenceControl__Group__2__Impl rule__DictionaryReferenceControl__Group__3 ;
public final void rule__DictionaryReferenceControl__Group__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21086:1: ( rule__DictionaryReferenceControl__Group__2__Impl rule__DictionaryReferenceControl__Group__3 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21087:2: rule__DictionaryReferenceControl__Group__2__Impl rule__DictionaryReferenceControl__Group__3
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryReferenceControl__Group__2__Impl_in_rule__DictionaryReferenceControl__Group__242996);
rule__DictionaryReferenceControl__Group__2__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryReferenceControl__Group__3_in_rule__DictionaryReferenceControl__Group__242999);
rule__DictionaryReferenceControl__Group__3();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryReferenceControl__Group__2"
// $ANTLR start "rule__DictionaryReferenceControl__Group__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21094:1: rule__DictionaryReferenceControl__Group__2__Impl : ( ( rule__DictionaryReferenceControl__NameAssignment_2 )? ) ;
public final void rule__DictionaryReferenceControl__Group__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21098:1: ( ( ( rule__DictionaryReferenceControl__NameAssignment_2 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21099:1: ( ( rule__DictionaryReferenceControl__NameAssignment_2 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21099:1: ( ( rule__DictionaryReferenceControl__NameAssignment_2 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21100:1: ( rule__DictionaryReferenceControl__NameAssignment_2 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryReferenceControlAccess().getNameAssignment_2());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21101:1: ( rule__DictionaryReferenceControl__NameAssignment_2 )?
int alt170=2;
int LA170_0 = input.LA(1);
if ( (LA170_0==RULE_ID) ) {
alt170=1;
}
switch (alt170) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21101:2: rule__DictionaryReferenceControl__NameAssignment_2
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryReferenceControl__NameAssignment_2_in_rule__DictionaryReferenceControl__Group__2__Impl43026);
rule__DictionaryReferenceControl__NameAssignment_2();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryReferenceControlAccess().getNameAssignment_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryReferenceControl__Group__2__Impl"
// $ANTLR start "rule__DictionaryReferenceControl__Group__3"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21111:1: rule__DictionaryReferenceControl__Group__3 : rule__DictionaryReferenceControl__Group__3__Impl rule__DictionaryReferenceControl__Group__4 ;
public final void rule__DictionaryReferenceControl__Group__3() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21115:1: ( rule__DictionaryReferenceControl__Group__3__Impl rule__DictionaryReferenceControl__Group__4 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21116:2: rule__DictionaryReferenceControl__Group__3__Impl rule__DictionaryReferenceControl__Group__4
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryReferenceControl__Group__3__Impl_in_rule__DictionaryReferenceControl__Group__343057);
rule__DictionaryReferenceControl__Group__3__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryReferenceControl__Group__4_in_rule__DictionaryReferenceControl__Group__343060);
rule__DictionaryReferenceControl__Group__4();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryReferenceControl__Group__3"
// $ANTLR start "rule__DictionaryReferenceControl__Group__3__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21123:1: rule__DictionaryReferenceControl__Group__3__Impl : ( ( rule__DictionaryReferenceControl__Group_3__0 )? ) ;
public final void rule__DictionaryReferenceControl__Group__3__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21127:1: ( ( ( rule__DictionaryReferenceControl__Group_3__0 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21128:1: ( ( rule__DictionaryReferenceControl__Group_3__0 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21128:1: ( ( rule__DictionaryReferenceControl__Group_3__0 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21129:1: ( rule__DictionaryReferenceControl__Group_3__0 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryReferenceControlAccess().getGroup_3());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21130:1: ( rule__DictionaryReferenceControl__Group_3__0 )?
int alt171=2;
int LA171_0 = input.LA(1);
if ( (LA171_0==126) ) {
alt171=1;
}
switch (alt171) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21130:2: rule__DictionaryReferenceControl__Group_3__0
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryReferenceControl__Group_3__0_in_rule__DictionaryReferenceControl__Group__3__Impl43087);
rule__DictionaryReferenceControl__Group_3__0();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryReferenceControlAccess().getGroup_3());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryReferenceControl__Group__3__Impl"
// $ANTLR start "rule__DictionaryReferenceControl__Group__4"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21140:1: rule__DictionaryReferenceControl__Group__4 : rule__DictionaryReferenceControl__Group__4__Impl ;
public final void rule__DictionaryReferenceControl__Group__4() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21144:1: ( rule__DictionaryReferenceControl__Group__4__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21145:2: rule__DictionaryReferenceControl__Group__4__Impl
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryReferenceControl__Group__4__Impl_in_rule__DictionaryReferenceControl__Group__443118);
rule__DictionaryReferenceControl__Group__4__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryReferenceControl__Group__4"
// $ANTLR start "rule__DictionaryReferenceControl__Group__4__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21151:1: rule__DictionaryReferenceControl__Group__4__Impl : ( ( rule__DictionaryReferenceControl__Group_4__0 )? ) ;
public final void rule__DictionaryReferenceControl__Group__4__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21155:1: ( ( ( rule__DictionaryReferenceControl__Group_4__0 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21156:1: ( ( rule__DictionaryReferenceControl__Group_4__0 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21156:1: ( ( rule__DictionaryReferenceControl__Group_4__0 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21157:1: ( rule__DictionaryReferenceControl__Group_4__0 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryReferenceControlAccess().getGroup_4());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21158:1: ( rule__DictionaryReferenceControl__Group_4__0 )?
int alt172=2;
int LA172_0 = input.LA(1);
if ( (LA172_0==67) ) {
alt172=1;
}
switch (alt172) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21158:2: rule__DictionaryReferenceControl__Group_4__0
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryReferenceControl__Group_4__0_in_rule__DictionaryReferenceControl__Group__4__Impl43145);
rule__DictionaryReferenceControl__Group_4__0();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryReferenceControlAccess().getGroup_4());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryReferenceControl__Group__4__Impl"
// $ANTLR start "rule__DictionaryReferenceControl__Group_3__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21178:1: rule__DictionaryReferenceControl__Group_3__0 : rule__DictionaryReferenceControl__Group_3__0__Impl rule__DictionaryReferenceControl__Group_3__1 ;
public final void rule__DictionaryReferenceControl__Group_3__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21182:1: ( rule__DictionaryReferenceControl__Group_3__0__Impl rule__DictionaryReferenceControl__Group_3__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21183:2: rule__DictionaryReferenceControl__Group_3__0__Impl rule__DictionaryReferenceControl__Group_3__1
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryReferenceControl__Group_3__0__Impl_in_rule__DictionaryReferenceControl__Group_3__043186);
rule__DictionaryReferenceControl__Group_3__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryReferenceControl__Group_3__1_in_rule__DictionaryReferenceControl__Group_3__043189);
rule__DictionaryReferenceControl__Group_3__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryReferenceControl__Group_3__0"
// $ANTLR start "rule__DictionaryReferenceControl__Group_3__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21190:1: rule__DictionaryReferenceControl__Group_3__0__Impl : ( 'ref' ) ;
public final void rule__DictionaryReferenceControl__Group_3__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21194:1: ( ( 'ref' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21195:1: ( 'ref' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21195:1: ( 'ref' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21196:1: 'ref'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryReferenceControlAccess().getRefKeyword_3_0());
}
match(input,126,FollowSets001.FOLLOW_126_in_rule__DictionaryReferenceControl__Group_3__0__Impl43217); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryReferenceControlAccess().getRefKeyword_3_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryReferenceControl__Group_3__0__Impl"
// $ANTLR start "rule__DictionaryReferenceControl__Group_3__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21209:1: rule__DictionaryReferenceControl__Group_3__1 : rule__DictionaryReferenceControl__Group_3__1__Impl ;
public final void rule__DictionaryReferenceControl__Group_3__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21213:1: ( rule__DictionaryReferenceControl__Group_3__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21214:2: rule__DictionaryReferenceControl__Group_3__1__Impl
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryReferenceControl__Group_3__1__Impl_in_rule__DictionaryReferenceControl__Group_3__143248);
rule__DictionaryReferenceControl__Group_3__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryReferenceControl__Group_3__1"
// $ANTLR start "rule__DictionaryReferenceControl__Group_3__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21220:1: rule__DictionaryReferenceControl__Group_3__1__Impl : ( ( rule__DictionaryReferenceControl__RefAssignment_3_1 ) ) ;
public final void rule__DictionaryReferenceControl__Group_3__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21224:1: ( ( ( rule__DictionaryReferenceControl__RefAssignment_3_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21225:1: ( ( rule__DictionaryReferenceControl__RefAssignment_3_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21225:1: ( ( rule__DictionaryReferenceControl__RefAssignment_3_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21226:1: ( rule__DictionaryReferenceControl__RefAssignment_3_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryReferenceControlAccess().getRefAssignment_3_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21227:1: ( rule__DictionaryReferenceControl__RefAssignment_3_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21227:2: rule__DictionaryReferenceControl__RefAssignment_3_1
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryReferenceControl__RefAssignment_3_1_in_rule__DictionaryReferenceControl__Group_3__1__Impl43275);
rule__DictionaryReferenceControl__RefAssignment_3_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryReferenceControlAccess().getRefAssignment_3_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryReferenceControl__Group_3__1__Impl"
// $ANTLR start "rule__DictionaryReferenceControl__Group_4__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21241:1: rule__DictionaryReferenceControl__Group_4__0 : rule__DictionaryReferenceControl__Group_4__0__Impl rule__DictionaryReferenceControl__Group_4__1 ;
public final void rule__DictionaryReferenceControl__Group_4__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21245:1: ( rule__DictionaryReferenceControl__Group_4__0__Impl rule__DictionaryReferenceControl__Group_4__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21246:2: rule__DictionaryReferenceControl__Group_4__0__Impl rule__DictionaryReferenceControl__Group_4__1
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryReferenceControl__Group_4__0__Impl_in_rule__DictionaryReferenceControl__Group_4__043309);
rule__DictionaryReferenceControl__Group_4__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryReferenceControl__Group_4__1_in_rule__DictionaryReferenceControl__Group_4__043312);
rule__DictionaryReferenceControl__Group_4__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryReferenceControl__Group_4__0"
// $ANTLR start "rule__DictionaryReferenceControl__Group_4__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21253:1: rule__DictionaryReferenceControl__Group_4__0__Impl : ( '{' ) ;
public final void rule__DictionaryReferenceControl__Group_4__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21257:1: ( ( '{' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21258:1: ( '{' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21258:1: ( '{' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21259:1: '{'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryReferenceControlAccess().getLeftCurlyBracketKeyword_4_0());
}
match(input,67,FollowSets001.FOLLOW_67_in_rule__DictionaryReferenceControl__Group_4__0__Impl43340); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryReferenceControlAccess().getLeftCurlyBracketKeyword_4_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryReferenceControl__Group_4__0__Impl"
// $ANTLR start "rule__DictionaryReferenceControl__Group_4__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21272:1: rule__DictionaryReferenceControl__Group_4__1 : rule__DictionaryReferenceControl__Group_4__1__Impl rule__DictionaryReferenceControl__Group_4__2 ;
public final void rule__DictionaryReferenceControl__Group_4__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21276:1: ( rule__DictionaryReferenceControl__Group_4__1__Impl rule__DictionaryReferenceControl__Group_4__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21277:2: rule__DictionaryReferenceControl__Group_4__1__Impl rule__DictionaryReferenceControl__Group_4__2
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryReferenceControl__Group_4__1__Impl_in_rule__DictionaryReferenceControl__Group_4__143371);
rule__DictionaryReferenceControl__Group_4__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryReferenceControl__Group_4__2_in_rule__DictionaryReferenceControl__Group_4__143374);
rule__DictionaryReferenceControl__Group_4__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryReferenceControl__Group_4__1"
// $ANTLR start "rule__DictionaryReferenceControl__Group_4__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21284:1: rule__DictionaryReferenceControl__Group_4__1__Impl : ( ( rule__DictionaryReferenceControl__BaseControlAssignment_4_1 ) ) ;
public final void rule__DictionaryReferenceControl__Group_4__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21288:1: ( ( ( rule__DictionaryReferenceControl__BaseControlAssignment_4_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21289:1: ( ( rule__DictionaryReferenceControl__BaseControlAssignment_4_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21289:1: ( ( rule__DictionaryReferenceControl__BaseControlAssignment_4_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21290:1: ( rule__DictionaryReferenceControl__BaseControlAssignment_4_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryReferenceControlAccess().getBaseControlAssignment_4_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21291:1: ( rule__DictionaryReferenceControl__BaseControlAssignment_4_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21291:2: rule__DictionaryReferenceControl__BaseControlAssignment_4_1
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryReferenceControl__BaseControlAssignment_4_1_in_rule__DictionaryReferenceControl__Group_4__1__Impl43401);
rule__DictionaryReferenceControl__BaseControlAssignment_4_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryReferenceControlAccess().getBaseControlAssignment_4_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryReferenceControl__Group_4__1__Impl"
// $ANTLR start "rule__DictionaryReferenceControl__Group_4__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21301:1: rule__DictionaryReferenceControl__Group_4__2 : rule__DictionaryReferenceControl__Group_4__2__Impl rule__DictionaryReferenceControl__Group_4__3 ;
public final void rule__DictionaryReferenceControl__Group_4__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21305:1: ( rule__DictionaryReferenceControl__Group_4__2__Impl rule__DictionaryReferenceControl__Group_4__3 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21306:2: rule__DictionaryReferenceControl__Group_4__2__Impl rule__DictionaryReferenceControl__Group_4__3
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryReferenceControl__Group_4__2__Impl_in_rule__DictionaryReferenceControl__Group_4__243431);
rule__DictionaryReferenceControl__Group_4__2__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryReferenceControl__Group_4__3_in_rule__DictionaryReferenceControl__Group_4__243434);
rule__DictionaryReferenceControl__Group_4__3();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryReferenceControl__Group_4__2"
// $ANTLR start "rule__DictionaryReferenceControl__Group_4__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21313:1: rule__DictionaryReferenceControl__Group_4__2__Impl : ( ( rule__DictionaryReferenceControl__Group_4_2__0 )? ) ;
public final void rule__DictionaryReferenceControl__Group_4__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21317:1: ( ( ( rule__DictionaryReferenceControl__Group_4_2__0 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21318:1: ( ( rule__DictionaryReferenceControl__Group_4_2__0 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21318:1: ( ( rule__DictionaryReferenceControl__Group_4_2__0 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21319:1: ( rule__DictionaryReferenceControl__Group_4_2__0 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryReferenceControlAccess().getGroup_4_2());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21320:1: ( rule__DictionaryReferenceControl__Group_4_2__0 )?
int alt173=2;
int LA173_0 = input.LA(1);
if ( (LA173_0==101) ) {
alt173=1;
}
switch (alt173) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21320:2: rule__DictionaryReferenceControl__Group_4_2__0
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryReferenceControl__Group_4_2__0_in_rule__DictionaryReferenceControl__Group_4__2__Impl43461);
rule__DictionaryReferenceControl__Group_4_2__0();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryReferenceControlAccess().getGroup_4_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryReferenceControl__Group_4__2__Impl"
// $ANTLR start "rule__DictionaryReferenceControl__Group_4__3"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21330:1: rule__DictionaryReferenceControl__Group_4__3 : rule__DictionaryReferenceControl__Group_4__3__Impl rule__DictionaryReferenceControl__Group_4__4 ;
public final void rule__DictionaryReferenceControl__Group_4__3() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21334:1: ( rule__DictionaryReferenceControl__Group_4__3__Impl rule__DictionaryReferenceControl__Group_4__4 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21335:2: rule__DictionaryReferenceControl__Group_4__3__Impl rule__DictionaryReferenceControl__Group_4__4
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryReferenceControl__Group_4__3__Impl_in_rule__DictionaryReferenceControl__Group_4__343492);
rule__DictionaryReferenceControl__Group_4__3__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryReferenceControl__Group_4__4_in_rule__DictionaryReferenceControl__Group_4__343495);
rule__DictionaryReferenceControl__Group_4__4();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryReferenceControl__Group_4__3"
// $ANTLR start "rule__DictionaryReferenceControl__Group_4__3__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21342:1: rule__DictionaryReferenceControl__Group_4__3__Impl : ( ( rule__DictionaryReferenceControl__Group_4_3__0 )? ) ;
public final void rule__DictionaryReferenceControl__Group_4__3__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21346:1: ( ( ( rule__DictionaryReferenceControl__Group_4_3__0 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21347:1: ( ( rule__DictionaryReferenceControl__Group_4_3__0 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21347:1: ( ( rule__DictionaryReferenceControl__Group_4_3__0 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21348:1: ( rule__DictionaryReferenceControl__Group_4_3__0 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryReferenceControlAccess().getGroup_4_3());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21349:1: ( rule__DictionaryReferenceControl__Group_4_3__0 )?
int alt174=2;
int LA174_0 = input.LA(1);
if ( (LA174_0==137) ) {
alt174=1;
}
switch (alt174) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21349:2: rule__DictionaryReferenceControl__Group_4_3__0
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryReferenceControl__Group_4_3__0_in_rule__DictionaryReferenceControl__Group_4__3__Impl43522);
rule__DictionaryReferenceControl__Group_4_3__0();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryReferenceControlAccess().getGroup_4_3());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryReferenceControl__Group_4__3__Impl"
// $ANTLR start "rule__DictionaryReferenceControl__Group_4__4"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21359:1: rule__DictionaryReferenceControl__Group_4__4 : rule__DictionaryReferenceControl__Group_4__4__Impl rule__DictionaryReferenceControl__Group_4__5 ;
public final void rule__DictionaryReferenceControl__Group_4__4() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21363:1: ( rule__DictionaryReferenceControl__Group_4__4__Impl rule__DictionaryReferenceControl__Group_4__5 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21364:2: rule__DictionaryReferenceControl__Group_4__4__Impl rule__DictionaryReferenceControl__Group_4__5
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryReferenceControl__Group_4__4__Impl_in_rule__DictionaryReferenceControl__Group_4__443553);
rule__DictionaryReferenceControl__Group_4__4__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryReferenceControl__Group_4__5_in_rule__DictionaryReferenceControl__Group_4__443556);
rule__DictionaryReferenceControl__Group_4__5();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryReferenceControl__Group_4__4"
// $ANTLR start "rule__DictionaryReferenceControl__Group_4__4__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21371:1: rule__DictionaryReferenceControl__Group_4__4__Impl : ( ( rule__DictionaryReferenceControl__Group_4_4__0 )? ) ;
public final void rule__DictionaryReferenceControl__Group_4__4__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21375:1: ( ( ( rule__DictionaryReferenceControl__Group_4_4__0 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21376:1: ( ( rule__DictionaryReferenceControl__Group_4_4__0 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21376:1: ( ( rule__DictionaryReferenceControl__Group_4_4__0 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21377:1: ( rule__DictionaryReferenceControl__Group_4_4__0 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryReferenceControlAccess().getGroup_4_4());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21378:1: ( rule__DictionaryReferenceControl__Group_4_4__0 )?
int alt175=2;
int LA175_0 = input.LA(1);
if ( (LA175_0==103) ) {
alt175=1;
}
switch (alt175) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21378:2: rule__DictionaryReferenceControl__Group_4_4__0
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryReferenceControl__Group_4_4__0_in_rule__DictionaryReferenceControl__Group_4__4__Impl43583);
rule__DictionaryReferenceControl__Group_4_4__0();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryReferenceControlAccess().getGroup_4_4());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryReferenceControl__Group_4__4__Impl"
// $ANTLR start "rule__DictionaryReferenceControl__Group_4__5"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21388:1: rule__DictionaryReferenceControl__Group_4__5 : rule__DictionaryReferenceControl__Group_4__5__Impl ;
public final void rule__DictionaryReferenceControl__Group_4__5() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21392:1: ( rule__DictionaryReferenceControl__Group_4__5__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21393:2: rule__DictionaryReferenceControl__Group_4__5__Impl
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryReferenceControl__Group_4__5__Impl_in_rule__DictionaryReferenceControl__Group_4__543614);
rule__DictionaryReferenceControl__Group_4__5__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryReferenceControl__Group_4__5"
// $ANTLR start "rule__DictionaryReferenceControl__Group_4__5__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21399:1: rule__DictionaryReferenceControl__Group_4__5__Impl : ( '}' ) ;
public final void rule__DictionaryReferenceControl__Group_4__5__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21403:1: ( ( '}' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21404:1: ( '}' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21404:1: ( '}' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21405:1: '}'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryReferenceControlAccess().getRightCurlyBracketKeyword_4_5());
}
match(input,68,FollowSets001.FOLLOW_68_in_rule__DictionaryReferenceControl__Group_4__5__Impl43642); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryReferenceControlAccess().getRightCurlyBracketKeyword_4_5());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryReferenceControl__Group_4__5__Impl"
// $ANTLR start "rule__DictionaryReferenceControl__Group_4_2__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21430:1: rule__DictionaryReferenceControl__Group_4_2__0 : rule__DictionaryReferenceControl__Group_4_2__0__Impl rule__DictionaryReferenceControl__Group_4_2__1 ;
public final void rule__DictionaryReferenceControl__Group_4_2__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21434:1: ( rule__DictionaryReferenceControl__Group_4_2__0__Impl rule__DictionaryReferenceControl__Group_4_2__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21435:2: rule__DictionaryReferenceControl__Group_4_2__0__Impl rule__DictionaryReferenceControl__Group_4_2__1
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryReferenceControl__Group_4_2__0__Impl_in_rule__DictionaryReferenceControl__Group_4_2__043685);
rule__DictionaryReferenceControl__Group_4_2__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryReferenceControl__Group_4_2__1_in_rule__DictionaryReferenceControl__Group_4_2__043688);
rule__DictionaryReferenceControl__Group_4_2__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryReferenceControl__Group_4_2__0"
// $ANTLR start "rule__DictionaryReferenceControl__Group_4_2__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21442:1: rule__DictionaryReferenceControl__Group_4_2__0__Impl : ( 'dictionary' ) ;
public final void rule__DictionaryReferenceControl__Group_4_2__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21446:1: ( ( 'dictionary' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21447:1: ( 'dictionary' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21447:1: ( 'dictionary' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21448:1: 'dictionary'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryReferenceControlAccess().getDictionaryKeyword_4_2_0());
}
match(input,101,FollowSets001.FOLLOW_101_in_rule__DictionaryReferenceControl__Group_4_2__0__Impl43716); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryReferenceControlAccess().getDictionaryKeyword_4_2_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryReferenceControl__Group_4_2__0__Impl"
// $ANTLR start "rule__DictionaryReferenceControl__Group_4_2__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21461:1: rule__DictionaryReferenceControl__Group_4_2__1 : rule__DictionaryReferenceControl__Group_4_2__1__Impl ;
public final void rule__DictionaryReferenceControl__Group_4_2__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21465:1: ( rule__DictionaryReferenceControl__Group_4_2__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21466:2: rule__DictionaryReferenceControl__Group_4_2__1__Impl
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryReferenceControl__Group_4_2__1__Impl_in_rule__DictionaryReferenceControl__Group_4_2__143747);
rule__DictionaryReferenceControl__Group_4_2__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryReferenceControl__Group_4_2__1"
// $ANTLR start "rule__DictionaryReferenceControl__Group_4_2__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21472:1: rule__DictionaryReferenceControl__Group_4_2__1__Impl : ( ( rule__DictionaryReferenceControl__DictionaryAssignment_4_2_1 ) ) ;
public final void rule__DictionaryReferenceControl__Group_4_2__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21476:1: ( ( ( rule__DictionaryReferenceControl__DictionaryAssignment_4_2_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21477:1: ( ( rule__DictionaryReferenceControl__DictionaryAssignment_4_2_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21477:1: ( ( rule__DictionaryReferenceControl__DictionaryAssignment_4_2_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21478:1: ( rule__DictionaryReferenceControl__DictionaryAssignment_4_2_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryReferenceControlAccess().getDictionaryAssignment_4_2_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21479:1: ( rule__DictionaryReferenceControl__DictionaryAssignment_4_2_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21479:2: rule__DictionaryReferenceControl__DictionaryAssignment_4_2_1
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryReferenceControl__DictionaryAssignment_4_2_1_in_rule__DictionaryReferenceControl__Group_4_2__1__Impl43774);
rule__DictionaryReferenceControl__DictionaryAssignment_4_2_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryReferenceControlAccess().getDictionaryAssignment_4_2_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryReferenceControl__Group_4_2__1__Impl"
// $ANTLR start "rule__DictionaryReferenceControl__Group_4_3__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21493:1: rule__DictionaryReferenceControl__Group_4_3__0 : rule__DictionaryReferenceControl__Group_4_3__0__Impl rule__DictionaryReferenceControl__Group_4_3__1 ;
public final void rule__DictionaryReferenceControl__Group_4_3__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21497:1: ( rule__DictionaryReferenceControl__Group_4_3__0__Impl rule__DictionaryReferenceControl__Group_4_3__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21498:2: rule__DictionaryReferenceControl__Group_4_3__0__Impl rule__DictionaryReferenceControl__Group_4_3__1
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryReferenceControl__Group_4_3__0__Impl_in_rule__DictionaryReferenceControl__Group_4_3__043808);
rule__DictionaryReferenceControl__Group_4_3__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryReferenceControl__Group_4_3__1_in_rule__DictionaryReferenceControl__Group_4_3__043811);
rule__DictionaryReferenceControl__Group_4_3__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryReferenceControl__Group_4_3__0"
// $ANTLR start "rule__DictionaryReferenceControl__Group_4_3__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21505:1: rule__DictionaryReferenceControl__Group_4_3__0__Impl : ( 'controlType' ) ;
public final void rule__DictionaryReferenceControl__Group_4_3__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21509:1: ( ( 'controlType' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21510:1: ( 'controlType' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21510:1: ( 'controlType' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21511:1: 'controlType'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryReferenceControlAccess().getControlTypeKeyword_4_3_0());
}
match(input,137,FollowSets001.FOLLOW_137_in_rule__DictionaryReferenceControl__Group_4_3__0__Impl43839); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryReferenceControlAccess().getControlTypeKeyword_4_3_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryReferenceControl__Group_4_3__0__Impl"
// $ANTLR start "rule__DictionaryReferenceControl__Group_4_3__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21524:1: rule__DictionaryReferenceControl__Group_4_3__1 : rule__DictionaryReferenceControl__Group_4_3__1__Impl ;
public final void rule__DictionaryReferenceControl__Group_4_3__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21528:1: ( rule__DictionaryReferenceControl__Group_4_3__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21529:2: rule__DictionaryReferenceControl__Group_4_3__1__Impl
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryReferenceControl__Group_4_3__1__Impl_in_rule__DictionaryReferenceControl__Group_4_3__143870);
rule__DictionaryReferenceControl__Group_4_3__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryReferenceControl__Group_4_3__1"
// $ANTLR start "rule__DictionaryReferenceControl__Group_4_3__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21535:1: rule__DictionaryReferenceControl__Group_4_3__1__Impl : ( ( rule__DictionaryReferenceControl__ControlTypeAssignment_4_3_1 ) ) ;
public final void rule__DictionaryReferenceControl__Group_4_3__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21539:1: ( ( ( rule__DictionaryReferenceControl__ControlTypeAssignment_4_3_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21540:1: ( ( rule__DictionaryReferenceControl__ControlTypeAssignment_4_3_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21540:1: ( ( rule__DictionaryReferenceControl__ControlTypeAssignment_4_3_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21541:1: ( rule__DictionaryReferenceControl__ControlTypeAssignment_4_3_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryReferenceControlAccess().getControlTypeAssignment_4_3_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21542:1: ( rule__DictionaryReferenceControl__ControlTypeAssignment_4_3_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21542:2: rule__DictionaryReferenceControl__ControlTypeAssignment_4_3_1
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryReferenceControl__ControlTypeAssignment_4_3_1_in_rule__DictionaryReferenceControl__Group_4_3__1__Impl43897);
rule__DictionaryReferenceControl__ControlTypeAssignment_4_3_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryReferenceControlAccess().getControlTypeAssignment_4_3_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryReferenceControl__Group_4_3__1__Impl"
// $ANTLR start "rule__DictionaryReferenceControl__Group_4_4__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21556:1: rule__DictionaryReferenceControl__Group_4_4__0 : rule__DictionaryReferenceControl__Group_4_4__0__Impl rule__DictionaryReferenceControl__Group_4_4__1 ;
public final void rule__DictionaryReferenceControl__Group_4_4__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21560:1: ( rule__DictionaryReferenceControl__Group_4_4__0__Impl rule__DictionaryReferenceControl__Group_4_4__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21561:2: rule__DictionaryReferenceControl__Group_4_4__0__Impl rule__DictionaryReferenceControl__Group_4_4__1
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryReferenceControl__Group_4_4__0__Impl_in_rule__DictionaryReferenceControl__Group_4_4__043931);
rule__DictionaryReferenceControl__Group_4_4__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryReferenceControl__Group_4_4__1_in_rule__DictionaryReferenceControl__Group_4_4__043934);
rule__DictionaryReferenceControl__Group_4_4__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryReferenceControl__Group_4_4__0"
// $ANTLR start "rule__DictionaryReferenceControl__Group_4_4__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21568:1: rule__DictionaryReferenceControl__Group_4_4__0__Impl : ( 'labelcontrols' ) ;
public final void rule__DictionaryReferenceControl__Group_4_4__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21572:1: ( ( 'labelcontrols' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21573:1: ( 'labelcontrols' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21573:1: ( 'labelcontrols' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21574:1: 'labelcontrols'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryReferenceControlAccess().getLabelcontrolsKeyword_4_4_0());
}
match(input,103,FollowSets001.FOLLOW_103_in_rule__DictionaryReferenceControl__Group_4_4__0__Impl43962); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryReferenceControlAccess().getLabelcontrolsKeyword_4_4_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryReferenceControl__Group_4_4__0__Impl"
// $ANTLR start "rule__DictionaryReferenceControl__Group_4_4__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21587:1: rule__DictionaryReferenceControl__Group_4_4__1 : rule__DictionaryReferenceControl__Group_4_4__1__Impl rule__DictionaryReferenceControl__Group_4_4__2 ;
public final void rule__DictionaryReferenceControl__Group_4_4__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21591:1: ( rule__DictionaryReferenceControl__Group_4_4__1__Impl rule__DictionaryReferenceControl__Group_4_4__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21592:2: rule__DictionaryReferenceControl__Group_4_4__1__Impl rule__DictionaryReferenceControl__Group_4_4__2
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryReferenceControl__Group_4_4__1__Impl_in_rule__DictionaryReferenceControl__Group_4_4__143993);
rule__DictionaryReferenceControl__Group_4_4__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryReferenceControl__Group_4_4__2_in_rule__DictionaryReferenceControl__Group_4_4__143996);
rule__DictionaryReferenceControl__Group_4_4__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryReferenceControl__Group_4_4__1"
// $ANTLR start "rule__DictionaryReferenceControl__Group_4_4__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21599:1: rule__DictionaryReferenceControl__Group_4_4__1__Impl : ( '{' ) ;
public final void rule__DictionaryReferenceControl__Group_4_4__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21603:1: ( ( '{' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21604:1: ( '{' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21604:1: ( '{' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21605:1: '{'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryReferenceControlAccess().getLeftCurlyBracketKeyword_4_4_1());
}
match(input,67,FollowSets001.FOLLOW_67_in_rule__DictionaryReferenceControl__Group_4_4__1__Impl44024); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryReferenceControlAccess().getLeftCurlyBracketKeyword_4_4_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryReferenceControl__Group_4_4__1__Impl"
// $ANTLR start "rule__DictionaryReferenceControl__Group_4_4__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21618:1: rule__DictionaryReferenceControl__Group_4_4__2 : rule__DictionaryReferenceControl__Group_4_4__2__Impl rule__DictionaryReferenceControl__Group_4_4__3 ;
public final void rule__DictionaryReferenceControl__Group_4_4__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21622:1: ( rule__DictionaryReferenceControl__Group_4_4__2__Impl rule__DictionaryReferenceControl__Group_4_4__3 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21623:2: rule__DictionaryReferenceControl__Group_4_4__2__Impl rule__DictionaryReferenceControl__Group_4_4__3
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryReferenceControl__Group_4_4__2__Impl_in_rule__DictionaryReferenceControl__Group_4_4__244055);
rule__DictionaryReferenceControl__Group_4_4__2__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryReferenceControl__Group_4_4__3_in_rule__DictionaryReferenceControl__Group_4_4__244058);
rule__DictionaryReferenceControl__Group_4_4__3();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryReferenceControl__Group_4_4__2"
// $ANTLR start "rule__DictionaryReferenceControl__Group_4_4__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21630:1: rule__DictionaryReferenceControl__Group_4_4__2__Impl : ( ( rule__DictionaryReferenceControl__LabelcontrolsAssignment_4_4_2 )* ) ;
public final void rule__DictionaryReferenceControl__Group_4_4__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21634:1: ( ( ( rule__DictionaryReferenceControl__LabelcontrolsAssignment_4_4_2 )* ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21635:1: ( ( rule__DictionaryReferenceControl__LabelcontrolsAssignment_4_4_2 )* )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21635:1: ( ( rule__DictionaryReferenceControl__LabelcontrolsAssignment_4_4_2 )* )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21636:1: ( rule__DictionaryReferenceControl__LabelcontrolsAssignment_4_4_2 )*
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryReferenceControlAccess().getLabelcontrolsAssignment_4_4_2());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21637:1: ( rule__DictionaryReferenceControl__LabelcontrolsAssignment_4_4_2 )*
loop176:
do {
int alt176=2;
int LA176_0 = input.LA(1);
if ( (LA176_0==125||LA176_0==127||LA176_0==129||(LA176_0>=131 && LA176_0<=136)||LA176_0==138) ) {
alt176=1;
}
switch (alt176) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21637:2: rule__DictionaryReferenceControl__LabelcontrolsAssignment_4_4_2
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryReferenceControl__LabelcontrolsAssignment_4_4_2_in_rule__DictionaryReferenceControl__Group_4_4__2__Impl44085);
rule__DictionaryReferenceControl__LabelcontrolsAssignment_4_4_2();
state._fsp--;
if (state.failed) return ;
}
break;
default :
break loop176;
}
} while (true);
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryReferenceControlAccess().getLabelcontrolsAssignment_4_4_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryReferenceControl__Group_4_4__2__Impl"
// $ANTLR start "rule__DictionaryReferenceControl__Group_4_4__3"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21647:1: rule__DictionaryReferenceControl__Group_4_4__3 : rule__DictionaryReferenceControl__Group_4_4__3__Impl ;
public final void rule__DictionaryReferenceControl__Group_4_4__3() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21651:1: ( rule__DictionaryReferenceControl__Group_4_4__3__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21652:2: rule__DictionaryReferenceControl__Group_4_4__3__Impl
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryReferenceControl__Group_4_4__3__Impl_in_rule__DictionaryReferenceControl__Group_4_4__344116);
rule__DictionaryReferenceControl__Group_4_4__3__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryReferenceControl__Group_4_4__3"
// $ANTLR start "rule__DictionaryReferenceControl__Group_4_4__3__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21658:1: rule__DictionaryReferenceControl__Group_4_4__3__Impl : ( '}' ) ;
public final void rule__DictionaryReferenceControl__Group_4_4__3__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21662:1: ( ( '}' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21663:1: ( '}' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21663:1: ( '}' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21664:1: '}'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryReferenceControlAccess().getRightCurlyBracketKeyword_4_4_3());
}
match(input,68,FollowSets001.FOLLOW_68_in_rule__DictionaryReferenceControl__Group_4_4__3__Impl44144); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryReferenceControlAccess().getRightCurlyBracketKeyword_4_4_3());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryReferenceControl__Group_4_4__3__Impl"
// $ANTLR start "rule__DictionaryFileControl__Group__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21685:1: rule__DictionaryFileControl__Group__0 : rule__DictionaryFileControl__Group__0__Impl rule__DictionaryFileControl__Group__1 ;
public final void rule__DictionaryFileControl__Group__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21689:1: ( rule__DictionaryFileControl__Group__0__Impl rule__DictionaryFileControl__Group__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21690:2: rule__DictionaryFileControl__Group__0__Impl rule__DictionaryFileControl__Group__1
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryFileControl__Group__0__Impl_in_rule__DictionaryFileControl__Group__044183);
rule__DictionaryFileControl__Group__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryFileControl__Group__1_in_rule__DictionaryFileControl__Group__044186);
rule__DictionaryFileControl__Group__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryFileControl__Group__0"
// $ANTLR start "rule__DictionaryFileControl__Group__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21697:1: rule__DictionaryFileControl__Group__0__Impl : ( () ) ;
public final void rule__DictionaryFileControl__Group__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21701:1: ( ( () ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21702:1: ( () )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21702:1: ( () )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21703:1: ()
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryFileControlAccess().getDictionaryFileControlAction_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21704:1: ()
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21706:1:
{
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryFileControlAccess().getDictionaryFileControlAction_0());
}
}
}
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryFileControl__Group__0__Impl"
// $ANTLR start "rule__DictionaryFileControl__Group__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21716:1: rule__DictionaryFileControl__Group__1 : rule__DictionaryFileControl__Group__1__Impl rule__DictionaryFileControl__Group__2 ;
public final void rule__DictionaryFileControl__Group__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21720:1: ( rule__DictionaryFileControl__Group__1__Impl rule__DictionaryFileControl__Group__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21721:2: rule__DictionaryFileControl__Group__1__Impl rule__DictionaryFileControl__Group__2
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryFileControl__Group__1__Impl_in_rule__DictionaryFileControl__Group__144244);
rule__DictionaryFileControl__Group__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryFileControl__Group__2_in_rule__DictionaryFileControl__Group__144247);
rule__DictionaryFileControl__Group__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryFileControl__Group__1"
// $ANTLR start "rule__DictionaryFileControl__Group__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21728:1: rule__DictionaryFileControl__Group__1__Impl : ( 'filecontrol' ) ;
public final void rule__DictionaryFileControl__Group__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21732:1: ( ( 'filecontrol' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21733:1: ( 'filecontrol' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21733:1: ( 'filecontrol' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21734:1: 'filecontrol'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryFileControlAccess().getFilecontrolKeyword_1());
}
match(input,138,FollowSets001.FOLLOW_138_in_rule__DictionaryFileControl__Group__1__Impl44275); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryFileControlAccess().getFilecontrolKeyword_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryFileControl__Group__1__Impl"
// $ANTLR start "rule__DictionaryFileControl__Group__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21747:1: rule__DictionaryFileControl__Group__2 : rule__DictionaryFileControl__Group__2__Impl rule__DictionaryFileControl__Group__3 ;
public final void rule__DictionaryFileControl__Group__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21751:1: ( rule__DictionaryFileControl__Group__2__Impl rule__DictionaryFileControl__Group__3 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21752:2: rule__DictionaryFileControl__Group__2__Impl rule__DictionaryFileControl__Group__3
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryFileControl__Group__2__Impl_in_rule__DictionaryFileControl__Group__244306);
rule__DictionaryFileControl__Group__2__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryFileControl__Group__3_in_rule__DictionaryFileControl__Group__244309);
rule__DictionaryFileControl__Group__3();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryFileControl__Group__2"
// $ANTLR start "rule__DictionaryFileControl__Group__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21759:1: rule__DictionaryFileControl__Group__2__Impl : ( ( rule__DictionaryFileControl__NameAssignment_2 )? ) ;
public final void rule__DictionaryFileControl__Group__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21763:1: ( ( ( rule__DictionaryFileControl__NameAssignment_2 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21764:1: ( ( rule__DictionaryFileControl__NameAssignment_2 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21764:1: ( ( rule__DictionaryFileControl__NameAssignment_2 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21765:1: ( rule__DictionaryFileControl__NameAssignment_2 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryFileControlAccess().getNameAssignment_2());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21766:1: ( rule__DictionaryFileControl__NameAssignment_2 )?
int alt177=2;
int LA177_0 = input.LA(1);
if ( (LA177_0==RULE_ID) ) {
alt177=1;
}
switch (alt177) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21766:2: rule__DictionaryFileControl__NameAssignment_2
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryFileControl__NameAssignment_2_in_rule__DictionaryFileControl__Group__2__Impl44336);
rule__DictionaryFileControl__NameAssignment_2();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryFileControlAccess().getNameAssignment_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryFileControl__Group__2__Impl"
// $ANTLR start "rule__DictionaryFileControl__Group__3"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21776:1: rule__DictionaryFileControl__Group__3 : rule__DictionaryFileControl__Group__3__Impl rule__DictionaryFileControl__Group__4 ;
public final void rule__DictionaryFileControl__Group__3() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21780:1: ( rule__DictionaryFileControl__Group__3__Impl rule__DictionaryFileControl__Group__4 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21781:2: rule__DictionaryFileControl__Group__3__Impl rule__DictionaryFileControl__Group__4
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryFileControl__Group__3__Impl_in_rule__DictionaryFileControl__Group__344367);
rule__DictionaryFileControl__Group__3__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryFileControl__Group__4_in_rule__DictionaryFileControl__Group__344370);
rule__DictionaryFileControl__Group__4();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryFileControl__Group__3"
// $ANTLR start "rule__DictionaryFileControl__Group__3__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21788:1: rule__DictionaryFileControl__Group__3__Impl : ( ( rule__DictionaryFileControl__Group_3__0 )? ) ;
public final void rule__DictionaryFileControl__Group__3__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21792:1: ( ( ( rule__DictionaryFileControl__Group_3__0 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21793:1: ( ( rule__DictionaryFileControl__Group_3__0 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21793:1: ( ( rule__DictionaryFileControl__Group_3__0 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21794:1: ( rule__DictionaryFileControl__Group_3__0 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryFileControlAccess().getGroup_3());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21795:1: ( rule__DictionaryFileControl__Group_3__0 )?
int alt178=2;
int LA178_0 = input.LA(1);
if ( (LA178_0==126) ) {
alt178=1;
}
switch (alt178) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21795:2: rule__DictionaryFileControl__Group_3__0
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryFileControl__Group_3__0_in_rule__DictionaryFileControl__Group__3__Impl44397);
rule__DictionaryFileControl__Group_3__0();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryFileControlAccess().getGroup_3());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryFileControl__Group__3__Impl"
// $ANTLR start "rule__DictionaryFileControl__Group__4"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21805:1: rule__DictionaryFileControl__Group__4 : rule__DictionaryFileControl__Group__4__Impl ;
public final void rule__DictionaryFileControl__Group__4() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21809:1: ( rule__DictionaryFileControl__Group__4__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21810:2: rule__DictionaryFileControl__Group__4__Impl
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryFileControl__Group__4__Impl_in_rule__DictionaryFileControl__Group__444428);
rule__DictionaryFileControl__Group__4__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryFileControl__Group__4"
// $ANTLR start "rule__DictionaryFileControl__Group__4__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21816:1: rule__DictionaryFileControl__Group__4__Impl : ( ( rule__DictionaryFileControl__Group_4__0 )? ) ;
public final void rule__DictionaryFileControl__Group__4__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21820:1: ( ( ( rule__DictionaryFileControl__Group_4__0 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21821:1: ( ( rule__DictionaryFileControl__Group_4__0 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21821:1: ( ( rule__DictionaryFileControl__Group_4__0 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21822:1: ( rule__DictionaryFileControl__Group_4__0 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryFileControlAccess().getGroup_4());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21823:1: ( rule__DictionaryFileControl__Group_4__0 )?
int alt179=2;
int LA179_0 = input.LA(1);
if ( (LA179_0==67) ) {
alt179=1;
}
switch (alt179) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21823:2: rule__DictionaryFileControl__Group_4__0
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryFileControl__Group_4__0_in_rule__DictionaryFileControl__Group__4__Impl44455);
rule__DictionaryFileControl__Group_4__0();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryFileControlAccess().getGroup_4());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryFileControl__Group__4__Impl"
// $ANTLR start "rule__DictionaryFileControl__Group_3__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21843:1: rule__DictionaryFileControl__Group_3__0 : rule__DictionaryFileControl__Group_3__0__Impl rule__DictionaryFileControl__Group_3__1 ;
public final void rule__DictionaryFileControl__Group_3__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21847:1: ( rule__DictionaryFileControl__Group_3__0__Impl rule__DictionaryFileControl__Group_3__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21848:2: rule__DictionaryFileControl__Group_3__0__Impl rule__DictionaryFileControl__Group_3__1
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryFileControl__Group_3__0__Impl_in_rule__DictionaryFileControl__Group_3__044496);
rule__DictionaryFileControl__Group_3__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryFileControl__Group_3__1_in_rule__DictionaryFileControl__Group_3__044499);
rule__DictionaryFileControl__Group_3__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryFileControl__Group_3__0"
// $ANTLR start "rule__DictionaryFileControl__Group_3__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21855:1: rule__DictionaryFileControl__Group_3__0__Impl : ( 'ref' ) ;
public final void rule__DictionaryFileControl__Group_3__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21859:1: ( ( 'ref' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21860:1: ( 'ref' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21860:1: ( 'ref' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21861:1: 'ref'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryFileControlAccess().getRefKeyword_3_0());
}
match(input,126,FollowSets001.FOLLOW_126_in_rule__DictionaryFileControl__Group_3__0__Impl44527); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryFileControlAccess().getRefKeyword_3_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryFileControl__Group_3__0__Impl"
// $ANTLR start "rule__DictionaryFileControl__Group_3__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21874:1: rule__DictionaryFileControl__Group_3__1 : rule__DictionaryFileControl__Group_3__1__Impl ;
public final void rule__DictionaryFileControl__Group_3__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21878:1: ( rule__DictionaryFileControl__Group_3__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21879:2: rule__DictionaryFileControl__Group_3__1__Impl
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryFileControl__Group_3__1__Impl_in_rule__DictionaryFileControl__Group_3__144558);
rule__DictionaryFileControl__Group_3__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryFileControl__Group_3__1"
// $ANTLR start "rule__DictionaryFileControl__Group_3__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21885:1: rule__DictionaryFileControl__Group_3__1__Impl : ( ( rule__DictionaryFileControl__RefAssignment_3_1 ) ) ;
public final void rule__DictionaryFileControl__Group_3__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21889:1: ( ( ( rule__DictionaryFileControl__RefAssignment_3_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21890:1: ( ( rule__DictionaryFileControl__RefAssignment_3_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21890:1: ( ( rule__DictionaryFileControl__RefAssignment_3_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21891:1: ( rule__DictionaryFileControl__RefAssignment_3_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryFileControlAccess().getRefAssignment_3_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21892:1: ( rule__DictionaryFileControl__RefAssignment_3_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21892:2: rule__DictionaryFileControl__RefAssignment_3_1
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryFileControl__RefAssignment_3_1_in_rule__DictionaryFileControl__Group_3__1__Impl44585);
rule__DictionaryFileControl__RefAssignment_3_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryFileControlAccess().getRefAssignment_3_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryFileControl__Group_3__1__Impl"
// $ANTLR start "rule__DictionaryFileControl__Group_4__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21906:1: rule__DictionaryFileControl__Group_4__0 : rule__DictionaryFileControl__Group_4__0__Impl rule__DictionaryFileControl__Group_4__1 ;
public final void rule__DictionaryFileControl__Group_4__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21910:1: ( rule__DictionaryFileControl__Group_4__0__Impl rule__DictionaryFileControl__Group_4__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21911:2: rule__DictionaryFileControl__Group_4__0__Impl rule__DictionaryFileControl__Group_4__1
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryFileControl__Group_4__0__Impl_in_rule__DictionaryFileControl__Group_4__044619);
rule__DictionaryFileControl__Group_4__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryFileControl__Group_4__1_in_rule__DictionaryFileControl__Group_4__044622);
rule__DictionaryFileControl__Group_4__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryFileControl__Group_4__0"
// $ANTLR start "rule__DictionaryFileControl__Group_4__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21918:1: rule__DictionaryFileControl__Group_4__0__Impl : ( '{' ) ;
public final void rule__DictionaryFileControl__Group_4__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21922:1: ( ( '{' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21923:1: ( '{' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21923:1: ( '{' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21924:1: '{'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryFileControlAccess().getLeftCurlyBracketKeyword_4_0());
}
match(input,67,FollowSets001.FOLLOW_67_in_rule__DictionaryFileControl__Group_4__0__Impl44650); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryFileControlAccess().getLeftCurlyBracketKeyword_4_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryFileControl__Group_4__0__Impl"
// $ANTLR start "rule__DictionaryFileControl__Group_4__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21937:1: rule__DictionaryFileControl__Group_4__1 : rule__DictionaryFileControl__Group_4__1__Impl rule__DictionaryFileControl__Group_4__2 ;
public final void rule__DictionaryFileControl__Group_4__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21941:1: ( rule__DictionaryFileControl__Group_4__1__Impl rule__DictionaryFileControl__Group_4__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21942:2: rule__DictionaryFileControl__Group_4__1__Impl rule__DictionaryFileControl__Group_4__2
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryFileControl__Group_4__1__Impl_in_rule__DictionaryFileControl__Group_4__144681);
rule__DictionaryFileControl__Group_4__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets001.FOLLOW_rule__DictionaryFileControl__Group_4__2_in_rule__DictionaryFileControl__Group_4__144684);
rule__DictionaryFileControl__Group_4__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryFileControl__Group_4__1"
// $ANTLR start "rule__DictionaryFileControl__Group_4__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21949:1: rule__DictionaryFileControl__Group_4__1__Impl : ( ( rule__DictionaryFileControl__BaseControlAssignment_4_1 ) ) ;
public final void rule__DictionaryFileControl__Group_4__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21953:1: ( ( ( rule__DictionaryFileControl__BaseControlAssignment_4_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21954:1: ( ( rule__DictionaryFileControl__BaseControlAssignment_4_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21954:1: ( ( rule__DictionaryFileControl__BaseControlAssignment_4_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21955:1: ( rule__DictionaryFileControl__BaseControlAssignment_4_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryFileControlAccess().getBaseControlAssignment_4_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21956:1: ( rule__DictionaryFileControl__BaseControlAssignment_4_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21956:2: rule__DictionaryFileControl__BaseControlAssignment_4_1
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryFileControl__BaseControlAssignment_4_1_in_rule__DictionaryFileControl__Group_4__1__Impl44711);
rule__DictionaryFileControl__BaseControlAssignment_4_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryFileControlAccess().getBaseControlAssignment_4_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryFileControl__Group_4__1__Impl"
// $ANTLR start "rule__DictionaryFileControl__Group_4__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21966:1: rule__DictionaryFileControl__Group_4__2 : rule__DictionaryFileControl__Group_4__2__Impl ;
public final void rule__DictionaryFileControl__Group_4__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21970:1: ( rule__DictionaryFileControl__Group_4__2__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21971:2: rule__DictionaryFileControl__Group_4__2__Impl
{
pushFollow(FollowSets001.FOLLOW_rule__DictionaryFileControl__Group_4__2__Impl_in_rule__DictionaryFileControl__Group_4__244741);
rule__DictionaryFileControl__Group_4__2__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryFileControl__Group_4__2"
// $ANTLR start "rule__DictionaryFileControl__Group_4__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21977:1: rule__DictionaryFileControl__Group_4__2__Impl : ( '}' ) ;
public final void rule__DictionaryFileControl__Group_4__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21981:1: ( ( '}' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21982:1: ( '}' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21982:1: ( '}' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:21983:1: '}'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryFileControlAccess().getRightCurlyBracketKeyword_4_2());
}
match(input,68,FollowSets001.FOLLOW_68_in_rule__DictionaryFileControl__Group_4__2__Impl44769); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryFileControlAccess().getRightCurlyBracketKeyword_4_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryFileControl__Group_4__2__Impl"
// $ANTLR start "rule__Module__Group__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22002:1: rule__Module__Group__0 : rule__Module__Group__0__Impl rule__Module__Group__1 ;
public final void rule__Module__Group__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22006:1: ( rule__Module__Group__0__Impl rule__Module__Group__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22007:2: rule__Module__Group__0__Impl rule__Module__Group__1
{
pushFollow(FollowSets001.FOLLOW_rule__Module__Group__0__Impl_in_rule__Module__Group__044806);
rule__Module__Group__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__Module__Group__1_in_rule__Module__Group__044809);
rule__Module__Group__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Module__Group__0"
// $ANTLR start "rule__Module__Group__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22014:1: rule__Module__Group__0__Impl : ( 'module' ) ;
public final void rule__Module__Group__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22018:1: ( ( 'module' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22019:1: ( 'module' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22019:1: ( 'module' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22020:1: 'module'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getModuleAccess().getModuleKeyword_0());
}
match(input,139,FollowSets002.FOLLOW_139_in_rule__Module__Group__0__Impl44837); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getModuleAccess().getModuleKeyword_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Module__Group__0__Impl"
// $ANTLR start "rule__Module__Group__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22033:1: rule__Module__Group__1 : rule__Module__Group__1__Impl rule__Module__Group__2 ;
public final void rule__Module__Group__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22037:1: ( rule__Module__Group__1__Impl rule__Module__Group__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22038:2: rule__Module__Group__1__Impl rule__Module__Group__2
{
pushFollow(FollowSets002.FOLLOW_rule__Module__Group__1__Impl_in_rule__Module__Group__144868);
rule__Module__Group__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__Module__Group__2_in_rule__Module__Group__144871);
rule__Module__Group__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Module__Group__1"
// $ANTLR start "rule__Module__Group__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22045:1: rule__Module__Group__1__Impl : ( ( rule__Module__NameAssignment_1 ) ) ;
public final void rule__Module__Group__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22049:1: ( ( ( rule__Module__NameAssignment_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22050:1: ( ( rule__Module__NameAssignment_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22050:1: ( ( rule__Module__NameAssignment_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22051:1: ( rule__Module__NameAssignment_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getModuleAccess().getNameAssignment_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22052:1: ( rule__Module__NameAssignment_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22052:2: rule__Module__NameAssignment_1
{
pushFollow(FollowSets002.FOLLOW_rule__Module__NameAssignment_1_in_rule__Module__Group__1__Impl44898);
rule__Module__NameAssignment_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getModuleAccess().getNameAssignment_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Module__Group__1__Impl"
// $ANTLR start "rule__Module__Group__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22062:1: rule__Module__Group__2 : rule__Module__Group__2__Impl rule__Module__Group__3 ;
public final void rule__Module__Group__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22066:1: ( rule__Module__Group__2__Impl rule__Module__Group__3 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22067:2: rule__Module__Group__2__Impl rule__Module__Group__3
{
pushFollow(FollowSets002.FOLLOW_rule__Module__Group__2__Impl_in_rule__Module__Group__244928);
rule__Module__Group__2__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__Module__Group__3_in_rule__Module__Group__244931);
rule__Module__Group__3();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Module__Group__2"
// $ANTLR start "rule__Module__Group__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22074:1: rule__Module__Group__2__Impl : ( '{' ) ;
public final void rule__Module__Group__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22078:1: ( ( '{' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22079:1: ( '{' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22079:1: ( '{' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22080:1: '{'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getModuleAccess().getLeftCurlyBracketKeyword_2());
}
match(input,67,FollowSets002.FOLLOW_67_in_rule__Module__Group__2__Impl44959); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getModuleAccess().getLeftCurlyBracketKeyword_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Module__Group__2__Impl"
// $ANTLR start "rule__Module__Group__3"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22093:1: rule__Module__Group__3 : rule__Module__Group__3__Impl rule__Module__Group__4 ;
public final void rule__Module__Group__3() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22097:1: ( rule__Module__Group__3__Impl rule__Module__Group__4 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22098:2: rule__Module__Group__3__Impl rule__Module__Group__4
{
pushFollow(FollowSets002.FOLLOW_rule__Module__Group__3__Impl_in_rule__Module__Group__344990);
rule__Module__Group__3__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__Module__Group__4_in_rule__Module__Group__344993);
rule__Module__Group__4();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Module__Group__3"
// $ANTLR start "rule__Module__Group__3__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22105:1: rule__Module__Group__3__Impl : ( 'moduledefinition' ) ;
public final void rule__Module__Group__3__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22109:1: ( ( 'moduledefinition' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22110:1: ( 'moduledefinition' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22110:1: ( 'moduledefinition' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22111:1: 'moduledefinition'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getModuleAccess().getModuledefinitionKeyword_3());
}
match(input,140,FollowSets002.FOLLOW_140_in_rule__Module__Group__3__Impl45021); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getModuleAccess().getModuledefinitionKeyword_3());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Module__Group__3__Impl"
// $ANTLR start "rule__Module__Group__4"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22124:1: rule__Module__Group__4 : rule__Module__Group__4__Impl rule__Module__Group__5 ;
public final void rule__Module__Group__4() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22128:1: ( rule__Module__Group__4__Impl rule__Module__Group__5 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22129:2: rule__Module__Group__4__Impl rule__Module__Group__5
{
pushFollow(FollowSets002.FOLLOW_rule__Module__Group__4__Impl_in_rule__Module__Group__445052);
rule__Module__Group__4__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__Module__Group__5_in_rule__Module__Group__445055);
rule__Module__Group__5();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Module__Group__4"
// $ANTLR start "rule__Module__Group__4__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22136:1: rule__Module__Group__4__Impl : ( ( rule__Module__ModuledefinitionAssignment_4 ) ) ;
public final void rule__Module__Group__4__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22140:1: ( ( ( rule__Module__ModuledefinitionAssignment_4 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22141:1: ( ( rule__Module__ModuledefinitionAssignment_4 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22141:1: ( ( rule__Module__ModuledefinitionAssignment_4 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22142:1: ( rule__Module__ModuledefinitionAssignment_4 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getModuleAccess().getModuledefinitionAssignment_4());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22143:1: ( rule__Module__ModuledefinitionAssignment_4 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22143:2: rule__Module__ModuledefinitionAssignment_4
{
pushFollow(FollowSets002.FOLLOW_rule__Module__ModuledefinitionAssignment_4_in_rule__Module__Group__4__Impl45082);
rule__Module__ModuledefinitionAssignment_4();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getModuleAccess().getModuledefinitionAssignment_4());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Module__Group__4__Impl"
// $ANTLR start "rule__Module__Group__5"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22153:1: rule__Module__Group__5 : rule__Module__Group__5__Impl rule__Module__Group__6 ;
public final void rule__Module__Group__5() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22157:1: ( rule__Module__Group__5__Impl rule__Module__Group__6 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22158:2: rule__Module__Group__5__Impl rule__Module__Group__6
{
pushFollow(FollowSets002.FOLLOW_rule__Module__Group__5__Impl_in_rule__Module__Group__545112);
rule__Module__Group__5__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__Module__Group__6_in_rule__Module__Group__545115);
rule__Module__Group__6();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Module__Group__5"
// $ANTLR start "rule__Module__Group__5__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22165:1: rule__Module__Group__5__Impl : ( ( rule__Module__Group_5__0 )? ) ;
public final void rule__Module__Group__5__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22169:1: ( ( ( rule__Module__Group_5__0 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22170:1: ( ( rule__Module__Group_5__0 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22170:1: ( ( rule__Module__Group_5__0 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22171:1: ( rule__Module__Group_5__0 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getModuleAccess().getGroup_5());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22172:1: ( rule__Module__Group_5__0 )?
int alt180=2;
int LA180_0 = input.LA(1);
if ( (LA180_0==141) ) {
alt180=1;
}
switch (alt180) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22172:2: rule__Module__Group_5__0
{
pushFollow(FollowSets002.FOLLOW_rule__Module__Group_5__0_in_rule__Module__Group__5__Impl45142);
rule__Module__Group_5__0();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getModuleAccess().getGroup_5());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Module__Group__5__Impl"
// $ANTLR start "rule__Module__Group__6"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22182:1: rule__Module__Group__6 : rule__Module__Group__6__Impl ;
public final void rule__Module__Group__6() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22186:1: ( rule__Module__Group__6__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22187:2: rule__Module__Group__6__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__Module__Group__6__Impl_in_rule__Module__Group__645173);
rule__Module__Group__6__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Module__Group__6"
// $ANTLR start "rule__Module__Group__6__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22193:1: rule__Module__Group__6__Impl : ( '}' ) ;
public final void rule__Module__Group__6__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22197:1: ( ( '}' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22198:1: ( '}' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22198:1: ( '}' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22199:1: '}'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getModuleAccess().getRightCurlyBracketKeyword_6());
}
match(input,68,FollowSets002.FOLLOW_68_in_rule__Module__Group__6__Impl45201); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getModuleAccess().getRightCurlyBracketKeyword_6());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Module__Group__6__Impl"
// $ANTLR start "rule__Module__Group_5__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22226:1: rule__Module__Group_5__0 : rule__Module__Group_5__0__Impl rule__Module__Group_5__1 ;
public final void rule__Module__Group_5__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22230:1: ( rule__Module__Group_5__0__Impl rule__Module__Group_5__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22231:2: rule__Module__Group_5__0__Impl rule__Module__Group_5__1
{
pushFollow(FollowSets002.FOLLOW_rule__Module__Group_5__0__Impl_in_rule__Module__Group_5__045246);
rule__Module__Group_5__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__Module__Group_5__1_in_rule__Module__Group_5__045249);
rule__Module__Group_5__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Module__Group_5__0"
// $ANTLR start "rule__Module__Group_5__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22238:1: rule__Module__Group_5__0__Impl : ( 'parameters' ) ;
public final void rule__Module__Group_5__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22242:1: ( ( 'parameters' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22243:1: ( 'parameters' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22243:1: ( 'parameters' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22244:1: 'parameters'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getModuleAccess().getParametersKeyword_5_0());
}
match(input,141,FollowSets002.FOLLOW_141_in_rule__Module__Group_5__0__Impl45277); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getModuleAccess().getParametersKeyword_5_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Module__Group_5__0__Impl"
// $ANTLR start "rule__Module__Group_5__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22257:1: rule__Module__Group_5__1 : rule__Module__Group_5__1__Impl rule__Module__Group_5__2 ;
public final void rule__Module__Group_5__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22261:1: ( rule__Module__Group_5__1__Impl rule__Module__Group_5__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22262:2: rule__Module__Group_5__1__Impl rule__Module__Group_5__2
{
pushFollow(FollowSets002.FOLLOW_rule__Module__Group_5__1__Impl_in_rule__Module__Group_5__145308);
rule__Module__Group_5__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__Module__Group_5__2_in_rule__Module__Group_5__145311);
rule__Module__Group_5__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Module__Group_5__1"
// $ANTLR start "rule__Module__Group_5__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22269:1: rule__Module__Group_5__1__Impl : ( '{' ) ;
public final void rule__Module__Group_5__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22273:1: ( ( '{' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22274:1: ( '{' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22274:1: ( '{' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22275:1: '{'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getModuleAccess().getLeftCurlyBracketKeyword_5_1());
}
match(input,67,FollowSets002.FOLLOW_67_in_rule__Module__Group_5__1__Impl45339); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getModuleAccess().getLeftCurlyBracketKeyword_5_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Module__Group_5__1__Impl"
// $ANTLR start "rule__Module__Group_5__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22288:1: rule__Module__Group_5__2 : rule__Module__Group_5__2__Impl rule__Module__Group_5__3 ;
public final void rule__Module__Group_5__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22292:1: ( rule__Module__Group_5__2__Impl rule__Module__Group_5__3 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22293:2: rule__Module__Group_5__2__Impl rule__Module__Group_5__3
{
pushFollow(FollowSets002.FOLLOW_rule__Module__Group_5__2__Impl_in_rule__Module__Group_5__245370);
rule__Module__Group_5__2__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__Module__Group_5__3_in_rule__Module__Group_5__245373);
rule__Module__Group_5__3();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Module__Group_5__2"
// $ANTLR start "rule__Module__Group_5__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22300:1: rule__Module__Group_5__2__Impl : ( ( rule__Module__ModuleParametersAssignment_5_2 )* ) ;
public final void rule__Module__Group_5__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22304:1: ( ( ( rule__Module__ModuleParametersAssignment_5_2 )* ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22305:1: ( ( rule__Module__ModuleParametersAssignment_5_2 )* )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22305:1: ( ( rule__Module__ModuleParametersAssignment_5_2 )* )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22306:1: ( rule__Module__ModuleParametersAssignment_5_2 )*
{
if ( state.backtracking==0 ) {
before(grammarAccess.getModuleAccess().getModuleParametersAssignment_5_2());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22307:1: ( rule__Module__ModuleParametersAssignment_5_2 )*
loop181:
do {
int alt181=2;
int LA181_0 = input.LA(1);
if ( (LA181_0==RULE_ID) ) {
alt181=1;
}
switch (alt181) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22307:2: rule__Module__ModuleParametersAssignment_5_2
{
pushFollow(FollowSets002.FOLLOW_rule__Module__ModuleParametersAssignment_5_2_in_rule__Module__Group_5__2__Impl45400);
rule__Module__ModuleParametersAssignment_5_2();
state._fsp--;
if (state.failed) return ;
}
break;
default :
break loop181;
}
} while (true);
if ( state.backtracking==0 ) {
after(grammarAccess.getModuleAccess().getModuleParametersAssignment_5_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Module__Group_5__2__Impl"
// $ANTLR start "rule__Module__Group_5__3"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22317:1: rule__Module__Group_5__3 : rule__Module__Group_5__3__Impl ;
public final void rule__Module__Group_5__3() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22321:1: ( rule__Module__Group_5__3__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22322:2: rule__Module__Group_5__3__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__Module__Group_5__3__Impl_in_rule__Module__Group_5__345431);
rule__Module__Group_5__3__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Module__Group_5__3"
// $ANTLR start "rule__Module__Group_5__3__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22328:1: rule__Module__Group_5__3__Impl : ( '}' ) ;
public final void rule__Module__Group_5__3__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22332:1: ( ( '}' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22333:1: ( '}' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22333:1: ( '}' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22334:1: '}'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getModuleAccess().getRightCurlyBracketKeyword_5_3());
}
match(input,68,FollowSets002.FOLLOW_68_in_rule__Module__Group_5__3__Impl45459); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getModuleAccess().getRightCurlyBracketKeyword_5_3());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Module__Group_5__3__Impl"
// $ANTLR start "rule__ModuleParameter__Group__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22355:1: rule__ModuleParameter__Group__0 : rule__ModuleParameter__Group__0__Impl rule__ModuleParameter__Group__1 ;
public final void rule__ModuleParameter__Group__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22359:1: ( rule__ModuleParameter__Group__0__Impl rule__ModuleParameter__Group__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22360:2: rule__ModuleParameter__Group__0__Impl rule__ModuleParameter__Group__1
{
pushFollow(FollowSets002.FOLLOW_rule__ModuleParameter__Group__0__Impl_in_rule__ModuleParameter__Group__045498);
rule__ModuleParameter__Group__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__ModuleParameter__Group__1_in_rule__ModuleParameter__Group__045501);
rule__ModuleParameter__Group__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ModuleParameter__Group__0"
// $ANTLR start "rule__ModuleParameter__Group__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22367:1: rule__ModuleParameter__Group__0__Impl : ( ( rule__ModuleParameter__ModuleDefinitionParameterAssignment_0 ) ) ;
public final void rule__ModuleParameter__Group__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22371:1: ( ( ( rule__ModuleParameter__ModuleDefinitionParameterAssignment_0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22372:1: ( ( rule__ModuleParameter__ModuleDefinitionParameterAssignment_0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22372:1: ( ( rule__ModuleParameter__ModuleDefinitionParameterAssignment_0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22373:1: ( rule__ModuleParameter__ModuleDefinitionParameterAssignment_0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getModuleParameterAccess().getModuleDefinitionParameterAssignment_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22374:1: ( rule__ModuleParameter__ModuleDefinitionParameterAssignment_0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22374:2: rule__ModuleParameter__ModuleDefinitionParameterAssignment_0
{
pushFollow(FollowSets002.FOLLOW_rule__ModuleParameter__ModuleDefinitionParameterAssignment_0_in_rule__ModuleParameter__Group__0__Impl45528);
rule__ModuleParameter__ModuleDefinitionParameterAssignment_0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getModuleParameterAccess().getModuleDefinitionParameterAssignment_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ModuleParameter__Group__0__Impl"
// $ANTLR start "rule__ModuleParameter__Group__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22384:1: rule__ModuleParameter__Group__1 : rule__ModuleParameter__Group__1__Impl rule__ModuleParameter__Group__2 ;
public final void rule__ModuleParameter__Group__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22388:1: ( rule__ModuleParameter__Group__1__Impl rule__ModuleParameter__Group__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22389:2: rule__ModuleParameter__Group__1__Impl rule__ModuleParameter__Group__2
{
pushFollow(FollowSets002.FOLLOW_rule__ModuleParameter__Group__1__Impl_in_rule__ModuleParameter__Group__145558);
rule__ModuleParameter__Group__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__ModuleParameter__Group__2_in_rule__ModuleParameter__Group__145561);
rule__ModuleParameter__Group__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ModuleParameter__Group__1"
// $ANTLR start "rule__ModuleParameter__Group__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22396:1: rule__ModuleParameter__Group__1__Impl : ( '=' ) ;
public final void rule__ModuleParameter__Group__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22400:1: ( ( '=' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22401:1: ( '=' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22401:1: ( '=' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22402:1: '='
{
if ( state.backtracking==0 ) {
before(grammarAccess.getModuleParameterAccess().getEqualsSignKeyword_1());
}
match(input,13,FollowSets002.FOLLOW_13_in_rule__ModuleParameter__Group__1__Impl45589); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getModuleParameterAccess().getEqualsSignKeyword_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ModuleParameter__Group__1__Impl"
// $ANTLR start "rule__ModuleParameter__Group__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22415:1: rule__ModuleParameter__Group__2 : rule__ModuleParameter__Group__2__Impl ;
public final void rule__ModuleParameter__Group__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22419:1: ( rule__ModuleParameter__Group__2__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22420:2: rule__ModuleParameter__Group__2__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__ModuleParameter__Group__2__Impl_in_rule__ModuleParameter__Group__245620);
rule__ModuleParameter__Group__2__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ModuleParameter__Group__2"
// $ANTLR start "rule__ModuleParameter__Group__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22426:1: rule__ModuleParameter__Group__2__Impl : ( ( rule__ModuleParameter__ValueAssignment_2 ) ) ;
public final void rule__ModuleParameter__Group__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22430:1: ( ( ( rule__ModuleParameter__ValueAssignment_2 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22431:1: ( ( rule__ModuleParameter__ValueAssignment_2 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22431:1: ( ( rule__ModuleParameter__ValueAssignment_2 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22432:1: ( rule__ModuleParameter__ValueAssignment_2 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getModuleParameterAccess().getValueAssignment_2());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22433:1: ( rule__ModuleParameter__ValueAssignment_2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22433:2: rule__ModuleParameter__ValueAssignment_2
{
pushFollow(FollowSets002.FOLLOW_rule__ModuleParameter__ValueAssignment_2_in_rule__ModuleParameter__Group__2__Impl45647);
rule__ModuleParameter__ValueAssignment_2();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getModuleParameterAccess().getValueAssignment_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ModuleParameter__Group__2__Impl"
// $ANTLR start "rule__ModuleDefinition__Group__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22449:1: rule__ModuleDefinition__Group__0 : rule__ModuleDefinition__Group__0__Impl rule__ModuleDefinition__Group__1 ;
public final void rule__ModuleDefinition__Group__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22453:1: ( rule__ModuleDefinition__Group__0__Impl rule__ModuleDefinition__Group__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22454:2: rule__ModuleDefinition__Group__0__Impl rule__ModuleDefinition__Group__1
{
pushFollow(FollowSets002.FOLLOW_rule__ModuleDefinition__Group__0__Impl_in_rule__ModuleDefinition__Group__045683);
rule__ModuleDefinition__Group__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__ModuleDefinition__Group__1_in_rule__ModuleDefinition__Group__045686);
rule__ModuleDefinition__Group__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ModuleDefinition__Group__0"
// $ANTLR start "rule__ModuleDefinition__Group__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22461:1: rule__ModuleDefinition__Group__0__Impl : ( 'moduledefinition' ) ;
public final void rule__ModuleDefinition__Group__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22465:1: ( ( 'moduledefinition' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22466:1: ( 'moduledefinition' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22466:1: ( 'moduledefinition' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22467:1: 'moduledefinition'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getModuleDefinitionAccess().getModuledefinitionKeyword_0());
}
match(input,140,FollowSets002.FOLLOW_140_in_rule__ModuleDefinition__Group__0__Impl45714); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getModuleDefinitionAccess().getModuledefinitionKeyword_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ModuleDefinition__Group__0__Impl"
// $ANTLR start "rule__ModuleDefinition__Group__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22480:1: rule__ModuleDefinition__Group__1 : rule__ModuleDefinition__Group__1__Impl rule__ModuleDefinition__Group__2 ;
public final void rule__ModuleDefinition__Group__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22484:1: ( rule__ModuleDefinition__Group__1__Impl rule__ModuleDefinition__Group__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22485:2: rule__ModuleDefinition__Group__1__Impl rule__ModuleDefinition__Group__2
{
pushFollow(FollowSets002.FOLLOW_rule__ModuleDefinition__Group__1__Impl_in_rule__ModuleDefinition__Group__145745);
rule__ModuleDefinition__Group__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__ModuleDefinition__Group__2_in_rule__ModuleDefinition__Group__145748);
rule__ModuleDefinition__Group__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ModuleDefinition__Group__1"
// $ANTLR start "rule__ModuleDefinition__Group__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22492:1: rule__ModuleDefinition__Group__1__Impl : ( ( rule__ModuleDefinition__NameAssignment_1 ) ) ;
public final void rule__ModuleDefinition__Group__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22496:1: ( ( ( rule__ModuleDefinition__NameAssignment_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22497:1: ( ( rule__ModuleDefinition__NameAssignment_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22497:1: ( ( rule__ModuleDefinition__NameAssignment_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22498:1: ( rule__ModuleDefinition__NameAssignment_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getModuleDefinitionAccess().getNameAssignment_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22499:1: ( rule__ModuleDefinition__NameAssignment_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22499:2: rule__ModuleDefinition__NameAssignment_1
{
pushFollow(FollowSets002.FOLLOW_rule__ModuleDefinition__NameAssignment_1_in_rule__ModuleDefinition__Group__1__Impl45775);
rule__ModuleDefinition__NameAssignment_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getModuleDefinitionAccess().getNameAssignment_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ModuleDefinition__Group__1__Impl"
// $ANTLR start "rule__ModuleDefinition__Group__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22509:1: rule__ModuleDefinition__Group__2 : rule__ModuleDefinition__Group__2__Impl rule__ModuleDefinition__Group__3 ;
public final void rule__ModuleDefinition__Group__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22513:1: ( rule__ModuleDefinition__Group__2__Impl rule__ModuleDefinition__Group__3 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22514:2: rule__ModuleDefinition__Group__2__Impl rule__ModuleDefinition__Group__3
{
pushFollow(FollowSets002.FOLLOW_rule__ModuleDefinition__Group__2__Impl_in_rule__ModuleDefinition__Group__245805);
rule__ModuleDefinition__Group__2__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__ModuleDefinition__Group__3_in_rule__ModuleDefinition__Group__245808);
rule__ModuleDefinition__Group__3();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ModuleDefinition__Group__2"
// $ANTLR start "rule__ModuleDefinition__Group__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22521:1: rule__ModuleDefinition__Group__2__Impl : ( '{' ) ;
public final void rule__ModuleDefinition__Group__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22525:1: ( ( '{' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22526:1: ( '{' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22526:1: ( '{' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22527:1: '{'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getModuleDefinitionAccess().getLeftCurlyBracketKeyword_2());
}
match(input,67,FollowSets002.FOLLOW_67_in_rule__ModuleDefinition__Group__2__Impl45836); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getModuleDefinitionAccess().getLeftCurlyBracketKeyword_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ModuleDefinition__Group__2__Impl"
// $ANTLR start "rule__ModuleDefinition__Group__3"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22540:1: rule__ModuleDefinition__Group__3 : rule__ModuleDefinition__Group__3__Impl rule__ModuleDefinition__Group__4 ;
public final void rule__ModuleDefinition__Group__3() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22544:1: ( rule__ModuleDefinition__Group__3__Impl rule__ModuleDefinition__Group__4 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22545:2: rule__ModuleDefinition__Group__3__Impl rule__ModuleDefinition__Group__4
{
pushFollow(FollowSets002.FOLLOW_rule__ModuleDefinition__Group__3__Impl_in_rule__ModuleDefinition__Group__345867);
rule__ModuleDefinition__Group__3__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__ModuleDefinition__Group__4_in_rule__ModuleDefinition__Group__345870);
rule__ModuleDefinition__Group__4();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ModuleDefinition__Group__3"
// $ANTLR start "rule__ModuleDefinition__Group__3__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22552:1: rule__ModuleDefinition__Group__3__Impl : ( ( rule__ModuleDefinition__Group_3__0 )? ) ;
public final void rule__ModuleDefinition__Group__3__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22556:1: ( ( ( rule__ModuleDefinition__Group_3__0 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22557:1: ( ( rule__ModuleDefinition__Group_3__0 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22557:1: ( ( rule__ModuleDefinition__Group_3__0 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22558:1: ( rule__ModuleDefinition__Group_3__0 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getModuleDefinitionAccess().getGroup_3());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22559:1: ( rule__ModuleDefinition__Group_3__0 )?
int alt182=2;
int LA182_0 = input.LA(1);
if ( (LA182_0==141) ) {
alt182=1;
}
switch (alt182) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22559:2: rule__ModuleDefinition__Group_3__0
{
pushFollow(FollowSets002.FOLLOW_rule__ModuleDefinition__Group_3__0_in_rule__ModuleDefinition__Group__3__Impl45897);
rule__ModuleDefinition__Group_3__0();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getModuleDefinitionAccess().getGroup_3());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ModuleDefinition__Group__3__Impl"
// $ANTLR start "rule__ModuleDefinition__Group__4"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22569:1: rule__ModuleDefinition__Group__4 : rule__ModuleDefinition__Group__4__Impl ;
public final void rule__ModuleDefinition__Group__4() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22573:1: ( rule__ModuleDefinition__Group__4__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22574:2: rule__ModuleDefinition__Group__4__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__ModuleDefinition__Group__4__Impl_in_rule__ModuleDefinition__Group__445928);
rule__ModuleDefinition__Group__4__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ModuleDefinition__Group__4"
// $ANTLR start "rule__ModuleDefinition__Group__4__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22580:1: rule__ModuleDefinition__Group__4__Impl : ( '}' ) ;
public final void rule__ModuleDefinition__Group__4__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22584:1: ( ( '}' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22585:1: ( '}' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22585:1: ( '}' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22586:1: '}'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getModuleDefinitionAccess().getRightCurlyBracketKeyword_4());
}
match(input,68,FollowSets002.FOLLOW_68_in_rule__ModuleDefinition__Group__4__Impl45956); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getModuleDefinitionAccess().getRightCurlyBracketKeyword_4());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ModuleDefinition__Group__4__Impl"
// $ANTLR start "rule__ModuleDefinition__Group_3__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22609:1: rule__ModuleDefinition__Group_3__0 : rule__ModuleDefinition__Group_3__0__Impl rule__ModuleDefinition__Group_3__1 ;
public final void rule__ModuleDefinition__Group_3__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22613:1: ( rule__ModuleDefinition__Group_3__0__Impl rule__ModuleDefinition__Group_3__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22614:2: rule__ModuleDefinition__Group_3__0__Impl rule__ModuleDefinition__Group_3__1
{
pushFollow(FollowSets002.FOLLOW_rule__ModuleDefinition__Group_3__0__Impl_in_rule__ModuleDefinition__Group_3__045997);
rule__ModuleDefinition__Group_3__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__ModuleDefinition__Group_3__1_in_rule__ModuleDefinition__Group_3__046000);
rule__ModuleDefinition__Group_3__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ModuleDefinition__Group_3__0"
// $ANTLR start "rule__ModuleDefinition__Group_3__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22621:1: rule__ModuleDefinition__Group_3__0__Impl : ( 'parameters' ) ;
public final void rule__ModuleDefinition__Group_3__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22625:1: ( ( 'parameters' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22626:1: ( 'parameters' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22626:1: ( 'parameters' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22627:1: 'parameters'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getModuleDefinitionAccess().getParametersKeyword_3_0());
}
match(input,141,FollowSets002.FOLLOW_141_in_rule__ModuleDefinition__Group_3__0__Impl46028); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getModuleDefinitionAccess().getParametersKeyword_3_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ModuleDefinition__Group_3__0__Impl"
// $ANTLR start "rule__ModuleDefinition__Group_3__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22640:1: rule__ModuleDefinition__Group_3__1 : rule__ModuleDefinition__Group_3__1__Impl rule__ModuleDefinition__Group_3__2 ;
public final void rule__ModuleDefinition__Group_3__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22644:1: ( rule__ModuleDefinition__Group_3__1__Impl rule__ModuleDefinition__Group_3__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22645:2: rule__ModuleDefinition__Group_3__1__Impl rule__ModuleDefinition__Group_3__2
{
pushFollow(FollowSets002.FOLLOW_rule__ModuleDefinition__Group_3__1__Impl_in_rule__ModuleDefinition__Group_3__146059);
rule__ModuleDefinition__Group_3__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__ModuleDefinition__Group_3__2_in_rule__ModuleDefinition__Group_3__146062);
rule__ModuleDefinition__Group_3__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ModuleDefinition__Group_3__1"
// $ANTLR start "rule__ModuleDefinition__Group_3__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22652:1: rule__ModuleDefinition__Group_3__1__Impl : ( '{' ) ;
public final void rule__ModuleDefinition__Group_3__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22656:1: ( ( '{' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22657:1: ( '{' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22657:1: ( '{' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22658:1: '{'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getModuleDefinitionAccess().getLeftCurlyBracketKeyword_3_1());
}
match(input,67,FollowSets002.FOLLOW_67_in_rule__ModuleDefinition__Group_3__1__Impl46090); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getModuleDefinitionAccess().getLeftCurlyBracketKeyword_3_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ModuleDefinition__Group_3__1__Impl"
// $ANTLR start "rule__ModuleDefinition__Group_3__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22671:1: rule__ModuleDefinition__Group_3__2 : rule__ModuleDefinition__Group_3__2__Impl rule__ModuleDefinition__Group_3__3 ;
public final void rule__ModuleDefinition__Group_3__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22675:1: ( rule__ModuleDefinition__Group_3__2__Impl rule__ModuleDefinition__Group_3__3 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22676:2: rule__ModuleDefinition__Group_3__2__Impl rule__ModuleDefinition__Group_3__3
{
pushFollow(FollowSets002.FOLLOW_rule__ModuleDefinition__Group_3__2__Impl_in_rule__ModuleDefinition__Group_3__246121);
rule__ModuleDefinition__Group_3__2__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__ModuleDefinition__Group_3__3_in_rule__ModuleDefinition__Group_3__246124);
rule__ModuleDefinition__Group_3__3();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ModuleDefinition__Group_3__2"
// $ANTLR start "rule__ModuleDefinition__Group_3__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22683:1: rule__ModuleDefinition__Group_3__2__Impl : ( ( rule__ModuleDefinition__ModuleDefinitionParametersAssignment_3_2 )* ) ;
public final void rule__ModuleDefinition__Group_3__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22687:1: ( ( ( rule__ModuleDefinition__ModuleDefinitionParametersAssignment_3_2 )* ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22688:1: ( ( rule__ModuleDefinition__ModuleDefinitionParametersAssignment_3_2 )* )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22688:1: ( ( rule__ModuleDefinition__ModuleDefinitionParametersAssignment_3_2 )* )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22689:1: ( rule__ModuleDefinition__ModuleDefinitionParametersAssignment_3_2 )*
{
if ( state.backtracking==0 ) {
before(grammarAccess.getModuleDefinitionAccess().getModuleDefinitionParametersAssignment_3_2());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22690:1: ( rule__ModuleDefinition__ModuleDefinitionParametersAssignment_3_2 )*
loop183:
do {
int alt183=2;
int LA183_0 = input.LA(1);
if ( (LA183_0==142) ) {
alt183=1;
}
switch (alt183) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22690:2: rule__ModuleDefinition__ModuleDefinitionParametersAssignment_3_2
{
pushFollow(FollowSets002.FOLLOW_rule__ModuleDefinition__ModuleDefinitionParametersAssignment_3_2_in_rule__ModuleDefinition__Group_3__2__Impl46151);
rule__ModuleDefinition__ModuleDefinitionParametersAssignment_3_2();
state._fsp--;
if (state.failed) return ;
}
break;
default :
break loop183;
}
} while (true);
if ( state.backtracking==0 ) {
after(grammarAccess.getModuleDefinitionAccess().getModuleDefinitionParametersAssignment_3_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ModuleDefinition__Group_3__2__Impl"
// $ANTLR start "rule__ModuleDefinition__Group_3__3"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22700:1: rule__ModuleDefinition__Group_3__3 : rule__ModuleDefinition__Group_3__3__Impl ;
public final void rule__ModuleDefinition__Group_3__3() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22704:1: ( rule__ModuleDefinition__Group_3__3__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22705:2: rule__ModuleDefinition__Group_3__3__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__ModuleDefinition__Group_3__3__Impl_in_rule__ModuleDefinition__Group_3__346182);
rule__ModuleDefinition__Group_3__3__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ModuleDefinition__Group_3__3"
// $ANTLR start "rule__ModuleDefinition__Group_3__3__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22711:1: rule__ModuleDefinition__Group_3__3__Impl : ( '}' ) ;
public final void rule__ModuleDefinition__Group_3__3__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22715:1: ( ( '}' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22716:1: ( '}' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22716:1: ( '}' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22717:1: '}'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getModuleDefinitionAccess().getRightCurlyBracketKeyword_3_3());
}
match(input,68,FollowSets002.FOLLOW_68_in_rule__ModuleDefinition__Group_3__3__Impl46210); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getModuleDefinitionAccess().getRightCurlyBracketKeyword_3_3());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ModuleDefinition__Group_3__3__Impl"
// $ANTLR start "rule__ModuleDefinitionParameter__Group__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22738:1: rule__ModuleDefinitionParameter__Group__0 : rule__ModuleDefinitionParameter__Group__0__Impl rule__ModuleDefinitionParameter__Group__1 ;
public final void rule__ModuleDefinitionParameter__Group__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22742:1: ( rule__ModuleDefinitionParameter__Group__0__Impl rule__ModuleDefinitionParameter__Group__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22743:2: rule__ModuleDefinitionParameter__Group__0__Impl rule__ModuleDefinitionParameter__Group__1
{
pushFollow(FollowSets002.FOLLOW_rule__ModuleDefinitionParameter__Group__0__Impl_in_rule__ModuleDefinitionParameter__Group__046249);
rule__ModuleDefinitionParameter__Group__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__ModuleDefinitionParameter__Group__1_in_rule__ModuleDefinitionParameter__Group__046252);
rule__ModuleDefinitionParameter__Group__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ModuleDefinitionParameter__Group__0"
// $ANTLR start "rule__ModuleDefinitionParameter__Group__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22750:1: rule__ModuleDefinitionParameter__Group__0__Impl : ( 'parameter' ) ;
public final void rule__ModuleDefinitionParameter__Group__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22754:1: ( ( 'parameter' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22755:1: ( 'parameter' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22755:1: ( 'parameter' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22756:1: 'parameter'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getModuleDefinitionParameterAccess().getParameterKeyword_0());
}
match(input,142,FollowSets002.FOLLOW_142_in_rule__ModuleDefinitionParameter__Group__0__Impl46280); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getModuleDefinitionParameterAccess().getParameterKeyword_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ModuleDefinitionParameter__Group__0__Impl"
// $ANTLR start "rule__ModuleDefinitionParameter__Group__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22769:1: rule__ModuleDefinitionParameter__Group__1 : rule__ModuleDefinitionParameter__Group__1__Impl rule__ModuleDefinitionParameter__Group__2 ;
public final void rule__ModuleDefinitionParameter__Group__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22773:1: ( rule__ModuleDefinitionParameter__Group__1__Impl rule__ModuleDefinitionParameter__Group__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22774:2: rule__ModuleDefinitionParameter__Group__1__Impl rule__ModuleDefinitionParameter__Group__2
{
pushFollow(FollowSets002.FOLLOW_rule__ModuleDefinitionParameter__Group__1__Impl_in_rule__ModuleDefinitionParameter__Group__146311);
rule__ModuleDefinitionParameter__Group__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__ModuleDefinitionParameter__Group__2_in_rule__ModuleDefinitionParameter__Group__146314);
rule__ModuleDefinitionParameter__Group__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ModuleDefinitionParameter__Group__1"
// $ANTLR start "rule__ModuleDefinitionParameter__Group__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22781:1: rule__ModuleDefinitionParameter__Group__1__Impl : ( ( rule__ModuleDefinitionParameter__NameAssignment_1 ) ) ;
public final void rule__ModuleDefinitionParameter__Group__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22785:1: ( ( ( rule__ModuleDefinitionParameter__NameAssignment_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22786:1: ( ( rule__ModuleDefinitionParameter__NameAssignment_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22786:1: ( ( rule__ModuleDefinitionParameter__NameAssignment_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22787:1: ( rule__ModuleDefinitionParameter__NameAssignment_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getModuleDefinitionParameterAccess().getNameAssignment_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22788:1: ( rule__ModuleDefinitionParameter__NameAssignment_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22788:2: rule__ModuleDefinitionParameter__NameAssignment_1
{
pushFollow(FollowSets002.FOLLOW_rule__ModuleDefinitionParameter__NameAssignment_1_in_rule__ModuleDefinitionParameter__Group__1__Impl46341);
rule__ModuleDefinitionParameter__NameAssignment_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getModuleDefinitionParameterAccess().getNameAssignment_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ModuleDefinitionParameter__Group__1__Impl"
// $ANTLR start "rule__ModuleDefinitionParameter__Group__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22798:1: rule__ModuleDefinitionParameter__Group__2 : rule__ModuleDefinitionParameter__Group__2__Impl rule__ModuleDefinitionParameter__Group__3 ;
public final void rule__ModuleDefinitionParameter__Group__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22802:1: ( rule__ModuleDefinitionParameter__Group__2__Impl rule__ModuleDefinitionParameter__Group__3 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22803:2: rule__ModuleDefinitionParameter__Group__2__Impl rule__ModuleDefinitionParameter__Group__3
{
pushFollow(FollowSets002.FOLLOW_rule__ModuleDefinitionParameter__Group__2__Impl_in_rule__ModuleDefinitionParameter__Group__246371);
rule__ModuleDefinitionParameter__Group__2__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__ModuleDefinitionParameter__Group__3_in_rule__ModuleDefinitionParameter__Group__246374);
rule__ModuleDefinitionParameter__Group__3();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ModuleDefinitionParameter__Group__2"
// $ANTLR start "rule__ModuleDefinitionParameter__Group__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22810:1: rule__ModuleDefinitionParameter__Group__2__Impl : ( '{' ) ;
public final void rule__ModuleDefinitionParameter__Group__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22814:1: ( ( '{' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22815:1: ( '{' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22815:1: ( '{' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22816:1: '{'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getModuleDefinitionParameterAccess().getLeftCurlyBracketKeyword_2());
}
match(input,67,FollowSets002.FOLLOW_67_in_rule__ModuleDefinitionParameter__Group__2__Impl46402); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getModuleDefinitionParameterAccess().getLeftCurlyBracketKeyword_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ModuleDefinitionParameter__Group__2__Impl"
// $ANTLR start "rule__ModuleDefinitionParameter__Group__3"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22829:1: rule__ModuleDefinitionParameter__Group__3 : rule__ModuleDefinitionParameter__Group__3__Impl rule__ModuleDefinitionParameter__Group__4 ;
public final void rule__ModuleDefinitionParameter__Group__3() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22833:1: ( rule__ModuleDefinitionParameter__Group__3__Impl rule__ModuleDefinitionParameter__Group__4 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22834:2: rule__ModuleDefinitionParameter__Group__3__Impl rule__ModuleDefinitionParameter__Group__4
{
pushFollow(FollowSets002.FOLLOW_rule__ModuleDefinitionParameter__Group__3__Impl_in_rule__ModuleDefinitionParameter__Group__346433);
rule__ModuleDefinitionParameter__Group__3__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__ModuleDefinitionParameter__Group__4_in_rule__ModuleDefinitionParameter__Group__346436);
rule__ModuleDefinitionParameter__Group__4();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ModuleDefinitionParameter__Group__3"
// $ANTLR start "rule__ModuleDefinitionParameter__Group__3__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22841:1: rule__ModuleDefinitionParameter__Group__3__Impl : ( 'type' ) ;
public final void rule__ModuleDefinitionParameter__Group__3__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22845:1: ( ( 'type' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22846:1: ( 'type' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22846:1: ( 'type' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22847:1: 'type'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getModuleDefinitionParameterAccess().getTypeKeyword_3());
}
match(input,121,FollowSets002.FOLLOW_121_in_rule__ModuleDefinitionParameter__Group__3__Impl46464); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getModuleDefinitionParameterAccess().getTypeKeyword_3());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ModuleDefinitionParameter__Group__3__Impl"
// $ANTLR start "rule__ModuleDefinitionParameter__Group__4"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22860:1: rule__ModuleDefinitionParameter__Group__4 : rule__ModuleDefinitionParameter__Group__4__Impl rule__ModuleDefinitionParameter__Group__5 ;
public final void rule__ModuleDefinitionParameter__Group__4() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22864:1: ( rule__ModuleDefinitionParameter__Group__4__Impl rule__ModuleDefinitionParameter__Group__5 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22865:2: rule__ModuleDefinitionParameter__Group__4__Impl rule__ModuleDefinitionParameter__Group__5
{
pushFollow(FollowSets002.FOLLOW_rule__ModuleDefinitionParameter__Group__4__Impl_in_rule__ModuleDefinitionParameter__Group__446495);
rule__ModuleDefinitionParameter__Group__4__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__ModuleDefinitionParameter__Group__5_in_rule__ModuleDefinitionParameter__Group__446498);
rule__ModuleDefinitionParameter__Group__5();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ModuleDefinitionParameter__Group__4"
// $ANTLR start "rule__ModuleDefinitionParameter__Group__4__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22872:1: rule__ModuleDefinitionParameter__Group__4__Impl : ( ( rule__ModuleDefinitionParameter__TypeAssignment_4 ) ) ;
public final void rule__ModuleDefinitionParameter__Group__4__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22876:1: ( ( ( rule__ModuleDefinitionParameter__TypeAssignment_4 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22877:1: ( ( rule__ModuleDefinitionParameter__TypeAssignment_4 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22877:1: ( ( rule__ModuleDefinitionParameter__TypeAssignment_4 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22878:1: ( rule__ModuleDefinitionParameter__TypeAssignment_4 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getModuleDefinitionParameterAccess().getTypeAssignment_4());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22879:1: ( rule__ModuleDefinitionParameter__TypeAssignment_4 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22879:2: rule__ModuleDefinitionParameter__TypeAssignment_4
{
pushFollow(FollowSets002.FOLLOW_rule__ModuleDefinitionParameter__TypeAssignment_4_in_rule__ModuleDefinitionParameter__Group__4__Impl46525);
rule__ModuleDefinitionParameter__TypeAssignment_4();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getModuleDefinitionParameterAccess().getTypeAssignment_4());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ModuleDefinitionParameter__Group__4__Impl"
// $ANTLR start "rule__ModuleDefinitionParameter__Group__5"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22889:1: rule__ModuleDefinitionParameter__Group__5 : rule__ModuleDefinitionParameter__Group__5__Impl ;
public final void rule__ModuleDefinitionParameter__Group__5() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22893:1: ( rule__ModuleDefinitionParameter__Group__5__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22894:2: rule__ModuleDefinitionParameter__Group__5__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__ModuleDefinitionParameter__Group__5__Impl_in_rule__ModuleDefinitionParameter__Group__546555);
rule__ModuleDefinitionParameter__Group__5__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ModuleDefinitionParameter__Group__5"
// $ANTLR start "rule__ModuleDefinitionParameter__Group__5__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22900:1: rule__ModuleDefinitionParameter__Group__5__Impl : ( '}' ) ;
public final void rule__ModuleDefinitionParameter__Group__5__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22904:1: ( ( '}' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22905:1: ( '}' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22905:1: ( '}' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22906:1: '}'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getModuleDefinitionParameterAccess().getRightCurlyBracketKeyword_5());
}
match(input,68,FollowSets002.FOLLOW_68_in_rule__ModuleDefinitionParameter__Group__5__Impl46583); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getModuleDefinitionParameterAccess().getRightCurlyBracketKeyword_5());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ModuleDefinitionParameter__Group__5__Impl"
// $ANTLR start "rule__ServiceOptions__Group__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22931:1: rule__ServiceOptions__Group__0 : rule__ServiceOptions__Group__0__Impl rule__ServiceOptions__Group__1 ;
public final void rule__ServiceOptions__Group__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22935:1: ( rule__ServiceOptions__Group__0__Impl rule__ServiceOptions__Group__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22936:2: rule__ServiceOptions__Group__0__Impl rule__ServiceOptions__Group__1
{
pushFollow(FollowSets002.FOLLOW_rule__ServiceOptions__Group__0__Impl_in_rule__ServiceOptions__Group__046626);
rule__ServiceOptions__Group__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__ServiceOptions__Group__1_in_rule__ServiceOptions__Group__046629);
rule__ServiceOptions__Group__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ServiceOptions__Group__0"
// $ANTLR start "rule__ServiceOptions__Group__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22943:1: rule__ServiceOptions__Group__0__Impl : ( () ) ;
public final void rule__ServiceOptions__Group__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22947:1: ( ( () ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22948:1: ( () )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22948:1: ( () )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22949:1: ()
{
if ( state.backtracking==0 ) {
before(grammarAccess.getServiceOptionsAccess().getServiceOptionsAction_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22950:1: ()
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22952:1:
{
}
if ( state.backtracking==0 ) {
after(grammarAccess.getServiceOptionsAccess().getServiceOptionsAction_0());
}
}
}
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ServiceOptions__Group__0__Impl"
// $ANTLR start "rule__ServiceOptions__Group__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22962:1: rule__ServiceOptions__Group__1 : rule__ServiceOptions__Group__1__Impl ;
public final void rule__ServiceOptions__Group__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22966:1: ( rule__ServiceOptions__Group__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22967:2: rule__ServiceOptions__Group__1__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__ServiceOptions__Group__1__Impl_in_rule__ServiceOptions__Group__146687);
rule__ServiceOptions__Group__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ServiceOptions__Group__1"
// $ANTLR start "rule__ServiceOptions__Group__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22973:1: rule__ServiceOptions__Group__1__Impl : ( ( rule__ServiceOptions__NonpublicAssignment_1 )? ) ;
public final void rule__ServiceOptions__Group__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22977:1: ( ( ( rule__ServiceOptions__NonpublicAssignment_1 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22978:1: ( ( rule__ServiceOptions__NonpublicAssignment_1 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22978:1: ( ( rule__ServiceOptions__NonpublicAssignment_1 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22979:1: ( rule__ServiceOptions__NonpublicAssignment_1 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getServiceOptionsAccess().getNonpublicAssignment_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22980:1: ( rule__ServiceOptions__NonpublicAssignment_1 )?
int alt184=2;
int LA184_0 = input.LA(1);
if ( (LA184_0==180) ) {
alt184=1;
}
switch (alt184) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22980:2: rule__ServiceOptions__NonpublicAssignment_1
{
pushFollow(FollowSets002.FOLLOW_rule__ServiceOptions__NonpublicAssignment_1_in_rule__ServiceOptions__Group__1__Impl46714);
rule__ServiceOptions__NonpublicAssignment_1();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getServiceOptionsAccess().getNonpublicAssignment_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ServiceOptions__Group__1__Impl"
// $ANTLR start "rule__ServiceMethod__Group__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22994:1: rule__ServiceMethod__Group__0 : rule__ServiceMethod__Group__0__Impl rule__ServiceMethod__Group__1 ;
public final void rule__ServiceMethod__Group__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22998:1: ( rule__ServiceMethod__Group__0__Impl rule__ServiceMethod__Group__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:22999:2: rule__ServiceMethod__Group__0__Impl rule__ServiceMethod__Group__1
{
pushFollow(FollowSets002.FOLLOW_rule__ServiceMethod__Group__0__Impl_in_rule__ServiceMethod__Group__046749);
rule__ServiceMethod__Group__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__ServiceMethod__Group__1_in_rule__ServiceMethod__Group__046752);
rule__ServiceMethod__Group__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ServiceMethod__Group__0"
// $ANTLR start "rule__ServiceMethod__Group__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23006:1: rule__ServiceMethod__Group__0__Impl : ( 'method' ) ;
public final void rule__ServiceMethod__Group__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23010:1: ( ( 'method' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23011:1: ( 'method' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23011:1: ( 'method' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23012:1: 'method'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getServiceMethodAccess().getMethodKeyword_0());
}
match(input,143,FollowSets002.FOLLOW_143_in_rule__ServiceMethod__Group__0__Impl46780); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getServiceMethodAccess().getMethodKeyword_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ServiceMethod__Group__0__Impl"
// $ANTLR start "rule__ServiceMethod__Group__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23025:1: rule__ServiceMethod__Group__1 : rule__ServiceMethod__Group__1__Impl rule__ServiceMethod__Group__2 ;
public final void rule__ServiceMethod__Group__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23029:1: ( rule__ServiceMethod__Group__1__Impl rule__ServiceMethod__Group__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23030:2: rule__ServiceMethod__Group__1__Impl rule__ServiceMethod__Group__2
{
pushFollow(FollowSets002.FOLLOW_rule__ServiceMethod__Group__1__Impl_in_rule__ServiceMethod__Group__146811);
rule__ServiceMethod__Group__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__ServiceMethod__Group__2_in_rule__ServiceMethod__Group__146814);
rule__ServiceMethod__Group__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ServiceMethod__Group__1"
// $ANTLR start "rule__ServiceMethod__Group__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23037:1: rule__ServiceMethod__Group__1__Impl : ( ( rule__ServiceMethod__Group_1__0 )? ) ;
public final void rule__ServiceMethod__Group__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23041:1: ( ( ( rule__ServiceMethod__Group_1__0 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23042:1: ( ( rule__ServiceMethod__Group_1__0 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23042:1: ( ( rule__ServiceMethod__Group_1__0 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23043:1: ( rule__ServiceMethod__Group_1__0 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getServiceMethodAccess().getGroup_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23044:1: ( rule__ServiceMethod__Group_1__0 )?
int alt185=2;
int LA185_0 = input.LA(1);
if ( (LA185_0==27) ) {
alt185=1;
}
switch (alt185) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23044:2: rule__ServiceMethod__Group_1__0
{
pushFollow(FollowSets002.FOLLOW_rule__ServiceMethod__Group_1__0_in_rule__ServiceMethod__Group__1__Impl46841);
rule__ServiceMethod__Group_1__0();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getServiceMethodAccess().getGroup_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ServiceMethod__Group__1__Impl"
// $ANTLR start "rule__ServiceMethod__Group__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23054:1: rule__ServiceMethod__Group__2 : rule__ServiceMethod__Group__2__Impl rule__ServiceMethod__Group__3 ;
public final void rule__ServiceMethod__Group__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23058:1: ( rule__ServiceMethod__Group__2__Impl rule__ServiceMethod__Group__3 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23059:2: rule__ServiceMethod__Group__2__Impl rule__ServiceMethod__Group__3
{
pushFollow(FollowSets002.FOLLOW_rule__ServiceMethod__Group__2__Impl_in_rule__ServiceMethod__Group__246872);
rule__ServiceMethod__Group__2__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__ServiceMethod__Group__3_in_rule__ServiceMethod__Group__246875);
rule__ServiceMethod__Group__3();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ServiceMethod__Group__2"
// $ANTLR start "rule__ServiceMethod__Group__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23066:1: rule__ServiceMethod__Group__2__Impl : ( ( rule__ServiceMethod__ReturnTypeAssignment_2 ) ) ;
public final void rule__ServiceMethod__Group__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23070:1: ( ( ( rule__ServiceMethod__ReturnTypeAssignment_2 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23071:1: ( ( rule__ServiceMethod__ReturnTypeAssignment_2 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23071:1: ( ( rule__ServiceMethod__ReturnTypeAssignment_2 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23072:1: ( rule__ServiceMethod__ReturnTypeAssignment_2 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getServiceMethodAccess().getReturnTypeAssignment_2());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23073:1: ( rule__ServiceMethod__ReturnTypeAssignment_2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23073:2: rule__ServiceMethod__ReturnTypeAssignment_2
{
pushFollow(FollowSets002.FOLLOW_rule__ServiceMethod__ReturnTypeAssignment_2_in_rule__ServiceMethod__Group__2__Impl46902);
rule__ServiceMethod__ReturnTypeAssignment_2();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getServiceMethodAccess().getReturnTypeAssignment_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ServiceMethod__Group__2__Impl"
// $ANTLR start "rule__ServiceMethod__Group__3"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23083:1: rule__ServiceMethod__Group__3 : rule__ServiceMethod__Group__3__Impl rule__ServiceMethod__Group__4 ;
public final void rule__ServiceMethod__Group__3() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23087:1: ( rule__ServiceMethod__Group__3__Impl rule__ServiceMethod__Group__4 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23088:2: rule__ServiceMethod__Group__3__Impl rule__ServiceMethod__Group__4
{
pushFollow(FollowSets002.FOLLOW_rule__ServiceMethod__Group__3__Impl_in_rule__ServiceMethod__Group__346932);
rule__ServiceMethod__Group__3__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__ServiceMethod__Group__4_in_rule__ServiceMethod__Group__346935);
rule__ServiceMethod__Group__4();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ServiceMethod__Group__3"
// $ANTLR start "rule__ServiceMethod__Group__3__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23095:1: rule__ServiceMethod__Group__3__Impl : ( ( rule__ServiceMethod__NameAssignment_3 ) ) ;
public final void rule__ServiceMethod__Group__3__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23099:1: ( ( ( rule__ServiceMethod__NameAssignment_3 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23100:1: ( ( rule__ServiceMethod__NameAssignment_3 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23100:1: ( ( rule__ServiceMethod__NameAssignment_3 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23101:1: ( rule__ServiceMethod__NameAssignment_3 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getServiceMethodAccess().getNameAssignment_3());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23102:1: ( rule__ServiceMethod__NameAssignment_3 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23102:2: rule__ServiceMethod__NameAssignment_3
{
pushFollow(FollowSets002.FOLLOW_rule__ServiceMethod__NameAssignment_3_in_rule__ServiceMethod__Group__3__Impl46962);
rule__ServiceMethod__NameAssignment_3();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getServiceMethodAccess().getNameAssignment_3());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ServiceMethod__Group__3__Impl"
// $ANTLR start "rule__ServiceMethod__Group__4"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23112:1: rule__ServiceMethod__Group__4 : rule__ServiceMethod__Group__4__Impl rule__ServiceMethod__Group__5 ;
public final void rule__ServiceMethod__Group__4() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23116:1: ( rule__ServiceMethod__Group__4__Impl rule__ServiceMethod__Group__5 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23117:2: rule__ServiceMethod__Group__4__Impl rule__ServiceMethod__Group__5
{
pushFollow(FollowSets002.FOLLOW_rule__ServiceMethod__Group__4__Impl_in_rule__ServiceMethod__Group__446992);
rule__ServiceMethod__Group__4__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__ServiceMethod__Group__5_in_rule__ServiceMethod__Group__446995);
rule__ServiceMethod__Group__5();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ServiceMethod__Group__4"
// $ANTLR start "rule__ServiceMethod__Group__4__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23124:1: rule__ServiceMethod__Group__4__Impl : ( '(' ) ;
public final void rule__ServiceMethod__Group__4__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23128:1: ( ( '(' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23129:1: ( '(' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23129:1: ( '(' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23130:1: '('
{
if ( state.backtracking==0 ) {
before(grammarAccess.getServiceMethodAccess().getLeftParenthesisKeyword_4());
}
match(input,144,FollowSets002.FOLLOW_144_in_rule__ServiceMethod__Group__4__Impl47023); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getServiceMethodAccess().getLeftParenthesisKeyword_4());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ServiceMethod__Group__4__Impl"
// $ANTLR start "rule__ServiceMethod__Group__5"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23143:1: rule__ServiceMethod__Group__5 : rule__ServiceMethod__Group__5__Impl rule__ServiceMethod__Group__6 ;
public final void rule__ServiceMethod__Group__5() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23147:1: ( rule__ServiceMethod__Group__5__Impl rule__ServiceMethod__Group__6 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23148:2: rule__ServiceMethod__Group__5__Impl rule__ServiceMethod__Group__6
{
pushFollow(FollowSets002.FOLLOW_rule__ServiceMethod__Group__5__Impl_in_rule__ServiceMethod__Group__547054);
rule__ServiceMethod__Group__5__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__ServiceMethod__Group__6_in_rule__ServiceMethod__Group__547057);
rule__ServiceMethod__Group__6();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ServiceMethod__Group__5"
// $ANTLR start "rule__ServiceMethod__Group__5__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23155:1: rule__ServiceMethod__Group__5__Impl : ( ( rule__ServiceMethod__Group_5__0 )? ) ;
public final void rule__ServiceMethod__Group__5__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23159:1: ( ( ( rule__ServiceMethod__Group_5__0 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23160:1: ( ( rule__ServiceMethod__Group_5__0 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23160:1: ( ( rule__ServiceMethod__Group_5__0 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23161:1: ( rule__ServiceMethod__Group_5__0 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getServiceMethodAccess().getGroup_5());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23162:1: ( rule__ServiceMethod__Group_5__0 )?
int alt186=2;
int LA186_0 = input.LA(1);
if ( (LA186_0==RULE_ID||LA186_0==31||LA186_0==144) ) {
alt186=1;
}
switch (alt186) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23162:2: rule__ServiceMethod__Group_5__0
{
pushFollow(FollowSets002.FOLLOW_rule__ServiceMethod__Group_5__0_in_rule__ServiceMethod__Group__5__Impl47084);
rule__ServiceMethod__Group_5__0();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getServiceMethodAccess().getGroup_5());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ServiceMethod__Group__5__Impl"
// $ANTLR start "rule__ServiceMethod__Group__6"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23172:1: rule__ServiceMethod__Group__6 : rule__ServiceMethod__Group__6__Impl ;
public final void rule__ServiceMethod__Group__6() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23176:1: ( rule__ServiceMethod__Group__6__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23177:2: rule__ServiceMethod__Group__6__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__ServiceMethod__Group__6__Impl_in_rule__ServiceMethod__Group__647115);
rule__ServiceMethod__Group__6__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ServiceMethod__Group__6"
// $ANTLR start "rule__ServiceMethod__Group__6__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23183:1: rule__ServiceMethod__Group__6__Impl : ( ')' ) ;
public final void rule__ServiceMethod__Group__6__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23187:1: ( ( ')' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23188:1: ( ')' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23188:1: ( ')' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23189:1: ')'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getServiceMethodAccess().getRightParenthesisKeyword_6());
}
match(input,145,FollowSets002.FOLLOW_145_in_rule__ServiceMethod__Group__6__Impl47143); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getServiceMethodAccess().getRightParenthesisKeyword_6());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ServiceMethod__Group__6__Impl"
// $ANTLR start "rule__ServiceMethod__Group_1__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23216:1: rule__ServiceMethod__Group_1__0 : rule__ServiceMethod__Group_1__0__Impl rule__ServiceMethod__Group_1__1 ;
public final void rule__ServiceMethod__Group_1__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23220:1: ( rule__ServiceMethod__Group_1__0__Impl rule__ServiceMethod__Group_1__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23221:2: rule__ServiceMethod__Group_1__0__Impl rule__ServiceMethod__Group_1__1
{
pushFollow(FollowSets002.FOLLOW_rule__ServiceMethod__Group_1__0__Impl_in_rule__ServiceMethod__Group_1__047188);
rule__ServiceMethod__Group_1__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__ServiceMethod__Group_1__1_in_rule__ServiceMethod__Group_1__047191);
rule__ServiceMethod__Group_1__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ServiceMethod__Group_1__0"
// $ANTLR start "rule__ServiceMethod__Group_1__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23228:1: rule__ServiceMethod__Group_1__0__Impl : ( '<' ) ;
public final void rule__ServiceMethod__Group_1__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23232:1: ( ( '<' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23233:1: ( '<' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23233:1: ( '<' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23234:1: '<'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getServiceMethodAccess().getLessThanSignKeyword_1_0());
}
match(input,27,FollowSets002.FOLLOW_27_in_rule__ServiceMethod__Group_1__0__Impl47219); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getServiceMethodAccess().getLessThanSignKeyword_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ServiceMethod__Group_1__0__Impl"
// $ANTLR start "rule__ServiceMethod__Group_1__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23247:1: rule__ServiceMethod__Group_1__1 : rule__ServiceMethod__Group_1__1__Impl rule__ServiceMethod__Group_1__2 ;
public final void rule__ServiceMethod__Group_1__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23251:1: ( rule__ServiceMethod__Group_1__1__Impl rule__ServiceMethod__Group_1__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23252:2: rule__ServiceMethod__Group_1__1__Impl rule__ServiceMethod__Group_1__2
{
pushFollow(FollowSets002.FOLLOW_rule__ServiceMethod__Group_1__1__Impl_in_rule__ServiceMethod__Group_1__147250);
rule__ServiceMethod__Group_1__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__ServiceMethod__Group_1__2_in_rule__ServiceMethod__Group_1__147253);
rule__ServiceMethod__Group_1__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ServiceMethod__Group_1__1"
// $ANTLR start "rule__ServiceMethod__Group_1__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23259:1: rule__ServiceMethod__Group_1__1__Impl : ( ( rule__ServiceMethod__TypeParameterAssignment_1_1 ) ) ;
public final void rule__ServiceMethod__Group_1__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23263:1: ( ( ( rule__ServiceMethod__TypeParameterAssignment_1_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23264:1: ( ( rule__ServiceMethod__TypeParameterAssignment_1_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23264:1: ( ( rule__ServiceMethod__TypeParameterAssignment_1_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23265:1: ( rule__ServiceMethod__TypeParameterAssignment_1_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getServiceMethodAccess().getTypeParameterAssignment_1_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23266:1: ( rule__ServiceMethod__TypeParameterAssignment_1_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23266:2: rule__ServiceMethod__TypeParameterAssignment_1_1
{
pushFollow(FollowSets002.FOLLOW_rule__ServiceMethod__TypeParameterAssignment_1_1_in_rule__ServiceMethod__Group_1__1__Impl47280);
rule__ServiceMethod__TypeParameterAssignment_1_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getServiceMethodAccess().getTypeParameterAssignment_1_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ServiceMethod__Group_1__1__Impl"
// $ANTLR start "rule__ServiceMethod__Group_1__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23276:1: rule__ServiceMethod__Group_1__2 : rule__ServiceMethod__Group_1__2__Impl ;
public final void rule__ServiceMethod__Group_1__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23280:1: ( rule__ServiceMethod__Group_1__2__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23281:2: rule__ServiceMethod__Group_1__2__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__ServiceMethod__Group_1__2__Impl_in_rule__ServiceMethod__Group_1__247310);
rule__ServiceMethod__Group_1__2__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ServiceMethod__Group_1__2"
// $ANTLR start "rule__ServiceMethod__Group_1__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23287:1: rule__ServiceMethod__Group_1__2__Impl : ( '>' ) ;
public final void rule__ServiceMethod__Group_1__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23291:1: ( ( '>' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23292:1: ( '>' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23292:1: ( '>' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23293:1: '>'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getServiceMethodAccess().getGreaterThanSignKeyword_1_2());
}
match(input,26,FollowSets002.FOLLOW_26_in_rule__ServiceMethod__Group_1__2__Impl47338); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getServiceMethodAccess().getGreaterThanSignKeyword_1_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ServiceMethod__Group_1__2__Impl"
// $ANTLR start "rule__ServiceMethod__Group_5__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23312:1: rule__ServiceMethod__Group_5__0 : rule__ServiceMethod__Group_5__0__Impl rule__ServiceMethod__Group_5__1 ;
public final void rule__ServiceMethod__Group_5__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23316:1: ( rule__ServiceMethod__Group_5__0__Impl rule__ServiceMethod__Group_5__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23317:2: rule__ServiceMethod__Group_5__0__Impl rule__ServiceMethod__Group_5__1
{
pushFollow(FollowSets002.FOLLOW_rule__ServiceMethod__Group_5__0__Impl_in_rule__ServiceMethod__Group_5__047375);
rule__ServiceMethod__Group_5__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__ServiceMethod__Group_5__1_in_rule__ServiceMethod__Group_5__047378);
rule__ServiceMethod__Group_5__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ServiceMethod__Group_5__0"
// $ANTLR start "rule__ServiceMethod__Group_5__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23324:1: rule__ServiceMethod__Group_5__0__Impl : ( ( rule__ServiceMethod__ParamsAssignment_5_0 ) ) ;
public final void rule__ServiceMethod__Group_5__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23328:1: ( ( ( rule__ServiceMethod__ParamsAssignment_5_0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23329:1: ( ( rule__ServiceMethod__ParamsAssignment_5_0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23329:1: ( ( rule__ServiceMethod__ParamsAssignment_5_0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23330:1: ( rule__ServiceMethod__ParamsAssignment_5_0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getServiceMethodAccess().getParamsAssignment_5_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23331:1: ( rule__ServiceMethod__ParamsAssignment_5_0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23331:2: rule__ServiceMethod__ParamsAssignment_5_0
{
pushFollow(FollowSets002.FOLLOW_rule__ServiceMethod__ParamsAssignment_5_0_in_rule__ServiceMethod__Group_5__0__Impl47405);
rule__ServiceMethod__ParamsAssignment_5_0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getServiceMethodAccess().getParamsAssignment_5_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ServiceMethod__Group_5__0__Impl"
// $ANTLR start "rule__ServiceMethod__Group_5__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23341:1: rule__ServiceMethod__Group_5__1 : rule__ServiceMethod__Group_5__1__Impl ;
public final void rule__ServiceMethod__Group_5__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23345:1: ( rule__ServiceMethod__Group_5__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23346:2: rule__ServiceMethod__Group_5__1__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__ServiceMethod__Group_5__1__Impl_in_rule__ServiceMethod__Group_5__147435);
rule__ServiceMethod__Group_5__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ServiceMethod__Group_5__1"
// $ANTLR start "rule__ServiceMethod__Group_5__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23352:1: rule__ServiceMethod__Group_5__1__Impl : ( ( rule__ServiceMethod__Group_5_1__0 )* ) ;
public final void rule__ServiceMethod__Group_5__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23356:1: ( ( ( rule__ServiceMethod__Group_5_1__0 )* ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23357:1: ( ( rule__ServiceMethod__Group_5_1__0 )* )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23357:1: ( ( rule__ServiceMethod__Group_5_1__0 )* )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23358:1: ( rule__ServiceMethod__Group_5_1__0 )*
{
if ( state.backtracking==0 ) {
before(grammarAccess.getServiceMethodAccess().getGroup_5_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23359:1: ( rule__ServiceMethod__Group_5_1__0 )*
loop187:
do {
int alt187=2;
int LA187_0 = input.LA(1);
if ( (LA187_0==72) ) {
alt187=1;
}
switch (alt187) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23359:2: rule__ServiceMethod__Group_5_1__0
{
pushFollow(FollowSets002.FOLLOW_rule__ServiceMethod__Group_5_1__0_in_rule__ServiceMethod__Group_5__1__Impl47462);
rule__ServiceMethod__Group_5_1__0();
state._fsp--;
if (state.failed) return ;
}
break;
default :
break loop187;
}
} while (true);
if ( state.backtracking==0 ) {
after(grammarAccess.getServiceMethodAccess().getGroup_5_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ServiceMethod__Group_5__1__Impl"
// $ANTLR start "rule__ServiceMethod__Group_5_1__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23373:1: rule__ServiceMethod__Group_5_1__0 : rule__ServiceMethod__Group_5_1__0__Impl rule__ServiceMethod__Group_5_1__1 ;
public final void rule__ServiceMethod__Group_5_1__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23377:1: ( rule__ServiceMethod__Group_5_1__0__Impl rule__ServiceMethod__Group_5_1__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23378:2: rule__ServiceMethod__Group_5_1__0__Impl rule__ServiceMethod__Group_5_1__1
{
pushFollow(FollowSets002.FOLLOW_rule__ServiceMethod__Group_5_1__0__Impl_in_rule__ServiceMethod__Group_5_1__047497);
rule__ServiceMethod__Group_5_1__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__ServiceMethod__Group_5_1__1_in_rule__ServiceMethod__Group_5_1__047500);
rule__ServiceMethod__Group_5_1__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ServiceMethod__Group_5_1__0"
// $ANTLR start "rule__ServiceMethod__Group_5_1__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23385:1: rule__ServiceMethod__Group_5_1__0__Impl : ( ', ' ) ;
public final void rule__ServiceMethod__Group_5_1__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23389:1: ( ( ', ' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23390:1: ( ', ' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23390:1: ( ', ' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23391:1: ', '
{
if ( state.backtracking==0 ) {
before(grammarAccess.getServiceMethodAccess().getCommaSpaceKeyword_5_1_0());
}
match(input,72,FollowSets002.FOLLOW_72_in_rule__ServiceMethod__Group_5_1__0__Impl47528); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getServiceMethodAccess().getCommaSpaceKeyword_5_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ServiceMethod__Group_5_1__0__Impl"
// $ANTLR start "rule__ServiceMethod__Group_5_1__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23404:1: rule__ServiceMethod__Group_5_1__1 : rule__ServiceMethod__Group_5_1__1__Impl ;
public final void rule__ServiceMethod__Group_5_1__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23408:1: ( rule__ServiceMethod__Group_5_1__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23409:2: rule__ServiceMethod__Group_5_1__1__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__ServiceMethod__Group_5_1__1__Impl_in_rule__ServiceMethod__Group_5_1__147559);
rule__ServiceMethod__Group_5_1__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ServiceMethod__Group_5_1__1"
// $ANTLR start "rule__ServiceMethod__Group_5_1__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23415:1: rule__ServiceMethod__Group_5_1__1__Impl : ( ( rule__ServiceMethod__ParamsAssignment_5_1_1 ) ) ;
public final void rule__ServiceMethod__Group_5_1__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23419:1: ( ( ( rule__ServiceMethod__ParamsAssignment_5_1_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23420:1: ( ( rule__ServiceMethod__ParamsAssignment_5_1_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23420:1: ( ( rule__ServiceMethod__ParamsAssignment_5_1_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23421:1: ( rule__ServiceMethod__ParamsAssignment_5_1_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getServiceMethodAccess().getParamsAssignment_5_1_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23422:1: ( rule__ServiceMethod__ParamsAssignment_5_1_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23422:2: rule__ServiceMethod__ParamsAssignment_5_1_1
{
pushFollow(FollowSets002.FOLLOW_rule__ServiceMethod__ParamsAssignment_5_1_1_in_rule__ServiceMethod__Group_5_1__1__Impl47586);
rule__ServiceMethod__ParamsAssignment_5_1_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getServiceMethodAccess().getParamsAssignment_5_1_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ServiceMethod__Group_5_1__1__Impl"
// $ANTLR start "rule__Service__Group__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23436:1: rule__Service__Group__0 : rule__Service__Group__0__Impl rule__Service__Group__1 ;
public final void rule__Service__Group__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23440:1: ( rule__Service__Group__0__Impl rule__Service__Group__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23441:2: rule__Service__Group__0__Impl rule__Service__Group__1
{
pushFollow(FollowSets002.FOLLOW_rule__Service__Group__0__Impl_in_rule__Service__Group__047620);
rule__Service__Group__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__Service__Group__1_in_rule__Service__Group__047623);
rule__Service__Group__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Service__Group__0"
// $ANTLR start "rule__Service__Group__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23448:1: rule__Service__Group__0__Impl : ( () ) ;
public final void rule__Service__Group__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23452:1: ( ( () ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23453:1: ( () )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23453:1: ( () )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23454:1: ()
{
if ( state.backtracking==0 ) {
before(grammarAccess.getServiceAccess().getServiceAction_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23455:1: ()
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23457:1:
{
}
if ( state.backtracking==0 ) {
after(grammarAccess.getServiceAccess().getServiceAction_0());
}
}
}
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Service__Group__0__Impl"
// $ANTLR start "rule__Service__Group__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23467:1: rule__Service__Group__1 : rule__Service__Group__1__Impl rule__Service__Group__2 ;
public final void rule__Service__Group__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23471:1: ( rule__Service__Group__1__Impl rule__Service__Group__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23472:2: rule__Service__Group__1__Impl rule__Service__Group__2
{
pushFollow(FollowSets002.FOLLOW_rule__Service__Group__1__Impl_in_rule__Service__Group__147681);
rule__Service__Group__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__Service__Group__2_in_rule__Service__Group__147684);
rule__Service__Group__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Service__Group__1"
// $ANTLR start "rule__Service__Group__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23479:1: rule__Service__Group__1__Impl : ( 'service' ) ;
public final void rule__Service__Group__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23483:1: ( ( 'service' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23484:1: ( 'service' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23484:1: ( 'service' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23485:1: 'service'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getServiceAccess().getServiceKeyword_1());
}
match(input,146,FollowSets002.FOLLOW_146_in_rule__Service__Group__1__Impl47712); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getServiceAccess().getServiceKeyword_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Service__Group__1__Impl"
// $ANTLR start "rule__Service__Group__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23498:1: rule__Service__Group__2 : rule__Service__Group__2__Impl rule__Service__Group__3 ;
public final void rule__Service__Group__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23502:1: ( rule__Service__Group__2__Impl rule__Service__Group__3 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23503:2: rule__Service__Group__2__Impl rule__Service__Group__3
{
pushFollow(FollowSets002.FOLLOW_rule__Service__Group__2__Impl_in_rule__Service__Group__247743);
rule__Service__Group__2__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__Service__Group__3_in_rule__Service__Group__247746);
rule__Service__Group__3();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Service__Group__2"
// $ANTLR start "rule__Service__Group__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23510:1: rule__Service__Group__2__Impl : ( ( rule__Service__NameAssignment_2 ) ) ;
public final void rule__Service__Group__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23514:1: ( ( ( rule__Service__NameAssignment_2 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23515:1: ( ( rule__Service__NameAssignment_2 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23515:1: ( ( rule__Service__NameAssignment_2 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23516:1: ( rule__Service__NameAssignment_2 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getServiceAccess().getNameAssignment_2());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23517:1: ( rule__Service__NameAssignment_2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23517:2: rule__Service__NameAssignment_2
{
pushFollow(FollowSets002.FOLLOW_rule__Service__NameAssignment_2_in_rule__Service__Group__2__Impl47773);
rule__Service__NameAssignment_2();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getServiceAccess().getNameAssignment_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Service__Group__2__Impl"
// $ANTLR start "rule__Service__Group__3"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23527:1: rule__Service__Group__3 : rule__Service__Group__3__Impl rule__Service__Group__4 ;
public final void rule__Service__Group__3() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23531:1: ( rule__Service__Group__3__Impl rule__Service__Group__4 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23532:2: rule__Service__Group__3__Impl rule__Service__Group__4
{
pushFollow(FollowSets002.FOLLOW_rule__Service__Group__3__Impl_in_rule__Service__Group__347803);
rule__Service__Group__3__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__Service__Group__4_in_rule__Service__Group__347806);
rule__Service__Group__4();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Service__Group__3"
// $ANTLR start "rule__Service__Group__3__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23539:1: rule__Service__Group__3__Impl : ( '{' ) ;
public final void rule__Service__Group__3__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23543:1: ( ( '{' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23544:1: ( '{' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23544:1: ( '{' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23545:1: '{'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getServiceAccess().getLeftCurlyBracketKeyword_3());
}
match(input,67,FollowSets002.FOLLOW_67_in_rule__Service__Group__3__Impl47834); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getServiceAccess().getLeftCurlyBracketKeyword_3());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Service__Group__3__Impl"
// $ANTLR start "rule__Service__Group__4"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23558:1: rule__Service__Group__4 : rule__Service__Group__4__Impl rule__Service__Group__5 ;
public final void rule__Service__Group__4() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23562:1: ( rule__Service__Group__4__Impl rule__Service__Group__5 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23563:2: rule__Service__Group__4__Impl rule__Service__Group__5
{
pushFollow(FollowSets002.FOLLOW_rule__Service__Group__4__Impl_in_rule__Service__Group__447865);
rule__Service__Group__4__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__Service__Group__5_in_rule__Service__Group__447868);
rule__Service__Group__5();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Service__Group__4"
// $ANTLR start "rule__Service__Group__4__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23570:1: rule__Service__Group__4__Impl : ( ( rule__Service__Group_4__0 )? ) ;
public final void rule__Service__Group__4__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23574:1: ( ( ( rule__Service__Group_4__0 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23575:1: ( ( rule__Service__Group_4__0 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23575:1: ( ( rule__Service__Group_4__0 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23576:1: ( rule__Service__Group_4__0 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getServiceAccess().getGroup_4());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23577:1: ( rule__Service__Group_4__0 )?
int alt188=2;
int LA188_0 = input.LA(1);
if ( (LA188_0==147) ) {
alt188=1;
}
switch (alt188) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23577:2: rule__Service__Group_4__0
{
pushFollow(FollowSets002.FOLLOW_rule__Service__Group_4__0_in_rule__Service__Group__4__Impl47895);
rule__Service__Group_4__0();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getServiceAccess().getGroup_4());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Service__Group__4__Impl"
// $ANTLR start "rule__Service__Group__5"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23587:1: rule__Service__Group__5 : rule__Service__Group__5__Impl rule__Service__Group__6 ;
public final void rule__Service__Group__5() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23591:1: ( rule__Service__Group__5__Impl rule__Service__Group__6 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23592:2: rule__Service__Group__5__Impl rule__Service__Group__6
{
pushFollow(FollowSets002.FOLLOW_rule__Service__Group__5__Impl_in_rule__Service__Group__547926);
rule__Service__Group__5__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__Service__Group__6_in_rule__Service__Group__547929);
rule__Service__Group__6();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Service__Group__5"
// $ANTLR start "rule__Service__Group__5__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23599:1: rule__Service__Group__5__Impl : ( ( rule__Service__RemoteMethodsAssignment_5 )* ) ;
public final void rule__Service__Group__5__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23603:1: ( ( ( rule__Service__RemoteMethodsAssignment_5 )* ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23604:1: ( ( rule__Service__RemoteMethodsAssignment_5 )* )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23604:1: ( ( rule__Service__RemoteMethodsAssignment_5 )* )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23605:1: ( rule__Service__RemoteMethodsAssignment_5 )*
{
if ( state.backtracking==0 ) {
before(grammarAccess.getServiceAccess().getRemoteMethodsAssignment_5());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23606:1: ( rule__Service__RemoteMethodsAssignment_5 )*
loop189:
do {
int alt189=2;
int LA189_0 = input.LA(1);
if ( (LA189_0==143) ) {
alt189=1;
}
switch (alt189) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23606:2: rule__Service__RemoteMethodsAssignment_5
{
pushFollow(FollowSets002.FOLLOW_rule__Service__RemoteMethodsAssignment_5_in_rule__Service__Group__5__Impl47956);
rule__Service__RemoteMethodsAssignment_5();
state._fsp--;
if (state.failed) return ;
}
break;
default :
break loop189;
}
} while (true);
if ( state.backtracking==0 ) {
after(grammarAccess.getServiceAccess().getRemoteMethodsAssignment_5());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Service__Group__5__Impl"
// $ANTLR start "rule__Service__Group__6"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23616:1: rule__Service__Group__6 : rule__Service__Group__6__Impl ;
public final void rule__Service__Group__6() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23620:1: ( rule__Service__Group__6__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23621:2: rule__Service__Group__6__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__Service__Group__6__Impl_in_rule__Service__Group__647987);
rule__Service__Group__6__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Service__Group__6"
// $ANTLR start "rule__Service__Group__6__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23627:1: rule__Service__Group__6__Impl : ( '}' ) ;
public final void rule__Service__Group__6__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23631:1: ( ( '}' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23632:1: ( '}' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23632:1: ( '}' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23633:1: '}'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getServiceAccess().getRightCurlyBracketKeyword_6());
}
match(input,68,FollowSets002.FOLLOW_68_in_rule__Service__Group__6__Impl48015); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getServiceAccess().getRightCurlyBracketKeyword_6());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Service__Group__6__Impl"
// $ANTLR start "rule__Service__Group_4__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23660:1: rule__Service__Group_4__0 : rule__Service__Group_4__0__Impl rule__Service__Group_4__1 ;
public final void rule__Service__Group_4__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23664:1: ( rule__Service__Group_4__0__Impl rule__Service__Group_4__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23665:2: rule__Service__Group_4__0__Impl rule__Service__Group_4__1
{
pushFollow(FollowSets002.FOLLOW_rule__Service__Group_4__0__Impl_in_rule__Service__Group_4__048060);
rule__Service__Group_4__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__Service__Group_4__1_in_rule__Service__Group_4__048063);
rule__Service__Group_4__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Service__Group_4__0"
// $ANTLR start "rule__Service__Group_4__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23672:1: rule__Service__Group_4__0__Impl : ( 'options1' ) ;
public final void rule__Service__Group_4__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23676:1: ( ( 'options1' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23677:1: ( 'options1' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23677:1: ( 'options1' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23678:1: 'options1'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getServiceAccess().getOptions1Keyword_4_0());
}
match(input,147,FollowSets002.FOLLOW_147_in_rule__Service__Group_4__0__Impl48091); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getServiceAccess().getOptions1Keyword_4_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Service__Group_4__0__Impl"
// $ANTLR start "rule__Service__Group_4__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23691:1: rule__Service__Group_4__1 : rule__Service__Group_4__1__Impl rule__Service__Group_4__2 ;
public final void rule__Service__Group_4__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23695:1: ( rule__Service__Group_4__1__Impl rule__Service__Group_4__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23696:2: rule__Service__Group_4__1__Impl rule__Service__Group_4__2
{
pushFollow(FollowSets002.FOLLOW_rule__Service__Group_4__1__Impl_in_rule__Service__Group_4__148122);
rule__Service__Group_4__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__Service__Group_4__2_in_rule__Service__Group_4__148125);
rule__Service__Group_4__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Service__Group_4__1"
// $ANTLR start "rule__Service__Group_4__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23703:1: rule__Service__Group_4__1__Impl : ( '{' ) ;
public final void rule__Service__Group_4__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23707:1: ( ( '{' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23708:1: ( '{' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23708:1: ( '{' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23709:1: '{'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getServiceAccess().getLeftCurlyBracketKeyword_4_1());
}
match(input,67,FollowSets002.FOLLOW_67_in_rule__Service__Group_4__1__Impl48153); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getServiceAccess().getLeftCurlyBracketKeyword_4_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Service__Group_4__1__Impl"
// $ANTLR start "rule__Service__Group_4__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23722:1: rule__Service__Group_4__2 : rule__Service__Group_4__2__Impl rule__Service__Group_4__3 ;
public final void rule__Service__Group_4__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23726:1: ( rule__Service__Group_4__2__Impl rule__Service__Group_4__3 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23727:2: rule__Service__Group_4__2__Impl rule__Service__Group_4__3
{
pushFollow(FollowSets002.FOLLOW_rule__Service__Group_4__2__Impl_in_rule__Service__Group_4__248184);
rule__Service__Group_4__2__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__Service__Group_4__3_in_rule__Service__Group_4__248187);
rule__Service__Group_4__3();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Service__Group_4__2"
// $ANTLR start "rule__Service__Group_4__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23734:1: rule__Service__Group_4__2__Impl : ( ( rule__Service__RemoteServiceOptionsAssignment_4_2 ) ) ;
public final void rule__Service__Group_4__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23738:1: ( ( ( rule__Service__RemoteServiceOptionsAssignment_4_2 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23739:1: ( ( rule__Service__RemoteServiceOptionsAssignment_4_2 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23739:1: ( ( rule__Service__RemoteServiceOptionsAssignment_4_2 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23740:1: ( rule__Service__RemoteServiceOptionsAssignment_4_2 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getServiceAccess().getRemoteServiceOptionsAssignment_4_2());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23741:1: ( rule__Service__RemoteServiceOptionsAssignment_4_2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23741:2: rule__Service__RemoteServiceOptionsAssignment_4_2
{
pushFollow(FollowSets002.FOLLOW_rule__Service__RemoteServiceOptionsAssignment_4_2_in_rule__Service__Group_4__2__Impl48214);
rule__Service__RemoteServiceOptionsAssignment_4_2();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getServiceAccess().getRemoteServiceOptionsAssignment_4_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Service__Group_4__2__Impl"
// $ANTLR start "rule__Service__Group_4__3"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23751:1: rule__Service__Group_4__3 : rule__Service__Group_4__3__Impl ;
public final void rule__Service__Group_4__3() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23755:1: ( rule__Service__Group_4__3__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23756:2: rule__Service__Group_4__3__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__Service__Group_4__3__Impl_in_rule__Service__Group_4__348244);
rule__Service__Group_4__3__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Service__Group_4__3"
// $ANTLR start "rule__Service__Group_4__3__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23762:1: rule__Service__Group_4__3__Impl : ( '}' ) ;
public final void rule__Service__Group_4__3__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23766:1: ( ( '}' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23767:1: ( '}' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23767:1: ( '}' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23768:1: '}'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getServiceAccess().getRightCurlyBracketKeyword_4_3());
}
match(input,68,FollowSets002.FOLLOW_68_in_rule__Service__Group_4__3__Impl48272); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getServiceAccess().getRightCurlyBracketKeyword_4_3());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Service__Group_4__3__Impl"
// $ANTLR start "rule__NavigationNode__Group__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23789:1: rule__NavigationNode__Group__0 : rule__NavigationNode__Group__0__Impl rule__NavigationNode__Group__1 ;
public final void rule__NavigationNode__Group__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23793:1: ( rule__NavigationNode__Group__0__Impl rule__NavigationNode__Group__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23794:2: rule__NavigationNode__Group__0__Impl rule__NavigationNode__Group__1
{
pushFollow(FollowSets002.FOLLOW_rule__NavigationNode__Group__0__Impl_in_rule__NavigationNode__Group__048311);
rule__NavigationNode__Group__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__NavigationNode__Group__1_in_rule__NavigationNode__Group__048314);
rule__NavigationNode__Group__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__NavigationNode__Group__0"
// $ANTLR start "rule__NavigationNode__Group__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23801:1: rule__NavigationNode__Group__0__Impl : ( 'navigationnode' ) ;
public final void rule__NavigationNode__Group__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23805:1: ( ( 'navigationnode' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23806:1: ( 'navigationnode' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23806:1: ( 'navigationnode' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23807:1: 'navigationnode'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getNavigationNodeAccess().getNavigationnodeKeyword_0());
}
match(input,148,FollowSets002.FOLLOW_148_in_rule__NavigationNode__Group__0__Impl48342); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getNavigationNodeAccess().getNavigationnodeKeyword_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__NavigationNode__Group__0__Impl"
// $ANTLR start "rule__NavigationNode__Group__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23820:1: rule__NavigationNode__Group__1 : rule__NavigationNode__Group__1__Impl rule__NavigationNode__Group__2 ;
public final void rule__NavigationNode__Group__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23824:1: ( rule__NavigationNode__Group__1__Impl rule__NavigationNode__Group__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23825:2: rule__NavigationNode__Group__1__Impl rule__NavigationNode__Group__2
{
pushFollow(FollowSets002.FOLLOW_rule__NavigationNode__Group__1__Impl_in_rule__NavigationNode__Group__148373);
rule__NavigationNode__Group__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__NavigationNode__Group__2_in_rule__NavigationNode__Group__148376);
rule__NavigationNode__Group__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__NavigationNode__Group__1"
// $ANTLR start "rule__NavigationNode__Group__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23832:1: rule__NavigationNode__Group__1__Impl : ( ( rule__NavigationNode__NameAssignment_1 ) ) ;
public final void rule__NavigationNode__Group__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23836:1: ( ( ( rule__NavigationNode__NameAssignment_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23837:1: ( ( rule__NavigationNode__NameAssignment_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23837:1: ( ( rule__NavigationNode__NameAssignment_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23838:1: ( rule__NavigationNode__NameAssignment_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getNavigationNodeAccess().getNameAssignment_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23839:1: ( rule__NavigationNode__NameAssignment_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23839:2: rule__NavigationNode__NameAssignment_1
{
pushFollow(FollowSets002.FOLLOW_rule__NavigationNode__NameAssignment_1_in_rule__NavigationNode__Group__1__Impl48403);
rule__NavigationNode__NameAssignment_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getNavigationNodeAccess().getNameAssignment_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__NavigationNode__Group__1__Impl"
// $ANTLR start "rule__NavigationNode__Group__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23849:1: rule__NavigationNode__Group__2 : rule__NavigationNode__Group__2__Impl rule__NavigationNode__Group__3 ;
public final void rule__NavigationNode__Group__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23853:1: ( rule__NavigationNode__Group__2__Impl rule__NavigationNode__Group__3 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23854:2: rule__NavigationNode__Group__2__Impl rule__NavigationNode__Group__3
{
pushFollow(FollowSets002.FOLLOW_rule__NavigationNode__Group__2__Impl_in_rule__NavigationNode__Group__248433);
rule__NavigationNode__Group__2__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__NavigationNode__Group__3_in_rule__NavigationNode__Group__248436);
rule__NavigationNode__Group__3();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__NavigationNode__Group__2"
// $ANTLR start "rule__NavigationNode__Group__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23861:1: rule__NavigationNode__Group__2__Impl : ( '{' ) ;
public final void rule__NavigationNode__Group__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23865:1: ( ( '{' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23866:1: ( '{' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23866:1: ( '{' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23867:1: '{'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getNavigationNodeAccess().getLeftCurlyBracketKeyword_2());
}
match(input,67,FollowSets002.FOLLOW_67_in_rule__NavigationNode__Group__2__Impl48464); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getNavigationNodeAccess().getLeftCurlyBracketKeyword_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__NavigationNode__Group__2__Impl"
// $ANTLR start "rule__NavigationNode__Group__3"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23880:1: rule__NavigationNode__Group__3 : rule__NavigationNode__Group__3__Impl rule__NavigationNode__Group__4 ;
public final void rule__NavigationNode__Group__3() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23884:1: ( rule__NavigationNode__Group__3__Impl rule__NavigationNode__Group__4 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23885:2: rule__NavigationNode__Group__3__Impl rule__NavigationNode__Group__4
{
pushFollow(FollowSets002.FOLLOW_rule__NavigationNode__Group__3__Impl_in_rule__NavigationNode__Group__348495);
rule__NavigationNode__Group__3__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__NavigationNode__Group__4_in_rule__NavigationNode__Group__348498);
rule__NavigationNode__Group__4();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__NavigationNode__Group__3"
// $ANTLR start "rule__NavigationNode__Group__3__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23892:1: rule__NavigationNode__Group__3__Impl : ( ( rule__NavigationNode__Group_3__0 )? ) ;
public final void rule__NavigationNode__Group__3__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23896:1: ( ( ( rule__NavigationNode__Group_3__0 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23897:1: ( ( rule__NavigationNode__Group_3__0 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23897:1: ( ( rule__NavigationNode__Group_3__0 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23898:1: ( rule__NavigationNode__Group_3__0 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getNavigationNodeAccess().getGroup_3());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23899:1: ( rule__NavigationNode__Group_3__0 )?
int alt190=2;
int LA190_0 = input.LA(1);
if ( (LA190_0==75) ) {
alt190=1;
}
switch (alt190) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23899:2: rule__NavigationNode__Group_3__0
{
pushFollow(FollowSets002.FOLLOW_rule__NavigationNode__Group_3__0_in_rule__NavigationNode__Group__3__Impl48525);
rule__NavigationNode__Group_3__0();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getNavigationNodeAccess().getGroup_3());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__NavigationNode__Group__3__Impl"
// $ANTLR start "rule__NavigationNode__Group__4"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23909:1: rule__NavigationNode__Group__4 : rule__NavigationNode__Group__4__Impl rule__NavigationNode__Group__5 ;
public final void rule__NavigationNode__Group__4() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23913:1: ( rule__NavigationNode__Group__4__Impl rule__NavigationNode__Group__5 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23914:2: rule__NavigationNode__Group__4__Impl rule__NavigationNode__Group__5
{
pushFollow(FollowSets002.FOLLOW_rule__NavigationNode__Group__4__Impl_in_rule__NavigationNode__Group__448556);
rule__NavigationNode__Group__4__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__NavigationNode__Group__5_in_rule__NavigationNode__Group__448559);
rule__NavigationNode__Group__5();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__NavigationNode__Group__4"
// $ANTLR start "rule__NavigationNode__Group__4__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23921:1: rule__NavigationNode__Group__4__Impl : ( ( rule__NavigationNode__Group_4__0 )? ) ;
public final void rule__NavigationNode__Group__4__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23925:1: ( ( ( rule__NavigationNode__Group_4__0 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23926:1: ( ( rule__NavigationNode__Group_4__0 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23926:1: ( ( rule__NavigationNode__Group_4__0 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23927:1: ( rule__NavigationNode__Group_4__0 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getNavigationNodeAccess().getGroup_4());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23928:1: ( rule__NavigationNode__Group_4__0 )?
int alt191=2;
int LA191_0 = input.LA(1);
if ( (LA191_0==149) ) {
alt191=1;
}
switch (alt191) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23928:2: rule__NavigationNode__Group_4__0
{
pushFollow(FollowSets002.FOLLOW_rule__NavigationNode__Group_4__0_in_rule__NavigationNode__Group__4__Impl48586);
rule__NavigationNode__Group_4__0();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getNavigationNodeAccess().getGroup_4());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__NavigationNode__Group__4__Impl"
// $ANTLR start "rule__NavigationNode__Group__5"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23938:1: rule__NavigationNode__Group__5 : rule__NavigationNode__Group__5__Impl rule__NavigationNode__Group__6 ;
public final void rule__NavigationNode__Group__5() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23942:1: ( rule__NavigationNode__Group__5__Impl rule__NavigationNode__Group__6 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23943:2: rule__NavigationNode__Group__5__Impl rule__NavigationNode__Group__6
{
pushFollow(FollowSets002.FOLLOW_rule__NavigationNode__Group__5__Impl_in_rule__NavigationNode__Group__548617);
rule__NavigationNode__Group__5__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__NavigationNode__Group__6_in_rule__NavigationNode__Group__548620);
rule__NavigationNode__Group__6();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__NavigationNode__Group__5"
// $ANTLR start "rule__NavigationNode__Group__5__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23950:1: rule__NavigationNode__Group__5__Impl : ( ( rule__NavigationNode__Group_5__0 )? ) ;
public final void rule__NavigationNode__Group__5__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23954:1: ( ( ( rule__NavigationNode__Group_5__0 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23955:1: ( ( rule__NavigationNode__Group_5__0 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23955:1: ( ( rule__NavigationNode__Group_5__0 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23956:1: ( rule__NavigationNode__Group_5__0 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getNavigationNodeAccess().getGroup_5());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23957:1: ( rule__NavigationNode__Group_5__0 )?
int alt192=2;
int LA192_0 = input.LA(1);
if ( (LA192_0==139) ) {
alt192=1;
}
switch (alt192) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23957:2: rule__NavigationNode__Group_5__0
{
pushFollow(FollowSets002.FOLLOW_rule__NavigationNode__Group_5__0_in_rule__NavigationNode__Group__5__Impl48647);
rule__NavigationNode__Group_5__0();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getNavigationNodeAccess().getGroup_5());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__NavigationNode__Group__5__Impl"
// $ANTLR start "rule__NavigationNode__Group__6"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23967:1: rule__NavigationNode__Group__6 : rule__NavigationNode__Group__6__Impl rule__NavigationNode__Group__7 ;
public final void rule__NavigationNode__Group__6() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23971:1: ( rule__NavigationNode__Group__6__Impl rule__NavigationNode__Group__7 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23972:2: rule__NavigationNode__Group__6__Impl rule__NavigationNode__Group__7
{
pushFollow(FollowSets002.FOLLOW_rule__NavigationNode__Group__6__Impl_in_rule__NavigationNode__Group__648678);
rule__NavigationNode__Group__6__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__NavigationNode__Group__7_in_rule__NavigationNode__Group__648681);
rule__NavigationNode__Group__7();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__NavigationNode__Group__6"
// $ANTLR start "rule__NavigationNode__Group__6__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23979:1: rule__NavigationNode__Group__6__Impl : ( ( rule__NavigationNode__Group_6__0 )? ) ;
public final void rule__NavigationNode__Group__6__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23983:1: ( ( ( rule__NavigationNode__Group_6__0 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23984:1: ( ( rule__NavigationNode__Group_6__0 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23984:1: ( ( rule__NavigationNode__Group_6__0 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23985:1: ( rule__NavigationNode__Group_6__0 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getNavigationNodeAccess().getGroup_6());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23986:1: ( rule__NavigationNode__Group_6__0 )?
int alt193=2;
int LA193_0 = input.LA(1);
if ( (LA193_0==150) ) {
alt193=1;
}
switch (alt193) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23986:2: rule__NavigationNode__Group_6__0
{
pushFollow(FollowSets002.FOLLOW_rule__NavigationNode__Group_6__0_in_rule__NavigationNode__Group__6__Impl48708);
rule__NavigationNode__Group_6__0();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getNavigationNodeAccess().getGroup_6());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__NavigationNode__Group__6__Impl"
// $ANTLR start "rule__NavigationNode__Group__7"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:23996:1: rule__NavigationNode__Group__7 : rule__NavigationNode__Group__7__Impl rule__NavigationNode__Group__8 ;
public final void rule__NavigationNode__Group__7() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24000:1: ( rule__NavigationNode__Group__7__Impl rule__NavigationNode__Group__8 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24001:2: rule__NavigationNode__Group__7__Impl rule__NavigationNode__Group__8
{
pushFollow(FollowSets002.FOLLOW_rule__NavigationNode__Group__7__Impl_in_rule__NavigationNode__Group__748739);
rule__NavigationNode__Group__7__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__NavigationNode__Group__8_in_rule__NavigationNode__Group__748742);
rule__NavigationNode__Group__8();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__NavigationNode__Group__7"
// $ANTLR start "rule__NavigationNode__Group__7__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24008:1: rule__NavigationNode__Group__7__Impl : ( ( rule__NavigationNode__Group_7__0 )? ) ;
public final void rule__NavigationNode__Group__7__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24012:1: ( ( ( rule__NavigationNode__Group_7__0 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24013:1: ( ( rule__NavigationNode__Group_7__0 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24013:1: ( ( rule__NavigationNode__Group_7__0 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24014:1: ( rule__NavigationNode__Group_7__0 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getNavigationNodeAccess().getGroup_7());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24015:1: ( rule__NavigationNode__Group_7__0 )?
int alt194=2;
int LA194_0 = input.LA(1);
if ( (LA194_0==151) ) {
alt194=1;
}
switch (alt194) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24015:2: rule__NavigationNode__Group_7__0
{
pushFollow(FollowSets002.FOLLOW_rule__NavigationNode__Group_7__0_in_rule__NavigationNode__Group__7__Impl48769);
rule__NavigationNode__Group_7__0();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getNavigationNodeAccess().getGroup_7());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__NavigationNode__Group__7__Impl"
// $ANTLR start "rule__NavigationNode__Group__8"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24025:1: rule__NavigationNode__Group__8 : rule__NavigationNode__Group__8__Impl rule__NavigationNode__Group__9 ;
public final void rule__NavigationNode__Group__8() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24029:1: ( rule__NavigationNode__Group__8__Impl rule__NavigationNode__Group__9 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24030:2: rule__NavigationNode__Group__8__Impl rule__NavigationNode__Group__9
{
pushFollow(FollowSets002.FOLLOW_rule__NavigationNode__Group__8__Impl_in_rule__NavigationNode__Group__848800);
rule__NavigationNode__Group__8__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__NavigationNode__Group__9_in_rule__NavigationNode__Group__848803);
rule__NavigationNode__Group__9();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__NavigationNode__Group__8"
// $ANTLR start "rule__NavigationNode__Group__8__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24037:1: rule__NavigationNode__Group__8__Impl : ( ( rule__NavigationNode__NavigationNodesAssignment_8 )* ) ;
public final void rule__NavigationNode__Group__8__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24041:1: ( ( ( rule__NavigationNode__NavigationNodesAssignment_8 )* ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24042:1: ( ( rule__NavigationNode__NavigationNodesAssignment_8 )* )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24042:1: ( ( rule__NavigationNode__NavigationNodesAssignment_8 )* )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24043:1: ( rule__NavigationNode__NavigationNodesAssignment_8 )*
{
if ( state.backtracking==0 ) {
before(grammarAccess.getNavigationNodeAccess().getNavigationNodesAssignment_8());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24044:1: ( rule__NavigationNode__NavigationNodesAssignment_8 )*
loop195:
do {
int alt195=2;
int LA195_0 = input.LA(1);
if ( (LA195_0==148) ) {
alt195=1;
}
switch (alt195) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24044:2: rule__NavigationNode__NavigationNodesAssignment_8
{
pushFollow(FollowSets002.FOLLOW_rule__NavigationNode__NavigationNodesAssignment_8_in_rule__NavigationNode__Group__8__Impl48830);
rule__NavigationNode__NavigationNodesAssignment_8();
state._fsp--;
if (state.failed) return ;
}
break;
default :
break loop195;
}
} while (true);
if ( state.backtracking==0 ) {
after(grammarAccess.getNavigationNodeAccess().getNavigationNodesAssignment_8());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__NavigationNode__Group__8__Impl"
// $ANTLR start "rule__NavigationNode__Group__9"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24054:1: rule__NavigationNode__Group__9 : rule__NavigationNode__Group__9__Impl ;
public final void rule__NavigationNode__Group__9() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24058:1: ( rule__NavigationNode__Group__9__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24059:2: rule__NavigationNode__Group__9__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__NavigationNode__Group__9__Impl_in_rule__NavigationNode__Group__948861);
rule__NavigationNode__Group__9__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__NavigationNode__Group__9"
// $ANTLR start "rule__NavigationNode__Group__9__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24065:1: rule__NavigationNode__Group__9__Impl : ( '}' ) ;
public final void rule__NavigationNode__Group__9__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24069:1: ( ( '}' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24070:1: ( '}' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24070:1: ( '}' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24071:1: '}'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getNavigationNodeAccess().getRightCurlyBracketKeyword_9());
}
match(input,68,FollowSets002.FOLLOW_68_in_rule__NavigationNode__Group__9__Impl48889); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getNavigationNodeAccess().getRightCurlyBracketKeyword_9());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__NavigationNode__Group__9__Impl"
// $ANTLR start "rule__NavigationNode__Group_3__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24104:1: rule__NavigationNode__Group_3__0 : rule__NavigationNode__Group_3__0__Impl rule__NavigationNode__Group_3__1 ;
public final void rule__NavigationNode__Group_3__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24108:1: ( rule__NavigationNode__Group_3__0__Impl rule__NavigationNode__Group_3__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24109:2: rule__NavigationNode__Group_3__0__Impl rule__NavigationNode__Group_3__1
{
pushFollow(FollowSets002.FOLLOW_rule__NavigationNode__Group_3__0__Impl_in_rule__NavigationNode__Group_3__048940);
rule__NavigationNode__Group_3__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__NavigationNode__Group_3__1_in_rule__NavigationNode__Group_3__048943);
rule__NavigationNode__Group_3__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__NavigationNode__Group_3__0"
// $ANTLR start "rule__NavigationNode__Group_3__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24116:1: rule__NavigationNode__Group_3__0__Impl : ( 'label' ) ;
public final void rule__NavigationNode__Group_3__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24120:1: ( ( 'label' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24121:1: ( 'label' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24121:1: ( 'label' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24122:1: 'label'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getNavigationNodeAccess().getLabelKeyword_3_0());
}
match(input,75,FollowSets002.FOLLOW_75_in_rule__NavigationNode__Group_3__0__Impl48971); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getNavigationNodeAccess().getLabelKeyword_3_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__NavigationNode__Group_3__0__Impl"
// $ANTLR start "rule__NavigationNode__Group_3__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24135:1: rule__NavigationNode__Group_3__1 : rule__NavigationNode__Group_3__1__Impl ;
public final void rule__NavigationNode__Group_3__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24139:1: ( rule__NavigationNode__Group_3__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24140:2: rule__NavigationNode__Group_3__1__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__NavigationNode__Group_3__1__Impl_in_rule__NavigationNode__Group_3__149002);
rule__NavigationNode__Group_3__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__NavigationNode__Group_3__1"
// $ANTLR start "rule__NavigationNode__Group_3__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24146:1: rule__NavigationNode__Group_3__1__Impl : ( ( rule__NavigationNode__LabelAssignment_3_1 ) ) ;
public final void rule__NavigationNode__Group_3__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24150:1: ( ( ( rule__NavigationNode__LabelAssignment_3_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24151:1: ( ( rule__NavigationNode__LabelAssignment_3_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24151:1: ( ( rule__NavigationNode__LabelAssignment_3_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24152:1: ( rule__NavigationNode__LabelAssignment_3_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getNavigationNodeAccess().getLabelAssignment_3_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24153:1: ( rule__NavigationNode__LabelAssignment_3_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24153:2: rule__NavigationNode__LabelAssignment_3_1
{
pushFollow(FollowSets002.FOLLOW_rule__NavigationNode__LabelAssignment_3_1_in_rule__NavigationNode__Group_3__1__Impl49029);
rule__NavigationNode__LabelAssignment_3_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getNavigationNodeAccess().getLabelAssignment_3_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__NavigationNode__Group_3__1__Impl"
// $ANTLR start "rule__NavigationNode__Group_4__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24167:1: rule__NavigationNode__Group_4__0 : rule__NavigationNode__Group_4__0__Impl rule__NavigationNode__Group_4__1 ;
public final void rule__NavigationNode__Group_4__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24171:1: ( rule__NavigationNode__Group_4__0__Impl rule__NavigationNode__Group_4__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24172:2: rule__NavigationNode__Group_4__0__Impl rule__NavigationNode__Group_4__1
{
pushFollow(FollowSets002.FOLLOW_rule__NavigationNode__Group_4__0__Impl_in_rule__NavigationNode__Group_4__049063);
rule__NavigationNode__Group_4__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__NavigationNode__Group_4__1_in_rule__NavigationNode__Group_4__049066);
rule__NavigationNode__Group_4__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__NavigationNode__Group_4__0"
// $ANTLR start "rule__NavigationNode__Group_4__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24179:1: rule__NavigationNode__Group_4__0__Impl : ( 'moduleDefinition' ) ;
public final void rule__NavigationNode__Group_4__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24183:1: ( ( 'moduleDefinition' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24184:1: ( 'moduleDefinition' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24184:1: ( 'moduleDefinition' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24185:1: 'moduleDefinition'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getNavigationNodeAccess().getModuleDefinitionKeyword_4_0());
}
match(input,149,FollowSets002.FOLLOW_149_in_rule__NavigationNode__Group_4__0__Impl49094); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getNavigationNodeAccess().getModuleDefinitionKeyword_4_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__NavigationNode__Group_4__0__Impl"
// $ANTLR start "rule__NavigationNode__Group_4__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24198:1: rule__NavigationNode__Group_4__1 : rule__NavigationNode__Group_4__1__Impl ;
public final void rule__NavigationNode__Group_4__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24202:1: ( rule__NavigationNode__Group_4__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24203:2: rule__NavigationNode__Group_4__1__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__NavigationNode__Group_4__1__Impl_in_rule__NavigationNode__Group_4__149125);
rule__NavigationNode__Group_4__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__NavigationNode__Group_4__1"
// $ANTLR start "rule__NavigationNode__Group_4__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24209:1: rule__NavigationNode__Group_4__1__Impl : ( ( rule__NavigationNode__ModuleDefinitionAssignment_4_1 ) ) ;
public final void rule__NavigationNode__Group_4__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24213:1: ( ( ( rule__NavigationNode__ModuleDefinitionAssignment_4_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24214:1: ( ( rule__NavigationNode__ModuleDefinitionAssignment_4_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24214:1: ( ( rule__NavigationNode__ModuleDefinitionAssignment_4_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24215:1: ( rule__NavigationNode__ModuleDefinitionAssignment_4_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getNavigationNodeAccess().getModuleDefinitionAssignment_4_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24216:1: ( rule__NavigationNode__ModuleDefinitionAssignment_4_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24216:2: rule__NavigationNode__ModuleDefinitionAssignment_4_1
{
pushFollow(FollowSets002.FOLLOW_rule__NavigationNode__ModuleDefinitionAssignment_4_1_in_rule__NavigationNode__Group_4__1__Impl49152);
rule__NavigationNode__ModuleDefinitionAssignment_4_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getNavigationNodeAccess().getModuleDefinitionAssignment_4_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__NavigationNode__Group_4__1__Impl"
// $ANTLR start "rule__NavigationNode__Group_5__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24230:1: rule__NavigationNode__Group_5__0 : rule__NavigationNode__Group_5__0__Impl rule__NavigationNode__Group_5__1 ;
public final void rule__NavigationNode__Group_5__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24234:1: ( rule__NavigationNode__Group_5__0__Impl rule__NavigationNode__Group_5__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24235:2: rule__NavigationNode__Group_5__0__Impl rule__NavigationNode__Group_5__1
{
pushFollow(FollowSets002.FOLLOW_rule__NavigationNode__Group_5__0__Impl_in_rule__NavigationNode__Group_5__049186);
rule__NavigationNode__Group_5__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__NavigationNode__Group_5__1_in_rule__NavigationNode__Group_5__049189);
rule__NavigationNode__Group_5__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__NavigationNode__Group_5__0"
// $ANTLR start "rule__NavigationNode__Group_5__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24242:1: rule__NavigationNode__Group_5__0__Impl : ( 'module' ) ;
public final void rule__NavigationNode__Group_5__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24246:1: ( ( 'module' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24247:1: ( 'module' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24247:1: ( 'module' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24248:1: 'module'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getNavigationNodeAccess().getModuleKeyword_5_0());
}
match(input,139,FollowSets002.FOLLOW_139_in_rule__NavigationNode__Group_5__0__Impl49217); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getNavigationNodeAccess().getModuleKeyword_5_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__NavigationNode__Group_5__0__Impl"
// $ANTLR start "rule__NavigationNode__Group_5__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24261:1: rule__NavigationNode__Group_5__1 : rule__NavigationNode__Group_5__1__Impl ;
public final void rule__NavigationNode__Group_5__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24265:1: ( rule__NavigationNode__Group_5__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24266:2: rule__NavigationNode__Group_5__1__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__NavigationNode__Group_5__1__Impl_in_rule__NavigationNode__Group_5__149248);
rule__NavigationNode__Group_5__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__NavigationNode__Group_5__1"
// $ANTLR start "rule__NavigationNode__Group_5__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24272:1: rule__NavigationNode__Group_5__1__Impl : ( ( rule__NavigationNode__ModuleAssignment_5_1 ) ) ;
public final void rule__NavigationNode__Group_5__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24276:1: ( ( ( rule__NavigationNode__ModuleAssignment_5_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24277:1: ( ( rule__NavigationNode__ModuleAssignment_5_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24277:1: ( ( rule__NavigationNode__ModuleAssignment_5_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24278:1: ( rule__NavigationNode__ModuleAssignment_5_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getNavigationNodeAccess().getModuleAssignment_5_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24279:1: ( rule__NavigationNode__ModuleAssignment_5_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24279:2: rule__NavigationNode__ModuleAssignment_5_1
{
pushFollow(FollowSets002.FOLLOW_rule__NavigationNode__ModuleAssignment_5_1_in_rule__NavigationNode__Group_5__1__Impl49275);
rule__NavigationNode__ModuleAssignment_5_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getNavigationNodeAccess().getModuleAssignment_5_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__NavigationNode__Group_5__1__Impl"
// $ANTLR start "rule__NavigationNode__Group_6__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24293:1: rule__NavigationNode__Group_6__0 : rule__NavigationNode__Group_6__0__Impl rule__NavigationNode__Group_6__1 ;
public final void rule__NavigationNode__Group_6__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24297:1: ( rule__NavigationNode__Group_6__0__Impl rule__NavigationNode__Group_6__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24298:2: rule__NavigationNode__Group_6__0__Impl rule__NavigationNode__Group_6__1
{
pushFollow(FollowSets002.FOLLOW_rule__NavigationNode__Group_6__0__Impl_in_rule__NavigationNode__Group_6__049309);
rule__NavigationNode__Group_6__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__NavigationNode__Group_6__1_in_rule__NavigationNode__Group_6__049312);
rule__NavigationNode__Group_6__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__NavigationNode__Group_6__0"
// $ANTLR start "rule__NavigationNode__Group_6__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24305:1: rule__NavigationNode__Group_6__0__Impl : ( 'dictionaryEditor' ) ;
public final void rule__NavigationNode__Group_6__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24309:1: ( ( 'dictionaryEditor' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24310:1: ( 'dictionaryEditor' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24310:1: ( 'dictionaryEditor' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24311:1: 'dictionaryEditor'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getNavigationNodeAccess().getDictionaryEditorKeyword_6_0());
}
match(input,150,FollowSets002.FOLLOW_150_in_rule__NavigationNode__Group_6__0__Impl49340); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getNavigationNodeAccess().getDictionaryEditorKeyword_6_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__NavigationNode__Group_6__0__Impl"
// $ANTLR start "rule__NavigationNode__Group_6__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24324:1: rule__NavigationNode__Group_6__1 : rule__NavigationNode__Group_6__1__Impl ;
public final void rule__NavigationNode__Group_6__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24328:1: ( rule__NavigationNode__Group_6__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24329:2: rule__NavigationNode__Group_6__1__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__NavigationNode__Group_6__1__Impl_in_rule__NavigationNode__Group_6__149371);
rule__NavigationNode__Group_6__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__NavigationNode__Group_6__1"
// $ANTLR start "rule__NavigationNode__Group_6__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24335:1: rule__NavigationNode__Group_6__1__Impl : ( ( rule__NavigationNode__DictionaryEditorAssignment_6_1 ) ) ;
public final void rule__NavigationNode__Group_6__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24339:1: ( ( ( rule__NavigationNode__DictionaryEditorAssignment_6_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24340:1: ( ( rule__NavigationNode__DictionaryEditorAssignment_6_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24340:1: ( ( rule__NavigationNode__DictionaryEditorAssignment_6_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24341:1: ( rule__NavigationNode__DictionaryEditorAssignment_6_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getNavigationNodeAccess().getDictionaryEditorAssignment_6_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24342:1: ( rule__NavigationNode__DictionaryEditorAssignment_6_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24342:2: rule__NavigationNode__DictionaryEditorAssignment_6_1
{
pushFollow(FollowSets002.FOLLOW_rule__NavigationNode__DictionaryEditorAssignment_6_1_in_rule__NavigationNode__Group_6__1__Impl49398);
rule__NavigationNode__DictionaryEditorAssignment_6_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getNavigationNodeAccess().getDictionaryEditorAssignment_6_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__NavigationNode__Group_6__1__Impl"
// $ANTLR start "rule__NavigationNode__Group_7__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24356:1: rule__NavigationNode__Group_7__0 : rule__NavigationNode__Group_7__0__Impl rule__NavigationNode__Group_7__1 ;
public final void rule__NavigationNode__Group_7__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24360:1: ( rule__NavigationNode__Group_7__0__Impl rule__NavigationNode__Group_7__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24361:2: rule__NavigationNode__Group_7__0__Impl rule__NavigationNode__Group_7__1
{
pushFollow(FollowSets002.FOLLOW_rule__NavigationNode__Group_7__0__Impl_in_rule__NavigationNode__Group_7__049432);
rule__NavigationNode__Group_7__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__NavigationNode__Group_7__1_in_rule__NavigationNode__Group_7__049435);
rule__NavigationNode__Group_7__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__NavigationNode__Group_7__0"
// $ANTLR start "rule__NavigationNode__Group_7__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24368:1: rule__NavigationNode__Group_7__0__Impl : ( 'dictionarySearch' ) ;
public final void rule__NavigationNode__Group_7__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24372:1: ( ( 'dictionarySearch' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24373:1: ( 'dictionarySearch' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24373:1: ( 'dictionarySearch' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24374:1: 'dictionarySearch'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getNavigationNodeAccess().getDictionarySearchKeyword_7_0());
}
match(input,151,FollowSets002.FOLLOW_151_in_rule__NavigationNode__Group_7__0__Impl49463); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getNavigationNodeAccess().getDictionarySearchKeyword_7_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__NavigationNode__Group_7__0__Impl"
// $ANTLR start "rule__NavigationNode__Group_7__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24387:1: rule__NavigationNode__Group_7__1 : rule__NavigationNode__Group_7__1__Impl ;
public final void rule__NavigationNode__Group_7__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24391:1: ( rule__NavigationNode__Group_7__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24392:2: rule__NavigationNode__Group_7__1__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__NavigationNode__Group_7__1__Impl_in_rule__NavigationNode__Group_7__149494);
rule__NavigationNode__Group_7__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__NavigationNode__Group_7__1"
// $ANTLR start "rule__NavigationNode__Group_7__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24398:1: rule__NavigationNode__Group_7__1__Impl : ( ( rule__NavigationNode__DictionarySearchAssignment_7_1 ) ) ;
public final void rule__NavigationNode__Group_7__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24402:1: ( ( ( rule__NavigationNode__DictionarySearchAssignment_7_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24403:1: ( ( rule__NavigationNode__DictionarySearchAssignment_7_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24403:1: ( ( rule__NavigationNode__DictionarySearchAssignment_7_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24404:1: ( rule__NavigationNode__DictionarySearchAssignment_7_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getNavigationNodeAccess().getDictionarySearchAssignment_7_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24405:1: ( rule__NavigationNode__DictionarySearchAssignment_7_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24405:2: rule__NavigationNode__DictionarySearchAssignment_7_1
{
pushFollow(FollowSets002.FOLLOW_rule__NavigationNode__DictionarySearchAssignment_7_1_in_rule__NavigationNode__Group_7__1__Impl49521);
rule__NavigationNode__DictionarySearchAssignment_7_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getNavigationNodeAccess().getDictionarySearchAssignment_7_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__NavigationNode__Group_7__1__Impl"
// $ANTLR start "rule__XAssignment__Group_0__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24419:1: rule__XAssignment__Group_0__0 : rule__XAssignment__Group_0__0__Impl rule__XAssignment__Group_0__1 ;
public final void rule__XAssignment__Group_0__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24423:1: ( rule__XAssignment__Group_0__0__Impl rule__XAssignment__Group_0__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24424:2: rule__XAssignment__Group_0__0__Impl rule__XAssignment__Group_0__1
{
pushFollow(FollowSets002.FOLLOW_rule__XAssignment__Group_0__0__Impl_in_rule__XAssignment__Group_0__049555);
rule__XAssignment__Group_0__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XAssignment__Group_0__1_in_rule__XAssignment__Group_0__049558);
rule__XAssignment__Group_0__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XAssignment__Group_0__0"
// $ANTLR start "rule__XAssignment__Group_0__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24431:1: rule__XAssignment__Group_0__0__Impl : ( () ) ;
public final void rule__XAssignment__Group_0__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24435:1: ( ( () ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24436:1: ( () )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24436:1: ( () )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24437:1: ()
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXAssignmentAccess().getXAssignmentAction_0_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24438:1: ()
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24440:1:
{
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXAssignmentAccess().getXAssignmentAction_0_0());
}
}
}
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XAssignment__Group_0__0__Impl"
// $ANTLR start "rule__XAssignment__Group_0__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24450:1: rule__XAssignment__Group_0__1 : rule__XAssignment__Group_0__1__Impl rule__XAssignment__Group_0__2 ;
public final void rule__XAssignment__Group_0__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24454:1: ( rule__XAssignment__Group_0__1__Impl rule__XAssignment__Group_0__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24455:2: rule__XAssignment__Group_0__1__Impl rule__XAssignment__Group_0__2
{
pushFollow(FollowSets002.FOLLOW_rule__XAssignment__Group_0__1__Impl_in_rule__XAssignment__Group_0__149616);
rule__XAssignment__Group_0__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XAssignment__Group_0__2_in_rule__XAssignment__Group_0__149619);
rule__XAssignment__Group_0__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XAssignment__Group_0__1"
// $ANTLR start "rule__XAssignment__Group_0__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24462:1: rule__XAssignment__Group_0__1__Impl : ( ( rule__XAssignment__FeatureAssignment_0_1 ) ) ;
public final void rule__XAssignment__Group_0__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24466:1: ( ( ( rule__XAssignment__FeatureAssignment_0_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24467:1: ( ( rule__XAssignment__FeatureAssignment_0_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24467:1: ( ( rule__XAssignment__FeatureAssignment_0_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24468:1: ( rule__XAssignment__FeatureAssignment_0_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXAssignmentAccess().getFeatureAssignment_0_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24469:1: ( rule__XAssignment__FeatureAssignment_0_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24469:2: rule__XAssignment__FeatureAssignment_0_1
{
pushFollow(FollowSets002.FOLLOW_rule__XAssignment__FeatureAssignment_0_1_in_rule__XAssignment__Group_0__1__Impl49646);
rule__XAssignment__FeatureAssignment_0_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXAssignmentAccess().getFeatureAssignment_0_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XAssignment__Group_0__1__Impl"
// $ANTLR start "rule__XAssignment__Group_0__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24479:1: rule__XAssignment__Group_0__2 : rule__XAssignment__Group_0__2__Impl rule__XAssignment__Group_0__3 ;
public final void rule__XAssignment__Group_0__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24483:1: ( rule__XAssignment__Group_0__2__Impl rule__XAssignment__Group_0__3 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24484:2: rule__XAssignment__Group_0__2__Impl rule__XAssignment__Group_0__3
{
pushFollow(FollowSets002.FOLLOW_rule__XAssignment__Group_0__2__Impl_in_rule__XAssignment__Group_0__249676);
rule__XAssignment__Group_0__2__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XAssignment__Group_0__3_in_rule__XAssignment__Group_0__249679);
rule__XAssignment__Group_0__3();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XAssignment__Group_0__2"
// $ANTLR start "rule__XAssignment__Group_0__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24491:1: rule__XAssignment__Group_0__2__Impl : ( ruleOpSingleAssign ) ;
public final void rule__XAssignment__Group_0__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24495:1: ( ( ruleOpSingleAssign ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24496:1: ( ruleOpSingleAssign )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24496:1: ( ruleOpSingleAssign )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24497:1: ruleOpSingleAssign
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXAssignmentAccess().getOpSingleAssignParserRuleCall_0_2());
}
pushFollow(FollowSets002.FOLLOW_ruleOpSingleAssign_in_rule__XAssignment__Group_0__2__Impl49706);
ruleOpSingleAssign();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXAssignmentAccess().getOpSingleAssignParserRuleCall_0_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XAssignment__Group_0__2__Impl"
// $ANTLR start "rule__XAssignment__Group_0__3"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24508:1: rule__XAssignment__Group_0__3 : rule__XAssignment__Group_0__3__Impl ;
public final void rule__XAssignment__Group_0__3() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24512:1: ( rule__XAssignment__Group_0__3__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24513:2: rule__XAssignment__Group_0__3__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__XAssignment__Group_0__3__Impl_in_rule__XAssignment__Group_0__349735);
rule__XAssignment__Group_0__3__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XAssignment__Group_0__3"
// $ANTLR start "rule__XAssignment__Group_0__3__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24519:1: rule__XAssignment__Group_0__3__Impl : ( ( rule__XAssignment__ValueAssignment_0_3 ) ) ;
public final void rule__XAssignment__Group_0__3__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24523:1: ( ( ( rule__XAssignment__ValueAssignment_0_3 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24524:1: ( ( rule__XAssignment__ValueAssignment_0_3 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24524:1: ( ( rule__XAssignment__ValueAssignment_0_3 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24525:1: ( rule__XAssignment__ValueAssignment_0_3 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXAssignmentAccess().getValueAssignment_0_3());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24526:1: ( rule__XAssignment__ValueAssignment_0_3 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24526:2: rule__XAssignment__ValueAssignment_0_3
{
pushFollow(FollowSets002.FOLLOW_rule__XAssignment__ValueAssignment_0_3_in_rule__XAssignment__Group_0__3__Impl49762);
rule__XAssignment__ValueAssignment_0_3();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXAssignmentAccess().getValueAssignment_0_3());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XAssignment__Group_0__3__Impl"
// $ANTLR start "rule__XAssignment__Group_1__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24544:1: rule__XAssignment__Group_1__0 : rule__XAssignment__Group_1__0__Impl rule__XAssignment__Group_1__1 ;
public final void rule__XAssignment__Group_1__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24548:1: ( rule__XAssignment__Group_1__0__Impl rule__XAssignment__Group_1__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24549:2: rule__XAssignment__Group_1__0__Impl rule__XAssignment__Group_1__1
{
pushFollow(FollowSets002.FOLLOW_rule__XAssignment__Group_1__0__Impl_in_rule__XAssignment__Group_1__049800);
rule__XAssignment__Group_1__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XAssignment__Group_1__1_in_rule__XAssignment__Group_1__049803);
rule__XAssignment__Group_1__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XAssignment__Group_1__0"
// $ANTLR start "rule__XAssignment__Group_1__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24556:1: rule__XAssignment__Group_1__0__Impl : ( ruleXOrExpression ) ;
public final void rule__XAssignment__Group_1__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24560:1: ( ( ruleXOrExpression ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24561:1: ( ruleXOrExpression )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24561:1: ( ruleXOrExpression )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24562:1: ruleXOrExpression
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXAssignmentAccess().getXOrExpressionParserRuleCall_1_0());
}
pushFollow(FollowSets002.FOLLOW_ruleXOrExpression_in_rule__XAssignment__Group_1__0__Impl49830);
ruleXOrExpression();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXAssignmentAccess().getXOrExpressionParserRuleCall_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XAssignment__Group_1__0__Impl"
// $ANTLR start "rule__XAssignment__Group_1__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24573:1: rule__XAssignment__Group_1__1 : rule__XAssignment__Group_1__1__Impl ;
public final void rule__XAssignment__Group_1__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24577:1: ( rule__XAssignment__Group_1__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24578:2: rule__XAssignment__Group_1__1__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__XAssignment__Group_1__1__Impl_in_rule__XAssignment__Group_1__149859);
rule__XAssignment__Group_1__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XAssignment__Group_1__1"
// $ANTLR start "rule__XAssignment__Group_1__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24584:1: rule__XAssignment__Group_1__1__Impl : ( ( rule__XAssignment__Group_1_1__0 )? ) ;
public final void rule__XAssignment__Group_1__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24588:1: ( ( ( rule__XAssignment__Group_1_1__0 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24589:1: ( ( rule__XAssignment__Group_1_1__0 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24589:1: ( ( rule__XAssignment__Group_1_1__0 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24590:1: ( rule__XAssignment__Group_1_1__0 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXAssignmentAccess().getGroup_1_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24591:1: ( rule__XAssignment__Group_1_1__0 )?
int alt196=2;
alt196 = dfa196.predict(input);
switch (alt196) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24591:2: rule__XAssignment__Group_1_1__0
{
pushFollow(FollowSets002.FOLLOW_rule__XAssignment__Group_1_1__0_in_rule__XAssignment__Group_1__1__Impl49886);
rule__XAssignment__Group_1_1__0();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXAssignmentAccess().getGroup_1_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XAssignment__Group_1__1__Impl"
// $ANTLR start "rule__XAssignment__Group_1_1__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24605:1: rule__XAssignment__Group_1_1__0 : rule__XAssignment__Group_1_1__0__Impl rule__XAssignment__Group_1_1__1 ;
public final void rule__XAssignment__Group_1_1__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24609:1: ( rule__XAssignment__Group_1_1__0__Impl rule__XAssignment__Group_1_1__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24610:2: rule__XAssignment__Group_1_1__0__Impl rule__XAssignment__Group_1_1__1
{
pushFollow(FollowSets002.FOLLOW_rule__XAssignment__Group_1_1__0__Impl_in_rule__XAssignment__Group_1_1__049921);
rule__XAssignment__Group_1_1__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XAssignment__Group_1_1__1_in_rule__XAssignment__Group_1_1__049924);
rule__XAssignment__Group_1_1__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XAssignment__Group_1_1__0"
// $ANTLR start "rule__XAssignment__Group_1_1__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24617:1: rule__XAssignment__Group_1_1__0__Impl : ( ( rule__XAssignment__Group_1_1_0__0 ) ) ;
public final void rule__XAssignment__Group_1_1__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24621:1: ( ( ( rule__XAssignment__Group_1_1_0__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24622:1: ( ( rule__XAssignment__Group_1_1_0__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24622:1: ( ( rule__XAssignment__Group_1_1_0__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24623:1: ( rule__XAssignment__Group_1_1_0__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXAssignmentAccess().getGroup_1_1_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24624:1: ( rule__XAssignment__Group_1_1_0__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24624:2: rule__XAssignment__Group_1_1_0__0
{
pushFollow(FollowSets002.FOLLOW_rule__XAssignment__Group_1_1_0__0_in_rule__XAssignment__Group_1_1__0__Impl49951);
rule__XAssignment__Group_1_1_0__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXAssignmentAccess().getGroup_1_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XAssignment__Group_1_1__0__Impl"
// $ANTLR start "rule__XAssignment__Group_1_1__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24634:1: rule__XAssignment__Group_1_1__1 : rule__XAssignment__Group_1_1__1__Impl ;
public final void rule__XAssignment__Group_1_1__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24638:1: ( rule__XAssignment__Group_1_1__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24639:2: rule__XAssignment__Group_1_1__1__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__XAssignment__Group_1_1__1__Impl_in_rule__XAssignment__Group_1_1__149981);
rule__XAssignment__Group_1_1__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XAssignment__Group_1_1__1"
// $ANTLR start "rule__XAssignment__Group_1_1__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24645:1: rule__XAssignment__Group_1_1__1__Impl : ( ( rule__XAssignment__RightOperandAssignment_1_1_1 ) ) ;
public final void rule__XAssignment__Group_1_1__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24649:1: ( ( ( rule__XAssignment__RightOperandAssignment_1_1_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24650:1: ( ( rule__XAssignment__RightOperandAssignment_1_1_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24650:1: ( ( rule__XAssignment__RightOperandAssignment_1_1_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24651:1: ( rule__XAssignment__RightOperandAssignment_1_1_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXAssignmentAccess().getRightOperandAssignment_1_1_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24652:1: ( rule__XAssignment__RightOperandAssignment_1_1_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24652:2: rule__XAssignment__RightOperandAssignment_1_1_1
{
pushFollow(FollowSets002.FOLLOW_rule__XAssignment__RightOperandAssignment_1_1_1_in_rule__XAssignment__Group_1_1__1__Impl50008);
rule__XAssignment__RightOperandAssignment_1_1_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXAssignmentAccess().getRightOperandAssignment_1_1_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XAssignment__Group_1_1__1__Impl"
// $ANTLR start "rule__XAssignment__Group_1_1_0__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24666:1: rule__XAssignment__Group_1_1_0__0 : rule__XAssignment__Group_1_1_0__0__Impl ;
public final void rule__XAssignment__Group_1_1_0__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24670:1: ( rule__XAssignment__Group_1_1_0__0__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24671:2: rule__XAssignment__Group_1_1_0__0__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__XAssignment__Group_1_1_0__0__Impl_in_rule__XAssignment__Group_1_1_0__050042);
rule__XAssignment__Group_1_1_0__0__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XAssignment__Group_1_1_0__0"
// $ANTLR start "rule__XAssignment__Group_1_1_0__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24677:1: rule__XAssignment__Group_1_1_0__0__Impl : ( ( rule__XAssignment__Group_1_1_0_0__0 ) ) ;
public final void rule__XAssignment__Group_1_1_0__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24681:1: ( ( ( rule__XAssignment__Group_1_1_0_0__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24682:1: ( ( rule__XAssignment__Group_1_1_0_0__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24682:1: ( ( rule__XAssignment__Group_1_1_0_0__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24683:1: ( rule__XAssignment__Group_1_1_0_0__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXAssignmentAccess().getGroup_1_1_0_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24684:1: ( rule__XAssignment__Group_1_1_0_0__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24684:2: rule__XAssignment__Group_1_1_0_0__0
{
pushFollow(FollowSets002.FOLLOW_rule__XAssignment__Group_1_1_0_0__0_in_rule__XAssignment__Group_1_1_0__0__Impl50069);
rule__XAssignment__Group_1_1_0_0__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXAssignmentAccess().getGroup_1_1_0_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XAssignment__Group_1_1_0__0__Impl"
// $ANTLR start "rule__XAssignment__Group_1_1_0_0__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24696:1: rule__XAssignment__Group_1_1_0_0__0 : rule__XAssignment__Group_1_1_0_0__0__Impl rule__XAssignment__Group_1_1_0_0__1 ;
public final void rule__XAssignment__Group_1_1_0_0__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24700:1: ( rule__XAssignment__Group_1_1_0_0__0__Impl rule__XAssignment__Group_1_1_0_0__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24701:2: rule__XAssignment__Group_1_1_0_0__0__Impl rule__XAssignment__Group_1_1_0_0__1
{
pushFollow(FollowSets002.FOLLOW_rule__XAssignment__Group_1_1_0_0__0__Impl_in_rule__XAssignment__Group_1_1_0_0__050101);
rule__XAssignment__Group_1_1_0_0__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XAssignment__Group_1_1_0_0__1_in_rule__XAssignment__Group_1_1_0_0__050104);
rule__XAssignment__Group_1_1_0_0__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XAssignment__Group_1_1_0_0__0"
// $ANTLR start "rule__XAssignment__Group_1_1_0_0__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24708:1: rule__XAssignment__Group_1_1_0_0__0__Impl : ( () ) ;
public final void rule__XAssignment__Group_1_1_0_0__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24712:1: ( ( () ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24713:1: ( () )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24713:1: ( () )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24714:1: ()
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXAssignmentAccess().getXBinaryOperationLeftOperandAction_1_1_0_0_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24715:1: ()
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24717:1:
{
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXAssignmentAccess().getXBinaryOperationLeftOperandAction_1_1_0_0_0());
}
}
}
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XAssignment__Group_1_1_0_0__0__Impl"
// $ANTLR start "rule__XAssignment__Group_1_1_0_0__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24727:1: rule__XAssignment__Group_1_1_0_0__1 : rule__XAssignment__Group_1_1_0_0__1__Impl ;
public final void rule__XAssignment__Group_1_1_0_0__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24731:1: ( rule__XAssignment__Group_1_1_0_0__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24732:2: rule__XAssignment__Group_1_1_0_0__1__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__XAssignment__Group_1_1_0_0__1__Impl_in_rule__XAssignment__Group_1_1_0_0__150162);
rule__XAssignment__Group_1_1_0_0__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XAssignment__Group_1_1_0_0__1"
// $ANTLR start "rule__XAssignment__Group_1_1_0_0__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24738:1: rule__XAssignment__Group_1_1_0_0__1__Impl : ( ( rule__XAssignment__FeatureAssignment_1_1_0_0_1 ) ) ;
public final void rule__XAssignment__Group_1_1_0_0__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24742:1: ( ( ( rule__XAssignment__FeatureAssignment_1_1_0_0_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24743:1: ( ( rule__XAssignment__FeatureAssignment_1_1_0_0_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24743:1: ( ( rule__XAssignment__FeatureAssignment_1_1_0_0_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24744:1: ( rule__XAssignment__FeatureAssignment_1_1_0_0_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXAssignmentAccess().getFeatureAssignment_1_1_0_0_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24745:1: ( rule__XAssignment__FeatureAssignment_1_1_0_0_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24745:2: rule__XAssignment__FeatureAssignment_1_1_0_0_1
{
pushFollow(FollowSets002.FOLLOW_rule__XAssignment__FeatureAssignment_1_1_0_0_1_in_rule__XAssignment__Group_1_1_0_0__1__Impl50189);
rule__XAssignment__FeatureAssignment_1_1_0_0_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXAssignmentAccess().getFeatureAssignment_1_1_0_0_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XAssignment__Group_1_1_0_0__1__Impl"
// $ANTLR start "rule__OpMultiAssign__Group_5__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24759:1: rule__OpMultiAssign__Group_5__0 : rule__OpMultiAssign__Group_5__0__Impl rule__OpMultiAssign__Group_5__1 ;
public final void rule__OpMultiAssign__Group_5__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24763:1: ( rule__OpMultiAssign__Group_5__0__Impl rule__OpMultiAssign__Group_5__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24764:2: rule__OpMultiAssign__Group_5__0__Impl rule__OpMultiAssign__Group_5__1
{
pushFollow(FollowSets002.FOLLOW_rule__OpMultiAssign__Group_5__0__Impl_in_rule__OpMultiAssign__Group_5__050223);
rule__OpMultiAssign__Group_5__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__OpMultiAssign__Group_5__1_in_rule__OpMultiAssign__Group_5__050226);
rule__OpMultiAssign__Group_5__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__OpMultiAssign__Group_5__0"
// $ANTLR start "rule__OpMultiAssign__Group_5__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24771:1: rule__OpMultiAssign__Group_5__0__Impl : ( '<' ) ;
public final void rule__OpMultiAssign__Group_5__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24775:1: ( ( '<' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24776:1: ( '<' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24776:1: ( '<' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24777:1: '<'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getOpMultiAssignAccess().getLessThanSignKeyword_5_0());
}
match(input,27,FollowSets002.FOLLOW_27_in_rule__OpMultiAssign__Group_5__0__Impl50254); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getOpMultiAssignAccess().getLessThanSignKeyword_5_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__OpMultiAssign__Group_5__0__Impl"
// $ANTLR start "rule__OpMultiAssign__Group_5__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24790:1: rule__OpMultiAssign__Group_5__1 : rule__OpMultiAssign__Group_5__1__Impl rule__OpMultiAssign__Group_5__2 ;
public final void rule__OpMultiAssign__Group_5__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24794:1: ( rule__OpMultiAssign__Group_5__1__Impl rule__OpMultiAssign__Group_5__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24795:2: rule__OpMultiAssign__Group_5__1__Impl rule__OpMultiAssign__Group_5__2
{
pushFollow(FollowSets002.FOLLOW_rule__OpMultiAssign__Group_5__1__Impl_in_rule__OpMultiAssign__Group_5__150285);
rule__OpMultiAssign__Group_5__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__OpMultiAssign__Group_5__2_in_rule__OpMultiAssign__Group_5__150288);
rule__OpMultiAssign__Group_5__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__OpMultiAssign__Group_5__1"
// $ANTLR start "rule__OpMultiAssign__Group_5__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24802:1: rule__OpMultiAssign__Group_5__1__Impl : ( '<' ) ;
public final void rule__OpMultiAssign__Group_5__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24806:1: ( ( '<' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24807:1: ( '<' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24807:1: ( '<' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24808:1: '<'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getOpMultiAssignAccess().getLessThanSignKeyword_5_1());
}
match(input,27,FollowSets002.FOLLOW_27_in_rule__OpMultiAssign__Group_5__1__Impl50316); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getOpMultiAssignAccess().getLessThanSignKeyword_5_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__OpMultiAssign__Group_5__1__Impl"
// $ANTLR start "rule__OpMultiAssign__Group_5__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24821:1: rule__OpMultiAssign__Group_5__2 : rule__OpMultiAssign__Group_5__2__Impl ;
public final void rule__OpMultiAssign__Group_5__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24825:1: ( rule__OpMultiAssign__Group_5__2__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24826:2: rule__OpMultiAssign__Group_5__2__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__OpMultiAssign__Group_5__2__Impl_in_rule__OpMultiAssign__Group_5__250347);
rule__OpMultiAssign__Group_5__2__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__OpMultiAssign__Group_5__2"
// $ANTLR start "rule__OpMultiAssign__Group_5__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24832:1: rule__OpMultiAssign__Group_5__2__Impl : ( '=' ) ;
public final void rule__OpMultiAssign__Group_5__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24836:1: ( ( '=' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24837:1: ( '=' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24837:1: ( '=' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24838:1: '='
{
if ( state.backtracking==0 ) {
before(grammarAccess.getOpMultiAssignAccess().getEqualsSignKeyword_5_2());
}
match(input,13,FollowSets002.FOLLOW_13_in_rule__OpMultiAssign__Group_5__2__Impl50375); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getOpMultiAssignAccess().getEqualsSignKeyword_5_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__OpMultiAssign__Group_5__2__Impl"
// $ANTLR start "rule__OpMultiAssign__Group_6__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24857:1: rule__OpMultiAssign__Group_6__0 : rule__OpMultiAssign__Group_6__0__Impl rule__OpMultiAssign__Group_6__1 ;
public final void rule__OpMultiAssign__Group_6__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24861:1: ( rule__OpMultiAssign__Group_6__0__Impl rule__OpMultiAssign__Group_6__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24862:2: rule__OpMultiAssign__Group_6__0__Impl rule__OpMultiAssign__Group_6__1
{
pushFollow(FollowSets002.FOLLOW_rule__OpMultiAssign__Group_6__0__Impl_in_rule__OpMultiAssign__Group_6__050412);
rule__OpMultiAssign__Group_6__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__OpMultiAssign__Group_6__1_in_rule__OpMultiAssign__Group_6__050415);
rule__OpMultiAssign__Group_6__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__OpMultiAssign__Group_6__0"
// $ANTLR start "rule__OpMultiAssign__Group_6__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24869:1: rule__OpMultiAssign__Group_6__0__Impl : ( '>' ) ;
public final void rule__OpMultiAssign__Group_6__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24873:1: ( ( '>' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24874:1: ( '>' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24874:1: ( '>' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24875:1: '>'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getOpMultiAssignAccess().getGreaterThanSignKeyword_6_0());
}
match(input,26,FollowSets002.FOLLOW_26_in_rule__OpMultiAssign__Group_6__0__Impl50443); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getOpMultiAssignAccess().getGreaterThanSignKeyword_6_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__OpMultiAssign__Group_6__0__Impl"
// $ANTLR start "rule__OpMultiAssign__Group_6__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24888:1: rule__OpMultiAssign__Group_6__1 : rule__OpMultiAssign__Group_6__1__Impl rule__OpMultiAssign__Group_6__2 ;
public final void rule__OpMultiAssign__Group_6__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24892:1: ( rule__OpMultiAssign__Group_6__1__Impl rule__OpMultiAssign__Group_6__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24893:2: rule__OpMultiAssign__Group_6__1__Impl rule__OpMultiAssign__Group_6__2
{
pushFollow(FollowSets002.FOLLOW_rule__OpMultiAssign__Group_6__1__Impl_in_rule__OpMultiAssign__Group_6__150474);
rule__OpMultiAssign__Group_6__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__OpMultiAssign__Group_6__2_in_rule__OpMultiAssign__Group_6__150477);
rule__OpMultiAssign__Group_6__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__OpMultiAssign__Group_6__1"
// $ANTLR start "rule__OpMultiAssign__Group_6__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24900:1: rule__OpMultiAssign__Group_6__1__Impl : ( ( '>' )? ) ;
public final void rule__OpMultiAssign__Group_6__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24904:1: ( ( ( '>' )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24905:1: ( ( '>' )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24905:1: ( ( '>' )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24906:1: ( '>' )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getOpMultiAssignAccess().getGreaterThanSignKeyword_6_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24907:1: ( '>' )?
int alt197=2;
int LA197_0 = input.LA(1);
if ( (LA197_0==26) ) {
alt197=1;
}
switch (alt197) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24908:2: '>'
{
match(input,26,FollowSets002.FOLLOW_26_in_rule__OpMultiAssign__Group_6__1__Impl50506); if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getOpMultiAssignAccess().getGreaterThanSignKeyword_6_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__OpMultiAssign__Group_6__1__Impl"
// $ANTLR start "rule__OpMultiAssign__Group_6__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24919:1: rule__OpMultiAssign__Group_6__2 : rule__OpMultiAssign__Group_6__2__Impl ;
public final void rule__OpMultiAssign__Group_6__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24923:1: ( rule__OpMultiAssign__Group_6__2__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24924:2: rule__OpMultiAssign__Group_6__2__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__OpMultiAssign__Group_6__2__Impl_in_rule__OpMultiAssign__Group_6__250539);
rule__OpMultiAssign__Group_6__2__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__OpMultiAssign__Group_6__2"
// $ANTLR start "rule__OpMultiAssign__Group_6__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24930:1: rule__OpMultiAssign__Group_6__2__Impl : ( '>=' ) ;
public final void rule__OpMultiAssign__Group_6__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24934:1: ( ( '>=' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24935:1: ( '>=' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24935:1: ( '>=' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24936:1: '>='
{
if ( state.backtracking==0 ) {
before(grammarAccess.getOpMultiAssignAccess().getGreaterThanSignEqualsSignKeyword_6_2());
}
match(input,25,FollowSets002.FOLLOW_25_in_rule__OpMultiAssign__Group_6__2__Impl50567); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getOpMultiAssignAccess().getGreaterThanSignEqualsSignKeyword_6_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__OpMultiAssign__Group_6__2__Impl"
// $ANTLR start "rule__XOrExpression__Group__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24955:1: rule__XOrExpression__Group__0 : rule__XOrExpression__Group__0__Impl rule__XOrExpression__Group__1 ;
public final void rule__XOrExpression__Group__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24959:1: ( rule__XOrExpression__Group__0__Impl rule__XOrExpression__Group__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24960:2: rule__XOrExpression__Group__0__Impl rule__XOrExpression__Group__1
{
pushFollow(FollowSets002.FOLLOW_rule__XOrExpression__Group__0__Impl_in_rule__XOrExpression__Group__050604);
rule__XOrExpression__Group__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XOrExpression__Group__1_in_rule__XOrExpression__Group__050607);
rule__XOrExpression__Group__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XOrExpression__Group__0"
// $ANTLR start "rule__XOrExpression__Group__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24967:1: rule__XOrExpression__Group__0__Impl : ( ruleXAndExpression ) ;
public final void rule__XOrExpression__Group__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24971:1: ( ( ruleXAndExpression ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24972:1: ( ruleXAndExpression )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24972:1: ( ruleXAndExpression )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24973:1: ruleXAndExpression
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXOrExpressionAccess().getXAndExpressionParserRuleCall_0());
}
pushFollow(FollowSets002.FOLLOW_ruleXAndExpression_in_rule__XOrExpression__Group__0__Impl50634);
ruleXAndExpression();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXOrExpressionAccess().getXAndExpressionParserRuleCall_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XOrExpression__Group__0__Impl"
// $ANTLR start "rule__XOrExpression__Group__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24984:1: rule__XOrExpression__Group__1 : rule__XOrExpression__Group__1__Impl ;
public final void rule__XOrExpression__Group__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24988:1: ( rule__XOrExpression__Group__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24989:2: rule__XOrExpression__Group__1__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__XOrExpression__Group__1__Impl_in_rule__XOrExpression__Group__150663);
rule__XOrExpression__Group__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XOrExpression__Group__1"
// $ANTLR start "rule__XOrExpression__Group__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24995:1: rule__XOrExpression__Group__1__Impl : ( ( rule__XOrExpression__Group_1__0 )* ) ;
public final void rule__XOrExpression__Group__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24999:1: ( ( ( rule__XOrExpression__Group_1__0 )* ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25000:1: ( ( rule__XOrExpression__Group_1__0 )* )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25000:1: ( ( rule__XOrExpression__Group_1__0 )* )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25001:1: ( rule__XOrExpression__Group_1__0 )*
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXOrExpressionAccess().getGroup_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25002:1: ( rule__XOrExpression__Group_1__0 )*
loop198:
do {
int alt198=2;
int LA198_0 = input.LA(1);
if ( (LA198_0==14) ) {
int LA198_2 = input.LA(2);
if ( (synpred284_InternalMango()) ) {
alt198=1;
}
}
switch (alt198) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25002:2: rule__XOrExpression__Group_1__0
{
pushFollow(FollowSets002.FOLLOW_rule__XOrExpression__Group_1__0_in_rule__XOrExpression__Group__1__Impl50690);
rule__XOrExpression__Group_1__0();
state._fsp--;
if (state.failed) return ;
}
break;
default :
break loop198;
}
} while (true);
if ( state.backtracking==0 ) {
after(grammarAccess.getXOrExpressionAccess().getGroup_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XOrExpression__Group__1__Impl"
// $ANTLR start "rule__XOrExpression__Group_1__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25016:1: rule__XOrExpression__Group_1__0 : rule__XOrExpression__Group_1__0__Impl rule__XOrExpression__Group_1__1 ;
public final void rule__XOrExpression__Group_1__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25020:1: ( rule__XOrExpression__Group_1__0__Impl rule__XOrExpression__Group_1__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25021:2: rule__XOrExpression__Group_1__0__Impl rule__XOrExpression__Group_1__1
{
pushFollow(FollowSets002.FOLLOW_rule__XOrExpression__Group_1__0__Impl_in_rule__XOrExpression__Group_1__050725);
rule__XOrExpression__Group_1__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XOrExpression__Group_1__1_in_rule__XOrExpression__Group_1__050728);
rule__XOrExpression__Group_1__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XOrExpression__Group_1__0"
// $ANTLR start "rule__XOrExpression__Group_1__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25028:1: rule__XOrExpression__Group_1__0__Impl : ( ( rule__XOrExpression__Group_1_0__0 ) ) ;
public final void rule__XOrExpression__Group_1__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25032:1: ( ( ( rule__XOrExpression__Group_1_0__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25033:1: ( ( rule__XOrExpression__Group_1_0__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25033:1: ( ( rule__XOrExpression__Group_1_0__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25034:1: ( rule__XOrExpression__Group_1_0__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXOrExpressionAccess().getGroup_1_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25035:1: ( rule__XOrExpression__Group_1_0__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25035:2: rule__XOrExpression__Group_1_0__0
{
pushFollow(FollowSets002.FOLLOW_rule__XOrExpression__Group_1_0__0_in_rule__XOrExpression__Group_1__0__Impl50755);
rule__XOrExpression__Group_1_0__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXOrExpressionAccess().getGroup_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XOrExpression__Group_1__0__Impl"
// $ANTLR start "rule__XOrExpression__Group_1__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25045:1: rule__XOrExpression__Group_1__1 : rule__XOrExpression__Group_1__1__Impl ;
public final void rule__XOrExpression__Group_1__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25049:1: ( rule__XOrExpression__Group_1__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25050:2: rule__XOrExpression__Group_1__1__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__XOrExpression__Group_1__1__Impl_in_rule__XOrExpression__Group_1__150785);
rule__XOrExpression__Group_1__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XOrExpression__Group_1__1"
// $ANTLR start "rule__XOrExpression__Group_1__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25056:1: rule__XOrExpression__Group_1__1__Impl : ( ( rule__XOrExpression__RightOperandAssignment_1_1 ) ) ;
public final void rule__XOrExpression__Group_1__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25060:1: ( ( ( rule__XOrExpression__RightOperandAssignment_1_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25061:1: ( ( rule__XOrExpression__RightOperandAssignment_1_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25061:1: ( ( rule__XOrExpression__RightOperandAssignment_1_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25062:1: ( rule__XOrExpression__RightOperandAssignment_1_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXOrExpressionAccess().getRightOperandAssignment_1_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25063:1: ( rule__XOrExpression__RightOperandAssignment_1_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25063:2: rule__XOrExpression__RightOperandAssignment_1_1
{
pushFollow(FollowSets002.FOLLOW_rule__XOrExpression__RightOperandAssignment_1_1_in_rule__XOrExpression__Group_1__1__Impl50812);
rule__XOrExpression__RightOperandAssignment_1_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXOrExpressionAccess().getRightOperandAssignment_1_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XOrExpression__Group_1__1__Impl"
// $ANTLR start "rule__XOrExpression__Group_1_0__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25077:1: rule__XOrExpression__Group_1_0__0 : rule__XOrExpression__Group_1_0__0__Impl ;
public final void rule__XOrExpression__Group_1_0__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25081:1: ( rule__XOrExpression__Group_1_0__0__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25082:2: rule__XOrExpression__Group_1_0__0__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__XOrExpression__Group_1_0__0__Impl_in_rule__XOrExpression__Group_1_0__050846);
rule__XOrExpression__Group_1_0__0__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XOrExpression__Group_1_0__0"
// $ANTLR start "rule__XOrExpression__Group_1_0__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25088:1: rule__XOrExpression__Group_1_0__0__Impl : ( ( rule__XOrExpression__Group_1_0_0__0 ) ) ;
public final void rule__XOrExpression__Group_1_0__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25092:1: ( ( ( rule__XOrExpression__Group_1_0_0__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25093:1: ( ( rule__XOrExpression__Group_1_0_0__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25093:1: ( ( rule__XOrExpression__Group_1_0_0__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25094:1: ( rule__XOrExpression__Group_1_0_0__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXOrExpressionAccess().getGroup_1_0_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25095:1: ( rule__XOrExpression__Group_1_0_0__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25095:2: rule__XOrExpression__Group_1_0_0__0
{
pushFollow(FollowSets002.FOLLOW_rule__XOrExpression__Group_1_0_0__0_in_rule__XOrExpression__Group_1_0__0__Impl50873);
rule__XOrExpression__Group_1_0_0__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXOrExpressionAccess().getGroup_1_0_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XOrExpression__Group_1_0__0__Impl"
// $ANTLR start "rule__XOrExpression__Group_1_0_0__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25107:1: rule__XOrExpression__Group_1_0_0__0 : rule__XOrExpression__Group_1_0_0__0__Impl rule__XOrExpression__Group_1_0_0__1 ;
public final void rule__XOrExpression__Group_1_0_0__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25111:1: ( rule__XOrExpression__Group_1_0_0__0__Impl rule__XOrExpression__Group_1_0_0__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25112:2: rule__XOrExpression__Group_1_0_0__0__Impl rule__XOrExpression__Group_1_0_0__1
{
pushFollow(FollowSets002.FOLLOW_rule__XOrExpression__Group_1_0_0__0__Impl_in_rule__XOrExpression__Group_1_0_0__050905);
rule__XOrExpression__Group_1_0_0__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XOrExpression__Group_1_0_0__1_in_rule__XOrExpression__Group_1_0_0__050908);
rule__XOrExpression__Group_1_0_0__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XOrExpression__Group_1_0_0__0"
// $ANTLR start "rule__XOrExpression__Group_1_0_0__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25119:1: rule__XOrExpression__Group_1_0_0__0__Impl : ( () ) ;
public final void rule__XOrExpression__Group_1_0_0__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25123:1: ( ( () ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25124:1: ( () )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25124:1: ( () )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25125:1: ()
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXOrExpressionAccess().getXBinaryOperationLeftOperandAction_1_0_0_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25126:1: ()
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25128:1:
{
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXOrExpressionAccess().getXBinaryOperationLeftOperandAction_1_0_0_0());
}
}
}
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XOrExpression__Group_1_0_0__0__Impl"
// $ANTLR start "rule__XOrExpression__Group_1_0_0__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25138:1: rule__XOrExpression__Group_1_0_0__1 : rule__XOrExpression__Group_1_0_0__1__Impl ;
public final void rule__XOrExpression__Group_1_0_0__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25142:1: ( rule__XOrExpression__Group_1_0_0__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25143:2: rule__XOrExpression__Group_1_0_0__1__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__XOrExpression__Group_1_0_0__1__Impl_in_rule__XOrExpression__Group_1_0_0__150966);
rule__XOrExpression__Group_1_0_0__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XOrExpression__Group_1_0_0__1"
// $ANTLR start "rule__XOrExpression__Group_1_0_0__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25149:1: rule__XOrExpression__Group_1_0_0__1__Impl : ( ( rule__XOrExpression__FeatureAssignment_1_0_0_1 ) ) ;
public final void rule__XOrExpression__Group_1_0_0__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25153:1: ( ( ( rule__XOrExpression__FeatureAssignment_1_0_0_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25154:1: ( ( rule__XOrExpression__FeatureAssignment_1_0_0_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25154:1: ( ( rule__XOrExpression__FeatureAssignment_1_0_0_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25155:1: ( rule__XOrExpression__FeatureAssignment_1_0_0_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXOrExpressionAccess().getFeatureAssignment_1_0_0_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25156:1: ( rule__XOrExpression__FeatureAssignment_1_0_0_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25156:2: rule__XOrExpression__FeatureAssignment_1_0_0_1
{
pushFollow(FollowSets002.FOLLOW_rule__XOrExpression__FeatureAssignment_1_0_0_1_in_rule__XOrExpression__Group_1_0_0__1__Impl50993);
rule__XOrExpression__FeatureAssignment_1_0_0_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXOrExpressionAccess().getFeatureAssignment_1_0_0_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XOrExpression__Group_1_0_0__1__Impl"
// $ANTLR start "rule__XAndExpression__Group__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25170:1: rule__XAndExpression__Group__0 : rule__XAndExpression__Group__0__Impl rule__XAndExpression__Group__1 ;
public final void rule__XAndExpression__Group__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25174:1: ( rule__XAndExpression__Group__0__Impl rule__XAndExpression__Group__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25175:2: rule__XAndExpression__Group__0__Impl rule__XAndExpression__Group__1
{
pushFollow(FollowSets002.FOLLOW_rule__XAndExpression__Group__0__Impl_in_rule__XAndExpression__Group__051027);
rule__XAndExpression__Group__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XAndExpression__Group__1_in_rule__XAndExpression__Group__051030);
rule__XAndExpression__Group__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XAndExpression__Group__0"
// $ANTLR start "rule__XAndExpression__Group__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25182:1: rule__XAndExpression__Group__0__Impl : ( ruleXEqualityExpression ) ;
public final void rule__XAndExpression__Group__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25186:1: ( ( ruleXEqualityExpression ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25187:1: ( ruleXEqualityExpression )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25187:1: ( ruleXEqualityExpression )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25188:1: ruleXEqualityExpression
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXAndExpressionAccess().getXEqualityExpressionParserRuleCall_0());
}
pushFollow(FollowSets002.FOLLOW_ruleXEqualityExpression_in_rule__XAndExpression__Group__0__Impl51057);
ruleXEqualityExpression();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXAndExpressionAccess().getXEqualityExpressionParserRuleCall_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XAndExpression__Group__0__Impl"
// $ANTLR start "rule__XAndExpression__Group__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25199:1: rule__XAndExpression__Group__1 : rule__XAndExpression__Group__1__Impl ;
public final void rule__XAndExpression__Group__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25203:1: ( rule__XAndExpression__Group__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25204:2: rule__XAndExpression__Group__1__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__XAndExpression__Group__1__Impl_in_rule__XAndExpression__Group__151086);
rule__XAndExpression__Group__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XAndExpression__Group__1"
// $ANTLR start "rule__XAndExpression__Group__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25210:1: rule__XAndExpression__Group__1__Impl : ( ( rule__XAndExpression__Group_1__0 )* ) ;
public final void rule__XAndExpression__Group__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25214:1: ( ( ( rule__XAndExpression__Group_1__0 )* ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25215:1: ( ( rule__XAndExpression__Group_1__0 )* )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25215:1: ( ( rule__XAndExpression__Group_1__0 )* )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25216:1: ( rule__XAndExpression__Group_1__0 )*
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXAndExpressionAccess().getGroup_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25217:1: ( rule__XAndExpression__Group_1__0 )*
loop199:
do {
int alt199=2;
int LA199_0 = input.LA(1);
if ( (LA199_0==15) ) {
int LA199_2 = input.LA(2);
if ( (synpred285_InternalMango()) ) {
alt199=1;
}
}
switch (alt199) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25217:2: rule__XAndExpression__Group_1__0
{
pushFollow(FollowSets002.FOLLOW_rule__XAndExpression__Group_1__0_in_rule__XAndExpression__Group__1__Impl51113);
rule__XAndExpression__Group_1__0();
state._fsp--;
if (state.failed) return ;
}
break;
default :
break loop199;
}
} while (true);
if ( state.backtracking==0 ) {
after(grammarAccess.getXAndExpressionAccess().getGroup_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XAndExpression__Group__1__Impl"
// $ANTLR start "rule__XAndExpression__Group_1__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25231:1: rule__XAndExpression__Group_1__0 : rule__XAndExpression__Group_1__0__Impl rule__XAndExpression__Group_1__1 ;
public final void rule__XAndExpression__Group_1__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25235:1: ( rule__XAndExpression__Group_1__0__Impl rule__XAndExpression__Group_1__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25236:2: rule__XAndExpression__Group_1__0__Impl rule__XAndExpression__Group_1__1
{
pushFollow(FollowSets002.FOLLOW_rule__XAndExpression__Group_1__0__Impl_in_rule__XAndExpression__Group_1__051148);
rule__XAndExpression__Group_1__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XAndExpression__Group_1__1_in_rule__XAndExpression__Group_1__051151);
rule__XAndExpression__Group_1__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XAndExpression__Group_1__0"
// $ANTLR start "rule__XAndExpression__Group_1__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25243:1: rule__XAndExpression__Group_1__0__Impl : ( ( rule__XAndExpression__Group_1_0__0 ) ) ;
public final void rule__XAndExpression__Group_1__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25247:1: ( ( ( rule__XAndExpression__Group_1_0__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25248:1: ( ( rule__XAndExpression__Group_1_0__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25248:1: ( ( rule__XAndExpression__Group_1_0__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25249:1: ( rule__XAndExpression__Group_1_0__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXAndExpressionAccess().getGroup_1_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25250:1: ( rule__XAndExpression__Group_1_0__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25250:2: rule__XAndExpression__Group_1_0__0
{
pushFollow(FollowSets002.FOLLOW_rule__XAndExpression__Group_1_0__0_in_rule__XAndExpression__Group_1__0__Impl51178);
rule__XAndExpression__Group_1_0__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXAndExpressionAccess().getGroup_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XAndExpression__Group_1__0__Impl"
// $ANTLR start "rule__XAndExpression__Group_1__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25260:1: rule__XAndExpression__Group_1__1 : rule__XAndExpression__Group_1__1__Impl ;
public final void rule__XAndExpression__Group_1__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25264:1: ( rule__XAndExpression__Group_1__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25265:2: rule__XAndExpression__Group_1__1__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__XAndExpression__Group_1__1__Impl_in_rule__XAndExpression__Group_1__151208);
rule__XAndExpression__Group_1__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XAndExpression__Group_1__1"
// $ANTLR start "rule__XAndExpression__Group_1__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25271:1: rule__XAndExpression__Group_1__1__Impl : ( ( rule__XAndExpression__RightOperandAssignment_1_1 ) ) ;
public final void rule__XAndExpression__Group_1__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25275:1: ( ( ( rule__XAndExpression__RightOperandAssignment_1_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25276:1: ( ( rule__XAndExpression__RightOperandAssignment_1_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25276:1: ( ( rule__XAndExpression__RightOperandAssignment_1_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25277:1: ( rule__XAndExpression__RightOperandAssignment_1_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXAndExpressionAccess().getRightOperandAssignment_1_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25278:1: ( rule__XAndExpression__RightOperandAssignment_1_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25278:2: rule__XAndExpression__RightOperandAssignment_1_1
{
pushFollow(FollowSets002.FOLLOW_rule__XAndExpression__RightOperandAssignment_1_1_in_rule__XAndExpression__Group_1__1__Impl51235);
rule__XAndExpression__RightOperandAssignment_1_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXAndExpressionAccess().getRightOperandAssignment_1_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XAndExpression__Group_1__1__Impl"
// $ANTLR start "rule__XAndExpression__Group_1_0__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25292:1: rule__XAndExpression__Group_1_0__0 : rule__XAndExpression__Group_1_0__0__Impl ;
public final void rule__XAndExpression__Group_1_0__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25296:1: ( rule__XAndExpression__Group_1_0__0__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25297:2: rule__XAndExpression__Group_1_0__0__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__XAndExpression__Group_1_0__0__Impl_in_rule__XAndExpression__Group_1_0__051269);
rule__XAndExpression__Group_1_0__0__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XAndExpression__Group_1_0__0"
// $ANTLR start "rule__XAndExpression__Group_1_0__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25303:1: rule__XAndExpression__Group_1_0__0__Impl : ( ( rule__XAndExpression__Group_1_0_0__0 ) ) ;
public final void rule__XAndExpression__Group_1_0__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25307:1: ( ( ( rule__XAndExpression__Group_1_0_0__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25308:1: ( ( rule__XAndExpression__Group_1_0_0__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25308:1: ( ( rule__XAndExpression__Group_1_0_0__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25309:1: ( rule__XAndExpression__Group_1_0_0__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXAndExpressionAccess().getGroup_1_0_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25310:1: ( rule__XAndExpression__Group_1_0_0__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25310:2: rule__XAndExpression__Group_1_0_0__0
{
pushFollow(FollowSets002.FOLLOW_rule__XAndExpression__Group_1_0_0__0_in_rule__XAndExpression__Group_1_0__0__Impl51296);
rule__XAndExpression__Group_1_0_0__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXAndExpressionAccess().getGroup_1_0_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XAndExpression__Group_1_0__0__Impl"
// $ANTLR start "rule__XAndExpression__Group_1_0_0__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25322:1: rule__XAndExpression__Group_1_0_0__0 : rule__XAndExpression__Group_1_0_0__0__Impl rule__XAndExpression__Group_1_0_0__1 ;
public final void rule__XAndExpression__Group_1_0_0__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25326:1: ( rule__XAndExpression__Group_1_0_0__0__Impl rule__XAndExpression__Group_1_0_0__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25327:2: rule__XAndExpression__Group_1_0_0__0__Impl rule__XAndExpression__Group_1_0_0__1
{
pushFollow(FollowSets002.FOLLOW_rule__XAndExpression__Group_1_0_0__0__Impl_in_rule__XAndExpression__Group_1_0_0__051328);
rule__XAndExpression__Group_1_0_0__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XAndExpression__Group_1_0_0__1_in_rule__XAndExpression__Group_1_0_0__051331);
rule__XAndExpression__Group_1_0_0__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XAndExpression__Group_1_0_0__0"
// $ANTLR start "rule__XAndExpression__Group_1_0_0__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25334:1: rule__XAndExpression__Group_1_0_0__0__Impl : ( () ) ;
public final void rule__XAndExpression__Group_1_0_0__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25338:1: ( ( () ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25339:1: ( () )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25339:1: ( () )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25340:1: ()
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXAndExpressionAccess().getXBinaryOperationLeftOperandAction_1_0_0_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25341:1: ()
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25343:1:
{
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXAndExpressionAccess().getXBinaryOperationLeftOperandAction_1_0_0_0());
}
}
}
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XAndExpression__Group_1_0_0__0__Impl"
// $ANTLR start "rule__XAndExpression__Group_1_0_0__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25353:1: rule__XAndExpression__Group_1_0_0__1 : rule__XAndExpression__Group_1_0_0__1__Impl ;
public final void rule__XAndExpression__Group_1_0_0__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25357:1: ( rule__XAndExpression__Group_1_0_0__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25358:2: rule__XAndExpression__Group_1_0_0__1__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__XAndExpression__Group_1_0_0__1__Impl_in_rule__XAndExpression__Group_1_0_0__151389);
rule__XAndExpression__Group_1_0_0__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XAndExpression__Group_1_0_0__1"
// $ANTLR start "rule__XAndExpression__Group_1_0_0__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25364:1: rule__XAndExpression__Group_1_0_0__1__Impl : ( ( rule__XAndExpression__FeatureAssignment_1_0_0_1 ) ) ;
public final void rule__XAndExpression__Group_1_0_0__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25368:1: ( ( ( rule__XAndExpression__FeatureAssignment_1_0_0_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25369:1: ( ( rule__XAndExpression__FeatureAssignment_1_0_0_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25369:1: ( ( rule__XAndExpression__FeatureAssignment_1_0_0_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25370:1: ( rule__XAndExpression__FeatureAssignment_1_0_0_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXAndExpressionAccess().getFeatureAssignment_1_0_0_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25371:1: ( rule__XAndExpression__FeatureAssignment_1_0_0_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25371:2: rule__XAndExpression__FeatureAssignment_1_0_0_1
{
pushFollow(FollowSets002.FOLLOW_rule__XAndExpression__FeatureAssignment_1_0_0_1_in_rule__XAndExpression__Group_1_0_0__1__Impl51416);
rule__XAndExpression__FeatureAssignment_1_0_0_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXAndExpressionAccess().getFeatureAssignment_1_0_0_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XAndExpression__Group_1_0_0__1__Impl"
// $ANTLR start "rule__XEqualityExpression__Group__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25385:1: rule__XEqualityExpression__Group__0 : rule__XEqualityExpression__Group__0__Impl rule__XEqualityExpression__Group__1 ;
public final void rule__XEqualityExpression__Group__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25389:1: ( rule__XEqualityExpression__Group__0__Impl rule__XEqualityExpression__Group__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25390:2: rule__XEqualityExpression__Group__0__Impl rule__XEqualityExpression__Group__1
{
pushFollow(FollowSets002.FOLLOW_rule__XEqualityExpression__Group__0__Impl_in_rule__XEqualityExpression__Group__051450);
rule__XEqualityExpression__Group__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XEqualityExpression__Group__1_in_rule__XEqualityExpression__Group__051453);
rule__XEqualityExpression__Group__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XEqualityExpression__Group__0"
// $ANTLR start "rule__XEqualityExpression__Group__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25397:1: rule__XEqualityExpression__Group__0__Impl : ( ruleXRelationalExpression ) ;
public final void rule__XEqualityExpression__Group__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25401:1: ( ( ruleXRelationalExpression ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25402:1: ( ruleXRelationalExpression )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25402:1: ( ruleXRelationalExpression )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25403:1: ruleXRelationalExpression
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXEqualityExpressionAccess().getXRelationalExpressionParserRuleCall_0());
}
pushFollow(FollowSets002.FOLLOW_ruleXRelationalExpression_in_rule__XEqualityExpression__Group__0__Impl51480);
ruleXRelationalExpression();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXEqualityExpressionAccess().getXRelationalExpressionParserRuleCall_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XEqualityExpression__Group__0__Impl"
// $ANTLR start "rule__XEqualityExpression__Group__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25414:1: rule__XEqualityExpression__Group__1 : rule__XEqualityExpression__Group__1__Impl ;
public final void rule__XEqualityExpression__Group__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25418:1: ( rule__XEqualityExpression__Group__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25419:2: rule__XEqualityExpression__Group__1__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__XEqualityExpression__Group__1__Impl_in_rule__XEqualityExpression__Group__151509);
rule__XEqualityExpression__Group__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XEqualityExpression__Group__1"
// $ANTLR start "rule__XEqualityExpression__Group__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25425:1: rule__XEqualityExpression__Group__1__Impl : ( ( rule__XEqualityExpression__Group_1__0 )* ) ;
public final void rule__XEqualityExpression__Group__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25429:1: ( ( ( rule__XEqualityExpression__Group_1__0 )* ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25430:1: ( ( rule__XEqualityExpression__Group_1__0 )* )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25430:1: ( ( rule__XEqualityExpression__Group_1__0 )* )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25431:1: ( rule__XEqualityExpression__Group_1__0 )*
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXEqualityExpressionAccess().getGroup_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25432:1: ( rule__XEqualityExpression__Group_1__0 )*
loop200:
do {
int alt200=2;
switch ( input.LA(1) ) {
case 21:
{
int LA200_2 = input.LA(2);
if ( (synpred286_InternalMango()) ) {
alt200=1;
}
}
break;
case 22:
{
int LA200_3 = input.LA(2);
if ( (synpred286_InternalMango()) ) {
alt200=1;
}
}
break;
case 23:
{
int LA200_4 = input.LA(2);
if ( (synpred286_InternalMango()) ) {
alt200=1;
}
}
break;
case 24:
{
int LA200_5 = input.LA(2);
if ( (synpred286_InternalMango()) ) {
alt200=1;
}
}
break;
}
switch (alt200) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25432:2: rule__XEqualityExpression__Group_1__0
{
pushFollow(FollowSets002.FOLLOW_rule__XEqualityExpression__Group_1__0_in_rule__XEqualityExpression__Group__1__Impl51536);
rule__XEqualityExpression__Group_1__0();
state._fsp--;
if (state.failed) return ;
}
break;
default :
break loop200;
}
} while (true);
if ( state.backtracking==0 ) {
after(grammarAccess.getXEqualityExpressionAccess().getGroup_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XEqualityExpression__Group__1__Impl"
// $ANTLR start "rule__XEqualityExpression__Group_1__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25446:1: rule__XEqualityExpression__Group_1__0 : rule__XEqualityExpression__Group_1__0__Impl rule__XEqualityExpression__Group_1__1 ;
public final void rule__XEqualityExpression__Group_1__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25450:1: ( rule__XEqualityExpression__Group_1__0__Impl rule__XEqualityExpression__Group_1__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25451:2: rule__XEqualityExpression__Group_1__0__Impl rule__XEqualityExpression__Group_1__1
{
pushFollow(FollowSets002.FOLLOW_rule__XEqualityExpression__Group_1__0__Impl_in_rule__XEqualityExpression__Group_1__051571);
rule__XEqualityExpression__Group_1__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XEqualityExpression__Group_1__1_in_rule__XEqualityExpression__Group_1__051574);
rule__XEqualityExpression__Group_1__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XEqualityExpression__Group_1__0"
// $ANTLR start "rule__XEqualityExpression__Group_1__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25458:1: rule__XEqualityExpression__Group_1__0__Impl : ( ( rule__XEqualityExpression__Group_1_0__0 ) ) ;
public final void rule__XEqualityExpression__Group_1__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25462:1: ( ( ( rule__XEqualityExpression__Group_1_0__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25463:1: ( ( rule__XEqualityExpression__Group_1_0__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25463:1: ( ( rule__XEqualityExpression__Group_1_0__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25464:1: ( rule__XEqualityExpression__Group_1_0__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXEqualityExpressionAccess().getGroup_1_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25465:1: ( rule__XEqualityExpression__Group_1_0__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25465:2: rule__XEqualityExpression__Group_1_0__0
{
pushFollow(FollowSets002.FOLLOW_rule__XEqualityExpression__Group_1_0__0_in_rule__XEqualityExpression__Group_1__0__Impl51601);
rule__XEqualityExpression__Group_1_0__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXEqualityExpressionAccess().getGroup_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XEqualityExpression__Group_1__0__Impl"
// $ANTLR start "rule__XEqualityExpression__Group_1__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25475:1: rule__XEqualityExpression__Group_1__1 : rule__XEqualityExpression__Group_1__1__Impl ;
public final void rule__XEqualityExpression__Group_1__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25479:1: ( rule__XEqualityExpression__Group_1__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25480:2: rule__XEqualityExpression__Group_1__1__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__XEqualityExpression__Group_1__1__Impl_in_rule__XEqualityExpression__Group_1__151631);
rule__XEqualityExpression__Group_1__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XEqualityExpression__Group_1__1"
// $ANTLR start "rule__XEqualityExpression__Group_1__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25486:1: rule__XEqualityExpression__Group_1__1__Impl : ( ( rule__XEqualityExpression__RightOperandAssignment_1_1 ) ) ;
public final void rule__XEqualityExpression__Group_1__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25490:1: ( ( ( rule__XEqualityExpression__RightOperandAssignment_1_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25491:1: ( ( rule__XEqualityExpression__RightOperandAssignment_1_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25491:1: ( ( rule__XEqualityExpression__RightOperandAssignment_1_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25492:1: ( rule__XEqualityExpression__RightOperandAssignment_1_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXEqualityExpressionAccess().getRightOperandAssignment_1_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25493:1: ( rule__XEqualityExpression__RightOperandAssignment_1_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25493:2: rule__XEqualityExpression__RightOperandAssignment_1_1
{
pushFollow(FollowSets002.FOLLOW_rule__XEqualityExpression__RightOperandAssignment_1_1_in_rule__XEqualityExpression__Group_1__1__Impl51658);
rule__XEqualityExpression__RightOperandAssignment_1_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXEqualityExpressionAccess().getRightOperandAssignment_1_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XEqualityExpression__Group_1__1__Impl"
// $ANTLR start "rule__XEqualityExpression__Group_1_0__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25507:1: rule__XEqualityExpression__Group_1_0__0 : rule__XEqualityExpression__Group_1_0__0__Impl ;
public final void rule__XEqualityExpression__Group_1_0__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25511:1: ( rule__XEqualityExpression__Group_1_0__0__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25512:2: rule__XEqualityExpression__Group_1_0__0__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__XEqualityExpression__Group_1_0__0__Impl_in_rule__XEqualityExpression__Group_1_0__051692);
rule__XEqualityExpression__Group_1_0__0__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XEqualityExpression__Group_1_0__0"
// $ANTLR start "rule__XEqualityExpression__Group_1_0__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25518:1: rule__XEqualityExpression__Group_1_0__0__Impl : ( ( rule__XEqualityExpression__Group_1_0_0__0 ) ) ;
public final void rule__XEqualityExpression__Group_1_0__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25522:1: ( ( ( rule__XEqualityExpression__Group_1_0_0__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25523:1: ( ( rule__XEqualityExpression__Group_1_0_0__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25523:1: ( ( rule__XEqualityExpression__Group_1_0_0__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25524:1: ( rule__XEqualityExpression__Group_1_0_0__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXEqualityExpressionAccess().getGroup_1_0_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25525:1: ( rule__XEqualityExpression__Group_1_0_0__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25525:2: rule__XEqualityExpression__Group_1_0_0__0
{
pushFollow(FollowSets002.FOLLOW_rule__XEqualityExpression__Group_1_0_0__0_in_rule__XEqualityExpression__Group_1_0__0__Impl51719);
rule__XEqualityExpression__Group_1_0_0__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXEqualityExpressionAccess().getGroup_1_0_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XEqualityExpression__Group_1_0__0__Impl"
// $ANTLR start "rule__XEqualityExpression__Group_1_0_0__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25537:1: rule__XEqualityExpression__Group_1_0_0__0 : rule__XEqualityExpression__Group_1_0_0__0__Impl rule__XEqualityExpression__Group_1_0_0__1 ;
public final void rule__XEqualityExpression__Group_1_0_0__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25541:1: ( rule__XEqualityExpression__Group_1_0_0__0__Impl rule__XEqualityExpression__Group_1_0_0__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25542:2: rule__XEqualityExpression__Group_1_0_0__0__Impl rule__XEqualityExpression__Group_1_0_0__1
{
pushFollow(FollowSets002.FOLLOW_rule__XEqualityExpression__Group_1_0_0__0__Impl_in_rule__XEqualityExpression__Group_1_0_0__051751);
rule__XEqualityExpression__Group_1_0_0__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XEqualityExpression__Group_1_0_0__1_in_rule__XEqualityExpression__Group_1_0_0__051754);
rule__XEqualityExpression__Group_1_0_0__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XEqualityExpression__Group_1_0_0__0"
// $ANTLR start "rule__XEqualityExpression__Group_1_0_0__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25549:1: rule__XEqualityExpression__Group_1_0_0__0__Impl : ( () ) ;
public final void rule__XEqualityExpression__Group_1_0_0__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25553:1: ( ( () ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25554:1: ( () )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25554:1: ( () )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25555:1: ()
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXEqualityExpressionAccess().getXBinaryOperationLeftOperandAction_1_0_0_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25556:1: ()
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25558:1:
{
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXEqualityExpressionAccess().getXBinaryOperationLeftOperandAction_1_0_0_0());
}
}
}
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XEqualityExpression__Group_1_0_0__0__Impl"
// $ANTLR start "rule__XEqualityExpression__Group_1_0_0__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25568:1: rule__XEqualityExpression__Group_1_0_0__1 : rule__XEqualityExpression__Group_1_0_0__1__Impl ;
public final void rule__XEqualityExpression__Group_1_0_0__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25572:1: ( rule__XEqualityExpression__Group_1_0_0__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25573:2: rule__XEqualityExpression__Group_1_0_0__1__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__XEqualityExpression__Group_1_0_0__1__Impl_in_rule__XEqualityExpression__Group_1_0_0__151812);
rule__XEqualityExpression__Group_1_0_0__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XEqualityExpression__Group_1_0_0__1"
// $ANTLR start "rule__XEqualityExpression__Group_1_0_0__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25579:1: rule__XEqualityExpression__Group_1_0_0__1__Impl : ( ( rule__XEqualityExpression__FeatureAssignment_1_0_0_1 ) ) ;
public final void rule__XEqualityExpression__Group_1_0_0__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25583:1: ( ( ( rule__XEqualityExpression__FeatureAssignment_1_0_0_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25584:1: ( ( rule__XEqualityExpression__FeatureAssignment_1_0_0_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25584:1: ( ( rule__XEqualityExpression__FeatureAssignment_1_0_0_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25585:1: ( rule__XEqualityExpression__FeatureAssignment_1_0_0_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXEqualityExpressionAccess().getFeatureAssignment_1_0_0_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25586:1: ( rule__XEqualityExpression__FeatureAssignment_1_0_0_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25586:2: rule__XEqualityExpression__FeatureAssignment_1_0_0_1
{
pushFollow(FollowSets002.FOLLOW_rule__XEqualityExpression__FeatureAssignment_1_0_0_1_in_rule__XEqualityExpression__Group_1_0_0__1__Impl51839);
rule__XEqualityExpression__FeatureAssignment_1_0_0_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXEqualityExpressionAccess().getFeatureAssignment_1_0_0_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XEqualityExpression__Group_1_0_0__1__Impl"
// $ANTLR start "rule__XRelationalExpression__Group__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25600:1: rule__XRelationalExpression__Group__0 : rule__XRelationalExpression__Group__0__Impl rule__XRelationalExpression__Group__1 ;
public final void rule__XRelationalExpression__Group__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25604:1: ( rule__XRelationalExpression__Group__0__Impl rule__XRelationalExpression__Group__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25605:2: rule__XRelationalExpression__Group__0__Impl rule__XRelationalExpression__Group__1
{
pushFollow(FollowSets002.FOLLOW_rule__XRelationalExpression__Group__0__Impl_in_rule__XRelationalExpression__Group__051873);
rule__XRelationalExpression__Group__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XRelationalExpression__Group__1_in_rule__XRelationalExpression__Group__051876);
rule__XRelationalExpression__Group__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XRelationalExpression__Group__0"
// $ANTLR start "rule__XRelationalExpression__Group__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25612:1: rule__XRelationalExpression__Group__0__Impl : ( ruleXOtherOperatorExpression ) ;
public final void rule__XRelationalExpression__Group__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25616:1: ( ( ruleXOtherOperatorExpression ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25617:1: ( ruleXOtherOperatorExpression )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25617:1: ( ruleXOtherOperatorExpression )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25618:1: ruleXOtherOperatorExpression
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXRelationalExpressionAccess().getXOtherOperatorExpressionParserRuleCall_0());
}
pushFollow(FollowSets002.FOLLOW_ruleXOtherOperatorExpression_in_rule__XRelationalExpression__Group__0__Impl51903);
ruleXOtherOperatorExpression();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXRelationalExpressionAccess().getXOtherOperatorExpressionParserRuleCall_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XRelationalExpression__Group__0__Impl"
// $ANTLR start "rule__XRelationalExpression__Group__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25629:1: rule__XRelationalExpression__Group__1 : rule__XRelationalExpression__Group__1__Impl ;
public final void rule__XRelationalExpression__Group__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25633:1: ( rule__XRelationalExpression__Group__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25634:2: rule__XRelationalExpression__Group__1__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__XRelationalExpression__Group__1__Impl_in_rule__XRelationalExpression__Group__151932);
rule__XRelationalExpression__Group__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XRelationalExpression__Group__1"
// $ANTLR start "rule__XRelationalExpression__Group__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25640:1: rule__XRelationalExpression__Group__1__Impl : ( ( rule__XRelationalExpression__Alternatives_1 )* ) ;
public final void rule__XRelationalExpression__Group__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25644:1: ( ( ( rule__XRelationalExpression__Alternatives_1 )* ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25645:1: ( ( rule__XRelationalExpression__Alternatives_1 )* )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25645:1: ( ( rule__XRelationalExpression__Alternatives_1 )* )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25646:1: ( rule__XRelationalExpression__Alternatives_1 )*
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXRelationalExpressionAccess().getAlternatives_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25647:1: ( rule__XRelationalExpression__Alternatives_1 )*
loop201:
do {
int alt201=2;
switch ( input.LA(1) ) {
case 27:
{
int LA201_2 = input.LA(2);
if ( (synpred287_InternalMango()) ) {
alt201=1;
}
}
break;
case 26:
{
int LA201_3 = input.LA(2);
if ( (synpred287_InternalMango()) ) {
alt201=1;
}
}
break;
case 152:
{
int LA201_4 = input.LA(2);
if ( (synpred287_InternalMango()) ) {
alt201=1;
}
}
break;
case 25:
{
int LA201_5 = input.LA(2);
if ( (synpred287_InternalMango()) ) {
alt201=1;
}
}
break;
}
switch (alt201) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25647:2: rule__XRelationalExpression__Alternatives_1
{
pushFollow(FollowSets002.FOLLOW_rule__XRelationalExpression__Alternatives_1_in_rule__XRelationalExpression__Group__1__Impl51959);
rule__XRelationalExpression__Alternatives_1();
state._fsp--;
if (state.failed) return ;
}
break;
default :
break loop201;
}
} while (true);
if ( state.backtracking==0 ) {
after(grammarAccess.getXRelationalExpressionAccess().getAlternatives_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XRelationalExpression__Group__1__Impl"
// $ANTLR start "rule__XRelationalExpression__Group_1_0__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25661:1: rule__XRelationalExpression__Group_1_0__0 : rule__XRelationalExpression__Group_1_0__0__Impl rule__XRelationalExpression__Group_1_0__1 ;
public final void rule__XRelationalExpression__Group_1_0__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25665:1: ( rule__XRelationalExpression__Group_1_0__0__Impl rule__XRelationalExpression__Group_1_0__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25666:2: rule__XRelationalExpression__Group_1_0__0__Impl rule__XRelationalExpression__Group_1_0__1
{
pushFollow(FollowSets002.FOLLOW_rule__XRelationalExpression__Group_1_0__0__Impl_in_rule__XRelationalExpression__Group_1_0__051994);
rule__XRelationalExpression__Group_1_0__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XRelationalExpression__Group_1_0__1_in_rule__XRelationalExpression__Group_1_0__051997);
rule__XRelationalExpression__Group_1_0__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XRelationalExpression__Group_1_0__0"
// $ANTLR start "rule__XRelationalExpression__Group_1_0__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25673:1: rule__XRelationalExpression__Group_1_0__0__Impl : ( ( rule__XRelationalExpression__Group_1_0_0__0 ) ) ;
public final void rule__XRelationalExpression__Group_1_0__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25677:1: ( ( ( rule__XRelationalExpression__Group_1_0_0__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25678:1: ( ( rule__XRelationalExpression__Group_1_0_0__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25678:1: ( ( rule__XRelationalExpression__Group_1_0_0__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25679:1: ( rule__XRelationalExpression__Group_1_0_0__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXRelationalExpressionAccess().getGroup_1_0_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25680:1: ( rule__XRelationalExpression__Group_1_0_0__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25680:2: rule__XRelationalExpression__Group_1_0_0__0
{
pushFollow(FollowSets002.FOLLOW_rule__XRelationalExpression__Group_1_0_0__0_in_rule__XRelationalExpression__Group_1_0__0__Impl52024);
rule__XRelationalExpression__Group_1_0_0__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXRelationalExpressionAccess().getGroup_1_0_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XRelationalExpression__Group_1_0__0__Impl"
// $ANTLR start "rule__XRelationalExpression__Group_1_0__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25690:1: rule__XRelationalExpression__Group_1_0__1 : rule__XRelationalExpression__Group_1_0__1__Impl ;
public final void rule__XRelationalExpression__Group_1_0__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25694:1: ( rule__XRelationalExpression__Group_1_0__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25695:2: rule__XRelationalExpression__Group_1_0__1__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__XRelationalExpression__Group_1_0__1__Impl_in_rule__XRelationalExpression__Group_1_0__152054);
rule__XRelationalExpression__Group_1_0__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XRelationalExpression__Group_1_0__1"
// $ANTLR start "rule__XRelationalExpression__Group_1_0__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25701:1: rule__XRelationalExpression__Group_1_0__1__Impl : ( ( rule__XRelationalExpression__TypeAssignment_1_0_1 ) ) ;
public final void rule__XRelationalExpression__Group_1_0__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25705:1: ( ( ( rule__XRelationalExpression__TypeAssignment_1_0_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25706:1: ( ( rule__XRelationalExpression__TypeAssignment_1_0_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25706:1: ( ( rule__XRelationalExpression__TypeAssignment_1_0_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25707:1: ( rule__XRelationalExpression__TypeAssignment_1_0_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXRelationalExpressionAccess().getTypeAssignment_1_0_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25708:1: ( rule__XRelationalExpression__TypeAssignment_1_0_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25708:2: rule__XRelationalExpression__TypeAssignment_1_0_1
{
pushFollow(FollowSets002.FOLLOW_rule__XRelationalExpression__TypeAssignment_1_0_1_in_rule__XRelationalExpression__Group_1_0__1__Impl52081);
rule__XRelationalExpression__TypeAssignment_1_0_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXRelationalExpressionAccess().getTypeAssignment_1_0_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XRelationalExpression__Group_1_0__1__Impl"
// $ANTLR start "rule__XRelationalExpression__Group_1_0_0__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25722:1: rule__XRelationalExpression__Group_1_0_0__0 : rule__XRelationalExpression__Group_1_0_0__0__Impl ;
public final void rule__XRelationalExpression__Group_1_0_0__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25726:1: ( rule__XRelationalExpression__Group_1_0_0__0__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25727:2: rule__XRelationalExpression__Group_1_0_0__0__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__XRelationalExpression__Group_1_0_0__0__Impl_in_rule__XRelationalExpression__Group_1_0_0__052115);
rule__XRelationalExpression__Group_1_0_0__0__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XRelationalExpression__Group_1_0_0__0"
// $ANTLR start "rule__XRelationalExpression__Group_1_0_0__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25733:1: rule__XRelationalExpression__Group_1_0_0__0__Impl : ( ( rule__XRelationalExpression__Group_1_0_0_0__0 ) ) ;
public final void rule__XRelationalExpression__Group_1_0_0__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25737:1: ( ( ( rule__XRelationalExpression__Group_1_0_0_0__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25738:1: ( ( rule__XRelationalExpression__Group_1_0_0_0__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25738:1: ( ( rule__XRelationalExpression__Group_1_0_0_0__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25739:1: ( rule__XRelationalExpression__Group_1_0_0_0__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXRelationalExpressionAccess().getGroup_1_0_0_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25740:1: ( rule__XRelationalExpression__Group_1_0_0_0__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25740:2: rule__XRelationalExpression__Group_1_0_0_0__0
{
pushFollow(FollowSets002.FOLLOW_rule__XRelationalExpression__Group_1_0_0_0__0_in_rule__XRelationalExpression__Group_1_0_0__0__Impl52142);
rule__XRelationalExpression__Group_1_0_0_0__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXRelationalExpressionAccess().getGroup_1_0_0_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XRelationalExpression__Group_1_0_0__0__Impl"
// $ANTLR start "rule__XRelationalExpression__Group_1_0_0_0__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25752:1: rule__XRelationalExpression__Group_1_0_0_0__0 : rule__XRelationalExpression__Group_1_0_0_0__0__Impl rule__XRelationalExpression__Group_1_0_0_0__1 ;
public final void rule__XRelationalExpression__Group_1_0_0_0__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25756:1: ( rule__XRelationalExpression__Group_1_0_0_0__0__Impl rule__XRelationalExpression__Group_1_0_0_0__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25757:2: rule__XRelationalExpression__Group_1_0_0_0__0__Impl rule__XRelationalExpression__Group_1_0_0_0__1
{
pushFollow(FollowSets002.FOLLOW_rule__XRelationalExpression__Group_1_0_0_0__0__Impl_in_rule__XRelationalExpression__Group_1_0_0_0__052174);
rule__XRelationalExpression__Group_1_0_0_0__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XRelationalExpression__Group_1_0_0_0__1_in_rule__XRelationalExpression__Group_1_0_0_0__052177);
rule__XRelationalExpression__Group_1_0_0_0__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XRelationalExpression__Group_1_0_0_0__0"
// $ANTLR start "rule__XRelationalExpression__Group_1_0_0_0__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25764:1: rule__XRelationalExpression__Group_1_0_0_0__0__Impl : ( () ) ;
public final void rule__XRelationalExpression__Group_1_0_0_0__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25768:1: ( ( () ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25769:1: ( () )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25769:1: ( () )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25770:1: ()
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXRelationalExpressionAccess().getXInstanceOfExpressionExpressionAction_1_0_0_0_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25771:1: ()
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25773:1:
{
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXRelationalExpressionAccess().getXInstanceOfExpressionExpressionAction_1_0_0_0_0());
}
}
}
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XRelationalExpression__Group_1_0_0_0__0__Impl"
// $ANTLR start "rule__XRelationalExpression__Group_1_0_0_0__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25783:1: rule__XRelationalExpression__Group_1_0_0_0__1 : rule__XRelationalExpression__Group_1_0_0_0__1__Impl ;
public final void rule__XRelationalExpression__Group_1_0_0_0__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25787:1: ( rule__XRelationalExpression__Group_1_0_0_0__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25788:2: rule__XRelationalExpression__Group_1_0_0_0__1__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__XRelationalExpression__Group_1_0_0_0__1__Impl_in_rule__XRelationalExpression__Group_1_0_0_0__152235);
rule__XRelationalExpression__Group_1_0_0_0__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XRelationalExpression__Group_1_0_0_0__1"
// $ANTLR start "rule__XRelationalExpression__Group_1_0_0_0__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25794:1: rule__XRelationalExpression__Group_1_0_0_0__1__Impl : ( 'instanceof' ) ;
public final void rule__XRelationalExpression__Group_1_0_0_0__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25798:1: ( ( 'instanceof' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25799:1: ( 'instanceof' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25799:1: ( 'instanceof' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25800:1: 'instanceof'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXRelationalExpressionAccess().getInstanceofKeyword_1_0_0_0_1());
}
match(input,152,FollowSets002.FOLLOW_152_in_rule__XRelationalExpression__Group_1_0_0_0__1__Impl52263); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXRelationalExpressionAccess().getInstanceofKeyword_1_0_0_0_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XRelationalExpression__Group_1_0_0_0__1__Impl"
// $ANTLR start "rule__XRelationalExpression__Group_1_1__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25817:1: rule__XRelationalExpression__Group_1_1__0 : rule__XRelationalExpression__Group_1_1__0__Impl rule__XRelationalExpression__Group_1_1__1 ;
public final void rule__XRelationalExpression__Group_1_1__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25821:1: ( rule__XRelationalExpression__Group_1_1__0__Impl rule__XRelationalExpression__Group_1_1__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25822:2: rule__XRelationalExpression__Group_1_1__0__Impl rule__XRelationalExpression__Group_1_1__1
{
pushFollow(FollowSets002.FOLLOW_rule__XRelationalExpression__Group_1_1__0__Impl_in_rule__XRelationalExpression__Group_1_1__052298);
rule__XRelationalExpression__Group_1_1__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XRelationalExpression__Group_1_1__1_in_rule__XRelationalExpression__Group_1_1__052301);
rule__XRelationalExpression__Group_1_1__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XRelationalExpression__Group_1_1__0"
// $ANTLR start "rule__XRelationalExpression__Group_1_1__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25829:1: rule__XRelationalExpression__Group_1_1__0__Impl : ( ( rule__XRelationalExpression__Group_1_1_0__0 ) ) ;
public final void rule__XRelationalExpression__Group_1_1__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25833:1: ( ( ( rule__XRelationalExpression__Group_1_1_0__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25834:1: ( ( rule__XRelationalExpression__Group_1_1_0__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25834:1: ( ( rule__XRelationalExpression__Group_1_1_0__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25835:1: ( rule__XRelationalExpression__Group_1_1_0__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXRelationalExpressionAccess().getGroup_1_1_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25836:1: ( rule__XRelationalExpression__Group_1_1_0__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25836:2: rule__XRelationalExpression__Group_1_1_0__0
{
pushFollow(FollowSets002.FOLLOW_rule__XRelationalExpression__Group_1_1_0__0_in_rule__XRelationalExpression__Group_1_1__0__Impl52328);
rule__XRelationalExpression__Group_1_1_0__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXRelationalExpressionAccess().getGroup_1_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XRelationalExpression__Group_1_1__0__Impl"
// $ANTLR start "rule__XRelationalExpression__Group_1_1__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25846:1: rule__XRelationalExpression__Group_1_1__1 : rule__XRelationalExpression__Group_1_1__1__Impl ;
public final void rule__XRelationalExpression__Group_1_1__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25850:1: ( rule__XRelationalExpression__Group_1_1__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25851:2: rule__XRelationalExpression__Group_1_1__1__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__XRelationalExpression__Group_1_1__1__Impl_in_rule__XRelationalExpression__Group_1_1__152358);
rule__XRelationalExpression__Group_1_1__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XRelationalExpression__Group_1_1__1"
// $ANTLR start "rule__XRelationalExpression__Group_1_1__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25857:1: rule__XRelationalExpression__Group_1_1__1__Impl : ( ( rule__XRelationalExpression__RightOperandAssignment_1_1_1 ) ) ;
public final void rule__XRelationalExpression__Group_1_1__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25861:1: ( ( ( rule__XRelationalExpression__RightOperandAssignment_1_1_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25862:1: ( ( rule__XRelationalExpression__RightOperandAssignment_1_1_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25862:1: ( ( rule__XRelationalExpression__RightOperandAssignment_1_1_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25863:1: ( rule__XRelationalExpression__RightOperandAssignment_1_1_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXRelationalExpressionAccess().getRightOperandAssignment_1_1_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25864:1: ( rule__XRelationalExpression__RightOperandAssignment_1_1_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25864:2: rule__XRelationalExpression__RightOperandAssignment_1_1_1
{
pushFollow(FollowSets002.FOLLOW_rule__XRelationalExpression__RightOperandAssignment_1_1_1_in_rule__XRelationalExpression__Group_1_1__1__Impl52385);
rule__XRelationalExpression__RightOperandAssignment_1_1_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXRelationalExpressionAccess().getRightOperandAssignment_1_1_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XRelationalExpression__Group_1_1__1__Impl"
// $ANTLR start "rule__XRelationalExpression__Group_1_1_0__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25878:1: rule__XRelationalExpression__Group_1_1_0__0 : rule__XRelationalExpression__Group_1_1_0__0__Impl ;
public final void rule__XRelationalExpression__Group_1_1_0__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25882:1: ( rule__XRelationalExpression__Group_1_1_0__0__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25883:2: rule__XRelationalExpression__Group_1_1_0__0__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__XRelationalExpression__Group_1_1_0__0__Impl_in_rule__XRelationalExpression__Group_1_1_0__052419);
rule__XRelationalExpression__Group_1_1_0__0__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XRelationalExpression__Group_1_1_0__0"
// $ANTLR start "rule__XRelationalExpression__Group_1_1_0__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25889:1: rule__XRelationalExpression__Group_1_1_0__0__Impl : ( ( rule__XRelationalExpression__Group_1_1_0_0__0 ) ) ;
public final void rule__XRelationalExpression__Group_1_1_0__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25893:1: ( ( ( rule__XRelationalExpression__Group_1_1_0_0__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25894:1: ( ( rule__XRelationalExpression__Group_1_1_0_0__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25894:1: ( ( rule__XRelationalExpression__Group_1_1_0_0__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25895:1: ( rule__XRelationalExpression__Group_1_1_0_0__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXRelationalExpressionAccess().getGroup_1_1_0_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25896:1: ( rule__XRelationalExpression__Group_1_1_0_0__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25896:2: rule__XRelationalExpression__Group_1_1_0_0__0
{
pushFollow(FollowSets002.FOLLOW_rule__XRelationalExpression__Group_1_1_0_0__0_in_rule__XRelationalExpression__Group_1_1_0__0__Impl52446);
rule__XRelationalExpression__Group_1_1_0_0__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXRelationalExpressionAccess().getGroup_1_1_0_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XRelationalExpression__Group_1_1_0__0__Impl"
// $ANTLR start "rule__XRelationalExpression__Group_1_1_0_0__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25908:1: rule__XRelationalExpression__Group_1_1_0_0__0 : rule__XRelationalExpression__Group_1_1_0_0__0__Impl rule__XRelationalExpression__Group_1_1_0_0__1 ;
public final void rule__XRelationalExpression__Group_1_1_0_0__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25912:1: ( rule__XRelationalExpression__Group_1_1_0_0__0__Impl rule__XRelationalExpression__Group_1_1_0_0__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25913:2: rule__XRelationalExpression__Group_1_1_0_0__0__Impl rule__XRelationalExpression__Group_1_1_0_0__1
{
pushFollow(FollowSets002.FOLLOW_rule__XRelationalExpression__Group_1_1_0_0__0__Impl_in_rule__XRelationalExpression__Group_1_1_0_0__052478);
rule__XRelationalExpression__Group_1_1_0_0__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XRelationalExpression__Group_1_1_0_0__1_in_rule__XRelationalExpression__Group_1_1_0_0__052481);
rule__XRelationalExpression__Group_1_1_0_0__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XRelationalExpression__Group_1_1_0_0__0"
// $ANTLR start "rule__XRelationalExpression__Group_1_1_0_0__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25920:1: rule__XRelationalExpression__Group_1_1_0_0__0__Impl : ( () ) ;
public final void rule__XRelationalExpression__Group_1_1_0_0__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25924:1: ( ( () ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25925:1: ( () )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25925:1: ( () )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25926:1: ()
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXRelationalExpressionAccess().getXBinaryOperationLeftOperandAction_1_1_0_0_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25927:1: ()
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25929:1:
{
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXRelationalExpressionAccess().getXBinaryOperationLeftOperandAction_1_1_0_0_0());
}
}
}
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XRelationalExpression__Group_1_1_0_0__0__Impl"
// $ANTLR start "rule__XRelationalExpression__Group_1_1_0_0__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25939:1: rule__XRelationalExpression__Group_1_1_0_0__1 : rule__XRelationalExpression__Group_1_1_0_0__1__Impl ;
public final void rule__XRelationalExpression__Group_1_1_0_0__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25943:1: ( rule__XRelationalExpression__Group_1_1_0_0__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25944:2: rule__XRelationalExpression__Group_1_1_0_0__1__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__XRelationalExpression__Group_1_1_0_0__1__Impl_in_rule__XRelationalExpression__Group_1_1_0_0__152539);
rule__XRelationalExpression__Group_1_1_0_0__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XRelationalExpression__Group_1_1_0_0__1"
// $ANTLR start "rule__XRelationalExpression__Group_1_1_0_0__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25950:1: rule__XRelationalExpression__Group_1_1_0_0__1__Impl : ( ( rule__XRelationalExpression__FeatureAssignment_1_1_0_0_1 ) ) ;
public final void rule__XRelationalExpression__Group_1_1_0_0__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25954:1: ( ( ( rule__XRelationalExpression__FeatureAssignment_1_1_0_0_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25955:1: ( ( rule__XRelationalExpression__FeatureAssignment_1_1_0_0_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25955:1: ( ( rule__XRelationalExpression__FeatureAssignment_1_1_0_0_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25956:1: ( rule__XRelationalExpression__FeatureAssignment_1_1_0_0_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXRelationalExpressionAccess().getFeatureAssignment_1_1_0_0_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25957:1: ( rule__XRelationalExpression__FeatureAssignment_1_1_0_0_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25957:2: rule__XRelationalExpression__FeatureAssignment_1_1_0_0_1
{
pushFollow(FollowSets002.FOLLOW_rule__XRelationalExpression__FeatureAssignment_1_1_0_0_1_in_rule__XRelationalExpression__Group_1_1_0_0__1__Impl52566);
rule__XRelationalExpression__FeatureAssignment_1_1_0_0_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXRelationalExpressionAccess().getFeatureAssignment_1_1_0_0_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XRelationalExpression__Group_1_1_0_0__1__Impl"
// $ANTLR start "rule__OpCompare__Group_1__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25971:1: rule__OpCompare__Group_1__0 : rule__OpCompare__Group_1__0__Impl rule__OpCompare__Group_1__1 ;
public final void rule__OpCompare__Group_1__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25975:1: ( rule__OpCompare__Group_1__0__Impl rule__OpCompare__Group_1__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25976:2: rule__OpCompare__Group_1__0__Impl rule__OpCompare__Group_1__1
{
pushFollow(FollowSets002.FOLLOW_rule__OpCompare__Group_1__0__Impl_in_rule__OpCompare__Group_1__052600);
rule__OpCompare__Group_1__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__OpCompare__Group_1__1_in_rule__OpCompare__Group_1__052603);
rule__OpCompare__Group_1__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__OpCompare__Group_1__0"
// $ANTLR start "rule__OpCompare__Group_1__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25983:1: rule__OpCompare__Group_1__0__Impl : ( '<' ) ;
public final void rule__OpCompare__Group_1__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25987:1: ( ( '<' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25988:1: ( '<' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25988:1: ( '<' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25989:1: '<'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getOpCompareAccess().getLessThanSignKeyword_1_0());
}
match(input,27,FollowSets002.FOLLOW_27_in_rule__OpCompare__Group_1__0__Impl52631); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getOpCompareAccess().getLessThanSignKeyword_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__OpCompare__Group_1__0__Impl"
// $ANTLR start "rule__OpCompare__Group_1__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26002:1: rule__OpCompare__Group_1__1 : rule__OpCompare__Group_1__1__Impl ;
public final void rule__OpCompare__Group_1__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26006:1: ( rule__OpCompare__Group_1__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26007:2: rule__OpCompare__Group_1__1__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__OpCompare__Group_1__1__Impl_in_rule__OpCompare__Group_1__152662);
rule__OpCompare__Group_1__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__OpCompare__Group_1__1"
// $ANTLR start "rule__OpCompare__Group_1__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26013:1: rule__OpCompare__Group_1__1__Impl : ( '=' ) ;
public final void rule__OpCompare__Group_1__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26017:1: ( ( '=' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26018:1: ( '=' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26018:1: ( '=' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26019:1: '='
{
if ( state.backtracking==0 ) {
before(grammarAccess.getOpCompareAccess().getEqualsSignKeyword_1_1());
}
match(input,13,FollowSets002.FOLLOW_13_in_rule__OpCompare__Group_1__1__Impl52690); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getOpCompareAccess().getEqualsSignKeyword_1_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__OpCompare__Group_1__1__Impl"
// $ANTLR start "rule__XOtherOperatorExpression__Group__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26036:1: rule__XOtherOperatorExpression__Group__0 : rule__XOtherOperatorExpression__Group__0__Impl rule__XOtherOperatorExpression__Group__1 ;
public final void rule__XOtherOperatorExpression__Group__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26040:1: ( rule__XOtherOperatorExpression__Group__0__Impl rule__XOtherOperatorExpression__Group__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26041:2: rule__XOtherOperatorExpression__Group__0__Impl rule__XOtherOperatorExpression__Group__1
{
pushFollow(FollowSets002.FOLLOW_rule__XOtherOperatorExpression__Group__0__Impl_in_rule__XOtherOperatorExpression__Group__052725);
rule__XOtherOperatorExpression__Group__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XOtherOperatorExpression__Group__1_in_rule__XOtherOperatorExpression__Group__052728);
rule__XOtherOperatorExpression__Group__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XOtherOperatorExpression__Group__0"
// $ANTLR start "rule__XOtherOperatorExpression__Group__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26048:1: rule__XOtherOperatorExpression__Group__0__Impl : ( ruleXAdditiveExpression ) ;
public final void rule__XOtherOperatorExpression__Group__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26052:1: ( ( ruleXAdditiveExpression ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26053:1: ( ruleXAdditiveExpression )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26053:1: ( ruleXAdditiveExpression )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26054:1: ruleXAdditiveExpression
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXOtherOperatorExpressionAccess().getXAdditiveExpressionParserRuleCall_0());
}
pushFollow(FollowSets002.FOLLOW_ruleXAdditiveExpression_in_rule__XOtherOperatorExpression__Group__0__Impl52755);
ruleXAdditiveExpression();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXOtherOperatorExpressionAccess().getXAdditiveExpressionParserRuleCall_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XOtherOperatorExpression__Group__0__Impl"
// $ANTLR start "rule__XOtherOperatorExpression__Group__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26065:1: rule__XOtherOperatorExpression__Group__1 : rule__XOtherOperatorExpression__Group__1__Impl ;
public final void rule__XOtherOperatorExpression__Group__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26069:1: ( rule__XOtherOperatorExpression__Group__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26070:2: rule__XOtherOperatorExpression__Group__1__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__XOtherOperatorExpression__Group__1__Impl_in_rule__XOtherOperatorExpression__Group__152784);
rule__XOtherOperatorExpression__Group__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XOtherOperatorExpression__Group__1"
// $ANTLR start "rule__XOtherOperatorExpression__Group__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26076:1: rule__XOtherOperatorExpression__Group__1__Impl : ( ( rule__XOtherOperatorExpression__Group_1__0 )* ) ;
public final void rule__XOtherOperatorExpression__Group__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26080:1: ( ( ( rule__XOtherOperatorExpression__Group_1__0 )* ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26081:1: ( ( rule__XOtherOperatorExpression__Group_1__0 )* )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26081:1: ( ( rule__XOtherOperatorExpression__Group_1__0 )* )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26082:1: ( rule__XOtherOperatorExpression__Group_1__0 )*
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXOtherOperatorExpressionAccess().getGroup_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26083:1: ( rule__XOtherOperatorExpression__Group_1__0 )*
loop202:
do {
int alt202=2;
alt202 = dfa202.predict(input);
switch (alt202) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26083:2: rule__XOtherOperatorExpression__Group_1__0
{
pushFollow(FollowSets002.FOLLOW_rule__XOtherOperatorExpression__Group_1__0_in_rule__XOtherOperatorExpression__Group__1__Impl52811);
rule__XOtherOperatorExpression__Group_1__0();
state._fsp--;
if (state.failed) return ;
}
break;
default :
break loop202;
}
} while (true);
if ( state.backtracking==0 ) {
after(grammarAccess.getXOtherOperatorExpressionAccess().getGroup_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XOtherOperatorExpression__Group__1__Impl"
// $ANTLR start "rule__XOtherOperatorExpression__Group_1__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26097:1: rule__XOtherOperatorExpression__Group_1__0 : rule__XOtherOperatorExpression__Group_1__0__Impl rule__XOtherOperatorExpression__Group_1__1 ;
public final void rule__XOtherOperatorExpression__Group_1__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26101:1: ( rule__XOtherOperatorExpression__Group_1__0__Impl rule__XOtherOperatorExpression__Group_1__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26102:2: rule__XOtherOperatorExpression__Group_1__0__Impl rule__XOtherOperatorExpression__Group_1__1
{
pushFollow(FollowSets002.FOLLOW_rule__XOtherOperatorExpression__Group_1__0__Impl_in_rule__XOtherOperatorExpression__Group_1__052846);
rule__XOtherOperatorExpression__Group_1__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XOtherOperatorExpression__Group_1__1_in_rule__XOtherOperatorExpression__Group_1__052849);
rule__XOtherOperatorExpression__Group_1__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XOtherOperatorExpression__Group_1__0"
// $ANTLR start "rule__XOtherOperatorExpression__Group_1__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26109:1: rule__XOtherOperatorExpression__Group_1__0__Impl : ( ( rule__XOtherOperatorExpression__Group_1_0__0 ) ) ;
public final void rule__XOtherOperatorExpression__Group_1__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26113:1: ( ( ( rule__XOtherOperatorExpression__Group_1_0__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26114:1: ( ( rule__XOtherOperatorExpression__Group_1_0__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26114:1: ( ( rule__XOtherOperatorExpression__Group_1_0__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26115:1: ( rule__XOtherOperatorExpression__Group_1_0__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXOtherOperatorExpressionAccess().getGroup_1_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26116:1: ( rule__XOtherOperatorExpression__Group_1_0__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26116:2: rule__XOtherOperatorExpression__Group_1_0__0
{
pushFollow(FollowSets002.FOLLOW_rule__XOtherOperatorExpression__Group_1_0__0_in_rule__XOtherOperatorExpression__Group_1__0__Impl52876);
rule__XOtherOperatorExpression__Group_1_0__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXOtherOperatorExpressionAccess().getGroup_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XOtherOperatorExpression__Group_1__0__Impl"
// $ANTLR start "rule__XOtherOperatorExpression__Group_1__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26126:1: rule__XOtherOperatorExpression__Group_1__1 : rule__XOtherOperatorExpression__Group_1__1__Impl ;
public final void rule__XOtherOperatorExpression__Group_1__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26130:1: ( rule__XOtherOperatorExpression__Group_1__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26131:2: rule__XOtherOperatorExpression__Group_1__1__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__XOtherOperatorExpression__Group_1__1__Impl_in_rule__XOtherOperatorExpression__Group_1__152906);
rule__XOtherOperatorExpression__Group_1__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XOtherOperatorExpression__Group_1__1"
// $ANTLR start "rule__XOtherOperatorExpression__Group_1__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26137:1: rule__XOtherOperatorExpression__Group_1__1__Impl : ( ( rule__XOtherOperatorExpression__RightOperandAssignment_1_1 ) ) ;
public final void rule__XOtherOperatorExpression__Group_1__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26141:1: ( ( ( rule__XOtherOperatorExpression__RightOperandAssignment_1_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26142:1: ( ( rule__XOtherOperatorExpression__RightOperandAssignment_1_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26142:1: ( ( rule__XOtherOperatorExpression__RightOperandAssignment_1_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26143:1: ( rule__XOtherOperatorExpression__RightOperandAssignment_1_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXOtherOperatorExpressionAccess().getRightOperandAssignment_1_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26144:1: ( rule__XOtherOperatorExpression__RightOperandAssignment_1_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26144:2: rule__XOtherOperatorExpression__RightOperandAssignment_1_1
{
pushFollow(FollowSets002.FOLLOW_rule__XOtherOperatorExpression__RightOperandAssignment_1_1_in_rule__XOtherOperatorExpression__Group_1__1__Impl52933);
rule__XOtherOperatorExpression__RightOperandAssignment_1_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXOtherOperatorExpressionAccess().getRightOperandAssignment_1_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XOtherOperatorExpression__Group_1__1__Impl"
// $ANTLR start "rule__XOtherOperatorExpression__Group_1_0__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26158:1: rule__XOtherOperatorExpression__Group_1_0__0 : rule__XOtherOperatorExpression__Group_1_0__0__Impl ;
public final void rule__XOtherOperatorExpression__Group_1_0__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26162:1: ( rule__XOtherOperatorExpression__Group_1_0__0__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26163:2: rule__XOtherOperatorExpression__Group_1_0__0__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__XOtherOperatorExpression__Group_1_0__0__Impl_in_rule__XOtherOperatorExpression__Group_1_0__052967);
rule__XOtherOperatorExpression__Group_1_0__0__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XOtherOperatorExpression__Group_1_0__0"
// $ANTLR start "rule__XOtherOperatorExpression__Group_1_0__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26169:1: rule__XOtherOperatorExpression__Group_1_0__0__Impl : ( ( rule__XOtherOperatorExpression__Group_1_0_0__0 ) ) ;
public final void rule__XOtherOperatorExpression__Group_1_0__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26173:1: ( ( ( rule__XOtherOperatorExpression__Group_1_0_0__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26174:1: ( ( rule__XOtherOperatorExpression__Group_1_0_0__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26174:1: ( ( rule__XOtherOperatorExpression__Group_1_0_0__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26175:1: ( rule__XOtherOperatorExpression__Group_1_0_0__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXOtherOperatorExpressionAccess().getGroup_1_0_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26176:1: ( rule__XOtherOperatorExpression__Group_1_0_0__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26176:2: rule__XOtherOperatorExpression__Group_1_0_0__0
{
pushFollow(FollowSets002.FOLLOW_rule__XOtherOperatorExpression__Group_1_0_0__0_in_rule__XOtherOperatorExpression__Group_1_0__0__Impl52994);
rule__XOtherOperatorExpression__Group_1_0_0__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXOtherOperatorExpressionAccess().getGroup_1_0_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XOtherOperatorExpression__Group_1_0__0__Impl"
// $ANTLR start "rule__XOtherOperatorExpression__Group_1_0_0__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26188:1: rule__XOtherOperatorExpression__Group_1_0_0__0 : rule__XOtherOperatorExpression__Group_1_0_0__0__Impl rule__XOtherOperatorExpression__Group_1_0_0__1 ;
public final void rule__XOtherOperatorExpression__Group_1_0_0__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26192:1: ( rule__XOtherOperatorExpression__Group_1_0_0__0__Impl rule__XOtherOperatorExpression__Group_1_0_0__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26193:2: rule__XOtherOperatorExpression__Group_1_0_0__0__Impl rule__XOtherOperatorExpression__Group_1_0_0__1
{
pushFollow(FollowSets002.FOLLOW_rule__XOtherOperatorExpression__Group_1_0_0__0__Impl_in_rule__XOtherOperatorExpression__Group_1_0_0__053026);
rule__XOtherOperatorExpression__Group_1_0_0__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XOtherOperatorExpression__Group_1_0_0__1_in_rule__XOtherOperatorExpression__Group_1_0_0__053029);
rule__XOtherOperatorExpression__Group_1_0_0__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XOtherOperatorExpression__Group_1_0_0__0"
// $ANTLR start "rule__XOtherOperatorExpression__Group_1_0_0__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26200:1: rule__XOtherOperatorExpression__Group_1_0_0__0__Impl : ( () ) ;
public final void rule__XOtherOperatorExpression__Group_1_0_0__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26204:1: ( ( () ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26205:1: ( () )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26205:1: ( () )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26206:1: ()
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXOtherOperatorExpressionAccess().getXBinaryOperationLeftOperandAction_1_0_0_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26207:1: ()
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26209:1:
{
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXOtherOperatorExpressionAccess().getXBinaryOperationLeftOperandAction_1_0_0_0());
}
}
}
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XOtherOperatorExpression__Group_1_0_0__0__Impl"
// $ANTLR start "rule__XOtherOperatorExpression__Group_1_0_0__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26219:1: rule__XOtherOperatorExpression__Group_1_0_0__1 : rule__XOtherOperatorExpression__Group_1_0_0__1__Impl ;
public final void rule__XOtherOperatorExpression__Group_1_0_0__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26223:1: ( rule__XOtherOperatorExpression__Group_1_0_0__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26224:2: rule__XOtherOperatorExpression__Group_1_0_0__1__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__XOtherOperatorExpression__Group_1_0_0__1__Impl_in_rule__XOtherOperatorExpression__Group_1_0_0__153087);
rule__XOtherOperatorExpression__Group_1_0_0__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XOtherOperatorExpression__Group_1_0_0__1"
// $ANTLR start "rule__XOtherOperatorExpression__Group_1_0_0__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26230:1: rule__XOtherOperatorExpression__Group_1_0_0__1__Impl : ( ( rule__XOtherOperatorExpression__FeatureAssignment_1_0_0_1 ) ) ;
public final void rule__XOtherOperatorExpression__Group_1_0_0__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26234:1: ( ( ( rule__XOtherOperatorExpression__FeatureAssignment_1_0_0_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26235:1: ( ( rule__XOtherOperatorExpression__FeatureAssignment_1_0_0_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26235:1: ( ( rule__XOtherOperatorExpression__FeatureAssignment_1_0_0_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26236:1: ( rule__XOtherOperatorExpression__FeatureAssignment_1_0_0_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXOtherOperatorExpressionAccess().getFeatureAssignment_1_0_0_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26237:1: ( rule__XOtherOperatorExpression__FeatureAssignment_1_0_0_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26237:2: rule__XOtherOperatorExpression__FeatureAssignment_1_0_0_1
{
pushFollow(FollowSets002.FOLLOW_rule__XOtherOperatorExpression__FeatureAssignment_1_0_0_1_in_rule__XOtherOperatorExpression__Group_1_0_0__1__Impl53114);
rule__XOtherOperatorExpression__FeatureAssignment_1_0_0_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXOtherOperatorExpressionAccess().getFeatureAssignment_1_0_0_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XOtherOperatorExpression__Group_1_0_0__1__Impl"
// $ANTLR start "rule__OpOther__Group_2__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26251:1: rule__OpOther__Group_2__0 : rule__OpOther__Group_2__0__Impl rule__OpOther__Group_2__1 ;
public final void rule__OpOther__Group_2__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26255:1: ( rule__OpOther__Group_2__0__Impl rule__OpOther__Group_2__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26256:2: rule__OpOther__Group_2__0__Impl rule__OpOther__Group_2__1
{
pushFollow(FollowSets002.FOLLOW_rule__OpOther__Group_2__0__Impl_in_rule__OpOther__Group_2__053148);
rule__OpOther__Group_2__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__OpOther__Group_2__1_in_rule__OpOther__Group_2__053151);
rule__OpOther__Group_2__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__OpOther__Group_2__0"
// $ANTLR start "rule__OpOther__Group_2__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26263:1: rule__OpOther__Group_2__0__Impl : ( '>' ) ;
public final void rule__OpOther__Group_2__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26267:1: ( ( '>' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26268:1: ( '>' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26268:1: ( '>' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26269:1: '>'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getOpOtherAccess().getGreaterThanSignKeyword_2_0());
}
match(input,26,FollowSets002.FOLLOW_26_in_rule__OpOther__Group_2__0__Impl53179); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getOpOtherAccess().getGreaterThanSignKeyword_2_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__OpOther__Group_2__0__Impl"
// $ANTLR start "rule__OpOther__Group_2__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26282:1: rule__OpOther__Group_2__1 : rule__OpOther__Group_2__1__Impl ;
public final void rule__OpOther__Group_2__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26286:1: ( rule__OpOther__Group_2__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26287:2: rule__OpOther__Group_2__1__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__OpOther__Group_2__1__Impl_in_rule__OpOther__Group_2__153210);
rule__OpOther__Group_2__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__OpOther__Group_2__1"
// $ANTLR start "rule__OpOther__Group_2__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26293:1: rule__OpOther__Group_2__1__Impl : ( '..' ) ;
public final void rule__OpOther__Group_2__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26297:1: ( ( '..' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26298:1: ( '..' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26298:1: ( '..' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26299:1: '..'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getOpOtherAccess().getFullStopFullStopKeyword_2_1());
}
match(input,30,FollowSets002.FOLLOW_30_in_rule__OpOther__Group_2__1__Impl53238); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getOpOtherAccess().getFullStopFullStopKeyword_2_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__OpOther__Group_2__1__Impl"
// $ANTLR start "rule__OpOther__Group_5__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26316:1: rule__OpOther__Group_5__0 : rule__OpOther__Group_5__0__Impl rule__OpOther__Group_5__1 ;
public final void rule__OpOther__Group_5__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26320:1: ( rule__OpOther__Group_5__0__Impl rule__OpOther__Group_5__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26321:2: rule__OpOther__Group_5__0__Impl rule__OpOther__Group_5__1
{
pushFollow(FollowSets002.FOLLOW_rule__OpOther__Group_5__0__Impl_in_rule__OpOther__Group_5__053273);
rule__OpOther__Group_5__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__OpOther__Group_5__1_in_rule__OpOther__Group_5__053276);
rule__OpOther__Group_5__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__OpOther__Group_5__0"
// $ANTLR start "rule__OpOther__Group_5__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26328:1: rule__OpOther__Group_5__0__Impl : ( '>' ) ;
public final void rule__OpOther__Group_5__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26332:1: ( ( '>' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26333:1: ( '>' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26333:1: ( '>' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26334:1: '>'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getOpOtherAccess().getGreaterThanSignKeyword_5_0());
}
match(input,26,FollowSets002.FOLLOW_26_in_rule__OpOther__Group_5__0__Impl53304); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getOpOtherAccess().getGreaterThanSignKeyword_5_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__OpOther__Group_5__0__Impl"
// $ANTLR start "rule__OpOther__Group_5__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26347:1: rule__OpOther__Group_5__1 : rule__OpOther__Group_5__1__Impl ;
public final void rule__OpOther__Group_5__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26351:1: ( rule__OpOther__Group_5__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26352:2: rule__OpOther__Group_5__1__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__OpOther__Group_5__1__Impl_in_rule__OpOther__Group_5__153335);
rule__OpOther__Group_5__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__OpOther__Group_5__1"
// $ANTLR start "rule__OpOther__Group_5__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26358:1: rule__OpOther__Group_5__1__Impl : ( ( rule__OpOther__Alternatives_5_1 ) ) ;
public final void rule__OpOther__Group_5__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26362:1: ( ( ( rule__OpOther__Alternatives_5_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26363:1: ( ( rule__OpOther__Alternatives_5_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26363:1: ( ( rule__OpOther__Alternatives_5_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26364:1: ( rule__OpOther__Alternatives_5_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getOpOtherAccess().getAlternatives_5_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26365:1: ( rule__OpOther__Alternatives_5_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26365:2: rule__OpOther__Alternatives_5_1
{
pushFollow(FollowSets002.FOLLOW_rule__OpOther__Alternatives_5_1_in_rule__OpOther__Group_5__1__Impl53362);
rule__OpOther__Alternatives_5_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getOpOtherAccess().getAlternatives_5_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__OpOther__Group_5__1__Impl"
// $ANTLR start "rule__OpOther__Group_5_1_0__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26379:1: rule__OpOther__Group_5_1_0__0 : rule__OpOther__Group_5_1_0__0__Impl ;
public final void rule__OpOther__Group_5_1_0__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26383:1: ( rule__OpOther__Group_5_1_0__0__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26384:2: rule__OpOther__Group_5_1_0__0__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__OpOther__Group_5_1_0__0__Impl_in_rule__OpOther__Group_5_1_0__053396);
rule__OpOther__Group_5_1_0__0__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__OpOther__Group_5_1_0__0"
// $ANTLR start "rule__OpOther__Group_5_1_0__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26390:1: rule__OpOther__Group_5_1_0__0__Impl : ( ( rule__OpOther__Group_5_1_0_0__0 ) ) ;
public final void rule__OpOther__Group_5_1_0__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26394:1: ( ( ( rule__OpOther__Group_5_1_0_0__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26395:1: ( ( rule__OpOther__Group_5_1_0_0__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26395:1: ( ( rule__OpOther__Group_5_1_0_0__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26396:1: ( rule__OpOther__Group_5_1_0_0__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getOpOtherAccess().getGroup_5_1_0_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26397:1: ( rule__OpOther__Group_5_1_0_0__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26397:2: rule__OpOther__Group_5_1_0_0__0
{
pushFollow(FollowSets002.FOLLOW_rule__OpOther__Group_5_1_0_0__0_in_rule__OpOther__Group_5_1_0__0__Impl53423);
rule__OpOther__Group_5_1_0_0__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getOpOtherAccess().getGroup_5_1_0_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__OpOther__Group_5_1_0__0__Impl"
// $ANTLR start "rule__OpOther__Group_5_1_0_0__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26409:1: rule__OpOther__Group_5_1_0_0__0 : rule__OpOther__Group_5_1_0_0__0__Impl rule__OpOther__Group_5_1_0_0__1 ;
public final void rule__OpOther__Group_5_1_0_0__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26413:1: ( rule__OpOther__Group_5_1_0_0__0__Impl rule__OpOther__Group_5_1_0_0__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26414:2: rule__OpOther__Group_5_1_0_0__0__Impl rule__OpOther__Group_5_1_0_0__1
{
pushFollow(FollowSets002.FOLLOW_rule__OpOther__Group_5_1_0_0__0__Impl_in_rule__OpOther__Group_5_1_0_0__053455);
rule__OpOther__Group_5_1_0_0__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__OpOther__Group_5_1_0_0__1_in_rule__OpOther__Group_5_1_0_0__053458);
rule__OpOther__Group_5_1_0_0__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__OpOther__Group_5_1_0_0__0"
// $ANTLR start "rule__OpOther__Group_5_1_0_0__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26421:1: rule__OpOther__Group_5_1_0_0__0__Impl : ( '>' ) ;
public final void rule__OpOther__Group_5_1_0_0__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26425:1: ( ( '>' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26426:1: ( '>' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26426:1: ( '>' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26427:1: '>'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getOpOtherAccess().getGreaterThanSignKeyword_5_1_0_0_0());
}
match(input,26,FollowSets002.FOLLOW_26_in_rule__OpOther__Group_5_1_0_0__0__Impl53486); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getOpOtherAccess().getGreaterThanSignKeyword_5_1_0_0_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__OpOther__Group_5_1_0_0__0__Impl"
// $ANTLR start "rule__OpOther__Group_5_1_0_0__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26440:1: rule__OpOther__Group_5_1_0_0__1 : rule__OpOther__Group_5_1_0_0__1__Impl ;
public final void rule__OpOther__Group_5_1_0_0__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26444:1: ( rule__OpOther__Group_5_1_0_0__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26445:2: rule__OpOther__Group_5_1_0_0__1__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__OpOther__Group_5_1_0_0__1__Impl_in_rule__OpOther__Group_5_1_0_0__153517);
rule__OpOther__Group_5_1_0_0__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__OpOther__Group_5_1_0_0__1"
// $ANTLR start "rule__OpOther__Group_5_1_0_0__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26451:1: rule__OpOther__Group_5_1_0_0__1__Impl : ( '>' ) ;
public final void rule__OpOther__Group_5_1_0_0__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26455:1: ( ( '>' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26456:1: ( '>' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26456:1: ( '>' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26457:1: '>'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getOpOtherAccess().getGreaterThanSignKeyword_5_1_0_0_1());
}
match(input,26,FollowSets002.FOLLOW_26_in_rule__OpOther__Group_5_1_0_0__1__Impl53545); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getOpOtherAccess().getGreaterThanSignKeyword_5_1_0_0_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__OpOther__Group_5_1_0_0__1__Impl"
// $ANTLR start "rule__OpOther__Group_6__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26474:1: rule__OpOther__Group_6__0 : rule__OpOther__Group_6__0__Impl rule__OpOther__Group_6__1 ;
public final void rule__OpOther__Group_6__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26478:1: ( rule__OpOther__Group_6__0__Impl rule__OpOther__Group_6__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26479:2: rule__OpOther__Group_6__0__Impl rule__OpOther__Group_6__1
{
pushFollow(FollowSets002.FOLLOW_rule__OpOther__Group_6__0__Impl_in_rule__OpOther__Group_6__053580);
rule__OpOther__Group_6__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__OpOther__Group_6__1_in_rule__OpOther__Group_6__053583);
rule__OpOther__Group_6__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__OpOther__Group_6__0"
// $ANTLR start "rule__OpOther__Group_6__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26486:1: rule__OpOther__Group_6__0__Impl : ( '<' ) ;
public final void rule__OpOther__Group_6__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26490:1: ( ( '<' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26491:1: ( '<' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26491:1: ( '<' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26492:1: '<'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getOpOtherAccess().getLessThanSignKeyword_6_0());
}
match(input,27,FollowSets002.FOLLOW_27_in_rule__OpOther__Group_6__0__Impl53611); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getOpOtherAccess().getLessThanSignKeyword_6_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__OpOther__Group_6__0__Impl"
// $ANTLR start "rule__OpOther__Group_6__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26505:1: rule__OpOther__Group_6__1 : rule__OpOther__Group_6__1__Impl ;
public final void rule__OpOther__Group_6__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26509:1: ( rule__OpOther__Group_6__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26510:2: rule__OpOther__Group_6__1__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__OpOther__Group_6__1__Impl_in_rule__OpOther__Group_6__153642);
rule__OpOther__Group_6__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__OpOther__Group_6__1"
// $ANTLR start "rule__OpOther__Group_6__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26516:1: rule__OpOther__Group_6__1__Impl : ( ( rule__OpOther__Alternatives_6_1 ) ) ;
public final void rule__OpOther__Group_6__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26520:1: ( ( ( rule__OpOther__Alternatives_6_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26521:1: ( ( rule__OpOther__Alternatives_6_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26521:1: ( ( rule__OpOther__Alternatives_6_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26522:1: ( rule__OpOther__Alternatives_6_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getOpOtherAccess().getAlternatives_6_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26523:1: ( rule__OpOther__Alternatives_6_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26523:2: rule__OpOther__Alternatives_6_1
{
pushFollow(FollowSets002.FOLLOW_rule__OpOther__Alternatives_6_1_in_rule__OpOther__Group_6__1__Impl53669);
rule__OpOther__Alternatives_6_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getOpOtherAccess().getAlternatives_6_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__OpOther__Group_6__1__Impl"
// $ANTLR start "rule__OpOther__Group_6_1_0__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26537:1: rule__OpOther__Group_6_1_0__0 : rule__OpOther__Group_6_1_0__0__Impl ;
public final void rule__OpOther__Group_6_1_0__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26541:1: ( rule__OpOther__Group_6_1_0__0__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26542:2: rule__OpOther__Group_6_1_0__0__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__OpOther__Group_6_1_0__0__Impl_in_rule__OpOther__Group_6_1_0__053703);
rule__OpOther__Group_6_1_0__0__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__OpOther__Group_6_1_0__0"
// $ANTLR start "rule__OpOther__Group_6_1_0__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26548:1: rule__OpOther__Group_6_1_0__0__Impl : ( ( rule__OpOther__Group_6_1_0_0__0 ) ) ;
public final void rule__OpOther__Group_6_1_0__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26552:1: ( ( ( rule__OpOther__Group_6_1_0_0__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26553:1: ( ( rule__OpOther__Group_6_1_0_0__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26553:1: ( ( rule__OpOther__Group_6_1_0_0__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26554:1: ( rule__OpOther__Group_6_1_0_0__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getOpOtherAccess().getGroup_6_1_0_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26555:1: ( rule__OpOther__Group_6_1_0_0__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26555:2: rule__OpOther__Group_6_1_0_0__0
{
pushFollow(FollowSets002.FOLLOW_rule__OpOther__Group_6_1_0_0__0_in_rule__OpOther__Group_6_1_0__0__Impl53730);
rule__OpOther__Group_6_1_0_0__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getOpOtherAccess().getGroup_6_1_0_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__OpOther__Group_6_1_0__0__Impl"
// $ANTLR start "rule__OpOther__Group_6_1_0_0__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26567:1: rule__OpOther__Group_6_1_0_0__0 : rule__OpOther__Group_6_1_0_0__0__Impl rule__OpOther__Group_6_1_0_0__1 ;
public final void rule__OpOther__Group_6_1_0_0__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26571:1: ( rule__OpOther__Group_6_1_0_0__0__Impl rule__OpOther__Group_6_1_0_0__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26572:2: rule__OpOther__Group_6_1_0_0__0__Impl rule__OpOther__Group_6_1_0_0__1
{
pushFollow(FollowSets002.FOLLOW_rule__OpOther__Group_6_1_0_0__0__Impl_in_rule__OpOther__Group_6_1_0_0__053762);
rule__OpOther__Group_6_1_0_0__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__OpOther__Group_6_1_0_0__1_in_rule__OpOther__Group_6_1_0_0__053765);
rule__OpOther__Group_6_1_0_0__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__OpOther__Group_6_1_0_0__0"
// $ANTLR start "rule__OpOther__Group_6_1_0_0__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26579:1: rule__OpOther__Group_6_1_0_0__0__Impl : ( '<' ) ;
public final void rule__OpOther__Group_6_1_0_0__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26583:1: ( ( '<' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26584:1: ( '<' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26584:1: ( '<' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26585:1: '<'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getOpOtherAccess().getLessThanSignKeyword_6_1_0_0_0());
}
match(input,27,FollowSets002.FOLLOW_27_in_rule__OpOther__Group_6_1_0_0__0__Impl53793); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getOpOtherAccess().getLessThanSignKeyword_6_1_0_0_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__OpOther__Group_6_1_0_0__0__Impl"
// $ANTLR start "rule__OpOther__Group_6_1_0_0__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26598:1: rule__OpOther__Group_6_1_0_0__1 : rule__OpOther__Group_6_1_0_0__1__Impl ;
public final void rule__OpOther__Group_6_1_0_0__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26602:1: ( rule__OpOther__Group_6_1_0_0__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26603:2: rule__OpOther__Group_6_1_0_0__1__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__OpOther__Group_6_1_0_0__1__Impl_in_rule__OpOther__Group_6_1_0_0__153824);
rule__OpOther__Group_6_1_0_0__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__OpOther__Group_6_1_0_0__1"
// $ANTLR start "rule__OpOther__Group_6_1_0_0__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26609:1: rule__OpOther__Group_6_1_0_0__1__Impl : ( '<' ) ;
public final void rule__OpOther__Group_6_1_0_0__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26613:1: ( ( '<' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26614:1: ( '<' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26614:1: ( '<' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26615:1: '<'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getOpOtherAccess().getLessThanSignKeyword_6_1_0_0_1());
}
match(input,27,FollowSets002.FOLLOW_27_in_rule__OpOther__Group_6_1_0_0__1__Impl53852); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getOpOtherAccess().getLessThanSignKeyword_6_1_0_0_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__OpOther__Group_6_1_0_0__1__Impl"
// $ANTLR start "rule__XAdditiveExpression__Group__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26632:1: rule__XAdditiveExpression__Group__0 : rule__XAdditiveExpression__Group__0__Impl rule__XAdditiveExpression__Group__1 ;
public final void rule__XAdditiveExpression__Group__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26636:1: ( rule__XAdditiveExpression__Group__0__Impl rule__XAdditiveExpression__Group__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26637:2: rule__XAdditiveExpression__Group__0__Impl rule__XAdditiveExpression__Group__1
{
pushFollow(FollowSets002.FOLLOW_rule__XAdditiveExpression__Group__0__Impl_in_rule__XAdditiveExpression__Group__053887);
rule__XAdditiveExpression__Group__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XAdditiveExpression__Group__1_in_rule__XAdditiveExpression__Group__053890);
rule__XAdditiveExpression__Group__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XAdditiveExpression__Group__0"
// $ANTLR start "rule__XAdditiveExpression__Group__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26644:1: rule__XAdditiveExpression__Group__0__Impl : ( ruleXMultiplicativeExpression ) ;
public final void rule__XAdditiveExpression__Group__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26648:1: ( ( ruleXMultiplicativeExpression ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26649:1: ( ruleXMultiplicativeExpression )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26649:1: ( ruleXMultiplicativeExpression )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26650:1: ruleXMultiplicativeExpression
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXAdditiveExpressionAccess().getXMultiplicativeExpressionParserRuleCall_0());
}
pushFollow(FollowSets002.FOLLOW_ruleXMultiplicativeExpression_in_rule__XAdditiveExpression__Group__0__Impl53917);
ruleXMultiplicativeExpression();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXAdditiveExpressionAccess().getXMultiplicativeExpressionParserRuleCall_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XAdditiveExpression__Group__0__Impl"
// $ANTLR start "rule__XAdditiveExpression__Group__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26661:1: rule__XAdditiveExpression__Group__1 : rule__XAdditiveExpression__Group__1__Impl ;
public final void rule__XAdditiveExpression__Group__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26665:1: ( rule__XAdditiveExpression__Group__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26666:2: rule__XAdditiveExpression__Group__1__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__XAdditiveExpression__Group__1__Impl_in_rule__XAdditiveExpression__Group__153946);
rule__XAdditiveExpression__Group__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XAdditiveExpression__Group__1"
// $ANTLR start "rule__XAdditiveExpression__Group__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26672:1: rule__XAdditiveExpression__Group__1__Impl : ( ( rule__XAdditiveExpression__Group_1__0 )* ) ;
public final void rule__XAdditiveExpression__Group__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26676:1: ( ( ( rule__XAdditiveExpression__Group_1__0 )* ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26677:1: ( ( rule__XAdditiveExpression__Group_1__0 )* )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26677:1: ( ( rule__XAdditiveExpression__Group_1__0 )* )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26678:1: ( rule__XAdditiveExpression__Group_1__0 )*
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXAdditiveExpressionAccess().getGroup_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26679:1: ( rule__XAdditiveExpression__Group_1__0 )*
loop203:
do {
int alt203=2;
int LA203_0 = input.LA(1);
if ( (LA203_0==35) ) {
int LA203_2 = input.LA(2);
if ( (synpred289_InternalMango()) ) {
alt203=1;
}
}
else if ( (LA203_0==34) ) {
int LA203_3 = input.LA(2);
if ( (synpred289_InternalMango()) ) {
alt203=1;
}
}
switch (alt203) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26679:2: rule__XAdditiveExpression__Group_1__0
{
pushFollow(FollowSets002.FOLLOW_rule__XAdditiveExpression__Group_1__0_in_rule__XAdditiveExpression__Group__1__Impl53973);
rule__XAdditiveExpression__Group_1__0();
state._fsp--;
if (state.failed) return ;
}
break;
default :
break loop203;
}
} while (true);
if ( state.backtracking==0 ) {
after(grammarAccess.getXAdditiveExpressionAccess().getGroup_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XAdditiveExpression__Group__1__Impl"
// $ANTLR start "rule__XAdditiveExpression__Group_1__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26693:1: rule__XAdditiveExpression__Group_1__0 : rule__XAdditiveExpression__Group_1__0__Impl rule__XAdditiveExpression__Group_1__1 ;
public final void rule__XAdditiveExpression__Group_1__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26697:1: ( rule__XAdditiveExpression__Group_1__0__Impl rule__XAdditiveExpression__Group_1__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26698:2: rule__XAdditiveExpression__Group_1__0__Impl rule__XAdditiveExpression__Group_1__1
{
pushFollow(FollowSets002.FOLLOW_rule__XAdditiveExpression__Group_1__0__Impl_in_rule__XAdditiveExpression__Group_1__054008);
rule__XAdditiveExpression__Group_1__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XAdditiveExpression__Group_1__1_in_rule__XAdditiveExpression__Group_1__054011);
rule__XAdditiveExpression__Group_1__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XAdditiveExpression__Group_1__0"
// $ANTLR start "rule__XAdditiveExpression__Group_1__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26705:1: rule__XAdditiveExpression__Group_1__0__Impl : ( ( rule__XAdditiveExpression__Group_1_0__0 ) ) ;
public final void rule__XAdditiveExpression__Group_1__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26709:1: ( ( ( rule__XAdditiveExpression__Group_1_0__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26710:1: ( ( rule__XAdditiveExpression__Group_1_0__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26710:1: ( ( rule__XAdditiveExpression__Group_1_0__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26711:1: ( rule__XAdditiveExpression__Group_1_0__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXAdditiveExpressionAccess().getGroup_1_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26712:1: ( rule__XAdditiveExpression__Group_1_0__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26712:2: rule__XAdditiveExpression__Group_1_0__0
{
pushFollow(FollowSets002.FOLLOW_rule__XAdditiveExpression__Group_1_0__0_in_rule__XAdditiveExpression__Group_1__0__Impl54038);
rule__XAdditiveExpression__Group_1_0__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXAdditiveExpressionAccess().getGroup_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XAdditiveExpression__Group_1__0__Impl"
// $ANTLR start "rule__XAdditiveExpression__Group_1__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26722:1: rule__XAdditiveExpression__Group_1__1 : rule__XAdditiveExpression__Group_1__1__Impl ;
public final void rule__XAdditiveExpression__Group_1__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26726:1: ( rule__XAdditiveExpression__Group_1__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26727:2: rule__XAdditiveExpression__Group_1__1__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__XAdditiveExpression__Group_1__1__Impl_in_rule__XAdditiveExpression__Group_1__154068);
rule__XAdditiveExpression__Group_1__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XAdditiveExpression__Group_1__1"
// $ANTLR start "rule__XAdditiveExpression__Group_1__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26733:1: rule__XAdditiveExpression__Group_1__1__Impl : ( ( rule__XAdditiveExpression__RightOperandAssignment_1_1 ) ) ;
public final void rule__XAdditiveExpression__Group_1__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26737:1: ( ( ( rule__XAdditiveExpression__RightOperandAssignment_1_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26738:1: ( ( rule__XAdditiveExpression__RightOperandAssignment_1_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26738:1: ( ( rule__XAdditiveExpression__RightOperandAssignment_1_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26739:1: ( rule__XAdditiveExpression__RightOperandAssignment_1_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXAdditiveExpressionAccess().getRightOperandAssignment_1_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26740:1: ( rule__XAdditiveExpression__RightOperandAssignment_1_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26740:2: rule__XAdditiveExpression__RightOperandAssignment_1_1
{
pushFollow(FollowSets002.FOLLOW_rule__XAdditiveExpression__RightOperandAssignment_1_1_in_rule__XAdditiveExpression__Group_1__1__Impl54095);
rule__XAdditiveExpression__RightOperandAssignment_1_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXAdditiveExpressionAccess().getRightOperandAssignment_1_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XAdditiveExpression__Group_1__1__Impl"
// $ANTLR start "rule__XAdditiveExpression__Group_1_0__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26754:1: rule__XAdditiveExpression__Group_1_0__0 : rule__XAdditiveExpression__Group_1_0__0__Impl ;
public final void rule__XAdditiveExpression__Group_1_0__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26758:1: ( rule__XAdditiveExpression__Group_1_0__0__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26759:2: rule__XAdditiveExpression__Group_1_0__0__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__XAdditiveExpression__Group_1_0__0__Impl_in_rule__XAdditiveExpression__Group_1_0__054129);
rule__XAdditiveExpression__Group_1_0__0__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XAdditiveExpression__Group_1_0__0"
// $ANTLR start "rule__XAdditiveExpression__Group_1_0__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26765:1: rule__XAdditiveExpression__Group_1_0__0__Impl : ( ( rule__XAdditiveExpression__Group_1_0_0__0 ) ) ;
public final void rule__XAdditiveExpression__Group_1_0__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26769:1: ( ( ( rule__XAdditiveExpression__Group_1_0_0__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26770:1: ( ( rule__XAdditiveExpression__Group_1_0_0__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26770:1: ( ( rule__XAdditiveExpression__Group_1_0_0__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26771:1: ( rule__XAdditiveExpression__Group_1_0_0__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXAdditiveExpressionAccess().getGroup_1_0_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26772:1: ( rule__XAdditiveExpression__Group_1_0_0__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26772:2: rule__XAdditiveExpression__Group_1_0_0__0
{
pushFollow(FollowSets002.FOLLOW_rule__XAdditiveExpression__Group_1_0_0__0_in_rule__XAdditiveExpression__Group_1_0__0__Impl54156);
rule__XAdditiveExpression__Group_1_0_0__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXAdditiveExpressionAccess().getGroup_1_0_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XAdditiveExpression__Group_1_0__0__Impl"
// $ANTLR start "rule__XAdditiveExpression__Group_1_0_0__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26784:1: rule__XAdditiveExpression__Group_1_0_0__0 : rule__XAdditiveExpression__Group_1_0_0__0__Impl rule__XAdditiveExpression__Group_1_0_0__1 ;
public final void rule__XAdditiveExpression__Group_1_0_0__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26788:1: ( rule__XAdditiveExpression__Group_1_0_0__0__Impl rule__XAdditiveExpression__Group_1_0_0__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26789:2: rule__XAdditiveExpression__Group_1_0_0__0__Impl rule__XAdditiveExpression__Group_1_0_0__1
{
pushFollow(FollowSets002.FOLLOW_rule__XAdditiveExpression__Group_1_0_0__0__Impl_in_rule__XAdditiveExpression__Group_1_0_0__054188);
rule__XAdditiveExpression__Group_1_0_0__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XAdditiveExpression__Group_1_0_0__1_in_rule__XAdditiveExpression__Group_1_0_0__054191);
rule__XAdditiveExpression__Group_1_0_0__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XAdditiveExpression__Group_1_0_0__0"
// $ANTLR start "rule__XAdditiveExpression__Group_1_0_0__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26796:1: rule__XAdditiveExpression__Group_1_0_0__0__Impl : ( () ) ;
public final void rule__XAdditiveExpression__Group_1_0_0__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26800:1: ( ( () ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26801:1: ( () )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26801:1: ( () )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26802:1: ()
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXAdditiveExpressionAccess().getXBinaryOperationLeftOperandAction_1_0_0_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26803:1: ()
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26805:1:
{
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXAdditiveExpressionAccess().getXBinaryOperationLeftOperandAction_1_0_0_0());
}
}
}
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XAdditiveExpression__Group_1_0_0__0__Impl"
// $ANTLR start "rule__XAdditiveExpression__Group_1_0_0__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26815:1: rule__XAdditiveExpression__Group_1_0_0__1 : rule__XAdditiveExpression__Group_1_0_0__1__Impl ;
public final void rule__XAdditiveExpression__Group_1_0_0__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26819:1: ( rule__XAdditiveExpression__Group_1_0_0__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26820:2: rule__XAdditiveExpression__Group_1_0_0__1__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__XAdditiveExpression__Group_1_0_0__1__Impl_in_rule__XAdditiveExpression__Group_1_0_0__154249);
rule__XAdditiveExpression__Group_1_0_0__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XAdditiveExpression__Group_1_0_0__1"
// $ANTLR start "rule__XAdditiveExpression__Group_1_0_0__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26826:1: rule__XAdditiveExpression__Group_1_0_0__1__Impl : ( ( rule__XAdditiveExpression__FeatureAssignment_1_0_0_1 ) ) ;
public final void rule__XAdditiveExpression__Group_1_0_0__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26830:1: ( ( ( rule__XAdditiveExpression__FeatureAssignment_1_0_0_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26831:1: ( ( rule__XAdditiveExpression__FeatureAssignment_1_0_0_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26831:1: ( ( rule__XAdditiveExpression__FeatureAssignment_1_0_0_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26832:1: ( rule__XAdditiveExpression__FeatureAssignment_1_0_0_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXAdditiveExpressionAccess().getFeatureAssignment_1_0_0_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26833:1: ( rule__XAdditiveExpression__FeatureAssignment_1_0_0_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26833:2: rule__XAdditiveExpression__FeatureAssignment_1_0_0_1
{
pushFollow(FollowSets002.FOLLOW_rule__XAdditiveExpression__FeatureAssignment_1_0_0_1_in_rule__XAdditiveExpression__Group_1_0_0__1__Impl54276);
rule__XAdditiveExpression__FeatureAssignment_1_0_0_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXAdditiveExpressionAccess().getFeatureAssignment_1_0_0_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XAdditiveExpression__Group_1_0_0__1__Impl"
// $ANTLR start "rule__XMultiplicativeExpression__Group__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26847:1: rule__XMultiplicativeExpression__Group__0 : rule__XMultiplicativeExpression__Group__0__Impl rule__XMultiplicativeExpression__Group__1 ;
public final void rule__XMultiplicativeExpression__Group__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26851:1: ( rule__XMultiplicativeExpression__Group__0__Impl rule__XMultiplicativeExpression__Group__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26852:2: rule__XMultiplicativeExpression__Group__0__Impl rule__XMultiplicativeExpression__Group__1
{
pushFollow(FollowSets002.FOLLOW_rule__XMultiplicativeExpression__Group__0__Impl_in_rule__XMultiplicativeExpression__Group__054310);
rule__XMultiplicativeExpression__Group__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XMultiplicativeExpression__Group__1_in_rule__XMultiplicativeExpression__Group__054313);
rule__XMultiplicativeExpression__Group__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XMultiplicativeExpression__Group__0"
// $ANTLR start "rule__XMultiplicativeExpression__Group__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26859:1: rule__XMultiplicativeExpression__Group__0__Impl : ( ruleXUnaryOperation ) ;
public final void rule__XMultiplicativeExpression__Group__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26863:1: ( ( ruleXUnaryOperation ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26864:1: ( ruleXUnaryOperation )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26864:1: ( ruleXUnaryOperation )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26865:1: ruleXUnaryOperation
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXMultiplicativeExpressionAccess().getXUnaryOperationParserRuleCall_0());
}
pushFollow(FollowSets002.FOLLOW_ruleXUnaryOperation_in_rule__XMultiplicativeExpression__Group__0__Impl54340);
ruleXUnaryOperation();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXMultiplicativeExpressionAccess().getXUnaryOperationParserRuleCall_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XMultiplicativeExpression__Group__0__Impl"
// $ANTLR start "rule__XMultiplicativeExpression__Group__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26876:1: rule__XMultiplicativeExpression__Group__1 : rule__XMultiplicativeExpression__Group__1__Impl ;
public final void rule__XMultiplicativeExpression__Group__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26880:1: ( rule__XMultiplicativeExpression__Group__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26881:2: rule__XMultiplicativeExpression__Group__1__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__XMultiplicativeExpression__Group__1__Impl_in_rule__XMultiplicativeExpression__Group__154369);
rule__XMultiplicativeExpression__Group__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XMultiplicativeExpression__Group__1"
// $ANTLR start "rule__XMultiplicativeExpression__Group__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26887:1: rule__XMultiplicativeExpression__Group__1__Impl : ( ( rule__XMultiplicativeExpression__Group_1__0 )* ) ;
public final void rule__XMultiplicativeExpression__Group__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26891:1: ( ( ( rule__XMultiplicativeExpression__Group_1__0 )* ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26892:1: ( ( rule__XMultiplicativeExpression__Group_1__0 )* )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26892:1: ( ( rule__XMultiplicativeExpression__Group_1__0 )* )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26893:1: ( rule__XMultiplicativeExpression__Group_1__0 )*
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXMultiplicativeExpressionAccess().getGroup_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26894:1: ( rule__XMultiplicativeExpression__Group_1__0 )*
loop204:
do {
int alt204=2;
switch ( input.LA(1) ) {
case 36:
{
int LA204_2 = input.LA(2);
if ( (synpred290_InternalMango()) ) {
alt204=1;
}
}
break;
case 37:
{
int LA204_3 = input.LA(2);
if ( (synpred290_InternalMango()) ) {
alt204=1;
}
}
break;
case 38:
{
int LA204_4 = input.LA(2);
if ( (synpred290_InternalMango()) ) {
alt204=1;
}
}
break;
case 39:
{
int LA204_5 = input.LA(2);
if ( (synpred290_InternalMango()) ) {
alt204=1;
}
}
break;
}
switch (alt204) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26894:2: rule__XMultiplicativeExpression__Group_1__0
{
pushFollow(FollowSets002.FOLLOW_rule__XMultiplicativeExpression__Group_1__0_in_rule__XMultiplicativeExpression__Group__1__Impl54396);
rule__XMultiplicativeExpression__Group_1__0();
state._fsp--;
if (state.failed) return ;
}
break;
default :
break loop204;
}
} while (true);
if ( state.backtracking==0 ) {
after(grammarAccess.getXMultiplicativeExpressionAccess().getGroup_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XMultiplicativeExpression__Group__1__Impl"
// $ANTLR start "rule__XMultiplicativeExpression__Group_1__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26908:1: rule__XMultiplicativeExpression__Group_1__0 : rule__XMultiplicativeExpression__Group_1__0__Impl rule__XMultiplicativeExpression__Group_1__1 ;
public final void rule__XMultiplicativeExpression__Group_1__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26912:1: ( rule__XMultiplicativeExpression__Group_1__0__Impl rule__XMultiplicativeExpression__Group_1__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26913:2: rule__XMultiplicativeExpression__Group_1__0__Impl rule__XMultiplicativeExpression__Group_1__1
{
pushFollow(FollowSets002.FOLLOW_rule__XMultiplicativeExpression__Group_1__0__Impl_in_rule__XMultiplicativeExpression__Group_1__054431);
rule__XMultiplicativeExpression__Group_1__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XMultiplicativeExpression__Group_1__1_in_rule__XMultiplicativeExpression__Group_1__054434);
rule__XMultiplicativeExpression__Group_1__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XMultiplicativeExpression__Group_1__0"
// $ANTLR start "rule__XMultiplicativeExpression__Group_1__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26920:1: rule__XMultiplicativeExpression__Group_1__0__Impl : ( ( rule__XMultiplicativeExpression__Group_1_0__0 ) ) ;
public final void rule__XMultiplicativeExpression__Group_1__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26924:1: ( ( ( rule__XMultiplicativeExpression__Group_1_0__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26925:1: ( ( rule__XMultiplicativeExpression__Group_1_0__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26925:1: ( ( rule__XMultiplicativeExpression__Group_1_0__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26926:1: ( rule__XMultiplicativeExpression__Group_1_0__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXMultiplicativeExpressionAccess().getGroup_1_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26927:1: ( rule__XMultiplicativeExpression__Group_1_0__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26927:2: rule__XMultiplicativeExpression__Group_1_0__0
{
pushFollow(FollowSets002.FOLLOW_rule__XMultiplicativeExpression__Group_1_0__0_in_rule__XMultiplicativeExpression__Group_1__0__Impl54461);
rule__XMultiplicativeExpression__Group_1_0__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXMultiplicativeExpressionAccess().getGroup_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XMultiplicativeExpression__Group_1__0__Impl"
// $ANTLR start "rule__XMultiplicativeExpression__Group_1__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26937:1: rule__XMultiplicativeExpression__Group_1__1 : rule__XMultiplicativeExpression__Group_1__1__Impl ;
public final void rule__XMultiplicativeExpression__Group_1__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26941:1: ( rule__XMultiplicativeExpression__Group_1__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26942:2: rule__XMultiplicativeExpression__Group_1__1__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__XMultiplicativeExpression__Group_1__1__Impl_in_rule__XMultiplicativeExpression__Group_1__154491);
rule__XMultiplicativeExpression__Group_1__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XMultiplicativeExpression__Group_1__1"
// $ANTLR start "rule__XMultiplicativeExpression__Group_1__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26948:1: rule__XMultiplicativeExpression__Group_1__1__Impl : ( ( rule__XMultiplicativeExpression__RightOperandAssignment_1_1 ) ) ;
public final void rule__XMultiplicativeExpression__Group_1__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26952:1: ( ( ( rule__XMultiplicativeExpression__RightOperandAssignment_1_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26953:1: ( ( rule__XMultiplicativeExpression__RightOperandAssignment_1_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26953:1: ( ( rule__XMultiplicativeExpression__RightOperandAssignment_1_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26954:1: ( rule__XMultiplicativeExpression__RightOperandAssignment_1_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXMultiplicativeExpressionAccess().getRightOperandAssignment_1_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26955:1: ( rule__XMultiplicativeExpression__RightOperandAssignment_1_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26955:2: rule__XMultiplicativeExpression__RightOperandAssignment_1_1
{
pushFollow(FollowSets002.FOLLOW_rule__XMultiplicativeExpression__RightOperandAssignment_1_1_in_rule__XMultiplicativeExpression__Group_1__1__Impl54518);
rule__XMultiplicativeExpression__RightOperandAssignment_1_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXMultiplicativeExpressionAccess().getRightOperandAssignment_1_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XMultiplicativeExpression__Group_1__1__Impl"
// $ANTLR start "rule__XMultiplicativeExpression__Group_1_0__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26969:1: rule__XMultiplicativeExpression__Group_1_0__0 : rule__XMultiplicativeExpression__Group_1_0__0__Impl ;
public final void rule__XMultiplicativeExpression__Group_1_0__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26973:1: ( rule__XMultiplicativeExpression__Group_1_0__0__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26974:2: rule__XMultiplicativeExpression__Group_1_0__0__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__XMultiplicativeExpression__Group_1_0__0__Impl_in_rule__XMultiplicativeExpression__Group_1_0__054552);
rule__XMultiplicativeExpression__Group_1_0__0__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XMultiplicativeExpression__Group_1_0__0"
// $ANTLR start "rule__XMultiplicativeExpression__Group_1_0__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26980:1: rule__XMultiplicativeExpression__Group_1_0__0__Impl : ( ( rule__XMultiplicativeExpression__Group_1_0_0__0 ) ) ;
public final void rule__XMultiplicativeExpression__Group_1_0__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26984:1: ( ( ( rule__XMultiplicativeExpression__Group_1_0_0__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26985:1: ( ( rule__XMultiplicativeExpression__Group_1_0_0__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26985:1: ( ( rule__XMultiplicativeExpression__Group_1_0_0__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26986:1: ( rule__XMultiplicativeExpression__Group_1_0_0__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXMultiplicativeExpressionAccess().getGroup_1_0_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26987:1: ( rule__XMultiplicativeExpression__Group_1_0_0__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26987:2: rule__XMultiplicativeExpression__Group_1_0_0__0
{
pushFollow(FollowSets002.FOLLOW_rule__XMultiplicativeExpression__Group_1_0_0__0_in_rule__XMultiplicativeExpression__Group_1_0__0__Impl54579);
rule__XMultiplicativeExpression__Group_1_0_0__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXMultiplicativeExpressionAccess().getGroup_1_0_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XMultiplicativeExpression__Group_1_0__0__Impl"
// $ANTLR start "rule__XMultiplicativeExpression__Group_1_0_0__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26999:1: rule__XMultiplicativeExpression__Group_1_0_0__0 : rule__XMultiplicativeExpression__Group_1_0_0__0__Impl rule__XMultiplicativeExpression__Group_1_0_0__1 ;
public final void rule__XMultiplicativeExpression__Group_1_0_0__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27003:1: ( rule__XMultiplicativeExpression__Group_1_0_0__0__Impl rule__XMultiplicativeExpression__Group_1_0_0__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27004:2: rule__XMultiplicativeExpression__Group_1_0_0__0__Impl rule__XMultiplicativeExpression__Group_1_0_0__1
{
pushFollow(FollowSets002.FOLLOW_rule__XMultiplicativeExpression__Group_1_0_0__0__Impl_in_rule__XMultiplicativeExpression__Group_1_0_0__054611);
rule__XMultiplicativeExpression__Group_1_0_0__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XMultiplicativeExpression__Group_1_0_0__1_in_rule__XMultiplicativeExpression__Group_1_0_0__054614);
rule__XMultiplicativeExpression__Group_1_0_0__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XMultiplicativeExpression__Group_1_0_0__0"
// $ANTLR start "rule__XMultiplicativeExpression__Group_1_0_0__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27011:1: rule__XMultiplicativeExpression__Group_1_0_0__0__Impl : ( () ) ;
public final void rule__XMultiplicativeExpression__Group_1_0_0__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27015:1: ( ( () ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27016:1: ( () )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27016:1: ( () )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27017:1: ()
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXMultiplicativeExpressionAccess().getXBinaryOperationLeftOperandAction_1_0_0_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27018:1: ()
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27020:1:
{
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXMultiplicativeExpressionAccess().getXBinaryOperationLeftOperandAction_1_0_0_0());
}
}
}
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XMultiplicativeExpression__Group_1_0_0__0__Impl"
// $ANTLR start "rule__XMultiplicativeExpression__Group_1_0_0__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27030:1: rule__XMultiplicativeExpression__Group_1_0_0__1 : rule__XMultiplicativeExpression__Group_1_0_0__1__Impl ;
public final void rule__XMultiplicativeExpression__Group_1_0_0__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27034:1: ( rule__XMultiplicativeExpression__Group_1_0_0__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27035:2: rule__XMultiplicativeExpression__Group_1_0_0__1__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__XMultiplicativeExpression__Group_1_0_0__1__Impl_in_rule__XMultiplicativeExpression__Group_1_0_0__154672);
rule__XMultiplicativeExpression__Group_1_0_0__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XMultiplicativeExpression__Group_1_0_0__1"
// $ANTLR start "rule__XMultiplicativeExpression__Group_1_0_0__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27041:1: rule__XMultiplicativeExpression__Group_1_0_0__1__Impl : ( ( rule__XMultiplicativeExpression__FeatureAssignment_1_0_0_1 ) ) ;
public final void rule__XMultiplicativeExpression__Group_1_0_0__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27045:1: ( ( ( rule__XMultiplicativeExpression__FeatureAssignment_1_0_0_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27046:1: ( ( rule__XMultiplicativeExpression__FeatureAssignment_1_0_0_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27046:1: ( ( rule__XMultiplicativeExpression__FeatureAssignment_1_0_0_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27047:1: ( rule__XMultiplicativeExpression__FeatureAssignment_1_0_0_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXMultiplicativeExpressionAccess().getFeatureAssignment_1_0_0_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27048:1: ( rule__XMultiplicativeExpression__FeatureAssignment_1_0_0_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27048:2: rule__XMultiplicativeExpression__FeatureAssignment_1_0_0_1
{
pushFollow(FollowSets002.FOLLOW_rule__XMultiplicativeExpression__FeatureAssignment_1_0_0_1_in_rule__XMultiplicativeExpression__Group_1_0_0__1__Impl54699);
rule__XMultiplicativeExpression__FeatureAssignment_1_0_0_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXMultiplicativeExpressionAccess().getFeatureAssignment_1_0_0_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XMultiplicativeExpression__Group_1_0_0__1__Impl"
// $ANTLR start "rule__XUnaryOperation__Group_0__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27062:1: rule__XUnaryOperation__Group_0__0 : rule__XUnaryOperation__Group_0__0__Impl rule__XUnaryOperation__Group_0__1 ;
public final void rule__XUnaryOperation__Group_0__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27066:1: ( rule__XUnaryOperation__Group_0__0__Impl rule__XUnaryOperation__Group_0__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27067:2: rule__XUnaryOperation__Group_0__0__Impl rule__XUnaryOperation__Group_0__1
{
pushFollow(FollowSets002.FOLLOW_rule__XUnaryOperation__Group_0__0__Impl_in_rule__XUnaryOperation__Group_0__054733);
rule__XUnaryOperation__Group_0__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XUnaryOperation__Group_0__1_in_rule__XUnaryOperation__Group_0__054736);
rule__XUnaryOperation__Group_0__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XUnaryOperation__Group_0__0"
// $ANTLR start "rule__XUnaryOperation__Group_0__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27074:1: rule__XUnaryOperation__Group_0__0__Impl : ( () ) ;
public final void rule__XUnaryOperation__Group_0__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27078:1: ( ( () ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27079:1: ( () )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27079:1: ( () )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27080:1: ()
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXUnaryOperationAccess().getXUnaryOperationAction_0_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27081:1: ()
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27083:1:
{
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXUnaryOperationAccess().getXUnaryOperationAction_0_0());
}
}
}
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XUnaryOperation__Group_0__0__Impl"
// $ANTLR start "rule__XUnaryOperation__Group_0__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27093:1: rule__XUnaryOperation__Group_0__1 : rule__XUnaryOperation__Group_0__1__Impl rule__XUnaryOperation__Group_0__2 ;
public final void rule__XUnaryOperation__Group_0__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27097:1: ( rule__XUnaryOperation__Group_0__1__Impl rule__XUnaryOperation__Group_0__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27098:2: rule__XUnaryOperation__Group_0__1__Impl rule__XUnaryOperation__Group_0__2
{
pushFollow(FollowSets002.FOLLOW_rule__XUnaryOperation__Group_0__1__Impl_in_rule__XUnaryOperation__Group_0__154794);
rule__XUnaryOperation__Group_0__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XUnaryOperation__Group_0__2_in_rule__XUnaryOperation__Group_0__154797);
rule__XUnaryOperation__Group_0__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XUnaryOperation__Group_0__1"
// $ANTLR start "rule__XUnaryOperation__Group_0__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27105:1: rule__XUnaryOperation__Group_0__1__Impl : ( ( rule__XUnaryOperation__FeatureAssignment_0_1 ) ) ;
public final void rule__XUnaryOperation__Group_0__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27109:1: ( ( ( rule__XUnaryOperation__FeatureAssignment_0_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27110:1: ( ( rule__XUnaryOperation__FeatureAssignment_0_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27110:1: ( ( rule__XUnaryOperation__FeatureAssignment_0_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27111:1: ( rule__XUnaryOperation__FeatureAssignment_0_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXUnaryOperationAccess().getFeatureAssignment_0_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27112:1: ( rule__XUnaryOperation__FeatureAssignment_0_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27112:2: rule__XUnaryOperation__FeatureAssignment_0_1
{
pushFollow(FollowSets002.FOLLOW_rule__XUnaryOperation__FeatureAssignment_0_1_in_rule__XUnaryOperation__Group_0__1__Impl54824);
rule__XUnaryOperation__FeatureAssignment_0_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXUnaryOperationAccess().getFeatureAssignment_0_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XUnaryOperation__Group_0__1__Impl"
// $ANTLR start "rule__XUnaryOperation__Group_0__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27122:1: rule__XUnaryOperation__Group_0__2 : rule__XUnaryOperation__Group_0__2__Impl ;
public final void rule__XUnaryOperation__Group_0__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27126:1: ( rule__XUnaryOperation__Group_0__2__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27127:2: rule__XUnaryOperation__Group_0__2__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__XUnaryOperation__Group_0__2__Impl_in_rule__XUnaryOperation__Group_0__254854);
rule__XUnaryOperation__Group_0__2__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XUnaryOperation__Group_0__2"
// $ANTLR start "rule__XUnaryOperation__Group_0__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27133:1: rule__XUnaryOperation__Group_0__2__Impl : ( ( rule__XUnaryOperation__OperandAssignment_0_2 ) ) ;
public final void rule__XUnaryOperation__Group_0__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27137:1: ( ( ( rule__XUnaryOperation__OperandAssignment_0_2 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27138:1: ( ( rule__XUnaryOperation__OperandAssignment_0_2 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27138:1: ( ( rule__XUnaryOperation__OperandAssignment_0_2 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27139:1: ( rule__XUnaryOperation__OperandAssignment_0_2 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXUnaryOperationAccess().getOperandAssignment_0_2());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27140:1: ( rule__XUnaryOperation__OperandAssignment_0_2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27140:2: rule__XUnaryOperation__OperandAssignment_0_2
{
pushFollow(FollowSets002.FOLLOW_rule__XUnaryOperation__OperandAssignment_0_2_in_rule__XUnaryOperation__Group_0__2__Impl54881);
rule__XUnaryOperation__OperandAssignment_0_2();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXUnaryOperationAccess().getOperandAssignment_0_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XUnaryOperation__Group_0__2__Impl"
// $ANTLR start "rule__XCastedExpression__Group__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27156:1: rule__XCastedExpression__Group__0 : rule__XCastedExpression__Group__0__Impl rule__XCastedExpression__Group__1 ;
public final void rule__XCastedExpression__Group__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27160:1: ( rule__XCastedExpression__Group__0__Impl rule__XCastedExpression__Group__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27161:2: rule__XCastedExpression__Group__0__Impl rule__XCastedExpression__Group__1
{
pushFollow(FollowSets002.FOLLOW_rule__XCastedExpression__Group__0__Impl_in_rule__XCastedExpression__Group__054917);
rule__XCastedExpression__Group__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XCastedExpression__Group__1_in_rule__XCastedExpression__Group__054920);
rule__XCastedExpression__Group__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XCastedExpression__Group__0"
// $ANTLR start "rule__XCastedExpression__Group__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27168:1: rule__XCastedExpression__Group__0__Impl : ( ruleXPostfixOperation ) ;
public final void rule__XCastedExpression__Group__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27172:1: ( ( ruleXPostfixOperation ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27173:1: ( ruleXPostfixOperation )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27173:1: ( ruleXPostfixOperation )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27174:1: ruleXPostfixOperation
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXCastedExpressionAccess().getXPostfixOperationParserRuleCall_0());
}
pushFollow(FollowSets002.FOLLOW_ruleXPostfixOperation_in_rule__XCastedExpression__Group__0__Impl54947);
ruleXPostfixOperation();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXCastedExpressionAccess().getXPostfixOperationParserRuleCall_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XCastedExpression__Group__0__Impl"
// $ANTLR start "rule__XCastedExpression__Group__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27185:1: rule__XCastedExpression__Group__1 : rule__XCastedExpression__Group__1__Impl ;
public final void rule__XCastedExpression__Group__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27189:1: ( rule__XCastedExpression__Group__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27190:2: rule__XCastedExpression__Group__1__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__XCastedExpression__Group__1__Impl_in_rule__XCastedExpression__Group__154976);
rule__XCastedExpression__Group__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XCastedExpression__Group__1"
// $ANTLR start "rule__XCastedExpression__Group__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27196:1: rule__XCastedExpression__Group__1__Impl : ( ( rule__XCastedExpression__Group_1__0 )* ) ;
public final void rule__XCastedExpression__Group__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27200:1: ( ( ( rule__XCastedExpression__Group_1__0 )* ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27201:1: ( ( rule__XCastedExpression__Group_1__0 )* )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27201:1: ( ( rule__XCastedExpression__Group_1__0 )* )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27202:1: ( rule__XCastedExpression__Group_1__0 )*
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXCastedExpressionAccess().getGroup_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27203:1: ( rule__XCastedExpression__Group_1__0 )*
loop205:
do {
int alt205=2;
int LA205_0 = input.LA(1);
if ( (LA205_0==153) ) {
int LA205_2 = input.LA(2);
if ( (synpred291_InternalMango()) ) {
alt205=1;
}
}
switch (alt205) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27203:2: rule__XCastedExpression__Group_1__0
{
pushFollow(FollowSets002.FOLLOW_rule__XCastedExpression__Group_1__0_in_rule__XCastedExpression__Group__1__Impl55003);
rule__XCastedExpression__Group_1__0();
state._fsp--;
if (state.failed) return ;
}
break;
default :
break loop205;
}
} while (true);
if ( state.backtracking==0 ) {
after(grammarAccess.getXCastedExpressionAccess().getGroup_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XCastedExpression__Group__1__Impl"
// $ANTLR start "rule__XCastedExpression__Group_1__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27217:1: rule__XCastedExpression__Group_1__0 : rule__XCastedExpression__Group_1__0__Impl rule__XCastedExpression__Group_1__1 ;
public final void rule__XCastedExpression__Group_1__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27221:1: ( rule__XCastedExpression__Group_1__0__Impl rule__XCastedExpression__Group_1__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27222:2: rule__XCastedExpression__Group_1__0__Impl rule__XCastedExpression__Group_1__1
{
pushFollow(FollowSets002.FOLLOW_rule__XCastedExpression__Group_1__0__Impl_in_rule__XCastedExpression__Group_1__055038);
rule__XCastedExpression__Group_1__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XCastedExpression__Group_1__1_in_rule__XCastedExpression__Group_1__055041);
rule__XCastedExpression__Group_1__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XCastedExpression__Group_1__0"
// $ANTLR start "rule__XCastedExpression__Group_1__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27229:1: rule__XCastedExpression__Group_1__0__Impl : ( ( rule__XCastedExpression__Group_1_0__0 ) ) ;
public final void rule__XCastedExpression__Group_1__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27233:1: ( ( ( rule__XCastedExpression__Group_1_0__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27234:1: ( ( rule__XCastedExpression__Group_1_0__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27234:1: ( ( rule__XCastedExpression__Group_1_0__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27235:1: ( rule__XCastedExpression__Group_1_0__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXCastedExpressionAccess().getGroup_1_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27236:1: ( rule__XCastedExpression__Group_1_0__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27236:2: rule__XCastedExpression__Group_1_0__0
{
pushFollow(FollowSets002.FOLLOW_rule__XCastedExpression__Group_1_0__0_in_rule__XCastedExpression__Group_1__0__Impl55068);
rule__XCastedExpression__Group_1_0__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXCastedExpressionAccess().getGroup_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XCastedExpression__Group_1__0__Impl"
// $ANTLR start "rule__XCastedExpression__Group_1__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27246:1: rule__XCastedExpression__Group_1__1 : rule__XCastedExpression__Group_1__1__Impl ;
public final void rule__XCastedExpression__Group_1__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27250:1: ( rule__XCastedExpression__Group_1__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27251:2: rule__XCastedExpression__Group_1__1__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__XCastedExpression__Group_1__1__Impl_in_rule__XCastedExpression__Group_1__155098);
rule__XCastedExpression__Group_1__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XCastedExpression__Group_1__1"
// $ANTLR start "rule__XCastedExpression__Group_1__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27257:1: rule__XCastedExpression__Group_1__1__Impl : ( ( rule__XCastedExpression__TypeAssignment_1_1 ) ) ;
public final void rule__XCastedExpression__Group_1__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27261:1: ( ( ( rule__XCastedExpression__TypeAssignment_1_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27262:1: ( ( rule__XCastedExpression__TypeAssignment_1_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27262:1: ( ( rule__XCastedExpression__TypeAssignment_1_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27263:1: ( rule__XCastedExpression__TypeAssignment_1_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXCastedExpressionAccess().getTypeAssignment_1_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27264:1: ( rule__XCastedExpression__TypeAssignment_1_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27264:2: rule__XCastedExpression__TypeAssignment_1_1
{
pushFollow(FollowSets002.FOLLOW_rule__XCastedExpression__TypeAssignment_1_1_in_rule__XCastedExpression__Group_1__1__Impl55125);
rule__XCastedExpression__TypeAssignment_1_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXCastedExpressionAccess().getTypeAssignment_1_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XCastedExpression__Group_1__1__Impl"
// $ANTLR start "rule__XCastedExpression__Group_1_0__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27278:1: rule__XCastedExpression__Group_1_0__0 : rule__XCastedExpression__Group_1_0__0__Impl ;
public final void rule__XCastedExpression__Group_1_0__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27282:1: ( rule__XCastedExpression__Group_1_0__0__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27283:2: rule__XCastedExpression__Group_1_0__0__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__XCastedExpression__Group_1_0__0__Impl_in_rule__XCastedExpression__Group_1_0__055159);
rule__XCastedExpression__Group_1_0__0__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XCastedExpression__Group_1_0__0"
// $ANTLR start "rule__XCastedExpression__Group_1_0__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27289:1: rule__XCastedExpression__Group_1_0__0__Impl : ( ( rule__XCastedExpression__Group_1_0_0__0 ) ) ;
public final void rule__XCastedExpression__Group_1_0__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27293:1: ( ( ( rule__XCastedExpression__Group_1_0_0__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27294:1: ( ( rule__XCastedExpression__Group_1_0_0__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27294:1: ( ( rule__XCastedExpression__Group_1_0_0__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27295:1: ( rule__XCastedExpression__Group_1_0_0__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXCastedExpressionAccess().getGroup_1_0_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27296:1: ( rule__XCastedExpression__Group_1_0_0__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27296:2: rule__XCastedExpression__Group_1_0_0__0
{
pushFollow(FollowSets002.FOLLOW_rule__XCastedExpression__Group_1_0_0__0_in_rule__XCastedExpression__Group_1_0__0__Impl55186);
rule__XCastedExpression__Group_1_0_0__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXCastedExpressionAccess().getGroup_1_0_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XCastedExpression__Group_1_0__0__Impl"
// $ANTLR start "rule__XCastedExpression__Group_1_0_0__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27308:1: rule__XCastedExpression__Group_1_0_0__0 : rule__XCastedExpression__Group_1_0_0__0__Impl rule__XCastedExpression__Group_1_0_0__1 ;
public final void rule__XCastedExpression__Group_1_0_0__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27312:1: ( rule__XCastedExpression__Group_1_0_0__0__Impl rule__XCastedExpression__Group_1_0_0__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27313:2: rule__XCastedExpression__Group_1_0_0__0__Impl rule__XCastedExpression__Group_1_0_0__1
{
pushFollow(FollowSets002.FOLLOW_rule__XCastedExpression__Group_1_0_0__0__Impl_in_rule__XCastedExpression__Group_1_0_0__055218);
rule__XCastedExpression__Group_1_0_0__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XCastedExpression__Group_1_0_0__1_in_rule__XCastedExpression__Group_1_0_0__055221);
rule__XCastedExpression__Group_1_0_0__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XCastedExpression__Group_1_0_0__0"
// $ANTLR start "rule__XCastedExpression__Group_1_0_0__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27320:1: rule__XCastedExpression__Group_1_0_0__0__Impl : ( () ) ;
public final void rule__XCastedExpression__Group_1_0_0__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27324:1: ( ( () ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27325:1: ( () )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27325:1: ( () )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27326:1: ()
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXCastedExpressionAccess().getXCastedExpressionTargetAction_1_0_0_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27327:1: ()
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27329:1:
{
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXCastedExpressionAccess().getXCastedExpressionTargetAction_1_0_0_0());
}
}
}
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XCastedExpression__Group_1_0_0__0__Impl"
// $ANTLR start "rule__XCastedExpression__Group_1_0_0__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27339:1: rule__XCastedExpression__Group_1_0_0__1 : rule__XCastedExpression__Group_1_0_0__1__Impl ;
public final void rule__XCastedExpression__Group_1_0_0__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27343:1: ( rule__XCastedExpression__Group_1_0_0__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27344:2: rule__XCastedExpression__Group_1_0_0__1__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__XCastedExpression__Group_1_0_0__1__Impl_in_rule__XCastedExpression__Group_1_0_0__155279);
rule__XCastedExpression__Group_1_0_0__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XCastedExpression__Group_1_0_0__1"
// $ANTLR start "rule__XCastedExpression__Group_1_0_0__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27350:1: rule__XCastedExpression__Group_1_0_0__1__Impl : ( 'as' ) ;
public final void rule__XCastedExpression__Group_1_0_0__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27354:1: ( ( 'as' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27355:1: ( 'as' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27355:1: ( 'as' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27356:1: 'as'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXCastedExpressionAccess().getAsKeyword_1_0_0_1());
}
match(input,153,FollowSets002.FOLLOW_153_in_rule__XCastedExpression__Group_1_0_0__1__Impl55307); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXCastedExpressionAccess().getAsKeyword_1_0_0_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XCastedExpression__Group_1_0_0__1__Impl"
// $ANTLR start "rule__XPostfixOperation__Group__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27373:1: rule__XPostfixOperation__Group__0 : rule__XPostfixOperation__Group__0__Impl rule__XPostfixOperation__Group__1 ;
public final void rule__XPostfixOperation__Group__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27377:1: ( rule__XPostfixOperation__Group__0__Impl rule__XPostfixOperation__Group__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27378:2: rule__XPostfixOperation__Group__0__Impl rule__XPostfixOperation__Group__1
{
pushFollow(FollowSets002.FOLLOW_rule__XPostfixOperation__Group__0__Impl_in_rule__XPostfixOperation__Group__055342);
rule__XPostfixOperation__Group__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XPostfixOperation__Group__1_in_rule__XPostfixOperation__Group__055345);
rule__XPostfixOperation__Group__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XPostfixOperation__Group__0"
// $ANTLR start "rule__XPostfixOperation__Group__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27385:1: rule__XPostfixOperation__Group__0__Impl : ( ruleXMemberFeatureCall ) ;
public final void rule__XPostfixOperation__Group__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27389:1: ( ( ruleXMemberFeatureCall ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27390:1: ( ruleXMemberFeatureCall )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27390:1: ( ruleXMemberFeatureCall )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27391:1: ruleXMemberFeatureCall
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXPostfixOperationAccess().getXMemberFeatureCallParserRuleCall_0());
}
pushFollow(FollowSets002.FOLLOW_ruleXMemberFeatureCall_in_rule__XPostfixOperation__Group__0__Impl55372);
ruleXMemberFeatureCall();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXPostfixOperationAccess().getXMemberFeatureCallParserRuleCall_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XPostfixOperation__Group__0__Impl"
// $ANTLR start "rule__XPostfixOperation__Group__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27402:1: rule__XPostfixOperation__Group__1 : rule__XPostfixOperation__Group__1__Impl ;
public final void rule__XPostfixOperation__Group__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27406:1: ( rule__XPostfixOperation__Group__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27407:2: rule__XPostfixOperation__Group__1__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__XPostfixOperation__Group__1__Impl_in_rule__XPostfixOperation__Group__155401);
rule__XPostfixOperation__Group__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XPostfixOperation__Group__1"
// $ANTLR start "rule__XPostfixOperation__Group__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27413:1: rule__XPostfixOperation__Group__1__Impl : ( ( rule__XPostfixOperation__Group_1__0 )? ) ;
public final void rule__XPostfixOperation__Group__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27417:1: ( ( ( rule__XPostfixOperation__Group_1__0 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27418:1: ( ( rule__XPostfixOperation__Group_1__0 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27418:1: ( ( rule__XPostfixOperation__Group_1__0 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27419:1: ( rule__XPostfixOperation__Group_1__0 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXPostfixOperationAccess().getGroup_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27420:1: ( rule__XPostfixOperation__Group_1__0 )?
int alt206=2;
int LA206_0 = input.LA(1);
if ( (LA206_0==41) ) {
int LA206_1 = input.LA(2);
if ( (synpred292_InternalMango()) ) {
alt206=1;
}
}
else if ( (LA206_0==42) ) {
int LA206_2 = input.LA(2);
if ( (synpred292_InternalMango()) ) {
alt206=1;
}
}
switch (alt206) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27420:2: rule__XPostfixOperation__Group_1__0
{
pushFollow(FollowSets002.FOLLOW_rule__XPostfixOperation__Group_1__0_in_rule__XPostfixOperation__Group__1__Impl55428);
rule__XPostfixOperation__Group_1__0();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXPostfixOperationAccess().getGroup_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XPostfixOperation__Group__1__Impl"
// $ANTLR start "rule__XPostfixOperation__Group_1__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27434:1: rule__XPostfixOperation__Group_1__0 : rule__XPostfixOperation__Group_1__0__Impl ;
public final void rule__XPostfixOperation__Group_1__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27438:1: ( rule__XPostfixOperation__Group_1__0__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27439:2: rule__XPostfixOperation__Group_1__0__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__XPostfixOperation__Group_1__0__Impl_in_rule__XPostfixOperation__Group_1__055463);
rule__XPostfixOperation__Group_1__0__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XPostfixOperation__Group_1__0"
// $ANTLR start "rule__XPostfixOperation__Group_1__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27445:1: rule__XPostfixOperation__Group_1__0__Impl : ( ( rule__XPostfixOperation__Group_1_0__0 ) ) ;
public final void rule__XPostfixOperation__Group_1__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27449:1: ( ( ( rule__XPostfixOperation__Group_1_0__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27450:1: ( ( rule__XPostfixOperation__Group_1_0__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27450:1: ( ( rule__XPostfixOperation__Group_1_0__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27451:1: ( rule__XPostfixOperation__Group_1_0__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXPostfixOperationAccess().getGroup_1_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27452:1: ( rule__XPostfixOperation__Group_1_0__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27452:2: rule__XPostfixOperation__Group_1_0__0
{
pushFollow(FollowSets002.FOLLOW_rule__XPostfixOperation__Group_1_0__0_in_rule__XPostfixOperation__Group_1__0__Impl55490);
rule__XPostfixOperation__Group_1_0__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXPostfixOperationAccess().getGroup_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XPostfixOperation__Group_1__0__Impl"
// $ANTLR start "rule__XPostfixOperation__Group_1_0__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27464:1: rule__XPostfixOperation__Group_1_0__0 : rule__XPostfixOperation__Group_1_0__0__Impl rule__XPostfixOperation__Group_1_0__1 ;
public final void rule__XPostfixOperation__Group_1_0__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27468:1: ( rule__XPostfixOperation__Group_1_0__0__Impl rule__XPostfixOperation__Group_1_0__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27469:2: rule__XPostfixOperation__Group_1_0__0__Impl rule__XPostfixOperation__Group_1_0__1
{
pushFollow(FollowSets002.FOLLOW_rule__XPostfixOperation__Group_1_0__0__Impl_in_rule__XPostfixOperation__Group_1_0__055522);
rule__XPostfixOperation__Group_1_0__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XPostfixOperation__Group_1_0__1_in_rule__XPostfixOperation__Group_1_0__055525);
rule__XPostfixOperation__Group_1_0__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XPostfixOperation__Group_1_0__0"
// $ANTLR start "rule__XPostfixOperation__Group_1_0__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27476:1: rule__XPostfixOperation__Group_1_0__0__Impl : ( () ) ;
public final void rule__XPostfixOperation__Group_1_0__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27480:1: ( ( () ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27481:1: ( () )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27481:1: ( () )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27482:1: ()
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXPostfixOperationAccess().getXPostfixOperationOperandAction_1_0_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27483:1: ()
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27485:1:
{
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXPostfixOperationAccess().getXPostfixOperationOperandAction_1_0_0());
}
}
}
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XPostfixOperation__Group_1_0__0__Impl"
// $ANTLR start "rule__XPostfixOperation__Group_1_0__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27495:1: rule__XPostfixOperation__Group_1_0__1 : rule__XPostfixOperation__Group_1_0__1__Impl ;
public final void rule__XPostfixOperation__Group_1_0__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27499:1: ( rule__XPostfixOperation__Group_1_0__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27500:2: rule__XPostfixOperation__Group_1_0__1__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__XPostfixOperation__Group_1_0__1__Impl_in_rule__XPostfixOperation__Group_1_0__155583);
rule__XPostfixOperation__Group_1_0__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XPostfixOperation__Group_1_0__1"
// $ANTLR start "rule__XPostfixOperation__Group_1_0__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27506:1: rule__XPostfixOperation__Group_1_0__1__Impl : ( ( rule__XPostfixOperation__FeatureAssignment_1_0_1 ) ) ;
public final void rule__XPostfixOperation__Group_1_0__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27510:1: ( ( ( rule__XPostfixOperation__FeatureAssignment_1_0_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27511:1: ( ( rule__XPostfixOperation__FeatureAssignment_1_0_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27511:1: ( ( rule__XPostfixOperation__FeatureAssignment_1_0_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27512:1: ( rule__XPostfixOperation__FeatureAssignment_1_0_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXPostfixOperationAccess().getFeatureAssignment_1_0_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27513:1: ( rule__XPostfixOperation__FeatureAssignment_1_0_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27513:2: rule__XPostfixOperation__FeatureAssignment_1_0_1
{
pushFollow(FollowSets002.FOLLOW_rule__XPostfixOperation__FeatureAssignment_1_0_1_in_rule__XPostfixOperation__Group_1_0__1__Impl55610);
rule__XPostfixOperation__FeatureAssignment_1_0_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXPostfixOperationAccess().getFeatureAssignment_1_0_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XPostfixOperation__Group_1_0__1__Impl"
// $ANTLR start "rule__XMemberFeatureCall__Group__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27527:1: rule__XMemberFeatureCall__Group__0 : rule__XMemberFeatureCall__Group__0__Impl rule__XMemberFeatureCall__Group__1 ;
public final void rule__XMemberFeatureCall__Group__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27531:1: ( rule__XMemberFeatureCall__Group__0__Impl rule__XMemberFeatureCall__Group__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27532:2: rule__XMemberFeatureCall__Group__0__Impl rule__XMemberFeatureCall__Group__1
{
pushFollow(FollowSets002.FOLLOW_rule__XMemberFeatureCall__Group__0__Impl_in_rule__XMemberFeatureCall__Group__055644);
rule__XMemberFeatureCall__Group__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XMemberFeatureCall__Group__1_in_rule__XMemberFeatureCall__Group__055647);
rule__XMemberFeatureCall__Group__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XMemberFeatureCall__Group__0"
// $ANTLR start "rule__XMemberFeatureCall__Group__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27539:1: rule__XMemberFeatureCall__Group__0__Impl : ( ruleXPrimaryExpression ) ;
public final void rule__XMemberFeatureCall__Group__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27543:1: ( ( ruleXPrimaryExpression ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27544:1: ( ruleXPrimaryExpression )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27544:1: ( ruleXPrimaryExpression )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27545:1: ruleXPrimaryExpression
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXMemberFeatureCallAccess().getXPrimaryExpressionParserRuleCall_0());
}
pushFollow(FollowSets002.FOLLOW_ruleXPrimaryExpression_in_rule__XMemberFeatureCall__Group__0__Impl55674);
ruleXPrimaryExpression();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXMemberFeatureCallAccess().getXPrimaryExpressionParserRuleCall_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XMemberFeatureCall__Group__0__Impl"
// $ANTLR start "rule__XMemberFeatureCall__Group__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27556:1: rule__XMemberFeatureCall__Group__1 : rule__XMemberFeatureCall__Group__1__Impl ;
public final void rule__XMemberFeatureCall__Group__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27560:1: ( rule__XMemberFeatureCall__Group__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27561:2: rule__XMemberFeatureCall__Group__1__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__XMemberFeatureCall__Group__1__Impl_in_rule__XMemberFeatureCall__Group__155703);
rule__XMemberFeatureCall__Group__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XMemberFeatureCall__Group__1"
// $ANTLR start "rule__XMemberFeatureCall__Group__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27567:1: rule__XMemberFeatureCall__Group__1__Impl : ( ( rule__XMemberFeatureCall__Alternatives_1 )* ) ;
public final void rule__XMemberFeatureCall__Group__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27571:1: ( ( ( rule__XMemberFeatureCall__Alternatives_1 )* ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27572:1: ( ( rule__XMemberFeatureCall__Alternatives_1 )* )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27572:1: ( ( rule__XMemberFeatureCall__Alternatives_1 )* )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27573:1: ( rule__XMemberFeatureCall__Alternatives_1 )*
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXMemberFeatureCallAccess().getAlternatives_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27574:1: ( rule__XMemberFeatureCall__Alternatives_1 )*
loop207:
do {
int alt207=2;
switch ( input.LA(1) ) {
case 43:
{
int LA207_2 = input.LA(2);
if ( (synpred293_InternalMango()) ) {
alt207=1;
}
}
break;
case 181:
{
int LA207_3 = input.LA(2);
if ( (synpred293_InternalMango()) ) {
alt207=1;
}
}
break;
case 182:
{
int LA207_4 = input.LA(2);
if ( (synpred293_InternalMango()) ) {
alt207=1;
}
}
break;
}
switch (alt207) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27574:2: rule__XMemberFeatureCall__Alternatives_1
{
pushFollow(FollowSets002.FOLLOW_rule__XMemberFeatureCall__Alternatives_1_in_rule__XMemberFeatureCall__Group__1__Impl55730);
rule__XMemberFeatureCall__Alternatives_1();
state._fsp--;
if (state.failed) return ;
}
break;
default :
break loop207;
}
} while (true);
if ( state.backtracking==0 ) {
after(grammarAccess.getXMemberFeatureCallAccess().getAlternatives_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XMemberFeatureCall__Group__1__Impl"
// $ANTLR start "rule__XMemberFeatureCall__Group_1_0__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27588:1: rule__XMemberFeatureCall__Group_1_0__0 : rule__XMemberFeatureCall__Group_1_0__0__Impl rule__XMemberFeatureCall__Group_1_0__1 ;
public final void rule__XMemberFeatureCall__Group_1_0__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27592:1: ( rule__XMemberFeatureCall__Group_1_0__0__Impl rule__XMemberFeatureCall__Group_1_0__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27593:2: rule__XMemberFeatureCall__Group_1_0__0__Impl rule__XMemberFeatureCall__Group_1_0__1
{
pushFollow(FollowSets002.FOLLOW_rule__XMemberFeatureCall__Group_1_0__0__Impl_in_rule__XMemberFeatureCall__Group_1_0__055765);
rule__XMemberFeatureCall__Group_1_0__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XMemberFeatureCall__Group_1_0__1_in_rule__XMemberFeatureCall__Group_1_0__055768);
rule__XMemberFeatureCall__Group_1_0__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XMemberFeatureCall__Group_1_0__0"
// $ANTLR start "rule__XMemberFeatureCall__Group_1_0__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27600:1: rule__XMemberFeatureCall__Group_1_0__0__Impl : ( ( rule__XMemberFeatureCall__Group_1_0_0__0 ) ) ;
public final void rule__XMemberFeatureCall__Group_1_0__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27604:1: ( ( ( rule__XMemberFeatureCall__Group_1_0_0__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27605:1: ( ( rule__XMemberFeatureCall__Group_1_0_0__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27605:1: ( ( rule__XMemberFeatureCall__Group_1_0_0__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27606:1: ( rule__XMemberFeatureCall__Group_1_0_0__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXMemberFeatureCallAccess().getGroup_1_0_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27607:1: ( rule__XMemberFeatureCall__Group_1_0_0__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27607:2: rule__XMemberFeatureCall__Group_1_0_0__0
{
pushFollow(FollowSets002.FOLLOW_rule__XMemberFeatureCall__Group_1_0_0__0_in_rule__XMemberFeatureCall__Group_1_0__0__Impl55795);
rule__XMemberFeatureCall__Group_1_0_0__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXMemberFeatureCallAccess().getGroup_1_0_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XMemberFeatureCall__Group_1_0__0__Impl"
// $ANTLR start "rule__XMemberFeatureCall__Group_1_0__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27617:1: rule__XMemberFeatureCall__Group_1_0__1 : rule__XMemberFeatureCall__Group_1_0__1__Impl ;
public final void rule__XMemberFeatureCall__Group_1_0__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27621:1: ( rule__XMemberFeatureCall__Group_1_0__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27622:2: rule__XMemberFeatureCall__Group_1_0__1__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__XMemberFeatureCall__Group_1_0__1__Impl_in_rule__XMemberFeatureCall__Group_1_0__155825);
rule__XMemberFeatureCall__Group_1_0__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XMemberFeatureCall__Group_1_0__1"
// $ANTLR start "rule__XMemberFeatureCall__Group_1_0__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27628:1: rule__XMemberFeatureCall__Group_1_0__1__Impl : ( ( rule__XMemberFeatureCall__ValueAssignment_1_0_1 ) ) ;
public final void rule__XMemberFeatureCall__Group_1_0__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27632:1: ( ( ( rule__XMemberFeatureCall__ValueAssignment_1_0_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27633:1: ( ( rule__XMemberFeatureCall__ValueAssignment_1_0_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27633:1: ( ( rule__XMemberFeatureCall__ValueAssignment_1_0_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27634:1: ( rule__XMemberFeatureCall__ValueAssignment_1_0_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXMemberFeatureCallAccess().getValueAssignment_1_0_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27635:1: ( rule__XMemberFeatureCall__ValueAssignment_1_0_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27635:2: rule__XMemberFeatureCall__ValueAssignment_1_0_1
{
pushFollow(FollowSets002.FOLLOW_rule__XMemberFeatureCall__ValueAssignment_1_0_1_in_rule__XMemberFeatureCall__Group_1_0__1__Impl55852);
rule__XMemberFeatureCall__ValueAssignment_1_0_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXMemberFeatureCallAccess().getValueAssignment_1_0_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XMemberFeatureCall__Group_1_0__1__Impl"
// $ANTLR start "rule__XMemberFeatureCall__Group_1_0_0__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27649:1: rule__XMemberFeatureCall__Group_1_0_0__0 : rule__XMemberFeatureCall__Group_1_0_0__0__Impl ;
public final void rule__XMemberFeatureCall__Group_1_0_0__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27653:1: ( rule__XMemberFeatureCall__Group_1_0_0__0__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27654:2: rule__XMemberFeatureCall__Group_1_0_0__0__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__XMemberFeatureCall__Group_1_0_0__0__Impl_in_rule__XMemberFeatureCall__Group_1_0_0__055886);
rule__XMemberFeatureCall__Group_1_0_0__0__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XMemberFeatureCall__Group_1_0_0__0"
// $ANTLR start "rule__XMemberFeatureCall__Group_1_0_0__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27660:1: rule__XMemberFeatureCall__Group_1_0_0__0__Impl : ( ( rule__XMemberFeatureCall__Group_1_0_0_0__0 ) ) ;
public final void rule__XMemberFeatureCall__Group_1_0_0__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27664:1: ( ( ( rule__XMemberFeatureCall__Group_1_0_0_0__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27665:1: ( ( rule__XMemberFeatureCall__Group_1_0_0_0__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27665:1: ( ( rule__XMemberFeatureCall__Group_1_0_0_0__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27666:1: ( rule__XMemberFeatureCall__Group_1_0_0_0__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXMemberFeatureCallAccess().getGroup_1_0_0_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27667:1: ( rule__XMemberFeatureCall__Group_1_0_0_0__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27667:2: rule__XMemberFeatureCall__Group_1_0_0_0__0
{
pushFollow(FollowSets002.FOLLOW_rule__XMemberFeatureCall__Group_1_0_0_0__0_in_rule__XMemberFeatureCall__Group_1_0_0__0__Impl55913);
rule__XMemberFeatureCall__Group_1_0_0_0__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXMemberFeatureCallAccess().getGroup_1_0_0_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XMemberFeatureCall__Group_1_0_0__0__Impl"
// $ANTLR start "rule__XMemberFeatureCall__Group_1_0_0_0__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27679:1: rule__XMemberFeatureCall__Group_1_0_0_0__0 : rule__XMemberFeatureCall__Group_1_0_0_0__0__Impl rule__XMemberFeatureCall__Group_1_0_0_0__1 ;
public final void rule__XMemberFeatureCall__Group_1_0_0_0__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27683:1: ( rule__XMemberFeatureCall__Group_1_0_0_0__0__Impl rule__XMemberFeatureCall__Group_1_0_0_0__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27684:2: rule__XMemberFeatureCall__Group_1_0_0_0__0__Impl rule__XMemberFeatureCall__Group_1_0_0_0__1
{
pushFollow(FollowSets002.FOLLOW_rule__XMemberFeatureCall__Group_1_0_0_0__0__Impl_in_rule__XMemberFeatureCall__Group_1_0_0_0__055945);
rule__XMemberFeatureCall__Group_1_0_0_0__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XMemberFeatureCall__Group_1_0_0_0__1_in_rule__XMemberFeatureCall__Group_1_0_0_0__055948);
rule__XMemberFeatureCall__Group_1_0_0_0__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XMemberFeatureCall__Group_1_0_0_0__0"
// $ANTLR start "rule__XMemberFeatureCall__Group_1_0_0_0__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27691:1: rule__XMemberFeatureCall__Group_1_0_0_0__0__Impl : ( () ) ;
public final void rule__XMemberFeatureCall__Group_1_0_0_0__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27695:1: ( ( () ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27696:1: ( () )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27696:1: ( () )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27697:1: ()
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXMemberFeatureCallAccess().getXAssignmentAssignableAction_1_0_0_0_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27698:1: ()
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27700:1:
{
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXMemberFeatureCallAccess().getXAssignmentAssignableAction_1_0_0_0_0());
}
}
}
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XMemberFeatureCall__Group_1_0_0_0__0__Impl"
// $ANTLR start "rule__XMemberFeatureCall__Group_1_0_0_0__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27710:1: rule__XMemberFeatureCall__Group_1_0_0_0__1 : rule__XMemberFeatureCall__Group_1_0_0_0__1__Impl rule__XMemberFeatureCall__Group_1_0_0_0__2 ;
public final void rule__XMemberFeatureCall__Group_1_0_0_0__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27714:1: ( rule__XMemberFeatureCall__Group_1_0_0_0__1__Impl rule__XMemberFeatureCall__Group_1_0_0_0__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27715:2: rule__XMemberFeatureCall__Group_1_0_0_0__1__Impl rule__XMemberFeatureCall__Group_1_0_0_0__2
{
pushFollow(FollowSets002.FOLLOW_rule__XMemberFeatureCall__Group_1_0_0_0__1__Impl_in_rule__XMemberFeatureCall__Group_1_0_0_0__156006);
rule__XMemberFeatureCall__Group_1_0_0_0__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XMemberFeatureCall__Group_1_0_0_0__2_in_rule__XMemberFeatureCall__Group_1_0_0_0__156009);
rule__XMemberFeatureCall__Group_1_0_0_0__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XMemberFeatureCall__Group_1_0_0_0__1"
// $ANTLR start "rule__XMemberFeatureCall__Group_1_0_0_0__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27722:1: rule__XMemberFeatureCall__Group_1_0_0_0__1__Impl : ( ( rule__XMemberFeatureCall__Alternatives_1_0_0_0_1 ) ) ;
public final void rule__XMemberFeatureCall__Group_1_0_0_0__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27726:1: ( ( ( rule__XMemberFeatureCall__Alternatives_1_0_0_0_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27727:1: ( ( rule__XMemberFeatureCall__Alternatives_1_0_0_0_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27727:1: ( ( rule__XMemberFeatureCall__Alternatives_1_0_0_0_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27728:1: ( rule__XMemberFeatureCall__Alternatives_1_0_0_0_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXMemberFeatureCallAccess().getAlternatives_1_0_0_0_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27729:1: ( rule__XMemberFeatureCall__Alternatives_1_0_0_0_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27729:2: rule__XMemberFeatureCall__Alternatives_1_0_0_0_1
{
pushFollow(FollowSets002.FOLLOW_rule__XMemberFeatureCall__Alternatives_1_0_0_0_1_in_rule__XMemberFeatureCall__Group_1_0_0_0__1__Impl56036);
rule__XMemberFeatureCall__Alternatives_1_0_0_0_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXMemberFeatureCallAccess().getAlternatives_1_0_0_0_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XMemberFeatureCall__Group_1_0_0_0__1__Impl"
// $ANTLR start "rule__XMemberFeatureCall__Group_1_0_0_0__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27739:1: rule__XMemberFeatureCall__Group_1_0_0_0__2 : rule__XMemberFeatureCall__Group_1_0_0_0__2__Impl rule__XMemberFeatureCall__Group_1_0_0_0__3 ;
public final void rule__XMemberFeatureCall__Group_1_0_0_0__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27743:1: ( rule__XMemberFeatureCall__Group_1_0_0_0__2__Impl rule__XMemberFeatureCall__Group_1_0_0_0__3 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27744:2: rule__XMemberFeatureCall__Group_1_0_0_0__2__Impl rule__XMemberFeatureCall__Group_1_0_0_0__3
{
pushFollow(FollowSets002.FOLLOW_rule__XMemberFeatureCall__Group_1_0_0_0__2__Impl_in_rule__XMemberFeatureCall__Group_1_0_0_0__256066);
rule__XMemberFeatureCall__Group_1_0_0_0__2__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XMemberFeatureCall__Group_1_0_0_0__3_in_rule__XMemberFeatureCall__Group_1_0_0_0__256069);
rule__XMemberFeatureCall__Group_1_0_0_0__3();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XMemberFeatureCall__Group_1_0_0_0__2"
// $ANTLR start "rule__XMemberFeatureCall__Group_1_0_0_0__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27751:1: rule__XMemberFeatureCall__Group_1_0_0_0__2__Impl : ( ( rule__XMemberFeatureCall__FeatureAssignment_1_0_0_0_2 ) ) ;
public final void rule__XMemberFeatureCall__Group_1_0_0_0__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27755:1: ( ( ( rule__XMemberFeatureCall__FeatureAssignment_1_0_0_0_2 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27756:1: ( ( rule__XMemberFeatureCall__FeatureAssignment_1_0_0_0_2 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27756:1: ( ( rule__XMemberFeatureCall__FeatureAssignment_1_0_0_0_2 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27757:1: ( rule__XMemberFeatureCall__FeatureAssignment_1_0_0_0_2 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXMemberFeatureCallAccess().getFeatureAssignment_1_0_0_0_2());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27758:1: ( rule__XMemberFeatureCall__FeatureAssignment_1_0_0_0_2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27758:2: rule__XMemberFeatureCall__FeatureAssignment_1_0_0_0_2
{
pushFollow(FollowSets002.FOLLOW_rule__XMemberFeatureCall__FeatureAssignment_1_0_0_0_2_in_rule__XMemberFeatureCall__Group_1_0_0_0__2__Impl56096);
rule__XMemberFeatureCall__FeatureAssignment_1_0_0_0_2();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXMemberFeatureCallAccess().getFeatureAssignment_1_0_0_0_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XMemberFeatureCall__Group_1_0_0_0__2__Impl"
// $ANTLR start "rule__XMemberFeatureCall__Group_1_0_0_0__3"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27768:1: rule__XMemberFeatureCall__Group_1_0_0_0__3 : rule__XMemberFeatureCall__Group_1_0_0_0__3__Impl ;
public final void rule__XMemberFeatureCall__Group_1_0_0_0__3() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27772:1: ( rule__XMemberFeatureCall__Group_1_0_0_0__3__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27773:2: rule__XMemberFeatureCall__Group_1_0_0_0__3__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__XMemberFeatureCall__Group_1_0_0_0__3__Impl_in_rule__XMemberFeatureCall__Group_1_0_0_0__356126);
rule__XMemberFeatureCall__Group_1_0_0_0__3__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XMemberFeatureCall__Group_1_0_0_0__3"
// $ANTLR start "rule__XMemberFeatureCall__Group_1_0_0_0__3__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27779:1: rule__XMemberFeatureCall__Group_1_0_0_0__3__Impl : ( ruleOpSingleAssign ) ;
public final void rule__XMemberFeatureCall__Group_1_0_0_0__3__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27783:1: ( ( ruleOpSingleAssign ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27784:1: ( ruleOpSingleAssign )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27784:1: ( ruleOpSingleAssign )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27785:1: ruleOpSingleAssign
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXMemberFeatureCallAccess().getOpSingleAssignParserRuleCall_1_0_0_0_3());
}
pushFollow(FollowSets002.FOLLOW_ruleOpSingleAssign_in_rule__XMemberFeatureCall__Group_1_0_0_0__3__Impl56153);
ruleOpSingleAssign();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXMemberFeatureCallAccess().getOpSingleAssignParserRuleCall_1_0_0_0_3());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XMemberFeatureCall__Group_1_0_0_0__3__Impl"
// $ANTLR start "rule__XMemberFeatureCall__Group_1_1__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27804:1: rule__XMemberFeatureCall__Group_1_1__0 : rule__XMemberFeatureCall__Group_1_1__0__Impl rule__XMemberFeatureCall__Group_1_1__1 ;
public final void rule__XMemberFeatureCall__Group_1_1__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27808:1: ( rule__XMemberFeatureCall__Group_1_1__0__Impl rule__XMemberFeatureCall__Group_1_1__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27809:2: rule__XMemberFeatureCall__Group_1_1__0__Impl rule__XMemberFeatureCall__Group_1_1__1
{
pushFollow(FollowSets002.FOLLOW_rule__XMemberFeatureCall__Group_1_1__0__Impl_in_rule__XMemberFeatureCall__Group_1_1__056190);
rule__XMemberFeatureCall__Group_1_1__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XMemberFeatureCall__Group_1_1__1_in_rule__XMemberFeatureCall__Group_1_1__056193);
rule__XMemberFeatureCall__Group_1_1__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XMemberFeatureCall__Group_1_1__0"
// $ANTLR start "rule__XMemberFeatureCall__Group_1_1__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27816:1: rule__XMemberFeatureCall__Group_1_1__0__Impl : ( ( rule__XMemberFeatureCall__Group_1_1_0__0 ) ) ;
public final void rule__XMemberFeatureCall__Group_1_1__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27820:1: ( ( ( rule__XMemberFeatureCall__Group_1_1_0__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27821:1: ( ( rule__XMemberFeatureCall__Group_1_1_0__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27821:1: ( ( rule__XMemberFeatureCall__Group_1_1_0__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27822:1: ( rule__XMemberFeatureCall__Group_1_1_0__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXMemberFeatureCallAccess().getGroup_1_1_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27823:1: ( rule__XMemberFeatureCall__Group_1_1_0__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27823:2: rule__XMemberFeatureCall__Group_1_1_0__0
{
pushFollow(FollowSets002.FOLLOW_rule__XMemberFeatureCall__Group_1_1_0__0_in_rule__XMemberFeatureCall__Group_1_1__0__Impl56220);
rule__XMemberFeatureCall__Group_1_1_0__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXMemberFeatureCallAccess().getGroup_1_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XMemberFeatureCall__Group_1_1__0__Impl"
// $ANTLR start "rule__XMemberFeatureCall__Group_1_1__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27833:1: rule__XMemberFeatureCall__Group_1_1__1 : rule__XMemberFeatureCall__Group_1_1__1__Impl rule__XMemberFeatureCall__Group_1_1__2 ;
public final void rule__XMemberFeatureCall__Group_1_1__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27837:1: ( rule__XMemberFeatureCall__Group_1_1__1__Impl rule__XMemberFeatureCall__Group_1_1__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27838:2: rule__XMemberFeatureCall__Group_1_1__1__Impl rule__XMemberFeatureCall__Group_1_1__2
{
pushFollow(FollowSets002.FOLLOW_rule__XMemberFeatureCall__Group_1_1__1__Impl_in_rule__XMemberFeatureCall__Group_1_1__156250);
rule__XMemberFeatureCall__Group_1_1__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XMemberFeatureCall__Group_1_1__2_in_rule__XMemberFeatureCall__Group_1_1__156253);
rule__XMemberFeatureCall__Group_1_1__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XMemberFeatureCall__Group_1_1__1"
// $ANTLR start "rule__XMemberFeatureCall__Group_1_1__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27845:1: rule__XMemberFeatureCall__Group_1_1__1__Impl : ( ( rule__XMemberFeatureCall__Group_1_1_1__0 )? ) ;
public final void rule__XMemberFeatureCall__Group_1_1__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27849:1: ( ( ( rule__XMemberFeatureCall__Group_1_1_1__0 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27850:1: ( ( rule__XMemberFeatureCall__Group_1_1_1__0 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27850:1: ( ( rule__XMemberFeatureCall__Group_1_1_1__0 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27851:1: ( rule__XMemberFeatureCall__Group_1_1_1__0 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXMemberFeatureCallAccess().getGroup_1_1_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27852:1: ( rule__XMemberFeatureCall__Group_1_1_1__0 )?
int alt208=2;
int LA208_0 = input.LA(1);
if ( (LA208_0==27) ) {
alt208=1;
}
switch (alt208) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27852:2: rule__XMemberFeatureCall__Group_1_1_1__0
{
pushFollow(FollowSets002.FOLLOW_rule__XMemberFeatureCall__Group_1_1_1__0_in_rule__XMemberFeatureCall__Group_1_1__1__Impl56280);
rule__XMemberFeatureCall__Group_1_1_1__0();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXMemberFeatureCallAccess().getGroup_1_1_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XMemberFeatureCall__Group_1_1__1__Impl"
// $ANTLR start "rule__XMemberFeatureCall__Group_1_1__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27862:1: rule__XMemberFeatureCall__Group_1_1__2 : rule__XMemberFeatureCall__Group_1_1__2__Impl rule__XMemberFeatureCall__Group_1_1__3 ;
public final void rule__XMemberFeatureCall__Group_1_1__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27866:1: ( rule__XMemberFeatureCall__Group_1_1__2__Impl rule__XMemberFeatureCall__Group_1_1__3 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27867:2: rule__XMemberFeatureCall__Group_1_1__2__Impl rule__XMemberFeatureCall__Group_1_1__3
{
pushFollow(FollowSets002.FOLLOW_rule__XMemberFeatureCall__Group_1_1__2__Impl_in_rule__XMemberFeatureCall__Group_1_1__256311);
rule__XMemberFeatureCall__Group_1_1__2__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XMemberFeatureCall__Group_1_1__3_in_rule__XMemberFeatureCall__Group_1_1__256314);
rule__XMemberFeatureCall__Group_1_1__3();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XMemberFeatureCall__Group_1_1__2"
// $ANTLR start "rule__XMemberFeatureCall__Group_1_1__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27874:1: rule__XMemberFeatureCall__Group_1_1__2__Impl : ( ( rule__XMemberFeatureCall__FeatureAssignment_1_1_2 ) ) ;
public final void rule__XMemberFeatureCall__Group_1_1__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27878:1: ( ( ( rule__XMemberFeatureCall__FeatureAssignment_1_1_2 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27879:1: ( ( rule__XMemberFeatureCall__FeatureAssignment_1_1_2 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27879:1: ( ( rule__XMemberFeatureCall__FeatureAssignment_1_1_2 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27880:1: ( rule__XMemberFeatureCall__FeatureAssignment_1_1_2 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXMemberFeatureCallAccess().getFeatureAssignment_1_1_2());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27881:1: ( rule__XMemberFeatureCall__FeatureAssignment_1_1_2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27881:2: rule__XMemberFeatureCall__FeatureAssignment_1_1_2
{
pushFollow(FollowSets002.FOLLOW_rule__XMemberFeatureCall__FeatureAssignment_1_1_2_in_rule__XMemberFeatureCall__Group_1_1__2__Impl56341);
rule__XMemberFeatureCall__FeatureAssignment_1_1_2();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXMemberFeatureCallAccess().getFeatureAssignment_1_1_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XMemberFeatureCall__Group_1_1__2__Impl"
// $ANTLR start "rule__XMemberFeatureCall__Group_1_1__3"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27891:1: rule__XMemberFeatureCall__Group_1_1__3 : rule__XMemberFeatureCall__Group_1_1__3__Impl rule__XMemberFeatureCall__Group_1_1__4 ;
public final void rule__XMemberFeatureCall__Group_1_1__3() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27895:1: ( rule__XMemberFeatureCall__Group_1_1__3__Impl rule__XMemberFeatureCall__Group_1_1__4 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27896:2: rule__XMemberFeatureCall__Group_1_1__3__Impl rule__XMemberFeatureCall__Group_1_1__4
{
pushFollow(FollowSets002.FOLLOW_rule__XMemberFeatureCall__Group_1_1__3__Impl_in_rule__XMemberFeatureCall__Group_1_1__356371);
rule__XMemberFeatureCall__Group_1_1__3__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XMemberFeatureCall__Group_1_1__4_in_rule__XMemberFeatureCall__Group_1_1__356374);
rule__XMemberFeatureCall__Group_1_1__4();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XMemberFeatureCall__Group_1_1__3"
// $ANTLR start "rule__XMemberFeatureCall__Group_1_1__3__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27903:1: rule__XMemberFeatureCall__Group_1_1__3__Impl : ( ( rule__XMemberFeatureCall__Group_1_1_3__0 )? ) ;
public final void rule__XMemberFeatureCall__Group_1_1__3__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27907:1: ( ( ( rule__XMemberFeatureCall__Group_1_1_3__0 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27908:1: ( ( rule__XMemberFeatureCall__Group_1_1_3__0 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27908:1: ( ( rule__XMemberFeatureCall__Group_1_1_3__0 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27909:1: ( rule__XMemberFeatureCall__Group_1_1_3__0 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXMemberFeatureCallAccess().getGroup_1_1_3());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27910:1: ( rule__XMemberFeatureCall__Group_1_1_3__0 )?
int alt209=2;
alt209 = dfa209.predict(input);
switch (alt209) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27910:2: rule__XMemberFeatureCall__Group_1_1_3__0
{
pushFollow(FollowSets002.FOLLOW_rule__XMemberFeatureCall__Group_1_1_3__0_in_rule__XMemberFeatureCall__Group_1_1__3__Impl56401);
rule__XMemberFeatureCall__Group_1_1_3__0();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXMemberFeatureCallAccess().getGroup_1_1_3());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XMemberFeatureCall__Group_1_1__3__Impl"
// $ANTLR start "rule__XMemberFeatureCall__Group_1_1__4"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27920:1: rule__XMemberFeatureCall__Group_1_1__4 : rule__XMemberFeatureCall__Group_1_1__4__Impl ;
public final void rule__XMemberFeatureCall__Group_1_1__4() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27924:1: ( rule__XMemberFeatureCall__Group_1_1__4__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27925:2: rule__XMemberFeatureCall__Group_1_1__4__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__XMemberFeatureCall__Group_1_1__4__Impl_in_rule__XMemberFeatureCall__Group_1_1__456432);
rule__XMemberFeatureCall__Group_1_1__4__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XMemberFeatureCall__Group_1_1__4"
// $ANTLR start "rule__XMemberFeatureCall__Group_1_1__4__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27931:1: rule__XMemberFeatureCall__Group_1_1__4__Impl : ( ( rule__XMemberFeatureCall__MemberCallArgumentsAssignment_1_1_4 )? ) ;
public final void rule__XMemberFeatureCall__Group_1_1__4__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27935:1: ( ( ( rule__XMemberFeatureCall__MemberCallArgumentsAssignment_1_1_4 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27936:1: ( ( rule__XMemberFeatureCall__MemberCallArgumentsAssignment_1_1_4 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27936:1: ( ( rule__XMemberFeatureCall__MemberCallArgumentsAssignment_1_1_4 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27937:1: ( rule__XMemberFeatureCall__MemberCallArgumentsAssignment_1_1_4 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXMemberFeatureCallAccess().getMemberCallArgumentsAssignment_1_1_4());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27938:1: ( rule__XMemberFeatureCall__MemberCallArgumentsAssignment_1_1_4 )?
int alt210=2;
alt210 = dfa210.predict(input);
switch (alt210) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27938:2: rule__XMemberFeatureCall__MemberCallArgumentsAssignment_1_1_4
{
pushFollow(FollowSets002.FOLLOW_rule__XMemberFeatureCall__MemberCallArgumentsAssignment_1_1_4_in_rule__XMemberFeatureCall__Group_1_1__4__Impl56459);
rule__XMemberFeatureCall__MemberCallArgumentsAssignment_1_1_4();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXMemberFeatureCallAccess().getMemberCallArgumentsAssignment_1_1_4());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XMemberFeatureCall__Group_1_1__4__Impl"
// $ANTLR start "rule__XMemberFeatureCall__Group_1_1_0__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27958:1: rule__XMemberFeatureCall__Group_1_1_0__0 : rule__XMemberFeatureCall__Group_1_1_0__0__Impl ;
public final void rule__XMemberFeatureCall__Group_1_1_0__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27962:1: ( rule__XMemberFeatureCall__Group_1_1_0__0__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27963:2: rule__XMemberFeatureCall__Group_1_1_0__0__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__XMemberFeatureCall__Group_1_1_0__0__Impl_in_rule__XMemberFeatureCall__Group_1_1_0__056500);
rule__XMemberFeatureCall__Group_1_1_0__0__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XMemberFeatureCall__Group_1_1_0__0"
// $ANTLR start "rule__XMemberFeatureCall__Group_1_1_0__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27969:1: rule__XMemberFeatureCall__Group_1_1_0__0__Impl : ( ( rule__XMemberFeatureCall__Group_1_1_0_0__0 ) ) ;
public final void rule__XMemberFeatureCall__Group_1_1_0__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27973:1: ( ( ( rule__XMemberFeatureCall__Group_1_1_0_0__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27974:1: ( ( rule__XMemberFeatureCall__Group_1_1_0_0__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27974:1: ( ( rule__XMemberFeatureCall__Group_1_1_0_0__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27975:1: ( rule__XMemberFeatureCall__Group_1_1_0_0__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXMemberFeatureCallAccess().getGroup_1_1_0_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27976:1: ( rule__XMemberFeatureCall__Group_1_1_0_0__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27976:2: rule__XMemberFeatureCall__Group_1_1_0_0__0
{
pushFollow(FollowSets002.FOLLOW_rule__XMemberFeatureCall__Group_1_1_0_0__0_in_rule__XMemberFeatureCall__Group_1_1_0__0__Impl56527);
rule__XMemberFeatureCall__Group_1_1_0_0__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXMemberFeatureCallAccess().getGroup_1_1_0_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XMemberFeatureCall__Group_1_1_0__0__Impl"
// $ANTLR start "rule__XMemberFeatureCall__Group_1_1_0_0__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27988:1: rule__XMemberFeatureCall__Group_1_1_0_0__0 : rule__XMemberFeatureCall__Group_1_1_0_0__0__Impl rule__XMemberFeatureCall__Group_1_1_0_0__1 ;
public final void rule__XMemberFeatureCall__Group_1_1_0_0__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27992:1: ( rule__XMemberFeatureCall__Group_1_1_0_0__0__Impl rule__XMemberFeatureCall__Group_1_1_0_0__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27993:2: rule__XMemberFeatureCall__Group_1_1_0_0__0__Impl rule__XMemberFeatureCall__Group_1_1_0_0__1
{
pushFollow(FollowSets002.FOLLOW_rule__XMemberFeatureCall__Group_1_1_0_0__0__Impl_in_rule__XMemberFeatureCall__Group_1_1_0_0__056559);
rule__XMemberFeatureCall__Group_1_1_0_0__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XMemberFeatureCall__Group_1_1_0_0__1_in_rule__XMemberFeatureCall__Group_1_1_0_0__056562);
rule__XMemberFeatureCall__Group_1_1_0_0__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XMemberFeatureCall__Group_1_1_0_0__0"
// $ANTLR start "rule__XMemberFeatureCall__Group_1_1_0_0__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28000:1: rule__XMemberFeatureCall__Group_1_1_0_0__0__Impl : ( () ) ;
public final void rule__XMemberFeatureCall__Group_1_1_0_0__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28004:1: ( ( () ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28005:1: ( () )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28005:1: ( () )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28006:1: ()
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXMemberFeatureCallAccess().getXMemberFeatureCallMemberCallTargetAction_1_1_0_0_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28007:1: ()
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28009:1:
{
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXMemberFeatureCallAccess().getXMemberFeatureCallMemberCallTargetAction_1_1_0_0_0());
}
}
}
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XMemberFeatureCall__Group_1_1_0_0__0__Impl"
// $ANTLR start "rule__XMemberFeatureCall__Group_1_1_0_0__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28019:1: rule__XMemberFeatureCall__Group_1_1_0_0__1 : rule__XMemberFeatureCall__Group_1_1_0_0__1__Impl ;
public final void rule__XMemberFeatureCall__Group_1_1_0_0__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28023:1: ( rule__XMemberFeatureCall__Group_1_1_0_0__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28024:2: rule__XMemberFeatureCall__Group_1_1_0_0__1__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__XMemberFeatureCall__Group_1_1_0_0__1__Impl_in_rule__XMemberFeatureCall__Group_1_1_0_0__156620);
rule__XMemberFeatureCall__Group_1_1_0_0__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XMemberFeatureCall__Group_1_1_0_0__1"
// $ANTLR start "rule__XMemberFeatureCall__Group_1_1_0_0__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28030:1: rule__XMemberFeatureCall__Group_1_1_0_0__1__Impl : ( ( rule__XMemberFeatureCall__Alternatives_1_1_0_0_1 ) ) ;
public final void rule__XMemberFeatureCall__Group_1_1_0_0__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28034:1: ( ( ( rule__XMemberFeatureCall__Alternatives_1_1_0_0_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28035:1: ( ( rule__XMemberFeatureCall__Alternatives_1_1_0_0_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28035:1: ( ( rule__XMemberFeatureCall__Alternatives_1_1_0_0_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28036:1: ( rule__XMemberFeatureCall__Alternatives_1_1_0_0_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXMemberFeatureCallAccess().getAlternatives_1_1_0_0_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28037:1: ( rule__XMemberFeatureCall__Alternatives_1_1_0_0_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28037:2: rule__XMemberFeatureCall__Alternatives_1_1_0_0_1
{
pushFollow(FollowSets002.FOLLOW_rule__XMemberFeatureCall__Alternatives_1_1_0_0_1_in_rule__XMemberFeatureCall__Group_1_1_0_0__1__Impl56647);
rule__XMemberFeatureCall__Alternatives_1_1_0_0_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXMemberFeatureCallAccess().getAlternatives_1_1_0_0_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XMemberFeatureCall__Group_1_1_0_0__1__Impl"
// $ANTLR start "rule__XMemberFeatureCall__Group_1_1_1__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28051:1: rule__XMemberFeatureCall__Group_1_1_1__0 : rule__XMemberFeatureCall__Group_1_1_1__0__Impl rule__XMemberFeatureCall__Group_1_1_1__1 ;
public final void rule__XMemberFeatureCall__Group_1_1_1__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28055:1: ( rule__XMemberFeatureCall__Group_1_1_1__0__Impl rule__XMemberFeatureCall__Group_1_1_1__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28056:2: rule__XMemberFeatureCall__Group_1_1_1__0__Impl rule__XMemberFeatureCall__Group_1_1_1__1
{
pushFollow(FollowSets002.FOLLOW_rule__XMemberFeatureCall__Group_1_1_1__0__Impl_in_rule__XMemberFeatureCall__Group_1_1_1__056681);
rule__XMemberFeatureCall__Group_1_1_1__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XMemberFeatureCall__Group_1_1_1__1_in_rule__XMemberFeatureCall__Group_1_1_1__056684);
rule__XMemberFeatureCall__Group_1_1_1__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XMemberFeatureCall__Group_1_1_1__0"
// $ANTLR start "rule__XMemberFeatureCall__Group_1_1_1__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28063:1: rule__XMemberFeatureCall__Group_1_1_1__0__Impl : ( '<' ) ;
public final void rule__XMemberFeatureCall__Group_1_1_1__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28067:1: ( ( '<' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28068:1: ( '<' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28068:1: ( '<' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28069:1: '<'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXMemberFeatureCallAccess().getLessThanSignKeyword_1_1_1_0());
}
match(input,27,FollowSets002.FOLLOW_27_in_rule__XMemberFeatureCall__Group_1_1_1__0__Impl56712); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXMemberFeatureCallAccess().getLessThanSignKeyword_1_1_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XMemberFeatureCall__Group_1_1_1__0__Impl"
// $ANTLR start "rule__XMemberFeatureCall__Group_1_1_1__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28082:1: rule__XMemberFeatureCall__Group_1_1_1__1 : rule__XMemberFeatureCall__Group_1_1_1__1__Impl rule__XMemberFeatureCall__Group_1_1_1__2 ;
public final void rule__XMemberFeatureCall__Group_1_1_1__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28086:1: ( rule__XMemberFeatureCall__Group_1_1_1__1__Impl rule__XMemberFeatureCall__Group_1_1_1__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28087:2: rule__XMemberFeatureCall__Group_1_1_1__1__Impl rule__XMemberFeatureCall__Group_1_1_1__2
{
pushFollow(FollowSets002.FOLLOW_rule__XMemberFeatureCall__Group_1_1_1__1__Impl_in_rule__XMemberFeatureCall__Group_1_1_1__156743);
rule__XMemberFeatureCall__Group_1_1_1__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XMemberFeatureCall__Group_1_1_1__2_in_rule__XMemberFeatureCall__Group_1_1_1__156746);
rule__XMemberFeatureCall__Group_1_1_1__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XMemberFeatureCall__Group_1_1_1__1"
// $ANTLR start "rule__XMemberFeatureCall__Group_1_1_1__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28094:1: rule__XMemberFeatureCall__Group_1_1_1__1__Impl : ( ( rule__XMemberFeatureCall__TypeArgumentsAssignment_1_1_1_1 ) ) ;
public final void rule__XMemberFeatureCall__Group_1_1_1__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28098:1: ( ( ( rule__XMemberFeatureCall__TypeArgumentsAssignment_1_1_1_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28099:1: ( ( rule__XMemberFeatureCall__TypeArgumentsAssignment_1_1_1_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28099:1: ( ( rule__XMemberFeatureCall__TypeArgumentsAssignment_1_1_1_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28100:1: ( rule__XMemberFeatureCall__TypeArgumentsAssignment_1_1_1_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXMemberFeatureCallAccess().getTypeArgumentsAssignment_1_1_1_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28101:1: ( rule__XMemberFeatureCall__TypeArgumentsAssignment_1_1_1_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28101:2: rule__XMemberFeatureCall__TypeArgumentsAssignment_1_1_1_1
{
pushFollow(FollowSets002.FOLLOW_rule__XMemberFeatureCall__TypeArgumentsAssignment_1_1_1_1_in_rule__XMemberFeatureCall__Group_1_1_1__1__Impl56773);
rule__XMemberFeatureCall__TypeArgumentsAssignment_1_1_1_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXMemberFeatureCallAccess().getTypeArgumentsAssignment_1_1_1_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XMemberFeatureCall__Group_1_1_1__1__Impl"
// $ANTLR start "rule__XMemberFeatureCall__Group_1_1_1__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28111:1: rule__XMemberFeatureCall__Group_1_1_1__2 : rule__XMemberFeatureCall__Group_1_1_1__2__Impl rule__XMemberFeatureCall__Group_1_1_1__3 ;
public final void rule__XMemberFeatureCall__Group_1_1_1__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28115:1: ( rule__XMemberFeatureCall__Group_1_1_1__2__Impl rule__XMemberFeatureCall__Group_1_1_1__3 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28116:2: rule__XMemberFeatureCall__Group_1_1_1__2__Impl rule__XMemberFeatureCall__Group_1_1_1__3
{
pushFollow(FollowSets002.FOLLOW_rule__XMemberFeatureCall__Group_1_1_1__2__Impl_in_rule__XMemberFeatureCall__Group_1_1_1__256803);
rule__XMemberFeatureCall__Group_1_1_1__2__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XMemberFeatureCall__Group_1_1_1__3_in_rule__XMemberFeatureCall__Group_1_1_1__256806);
rule__XMemberFeatureCall__Group_1_1_1__3();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XMemberFeatureCall__Group_1_1_1__2"
// $ANTLR start "rule__XMemberFeatureCall__Group_1_1_1__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28123:1: rule__XMemberFeatureCall__Group_1_1_1__2__Impl : ( ( rule__XMemberFeatureCall__Group_1_1_1_2__0 )* ) ;
public final void rule__XMemberFeatureCall__Group_1_1_1__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28127:1: ( ( ( rule__XMemberFeatureCall__Group_1_1_1_2__0 )* ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28128:1: ( ( rule__XMemberFeatureCall__Group_1_1_1_2__0 )* )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28128:1: ( ( rule__XMemberFeatureCall__Group_1_1_1_2__0 )* )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28129:1: ( rule__XMemberFeatureCall__Group_1_1_1_2__0 )*
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXMemberFeatureCallAccess().getGroup_1_1_1_2());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28130:1: ( rule__XMemberFeatureCall__Group_1_1_1_2__0 )*
loop211:
do {
int alt211=2;
int LA211_0 = input.LA(1);
if ( (LA211_0==154) ) {
alt211=1;
}
switch (alt211) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28130:2: rule__XMemberFeatureCall__Group_1_1_1_2__0
{
pushFollow(FollowSets002.FOLLOW_rule__XMemberFeatureCall__Group_1_1_1_2__0_in_rule__XMemberFeatureCall__Group_1_1_1__2__Impl56833);
rule__XMemberFeatureCall__Group_1_1_1_2__0();
state._fsp--;
if (state.failed) return ;
}
break;
default :
break loop211;
}
} while (true);
if ( state.backtracking==0 ) {
after(grammarAccess.getXMemberFeatureCallAccess().getGroup_1_1_1_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XMemberFeatureCall__Group_1_1_1__2__Impl"
// $ANTLR start "rule__XMemberFeatureCall__Group_1_1_1__3"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28140:1: rule__XMemberFeatureCall__Group_1_1_1__3 : rule__XMemberFeatureCall__Group_1_1_1__3__Impl ;
public final void rule__XMemberFeatureCall__Group_1_1_1__3() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28144:1: ( rule__XMemberFeatureCall__Group_1_1_1__3__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28145:2: rule__XMemberFeatureCall__Group_1_1_1__3__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__XMemberFeatureCall__Group_1_1_1__3__Impl_in_rule__XMemberFeatureCall__Group_1_1_1__356864);
rule__XMemberFeatureCall__Group_1_1_1__3__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XMemberFeatureCall__Group_1_1_1__3"
// $ANTLR start "rule__XMemberFeatureCall__Group_1_1_1__3__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28151:1: rule__XMemberFeatureCall__Group_1_1_1__3__Impl : ( '>' ) ;
public final void rule__XMemberFeatureCall__Group_1_1_1__3__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28155:1: ( ( '>' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28156:1: ( '>' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28156:1: ( '>' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28157:1: '>'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXMemberFeatureCallAccess().getGreaterThanSignKeyword_1_1_1_3());
}
match(input,26,FollowSets002.FOLLOW_26_in_rule__XMemberFeatureCall__Group_1_1_1__3__Impl56892); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXMemberFeatureCallAccess().getGreaterThanSignKeyword_1_1_1_3());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XMemberFeatureCall__Group_1_1_1__3__Impl"
// $ANTLR start "rule__XMemberFeatureCall__Group_1_1_1_2__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28178:1: rule__XMemberFeatureCall__Group_1_1_1_2__0 : rule__XMemberFeatureCall__Group_1_1_1_2__0__Impl rule__XMemberFeatureCall__Group_1_1_1_2__1 ;
public final void rule__XMemberFeatureCall__Group_1_1_1_2__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28182:1: ( rule__XMemberFeatureCall__Group_1_1_1_2__0__Impl rule__XMemberFeatureCall__Group_1_1_1_2__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28183:2: rule__XMemberFeatureCall__Group_1_1_1_2__0__Impl rule__XMemberFeatureCall__Group_1_1_1_2__1
{
pushFollow(FollowSets002.FOLLOW_rule__XMemberFeatureCall__Group_1_1_1_2__0__Impl_in_rule__XMemberFeatureCall__Group_1_1_1_2__056931);
rule__XMemberFeatureCall__Group_1_1_1_2__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XMemberFeatureCall__Group_1_1_1_2__1_in_rule__XMemberFeatureCall__Group_1_1_1_2__056934);
rule__XMemberFeatureCall__Group_1_1_1_2__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XMemberFeatureCall__Group_1_1_1_2__0"
// $ANTLR start "rule__XMemberFeatureCall__Group_1_1_1_2__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28190:1: rule__XMemberFeatureCall__Group_1_1_1_2__0__Impl : ( ',' ) ;
public final void rule__XMemberFeatureCall__Group_1_1_1_2__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28194:1: ( ( ',' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28195:1: ( ',' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28195:1: ( ',' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28196:1: ','
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXMemberFeatureCallAccess().getCommaKeyword_1_1_1_2_0());
}
match(input,154,FollowSets002.FOLLOW_154_in_rule__XMemberFeatureCall__Group_1_1_1_2__0__Impl56962); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXMemberFeatureCallAccess().getCommaKeyword_1_1_1_2_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XMemberFeatureCall__Group_1_1_1_2__0__Impl"
// $ANTLR start "rule__XMemberFeatureCall__Group_1_1_1_2__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28209:1: rule__XMemberFeatureCall__Group_1_1_1_2__1 : rule__XMemberFeatureCall__Group_1_1_1_2__1__Impl ;
public final void rule__XMemberFeatureCall__Group_1_1_1_2__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28213:1: ( rule__XMemberFeatureCall__Group_1_1_1_2__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28214:2: rule__XMemberFeatureCall__Group_1_1_1_2__1__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__XMemberFeatureCall__Group_1_1_1_2__1__Impl_in_rule__XMemberFeatureCall__Group_1_1_1_2__156993);
rule__XMemberFeatureCall__Group_1_1_1_2__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XMemberFeatureCall__Group_1_1_1_2__1"
// $ANTLR start "rule__XMemberFeatureCall__Group_1_1_1_2__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28220:1: rule__XMemberFeatureCall__Group_1_1_1_2__1__Impl : ( ( rule__XMemberFeatureCall__TypeArgumentsAssignment_1_1_1_2_1 ) ) ;
public final void rule__XMemberFeatureCall__Group_1_1_1_2__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28224:1: ( ( ( rule__XMemberFeatureCall__TypeArgumentsAssignment_1_1_1_2_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28225:1: ( ( rule__XMemberFeatureCall__TypeArgumentsAssignment_1_1_1_2_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28225:1: ( ( rule__XMemberFeatureCall__TypeArgumentsAssignment_1_1_1_2_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28226:1: ( rule__XMemberFeatureCall__TypeArgumentsAssignment_1_1_1_2_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXMemberFeatureCallAccess().getTypeArgumentsAssignment_1_1_1_2_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28227:1: ( rule__XMemberFeatureCall__TypeArgumentsAssignment_1_1_1_2_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28227:2: rule__XMemberFeatureCall__TypeArgumentsAssignment_1_1_1_2_1
{
pushFollow(FollowSets002.FOLLOW_rule__XMemberFeatureCall__TypeArgumentsAssignment_1_1_1_2_1_in_rule__XMemberFeatureCall__Group_1_1_1_2__1__Impl57020);
rule__XMemberFeatureCall__TypeArgumentsAssignment_1_1_1_2_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXMemberFeatureCallAccess().getTypeArgumentsAssignment_1_1_1_2_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XMemberFeatureCall__Group_1_1_1_2__1__Impl"
// $ANTLR start "rule__XMemberFeatureCall__Group_1_1_3__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28241:1: rule__XMemberFeatureCall__Group_1_1_3__0 : rule__XMemberFeatureCall__Group_1_1_3__0__Impl rule__XMemberFeatureCall__Group_1_1_3__1 ;
public final void rule__XMemberFeatureCall__Group_1_1_3__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28245:1: ( rule__XMemberFeatureCall__Group_1_1_3__0__Impl rule__XMemberFeatureCall__Group_1_1_3__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28246:2: rule__XMemberFeatureCall__Group_1_1_3__0__Impl rule__XMemberFeatureCall__Group_1_1_3__1
{
pushFollow(FollowSets002.FOLLOW_rule__XMemberFeatureCall__Group_1_1_3__0__Impl_in_rule__XMemberFeatureCall__Group_1_1_3__057054);
rule__XMemberFeatureCall__Group_1_1_3__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XMemberFeatureCall__Group_1_1_3__1_in_rule__XMemberFeatureCall__Group_1_1_3__057057);
rule__XMemberFeatureCall__Group_1_1_3__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XMemberFeatureCall__Group_1_1_3__0"
// $ANTLR start "rule__XMemberFeatureCall__Group_1_1_3__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28253:1: rule__XMemberFeatureCall__Group_1_1_3__0__Impl : ( ( rule__XMemberFeatureCall__ExplicitOperationCallAssignment_1_1_3_0 ) ) ;
public final void rule__XMemberFeatureCall__Group_1_1_3__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28257:1: ( ( ( rule__XMemberFeatureCall__ExplicitOperationCallAssignment_1_1_3_0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28258:1: ( ( rule__XMemberFeatureCall__ExplicitOperationCallAssignment_1_1_3_0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28258:1: ( ( rule__XMemberFeatureCall__ExplicitOperationCallAssignment_1_1_3_0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28259:1: ( rule__XMemberFeatureCall__ExplicitOperationCallAssignment_1_1_3_0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXMemberFeatureCallAccess().getExplicitOperationCallAssignment_1_1_3_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28260:1: ( rule__XMemberFeatureCall__ExplicitOperationCallAssignment_1_1_3_0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28260:2: rule__XMemberFeatureCall__ExplicitOperationCallAssignment_1_1_3_0
{
pushFollow(FollowSets002.FOLLOW_rule__XMemberFeatureCall__ExplicitOperationCallAssignment_1_1_3_0_in_rule__XMemberFeatureCall__Group_1_1_3__0__Impl57084);
rule__XMemberFeatureCall__ExplicitOperationCallAssignment_1_1_3_0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXMemberFeatureCallAccess().getExplicitOperationCallAssignment_1_1_3_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XMemberFeatureCall__Group_1_1_3__0__Impl"
// $ANTLR start "rule__XMemberFeatureCall__Group_1_1_3__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28270:1: rule__XMemberFeatureCall__Group_1_1_3__1 : rule__XMemberFeatureCall__Group_1_1_3__1__Impl rule__XMemberFeatureCall__Group_1_1_3__2 ;
public final void rule__XMemberFeatureCall__Group_1_1_3__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28274:1: ( rule__XMemberFeatureCall__Group_1_1_3__1__Impl rule__XMemberFeatureCall__Group_1_1_3__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28275:2: rule__XMemberFeatureCall__Group_1_1_3__1__Impl rule__XMemberFeatureCall__Group_1_1_3__2
{
pushFollow(FollowSets002.FOLLOW_rule__XMemberFeatureCall__Group_1_1_3__1__Impl_in_rule__XMemberFeatureCall__Group_1_1_3__157114);
rule__XMemberFeatureCall__Group_1_1_3__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XMemberFeatureCall__Group_1_1_3__2_in_rule__XMemberFeatureCall__Group_1_1_3__157117);
rule__XMemberFeatureCall__Group_1_1_3__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XMemberFeatureCall__Group_1_1_3__1"
// $ANTLR start "rule__XMemberFeatureCall__Group_1_1_3__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28282:1: rule__XMemberFeatureCall__Group_1_1_3__1__Impl : ( ( rule__XMemberFeatureCall__Alternatives_1_1_3_1 )? ) ;
public final void rule__XMemberFeatureCall__Group_1_1_3__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28286:1: ( ( ( rule__XMemberFeatureCall__Alternatives_1_1_3_1 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28287:1: ( ( rule__XMemberFeatureCall__Alternatives_1_1_3_1 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28287:1: ( ( rule__XMemberFeatureCall__Alternatives_1_1_3_1 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28288:1: ( rule__XMemberFeatureCall__Alternatives_1_1_3_1 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXMemberFeatureCallAccess().getAlternatives_1_1_3_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28289:1: ( rule__XMemberFeatureCall__Alternatives_1_1_3_1 )?
int alt212=2;
int LA212_0 = input.LA(1);
if ( ((LA212_0>=RULE_ID && LA212_0<=RULE_STRING)||LA212_0==27||LA212_0==31||(LA212_0>=34 && LA212_0<=35)||LA212_0==40||(LA212_0>=45 && LA212_0<=50)||LA212_0==67||LA212_0==144||(LA212_0>=155 && LA212_0<=156)||LA212_0==159||LA212_0==161||(LA212_0>=165 && LA212_0<=173)||LA212_0==175||LA212_0==183||LA212_0==185) ) {
alt212=1;
}
switch (alt212) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28289:2: rule__XMemberFeatureCall__Alternatives_1_1_3_1
{
pushFollow(FollowSets002.FOLLOW_rule__XMemberFeatureCall__Alternatives_1_1_3_1_in_rule__XMemberFeatureCall__Group_1_1_3__1__Impl57144);
rule__XMemberFeatureCall__Alternatives_1_1_3_1();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXMemberFeatureCallAccess().getAlternatives_1_1_3_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XMemberFeatureCall__Group_1_1_3__1__Impl"
// $ANTLR start "rule__XMemberFeatureCall__Group_1_1_3__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28299:1: rule__XMemberFeatureCall__Group_1_1_3__2 : rule__XMemberFeatureCall__Group_1_1_3__2__Impl ;
public final void rule__XMemberFeatureCall__Group_1_1_3__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28303:1: ( rule__XMemberFeatureCall__Group_1_1_3__2__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28304:2: rule__XMemberFeatureCall__Group_1_1_3__2__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__XMemberFeatureCall__Group_1_1_3__2__Impl_in_rule__XMemberFeatureCall__Group_1_1_3__257175);
rule__XMemberFeatureCall__Group_1_1_3__2__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XMemberFeatureCall__Group_1_1_3__2"
// $ANTLR start "rule__XMemberFeatureCall__Group_1_1_3__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28310:1: rule__XMemberFeatureCall__Group_1_1_3__2__Impl : ( ')' ) ;
public final void rule__XMemberFeatureCall__Group_1_1_3__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28314:1: ( ( ')' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28315:1: ( ')' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28315:1: ( ')' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28316:1: ')'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXMemberFeatureCallAccess().getRightParenthesisKeyword_1_1_3_2());
}
match(input,145,FollowSets002.FOLLOW_145_in_rule__XMemberFeatureCall__Group_1_1_3__2__Impl57203); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXMemberFeatureCallAccess().getRightParenthesisKeyword_1_1_3_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XMemberFeatureCall__Group_1_1_3__2__Impl"
// $ANTLR start "rule__XMemberFeatureCall__Group_1_1_3_1_1__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28335:1: rule__XMemberFeatureCall__Group_1_1_3_1_1__0 : rule__XMemberFeatureCall__Group_1_1_3_1_1__0__Impl rule__XMemberFeatureCall__Group_1_1_3_1_1__1 ;
public final void rule__XMemberFeatureCall__Group_1_1_3_1_1__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28339:1: ( rule__XMemberFeatureCall__Group_1_1_3_1_1__0__Impl rule__XMemberFeatureCall__Group_1_1_3_1_1__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28340:2: rule__XMemberFeatureCall__Group_1_1_3_1_1__0__Impl rule__XMemberFeatureCall__Group_1_1_3_1_1__1
{
pushFollow(FollowSets002.FOLLOW_rule__XMemberFeatureCall__Group_1_1_3_1_1__0__Impl_in_rule__XMemberFeatureCall__Group_1_1_3_1_1__057240);
rule__XMemberFeatureCall__Group_1_1_3_1_1__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XMemberFeatureCall__Group_1_1_3_1_1__1_in_rule__XMemberFeatureCall__Group_1_1_3_1_1__057243);
rule__XMemberFeatureCall__Group_1_1_3_1_1__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XMemberFeatureCall__Group_1_1_3_1_1__0"
// $ANTLR start "rule__XMemberFeatureCall__Group_1_1_3_1_1__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28347:1: rule__XMemberFeatureCall__Group_1_1_3_1_1__0__Impl : ( ( rule__XMemberFeatureCall__MemberCallArgumentsAssignment_1_1_3_1_1_0 ) ) ;
public final void rule__XMemberFeatureCall__Group_1_1_3_1_1__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28351:1: ( ( ( rule__XMemberFeatureCall__MemberCallArgumentsAssignment_1_1_3_1_1_0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28352:1: ( ( rule__XMemberFeatureCall__MemberCallArgumentsAssignment_1_1_3_1_1_0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28352:1: ( ( rule__XMemberFeatureCall__MemberCallArgumentsAssignment_1_1_3_1_1_0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28353:1: ( rule__XMemberFeatureCall__MemberCallArgumentsAssignment_1_1_3_1_1_0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXMemberFeatureCallAccess().getMemberCallArgumentsAssignment_1_1_3_1_1_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28354:1: ( rule__XMemberFeatureCall__MemberCallArgumentsAssignment_1_1_3_1_1_0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28354:2: rule__XMemberFeatureCall__MemberCallArgumentsAssignment_1_1_3_1_1_0
{
pushFollow(FollowSets002.FOLLOW_rule__XMemberFeatureCall__MemberCallArgumentsAssignment_1_1_3_1_1_0_in_rule__XMemberFeatureCall__Group_1_1_3_1_1__0__Impl57270);
rule__XMemberFeatureCall__MemberCallArgumentsAssignment_1_1_3_1_1_0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXMemberFeatureCallAccess().getMemberCallArgumentsAssignment_1_1_3_1_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XMemberFeatureCall__Group_1_1_3_1_1__0__Impl"
// $ANTLR start "rule__XMemberFeatureCall__Group_1_1_3_1_1__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28364:1: rule__XMemberFeatureCall__Group_1_1_3_1_1__1 : rule__XMemberFeatureCall__Group_1_1_3_1_1__1__Impl ;
public final void rule__XMemberFeatureCall__Group_1_1_3_1_1__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28368:1: ( rule__XMemberFeatureCall__Group_1_1_3_1_1__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28369:2: rule__XMemberFeatureCall__Group_1_1_3_1_1__1__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__XMemberFeatureCall__Group_1_1_3_1_1__1__Impl_in_rule__XMemberFeatureCall__Group_1_1_3_1_1__157300);
rule__XMemberFeatureCall__Group_1_1_3_1_1__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XMemberFeatureCall__Group_1_1_3_1_1__1"
// $ANTLR start "rule__XMemberFeatureCall__Group_1_1_3_1_1__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28375:1: rule__XMemberFeatureCall__Group_1_1_3_1_1__1__Impl : ( ( rule__XMemberFeatureCall__Group_1_1_3_1_1_1__0 )* ) ;
public final void rule__XMemberFeatureCall__Group_1_1_3_1_1__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28379:1: ( ( ( rule__XMemberFeatureCall__Group_1_1_3_1_1_1__0 )* ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28380:1: ( ( rule__XMemberFeatureCall__Group_1_1_3_1_1_1__0 )* )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28380:1: ( ( rule__XMemberFeatureCall__Group_1_1_3_1_1_1__0 )* )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28381:1: ( rule__XMemberFeatureCall__Group_1_1_3_1_1_1__0 )*
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXMemberFeatureCallAccess().getGroup_1_1_3_1_1_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28382:1: ( rule__XMemberFeatureCall__Group_1_1_3_1_1_1__0 )*
loop213:
do {
int alt213=2;
int LA213_0 = input.LA(1);
if ( (LA213_0==154) ) {
alt213=1;
}
switch (alt213) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28382:2: rule__XMemberFeatureCall__Group_1_1_3_1_1_1__0
{
pushFollow(FollowSets002.FOLLOW_rule__XMemberFeatureCall__Group_1_1_3_1_1_1__0_in_rule__XMemberFeatureCall__Group_1_1_3_1_1__1__Impl57327);
rule__XMemberFeatureCall__Group_1_1_3_1_1_1__0();
state._fsp--;
if (state.failed) return ;
}
break;
default :
break loop213;
}
} while (true);
if ( state.backtracking==0 ) {
after(grammarAccess.getXMemberFeatureCallAccess().getGroup_1_1_3_1_1_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XMemberFeatureCall__Group_1_1_3_1_1__1__Impl"
// $ANTLR start "rule__XMemberFeatureCall__Group_1_1_3_1_1_1__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28396:1: rule__XMemberFeatureCall__Group_1_1_3_1_1_1__0 : rule__XMemberFeatureCall__Group_1_1_3_1_1_1__0__Impl rule__XMemberFeatureCall__Group_1_1_3_1_1_1__1 ;
public final void rule__XMemberFeatureCall__Group_1_1_3_1_1_1__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28400:1: ( rule__XMemberFeatureCall__Group_1_1_3_1_1_1__0__Impl rule__XMemberFeatureCall__Group_1_1_3_1_1_1__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28401:2: rule__XMemberFeatureCall__Group_1_1_3_1_1_1__0__Impl rule__XMemberFeatureCall__Group_1_1_3_1_1_1__1
{
pushFollow(FollowSets002.FOLLOW_rule__XMemberFeatureCall__Group_1_1_3_1_1_1__0__Impl_in_rule__XMemberFeatureCall__Group_1_1_3_1_1_1__057362);
rule__XMemberFeatureCall__Group_1_1_3_1_1_1__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XMemberFeatureCall__Group_1_1_3_1_1_1__1_in_rule__XMemberFeatureCall__Group_1_1_3_1_1_1__057365);
rule__XMemberFeatureCall__Group_1_1_3_1_1_1__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XMemberFeatureCall__Group_1_1_3_1_1_1__0"
// $ANTLR start "rule__XMemberFeatureCall__Group_1_1_3_1_1_1__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28408:1: rule__XMemberFeatureCall__Group_1_1_3_1_1_1__0__Impl : ( ',' ) ;
public final void rule__XMemberFeatureCall__Group_1_1_3_1_1_1__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28412:1: ( ( ',' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28413:1: ( ',' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28413:1: ( ',' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28414:1: ','
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXMemberFeatureCallAccess().getCommaKeyword_1_1_3_1_1_1_0());
}
match(input,154,FollowSets002.FOLLOW_154_in_rule__XMemberFeatureCall__Group_1_1_3_1_1_1__0__Impl57393); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXMemberFeatureCallAccess().getCommaKeyword_1_1_3_1_1_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XMemberFeatureCall__Group_1_1_3_1_1_1__0__Impl"
// $ANTLR start "rule__XMemberFeatureCall__Group_1_1_3_1_1_1__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28427:1: rule__XMemberFeatureCall__Group_1_1_3_1_1_1__1 : rule__XMemberFeatureCall__Group_1_1_3_1_1_1__1__Impl ;
public final void rule__XMemberFeatureCall__Group_1_1_3_1_1_1__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28431:1: ( rule__XMemberFeatureCall__Group_1_1_3_1_1_1__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28432:2: rule__XMemberFeatureCall__Group_1_1_3_1_1_1__1__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__XMemberFeatureCall__Group_1_1_3_1_1_1__1__Impl_in_rule__XMemberFeatureCall__Group_1_1_3_1_1_1__157424);
rule__XMemberFeatureCall__Group_1_1_3_1_1_1__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XMemberFeatureCall__Group_1_1_3_1_1_1__1"
// $ANTLR start "rule__XMemberFeatureCall__Group_1_1_3_1_1_1__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28438:1: rule__XMemberFeatureCall__Group_1_1_3_1_1_1__1__Impl : ( ( rule__XMemberFeatureCall__MemberCallArgumentsAssignment_1_1_3_1_1_1_1 ) ) ;
public final void rule__XMemberFeatureCall__Group_1_1_3_1_1_1__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28442:1: ( ( ( rule__XMemberFeatureCall__MemberCallArgumentsAssignment_1_1_3_1_1_1_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28443:1: ( ( rule__XMemberFeatureCall__MemberCallArgumentsAssignment_1_1_3_1_1_1_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28443:1: ( ( rule__XMemberFeatureCall__MemberCallArgumentsAssignment_1_1_3_1_1_1_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28444:1: ( rule__XMemberFeatureCall__MemberCallArgumentsAssignment_1_1_3_1_1_1_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXMemberFeatureCallAccess().getMemberCallArgumentsAssignment_1_1_3_1_1_1_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28445:1: ( rule__XMemberFeatureCall__MemberCallArgumentsAssignment_1_1_3_1_1_1_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28445:2: rule__XMemberFeatureCall__MemberCallArgumentsAssignment_1_1_3_1_1_1_1
{
pushFollow(FollowSets002.FOLLOW_rule__XMemberFeatureCall__MemberCallArgumentsAssignment_1_1_3_1_1_1_1_in_rule__XMemberFeatureCall__Group_1_1_3_1_1_1__1__Impl57451);
rule__XMemberFeatureCall__MemberCallArgumentsAssignment_1_1_3_1_1_1_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXMemberFeatureCallAccess().getMemberCallArgumentsAssignment_1_1_3_1_1_1_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XMemberFeatureCall__Group_1_1_3_1_1_1__1__Impl"
// $ANTLR start "rule__XSetLiteral__Group__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28459:1: rule__XSetLiteral__Group__0 : rule__XSetLiteral__Group__0__Impl rule__XSetLiteral__Group__1 ;
public final void rule__XSetLiteral__Group__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28463:1: ( rule__XSetLiteral__Group__0__Impl rule__XSetLiteral__Group__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28464:2: rule__XSetLiteral__Group__0__Impl rule__XSetLiteral__Group__1
{
pushFollow(FollowSets002.FOLLOW_rule__XSetLiteral__Group__0__Impl_in_rule__XSetLiteral__Group__057485);
rule__XSetLiteral__Group__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XSetLiteral__Group__1_in_rule__XSetLiteral__Group__057488);
rule__XSetLiteral__Group__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XSetLiteral__Group__0"
// $ANTLR start "rule__XSetLiteral__Group__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28471:1: rule__XSetLiteral__Group__0__Impl : ( () ) ;
public final void rule__XSetLiteral__Group__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28475:1: ( ( () ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28476:1: ( () )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28476:1: ( () )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28477:1: ()
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXSetLiteralAccess().getXSetLiteralAction_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28478:1: ()
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28480:1:
{
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXSetLiteralAccess().getXSetLiteralAction_0());
}
}
}
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XSetLiteral__Group__0__Impl"
// $ANTLR start "rule__XSetLiteral__Group__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28490:1: rule__XSetLiteral__Group__1 : rule__XSetLiteral__Group__1__Impl rule__XSetLiteral__Group__2 ;
public final void rule__XSetLiteral__Group__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28494:1: ( rule__XSetLiteral__Group__1__Impl rule__XSetLiteral__Group__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28495:2: rule__XSetLiteral__Group__1__Impl rule__XSetLiteral__Group__2
{
pushFollow(FollowSets002.FOLLOW_rule__XSetLiteral__Group__1__Impl_in_rule__XSetLiteral__Group__157546);
rule__XSetLiteral__Group__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XSetLiteral__Group__2_in_rule__XSetLiteral__Group__157549);
rule__XSetLiteral__Group__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XSetLiteral__Group__1"
// $ANTLR start "rule__XSetLiteral__Group__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28502:1: rule__XSetLiteral__Group__1__Impl : ( '#' ) ;
public final void rule__XSetLiteral__Group__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28506:1: ( ( '#' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28507:1: ( '#' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28507:1: ( '#' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28508:1: '#'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXSetLiteralAccess().getNumberSignKeyword_1());
}
match(input,155,FollowSets002.FOLLOW_155_in_rule__XSetLiteral__Group__1__Impl57577); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXSetLiteralAccess().getNumberSignKeyword_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XSetLiteral__Group__1__Impl"
// $ANTLR start "rule__XSetLiteral__Group__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28521:1: rule__XSetLiteral__Group__2 : rule__XSetLiteral__Group__2__Impl rule__XSetLiteral__Group__3 ;
public final void rule__XSetLiteral__Group__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28525:1: ( rule__XSetLiteral__Group__2__Impl rule__XSetLiteral__Group__3 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28526:2: rule__XSetLiteral__Group__2__Impl rule__XSetLiteral__Group__3
{
pushFollow(FollowSets002.FOLLOW_rule__XSetLiteral__Group__2__Impl_in_rule__XSetLiteral__Group__257608);
rule__XSetLiteral__Group__2__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XSetLiteral__Group__3_in_rule__XSetLiteral__Group__257611);
rule__XSetLiteral__Group__3();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XSetLiteral__Group__2"
// $ANTLR start "rule__XSetLiteral__Group__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28533:1: rule__XSetLiteral__Group__2__Impl : ( '{' ) ;
public final void rule__XSetLiteral__Group__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28537:1: ( ( '{' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28538:1: ( '{' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28538:1: ( '{' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28539:1: '{'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXSetLiteralAccess().getLeftCurlyBracketKeyword_2());
}
match(input,67,FollowSets002.FOLLOW_67_in_rule__XSetLiteral__Group__2__Impl57639); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXSetLiteralAccess().getLeftCurlyBracketKeyword_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XSetLiteral__Group__2__Impl"
// $ANTLR start "rule__XSetLiteral__Group__3"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28552:1: rule__XSetLiteral__Group__3 : rule__XSetLiteral__Group__3__Impl rule__XSetLiteral__Group__4 ;
public final void rule__XSetLiteral__Group__3() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28556:1: ( rule__XSetLiteral__Group__3__Impl rule__XSetLiteral__Group__4 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28557:2: rule__XSetLiteral__Group__3__Impl rule__XSetLiteral__Group__4
{
pushFollow(FollowSets002.FOLLOW_rule__XSetLiteral__Group__3__Impl_in_rule__XSetLiteral__Group__357670);
rule__XSetLiteral__Group__3__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XSetLiteral__Group__4_in_rule__XSetLiteral__Group__357673);
rule__XSetLiteral__Group__4();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XSetLiteral__Group__3"
// $ANTLR start "rule__XSetLiteral__Group__3__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28564:1: rule__XSetLiteral__Group__3__Impl : ( ( rule__XSetLiteral__Group_3__0 )? ) ;
public final void rule__XSetLiteral__Group__3__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28568:1: ( ( ( rule__XSetLiteral__Group_3__0 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28569:1: ( ( rule__XSetLiteral__Group_3__0 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28569:1: ( ( rule__XSetLiteral__Group_3__0 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28570:1: ( rule__XSetLiteral__Group_3__0 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXSetLiteralAccess().getGroup_3());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28571:1: ( rule__XSetLiteral__Group_3__0 )?
int alt214=2;
int LA214_0 = input.LA(1);
if ( ((LA214_0>=RULE_ID && LA214_0<=RULE_STRING)||LA214_0==27||(LA214_0>=34 && LA214_0<=35)||LA214_0==40||(LA214_0>=45 && LA214_0<=50)||LA214_0==67||LA214_0==144||(LA214_0>=155 && LA214_0<=156)||LA214_0==159||LA214_0==161||(LA214_0>=165 && LA214_0<=173)||LA214_0==175||LA214_0==185) ) {
alt214=1;
}
switch (alt214) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28571:2: rule__XSetLiteral__Group_3__0
{
pushFollow(FollowSets002.FOLLOW_rule__XSetLiteral__Group_3__0_in_rule__XSetLiteral__Group__3__Impl57700);
rule__XSetLiteral__Group_3__0();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXSetLiteralAccess().getGroup_3());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XSetLiteral__Group__3__Impl"
// $ANTLR start "rule__XSetLiteral__Group__4"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28581:1: rule__XSetLiteral__Group__4 : rule__XSetLiteral__Group__4__Impl ;
public final void rule__XSetLiteral__Group__4() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28585:1: ( rule__XSetLiteral__Group__4__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28586:2: rule__XSetLiteral__Group__4__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__XSetLiteral__Group__4__Impl_in_rule__XSetLiteral__Group__457731);
rule__XSetLiteral__Group__4__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XSetLiteral__Group__4"
// $ANTLR start "rule__XSetLiteral__Group__4__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28592:1: rule__XSetLiteral__Group__4__Impl : ( '}' ) ;
public final void rule__XSetLiteral__Group__4__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28596:1: ( ( '}' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28597:1: ( '}' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28597:1: ( '}' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28598:1: '}'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXSetLiteralAccess().getRightCurlyBracketKeyword_4());
}
match(input,68,FollowSets002.FOLLOW_68_in_rule__XSetLiteral__Group__4__Impl57759); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXSetLiteralAccess().getRightCurlyBracketKeyword_4());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XSetLiteral__Group__4__Impl"
// $ANTLR start "rule__XSetLiteral__Group_3__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28621:1: rule__XSetLiteral__Group_3__0 : rule__XSetLiteral__Group_3__0__Impl rule__XSetLiteral__Group_3__1 ;
public final void rule__XSetLiteral__Group_3__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28625:1: ( rule__XSetLiteral__Group_3__0__Impl rule__XSetLiteral__Group_3__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28626:2: rule__XSetLiteral__Group_3__0__Impl rule__XSetLiteral__Group_3__1
{
pushFollow(FollowSets002.FOLLOW_rule__XSetLiteral__Group_3__0__Impl_in_rule__XSetLiteral__Group_3__057800);
rule__XSetLiteral__Group_3__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XSetLiteral__Group_3__1_in_rule__XSetLiteral__Group_3__057803);
rule__XSetLiteral__Group_3__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XSetLiteral__Group_3__0"
// $ANTLR start "rule__XSetLiteral__Group_3__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28633:1: rule__XSetLiteral__Group_3__0__Impl : ( ( rule__XSetLiteral__ElementsAssignment_3_0 ) ) ;
public final void rule__XSetLiteral__Group_3__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28637:1: ( ( ( rule__XSetLiteral__ElementsAssignment_3_0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28638:1: ( ( rule__XSetLiteral__ElementsAssignment_3_0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28638:1: ( ( rule__XSetLiteral__ElementsAssignment_3_0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28639:1: ( rule__XSetLiteral__ElementsAssignment_3_0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXSetLiteralAccess().getElementsAssignment_3_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28640:1: ( rule__XSetLiteral__ElementsAssignment_3_0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28640:2: rule__XSetLiteral__ElementsAssignment_3_0
{
pushFollow(FollowSets002.FOLLOW_rule__XSetLiteral__ElementsAssignment_3_0_in_rule__XSetLiteral__Group_3__0__Impl57830);
rule__XSetLiteral__ElementsAssignment_3_0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXSetLiteralAccess().getElementsAssignment_3_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XSetLiteral__Group_3__0__Impl"
// $ANTLR start "rule__XSetLiteral__Group_3__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28650:1: rule__XSetLiteral__Group_3__1 : rule__XSetLiteral__Group_3__1__Impl ;
public final void rule__XSetLiteral__Group_3__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28654:1: ( rule__XSetLiteral__Group_3__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28655:2: rule__XSetLiteral__Group_3__1__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__XSetLiteral__Group_3__1__Impl_in_rule__XSetLiteral__Group_3__157860);
rule__XSetLiteral__Group_3__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XSetLiteral__Group_3__1"
// $ANTLR start "rule__XSetLiteral__Group_3__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28661:1: rule__XSetLiteral__Group_3__1__Impl : ( ( rule__XSetLiteral__Group_3_1__0 )* ) ;
public final void rule__XSetLiteral__Group_3__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28665:1: ( ( ( rule__XSetLiteral__Group_3_1__0 )* ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28666:1: ( ( rule__XSetLiteral__Group_3_1__0 )* )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28666:1: ( ( rule__XSetLiteral__Group_3_1__0 )* )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28667:1: ( rule__XSetLiteral__Group_3_1__0 )*
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXSetLiteralAccess().getGroup_3_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28668:1: ( rule__XSetLiteral__Group_3_1__0 )*
loop215:
do {
int alt215=2;
int LA215_0 = input.LA(1);
if ( (LA215_0==154) ) {
alt215=1;
}
switch (alt215) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28668:2: rule__XSetLiteral__Group_3_1__0
{
pushFollow(FollowSets002.FOLLOW_rule__XSetLiteral__Group_3_1__0_in_rule__XSetLiteral__Group_3__1__Impl57887);
rule__XSetLiteral__Group_3_1__0();
state._fsp--;
if (state.failed) return ;
}
break;
default :
break loop215;
}
} while (true);
if ( state.backtracking==0 ) {
after(grammarAccess.getXSetLiteralAccess().getGroup_3_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XSetLiteral__Group_3__1__Impl"
// $ANTLR start "rule__XSetLiteral__Group_3_1__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28682:1: rule__XSetLiteral__Group_3_1__0 : rule__XSetLiteral__Group_3_1__0__Impl rule__XSetLiteral__Group_3_1__1 ;
public final void rule__XSetLiteral__Group_3_1__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28686:1: ( rule__XSetLiteral__Group_3_1__0__Impl rule__XSetLiteral__Group_3_1__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28687:2: rule__XSetLiteral__Group_3_1__0__Impl rule__XSetLiteral__Group_3_1__1
{
pushFollow(FollowSets002.FOLLOW_rule__XSetLiteral__Group_3_1__0__Impl_in_rule__XSetLiteral__Group_3_1__057922);
rule__XSetLiteral__Group_3_1__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XSetLiteral__Group_3_1__1_in_rule__XSetLiteral__Group_3_1__057925);
rule__XSetLiteral__Group_3_1__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XSetLiteral__Group_3_1__0"
// $ANTLR start "rule__XSetLiteral__Group_3_1__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28694:1: rule__XSetLiteral__Group_3_1__0__Impl : ( ',' ) ;
public final void rule__XSetLiteral__Group_3_1__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28698:1: ( ( ',' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28699:1: ( ',' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28699:1: ( ',' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28700:1: ','
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXSetLiteralAccess().getCommaKeyword_3_1_0());
}
match(input,154,FollowSets002.FOLLOW_154_in_rule__XSetLiteral__Group_3_1__0__Impl57953); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXSetLiteralAccess().getCommaKeyword_3_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XSetLiteral__Group_3_1__0__Impl"
// $ANTLR start "rule__XSetLiteral__Group_3_1__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28713:1: rule__XSetLiteral__Group_3_1__1 : rule__XSetLiteral__Group_3_1__1__Impl ;
public final void rule__XSetLiteral__Group_3_1__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28717:1: ( rule__XSetLiteral__Group_3_1__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28718:2: rule__XSetLiteral__Group_3_1__1__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__XSetLiteral__Group_3_1__1__Impl_in_rule__XSetLiteral__Group_3_1__157984);
rule__XSetLiteral__Group_3_1__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XSetLiteral__Group_3_1__1"
// $ANTLR start "rule__XSetLiteral__Group_3_1__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28724:1: rule__XSetLiteral__Group_3_1__1__Impl : ( ( rule__XSetLiteral__ElementsAssignment_3_1_1 ) ) ;
public final void rule__XSetLiteral__Group_3_1__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28728:1: ( ( ( rule__XSetLiteral__ElementsAssignment_3_1_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28729:1: ( ( rule__XSetLiteral__ElementsAssignment_3_1_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28729:1: ( ( rule__XSetLiteral__ElementsAssignment_3_1_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28730:1: ( rule__XSetLiteral__ElementsAssignment_3_1_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXSetLiteralAccess().getElementsAssignment_3_1_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28731:1: ( rule__XSetLiteral__ElementsAssignment_3_1_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28731:2: rule__XSetLiteral__ElementsAssignment_3_1_1
{
pushFollow(FollowSets002.FOLLOW_rule__XSetLiteral__ElementsAssignment_3_1_1_in_rule__XSetLiteral__Group_3_1__1__Impl58011);
rule__XSetLiteral__ElementsAssignment_3_1_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXSetLiteralAccess().getElementsAssignment_3_1_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XSetLiteral__Group_3_1__1__Impl"
// $ANTLR start "rule__XListLiteral__Group__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28745:1: rule__XListLiteral__Group__0 : rule__XListLiteral__Group__0__Impl rule__XListLiteral__Group__1 ;
public final void rule__XListLiteral__Group__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28749:1: ( rule__XListLiteral__Group__0__Impl rule__XListLiteral__Group__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28750:2: rule__XListLiteral__Group__0__Impl rule__XListLiteral__Group__1
{
pushFollow(FollowSets002.FOLLOW_rule__XListLiteral__Group__0__Impl_in_rule__XListLiteral__Group__058045);
rule__XListLiteral__Group__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XListLiteral__Group__1_in_rule__XListLiteral__Group__058048);
rule__XListLiteral__Group__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XListLiteral__Group__0"
// $ANTLR start "rule__XListLiteral__Group__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28757:1: rule__XListLiteral__Group__0__Impl : ( () ) ;
public final void rule__XListLiteral__Group__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28761:1: ( ( () ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28762:1: ( () )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28762:1: ( () )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28763:1: ()
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXListLiteralAccess().getXListLiteralAction_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28764:1: ()
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28766:1:
{
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXListLiteralAccess().getXListLiteralAction_0());
}
}
}
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XListLiteral__Group__0__Impl"
// $ANTLR start "rule__XListLiteral__Group__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28776:1: rule__XListLiteral__Group__1 : rule__XListLiteral__Group__1__Impl rule__XListLiteral__Group__2 ;
public final void rule__XListLiteral__Group__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28780:1: ( rule__XListLiteral__Group__1__Impl rule__XListLiteral__Group__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28781:2: rule__XListLiteral__Group__1__Impl rule__XListLiteral__Group__2
{
pushFollow(FollowSets002.FOLLOW_rule__XListLiteral__Group__1__Impl_in_rule__XListLiteral__Group__158106);
rule__XListLiteral__Group__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XListLiteral__Group__2_in_rule__XListLiteral__Group__158109);
rule__XListLiteral__Group__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XListLiteral__Group__1"
// $ANTLR start "rule__XListLiteral__Group__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28788:1: rule__XListLiteral__Group__1__Impl : ( '#' ) ;
public final void rule__XListLiteral__Group__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28792:1: ( ( '#' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28793:1: ( '#' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28793:1: ( '#' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28794:1: '#'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXListLiteralAccess().getNumberSignKeyword_1());
}
match(input,155,FollowSets002.FOLLOW_155_in_rule__XListLiteral__Group__1__Impl58137); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXListLiteralAccess().getNumberSignKeyword_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XListLiteral__Group__1__Impl"
// $ANTLR start "rule__XListLiteral__Group__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28807:1: rule__XListLiteral__Group__2 : rule__XListLiteral__Group__2__Impl rule__XListLiteral__Group__3 ;
public final void rule__XListLiteral__Group__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28811:1: ( rule__XListLiteral__Group__2__Impl rule__XListLiteral__Group__3 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28812:2: rule__XListLiteral__Group__2__Impl rule__XListLiteral__Group__3
{
pushFollow(FollowSets002.FOLLOW_rule__XListLiteral__Group__2__Impl_in_rule__XListLiteral__Group__258168);
rule__XListLiteral__Group__2__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XListLiteral__Group__3_in_rule__XListLiteral__Group__258171);
rule__XListLiteral__Group__3();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XListLiteral__Group__2"
// $ANTLR start "rule__XListLiteral__Group__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28819:1: rule__XListLiteral__Group__2__Impl : ( '[' ) ;
public final void rule__XListLiteral__Group__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28823:1: ( ( '[' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28824:1: ( '[' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28824:1: ( '[' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28825:1: '['
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXListLiteralAccess().getLeftSquareBracketKeyword_2());
}
match(input,156,FollowSets002.FOLLOW_156_in_rule__XListLiteral__Group__2__Impl58199); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXListLiteralAccess().getLeftSquareBracketKeyword_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XListLiteral__Group__2__Impl"
// $ANTLR start "rule__XListLiteral__Group__3"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28838:1: rule__XListLiteral__Group__3 : rule__XListLiteral__Group__3__Impl rule__XListLiteral__Group__4 ;
public final void rule__XListLiteral__Group__3() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28842:1: ( rule__XListLiteral__Group__3__Impl rule__XListLiteral__Group__4 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28843:2: rule__XListLiteral__Group__3__Impl rule__XListLiteral__Group__4
{
pushFollow(FollowSets002.FOLLOW_rule__XListLiteral__Group__3__Impl_in_rule__XListLiteral__Group__358230);
rule__XListLiteral__Group__3__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XListLiteral__Group__4_in_rule__XListLiteral__Group__358233);
rule__XListLiteral__Group__4();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XListLiteral__Group__3"
// $ANTLR start "rule__XListLiteral__Group__3__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28850:1: rule__XListLiteral__Group__3__Impl : ( ( rule__XListLiteral__Group_3__0 )? ) ;
public final void rule__XListLiteral__Group__3__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28854:1: ( ( ( rule__XListLiteral__Group_3__0 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28855:1: ( ( rule__XListLiteral__Group_3__0 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28855:1: ( ( rule__XListLiteral__Group_3__0 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28856:1: ( rule__XListLiteral__Group_3__0 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXListLiteralAccess().getGroup_3());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28857:1: ( rule__XListLiteral__Group_3__0 )?
int alt216=2;
int LA216_0 = input.LA(1);
if ( ((LA216_0>=RULE_ID && LA216_0<=RULE_STRING)||LA216_0==27||(LA216_0>=34 && LA216_0<=35)||LA216_0==40||(LA216_0>=45 && LA216_0<=50)||LA216_0==67||LA216_0==144||(LA216_0>=155 && LA216_0<=156)||LA216_0==159||LA216_0==161||(LA216_0>=165 && LA216_0<=173)||LA216_0==175||LA216_0==185) ) {
alt216=1;
}
switch (alt216) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28857:2: rule__XListLiteral__Group_3__0
{
pushFollow(FollowSets002.FOLLOW_rule__XListLiteral__Group_3__0_in_rule__XListLiteral__Group__3__Impl58260);
rule__XListLiteral__Group_3__0();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXListLiteralAccess().getGroup_3());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XListLiteral__Group__3__Impl"
// $ANTLR start "rule__XListLiteral__Group__4"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28867:1: rule__XListLiteral__Group__4 : rule__XListLiteral__Group__4__Impl ;
public final void rule__XListLiteral__Group__4() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28871:1: ( rule__XListLiteral__Group__4__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28872:2: rule__XListLiteral__Group__4__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__XListLiteral__Group__4__Impl_in_rule__XListLiteral__Group__458291);
rule__XListLiteral__Group__4__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XListLiteral__Group__4"
// $ANTLR start "rule__XListLiteral__Group__4__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28878:1: rule__XListLiteral__Group__4__Impl : ( ']' ) ;
public final void rule__XListLiteral__Group__4__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28882:1: ( ( ']' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28883:1: ( ']' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28883:1: ( ']' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28884:1: ']'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXListLiteralAccess().getRightSquareBracketKeyword_4());
}
match(input,157,FollowSets002.FOLLOW_157_in_rule__XListLiteral__Group__4__Impl58319); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXListLiteralAccess().getRightSquareBracketKeyword_4());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XListLiteral__Group__4__Impl"
// $ANTLR start "rule__XListLiteral__Group_3__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28907:1: rule__XListLiteral__Group_3__0 : rule__XListLiteral__Group_3__0__Impl rule__XListLiteral__Group_3__1 ;
public final void rule__XListLiteral__Group_3__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28911:1: ( rule__XListLiteral__Group_3__0__Impl rule__XListLiteral__Group_3__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28912:2: rule__XListLiteral__Group_3__0__Impl rule__XListLiteral__Group_3__1
{
pushFollow(FollowSets002.FOLLOW_rule__XListLiteral__Group_3__0__Impl_in_rule__XListLiteral__Group_3__058360);
rule__XListLiteral__Group_3__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XListLiteral__Group_3__1_in_rule__XListLiteral__Group_3__058363);
rule__XListLiteral__Group_3__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XListLiteral__Group_3__0"
// $ANTLR start "rule__XListLiteral__Group_3__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28919:1: rule__XListLiteral__Group_3__0__Impl : ( ( rule__XListLiteral__ElementsAssignment_3_0 ) ) ;
public final void rule__XListLiteral__Group_3__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28923:1: ( ( ( rule__XListLiteral__ElementsAssignment_3_0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28924:1: ( ( rule__XListLiteral__ElementsAssignment_3_0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28924:1: ( ( rule__XListLiteral__ElementsAssignment_3_0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28925:1: ( rule__XListLiteral__ElementsAssignment_3_0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXListLiteralAccess().getElementsAssignment_3_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28926:1: ( rule__XListLiteral__ElementsAssignment_3_0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28926:2: rule__XListLiteral__ElementsAssignment_3_0
{
pushFollow(FollowSets002.FOLLOW_rule__XListLiteral__ElementsAssignment_3_0_in_rule__XListLiteral__Group_3__0__Impl58390);
rule__XListLiteral__ElementsAssignment_3_0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXListLiteralAccess().getElementsAssignment_3_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XListLiteral__Group_3__0__Impl"
// $ANTLR start "rule__XListLiteral__Group_3__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28936:1: rule__XListLiteral__Group_3__1 : rule__XListLiteral__Group_3__1__Impl ;
public final void rule__XListLiteral__Group_3__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28940:1: ( rule__XListLiteral__Group_3__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28941:2: rule__XListLiteral__Group_3__1__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__XListLiteral__Group_3__1__Impl_in_rule__XListLiteral__Group_3__158420);
rule__XListLiteral__Group_3__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XListLiteral__Group_3__1"
// $ANTLR start "rule__XListLiteral__Group_3__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28947:1: rule__XListLiteral__Group_3__1__Impl : ( ( rule__XListLiteral__Group_3_1__0 )* ) ;
public final void rule__XListLiteral__Group_3__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28951:1: ( ( ( rule__XListLiteral__Group_3_1__0 )* ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28952:1: ( ( rule__XListLiteral__Group_3_1__0 )* )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28952:1: ( ( rule__XListLiteral__Group_3_1__0 )* )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28953:1: ( rule__XListLiteral__Group_3_1__0 )*
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXListLiteralAccess().getGroup_3_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28954:1: ( rule__XListLiteral__Group_3_1__0 )*
loop217:
do {
int alt217=2;
int LA217_0 = input.LA(1);
if ( (LA217_0==154) ) {
alt217=1;
}
switch (alt217) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28954:2: rule__XListLiteral__Group_3_1__0
{
pushFollow(FollowSets002.FOLLOW_rule__XListLiteral__Group_3_1__0_in_rule__XListLiteral__Group_3__1__Impl58447);
rule__XListLiteral__Group_3_1__0();
state._fsp--;
if (state.failed) return ;
}
break;
default :
break loop217;
}
} while (true);
if ( state.backtracking==0 ) {
after(grammarAccess.getXListLiteralAccess().getGroup_3_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XListLiteral__Group_3__1__Impl"
// $ANTLR start "rule__XListLiteral__Group_3_1__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28968:1: rule__XListLiteral__Group_3_1__0 : rule__XListLiteral__Group_3_1__0__Impl rule__XListLiteral__Group_3_1__1 ;
public final void rule__XListLiteral__Group_3_1__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28972:1: ( rule__XListLiteral__Group_3_1__0__Impl rule__XListLiteral__Group_3_1__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28973:2: rule__XListLiteral__Group_3_1__0__Impl rule__XListLiteral__Group_3_1__1
{
pushFollow(FollowSets002.FOLLOW_rule__XListLiteral__Group_3_1__0__Impl_in_rule__XListLiteral__Group_3_1__058482);
rule__XListLiteral__Group_3_1__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XListLiteral__Group_3_1__1_in_rule__XListLiteral__Group_3_1__058485);
rule__XListLiteral__Group_3_1__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XListLiteral__Group_3_1__0"
// $ANTLR start "rule__XListLiteral__Group_3_1__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28980:1: rule__XListLiteral__Group_3_1__0__Impl : ( ',' ) ;
public final void rule__XListLiteral__Group_3_1__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28984:1: ( ( ',' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28985:1: ( ',' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28985:1: ( ',' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28986:1: ','
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXListLiteralAccess().getCommaKeyword_3_1_0());
}
match(input,154,FollowSets002.FOLLOW_154_in_rule__XListLiteral__Group_3_1__0__Impl58513); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXListLiteralAccess().getCommaKeyword_3_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XListLiteral__Group_3_1__0__Impl"
// $ANTLR start "rule__XListLiteral__Group_3_1__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:28999:1: rule__XListLiteral__Group_3_1__1 : rule__XListLiteral__Group_3_1__1__Impl ;
public final void rule__XListLiteral__Group_3_1__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29003:1: ( rule__XListLiteral__Group_3_1__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29004:2: rule__XListLiteral__Group_3_1__1__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__XListLiteral__Group_3_1__1__Impl_in_rule__XListLiteral__Group_3_1__158544);
rule__XListLiteral__Group_3_1__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XListLiteral__Group_3_1__1"
// $ANTLR start "rule__XListLiteral__Group_3_1__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29010:1: rule__XListLiteral__Group_3_1__1__Impl : ( ( rule__XListLiteral__ElementsAssignment_3_1_1 ) ) ;
public final void rule__XListLiteral__Group_3_1__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29014:1: ( ( ( rule__XListLiteral__ElementsAssignment_3_1_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29015:1: ( ( rule__XListLiteral__ElementsAssignment_3_1_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29015:1: ( ( rule__XListLiteral__ElementsAssignment_3_1_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29016:1: ( rule__XListLiteral__ElementsAssignment_3_1_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXListLiteralAccess().getElementsAssignment_3_1_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29017:1: ( rule__XListLiteral__ElementsAssignment_3_1_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29017:2: rule__XListLiteral__ElementsAssignment_3_1_1
{
pushFollow(FollowSets002.FOLLOW_rule__XListLiteral__ElementsAssignment_3_1_1_in_rule__XListLiteral__Group_3_1__1__Impl58571);
rule__XListLiteral__ElementsAssignment_3_1_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXListLiteralAccess().getElementsAssignment_3_1_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XListLiteral__Group_3_1__1__Impl"
// $ANTLR start "rule__XClosure__Group__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29031:1: rule__XClosure__Group__0 : rule__XClosure__Group__0__Impl rule__XClosure__Group__1 ;
public final void rule__XClosure__Group__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29035:1: ( rule__XClosure__Group__0__Impl rule__XClosure__Group__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29036:2: rule__XClosure__Group__0__Impl rule__XClosure__Group__1
{
pushFollow(FollowSets002.FOLLOW_rule__XClosure__Group__0__Impl_in_rule__XClosure__Group__058605);
rule__XClosure__Group__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XClosure__Group__1_in_rule__XClosure__Group__058608);
rule__XClosure__Group__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XClosure__Group__0"
// $ANTLR start "rule__XClosure__Group__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29043:1: rule__XClosure__Group__0__Impl : ( ( rule__XClosure__Group_0__0 ) ) ;
public final void rule__XClosure__Group__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29047:1: ( ( ( rule__XClosure__Group_0__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29048:1: ( ( rule__XClosure__Group_0__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29048:1: ( ( rule__XClosure__Group_0__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29049:1: ( rule__XClosure__Group_0__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXClosureAccess().getGroup_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29050:1: ( rule__XClosure__Group_0__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29050:2: rule__XClosure__Group_0__0
{
pushFollow(FollowSets002.FOLLOW_rule__XClosure__Group_0__0_in_rule__XClosure__Group__0__Impl58635);
rule__XClosure__Group_0__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXClosureAccess().getGroup_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XClosure__Group__0__Impl"
// $ANTLR start "rule__XClosure__Group__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29060:1: rule__XClosure__Group__1 : rule__XClosure__Group__1__Impl rule__XClosure__Group__2 ;
public final void rule__XClosure__Group__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29064:1: ( rule__XClosure__Group__1__Impl rule__XClosure__Group__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29065:2: rule__XClosure__Group__1__Impl rule__XClosure__Group__2
{
pushFollow(FollowSets002.FOLLOW_rule__XClosure__Group__1__Impl_in_rule__XClosure__Group__158665);
rule__XClosure__Group__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XClosure__Group__2_in_rule__XClosure__Group__158668);
rule__XClosure__Group__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XClosure__Group__1"
// $ANTLR start "rule__XClosure__Group__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29072:1: rule__XClosure__Group__1__Impl : ( ( rule__XClosure__Group_1__0 )? ) ;
public final void rule__XClosure__Group__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29076:1: ( ( ( rule__XClosure__Group_1__0 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29077:1: ( ( rule__XClosure__Group_1__0 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29077:1: ( ( rule__XClosure__Group_1__0 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29078:1: ( rule__XClosure__Group_1__0 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXClosureAccess().getGroup_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29079:1: ( rule__XClosure__Group_1__0 )?
int alt218=2;
alt218 = dfa218.predict(input);
switch (alt218) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29079:2: rule__XClosure__Group_1__0
{
pushFollow(FollowSets002.FOLLOW_rule__XClosure__Group_1__0_in_rule__XClosure__Group__1__Impl58695);
rule__XClosure__Group_1__0();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXClosureAccess().getGroup_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XClosure__Group__1__Impl"
// $ANTLR start "rule__XClosure__Group__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29089:1: rule__XClosure__Group__2 : rule__XClosure__Group__2__Impl rule__XClosure__Group__3 ;
public final void rule__XClosure__Group__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29093:1: ( rule__XClosure__Group__2__Impl rule__XClosure__Group__3 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29094:2: rule__XClosure__Group__2__Impl rule__XClosure__Group__3
{
pushFollow(FollowSets002.FOLLOW_rule__XClosure__Group__2__Impl_in_rule__XClosure__Group__258726);
rule__XClosure__Group__2__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XClosure__Group__3_in_rule__XClosure__Group__258729);
rule__XClosure__Group__3();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XClosure__Group__2"
// $ANTLR start "rule__XClosure__Group__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29101:1: rule__XClosure__Group__2__Impl : ( ( rule__XClosure__ExpressionAssignment_2 ) ) ;
public final void rule__XClosure__Group__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29105:1: ( ( ( rule__XClosure__ExpressionAssignment_2 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29106:1: ( ( rule__XClosure__ExpressionAssignment_2 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29106:1: ( ( rule__XClosure__ExpressionAssignment_2 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29107:1: ( rule__XClosure__ExpressionAssignment_2 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXClosureAccess().getExpressionAssignment_2());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29108:1: ( rule__XClosure__ExpressionAssignment_2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29108:2: rule__XClosure__ExpressionAssignment_2
{
pushFollow(FollowSets002.FOLLOW_rule__XClosure__ExpressionAssignment_2_in_rule__XClosure__Group__2__Impl58756);
rule__XClosure__ExpressionAssignment_2();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXClosureAccess().getExpressionAssignment_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XClosure__Group__2__Impl"
// $ANTLR start "rule__XClosure__Group__3"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29118:1: rule__XClosure__Group__3 : rule__XClosure__Group__3__Impl ;
public final void rule__XClosure__Group__3() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29122:1: ( rule__XClosure__Group__3__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29123:2: rule__XClosure__Group__3__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__XClosure__Group__3__Impl_in_rule__XClosure__Group__358786);
rule__XClosure__Group__3__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XClosure__Group__3"
// $ANTLR start "rule__XClosure__Group__3__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29129:1: rule__XClosure__Group__3__Impl : ( ']' ) ;
public final void rule__XClosure__Group__3__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29133:1: ( ( ']' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29134:1: ( ']' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29134:1: ( ']' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29135:1: ']'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXClosureAccess().getRightSquareBracketKeyword_3());
}
match(input,157,FollowSets002.FOLLOW_157_in_rule__XClosure__Group__3__Impl58814); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXClosureAccess().getRightSquareBracketKeyword_3());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XClosure__Group__3__Impl"
// $ANTLR start "rule__XClosure__Group_0__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29156:1: rule__XClosure__Group_0__0 : rule__XClosure__Group_0__0__Impl ;
public final void rule__XClosure__Group_0__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29160:1: ( rule__XClosure__Group_0__0__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29161:2: rule__XClosure__Group_0__0__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__XClosure__Group_0__0__Impl_in_rule__XClosure__Group_0__058853);
rule__XClosure__Group_0__0__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XClosure__Group_0__0"
// $ANTLR start "rule__XClosure__Group_0__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29167:1: rule__XClosure__Group_0__0__Impl : ( ( rule__XClosure__Group_0_0__0 ) ) ;
public final void rule__XClosure__Group_0__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29171:1: ( ( ( rule__XClosure__Group_0_0__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29172:1: ( ( rule__XClosure__Group_0_0__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29172:1: ( ( rule__XClosure__Group_0_0__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29173:1: ( rule__XClosure__Group_0_0__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXClosureAccess().getGroup_0_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29174:1: ( rule__XClosure__Group_0_0__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29174:2: rule__XClosure__Group_0_0__0
{
pushFollow(FollowSets002.FOLLOW_rule__XClosure__Group_0_0__0_in_rule__XClosure__Group_0__0__Impl58880);
rule__XClosure__Group_0_0__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXClosureAccess().getGroup_0_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XClosure__Group_0__0__Impl"
// $ANTLR start "rule__XClosure__Group_0_0__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29186:1: rule__XClosure__Group_0_0__0 : rule__XClosure__Group_0_0__0__Impl rule__XClosure__Group_0_0__1 ;
public final void rule__XClosure__Group_0_0__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29190:1: ( rule__XClosure__Group_0_0__0__Impl rule__XClosure__Group_0_0__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29191:2: rule__XClosure__Group_0_0__0__Impl rule__XClosure__Group_0_0__1
{
pushFollow(FollowSets002.FOLLOW_rule__XClosure__Group_0_0__0__Impl_in_rule__XClosure__Group_0_0__058912);
rule__XClosure__Group_0_0__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XClosure__Group_0_0__1_in_rule__XClosure__Group_0_0__058915);
rule__XClosure__Group_0_0__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XClosure__Group_0_0__0"
// $ANTLR start "rule__XClosure__Group_0_0__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29198:1: rule__XClosure__Group_0_0__0__Impl : ( () ) ;
public final void rule__XClosure__Group_0_0__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29202:1: ( ( () ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29203:1: ( () )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29203:1: ( () )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29204:1: ()
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXClosureAccess().getXClosureAction_0_0_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29205:1: ()
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29207:1:
{
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXClosureAccess().getXClosureAction_0_0_0());
}
}
}
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XClosure__Group_0_0__0__Impl"
// $ANTLR start "rule__XClosure__Group_0_0__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29217:1: rule__XClosure__Group_0_0__1 : rule__XClosure__Group_0_0__1__Impl ;
public final void rule__XClosure__Group_0_0__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29221:1: ( rule__XClosure__Group_0_0__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29222:2: rule__XClosure__Group_0_0__1__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__XClosure__Group_0_0__1__Impl_in_rule__XClosure__Group_0_0__158973);
rule__XClosure__Group_0_0__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XClosure__Group_0_0__1"
// $ANTLR start "rule__XClosure__Group_0_0__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29228:1: rule__XClosure__Group_0_0__1__Impl : ( '[' ) ;
public final void rule__XClosure__Group_0_0__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29232:1: ( ( '[' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29233:1: ( '[' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29233:1: ( '[' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29234:1: '['
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXClosureAccess().getLeftSquareBracketKeyword_0_0_1());
}
match(input,156,FollowSets002.FOLLOW_156_in_rule__XClosure__Group_0_0__1__Impl59001); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXClosureAccess().getLeftSquareBracketKeyword_0_0_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XClosure__Group_0_0__1__Impl"
// $ANTLR start "rule__XClosure__Group_1__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29251:1: rule__XClosure__Group_1__0 : rule__XClosure__Group_1__0__Impl ;
public final void rule__XClosure__Group_1__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29255:1: ( rule__XClosure__Group_1__0__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29256:2: rule__XClosure__Group_1__0__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__XClosure__Group_1__0__Impl_in_rule__XClosure__Group_1__059036);
rule__XClosure__Group_1__0__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XClosure__Group_1__0"
// $ANTLR start "rule__XClosure__Group_1__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29262:1: rule__XClosure__Group_1__0__Impl : ( ( rule__XClosure__Group_1_0__0 ) ) ;
public final void rule__XClosure__Group_1__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29266:1: ( ( ( rule__XClosure__Group_1_0__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29267:1: ( ( rule__XClosure__Group_1_0__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29267:1: ( ( rule__XClosure__Group_1_0__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29268:1: ( rule__XClosure__Group_1_0__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXClosureAccess().getGroup_1_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29269:1: ( rule__XClosure__Group_1_0__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29269:2: rule__XClosure__Group_1_0__0
{
pushFollow(FollowSets002.FOLLOW_rule__XClosure__Group_1_0__0_in_rule__XClosure__Group_1__0__Impl59063);
rule__XClosure__Group_1_0__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXClosureAccess().getGroup_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XClosure__Group_1__0__Impl"
// $ANTLR start "rule__XClosure__Group_1_0__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29281:1: rule__XClosure__Group_1_0__0 : rule__XClosure__Group_1_0__0__Impl rule__XClosure__Group_1_0__1 ;
public final void rule__XClosure__Group_1_0__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29285:1: ( rule__XClosure__Group_1_0__0__Impl rule__XClosure__Group_1_0__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29286:2: rule__XClosure__Group_1_0__0__Impl rule__XClosure__Group_1_0__1
{
pushFollow(FollowSets002.FOLLOW_rule__XClosure__Group_1_0__0__Impl_in_rule__XClosure__Group_1_0__059095);
rule__XClosure__Group_1_0__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XClosure__Group_1_0__1_in_rule__XClosure__Group_1_0__059098);
rule__XClosure__Group_1_0__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XClosure__Group_1_0__0"
// $ANTLR start "rule__XClosure__Group_1_0__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29293:1: rule__XClosure__Group_1_0__0__Impl : ( ( rule__XClosure__Group_1_0_0__0 )? ) ;
public final void rule__XClosure__Group_1_0__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29297:1: ( ( ( rule__XClosure__Group_1_0_0__0 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29298:1: ( ( rule__XClosure__Group_1_0_0__0 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29298:1: ( ( rule__XClosure__Group_1_0_0__0 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29299:1: ( rule__XClosure__Group_1_0_0__0 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXClosureAccess().getGroup_1_0_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29300:1: ( rule__XClosure__Group_1_0_0__0 )?
int alt219=2;
int LA219_0 = input.LA(1);
if ( (LA219_0==RULE_ID||LA219_0==31||LA219_0==144) ) {
alt219=1;
}
switch (alt219) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29300:2: rule__XClosure__Group_1_0_0__0
{
pushFollow(FollowSets002.FOLLOW_rule__XClosure__Group_1_0_0__0_in_rule__XClosure__Group_1_0__0__Impl59125);
rule__XClosure__Group_1_0_0__0();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXClosureAccess().getGroup_1_0_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XClosure__Group_1_0__0__Impl"
// $ANTLR start "rule__XClosure__Group_1_0__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29310:1: rule__XClosure__Group_1_0__1 : rule__XClosure__Group_1_0__1__Impl ;
public final void rule__XClosure__Group_1_0__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29314:1: ( rule__XClosure__Group_1_0__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29315:2: rule__XClosure__Group_1_0__1__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__XClosure__Group_1_0__1__Impl_in_rule__XClosure__Group_1_0__159156);
rule__XClosure__Group_1_0__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XClosure__Group_1_0__1"
// $ANTLR start "rule__XClosure__Group_1_0__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29321:1: rule__XClosure__Group_1_0__1__Impl : ( ( rule__XClosure__ExplicitSyntaxAssignment_1_0_1 ) ) ;
public final void rule__XClosure__Group_1_0__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29325:1: ( ( ( rule__XClosure__ExplicitSyntaxAssignment_1_0_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29326:1: ( ( rule__XClosure__ExplicitSyntaxAssignment_1_0_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29326:1: ( ( rule__XClosure__ExplicitSyntaxAssignment_1_0_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29327:1: ( rule__XClosure__ExplicitSyntaxAssignment_1_0_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXClosureAccess().getExplicitSyntaxAssignment_1_0_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29328:1: ( rule__XClosure__ExplicitSyntaxAssignment_1_0_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29328:2: rule__XClosure__ExplicitSyntaxAssignment_1_0_1
{
pushFollow(FollowSets002.FOLLOW_rule__XClosure__ExplicitSyntaxAssignment_1_0_1_in_rule__XClosure__Group_1_0__1__Impl59183);
rule__XClosure__ExplicitSyntaxAssignment_1_0_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXClosureAccess().getExplicitSyntaxAssignment_1_0_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XClosure__Group_1_0__1__Impl"
// $ANTLR start "rule__XClosure__Group_1_0_0__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29342:1: rule__XClosure__Group_1_0_0__0 : rule__XClosure__Group_1_0_0__0__Impl rule__XClosure__Group_1_0_0__1 ;
public final void rule__XClosure__Group_1_0_0__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29346:1: ( rule__XClosure__Group_1_0_0__0__Impl rule__XClosure__Group_1_0_0__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29347:2: rule__XClosure__Group_1_0_0__0__Impl rule__XClosure__Group_1_0_0__1
{
pushFollow(FollowSets002.FOLLOW_rule__XClosure__Group_1_0_0__0__Impl_in_rule__XClosure__Group_1_0_0__059217);
rule__XClosure__Group_1_0_0__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XClosure__Group_1_0_0__1_in_rule__XClosure__Group_1_0_0__059220);
rule__XClosure__Group_1_0_0__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XClosure__Group_1_0_0__0"
// $ANTLR start "rule__XClosure__Group_1_0_0__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29354:1: rule__XClosure__Group_1_0_0__0__Impl : ( ( rule__XClosure__DeclaredFormalParametersAssignment_1_0_0_0 ) ) ;
public final void rule__XClosure__Group_1_0_0__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29358:1: ( ( ( rule__XClosure__DeclaredFormalParametersAssignment_1_0_0_0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29359:1: ( ( rule__XClosure__DeclaredFormalParametersAssignment_1_0_0_0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29359:1: ( ( rule__XClosure__DeclaredFormalParametersAssignment_1_0_0_0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29360:1: ( rule__XClosure__DeclaredFormalParametersAssignment_1_0_0_0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXClosureAccess().getDeclaredFormalParametersAssignment_1_0_0_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29361:1: ( rule__XClosure__DeclaredFormalParametersAssignment_1_0_0_0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29361:2: rule__XClosure__DeclaredFormalParametersAssignment_1_0_0_0
{
pushFollow(FollowSets002.FOLLOW_rule__XClosure__DeclaredFormalParametersAssignment_1_0_0_0_in_rule__XClosure__Group_1_0_0__0__Impl59247);
rule__XClosure__DeclaredFormalParametersAssignment_1_0_0_0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXClosureAccess().getDeclaredFormalParametersAssignment_1_0_0_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XClosure__Group_1_0_0__0__Impl"
// $ANTLR start "rule__XClosure__Group_1_0_0__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29371:1: rule__XClosure__Group_1_0_0__1 : rule__XClosure__Group_1_0_0__1__Impl ;
public final void rule__XClosure__Group_1_0_0__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29375:1: ( rule__XClosure__Group_1_0_0__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29376:2: rule__XClosure__Group_1_0_0__1__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__XClosure__Group_1_0_0__1__Impl_in_rule__XClosure__Group_1_0_0__159277);
rule__XClosure__Group_1_0_0__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XClosure__Group_1_0_0__1"
// $ANTLR start "rule__XClosure__Group_1_0_0__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29382:1: rule__XClosure__Group_1_0_0__1__Impl : ( ( rule__XClosure__Group_1_0_0_1__0 )* ) ;
public final void rule__XClosure__Group_1_0_0__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29386:1: ( ( ( rule__XClosure__Group_1_0_0_1__0 )* ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29387:1: ( ( rule__XClosure__Group_1_0_0_1__0 )* )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29387:1: ( ( rule__XClosure__Group_1_0_0_1__0 )* )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29388:1: ( rule__XClosure__Group_1_0_0_1__0 )*
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXClosureAccess().getGroup_1_0_0_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29389:1: ( rule__XClosure__Group_1_0_0_1__0 )*
loop220:
do {
int alt220=2;
int LA220_0 = input.LA(1);
if ( (LA220_0==154) ) {
alt220=1;
}
switch (alt220) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29389:2: rule__XClosure__Group_1_0_0_1__0
{
pushFollow(FollowSets002.FOLLOW_rule__XClosure__Group_1_0_0_1__0_in_rule__XClosure__Group_1_0_0__1__Impl59304);
rule__XClosure__Group_1_0_0_1__0();
state._fsp--;
if (state.failed) return ;
}
break;
default :
break loop220;
}
} while (true);
if ( state.backtracking==0 ) {
after(grammarAccess.getXClosureAccess().getGroup_1_0_0_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XClosure__Group_1_0_0__1__Impl"
// $ANTLR start "rule__XClosure__Group_1_0_0_1__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29403:1: rule__XClosure__Group_1_0_0_1__0 : rule__XClosure__Group_1_0_0_1__0__Impl rule__XClosure__Group_1_0_0_1__1 ;
public final void rule__XClosure__Group_1_0_0_1__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29407:1: ( rule__XClosure__Group_1_0_0_1__0__Impl rule__XClosure__Group_1_0_0_1__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29408:2: rule__XClosure__Group_1_0_0_1__0__Impl rule__XClosure__Group_1_0_0_1__1
{
pushFollow(FollowSets002.FOLLOW_rule__XClosure__Group_1_0_0_1__0__Impl_in_rule__XClosure__Group_1_0_0_1__059339);
rule__XClosure__Group_1_0_0_1__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XClosure__Group_1_0_0_1__1_in_rule__XClosure__Group_1_0_0_1__059342);
rule__XClosure__Group_1_0_0_1__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XClosure__Group_1_0_0_1__0"
// $ANTLR start "rule__XClosure__Group_1_0_0_1__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29415:1: rule__XClosure__Group_1_0_0_1__0__Impl : ( ',' ) ;
public final void rule__XClosure__Group_1_0_0_1__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29419:1: ( ( ',' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29420:1: ( ',' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29420:1: ( ',' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29421:1: ','
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXClosureAccess().getCommaKeyword_1_0_0_1_0());
}
match(input,154,FollowSets002.FOLLOW_154_in_rule__XClosure__Group_1_0_0_1__0__Impl59370); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXClosureAccess().getCommaKeyword_1_0_0_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XClosure__Group_1_0_0_1__0__Impl"
// $ANTLR start "rule__XClosure__Group_1_0_0_1__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29434:1: rule__XClosure__Group_1_0_0_1__1 : rule__XClosure__Group_1_0_0_1__1__Impl ;
public final void rule__XClosure__Group_1_0_0_1__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29438:1: ( rule__XClosure__Group_1_0_0_1__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29439:2: rule__XClosure__Group_1_0_0_1__1__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__XClosure__Group_1_0_0_1__1__Impl_in_rule__XClosure__Group_1_0_0_1__159401);
rule__XClosure__Group_1_0_0_1__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XClosure__Group_1_0_0_1__1"
// $ANTLR start "rule__XClosure__Group_1_0_0_1__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29445:1: rule__XClosure__Group_1_0_0_1__1__Impl : ( ( rule__XClosure__DeclaredFormalParametersAssignment_1_0_0_1_1 ) ) ;
public final void rule__XClosure__Group_1_0_0_1__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29449:1: ( ( ( rule__XClosure__DeclaredFormalParametersAssignment_1_0_0_1_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29450:1: ( ( rule__XClosure__DeclaredFormalParametersAssignment_1_0_0_1_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29450:1: ( ( rule__XClosure__DeclaredFormalParametersAssignment_1_0_0_1_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29451:1: ( rule__XClosure__DeclaredFormalParametersAssignment_1_0_0_1_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXClosureAccess().getDeclaredFormalParametersAssignment_1_0_0_1_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29452:1: ( rule__XClosure__DeclaredFormalParametersAssignment_1_0_0_1_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29452:2: rule__XClosure__DeclaredFormalParametersAssignment_1_0_0_1_1
{
pushFollow(FollowSets002.FOLLOW_rule__XClosure__DeclaredFormalParametersAssignment_1_0_0_1_1_in_rule__XClosure__Group_1_0_0_1__1__Impl59428);
rule__XClosure__DeclaredFormalParametersAssignment_1_0_0_1_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXClosureAccess().getDeclaredFormalParametersAssignment_1_0_0_1_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XClosure__Group_1_0_0_1__1__Impl"
// $ANTLR start "rule__XExpressionInClosure__Group__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29466:1: rule__XExpressionInClosure__Group__0 : rule__XExpressionInClosure__Group__0__Impl rule__XExpressionInClosure__Group__1 ;
public final void rule__XExpressionInClosure__Group__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29470:1: ( rule__XExpressionInClosure__Group__0__Impl rule__XExpressionInClosure__Group__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29471:2: rule__XExpressionInClosure__Group__0__Impl rule__XExpressionInClosure__Group__1
{
pushFollow(FollowSets002.FOLLOW_rule__XExpressionInClosure__Group__0__Impl_in_rule__XExpressionInClosure__Group__059462);
rule__XExpressionInClosure__Group__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XExpressionInClosure__Group__1_in_rule__XExpressionInClosure__Group__059465);
rule__XExpressionInClosure__Group__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XExpressionInClosure__Group__0"
// $ANTLR start "rule__XExpressionInClosure__Group__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29478:1: rule__XExpressionInClosure__Group__0__Impl : ( () ) ;
public final void rule__XExpressionInClosure__Group__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29482:1: ( ( () ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29483:1: ( () )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29483:1: ( () )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29484:1: ()
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXExpressionInClosureAccess().getXBlockExpressionAction_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29485:1: ()
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29487:1:
{
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXExpressionInClosureAccess().getXBlockExpressionAction_0());
}
}
}
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XExpressionInClosure__Group__0__Impl"
// $ANTLR start "rule__XExpressionInClosure__Group__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29497:1: rule__XExpressionInClosure__Group__1 : rule__XExpressionInClosure__Group__1__Impl ;
public final void rule__XExpressionInClosure__Group__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29501:1: ( rule__XExpressionInClosure__Group__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29502:2: rule__XExpressionInClosure__Group__1__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__XExpressionInClosure__Group__1__Impl_in_rule__XExpressionInClosure__Group__159523);
rule__XExpressionInClosure__Group__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XExpressionInClosure__Group__1"
// $ANTLR start "rule__XExpressionInClosure__Group__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29508:1: rule__XExpressionInClosure__Group__1__Impl : ( ( rule__XExpressionInClosure__Group_1__0 )* ) ;
public final void rule__XExpressionInClosure__Group__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29512:1: ( ( ( rule__XExpressionInClosure__Group_1__0 )* ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29513:1: ( ( rule__XExpressionInClosure__Group_1__0 )* )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29513:1: ( ( rule__XExpressionInClosure__Group_1__0 )* )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29514:1: ( rule__XExpressionInClosure__Group_1__0 )*
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXExpressionInClosureAccess().getGroup_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29515:1: ( rule__XExpressionInClosure__Group_1__0 )*
loop221:
do {
int alt221=2;
int LA221_0 = input.LA(1);
if ( ((LA221_0>=RULE_ID && LA221_0<=RULE_STRING)||LA221_0==27||(LA221_0>=34 && LA221_0<=35)||LA221_0==40||(LA221_0>=44 && LA221_0<=50)||LA221_0==67||LA221_0==144||(LA221_0>=155 && LA221_0<=156)||LA221_0==159||LA221_0==161||(LA221_0>=165 && LA221_0<=173)||LA221_0==175||(LA221_0>=184 && LA221_0<=185)) ) {
alt221=1;
}
switch (alt221) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29515:2: rule__XExpressionInClosure__Group_1__0
{
pushFollow(FollowSets002.FOLLOW_rule__XExpressionInClosure__Group_1__0_in_rule__XExpressionInClosure__Group__1__Impl59550);
rule__XExpressionInClosure__Group_1__0();
state._fsp--;
if (state.failed) return ;
}
break;
default :
break loop221;
}
} while (true);
if ( state.backtracking==0 ) {
after(grammarAccess.getXExpressionInClosureAccess().getGroup_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XExpressionInClosure__Group__1__Impl"
// $ANTLR start "rule__XExpressionInClosure__Group_1__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29529:1: rule__XExpressionInClosure__Group_1__0 : rule__XExpressionInClosure__Group_1__0__Impl rule__XExpressionInClosure__Group_1__1 ;
public final void rule__XExpressionInClosure__Group_1__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29533:1: ( rule__XExpressionInClosure__Group_1__0__Impl rule__XExpressionInClosure__Group_1__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29534:2: rule__XExpressionInClosure__Group_1__0__Impl rule__XExpressionInClosure__Group_1__1
{
pushFollow(FollowSets002.FOLLOW_rule__XExpressionInClosure__Group_1__0__Impl_in_rule__XExpressionInClosure__Group_1__059585);
rule__XExpressionInClosure__Group_1__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XExpressionInClosure__Group_1__1_in_rule__XExpressionInClosure__Group_1__059588);
rule__XExpressionInClosure__Group_1__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XExpressionInClosure__Group_1__0"
// $ANTLR start "rule__XExpressionInClosure__Group_1__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29541:1: rule__XExpressionInClosure__Group_1__0__Impl : ( ( rule__XExpressionInClosure__ExpressionsAssignment_1_0 ) ) ;
public final void rule__XExpressionInClosure__Group_1__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29545:1: ( ( ( rule__XExpressionInClosure__ExpressionsAssignment_1_0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29546:1: ( ( rule__XExpressionInClosure__ExpressionsAssignment_1_0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29546:1: ( ( rule__XExpressionInClosure__ExpressionsAssignment_1_0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29547:1: ( rule__XExpressionInClosure__ExpressionsAssignment_1_0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXExpressionInClosureAccess().getExpressionsAssignment_1_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29548:1: ( rule__XExpressionInClosure__ExpressionsAssignment_1_0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29548:2: rule__XExpressionInClosure__ExpressionsAssignment_1_0
{
pushFollow(FollowSets002.FOLLOW_rule__XExpressionInClosure__ExpressionsAssignment_1_0_in_rule__XExpressionInClosure__Group_1__0__Impl59615);
rule__XExpressionInClosure__ExpressionsAssignment_1_0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXExpressionInClosureAccess().getExpressionsAssignment_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XExpressionInClosure__Group_1__0__Impl"
// $ANTLR start "rule__XExpressionInClosure__Group_1__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29558:1: rule__XExpressionInClosure__Group_1__1 : rule__XExpressionInClosure__Group_1__1__Impl ;
public final void rule__XExpressionInClosure__Group_1__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29562:1: ( rule__XExpressionInClosure__Group_1__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29563:2: rule__XExpressionInClosure__Group_1__1__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__XExpressionInClosure__Group_1__1__Impl_in_rule__XExpressionInClosure__Group_1__159645);
rule__XExpressionInClosure__Group_1__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XExpressionInClosure__Group_1__1"
// $ANTLR start "rule__XExpressionInClosure__Group_1__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29569:1: rule__XExpressionInClosure__Group_1__1__Impl : ( ( ';' )? ) ;
public final void rule__XExpressionInClosure__Group_1__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29573:1: ( ( ( ';' )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29574:1: ( ( ';' )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29574:1: ( ( ';' )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29575:1: ( ';' )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXExpressionInClosureAccess().getSemicolonKeyword_1_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29576:1: ( ';' )?
int alt222=2;
int LA222_0 = input.LA(1);
if ( (LA222_0==158) ) {
alt222=1;
}
switch (alt222) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29577:2: ';'
{
match(input,158,FollowSets002.FOLLOW_158_in_rule__XExpressionInClosure__Group_1__1__Impl59674); if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXExpressionInClosureAccess().getSemicolonKeyword_1_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XExpressionInClosure__Group_1__1__Impl"
// $ANTLR start "rule__XShortClosure__Group__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29592:1: rule__XShortClosure__Group__0 : rule__XShortClosure__Group__0__Impl rule__XShortClosure__Group__1 ;
public final void rule__XShortClosure__Group__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29596:1: ( rule__XShortClosure__Group__0__Impl rule__XShortClosure__Group__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29597:2: rule__XShortClosure__Group__0__Impl rule__XShortClosure__Group__1
{
pushFollow(FollowSets002.FOLLOW_rule__XShortClosure__Group__0__Impl_in_rule__XShortClosure__Group__059711);
rule__XShortClosure__Group__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XShortClosure__Group__1_in_rule__XShortClosure__Group__059714);
rule__XShortClosure__Group__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XShortClosure__Group__0"
// $ANTLR start "rule__XShortClosure__Group__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29604:1: rule__XShortClosure__Group__0__Impl : ( ( rule__XShortClosure__Group_0__0 ) ) ;
public final void rule__XShortClosure__Group__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29608:1: ( ( ( rule__XShortClosure__Group_0__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29609:1: ( ( rule__XShortClosure__Group_0__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29609:1: ( ( rule__XShortClosure__Group_0__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29610:1: ( rule__XShortClosure__Group_0__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXShortClosureAccess().getGroup_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29611:1: ( rule__XShortClosure__Group_0__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29611:2: rule__XShortClosure__Group_0__0
{
pushFollow(FollowSets002.FOLLOW_rule__XShortClosure__Group_0__0_in_rule__XShortClosure__Group__0__Impl59741);
rule__XShortClosure__Group_0__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXShortClosureAccess().getGroup_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XShortClosure__Group__0__Impl"
// $ANTLR start "rule__XShortClosure__Group__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29621:1: rule__XShortClosure__Group__1 : rule__XShortClosure__Group__1__Impl ;
public final void rule__XShortClosure__Group__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29625:1: ( rule__XShortClosure__Group__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29626:2: rule__XShortClosure__Group__1__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__XShortClosure__Group__1__Impl_in_rule__XShortClosure__Group__159771);
rule__XShortClosure__Group__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XShortClosure__Group__1"
// $ANTLR start "rule__XShortClosure__Group__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29632:1: rule__XShortClosure__Group__1__Impl : ( ( rule__XShortClosure__ExpressionAssignment_1 ) ) ;
public final void rule__XShortClosure__Group__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29636:1: ( ( ( rule__XShortClosure__ExpressionAssignment_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29637:1: ( ( rule__XShortClosure__ExpressionAssignment_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29637:1: ( ( rule__XShortClosure__ExpressionAssignment_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29638:1: ( rule__XShortClosure__ExpressionAssignment_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXShortClosureAccess().getExpressionAssignment_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29639:1: ( rule__XShortClosure__ExpressionAssignment_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29639:2: rule__XShortClosure__ExpressionAssignment_1
{
pushFollow(FollowSets002.FOLLOW_rule__XShortClosure__ExpressionAssignment_1_in_rule__XShortClosure__Group__1__Impl59798);
rule__XShortClosure__ExpressionAssignment_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXShortClosureAccess().getExpressionAssignment_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XShortClosure__Group__1__Impl"
// $ANTLR start "rule__XShortClosure__Group_0__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29653:1: rule__XShortClosure__Group_0__0 : rule__XShortClosure__Group_0__0__Impl ;
public final void rule__XShortClosure__Group_0__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29657:1: ( rule__XShortClosure__Group_0__0__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29658:2: rule__XShortClosure__Group_0__0__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__XShortClosure__Group_0__0__Impl_in_rule__XShortClosure__Group_0__059832);
rule__XShortClosure__Group_0__0__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XShortClosure__Group_0__0"
// $ANTLR start "rule__XShortClosure__Group_0__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29664:1: rule__XShortClosure__Group_0__0__Impl : ( ( rule__XShortClosure__Group_0_0__0 ) ) ;
public final void rule__XShortClosure__Group_0__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29668:1: ( ( ( rule__XShortClosure__Group_0_0__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29669:1: ( ( rule__XShortClosure__Group_0_0__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29669:1: ( ( rule__XShortClosure__Group_0_0__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29670:1: ( rule__XShortClosure__Group_0_0__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXShortClosureAccess().getGroup_0_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29671:1: ( rule__XShortClosure__Group_0_0__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29671:2: rule__XShortClosure__Group_0_0__0
{
pushFollow(FollowSets002.FOLLOW_rule__XShortClosure__Group_0_0__0_in_rule__XShortClosure__Group_0__0__Impl59859);
rule__XShortClosure__Group_0_0__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXShortClosureAccess().getGroup_0_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XShortClosure__Group_0__0__Impl"
// $ANTLR start "rule__XShortClosure__Group_0_0__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29683:1: rule__XShortClosure__Group_0_0__0 : rule__XShortClosure__Group_0_0__0__Impl rule__XShortClosure__Group_0_0__1 ;
public final void rule__XShortClosure__Group_0_0__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29687:1: ( rule__XShortClosure__Group_0_0__0__Impl rule__XShortClosure__Group_0_0__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29688:2: rule__XShortClosure__Group_0_0__0__Impl rule__XShortClosure__Group_0_0__1
{
pushFollow(FollowSets002.FOLLOW_rule__XShortClosure__Group_0_0__0__Impl_in_rule__XShortClosure__Group_0_0__059891);
rule__XShortClosure__Group_0_0__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XShortClosure__Group_0_0__1_in_rule__XShortClosure__Group_0_0__059894);
rule__XShortClosure__Group_0_0__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XShortClosure__Group_0_0__0"
// $ANTLR start "rule__XShortClosure__Group_0_0__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29695:1: rule__XShortClosure__Group_0_0__0__Impl : ( () ) ;
public final void rule__XShortClosure__Group_0_0__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29699:1: ( ( () ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29700:1: ( () )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29700:1: ( () )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29701:1: ()
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXShortClosureAccess().getXClosureAction_0_0_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29702:1: ()
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29704:1:
{
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXShortClosureAccess().getXClosureAction_0_0_0());
}
}
}
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XShortClosure__Group_0_0__0__Impl"
// $ANTLR start "rule__XShortClosure__Group_0_0__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29714:1: rule__XShortClosure__Group_0_0__1 : rule__XShortClosure__Group_0_0__1__Impl rule__XShortClosure__Group_0_0__2 ;
public final void rule__XShortClosure__Group_0_0__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29718:1: ( rule__XShortClosure__Group_0_0__1__Impl rule__XShortClosure__Group_0_0__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29719:2: rule__XShortClosure__Group_0_0__1__Impl rule__XShortClosure__Group_0_0__2
{
pushFollow(FollowSets002.FOLLOW_rule__XShortClosure__Group_0_0__1__Impl_in_rule__XShortClosure__Group_0_0__159952);
rule__XShortClosure__Group_0_0__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XShortClosure__Group_0_0__2_in_rule__XShortClosure__Group_0_0__159955);
rule__XShortClosure__Group_0_0__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XShortClosure__Group_0_0__1"
// $ANTLR start "rule__XShortClosure__Group_0_0__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29726:1: rule__XShortClosure__Group_0_0__1__Impl : ( ( rule__XShortClosure__Group_0_0_1__0 )? ) ;
public final void rule__XShortClosure__Group_0_0__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29730:1: ( ( ( rule__XShortClosure__Group_0_0_1__0 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29731:1: ( ( rule__XShortClosure__Group_0_0_1__0 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29731:1: ( ( rule__XShortClosure__Group_0_0_1__0 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29732:1: ( rule__XShortClosure__Group_0_0_1__0 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXShortClosureAccess().getGroup_0_0_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29733:1: ( rule__XShortClosure__Group_0_0_1__0 )?
int alt223=2;
int LA223_0 = input.LA(1);
if ( (LA223_0==RULE_ID||LA223_0==31||LA223_0==144) ) {
alt223=1;
}
switch (alt223) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29733:2: rule__XShortClosure__Group_0_0_1__0
{
pushFollow(FollowSets002.FOLLOW_rule__XShortClosure__Group_0_0_1__0_in_rule__XShortClosure__Group_0_0__1__Impl59982);
rule__XShortClosure__Group_0_0_1__0();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXShortClosureAccess().getGroup_0_0_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XShortClosure__Group_0_0__1__Impl"
// $ANTLR start "rule__XShortClosure__Group_0_0__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29743:1: rule__XShortClosure__Group_0_0__2 : rule__XShortClosure__Group_0_0__2__Impl ;
public final void rule__XShortClosure__Group_0_0__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29747:1: ( rule__XShortClosure__Group_0_0__2__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29748:2: rule__XShortClosure__Group_0_0__2__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__XShortClosure__Group_0_0__2__Impl_in_rule__XShortClosure__Group_0_0__260013);
rule__XShortClosure__Group_0_0__2__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XShortClosure__Group_0_0__2"
// $ANTLR start "rule__XShortClosure__Group_0_0__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29754:1: rule__XShortClosure__Group_0_0__2__Impl : ( ( rule__XShortClosure__ExplicitSyntaxAssignment_0_0_2 ) ) ;
public final void rule__XShortClosure__Group_0_0__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29758:1: ( ( ( rule__XShortClosure__ExplicitSyntaxAssignment_0_0_2 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29759:1: ( ( rule__XShortClosure__ExplicitSyntaxAssignment_0_0_2 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29759:1: ( ( rule__XShortClosure__ExplicitSyntaxAssignment_0_0_2 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29760:1: ( rule__XShortClosure__ExplicitSyntaxAssignment_0_0_2 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXShortClosureAccess().getExplicitSyntaxAssignment_0_0_2());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29761:1: ( rule__XShortClosure__ExplicitSyntaxAssignment_0_0_2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29761:2: rule__XShortClosure__ExplicitSyntaxAssignment_0_0_2
{
pushFollow(FollowSets002.FOLLOW_rule__XShortClosure__ExplicitSyntaxAssignment_0_0_2_in_rule__XShortClosure__Group_0_0__2__Impl60040);
rule__XShortClosure__ExplicitSyntaxAssignment_0_0_2();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXShortClosureAccess().getExplicitSyntaxAssignment_0_0_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XShortClosure__Group_0_0__2__Impl"
// $ANTLR start "rule__XShortClosure__Group_0_0_1__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29777:1: rule__XShortClosure__Group_0_0_1__0 : rule__XShortClosure__Group_0_0_1__0__Impl rule__XShortClosure__Group_0_0_1__1 ;
public final void rule__XShortClosure__Group_0_0_1__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29781:1: ( rule__XShortClosure__Group_0_0_1__0__Impl rule__XShortClosure__Group_0_0_1__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29782:2: rule__XShortClosure__Group_0_0_1__0__Impl rule__XShortClosure__Group_0_0_1__1
{
pushFollow(FollowSets002.FOLLOW_rule__XShortClosure__Group_0_0_1__0__Impl_in_rule__XShortClosure__Group_0_0_1__060076);
rule__XShortClosure__Group_0_0_1__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XShortClosure__Group_0_0_1__1_in_rule__XShortClosure__Group_0_0_1__060079);
rule__XShortClosure__Group_0_0_1__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XShortClosure__Group_0_0_1__0"
// $ANTLR start "rule__XShortClosure__Group_0_0_1__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29789:1: rule__XShortClosure__Group_0_0_1__0__Impl : ( ( rule__XShortClosure__DeclaredFormalParametersAssignment_0_0_1_0 ) ) ;
public final void rule__XShortClosure__Group_0_0_1__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29793:1: ( ( ( rule__XShortClosure__DeclaredFormalParametersAssignment_0_0_1_0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29794:1: ( ( rule__XShortClosure__DeclaredFormalParametersAssignment_0_0_1_0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29794:1: ( ( rule__XShortClosure__DeclaredFormalParametersAssignment_0_0_1_0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29795:1: ( rule__XShortClosure__DeclaredFormalParametersAssignment_0_0_1_0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXShortClosureAccess().getDeclaredFormalParametersAssignment_0_0_1_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29796:1: ( rule__XShortClosure__DeclaredFormalParametersAssignment_0_0_1_0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29796:2: rule__XShortClosure__DeclaredFormalParametersAssignment_0_0_1_0
{
pushFollow(FollowSets002.FOLLOW_rule__XShortClosure__DeclaredFormalParametersAssignment_0_0_1_0_in_rule__XShortClosure__Group_0_0_1__0__Impl60106);
rule__XShortClosure__DeclaredFormalParametersAssignment_0_0_1_0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXShortClosureAccess().getDeclaredFormalParametersAssignment_0_0_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XShortClosure__Group_0_0_1__0__Impl"
// $ANTLR start "rule__XShortClosure__Group_0_0_1__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29806:1: rule__XShortClosure__Group_0_0_1__1 : rule__XShortClosure__Group_0_0_1__1__Impl ;
public final void rule__XShortClosure__Group_0_0_1__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29810:1: ( rule__XShortClosure__Group_0_0_1__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29811:2: rule__XShortClosure__Group_0_0_1__1__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__XShortClosure__Group_0_0_1__1__Impl_in_rule__XShortClosure__Group_0_0_1__160136);
rule__XShortClosure__Group_0_0_1__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XShortClosure__Group_0_0_1__1"
// $ANTLR start "rule__XShortClosure__Group_0_0_1__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29817:1: rule__XShortClosure__Group_0_0_1__1__Impl : ( ( rule__XShortClosure__Group_0_0_1_1__0 )* ) ;
public final void rule__XShortClosure__Group_0_0_1__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29821:1: ( ( ( rule__XShortClosure__Group_0_0_1_1__0 )* ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29822:1: ( ( rule__XShortClosure__Group_0_0_1_1__0 )* )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29822:1: ( ( rule__XShortClosure__Group_0_0_1_1__0 )* )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29823:1: ( rule__XShortClosure__Group_0_0_1_1__0 )*
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXShortClosureAccess().getGroup_0_0_1_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29824:1: ( rule__XShortClosure__Group_0_0_1_1__0 )*
loop224:
do {
int alt224=2;
int LA224_0 = input.LA(1);
if ( (LA224_0==154) ) {
alt224=1;
}
switch (alt224) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29824:2: rule__XShortClosure__Group_0_0_1_1__0
{
pushFollow(FollowSets002.FOLLOW_rule__XShortClosure__Group_0_0_1_1__0_in_rule__XShortClosure__Group_0_0_1__1__Impl60163);
rule__XShortClosure__Group_0_0_1_1__0();
state._fsp--;
if (state.failed) return ;
}
break;
default :
break loop224;
}
} while (true);
if ( state.backtracking==0 ) {
after(grammarAccess.getXShortClosureAccess().getGroup_0_0_1_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XShortClosure__Group_0_0_1__1__Impl"
// $ANTLR start "rule__XShortClosure__Group_0_0_1_1__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29838:1: rule__XShortClosure__Group_0_0_1_1__0 : rule__XShortClosure__Group_0_0_1_1__0__Impl rule__XShortClosure__Group_0_0_1_1__1 ;
public final void rule__XShortClosure__Group_0_0_1_1__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29842:1: ( rule__XShortClosure__Group_0_0_1_1__0__Impl rule__XShortClosure__Group_0_0_1_1__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29843:2: rule__XShortClosure__Group_0_0_1_1__0__Impl rule__XShortClosure__Group_0_0_1_1__1
{
pushFollow(FollowSets002.FOLLOW_rule__XShortClosure__Group_0_0_1_1__0__Impl_in_rule__XShortClosure__Group_0_0_1_1__060198);
rule__XShortClosure__Group_0_0_1_1__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XShortClosure__Group_0_0_1_1__1_in_rule__XShortClosure__Group_0_0_1_1__060201);
rule__XShortClosure__Group_0_0_1_1__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XShortClosure__Group_0_0_1_1__0"
// $ANTLR start "rule__XShortClosure__Group_0_0_1_1__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29850:1: rule__XShortClosure__Group_0_0_1_1__0__Impl : ( ',' ) ;
public final void rule__XShortClosure__Group_0_0_1_1__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29854:1: ( ( ',' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29855:1: ( ',' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29855:1: ( ',' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29856:1: ','
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXShortClosureAccess().getCommaKeyword_0_0_1_1_0());
}
match(input,154,FollowSets002.FOLLOW_154_in_rule__XShortClosure__Group_0_0_1_1__0__Impl60229); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXShortClosureAccess().getCommaKeyword_0_0_1_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XShortClosure__Group_0_0_1_1__0__Impl"
// $ANTLR start "rule__XShortClosure__Group_0_0_1_1__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29869:1: rule__XShortClosure__Group_0_0_1_1__1 : rule__XShortClosure__Group_0_0_1_1__1__Impl ;
public final void rule__XShortClosure__Group_0_0_1_1__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29873:1: ( rule__XShortClosure__Group_0_0_1_1__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29874:2: rule__XShortClosure__Group_0_0_1_1__1__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__XShortClosure__Group_0_0_1_1__1__Impl_in_rule__XShortClosure__Group_0_0_1_1__160260);
rule__XShortClosure__Group_0_0_1_1__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XShortClosure__Group_0_0_1_1__1"
// $ANTLR start "rule__XShortClosure__Group_0_0_1_1__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29880:1: rule__XShortClosure__Group_0_0_1_1__1__Impl : ( ( rule__XShortClosure__DeclaredFormalParametersAssignment_0_0_1_1_1 ) ) ;
public final void rule__XShortClosure__Group_0_0_1_1__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29884:1: ( ( ( rule__XShortClosure__DeclaredFormalParametersAssignment_0_0_1_1_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29885:1: ( ( rule__XShortClosure__DeclaredFormalParametersAssignment_0_0_1_1_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29885:1: ( ( rule__XShortClosure__DeclaredFormalParametersAssignment_0_0_1_1_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29886:1: ( rule__XShortClosure__DeclaredFormalParametersAssignment_0_0_1_1_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXShortClosureAccess().getDeclaredFormalParametersAssignment_0_0_1_1_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29887:1: ( rule__XShortClosure__DeclaredFormalParametersAssignment_0_0_1_1_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29887:2: rule__XShortClosure__DeclaredFormalParametersAssignment_0_0_1_1_1
{
pushFollow(FollowSets002.FOLLOW_rule__XShortClosure__DeclaredFormalParametersAssignment_0_0_1_1_1_in_rule__XShortClosure__Group_0_0_1_1__1__Impl60287);
rule__XShortClosure__DeclaredFormalParametersAssignment_0_0_1_1_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXShortClosureAccess().getDeclaredFormalParametersAssignment_0_0_1_1_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XShortClosure__Group_0_0_1_1__1__Impl"
// $ANTLR start "rule__XParenthesizedExpression__Group__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29901:1: rule__XParenthesizedExpression__Group__0 : rule__XParenthesizedExpression__Group__0__Impl rule__XParenthesizedExpression__Group__1 ;
public final void rule__XParenthesizedExpression__Group__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29905:1: ( rule__XParenthesizedExpression__Group__0__Impl rule__XParenthesizedExpression__Group__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29906:2: rule__XParenthesizedExpression__Group__0__Impl rule__XParenthesizedExpression__Group__1
{
pushFollow(FollowSets002.FOLLOW_rule__XParenthesizedExpression__Group__0__Impl_in_rule__XParenthesizedExpression__Group__060321);
rule__XParenthesizedExpression__Group__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XParenthesizedExpression__Group__1_in_rule__XParenthesizedExpression__Group__060324);
rule__XParenthesizedExpression__Group__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XParenthesizedExpression__Group__0"
// $ANTLR start "rule__XParenthesizedExpression__Group__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29913:1: rule__XParenthesizedExpression__Group__0__Impl : ( '(' ) ;
public final void rule__XParenthesizedExpression__Group__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29917:1: ( ( '(' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29918:1: ( '(' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29918:1: ( '(' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29919:1: '('
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXParenthesizedExpressionAccess().getLeftParenthesisKeyword_0());
}
match(input,144,FollowSets002.FOLLOW_144_in_rule__XParenthesizedExpression__Group__0__Impl60352); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXParenthesizedExpressionAccess().getLeftParenthesisKeyword_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XParenthesizedExpression__Group__0__Impl"
// $ANTLR start "rule__XParenthesizedExpression__Group__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29932:1: rule__XParenthesizedExpression__Group__1 : rule__XParenthesizedExpression__Group__1__Impl rule__XParenthesizedExpression__Group__2 ;
public final void rule__XParenthesizedExpression__Group__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29936:1: ( rule__XParenthesizedExpression__Group__1__Impl rule__XParenthesizedExpression__Group__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29937:2: rule__XParenthesizedExpression__Group__1__Impl rule__XParenthesizedExpression__Group__2
{
pushFollow(FollowSets002.FOLLOW_rule__XParenthesizedExpression__Group__1__Impl_in_rule__XParenthesizedExpression__Group__160383);
rule__XParenthesizedExpression__Group__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XParenthesizedExpression__Group__2_in_rule__XParenthesizedExpression__Group__160386);
rule__XParenthesizedExpression__Group__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XParenthesizedExpression__Group__1"
// $ANTLR start "rule__XParenthesizedExpression__Group__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29944:1: rule__XParenthesizedExpression__Group__1__Impl : ( ruleXExpression ) ;
public final void rule__XParenthesizedExpression__Group__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29948:1: ( ( ruleXExpression ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29949:1: ( ruleXExpression )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29949:1: ( ruleXExpression )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29950:1: ruleXExpression
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXParenthesizedExpressionAccess().getXExpressionParserRuleCall_1());
}
pushFollow(FollowSets002.FOLLOW_ruleXExpression_in_rule__XParenthesizedExpression__Group__1__Impl60413);
ruleXExpression();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXParenthesizedExpressionAccess().getXExpressionParserRuleCall_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XParenthesizedExpression__Group__1__Impl"
// $ANTLR start "rule__XParenthesizedExpression__Group__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29961:1: rule__XParenthesizedExpression__Group__2 : rule__XParenthesizedExpression__Group__2__Impl ;
public final void rule__XParenthesizedExpression__Group__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29965:1: ( rule__XParenthesizedExpression__Group__2__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29966:2: rule__XParenthesizedExpression__Group__2__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__XParenthesizedExpression__Group__2__Impl_in_rule__XParenthesizedExpression__Group__260442);
rule__XParenthesizedExpression__Group__2__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XParenthesizedExpression__Group__2"
// $ANTLR start "rule__XParenthesizedExpression__Group__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29972:1: rule__XParenthesizedExpression__Group__2__Impl : ( ')' ) ;
public final void rule__XParenthesizedExpression__Group__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29976:1: ( ( ')' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29977:1: ( ')' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29977:1: ( ')' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29978:1: ')'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXParenthesizedExpressionAccess().getRightParenthesisKeyword_2());
}
match(input,145,FollowSets002.FOLLOW_145_in_rule__XParenthesizedExpression__Group__2__Impl60470); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXParenthesizedExpressionAccess().getRightParenthesisKeyword_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XParenthesizedExpression__Group__2__Impl"
// $ANTLR start "rule__XIfExpression__Group__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29997:1: rule__XIfExpression__Group__0 : rule__XIfExpression__Group__0__Impl rule__XIfExpression__Group__1 ;
public final void rule__XIfExpression__Group__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30001:1: ( rule__XIfExpression__Group__0__Impl rule__XIfExpression__Group__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30002:2: rule__XIfExpression__Group__0__Impl rule__XIfExpression__Group__1
{
pushFollow(FollowSets002.FOLLOW_rule__XIfExpression__Group__0__Impl_in_rule__XIfExpression__Group__060507);
rule__XIfExpression__Group__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XIfExpression__Group__1_in_rule__XIfExpression__Group__060510);
rule__XIfExpression__Group__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XIfExpression__Group__0"
// $ANTLR start "rule__XIfExpression__Group__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30009:1: rule__XIfExpression__Group__0__Impl : ( () ) ;
public final void rule__XIfExpression__Group__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30013:1: ( ( () ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30014:1: ( () )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30014:1: ( () )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30015:1: ()
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXIfExpressionAccess().getXIfExpressionAction_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30016:1: ()
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30018:1:
{
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXIfExpressionAccess().getXIfExpressionAction_0());
}
}
}
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XIfExpression__Group__0__Impl"
// $ANTLR start "rule__XIfExpression__Group__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30028:1: rule__XIfExpression__Group__1 : rule__XIfExpression__Group__1__Impl rule__XIfExpression__Group__2 ;
public final void rule__XIfExpression__Group__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30032:1: ( rule__XIfExpression__Group__1__Impl rule__XIfExpression__Group__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30033:2: rule__XIfExpression__Group__1__Impl rule__XIfExpression__Group__2
{
pushFollow(FollowSets002.FOLLOW_rule__XIfExpression__Group__1__Impl_in_rule__XIfExpression__Group__160568);
rule__XIfExpression__Group__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XIfExpression__Group__2_in_rule__XIfExpression__Group__160571);
rule__XIfExpression__Group__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XIfExpression__Group__1"
// $ANTLR start "rule__XIfExpression__Group__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30040:1: rule__XIfExpression__Group__1__Impl : ( 'if' ) ;
public final void rule__XIfExpression__Group__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30044:1: ( ( 'if' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30045:1: ( 'if' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30045:1: ( 'if' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30046:1: 'if'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXIfExpressionAccess().getIfKeyword_1());
}
match(input,159,FollowSets002.FOLLOW_159_in_rule__XIfExpression__Group__1__Impl60599); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXIfExpressionAccess().getIfKeyword_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XIfExpression__Group__1__Impl"
// $ANTLR start "rule__XIfExpression__Group__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30059:1: rule__XIfExpression__Group__2 : rule__XIfExpression__Group__2__Impl rule__XIfExpression__Group__3 ;
public final void rule__XIfExpression__Group__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30063:1: ( rule__XIfExpression__Group__2__Impl rule__XIfExpression__Group__3 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30064:2: rule__XIfExpression__Group__2__Impl rule__XIfExpression__Group__3
{
pushFollow(FollowSets002.FOLLOW_rule__XIfExpression__Group__2__Impl_in_rule__XIfExpression__Group__260630);
rule__XIfExpression__Group__2__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XIfExpression__Group__3_in_rule__XIfExpression__Group__260633);
rule__XIfExpression__Group__3();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XIfExpression__Group__2"
// $ANTLR start "rule__XIfExpression__Group__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30071:1: rule__XIfExpression__Group__2__Impl : ( '(' ) ;
public final void rule__XIfExpression__Group__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30075:1: ( ( '(' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30076:1: ( '(' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30076:1: ( '(' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30077:1: '('
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXIfExpressionAccess().getLeftParenthesisKeyword_2());
}
match(input,144,FollowSets002.FOLLOW_144_in_rule__XIfExpression__Group__2__Impl60661); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXIfExpressionAccess().getLeftParenthesisKeyword_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XIfExpression__Group__2__Impl"
// $ANTLR start "rule__XIfExpression__Group__3"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30090:1: rule__XIfExpression__Group__3 : rule__XIfExpression__Group__3__Impl rule__XIfExpression__Group__4 ;
public final void rule__XIfExpression__Group__3() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30094:1: ( rule__XIfExpression__Group__3__Impl rule__XIfExpression__Group__4 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30095:2: rule__XIfExpression__Group__3__Impl rule__XIfExpression__Group__4
{
pushFollow(FollowSets002.FOLLOW_rule__XIfExpression__Group__3__Impl_in_rule__XIfExpression__Group__360692);
rule__XIfExpression__Group__3__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XIfExpression__Group__4_in_rule__XIfExpression__Group__360695);
rule__XIfExpression__Group__4();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XIfExpression__Group__3"
// $ANTLR start "rule__XIfExpression__Group__3__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30102:1: rule__XIfExpression__Group__3__Impl : ( ( rule__XIfExpression__IfAssignment_3 ) ) ;
public final void rule__XIfExpression__Group__3__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30106:1: ( ( ( rule__XIfExpression__IfAssignment_3 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30107:1: ( ( rule__XIfExpression__IfAssignment_3 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30107:1: ( ( rule__XIfExpression__IfAssignment_3 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30108:1: ( rule__XIfExpression__IfAssignment_3 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXIfExpressionAccess().getIfAssignment_3());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30109:1: ( rule__XIfExpression__IfAssignment_3 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30109:2: rule__XIfExpression__IfAssignment_3
{
pushFollow(FollowSets002.FOLLOW_rule__XIfExpression__IfAssignment_3_in_rule__XIfExpression__Group__3__Impl60722);
rule__XIfExpression__IfAssignment_3();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXIfExpressionAccess().getIfAssignment_3());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XIfExpression__Group__3__Impl"
// $ANTLR start "rule__XIfExpression__Group__4"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30119:1: rule__XIfExpression__Group__4 : rule__XIfExpression__Group__4__Impl rule__XIfExpression__Group__5 ;
public final void rule__XIfExpression__Group__4() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30123:1: ( rule__XIfExpression__Group__4__Impl rule__XIfExpression__Group__5 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30124:2: rule__XIfExpression__Group__4__Impl rule__XIfExpression__Group__5
{
pushFollow(FollowSets002.FOLLOW_rule__XIfExpression__Group__4__Impl_in_rule__XIfExpression__Group__460752);
rule__XIfExpression__Group__4__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XIfExpression__Group__5_in_rule__XIfExpression__Group__460755);
rule__XIfExpression__Group__5();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XIfExpression__Group__4"
// $ANTLR start "rule__XIfExpression__Group__4__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30131:1: rule__XIfExpression__Group__4__Impl : ( ')' ) ;
public final void rule__XIfExpression__Group__4__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30135:1: ( ( ')' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30136:1: ( ')' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30136:1: ( ')' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30137:1: ')'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXIfExpressionAccess().getRightParenthesisKeyword_4());
}
match(input,145,FollowSets002.FOLLOW_145_in_rule__XIfExpression__Group__4__Impl60783); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXIfExpressionAccess().getRightParenthesisKeyword_4());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XIfExpression__Group__4__Impl"
// $ANTLR start "rule__XIfExpression__Group__5"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30150:1: rule__XIfExpression__Group__5 : rule__XIfExpression__Group__5__Impl rule__XIfExpression__Group__6 ;
public final void rule__XIfExpression__Group__5() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30154:1: ( rule__XIfExpression__Group__5__Impl rule__XIfExpression__Group__6 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30155:2: rule__XIfExpression__Group__5__Impl rule__XIfExpression__Group__6
{
pushFollow(FollowSets002.FOLLOW_rule__XIfExpression__Group__5__Impl_in_rule__XIfExpression__Group__560814);
rule__XIfExpression__Group__5__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XIfExpression__Group__6_in_rule__XIfExpression__Group__560817);
rule__XIfExpression__Group__6();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XIfExpression__Group__5"
// $ANTLR start "rule__XIfExpression__Group__5__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30162:1: rule__XIfExpression__Group__5__Impl : ( ( rule__XIfExpression__ThenAssignment_5 ) ) ;
public final void rule__XIfExpression__Group__5__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30166:1: ( ( ( rule__XIfExpression__ThenAssignment_5 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30167:1: ( ( rule__XIfExpression__ThenAssignment_5 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30167:1: ( ( rule__XIfExpression__ThenAssignment_5 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30168:1: ( rule__XIfExpression__ThenAssignment_5 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXIfExpressionAccess().getThenAssignment_5());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30169:1: ( rule__XIfExpression__ThenAssignment_5 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30169:2: rule__XIfExpression__ThenAssignment_5
{
pushFollow(FollowSets002.FOLLOW_rule__XIfExpression__ThenAssignment_5_in_rule__XIfExpression__Group__5__Impl60844);
rule__XIfExpression__ThenAssignment_5();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXIfExpressionAccess().getThenAssignment_5());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XIfExpression__Group__5__Impl"
// $ANTLR start "rule__XIfExpression__Group__6"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30179:1: rule__XIfExpression__Group__6 : rule__XIfExpression__Group__6__Impl ;
public final void rule__XIfExpression__Group__6() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30183:1: ( rule__XIfExpression__Group__6__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30184:2: rule__XIfExpression__Group__6__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__XIfExpression__Group__6__Impl_in_rule__XIfExpression__Group__660874);
rule__XIfExpression__Group__6__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XIfExpression__Group__6"
// $ANTLR start "rule__XIfExpression__Group__6__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30190:1: rule__XIfExpression__Group__6__Impl : ( ( rule__XIfExpression__Group_6__0 )? ) ;
public final void rule__XIfExpression__Group__6__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30194:1: ( ( ( rule__XIfExpression__Group_6__0 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30195:1: ( ( rule__XIfExpression__Group_6__0 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30195:1: ( ( rule__XIfExpression__Group_6__0 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30196:1: ( rule__XIfExpression__Group_6__0 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXIfExpressionAccess().getGroup_6());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30197:1: ( rule__XIfExpression__Group_6__0 )?
int alt225=2;
int LA225_0 = input.LA(1);
if ( (LA225_0==160) ) {
int LA225_1 = input.LA(2);
if ( (synpred311_InternalMango()) ) {
alt225=1;
}
}
switch (alt225) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30197:2: rule__XIfExpression__Group_6__0
{
pushFollow(FollowSets002.FOLLOW_rule__XIfExpression__Group_6__0_in_rule__XIfExpression__Group__6__Impl60901);
rule__XIfExpression__Group_6__0();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXIfExpressionAccess().getGroup_6());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XIfExpression__Group__6__Impl"
// $ANTLR start "rule__XIfExpression__Group_6__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30221:1: rule__XIfExpression__Group_6__0 : rule__XIfExpression__Group_6__0__Impl rule__XIfExpression__Group_6__1 ;
public final void rule__XIfExpression__Group_6__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30225:1: ( rule__XIfExpression__Group_6__0__Impl rule__XIfExpression__Group_6__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30226:2: rule__XIfExpression__Group_6__0__Impl rule__XIfExpression__Group_6__1
{
pushFollow(FollowSets002.FOLLOW_rule__XIfExpression__Group_6__0__Impl_in_rule__XIfExpression__Group_6__060946);
rule__XIfExpression__Group_6__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XIfExpression__Group_6__1_in_rule__XIfExpression__Group_6__060949);
rule__XIfExpression__Group_6__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XIfExpression__Group_6__0"
// $ANTLR start "rule__XIfExpression__Group_6__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30233:1: rule__XIfExpression__Group_6__0__Impl : ( ( 'else' ) ) ;
public final void rule__XIfExpression__Group_6__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30237:1: ( ( ( 'else' ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30238:1: ( ( 'else' ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30238:1: ( ( 'else' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30239:1: ( 'else' )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXIfExpressionAccess().getElseKeyword_6_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30240:1: ( 'else' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30241:2: 'else'
{
match(input,160,FollowSets002.FOLLOW_160_in_rule__XIfExpression__Group_6__0__Impl60978); if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXIfExpressionAccess().getElseKeyword_6_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XIfExpression__Group_6__0__Impl"
// $ANTLR start "rule__XIfExpression__Group_6__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30252:1: rule__XIfExpression__Group_6__1 : rule__XIfExpression__Group_6__1__Impl ;
public final void rule__XIfExpression__Group_6__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30256:1: ( rule__XIfExpression__Group_6__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30257:2: rule__XIfExpression__Group_6__1__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__XIfExpression__Group_6__1__Impl_in_rule__XIfExpression__Group_6__161010);
rule__XIfExpression__Group_6__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XIfExpression__Group_6__1"
// $ANTLR start "rule__XIfExpression__Group_6__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30263:1: rule__XIfExpression__Group_6__1__Impl : ( ( rule__XIfExpression__ElseAssignment_6_1 ) ) ;
public final void rule__XIfExpression__Group_6__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30267:1: ( ( ( rule__XIfExpression__ElseAssignment_6_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30268:1: ( ( rule__XIfExpression__ElseAssignment_6_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30268:1: ( ( rule__XIfExpression__ElseAssignment_6_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30269:1: ( rule__XIfExpression__ElseAssignment_6_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXIfExpressionAccess().getElseAssignment_6_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30270:1: ( rule__XIfExpression__ElseAssignment_6_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30270:2: rule__XIfExpression__ElseAssignment_6_1
{
pushFollow(FollowSets002.FOLLOW_rule__XIfExpression__ElseAssignment_6_1_in_rule__XIfExpression__Group_6__1__Impl61037);
rule__XIfExpression__ElseAssignment_6_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXIfExpressionAccess().getElseAssignment_6_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XIfExpression__Group_6__1__Impl"
// $ANTLR start "rule__XSwitchExpression__Group__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30284:1: rule__XSwitchExpression__Group__0 : rule__XSwitchExpression__Group__0__Impl rule__XSwitchExpression__Group__1 ;
public final void rule__XSwitchExpression__Group__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30288:1: ( rule__XSwitchExpression__Group__0__Impl rule__XSwitchExpression__Group__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30289:2: rule__XSwitchExpression__Group__0__Impl rule__XSwitchExpression__Group__1
{
pushFollow(FollowSets002.FOLLOW_rule__XSwitchExpression__Group__0__Impl_in_rule__XSwitchExpression__Group__061071);
rule__XSwitchExpression__Group__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XSwitchExpression__Group__1_in_rule__XSwitchExpression__Group__061074);
rule__XSwitchExpression__Group__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XSwitchExpression__Group__0"
// $ANTLR start "rule__XSwitchExpression__Group__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30296:1: rule__XSwitchExpression__Group__0__Impl : ( () ) ;
public final void rule__XSwitchExpression__Group__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30300:1: ( ( () ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30301:1: ( () )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30301:1: ( () )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30302:1: ()
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXSwitchExpressionAccess().getXSwitchExpressionAction_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30303:1: ()
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30305:1:
{
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXSwitchExpressionAccess().getXSwitchExpressionAction_0());
}
}
}
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XSwitchExpression__Group__0__Impl"
// $ANTLR start "rule__XSwitchExpression__Group__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30315:1: rule__XSwitchExpression__Group__1 : rule__XSwitchExpression__Group__1__Impl rule__XSwitchExpression__Group__2 ;
public final void rule__XSwitchExpression__Group__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30319:1: ( rule__XSwitchExpression__Group__1__Impl rule__XSwitchExpression__Group__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30320:2: rule__XSwitchExpression__Group__1__Impl rule__XSwitchExpression__Group__2
{
pushFollow(FollowSets002.FOLLOW_rule__XSwitchExpression__Group__1__Impl_in_rule__XSwitchExpression__Group__161132);
rule__XSwitchExpression__Group__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XSwitchExpression__Group__2_in_rule__XSwitchExpression__Group__161135);
rule__XSwitchExpression__Group__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XSwitchExpression__Group__1"
// $ANTLR start "rule__XSwitchExpression__Group__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30327:1: rule__XSwitchExpression__Group__1__Impl : ( 'switch' ) ;
public final void rule__XSwitchExpression__Group__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30331:1: ( ( 'switch' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30332:1: ( 'switch' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30332:1: ( 'switch' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30333:1: 'switch'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXSwitchExpressionAccess().getSwitchKeyword_1());
}
match(input,161,FollowSets002.FOLLOW_161_in_rule__XSwitchExpression__Group__1__Impl61163); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXSwitchExpressionAccess().getSwitchKeyword_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XSwitchExpression__Group__1__Impl"
// $ANTLR start "rule__XSwitchExpression__Group__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30346:1: rule__XSwitchExpression__Group__2 : rule__XSwitchExpression__Group__2__Impl rule__XSwitchExpression__Group__3 ;
public final void rule__XSwitchExpression__Group__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30350:1: ( rule__XSwitchExpression__Group__2__Impl rule__XSwitchExpression__Group__3 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30351:2: rule__XSwitchExpression__Group__2__Impl rule__XSwitchExpression__Group__3
{
pushFollow(FollowSets002.FOLLOW_rule__XSwitchExpression__Group__2__Impl_in_rule__XSwitchExpression__Group__261194);
rule__XSwitchExpression__Group__2__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XSwitchExpression__Group__3_in_rule__XSwitchExpression__Group__261197);
rule__XSwitchExpression__Group__3();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XSwitchExpression__Group__2"
// $ANTLR start "rule__XSwitchExpression__Group__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30358:1: rule__XSwitchExpression__Group__2__Impl : ( ( rule__XSwitchExpression__Alternatives_2 ) ) ;
public final void rule__XSwitchExpression__Group__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30362:1: ( ( ( rule__XSwitchExpression__Alternatives_2 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30363:1: ( ( rule__XSwitchExpression__Alternatives_2 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30363:1: ( ( rule__XSwitchExpression__Alternatives_2 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30364:1: ( rule__XSwitchExpression__Alternatives_2 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXSwitchExpressionAccess().getAlternatives_2());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30365:1: ( rule__XSwitchExpression__Alternatives_2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30365:2: rule__XSwitchExpression__Alternatives_2
{
pushFollow(FollowSets002.FOLLOW_rule__XSwitchExpression__Alternatives_2_in_rule__XSwitchExpression__Group__2__Impl61224);
rule__XSwitchExpression__Alternatives_2();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXSwitchExpressionAccess().getAlternatives_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XSwitchExpression__Group__2__Impl"
// $ANTLR start "rule__XSwitchExpression__Group__3"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30375:1: rule__XSwitchExpression__Group__3 : rule__XSwitchExpression__Group__3__Impl rule__XSwitchExpression__Group__4 ;
public final void rule__XSwitchExpression__Group__3() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30379:1: ( rule__XSwitchExpression__Group__3__Impl rule__XSwitchExpression__Group__4 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30380:2: rule__XSwitchExpression__Group__3__Impl rule__XSwitchExpression__Group__4
{
pushFollow(FollowSets002.FOLLOW_rule__XSwitchExpression__Group__3__Impl_in_rule__XSwitchExpression__Group__361254);
rule__XSwitchExpression__Group__3__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XSwitchExpression__Group__4_in_rule__XSwitchExpression__Group__361257);
rule__XSwitchExpression__Group__4();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XSwitchExpression__Group__3"
// $ANTLR start "rule__XSwitchExpression__Group__3__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30387:1: rule__XSwitchExpression__Group__3__Impl : ( '{' ) ;
public final void rule__XSwitchExpression__Group__3__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30391:1: ( ( '{' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30392:1: ( '{' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30392:1: ( '{' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30393:1: '{'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXSwitchExpressionAccess().getLeftCurlyBracketKeyword_3());
}
match(input,67,FollowSets002.FOLLOW_67_in_rule__XSwitchExpression__Group__3__Impl61285); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXSwitchExpressionAccess().getLeftCurlyBracketKeyword_3());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XSwitchExpression__Group__3__Impl"
// $ANTLR start "rule__XSwitchExpression__Group__4"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30406:1: rule__XSwitchExpression__Group__4 : rule__XSwitchExpression__Group__4__Impl rule__XSwitchExpression__Group__5 ;
public final void rule__XSwitchExpression__Group__4() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30410:1: ( rule__XSwitchExpression__Group__4__Impl rule__XSwitchExpression__Group__5 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30411:2: rule__XSwitchExpression__Group__4__Impl rule__XSwitchExpression__Group__5
{
pushFollow(FollowSets002.FOLLOW_rule__XSwitchExpression__Group__4__Impl_in_rule__XSwitchExpression__Group__461316);
rule__XSwitchExpression__Group__4__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XSwitchExpression__Group__5_in_rule__XSwitchExpression__Group__461319);
rule__XSwitchExpression__Group__5();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XSwitchExpression__Group__4"
// $ANTLR start "rule__XSwitchExpression__Group__4__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30418:1: rule__XSwitchExpression__Group__4__Impl : ( ( rule__XSwitchExpression__CasesAssignment_4 )* ) ;
public final void rule__XSwitchExpression__Group__4__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30422:1: ( ( ( rule__XSwitchExpression__CasesAssignment_4 )* ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30423:1: ( ( rule__XSwitchExpression__CasesAssignment_4 )* )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30423:1: ( ( rule__XSwitchExpression__CasesAssignment_4 )* )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30424:1: ( rule__XSwitchExpression__CasesAssignment_4 )*
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXSwitchExpressionAccess().getCasesAssignment_4());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30425:1: ( rule__XSwitchExpression__CasesAssignment_4 )*
loop226:
do {
int alt226=2;
int LA226_0 = input.LA(1);
if ( (LA226_0==RULE_ID||LA226_0==31||LA226_0==144||LA226_0==154||LA226_0==162||LA226_0==164) ) {
alt226=1;
}
switch (alt226) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30425:2: rule__XSwitchExpression__CasesAssignment_4
{
pushFollow(FollowSets002.FOLLOW_rule__XSwitchExpression__CasesAssignment_4_in_rule__XSwitchExpression__Group__4__Impl61346);
rule__XSwitchExpression__CasesAssignment_4();
state._fsp--;
if (state.failed) return ;
}
break;
default :
break loop226;
}
} while (true);
if ( state.backtracking==0 ) {
after(grammarAccess.getXSwitchExpressionAccess().getCasesAssignment_4());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XSwitchExpression__Group__4__Impl"
// $ANTLR start "rule__XSwitchExpression__Group__5"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30435:1: rule__XSwitchExpression__Group__5 : rule__XSwitchExpression__Group__5__Impl rule__XSwitchExpression__Group__6 ;
public final void rule__XSwitchExpression__Group__5() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30439:1: ( rule__XSwitchExpression__Group__5__Impl rule__XSwitchExpression__Group__6 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30440:2: rule__XSwitchExpression__Group__5__Impl rule__XSwitchExpression__Group__6
{
pushFollow(FollowSets002.FOLLOW_rule__XSwitchExpression__Group__5__Impl_in_rule__XSwitchExpression__Group__561377);
rule__XSwitchExpression__Group__5__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XSwitchExpression__Group__6_in_rule__XSwitchExpression__Group__561380);
rule__XSwitchExpression__Group__6();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XSwitchExpression__Group__5"
// $ANTLR start "rule__XSwitchExpression__Group__5__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30447:1: rule__XSwitchExpression__Group__5__Impl : ( ( rule__XSwitchExpression__Group_5__0 )? ) ;
public final void rule__XSwitchExpression__Group__5__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30451:1: ( ( ( rule__XSwitchExpression__Group_5__0 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30452:1: ( ( rule__XSwitchExpression__Group_5__0 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30452:1: ( ( rule__XSwitchExpression__Group_5__0 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30453:1: ( rule__XSwitchExpression__Group_5__0 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXSwitchExpressionAccess().getGroup_5());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30454:1: ( rule__XSwitchExpression__Group_5__0 )?
int alt227=2;
int LA227_0 = input.LA(1);
if ( (LA227_0==163) ) {
alt227=1;
}
switch (alt227) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30454:2: rule__XSwitchExpression__Group_5__0
{
pushFollow(FollowSets002.FOLLOW_rule__XSwitchExpression__Group_5__0_in_rule__XSwitchExpression__Group__5__Impl61407);
rule__XSwitchExpression__Group_5__0();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXSwitchExpressionAccess().getGroup_5());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XSwitchExpression__Group__5__Impl"
// $ANTLR start "rule__XSwitchExpression__Group__6"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30464:1: rule__XSwitchExpression__Group__6 : rule__XSwitchExpression__Group__6__Impl ;
public final void rule__XSwitchExpression__Group__6() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30468:1: ( rule__XSwitchExpression__Group__6__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30469:2: rule__XSwitchExpression__Group__6__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__XSwitchExpression__Group__6__Impl_in_rule__XSwitchExpression__Group__661438);
rule__XSwitchExpression__Group__6__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XSwitchExpression__Group__6"
// $ANTLR start "rule__XSwitchExpression__Group__6__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30475:1: rule__XSwitchExpression__Group__6__Impl : ( '}' ) ;
public final void rule__XSwitchExpression__Group__6__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30479:1: ( ( '}' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30480:1: ( '}' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30480:1: ( '}' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30481:1: '}'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXSwitchExpressionAccess().getRightCurlyBracketKeyword_6());
}
match(input,68,FollowSets002.FOLLOW_68_in_rule__XSwitchExpression__Group__6__Impl61466); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXSwitchExpressionAccess().getRightCurlyBracketKeyword_6());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XSwitchExpression__Group__6__Impl"
// $ANTLR start "rule__XSwitchExpression__Group_2_0__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30508:1: rule__XSwitchExpression__Group_2_0__0 : rule__XSwitchExpression__Group_2_0__0__Impl rule__XSwitchExpression__Group_2_0__1 ;
public final void rule__XSwitchExpression__Group_2_0__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30512:1: ( rule__XSwitchExpression__Group_2_0__0__Impl rule__XSwitchExpression__Group_2_0__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30513:2: rule__XSwitchExpression__Group_2_0__0__Impl rule__XSwitchExpression__Group_2_0__1
{
pushFollow(FollowSets002.FOLLOW_rule__XSwitchExpression__Group_2_0__0__Impl_in_rule__XSwitchExpression__Group_2_0__061511);
rule__XSwitchExpression__Group_2_0__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XSwitchExpression__Group_2_0__1_in_rule__XSwitchExpression__Group_2_0__061514);
rule__XSwitchExpression__Group_2_0__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XSwitchExpression__Group_2_0__0"
// $ANTLR start "rule__XSwitchExpression__Group_2_0__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30520:1: rule__XSwitchExpression__Group_2_0__0__Impl : ( ( rule__XSwitchExpression__Group_2_0_0__0 ) ) ;
public final void rule__XSwitchExpression__Group_2_0__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30524:1: ( ( ( rule__XSwitchExpression__Group_2_0_0__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30525:1: ( ( rule__XSwitchExpression__Group_2_0_0__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30525:1: ( ( rule__XSwitchExpression__Group_2_0_0__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30526:1: ( rule__XSwitchExpression__Group_2_0_0__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXSwitchExpressionAccess().getGroup_2_0_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30527:1: ( rule__XSwitchExpression__Group_2_0_0__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30527:2: rule__XSwitchExpression__Group_2_0_0__0
{
pushFollow(FollowSets002.FOLLOW_rule__XSwitchExpression__Group_2_0_0__0_in_rule__XSwitchExpression__Group_2_0__0__Impl61541);
rule__XSwitchExpression__Group_2_0_0__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXSwitchExpressionAccess().getGroup_2_0_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XSwitchExpression__Group_2_0__0__Impl"
// $ANTLR start "rule__XSwitchExpression__Group_2_0__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30537:1: rule__XSwitchExpression__Group_2_0__1 : rule__XSwitchExpression__Group_2_0__1__Impl rule__XSwitchExpression__Group_2_0__2 ;
public final void rule__XSwitchExpression__Group_2_0__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30541:1: ( rule__XSwitchExpression__Group_2_0__1__Impl rule__XSwitchExpression__Group_2_0__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30542:2: rule__XSwitchExpression__Group_2_0__1__Impl rule__XSwitchExpression__Group_2_0__2
{
pushFollow(FollowSets002.FOLLOW_rule__XSwitchExpression__Group_2_0__1__Impl_in_rule__XSwitchExpression__Group_2_0__161571);
rule__XSwitchExpression__Group_2_0__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XSwitchExpression__Group_2_0__2_in_rule__XSwitchExpression__Group_2_0__161574);
rule__XSwitchExpression__Group_2_0__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XSwitchExpression__Group_2_0__1"
// $ANTLR start "rule__XSwitchExpression__Group_2_0__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30549:1: rule__XSwitchExpression__Group_2_0__1__Impl : ( ( rule__XSwitchExpression__SwitchAssignment_2_0_1 ) ) ;
public final void rule__XSwitchExpression__Group_2_0__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30553:1: ( ( ( rule__XSwitchExpression__SwitchAssignment_2_0_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30554:1: ( ( rule__XSwitchExpression__SwitchAssignment_2_0_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30554:1: ( ( rule__XSwitchExpression__SwitchAssignment_2_0_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30555:1: ( rule__XSwitchExpression__SwitchAssignment_2_0_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXSwitchExpressionAccess().getSwitchAssignment_2_0_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30556:1: ( rule__XSwitchExpression__SwitchAssignment_2_0_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30556:2: rule__XSwitchExpression__SwitchAssignment_2_0_1
{
pushFollow(FollowSets002.FOLLOW_rule__XSwitchExpression__SwitchAssignment_2_0_1_in_rule__XSwitchExpression__Group_2_0__1__Impl61601);
rule__XSwitchExpression__SwitchAssignment_2_0_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXSwitchExpressionAccess().getSwitchAssignment_2_0_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XSwitchExpression__Group_2_0__1__Impl"
// $ANTLR start "rule__XSwitchExpression__Group_2_0__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30566:1: rule__XSwitchExpression__Group_2_0__2 : rule__XSwitchExpression__Group_2_0__2__Impl ;
public final void rule__XSwitchExpression__Group_2_0__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30570:1: ( rule__XSwitchExpression__Group_2_0__2__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30571:2: rule__XSwitchExpression__Group_2_0__2__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__XSwitchExpression__Group_2_0__2__Impl_in_rule__XSwitchExpression__Group_2_0__261631);
rule__XSwitchExpression__Group_2_0__2__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XSwitchExpression__Group_2_0__2"
// $ANTLR start "rule__XSwitchExpression__Group_2_0__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30577:1: rule__XSwitchExpression__Group_2_0__2__Impl : ( ')' ) ;
public final void rule__XSwitchExpression__Group_2_0__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30581:1: ( ( ')' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30582:1: ( ')' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30582:1: ( ')' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30583:1: ')'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXSwitchExpressionAccess().getRightParenthesisKeyword_2_0_2());
}
match(input,145,FollowSets002.FOLLOW_145_in_rule__XSwitchExpression__Group_2_0__2__Impl61659); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXSwitchExpressionAccess().getRightParenthesisKeyword_2_0_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XSwitchExpression__Group_2_0__2__Impl"
// $ANTLR start "rule__XSwitchExpression__Group_2_0_0__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30602:1: rule__XSwitchExpression__Group_2_0_0__0 : rule__XSwitchExpression__Group_2_0_0__0__Impl ;
public final void rule__XSwitchExpression__Group_2_0_0__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30606:1: ( rule__XSwitchExpression__Group_2_0_0__0__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30607:2: rule__XSwitchExpression__Group_2_0_0__0__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__XSwitchExpression__Group_2_0_0__0__Impl_in_rule__XSwitchExpression__Group_2_0_0__061696);
rule__XSwitchExpression__Group_2_0_0__0__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XSwitchExpression__Group_2_0_0__0"
// $ANTLR start "rule__XSwitchExpression__Group_2_0_0__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30613:1: rule__XSwitchExpression__Group_2_0_0__0__Impl : ( ( rule__XSwitchExpression__Group_2_0_0_0__0 ) ) ;
public final void rule__XSwitchExpression__Group_2_0_0__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30617:1: ( ( ( rule__XSwitchExpression__Group_2_0_0_0__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30618:1: ( ( rule__XSwitchExpression__Group_2_0_0_0__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30618:1: ( ( rule__XSwitchExpression__Group_2_0_0_0__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30619:1: ( rule__XSwitchExpression__Group_2_0_0_0__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXSwitchExpressionAccess().getGroup_2_0_0_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30620:1: ( rule__XSwitchExpression__Group_2_0_0_0__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30620:2: rule__XSwitchExpression__Group_2_0_0_0__0
{
pushFollow(FollowSets002.FOLLOW_rule__XSwitchExpression__Group_2_0_0_0__0_in_rule__XSwitchExpression__Group_2_0_0__0__Impl61723);
rule__XSwitchExpression__Group_2_0_0_0__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXSwitchExpressionAccess().getGroup_2_0_0_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XSwitchExpression__Group_2_0_0__0__Impl"
// $ANTLR start "rule__XSwitchExpression__Group_2_0_0_0__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30632:1: rule__XSwitchExpression__Group_2_0_0_0__0 : rule__XSwitchExpression__Group_2_0_0_0__0__Impl rule__XSwitchExpression__Group_2_0_0_0__1 ;
public final void rule__XSwitchExpression__Group_2_0_0_0__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30636:1: ( rule__XSwitchExpression__Group_2_0_0_0__0__Impl rule__XSwitchExpression__Group_2_0_0_0__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30637:2: rule__XSwitchExpression__Group_2_0_0_0__0__Impl rule__XSwitchExpression__Group_2_0_0_0__1
{
pushFollow(FollowSets002.FOLLOW_rule__XSwitchExpression__Group_2_0_0_0__0__Impl_in_rule__XSwitchExpression__Group_2_0_0_0__061755);
rule__XSwitchExpression__Group_2_0_0_0__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XSwitchExpression__Group_2_0_0_0__1_in_rule__XSwitchExpression__Group_2_0_0_0__061758);
rule__XSwitchExpression__Group_2_0_0_0__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XSwitchExpression__Group_2_0_0_0__0"
// $ANTLR start "rule__XSwitchExpression__Group_2_0_0_0__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30644:1: rule__XSwitchExpression__Group_2_0_0_0__0__Impl : ( '(' ) ;
public final void rule__XSwitchExpression__Group_2_0_0_0__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30648:1: ( ( '(' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30649:1: ( '(' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30649:1: ( '(' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30650:1: '('
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXSwitchExpressionAccess().getLeftParenthesisKeyword_2_0_0_0_0());
}
match(input,144,FollowSets002.FOLLOW_144_in_rule__XSwitchExpression__Group_2_0_0_0__0__Impl61786); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXSwitchExpressionAccess().getLeftParenthesisKeyword_2_0_0_0_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XSwitchExpression__Group_2_0_0_0__0__Impl"
// $ANTLR start "rule__XSwitchExpression__Group_2_0_0_0__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30663:1: rule__XSwitchExpression__Group_2_0_0_0__1 : rule__XSwitchExpression__Group_2_0_0_0__1__Impl rule__XSwitchExpression__Group_2_0_0_0__2 ;
public final void rule__XSwitchExpression__Group_2_0_0_0__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30667:1: ( rule__XSwitchExpression__Group_2_0_0_0__1__Impl rule__XSwitchExpression__Group_2_0_0_0__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30668:2: rule__XSwitchExpression__Group_2_0_0_0__1__Impl rule__XSwitchExpression__Group_2_0_0_0__2
{
pushFollow(FollowSets002.FOLLOW_rule__XSwitchExpression__Group_2_0_0_0__1__Impl_in_rule__XSwitchExpression__Group_2_0_0_0__161817);
rule__XSwitchExpression__Group_2_0_0_0__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XSwitchExpression__Group_2_0_0_0__2_in_rule__XSwitchExpression__Group_2_0_0_0__161820);
rule__XSwitchExpression__Group_2_0_0_0__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XSwitchExpression__Group_2_0_0_0__1"
// $ANTLR start "rule__XSwitchExpression__Group_2_0_0_0__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30675:1: rule__XSwitchExpression__Group_2_0_0_0__1__Impl : ( ( rule__XSwitchExpression__DeclaredParamAssignment_2_0_0_0_1 ) ) ;
public final void rule__XSwitchExpression__Group_2_0_0_0__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30679:1: ( ( ( rule__XSwitchExpression__DeclaredParamAssignment_2_0_0_0_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30680:1: ( ( rule__XSwitchExpression__DeclaredParamAssignment_2_0_0_0_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30680:1: ( ( rule__XSwitchExpression__DeclaredParamAssignment_2_0_0_0_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30681:1: ( rule__XSwitchExpression__DeclaredParamAssignment_2_0_0_0_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXSwitchExpressionAccess().getDeclaredParamAssignment_2_0_0_0_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30682:1: ( rule__XSwitchExpression__DeclaredParamAssignment_2_0_0_0_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30682:2: rule__XSwitchExpression__DeclaredParamAssignment_2_0_0_0_1
{
pushFollow(FollowSets002.FOLLOW_rule__XSwitchExpression__DeclaredParamAssignment_2_0_0_0_1_in_rule__XSwitchExpression__Group_2_0_0_0__1__Impl61847);
rule__XSwitchExpression__DeclaredParamAssignment_2_0_0_0_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXSwitchExpressionAccess().getDeclaredParamAssignment_2_0_0_0_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XSwitchExpression__Group_2_0_0_0__1__Impl"
// $ANTLR start "rule__XSwitchExpression__Group_2_0_0_0__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30692:1: rule__XSwitchExpression__Group_2_0_0_0__2 : rule__XSwitchExpression__Group_2_0_0_0__2__Impl ;
public final void rule__XSwitchExpression__Group_2_0_0_0__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30696:1: ( rule__XSwitchExpression__Group_2_0_0_0__2__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30697:2: rule__XSwitchExpression__Group_2_0_0_0__2__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__XSwitchExpression__Group_2_0_0_0__2__Impl_in_rule__XSwitchExpression__Group_2_0_0_0__261877);
rule__XSwitchExpression__Group_2_0_0_0__2__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XSwitchExpression__Group_2_0_0_0__2"
// $ANTLR start "rule__XSwitchExpression__Group_2_0_0_0__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30703:1: rule__XSwitchExpression__Group_2_0_0_0__2__Impl : ( ':' ) ;
public final void rule__XSwitchExpression__Group_2_0_0_0__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30707:1: ( ( ':' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30708:1: ( ':' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30708:1: ( ':' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30709:1: ':'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXSwitchExpressionAccess().getColonKeyword_2_0_0_0_2());
}
match(input,162,FollowSets002.FOLLOW_162_in_rule__XSwitchExpression__Group_2_0_0_0__2__Impl61905); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXSwitchExpressionAccess().getColonKeyword_2_0_0_0_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XSwitchExpression__Group_2_0_0_0__2__Impl"
// $ANTLR start "rule__XSwitchExpression__Group_2_1__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30728:1: rule__XSwitchExpression__Group_2_1__0 : rule__XSwitchExpression__Group_2_1__0__Impl rule__XSwitchExpression__Group_2_1__1 ;
public final void rule__XSwitchExpression__Group_2_1__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30732:1: ( rule__XSwitchExpression__Group_2_1__0__Impl rule__XSwitchExpression__Group_2_1__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30733:2: rule__XSwitchExpression__Group_2_1__0__Impl rule__XSwitchExpression__Group_2_1__1
{
pushFollow(FollowSets002.FOLLOW_rule__XSwitchExpression__Group_2_1__0__Impl_in_rule__XSwitchExpression__Group_2_1__061942);
rule__XSwitchExpression__Group_2_1__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XSwitchExpression__Group_2_1__1_in_rule__XSwitchExpression__Group_2_1__061945);
rule__XSwitchExpression__Group_2_1__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XSwitchExpression__Group_2_1__0"
// $ANTLR start "rule__XSwitchExpression__Group_2_1__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30740:1: rule__XSwitchExpression__Group_2_1__0__Impl : ( ( rule__XSwitchExpression__Group_2_1_0__0 )? ) ;
public final void rule__XSwitchExpression__Group_2_1__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30744:1: ( ( ( rule__XSwitchExpression__Group_2_1_0__0 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30745:1: ( ( rule__XSwitchExpression__Group_2_1_0__0 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30745:1: ( ( rule__XSwitchExpression__Group_2_1_0__0 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30746:1: ( rule__XSwitchExpression__Group_2_1_0__0 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXSwitchExpressionAccess().getGroup_2_1_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30747:1: ( rule__XSwitchExpression__Group_2_1_0__0 )?
int alt228=2;
alt228 = dfa228.predict(input);
switch (alt228) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30747:2: rule__XSwitchExpression__Group_2_1_0__0
{
pushFollow(FollowSets002.FOLLOW_rule__XSwitchExpression__Group_2_1_0__0_in_rule__XSwitchExpression__Group_2_1__0__Impl61972);
rule__XSwitchExpression__Group_2_1_0__0();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXSwitchExpressionAccess().getGroup_2_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XSwitchExpression__Group_2_1__0__Impl"
// $ANTLR start "rule__XSwitchExpression__Group_2_1__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30757:1: rule__XSwitchExpression__Group_2_1__1 : rule__XSwitchExpression__Group_2_1__1__Impl ;
public final void rule__XSwitchExpression__Group_2_1__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30761:1: ( rule__XSwitchExpression__Group_2_1__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30762:2: rule__XSwitchExpression__Group_2_1__1__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__XSwitchExpression__Group_2_1__1__Impl_in_rule__XSwitchExpression__Group_2_1__162003);
rule__XSwitchExpression__Group_2_1__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XSwitchExpression__Group_2_1__1"
// $ANTLR start "rule__XSwitchExpression__Group_2_1__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30768:1: rule__XSwitchExpression__Group_2_1__1__Impl : ( ( rule__XSwitchExpression__SwitchAssignment_2_1_1 ) ) ;
public final void rule__XSwitchExpression__Group_2_1__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30772:1: ( ( ( rule__XSwitchExpression__SwitchAssignment_2_1_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30773:1: ( ( rule__XSwitchExpression__SwitchAssignment_2_1_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30773:1: ( ( rule__XSwitchExpression__SwitchAssignment_2_1_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30774:1: ( rule__XSwitchExpression__SwitchAssignment_2_1_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXSwitchExpressionAccess().getSwitchAssignment_2_1_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30775:1: ( rule__XSwitchExpression__SwitchAssignment_2_1_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30775:2: rule__XSwitchExpression__SwitchAssignment_2_1_1
{
pushFollow(FollowSets002.FOLLOW_rule__XSwitchExpression__SwitchAssignment_2_1_1_in_rule__XSwitchExpression__Group_2_1__1__Impl62030);
rule__XSwitchExpression__SwitchAssignment_2_1_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXSwitchExpressionAccess().getSwitchAssignment_2_1_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XSwitchExpression__Group_2_1__1__Impl"
// $ANTLR start "rule__XSwitchExpression__Group_2_1_0__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30789:1: rule__XSwitchExpression__Group_2_1_0__0 : rule__XSwitchExpression__Group_2_1_0__0__Impl ;
public final void rule__XSwitchExpression__Group_2_1_0__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30793:1: ( rule__XSwitchExpression__Group_2_1_0__0__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30794:2: rule__XSwitchExpression__Group_2_1_0__0__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__XSwitchExpression__Group_2_1_0__0__Impl_in_rule__XSwitchExpression__Group_2_1_0__062064);
rule__XSwitchExpression__Group_2_1_0__0__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XSwitchExpression__Group_2_1_0__0"
// $ANTLR start "rule__XSwitchExpression__Group_2_1_0__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30800:1: rule__XSwitchExpression__Group_2_1_0__0__Impl : ( ( rule__XSwitchExpression__Group_2_1_0_0__0 ) ) ;
public final void rule__XSwitchExpression__Group_2_1_0__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30804:1: ( ( ( rule__XSwitchExpression__Group_2_1_0_0__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30805:1: ( ( rule__XSwitchExpression__Group_2_1_0_0__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30805:1: ( ( rule__XSwitchExpression__Group_2_1_0_0__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30806:1: ( rule__XSwitchExpression__Group_2_1_0_0__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXSwitchExpressionAccess().getGroup_2_1_0_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30807:1: ( rule__XSwitchExpression__Group_2_1_0_0__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30807:2: rule__XSwitchExpression__Group_2_1_0_0__0
{
pushFollow(FollowSets002.FOLLOW_rule__XSwitchExpression__Group_2_1_0_0__0_in_rule__XSwitchExpression__Group_2_1_0__0__Impl62091);
rule__XSwitchExpression__Group_2_1_0_0__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXSwitchExpressionAccess().getGroup_2_1_0_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XSwitchExpression__Group_2_1_0__0__Impl"
// $ANTLR start "rule__XSwitchExpression__Group_2_1_0_0__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30819:1: rule__XSwitchExpression__Group_2_1_0_0__0 : rule__XSwitchExpression__Group_2_1_0_0__0__Impl rule__XSwitchExpression__Group_2_1_0_0__1 ;
public final void rule__XSwitchExpression__Group_2_1_0_0__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30823:1: ( rule__XSwitchExpression__Group_2_1_0_0__0__Impl rule__XSwitchExpression__Group_2_1_0_0__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30824:2: rule__XSwitchExpression__Group_2_1_0_0__0__Impl rule__XSwitchExpression__Group_2_1_0_0__1
{
pushFollow(FollowSets002.FOLLOW_rule__XSwitchExpression__Group_2_1_0_0__0__Impl_in_rule__XSwitchExpression__Group_2_1_0_0__062123);
rule__XSwitchExpression__Group_2_1_0_0__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XSwitchExpression__Group_2_1_0_0__1_in_rule__XSwitchExpression__Group_2_1_0_0__062126);
rule__XSwitchExpression__Group_2_1_0_0__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XSwitchExpression__Group_2_1_0_0__0"
// $ANTLR start "rule__XSwitchExpression__Group_2_1_0_0__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30831:1: rule__XSwitchExpression__Group_2_1_0_0__0__Impl : ( ( rule__XSwitchExpression__DeclaredParamAssignment_2_1_0_0_0 ) ) ;
public final void rule__XSwitchExpression__Group_2_1_0_0__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30835:1: ( ( ( rule__XSwitchExpression__DeclaredParamAssignment_2_1_0_0_0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30836:1: ( ( rule__XSwitchExpression__DeclaredParamAssignment_2_1_0_0_0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30836:1: ( ( rule__XSwitchExpression__DeclaredParamAssignment_2_1_0_0_0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30837:1: ( rule__XSwitchExpression__DeclaredParamAssignment_2_1_0_0_0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXSwitchExpressionAccess().getDeclaredParamAssignment_2_1_0_0_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30838:1: ( rule__XSwitchExpression__DeclaredParamAssignment_2_1_0_0_0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30838:2: rule__XSwitchExpression__DeclaredParamAssignment_2_1_0_0_0
{
pushFollow(FollowSets002.FOLLOW_rule__XSwitchExpression__DeclaredParamAssignment_2_1_0_0_0_in_rule__XSwitchExpression__Group_2_1_0_0__0__Impl62153);
rule__XSwitchExpression__DeclaredParamAssignment_2_1_0_0_0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXSwitchExpressionAccess().getDeclaredParamAssignment_2_1_0_0_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XSwitchExpression__Group_2_1_0_0__0__Impl"
// $ANTLR start "rule__XSwitchExpression__Group_2_1_0_0__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30848:1: rule__XSwitchExpression__Group_2_1_0_0__1 : rule__XSwitchExpression__Group_2_1_0_0__1__Impl ;
public final void rule__XSwitchExpression__Group_2_1_0_0__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30852:1: ( rule__XSwitchExpression__Group_2_1_0_0__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30853:2: rule__XSwitchExpression__Group_2_1_0_0__1__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__XSwitchExpression__Group_2_1_0_0__1__Impl_in_rule__XSwitchExpression__Group_2_1_0_0__162183);
rule__XSwitchExpression__Group_2_1_0_0__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XSwitchExpression__Group_2_1_0_0__1"
// $ANTLR start "rule__XSwitchExpression__Group_2_1_0_0__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30859:1: rule__XSwitchExpression__Group_2_1_0_0__1__Impl : ( ':' ) ;
public final void rule__XSwitchExpression__Group_2_1_0_0__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30863:1: ( ( ':' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30864:1: ( ':' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30864:1: ( ':' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30865:1: ':'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXSwitchExpressionAccess().getColonKeyword_2_1_0_0_1());
}
match(input,162,FollowSets002.FOLLOW_162_in_rule__XSwitchExpression__Group_2_1_0_0__1__Impl62211); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXSwitchExpressionAccess().getColonKeyword_2_1_0_0_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XSwitchExpression__Group_2_1_0_0__1__Impl"
// $ANTLR start "rule__XSwitchExpression__Group_5__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30882:1: rule__XSwitchExpression__Group_5__0 : rule__XSwitchExpression__Group_5__0__Impl rule__XSwitchExpression__Group_5__1 ;
public final void rule__XSwitchExpression__Group_5__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30886:1: ( rule__XSwitchExpression__Group_5__0__Impl rule__XSwitchExpression__Group_5__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30887:2: rule__XSwitchExpression__Group_5__0__Impl rule__XSwitchExpression__Group_5__1
{
pushFollow(FollowSets002.FOLLOW_rule__XSwitchExpression__Group_5__0__Impl_in_rule__XSwitchExpression__Group_5__062246);
rule__XSwitchExpression__Group_5__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XSwitchExpression__Group_5__1_in_rule__XSwitchExpression__Group_5__062249);
rule__XSwitchExpression__Group_5__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XSwitchExpression__Group_5__0"
// $ANTLR start "rule__XSwitchExpression__Group_5__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30894:1: rule__XSwitchExpression__Group_5__0__Impl : ( 'default' ) ;
public final void rule__XSwitchExpression__Group_5__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30898:1: ( ( 'default' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30899:1: ( 'default' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30899:1: ( 'default' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30900:1: 'default'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXSwitchExpressionAccess().getDefaultKeyword_5_0());
}
match(input,163,FollowSets002.FOLLOW_163_in_rule__XSwitchExpression__Group_5__0__Impl62277); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXSwitchExpressionAccess().getDefaultKeyword_5_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XSwitchExpression__Group_5__0__Impl"
// $ANTLR start "rule__XSwitchExpression__Group_5__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30913:1: rule__XSwitchExpression__Group_5__1 : rule__XSwitchExpression__Group_5__1__Impl rule__XSwitchExpression__Group_5__2 ;
public final void rule__XSwitchExpression__Group_5__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30917:1: ( rule__XSwitchExpression__Group_5__1__Impl rule__XSwitchExpression__Group_5__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30918:2: rule__XSwitchExpression__Group_5__1__Impl rule__XSwitchExpression__Group_5__2
{
pushFollow(FollowSets002.FOLLOW_rule__XSwitchExpression__Group_5__1__Impl_in_rule__XSwitchExpression__Group_5__162308);
rule__XSwitchExpression__Group_5__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XSwitchExpression__Group_5__2_in_rule__XSwitchExpression__Group_5__162311);
rule__XSwitchExpression__Group_5__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XSwitchExpression__Group_5__1"
// $ANTLR start "rule__XSwitchExpression__Group_5__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30925:1: rule__XSwitchExpression__Group_5__1__Impl : ( ':' ) ;
public final void rule__XSwitchExpression__Group_5__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30929:1: ( ( ':' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30930:1: ( ':' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30930:1: ( ':' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30931:1: ':'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXSwitchExpressionAccess().getColonKeyword_5_1());
}
match(input,162,FollowSets002.FOLLOW_162_in_rule__XSwitchExpression__Group_5__1__Impl62339); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXSwitchExpressionAccess().getColonKeyword_5_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XSwitchExpression__Group_5__1__Impl"
// $ANTLR start "rule__XSwitchExpression__Group_5__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30944:1: rule__XSwitchExpression__Group_5__2 : rule__XSwitchExpression__Group_5__2__Impl ;
public final void rule__XSwitchExpression__Group_5__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30948:1: ( rule__XSwitchExpression__Group_5__2__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30949:2: rule__XSwitchExpression__Group_5__2__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__XSwitchExpression__Group_5__2__Impl_in_rule__XSwitchExpression__Group_5__262370);
rule__XSwitchExpression__Group_5__2__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XSwitchExpression__Group_5__2"
// $ANTLR start "rule__XSwitchExpression__Group_5__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30955:1: rule__XSwitchExpression__Group_5__2__Impl : ( ( rule__XSwitchExpression__DefaultAssignment_5_2 ) ) ;
public final void rule__XSwitchExpression__Group_5__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30959:1: ( ( ( rule__XSwitchExpression__DefaultAssignment_5_2 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30960:1: ( ( rule__XSwitchExpression__DefaultAssignment_5_2 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30960:1: ( ( rule__XSwitchExpression__DefaultAssignment_5_2 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30961:1: ( rule__XSwitchExpression__DefaultAssignment_5_2 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXSwitchExpressionAccess().getDefaultAssignment_5_2());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30962:1: ( rule__XSwitchExpression__DefaultAssignment_5_2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30962:2: rule__XSwitchExpression__DefaultAssignment_5_2
{
pushFollow(FollowSets002.FOLLOW_rule__XSwitchExpression__DefaultAssignment_5_2_in_rule__XSwitchExpression__Group_5__2__Impl62397);
rule__XSwitchExpression__DefaultAssignment_5_2();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXSwitchExpressionAccess().getDefaultAssignment_5_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XSwitchExpression__Group_5__2__Impl"
// $ANTLR start "rule__XCasePart__Group__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30978:1: rule__XCasePart__Group__0 : rule__XCasePart__Group__0__Impl rule__XCasePart__Group__1 ;
public final void rule__XCasePart__Group__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30982:1: ( rule__XCasePart__Group__0__Impl rule__XCasePart__Group__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30983:2: rule__XCasePart__Group__0__Impl rule__XCasePart__Group__1
{
pushFollow(FollowSets002.FOLLOW_rule__XCasePart__Group__0__Impl_in_rule__XCasePart__Group__062433);
rule__XCasePart__Group__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XCasePart__Group__1_in_rule__XCasePart__Group__062436);
rule__XCasePart__Group__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XCasePart__Group__0"
// $ANTLR start "rule__XCasePart__Group__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30990:1: rule__XCasePart__Group__0__Impl : ( () ) ;
public final void rule__XCasePart__Group__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30994:1: ( ( () ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30995:1: ( () )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30995:1: ( () )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30996:1: ()
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXCasePartAccess().getXCasePartAction_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30997:1: ()
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30999:1:
{
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXCasePartAccess().getXCasePartAction_0());
}
}
}
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XCasePart__Group__0__Impl"
// $ANTLR start "rule__XCasePart__Group__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31009:1: rule__XCasePart__Group__1 : rule__XCasePart__Group__1__Impl rule__XCasePart__Group__2 ;
public final void rule__XCasePart__Group__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31013:1: ( rule__XCasePart__Group__1__Impl rule__XCasePart__Group__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31014:2: rule__XCasePart__Group__1__Impl rule__XCasePart__Group__2
{
pushFollow(FollowSets002.FOLLOW_rule__XCasePart__Group__1__Impl_in_rule__XCasePart__Group__162494);
rule__XCasePart__Group__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XCasePart__Group__2_in_rule__XCasePart__Group__162497);
rule__XCasePart__Group__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XCasePart__Group__1"
// $ANTLR start "rule__XCasePart__Group__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31021:1: rule__XCasePart__Group__1__Impl : ( ( rule__XCasePart__TypeGuardAssignment_1 )? ) ;
public final void rule__XCasePart__Group__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31025:1: ( ( ( rule__XCasePart__TypeGuardAssignment_1 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31026:1: ( ( rule__XCasePart__TypeGuardAssignment_1 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31026:1: ( ( rule__XCasePart__TypeGuardAssignment_1 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31027:1: ( rule__XCasePart__TypeGuardAssignment_1 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXCasePartAccess().getTypeGuardAssignment_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31028:1: ( rule__XCasePart__TypeGuardAssignment_1 )?
int alt229=2;
int LA229_0 = input.LA(1);
if ( (LA229_0==RULE_ID||LA229_0==31||LA229_0==144) ) {
alt229=1;
}
switch (alt229) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31028:2: rule__XCasePart__TypeGuardAssignment_1
{
pushFollow(FollowSets002.FOLLOW_rule__XCasePart__TypeGuardAssignment_1_in_rule__XCasePart__Group__1__Impl62524);
rule__XCasePart__TypeGuardAssignment_1();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXCasePartAccess().getTypeGuardAssignment_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XCasePart__Group__1__Impl"
// $ANTLR start "rule__XCasePart__Group__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31038:1: rule__XCasePart__Group__2 : rule__XCasePart__Group__2__Impl rule__XCasePart__Group__3 ;
public final void rule__XCasePart__Group__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31042:1: ( rule__XCasePart__Group__2__Impl rule__XCasePart__Group__3 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31043:2: rule__XCasePart__Group__2__Impl rule__XCasePart__Group__3
{
pushFollow(FollowSets002.FOLLOW_rule__XCasePart__Group__2__Impl_in_rule__XCasePart__Group__262555);
rule__XCasePart__Group__2__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XCasePart__Group__3_in_rule__XCasePart__Group__262558);
rule__XCasePart__Group__3();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XCasePart__Group__2"
// $ANTLR start "rule__XCasePart__Group__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31050:1: rule__XCasePart__Group__2__Impl : ( ( rule__XCasePart__Group_2__0 )? ) ;
public final void rule__XCasePart__Group__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31054:1: ( ( ( rule__XCasePart__Group_2__0 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31055:1: ( ( rule__XCasePart__Group_2__0 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31055:1: ( ( rule__XCasePart__Group_2__0 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31056:1: ( rule__XCasePart__Group_2__0 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXCasePartAccess().getGroup_2());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31057:1: ( rule__XCasePart__Group_2__0 )?
int alt230=2;
int LA230_0 = input.LA(1);
if ( (LA230_0==164) ) {
alt230=1;
}
switch (alt230) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31057:2: rule__XCasePart__Group_2__0
{
pushFollow(FollowSets002.FOLLOW_rule__XCasePart__Group_2__0_in_rule__XCasePart__Group__2__Impl62585);
rule__XCasePart__Group_2__0();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXCasePartAccess().getGroup_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XCasePart__Group__2__Impl"
// $ANTLR start "rule__XCasePart__Group__3"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31067:1: rule__XCasePart__Group__3 : rule__XCasePart__Group__3__Impl ;
public final void rule__XCasePart__Group__3() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31071:1: ( rule__XCasePart__Group__3__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31072:2: rule__XCasePart__Group__3__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__XCasePart__Group__3__Impl_in_rule__XCasePart__Group__362616);
rule__XCasePart__Group__3__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XCasePart__Group__3"
// $ANTLR start "rule__XCasePart__Group__3__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31078:1: rule__XCasePart__Group__3__Impl : ( ( rule__XCasePart__Alternatives_3 ) ) ;
public final void rule__XCasePart__Group__3__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31082:1: ( ( ( rule__XCasePart__Alternatives_3 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31083:1: ( ( rule__XCasePart__Alternatives_3 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31083:1: ( ( rule__XCasePart__Alternatives_3 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31084:1: ( rule__XCasePart__Alternatives_3 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXCasePartAccess().getAlternatives_3());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31085:1: ( rule__XCasePart__Alternatives_3 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31085:2: rule__XCasePart__Alternatives_3
{
pushFollow(FollowSets002.FOLLOW_rule__XCasePart__Alternatives_3_in_rule__XCasePart__Group__3__Impl62643);
rule__XCasePart__Alternatives_3();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXCasePartAccess().getAlternatives_3());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XCasePart__Group__3__Impl"
// $ANTLR start "rule__XCasePart__Group_2__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31103:1: rule__XCasePart__Group_2__0 : rule__XCasePart__Group_2__0__Impl rule__XCasePart__Group_2__1 ;
public final void rule__XCasePart__Group_2__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31107:1: ( rule__XCasePart__Group_2__0__Impl rule__XCasePart__Group_2__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31108:2: rule__XCasePart__Group_2__0__Impl rule__XCasePart__Group_2__1
{
pushFollow(FollowSets002.FOLLOW_rule__XCasePart__Group_2__0__Impl_in_rule__XCasePart__Group_2__062681);
rule__XCasePart__Group_2__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XCasePart__Group_2__1_in_rule__XCasePart__Group_2__062684);
rule__XCasePart__Group_2__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XCasePart__Group_2__0"
// $ANTLR start "rule__XCasePart__Group_2__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31115:1: rule__XCasePart__Group_2__0__Impl : ( 'case' ) ;
public final void rule__XCasePart__Group_2__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31119:1: ( ( 'case' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31120:1: ( 'case' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31120:1: ( 'case' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31121:1: 'case'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXCasePartAccess().getCaseKeyword_2_0());
}
match(input,164,FollowSets002.FOLLOW_164_in_rule__XCasePart__Group_2__0__Impl62712); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXCasePartAccess().getCaseKeyword_2_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XCasePart__Group_2__0__Impl"
// $ANTLR start "rule__XCasePart__Group_2__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31134:1: rule__XCasePart__Group_2__1 : rule__XCasePart__Group_2__1__Impl ;
public final void rule__XCasePart__Group_2__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31138:1: ( rule__XCasePart__Group_2__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31139:2: rule__XCasePart__Group_2__1__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__XCasePart__Group_2__1__Impl_in_rule__XCasePart__Group_2__162743);
rule__XCasePart__Group_2__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XCasePart__Group_2__1"
// $ANTLR start "rule__XCasePart__Group_2__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31145:1: rule__XCasePart__Group_2__1__Impl : ( ( rule__XCasePart__CaseAssignment_2_1 ) ) ;
public final void rule__XCasePart__Group_2__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31149:1: ( ( ( rule__XCasePart__CaseAssignment_2_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31150:1: ( ( rule__XCasePart__CaseAssignment_2_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31150:1: ( ( rule__XCasePart__CaseAssignment_2_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31151:1: ( rule__XCasePart__CaseAssignment_2_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXCasePartAccess().getCaseAssignment_2_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31152:1: ( rule__XCasePart__CaseAssignment_2_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31152:2: rule__XCasePart__CaseAssignment_2_1
{
pushFollow(FollowSets002.FOLLOW_rule__XCasePart__CaseAssignment_2_1_in_rule__XCasePart__Group_2__1__Impl62770);
rule__XCasePart__CaseAssignment_2_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXCasePartAccess().getCaseAssignment_2_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XCasePart__Group_2__1__Impl"
// $ANTLR start "rule__XCasePart__Group_3_0__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31166:1: rule__XCasePart__Group_3_0__0 : rule__XCasePart__Group_3_0__0__Impl rule__XCasePart__Group_3_0__1 ;
public final void rule__XCasePart__Group_3_0__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31170:1: ( rule__XCasePart__Group_3_0__0__Impl rule__XCasePart__Group_3_0__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31171:2: rule__XCasePart__Group_3_0__0__Impl rule__XCasePart__Group_3_0__1
{
pushFollow(FollowSets002.FOLLOW_rule__XCasePart__Group_3_0__0__Impl_in_rule__XCasePart__Group_3_0__062804);
rule__XCasePart__Group_3_0__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XCasePart__Group_3_0__1_in_rule__XCasePart__Group_3_0__062807);
rule__XCasePart__Group_3_0__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XCasePart__Group_3_0__0"
// $ANTLR start "rule__XCasePart__Group_3_0__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31178:1: rule__XCasePart__Group_3_0__0__Impl : ( ':' ) ;
public final void rule__XCasePart__Group_3_0__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31182:1: ( ( ':' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31183:1: ( ':' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31183:1: ( ':' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31184:1: ':'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXCasePartAccess().getColonKeyword_3_0_0());
}
match(input,162,FollowSets002.FOLLOW_162_in_rule__XCasePart__Group_3_0__0__Impl62835); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXCasePartAccess().getColonKeyword_3_0_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XCasePart__Group_3_0__0__Impl"
// $ANTLR start "rule__XCasePart__Group_3_0__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31197:1: rule__XCasePart__Group_3_0__1 : rule__XCasePart__Group_3_0__1__Impl ;
public final void rule__XCasePart__Group_3_0__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31201:1: ( rule__XCasePart__Group_3_0__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31202:2: rule__XCasePart__Group_3_0__1__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__XCasePart__Group_3_0__1__Impl_in_rule__XCasePart__Group_3_0__162866);
rule__XCasePart__Group_3_0__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XCasePart__Group_3_0__1"
// $ANTLR start "rule__XCasePart__Group_3_0__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31208:1: rule__XCasePart__Group_3_0__1__Impl : ( ( rule__XCasePart__ThenAssignment_3_0_1 ) ) ;
public final void rule__XCasePart__Group_3_0__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31212:1: ( ( ( rule__XCasePart__ThenAssignment_3_0_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31213:1: ( ( rule__XCasePart__ThenAssignment_3_0_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31213:1: ( ( rule__XCasePart__ThenAssignment_3_0_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31214:1: ( rule__XCasePart__ThenAssignment_3_0_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXCasePartAccess().getThenAssignment_3_0_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31215:1: ( rule__XCasePart__ThenAssignment_3_0_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31215:2: rule__XCasePart__ThenAssignment_3_0_1
{
pushFollow(FollowSets002.FOLLOW_rule__XCasePart__ThenAssignment_3_0_1_in_rule__XCasePart__Group_3_0__1__Impl62893);
rule__XCasePart__ThenAssignment_3_0_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXCasePartAccess().getThenAssignment_3_0_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XCasePart__Group_3_0__1__Impl"
// $ANTLR start "rule__XForLoopExpression__Group__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31229:1: rule__XForLoopExpression__Group__0 : rule__XForLoopExpression__Group__0__Impl rule__XForLoopExpression__Group__1 ;
public final void rule__XForLoopExpression__Group__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31233:1: ( rule__XForLoopExpression__Group__0__Impl rule__XForLoopExpression__Group__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31234:2: rule__XForLoopExpression__Group__0__Impl rule__XForLoopExpression__Group__1
{
pushFollow(FollowSets002.FOLLOW_rule__XForLoopExpression__Group__0__Impl_in_rule__XForLoopExpression__Group__062927);
rule__XForLoopExpression__Group__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XForLoopExpression__Group__1_in_rule__XForLoopExpression__Group__062930);
rule__XForLoopExpression__Group__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XForLoopExpression__Group__0"
// $ANTLR start "rule__XForLoopExpression__Group__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31241:1: rule__XForLoopExpression__Group__0__Impl : ( ( rule__XForLoopExpression__Group_0__0 ) ) ;
public final void rule__XForLoopExpression__Group__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31245:1: ( ( ( rule__XForLoopExpression__Group_0__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31246:1: ( ( rule__XForLoopExpression__Group_0__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31246:1: ( ( rule__XForLoopExpression__Group_0__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31247:1: ( rule__XForLoopExpression__Group_0__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXForLoopExpressionAccess().getGroup_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31248:1: ( rule__XForLoopExpression__Group_0__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31248:2: rule__XForLoopExpression__Group_0__0
{
pushFollow(FollowSets002.FOLLOW_rule__XForLoopExpression__Group_0__0_in_rule__XForLoopExpression__Group__0__Impl62957);
rule__XForLoopExpression__Group_0__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXForLoopExpressionAccess().getGroup_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XForLoopExpression__Group__0__Impl"
// $ANTLR start "rule__XForLoopExpression__Group__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31258:1: rule__XForLoopExpression__Group__1 : rule__XForLoopExpression__Group__1__Impl rule__XForLoopExpression__Group__2 ;
public final void rule__XForLoopExpression__Group__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31262:1: ( rule__XForLoopExpression__Group__1__Impl rule__XForLoopExpression__Group__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31263:2: rule__XForLoopExpression__Group__1__Impl rule__XForLoopExpression__Group__2
{
pushFollow(FollowSets002.FOLLOW_rule__XForLoopExpression__Group__1__Impl_in_rule__XForLoopExpression__Group__162987);
rule__XForLoopExpression__Group__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XForLoopExpression__Group__2_in_rule__XForLoopExpression__Group__162990);
rule__XForLoopExpression__Group__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XForLoopExpression__Group__1"
// $ANTLR start "rule__XForLoopExpression__Group__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31270:1: rule__XForLoopExpression__Group__1__Impl : ( ( rule__XForLoopExpression__ForExpressionAssignment_1 ) ) ;
public final void rule__XForLoopExpression__Group__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31274:1: ( ( ( rule__XForLoopExpression__ForExpressionAssignment_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31275:1: ( ( rule__XForLoopExpression__ForExpressionAssignment_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31275:1: ( ( rule__XForLoopExpression__ForExpressionAssignment_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31276:1: ( rule__XForLoopExpression__ForExpressionAssignment_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXForLoopExpressionAccess().getForExpressionAssignment_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31277:1: ( rule__XForLoopExpression__ForExpressionAssignment_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31277:2: rule__XForLoopExpression__ForExpressionAssignment_1
{
pushFollow(FollowSets002.FOLLOW_rule__XForLoopExpression__ForExpressionAssignment_1_in_rule__XForLoopExpression__Group__1__Impl63017);
rule__XForLoopExpression__ForExpressionAssignment_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXForLoopExpressionAccess().getForExpressionAssignment_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XForLoopExpression__Group__1__Impl"
// $ANTLR start "rule__XForLoopExpression__Group__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31287:1: rule__XForLoopExpression__Group__2 : rule__XForLoopExpression__Group__2__Impl rule__XForLoopExpression__Group__3 ;
public final void rule__XForLoopExpression__Group__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31291:1: ( rule__XForLoopExpression__Group__2__Impl rule__XForLoopExpression__Group__3 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31292:2: rule__XForLoopExpression__Group__2__Impl rule__XForLoopExpression__Group__3
{
pushFollow(FollowSets002.FOLLOW_rule__XForLoopExpression__Group__2__Impl_in_rule__XForLoopExpression__Group__263047);
rule__XForLoopExpression__Group__2__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XForLoopExpression__Group__3_in_rule__XForLoopExpression__Group__263050);
rule__XForLoopExpression__Group__3();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XForLoopExpression__Group__2"
// $ANTLR start "rule__XForLoopExpression__Group__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31299:1: rule__XForLoopExpression__Group__2__Impl : ( ')' ) ;
public final void rule__XForLoopExpression__Group__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31303:1: ( ( ')' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31304:1: ( ')' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31304:1: ( ')' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31305:1: ')'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXForLoopExpressionAccess().getRightParenthesisKeyword_2());
}
match(input,145,FollowSets002.FOLLOW_145_in_rule__XForLoopExpression__Group__2__Impl63078); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXForLoopExpressionAccess().getRightParenthesisKeyword_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XForLoopExpression__Group__2__Impl"
// $ANTLR start "rule__XForLoopExpression__Group__3"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31318:1: rule__XForLoopExpression__Group__3 : rule__XForLoopExpression__Group__3__Impl ;
public final void rule__XForLoopExpression__Group__3() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31322:1: ( rule__XForLoopExpression__Group__3__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31323:2: rule__XForLoopExpression__Group__3__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__XForLoopExpression__Group__3__Impl_in_rule__XForLoopExpression__Group__363109);
rule__XForLoopExpression__Group__3__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XForLoopExpression__Group__3"
// $ANTLR start "rule__XForLoopExpression__Group__3__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31329:1: rule__XForLoopExpression__Group__3__Impl : ( ( rule__XForLoopExpression__EachExpressionAssignment_3 ) ) ;
public final void rule__XForLoopExpression__Group__3__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31333:1: ( ( ( rule__XForLoopExpression__EachExpressionAssignment_3 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31334:1: ( ( rule__XForLoopExpression__EachExpressionAssignment_3 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31334:1: ( ( rule__XForLoopExpression__EachExpressionAssignment_3 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31335:1: ( rule__XForLoopExpression__EachExpressionAssignment_3 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXForLoopExpressionAccess().getEachExpressionAssignment_3());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31336:1: ( rule__XForLoopExpression__EachExpressionAssignment_3 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31336:2: rule__XForLoopExpression__EachExpressionAssignment_3
{
pushFollow(FollowSets002.FOLLOW_rule__XForLoopExpression__EachExpressionAssignment_3_in_rule__XForLoopExpression__Group__3__Impl63136);
rule__XForLoopExpression__EachExpressionAssignment_3();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXForLoopExpressionAccess().getEachExpressionAssignment_3());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XForLoopExpression__Group__3__Impl"
// $ANTLR start "rule__XForLoopExpression__Group_0__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31354:1: rule__XForLoopExpression__Group_0__0 : rule__XForLoopExpression__Group_0__0__Impl ;
public final void rule__XForLoopExpression__Group_0__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31358:1: ( rule__XForLoopExpression__Group_0__0__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31359:2: rule__XForLoopExpression__Group_0__0__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__XForLoopExpression__Group_0__0__Impl_in_rule__XForLoopExpression__Group_0__063174);
rule__XForLoopExpression__Group_0__0__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XForLoopExpression__Group_0__0"
// $ANTLR start "rule__XForLoopExpression__Group_0__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31365:1: rule__XForLoopExpression__Group_0__0__Impl : ( ( rule__XForLoopExpression__Group_0_0__0 ) ) ;
public final void rule__XForLoopExpression__Group_0__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31369:1: ( ( ( rule__XForLoopExpression__Group_0_0__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31370:1: ( ( rule__XForLoopExpression__Group_0_0__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31370:1: ( ( rule__XForLoopExpression__Group_0_0__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31371:1: ( rule__XForLoopExpression__Group_0_0__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXForLoopExpressionAccess().getGroup_0_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31372:1: ( rule__XForLoopExpression__Group_0_0__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31372:2: rule__XForLoopExpression__Group_0_0__0
{
pushFollow(FollowSets002.FOLLOW_rule__XForLoopExpression__Group_0_0__0_in_rule__XForLoopExpression__Group_0__0__Impl63201);
rule__XForLoopExpression__Group_0_0__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXForLoopExpressionAccess().getGroup_0_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XForLoopExpression__Group_0__0__Impl"
// $ANTLR start "rule__XForLoopExpression__Group_0_0__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31384:1: rule__XForLoopExpression__Group_0_0__0 : rule__XForLoopExpression__Group_0_0__0__Impl rule__XForLoopExpression__Group_0_0__1 ;
public final void rule__XForLoopExpression__Group_0_0__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31388:1: ( rule__XForLoopExpression__Group_0_0__0__Impl rule__XForLoopExpression__Group_0_0__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31389:2: rule__XForLoopExpression__Group_0_0__0__Impl rule__XForLoopExpression__Group_0_0__1
{
pushFollow(FollowSets002.FOLLOW_rule__XForLoopExpression__Group_0_0__0__Impl_in_rule__XForLoopExpression__Group_0_0__063233);
rule__XForLoopExpression__Group_0_0__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XForLoopExpression__Group_0_0__1_in_rule__XForLoopExpression__Group_0_0__063236);
rule__XForLoopExpression__Group_0_0__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XForLoopExpression__Group_0_0__0"
// $ANTLR start "rule__XForLoopExpression__Group_0_0__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31396:1: rule__XForLoopExpression__Group_0_0__0__Impl : ( () ) ;
public final void rule__XForLoopExpression__Group_0_0__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31400:1: ( ( () ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31401:1: ( () )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31401:1: ( () )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31402:1: ()
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXForLoopExpressionAccess().getXForLoopExpressionAction_0_0_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31403:1: ()
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31405:1:
{
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXForLoopExpressionAccess().getXForLoopExpressionAction_0_0_0());
}
}
}
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XForLoopExpression__Group_0_0__0__Impl"
// $ANTLR start "rule__XForLoopExpression__Group_0_0__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31415:1: rule__XForLoopExpression__Group_0_0__1 : rule__XForLoopExpression__Group_0_0__1__Impl rule__XForLoopExpression__Group_0_0__2 ;
public final void rule__XForLoopExpression__Group_0_0__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31419:1: ( rule__XForLoopExpression__Group_0_0__1__Impl rule__XForLoopExpression__Group_0_0__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31420:2: rule__XForLoopExpression__Group_0_0__1__Impl rule__XForLoopExpression__Group_0_0__2
{
pushFollow(FollowSets002.FOLLOW_rule__XForLoopExpression__Group_0_0__1__Impl_in_rule__XForLoopExpression__Group_0_0__163294);
rule__XForLoopExpression__Group_0_0__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XForLoopExpression__Group_0_0__2_in_rule__XForLoopExpression__Group_0_0__163297);
rule__XForLoopExpression__Group_0_0__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XForLoopExpression__Group_0_0__1"
// $ANTLR start "rule__XForLoopExpression__Group_0_0__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31427:1: rule__XForLoopExpression__Group_0_0__1__Impl : ( 'for' ) ;
public final void rule__XForLoopExpression__Group_0_0__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31431:1: ( ( 'for' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31432:1: ( 'for' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31432:1: ( 'for' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31433:1: 'for'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXForLoopExpressionAccess().getForKeyword_0_0_1());
}
match(input,165,FollowSets002.FOLLOW_165_in_rule__XForLoopExpression__Group_0_0__1__Impl63325); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXForLoopExpressionAccess().getForKeyword_0_0_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XForLoopExpression__Group_0_0__1__Impl"
// $ANTLR start "rule__XForLoopExpression__Group_0_0__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31446:1: rule__XForLoopExpression__Group_0_0__2 : rule__XForLoopExpression__Group_0_0__2__Impl rule__XForLoopExpression__Group_0_0__3 ;
public final void rule__XForLoopExpression__Group_0_0__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31450:1: ( rule__XForLoopExpression__Group_0_0__2__Impl rule__XForLoopExpression__Group_0_0__3 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31451:2: rule__XForLoopExpression__Group_0_0__2__Impl rule__XForLoopExpression__Group_0_0__3
{
pushFollow(FollowSets002.FOLLOW_rule__XForLoopExpression__Group_0_0__2__Impl_in_rule__XForLoopExpression__Group_0_0__263356);
rule__XForLoopExpression__Group_0_0__2__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XForLoopExpression__Group_0_0__3_in_rule__XForLoopExpression__Group_0_0__263359);
rule__XForLoopExpression__Group_0_0__3();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XForLoopExpression__Group_0_0__2"
// $ANTLR start "rule__XForLoopExpression__Group_0_0__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31458:1: rule__XForLoopExpression__Group_0_0__2__Impl : ( '(' ) ;
public final void rule__XForLoopExpression__Group_0_0__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31462:1: ( ( '(' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31463:1: ( '(' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31463:1: ( '(' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31464:1: '('
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXForLoopExpressionAccess().getLeftParenthesisKeyword_0_0_2());
}
match(input,144,FollowSets002.FOLLOW_144_in_rule__XForLoopExpression__Group_0_0__2__Impl63387); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXForLoopExpressionAccess().getLeftParenthesisKeyword_0_0_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XForLoopExpression__Group_0_0__2__Impl"
// $ANTLR start "rule__XForLoopExpression__Group_0_0__3"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31477:1: rule__XForLoopExpression__Group_0_0__3 : rule__XForLoopExpression__Group_0_0__3__Impl rule__XForLoopExpression__Group_0_0__4 ;
public final void rule__XForLoopExpression__Group_0_0__3() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31481:1: ( rule__XForLoopExpression__Group_0_0__3__Impl rule__XForLoopExpression__Group_0_0__4 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31482:2: rule__XForLoopExpression__Group_0_0__3__Impl rule__XForLoopExpression__Group_0_0__4
{
pushFollow(FollowSets002.FOLLOW_rule__XForLoopExpression__Group_0_0__3__Impl_in_rule__XForLoopExpression__Group_0_0__363418);
rule__XForLoopExpression__Group_0_0__3__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XForLoopExpression__Group_0_0__4_in_rule__XForLoopExpression__Group_0_0__363421);
rule__XForLoopExpression__Group_0_0__4();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XForLoopExpression__Group_0_0__3"
// $ANTLR start "rule__XForLoopExpression__Group_0_0__3__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31489:1: rule__XForLoopExpression__Group_0_0__3__Impl : ( ( rule__XForLoopExpression__DeclaredParamAssignment_0_0_3 ) ) ;
public final void rule__XForLoopExpression__Group_0_0__3__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31493:1: ( ( ( rule__XForLoopExpression__DeclaredParamAssignment_0_0_3 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31494:1: ( ( rule__XForLoopExpression__DeclaredParamAssignment_0_0_3 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31494:1: ( ( rule__XForLoopExpression__DeclaredParamAssignment_0_0_3 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31495:1: ( rule__XForLoopExpression__DeclaredParamAssignment_0_0_3 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXForLoopExpressionAccess().getDeclaredParamAssignment_0_0_3());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31496:1: ( rule__XForLoopExpression__DeclaredParamAssignment_0_0_3 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31496:2: rule__XForLoopExpression__DeclaredParamAssignment_0_0_3
{
pushFollow(FollowSets002.FOLLOW_rule__XForLoopExpression__DeclaredParamAssignment_0_0_3_in_rule__XForLoopExpression__Group_0_0__3__Impl63448);
rule__XForLoopExpression__DeclaredParamAssignment_0_0_3();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXForLoopExpressionAccess().getDeclaredParamAssignment_0_0_3());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XForLoopExpression__Group_0_0__3__Impl"
// $ANTLR start "rule__XForLoopExpression__Group_0_0__4"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31506:1: rule__XForLoopExpression__Group_0_0__4 : rule__XForLoopExpression__Group_0_0__4__Impl ;
public final void rule__XForLoopExpression__Group_0_0__4() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31510:1: ( rule__XForLoopExpression__Group_0_0__4__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31511:2: rule__XForLoopExpression__Group_0_0__4__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__XForLoopExpression__Group_0_0__4__Impl_in_rule__XForLoopExpression__Group_0_0__463478);
rule__XForLoopExpression__Group_0_0__4__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XForLoopExpression__Group_0_0__4"
// $ANTLR start "rule__XForLoopExpression__Group_0_0__4__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31517:1: rule__XForLoopExpression__Group_0_0__4__Impl : ( ':' ) ;
public final void rule__XForLoopExpression__Group_0_0__4__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31521:1: ( ( ':' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31522:1: ( ':' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31522:1: ( ':' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31523:1: ':'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXForLoopExpressionAccess().getColonKeyword_0_0_4());
}
match(input,162,FollowSets002.FOLLOW_162_in_rule__XForLoopExpression__Group_0_0__4__Impl63506); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXForLoopExpressionAccess().getColonKeyword_0_0_4());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XForLoopExpression__Group_0_0__4__Impl"
// $ANTLR start "rule__XBasicForLoopExpression__Group__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31546:1: rule__XBasicForLoopExpression__Group__0 : rule__XBasicForLoopExpression__Group__0__Impl rule__XBasicForLoopExpression__Group__1 ;
public final void rule__XBasicForLoopExpression__Group__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31550:1: ( rule__XBasicForLoopExpression__Group__0__Impl rule__XBasicForLoopExpression__Group__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31551:2: rule__XBasicForLoopExpression__Group__0__Impl rule__XBasicForLoopExpression__Group__1
{
pushFollow(FollowSets002.FOLLOW_rule__XBasicForLoopExpression__Group__0__Impl_in_rule__XBasicForLoopExpression__Group__063547);
rule__XBasicForLoopExpression__Group__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XBasicForLoopExpression__Group__1_in_rule__XBasicForLoopExpression__Group__063550);
rule__XBasicForLoopExpression__Group__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XBasicForLoopExpression__Group__0"
// $ANTLR start "rule__XBasicForLoopExpression__Group__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31558:1: rule__XBasicForLoopExpression__Group__0__Impl : ( () ) ;
public final void rule__XBasicForLoopExpression__Group__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31562:1: ( ( () ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31563:1: ( () )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31563:1: ( () )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31564:1: ()
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXBasicForLoopExpressionAccess().getXBasicForLoopExpressionAction_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31565:1: ()
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31567:1:
{
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXBasicForLoopExpressionAccess().getXBasicForLoopExpressionAction_0());
}
}
}
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XBasicForLoopExpression__Group__0__Impl"
// $ANTLR start "rule__XBasicForLoopExpression__Group__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31577:1: rule__XBasicForLoopExpression__Group__1 : rule__XBasicForLoopExpression__Group__1__Impl rule__XBasicForLoopExpression__Group__2 ;
public final void rule__XBasicForLoopExpression__Group__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31581:1: ( rule__XBasicForLoopExpression__Group__1__Impl rule__XBasicForLoopExpression__Group__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31582:2: rule__XBasicForLoopExpression__Group__1__Impl rule__XBasicForLoopExpression__Group__2
{
pushFollow(FollowSets002.FOLLOW_rule__XBasicForLoopExpression__Group__1__Impl_in_rule__XBasicForLoopExpression__Group__163608);
rule__XBasicForLoopExpression__Group__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XBasicForLoopExpression__Group__2_in_rule__XBasicForLoopExpression__Group__163611);
rule__XBasicForLoopExpression__Group__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XBasicForLoopExpression__Group__1"
// $ANTLR start "rule__XBasicForLoopExpression__Group__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31589:1: rule__XBasicForLoopExpression__Group__1__Impl : ( 'for' ) ;
public final void rule__XBasicForLoopExpression__Group__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31593:1: ( ( 'for' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31594:1: ( 'for' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31594:1: ( 'for' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31595:1: 'for'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXBasicForLoopExpressionAccess().getForKeyword_1());
}
match(input,165,FollowSets002.FOLLOW_165_in_rule__XBasicForLoopExpression__Group__1__Impl63639); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXBasicForLoopExpressionAccess().getForKeyword_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XBasicForLoopExpression__Group__1__Impl"
// $ANTLR start "rule__XBasicForLoopExpression__Group__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31608:1: rule__XBasicForLoopExpression__Group__2 : rule__XBasicForLoopExpression__Group__2__Impl rule__XBasicForLoopExpression__Group__3 ;
public final void rule__XBasicForLoopExpression__Group__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31612:1: ( rule__XBasicForLoopExpression__Group__2__Impl rule__XBasicForLoopExpression__Group__3 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31613:2: rule__XBasicForLoopExpression__Group__2__Impl rule__XBasicForLoopExpression__Group__3
{
pushFollow(FollowSets002.FOLLOW_rule__XBasicForLoopExpression__Group__2__Impl_in_rule__XBasicForLoopExpression__Group__263670);
rule__XBasicForLoopExpression__Group__2__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XBasicForLoopExpression__Group__3_in_rule__XBasicForLoopExpression__Group__263673);
rule__XBasicForLoopExpression__Group__3();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XBasicForLoopExpression__Group__2"
// $ANTLR start "rule__XBasicForLoopExpression__Group__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31620:1: rule__XBasicForLoopExpression__Group__2__Impl : ( '(' ) ;
public final void rule__XBasicForLoopExpression__Group__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31624:1: ( ( '(' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31625:1: ( '(' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31625:1: ( '(' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31626:1: '('
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXBasicForLoopExpressionAccess().getLeftParenthesisKeyword_2());
}
match(input,144,FollowSets002.FOLLOW_144_in_rule__XBasicForLoopExpression__Group__2__Impl63701); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXBasicForLoopExpressionAccess().getLeftParenthesisKeyword_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XBasicForLoopExpression__Group__2__Impl"
// $ANTLR start "rule__XBasicForLoopExpression__Group__3"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31639:1: rule__XBasicForLoopExpression__Group__3 : rule__XBasicForLoopExpression__Group__3__Impl rule__XBasicForLoopExpression__Group__4 ;
public final void rule__XBasicForLoopExpression__Group__3() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31643:1: ( rule__XBasicForLoopExpression__Group__3__Impl rule__XBasicForLoopExpression__Group__4 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31644:2: rule__XBasicForLoopExpression__Group__3__Impl rule__XBasicForLoopExpression__Group__4
{
pushFollow(FollowSets002.FOLLOW_rule__XBasicForLoopExpression__Group__3__Impl_in_rule__XBasicForLoopExpression__Group__363732);
rule__XBasicForLoopExpression__Group__3__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XBasicForLoopExpression__Group__4_in_rule__XBasicForLoopExpression__Group__363735);
rule__XBasicForLoopExpression__Group__4();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XBasicForLoopExpression__Group__3"
// $ANTLR start "rule__XBasicForLoopExpression__Group__3__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31651:1: rule__XBasicForLoopExpression__Group__3__Impl : ( ( rule__XBasicForLoopExpression__Group_3__0 )? ) ;
public final void rule__XBasicForLoopExpression__Group__3__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31655:1: ( ( ( rule__XBasicForLoopExpression__Group_3__0 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31656:1: ( ( rule__XBasicForLoopExpression__Group_3__0 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31656:1: ( ( rule__XBasicForLoopExpression__Group_3__0 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31657:1: ( rule__XBasicForLoopExpression__Group_3__0 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXBasicForLoopExpressionAccess().getGroup_3());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31658:1: ( rule__XBasicForLoopExpression__Group_3__0 )?
int alt231=2;
int LA231_0 = input.LA(1);
if ( ((LA231_0>=RULE_ID && LA231_0<=RULE_STRING)||LA231_0==27||(LA231_0>=34 && LA231_0<=35)||LA231_0==40||(LA231_0>=44 && LA231_0<=50)||LA231_0==67||LA231_0==144||(LA231_0>=155 && LA231_0<=156)||LA231_0==159||LA231_0==161||(LA231_0>=165 && LA231_0<=173)||LA231_0==175||(LA231_0>=184 && LA231_0<=185)) ) {
alt231=1;
}
switch (alt231) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31658:2: rule__XBasicForLoopExpression__Group_3__0
{
pushFollow(FollowSets002.FOLLOW_rule__XBasicForLoopExpression__Group_3__0_in_rule__XBasicForLoopExpression__Group__3__Impl63762);
rule__XBasicForLoopExpression__Group_3__0();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXBasicForLoopExpressionAccess().getGroup_3());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XBasicForLoopExpression__Group__3__Impl"
// $ANTLR start "rule__XBasicForLoopExpression__Group__4"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31668:1: rule__XBasicForLoopExpression__Group__4 : rule__XBasicForLoopExpression__Group__4__Impl rule__XBasicForLoopExpression__Group__5 ;
public final void rule__XBasicForLoopExpression__Group__4() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31672:1: ( rule__XBasicForLoopExpression__Group__4__Impl rule__XBasicForLoopExpression__Group__5 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31673:2: rule__XBasicForLoopExpression__Group__4__Impl rule__XBasicForLoopExpression__Group__5
{
pushFollow(FollowSets002.FOLLOW_rule__XBasicForLoopExpression__Group__4__Impl_in_rule__XBasicForLoopExpression__Group__463793);
rule__XBasicForLoopExpression__Group__4__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XBasicForLoopExpression__Group__5_in_rule__XBasicForLoopExpression__Group__463796);
rule__XBasicForLoopExpression__Group__5();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XBasicForLoopExpression__Group__4"
// $ANTLR start "rule__XBasicForLoopExpression__Group__4__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31680:1: rule__XBasicForLoopExpression__Group__4__Impl : ( ';' ) ;
public final void rule__XBasicForLoopExpression__Group__4__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31684:1: ( ( ';' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31685:1: ( ';' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31685:1: ( ';' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31686:1: ';'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXBasicForLoopExpressionAccess().getSemicolonKeyword_4());
}
match(input,158,FollowSets002.FOLLOW_158_in_rule__XBasicForLoopExpression__Group__4__Impl63824); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXBasicForLoopExpressionAccess().getSemicolonKeyword_4());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XBasicForLoopExpression__Group__4__Impl"
// $ANTLR start "rule__XBasicForLoopExpression__Group__5"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31699:1: rule__XBasicForLoopExpression__Group__5 : rule__XBasicForLoopExpression__Group__5__Impl rule__XBasicForLoopExpression__Group__6 ;
public final void rule__XBasicForLoopExpression__Group__5() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31703:1: ( rule__XBasicForLoopExpression__Group__5__Impl rule__XBasicForLoopExpression__Group__6 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31704:2: rule__XBasicForLoopExpression__Group__5__Impl rule__XBasicForLoopExpression__Group__6
{
pushFollow(FollowSets002.FOLLOW_rule__XBasicForLoopExpression__Group__5__Impl_in_rule__XBasicForLoopExpression__Group__563855);
rule__XBasicForLoopExpression__Group__5__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XBasicForLoopExpression__Group__6_in_rule__XBasicForLoopExpression__Group__563858);
rule__XBasicForLoopExpression__Group__6();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XBasicForLoopExpression__Group__5"
// $ANTLR start "rule__XBasicForLoopExpression__Group__5__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31711:1: rule__XBasicForLoopExpression__Group__5__Impl : ( ( rule__XBasicForLoopExpression__ExpressionAssignment_5 )? ) ;
public final void rule__XBasicForLoopExpression__Group__5__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31715:1: ( ( ( rule__XBasicForLoopExpression__ExpressionAssignment_5 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31716:1: ( ( rule__XBasicForLoopExpression__ExpressionAssignment_5 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31716:1: ( ( rule__XBasicForLoopExpression__ExpressionAssignment_5 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31717:1: ( rule__XBasicForLoopExpression__ExpressionAssignment_5 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXBasicForLoopExpressionAccess().getExpressionAssignment_5());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31718:1: ( rule__XBasicForLoopExpression__ExpressionAssignment_5 )?
int alt232=2;
int LA232_0 = input.LA(1);
if ( ((LA232_0>=RULE_ID && LA232_0<=RULE_STRING)||LA232_0==27||(LA232_0>=34 && LA232_0<=35)||LA232_0==40||(LA232_0>=45 && LA232_0<=50)||LA232_0==67||LA232_0==144||(LA232_0>=155 && LA232_0<=156)||LA232_0==159||LA232_0==161||(LA232_0>=165 && LA232_0<=173)||LA232_0==175||LA232_0==185) ) {
alt232=1;
}
switch (alt232) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31718:2: rule__XBasicForLoopExpression__ExpressionAssignment_5
{
pushFollow(FollowSets002.FOLLOW_rule__XBasicForLoopExpression__ExpressionAssignment_5_in_rule__XBasicForLoopExpression__Group__5__Impl63885);
rule__XBasicForLoopExpression__ExpressionAssignment_5();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXBasicForLoopExpressionAccess().getExpressionAssignment_5());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XBasicForLoopExpression__Group__5__Impl"
// $ANTLR start "rule__XBasicForLoopExpression__Group__6"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31728:1: rule__XBasicForLoopExpression__Group__6 : rule__XBasicForLoopExpression__Group__6__Impl rule__XBasicForLoopExpression__Group__7 ;
public final void rule__XBasicForLoopExpression__Group__6() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31732:1: ( rule__XBasicForLoopExpression__Group__6__Impl rule__XBasicForLoopExpression__Group__7 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31733:2: rule__XBasicForLoopExpression__Group__6__Impl rule__XBasicForLoopExpression__Group__7
{
pushFollow(FollowSets002.FOLLOW_rule__XBasicForLoopExpression__Group__6__Impl_in_rule__XBasicForLoopExpression__Group__663916);
rule__XBasicForLoopExpression__Group__6__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XBasicForLoopExpression__Group__7_in_rule__XBasicForLoopExpression__Group__663919);
rule__XBasicForLoopExpression__Group__7();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XBasicForLoopExpression__Group__6"
// $ANTLR start "rule__XBasicForLoopExpression__Group__6__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31740:1: rule__XBasicForLoopExpression__Group__6__Impl : ( ';' ) ;
public final void rule__XBasicForLoopExpression__Group__6__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31744:1: ( ( ';' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31745:1: ( ';' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31745:1: ( ';' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31746:1: ';'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXBasicForLoopExpressionAccess().getSemicolonKeyword_6());
}
match(input,158,FollowSets002.FOLLOW_158_in_rule__XBasicForLoopExpression__Group__6__Impl63947); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXBasicForLoopExpressionAccess().getSemicolonKeyword_6());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XBasicForLoopExpression__Group__6__Impl"
// $ANTLR start "rule__XBasicForLoopExpression__Group__7"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31759:1: rule__XBasicForLoopExpression__Group__7 : rule__XBasicForLoopExpression__Group__7__Impl rule__XBasicForLoopExpression__Group__8 ;
public final void rule__XBasicForLoopExpression__Group__7() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31763:1: ( rule__XBasicForLoopExpression__Group__7__Impl rule__XBasicForLoopExpression__Group__8 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31764:2: rule__XBasicForLoopExpression__Group__7__Impl rule__XBasicForLoopExpression__Group__8
{
pushFollow(FollowSets002.FOLLOW_rule__XBasicForLoopExpression__Group__7__Impl_in_rule__XBasicForLoopExpression__Group__763978);
rule__XBasicForLoopExpression__Group__7__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XBasicForLoopExpression__Group__8_in_rule__XBasicForLoopExpression__Group__763981);
rule__XBasicForLoopExpression__Group__8();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XBasicForLoopExpression__Group__7"
// $ANTLR start "rule__XBasicForLoopExpression__Group__7__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31771:1: rule__XBasicForLoopExpression__Group__7__Impl : ( ( rule__XBasicForLoopExpression__Group_7__0 )? ) ;
public final void rule__XBasicForLoopExpression__Group__7__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31775:1: ( ( ( rule__XBasicForLoopExpression__Group_7__0 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31776:1: ( ( rule__XBasicForLoopExpression__Group_7__0 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31776:1: ( ( rule__XBasicForLoopExpression__Group_7__0 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31777:1: ( rule__XBasicForLoopExpression__Group_7__0 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXBasicForLoopExpressionAccess().getGroup_7());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31778:1: ( rule__XBasicForLoopExpression__Group_7__0 )?
int alt233=2;
int LA233_0 = input.LA(1);
if ( ((LA233_0>=RULE_ID && LA233_0<=RULE_STRING)||LA233_0==27||(LA233_0>=34 && LA233_0<=35)||LA233_0==40||(LA233_0>=45 && LA233_0<=50)||LA233_0==67||LA233_0==144||(LA233_0>=155 && LA233_0<=156)||LA233_0==159||LA233_0==161||(LA233_0>=165 && LA233_0<=173)||LA233_0==175||LA233_0==185) ) {
alt233=1;
}
switch (alt233) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31778:2: rule__XBasicForLoopExpression__Group_7__0
{
pushFollow(FollowSets002.FOLLOW_rule__XBasicForLoopExpression__Group_7__0_in_rule__XBasicForLoopExpression__Group__7__Impl64008);
rule__XBasicForLoopExpression__Group_7__0();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXBasicForLoopExpressionAccess().getGroup_7());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XBasicForLoopExpression__Group__7__Impl"
// $ANTLR start "rule__XBasicForLoopExpression__Group__8"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31788:1: rule__XBasicForLoopExpression__Group__8 : rule__XBasicForLoopExpression__Group__8__Impl rule__XBasicForLoopExpression__Group__9 ;
public final void rule__XBasicForLoopExpression__Group__8() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31792:1: ( rule__XBasicForLoopExpression__Group__8__Impl rule__XBasicForLoopExpression__Group__9 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31793:2: rule__XBasicForLoopExpression__Group__8__Impl rule__XBasicForLoopExpression__Group__9
{
pushFollow(FollowSets002.FOLLOW_rule__XBasicForLoopExpression__Group__8__Impl_in_rule__XBasicForLoopExpression__Group__864039);
rule__XBasicForLoopExpression__Group__8__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XBasicForLoopExpression__Group__9_in_rule__XBasicForLoopExpression__Group__864042);
rule__XBasicForLoopExpression__Group__9();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XBasicForLoopExpression__Group__8"
// $ANTLR start "rule__XBasicForLoopExpression__Group__8__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31800:1: rule__XBasicForLoopExpression__Group__8__Impl : ( ')' ) ;
public final void rule__XBasicForLoopExpression__Group__8__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31804:1: ( ( ')' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31805:1: ( ')' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31805:1: ( ')' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31806:1: ')'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXBasicForLoopExpressionAccess().getRightParenthesisKeyword_8());
}
match(input,145,FollowSets002.FOLLOW_145_in_rule__XBasicForLoopExpression__Group__8__Impl64070); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXBasicForLoopExpressionAccess().getRightParenthesisKeyword_8());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XBasicForLoopExpression__Group__8__Impl"
// $ANTLR start "rule__XBasicForLoopExpression__Group__9"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31819:1: rule__XBasicForLoopExpression__Group__9 : rule__XBasicForLoopExpression__Group__9__Impl ;
public final void rule__XBasicForLoopExpression__Group__9() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31823:1: ( rule__XBasicForLoopExpression__Group__9__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31824:2: rule__XBasicForLoopExpression__Group__9__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__XBasicForLoopExpression__Group__9__Impl_in_rule__XBasicForLoopExpression__Group__964101);
rule__XBasicForLoopExpression__Group__9__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XBasicForLoopExpression__Group__9"
// $ANTLR start "rule__XBasicForLoopExpression__Group__9__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31830:1: rule__XBasicForLoopExpression__Group__9__Impl : ( ( rule__XBasicForLoopExpression__EachExpressionAssignment_9 ) ) ;
public final void rule__XBasicForLoopExpression__Group__9__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31834:1: ( ( ( rule__XBasicForLoopExpression__EachExpressionAssignment_9 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31835:1: ( ( rule__XBasicForLoopExpression__EachExpressionAssignment_9 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31835:1: ( ( rule__XBasicForLoopExpression__EachExpressionAssignment_9 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31836:1: ( rule__XBasicForLoopExpression__EachExpressionAssignment_9 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXBasicForLoopExpressionAccess().getEachExpressionAssignment_9());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31837:1: ( rule__XBasicForLoopExpression__EachExpressionAssignment_9 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31837:2: rule__XBasicForLoopExpression__EachExpressionAssignment_9
{
pushFollow(FollowSets002.FOLLOW_rule__XBasicForLoopExpression__EachExpressionAssignment_9_in_rule__XBasicForLoopExpression__Group__9__Impl64128);
rule__XBasicForLoopExpression__EachExpressionAssignment_9();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXBasicForLoopExpressionAccess().getEachExpressionAssignment_9());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XBasicForLoopExpression__Group__9__Impl"
// $ANTLR start "rule__XBasicForLoopExpression__Group_3__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31867:1: rule__XBasicForLoopExpression__Group_3__0 : rule__XBasicForLoopExpression__Group_3__0__Impl rule__XBasicForLoopExpression__Group_3__1 ;
public final void rule__XBasicForLoopExpression__Group_3__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31871:1: ( rule__XBasicForLoopExpression__Group_3__0__Impl rule__XBasicForLoopExpression__Group_3__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31872:2: rule__XBasicForLoopExpression__Group_3__0__Impl rule__XBasicForLoopExpression__Group_3__1
{
pushFollow(FollowSets002.FOLLOW_rule__XBasicForLoopExpression__Group_3__0__Impl_in_rule__XBasicForLoopExpression__Group_3__064178);
rule__XBasicForLoopExpression__Group_3__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XBasicForLoopExpression__Group_3__1_in_rule__XBasicForLoopExpression__Group_3__064181);
rule__XBasicForLoopExpression__Group_3__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XBasicForLoopExpression__Group_3__0"
// $ANTLR start "rule__XBasicForLoopExpression__Group_3__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31879:1: rule__XBasicForLoopExpression__Group_3__0__Impl : ( ( rule__XBasicForLoopExpression__InitExpressionsAssignment_3_0 ) ) ;
public final void rule__XBasicForLoopExpression__Group_3__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31883:1: ( ( ( rule__XBasicForLoopExpression__InitExpressionsAssignment_3_0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31884:1: ( ( rule__XBasicForLoopExpression__InitExpressionsAssignment_3_0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31884:1: ( ( rule__XBasicForLoopExpression__InitExpressionsAssignment_3_0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31885:1: ( rule__XBasicForLoopExpression__InitExpressionsAssignment_3_0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXBasicForLoopExpressionAccess().getInitExpressionsAssignment_3_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31886:1: ( rule__XBasicForLoopExpression__InitExpressionsAssignment_3_0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31886:2: rule__XBasicForLoopExpression__InitExpressionsAssignment_3_0
{
pushFollow(FollowSets002.FOLLOW_rule__XBasicForLoopExpression__InitExpressionsAssignment_3_0_in_rule__XBasicForLoopExpression__Group_3__0__Impl64208);
rule__XBasicForLoopExpression__InitExpressionsAssignment_3_0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXBasicForLoopExpressionAccess().getInitExpressionsAssignment_3_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XBasicForLoopExpression__Group_3__0__Impl"
// $ANTLR start "rule__XBasicForLoopExpression__Group_3__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31896:1: rule__XBasicForLoopExpression__Group_3__1 : rule__XBasicForLoopExpression__Group_3__1__Impl ;
public final void rule__XBasicForLoopExpression__Group_3__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31900:1: ( rule__XBasicForLoopExpression__Group_3__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31901:2: rule__XBasicForLoopExpression__Group_3__1__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__XBasicForLoopExpression__Group_3__1__Impl_in_rule__XBasicForLoopExpression__Group_3__164238);
rule__XBasicForLoopExpression__Group_3__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XBasicForLoopExpression__Group_3__1"
// $ANTLR start "rule__XBasicForLoopExpression__Group_3__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31907:1: rule__XBasicForLoopExpression__Group_3__1__Impl : ( ( rule__XBasicForLoopExpression__Group_3_1__0 )* ) ;
public final void rule__XBasicForLoopExpression__Group_3__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31911:1: ( ( ( rule__XBasicForLoopExpression__Group_3_1__0 )* ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31912:1: ( ( rule__XBasicForLoopExpression__Group_3_1__0 )* )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31912:1: ( ( rule__XBasicForLoopExpression__Group_3_1__0 )* )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31913:1: ( rule__XBasicForLoopExpression__Group_3_1__0 )*
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXBasicForLoopExpressionAccess().getGroup_3_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31914:1: ( rule__XBasicForLoopExpression__Group_3_1__0 )*
loop234:
do {
int alt234=2;
int LA234_0 = input.LA(1);
if ( (LA234_0==154) ) {
alt234=1;
}
switch (alt234) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31914:2: rule__XBasicForLoopExpression__Group_3_1__0
{
pushFollow(FollowSets002.FOLLOW_rule__XBasicForLoopExpression__Group_3_1__0_in_rule__XBasicForLoopExpression__Group_3__1__Impl64265);
rule__XBasicForLoopExpression__Group_3_1__0();
state._fsp--;
if (state.failed) return ;
}
break;
default :
break loop234;
}
} while (true);
if ( state.backtracking==0 ) {
after(grammarAccess.getXBasicForLoopExpressionAccess().getGroup_3_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XBasicForLoopExpression__Group_3__1__Impl"
// $ANTLR start "rule__XBasicForLoopExpression__Group_3_1__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31928:1: rule__XBasicForLoopExpression__Group_3_1__0 : rule__XBasicForLoopExpression__Group_3_1__0__Impl rule__XBasicForLoopExpression__Group_3_1__1 ;
public final void rule__XBasicForLoopExpression__Group_3_1__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31932:1: ( rule__XBasicForLoopExpression__Group_3_1__0__Impl rule__XBasicForLoopExpression__Group_3_1__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31933:2: rule__XBasicForLoopExpression__Group_3_1__0__Impl rule__XBasicForLoopExpression__Group_3_1__1
{
pushFollow(FollowSets002.FOLLOW_rule__XBasicForLoopExpression__Group_3_1__0__Impl_in_rule__XBasicForLoopExpression__Group_3_1__064300);
rule__XBasicForLoopExpression__Group_3_1__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XBasicForLoopExpression__Group_3_1__1_in_rule__XBasicForLoopExpression__Group_3_1__064303);
rule__XBasicForLoopExpression__Group_3_1__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XBasicForLoopExpression__Group_3_1__0"
// $ANTLR start "rule__XBasicForLoopExpression__Group_3_1__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31940:1: rule__XBasicForLoopExpression__Group_3_1__0__Impl : ( ',' ) ;
public final void rule__XBasicForLoopExpression__Group_3_1__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31944:1: ( ( ',' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31945:1: ( ',' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31945:1: ( ',' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31946:1: ','
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXBasicForLoopExpressionAccess().getCommaKeyword_3_1_0());
}
match(input,154,FollowSets002.FOLLOW_154_in_rule__XBasicForLoopExpression__Group_3_1__0__Impl64331); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXBasicForLoopExpressionAccess().getCommaKeyword_3_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XBasicForLoopExpression__Group_3_1__0__Impl"
// $ANTLR start "rule__XBasicForLoopExpression__Group_3_1__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31959:1: rule__XBasicForLoopExpression__Group_3_1__1 : rule__XBasicForLoopExpression__Group_3_1__1__Impl ;
public final void rule__XBasicForLoopExpression__Group_3_1__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31963:1: ( rule__XBasicForLoopExpression__Group_3_1__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31964:2: rule__XBasicForLoopExpression__Group_3_1__1__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__XBasicForLoopExpression__Group_3_1__1__Impl_in_rule__XBasicForLoopExpression__Group_3_1__164362);
rule__XBasicForLoopExpression__Group_3_1__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XBasicForLoopExpression__Group_3_1__1"
// $ANTLR start "rule__XBasicForLoopExpression__Group_3_1__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31970:1: rule__XBasicForLoopExpression__Group_3_1__1__Impl : ( ( rule__XBasicForLoopExpression__InitExpressionsAssignment_3_1_1 ) ) ;
public final void rule__XBasicForLoopExpression__Group_3_1__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31974:1: ( ( ( rule__XBasicForLoopExpression__InitExpressionsAssignment_3_1_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31975:1: ( ( rule__XBasicForLoopExpression__InitExpressionsAssignment_3_1_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31975:1: ( ( rule__XBasicForLoopExpression__InitExpressionsAssignment_3_1_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31976:1: ( rule__XBasicForLoopExpression__InitExpressionsAssignment_3_1_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXBasicForLoopExpressionAccess().getInitExpressionsAssignment_3_1_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31977:1: ( rule__XBasicForLoopExpression__InitExpressionsAssignment_3_1_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31977:2: rule__XBasicForLoopExpression__InitExpressionsAssignment_3_1_1
{
pushFollow(FollowSets002.FOLLOW_rule__XBasicForLoopExpression__InitExpressionsAssignment_3_1_1_in_rule__XBasicForLoopExpression__Group_3_1__1__Impl64389);
rule__XBasicForLoopExpression__InitExpressionsAssignment_3_1_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXBasicForLoopExpressionAccess().getInitExpressionsAssignment_3_1_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XBasicForLoopExpression__Group_3_1__1__Impl"
// $ANTLR start "rule__XBasicForLoopExpression__Group_7__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31991:1: rule__XBasicForLoopExpression__Group_7__0 : rule__XBasicForLoopExpression__Group_7__0__Impl rule__XBasicForLoopExpression__Group_7__1 ;
public final void rule__XBasicForLoopExpression__Group_7__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31995:1: ( rule__XBasicForLoopExpression__Group_7__0__Impl rule__XBasicForLoopExpression__Group_7__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:31996:2: rule__XBasicForLoopExpression__Group_7__0__Impl rule__XBasicForLoopExpression__Group_7__1
{
pushFollow(FollowSets002.FOLLOW_rule__XBasicForLoopExpression__Group_7__0__Impl_in_rule__XBasicForLoopExpression__Group_7__064423);
rule__XBasicForLoopExpression__Group_7__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XBasicForLoopExpression__Group_7__1_in_rule__XBasicForLoopExpression__Group_7__064426);
rule__XBasicForLoopExpression__Group_7__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XBasicForLoopExpression__Group_7__0"
// $ANTLR start "rule__XBasicForLoopExpression__Group_7__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32003:1: rule__XBasicForLoopExpression__Group_7__0__Impl : ( ( rule__XBasicForLoopExpression__UpdateExpressionsAssignment_7_0 ) ) ;
public final void rule__XBasicForLoopExpression__Group_7__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32007:1: ( ( ( rule__XBasicForLoopExpression__UpdateExpressionsAssignment_7_0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32008:1: ( ( rule__XBasicForLoopExpression__UpdateExpressionsAssignment_7_0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32008:1: ( ( rule__XBasicForLoopExpression__UpdateExpressionsAssignment_7_0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32009:1: ( rule__XBasicForLoopExpression__UpdateExpressionsAssignment_7_0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXBasicForLoopExpressionAccess().getUpdateExpressionsAssignment_7_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32010:1: ( rule__XBasicForLoopExpression__UpdateExpressionsAssignment_7_0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32010:2: rule__XBasicForLoopExpression__UpdateExpressionsAssignment_7_0
{
pushFollow(FollowSets002.FOLLOW_rule__XBasicForLoopExpression__UpdateExpressionsAssignment_7_0_in_rule__XBasicForLoopExpression__Group_7__0__Impl64453);
rule__XBasicForLoopExpression__UpdateExpressionsAssignment_7_0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXBasicForLoopExpressionAccess().getUpdateExpressionsAssignment_7_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XBasicForLoopExpression__Group_7__0__Impl"
// $ANTLR start "rule__XBasicForLoopExpression__Group_7__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32020:1: rule__XBasicForLoopExpression__Group_7__1 : rule__XBasicForLoopExpression__Group_7__1__Impl ;
public final void rule__XBasicForLoopExpression__Group_7__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32024:1: ( rule__XBasicForLoopExpression__Group_7__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32025:2: rule__XBasicForLoopExpression__Group_7__1__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__XBasicForLoopExpression__Group_7__1__Impl_in_rule__XBasicForLoopExpression__Group_7__164483);
rule__XBasicForLoopExpression__Group_7__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XBasicForLoopExpression__Group_7__1"
// $ANTLR start "rule__XBasicForLoopExpression__Group_7__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32031:1: rule__XBasicForLoopExpression__Group_7__1__Impl : ( ( rule__XBasicForLoopExpression__Group_7_1__0 )* ) ;
public final void rule__XBasicForLoopExpression__Group_7__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32035:1: ( ( ( rule__XBasicForLoopExpression__Group_7_1__0 )* ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32036:1: ( ( rule__XBasicForLoopExpression__Group_7_1__0 )* )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32036:1: ( ( rule__XBasicForLoopExpression__Group_7_1__0 )* )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32037:1: ( rule__XBasicForLoopExpression__Group_7_1__0 )*
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXBasicForLoopExpressionAccess().getGroup_7_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32038:1: ( rule__XBasicForLoopExpression__Group_7_1__0 )*
loop235:
do {
int alt235=2;
int LA235_0 = input.LA(1);
if ( (LA235_0==154) ) {
alt235=1;
}
switch (alt235) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32038:2: rule__XBasicForLoopExpression__Group_7_1__0
{
pushFollow(FollowSets002.FOLLOW_rule__XBasicForLoopExpression__Group_7_1__0_in_rule__XBasicForLoopExpression__Group_7__1__Impl64510);
rule__XBasicForLoopExpression__Group_7_1__0();
state._fsp--;
if (state.failed) return ;
}
break;
default :
break loop235;
}
} while (true);
if ( state.backtracking==0 ) {
after(grammarAccess.getXBasicForLoopExpressionAccess().getGroup_7_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XBasicForLoopExpression__Group_7__1__Impl"
// $ANTLR start "rule__XBasicForLoopExpression__Group_7_1__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32052:1: rule__XBasicForLoopExpression__Group_7_1__0 : rule__XBasicForLoopExpression__Group_7_1__0__Impl rule__XBasicForLoopExpression__Group_7_1__1 ;
public final void rule__XBasicForLoopExpression__Group_7_1__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32056:1: ( rule__XBasicForLoopExpression__Group_7_1__0__Impl rule__XBasicForLoopExpression__Group_7_1__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32057:2: rule__XBasicForLoopExpression__Group_7_1__0__Impl rule__XBasicForLoopExpression__Group_7_1__1
{
pushFollow(FollowSets002.FOLLOW_rule__XBasicForLoopExpression__Group_7_1__0__Impl_in_rule__XBasicForLoopExpression__Group_7_1__064545);
rule__XBasicForLoopExpression__Group_7_1__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XBasicForLoopExpression__Group_7_1__1_in_rule__XBasicForLoopExpression__Group_7_1__064548);
rule__XBasicForLoopExpression__Group_7_1__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XBasicForLoopExpression__Group_7_1__0"
// $ANTLR start "rule__XBasicForLoopExpression__Group_7_1__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32064:1: rule__XBasicForLoopExpression__Group_7_1__0__Impl : ( ',' ) ;
public final void rule__XBasicForLoopExpression__Group_7_1__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32068:1: ( ( ',' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32069:1: ( ',' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32069:1: ( ',' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32070:1: ','
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXBasicForLoopExpressionAccess().getCommaKeyword_7_1_0());
}
match(input,154,FollowSets002.FOLLOW_154_in_rule__XBasicForLoopExpression__Group_7_1__0__Impl64576); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXBasicForLoopExpressionAccess().getCommaKeyword_7_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XBasicForLoopExpression__Group_7_1__0__Impl"
}
@SuppressWarnings("all")
abstract class InternalMangoParser4 extends InternalMangoParser3 {
InternalMangoParser4(TokenStream input) {
this(input, new RecognizerSharedState());
}
InternalMangoParser4(TokenStream input, RecognizerSharedState state) {
super(input, state);
}
// $ANTLR start "rule__XBasicForLoopExpression__Group_7_1__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32083:1: rule__XBasicForLoopExpression__Group_7_1__1 : rule__XBasicForLoopExpression__Group_7_1__1__Impl ;
public final void rule__XBasicForLoopExpression__Group_7_1__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32087:1: ( rule__XBasicForLoopExpression__Group_7_1__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32088:2: rule__XBasicForLoopExpression__Group_7_1__1__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__XBasicForLoopExpression__Group_7_1__1__Impl_in_rule__XBasicForLoopExpression__Group_7_1__164607);
rule__XBasicForLoopExpression__Group_7_1__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XBasicForLoopExpression__Group_7_1__1"
// $ANTLR start "rule__XBasicForLoopExpression__Group_7_1__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32094:1: rule__XBasicForLoopExpression__Group_7_1__1__Impl : ( ( rule__XBasicForLoopExpression__UpdateExpressionsAssignment_7_1_1 ) ) ;
public final void rule__XBasicForLoopExpression__Group_7_1__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32098:1: ( ( ( rule__XBasicForLoopExpression__UpdateExpressionsAssignment_7_1_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32099:1: ( ( rule__XBasicForLoopExpression__UpdateExpressionsAssignment_7_1_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32099:1: ( ( rule__XBasicForLoopExpression__UpdateExpressionsAssignment_7_1_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32100:1: ( rule__XBasicForLoopExpression__UpdateExpressionsAssignment_7_1_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXBasicForLoopExpressionAccess().getUpdateExpressionsAssignment_7_1_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32101:1: ( rule__XBasicForLoopExpression__UpdateExpressionsAssignment_7_1_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32101:2: rule__XBasicForLoopExpression__UpdateExpressionsAssignment_7_1_1
{
pushFollow(FollowSets002.FOLLOW_rule__XBasicForLoopExpression__UpdateExpressionsAssignment_7_1_1_in_rule__XBasicForLoopExpression__Group_7_1__1__Impl64634);
rule__XBasicForLoopExpression__UpdateExpressionsAssignment_7_1_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXBasicForLoopExpressionAccess().getUpdateExpressionsAssignment_7_1_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XBasicForLoopExpression__Group_7_1__1__Impl"
// $ANTLR start "rule__XWhileExpression__Group__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32115:1: rule__XWhileExpression__Group__0 : rule__XWhileExpression__Group__0__Impl rule__XWhileExpression__Group__1 ;
public final void rule__XWhileExpression__Group__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32119:1: ( rule__XWhileExpression__Group__0__Impl rule__XWhileExpression__Group__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32120:2: rule__XWhileExpression__Group__0__Impl rule__XWhileExpression__Group__1
{
pushFollow(FollowSets002.FOLLOW_rule__XWhileExpression__Group__0__Impl_in_rule__XWhileExpression__Group__064668);
rule__XWhileExpression__Group__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XWhileExpression__Group__1_in_rule__XWhileExpression__Group__064671);
rule__XWhileExpression__Group__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XWhileExpression__Group__0"
// $ANTLR start "rule__XWhileExpression__Group__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32127:1: rule__XWhileExpression__Group__0__Impl : ( () ) ;
public final void rule__XWhileExpression__Group__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32131:1: ( ( () ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32132:1: ( () )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32132:1: ( () )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32133:1: ()
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXWhileExpressionAccess().getXWhileExpressionAction_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32134:1: ()
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32136:1:
{
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXWhileExpressionAccess().getXWhileExpressionAction_0());
}
}
}
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XWhileExpression__Group__0__Impl"
// $ANTLR start "rule__XWhileExpression__Group__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32146:1: rule__XWhileExpression__Group__1 : rule__XWhileExpression__Group__1__Impl rule__XWhileExpression__Group__2 ;
public final void rule__XWhileExpression__Group__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32150:1: ( rule__XWhileExpression__Group__1__Impl rule__XWhileExpression__Group__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32151:2: rule__XWhileExpression__Group__1__Impl rule__XWhileExpression__Group__2
{
pushFollow(FollowSets002.FOLLOW_rule__XWhileExpression__Group__1__Impl_in_rule__XWhileExpression__Group__164729);
rule__XWhileExpression__Group__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XWhileExpression__Group__2_in_rule__XWhileExpression__Group__164732);
rule__XWhileExpression__Group__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XWhileExpression__Group__1"
// $ANTLR start "rule__XWhileExpression__Group__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32158:1: rule__XWhileExpression__Group__1__Impl : ( 'while' ) ;
public final void rule__XWhileExpression__Group__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32162:1: ( ( 'while' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32163:1: ( 'while' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32163:1: ( 'while' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32164:1: 'while'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXWhileExpressionAccess().getWhileKeyword_1());
}
match(input,166,FollowSets002.FOLLOW_166_in_rule__XWhileExpression__Group__1__Impl64760); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXWhileExpressionAccess().getWhileKeyword_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XWhileExpression__Group__1__Impl"
// $ANTLR start "rule__XWhileExpression__Group__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32177:1: rule__XWhileExpression__Group__2 : rule__XWhileExpression__Group__2__Impl rule__XWhileExpression__Group__3 ;
public final void rule__XWhileExpression__Group__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32181:1: ( rule__XWhileExpression__Group__2__Impl rule__XWhileExpression__Group__3 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32182:2: rule__XWhileExpression__Group__2__Impl rule__XWhileExpression__Group__3
{
pushFollow(FollowSets002.FOLLOW_rule__XWhileExpression__Group__2__Impl_in_rule__XWhileExpression__Group__264791);
rule__XWhileExpression__Group__2__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XWhileExpression__Group__3_in_rule__XWhileExpression__Group__264794);
rule__XWhileExpression__Group__3();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XWhileExpression__Group__2"
// $ANTLR start "rule__XWhileExpression__Group__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32189:1: rule__XWhileExpression__Group__2__Impl : ( '(' ) ;
public final void rule__XWhileExpression__Group__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32193:1: ( ( '(' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32194:1: ( '(' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32194:1: ( '(' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32195:1: '('
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXWhileExpressionAccess().getLeftParenthesisKeyword_2());
}
match(input,144,FollowSets002.FOLLOW_144_in_rule__XWhileExpression__Group__2__Impl64822); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXWhileExpressionAccess().getLeftParenthesisKeyword_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XWhileExpression__Group__2__Impl"
// $ANTLR start "rule__XWhileExpression__Group__3"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32208:1: rule__XWhileExpression__Group__3 : rule__XWhileExpression__Group__3__Impl rule__XWhileExpression__Group__4 ;
public final void rule__XWhileExpression__Group__3() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32212:1: ( rule__XWhileExpression__Group__3__Impl rule__XWhileExpression__Group__4 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32213:2: rule__XWhileExpression__Group__3__Impl rule__XWhileExpression__Group__4
{
pushFollow(FollowSets002.FOLLOW_rule__XWhileExpression__Group__3__Impl_in_rule__XWhileExpression__Group__364853);
rule__XWhileExpression__Group__3__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XWhileExpression__Group__4_in_rule__XWhileExpression__Group__364856);
rule__XWhileExpression__Group__4();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XWhileExpression__Group__3"
// $ANTLR start "rule__XWhileExpression__Group__3__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32220:1: rule__XWhileExpression__Group__3__Impl : ( ( rule__XWhileExpression__PredicateAssignment_3 ) ) ;
public final void rule__XWhileExpression__Group__3__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32224:1: ( ( ( rule__XWhileExpression__PredicateAssignment_3 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32225:1: ( ( rule__XWhileExpression__PredicateAssignment_3 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32225:1: ( ( rule__XWhileExpression__PredicateAssignment_3 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32226:1: ( rule__XWhileExpression__PredicateAssignment_3 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXWhileExpressionAccess().getPredicateAssignment_3());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32227:1: ( rule__XWhileExpression__PredicateAssignment_3 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32227:2: rule__XWhileExpression__PredicateAssignment_3
{
pushFollow(FollowSets002.FOLLOW_rule__XWhileExpression__PredicateAssignment_3_in_rule__XWhileExpression__Group__3__Impl64883);
rule__XWhileExpression__PredicateAssignment_3();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXWhileExpressionAccess().getPredicateAssignment_3());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XWhileExpression__Group__3__Impl"
// $ANTLR start "rule__XWhileExpression__Group__4"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32237:1: rule__XWhileExpression__Group__4 : rule__XWhileExpression__Group__4__Impl rule__XWhileExpression__Group__5 ;
public final void rule__XWhileExpression__Group__4() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32241:1: ( rule__XWhileExpression__Group__4__Impl rule__XWhileExpression__Group__5 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32242:2: rule__XWhileExpression__Group__4__Impl rule__XWhileExpression__Group__5
{
pushFollow(FollowSets002.FOLLOW_rule__XWhileExpression__Group__4__Impl_in_rule__XWhileExpression__Group__464913);
rule__XWhileExpression__Group__4__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XWhileExpression__Group__5_in_rule__XWhileExpression__Group__464916);
rule__XWhileExpression__Group__5();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XWhileExpression__Group__4"
// $ANTLR start "rule__XWhileExpression__Group__4__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32249:1: rule__XWhileExpression__Group__4__Impl : ( ')' ) ;
public final void rule__XWhileExpression__Group__4__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32253:1: ( ( ')' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32254:1: ( ')' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32254:1: ( ')' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32255:1: ')'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXWhileExpressionAccess().getRightParenthesisKeyword_4());
}
match(input,145,FollowSets002.FOLLOW_145_in_rule__XWhileExpression__Group__4__Impl64944); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXWhileExpressionAccess().getRightParenthesisKeyword_4());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XWhileExpression__Group__4__Impl"
// $ANTLR start "rule__XWhileExpression__Group__5"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32268:1: rule__XWhileExpression__Group__5 : rule__XWhileExpression__Group__5__Impl ;
public final void rule__XWhileExpression__Group__5() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32272:1: ( rule__XWhileExpression__Group__5__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32273:2: rule__XWhileExpression__Group__5__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__XWhileExpression__Group__5__Impl_in_rule__XWhileExpression__Group__564975);
rule__XWhileExpression__Group__5__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XWhileExpression__Group__5"
// $ANTLR start "rule__XWhileExpression__Group__5__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32279:1: rule__XWhileExpression__Group__5__Impl : ( ( rule__XWhileExpression__BodyAssignment_5 ) ) ;
public final void rule__XWhileExpression__Group__5__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32283:1: ( ( ( rule__XWhileExpression__BodyAssignment_5 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32284:1: ( ( rule__XWhileExpression__BodyAssignment_5 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32284:1: ( ( rule__XWhileExpression__BodyAssignment_5 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32285:1: ( rule__XWhileExpression__BodyAssignment_5 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXWhileExpressionAccess().getBodyAssignment_5());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32286:1: ( rule__XWhileExpression__BodyAssignment_5 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32286:2: rule__XWhileExpression__BodyAssignment_5
{
pushFollow(FollowSets002.FOLLOW_rule__XWhileExpression__BodyAssignment_5_in_rule__XWhileExpression__Group__5__Impl65002);
rule__XWhileExpression__BodyAssignment_5();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXWhileExpressionAccess().getBodyAssignment_5());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XWhileExpression__Group__5__Impl"
// $ANTLR start "rule__XDoWhileExpression__Group__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32308:1: rule__XDoWhileExpression__Group__0 : rule__XDoWhileExpression__Group__0__Impl rule__XDoWhileExpression__Group__1 ;
public final void rule__XDoWhileExpression__Group__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32312:1: ( rule__XDoWhileExpression__Group__0__Impl rule__XDoWhileExpression__Group__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32313:2: rule__XDoWhileExpression__Group__0__Impl rule__XDoWhileExpression__Group__1
{
pushFollow(FollowSets002.FOLLOW_rule__XDoWhileExpression__Group__0__Impl_in_rule__XDoWhileExpression__Group__065044);
rule__XDoWhileExpression__Group__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XDoWhileExpression__Group__1_in_rule__XDoWhileExpression__Group__065047);
rule__XDoWhileExpression__Group__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XDoWhileExpression__Group__0"
// $ANTLR start "rule__XDoWhileExpression__Group__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32320:1: rule__XDoWhileExpression__Group__0__Impl : ( () ) ;
public final void rule__XDoWhileExpression__Group__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32324:1: ( ( () ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32325:1: ( () )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32325:1: ( () )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32326:1: ()
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXDoWhileExpressionAccess().getXDoWhileExpressionAction_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32327:1: ()
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32329:1:
{
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXDoWhileExpressionAccess().getXDoWhileExpressionAction_0());
}
}
}
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XDoWhileExpression__Group__0__Impl"
// $ANTLR start "rule__XDoWhileExpression__Group__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32339:1: rule__XDoWhileExpression__Group__1 : rule__XDoWhileExpression__Group__1__Impl rule__XDoWhileExpression__Group__2 ;
public final void rule__XDoWhileExpression__Group__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32343:1: ( rule__XDoWhileExpression__Group__1__Impl rule__XDoWhileExpression__Group__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32344:2: rule__XDoWhileExpression__Group__1__Impl rule__XDoWhileExpression__Group__2
{
pushFollow(FollowSets002.FOLLOW_rule__XDoWhileExpression__Group__1__Impl_in_rule__XDoWhileExpression__Group__165105);
rule__XDoWhileExpression__Group__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XDoWhileExpression__Group__2_in_rule__XDoWhileExpression__Group__165108);
rule__XDoWhileExpression__Group__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XDoWhileExpression__Group__1"
// $ANTLR start "rule__XDoWhileExpression__Group__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32351:1: rule__XDoWhileExpression__Group__1__Impl : ( 'do' ) ;
public final void rule__XDoWhileExpression__Group__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32355:1: ( ( 'do' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32356:1: ( 'do' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32356:1: ( 'do' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32357:1: 'do'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXDoWhileExpressionAccess().getDoKeyword_1());
}
match(input,167,FollowSets002.FOLLOW_167_in_rule__XDoWhileExpression__Group__1__Impl65136); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXDoWhileExpressionAccess().getDoKeyword_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XDoWhileExpression__Group__1__Impl"
// $ANTLR start "rule__XDoWhileExpression__Group__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32370:1: rule__XDoWhileExpression__Group__2 : rule__XDoWhileExpression__Group__2__Impl rule__XDoWhileExpression__Group__3 ;
public final void rule__XDoWhileExpression__Group__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32374:1: ( rule__XDoWhileExpression__Group__2__Impl rule__XDoWhileExpression__Group__3 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32375:2: rule__XDoWhileExpression__Group__2__Impl rule__XDoWhileExpression__Group__3
{
pushFollow(FollowSets002.FOLLOW_rule__XDoWhileExpression__Group__2__Impl_in_rule__XDoWhileExpression__Group__265167);
rule__XDoWhileExpression__Group__2__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XDoWhileExpression__Group__3_in_rule__XDoWhileExpression__Group__265170);
rule__XDoWhileExpression__Group__3();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XDoWhileExpression__Group__2"
// $ANTLR start "rule__XDoWhileExpression__Group__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32382:1: rule__XDoWhileExpression__Group__2__Impl : ( ( rule__XDoWhileExpression__BodyAssignment_2 ) ) ;
public final void rule__XDoWhileExpression__Group__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32386:1: ( ( ( rule__XDoWhileExpression__BodyAssignment_2 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32387:1: ( ( rule__XDoWhileExpression__BodyAssignment_2 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32387:1: ( ( rule__XDoWhileExpression__BodyAssignment_2 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32388:1: ( rule__XDoWhileExpression__BodyAssignment_2 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXDoWhileExpressionAccess().getBodyAssignment_2());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32389:1: ( rule__XDoWhileExpression__BodyAssignment_2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32389:2: rule__XDoWhileExpression__BodyAssignment_2
{
pushFollow(FollowSets002.FOLLOW_rule__XDoWhileExpression__BodyAssignment_2_in_rule__XDoWhileExpression__Group__2__Impl65197);
rule__XDoWhileExpression__BodyAssignment_2();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXDoWhileExpressionAccess().getBodyAssignment_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XDoWhileExpression__Group__2__Impl"
// $ANTLR start "rule__XDoWhileExpression__Group__3"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32399:1: rule__XDoWhileExpression__Group__3 : rule__XDoWhileExpression__Group__3__Impl rule__XDoWhileExpression__Group__4 ;
public final void rule__XDoWhileExpression__Group__3() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32403:1: ( rule__XDoWhileExpression__Group__3__Impl rule__XDoWhileExpression__Group__4 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32404:2: rule__XDoWhileExpression__Group__3__Impl rule__XDoWhileExpression__Group__4
{
pushFollow(FollowSets002.FOLLOW_rule__XDoWhileExpression__Group__3__Impl_in_rule__XDoWhileExpression__Group__365227);
rule__XDoWhileExpression__Group__3__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XDoWhileExpression__Group__4_in_rule__XDoWhileExpression__Group__365230);
rule__XDoWhileExpression__Group__4();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XDoWhileExpression__Group__3"
// $ANTLR start "rule__XDoWhileExpression__Group__3__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32411:1: rule__XDoWhileExpression__Group__3__Impl : ( 'while' ) ;
public final void rule__XDoWhileExpression__Group__3__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32415:1: ( ( 'while' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32416:1: ( 'while' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32416:1: ( 'while' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32417:1: 'while'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXDoWhileExpressionAccess().getWhileKeyword_3());
}
match(input,166,FollowSets002.FOLLOW_166_in_rule__XDoWhileExpression__Group__3__Impl65258); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXDoWhileExpressionAccess().getWhileKeyword_3());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XDoWhileExpression__Group__3__Impl"
// $ANTLR start "rule__XDoWhileExpression__Group__4"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32430:1: rule__XDoWhileExpression__Group__4 : rule__XDoWhileExpression__Group__4__Impl rule__XDoWhileExpression__Group__5 ;
public final void rule__XDoWhileExpression__Group__4() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32434:1: ( rule__XDoWhileExpression__Group__4__Impl rule__XDoWhileExpression__Group__5 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32435:2: rule__XDoWhileExpression__Group__4__Impl rule__XDoWhileExpression__Group__5
{
pushFollow(FollowSets002.FOLLOW_rule__XDoWhileExpression__Group__4__Impl_in_rule__XDoWhileExpression__Group__465289);
rule__XDoWhileExpression__Group__4__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XDoWhileExpression__Group__5_in_rule__XDoWhileExpression__Group__465292);
rule__XDoWhileExpression__Group__5();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XDoWhileExpression__Group__4"
// $ANTLR start "rule__XDoWhileExpression__Group__4__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32442:1: rule__XDoWhileExpression__Group__4__Impl : ( '(' ) ;
public final void rule__XDoWhileExpression__Group__4__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32446:1: ( ( '(' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32447:1: ( '(' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32447:1: ( '(' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32448:1: '('
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXDoWhileExpressionAccess().getLeftParenthesisKeyword_4());
}
match(input,144,FollowSets002.FOLLOW_144_in_rule__XDoWhileExpression__Group__4__Impl65320); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXDoWhileExpressionAccess().getLeftParenthesisKeyword_4());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XDoWhileExpression__Group__4__Impl"
// $ANTLR start "rule__XDoWhileExpression__Group__5"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32461:1: rule__XDoWhileExpression__Group__5 : rule__XDoWhileExpression__Group__5__Impl rule__XDoWhileExpression__Group__6 ;
public final void rule__XDoWhileExpression__Group__5() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32465:1: ( rule__XDoWhileExpression__Group__5__Impl rule__XDoWhileExpression__Group__6 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32466:2: rule__XDoWhileExpression__Group__5__Impl rule__XDoWhileExpression__Group__6
{
pushFollow(FollowSets002.FOLLOW_rule__XDoWhileExpression__Group__5__Impl_in_rule__XDoWhileExpression__Group__565351);
rule__XDoWhileExpression__Group__5__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XDoWhileExpression__Group__6_in_rule__XDoWhileExpression__Group__565354);
rule__XDoWhileExpression__Group__6();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XDoWhileExpression__Group__5"
// $ANTLR start "rule__XDoWhileExpression__Group__5__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32473:1: rule__XDoWhileExpression__Group__5__Impl : ( ( rule__XDoWhileExpression__PredicateAssignment_5 ) ) ;
public final void rule__XDoWhileExpression__Group__5__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32477:1: ( ( ( rule__XDoWhileExpression__PredicateAssignment_5 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32478:1: ( ( rule__XDoWhileExpression__PredicateAssignment_5 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32478:1: ( ( rule__XDoWhileExpression__PredicateAssignment_5 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32479:1: ( rule__XDoWhileExpression__PredicateAssignment_5 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXDoWhileExpressionAccess().getPredicateAssignment_5());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32480:1: ( rule__XDoWhileExpression__PredicateAssignment_5 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32480:2: rule__XDoWhileExpression__PredicateAssignment_5
{
pushFollow(FollowSets002.FOLLOW_rule__XDoWhileExpression__PredicateAssignment_5_in_rule__XDoWhileExpression__Group__5__Impl65381);
rule__XDoWhileExpression__PredicateAssignment_5();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXDoWhileExpressionAccess().getPredicateAssignment_5());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XDoWhileExpression__Group__5__Impl"
// $ANTLR start "rule__XDoWhileExpression__Group__6"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32490:1: rule__XDoWhileExpression__Group__6 : rule__XDoWhileExpression__Group__6__Impl ;
public final void rule__XDoWhileExpression__Group__6() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32494:1: ( rule__XDoWhileExpression__Group__6__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32495:2: rule__XDoWhileExpression__Group__6__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__XDoWhileExpression__Group__6__Impl_in_rule__XDoWhileExpression__Group__665411);
rule__XDoWhileExpression__Group__6__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XDoWhileExpression__Group__6"
// $ANTLR start "rule__XDoWhileExpression__Group__6__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32501:1: rule__XDoWhileExpression__Group__6__Impl : ( ')' ) ;
public final void rule__XDoWhileExpression__Group__6__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32505:1: ( ( ')' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32506:1: ( ')' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32506:1: ( ')' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32507:1: ')'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXDoWhileExpressionAccess().getRightParenthesisKeyword_6());
}
match(input,145,FollowSets002.FOLLOW_145_in_rule__XDoWhileExpression__Group__6__Impl65439); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXDoWhileExpressionAccess().getRightParenthesisKeyword_6());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XDoWhileExpression__Group__6__Impl"
// $ANTLR start "rule__XBlockExpression__Group__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32534:1: rule__XBlockExpression__Group__0 : rule__XBlockExpression__Group__0__Impl rule__XBlockExpression__Group__1 ;
public final void rule__XBlockExpression__Group__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32538:1: ( rule__XBlockExpression__Group__0__Impl rule__XBlockExpression__Group__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32539:2: rule__XBlockExpression__Group__0__Impl rule__XBlockExpression__Group__1
{
pushFollow(FollowSets002.FOLLOW_rule__XBlockExpression__Group__0__Impl_in_rule__XBlockExpression__Group__065484);
rule__XBlockExpression__Group__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XBlockExpression__Group__1_in_rule__XBlockExpression__Group__065487);
rule__XBlockExpression__Group__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XBlockExpression__Group__0"
// $ANTLR start "rule__XBlockExpression__Group__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32546:1: rule__XBlockExpression__Group__0__Impl : ( () ) ;
public final void rule__XBlockExpression__Group__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32550:1: ( ( () ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32551:1: ( () )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32551:1: ( () )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32552:1: ()
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXBlockExpressionAccess().getXBlockExpressionAction_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32553:1: ()
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32555:1:
{
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXBlockExpressionAccess().getXBlockExpressionAction_0());
}
}
}
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XBlockExpression__Group__0__Impl"
// $ANTLR start "rule__XBlockExpression__Group__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32565:1: rule__XBlockExpression__Group__1 : rule__XBlockExpression__Group__1__Impl rule__XBlockExpression__Group__2 ;
public final void rule__XBlockExpression__Group__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32569:1: ( rule__XBlockExpression__Group__1__Impl rule__XBlockExpression__Group__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32570:2: rule__XBlockExpression__Group__1__Impl rule__XBlockExpression__Group__2
{
pushFollow(FollowSets002.FOLLOW_rule__XBlockExpression__Group__1__Impl_in_rule__XBlockExpression__Group__165545);
rule__XBlockExpression__Group__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XBlockExpression__Group__2_in_rule__XBlockExpression__Group__165548);
rule__XBlockExpression__Group__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XBlockExpression__Group__1"
// $ANTLR start "rule__XBlockExpression__Group__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32577:1: rule__XBlockExpression__Group__1__Impl : ( '{' ) ;
public final void rule__XBlockExpression__Group__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32581:1: ( ( '{' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32582:1: ( '{' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32582:1: ( '{' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32583:1: '{'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXBlockExpressionAccess().getLeftCurlyBracketKeyword_1());
}
match(input,67,FollowSets002.FOLLOW_67_in_rule__XBlockExpression__Group__1__Impl65576); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXBlockExpressionAccess().getLeftCurlyBracketKeyword_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XBlockExpression__Group__1__Impl"
// $ANTLR start "rule__XBlockExpression__Group__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32596:1: rule__XBlockExpression__Group__2 : rule__XBlockExpression__Group__2__Impl rule__XBlockExpression__Group__3 ;
public final void rule__XBlockExpression__Group__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32600:1: ( rule__XBlockExpression__Group__2__Impl rule__XBlockExpression__Group__3 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32601:2: rule__XBlockExpression__Group__2__Impl rule__XBlockExpression__Group__3
{
pushFollow(FollowSets002.FOLLOW_rule__XBlockExpression__Group__2__Impl_in_rule__XBlockExpression__Group__265607);
rule__XBlockExpression__Group__2__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XBlockExpression__Group__3_in_rule__XBlockExpression__Group__265610);
rule__XBlockExpression__Group__3();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XBlockExpression__Group__2"
// $ANTLR start "rule__XBlockExpression__Group__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32608:1: rule__XBlockExpression__Group__2__Impl : ( ( rule__XBlockExpression__Group_2__0 )* ) ;
public final void rule__XBlockExpression__Group__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32612:1: ( ( ( rule__XBlockExpression__Group_2__0 )* ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32613:1: ( ( rule__XBlockExpression__Group_2__0 )* )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32613:1: ( ( rule__XBlockExpression__Group_2__0 )* )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32614:1: ( rule__XBlockExpression__Group_2__0 )*
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXBlockExpressionAccess().getGroup_2());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32615:1: ( rule__XBlockExpression__Group_2__0 )*
loop236:
do {
int alt236=2;
int LA236_0 = input.LA(1);
if ( ((LA236_0>=RULE_ID && LA236_0<=RULE_STRING)||LA236_0==27||(LA236_0>=34 && LA236_0<=35)||LA236_0==40||(LA236_0>=44 && LA236_0<=50)||LA236_0==67||LA236_0==144||(LA236_0>=155 && LA236_0<=156)||LA236_0==159||LA236_0==161||(LA236_0>=165 && LA236_0<=173)||LA236_0==175||(LA236_0>=184 && LA236_0<=185)) ) {
alt236=1;
}
switch (alt236) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32615:2: rule__XBlockExpression__Group_2__0
{
pushFollow(FollowSets002.FOLLOW_rule__XBlockExpression__Group_2__0_in_rule__XBlockExpression__Group__2__Impl65637);
rule__XBlockExpression__Group_2__0();
state._fsp--;
if (state.failed) return ;
}
break;
default :
break loop236;
}
} while (true);
if ( state.backtracking==0 ) {
after(grammarAccess.getXBlockExpressionAccess().getGroup_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XBlockExpression__Group__2__Impl"
// $ANTLR start "rule__XBlockExpression__Group__3"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32625:1: rule__XBlockExpression__Group__3 : rule__XBlockExpression__Group__3__Impl ;
public final void rule__XBlockExpression__Group__3() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32629:1: ( rule__XBlockExpression__Group__3__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32630:2: rule__XBlockExpression__Group__3__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__XBlockExpression__Group__3__Impl_in_rule__XBlockExpression__Group__365668);
rule__XBlockExpression__Group__3__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XBlockExpression__Group__3"
// $ANTLR start "rule__XBlockExpression__Group__3__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32636:1: rule__XBlockExpression__Group__3__Impl : ( '}' ) ;
public final void rule__XBlockExpression__Group__3__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32640:1: ( ( '}' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32641:1: ( '}' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32641:1: ( '}' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32642:1: '}'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXBlockExpressionAccess().getRightCurlyBracketKeyword_3());
}
match(input,68,FollowSets002.FOLLOW_68_in_rule__XBlockExpression__Group__3__Impl65696); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXBlockExpressionAccess().getRightCurlyBracketKeyword_3());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XBlockExpression__Group__3__Impl"
// $ANTLR start "rule__XBlockExpression__Group_2__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32663:1: rule__XBlockExpression__Group_2__0 : rule__XBlockExpression__Group_2__0__Impl rule__XBlockExpression__Group_2__1 ;
public final void rule__XBlockExpression__Group_2__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32667:1: ( rule__XBlockExpression__Group_2__0__Impl rule__XBlockExpression__Group_2__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32668:2: rule__XBlockExpression__Group_2__0__Impl rule__XBlockExpression__Group_2__1
{
pushFollow(FollowSets002.FOLLOW_rule__XBlockExpression__Group_2__0__Impl_in_rule__XBlockExpression__Group_2__065735);
rule__XBlockExpression__Group_2__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XBlockExpression__Group_2__1_in_rule__XBlockExpression__Group_2__065738);
rule__XBlockExpression__Group_2__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XBlockExpression__Group_2__0"
// $ANTLR start "rule__XBlockExpression__Group_2__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32675:1: rule__XBlockExpression__Group_2__0__Impl : ( ( rule__XBlockExpression__ExpressionsAssignment_2_0 ) ) ;
public final void rule__XBlockExpression__Group_2__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32679:1: ( ( ( rule__XBlockExpression__ExpressionsAssignment_2_0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32680:1: ( ( rule__XBlockExpression__ExpressionsAssignment_2_0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32680:1: ( ( rule__XBlockExpression__ExpressionsAssignment_2_0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32681:1: ( rule__XBlockExpression__ExpressionsAssignment_2_0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXBlockExpressionAccess().getExpressionsAssignment_2_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32682:1: ( rule__XBlockExpression__ExpressionsAssignment_2_0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32682:2: rule__XBlockExpression__ExpressionsAssignment_2_0
{
pushFollow(FollowSets002.FOLLOW_rule__XBlockExpression__ExpressionsAssignment_2_0_in_rule__XBlockExpression__Group_2__0__Impl65765);
rule__XBlockExpression__ExpressionsAssignment_2_0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXBlockExpressionAccess().getExpressionsAssignment_2_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XBlockExpression__Group_2__0__Impl"
// $ANTLR start "rule__XBlockExpression__Group_2__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32692:1: rule__XBlockExpression__Group_2__1 : rule__XBlockExpression__Group_2__1__Impl ;
public final void rule__XBlockExpression__Group_2__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32696:1: ( rule__XBlockExpression__Group_2__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32697:2: rule__XBlockExpression__Group_2__1__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__XBlockExpression__Group_2__1__Impl_in_rule__XBlockExpression__Group_2__165795);
rule__XBlockExpression__Group_2__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XBlockExpression__Group_2__1"
// $ANTLR start "rule__XBlockExpression__Group_2__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32703:1: rule__XBlockExpression__Group_2__1__Impl : ( ( ';' )? ) ;
public final void rule__XBlockExpression__Group_2__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32707:1: ( ( ( ';' )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32708:1: ( ( ';' )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32708:1: ( ( ';' )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32709:1: ( ';' )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXBlockExpressionAccess().getSemicolonKeyword_2_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32710:1: ( ';' )?
int alt237=2;
int LA237_0 = input.LA(1);
if ( (LA237_0==158) ) {
alt237=1;
}
switch (alt237) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32711:2: ';'
{
match(input,158,FollowSets002.FOLLOW_158_in_rule__XBlockExpression__Group_2__1__Impl65824); if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXBlockExpressionAccess().getSemicolonKeyword_2_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XBlockExpression__Group_2__1__Impl"
// $ANTLR start "rule__XVariableDeclaration__Group__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32726:1: rule__XVariableDeclaration__Group__0 : rule__XVariableDeclaration__Group__0__Impl rule__XVariableDeclaration__Group__1 ;
public final void rule__XVariableDeclaration__Group__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32730:1: ( rule__XVariableDeclaration__Group__0__Impl rule__XVariableDeclaration__Group__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32731:2: rule__XVariableDeclaration__Group__0__Impl rule__XVariableDeclaration__Group__1
{
pushFollow(FollowSets002.FOLLOW_rule__XVariableDeclaration__Group__0__Impl_in_rule__XVariableDeclaration__Group__065861);
rule__XVariableDeclaration__Group__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XVariableDeclaration__Group__1_in_rule__XVariableDeclaration__Group__065864);
rule__XVariableDeclaration__Group__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XVariableDeclaration__Group__0"
// $ANTLR start "rule__XVariableDeclaration__Group__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32738:1: rule__XVariableDeclaration__Group__0__Impl : ( () ) ;
public final void rule__XVariableDeclaration__Group__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32742:1: ( ( () ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32743:1: ( () )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32743:1: ( () )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32744:1: ()
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXVariableDeclarationAccess().getXVariableDeclarationAction_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32745:1: ()
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32747:1:
{
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXVariableDeclarationAccess().getXVariableDeclarationAction_0());
}
}
}
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XVariableDeclaration__Group__0__Impl"
// $ANTLR start "rule__XVariableDeclaration__Group__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32757:1: rule__XVariableDeclaration__Group__1 : rule__XVariableDeclaration__Group__1__Impl rule__XVariableDeclaration__Group__2 ;
public final void rule__XVariableDeclaration__Group__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32761:1: ( rule__XVariableDeclaration__Group__1__Impl rule__XVariableDeclaration__Group__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32762:2: rule__XVariableDeclaration__Group__1__Impl rule__XVariableDeclaration__Group__2
{
pushFollow(FollowSets002.FOLLOW_rule__XVariableDeclaration__Group__1__Impl_in_rule__XVariableDeclaration__Group__165922);
rule__XVariableDeclaration__Group__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XVariableDeclaration__Group__2_in_rule__XVariableDeclaration__Group__165925);
rule__XVariableDeclaration__Group__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XVariableDeclaration__Group__1"
// $ANTLR start "rule__XVariableDeclaration__Group__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32769:1: rule__XVariableDeclaration__Group__1__Impl : ( ( rule__XVariableDeclaration__Alternatives_1 ) ) ;
public final void rule__XVariableDeclaration__Group__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32773:1: ( ( ( rule__XVariableDeclaration__Alternatives_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32774:1: ( ( rule__XVariableDeclaration__Alternatives_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32774:1: ( ( rule__XVariableDeclaration__Alternatives_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32775:1: ( rule__XVariableDeclaration__Alternatives_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXVariableDeclarationAccess().getAlternatives_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32776:1: ( rule__XVariableDeclaration__Alternatives_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32776:2: rule__XVariableDeclaration__Alternatives_1
{
pushFollow(FollowSets002.FOLLOW_rule__XVariableDeclaration__Alternatives_1_in_rule__XVariableDeclaration__Group__1__Impl65952);
rule__XVariableDeclaration__Alternatives_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXVariableDeclarationAccess().getAlternatives_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XVariableDeclaration__Group__1__Impl"
// $ANTLR start "rule__XVariableDeclaration__Group__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32786:1: rule__XVariableDeclaration__Group__2 : rule__XVariableDeclaration__Group__2__Impl rule__XVariableDeclaration__Group__3 ;
public final void rule__XVariableDeclaration__Group__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32790:1: ( rule__XVariableDeclaration__Group__2__Impl rule__XVariableDeclaration__Group__3 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32791:2: rule__XVariableDeclaration__Group__2__Impl rule__XVariableDeclaration__Group__3
{
pushFollow(FollowSets002.FOLLOW_rule__XVariableDeclaration__Group__2__Impl_in_rule__XVariableDeclaration__Group__265982);
rule__XVariableDeclaration__Group__2__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XVariableDeclaration__Group__3_in_rule__XVariableDeclaration__Group__265985);
rule__XVariableDeclaration__Group__3();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XVariableDeclaration__Group__2"
// $ANTLR start "rule__XVariableDeclaration__Group__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32798:1: rule__XVariableDeclaration__Group__2__Impl : ( ( rule__XVariableDeclaration__Alternatives_2 ) ) ;
public final void rule__XVariableDeclaration__Group__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32802:1: ( ( ( rule__XVariableDeclaration__Alternatives_2 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32803:1: ( ( rule__XVariableDeclaration__Alternatives_2 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32803:1: ( ( rule__XVariableDeclaration__Alternatives_2 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32804:1: ( rule__XVariableDeclaration__Alternatives_2 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXVariableDeclarationAccess().getAlternatives_2());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32805:1: ( rule__XVariableDeclaration__Alternatives_2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32805:2: rule__XVariableDeclaration__Alternatives_2
{
pushFollow(FollowSets002.FOLLOW_rule__XVariableDeclaration__Alternatives_2_in_rule__XVariableDeclaration__Group__2__Impl66012);
rule__XVariableDeclaration__Alternatives_2();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXVariableDeclarationAccess().getAlternatives_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XVariableDeclaration__Group__2__Impl"
// $ANTLR start "rule__XVariableDeclaration__Group__3"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32815:1: rule__XVariableDeclaration__Group__3 : rule__XVariableDeclaration__Group__3__Impl ;
public final void rule__XVariableDeclaration__Group__3() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32819:1: ( rule__XVariableDeclaration__Group__3__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32820:2: rule__XVariableDeclaration__Group__3__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__XVariableDeclaration__Group__3__Impl_in_rule__XVariableDeclaration__Group__366042);
rule__XVariableDeclaration__Group__3__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XVariableDeclaration__Group__3"
// $ANTLR start "rule__XVariableDeclaration__Group__3__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32826:1: rule__XVariableDeclaration__Group__3__Impl : ( ( rule__XVariableDeclaration__Group_3__0 )? ) ;
public final void rule__XVariableDeclaration__Group__3__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32830:1: ( ( ( rule__XVariableDeclaration__Group_3__0 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32831:1: ( ( rule__XVariableDeclaration__Group_3__0 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32831:1: ( ( rule__XVariableDeclaration__Group_3__0 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32832:1: ( rule__XVariableDeclaration__Group_3__0 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXVariableDeclarationAccess().getGroup_3());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32833:1: ( rule__XVariableDeclaration__Group_3__0 )?
int alt238=2;
int LA238_0 = input.LA(1);
if ( (LA238_0==13) ) {
alt238=1;
}
switch (alt238) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32833:2: rule__XVariableDeclaration__Group_3__0
{
pushFollow(FollowSets002.FOLLOW_rule__XVariableDeclaration__Group_3__0_in_rule__XVariableDeclaration__Group__3__Impl66069);
rule__XVariableDeclaration__Group_3__0();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXVariableDeclarationAccess().getGroup_3());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XVariableDeclaration__Group__3__Impl"
// $ANTLR start "rule__XVariableDeclaration__Group_2_0__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32851:1: rule__XVariableDeclaration__Group_2_0__0 : rule__XVariableDeclaration__Group_2_0__0__Impl ;
public final void rule__XVariableDeclaration__Group_2_0__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32855:1: ( rule__XVariableDeclaration__Group_2_0__0__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32856:2: rule__XVariableDeclaration__Group_2_0__0__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__XVariableDeclaration__Group_2_0__0__Impl_in_rule__XVariableDeclaration__Group_2_0__066108);
rule__XVariableDeclaration__Group_2_0__0__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XVariableDeclaration__Group_2_0__0"
// $ANTLR start "rule__XVariableDeclaration__Group_2_0__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32862:1: rule__XVariableDeclaration__Group_2_0__0__Impl : ( ( rule__XVariableDeclaration__Group_2_0_0__0 ) ) ;
public final void rule__XVariableDeclaration__Group_2_0__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32866:1: ( ( ( rule__XVariableDeclaration__Group_2_0_0__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32867:1: ( ( rule__XVariableDeclaration__Group_2_0_0__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32867:1: ( ( rule__XVariableDeclaration__Group_2_0_0__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32868:1: ( rule__XVariableDeclaration__Group_2_0_0__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXVariableDeclarationAccess().getGroup_2_0_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32869:1: ( rule__XVariableDeclaration__Group_2_0_0__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32869:2: rule__XVariableDeclaration__Group_2_0_0__0
{
pushFollow(FollowSets002.FOLLOW_rule__XVariableDeclaration__Group_2_0_0__0_in_rule__XVariableDeclaration__Group_2_0__0__Impl66135);
rule__XVariableDeclaration__Group_2_0_0__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXVariableDeclarationAccess().getGroup_2_0_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XVariableDeclaration__Group_2_0__0__Impl"
// $ANTLR start "rule__XVariableDeclaration__Group_2_0_0__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32881:1: rule__XVariableDeclaration__Group_2_0_0__0 : rule__XVariableDeclaration__Group_2_0_0__0__Impl rule__XVariableDeclaration__Group_2_0_0__1 ;
public final void rule__XVariableDeclaration__Group_2_0_0__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32885:1: ( rule__XVariableDeclaration__Group_2_0_0__0__Impl rule__XVariableDeclaration__Group_2_0_0__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32886:2: rule__XVariableDeclaration__Group_2_0_0__0__Impl rule__XVariableDeclaration__Group_2_0_0__1
{
pushFollow(FollowSets002.FOLLOW_rule__XVariableDeclaration__Group_2_0_0__0__Impl_in_rule__XVariableDeclaration__Group_2_0_0__066167);
rule__XVariableDeclaration__Group_2_0_0__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XVariableDeclaration__Group_2_0_0__1_in_rule__XVariableDeclaration__Group_2_0_0__066170);
rule__XVariableDeclaration__Group_2_0_0__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XVariableDeclaration__Group_2_0_0__0"
// $ANTLR start "rule__XVariableDeclaration__Group_2_0_0__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32893:1: rule__XVariableDeclaration__Group_2_0_0__0__Impl : ( ( rule__XVariableDeclaration__TypeAssignment_2_0_0_0 ) ) ;
public final void rule__XVariableDeclaration__Group_2_0_0__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32897:1: ( ( ( rule__XVariableDeclaration__TypeAssignment_2_0_0_0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32898:1: ( ( rule__XVariableDeclaration__TypeAssignment_2_0_0_0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32898:1: ( ( rule__XVariableDeclaration__TypeAssignment_2_0_0_0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32899:1: ( rule__XVariableDeclaration__TypeAssignment_2_0_0_0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXVariableDeclarationAccess().getTypeAssignment_2_0_0_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32900:1: ( rule__XVariableDeclaration__TypeAssignment_2_0_0_0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32900:2: rule__XVariableDeclaration__TypeAssignment_2_0_0_0
{
pushFollow(FollowSets002.FOLLOW_rule__XVariableDeclaration__TypeAssignment_2_0_0_0_in_rule__XVariableDeclaration__Group_2_0_0__0__Impl66197);
rule__XVariableDeclaration__TypeAssignment_2_0_0_0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXVariableDeclarationAccess().getTypeAssignment_2_0_0_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XVariableDeclaration__Group_2_0_0__0__Impl"
// $ANTLR start "rule__XVariableDeclaration__Group_2_0_0__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32910:1: rule__XVariableDeclaration__Group_2_0_0__1 : rule__XVariableDeclaration__Group_2_0_0__1__Impl ;
public final void rule__XVariableDeclaration__Group_2_0_0__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32914:1: ( rule__XVariableDeclaration__Group_2_0_0__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32915:2: rule__XVariableDeclaration__Group_2_0_0__1__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__XVariableDeclaration__Group_2_0_0__1__Impl_in_rule__XVariableDeclaration__Group_2_0_0__166227);
rule__XVariableDeclaration__Group_2_0_0__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XVariableDeclaration__Group_2_0_0__1"
// $ANTLR start "rule__XVariableDeclaration__Group_2_0_0__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32921:1: rule__XVariableDeclaration__Group_2_0_0__1__Impl : ( ( rule__XVariableDeclaration__NameAssignment_2_0_0_1 ) ) ;
public final void rule__XVariableDeclaration__Group_2_0_0__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32925:1: ( ( ( rule__XVariableDeclaration__NameAssignment_2_0_0_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32926:1: ( ( rule__XVariableDeclaration__NameAssignment_2_0_0_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32926:1: ( ( rule__XVariableDeclaration__NameAssignment_2_0_0_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32927:1: ( rule__XVariableDeclaration__NameAssignment_2_0_0_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXVariableDeclarationAccess().getNameAssignment_2_0_0_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32928:1: ( rule__XVariableDeclaration__NameAssignment_2_0_0_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32928:2: rule__XVariableDeclaration__NameAssignment_2_0_0_1
{
pushFollow(FollowSets002.FOLLOW_rule__XVariableDeclaration__NameAssignment_2_0_0_1_in_rule__XVariableDeclaration__Group_2_0_0__1__Impl66254);
rule__XVariableDeclaration__NameAssignment_2_0_0_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXVariableDeclarationAccess().getNameAssignment_2_0_0_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XVariableDeclaration__Group_2_0_0__1__Impl"
// $ANTLR start "rule__XVariableDeclaration__Group_3__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32942:1: rule__XVariableDeclaration__Group_3__0 : rule__XVariableDeclaration__Group_3__0__Impl rule__XVariableDeclaration__Group_3__1 ;
public final void rule__XVariableDeclaration__Group_3__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32946:1: ( rule__XVariableDeclaration__Group_3__0__Impl rule__XVariableDeclaration__Group_3__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32947:2: rule__XVariableDeclaration__Group_3__0__Impl rule__XVariableDeclaration__Group_3__1
{
pushFollow(FollowSets002.FOLLOW_rule__XVariableDeclaration__Group_3__0__Impl_in_rule__XVariableDeclaration__Group_3__066288);
rule__XVariableDeclaration__Group_3__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XVariableDeclaration__Group_3__1_in_rule__XVariableDeclaration__Group_3__066291);
rule__XVariableDeclaration__Group_3__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XVariableDeclaration__Group_3__0"
// $ANTLR start "rule__XVariableDeclaration__Group_3__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32954:1: rule__XVariableDeclaration__Group_3__0__Impl : ( '=' ) ;
public final void rule__XVariableDeclaration__Group_3__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32958:1: ( ( '=' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32959:1: ( '=' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32959:1: ( '=' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32960:1: '='
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXVariableDeclarationAccess().getEqualsSignKeyword_3_0());
}
match(input,13,FollowSets002.FOLLOW_13_in_rule__XVariableDeclaration__Group_3__0__Impl66319); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXVariableDeclarationAccess().getEqualsSignKeyword_3_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XVariableDeclaration__Group_3__0__Impl"
// $ANTLR start "rule__XVariableDeclaration__Group_3__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32973:1: rule__XVariableDeclaration__Group_3__1 : rule__XVariableDeclaration__Group_3__1__Impl ;
public final void rule__XVariableDeclaration__Group_3__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32977:1: ( rule__XVariableDeclaration__Group_3__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32978:2: rule__XVariableDeclaration__Group_3__1__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__XVariableDeclaration__Group_3__1__Impl_in_rule__XVariableDeclaration__Group_3__166350);
rule__XVariableDeclaration__Group_3__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XVariableDeclaration__Group_3__1"
// $ANTLR start "rule__XVariableDeclaration__Group_3__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32984:1: rule__XVariableDeclaration__Group_3__1__Impl : ( ( rule__XVariableDeclaration__RightAssignment_3_1 ) ) ;
public final void rule__XVariableDeclaration__Group_3__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32988:1: ( ( ( rule__XVariableDeclaration__RightAssignment_3_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32989:1: ( ( rule__XVariableDeclaration__RightAssignment_3_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32989:1: ( ( rule__XVariableDeclaration__RightAssignment_3_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32990:1: ( rule__XVariableDeclaration__RightAssignment_3_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXVariableDeclarationAccess().getRightAssignment_3_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32991:1: ( rule__XVariableDeclaration__RightAssignment_3_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:32991:2: rule__XVariableDeclaration__RightAssignment_3_1
{
pushFollow(FollowSets002.FOLLOW_rule__XVariableDeclaration__RightAssignment_3_1_in_rule__XVariableDeclaration__Group_3__1__Impl66377);
rule__XVariableDeclaration__RightAssignment_3_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXVariableDeclarationAccess().getRightAssignment_3_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XVariableDeclaration__Group_3__1__Impl"
// $ANTLR start "rule__JvmFormalParameter__Group__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33005:1: rule__JvmFormalParameter__Group__0 : rule__JvmFormalParameter__Group__0__Impl rule__JvmFormalParameter__Group__1 ;
public final void rule__JvmFormalParameter__Group__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33009:1: ( rule__JvmFormalParameter__Group__0__Impl rule__JvmFormalParameter__Group__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33010:2: rule__JvmFormalParameter__Group__0__Impl rule__JvmFormalParameter__Group__1
{
pushFollow(FollowSets002.FOLLOW_rule__JvmFormalParameter__Group__0__Impl_in_rule__JvmFormalParameter__Group__066411);
rule__JvmFormalParameter__Group__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__JvmFormalParameter__Group__1_in_rule__JvmFormalParameter__Group__066414);
rule__JvmFormalParameter__Group__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__JvmFormalParameter__Group__0"
// $ANTLR start "rule__JvmFormalParameter__Group__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33017:1: rule__JvmFormalParameter__Group__0__Impl : ( ( rule__JvmFormalParameter__ParameterTypeAssignment_0 )? ) ;
public final void rule__JvmFormalParameter__Group__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33021:1: ( ( ( rule__JvmFormalParameter__ParameterTypeAssignment_0 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33022:1: ( ( rule__JvmFormalParameter__ParameterTypeAssignment_0 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33022:1: ( ( rule__JvmFormalParameter__ParameterTypeAssignment_0 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33023:1: ( rule__JvmFormalParameter__ParameterTypeAssignment_0 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getJvmFormalParameterAccess().getParameterTypeAssignment_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33024:1: ( rule__JvmFormalParameter__ParameterTypeAssignment_0 )?
int alt239=2;
int LA239_0 = input.LA(1);
if ( (LA239_0==RULE_ID) ) {
int LA239_1 = input.LA(2);
if ( (LA239_1==RULE_ID||LA239_1==27||LA239_1==43||LA239_1==156) ) {
alt239=1;
}
}
else if ( (LA239_0==31||LA239_0==144) ) {
alt239=1;
}
switch (alt239) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33024:2: rule__JvmFormalParameter__ParameterTypeAssignment_0
{
pushFollow(FollowSets002.FOLLOW_rule__JvmFormalParameter__ParameterTypeAssignment_0_in_rule__JvmFormalParameter__Group__0__Impl66441);
rule__JvmFormalParameter__ParameterTypeAssignment_0();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getJvmFormalParameterAccess().getParameterTypeAssignment_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__JvmFormalParameter__Group__0__Impl"
// $ANTLR start "rule__JvmFormalParameter__Group__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33034:1: rule__JvmFormalParameter__Group__1 : rule__JvmFormalParameter__Group__1__Impl ;
public final void rule__JvmFormalParameter__Group__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33038:1: ( rule__JvmFormalParameter__Group__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33039:2: rule__JvmFormalParameter__Group__1__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__JvmFormalParameter__Group__1__Impl_in_rule__JvmFormalParameter__Group__166472);
rule__JvmFormalParameter__Group__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__JvmFormalParameter__Group__1"
// $ANTLR start "rule__JvmFormalParameter__Group__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33045:1: rule__JvmFormalParameter__Group__1__Impl : ( ( rule__JvmFormalParameter__NameAssignment_1 ) ) ;
public final void rule__JvmFormalParameter__Group__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33049:1: ( ( ( rule__JvmFormalParameter__NameAssignment_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33050:1: ( ( rule__JvmFormalParameter__NameAssignment_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33050:1: ( ( rule__JvmFormalParameter__NameAssignment_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33051:1: ( rule__JvmFormalParameter__NameAssignment_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getJvmFormalParameterAccess().getNameAssignment_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33052:1: ( rule__JvmFormalParameter__NameAssignment_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33052:2: rule__JvmFormalParameter__NameAssignment_1
{
pushFollow(FollowSets002.FOLLOW_rule__JvmFormalParameter__NameAssignment_1_in_rule__JvmFormalParameter__Group__1__Impl66499);
rule__JvmFormalParameter__NameAssignment_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getJvmFormalParameterAccess().getNameAssignment_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__JvmFormalParameter__Group__1__Impl"
// $ANTLR start "rule__FullJvmFormalParameter__Group__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33066:1: rule__FullJvmFormalParameter__Group__0 : rule__FullJvmFormalParameter__Group__0__Impl rule__FullJvmFormalParameter__Group__1 ;
public final void rule__FullJvmFormalParameter__Group__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33070:1: ( rule__FullJvmFormalParameter__Group__0__Impl rule__FullJvmFormalParameter__Group__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33071:2: rule__FullJvmFormalParameter__Group__0__Impl rule__FullJvmFormalParameter__Group__1
{
pushFollow(FollowSets002.FOLLOW_rule__FullJvmFormalParameter__Group__0__Impl_in_rule__FullJvmFormalParameter__Group__066533);
rule__FullJvmFormalParameter__Group__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__FullJvmFormalParameter__Group__1_in_rule__FullJvmFormalParameter__Group__066536);
rule__FullJvmFormalParameter__Group__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__FullJvmFormalParameter__Group__0"
// $ANTLR start "rule__FullJvmFormalParameter__Group__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33078:1: rule__FullJvmFormalParameter__Group__0__Impl : ( ( rule__FullJvmFormalParameter__ParameterTypeAssignment_0 ) ) ;
public final void rule__FullJvmFormalParameter__Group__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33082:1: ( ( ( rule__FullJvmFormalParameter__ParameterTypeAssignment_0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33083:1: ( ( rule__FullJvmFormalParameter__ParameterTypeAssignment_0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33083:1: ( ( rule__FullJvmFormalParameter__ParameterTypeAssignment_0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33084:1: ( rule__FullJvmFormalParameter__ParameterTypeAssignment_0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getFullJvmFormalParameterAccess().getParameterTypeAssignment_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33085:1: ( rule__FullJvmFormalParameter__ParameterTypeAssignment_0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33085:2: rule__FullJvmFormalParameter__ParameterTypeAssignment_0
{
pushFollow(FollowSets002.FOLLOW_rule__FullJvmFormalParameter__ParameterTypeAssignment_0_in_rule__FullJvmFormalParameter__Group__0__Impl66563);
rule__FullJvmFormalParameter__ParameterTypeAssignment_0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getFullJvmFormalParameterAccess().getParameterTypeAssignment_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__FullJvmFormalParameter__Group__0__Impl"
// $ANTLR start "rule__FullJvmFormalParameter__Group__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33095:1: rule__FullJvmFormalParameter__Group__1 : rule__FullJvmFormalParameter__Group__1__Impl ;
public final void rule__FullJvmFormalParameter__Group__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33099:1: ( rule__FullJvmFormalParameter__Group__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33100:2: rule__FullJvmFormalParameter__Group__1__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__FullJvmFormalParameter__Group__1__Impl_in_rule__FullJvmFormalParameter__Group__166593);
rule__FullJvmFormalParameter__Group__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__FullJvmFormalParameter__Group__1"
// $ANTLR start "rule__FullJvmFormalParameter__Group__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33106:1: rule__FullJvmFormalParameter__Group__1__Impl : ( ( rule__FullJvmFormalParameter__NameAssignment_1 ) ) ;
public final void rule__FullJvmFormalParameter__Group__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33110:1: ( ( ( rule__FullJvmFormalParameter__NameAssignment_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33111:1: ( ( rule__FullJvmFormalParameter__NameAssignment_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33111:1: ( ( rule__FullJvmFormalParameter__NameAssignment_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33112:1: ( rule__FullJvmFormalParameter__NameAssignment_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getFullJvmFormalParameterAccess().getNameAssignment_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33113:1: ( rule__FullJvmFormalParameter__NameAssignment_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33113:2: rule__FullJvmFormalParameter__NameAssignment_1
{
pushFollow(FollowSets002.FOLLOW_rule__FullJvmFormalParameter__NameAssignment_1_in_rule__FullJvmFormalParameter__Group__1__Impl66620);
rule__FullJvmFormalParameter__NameAssignment_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getFullJvmFormalParameterAccess().getNameAssignment_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__FullJvmFormalParameter__Group__1__Impl"
// $ANTLR start "rule__XFeatureCall__Group__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33127:1: rule__XFeatureCall__Group__0 : rule__XFeatureCall__Group__0__Impl rule__XFeatureCall__Group__1 ;
public final void rule__XFeatureCall__Group__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33131:1: ( rule__XFeatureCall__Group__0__Impl rule__XFeatureCall__Group__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33132:2: rule__XFeatureCall__Group__0__Impl rule__XFeatureCall__Group__1
{
pushFollow(FollowSets002.FOLLOW_rule__XFeatureCall__Group__0__Impl_in_rule__XFeatureCall__Group__066654);
rule__XFeatureCall__Group__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XFeatureCall__Group__1_in_rule__XFeatureCall__Group__066657);
rule__XFeatureCall__Group__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XFeatureCall__Group__0"
// $ANTLR start "rule__XFeatureCall__Group__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33139:1: rule__XFeatureCall__Group__0__Impl : ( () ) ;
public final void rule__XFeatureCall__Group__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33143:1: ( ( () ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33144:1: ( () )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33144:1: ( () )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33145:1: ()
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXFeatureCallAccess().getXFeatureCallAction_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33146:1: ()
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33148:1:
{
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXFeatureCallAccess().getXFeatureCallAction_0());
}
}
}
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XFeatureCall__Group__0__Impl"
// $ANTLR start "rule__XFeatureCall__Group__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33158:1: rule__XFeatureCall__Group__1 : rule__XFeatureCall__Group__1__Impl rule__XFeatureCall__Group__2 ;
public final void rule__XFeatureCall__Group__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33162:1: ( rule__XFeatureCall__Group__1__Impl rule__XFeatureCall__Group__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33163:2: rule__XFeatureCall__Group__1__Impl rule__XFeatureCall__Group__2
{
pushFollow(FollowSets002.FOLLOW_rule__XFeatureCall__Group__1__Impl_in_rule__XFeatureCall__Group__166715);
rule__XFeatureCall__Group__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XFeatureCall__Group__2_in_rule__XFeatureCall__Group__166718);
rule__XFeatureCall__Group__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XFeatureCall__Group__1"
// $ANTLR start "rule__XFeatureCall__Group__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33170:1: rule__XFeatureCall__Group__1__Impl : ( ( rule__XFeatureCall__Group_1__0 )? ) ;
public final void rule__XFeatureCall__Group__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33174:1: ( ( ( rule__XFeatureCall__Group_1__0 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33175:1: ( ( rule__XFeatureCall__Group_1__0 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33175:1: ( ( rule__XFeatureCall__Group_1__0 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33176:1: ( rule__XFeatureCall__Group_1__0 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXFeatureCallAccess().getGroup_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33177:1: ( rule__XFeatureCall__Group_1__0 )?
int alt240=2;
int LA240_0 = input.LA(1);
if ( (LA240_0==27) ) {
alt240=1;
}
switch (alt240) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33177:2: rule__XFeatureCall__Group_1__0
{
pushFollow(FollowSets002.FOLLOW_rule__XFeatureCall__Group_1__0_in_rule__XFeatureCall__Group__1__Impl66745);
rule__XFeatureCall__Group_1__0();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXFeatureCallAccess().getGroup_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XFeatureCall__Group__1__Impl"
// $ANTLR start "rule__XFeatureCall__Group__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33187:1: rule__XFeatureCall__Group__2 : rule__XFeatureCall__Group__2__Impl rule__XFeatureCall__Group__3 ;
public final void rule__XFeatureCall__Group__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33191:1: ( rule__XFeatureCall__Group__2__Impl rule__XFeatureCall__Group__3 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33192:2: rule__XFeatureCall__Group__2__Impl rule__XFeatureCall__Group__3
{
pushFollow(FollowSets002.FOLLOW_rule__XFeatureCall__Group__2__Impl_in_rule__XFeatureCall__Group__266776);
rule__XFeatureCall__Group__2__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XFeatureCall__Group__3_in_rule__XFeatureCall__Group__266779);
rule__XFeatureCall__Group__3();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XFeatureCall__Group__2"
// $ANTLR start "rule__XFeatureCall__Group__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33199:1: rule__XFeatureCall__Group__2__Impl : ( ( rule__XFeatureCall__FeatureAssignment_2 ) ) ;
public final void rule__XFeatureCall__Group__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33203:1: ( ( ( rule__XFeatureCall__FeatureAssignment_2 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33204:1: ( ( rule__XFeatureCall__FeatureAssignment_2 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33204:1: ( ( rule__XFeatureCall__FeatureAssignment_2 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33205:1: ( rule__XFeatureCall__FeatureAssignment_2 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXFeatureCallAccess().getFeatureAssignment_2());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33206:1: ( rule__XFeatureCall__FeatureAssignment_2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33206:2: rule__XFeatureCall__FeatureAssignment_2
{
pushFollow(FollowSets002.FOLLOW_rule__XFeatureCall__FeatureAssignment_2_in_rule__XFeatureCall__Group__2__Impl66806);
rule__XFeatureCall__FeatureAssignment_2();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXFeatureCallAccess().getFeatureAssignment_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XFeatureCall__Group__2__Impl"
// $ANTLR start "rule__XFeatureCall__Group__3"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33216:1: rule__XFeatureCall__Group__3 : rule__XFeatureCall__Group__3__Impl rule__XFeatureCall__Group__4 ;
public final void rule__XFeatureCall__Group__3() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33220:1: ( rule__XFeatureCall__Group__3__Impl rule__XFeatureCall__Group__4 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33221:2: rule__XFeatureCall__Group__3__Impl rule__XFeatureCall__Group__4
{
pushFollow(FollowSets002.FOLLOW_rule__XFeatureCall__Group__3__Impl_in_rule__XFeatureCall__Group__366836);
rule__XFeatureCall__Group__3__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XFeatureCall__Group__4_in_rule__XFeatureCall__Group__366839);
rule__XFeatureCall__Group__4();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XFeatureCall__Group__3"
// $ANTLR start "rule__XFeatureCall__Group__3__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33228:1: rule__XFeatureCall__Group__3__Impl : ( ( rule__XFeatureCall__Group_3__0 )? ) ;
public final void rule__XFeatureCall__Group__3__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33232:1: ( ( ( rule__XFeatureCall__Group_3__0 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33233:1: ( ( rule__XFeatureCall__Group_3__0 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33233:1: ( ( rule__XFeatureCall__Group_3__0 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33234:1: ( rule__XFeatureCall__Group_3__0 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXFeatureCallAccess().getGroup_3());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33235:1: ( rule__XFeatureCall__Group_3__0 )?
int alt241=2;
alt241 = dfa241.predict(input);
switch (alt241) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33235:2: rule__XFeatureCall__Group_3__0
{
pushFollow(FollowSets002.FOLLOW_rule__XFeatureCall__Group_3__0_in_rule__XFeatureCall__Group__3__Impl66866);
rule__XFeatureCall__Group_3__0();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXFeatureCallAccess().getGroup_3());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XFeatureCall__Group__3__Impl"
// $ANTLR start "rule__XFeatureCall__Group__4"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33245:1: rule__XFeatureCall__Group__4 : rule__XFeatureCall__Group__4__Impl ;
public final void rule__XFeatureCall__Group__4() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33249:1: ( rule__XFeatureCall__Group__4__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33250:2: rule__XFeatureCall__Group__4__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__XFeatureCall__Group__4__Impl_in_rule__XFeatureCall__Group__466897);
rule__XFeatureCall__Group__4__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XFeatureCall__Group__4"
// $ANTLR start "rule__XFeatureCall__Group__4__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33256:1: rule__XFeatureCall__Group__4__Impl : ( ( rule__XFeatureCall__FeatureCallArgumentsAssignment_4 )? ) ;
public final void rule__XFeatureCall__Group__4__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33260:1: ( ( ( rule__XFeatureCall__FeatureCallArgumentsAssignment_4 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33261:1: ( ( rule__XFeatureCall__FeatureCallArgumentsAssignment_4 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33261:1: ( ( rule__XFeatureCall__FeatureCallArgumentsAssignment_4 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33262:1: ( rule__XFeatureCall__FeatureCallArgumentsAssignment_4 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXFeatureCallAccess().getFeatureCallArgumentsAssignment_4());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33263:1: ( rule__XFeatureCall__FeatureCallArgumentsAssignment_4 )?
int alt242=2;
alt242 = dfa242.predict(input);
switch (alt242) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33263:2: rule__XFeatureCall__FeatureCallArgumentsAssignment_4
{
pushFollow(FollowSets002.FOLLOW_rule__XFeatureCall__FeatureCallArgumentsAssignment_4_in_rule__XFeatureCall__Group__4__Impl66924);
rule__XFeatureCall__FeatureCallArgumentsAssignment_4();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXFeatureCallAccess().getFeatureCallArgumentsAssignment_4());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XFeatureCall__Group__4__Impl"
// $ANTLR start "rule__XFeatureCall__Group_1__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33283:1: rule__XFeatureCall__Group_1__0 : rule__XFeatureCall__Group_1__0__Impl rule__XFeatureCall__Group_1__1 ;
public final void rule__XFeatureCall__Group_1__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33287:1: ( rule__XFeatureCall__Group_1__0__Impl rule__XFeatureCall__Group_1__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33288:2: rule__XFeatureCall__Group_1__0__Impl rule__XFeatureCall__Group_1__1
{
pushFollow(FollowSets002.FOLLOW_rule__XFeatureCall__Group_1__0__Impl_in_rule__XFeatureCall__Group_1__066965);
rule__XFeatureCall__Group_1__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XFeatureCall__Group_1__1_in_rule__XFeatureCall__Group_1__066968);
rule__XFeatureCall__Group_1__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XFeatureCall__Group_1__0"
// $ANTLR start "rule__XFeatureCall__Group_1__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33295:1: rule__XFeatureCall__Group_1__0__Impl : ( '<' ) ;
public final void rule__XFeatureCall__Group_1__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33299:1: ( ( '<' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33300:1: ( '<' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33300:1: ( '<' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33301:1: '<'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXFeatureCallAccess().getLessThanSignKeyword_1_0());
}
match(input,27,FollowSets002.FOLLOW_27_in_rule__XFeatureCall__Group_1__0__Impl66996); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXFeatureCallAccess().getLessThanSignKeyword_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XFeatureCall__Group_1__0__Impl"
// $ANTLR start "rule__XFeatureCall__Group_1__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33314:1: rule__XFeatureCall__Group_1__1 : rule__XFeatureCall__Group_1__1__Impl rule__XFeatureCall__Group_1__2 ;
public final void rule__XFeatureCall__Group_1__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33318:1: ( rule__XFeatureCall__Group_1__1__Impl rule__XFeatureCall__Group_1__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33319:2: rule__XFeatureCall__Group_1__1__Impl rule__XFeatureCall__Group_1__2
{
pushFollow(FollowSets002.FOLLOW_rule__XFeatureCall__Group_1__1__Impl_in_rule__XFeatureCall__Group_1__167027);
rule__XFeatureCall__Group_1__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XFeatureCall__Group_1__2_in_rule__XFeatureCall__Group_1__167030);
rule__XFeatureCall__Group_1__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XFeatureCall__Group_1__1"
// $ANTLR start "rule__XFeatureCall__Group_1__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33326:1: rule__XFeatureCall__Group_1__1__Impl : ( ( rule__XFeatureCall__TypeArgumentsAssignment_1_1 ) ) ;
public final void rule__XFeatureCall__Group_1__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33330:1: ( ( ( rule__XFeatureCall__TypeArgumentsAssignment_1_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33331:1: ( ( rule__XFeatureCall__TypeArgumentsAssignment_1_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33331:1: ( ( rule__XFeatureCall__TypeArgumentsAssignment_1_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33332:1: ( rule__XFeatureCall__TypeArgumentsAssignment_1_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXFeatureCallAccess().getTypeArgumentsAssignment_1_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33333:1: ( rule__XFeatureCall__TypeArgumentsAssignment_1_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33333:2: rule__XFeatureCall__TypeArgumentsAssignment_1_1
{
pushFollow(FollowSets002.FOLLOW_rule__XFeatureCall__TypeArgumentsAssignment_1_1_in_rule__XFeatureCall__Group_1__1__Impl67057);
rule__XFeatureCall__TypeArgumentsAssignment_1_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXFeatureCallAccess().getTypeArgumentsAssignment_1_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XFeatureCall__Group_1__1__Impl"
// $ANTLR start "rule__XFeatureCall__Group_1__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33343:1: rule__XFeatureCall__Group_1__2 : rule__XFeatureCall__Group_1__2__Impl rule__XFeatureCall__Group_1__3 ;
public final void rule__XFeatureCall__Group_1__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33347:1: ( rule__XFeatureCall__Group_1__2__Impl rule__XFeatureCall__Group_1__3 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33348:2: rule__XFeatureCall__Group_1__2__Impl rule__XFeatureCall__Group_1__3
{
pushFollow(FollowSets002.FOLLOW_rule__XFeatureCall__Group_1__2__Impl_in_rule__XFeatureCall__Group_1__267087);
rule__XFeatureCall__Group_1__2__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XFeatureCall__Group_1__3_in_rule__XFeatureCall__Group_1__267090);
rule__XFeatureCall__Group_1__3();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XFeatureCall__Group_1__2"
// $ANTLR start "rule__XFeatureCall__Group_1__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33355:1: rule__XFeatureCall__Group_1__2__Impl : ( ( rule__XFeatureCall__Group_1_2__0 )* ) ;
public final void rule__XFeatureCall__Group_1__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33359:1: ( ( ( rule__XFeatureCall__Group_1_2__0 )* ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33360:1: ( ( rule__XFeatureCall__Group_1_2__0 )* )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33360:1: ( ( rule__XFeatureCall__Group_1_2__0 )* )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33361:1: ( rule__XFeatureCall__Group_1_2__0 )*
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXFeatureCallAccess().getGroup_1_2());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33362:1: ( rule__XFeatureCall__Group_1_2__0 )*
loop243:
do {
int alt243=2;
int LA243_0 = input.LA(1);
if ( (LA243_0==154) ) {
alt243=1;
}
switch (alt243) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33362:2: rule__XFeatureCall__Group_1_2__0
{
pushFollow(FollowSets002.FOLLOW_rule__XFeatureCall__Group_1_2__0_in_rule__XFeatureCall__Group_1__2__Impl67117);
rule__XFeatureCall__Group_1_2__0();
state._fsp--;
if (state.failed) return ;
}
break;
default :
break loop243;
}
} while (true);
if ( state.backtracking==0 ) {
after(grammarAccess.getXFeatureCallAccess().getGroup_1_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XFeatureCall__Group_1__2__Impl"
// $ANTLR start "rule__XFeatureCall__Group_1__3"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33372:1: rule__XFeatureCall__Group_1__3 : rule__XFeatureCall__Group_1__3__Impl ;
public final void rule__XFeatureCall__Group_1__3() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33376:1: ( rule__XFeatureCall__Group_1__3__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33377:2: rule__XFeatureCall__Group_1__3__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__XFeatureCall__Group_1__3__Impl_in_rule__XFeatureCall__Group_1__367148);
rule__XFeatureCall__Group_1__3__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XFeatureCall__Group_1__3"
// $ANTLR start "rule__XFeatureCall__Group_1__3__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33383:1: rule__XFeatureCall__Group_1__3__Impl : ( '>' ) ;
public final void rule__XFeatureCall__Group_1__3__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33387:1: ( ( '>' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33388:1: ( '>' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33388:1: ( '>' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33389:1: '>'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXFeatureCallAccess().getGreaterThanSignKeyword_1_3());
}
match(input,26,FollowSets002.FOLLOW_26_in_rule__XFeatureCall__Group_1__3__Impl67176); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXFeatureCallAccess().getGreaterThanSignKeyword_1_3());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XFeatureCall__Group_1__3__Impl"
// $ANTLR start "rule__XFeatureCall__Group_1_2__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33410:1: rule__XFeatureCall__Group_1_2__0 : rule__XFeatureCall__Group_1_2__0__Impl rule__XFeatureCall__Group_1_2__1 ;
public final void rule__XFeatureCall__Group_1_2__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33414:1: ( rule__XFeatureCall__Group_1_2__0__Impl rule__XFeatureCall__Group_1_2__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33415:2: rule__XFeatureCall__Group_1_2__0__Impl rule__XFeatureCall__Group_1_2__1
{
pushFollow(FollowSets002.FOLLOW_rule__XFeatureCall__Group_1_2__0__Impl_in_rule__XFeatureCall__Group_1_2__067215);
rule__XFeatureCall__Group_1_2__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XFeatureCall__Group_1_2__1_in_rule__XFeatureCall__Group_1_2__067218);
rule__XFeatureCall__Group_1_2__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XFeatureCall__Group_1_2__0"
// $ANTLR start "rule__XFeatureCall__Group_1_2__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33422:1: rule__XFeatureCall__Group_1_2__0__Impl : ( ',' ) ;
public final void rule__XFeatureCall__Group_1_2__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33426:1: ( ( ',' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33427:1: ( ',' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33427:1: ( ',' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33428:1: ','
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXFeatureCallAccess().getCommaKeyword_1_2_0());
}
match(input,154,FollowSets002.FOLLOW_154_in_rule__XFeatureCall__Group_1_2__0__Impl67246); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXFeatureCallAccess().getCommaKeyword_1_2_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XFeatureCall__Group_1_2__0__Impl"
// $ANTLR start "rule__XFeatureCall__Group_1_2__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33441:1: rule__XFeatureCall__Group_1_2__1 : rule__XFeatureCall__Group_1_2__1__Impl ;
public final void rule__XFeatureCall__Group_1_2__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33445:1: ( rule__XFeatureCall__Group_1_2__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33446:2: rule__XFeatureCall__Group_1_2__1__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__XFeatureCall__Group_1_2__1__Impl_in_rule__XFeatureCall__Group_1_2__167277);
rule__XFeatureCall__Group_1_2__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XFeatureCall__Group_1_2__1"
// $ANTLR start "rule__XFeatureCall__Group_1_2__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33452:1: rule__XFeatureCall__Group_1_2__1__Impl : ( ( rule__XFeatureCall__TypeArgumentsAssignment_1_2_1 ) ) ;
public final void rule__XFeatureCall__Group_1_2__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33456:1: ( ( ( rule__XFeatureCall__TypeArgumentsAssignment_1_2_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33457:1: ( ( rule__XFeatureCall__TypeArgumentsAssignment_1_2_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33457:1: ( ( rule__XFeatureCall__TypeArgumentsAssignment_1_2_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33458:1: ( rule__XFeatureCall__TypeArgumentsAssignment_1_2_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXFeatureCallAccess().getTypeArgumentsAssignment_1_2_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33459:1: ( rule__XFeatureCall__TypeArgumentsAssignment_1_2_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33459:2: rule__XFeatureCall__TypeArgumentsAssignment_1_2_1
{
pushFollow(FollowSets002.FOLLOW_rule__XFeatureCall__TypeArgumentsAssignment_1_2_1_in_rule__XFeatureCall__Group_1_2__1__Impl67304);
rule__XFeatureCall__TypeArgumentsAssignment_1_2_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXFeatureCallAccess().getTypeArgumentsAssignment_1_2_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XFeatureCall__Group_1_2__1__Impl"
// $ANTLR start "rule__XFeatureCall__Group_3__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33473:1: rule__XFeatureCall__Group_3__0 : rule__XFeatureCall__Group_3__0__Impl rule__XFeatureCall__Group_3__1 ;
public final void rule__XFeatureCall__Group_3__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33477:1: ( rule__XFeatureCall__Group_3__0__Impl rule__XFeatureCall__Group_3__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33478:2: rule__XFeatureCall__Group_3__0__Impl rule__XFeatureCall__Group_3__1
{
pushFollow(FollowSets002.FOLLOW_rule__XFeatureCall__Group_3__0__Impl_in_rule__XFeatureCall__Group_3__067338);
rule__XFeatureCall__Group_3__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XFeatureCall__Group_3__1_in_rule__XFeatureCall__Group_3__067341);
rule__XFeatureCall__Group_3__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XFeatureCall__Group_3__0"
// $ANTLR start "rule__XFeatureCall__Group_3__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33485:1: rule__XFeatureCall__Group_3__0__Impl : ( ( rule__XFeatureCall__ExplicitOperationCallAssignment_3_0 ) ) ;
public final void rule__XFeatureCall__Group_3__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33489:1: ( ( ( rule__XFeatureCall__ExplicitOperationCallAssignment_3_0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33490:1: ( ( rule__XFeatureCall__ExplicitOperationCallAssignment_3_0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33490:1: ( ( rule__XFeatureCall__ExplicitOperationCallAssignment_3_0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33491:1: ( rule__XFeatureCall__ExplicitOperationCallAssignment_3_0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXFeatureCallAccess().getExplicitOperationCallAssignment_3_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33492:1: ( rule__XFeatureCall__ExplicitOperationCallAssignment_3_0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33492:2: rule__XFeatureCall__ExplicitOperationCallAssignment_3_0
{
pushFollow(FollowSets002.FOLLOW_rule__XFeatureCall__ExplicitOperationCallAssignment_3_0_in_rule__XFeatureCall__Group_3__0__Impl67368);
rule__XFeatureCall__ExplicitOperationCallAssignment_3_0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXFeatureCallAccess().getExplicitOperationCallAssignment_3_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XFeatureCall__Group_3__0__Impl"
// $ANTLR start "rule__XFeatureCall__Group_3__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33502:1: rule__XFeatureCall__Group_3__1 : rule__XFeatureCall__Group_3__1__Impl rule__XFeatureCall__Group_3__2 ;
public final void rule__XFeatureCall__Group_3__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33506:1: ( rule__XFeatureCall__Group_3__1__Impl rule__XFeatureCall__Group_3__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33507:2: rule__XFeatureCall__Group_3__1__Impl rule__XFeatureCall__Group_3__2
{
pushFollow(FollowSets002.FOLLOW_rule__XFeatureCall__Group_3__1__Impl_in_rule__XFeatureCall__Group_3__167398);
rule__XFeatureCall__Group_3__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XFeatureCall__Group_3__2_in_rule__XFeatureCall__Group_3__167401);
rule__XFeatureCall__Group_3__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XFeatureCall__Group_3__1"
// $ANTLR start "rule__XFeatureCall__Group_3__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33514:1: rule__XFeatureCall__Group_3__1__Impl : ( ( rule__XFeatureCall__Alternatives_3_1 )? ) ;
public final void rule__XFeatureCall__Group_3__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33518:1: ( ( ( rule__XFeatureCall__Alternatives_3_1 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33519:1: ( ( rule__XFeatureCall__Alternatives_3_1 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33519:1: ( ( rule__XFeatureCall__Alternatives_3_1 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33520:1: ( rule__XFeatureCall__Alternatives_3_1 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXFeatureCallAccess().getAlternatives_3_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33521:1: ( rule__XFeatureCall__Alternatives_3_1 )?
int alt244=2;
int LA244_0 = input.LA(1);
if ( ((LA244_0>=RULE_ID && LA244_0<=RULE_STRING)||LA244_0==27||LA244_0==31||(LA244_0>=34 && LA244_0<=35)||LA244_0==40||(LA244_0>=45 && LA244_0<=50)||LA244_0==67||LA244_0==144||(LA244_0>=155 && LA244_0<=156)||LA244_0==159||LA244_0==161||(LA244_0>=165 && LA244_0<=173)||LA244_0==175||LA244_0==183||LA244_0==185) ) {
alt244=1;
}
switch (alt244) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33521:2: rule__XFeatureCall__Alternatives_3_1
{
pushFollow(FollowSets002.FOLLOW_rule__XFeatureCall__Alternatives_3_1_in_rule__XFeatureCall__Group_3__1__Impl67428);
rule__XFeatureCall__Alternatives_3_1();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXFeatureCallAccess().getAlternatives_3_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XFeatureCall__Group_3__1__Impl"
// $ANTLR start "rule__XFeatureCall__Group_3__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33531:1: rule__XFeatureCall__Group_3__2 : rule__XFeatureCall__Group_3__2__Impl ;
public final void rule__XFeatureCall__Group_3__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33535:1: ( rule__XFeatureCall__Group_3__2__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33536:2: rule__XFeatureCall__Group_3__2__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__XFeatureCall__Group_3__2__Impl_in_rule__XFeatureCall__Group_3__267459);
rule__XFeatureCall__Group_3__2__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XFeatureCall__Group_3__2"
// $ANTLR start "rule__XFeatureCall__Group_3__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33542:1: rule__XFeatureCall__Group_3__2__Impl : ( ')' ) ;
public final void rule__XFeatureCall__Group_3__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33546:1: ( ( ')' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33547:1: ( ')' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33547:1: ( ')' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33548:1: ')'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXFeatureCallAccess().getRightParenthesisKeyword_3_2());
}
match(input,145,FollowSets002.FOLLOW_145_in_rule__XFeatureCall__Group_3__2__Impl67487); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXFeatureCallAccess().getRightParenthesisKeyword_3_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XFeatureCall__Group_3__2__Impl"
// $ANTLR start "rule__XFeatureCall__Group_3_1_1__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33567:1: rule__XFeatureCall__Group_3_1_1__0 : rule__XFeatureCall__Group_3_1_1__0__Impl rule__XFeatureCall__Group_3_1_1__1 ;
public final void rule__XFeatureCall__Group_3_1_1__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33571:1: ( rule__XFeatureCall__Group_3_1_1__0__Impl rule__XFeatureCall__Group_3_1_1__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33572:2: rule__XFeatureCall__Group_3_1_1__0__Impl rule__XFeatureCall__Group_3_1_1__1
{
pushFollow(FollowSets002.FOLLOW_rule__XFeatureCall__Group_3_1_1__0__Impl_in_rule__XFeatureCall__Group_3_1_1__067524);
rule__XFeatureCall__Group_3_1_1__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XFeatureCall__Group_3_1_1__1_in_rule__XFeatureCall__Group_3_1_1__067527);
rule__XFeatureCall__Group_3_1_1__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XFeatureCall__Group_3_1_1__0"
// $ANTLR start "rule__XFeatureCall__Group_3_1_1__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33579:1: rule__XFeatureCall__Group_3_1_1__0__Impl : ( ( rule__XFeatureCall__FeatureCallArgumentsAssignment_3_1_1_0 ) ) ;
public final void rule__XFeatureCall__Group_3_1_1__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33583:1: ( ( ( rule__XFeatureCall__FeatureCallArgumentsAssignment_3_1_1_0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33584:1: ( ( rule__XFeatureCall__FeatureCallArgumentsAssignment_3_1_1_0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33584:1: ( ( rule__XFeatureCall__FeatureCallArgumentsAssignment_3_1_1_0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33585:1: ( rule__XFeatureCall__FeatureCallArgumentsAssignment_3_1_1_0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXFeatureCallAccess().getFeatureCallArgumentsAssignment_3_1_1_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33586:1: ( rule__XFeatureCall__FeatureCallArgumentsAssignment_3_1_1_0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33586:2: rule__XFeatureCall__FeatureCallArgumentsAssignment_3_1_1_0
{
pushFollow(FollowSets002.FOLLOW_rule__XFeatureCall__FeatureCallArgumentsAssignment_3_1_1_0_in_rule__XFeatureCall__Group_3_1_1__0__Impl67554);
rule__XFeatureCall__FeatureCallArgumentsAssignment_3_1_1_0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXFeatureCallAccess().getFeatureCallArgumentsAssignment_3_1_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XFeatureCall__Group_3_1_1__0__Impl"
// $ANTLR start "rule__XFeatureCall__Group_3_1_1__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33596:1: rule__XFeatureCall__Group_3_1_1__1 : rule__XFeatureCall__Group_3_1_1__1__Impl ;
public final void rule__XFeatureCall__Group_3_1_1__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33600:1: ( rule__XFeatureCall__Group_3_1_1__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33601:2: rule__XFeatureCall__Group_3_1_1__1__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__XFeatureCall__Group_3_1_1__1__Impl_in_rule__XFeatureCall__Group_3_1_1__167584);
rule__XFeatureCall__Group_3_1_1__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XFeatureCall__Group_3_1_1__1"
// $ANTLR start "rule__XFeatureCall__Group_3_1_1__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33607:1: rule__XFeatureCall__Group_3_1_1__1__Impl : ( ( rule__XFeatureCall__Group_3_1_1_1__0 )* ) ;
public final void rule__XFeatureCall__Group_3_1_1__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33611:1: ( ( ( rule__XFeatureCall__Group_3_1_1_1__0 )* ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33612:1: ( ( rule__XFeatureCall__Group_3_1_1_1__0 )* )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33612:1: ( ( rule__XFeatureCall__Group_3_1_1_1__0 )* )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33613:1: ( rule__XFeatureCall__Group_3_1_1_1__0 )*
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXFeatureCallAccess().getGroup_3_1_1_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33614:1: ( rule__XFeatureCall__Group_3_1_1_1__0 )*
loop245:
do {
int alt245=2;
int LA245_0 = input.LA(1);
if ( (LA245_0==154) ) {
alt245=1;
}
switch (alt245) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33614:2: rule__XFeatureCall__Group_3_1_1_1__0
{
pushFollow(FollowSets002.FOLLOW_rule__XFeatureCall__Group_3_1_1_1__0_in_rule__XFeatureCall__Group_3_1_1__1__Impl67611);
rule__XFeatureCall__Group_3_1_1_1__0();
state._fsp--;
if (state.failed) return ;
}
break;
default :
break loop245;
}
} while (true);
if ( state.backtracking==0 ) {
after(grammarAccess.getXFeatureCallAccess().getGroup_3_1_1_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XFeatureCall__Group_3_1_1__1__Impl"
// $ANTLR start "rule__XFeatureCall__Group_3_1_1_1__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33628:1: rule__XFeatureCall__Group_3_1_1_1__0 : rule__XFeatureCall__Group_3_1_1_1__0__Impl rule__XFeatureCall__Group_3_1_1_1__1 ;
public final void rule__XFeatureCall__Group_3_1_1_1__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33632:1: ( rule__XFeatureCall__Group_3_1_1_1__0__Impl rule__XFeatureCall__Group_3_1_1_1__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33633:2: rule__XFeatureCall__Group_3_1_1_1__0__Impl rule__XFeatureCall__Group_3_1_1_1__1
{
pushFollow(FollowSets002.FOLLOW_rule__XFeatureCall__Group_3_1_1_1__0__Impl_in_rule__XFeatureCall__Group_3_1_1_1__067646);
rule__XFeatureCall__Group_3_1_1_1__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XFeatureCall__Group_3_1_1_1__1_in_rule__XFeatureCall__Group_3_1_1_1__067649);
rule__XFeatureCall__Group_3_1_1_1__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XFeatureCall__Group_3_1_1_1__0"
// $ANTLR start "rule__XFeatureCall__Group_3_1_1_1__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33640:1: rule__XFeatureCall__Group_3_1_1_1__0__Impl : ( ',' ) ;
public final void rule__XFeatureCall__Group_3_1_1_1__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33644:1: ( ( ',' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33645:1: ( ',' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33645:1: ( ',' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33646:1: ','
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXFeatureCallAccess().getCommaKeyword_3_1_1_1_0());
}
match(input,154,FollowSets002.FOLLOW_154_in_rule__XFeatureCall__Group_3_1_1_1__0__Impl67677); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXFeatureCallAccess().getCommaKeyword_3_1_1_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XFeatureCall__Group_3_1_1_1__0__Impl"
// $ANTLR start "rule__XFeatureCall__Group_3_1_1_1__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33659:1: rule__XFeatureCall__Group_3_1_1_1__1 : rule__XFeatureCall__Group_3_1_1_1__1__Impl ;
public final void rule__XFeatureCall__Group_3_1_1_1__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33663:1: ( rule__XFeatureCall__Group_3_1_1_1__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33664:2: rule__XFeatureCall__Group_3_1_1_1__1__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__XFeatureCall__Group_3_1_1_1__1__Impl_in_rule__XFeatureCall__Group_3_1_1_1__167708);
rule__XFeatureCall__Group_3_1_1_1__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XFeatureCall__Group_3_1_1_1__1"
// $ANTLR start "rule__XFeatureCall__Group_3_1_1_1__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33670:1: rule__XFeatureCall__Group_3_1_1_1__1__Impl : ( ( rule__XFeatureCall__FeatureCallArgumentsAssignment_3_1_1_1_1 ) ) ;
public final void rule__XFeatureCall__Group_3_1_1_1__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33674:1: ( ( ( rule__XFeatureCall__FeatureCallArgumentsAssignment_3_1_1_1_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33675:1: ( ( rule__XFeatureCall__FeatureCallArgumentsAssignment_3_1_1_1_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33675:1: ( ( rule__XFeatureCall__FeatureCallArgumentsAssignment_3_1_1_1_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33676:1: ( rule__XFeatureCall__FeatureCallArgumentsAssignment_3_1_1_1_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXFeatureCallAccess().getFeatureCallArgumentsAssignment_3_1_1_1_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33677:1: ( rule__XFeatureCall__FeatureCallArgumentsAssignment_3_1_1_1_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33677:2: rule__XFeatureCall__FeatureCallArgumentsAssignment_3_1_1_1_1
{
pushFollow(FollowSets002.FOLLOW_rule__XFeatureCall__FeatureCallArgumentsAssignment_3_1_1_1_1_in_rule__XFeatureCall__Group_3_1_1_1__1__Impl67735);
rule__XFeatureCall__FeatureCallArgumentsAssignment_3_1_1_1_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXFeatureCallAccess().getFeatureCallArgumentsAssignment_3_1_1_1_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XFeatureCall__Group_3_1_1_1__1__Impl"
// $ANTLR start "rule__XConstructorCall__Group__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33691:1: rule__XConstructorCall__Group__0 : rule__XConstructorCall__Group__0__Impl rule__XConstructorCall__Group__1 ;
public final void rule__XConstructorCall__Group__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33695:1: ( rule__XConstructorCall__Group__0__Impl rule__XConstructorCall__Group__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33696:2: rule__XConstructorCall__Group__0__Impl rule__XConstructorCall__Group__1
{
pushFollow(FollowSets002.FOLLOW_rule__XConstructorCall__Group__0__Impl_in_rule__XConstructorCall__Group__067769);
rule__XConstructorCall__Group__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XConstructorCall__Group__1_in_rule__XConstructorCall__Group__067772);
rule__XConstructorCall__Group__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XConstructorCall__Group__0"
// $ANTLR start "rule__XConstructorCall__Group__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33703:1: rule__XConstructorCall__Group__0__Impl : ( () ) ;
public final void rule__XConstructorCall__Group__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33707:1: ( ( () ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33708:1: ( () )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33708:1: ( () )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33709:1: ()
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXConstructorCallAccess().getXConstructorCallAction_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33710:1: ()
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33712:1:
{
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXConstructorCallAccess().getXConstructorCallAction_0());
}
}
}
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XConstructorCall__Group__0__Impl"
// $ANTLR start "rule__XConstructorCall__Group__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33722:1: rule__XConstructorCall__Group__1 : rule__XConstructorCall__Group__1__Impl rule__XConstructorCall__Group__2 ;
public final void rule__XConstructorCall__Group__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33726:1: ( rule__XConstructorCall__Group__1__Impl rule__XConstructorCall__Group__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33727:2: rule__XConstructorCall__Group__1__Impl rule__XConstructorCall__Group__2
{
pushFollow(FollowSets002.FOLLOW_rule__XConstructorCall__Group__1__Impl_in_rule__XConstructorCall__Group__167830);
rule__XConstructorCall__Group__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XConstructorCall__Group__2_in_rule__XConstructorCall__Group__167833);
rule__XConstructorCall__Group__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XConstructorCall__Group__1"
// $ANTLR start "rule__XConstructorCall__Group__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33734:1: rule__XConstructorCall__Group__1__Impl : ( 'new' ) ;
public final void rule__XConstructorCall__Group__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33738:1: ( ( 'new' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33739:1: ( 'new' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33739:1: ( 'new' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33740:1: 'new'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXConstructorCallAccess().getNewKeyword_1());
}
match(input,168,FollowSets002.FOLLOW_168_in_rule__XConstructorCall__Group__1__Impl67861); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXConstructorCallAccess().getNewKeyword_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XConstructorCall__Group__1__Impl"
// $ANTLR start "rule__XConstructorCall__Group__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33753:1: rule__XConstructorCall__Group__2 : rule__XConstructorCall__Group__2__Impl rule__XConstructorCall__Group__3 ;
public final void rule__XConstructorCall__Group__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33757:1: ( rule__XConstructorCall__Group__2__Impl rule__XConstructorCall__Group__3 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33758:2: rule__XConstructorCall__Group__2__Impl rule__XConstructorCall__Group__3
{
pushFollow(FollowSets002.FOLLOW_rule__XConstructorCall__Group__2__Impl_in_rule__XConstructorCall__Group__267892);
rule__XConstructorCall__Group__2__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XConstructorCall__Group__3_in_rule__XConstructorCall__Group__267895);
rule__XConstructorCall__Group__3();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XConstructorCall__Group__2"
// $ANTLR start "rule__XConstructorCall__Group__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33765:1: rule__XConstructorCall__Group__2__Impl : ( ( rule__XConstructorCall__ConstructorAssignment_2 ) ) ;
public final void rule__XConstructorCall__Group__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33769:1: ( ( ( rule__XConstructorCall__ConstructorAssignment_2 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33770:1: ( ( rule__XConstructorCall__ConstructorAssignment_2 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33770:1: ( ( rule__XConstructorCall__ConstructorAssignment_2 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33771:1: ( rule__XConstructorCall__ConstructorAssignment_2 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXConstructorCallAccess().getConstructorAssignment_2());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33772:1: ( rule__XConstructorCall__ConstructorAssignment_2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33772:2: rule__XConstructorCall__ConstructorAssignment_2
{
pushFollow(FollowSets002.FOLLOW_rule__XConstructorCall__ConstructorAssignment_2_in_rule__XConstructorCall__Group__2__Impl67922);
rule__XConstructorCall__ConstructorAssignment_2();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXConstructorCallAccess().getConstructorAssignment_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XConstructorCall__Group__2__Impl"
// $ANTLR start "rule__XConstructorCall__Group__3"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33782:1: rule__XConstructorCall__Group__3 : rule__XConstructorCall__Group__3__Impl rule__XConstructorCall__Group__4 ;
public final void rule__XConstructorCall__Group__3() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33786:1: ( rule__XConstructorCall__Group__3__Impl rule__XConstructorCall__Group__4 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33787:2: rule__XConstructorCall__Group__3__Impl rule__XConstructorCall__Group__4
{
pushFollow(FollowSets002.FOLLOW_rule__XConstructorCall__Group__3__Impl_in_rule__XConstructorCall__Group__367952);
rule__XConstructorCall__Group__3__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XConstructorCall__Group__4_in_rule__XConstructorCall__Group__367955);
rule__XConstructorCall__Group__4();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XConstructorCall__Group__3"
// $ANTLR start "rule__XConstructorCall__Group__3__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33794:1: rule__XConstructorCall__Group__3__Impl : ( ( rule__XConstructorCall__Group_3__0 )? ) ;
public final void rule__XConstructorCall__Group__3__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33798:1: ( ( ( rule__XConstructorCall__Group_3__0 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33799:1: ( ( rule__XConstructorCall__Group_3__0 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33799:1: ( ( rule__XConstructorCall__Group_3__0 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33800:1: ( rule__XConstructorCall__Group_3__0 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXConstructorCallAccess().getGroup_3());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33801:1: ( rule__XConstructorCall__Group_3__0 )?
int alt246=2;
alt246 = dfa246.predict(input);
switch (alt246) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33801:2: rule__XConstructorCall__Group_3__0
{
pushFollow(FollowSets002.FOLLOW_rule__XConstructorCall__Group_3__0_in_rule__XConstructorCall__Group__3__Impl67982);
rule__XConstructorCall__Group_3__0();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXConstructorCallAccess().getGroup_3());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XConstructorCall__Group__3__Impl"
// $ANTLR start "rule__XConstructorCall__Group__4"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33811:1: rule__XConstructorCall__Group__4 : rule__XConstructorCall__Group__4__Impl rule__XConstructorCall__Group__5 ;
public final void rule__XConstructorCall__Group__4() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33815:1: ( rule__XConstructorCall__Group__4__Impl rule__XConstructorCall__Group__5 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33816:2: rule__XConstructorCall__Group__4__Impl rule__XConstructorCall__Group__5
{
pushFollow(FollowSets002.FOLLOW_rule__XConstructorCall__Group__4__Impl_in_rule__XConstructorCall__Group__468013);
rule__XConstructorCall__Group__4__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XConstructorCall__Group__5_in_rule__XConstructorCall__Group__468016);
rule__XConstructorCall__Group__5();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XConstructorCall__Group__4"
// $ANTLR start "rule__XConstructorCall__Group__4__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33823:1: rule__XConstructorCall__Group__4__Impl : ( ( rule__XConstructorCall__Group_4__0 )? ) ;
public final void rule__XConstructorCall__Group__4__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33827:1: ( ( ( rule__XConstructorCall__Group_4__0 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33828:1: ( ( rule__XConstructorCall__Group_4__0 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33828:1: ( ( rule__XConstructorCall__Group_4__0 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33829:1: ( rule__XConstructorCall__Group_4__0 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXConstructorCallAccess().getGroup_4());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33830:1: ( rule__XConstructorCall__Group_4__0 )?
int alt247=2;
alt247 = dfa247.predict(input);
switch (alt247) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33830:2: rule__XConstructorCall__Group_4__0
{
pushFollow(FollowSets002.FOLLOW_rule__XConstructorCall__Group_4__0_in_rule__XConstructorCall__Group__4__Impl68043);
rule__XConstructorCall__Group_4__0();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXConstructorCallAccess().getGroup_4());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XConstructorCall__Group__4__Impl"
// $ANTLR start "rule__XConstructorCall__Group__5"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33840:1: rule__XConstructorCall__Group__5 : rule__XConstructorCall__Group__5__Impl ;
public final void rule__XConstructorCall__Group__5() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33844:1: ( rule__XConstructorCall__Group__5__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33845:2: rule__XConstructorCall__Group__5__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__XConstructorCall__Group__5__Impl_in_rule__XConstructorCall__Group__568074);
rule__XConstructorCall__Group__5__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XConstructorCall__Group__5"
// $ANTLR start "rule__XConstructorCall__Group__5__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33851:1: rule__XConstructorCall__Group__5__Impl : ( ( rule__XConstructorCall__ArgumentsAssignment_5 )? ) ;
public final void rule__XConstructorCall__Group__5__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33855:1: ( ( ( rule__XConstructorCall__ArgumentsAssignment_5 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33856:1: ( ( rule__XConstructorCall__ArgumentsAssignment_5 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33856:1: ( ( rule__XConstructorCall__ArgumentsAssignment_5 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33857:1: ( rule__XConstructorCall__ArgumentsAssignment_5 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXConstructorCallAccess().getArgumentsAssignment_5());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33858:1: ( rule__XConstructorCall__ArgumentsAssignment_5 )?
int alt248=2;
alt248 = dfa248.predict(input);
switch (alt248) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33858:2: rule__XConstructorCall__ArgumentsAssignment_5
{
pushFollow(FollowSets002.FOLLOW_rule__XConstructorCall__ArgumentsAssignment_5_in_rule__XConstructorCall__Group__5__Impl68101);
rule__XConstructorCall__ArgumentsAssignment_5();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXConstructorCallAccess().getArgumentsAssignment_5());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XConstructorCall__Group__5__Impl"
// $ANTLR start "rule__XConstructorCall__Group_3__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33880:1: rule__XConstructorCall__Group_3__0 : rule__XConstructorCall__Group_3__0__Impl rule__XConstructorCall__Group_3__1 ;
public final void rule__XConstructorCall__Group_3__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33884:1: ( rule__XConstructorCall__Group_3__0__Impl rule__XConstructorCall__Group_3__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33885:2: rule__XConstructorCall__Group_3__0__Impl rule__XConstructorCall__Group_3__1
{
pushFollow(FollowSets002.FOLLOW_rule__XConstructorCall__Group_3__0__Impl_in_rule__XConstructorCall__Group_3__068144);
rule__XConstructorCall__Group_3__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XConstructorCall__Group_3__1_in_rule__XConstructorCall__Group_3__068147);
rule__XConstructorCall__Group_3__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XConstructorCall__Group_3__0"
// $ANTLR start "rule__XConstructorCall__Group_3__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33892:1: rule__XConstructorCall__Group_3__0__Impl : ( ( '<' ) ) ;
public final void rule__XConstructorCall__Group_3__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33896:1: ( ( ( '<' ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33897:1: ( ( '<' ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33897:1: ( ( '<' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33898:1: ( '<' )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXConstructorCallAccess().getLessThanSignKeyword_3_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33899:1: ( '<' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33900:2: '<'
{
match(input,27,FollowSets002.FOLLOW_27_in_rule__XConstructorCall__Group_3__0__Impl68176); if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXConstructorCallAccess().getLessThanSignKeyword_3_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XConstructorCall__Group_3__0__Impl"
// $ANTLR start "rule__XConstructorCall__Group_3__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33911:1: rule__XConstructorCall__Group_3__1 : rule__XConstructorCall__Group_3__1__Impl rule__XConstructorCall__Group_3__2 ;
public final void rule__XConstructorCall__Group_3__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33915:1: ( rule__XConstructorCall__Group_3__1__Impl rule__XConstructorCall__Group_3__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33916:2: rule__XConstructorCall__Group_3__1__Impl rule__XConstructorCall__Group_3__2
{
pushFollow(FollowSets002.FOLLOW_rule__XConstructorCall__Group_3__1__Impl_in_rule__XConstructorCall__Group_3__168208);
rule__XConstructorCall__Group_3__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XConstructorCall__Group_3__2_in_rule__XConstructorCall__Group_3__168211);
rule__XConstructorCall__Group_3__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XConstructorCall__Group_3__1"
// $ANTLR start "rule__XConstructorCall__Group_3__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33923:1: rule__XConstructorCall__Group_3__1__Impl : ( ( rule__XConstructorCall__TypeArgumentsAssignment_3_1 ) ) ;
public final void rule__XConstructorCall__Group_3__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33927:1: ( ( ( rule__XConstructorCall__TypeArgumentsAssignment_3_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33928:1: ( ( rule__XConstructorCall__TypeArgumentsAssignment_3_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33928:1: ( ( rule__XConstructorCall__TypeArgumentsAssignment_3_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33929:1: ( rule__XConstructorCall__TypeArgumentsAssignment_3_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXConstructorCallAccess().getTypeArgumentsAssignment_3_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33930:1: ( rule__XConstructorCall__TypeArgumentsAssignment_3_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33930:2: rule__XConstructorCall__TypeArgumentsAssignment_3_1
{
pushFollow(FollowSets002.FOLLOW_rule__XConstructorCall__TypeArgumentsAssignment_3_1_in_rule__XConstructorCall__Group_3__1__Impl68238);
rule__XConstructorCall__TypeArgumentsAssignment_3_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXConstructorCallAccess().getTypeArgumentsAssignment_3_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XConstructorCall__Group_3__1__Impl"
// $ANTLR start "rule__XConstructorCall__Group_3__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33940:1: rule__XConstructorCall__Group_3__2 : rule__XConstructorCall__Group_3__2__Impl rule__XConstructorCall__Group_3__3 ;
public final void rule__XConstructorCall__Group_3__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33944:1: ( rule__XConstructorCall__Group_3__2__Impl rule__XConstructorCall__Group_3__3 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33945:2: rule__XConstructorCall__Group_3__2__Impl rule__XConstructorCall__Group_3__3
{
pushFollow(FollowSets002.FOLLOW_rule__XConstructorCall__Group_3__2__Impl_in_rule__XConstructorCall__Group_3__268268);
rule__XConstructorCall__Group_3__2__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XConstructorCall__Group_3__3_in_rule__XConstructorCall__Group_3__268271);
rule__XConstructorCall__Group_3__3();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XConstructorCall__Group_3__2"
// $ANTLR start "rule__XConstructorCall__Group_3__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33952:1: rule__XConstructorCall__Group_3__2__Impl : ( ( rule__XConstructorCall__Group_3_2__0 )* ) ;
public final void rule__XConstructorCall__Group_3__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33956:1: ( ( ( rule__XConstructorCall__Group_3_2__0 )* ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33957:1: ( ( rule__XConstructorCall__Group_3_2__0 )* )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33957:1: ( ( rule__XConstructorCall__Group_3_2__0 )* )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33958:1: ( rule__XConstructorCall__Group_3_2__0 )*
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXConstructorCallAccess().getGroup_3_2());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33959:1: ( rule__XConstructorCall__Group_3_2__0 )*
loop249:
do {
int alt249=2;
int LA249_0 = input.LA(1);
if ( (LA249_0==154) ) {
alt249=1;
}
switch (alt249) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33959:2: rule__XConstructorCall__Group_3_2__0
{
pushFollow(FollowSets002.FOLLOW_rule__XConstructorCall__Group_3_2__0_in_rule__XConstructorCall__Group_3__2__Impl68298);
rule__XConstructorCall__Group_3_2__0();
state._fsp--;
if (state.failed) return ;
}
break;
default :
break loop249;
}
} while (true);
if ( state.backtracking==0 ) {
after(grammarAccess.getXConstructorCallAccess().getGroup_3_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XConstructorCall__Group_3__2__Impl"
// $ANTLR start "rule__XConstructorCall__Group_3__3"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33969:1: rule__XConstructorCall__Group_3__3 : rule__XConstructorCall__Group_3__3__Impl ;
public final void rule__XConstructorCall__Group_3__3() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33973:1: ( rule__XConstructorCall__Group_3__3__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33974:2: rule__XConstructorCall__Group_3__3__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__XConstructorCall__Group_3__3__Impl_in_rule__XConstructorCall__Group_3__368329);
rule__XConstructorCall__Group_3__3__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XConstructorCall__Group_3__3"
// $ANTLR start "rule__XConstructorCall__Group_3__3__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33980:1: rule__XConstructorCall__Group_3__3__Impl : ( '>' ) ;
public final void rule__XConstructorCall__Group_3__3__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33984:1: ( ( '>' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33985:1: ( '>' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33985:1: ( '>' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33986:1: '>'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXConstructorCallAccess().getGreaterThanSignKeyword_3_3());
}
match(input,26,FollowSets002.FOLLOW_26_in_rule__XConstructorCall__Group_3__3__Impl68357); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXConstructorCallAccess().getGreaterThanSignKeyword_3_3());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XConstructorCall__Group_3__3__Impl"
// $ANTLR start "rule__XConstructorCall__Group_3_2__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34007:1: rule__XConstructorCall__Group_3_2__0 : rule__XConstructorCall__Group_3_2__0__Impl rule__XConstructorCall__Group_3_2__1 ;
public final void rule__XConstructorCall__Group_3_2__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34011:1: ( rule__XConstructorCall__Group_3_2__0__Impl rule__XConstructorCall__Group_3_2__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34012:2: rule__XConstructorCall__Group_3_2__0__Impl rule__XConstructorCall__Group_3_2__1
{
pushFollow(FollowSets002.FOLLOW_rule__XConstructorCall__Group_3_2__0__Impl_in_rule__XConstructorCall__Group_3_2__068396);
rule__XConstructorCall__Group_3_2__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XConstructorCall__Group_3_2__1_in_rule__XConstructorCall__Group_3_2__068399);
rule__XConstructorCall__Group_3_2__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XConstructorCall__Group_3_2__0"
// $ANTLR start "rule__XConstructorCall__Group_3_2__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34019:1: rule__XConstructorCall__Group_3_2__0__Impl : ( ',' ) ;
public final void rule__XConstructorCall__Group_3_2__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34023:1: ( ( ',' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34024:1: ( ',' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34024:1: ( ',' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34025:1: ','
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXConstructorCallAccess().getCommaKeyword_3_2_0());
}
match(input,154,FollowSets002.FOLLOW_154_in_rule__XConstructorCall__Group_3_2__0__Impl68427); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXConstructorCallAccess().getCommaKeyword_3_2_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XConstructorCall__Group_3_2__0__Impl"
// $ANTLR start "rule__XConstructorCall__Group_3_2__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34038:1: rule__XConstructorCall__Group_3_2__1 : rule__XConstructorCall__Group_3_2__1__Impl ;
public final void rule__XConstructorCall__Group_3_2__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34042:1: ( rule__XConstructorCall__Group_3_2__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34043:2: rule__XConstructorCall__Group_3_2__1__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__XConstructorCall__Group_3_2__1__Impl_in_rule__XConstructorCall__Group_3_2__168458);
rule__XConstructorCall__Group_3_2__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XConstructorCall__Group_3_2__1"
// $ANTLR start "rule__XConstructorCall__Group_3_2__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34049:1: rule__XConstructorCall__Group_3_2__1__Impl : ( ( rule__XConstructorCall__TypeArgumentsAssignment_3_2_1 ) ) ;
public final void rule__XConstructorCall__Group_3_2__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34053:1: ( ( ( rule__XConstructorCall__TypeArgumentsAssignment_3_2_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34054:1: ( ( rule__XConstructorCall__TypeArgumentsAssignment_3_2_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34054:1: ( ( rule__XConstructorCall__TypeArgumentsAssignment_3_2_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34055:1: ( rule__XConstructorCall__TypeArgumentsAssignment_3_2_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXConstructorCallAccess().getTypeArgumentsAssignment_3_2_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34056:1: ( rule__XConstructorCall__TypeArgumentsAssignment_3_2_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34056:2: rule__XConstructorCall__TypeArgumentsAssignment_3_2_1
{
pushFollow(FollowSets002.FOLLOW_rule__XConstructorCall__TypeArgumentsAssignment_3_2_1_in_rule__XConstructorCall__Group_3_2__1__Impl68485);
rule__XConstructorCall__TypeArgumentsAssignment_3_2_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXConstructorCallAccess().getTypeArgumentsAssignment_3_2_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XConstructorCall__Group_3_2__1__Impl"
// $ANTLR start "rule__XConstructorCall__Group_4__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34070:1: rule__XConstructorCall__Group_4__0 : rule__XConstructorCall__Group_4__0__Impl rule__XConstructorCall__Group_4__1 ;
public final void rule__XConstructorCall__Group_4__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34074:1: ( rule__XConstructorCall__Group_4__0__Impl rule__XConstructorCall__Group_4__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34075:2: rule__XConstructorCall__Group_4__0__Impl rule__XConstructorCall__Group_4__1
{
pushFollow(FollowSets002.FOLLOW_rule__XConstructorCall__Group_4__0__Impl_in_rule__XConstructorCall__Group_4__068519);
rule__XConstructorCall__Group_4__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XConstructorCall__Group_4__1_in_rule__XConstructorCall__Group_4__068522);
rule__XConstructorCall__Group_4__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XConstructorCall__Group_4__0"
// $ANTLR start "rule__XConstructorCall__Group_4__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34082:1: rule__XConstructorCall__Group_4__0__Impl : ( ( rule__XConstructorCall__ExplicitConstructorCallAssignment_4_0 ) ) ;
public final void rule__XConstructorCall__Group_4__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34086:1: ( ( ( rule__XConstructorCall__ExplicitConstructorCallAssignment_4_0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34087:1: ( ( rule__XConstructorCall__ExplicitConstructorCallAssignment_4_0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34087:1: ( ( rule__XConstructorCall__ExplicitConstructorCallAssignment_4_0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34088:1: ( rule__XConstructorCall__ExplicitConstructorCallAssignment_4_0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXConstructorCallAccess().getExplicitConstructorCallAssignment_4_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34089:1: ( rule__XConstructorCall__ExplicitConstructorCallAssignment_4_0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34089:2: rule__XConstructorCall__ExplicitConstructorCallAssignment_4_0
{
pushFollow(FollowSets002.FOLLOW_rule__XConstructorCall__ExplicitConstructorCallAssignment_4_0_in_rule__XConstructorCall__Group_4__0__Impl68549);
rule__XConstructorCall__ExplicitConstructorCallAssignment_4_0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXConstructorCallAccess().getExplicitConstructorCallAssignment_4_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XConstructorCall__Group_4__0__Impl"
// $ANTLR start "rule__XConstructorCall__Group_4__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34099:1: rule__XConstructorCall__Group_4__1 : rule__XConstructorCall__Group_4__1__Impl rule__XConstructorCall__Group_4__2 ;
public final void rule__XConstructorCall__Group_4__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34103:1: ( rule__XConstructorCall__Group_4__1__Impl rule__XConstructorCall__Group_4__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34104:2: rule__XConstructorCall__Group_4__1__Impl rule__XConstructorCall__Group_4__2
{
pushFollow(FollowSets002.FOLLOW_rule__XConstructorCall__Group_4__1__Impl_in_rule__XConstructorCall__Group_4__168579);
rule__XConstructorCall__Group_4__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XConstructorCall__Group_4__2_in_rule__XConstructorCall__Group_4__168582);
rule__XConstructorCall__Group_4__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XConstructorCall__Group_4__1"
// $ANTLR start "rule__XConstructorCall__Group_4__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34111:1: rule__XConstructorCall__Group_4__1__Impl : ( ( rule__XConstructorCall__Alternatives_4_1 )? ) ;
public final void rule__XConstructorCall__Group_4__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34115:1: ( ( ( rule__XConstructorCall__Alternatives_4_1 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34116:1: ( ( rule__XConstructorCall__Alternatives_4_1 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34116:1: ( ( rule__XConstructorCall__Alternatives_4_1 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34117:1: ( rule__XConstructorCall__Alternatives_4_1 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXConstructorCallAccess().getAlternatives_4_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34118:1: ( rule__XConstructorCall__Alternatives_4_1 )?
int alt250=2;
int LA250_0 = input.LA(1);
if ( ((LA250_0>=RULE_ID && LA250_0<=RULE_STRING)||LA250_0==27||LA250_0==31||(LA250_0>=34 && LA250_0<=35)||LA250_0==40||(LA250_0>=45 && LA250_0<=50)||LA250_0==67||LA250_0==144||(LA250_0>=155 && LA250_0<=156)||LA250_0==159||LA250_0==161||(LA250_0>=165 && LA250_0<=173)||LA250_0==175||LA250_0==183||LA250_0==185) ) {
alt250=1;
}
switch (alt250) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34118:2: rule__XConstructorCall__Alternatives_4_1
{
pushFollow(FollowSets002.FOLLOW_rule__XConstructorCall__Alternatives_4_1_in_rule__XConstructorCall__Group_4__1__Impl68609);
rule__XConstructorCall__Alternatives_4_1();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXConstructorCallAccess().getAlternatives_4_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XConstructorCall__Group_4__1__Impl"
// $ANTLR start "rule__XConstructorCall__Group_4__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34128:1: rule__XConstructorCall__Group_4__2 : rule__XConstructorCall__Group_4__2__Impl ;
public final void rule__XConstructorCall__Group_4__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34132:1: ( rule__XConstructorCall__Group_4__2__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34133:2: rule__XConstructorCall__Group_4__2__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__XConstructorCall__Group_4__2__Impl_in_rule__XConstructorCall__Group_4__268640);
rule__XConstructorCall__Group_4__2__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XConstructorCall__Group_4__2"
// $ANTLR start "rule__XConstructorCall__Group_4__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34139:1: rule__XConstructorCall__Group_4__2__Impl : ( ')' ) ;
public final void rule__XConstructorCall__Group_4__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34143:1: ( ( ')' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34144:1: ( ')' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34144:1: ( ')' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34145:1: ')'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXConstructorCallAccess().getRightParenthesisKeyword_4_2());
}
match(input,145,FollowSets002.FOLLOW_145_in_rule__XConstructorCall__Group_4__2__Impl68668); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXConstructorCallAccess().getRightParenthesisKeyword_4_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XConstructorCall__Group_4__2__Impl"
// $ANTLR start "rule__XConstructorCall__Group_4_1_1__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34164:1: rule__XConstructorCall__Group_4_1_1__0 : rule__XConstructorCall__Group_4_1_1__0__Impl rule__XConstructorCall__Group_4_1_1__1 ;
public final void rule__XConstructorCall__Group_4_1_1__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34168:1: ( rule__XConstructorCall__Group_4_1_1__0__Impl rule__XConstructorCall__Group_4_1_1__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34169:2: rule__XConstructorCall__Group_4_1_1__0__Impl rule__XConstructorCall__Group_4_1_1__1
{
pushFollow(FollowSets002.FOLLOW_rule__XConstructorCall__Group_4_1_1__0__Impl_in_rule__XConstructorCall__Group_4_1_1__068705);
rule__XConstructorCall__Group_4_1_1__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XConstructorCall__Group_4_1_1__1_in_rule__XConstructorCall__Group_4_1_1__068708);
rule__XConstructorCall__Group_4_1_1__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XConstructorCall__Group_4_1_1__0"
// $ANTLR start "rule__XConstructorCall__Group_4_1_1__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34176:1: rule__XConstructorCall__Group_4_1_1__0__Impl : ( ( rule__XConstructorCall__ArgumentsAssignment_4_1_1_0 ) ) ;
public final void rule__XConstructorCall__Group_4_1_1__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34180:1: ( ( ( rule__XConstructorCall__ArgumentsAssignment_4_1_1_0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34181:1: ( ( rule__XConstructorCall__ArgumentsAssignment_4_1_1_0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34181:1: ( ( rule__XConstructorCall__ArgumentsAssignment_4_1_1_0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34182:1: ( rule__XConstructorCall__ArgumentsAssignment_4_1_1_0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXConstructorCallAccess().getArgumentsAssignment_4_1_1_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34183:1: ( rule__XConstructorCall__ArgumentsAssignment_4_1_1_0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34183:2: rule__XConstructorCall__ArgumentsAssignment_4_1_1_0
{
pushFollow(FollowSets002.FOLLOW_rule__XConstructorCall__ArgumentsAssignment_4_1_1_0_in_rule__XConstructorCall__Group_4_1_1__0__Impl68735);
rule__XConstructorCall__ArgumentsAssignment_4_1_1_0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXConstructorCallAccess().getArgumentsAssignment_4_1_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XConstructorCall__Group_4_1_1__0__Impl"
// $ANTLR start "rule__XConstructorCall__Group_4_1_1__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34193:1: rule__XConstructorCall__Group_4_1_1__1 : rule__XConstructorCall__Group_4_1_1__1__Impl ;
public final void rule__XConstructorCall__Group_4_1_1__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34197:1: ( rule__XConstructorCall__Group_4_1_1__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34198:2: rule__XConstructorCall__Group_4_1_1__1__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__XConstructorCall__Group_4_1_1__1__Impl_in_rule__XConstructorCall__Group_4_1_1__168765);
rule__XConstructorCall__Group_4_1_1__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XConstructorCall__Group_4_1_1__1"
// $ANTLR start "rule__XConstructorCall__Group_4_1_1__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34204:1: rule__XConstructorCall__Group_4_1_1__1__Impl : ( ( rule__XConstructorCall__Group_4_1_1_1__0 )* ) ;
public final void rule__XConstructorCall__Group_4_1_1__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34208:1: ( ( ( rule__XConstructorCall__Group_4_1_1_1__0 )* ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34209:1: ( ( rule__XConstructorCall__Group_4_1_1_1__0 )* )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34209:1: ( ( rule__XConstructorCall__Group_4_1_1_1__0 )* )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34210:1: ( rule__XConstructorCall__Group_4_1_1_1__0 )*
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXConstructorCallAccess().getGroup_4_1_1_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34211:1: ( rule__XConstructorCall__Group_4_1_1_1__0 )*
loop251:
do {
int alt251=2;
int LA251_0 = input.LA(1);
if ( (LA251_0==154) ) {
alt251=1;
}
switch (alt251) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34211:2: rule__XConstructorCall__Group_4_1_1_1__0
{
pushFollow(FollowSets002.FOLLOW_rule__XConstructorCall__Group_4_1_1_1__0_in_rule__XConstructorCall__Group_4_1_1__1__Impl68792);
rule__XConstructorCall__Group_4_1_1_1__0();
state._fsp--;
if (state.failed) return ;
}
break;
default :
break loop251;
}
} while (true);
if ( state.backtracking==0 ) {
after(grammarAccess.getXConstructorCallAccess().getGroup_4_1_1_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XConstructorCall__Group_4_1_1__1__Impl"
// $ANTLR start "rule__XConstructorCall__Group_4_1_1_1__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34225:1: rule__XConstructorCall__Group_4_1_1_1__0 : rule__XConstructorCall__Group_4_1_1_1__0__Impl rule__XConstructorCall__Group_4_1_1_1__1 ;
public final void rule__XConstructorCall__Group_4_1_1_1__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34229:1: ( rule__XConstructorCall__Group_4_1_1_1__0__Impl rule__XConstructorCall__Group_4_1_1_1__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34230:2: rule__XConstructorCall__Group_4_1_1_1__0__Impl rule__XConstructorCall__Group_4_1_1_1__1
{
pushFollow(FollowSets002.FOLLOW_rule__XConstructorCall__Group_4_1_1_1__0__Impl_in_rule__XConstructorCall__Group_4_1_1_1__068827);
rule__XConstructorCall__Group_4_1_1_1__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XConstructorCall__Group_4_1_1_1__1_in_rule__XConstructorCall__Group_4_1_1_1__068830);
rule__XConstructorCall__Group_4_1_1_1__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XConstructorCall__Group_4_1_1_1__0"
// $ANTLR start "rule__XConstructorCall__Group_4_1_1_1__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34237:1: rule__XConstructorCall__Group_4_1_1_1__0__Impl : ( ',' ) ;
public final void rule__XConstructorCall__Group_4_1_1_1__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34241:1: ( ( ',' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34242:1: ( ',' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34242:1: ( ',' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34243:1: ','
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXConstructorCallAccess().getCommaKeyword_4_1_1_1_0());
}
match(input,154,FollowSets002.FOLLOW_154_in_rule__XConstructorCall__Group_4_1_1_1__0__Impl68858); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXConstructorCallAccess().getCommaKeyword_4_1_1_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XConstructorCall__Group_4_1_1_1__0__Impl"
// $ANTLR start "rule__XConstructorCall__Group_4_1_1_1__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34256:1: rule__XConstructorCall__Group_4_1_1_1__1 : rule__XConstructorCall__Group_4_1_1_1__1__Impl ;
public final void rule__XConstructorCall__Group_4_1_1_1__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34260:1: ( rule__XConstructorCall__Group_4_1_1_1__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34261:2: rule__XConstructorCall__Group_4_1_1_1__1__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__XConstructorCall__Group_4_1_1_1__1__Impl_in_rule__XConstructorCall__Group_4_1_1_1__168889);
rule__XConstructorCall__Group_4_1_1_1__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XConstructorCall__Group_4_1_1_1__1"
// $ANTLR start "rule__XConstructorCall__Group_4_1_1_1__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34267:1: rule__XConstructorCall__Group_4_1_1_1__1__Impl : ( ( rule__XConstructorCall__ArgumentsAssignment_4_1_1_1_1 ) ) ;
public final void rule__XConstructorCall__Group_4_1_1_1__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34271:1: ( ( ( rule__XConstructorCall__ArgumentsAssignment_4_1_1_1_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34272:1: ( ( rule__XConstructorCall__ArgumentsAssignment_4_1_1_1_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34272:1: ( ( rule__XConstructorCall__ArgumentsAssignment_4_1_1_1_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34273:1: ( rule__XConstructorCall__ArgumentsAssignment_4_1_1_1_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXConstructorCallAccess().getArgumentsAssignment_4_1_1_1_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34274:1: ( rule__XConstructorCall__ArgumentsAssignment_4_1_1_1_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34274:2: rule__XConstructorCall__ArgumentsAssignment_4_1_1_1_1
{
pushFollow(FollowSets002.FOLLOW_rule__XConstructorCall__ArgumentsAssignment_4_1_1_1_1_in_rule__XConstructorCall__Group_4_1_1_1__1__Impl68916);
rule__XConstructorCall__ArgumentsAssignment_4_1_1_1_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXConstructorCallAccess().getArgumentsAssignment_4_1_1_1_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XConstructorCall__Group_4_1_1_1__1__Impl"
// $ANTLR start "rule__XBooleanLiteral__Group__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34288:1: rule__XBooleanLiteral__Group__0 : rule__XBooleanLiteral__Group__0__Impl rule__XBooleanLiteral__Group__1 ;
public final void rule__XBooleanLiteral__Group__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34292:1: ( rule__XBooleanLiteral__Group__0__Impl rule__XBooleanLiteral__Group__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34293:2: rule__XBooleanLiteral__Group__0__Impl rule__XBooleanLiteral__Group__1
{
pushFollow(FollowSets002.FOLLOW_rule__XBooleanLiteral__Group__0__Impl_in_rule__XBooleanLiteral__Group__068950);
rule__XBooleanLiteral__Group__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XBooleanLiteral__Group__1_in_rule__XBooleanLiteral__Group__068953);
rule__XBooleanLiteral__Group__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XBooleanLiteral__Group__0"
// $ANTLR start "rule__XBooleanLiteral__Group__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34300:1: rule__XBooleanLiteral__Group__0__Impl : ( () ) ;
public final void rule__XBooleanLiteral__Group__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34304:1: ( ( () ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34305:1: ( () )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34305:1: ( () )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34306:1: ()
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXBooleanLiteralAccess().getXBooleanLiteralAction_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34307:1: ()
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34309:1:
{
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXBooleanLiteralAccess().getXBooleanLiteralAction_0());
}
}
}
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XBooleanLiteral__Group__0__Impl"
// $ANTLR start "rule__XBooleanLiteral__Group__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34319:1: rule__XBooleanLiteral__Group__1 : rule__XBooleanLiteral__Group__1__Impl ;
public final void rule__XBooleanLiteral__Group__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34323:1: ( rule__XBooleanLiteral__Group__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34324:2: rule__XBooleanLiteral__Group__1__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__XBooleanLiteral__Group__1__Impl_in_rule__XBooleanLiteral__Group__169011);
rule__XBooleanLiteral__Group__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XBooleanLiteral__Group__1"
// $ANTLR start "rule__XBooleanLiteral__Group__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34330:1: rule__XBooleanLiteral__Group__1__Impl : ( ( rule__XBooleanLiteral__Alternatives_1 ) ) ;
public final void rule__XBooleanLiteral__Group__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34334:1: ( ( ( rule__XBooleanLiteral__Alternatives_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34335:1: ( ( rule__XBooleanLiteral__Alternatives_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34335:1: ( ( rule__XBooleanLiteral__Alternatives_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34336:1: ( rule__XBooleanLiteral__Alternatives_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXBooleanLiteralAccess().getAlternatives_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34337:1: ( rule__XBooleanLiteral__Alternatives_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34337:2: rule__XBooleanLiteral__Alternatives_1
{
pushFollow(FollowSets002.FOLLOW_rule__XBooleanLiteral__Alternatives_1_in_rule__XBooleanLiteral__Group__1__Impl69038);
rule__XBooleanLiteral__Alternatives_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXBooleanLiteralAccess().getAlternatives_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XBooleanLiteral__Group__1__Impl"
// $ANTLR start "rule__XNullLiteral__Group__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34351:1: rule__XNullLiteral__Group__0 : rule__XNullLiteral__Group__0__Impl rule__XNullLiteral__Group__1 ;
public final void rule__XNullLiteral__Group__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34355:1: ( rule__XNullLiteral__Group__0__Impl rule__XNullLiteral__Group__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34356:2: rule__XNullLiteral__Group__0__Impl rule__XNullLiteral__Group__1
{
pushFollow(FollowSets002.FOLLOW_rule__XNullLiteral__Group__0__Impl_in_rule__XNullLiteral__Group__069072);
rule__XNullLiteral__Group__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XNullLiteral__Group__1_in_rule__XNullLiteral__Group__069075);
rule__XNullLiteral__Group__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XNullLiteral__Group__0"
// $ANTLR start "rule__XNullLiteral__Group__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34363:1: rule__XNullLiteral__Group__0__Impl : ( () ) ;
public final void rule__XNullLiteral__Group__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34367:1: ( ( () ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34368:1: ( () )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34368:1: ( () )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34369:1: ()
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXNullLiteralAccess().getXNullLiteralAction_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34370:1: ()
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34372:1:
{
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXNullLiteralAccess().getXNullLiteralAction_0());
}
}
}
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XNullLiteral__Group__0__Impl"
// $ANTLR start "rule__XNullLiteral__Group__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34382:1: rule__XNullLiteral__Group__1 : rule__XNullLiteral__Group__1__Impl ;
public final void rule__XNullLiteral__Group__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34386:1: ( rule__XNullLiteral__Group__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34387:2: rule__XNullLiteral__Group__1__Impl
{
pushFollow(FollowSets002.FOLLOW_rule__XNullLiteral__Group__1__Impl_in_rule__XNullLiteral__Group__169133);
rule__XNullLiteral__Group__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XNullLiteral__Group__1"
// $ANTLR start "rule__XNullLiteral__Group__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34393:1: rule__XNullLiteral__Group__1__Impl : ( 'null' ) ;
public final void rule__XNullLiteral__Group__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34397:1: ( ( 'null' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34398:1: ( 'null' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34398:1: ( 'null' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34399:1: 'null'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXNullLiteralAccess().getNullKeyword_1());
}
match(input,169,FollowSets002.FOLLOW_169_in_rule__XNullLiteral__Group__1__Impl69161); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXNullLiteralAccess().getNullKeyword_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XNullLiteral__Group__1__Impl"
// $ANTLR start "rule__XNumberLiteral__Group__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34416:1: rule__XNumberLiteral__Group__0 : rule__XNumberLiteral__Group__0__Impl rule__XNumberLiteral__Group__1 ;
public final void rule__XNumberLiteral__Group__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34420:1: ( rule__XNumberLiteral__Group__0__Impl rule__XNumberLiteral__Group__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34421:2: rule__XNumberLiteral__Group__0__Impl rule__XNumberLiteral__Group__1
{
pushFollow(FollowSets002.FOLLOW_rule__XNumberLiteral__Group__0__Impl_in_rule__XNumberLiteral__Group__069196);
rule__XNumberLiteral__Group__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets002.FOLLOW_rule__XNumberLiteral__Group__1_in_rule__XNumberLiteral__Group__069199);
rule__XNumberLiteral__Group__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XNumberLiteral__Group__0"
// $ANTLR start "rule__XNumberLiteral__Group__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34428:1: rule__XNumberLiteral__Group__0__Impl : ( () ) ;
public final void rule__XNumberLiteral__Group__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34432:1: ( ( () ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34433:1: ( () )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34433:1: ( () )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34434:1: ()
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXNumberLiteralAccess().getXNumberLiteralAction_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34435:1: ()
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34437:1:
{
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXNumberLiteralAccess().getXNumberLiteralAction_0());
}
}
}
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XNumberLiteral__Group__0__Impl"
// $ANTLR start "rule__XNumberLiteral__Group__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34447:1: rule__XNumberLiteral__Group__1 : rule__XNumberLiteral__Group__1__Impl ;
public final void rule__XNumberLiteral__Group__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34451:1: ( rule__XNumberLiteral__Group__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34452:2: rule__XNumberLiteral__Group__1__Impl
{
pushFollow(FollowSets003.FOLLOW_rule__XNumberLiteral__Group__1__Impl_in_rule__XNumberLiteral__Group__169257);
rule__XNumberLiteral__Group__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XNumberLiteral__Group__1"
// $ANTLR start "rule__XNumberLiteral__Group__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34458:1: rule__XNumberLiteral__Group__1__Impl : ( ( rule__XNumberLiteral__ValueAssignment_1 ) ) ;
public final void rule__XNumberLiteral__Group__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34462:1: ( ( ( rule__XNumberLiteral__ValueAssignment_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34463:1: ( ( rule__XNumberLiteral__ValueAssignment_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34463:1: ( ( rule__XNumberLiteral__ValueAssignment_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34464:1: ( rule__XNumberLiteral__ValueAssignment_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXNumberLiteralAccess().getValueAssignment_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34465:1: ( rule__XNumberLiteral__ValueAssignment_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34465:2: rule__XNumberLiteral__ValueAssignment_1
{
pushFollow(FollowSets003.FOLLOW_rule__XNumberLiteral__ValueAssignment_1_in_rule__XNumberLiteral__Group__1__Impl69284);
rule__XNumberLiteral__ValueAssignment_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXNumberLiteralAccess().getValueAssignment_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XNumberLiteral__Group__1__Impl"
// $ANTLR start "rule__XStringLiteral__Group__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34479:1: rule__XStringLiteral__Group__0 : rule__XStringLiteral__Group__0__Impl rule__XStringLiteral__Group__1 ;
public final void rule__XStringLiteral__Group__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34483:1: ( rule__XStringLiteral__Group__0__Impl rule__XStringLiteral__Group__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34484:2: rule__XStringLiteral__Group__0__Impl rule__XStringLiteral__Group__1
{
pushFollow(FollowSets003.FOLLOW_rule__XStringLiteral__Group__0__Impl_in_rule__XStringLiteral__Group__069318);
rule__XStringLiteral__Group__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets003.FOLLOW_rule__XStringLiteral__Group__1_in_rule__XStringLiteral__Group__069321);
rule__XStringLiteral__Group__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XStringLiteral__Group__0"
// $ANTLR start "rule__XStringLiteral__Group__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34491:1: rule__XStringLiteral__Group__0__Impl : ( () ) ;
public final void rule__XStringLiteral__Group__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34495:1: ( ( () ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34496:1: ( () )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34496:1: ( () )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34497:1: ()
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXStringLiteralAccess().getXStringLiteralAction_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34498:1: ()
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34500:1:
{
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXStringLiteralAccess().getXStringLiteralAction_0());
}
}
}
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XStringLiteral__Group__0__Impl"
// $ANTLR start "rule__XStringLiteral__Group__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34510:1: rule__XStringLiteral__Group__1 : rule__XStringLiteral__Group__1__Impl ;
public final void rule__XStringLiteral__Group__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34514:1: ( rule__XStringLiteral__Group__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34515:2: rule__XStringLiteral__Group__1__Impl
{
pushFollow(FollowSets003.FOLLOW_rule__XStringLiteral__Group__1__Impl_in_rule__XStringLiteral__Group__169379);
rule__XStringLiteral__Group__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XStringLiteral__Group__1"
// $ANTLR start "rule__XStringLiteral__Group__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34521:1: rule__XStringLiteral__Group__1__Impl : ( ( rule__XStringLiteral__ValueAssignment_1 ) ) ;
public final void rule__XStringLiteral__Group__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34525:1: ( ( ( rule__XStringLiteral__ValueAssignment_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34526:1: ( ( rule__XStringLiteral__ValueAssignment_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34526:1: ( ( rule__XStringLiteral__ValueAssignment_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34527:1: ( rule__XStringLiteral__ValueAssignment_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXStringLiteralAccess().getValueAssignment_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34528:1: ( rule__XStringLiteral__ValueAssignment_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34528:2: rule__XStringLiteral__ValueAssignment_1
{
pushFollow(FollowSets003.FOLLOW_rule__XStringLiteral__ValueAssignment_1_in_rule__XStringLiteral__Group__1__Impl69406);
rule__XStringLiteral__ValueAssignment_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXStringLiteralAccess().getValueAssignment_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XStringLiteral__Group__1__Impl"
// $ANTLR start "rule__XTypeLiteral__Group__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34542:1: rule__XTypeLiteral__Group__0 : rule__XTypeLiteral__Group__0__Impl rule__XTypeLiteral__Group__1 ;
public final void rule__XTypeLiteral__Group__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34546:1: ( rule__XTypeLiteral__Group__0__Impl rule__XTypeLiteral__Group__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34547:2: rule__XTypeLiteral__Group__0__Impl rule__XTypeLiteral__Group__1
{
pushFollow(FollowSets003.FOLLOW_rule__XTypeLiteral__Group__0__Impl_in_rule__XTypeLiteral__Group__069440);
rule__XTypeLiteral__Group__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets003.FOLLOW_rule__XTypeLiteral__Group__1_in_rule__XTypeLiteral__Group__069443);
rule__XTypeLiteral__Group__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XTypeLiteral__Group__0"
// $ANTLR start "rule__XTypeLiteral__Group__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34554:1: rule__XTypeLiteral__Group__0__Impl : ( () ) ;
public final void rule__XTypeLiteral__Group__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34558:1: ( ( () ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34559:1: ( () )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34559:1: ( () )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34560:1: ()
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXTypeLiteralAccess().getXTypeLiteralAction_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34561:1: ()
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34563:1:
{
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXTypeLiteralAccess().getXTypeLiteralAction_0());
}
}
}
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XTypeLiteral__Group__0__Impl"
// $ANTLR start "rule__XTypeLiteral__Group__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34573:1: rule__XTypeLiteral__Group__1 : rule__XTypeLiteral__Group__1__Impl rule__XTypeLiteral__Group__2 ;
public final void rule__XTypeLiteral__Group__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34577:1: ( rule__XTypeLiteral__Group__1__Impl rule__XTypeLiteral__Group__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34578:2: rule__XTypeLiteral__Group__1__Impl rule__XTypeLiteral__Group__2
{
pushFollow(FollowSets003.FOLLOW_rule__XTypeLiteral__Group__1__Impl_in_rule__XTypeLiteral__Group__169501);
rule__XTypeLiteral__Group__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets003.FOLLOW_rule__XTypeLiteral__Group__2_in_rule__XTypeLiteral__Group__169504);
rule__XTypeLiteral__Group__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XTypeLiteral__Group__1"
// $ANTLR start "rule__XTypeLiteral__Group__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34585:1: rule__XTypeLiteral__Group__1__Impl : ( 'typeof' ) ;
public final void rule__XTypeLiteral__Group__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34589:1: ( ( 'typeof' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34590:1: ( 'typeof' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34590:1: ( 'typeof' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34591:1: 'typeof'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXTypeLiteralAccess().getTypeofKeyword_1());
}
match(input,170,FollowSets003.FOLLOW_170_in_rule__XTypeLiteral__Group__1__Impl69532); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXTypeLiteralAccess().getTypeofKeyword_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XTypeLiteral__Group__1__Impl"
// $ANTLR start "rule__XTypeLiteral__Group__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34604:1: rule__XTypeLiteral__Group__2 : rule__XTypeLiteral__Group__2__Impl rule__XTypeLiteral__Group__3 ;
public final void rule__XTypeLiteral__Group__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34608:1: ( rule__XTypeLiteral__Group__2__Impl rule__XTypeLiteral__Group__3 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34609:2: rule__XTypeLiteral__Group__2__Impl rule__XTypeLiteral__Group__3
{
pushFollow(FollowSets003.FOLLOW_rule__XTypeLiteral__Group__2__Impl_in_rule__XTypeLiteral__Group__269563);
rule__XTypeLiteral__Group__2__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets003.FOLLOW_rule__XTypeLiteral__Group__3_in_rule__XTypeLiteral__Group__269566);
rule__XTypeLiteral__Group__3();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XTypeLiteral__Group__2"
// $ANTLR start "rule__XTypeLiteral__Group__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34616:1: rule__XTypeLiteral__Group__2__Impl : ( '(' ) ;
public final void rule__XTypeLiteral__Group__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34620:1: ( ( '(' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34621:1: ( '(' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34621:1: ( '(' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34622:1: '('
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXTypeLiteralAccess().getLeftParenthesisKeyword_2());
}
match(input,144,FollowSets003.FOLLOW_144_in_rule__XTypeLiteral__Group__2__Impl69594); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXTypeLiteralAccess().getLeftParenthesisKeyword_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XTypeLiteral__Group__2__Impl"
// $ANTLR start "rule__XTypeLiteral__Group__3"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34635:1: rule__XTypeLiteral__Group__3 : rule__XTypeLiteral__Group__3__Impl rule__XTypeLiteral__Group__4 ;
public final void rule__XTypeLiteral__Group__3() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34639:1: ( rule__XTypeLiteral__Group__3__Impl rule__XTypeLiteral__Group__4 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34640:2: rule__XTypeLiteral__Group__3__Impl rule__XTypeLiteral__Group__4
{
pushFollow(FollowSets003.FOLLOW_rule__XTypeLiteral__Group__3__Impl_in_rule__XTypeLiteral__Group__369625);
rule__XTypeLiteral__Group__3__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets003.FOLLOW_rule__XTypeLiteral__Group__4_in_rule__XTypeLiteral__Group__369628);
rule__XTypeLiteral__Group__4();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XTypeLiteral__Group__3"
// $ANTLR start "rule__XTypeLiteral__Group__3__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34647:1: rule__XTypeLiteral__Group__3__Impl : ( ( rule__XTypeLiteral__TypeAssignment_3 ) ) ;
public final void rule__XTypeLiteral__Group__3__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34651:1: ( ( ( rule__XTypeLiteral__TypeAssignment_3 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34652:1: ( ( rule__XTypeLiteral__TypeAssignment_3 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34652:1: ( ( rule__XTypeLiteral__TypeAssignment_3 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34653:1: ( rule__XTypeLiteral__TypeAssignment_3 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXTypeLiteralAccess().getTypeAssignment_3());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34654:1: ( rule__XTypeLiteral__TypeAssignment_3 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34654:2: rule__XTypeLiteral__TypeAssignment_3
{
pushFollow(FollowSets003.FOLLOW_rule__XTypeLiteral__TypeAssignment_3_in_rule__XTypeLiteral__Group__3__Impl69655);
rule__XTypeLiteral__TypeAssignment_3();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXTypeLiteralAccess().getTypeAssignment_3());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XTypeLiteral__Group__3__Impl"
// $ANTLR start "rule__XTypeLiteral__Group__4"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34664:1: rule__XTypeLiteral__Group__4 : rule__XTypeLiteral__Group__4__Impl rule__XTypeLiteral__Group__5 ;
public final void rule__XTypeLiteral__Group__4() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34668:1: ( rule__XTypeLiteral__Group__4__Impl rule__XTypeLiteral__Group__5 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34669:2: rule__XTypeLiteral__Group__4__Impl rule__XTypeLiteral__Group__5
{
pushFollow(FollowSets003.FOLLOW_rule__XTypeLiteral__Group__4__Impl_in_rule__XTypeLiteral__Group__469685);
rule__XTypeLiteral__Group__4__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets003.FOLLOW_rule__XTypeLiteral__Group__5_in_rule__XTypeLiteral__Group__469688);
rule__XTypeLiteral__Group__5();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XTypeLiteral__Group__4"
// $ANTLR start "rule__XTypeLiteral__Group__4__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34676:1: rule__XTypeLiteral__Group__4__Impl : ( ( rule__XTypeLiteral__ArrayDimensionsAssignment_4 )* ) ;
public final void rule__XTypeLiteral__Group__4__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34680:1: ( ( ( rule__XTypeLiteral__ArrayDimensionsAssignment_4 )* ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34681:1: ( ( rule__XTypeLiteral__ArrayDimensionsAssignment_4 )* )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34681:1: ( ( rule__XTypeLiteral__ArrayDimensionsAssignment_4 )* )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34682:1: ( rule__XTypeLiteral__ArrayDimensionsAssignment_4 )*
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXTypeLiteralAccess().getArrayDimensionsAssignment_4());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34683:1: ( rule__XTypeLiteral__ArrayDimensionsAssignment_4 )*
loop252:
do {
int alt252=2;
int LA252_0 = input.LA(1);
if ( (LA252_0==156) ) {
alt252=1;
}
switch (alt252) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34683:2: rule__XTypeLiteral__ArrayDimensionsAssignment_4
{
pushFollow(FollowSets003.FOLLOW_rule__XTypeLiteral__ArrayDimensionsAssignment_4_in_rule__XTypeLiteral__Group__4__Impl69715);
rule__XTypeLiteral__ArrayDimensionsAssignment_4();
state._fsp--;
if (state.failed) return ;
}
break;
default :
break loop252;
}
} while (true);
if ( state.backtracking==0 ) {
after(grammarAccess.getXTypeLiteralAccess().getArrayDimensionsAssignment_4());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XTypeLiteral__Group__4__Impl"
// $ANTLR start "rule__XTypeLiteral__Group__5"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34693:1: rule__XTypeLiteral__Group__5 : rule__XTypeLiteral__Group__5__Impl ;
public final void rule__XTypeLiteral__Group__5() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34697:1: ( rule__XTypeLiteral__Group__5__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34698:2: rule__XTypeLiteral__Group__5__Impl
{
pushFollow(FollowSets003.FOLLOW_rule__XTypeLiteral__Group__5__Impl_in_rule__XTypeLiteral__Group__569746);
rule__XTypeLiteral__Group__5__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XTypeLiteral__Group__5"
// $ANTLR start "rule__XTypeLiteral__Group__5__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34704:1: rule__XTypeLiteral__Group__5__Impl : ( ')' ) ;
public final void rule__XTypeLiteral__Group__5__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34708:1: ( ( ')' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34709:1: ( ')' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34709:1: ( ')' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34710:1: ')'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXTypeLiteralAccess().getRightParenthesisKeyword_5());
}
match(input,145,FollowSets003.FOLLOW_145_in_rule__XTypeLiteral__Group__5__Impl69774); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXTypeLiteralAccess().getRightParenthesisKeyword_5());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XTypeLiteral__Group__5__Impl"
// $ANTLR start "rule__XThrowExpression__Group__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34735:1: rule__XThrowExpression__Group__0 : rule__XThrowExpression__Group__0__Impl rule__XThrowExpression__Group__1 ;
public final void rule__XThrowExpression__Group__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34739:1: ( rule__XThrowExpression__Group__0__Impl rule__XThrowExpression__Group__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34740:2: rule__XThrowExpression__Group__0__Impl rule__XThrowExpression__Group__1
{
pushFollow(FollowSets003.FOLLOW_rule__XThrowExpression__Group__0__Impl_in_rule__XThrowExpression__Group__069817);
rule__XThrowExpression__Group__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets003.FOLLOW_rule__XThrowExpression__Group__1_in_rule__XThrowExpression__Group__069820);
rule__XThrowExpression__Group__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XThrowExpression__Group__0"
// $ANTLR start "rule__XThrowExpression__Group__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34747:1: rule__XThrowExpression__Group__0__Impl : ( () ) ;
public final void rule__XThrowExpression__Group__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34751:1: ( ( () ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34752:1: ( () )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34752:1: ( () )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34753:1: ()
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXThrowExpressionAccess().getXThrowExpressionAction_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34754:1: ()
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34756:1:
{
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXThrowExpressionAccess().getXThrowExpressionAction_0());
}
}
}
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XThrowExpression__Group__0__Impl"
// $ANTLR start "rule__XThrowExpression__Group__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34766:1: rule__XThrowExpression__Group__1 : rule__XThrowExpression__Group__1__Impl rule__XThrowExpression__Group__2 ;
public final void rule__XThrowExpression__Group__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34770:1: ( rule__XThrowExpression__Group__1__Impl rule__XThrowExpression__Group__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34771:2: rule__XThrowExpression__Group__1__Impl rule__XThrowExpression__Group__2
{
pushFollow(FollowSets003.FOLLOW_rule__XThrowExpression__Group__1__Impl_in_rule__XThrowExpression__Group__169878);
rule__XThrowExpression__Group__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets003.FOLLOW_rule__XThrowExpression__Group__2_in_rule__XThrowExpression__Group__169881);
rule__XThrowExpression__Group__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XThrowExpression__Group__1"
// $ANTLR start "rule__XThrowExpression__Group__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34778:1: rule__XThrowExpression__Group__1__Impl : ( 'throw' ) ;
public final void rule__XThrowExpression__Group__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34782:1: ( ( 'throw' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34783:1: ( 'throw' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34783:1: ( 'throw' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34784:1: 'throw'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXThrowExpressionAccess().getThrowKeyword_1());
}
match(input,171,FollowSets003.FOLLOW_171_in_rule__XThrowExpression__Group__1__Impl69909); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXThrowExpressionAccess().getThrowKeyword_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XThrowExpression__Group__1__Impl"
// $ANTLR start "rule__XThrowExpression__Group__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34797:1: rule__XThrowExpression__Group__2 : rule__XThrowExpression__Group__2__Impl ;
public final void rule__XThrowExpression__Group__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34801:1: ( rule__XThrowExpression__Group__2__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34802:2: rule__XThrowExpression__Group__2__Impl
{
pushFollow(FollowSets003.FOLLOW_rule__XThrowExpression__Group__2__Impl_in_rule__XThrowExpression__Group__269940);
rule__XThrowExpression__Group__2__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XThrowExpression__Group__2"
// $ANTLR start "rule__XThrowExpression__Group__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34808:1: rule__XThrowExpression__Group__2__Impl : ( ( rule__XThrowExpression__ExpressionAssignment_2 ) ) ;
public final void rule__XThrowExpression__Group__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34812:1: ( ( ( rule__XThrowExpression__ExpressionAssignment_2 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34813:1: ( ( rule__XThrowExpression__ExpressionAssignment_2 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34813:1: ( ( rule__XThrowExpression__ExpressionAssignment_2 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34814:1: ( rule__XThrowExpression__ExpressionAssignment_2 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXThrowExpressionAccess().getExpressionAssignment_2());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34815:1: ( rule__XThrowExpression__ExpressionAssignment_2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34815:2: rule__XThrowExpression__ExpressionAssignment_2
{
pushFollow(FollowSets003.FOLLOW_rule__XThrowExpression__ExpressionAssignment_2_in_rule__XThrowExpression__Group__2__Impl69967);
rule__XThrowExpression__ExpressionAssignment_2();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXThrowExpressionAccess().getExpressionAssignment_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XThrowExpression__Group__2__Impl"
// $ANTLR start "rule__XReturnExpression__Group__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34831:1: rule__XReturnExpression__Group__0 : rule__XReturnExpression__Group__0__Impl rule__XReturnExpression__Group__1 ;
public final void rule__XReturnExpression__Group__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34835:1: ( rule__XReturnExpression__Group__0__Impl rule__XReturnExpression__Group__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34836:2: rule__XReturnExpression__Group__0__Impl rule__XReturnExpression__Group__1
{
pushFollow(FollowSets003.FOLLOW_rule__XReturnExpression__Group__0__Impl_in_rule__XReturnExpression__Group__070003);
rule__XReturnExpression__Group__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets003.FOLLOW_rule__XReturnExpression__Group__1_in_rule__XReturnExpression__Group__070006);
rule__XReturnExpression__Group__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XReturnExpression__Group__0"
// $ANTLR start "rule__XReturnExpression__Group__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34843:1: rule__XReturnExpression__Group__0__Impl : ( () ) ;
public final void rule__XReturnExpression__Group__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34847:1: ( ( () ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34848:1: ( () )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34848:1: ( () )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34849:1: ()
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXReturnExpressionAccess().getXReturnExpressionAction_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34850:1: ()
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34852:1:
{
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXReturnExpressionAccess().getXReturnExpressionAction_0());
}
}
}
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XReturnExpression__Group__0__Impl"
// $ANTLR start "rule__XReturnExpression__Group__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34862:1: rule__XReturnExpression__Group__1 : rule__XReturnExpression__Group__1__Impl rule__XReturnExpression__Group__2 ;
public final void rule__XReturnExpression__Group__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34866:1: ( rule__XReturnExpression__Group__1__Impl rule__XReturnExpression__Group__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34867:2: rule__XReturnExpression__Group__1__Impl rule__XReturnExpression__Group__2
{
pushFollow(FollowSets003.FOLLOW_rule__XReturnExpression__Group__1__Impl_in_rule__XReturnExpression__Group__170064);
rule__XReturnExpression__Group__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets003.FOLLOW_rule__XReturnExpression__Group__2_in_rule__XReturnExpression__Group__170067);
rule__XReturnExpression__Group__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XReturnExpression__Group__1"
// $ANTLR start "rule__XReturnExpression__Group__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34874:1: rule__XReturnExpression__Group__1__Impl : ( 'return' ) ;
public final void rule__XReturnExpression__Group__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34878:1: ( ( 'return' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34879:1: ( 'return' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34879:1: ( 'return' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34880:1: 'return'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXReturnExpressionAccess().getReturnKeyword_1());
}
match(input,172,FollowSets003.FOLLOW_172_in_rule__XReturnExpression__Group__1__Impl70095); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXReturnExpressionAccess().getReturnKeyword_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XReturnExpression__Group__1__Impl"
// $ANTLR start "rule__XReturnExpression__Group__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34893:1: rule__XReturnExpression__Group__2 : rule__XReturnExpression__Group__2__Impl ;
public final void rule__XReturnExpression__Group__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34897:1: ( rule__XReturnExpression__Group__2__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34898:2: rule__XReturnExpression__Group__2__Impl
{
pushFollow(FollowSets003.FOLLOW_rule__XReturnExpression__Group__2__Impl_in_rule__XReturnExpression__Group__270126);
rule__XReturnExpression__Group__2__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XReturnExpression__Group__2"
// $ANTLR start "rule__XReturnExpression__Group__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34904:1: rule__XReturnExpression__Group__2__Impl : ( ( rule__XReturnExpression__ExpressionAssignment_2 )? ) ;
public final void rule__XReturnExpression__Group__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34908:1: ( ( ( rule__XReturnExpression__ExpressionAssignment_2 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34909:1: ( ( rule__XReturnExpression__ExpressionAssignment_2 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34909:1: ( ( rule__XReturnExpression__ExpressionAssignment_2 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34910:1: ( rule__XReturnExpression__ExpressionAssignment_2 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXReturnExpressionAccess().getExpressionAssignment_2());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34911:1: ( rule__XReturnExpression__ExpressionAssignment_2 )?
int alt253=2;
alt253 = dfa253.predict(input);
switch (alt253) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34911:2: rule__XReturnExpression__ExpressionAssignment_2
{
pushFollow(FollowSets003.FOLLOW_rule__XReturnExpression__ExpressionAssignment_2_in_rule__XReturnExpression__Group__2__Impl70153);
rule__XReturnExpression__ExpressionAssignment_2();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXReturnExpressionAccess().getExpressionAssignment_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XReturnExpression__Group__2__Impl"
// $ANTLR start "rule__XTryCatchFinallyExpression__Group__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34927:1: rule__XTryCatchFinallyExpression__Group__0 : rule__XTryCatchFinallyExpression__Group__0__Impl rule__XTryCatchFinallyExpression__Group__1 ;
public final void rule__XTryCatchFinallyExpression__Group__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34931:1: ( rule__XTryCatchFinallyExpression__Group__0__Impl rule__XTryCatchFinallyExpression__Group__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34932:2: rule__XTryCatchFinallyExpression__Group__0__Impl rule__XTryCatchFinallyExpression__Group__1
{
pushFollow(FollowSets003.FOLLOW_rule__XTryCatchFinallyExpression__Group__0__Impl_in_rule__XTryCatchFinallyExpression__Group__070190);
rule__XTryCatchFinallyExpression__Group__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets003.FOLLOW_rule__XTryCatchFinallyExpression__Group__1_in_rule__XTryCatchFinallyExpression__Group__070193);
rule__XTryCatchFinallyExpression__Group__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XTryCatchFinallyExpression__Group__0"
// $ANTLR start "rule__XTryCatchFinallyExpression__Group__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34939:1: rule__XTryCatchFinallyExpression__Group__0__Impl : ( () ) ;
public final void rule__XTryCatchFinallyExpression__Group__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34943:1: ( ( () ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34944:1: ( () )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34944:1: ( () )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34945:1: ()
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXTryCatchFinallyExpressionAccess().getXTryCatchFinallyExpressionAction_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34946:1: ()
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34948:1:
{
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXTryCatchFinallyExpressionAccess().getXTryCatchFinallyExpressionAction_0());
}
}
}
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XTryCatchFinallyExpression__Group__0__Impl"
// $ANTLR start "rule__XTryCatchFinallyExpression__Group__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34958:1: rule__XTryCatchFinallyExpression__Group__1 : rule__XTryCatchFinallyExpression__Group__1__Impl rule__XTryCatchFinallyExpression__Group__2 ;
public final void rule__XTryCatchFinallyExpression__Group__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34962:1: ( rule__XTryCatchFinallyExpression__Group__1__Impl rule__XTryCatchFinallyExpression__Group__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34963:2: rule__XTryCatchFinallyExpression__Group__1__Impl rule__XTryCatchFinallyExpression__Group__2
{
pushFollow(FollowSets003.FOLLOW_rule__XTryCatchFinallyExpression__Group__1__Impl_in_rule__XTryCatchFinallyExpression__Group__170251);
rule__XTryCatchFinallyExpression__Group__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets003.FOLLOW_rule__XTryCatchFinallyExpression__Group__2_in_rule__XTryCatchFinallyExpression__Group__170254);
rule__XTryCatchFinallyExpression__Group__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XTryCatchFinallyExpression__Group__1"
// $ANTLR start "rule__XTryCatchFinallyExpression__Group__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34970:1: rule__XTryCatchFinallyExpression__Group__1__Impl : ( 'try' ) ;
public final void rule__XTryCatchFinallyExpression__Group__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34974:1: ( ( 'try' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34975:1: ( 'try' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34975:1: ( 'try' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34976:1: 'try'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXTryCatchFinallyExpressionAccess().getTryKeyword_1());
}
match(input,173,FollowSets003.FOLLOW_173_in_rule__XTryCatchFinallyExpression__Group__1__Impl70282); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXTryCatchFinallyExpressionAccess().getTryKeyword_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XTryCatchFinallyExpression__Group__1__Impl"
// $ANTLR start "rule__XTryCatchFinallyExpression__Group__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34989:1: rule__XTryCatchFinallyExpression__Group__2 : rule__XTryCatchFinallyExpression__Group__2__Impl rule__XTryCatchFinallyExpression__Group__3 ;
public final void rule__XTryCatchFinallyExpression__Group__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34993:1: ( rule__XTryCatchFinallyExpression__Group__2__Impl rule__XTryCatchFinallyExpression__Group__3 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34994:2: rule__XTryCatchFinallyExpression__Group__2__Impl rule__XTryCatchFinallyExpression__Group__3
{
pushFollow(FollowSets003.FOLLOW_rule__XTryCatchFinallyExpression__Group__2__Impl_in_rule__XTryCatchFinallyExpression__Group__270313);
rule__XTryCatchFinallyExpression__Group__2__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets003.FOLLOW_rule__XTryCatchFinallyExpression__Group__3_in_rule__XTryCatchFinallyExpression__Group__270316);
rule__XTryCatchFinallyExpression__Group__3();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XTryCatchFinallyExpression__Group__2"
// $ANTLR start "rule__XTryCatchFinallyExpression__Group__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35001:1: rule__XTryCatchFinallyExpression__Group__2__Impl : ( ( rule__XTryCatchFinallyExpression__ExpressionAssignment_2 ) ) ;
public final void rule__XTryCatchFinallyExpression__Group__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35005:1: ( ( ( rule__XTryCatchFinallyExpression__ExpressionAssignment_2 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35006:1: ( ( rule__XTryCatchFinallyExpression__ExpressionAssignment_2 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35006:1: ( ( rule__XTryCatchFinallyExpression__ExpressionAssignment_2 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35007:1: ( rule__XTryCatchFinallyExpression__ExpressionAssignment_2 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXTryCatchFinallyExpressionAccess().getExpressionAssignment_2());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35008:1: ( rule__XTryCatchFinallyExpression__ExpressionAssignment_2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35008:2: rule__XTryCatchFinallyExpression__ExpressionAssignment_2
{
pushFollow(FollowSets003.FOLLOW_rule__XTryCatchFinallyExpression__ExpressionAssignment_2_in_rule__XTryCatchFinallyExpression__Group__2__Impl70343);
rule__XTryCatchFinallyExpression__ExpressionAssignment_2();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXTryCatchFinallyExpressionAccess().getExpressionAssignment_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XTryCatchFinallyExpression__Group__2__Impl"
// $ANTLR start "rule__XTryCatchFinallyExpression__Group__3"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35018:1: rule__XTryCatchFinallyExpression__Group__3 : rule__XTryCatchFinallyExpression__Group__3__Impl ;
public final void rule__XTryCatchFinallyExpression__Group__3() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35022:1: ( rule__XTryCatchFinallyExpression__Group__3__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35023:2: rule__XTryCatchFinallyExpression__Group__3__Impl
{
pushFollow(FollowSets003.FOLLOW_rule__XTryCatchFinallyExpression__Group__3__Impl_in_rule__XTryCatchFinallyExpression__Group__370373);
rule__XTryCatchFinallyExpression__Group__3__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XTryCatchFinallyExpression__Group__3"
// $ANTLR start "rule__XTryCatchFinallyExpression__Group__3__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35029:1: rule__XTryCatchFinallyExpression__Group__3__Impl : ( ( rule__XTryCatchFinallyExpression__Alternatives_3 ) ) ;
public final void rule__XTryCatchFinallyExpression__Group__3__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35033:1: ( ( ( rule__XTryCatchFinallyExpression__Alternatives_3 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35034:1: ( ( rule__XTryCatchFinallyExpression__Alternatives_3 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35034:1: ( ( rule__XTryCatchFinallyExpression__Alternatives_3 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35035:1: ( rule__XTryCatchFinallyExpression__Alternatives_3 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXTryCatchFinallyExpressionAccess().getAlternatives_3());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35036:1: ( rule__XTryCatchFinallyExpression__Alternatives_3 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35036:2: rule__XTryCatchFinallyExpression__Alternatives_3
{
pushFollow(FollowSets003.FOLLOW_rule__XTryCatchFinallyExpression__Alternatives_3_in_rule__XTryCatchFinallyExpression__Group__3__Impl70400);
rule__XTryCatchFinallyExpression__Alternatives_3();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXTryCatchFinallyExpressionAccess().getAlternatives_3());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XTryCatchFinallyExpression__Group__3__Impl"
// $ANTLR start "rule__XTryCatchFinallyExpression__Group_3_0__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35054:1: rule__XTryCatchFinallyExpression__Group_3_0__0 : rule__XTryCatchFinallyExpression__Group_3_0__0__Impl rule__XTryCatchFinallyExpression__Group_3_0__1 ;
public final void rule__XTryCatchFinallyExpression__Group_3_0__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35058:1: ( rule__XTryCatchFinallyExpression__Group_3_0__0__Impl rule__XTryCatchFinallyExpression__Group_3_0__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35059:2: rule__XTryCatchFinallyExpression__Group_3_0__0__Impl rule__XTryCatchFinallyExpression__Group_3_0__1
{
pushFollow(FollowSets003.FOLLOW_rule__XTryCatchFinallyExpression__Group_3_0__0__Impl_in_rule__XTryCatchFinallyExpression__Group_3_0__070438);
rule__XTryCatchFinallyExpression__Group_3_0__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets003.FOLLOW_rule__XTryCatchFinallyExpression__Group_3_0__1_in_rule__XTryCatchFinallyExpression__Group_3_0__070441);
rule__XTryCatchFinallyExpression__Group_3_0__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XTryCatchFinallyExpression__Group_3_0__0"
// $ANTLR start "rule__XTryCatchFinallyExpression__Group_3_0__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35066:1: rule__XTryCatchFinallyExpression__Group_3_0__0__Impl : ( ( ( rule__XTryCatchFinallyExpression__CatchClausesAssignment_3_0_0 ) ) ( ( rule__XTryCatchFinallyExpression__CatchClausesAssignment_3_0_0 )* ) ) ;
public final void rule__XTryCatchFinallyExpression__Group_3_0__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35070:1: ( ( ( ( rule__XTryCatchFinallyExpression__CatchClausesAssignment_3_0_0 ) ) ( ( rule__XTryCatchFinallyExpression__CatchClausesAssignment_3_0_0 )* ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35071:1: ( ( ( rule__XTryCatchFinallyExpression__CatchClausesAssignment_3_0_0 ) ) ( ( rule__XTryCatchFinallyExpression__CatchClausesAssignment_3_0_0 )* ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35071:1: ( ( ( rule__XTryCatchFinallyExpression__CatchClausesAssignment_3_0_0 ) ) ( ( rule__XTryCatchFinallyExpression__CatchClausesAssignment_3_0_0 )* ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35072:1: ( ( rule__XTryCatchFinallyExpression__CatchClausesAssignment_3_0_0 ) ) ( ( rule__XTryCatchFinallyExpression__CatchClausesAssignment_3_0_0 )* )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35072:1: ( ( rule__XTryCatchFinallyExpression__CatchClausesAssignment_3_0_0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35073:1: ( rule__XTryCatchFinallyExpression__CatchClausesAssignment_3_0_0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXTryCatchFinallyExpressionAccess().getCatchClausesAssignment_3_0_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35074:1: ( rule__XTryCatchFinallyExpression__CatchClausesAssignment_3_0_0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35074:2: rule__XTryCatchFinallyExpression__CatchClausesAssignment_3_0_0
{
pushFollow(FollowSets003.FOLLOW_rule__XTryCatchFinallyExpression__CatchClausesAssignment_3_0_0_in_rule__XTryCatchFinallyExpression__Group_3_0__0__Impl70470);
rule__XTryCatchFinallyExpression__CatchClausesAssignment_3_0_0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXTryCatchFinallyExpressionAccess().getCatchClausesAssignment_3_0_0());
}
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35077:1: ( ( rule__XTryCatchFinallyExpression__CatchClausesAssignment_3_0_0 )* )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35078:1: ( rule__XTryCatchFinallyExpression__CatchClausesAssignment_3_0_0 )*
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXTryCatchFinallyExpressionAccess().getCatchClausesAssignment_3_0_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35079:1: ( rule__XTryCatchFinallyExpression__CatchClausesAssignment_3_0_0 )*
loop254:
do {
int alt254=2;
int LA254_0 = input.LA(1);
if ( (LA254_0==176) ) {
int LA254_2 = input.LA(2);
if ( (synpred340_InternalMango()) ) {
alt254=1;
}
}
switch (alt254) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35079:2: rule__XTryCatchFinallyExpression__CatchClausesAssignment_3_0_0
{
pushFollow(FollowSets003.FOLLOW_rule__XTryCatchFinallyExpression__CatchClausesAssignment_3_0_0_in_rule__XTryCatchFinallyExpression__Group_3_0__0__Impl70482);
rule__XTryCatchFinallyExpression__CatchClausesAssignment_3_0_0();
state._fsp--;
if (state.failed) return ;
}
break;
default :
break loop254;
}
} while (true);
if ( state.backtracking==0 ) {
after(grammarAccess.getXTryCatchFinallyExpressionAccess().getCatchClausesAssignment_3_0_0());
}
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XTryCatchFinallyExpression__Group_3_0__0__Impl"
// $ANTLR start "rule__XTryCatchFinallyExpression__Group_3_0__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35090:1: rule__XTryCatchFinallyExpression__Group_3_0__1 : rule__XTryCatchFinallyExpression__Group_3_0__1__Impl ;
public final void rule__XTryCatchFinallyExpression__Group_3_0__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35094:1: ( rule__XTryCatchFinallyExpression__Group_3_0__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35095:2: rule__XTryCatchFinallyExpression__Group_3_0__1__Impl
{
pushFollow(FollowSets003.FOLLOW_rule__XTryCatchFinallyExpression__Group_3_0__1__Impl_in_rule__XTryCatchFinallyExpression__Group_3_0__170515);
rule__XTryCatchFinallyExpression__Group_3_0__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XTryCatchFinallyExpression__Group_3_0__1"
// $ANTLR start "rule__XTryCatchFinallyExpression__Group_3_0__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35101:1: rule__XTryCatchFinallyExpression__Group_3_0__1__Impl : ( ( rule__XTryCatchFinallyExpression__Group_3_0_1__0 )? ) ;
public final void rule__XTryCatchFinallyExpression__Group_3_0__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35105:1: ( ( ( rule__XTryCatchFinallyExpression__Group_3_0_1__0 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35106:1: ( ( rule__XTryCatchFinallyExpression__Group_3_0_1__0 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35106:1: ( ( rule__XTryCatchFinallyExpression__Group_3_0_1__0 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35107:1: ( rule__XTryCatchFinallyExpression__Group_3_0_1__0 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXTryCatchFinallyExpressionAccess().getGroup_3_0_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35108:1: ( rule__XTryCatchFinallyExpression__Group_3_0_1__0 )?
int alt255=2;
int LA255_0 = input.LA(1);
if ( (LA255_0==174) ) {
int LA255_1 = input.LA(2);
if ( (synpred341_InternalMango()) ) {
alt255=1;
}
}
switch (alt255) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35108:2: rule__XTryCatchFinallyExpression__Group_3_0_1__0
{
pushFollow(FollowSets003.FOLLOW_rule__XTryCatchFinallyExpression__Group_3_0_1__0_in_rule__XTryCatchFinallyExpression__Group_3_0__1__Impl70542);
rule__XTryCatchFinallyExpression__Group_3_0_1__0();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXTryCatchFinallyExpressionAccess().getGroup_3_0_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XTryCatchFinallyExpression__Group_3_0__1__Impl"
// $ANTLR start "rule__XTryCatchFinallyExpression__Group_3_0_1__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35122:1: rule__XTryCatchFinallyExpression__Group_3_0_1__0 : rule__XTryCatchFinallyExpression__Group_3_0_1__0__Impl rule__XTryCatchFinallyExpression__Group_3_0_1__1 ;
public final void rule__XTryCatchFinallyExpression__Group_3_0_1__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35126:1: ( rule__XTryCatchFinallyExpression__Group_3_0_1__0__Impl rule__XTryCatchFinallyExpression__Group_3_0_1__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35127:2: rule__XTryCatchFinallyExpression__Group_3_0_1__0__Impl rule__XTryCatchFinallyExpression__Group_3_0_1__1
{
pushFollow(FollowSets003.FOLLOW_rule__XTryCatchFinallyExpression__Group_3_0_1__0__Impl_in_rule__XTryCatchFinallyExpression__Group_3_0_1__070577);
rule__XTryCatchFinallyExpression__Group_3_0_1__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets003.FOLLOW_rule__XTryCatchFinallyExpression__Group_3_0_1__1_in_rule__XTryCatchFinallyExpression__Group_3_0_1__070580);
rule__XTryCatchFinallyExpression__Group_3_0_1__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XTryCatchFinallyExpression__Group_3_0_1__0"
// $ANTLR start "rule__XTryCatchFinallyExpression__Group_3_0_1__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35134:1: rule__XTryCatchFinallyExpression__Group_3_0_1__0__Impl : ( ( 'finally' ) ) ;
public final void rule__XTryCatchFinallyExpression__Group_3_0_1__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35138:1: ( ( ( 'finally' ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35139:1: ( ( 'finally' ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35139:1: ( ( 'finally' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35140:1: ( 'finally' )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXTryCatchFinallyExpressionAccess().getFinallyKeyword_3_0_1_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35141:1: ( 'finally' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35142:2: 'finally'
{
match(input,174,FollowSets003.FOLLOW_174_in_rule__XTryCatchFinallyExpression__Group_3_0_1__0__Impl70609); if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXTryCatchFinallyExpressionAccess().getFinallyKeyword_3_0_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XTryCatchFinallyExpression__Group_3_0_1__0__Impl"
// $ANTLR start "rule__XTryCatchFinallyExpression__Group_3_0_1__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35153:1: rule__XTryCatchFinallyExpression__Group_3_0_1__1 : rule__XTryCatchFinallyExpression__Group_3_0_1__1__Impl ;
public final void rule__XTryCatchFinallyExpression__Group_3_0_1__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35157:1: ( rule__XTryCatchFinallyExpression__Group_3_0_1__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35158:2: rule__XTryCatchFinallyExpression__Group_3_0_1__1__Impl
{
pushFollow(FollowSets003.FOLLOW_rule__XTryCatchFinallyExpression__Group_3_0_1__1__Impl_in_rule__XTryCatchFinallyExpression__Group_3_0_1__170641);
rule__XTryCatchFinallyExpression__Group_3_0_1__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XTryCatchFinallyExpression__Group_3_0_1__1"
// $ANTLR start "rule__XTryCatchFinallyExpression__Group_3_0_1__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35164:1: rule__XTryCatchFinallyExpression__Group_3_0_1__1__Impl : ( ( rule__XTryCatchFinallyExpression__FinallyExpressionAssignment_3_0_1_1 ) ) ;
public final void rule__XTryCatchFinallyExpression__Group_3_0_1__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35168:1: ( ( ( rule__XTryCatchFinallyExpression__FinallyExpressionAssignment_3_0_1_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35169:1: ( ( rule__XTryCatchFinallyExpression__FinallyExpressionAssignment_3_0_1_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35169:1: ( ( rule__XTryCatchFinallyExpression__FinallyExpressionAssignment_3_0_1_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35170:1: ( rule__XTryCatchFinallyExpression__FinallyExpressionAssignment_3_0_1_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXTryCatchFinallyExpressionAccess().getFinallyExpressionAssignment_3_0_1_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35171:1: ( rule__XTryCatchFinallyExpression__FinallyExpressionAssignment_3_0_1_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35171:2: rule__XTryCatchFinallyExpression__FinallyExpressionAssignment_3_0_1_1
{
pushFollow(FollowSets003.FOLLOW_rule__XTryCatchFinallyExpression__FinallyExpressionAssignment_3_0_1_1_in_rule__XTryCatchFinallyExpression__Group_3_0_1__1__Impl70668);
rule__XTryCatchFinallyExpression__FinallyExpressionAssignment_3_0_1_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXTryCatchFinallyExpressionAccess().getFinallyExpressionAssignment_3_0_1_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XTryCatchFinallyExpression__Group_3_0_1__1__Impl"
// $ANTLR start "rule__XTryCatchFinallyExpression__Group_3_1__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35185:1: rule__XTryCatchFinallyExpression__Group_3_1__0 : rule__XTryCatchFinallyExpression__Group_3_1__0__Impl rule__XTryCatchFinallyExpression__Group_3_1__1 ;
public final void rule__XTryCatchFinallyExpression__Group_3_1__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35189:1: ( rule__XTryCatchFinallyExpression__Group_3_1__0__Impl rule__XTryCatchFinallyExpression__Group_3_1__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35190:2: rule__XTryCatchFinallyExpression__Group_3_1__0__Impl rule__XTryCatchFinallyExpression__Group_3_1__1
{
pushFollow(FollowSets003.FOLLOW_rule__XTryCatchFinallyExpression__Group_3_1__0__Impl_in_rule__XTryCatchFinallyExpression__Group_3_1__070702);
rule__XTryCatchFinallyExpression__Group_3_1__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets003.FOLLOW_rule__XTryCatchFinallyExpression__Group_3_1__1_in_rule__XTryCatchFinallyExpression__Group_3_1__070705);
rule__XTryCatchFinallyExpression__Group_3_1__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XTryCatchFinallyExpression__Group_3_1__0"
// $ANTLR start "rule__XTryCatchFinallyExpression__Group_3_1__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35197:1: rule__XTryCatchFinallyExpression__Group_3_1__0__Impl : ( 'finally' ) ;
public final void rule__XTryCatchFinallyExpression__Group_3_1__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35201:1: ( ( 'finally' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35202:1: ( 'finally' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35202:1: ( 'finally' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35203:1: 'finally'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXTryCatchFinallyExpressionAccess().getFinallyKeyword_3_1_0());
}
match(input,174,FollowSets003.FOLLOW_174_in_rule__XTryCatchFinallyExpression__Group_3_1__0__Impl70733); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXTryCatchFinallyExpressionAccess().getFinallyKeyword_3_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XTryCatchFinallyExpression__Group_3_1__0__Impl"
// $ANTLR start "rule__XTryCatchFinallyExpression__Group_3_1__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35216:1: rule__XTryCatchFinallyExpression__Group_3_1__1 : rule__XTryCatchFinallyExpression__Group_3_1__1__Impl ;
public final void rule__XTryCatchFinallyExpression__Group_3_1__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35220:1: ( rule__XTryCatchFinallyExpression__Group_3_1__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35221:2: rule__XTryCatchFinallyExpression__Group_3_1__1__Impl
{
pushFollow(FollowSets003.FOLLOW_rule__XTryCatchFinallyExpression__Group_3_1__1__Impl_in_rule__XTryCatchFinallyExpression__Group_3_1__170764);
rule__XTryCatchFinallyExpression__Group_3_1__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XTryCatchFinallyExpression__Group_3_1__1"
// $ANTLR start "rule__XTryCatchFinallyExpression__Group_3_1__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35227:1: rule__XTryCatchFinallyExpression__Group_3_1__1__Impl : ( ( rule__XTryCatchFinallyExpression__FinallyExpressionAssignment_3_1_1 ) ) ;
public final void rule__XTryCatchFinallyExpression__Group_3_1__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35231:1: ( ( ( rule__XTryCatchFinallyExpression__FinallyExpressionAssignment_3_1_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35232:1: ( ( rule__XTryCatchFinallyExpression__FinallyExpressionAssignment_3_1_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35232:1: ( ( rule__XTryCatchFinallyExpression__FinallyExpressionAssignment_3_1_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35233:1: ( rule__XTryCatchFinallyExpression__FinallyExpressionAssignment_3_1_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXTryCatchFinallyExpressionAccess().getFinallyExpressionAssignment_3_1_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35234:1: ( rule__XTryCatchFinallyExpression__FinallyExpressionAssignment_3_1_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35234:2: rule__XTryCatchFinallyExpression__FinallyExpressionAssignment_3_1_1
{
pushFollow(FollowSets003.FOLLOW_rule__XTryCatchFinallyExpression__FinallyExpressionAssignment_3_1_1_in_rule__XTryCatchFinallyExpression__Group_3_1__1__Impl70791);
rule__XTryCatchFinallyExpression__FinallyExpressionAssignment_3_1_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXTryCatchFinallyExpressionAccess().getFinallyExpressionAssignment_3_1_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XTryCatchFinallyExpression__Group_3_1__1__Impl"
// $ANTLR start "rule__XSynchronizedExpression__Group__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35248:1: rule__XSynchronizedExpression__Group__0 : rule__XSynchronizedExpression__Group__0__Impl rule__XSynchronizedExpression__Group__1 ;
public final void rule__XSynchronizedExpression__Group__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35252:1: ( rule__XSynchronizedExpression__Group__0__Impl rule__XSynchronizedExpression__Group__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35253:2: rule__XSynchronizedExpression__Group__0__Impl rule__XSynchronizedExpression__Group__1
{
pushFollow(FollowSets003.FOLLOW_rule__XSynchronizedExpression__Group__0__Impl_in_rule__XSynchronizedExpression__Group__070825);
rule__XSynchronizedExpression__Group__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets003.FOLLOW_rule__XSynchronizedExpression__Group__1_in_rule__XSynchronizedExpression__Group__070828);
rule__XSynchronizedExpression__Group__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XSynchronizedExpression__Group__0"
// $ANTLR start "rule__XSynchronizedExpression__Group__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35260:1: rule__XSynchronizedExpression__Group__0__Impl : ( ( rule__XSynchronizedExpression__Group_0__0 ) ) ;
public final void rule__XSynchronizedExpression__Group__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35264:1: ( ( ( rule__XSynchronizedExpression__Group_0__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35265:1: ( ( rule__XSynchronizedExpression__Group_0__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35265:1: ( ( rule__XSynchronizedExpression__Group_0__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35266:1: ( rule__XSynchronizedExpression__Group_0__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXSynchronizedExpressionAccess().getGroup_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35267:1: ( rule__XSynchronizedExpression__Group_0__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35267:2: rule__XSynchronizedExpression__Group_0__0
{
pushFollow(FollowSets003.FOLLOW_rule__XSynchronizedExpression__Group_0__0_in_rule__XSynchronizedExpression__Group__0__Impl70855);
rule__XSynchronizedExpression__Group_0__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXSynchronizedExpressionAccess().getGroup_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XSynchronizedExpression__Group__0__Impl"
// $ANTLR start "rule__XSynchronizedExpression__Group__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35277:1: rule__XSynchronizedExpression__Group__1 : rule__XSynchronizedExpression__Group__1__Impl rule__XSynchronizedExpression__Group__2 ;
public final void rule__XSynchronizedExpression__Group__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35281:1: ( rule__XSynchronizedExpression__Group__1__Impl rule__XSynchronizedExpression__Group__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35282:2: rule__XSynchronizedExpression__Group__1__Impl rule__XSynchronizedExpression__Group__2
{
pushFollow(FollowSets003.FOLLOW_rule__XSynchronizedExpression__Group__1__Impl_in_rule__XSynchronizedExpression__Group__170885);
rule__XSynchronizedExpression__Group__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets003.FOLLOW_rule__XSynchronizedExpression__Group__2_in_rule__XSynchronizedExpression__Group__170888);
rule__XSynchronizedExpression__Group__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XSynchronizedExpression__Group__1"
// $ANTLR start "rule__XSynchronizedExpression__Group__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35289:1: rule__XSynchronizedExpression__Group__1__Impl : ( ( rule__XSynchronizedExpression__ParamAssignment_1 ) ) ;
public final void rule__XSynchronizedExpression__Group__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35293:1: ( ( ( rule__XSynchronizedExpression__ParamAssignment_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35294:1: ( ( rule__XSynchronizedExpression__ParamAssignment_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35294:1: ( ( rule__XSynchronizedExpression__ParamAssignment_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35295:1: ( rule__XSynchronizedExpression__ParamAssignment_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXSynchronizedExpressionAccess().getParamAssignment_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35296:1: ( rule__XSynchronizedExpression__ParamAssignment_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35296:2: rule__XSynchronizedExpression__ParamAssignment_1
{
pushFollow(FollowSets003.FOLLOW_rule__XSynchronizedExpression__ParamAssignment_1_in_rule__XSynchronizedExpression__Group__1__Impl70915);
rule__XSynchronizedExpression__ParamAssignment_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXSynchronizedExpressionAccess().getParamAssignment_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XSynchronizedExpression__Group__1__Impl"
// $ANTLR start "rule__XSynchronizedExpression__Group__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35306:1: rule__XSynchronizedExpression__Group__2 : rule__XSynchronizedExpression__Group__2__Impl rule__XSynchronizedExpression__Group__3 ;
public final void rule__XSynchronizedExpression__Group__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35310:1: ( rule__XSynchronizedExpression__Group__2__Impl rule__XSynchronizedExpression__Group__3 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35311:2: rule__XSynchronizedExpression__Group__2__Impl rule__XSynchronizedExpression__Group__3
{
pushFollow(FollowSets003.FOLLOW_rule__XSynchronizedExpression__Group__2__Impl_in_rule__XSynchronizedExpression__Group__270945);
rule__XSynchronizedExpression__Group__2__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets003.FOLLOW_rule__XSynchronizedExpression__Group__3_in_rule__XSynchronizedExpression__Group__270948);
rule__XSynchronizedExpression__Group__3();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XSynchronizedExpression__Group__2"
// $ANTLR start "rule__XSynchronizedExpression__Group__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35318:1: rule__XSynchronizedExpression__Group__2__Impl : ( ')' ) ;
public final void rule__XSynchronizedExpression__Group__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35322:1: ( ( ')' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35323:1: ( ')' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35323:1: ( ')' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35324:1: ')'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXSynchronizedExpressionAccess().getRightParenthesisKeyword_2());
}
match(input,145,FollowSets003.FOLLOW_145_in_rule__XSynchronizedExpression__Group__2__Impl70976); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXSynchronizedExpressionAccess().getRightParenthesisKeyword_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XSynchronizedExpression__Group__2__Impl"
// $ANTLR start "rule__XSynchronizedExpression__Group__3"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35337:1: rule__XSynchronizedExpression__Group__3 : rule__XSynchronizedExpression__Group__3__Impl ;
public final void rule__XSynchronizedExpression__Group__3() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35341:1: ( rule__XSynchronizedExpression__Group__3__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35342:2: rule__XSynchronizedExpression__Group__3__Impl
{
pushFollow(FollowSets003.FOLLOW_rule__XSynchronizedExpression__Group__3__Impl_in_rule__XSynchronizedExpression__Group__371007);
rule__XSynchronizedExpression__Group__3__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XSynchronizedExpression__Group__3"
// $ANTLR start "rule__XSynchronizedExpression__Group__3__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35348:1: rule__XSynchronizedExpression__Group__3__Impl : ( ( rule__XSynchronizedExpression__ExpressionAssignment_3 ) ) ;
public final void rule__XSynchronizedExpression__Group__3__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35352:1: ( ( ( rule__XSynchronizedExpression__ExpressionAssignment_3 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35353:1: ( ( rule__XSynchronizedExpression__ExpressionAssignment_3 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35353:1: ( ( rule__XSynchronizedExpression__ExpressionAssignment_3 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35354:1: ( rule__XSynchronizedExpression__ExpressionAssignment_3 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXSynchronizedExpressionAccess().getExpressionAssignment_3());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35355:1: ( rule__XSynchronizedExpression__ExpressionAssignment_3 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35355:2: rule__XSynchronizedExpression__ExpressionAssignment_3
{
pushFollow(FollowSets003.FOLLOW_rule__XSynchronizedExpression__ExpressionAssignment_3_in_rule__XSynchronizedExpression__Group__3__Impl71034);
rule__XSynchronizedExpression__ExpressionAssignment_3();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXSynchronizedExpressionAccess().getExpressionAssignment_3());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XSynchronizedExpression__Group__3__Impl"
// $ANTLR start "rule__XSynchronizedExpression__Group_0__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35373:1: rule__XSynchronizedExpression__Group_0__0 : rule__XSynchronizedExpression__Group_0__0__Impl ;
public final void rule__XSynchronizedExpression__Group_0__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35377:1: ( rule__XSynchronizedExpression__Group_0__0__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35378:2: rule__XSynchronizedExpression__Group_0__0__Impl
{
pushFollow(FollowSets003.FOLLOW_rule__XSynchronizedExpression__Group_0__0__Impl_in_rule__XSynchronizedExpression__Group_0__071072);
rule__XSynchronizedExpression__Group_0__0__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XSynchronizedExpression__Group_0__0"
// $ANTLR start "rule__XSynchronizedExpression__Group_0__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35384:1: rule__XSynchronizedExpression__Group_0__0__Impl : ( ( rule__XSynchronizedExpression__Group_0_0__0 ) ) ;
public final void rule__XSynchronizedExpression__Group_0__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35388:1: ( ( ( rule__XSynchronizedExpression__Group_0_0__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35389:1: ( ( rule__XSynchronizedExpression__Group_0_0__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35389:1: ( ( rule__XSynchronizedExpression__Group_0_0__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35390:1: ( rule__XSynchronizedExpression__Group_0_0__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXSynchronizedExpressionAccess().getGroup_0_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35391:1: ( rule__XSynchronizedExpression__Group_0_0__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35391:2: rule__XSynchronizedExpression__Group_0_0__0
{
pushFollow(FollowSets003.FOLLOW_rule__XSynchronizedExpression__Group_0_0__0_in_rule__XSynchronizedExpression__Group_0__0__Impl71099);
rule__XSynchronizedExpression__Group_0_0__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXSynchronizedExpressionAccess().getGroup_0_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XSynchronizedExpression__Group_0__0__Impl"
// $ANTLR start "rule__XSynchronizedExpression__Group_0_0__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35403:1: rule__XSynchronizedExpression__Group_0_0__0 : rule__XSynchronizedExpression__Group_0_0__0__Impl rule__XSynchronizedExpression__Group_0_0__1 ;
public final void rule__XSynchronizedExpression__Group_0_0__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35407:1: ( rule__XSynchronizedExpression__Group_0_0__0__Impl rule__XSynchronizedExpression__Group_0_0__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35408:2: rule__XSynchronizedExpression__Group_0_0__0__Impl rule__XSynchronizedExpression__Group_0_0__1
{
pushFollow(FollowSets003.FOLLOW_rule__XSynchronizedExpression__Group_0_0__0__Impl_in_rule__XSynchronizedExpression__Group_0_0__071131);
rule__XSynchronizedExpression__Group_0_0__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets003.FOLLOW_rule__XSynchronizedExpression__Group_0_0__1_in_rule__XSynchronizedExpression__Group_0_0__071134);
rule__XSynchronizedExpression__Group_0_0__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XSynchronizedExpression__Group_0_0__0"
// $ANTLR start "rule__XSynchronizedExpression__Group_0_0__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35415:1: rule__XSynchronizedExpression__Group_0_0__0__Impl : ( () ) ;
public final void rule__XSynchronizedExpression__Group_0_0__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35419:1: ( ( () ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35420:1: ( () )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35420:1: ( () )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35421:1: ()
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXSynchronizedExpressionAccess().getXSynchronizedExpressionAction_0_0_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35422:1: ()
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35424:1:
{
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXSynchronizedExpressionAccess().getXSynchronizedExpressionAction_0_0_0());
}
}
}
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XSynchronizedExpression__Group_0_0__0__Impl"
// $ANTLR start "rule__XSynchronizedExpression__Group_0_0__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35434:1: rule__XSynchronizedExpression__Group_0_0__1 : rule__XSynchronizedExpression__Group_0_0__1__Impl rule__XSynchronizedExpression__Group_0_0__2 ;
public final void rule__XSynchronizedExpression__Group_0_0__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35438:1: ( rule__XSynchronizedExpression__Group_0_0__1__Impl rule__XSynchronizedExpression__Group_0_0__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35439:2: rule__XSynchronizedExpression__Group_0_0__1__Impl rule__XSynchronizedExpression__Group_0_0__2
{
pushFollow(FollowSets003.FOLLOW_rule__XSynchronizedExpression__Group_0_0__1__Impl_in_rule__XSynchronizedExpression__Group_0_0__171192);
rule__XSynchronizedExpression__Group_0_0__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets003.FOLLOW_rule__XSynchronizedExpression__Group_0_0__2_in_rule__XSynchronizedExpression__Group_0_0__171195);
rule__XSynchronizedExpression__Group_0_0__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XSynchronizedExpression__Group_0_0__1"
// $ANTLR start "rule__XSynchronizedExpression__Group_0_0__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35446:1: rule__XSynchronizedExpression__Group_0_0__1__Impl : ( 'synchronized' ) ;
public final void rule__XSynchronizedExpression__Group_0_0__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35450:1: ( ( 'synchronized' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35451:1: ( 'synchronized' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35451:1: ( 'synchronized' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35452:1: 'synchronized'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXSynchronizedExpressionAccess().getSynchronizedKeyword_0_0_1());
}
match(input,175,FollowSets003.FOLLOW_175_in_rule__XSynchronizedExpression__Group_0_0__1__Impl71223); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXSynchronizedExpressionAccess().getSynchronizedKeyword_0_0_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XSynchronizedExpression__Group_0_0__1__Impl"
// $ANTLR start "rule__XSynchronizedExpression__Group_0_0__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35465:1: rule__XSynchronizedExpression__Group_0_0__2 : rule__XSynchronizedExpression__Group_0_0__2__Impl ;
public final void rule__XSynchronizedExpression__Group_0_0__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35469:1: ( rule__XSynchronizedExpression__Group_0_0__2__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35470:2: rule__XSynchronizedExpression__Group_0_0__2__Impl
{
pushFollow(FollowSets003.FOLLOW_rule__XSynchronizedExpression__Group_0_0__2__Impl_in_rule__XSynchronizedExpression__Group_0_0__271254);
rule__XSynchronizedExpression__Group_0_0__2__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XSynchronizedExpression__Group_0_0__2"
// $ANTLR start "rule__XSynchronizedExpression__Group_0_0__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35476:1: rule__XSynchronizedExpression__Group_0_0__2__Impl : ( '(' ) ;
public final void rule__XSynchronizedExpression__Group_0_0__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35480:1: ( ( '(' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35481:1: ( '(' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35481:1: ( '(' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35482:1: '('
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXSynchronizedExpressionAccess().getLeftParenthesisKeyword_0_0_2());
}
match(input,144,FollowSets003.FOLLOW_144_in_rule__XSynchronizedExpression__Group_0_0__2__Impl71282); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXSynchronizedExpressionAccess().getLeftParenthesisKeyword_0_0_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XSynchronizedExpression__Group_0_0__2__Impl"
// $ANTLR start "rule__XCatchClause__Group__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35501:1: rule__XCatchClause__Group__0 : rule__XCatchClause__Group__0__Impl rule__XCatchClause__Group__1 ;
public final void rule__XCatchClause__Group__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35505:1: ( rule__XCatchClause__Group__0__Impl rule__XCatchClause__Group__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35506:2: rule__XCatchClause__Group__0__Impl rule__XCatchClause__Group__1
{
pushFollow(FollowSets003.FOLLOW_rule__XCatchClause__Group__0__Impl_in_rule__XCatchClause__Group__071319);
rule__XCatchClause__Group__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets003.FOLLOW_rule__XCatchClause__Group__1_in_rule__XCatchClause__Group__071322);
rule__XCatchClause__Group__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XCatchClause__Group__0"
// $ANTLR start "rule__XCatchClause__Group__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35513:1: rule__XCatchClause__Group__0__Impl : ( ( 'catch' ) ) ;
public final void rule__XCatchClause__Group__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35517:1: ( ( ( 'catch' ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35518:1: ( ( 'catch' ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35518:1: ( ( 'catch' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35519:1: ( 'catch' )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXCatchClauseAccess().getCatchKeyword_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35520:1: ( 'catch' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35521:2: 'catch'
{
match(input,176,FollowSets003.FOLLOW_176_in_rule__XCatchClause__Group__0__Impl71351); if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXCatchClauseAccess().getCatchKeyword_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XCatchClause__Group__0__Impl"
// $ANTLR start "rule__XCatchClause__Group__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35532:1: rule__XCatchClause__Group__1 : rule__XCatchClause__Group__1__Impl rule__XCatchClause__Group__2 ;
public final void rule__XCatchClause__Group__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35536:1: ( rule__XCatchClause__Group__1__Impl rule__XCatchClause__Group__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35537:2: rule__XCatchClause__Group__1__Impl rule__XCatchClause__Group__2
{
pushFollow(FollowSets003.FOLLOW_rule__XCatchClause__Group__1__Impl_in_rule__XCatchClause__Group__171383);
rule__XCatchClause__Group__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets003.FOLLOW_rule__XCatchClause__Group__2_in_rule__XCatchClause__Group__171386);
rule__XCatchClause__Group__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XCatchClause__Group__1"
// $ANTLR start "rule__XCatchClause__Group__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35544:1: rule__XCatchClause__Group__1__Impl : ( '(' ) ;
public final void rule__XCatchClause__Group__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35548:1: ( ( '(' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35549:1: ( '(' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35549:1: ( '(' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35550:1: '('
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXCatchClauseAccess().getLeftParenthesisKeyword_1());
}
match(input,144,FollowSets003.FOLLOW_144_in_rule__XCatchClause__Group__1__Impl71414); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXCatchClauseAccess().getLeftParenthesisKeyword_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XCatchClause__Group__1__Impl"
// $ANTLR start "rule__XCatchClause__Group__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35563:1: rule__XCatchClause__Group__2 : rule__XCatchClause__Group__2__Impl rule__XCatchClause__Group__3 ;
public final void rule__XCatchClause__Group__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35567:1: ( rule__XCatchClause__Group__2__Impl rule__XCatchClause__Group__3 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35568:2: rule__XCatchClause__Group__2__Impl rule__XCatchClause__Group__3
{
pushFollow(FollowSets003.FOLLOW_rule__XCatchClause__Group__2__Impl_in_rule__XCatchClause__Group__271445);
rule__XCatchClause__Group__2__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets003.FOLLOW_rule__XCatchClause__Group__3_in_rule__XCatchClause__Group__271448);
rule__XCatchClause__Group__3();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XCatchClause__Group__2"
// $ANTLR start "rule__XCatchClause__Group__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35575:1: rule__XCatchClause__Group__2__Impl : ( ( rule__XCatchClause__DeclaredParamAssignment_2 ) ) ;
public final void rule__XCatchClause__Group__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35579:1: ( ( ( rule__XCatchClause__DeclaredParamAssignment_2 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35580:1: ( ( rule__XCatchClause__DeclaredParamAssignment_2 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35580:1: ( ( rule__XCatchClause__DeclaredParamAssignment_2 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35581:1: ( rule__XCatchClause__DeclaredParamAssignment_2 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXCatchClauseAccess().getDeclaredParamAssignment_2());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35582:1: ( rule__XCatchClause__DeclaredParamAssignment_2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35582:2: rule__XCatchClause__DeclaredParamAssignment_2
{
pushFollow(FollowSets003.FOLLOW_rule__XCatchClause__DeclaredParamAssignment_2_in_rule__XCatchClause__Group__2__Impl71475);
rule__XCatchClause__DeclaredParamAssignment_2();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXCatchClauseAccess().getDeclaredParamAssignment_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XCatchClause__Group__2__Impl"
// $ANTLR start "rule__XCatchClause__Group__3"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35592:1: rule__XCatchClause__Group__3 : rule__XCatchClause__Group__3__Impl rule__XCatchClause__Group__4 ;
public final void rule__XCatchClause__Group__3() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35596:1: ( rule__XCatchClause__Group__3__Impl rule__XCatchClause__Group__4 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35597:2: rule__XCatchClause__Group__3__Impl rule__XCatchClause__Group__4
{
pushFollow(FollowSets003.FOLLOW_rule__XCatchClause__Group__3__Impl_in_rule__XCatchClause__Group__371505);
rule__XCatchClause__Group__3__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets003.FOLLOW_rule__XCatchClause__Group__4_in_rule__XCatchClause__Group__371508);
rule__XCatchClause__Group__4();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XCatchClause__Group__3"
// $ANTLR start "rule__XCatchClause__Group__3__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35604:1: rule__XCatchClause__Group__3__Impl : ( ')' ) ;
public final void rule__XCatchClause__Group__3__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35608:1: ( ( ')' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35609:1: ( ')' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35609:1: ( ')' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35610:1: ')'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXCatchClauseAccess().getRightParenthesisKeyword_3());
}
match(input,145,FollowSets003.FOLLOW_145_in_rule__XCatchClause__Group__3__Impl71536); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXCatchClauseAccess().getRightParenthesisKeyword_3());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XCatchClause__Group__3__Impl"
// $ANTLR start "rule__XCatchClause__Group__4"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35623:1: rule__XCatchClause__Group__4 : rule__XCatchClause__Group__4__Impl ;
public final void rule__XCatchClause__Group__4() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35627:1: ( rule__XCatchClause__Group__4__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35628:2: rule__XCatchClause__Group__4__Impl
{
pushFollow(FollowSets003.FOLLOW_rule__XCatchClause__Group__4__Impl_in_rule__XCatchClause__Group__471567);
rule__XCatchClause__Group__4__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XCatchClause__Group__4"
// $ANTLR start "rule__XCatchClause__Group__4__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35634:1: rule__XCatchClause__Group__4__Impl : ( ( rule__XCatchClause__ExpressionAssignment_4 ) ) ;
public final void rule__XCatchClause__Group__4__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35638:1: ( ( ( rule__XCatchClause__ExpressionAssignment_4 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35639:1: ( ( rule__XCatchClause__ExpressionAssignment_4 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35639:1: ( ( rule__XCatchClause__ExpressionAssignment_4 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35640:1: ( rule__XCatchClause__ExpressionAssignment_4 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXCatchClauseAccess().getExpressionAssignment_4());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35641:1: ( rule__XCatchClause__ExpressionAssignment_4 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35641:2: rule__XCatchClause__ExpressionAssignment_4
{
pushFollow(FollowSets003.FOLLOW_rule__XCatchClause__ExpressionAssignment_4_in_rule__XCatchClause__Group__4__Impl71594);
rule__XCatchClause__ExpressionAssignment_4();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXCatchClauseAccess().getExpressionAssignment_4());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XCatchClause__Group__4__Impl"
// $ANTLR start "rule__QualifiedName__Group__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35661:1: rule__QualifiedName__Group__0 : rule__QualifiedName__Group__0__Impl rule__QualifiedName__Group__1 ;
public final void rule__QualifiedName__Group__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35665:1: ( rule__QualifiedName__Group__0__Impl rule__QualifiedName__Group__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35666:2: rule__QualifiedName__Group__0__Impl rule__QualifiedName__Group__1
{
pushFollow(FollowSets003.FOLLOW_rule__QualifiedName__Group__0__Impl_in_rule__QualifiedName__Group__071634);
rule__QualifiedName__Group__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets003.FOLLOW_rule__QualifiedName__Group__1_in_rule__QualifiedName__Group__071637);
rule__QualifiedName__Group__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__QualifiedName__Group__0"
// $ANTLR start "rule__QualifiedName__Group__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35673:1: rule__QualifiedName__Group__0__Impl : ( ruleValidID ) ;
public final void rule__QualifiedName__Group__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35677:1: ( ( ruleValidID ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35678:1: ( ruleValidID )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35678:1: ( ruleValidID )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35679:1: ruleValidID
{
if ( state.backtracking==0 ) {
before(grammarAccess.getQualifiedNameAccess().getValidIDParserRuleCall_0());
}
pushFollow(FollowSets003.FOLLOW_ruleValidID_in_rule__QualifiedName__Group__0__Impl71664);
ruleValidID();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getQualifiedNameAccess().getValidIDParserRuleCall_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__QualifiedName__Group__0__Impl"
// $ANTLR start "rule__QualifiedName__Group__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35690:1: rule__QualifiedName__Group__1 : rule__QualifiedName__Group__1__Impl ;
public final void rule__QualifiedName__Group__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35694:1: ( rule__QualifiedName__Group__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35695:2: rule__QualifiedName__Group__1__Impl
{
pushFollow(FollowSets003.FOLLOW_rule__QualifiedName__Group__1__Impl_in_rule__QualifiedName__Group__171693);
rule__QualifiedName__Group__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__QualifiedName__Group__1"
// $ANTLR start "rule__QualifiedName__Group__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35701:1: rule__QualifiedName__Group__1__Impl : ( ( rule__QualifiedName__Group_1__0 )* ) ;
public final void rule__QualifiedName__Group__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35705:1: ( ( ( rule__QualifiedName__Group_1__0 )* ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35706:1: ( ( rule__QualifiedName__Group_1__0 )* )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35706:1: ( ( rule__QualifiedName__Group_1__0 )* )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35707:1: ( rule__QualifiedName__Group_1__0 )*
{
if ( state.backtracking==0 ) {
before(grammarAccess.getQualifiedNameAccess().getGroup_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35708:1: ( rule__QualifiedName__Group_1__0 )*
loop256:
do {
int alt256=2;
int LA256_0 = input.LA(1);
if ( (LA256_0==43) ) {
int LA256_2 = input.LA(2);
if ( (LA256_2==RULE_ID) ) {
int LA256_3 = input.LA(3);
if ( (synpred342_InternalMango()) ) {
alt256=1;
}
}
}
switch (alt256) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35708:2: rule__QualifiedName__Group_1__0
{
pushFollow(FollowSets003.FOLLOW_rule__QualifiedName__Group_1__0_in_rule__QualifiedName__Group__1__Impl71720);
rule__QualifiedName__Group_1__0();
state._fsp--;
if (state.failed) return ;
}
break;
default :
break loop256;
}
} while (true);
if ( state.backtracking==0 ) {
after(grammarAccess.getQualifiedNameAccess().getGroup_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__QualifiedName__Group__1__Impl"
// $ANTLR start "rule__QualifiedName__Group_1__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35722:1: rule__QualifiedName__Group_1__0 : rule__QualifiedName__Group_1__0__Impl rule__QualifiedName__Group_1__1 ;
public final void rule__QualifiedName__Group_1__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35726:1: ( rule__QualifiedName__Group_1__0__Impl rule__QualifiedName__Group_1__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35727:2: rule__QualifiedName__Group_1__0__Impl rule__QualifiedName__Group_1__1
{
pushFollow(FollowSets003.FOLLOW_rule__QualifiedName__Group_1__0__Impl_in_rule__QualifiedName__Group_1__071755);
rule__QualifiedName__Group_1__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets003.FOLLOW_rule__QualifiedName__Group_1__1_in_rule__QualifiedName__Group_1__071758);
rule__QualifiedName__Group_1__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__QualifiedName__Group_1__0"
// $ANTLR start "rule__QualifiedName__Group_1__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35734:1: rule__QualifiedName__Group_1__0__Impl : ( ( '.' ) ) ;
public final void rule__QualifiedName__Group_1__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35738:1: ( ( ( '.' ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35739:1: ( ( '.' ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35739:1: ( ( '.' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35740:1: ( '.' )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getQualifiedNameAccess().getFullStopKeyword_1_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35741:1: ( '.' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35742:2: '.'
{
match(input,43,FollowSets003.FOLLOW_43_in_rule__QualifiedName__Group_1__0__Impl71787); if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getQualifiedNameAccess().getFullStopKeyword_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__QualifiedName__Group_1__0__Impl"
// $ANTLR start "rule__QualifiedName__Group_1__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35753:1: rule__QualifiedName__Group_1__1 : rule__QualifiedName__Group_1__1__Impl ;
public final void rule__QualifiedName__Group_1__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35757:1: ( rule__QualifiedName__Group_1__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35758:2: rule__QualifiedName__Group_1__1__Impl
{
pushFollow(FollowSets003.FOLLOW_rule__QualifiedName__Group_1__1__Impl_in_rule__QualifiedName__Group_1__171819);
rule__QualifiedName__Group_1__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__QualifiedName__Group_1__1"
// $ANTLR start "rule__QualifiedName__Group_1__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35764:1: rule__QualifiedName__Group_1__1__Impl : ( ruleValidID ) ;
public final void rule__QualifiedName__Group_1__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35768:1: ( ( ruleValidID ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35769:1: ( ruleValidID )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35769:1: ( ruleValidID )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35770:1: ruleValidID
{
if ( state.backtracking==0 ) {
before(grammarAccess.getQualifiedNameAccess().getValidIDParserRuleCall_1_1());
}
pushFollow(FollowSets003.FOLLOW_ruleValidID_in_rule__QualifiedName__Group_1__1__Impl71846);
ruleValidID();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getQualifiedNameAccess().getValidIDParserRuleCall_1_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__QualifiedName__Group_1__1__Impl"
// $ANTLR start "rule__Number__Group_1__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35785:1: rule__Number__Group_1__0 : rule__Number__Group_1__0__Impl rule__Number__Group_1__1 ;
public final void rule__Number__Group_1__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35789:1: ( rule__Number__Group_1__0__Impl rule__Number__Group_1__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35790:2: rule__Number__Group_1__0__Impl rule__Number__Group_1__1
{
pushFollow(FollowSets003.FOLLOW_rule__Number__Group_1__0__Impl_in_rule__Number__Group_1__071879);
rule__Number__Group_1__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets003.FOLLOW_rule__Number__Group_1__1_in_rule__Number__Group_1__071882);
rule__Number__Group_1__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Number__Group_1__0"
// $ANTLR start "rule__Number__Group_1__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35797:1: rule__Number__Group_1__0__Impl : ( ( rule__Number__Alternatives_1_0 ) ) ;
public final void rule__Number__Group_1__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35801:1: ( ( ( rule__Number__Alternatives_1_0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35802:1: ( ( rule__Number__Alternatives_1_0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35802:1: ( ( rule__Number__Alternatives_1_0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35803:1: ( rule__Number__Alternatives_1_0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getNumberAccess().getAlternatives_1_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35804:1: ( rule__Number__Alternatives_1_0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35804:2: rule__Number__Alternatives_1_0
{
pushFollow(FollowSets003.FOLLOW_rule__Number__Alternatives_1_0_in_rule__Number__Group_1__0__Impl71909);
rule__Number__Alternatives_1_0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getNumberAccess().getAlternatives_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Number__Group_1__0__Impl"
// $ANTLR start "rule__Number__Group_1__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35814:1: rule__Number__Group_1__1 : rule__Number__Group_1__1__Impl ;
public final void rule__Number__Group_1__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35818:1: ( rule__Number__Group_1__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35819:2: rule__Number__Group_1__1__Impl
{
pushFollow(FollowSets003.FOLLOW_rule__Number__Group_1__1__Impl_in_rule__Number__Group_1__171939);
rule__Number__Group_1__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Number__Group_1__1"
// $ANTLR start "rule__Number__Group_1__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35825:1: rule__Number__Group_1__1__Impl : ( ( rule__Number__Group_1_1__0 )? ) ;
public final void rule__Number__Group_1__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35829:1: ( ( ( rule__Number__Group_1_1__0 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35830:1: ( ( rule__Number__Group_1_1__0 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35830:1: ( ( rule__Number__Group_1_1__0 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35831:1: ( rule__Number__Group_1_1__0 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getNumberAccess().getGroup_1_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35832:1: ( rule__Number__Group_1_1__0 )?
int alt257=2;
int LA257_0 = input.LA(1);
if ( (LA257_0==43) ) {
int LA257_1 = input.LA(2);
if ( ((LA257_1>=RULE_INT && LA257_1<=RULE_DECIMAL)) ) {
alt257=1;
}
}
switch (alt257) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35832:2: rule__Number__Group_1_1__0
{
pushFollow(FollowSets003.FOLLOW_rule__Number__Group_1_1__0_in_rule__Number__Group_1__1__Impl71966);
rule__Number__Group_1_1__0();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getNumberAccess().getGroup_1_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Number__Group_1__1__Impl"
// $ANTLR start "rule__Number__Group_1_1__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35846:1: rule__Number__Group_1_1__0 : rule__Number__Group_1_1__0__Impl rule__Number__Group_1_1__1 ;
public final void rule__Number__Group_1_1__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35850:1: ( rule__Number__Group_1_1__0__Impl rule__Number__Group_1_1__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35851:2: rule__Number__Group_1_1__0__Impl rule__Number__Group_1_1__1
{
pushFollow(FollowSets003.FOLLOW_rule__Number__Group_1_1__0__Impl_in_rule__Number__Group_1_1__072001);
rule__Number__Group_1_1__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets003.FOLLOW_rule__Number__Group_1_1__1_in_rule__Number__Group_1_1__072004);
rule__Number__Group_1_1__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Number__Group_1_1__0"
// $ANTLR start "rule__Number__Group_1_1__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35858:1: rule__Number__Group_1_1__0__Impl : ( '.' ) ;
public final void rule__Number__Group_1_1__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35862:1: ( ( '.' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35863:1: ( '.' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35863:1: ( '.' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35864:1: '.'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getNumberAccess().getFullStopKeyword_1_1_0());
}
match(input,43,FollowSets003.FOLLOW_43_in_rule__Number__Group_1_1__0__Impl72032); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getNumberAccess().getFullStopKeyword_1_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Number__Group_1_1__0__Impl"
// $ANTLR start "rule__Number__Group_1_1__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35877:1: rule__Number__Group_1_1__1 : rule__Number__Group_1_1__1__Impl ;
public final void rule__Number__Group_1_1__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35881:1: ( rule__Number__Group_1_1__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35882:2: rule__Number__Group_1_1__1__Impl
{
pushFollow(FollowSets003.FOLLOW_rule__Number__Group_1_1__1__Impl_in_rule__Number__Group_1_1__172063);
rule__Number__Group_1_1__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Number__Group_1_1__1"
// $ANTLR start "rule__Number__Group_1_1__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35888:1: rule__Number__Group_1_1__1__Impl : ( ( rule__Number__Alternatives_1_1_1 ) ) ;
public final void rule__Number__Group_1_1__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35892:1: ( ( ( rule__Number__Alternatives_1_1_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35893:1: ( ( rule__Number__Alternatives_1_1_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35893:1: ( ( rule__Number__Alternatives_1_1_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35894:1: ( rule__Number__Alternatives_1_1_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getNumberAccess().getAlternatives_1_1_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35895:1: ( rule__Number__Alternatives_1_1_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35895:2: rule__Number__Alternatives_1_1_1
{
pushFollow(FollowSets003.FOLLOW_rule__Number__Alternatives_1_1_1_in_rule__Number__Group_1_1__1__Impl72090);
rule__Number__Alternatives_1_1_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getNumberAccess().getAlternatives_1_1_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Number__Group_1_1__1__Impl"
// $ANTLR start "rule__JvmTypeReference__Group_0__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35910:1: rule__JvmTypeReference__Group_0__0 : rule__JvmTypeReference__Group_0__0__Impl rule__JvmTypeReference__Group_0__1 ;
public final void rule__JvmTypeReference__Group_0__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35914:1: ( rule__JvmTypeReference__Group_0__0__Impl rule__JvmTypeReference__Group_0__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35915:2: rule__JvmTypeReference__Group_0__0__Impl rule__JvmTypeReference__Group_0__1
{
pushFollow(FollowSets003.FOLLOW_rule__JvmTypeReference__Group_0__0__Impl_in_rule__JvmTypeReference__Group_0__072125);
rule__JvmTypeReference__Group_0__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets003.FOLLOW_rule__JvmTypeReference__Group_0__1_in_rule__JvmTypeReference__Group_0__072128);
rule__JvmTypeReference__Group_0__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__JvmTypeReference__Group_0__0"
// $ANTLR start "rule__JvmTypeReference__Group_0__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35922:1: rule__JvmTypeReference__Group_0__0__Impl : ( ruleJvmParameterizedTypeReference ) ;
public final void rule__JvmTypeReference__Group_0__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35926:1: ( ( ruleJvmParameterizedTypeReference ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35927:1: ( ruleJvmParameterizedTypeReference )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35927:1: ( ruleJvmParameterizedTypeReference )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35928:1: ruleJvmParameterizedTypeReference
{
if ( state.backtracking==0 ) {
before(grammarAccess.getJvmTypeReferenceAccess().getJvmParameterizedTypeReferenceParserRuleCall_0_0());
}
pushFollow(FollowSets003.FOLLOW_ruleJvmParameterizedTypeReference_in_rule__JvmTypeReference__Group_0__0__Impl72155);
ruleJvmParameterizedTypeReference();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getJvmTypeReferenceAccess().getJvmParameterizedTypeReferenceParserRuleCall_0_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__JvmTypeReference__Group_0__0__Impl"
// $ANTLR start "rule__JvmTypeReference__Group_0__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35939:1: rule__JvmTypeReference__Group_0__1 : rule__JvmTypeReference__Group_0__1__Impl ;
public final void rule__JvmTypeReference__Group_0__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35943:1: ( rule__JvmTypeReference__Group_0__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35944:2: rule__JvmTypeReference__Group_0__1__Impl
{
pushFollow(FollowSets003.FOLLOW_rule__JvmTypeReference__Group_0__1__Impl_in_rule__JvmTypeReference__Group_0__172184);
rule__JvmTypeReference__Group_0__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__JvmTypeReference__Group_0__1"
// $ANTLR start "rule__JvmTypeReference__Group_0__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35950:1: rule__JvmTypeReference__Group_0__1__Impl : ( ( rule__JvmTypeReference__Group_0_1__0 )* ) ;
public final void rule__JvmTypeReference__Group_0__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35954:1: ( ( ( rule__JvmTypeReference__Group_0_1__0 )* ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35955:1: ( ( rule__JvmTypeReference__Group_0_1__0 )* )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35955:1: ( ( rule__JvmTypeReference__Group_0_1__0 )* )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35956:1: ( rule__JvmTypeReference__Group_0_1__0 )*
{
if ( state.backtracking==0 ) {
before(grammarAccess.getJvmTypeReferenceAccess().getGroup_0_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35957:1: ( rule__JvmTypeReference__Group_0_1__0 )*
loop258:
do {
int alt258=2;
int LA258_0 = input.LA(1);
if ( (LA258_0==156) ) {
int LA258_2 = input.LA(2);
if ( (LA258_2==157) ) {
int LA258_3 = input.LA(3);
if ( (synpred344_InternalMango()) ) {
alt258=1;
}
}
}
switch (alt258) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35957:2: rule__JvmTypeReference__Group_0_1__0
{
pushFollow(FollowSets003.FOLLOW_rule__JvmTypeReference__Group_0_1__0_in_rule__JvmTypeReference__Group_0__1__Impl72211);
rule__JvmTypeReference__Group_0_1__0();
state._fsp--;
if (state.failed) return ;
}
break;
default :
break loop258;
}
} while (true);
if ( state.backtracking==0 ) {
after(grammarAccess.getJvmTypeReferenceAccess().getGroup_0_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__JvmTypeReference__Group_0__1__Impl"
// $ANTLR start "rule__JvmTypeReference__Group_0_1__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35971:1: rule__JvmTypeReference__Group_0_1__0 : rule__JvmTypeReference__Group_0_1__0__Impl ;
public final void rule__JvmTypeReference__Group_0_1__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35975:1: ( rule__JvmTypeReference__Group_0_1__0__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35976:2: rule__JvmTypeReference__Group_0_1__0__Impl
{
pushFollow(FollowSets003.FOLLOW_rule__JvmTypeReference__Group_0_1__0__Impl_in_rule__JvmTypeReference__Group_0_1__072246);
rule__JvmTypeReference__Group_0_1__0__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__JvmTypeReference__Group_0_1__0"
// $ANTLR start "rule__JvmTypeReference__Group_0_1__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35982:1: rule__JvmTypeReference__Group_0_1__0__Impl : ( ( rule__JvmTypeReference__Group_0_1_0__0 ) ) ;
public final void rule__JvmTypeReference__Group_0_1__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35986:1: ( ( ( rule__JvmTypeReference__Group_0_1_0__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35987:1: ( ( rule__JvmTypeReference__Group_0_1_0__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35987:1: ( ( rule__JvmTypeReference__Group_0_1_0__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35988:1: ( rule__JvmTypeReference__Group_0_1_0__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getJvmTypeReferenceAccess().getGroup_0_1_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35989:1: ( rule__JvmTypeReference__Group_0_1_0__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35989:2: rule__JvmTypeReference__Group_0_1_0__0
{
pushFollow(FollowSets003.FOLLOW_rule__JvmTypeReference__Group_0_1_0__0_in_rule__JvmTypeReference__Group_0_1__0__Impl72273);
rule__JvmTypeReference__Group_0_1_0__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getJvmTypeReferenceAccess().getGroup_0_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__JvmTypeReference__Group_0_1__0__Impl"
// $ANTLR start "rule__JvmTypeReference__Group_0_1_0__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36001:1: rule__JvmTypeReference__Group_0_1_0__0 : rule__JvmTypeReference__Group_0_1_0__0__Impl rule__JvmTypeReference__Group_0_1_0__1 ;
public final void rule__JvmTypeReference__Group_0_1_0__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36005:1: ( rule__JvmTypeReference__Group_0_1_0__0__Impl rule__JvmTypeReference__Group_0_1_0__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36006:2: rule__JvmTypeReference__Group_0_1_0__0__Impl rule__JvmTypeReference__Group_0_1_0__1
{
pushFollow(FollowSets003.FOLLOW_rule__JvmTypeReference__Group_0_1_0__0__Impl_in_rule__JvmTypeReference__Group_0_1_0__072305);
rule__JvmTypeReference__Group_0_1_0__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets003.FOLLOW_rule__JvmTypeReference__Group_0_1_0__1_in_rule__JvmTypeReference__Group_0_1_0__072308);
rule__JvmTypeReference__Group_0_1_0__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__JvmTypeReference__Group_0_1_0__0"
// $ANTLR start "rule__JvmTypeReference__Group_0_1_0__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36013:1: rule__JvmTypeReference__Group_0_1_0__0__Impl : ( () ) ;
public final void rule__JvmTypeReference__Group_0_1_0__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36017:1: ( ( () ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36018:1: ( () )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36018:1: ( () )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36019:1: ()
{
if ( state.backtracking==0 ) {
before(grammarAccess.getJvmTypeReferenceAccess().getJvmGenericArrayTypeReferenceComponentTypeAction_0_1_0_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36020:1: ()
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36022:1:
{
}
if ( state.backtracking==0 ) {
after(grammarAccess.getJvmTypeReferenceAccess().getJvmGenericArrayTypeReferenceComponentTypeAction_0_1_0_0());
}
}
}
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__JvmTypeReference__Group_0_1_0__0__Impl"
// $ANTLR start "rule__JvmTypeReference__Group_0_1_0__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36032:1: rule__JvmTypeReference__Group_0_1_0__1 : rule__JvmTypeReference__Group_0_1_0__1__Impl ;
public final void rule__JvmTypeReference__Group_0_1_0__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36036:1: ( rule__JvmTypeReference__Group_0_1_0__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36037:2: rule__JvmTypeReference__Group_0_1_0__1__Impl
{
pushFollow(FollowSets003.FOLLOW_rule__JvmTypeReference__Group_0_1_0__1__Impl_in_rule__JvmTypeReference__Group_0_1_0__172366);
rule__JvmTypeReference__Group_0_1_0__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__JvmTypeReference__Group_0_1_0__1"
// $ANTLR start "rule__JvmTypeReference__Group_0_1_0__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36043:1: rule__JvmTypeReference__Group_0_1_0__1__Impl : ( ruleArrayBrackets ) ;
public final void rule__JvmTypeReference__Group_0_1_0__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36047:1: ( ( ruleArrayBrackets ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36048:1: ( ruleArrayBrackets )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36048:1: ( ruleArrayBrackets )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36049:1: ruleArrayBrackets
{
if ( state.backtracking==0 ) {
before(grammarAccess.getJvmTypeReferenceAccess().getArrayBracketsParserRuleCall_0_1_0_1());
}
pushFollow(FollowSets003.FOLLOW_ruleArrayBrackets_in_rule__JvmTypeReference__Group_0_1_0__1__Impl72393);
ruleArrayBrackets();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getJvmTypeReferenceAccess().getArrayBracketsParserRuleCall_0_1_0_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__JvmTypeReference__Group_0_1_0__1__Impl"
// $ANTLR start "rule__ArrayBrackets__Group__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36064:1: rule__ArrayBrackets__Group__0 : rule__ArrayBrackets__Group__0__Impl rule__ArrayBrackets__Group__1 ;
public final void rule__ArrayBrackets__Group__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36068:1: ( rule__ArrayBrackets__Group__0__Impl rule__ArrayBrackets__Group__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36069:2: rule__ArrayBrackets__Group__0__Impl rule__ArrayBrackets__Group__1
{
pushFollow(FollowSets003.FOLLOW_rule__ArrayBrackets__Group__0__Impl_in_rule__ArrayBrackets__Group__072426);
rule__ArrayBrackets__Group__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets003.FOLLOW_rule__ArrayBrackets__Group__1_in_rule__ArrayBrackets__Group__072429);
rule__ArrayBrackets__Group__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ArrayBrackets__Group__0"
// $ANTLR start "rule__ArrayBrackets__Group__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36076:1: rule__ArrayBrackets__Group__0__Impl : ( '[' ) ;
public final void rule__ArrayBrackets__Group__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36080:1: ( ( '[' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36081:1: ( '[' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36081:1: ( '[' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36082:1: '['
{
if ( state.backtracking==0 ) {
before(grammarAccess.getArrayBracketsAccess().getLeftSquareBracketKeyword_0());
}
match(input,156,FollowSets003.FOLLOW_156_in_rule__ArrayBrackets__Group__0__Impl72457); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getArrayBracketsAccess().getLeftSquareBracketKeyword_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ArrayBrackets__Group__0__Impl"
// $ANTLR start "rule__ArrayBrackets__Group__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36095:1: rule__ArrayBrackets__Group__1 : rule__ArrayBrackets__Group__1__Impl ;
public final void rule__ArrayBrackets__Group__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36099:1: ( rule__ArrayBrackets__Group__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36100:2: rule__ArrayBrackets__Group__1__Impl
{
pushFollow(FollowSets003.FOLLOW_rule__ArrayBrackets__Group__1__Impl_in_rule__ArrayBrackets__Group__172488);
rule__ArrayBrackets__Group__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ArrayBrackets__Group__1"
// $ANTLR start "rule__ArrayBrackets__Group__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36106:1: rule__ArrayBrackets__Group__1__Impl : ( ']' ) ;
public final void rule__ArrayBrackets__Group__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36110:1: ( ( ']' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36111:1: ( ']' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36111:1: ( ']' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36112:1: ']'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getArrayBracketsAccess().getRightSquareBracketKeyword_1());
}
match(input,157,FollowSets003.FOLLOW_157_in_rule__ArrayBrackets__Group__1__Impl72516); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getArrayBracketsAccess().getRightSquareBracketKeyword_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ArrayBrackets__Group__1__Impl"
// $ANTLR start "rule__XFunctionTypeRef__Group__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36129:1: rule__XFunctionTypeRef__Group__0 : rule__XFunctionTypeRef__Group__0__Impl rule__XFunctionTypeRef__Group__1 ;
public final void rule__XFunctionTypeRef__Group__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36133:1: ( rule__XFunctionTypeRef__Group__0__Impl rule__XFunctionTypeRef__Group__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36134:2: rule__XFunctionTypeRef__Group__0__Impl rule__XFunctionTypeRef__Group__1
{
pushFollow(FollowSets003.FOLLOW_rule__XFunctionTypeRef__Group__0__Impl_in_rule__XFunctionTypeRef__Group__072551);
rule__XFunctionTypeRef__Group__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets003.FOLLOW_rule__XFunctionTypeRef__Group__1_in_rule__XFunctionTypeRef__Group__072554);
rule__XFunctionTypeRef__Group__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XFunctionTypeRef__Group__0"
// $ANTLR start "rule__XFunctionTypeRef__Group__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36141:1: rule__XFunctionTypeRef__Group__0__Impl : ( ( rule__XFunctionTypeRef__Group_0__0 )? ) ;
public final void rule__XFunctionTypeRef__Group__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36145:1: ( ( ( rule__XFunctionTypeRef__Group_0__0 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36146:1: ( ( rule__XFunctionTypeRef__Group_0__0 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36146:1: ( ( rule__XFunctionTypeRef__Group_0__0 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36147:1: ( rule__XFunctionTypeRef__Group_0__0 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXFunctionTypeRefAccess().getGroup_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36148:1: ( rule__XFunctionTypeRef__Group_0__0 )?
int alt259=2;
int LA259_0 = input.LA(1);
if ( (LA259_0==144) ) {
alt259=1;
}
switch (alt259) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36148:2: rule__XFunctionTypeRef__Group_0__0
{
pushFollow(FollowSets003.FOLLOW_rule__XFunctionTypeRef__Group_0__0_in_rule__XFunctionTypeRef__Group__0__Impl72581);
rule__XFunctionTypeRef__Group_0__0();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXFunctionTypeRefAccess().getGroup_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XFunctionTypeRef__Group__0__Impl"
// $ANTLR start "rule__XFunctionTypeRef__Group__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36158:1: rule__XFunctionTypeRef__Group__1 : rule__XFunctionTypeRef__Group__1__Impl rule__XFunctionTypeRef__Group__2 ;
public final void rule__XFunctionTypeRef__Group__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36162:1: ( rule__XFunctionTypeRef__Group__1__Impl rule__XFunctionTypeRef__Group__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36163:2: rule__XFunctionTypeRef__Group__1__Impl rule__XFunctionTypeRef__Group__2
{
pushFollow(FollowSets003.FOLLOW_rule__XFunctionTypeRef__Group__1__Impl_in_rule__XFunctionTypeRef__Group__172612);
rule__XFunctionTypeRef__Group__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets003.FOLLOW_rule__XFunctionTypeRef__Group__2_in_rule__XFunctionTypeRef__Group__172615);
rule__XFunctionTypeRef__Group__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XFunctionTypeRef__Group__1"
// $ANTLR start "rule__XFunctionTypeRef__Group__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36170:1: rule__XFunctionTypeRef__Group__1__Impl : ( '=>' ) ;
public final void rule__XFunctionTypeRef__Group__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36174:1: ( ( '=>' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36175:1: ( '=>' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36175:1: ( '=>' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36176:1: '=>'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXFunctionTypeRefAccess().getEqualsSignGreaterThanSignKeyword_1());
}
match(input,31,FollowSets003.FOLLOW_31_in_rule__XFunctionTypeRef__Group__1__Impl72643); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXFunctionTypeRefAccess().getEqualsSignGreaterThanSignKeyword_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XFunctionTypeRef__Group__1__Impl"
// $ANTLR start "rule__XFunctionTypeRef__Group__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36189:1: rule__XFunctionTypeRef__Group__2 : rule__XFunctionTypeRef__Group__2__Impl ;
public final void rule__XFunctionTypeRef__Group__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36193:1: ( rule__XFunctionTypeRef__Group__2__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36194:2: rule__XFunctionTypeRef__Group__2__Impl
{
pushFollow(FollowSets003.FOLLOW_rule__XFunctionTypeRef__Group__2__Impl_in_rule__XFunctionTypeRef__Group__272674);
rule__XFunctionTypeRef__Group__2__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XFunctionTypeRef__Group__2"
// $ANTLR start "rule__XFunctionTypeRef__Group__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36200:1: rule__XFunctionTypeRef__Group__2__Impl : ( ( rule__XFunctionTypeRef__ReturnTypeAssignment_2 ) ) ;
public final void rule__XFunctionTypeRef__Group__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36204:1: ( ( ( rule__XFunctionTypeRef__ReturnTypeAssignment_2 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36205:1: ( ( rule__XFunctionTypeRef__ReturnTypeAssignment_2 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36205:1: ( ( rule__XFunctionTypeRef__ReturnTypeAssignment_2 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36206:1: ( rule__XFunctionTypeRef__ReturnTypeAssignment_2 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXFunctionTypeRefAccess().getReturnTypeAssignment_2());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36207:1: ( rule__XFunctionTypeRef__ReturnTypeAssignment_2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36207:2: rule__XFunctionTypeRef__ReturnTypeAssignment_2
{
pushFollow(FollowSets003.FOLLOW_rule__XFunctionTypeRef__ReturnTypeAssignment_2_in_rule__XFunctionTypeRef__Group__2__Impl72701);
rule__XFunctionTypeRef__ReturnTypeAssignment_2();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXFunctionTypeRefAccess().getReturnTypeAssignment_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XFunctionTypeRef__Group__2__Impl"
// $ANTLR start "rule__XFunctionTypeRef__Group_0__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36223:1: rule__XFunctionTypeRef__Group_0__0 : rule__XFunctionTypeRef__Group_0__0__Impl rule__XFunctionTypeRef__Group_0__1 ;
public final void rule__XFunctionTypeRef__Group_0__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36227:1: ( rule__XFunctionTypeRef__Group_0__0__Impl rule__XFunctionTypeRef__Group_0__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36228:2: rule__XFunctionTypeRef__Group_0__0__Impl rule__XFunctionTypeRef__Group_0__1
{
pushFollow(FollowSets003.FOLLOW_rule__XFunctionTypeRef__Group_0__0__Impl_in_rule__XFunctionTypeRef__Group_0__072737);
rule__XFunctionTypeRef__Group_0__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets003.FOLLOW_rule__XFunctionTypeRef__Group_0__1_in_rule__XFunctionTypeRef__Group_0__072740);
rule__XFunctionTypeRef__Group_0__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XFunctionTypeRef__Group_0__0"
// $ANTLR start "rule__XFunctionTypeRef__Group_0__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36235:1: rule__XFunctionTypeRef__Group_0__0__Impl : ( '(' ) ;
public final void rule__XFunctionTypeRef__Group_0__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36239:1: ( ( '(' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36240:1: ( '(' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36240:1: ( '(' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36241:1: '('
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXFunctionTypeRefAccess().getLeftParenthesisKeyword_0_0());
}
match(input,144,FollowSets003.FOLLOW_144_in_rule__XFunctionTypeRef__Group_0__0__Impl72768); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXFunctionTypeRefAccess().getLeftParenthesisKeyword_0_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XFunctionTypeRef__Group_0__0__Impl"
// $ANTLR start "rule__XFunctionTypeRef__Group_0__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36254:1: rule__XFunctionTypeRef__Group_0__1 : rule__XFunctionTypeRef__Group_0__1__Impl rule__XFunctionTypeRef__Group_0__2 ;
public final void rule__XFunctionTypeRef__Group_0__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36258:1: ( rule__XFunctionTypeRef__Group_0__1__Impl rule__XFunctionTypeRef__Group_0__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36259:2: rule__XFunctionTypeRef__Group_0__1__Impl rule__XFunctionTypeRef__Group_0__2
{
pushFollow(FollowSets003.FOLLOW_rule__XFunctionTypeRef__Group_0__1__Impl_in_rule__XFunctionTypeRef__Group_0__172799);
rule__XFunctionTypeRef__Group_0__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets003.FOLLOW_rule__XFunctionTypeRef__Group_0__2_in_rule__XFunctionTypeRef__Group_0__172802);
rule__XFunctionTypeRef__Group_0__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XFunctionTypeRef__Group_0__1"
// $ANTLR start "rule__XFunctionTypeRef__Group_0__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36266:1: rule__XFunctionTypeRef__Group_0__1__Impl : ( ( rule__XFunctionTypeRef__Group_0_1__0 )? ) ;
public final void rule__XFunctionTypeRef__Group_0__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36270:1: ( ( ( rule__XFunctionTypeRef__Group_0_1__0 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36271:1: ( ( rule__XFunctionTypeRef__Group_0_1__0 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36271:1: ( ( rule__XFunctionTypeRef__Group_0_1__0 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36272:1: ( rule__XFunctionTypeRef__Group_0_1__0 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXFunctionTypeRefAccess().getGroup_0_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36273:1: ( rule__XFunctionTypeRef__Group_0_1__0 )?
int alt260=2;
int LA260_0 = input.LA(1);
if ( (LA260_0==RULE_ID||LA260_0==31||LA260_0==144) ) {
alt260=1;
}
switch (alt260) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36273:2: rule__XFunctionTypeRef__Group_0_1__0
{
pushFollow(FollowSets003.FOLLOW_rule__XFunctionTypeRef__Group_0_1__0_in_rule__XFunctionTypeRef__Group_0__1__Impl72829);
rule__XFunctionTypeRef__Group_0_1__0();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXFunctionTypeRefAccess().getGroup_0_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XFunctionTypeRef__Group_0__1__Impl"
// $ANTLR start "rule__XFunctionTypeRef__Group_0__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36283:1: rule__XFunctionTypeRef__Group_0__2 : rule__XFunctionTypeRef__Group_0__2__Impl ;
public final void rule__XFunctionTypeRef__Group_0__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36287:1: ( rule__XFunctionTypeRef__Group_0__2__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36288:2: rule__XFunctionTypeRef__Group_0__2__Impl
{
pushFollow(FollowSets003.FOLLOW_rule__XFunctionTypeRef__Group_0__2__Impl_in_rule__XFunctionTypeRef__Group_0__272860);
rule__XFunctionTypeRef__Group_0__2__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XFunctionTypeRef__Group_0__2"
// $ANTLR start "rule__XFunctionTypeRef__Group_0__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36294:1: rule__XFunctionTypeRef__Group_0__2__Impl : ( ')' ) ;
public final void rule__XFunctionTypeRef__Group_0__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36298:1: ( ( ')' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36299:1: ( ')' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36299:1: ( ')' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36300:1: ')'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXFunctionTypeRefAccess().getRightParenthesisKeyword_0_2());
}
match(input,145,FollowSets003.FOLLOW_145_in_rule__XFunctionTypeRef__Group_0__2__Impl72888); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXFunctionTypeRefAccess().getRightParenthesisKeyword_0_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XFunctionTypeRef__Group_0__2__Impl"
// $ANTLR start "rule__XFunctionTypeRef__Group_0_1__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36319:1: rule__XFunctionTypeRef__Group_0_1__0 : rule__XFunctionTypeRef__Group_0_1__0__Impl rule__XFunctionTypeRef__Group_0_1__1 ;
public final void rule__XFunctionTypeRef__Group_0_1__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36323:1: ( rule__XFunctionTypeRef__Group_0_1__0__Impl rule__XFunctionTypeRef__Group_0_1__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36324:2: rule__XFunctionTypeRef__Group_0_1__0__Impl rule__XFunctionTypeRef__Group_0_1__1
{
pushFollow(FollowSets003.FOLLOW_rule__XFunctionTypeRef__Group_0_1__0__Impl_in_rule__XFunctionTypeRef__Group_0_1__072925);
rule__XFunctionTypeRef__Group_0_1__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets003.FOLLOW_rule__XFunctionTypeRef__Group_0_1__1_in_rule__XFunctionTypeRef__Group_0_1__072928);
rule__XFunctionTypeRef__Group_0_1__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XFunctionTypeRef__Group_0_1__0"
// $ANTLR start "rule__XFunctionTypeRef__Group_0_1__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36331:1: rule__XFunctionTypeRef__Group_0_1__0__Impl : ( ( rule__XFunctionTypeRef__ParamTypesAssignment_0_1_0 ) ) ;
public final void rule__XFunctionTypeRef__Group_0_1__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36335:1: ( ( ( rule__XFunctionTypeRef__ParamTypesAssignment_0_1_0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36336:1: ( ( rule__XFunctionTypeRef__ParamTypesAssignment_0_1_0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36336:1: ( ( rule__XFunctionTypeRef__ParamTypesAssignment_0_1_0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36337:1: ( rule__XFunctionTypeRef__ParamTypesAssignment_0_1_0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXFunctionTypeRefAccess().getParamTypesAssignment_0_1_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36338:1: ( rule__XFunctionTypeRef__ParamTypesAssignment_0_1_0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36338:2: rule__XFunctionTypeRef__ParamTypesAssignment_0_1_0
{
pushFollow(FollowSets003.FOLLOW_rule__XFunctionTypeRef__ParamTypesAssignment_0_1_0_in_rule__XFunctionTypeRef__Group_0_1__0__Impl72955);
rule__XFunctionTypeRef__ParamTypesAssignment_0_1_0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXFunctionTypeRefAccess().getParamTypesAssignment_0_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XFunctionTypeRef__Group_0_1__0__Impl"
// $ANTLR start "rule__XFunctionTypeRef__Group_0_1__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36348:1: rule__XFunctionTypeRef__Group_0_1__1 : rule__XFunctionTypeRef__Group_0_1__1__Impl ;
public final void rule__XFunctionTypeRef__Group_0_1__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36352:1: ( rule__XFunctionTypeRef__Group_0_1__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36353:2: rule__XFunctionTypeRef__Group_0_1__1__Impl
{
pushFollow(FollowSets003.FOLLOW_rule__XFunctionTypeRef__Group_0_1__1__Impl_in_rule__XFunctionTypeRef__Group_0_1__172985);
rule__XFunctionTypeRef__Group_0_1__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XFunctionTypeRef__Group_0_1__1"
// $ANTLR start "rule__XFunctionTypeRef__Group_0_1__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36359:1: rule__XFunctionTypeRef__Group_0_1__1__Impl : ( ( rule__XFunctionTypeRef__Group_0_1_1__0 )* ) ;
public final void rule__XFunctionTypeRef__Group_0_1__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36363:1: ( ( ( rule__XFunctionTypeRef__Group_0_1_1__0 )* ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36364:1: ( ( rule__XFunctionTypeRef__Group_0_1_1__0 )* )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36364:1: ( ( rule__XFunctionTypeRef__Group_0_1_1__0 )* )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36365:1: ( rule__XFunctionTypeRef__Group_0_1_1__0 )*
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXFunctionTypeRefAccess().getGroup_0_1_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36366:1: ( rule__XFunctionTypeRef__Group_0_1_1__0 )*
loop261:
do {
int alt261=2;
int LA261_0 = input.LA(1);
if ( (LA261_0==154) ) {
alt261=1;
}
switch (alt261) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36366:2: rule__XFunctionTypeRef__Group_0_1_1__0
{
pushFollow(FollowSets003.FOLLOW_rule__XFunctionTypeRef__Group_0_1_1__0_in_rule__XFunctionTypeRef__Group_0_1__1__Impl73012);
rule__XFunctionTypeRef__Group_0_1_1__0();
state._fsp--;
if (state.failed) return ;
}
break;
default :
break loop261;
}
} while (true);
if ( state.backtracking==0 ) {
after(grammarAccess.getXFunctionTypeRefAccess().getGroup_0_1_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XFunctionTypeRef__Group_0_1__1__Impl"
// $ANTLR start "rule__XFunctionTypeRef__Group_0_1_1__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36380:1: rule__XFunctionTypeRef__Group_0_1_1__0 : rule__XFunctionTypeRef__Group_0_1_1__0__Impl rule__XFunctionTypeRef__Group_0_1_1__1 ;
public final void rule__XFunctionTypeRef__Group_0_1_1__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36384:1: ( rule__XFunctionTypeRef__Group_0_1_1__0__Impl rule__XFunctionTypeRef__Group_0_1_1__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36385:2: rule__XFunctionTypeRef__Group_0_1_1__0__Impl rule__XFunctionTypeRef__Group_0_1_1__1
{
pushFollow(FollowSets003.FOLLOW_rule__XFunctionTypeRef__Group_0_1_1__0__Impl_in_rule__XFunctionTypeRef__Group_0_1_1__073047);
rule__XFunctionTypeRef__Group_0_1_1__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets003.FOLLOW_rule__XFunctionTypeRef__Group_0_1_1__1_in_rule__XFunctionTypeRef__Group_0_1_1__073050);
rule__XFunctionTypeRef__Group_0_1_1__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XFunctionTypeRef__Group_0_1_1__0"
// $ANTLR start "rule__XFunctionTypeRef__Group_0_1_1__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36392:1: rule__XFunctionTypeRef__Group_0_1_1__0__Impl : ( ',' ) ;
public final void rule__XFunctionTypeRef__Group_0_1_1__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36396:1: ( ( ',' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36397:1: ( ',' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36397:1: ( ',' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36398:1: ','
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXFunctionTypeRefAccess().getCommaKeyword_0_1_1_0());
}
match(input,154,FollowSets003.FOLLOW_154_in_rule__XFunctionTypeRef__Group_0_1_1__0__Impl73078); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXFunctionTypeRefAccess().getCommaKeyword_0_1_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XFunctionTypeRef__Group_0_1_1__0__Impl"
// $ANTLR start "rule__XFunctionTypeRef__Group_0_1_1__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36411:1: rule__XFunctionTypeRef__Group_0_1_1__1 : rule__XFunctionTypeRef__Group_0_1_1__1__Impl ;
public final void rule__XFunctionTypeRef__Group_0_1_1__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36415:1: ( rule__XFunctionTypeRef__Group_0_1_1__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36416:2: rule__XFunctionTypeRef__Group_0_1_1__1__Impl
{
pushFollow(FollowSets003.FOLLOW_rule__XFunctionTypeRef__Group_0_1_1__1__Impl_in_rule__XFunctionTypeRef__Group_0_1_1__173109);
rule__XFunctionTypeRef__Group_0_1_1__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XFunctionTypeRef__Group_0_1_1__1"
// $ANTLR start "rule__XFunctionTypeRef__Group_0_1_1__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36422:1: rule__XFunctionTypeRef__Group_0_1_1__1__Impl : ( ( rule__XFunctionTypeRef__ParamTypesAssignment_0_1_1_1 ) ) ;
public final void rule__XFunctionTypeRef__Group_0_1_1__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36426:1: ( ( ( rule__XFunctionTypeRef__ParamTypesAssignment_0_1_1_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36427:1: ( ( rule__XFunctionTypeRef__ParamTypesAssignment_0_1_1_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36427:1: ( ( rule__XFunctionTypeRef__ParamTypesAssignment_0_1_1_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36428:1: ( rule__XFunctionTypeRef__ParamTypesAssignment_0_1_1_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXFunctionTypeRefAccess().getParamTypesAssignment_0_1_1_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36429:1: ( rule__XFunctionTypeRef__ParamTypesAssignment_0_1_1_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36429:2: rule__XFunctionTypeRef__ParamTypesAssignment_0_1_1_1
{
pushFollow(FollowSets003.FOLLOW_rule__XFunctionTypeRef__ParamTypesAssignment_0_1_1_1_in_rule__XFunctionTypeRef__Group_0_1_1__1__Impl73136);
rule__XFunctionTypeRef__ParamTypesAssignment_0_1_1_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXFunctionTypeRefAccess().getParamTypesAssignment_0_1_1_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XFunctionTypeRef__Group_0_1_1__1__Impl"
// $ANTLR start "rule__JvmParameterizedTypeReference__Group__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36443:1: rule__JvmParameterizedTypeReference__Group__0 : rule__JvmParameterizedTypeReference__Group__0__Impl rule__JvmParameterizedTypeReference__Group__1 ;
public final void rule__JvmParameterizedTypeReference__Group__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36447:1: ( rule__JvmParameterizedTypeReference__Group__0__Impl rule__JvmParameterizedTypeReference__Group__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36448:2: rule__JvmParameterizedTypeReference__Group__0__Impl rule__JvmParameterizedTypeReference__Group__1
{
pushFollow(FollowSets003.FOLLOW_rule__JvmParameterizedTypeReference__Group__0__Impl_in_rule__JvmParameterizedTypeReference__Group__073170);
rule__JvmParameterizedTypeReference__Group__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets003.FOLLOW_rule__JvmParameterizedTypeReference__Group__1_in_rule__JvmParameterizedTypeReference__Group__073173);
rule__JvmParameterizedTypeReference__Group__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__JvmParameterizedTypeReference__Group__0"
// $ANTLR start "rule__JvmParameterizedTypeReference__Group__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36455:1: rule__JvmParameterizedTypeReference__Group__0__Impl : ( ( rule__JvmParameterizedTypeReference__TypeAssignment_0 ) ) ;
public final void rule__JvmParameterizedTypeReference__Group__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36459:1: ( ( ( rule__JvmParameterizedTypeReference__TypeAssignment_0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36460:1: ( ( rule__JvmParameterizedTypeReference__TypeAssignment_0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36460:1: ( ( rule__JvmParameterizedTypeReference__TypeAssignment_0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36461:1: ( rule__JvmParameterizedTypeReference__TypeAssignment_0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getJvmParameterizedTypeReferenceAccess().getTypeAssignment_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36462:1: ( rule__JvmParameterizedTypeReference__TypeAssignment_0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36462:2: rule__JvmParameterizedTypeReference__TypeAssignment_0
{
pushFollow(FollowSets003.FOLLOW_rule__JvmParameterizedTypeReference__TypeAssignment_0_in_rule__JvmParameterizedTypeReference__Group__0__Impl73200);
rule__JvmParameterizedTypeReference__TypeAssignment_0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getJvmParameterizedTypeReferenceAccess().getTypeAssignment_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__JvmParameterizedTypeReference__Group__0__Impl"
// $ANTLR start "rule__JvmParameterizedTypeReference__Group__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36472:1: rule__JvmParameterizedTypeReference__Group__1 : rule__JvmParameterizedTypeReference__Group__1__Impl ;
public final void rule__JvmParameterizedTypeReference__Group__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36476:1: ( rule__JvmParameterizedTypeReference__Group__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36477:2: rule__JvmParameterizedTypeReference__Group__1__Impl
{
pushFollow(FollowSets003.FOLLOW_rule__JvmParameterizedTypeReference__Group__1__Impl_in_rule__JvmParameterizedTypeReference__Group__173230);
rule__JvmParameterizedTypeReference__Group__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__JvmParameterizedTypeReference__Group__1"
// $ANTLR start "rule__JvmParameterizedTypeReference__Group__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36483:1: rule__JvmParameterizedTypeReference__Group__1__Impl : ( ( rule__JvmParameterizedTypeReference__Group_1__0 )? ) ;
public final void rule__JvmParameterizedTypeReference__Group__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36487:1: ( ( ( rule__JvmParameterizedTypeReference__Group_1__0 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36488:1: ( ( rule__JvmParameterizedTypeReference__Group_1__0 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36488:1: ( ( rule__JvmParameterizedTypeReference__Group_1__0 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36489:1: ( rule__JvmParameterizedTypeReference__Group_1__0 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getJvmParameterizedTypeReferenceAccess().getGroup_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36490:1: ( rule__JvmParameterizedTypeReference__Group_1__0 )?
int alt262=2;
alt262 = dfa262.predict(input);
switch (alt262) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36490:2: rule__JvmParameterizedTypeReference__Group_1__0
{
pushFollow(FollowSets003.FOLLOW_rule__JvmParameterizedTypeReference__Group_1__0_in_rule__JvmParameterizedTypeReference__Group__1__Impl73257);
rule__JvmParameterizedTypeReference__Group_1__0();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getJvmParameterizedTypeReferenceAccess().getGroup_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__JvmParameterizedTypeReference__Group__1__Impl"
// $ANTLR start "rule__JvmParameterizedTypeReference__Group_1__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36504:1: rule__JvmParameterizedTypeReference__Group_1__0 : rule__JvmParameterizedTypeReference__Group_1__0__Impl rule__JvmParameterizedTypeReference__Group_1__1 ;
public final void rule__JvmParameterizedTypeReference__Group_1__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36508:1: ( rule__JvmParameterizedTypeReference__Group_1__0__Impl rule__JvmParameterizedTypeReference__Group_1__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36509:2: rule__JvmParameterizedTypeReference__Group_1__0__Impl rule__JvmParameterizedTypeReference__Group_1__1
{
pushFollow(FollowSets003.FOLLOW_rule__JvmParameterizedTypeReference__Group_1__0__Impl_in_rule__JvmParameterizedTypeReference__Group_1__073292);
rule__JvmParameterizedTypeReference__Group_1__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets003.FOLLOW_rule__JvmParameterizedTypeReference__Group_1__1_in_rule__JvmParameterizedTypeReference__Group_1__073295);
rule__JvmParameterizedTypeReference__Group_1__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__JvmParameterizedTypeReference__Group_1__0"
// $ANTLR start "rule__JvmParameterizedTypeReference__Group_1__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36516:1: rule__JvmParameterizedTypeReference__Group_1__0__Impl : ( ( '<' ) ) ;
public final void rule__JvmParameterizedTypeReference__Group_1__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36520:1: ( ( ( '<' ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36521:1: ( ( '<' ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36521:1: ( ( '<' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36522:1: ( '<' )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getJvmParameterizedTypeReferenceAccess().getLessThanSignKeyword_1_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36523:1: ( '<' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36524:2: '<'
{
match(input,27,FollowSets003.FOLLOW_27_in_rule__JvmParameterizedTypeReference__Group_1__0__Impl73324); if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getJvmParameterizedTypeReferenceAccess().getLessThanSignKeyword_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__JvmParameterizedTypeReference__Group_1__0__Impl"
// $ANTLR start "rule__JvmParameterizedTypeReference__Group_1__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36535:1: rule__JvmParameterizedTypeReference__Group_1__1 : rule__JvmParameterizedTypeReference__Group_1__1__Impl rule__JvmParameterizedTypeReference__Group_1__2 ;
public final void rule__JvmParameterizedTypeReference__Group_1__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36539:1: ( rule__JvmParameterizedTypeReference__Group_1__1__Impl rule__JvmParameterizedTypeReference__Group_1__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36540:2: rule__JvmParameterizedTypeReference__Group_1__1__Impl rule__JvmParameterizedTypeReference__Group_1__2
{
pushFollow(FollowSets003.FOLLOW_rule__JvmParameterizedTypeReference__Group_1__1__Impl_in_rule__JvmParameterizedTypeReference__Group_1__173356);
rule__JvmParameterizedTypeReference__Group_1__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets003.FOLLOW_rule__JvmParameterizedTypeReference__Group_1__2_in_rule__JvmParameterizedTypeReference__Group_1__173359);
rule__JvmParameterizedTypeReference__Group_1__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__JvmParameterizedTypeReference__Group_1__1"
// $ANTLR start "rule__JvmParameterizedTypeReference__Group_1__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36547:1: rule__JvmParameterizedTypeReference__Group_1__1__Impl : ( ( rule__JvmParameterizedTypeReference__ArgumentsAssignment_1_1 ) ) ;
public final void rule__JvmParameterizedTypeReference__Group_1__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36551:1: ( ( ( rule__JvmParameterizedTypeReference__ArgumentsAssignment_1_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36552:1: ( ( rule__JvmParameterizedTypeReference__ArgumentsAssignment_1_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36552:1: ( ( rule__JvmParameterizedTypeReference__ArgumentsAssignment_1_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36553:1: ( rule__JvmParameterizedTypeReference__ArgumentsAssignment_1_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getJvmParameterizedTypeReferenceAccess().getArgumentsAssignment_1_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36554:1: ( rule__JvmParameterizedTypeReference__ArgumentsAssignment_1_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36554:2: rule__JvmParameterizedTypeReference__ArgumentsAssignment_1_1
{
pushFollow(FollowSets003.FOLLOW_rule__JvmParameterizedTypeReference__ArgumentsAssignment_1_1_in_rule__JvmParameterizedTypeReference__Group_1__1__Impl73386);
rule__JvmParameterizedTypeReference__ArgumentsAssignment_1_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getJvmParameterizedTypeReferenceAccess().getArgumentsAssignment_1_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__JvmParameterizedTypeReference__Group_1__1__Impl"
// $ANTLR start "rule__JvmParameterizedTypeReference__Group_1__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36564:1: rule__JvmParameterizedTypeReference__Group_1__2 : rule__JvmParameterizedTypeReference__Group_1__2__Impl rule__JvmParameterizedTypeReference__Group_1__3 ;
public final void rule__JvmParameterizedTypeReference__Group_1__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36568:1: ( rule__JvmParameterizedTypeReference__Group_1__2__Impl rule__JvmParameterizedTypeReference__Group_1__3 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36569:2: rule__JvmParameterizedTypeReference__Group_1__2__Impl rule__JvmParameterizedTypeReference__Group_1__3
{
pushFollow(FollowSets003.FOLLOW_rule__JvmParameterizedTypeReference__Group_1__2__Impl_in_rule__JvmParameterizedTypeReference__Group_1__273416);
rule__JvmParameterizedTypeReference__Group_1__2__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets003.FOLLOW_rule__JvmParameterizedTypeReference__Group_1__3_in_rule__JvmParameterizedTypeReference__Group_1__273419);
rule__JvmParameterizedTypeReference__Group_1__3();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__JvmParameterizedTypeReference__Group_1__2"
// $ANTLR start "rule__JvmParameterizedTypeReference__Group_1__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36576:1: rule__JvmParameterizedTypeReference__Group_1__2__Impl : ( ( rule__JvmParameterizedTypeReference__Group_1_2__0 )* ) ;
public final void rule__JvmParameterizedTypeReference__Group_1__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36580:1: ( ( ( rule__JvmParameterizedTypeReference__Group_1_2__0 )* ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36581:1: ( ( rule__JvmParameterizedTypeReference__Group_1_2__0 )* )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36581:1: ( ( rule__JvmParameterizedTypeReference__Group_1_2__0 )* )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36582:1: ( rule__JvmParameterizedTypeReference__Group_1_2__0 )*
{
if ( state.backtracking==0 ) {
before(grammarAccess.getJvmParameterizedTypeReferenceAccess().getGroup_1_2());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36583:1: ( rule__JvmParameterizedTypeReference__Group_1_2__0 )*
loop263:
do {
int alt263=2;
int LA263_0 = input.LA(1);
if ( (LA263_0==154) ) {
alt263=1;
}
switch (alt263) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36583:2: rule__JvmParameterizedTypeReference__Group_1_2__0
{
pushFollow(FollowSets003.FOLLOW_rule__JvmParameterizedTypeReference__Group_1_2__0_in_rule__JvmParameterizedTypeReference__Group_1__2__Impl73446);
rule__JvmParameterizedTypeReference__Group_1_2__0();
state._fsp--;
if (state.failed) return ;
}
break;
default :
break loop263;
}
} while (true);
if ( state.backtracking==0 ) {
after(grammarAccess.getJvmParameterizedTypeReferenceAccess().getGroup_1_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__JvmParameterizedTypeReference__Group_1__2__Impl"
// $ANTLR start "rule__JvmParameterizedTypeReference__Group_1__3"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36593:1: rule__JvmParameterizedTypeReference__Group_1__3 : rule__JvmParameterizedTypeReference__Group_1__3__Impl rule__JvmParameterizedTypeReference__Group_1__4 ;
public final void rule__JvmParameterizedTypeReference__Group_1__3() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36597:1: ( rule__JvmParameterizedTypeReference__Group_1__3__Impl rule__JvmParameterizedTypeReference__Group_1__4 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36598:2: rule__JvmParameterizedTypeReference__Group_1__3__Impl rule__JvmParameterizedTypeReference__Group_1__4
{
pushFollow(FollowSets003.FOLLOW_rule__JvmParameterizedTypeReference__Group_1__3__Impl_in_rule__JvmParameterizedTypeReference__Group_1__373477);
rule__JvmParameterizedTypeReference__Group_1__3__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets003.FOLLOW_rule__JvmParameterizedTypeReference__Group_1__4_in_rule__JvmParameterizedTypeReference__Group_1__373480);
rule__JvmParameterizedTypeReference__Group_1__4();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__JvmParameterizedTypeReference__Group_1__3"
// $ANTLR start "rule__JvmParameterizedTypeReference__Group_1__3__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36605:1: rule__JvmParameterizedTypeReference__Group_1__3__Impl : ( '>' ) ;
public final void rule__JvmParameterizedTypeReference__Group_1__3__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36609:1: ( ( '>' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36610:1: ( '>' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36610:1: ( '>' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36611:1: '>'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getJvmParameterizedTypeReferenceAccess().getGreaterThanSignKeyword_1_3());
}
match(input,26,FollowSets003.FOLLOW_26_in_rule__JvmParameterizedTypeReference__Group_1__3__Impl73508); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getJvmParameterizedTypeReferenceAccess().getGreaterThanSignKeyword_1_3());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__JvmParameterizedTypeReference__Group_1__3__Impl"
// $ANTLR start "rule__JvmParameterizedTypeReference__Group_1__4"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36624:1: rule__JvmParameterizedTypeReference__Group_1__4 : rule__JvmParameterizedTypeReference__Group_1__4__Impl ;
public final void rule__JvmParameterizedTypeReference__Group_1__4() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36628:1: ( rule__JvmParameterizedTypeReference__Group_1__4__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36629:2: rule__JvmParameterizedTypeReference__Group_1__4__Impl
{
pushFollow(FollowSets003.FOLLOW_rule__JvmParameterizedTypeReference__Group_1__4__Impl_in_rule__JvmParameterizedTypeReference__Group_1__473539);
rule__JvmParameterizedTypeReference__Group_1__4__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__JvmParameterizedTypeReference__Group_1__4"
// $ANTLR start "rule__JvmParameterizedTypeReference__Group_1__4__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36635:1: rule__JvmParameterizedTypeReference__Group_1__4__Impl : ( ( rule__JvmParameterizedTypeReference__Group_1_4__0 )* ) ;
public final void rule__JvmParameterizedTypeReference__Group_1__4__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36639:1: ( ( ( rule__JvmParameterizedTypeReference__Group_1_4__0 )* ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36640:1: ( ( rule__JvmParameterizedTypeReference__Group_1_4__0 )* )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36640:1: ( ( rule__JvmParameterizedTypeReference__Group_1_4__0 )* )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36641:1: ( rule__JvmParameterizedTypeReference__Group_1_4__0 )*
{
if ( state.backtracking==0 ) {
before(grammarAccess.getJvmParameterizedTypeReferenceAccess().getGroup_1_4());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36642:1: ( rule__JvmParameterizedTypeReference__Group_1_4__0 )*
loop264:
do {
int alt264=2;
int LA264_0 = input.LA(1);
if ( (LA264_0==43) ) {
int LA264_2 = input.LA(2);
if ( (LA264_2==RULE_ID) ) {
int LA264_3 = input.LA(3);
if ( (synpred350_InternalMango()) ) {
alt264=1;
}
}
}
switch (alt264) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36642:2: rule__JvmParameterizedTypeReference__Group_1_4__0
{
pushFollow(FollowSets003.FOLLOW_rule__JvmParameterizedTypeReference__Group_1_4__0_in_rule__JvmParameterizedTypeReference__Group_1__4__Impl73566);
rule__JvmParameterizedTypeReference__Group_1_4__0();
state._fsp--;
if (state.failed) return ;
}
break;
default :
break loop264;
}
} while (true);
if ( state.backtracking==0 ) {
after(grammarAccess.getJvmParameterizedTypeReferenceAccess().getGroup_1_4());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__JvmParameterizedTypeReference__Group_1__4__Impl"
// $ANTLR start "rule__JvmParameterizedTypeReference__Group_1_2__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36662:1: rule__JvmParameterizedTypeReference__Group_1_2__0 : rule__JvmParameterizedTypeReference__Group_1_2__0__Impl rule__JvmParameterizedTypeReference__Group_1_2__1 ;
public final void rule__JvmParameterizedTypeReference__Group_1_2__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36666:1: ( rule__JvmParameterizedTypeReference__Group_1_2__0__Impl rule__JvmParameterizedTypeReference__Group_1_2__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36667:2: rule__JvmParameterizedTypeReference__Group_1_2__0__Impl rule__JvmParameterizedTypeReference__Group_1_2__1
{
pushFollow(FollowSets003.FOLLOW_rule__JvmParameterizedTypeReference__Group_1_2__0__Impl_in_rule__JvmParameterizedTypeReference__Group_1_2__073607);
rule__JvmParameterizedTypeReference__Group_1_2__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets003.FOLLOW_rule__JvmParameterizedTypeReference__Group_1_2__1_in_rule__JvmParameterizedTypeReference__Group_1_2__073610);
rule__JvmParameterizedTypeReference__Group_1_2__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__JvmParameterizedTypeReference__Group_1_2__0"
// $ANTLR start "rule__JvmParameterizedTypeReference__Group_1_2__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36674:1: rule__JvmParameterizedTypeReference__Group_1_2__0__Impl : ( ',' ) ;
public final void rule__JvmParameterizedTypeReference__Group_1_2__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36678:1: ( ( ',' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36679:1: ( ',' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36679:1: ( ',' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36680:1: ','
{
if ( state.backtracking==0 ) {
before(grammarAccess.getJvmParameterizedTypeReferenceAccess().getCommaKeyword_1_2_0());
}
match(input,154,FollowSets003.FOLLOW_154_in_rule__JvmParameterizedTypeReference__Group_1_2__0__Impl73638); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getJvmParameterizedTypeReferenceAccess().getCommaKeyword_1_2_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__JvmParameterizedTypeReference__Group_1_2__0__Impl"
// $ANTLR start "rule__JvmParameterizedTypeReference__Group_1_2__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36693:1: rule__JvmParameterizedTypeReference__Group_1_2__1 : rule__JvmParameterizedTypeReference__Group_1_2__1__Impl ;
public final void rule__JvmParameterizedTypeReference__Group_1_2__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36697:1: ( rule__JvmParameterizedTypeReference__Group_1_2__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36698:2: rule__JvmParameterizedTypeReference__Group_1_2__1__Impl
{
pushFollow(FollowSets003.FOLLOW_rule__JvmParameterizedTypeReference__Group_1_2__1__Impl_in_rule__JvmParameterizedTypeReference__Group_1_2__173669);
rule__JvmParameterizedTypeReference__Group_1_2__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__JvmParameterizedTypeReference__Group_1_2__1"
// $ANTLR start "rule__JvmParameterizedTypeReference__Group_1_2__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36704:1: rule__JvmParameterizedTypeReference__Group_1_2__1__Impl : ( ( rule__JvmParameterizedTypeReference__ArgumentsAssignment_1_2_1 ) ) ;
public final void rule__JvmParameterizedTypeReference__Group_1_2__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36708:1: ( ( ( rule__JvmParameterizedTypeReference__ArgumentsAssignment_1_2_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36709:1: ( ( rule__JvmParameterizedTypeReference__ArgumentsAssignment_1_2_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36709:1: ( ( rule__JvmParameterizedTypeReference__ArgumentsAssignment_1_2_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36710:1: ( rule__JvmParameterizedTypeReference__ArgumentsAssignment_1_2_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getJvmParameterizedTypeReferenceAccess().getArgumentsAssignment_1_2_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36711:1: ( rule__JvmParameterizedTypeReference__ArgumentsAssignment_1_2_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36711:2: rule__JvmParameterizedTypeReference__ArgumentsAssignment_1_2_1
{
pushFollow(FollowSets003.FOLLOW_rule__JvmParameterizedTypeReference__ArgumentsAssignment_1_2_1_in_rule__JvmParameterizedTypeReference__Group_1_2__1__Impl73696);
rule__JvmParameterizedTypeReference__ArgumentsAssignment_1_2_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getJvmParameterizedTypeReferenceAccess().getArgumentsAssignment_1_2_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__JvmParameterizedTypeReference__Group_1_2__1__Impl"
// $ANTLR start "rule__JvmParameterizedTypeReference__Group_1_4__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36725:1: rule__JvmParameterizedTypeReference__Group_1_4__0 : rule__JvmParameterizedTypeReference__Group_1_4__0__Impl rule__JvmParameterizedTypeReference__Group_1_4__1 ;
public final void rule__JvmParameterizedTypeReference__Group_1_4__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36729:1: ( rule__JvmParameterizedTypeReference__Group_1_4__0__Impl rule__JvmParameterizedTypeReference__Group_1_4__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36730:2: rule__JvmParameterizedTypeReference__Group_1_4__0__Impl rule__JvmParameterizedTypeReference__Group_1_4__1
{
pushFollow(FollowSets003.FOLLOW_rule__JvmParameterizedTypeReference__Group_1_4__0__Impl_in_rule__JvmParameterizedTypeReference__Group_1_4__073730);
rule__JvmParameterizedTypeReference__Group_1_4__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets003.FOLLOW_rule__JvmParameterizedTypeReference__Group_1_4__1_in_rule__JvmParameterizedTypeReference__Group_1_4__073733);
rule__JvmParameterizedTypeReference__Group_1_4__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__JvmParameterizedTypeReference__Group_1_4__0"
// $ANTLR start "rule__JvmParameterizedTypeReference__Group_1_4__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36737:1: rule__JvmParameterizedTypeReference__Group_1_4__0__Impl : ( ( rule__JvmParameterizedTypeReference__Group_1_4_0__0 ) ) ;
public final void rule__JvmParameterizedTypeReference__Group_1_4__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36741:1: ( ( ( rule__JvmParameterizedTypeReference__Group_1_4_0__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36742:1: ( ( rule__JvmParameterizedTypeReference__Group_1_4_0__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36742:1: ( ( rule__JvmParameterizedTypeReference__Group_1_4_0__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36743:1: ( rule__JvmParameterizedTypeReference__Group_1_4_0__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getJvmParameterizedTypeReferenceAccess().getGroup_1_4_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36744:1: ( rule__JvmParameterizedTypeReference__Group_1_4_0__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36744:2: rule__JvmParameterizedTypeReference__Group_1_4_0__0
{
pushFollow(FollowSets003.FOLLOW_rule__JvmParameterizedTypeReference__Group_1_4_0__0_in_rule__JvmParameterizedTypeReference__Group_1_4__0__Impl73760);
rule__JvmParameterizedTypeReference__Group_1_4_0__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getJvmParameterizedTypeReferenceAccess().getGroup_1_4_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__JvmParameterizedTypeReference__Group_1_4__0__Impl"
// $ANTLR start "rule__JvmParameterizedTypeReference__Group_1_4__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36754:1: rule__JvmParameterizedTypeReference__Group_1_4__1 : rule__JvmParameterizedTypeReference__Group_1_4__1__Impl rule__JvmParameterizedTypeReference__Group_1_4__2 ;
public final void rule__JvmParameterizedTypeReference__Group_1_4__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36758:1: ( rule__JvmParameterizedTypeReference__Group_1_4__1__Impl rule__JvmParameterizedTypeReference__Group_1_4__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36759:2: rule__JvmParameterizedTypeReference__Group_1_4__1__Impl rule__JvmParameterizedTypeReference__Group_1_4__2
{
pushFollow(FollowSets003.FOLLOW_rule__JvmParameterizedTypeReference__Group_1_4__1__Impl_in_rule__JvmParameterizedTypeReference__Group_1_4__173790);
rule__JvmParameterizedTypeReference__Group_1_4__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets003.FOLLOW_rule__JvmParameterizedTypeReference__Group_1_4__2_in_rule__JvmParameterizedTypeReference__Group_1_4__173793);
rule__JvmParameterizedTypeReference__Group_1_4__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__JvmParameterizedTypeReference__Group_1_4__1"
// $ANTLR start "rule__JvmParameterizedTypeReference__Group_1_4__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36766:1: rule__JvmParameterizedTypeReference__Group_1_4__1__Impl : ( ( rule__JvmParameterizedTypeReference__TypeAssignment_1_4_1 ) ) ;
public final void rule__JvmParameterizedTypeReference__Group_1_4__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36770:1: ( ( ( rule__JvmParameterizedTypeReference__TypeAssignment_1_4_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36771:1: ( ( rule__JvmParameterizedTypeReference__TypeAssignment_1_4_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36771:1: ( ( rule__JvmParameterizedTypeReference__TypeAssignment_1_4_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36772:1: ( rule__JvmParameterizedTypeReference__TypeAssignment_1_4_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getJvmParameterizedTypeReferenceAccess().getTypeAssignment_1_4_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36773:1: ( rule__JvmParameterizedTypeReference__TypeAssignment_1_4_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36773:2: rule__JvmParameterizedTypeReference__TypeAssignment_1_4_1
{
pushFollow(FollowSets003.FOLLOW_rule__JvmParameterizedTypeReference__TypeAssignment_1_4_1_in_rule__JvmParameterizedTypeReference__Group_1_4__1__Impl73820);
rule__JvmParameterizedTypeReference__TypeAssignment_1_4_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getJvmParameterizedTypeReferenceAccess().getTypeAssignment_1_4_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__JvmParameterizedTypeReference__Group_1_4__1__Impl"
// $ANTLR start "rule__JvmParameterizedTypeReference__Group_1_4__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36783:1: rule__JvmParameterizedTypeReference__Group_1_4__2 : rule__JvmParameterizedTypeReference__Group_1_4__2__Impl ;
public final void rule__JvmParameterizedTypeReference__Group_1_4__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36787:1: ( rule__JvmParameterizedTypeReference__Group_1_4__2__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36788:2: rule__JvmParameterizedTypeReference__Group_1_4__2__Impl
{
pushFollow(FollowSets003.FOLLOW_rule__JvmParameterizedTypeReference__Group_1_4__2__Impl_in_rule__JvmParameterizedTypeReference__Group_1_4__273850);
rule__JvmParameterizedTypeReference__Group_1_4__2__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__JvmParameterizedTypeReference__Group_1_4__2"
// $ANTLR start "rule__JvmParameterizedTypeReference__Group_1_4__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36794:1: rule__JvmParameterizedTypeReference__Group_1_4__2__Impl : ( ( rule__JvmParameterizedTypeReference__Group_1_4_2__0 )? ) ;
public final void rule__JvmParameterizedTypeReference__Group_1_4__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36798:1: ( ( ( rule__JvmParameterizedTypeReference__Group_1_4_2__0 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36799:1: ( ( rule__JvmParameterizedTypeReference__Group_1_4_2__0 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36799:1: ( ( rule__JvmParameterizedTypeReference__Group_1_4_2__0 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36800:1: ( rule__JvmParameterizedTypeReference__Group_1_4_2__0 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getJvmParameterizedTypeReferenceAccess().getGroup_1_4_2());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36801:1: ( rule__JvmParameterizedTypeReference__Group_1_4_2__0 )?
int alt265=2;
alt265 = dfa265.predict(input);
switch (alt265) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36801:2: rule__JvmParameterizedTypeReference__Group_1_4_2__0
{
pushFollow(FollowSets003.FOLLOW_rule__JvmParameterizedTypeReference__Group_1_4_2__0_in_rule__JvmParameterizedTypeReference__Group_1_4__2__Impl73877);
rule__JvmParameterizedTypeReference__Group_1_4_2__0();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getJvmParameterizedTypeReferenceAccess().getGroup_1_4_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__JvmParameterizedTypeReference__Group_1_4__2__Impl"
// $ANTLR start "rule__JvmParameterizedTypeReference__Group_1_4_0__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36817:1: rule__JvmParameterizedTypeReference__Group_1_4_0__0 : rule__JvmParameterizedTypeReference__Group_1_4_0__0__Impl ;
public final void rule__JvmParameterizedTypeReference__Group_1_4_0__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36821:1: ( rule__JvmParameterizedTypeReference__Group_1_4_0__0__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36822:2: rule__JvmParameterizedTypeReference__Group_1_4_0__0__Impl
{
pushFollow(FollowSets003.FOLLOW_rule__JvmParameterizedTypeReference__Group_1_4_0__0__Impl_in_rule__JvmParameterizedTypeReference__Group_1_4_0__073914);
rule__JvmParameterizedTypeReference__Group_1_4_0__0__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__JvmParameterizedTypeReference__Group_1_4_0__0"
// $ANTLR start "rule__JvmParameterizedTypeReference__Group_1_4_0__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36828:1: rule__JvmParameterizedTypeReference__Group_1_4_0__0__Impl : ( ( rule__JvmParameterizedTypeReference__Group_1_4_0_0__0 ) ) ;
public final void rule__JvmParameterizedTypeReference__Group_1_4_0__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36832:1: ( ( ( rule__JvmParameterizedTypeReference__Group_1_4_0_0__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36833:1: ( ( rule__JvmParameterizedTypeReference__Group_1_4_0_0__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36833:1: ( ( rule__JvmParameterizedTypeReference__Group_1_4_0_0__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36834:1: ( rule__JvmParameterizedTypeReference__Group_1_4_0_0__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getJvmParameterizedTypeReferenceAccess().getGroup_1_4_0_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36835:1: ( rule__JvmParameterizedTypeReference__Group_1_4_0_0__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36835:2: rule__JvmParameterizedTypeReference__Group_1_4_0_0__0
{
pushFollow(FollowSets003.FOLLOW_rule__JvmParameterizedTypeReference__Group_1_4_0_0__0_in_rule__JvmParameterizedTypeReference__Group_1_4_0__0__Impl73941);
rule__JvmParameterizedTypeReference__Group_1_4_0_0__0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getJvmParameterizedTypeReferenceAccess().getGroup_1_4_0_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__JvmParameterizedTypeReference__Group_1_4_0__0__Impl"
// $ANTLR start "rule__JvmParameterizedTypeReference__Group_1_4_0_0__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36847:1: rule__JvmParameterizedTypeReference__Group_1_4_0_0__0 : rule__JvmParameterizedTypeReference__Group_1_4_0_0__0__Impl rule__JvmParameterizedTypeReference__Group_1_4_0_0__1 ;
public final void rule__JvmParameterizedTypeReference__Group_1_4_0_0__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36851:1: ( rule__JvmParameterizedTypeReference__Group_1_4_0_0__0__Impl rule__JvmParameterizedTypeReference__Group_1_4_0_0__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36852:2: rule__JvmParameterizedTypeReference__Group_1_4_0_0__0__Impl rule__JvmParameterizedTypeReference__Group_1_4_0_0__1
{
pushFollow(FollowSets003.FOLLOW_rule__JvmParameterizedTypeReference__Group_1_4_0_0__0__Impl_in_rule__JvmParameterizedTypeReference__Group_1_4_0_0__073973);
rule__JvmParameterizedTypeReference__Group_1_4_0_0__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets003.FOLLOW_rule__JvmParameterizedTypeReference__Group_1_4_0_0__1_in_rule__JvmParameterizedTypeReference__Group_1_4_0_0__073976);
rule__JvmParameterizedTypeReference__Group_1_4_0_0__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__JvmParameterizedTypeReference__Group_1_4_0_0__0"
// $ANTLR start "rule__JvmParameterizedTypeReference__Group_1_4_0_0__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36859:1: rule__JvmParameterizedTypeReference__Group_1_4_0_0__0__Impl : ( () ) ;
public final void rule__JvmParameterizedTypeReference__Group_1_4_0_0__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36863:1: ( ( () ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36864:1: ( () )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36864:1: ( () )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36865:1: ()
{
if ( state.backtracking==0 ) {
before(grammarAccess.getJvmParameterizedTypeReferenceAccess().getJvmInnerTypeReferenceOuterAction_1_4_0_0_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36866:1: ()
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36868:1:
{
}
if ( state.backtracking==0 ) {
after(grammarAccess.getJvmParameterizedTypeReferenceAccess().getJvmInnerTypeReferenceOuterAction_1_4_0_0_0());
}
}
}
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__JvmParameterizedTypeReference__Group_1_4_0_0__0__Impl"
// $ANTLR start "rule__JvmParameterizedTypeReference__Group_1_4_0_0__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36878:1: rule__JvmParameterizedTypeReference__Group_1_4_0_0__1 : rule__JvmParameterizedTypeReference__Group_1_4_0_0__1__Impl ;
public final void rule__JvmParameterizedTypeReference__Group_1_4_0_0__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36882:1: ( rule__JvmParameterizedTypeReference__Group_1_4_0_0__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36883:2: rule__JvmParameterizedTypeReference__Group_1_4_0_0__1__Impl
{
pushFollow(FollowSets003.FOLLOW_rule__JvmParameterizedTypeReference__Group_1_4_0_0__1__Impl_in_rule__JvmParameterizedTypeReference__Group_1_4_0_0__174034);
rule__JvmParameterizedTypeReference__Group_1_4_0_0__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__JvmParameterizedTypeReference__Group_1_4_0_0__1"
// $ANTLR start "rule__JvmParameterizedTypeReference__Group_1_4_0_0__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36889:1: rule__JvmParameterizedTypeReference__Group_1_4_0_0__1__Impl : ( '.' ) ;
public final void rule__JvmParameterizedTypeReference__Group_1_4_0_0__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36893:1: ( ( '.' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36894:1: ( '.' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36894:1: ( '.' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36895:1: '.'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getJvmParameterizedTypeReferenceAccess().getFullStopKeyword_1_4_0_0_1());
}
match(input,43,FollowSets003.FOLLOW_43_in_rule__JvmParameterizedTypeReference__Group_1_4_0_0__1__Impl74062); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getJvmParameterizedTypeReferenceAccess().getFullStopKeyword_1_4_0_0_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__JvmParameterizedTypeReference__Group_1_4_0_0__1__Impl"
// $ANTLR start "rule__JvmParameterizedTypeReference__Group_1_4_2__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36912:1: rule__JvmParameterizedTypeReference__Group_1_4_2__0 : rule__JvmParameterizedTypeReference__Group_1_4_2__0__Impl rule__JvmParameterizedTypeReference__Group_1_4_2__1 ;
public final void rule__JvmParameterizedTypeReference__Group_1_4_2__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36916:1: ( rule__JvmParameterizedTypeReference__Group_1_4_2__0__Impl rule__JvmParameterizedTypeReference__Group_1_4_2__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36917:2: rule__JvmParameterizedTypeReference__Group_1_4_2__0__Impl rule__JvmParameterizedTypeReference__Group_1_4_2__1
{
pushFollow(FollowSets003.FOLLOW_rule__JvmParameterizedTypeReference__Group_1_4_2__0__Impl_in_rule__JvmParameterizedTypeReference__Group_1_4_2__074097);
rule__JvmParameterizedTypeReference__Group_1_4_2__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets003.FOLLOW_rule__JvmParameterizedTypeReference__Group_1_4_2__1_in_rule__JvmParameterizedTypeReference__Group_1_4_2__074100);
rule__JvmParameterizedTypeReference__Group_1_4_2__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__JvmParameterizedTypeReference__Group_1_4_2__0"
// $ANTLR start "rule__JvmParameterizedTypeReference__Group_1_4_2__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36924:1: rule__JvmParameterizedTypeReference__Group_1_4_2__0__Impl : ( ( '<' ) ) ;
public final void rule__JvmParameterizedTypeReference__Group_1_4_2__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36928:1: ( ( ( '<' ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36929:1: ( ( '<' ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36929:1: ( ( '<' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36930:1: ( '<' )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getJvmParameterizedTypeReferenceAccess().getLessThanSignKeyword_1_4_2_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36931:1: ( '<' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36932:2: '<'
{
match(input,27,FollowSets003.FOLLOW_27_in_rule__JvmParameterizedTypeReference__Group_1_4_2__0__Impl74129); if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getJvmParameterizedTypeReferenceAccess().getLessThanSignKeyword_1_4_2_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__JvmParameterizedTypeReference__Group_1_4_2__0__Impl"
// $ANTLR start "rule__JvmParameterizedTypeReference__Group_1_4_2__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36943:1: rule__JvmParameterizedTypeReference__Group_1_4_2__1 : rule__JvmParameterizedTypeReference__Group_1_4_2__1__Impl rule__JvmParameterizedTypeReference__Group_1_4_2__2 ;
public final void rule__JvmParameterizedTypeReference__Group_1_4_2__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36947:1: ( rule__JvmParameterizedTypeReference__Group_1_4_2__1__Impl rule__JvmParameterizedTypeReference__Group_1_4_2__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36948:2: rule__JvmParameterizedTypeReference__Group_1_4_2__1__Impl rule__JvmParameterizedTypeReference__Group_1_4_2__2
{
pushFollow(FollowSets003.FOLLOW_rule__JvmParameterizedTypeReference__Group_1_4_2__1__Impl_in_rule__JvmParameterizedTypeReference__Group_1_4_2__174161);
rule__JvmParameterizedTypeReference__Group_1_4_2__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets003.FOLLOW_rule__JvmParameterizedTypeReference__Group_1_4_2__2_in_rule__JvmParameterizedTypeReference__Group_1_4_2__174164);
rule__JvmParameterizedTypeReference__Group_1_4_2__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__JvmParameterizedTypeReference__Group_1_4_2__1"
// $ANTLR start "rule__JvmParameterizedTypeReference__Group_1_4_2__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36955:1: rule__JvmParameterizedTypeReference__Group_1_4_2__1__Impl : ( ( rule__JvmParameterizedTypeReference__ArgumentsAssignment_1_4_2_1 ) ) ;
public final void rule__JvmParameterizedTypeReference__Group_1_4_2__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36959:1: ( ( ( rule__JvmParameterizedTypeReference__ArgumentsAssignment_1_4_2_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36960:1: ( ( rule__JvmParameterizedTypeReference__ArgumentsAssignment_1_4_2_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36960:1: ( ( rule__JvmParameterizedTypeReference__ArgumentsAssignment_1_4_2_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36961:1: ( rule__JvmParameterizedTypeReference__ArgumentsAssignment_1_4_2_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getJvmParameterizedTypeReferenceAccess().getArgumentsAssignment_1_4_2_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36962:1: ( rule__JvmParameterizedTypeReference__ArgumentsAssignment_1_4_2_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36962:2: rule__JvmParameterizedTypeReference__ArgumentsAssignment_1_4_2_1
{
pushFollow(FollowSets003.FOLLOW_rule__JvmParameterizedTypeReference__ArgumentsAssignment_1_4_2_1_in_rule__JvmParameterizedTypeReference__Group_1_4_2__1__Impl74191);
rule__JvmParameterizedTypeReference__ArgumentsAssignment_1_4_2_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getJvmParameterizedTypeReferenceAccess().getArgumentsAssignment_1_4_2_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__JvmParameterizedTypeReference__Group_1_4_2__1__Impl"
// $ANTLR start "rule__JvmParameterizedTypeReference__Group_1_4_2__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36972:1: rule__JvmParameterizedTypeReference__Group_1_4_2__2 : rule__JvmParameterizedTypeReference__Group_1_4_2__2__Impl rule__JvmParameterizedTypeReference__Group_1_4_2__3 ;
public final void rule__JvmParameterizedTypeReference__Group_1_4_2__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36976:1: ( rule__JvmParameterizedTypeReference__Group_1_4_2__2__Impl rule__JvmParameterizedTypeReference__Group_1_4_2__3 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36977:2: rule__JvmParameterizedTypeReference__Group_1_4_2__2__Impl rule__JvmParameterizedTypeReference__Group_1_4_2__3
{
pushFollow(FollowSets003.FOLLOW_rule__JvmParameterizedTypeReference__Group_1_4_2__2__Impl_in_rule__JvmParameterizedTypeReference__Group_1_4_2__274221);
rule__JvmParameterizedTypeReference__Group_1_4_2__2__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets003.FOLLOW_rule__JvmParameterizedTypeReference__Group_1_4_2__3_in_rule__JvmParameterizedTypeReference__Group_1_4_2__274224);
rule__JvmParameterizedTypeReference__Group_1_4_2__3();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__JvmParameterizedTypeReference__Group_1_4_2__2"
// $ANTLR start "rule__JvmParameterizedTypeReference__Group_1_4_2__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36984:1: rule__JvmParameterizedTypeReference__Group_1_4_2__2__Impl : ( ( rule__JvmParameterizedTypeReference__Group_1_4_2_2__0 )* ) ;
public final void rule__JvmParameterizedTypeReference__Group_1_4_2__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36988:1: ( ( ( rule__JvmParameterizedTypeReference__Group_1_4_2_2__0 )* ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36989:1: ( ( rule__JvmParameterizedTypeReference__Group_1_4_2_2__0 )* )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36989:1: ( ( rule__JvmParameterizedTypeReference__Group_1_4_2_2__0 )* )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36990:1: ( rule__JvmParameterizedTypeReference__Group_1_4_2_2__0 )*
{
if ( state.backtracking==0 ) {
before(grammarAccess.getJvmParameterizedTypeReferenceAccess().getGroup_1_4_2_2());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36991:1: ( rule__JvmParameterizedTypeReference__Group_1_4_2_2__0 )*
loop266:
do {
int alt266=2;
int LA266_0 = input.LA(1);
if ( (LA266_0==154) ) {
alt266=1;
}
switch (alt266) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36991:2: rule__JvmParameterizedTypeReference__Group_1_4_2_2__0
{
pushFollow(FollowSets003.FOLLOW_rule__JvmParameterizedTypeReference__Group_1_4_2_2__0_in_rule__JvmParameterizedTypeReference__Group_1_4_2__2__Impl74251);
rule__JvmParameterizedTypeReference__Group_1_4_2_2__0();
state._fsp--;
if (state.failed) return ;
}
break;
default :
break loop266;
}
} while (true);
if ( state.backtracking==0 ) {
after(grammarAccess.getJvmParameterizedTypeReferenceAccess().getGroup_1_4_2_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__JvmParameterizedTypeReference__Group_1_4_2__2__Impl"
// $ANTLR start "rule__JvmParameterizedTypeReference__Group_1_4_2__3"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37001:1: rule__JvmParameterizedTypeReference__Group_1_4_2__3 : rule__JvmParameterizedTypeReference__Group_1_4_2__3__Impl ;
public final void rule__JvmParameterizedTypeReference__Group_1_4_2__3() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37005:1: ( rule__JvmParameterizedTypeReference__Group_1_4_2__3__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37006:2: rule__JvmParameterizedTypeReference__Group_1_4_2__3__Impl
{
pushFollow(FollowSets003.FOLLOW_rule__JvmParameterizedTypeReference__Group_1_4_2__3__Impl_in_rule__JvmParameterizedTypeReference__Group_1_4_2__374282);
rule__JvmParameterizedTypeReference__Group_1_4_2__3__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__JvmParameterizedTypeReference__Group_1_4_2__3"
// $ANTLR start "rule__JvmParameterizedTypeReference__Group_1_4_2__3__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37012:1: rule__JvmParameterizedTypeReference__Group_1_4_2__3__Impl : ( '>' ) ;
public final void rule__JvmParameterizedTypeReference__Group_1_4_2__3__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37016:1: ( ( '>' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37017:1: ( '>' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37017:1: ( '>' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37018:1: '>'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getJvmParameterizedTypeReferenceAccess().getGreaterThanSignKeyword_1_4_2_3());
}
match(input,26,FollowSets003.FOLLOW_26_in_rule__JvmParameterizedTypeReference__Group_1_4_2__3__Impl74310); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getJvmParameterizedTypeReferenceAccess().getGreaterThanSignKeyword_1_4_2_3());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__JvmParameterizedTypeReference__Group_1_4_2__3__Impl"
// $ANTLR start "rule__JvmParameterizedTypeReference__Group_1_4_2_2__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37039:1: rule__JvmParameterizedTypeReference__Group_1_4_2_2__0 : rule__JvmParameterizedTypeReference__Group_1_4_2_2__0__Impl rule__JvmParameterizedTypeReference__Group_1_4_2_2__1 ;
public final void rule__JvmParameterizedTypeReference__Group_1_4_2_2__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37043:1: ( rule__JvmParameterizedTypeReference__Group_1_4_2_2__0__Impl rule__JvmParameterizedTypeReference__Group_1_4_2_2__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37044:2: rule__JvmParameterizedTypeReference__Group_1_4_2_2__0__Impl rule__JvmParameterizedTypeReference__Group_1_4_2_2__1
{
pushFollow(FollowSets003.FOLLOW_rule__JvmParameterizedTypeReference__Group_1_4_2_2__0__Impl_in_rule__JvmParameterizedTypeReference__Group_1_4_2_2__074349);
rule__JvmParameterizedTypeReference__Group_1_4_2_2__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets003.FOLLOW_rule__JvmParameterizedTypeReference__Group_1_4_2_2__1_in_rule__JvmParameterizedTypeReference__Group_1_4_2_2__074352);
rule__JvmParameterizedTypeReference__Group_1_4_2_2__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__JvmParameterizedTypeReference__Group_1_4_2_2__0"
// $ANTLR start "rule__JvmParameterizedTypeReference__Group_1_4_2_2__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37051:1: rule__JvmParameterizedTypeReference__Group_1_4_2_2__0__Impl : ( ',' ) ;
public final void rule__JvmParameterizedTypeReference__Group_1_4_2_2__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37055:1: ( ( ',' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37056:1: ( ',' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37056:1: ( ',' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37057:1: ','
{
if ( state.backtracking==0 ) {
before(grammarAccess.getJvmParameterizedTypeReferenceAccess().getCommaKeyword_1_4_2_2_0());
}
match(input,154,FollowSets003.FOLLOW_154_in_rule__JvmParameterizedTypeReference__Group_1_4_2_2__0__Impl74380); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getJvmParameterizedTypeReferenceAccess().getCommaKeyword_1_4_2_2_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__JvmParameterizedTypeReference__Group_1_4_2_2__0__Impl"
// $ANTLR start "rule__JvmParameterizedTypeReference__Group_1_4_2_2__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37070:1: rule__JvmParameterizedTypeReference__Group_1_4_2_2__1 : rule__JvmParameterizedTypeReference__Group_1_4_2_2__1__Impl ;
public final void rule__JvmParameterizedTypeReference__Group_1_4_2_2__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37074:1: ( rule__JvmParameterizedTypeReference__Group_1_4_2_2__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37075:2: rule__JvmParameterizedTypeReference__Group_1_4_2_2__1__Impl
{
pushFollow(FollowSets003.FOLLOW_rule__JvmParameterizedTypeReference__Group_1_4_2_2__1__Impl_in_rule__JvmParameterizedTypeReference__Group_1_4_2_2__174411);
rule__JvmParameterizedTypeReference__Group_1_4_2_2__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__JvmParameterizedTypeReference__Group_1_4_2_2__1"
// $ANTLR start "rule__JvmParameterizedTypeReference__Group_1_4_2_2__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37081:1: rule__JvmParameterizedTypeReference__Group_1_4_2_2__1__Impl : ( ( rule__JvmParameterizedTypeReference__ArgumentsAssignment_1_4_2_2_1 ) ) ;
public final void rule__JvmParameterizedTypeReference__Group_1_4_2_2__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37085:1: ( ( ( rule__JvmParameterizedTypeReference__ArgumentsAssignment_1_4_2_2_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37086:1: ( ( rule__JvmParameterizedTypeReference__ArgumentsAssignment_1_4_2_2_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37086:1: ( ( rule__JvmParameterizedTypeReference__ArgumentsAssignment_1_4_2_2_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37087:1: ( rule__JvmParameterizedTypeReference__ArgumentsAssignment_1_4_2_2_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getJvmParameterizedTypeReferenceAccess().getArgumentsAssignment_1_4_2_2_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37088:1: ( rule__JvmParameterizedTypeReference__ArgumentsAssignment_1_4_2_2_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37088:2: rule__JvmParameterizedTypeReference__ArgumentsAssignment_1_4_2_2_1
{
pushFollow(FollowSets003.FOLLOW_rule__JvmParameterizedTypeReference__ArgumentsAssignment_1_4_2_2_1_in_rule__JvmParameterizedTypeReference__Group_1_4_2_2__1__Impl74438);
rule__JvmParameterizedTypeReference__ArgumentsAssignment_1_4_2_2_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getJvmParameterizedTypeReferenceAccess().getArgumentsAssignment_1_4_2_2_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__JvmParameterizedTypeReference__Group_1_4_2_2__1__Impl"
// $ANTLR start "rule__JvmWildcardTypeReference__Group__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37102:1: rule__JvmWildcardTypeReference__Group__0 : rule__JvmWildcardTypeReference__Group__0__Impl rule__JvmWildcardTypeReference__Group__1 ;
public final void rule__JvmWildcardTypeReference__Group__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37106:1: ( rule__JvmWildcardTypeReference__Group__0__Impl rule__JvmWildcardTypeReference__Group__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37107:2: rule__JvmWildcardTypeReference__Group__0__Impl rule__JvmWildcardTypeReference__Group__1
{
pushFollow(FollowSets003.FOLLOW_rule__JvmWildcardTypeReference__Group__0__Impl_in_rule__JvmWildcardTypeReference__Group__074472);
rule__JvmWildcardTypeReference__Group__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets003.FOLLOW_rule__JvmWildcardTypeReference__Group__1_in_rule__JvmWildcardTypeReference__Group__074475);
rule__JvmWildcardTypeReference__Group__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__JvmWildcardTypeReference__Group__0"
// $ANTLR start "rule__JvmWildcardTypeReference__Group__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37114:1: rule__JvmWildcardTypeReference__Group__0__Impl : ( () ) ;
public final void rule__JvmWildcardTypeReference__Group__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37118:1: ( ( () ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37119:1: ( () )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37119:1: ( () )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37120:1: ()
{
if ( state.backtracking==0 ) {
before(grammarAccess.getJvmWildcardTypeReferenceAccess().getJvmWildcardTypeReferenceAction_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37121:1: ()
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37123:1:
{
}
if ( state.backtracking==0 ) {
after(grammarAccess.getJvmWildcardTypeReferenceAccess().getJvmWildcardTypeReferenceAction_0());
}
}
}
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__JvmWildcardTypeReference__Group__0__Impl"
// $ANTLR start "rule__JvmWildcardTypeReference__Group__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37133:1: rule__JvmWildcardTypeReference__Group__1 : rule__JvmWildcardTypeReference__Group__1__Impl rule__JvmWildcardTypeReference__Group__2 ;
public final void rule__JvmWildcardTypeReference__Group__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37137:1: ( rule__JvmWildcardTypeReference__Group__1__Impl rule__JvmWildcardTypeReference__Group__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37138:2: rule__JvmWildcardTypeReference__Group__1__Impl rule__JvmWildcardTypeReference__Group__2
{
pushFollow(FollowSets003.FOLLOW_rule__JvmWildcardTypeReference__Group__1__Impl_in_rule__JvmWildcardTypeReference__Group__174533);
rule__JvmWildcardTypeReference__Group__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets003.FOLLOW_rule__JvmWildcardTypeReference__Group__2_in_rule__JvmWildcardTypeReference__Group__174536);
rule__JvmWildcardTypeReference__Group__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__JvmWildcardTypeReference__Group__1"
// $ANTLR start "rule__JvmWildcardTypeReference__Group__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37145:1: rule__JvmWildcardTypeReference__Group__1__Impl : ( '?' ) ;
public final void rule__JvmWildcardTypeReference__Group__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37149:1: ( ( '?' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37150:1: ( '?' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37150:1: ( '?' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37151:1: '?'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getJvmWildcardTypeReferenceAccess().getQuestionMarkKeyword_1());
}
match(input,177,FollowSets003.FOLLOW_177_in_rule__JvmWildcardTypeReference__Group__1__Impl74564); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getJvmWildcardTypeReferenceAccess().getQuestionMarkKeyword_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__JvmWildcardTypeReference__Group__1__Impl"
// $ANTLR start "rule__JvmWildcardTypeReference__Group__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37164:1: rule__JvmWildcardTypeReference__Group__2 : rule__JvmWildcardTypeReference__Group__2__Impl ;
public final void rule__JvmWildcardTypeReference__Group__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37168:1: ( rule__JvmWildcardTypeReference__Group__2__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37169:2: rule__JvmWildcardTypeReference__Group__2__Impl
{
pushFollow(FollowSets003.FOLLOW_rule__JvmWildcardTypeReference__Group__2__Impl_in_rule__JvmWildcardTypeReference__Group__274595);
rule__JvmWildcardTypeReference__Group__2__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__JvmWildcardTypeReference__Group__2"
// $ANTLR start "rule__JvmWildcardTypeReference__Group__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37175:1: rule__JvmWildcardTypeReference__Group__2__Impl : ( ( rule__JvmWildcardTypeReference__Alternatives_2 )? ) ;
public final void rule__JvmWildcardTypeReference__Group__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37179:1: ( ( ( rule__JvmWildcardTypeReference__Alternatives_2 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37180:1: ( ( rule__JvmWildcardTypeReference__Alternatives_2 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37180:1: ( ( rule__JvmWildcardTypeReference__Alternatives_2 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37181:1: ( rule__JvmWildcardTypeReference__Alternatives_2 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getJvmWildcardTypeReferenceAccess().getAlternatives_2());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37182:1: ( rule__JvmWildcardTypeReference__Alternatives_2 )?
int alt267=2;
int LA267_0 = input.LA(1);
if ( (LA267_0==45||LA267_0==49) ) {
alt267=1;
}
switch (alt267) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37182:2: rule__JvmWildcardTypeReference__Alternatives_2
{
pushFollow(FollowSets003.FOLLOW_rule__JvmWildcardTypeReference__Alternatives_2_in_rule__JvmWildcardTypeReference__Group__2__Impl74622);
rule__JvmWildcardTypeReference__Alternatives_2();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getJvmWildcardTypeReferenceAccess().getAlternatives_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__JvmWildcardTypeReference__Group__2__Impl"
// $ANTLR start "rule__JvmWildcardTypeReference__Group_2_0__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37198:1: rule__JvmWildcardTypeReference__Group_2_0__0 : rule__JvmWildcardTypeReference__Group_2_0__0__Impl rule__JvmWildcardTypeReference__Group_2_0__1 ;
public final void rule__JvmWildcardTypeReference__Group_2_0__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37202:1: ( rule__JvmWildcardTypeReference__Group_2_0__0__Impl rule__JvmWildcardTypeReference__Group_2_0__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37203:2: rule__JvmWildcardTypeReference__Group_2_0__0__Impl rule__JvmWildcardTypeReference__Group_2_0__1
{
pushFollow(FollowSets003.FOLLOW_rule__JvmWildcardTypeReference__Group_2_0__0__Impl_in_rule__JvmWildcardTypeReference__Group_2_0__074659);
rule__JvmWildcardTypeReference__Group_2_0__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets003.FOLLOW_rule__JvmWildcardTypeReference__Group_2_0__1_in_rule__JvmWildcardTypeReference__Group_2_0__074662);
rule__JvmWildcardTypeReference__Group_2_0__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__JvmWildcardTypeReference__Group_2_0__0"
// $ANTLR start "rule__JvmWildcardTypeReference__Group_2_0__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37210:1: rule__JvmWildcardTypeReference__Group_2_0__0__Impl : ( ( rule__JvmWildcardTypeReference__ConstraintsAssignment_2_0_0 ) ) ;
public final void rule__JvmWildcardTypeReference__Group_2_0__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37214:1: ( ( ( rule__JvmWildcardTypeReference__ConstraintsAssignment_2_0_0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37215:1: ( ( rule__JvmWildcardTypeReference__ConstraintsAssignment_2_0_0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37215:1: ( ( rule__JvmWildcardTypeReference__ConstraintsAssignment_2_0_0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37216:1: ( rule__JvmWildcardTypeReference__ConstraintsAssignment_2_0_0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getJvmWildcardTypeReferenceAccess().getConstraintsAssignment_2_0_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37217:1: ( rule__JvmWildcardTypeReference__ConstraintsAssignment_2_0_0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37217:2: rule__JvmWildcardTypeReference__ConstraintsAssignment_2_0_0
{
pushFollow(FollowSets003.FOLLOW_rule__JvmWildcardTypeReference__ConstraintsAssignment_2_0_0_in_rule__JvmWildcardTypeReference__Group_2_0__0__Impl74689);
rule__JvmWildcardTypeReference__ConstraintsAssignment_2_0_0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getJvmWildcardTypeReferenceAccess().getConstraintsAssignment_2_0_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__JvmWildcardTypeReference__Group_2_0__0__Impl"
// $ANTLR start "rule__JvmWildcardTypeReference__Group_2_0__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37227:1: rule__JvmWildcardTypeReference__Group_2_0__1 : rule__JvmWildcardTypeReference__Group_2_0__1__Impl ;
public final void rule__JvmWildcardTypeReference__Group_2_0__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37231:1: ( rule__JvmWildcardTypeReference__Group_2_0__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37232:2: rule__JvmWildcardTypeReference__Group_2_0__1__Impl
{
pushFollow(FollowSets003.FOLLOW_rule__JvmWildcardTypeReference__Group_2_0__1__Impl_in_rule__JvmWildcardTypeReference__Group_2_0__174719);
rule__JvmWildcardTypeReference__Group_2_0__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__JvmWildcardTypeReference__Group_2_0__1"
// $ANTLR start "rule__JvmWildcardTypeReference__Group_2_0__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37238:1: rule__JvmWildcardTypeReference__Group_2_0__1__Impl : ( ( rule__JvmWildcardTypeReference__ConstraintsAssignment_2_0_1 )* ) ;
public final void rule__JvmWildcardTypeReference__Group_2_0__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37242:1: ( ( ( rule__JvmWildcardTypeReference__ConstraintsAssignment_2_0_1 )* ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37243:1: ( ( rule__JvmWildcardTypeReference__ConstraintsAssignment_2_0_1 )* )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37243:1: ( ( rule__JvmWildcardTypeReference__ConstraintsAssignment_2_0_1 )* )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37244:1: ( rule__JvmWildcardTypeReference__ConstraintsAssignment_2_0_1 )*
{
if ( state.backtracking==0 ) {
before(grammarAccess.getJvmWildcardTypeReferenceAccess().getConstraintsAssignment_2_0_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37245:1: ( rule__JvmWildcardTypeReference__ConstraintsAssignment_2_0_1 )*
loop268:
do {
int alt268=2;
int LA268_0 = input.LA(1);
if ( (LA268_0==178) ) {
alt268=1;
}
switch (alt268) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37245:2: rule__JvmWildcardTypeReference__ConstraintsAssignment_2_0_1
{
pushFollow(FollowSets003.FOLLOW_rule__JvmWildcardTypeReference__ConstraintsAssignment_2_0_1_in_rule__JvmWildcardTypeReference__Group_2_0__1__Impl74746);
rule__JvmWildcardTypeReference__ConstraintsAssignment_2_0_1();
state._fsp--;
if (state.failed) return ;
}
break;
default :
break loop268;
}
} while (true);
if ( state.backtracking==0 ) {
after(grammarAccess.getJvmWildcardTypeReferenceAccess().getConstraintsAssignment_2_0_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__JvmWildcardTypeReference__Group_2_0__1__Impl"
// $ANTLR start "rule__JvmWildcardTypeReference__Group_2_1__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37259:1: rule__JvmWildcardTypeReference__Group_2_1__0 : rule__JvmWildcardTypeReference__Group_2_1__0__Impl rule__JvmWildcardTypeReference__Group_2_1__1 ;
public final void rule__JvmWildcardTypeReference__Group_2_1__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37263:1: ( rule__JvmWildcardTypeReference__Group_2_1__0__Impl rule__JvmWildcardTypeReference__Group_2_1__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37264:2: rule__JvmWildcardTypeReference__Group_2_1__0__Impl rule__JvmWildcardTypeReference__Group_2_1__1
{
pushFollow(FollowSets003.FOLLOW_rule__JvmWildcardTypeReference__Group_2_1__0__Impl_in_rule__JvmWildcardTypeReference__Group_2_1__074781);
rule__JvmWildcardTypeReference__Group_2_1__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets003.FOLLOW_rule__JvmWildcardTypeReference__Group_2_1__1_in_rule__JvmWildcardTypeReference__Group_2_1__074784);
rule__JvmWildcardTypeReference__Group_2_1__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__JvmWildcardTypeReference__Group_2_1__0"
// $ANTLR start "rule__JvmWildcardTypeReference__Group_2_1__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37271:1: rule__JvmWildcardTypeReference__Group_2_1__0__Impl : ( ( rule__JvmWildcardTypeReference__ConstraintsAssignment_2_1_0 ) ) ;
public final void rule__JvmWildcardTypeReference__Group_2_1__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37275:1: ( ( ( rule__JvmWildcardTypeReference__ConstraintsAssignment_2_1_0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37276:1: ( ( rule__JvmWildcardTypeReference__ConstraintsAssignment_2_1_0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37276:1: ( ( rule__JvmWildcardTypeReference__ConstraintsAssignment_2_1_0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37277:1: ( rule__JvmWildcardTypeReference__ConstraintsAssignment_2_1_0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getJvmWildcardTypeReferenceAccess().getConstraintsAssignment_2_1_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37278:1: ( rule__JvmWildcardTypeReference__ConstraintsAssignment_2_1_0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37278:2: rule__JvmWildcardTypeReference__ConstraintsAssignment_2_1_0
{
pushFollow(FollowSets003.FOLLOW_rule__JvmWildcardTypeReference__ConstraintsAssignment_2_1_0_in_rule__JvmWildcardTypeReference__Group_2_1__0__Impl74811);
rule__JvmWildcardTypeReference__ConstraintsAssignment_2_1_0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getJvmWildcardTypeReferenceAccess().getConstraintsAssignment_2_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__JvmWildcardTypeReference__Group_2_1__0__Impl"
// $ANTLR start "rule__JvmWildcardTypeReference__Group_2_1__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37288:1: rule__JvmWildcardTypeReference__Group_2_1__1 : rule__JvmWildcardTypeReference__Group_2_1__1__Impl ;
public final void rule__JvmWildcardTypeReference__Group_2_1__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37292:1: ( rule__JvmWildcardTypeReference__Group_2_1__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37293:2: rule__JvmWildcardTypeReference__Group_2_1__1__Impl
{
pushFollow(FollowSets003.FOLLOW_rule__JvmWildcardTypeReference__Group_2_1__1__Impl_in_rule__JvmWildcardTypeReference__Group_2_1__174841);
rule__JvmWildcardTypeReference__Group_2_1__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__JvmWildcardTypeReference__Group_2_1__1"
// $ANTLR start "rule__JvmWildcardTypeReference__Group_2_1__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37299:1: rule__JvmWildcardTypeReference__Group_2_1__1__Impl : ( ( rule__JvmWildcardTypeReference__ConstraintsAssignment_2_1_1 )* ) ;
public final void rule__JvmWildcardTypeReference__Group_2_1__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37303:1: ( ( ( rule__JvmWildcardTypeReference__ConstraintsAssignment_2_1_1 )* ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37304:1: ( ( rule__JvmWildcardTypeReference__ConstraintsAssignment_2_1_1 )* )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37304:1: ( ( rule__JvmWildcardTypeReference__ConstraintsAssignment_2_1_1 )* )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37305:1: ( rule__JvmWildcardTypeReference__ConstraintsAssignment_2_1_1 )*
{
if ( state.backtracking==0 ) {
before(grammarAccess.getJvmWildcardTypeReferenceAccess().getConstraintsAssignment_2_1_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37306:1: ( rule__JvmWildcardTypeReference__ConstraintsAssignment_2_1_1 )*
loop269:
do {
int alt269=2;
int LA269_0 = input.LA(1);
if ( (LA269_0==178) ) {
alt269=1;
}
switch (alt269) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37306:2: rule__JvmWildcardTypeReference__ConstraintsAssignment_2_1_1
{
pushFollow(FollowSets003.FOLLOW_rule__JvmWildcardTypeReference__ConstraintsAssignment_2_1_1_in_rule__JvmWildcardTypeReference__Group_2_1__1__Impl74868);
rule__JvmWildcardTypeReference__ConstraintsAssignment_2_1_1();
state._fsp--;
if (state.failed) return ;
}
break;
default :
break loop269;
}
} while (true);
if ( state.backtracking==0 ) {
after(grammarAccess.getJvmWildcardTypeReferenceAccess().getConstraintsAssignment_2_1_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__JvmWildcardTypeReference__Group_2_1__1__Impl"
// $ANTLR start "rule__JvmUpperBound__Group__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37320:1: rule__JvmUpperBound__Group__0 : rule__JvmUpperBound__Group__0__Impl rule__JvmUpperBound__Group__1 ;
public final void rule__JvmUpperBound__Group__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37324:1: ( rule__JvmUpperBound__Group__0__Impl rule__JvmUpperBound__Group__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37325:2: rule__JvmUpperBound__Group__0__Impl rule__JvmUpperBound__Group__1
{
pushFollow(FollowSets003.FOLLOW_rule__JvmUpperBound__Group__0__Impl_in_rule__JvmUpperBound__Group__074903);
rule__JvmUpperBound__Group__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets003.FOLLOW_rule__JvmUpperBound__Group__1_in_rule__JvmUpperBound__Group__074906);
rule__JvmUpperBound__Group__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__JvmUpperBound__Group__0"
// $ANTLR start "rule__JvmUpperBound__Group__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37332:1: rule__JvmUpperBound__Group__0__Impl : ( 'extends' ) ;
public final void rule__JvmUpperBound__Group__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37336:1: ( ( 'extends' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37337:1: ( 'extends' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37337:1: ( 'extends' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37338:1: 'extends'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getJvmUpperBoundAccess().getExtendsKeyword_0());
}
match(input,45,FollowSets003.FOLLOW_45_in_rule__JvmUpperBound__Group__0__Impl74934); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getJvmUpperBoundAccess().getExtendsKeyword_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__JvmUpperBound__Group__0__Impl"
// $ANTLR start "rule__JvmUpperBound__Group__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37351:1: rule__JvmUpperBound__Group__1 : rule__JvmUpperBound__Group__1__Impl ;
public final void rule__JvmUpperBound__Group__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37355:1: ( rule__JvmUpperBound__Group__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37356:2: rule__JvmUpperBound__Group__1__Impl
{
pushFollow(FollowSets003.FOLLOW_rule__JvmUpperBound__Group__1__Impl_in_rule__JvmUpperBound__Group__174965);
rule__JvmUpperBound__Group__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__JvmUpperBound__Group__1"
// $ANTLR start "rule__JvmUpperBound__Group__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37362:1: rule__JvmUpperBound__Group__1__Impl : ( ( rule__JvmUpperBound__TypeReferenceAssignment_1 ) ) ;
public final void rule__JvmUpperBound__Group__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37366:1: ( ( ( rule__JvmUpperBound__TypeReferenceAssignment_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37367:1: ( ( rule__JvmUpperBound__TypeReferenceAssignment_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37367:1: ( ( rule__JvmUpperBound__TypeReferenceAssignment_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37368:1: ( rule__JvmUpperBound__TypeReferenceAssignment_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getJvmUpperBoundAccess().getTypeReferenceAssignment_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37369:1: ( rule__JvmUpperBound__TypeReferenceAssignment_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37369:2: rule__JvmUpperBound__TypeReferenceAssignment_1
{
pushFollow(FollowSets003.FOLLOW_rule__JvmUpperBound__TypeReferenceAssignment_1_in_rule__JvmUpperBound__Group__1__Impl74992);
rule__JvmUpperBound__TypeReferenceAssignment_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getJvmUpperBoundAccess().getTypeReferenceAssignment_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__JvmUpperBound__Group__1__Impl"
// $ANTLR start "rule__JvmUpperBoundAnded__Group__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37383:1: rule__JvmUpperBoundAnded__Group__0 : rule__JvmUpperBoundAnded__Group__0__Impl rule__JvmUpperBoundAnded__Group__1 ;
public final void rule__JvmUpperBoundAnded__Group__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37387:1: ( rule__JvmUpperBoundAnded__Group__0__Impl rule__JvmUpperBoundAnded__Group__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37388:2: rule__JvmUpperBoundAnded__Group__0__Impl rule__JvmUpperBoundAnded__Group__1
{
pushFollow(FollowSets003.FOLLOW_rule__JvmUpperBoundAnded__Group__0__Impl_in_rule__JvmUpperBoundAnded__Group__075026);
rule__JvmUpperBoundAnded__Group__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets003.FOLLOW_rule__JvmUpperBoundAnded__Group__1_in_rule__JvmUpperBoundAnded__Group__075029);
rule__JvmUpperBoundAnded__Group__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__JvmUpperBoundAnded__Group__0"
// $ANTLR start "rule__JvmUpperBoundAnded__Group__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37395:1: rule__JvmUpperBoundAnded__Group__0__Impl : ( '&' ) ;
public final void rule__JvmUpperBoundAnded__Group__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37399:1: ( ( '&' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37400:1: ( '&' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37400:1: ( '&' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37401:1: '&'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getJvmUpperBoundAndedAccess().getAmpersandKeyword_0());
}
match(input,178,FollowSets003.FOLLOW_178_in_rule__JvmUpperBoundAnded__Group__0__Impl75057); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getJvmUpperBoundAndedAccess().getAmpersandKeyword_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__JvmUpperBoundAnded__Group__0__Impl"
// $ANTLR start "rule__JvmUpperBoundAnded__Group__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37414:1: rule__JvmUpperBoundAnded__Group__1 : rule__JvmUpperBoundAnded__Group__1__Impl ;
public final void rule__JvmUpperBoundAnded__Group__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37418:1: ( rule__JvmUpperBoundAnded__Group__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37419:2: rule__JvmUpperBoundAnded__Group__1__Impl
{
pushFollow(FollowSets003.FOLLOW_rule__JvmUpperBoundAnded__Group__1__Impl_in_rule__JvmUpperBoundAnded__Group__175088);
rule__JvmUpperBoundAnded__Group__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__JvmUpperBoundAnded__Group__1"
// $ANTLR start "rule__JvmUpperBoundAnded__Group__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37425:1: rule__JvmUpperBoundAnded__Group__1__Impl : ( ( rule__JvmUpperBoundAnded__TypeReferenceAssignment_1 ) ) ;
public final void rule__JvmUpperBoundAnded__Group__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37429:1: ( ( ( rule__JvmUpperBoundAnded__TypeReferenceAssignment_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37430:1: ( ( rule__JvmUpperBoundAnded__TypeReferenceAssignment_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37430:1: ( ( rule__JvmUpperBoundAnded__TypeReferenceAssignment_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37431:1: ( rule__JvmUpperBoundAnded__TypeReferenceAssignment_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getJvmUpperBoundAndedAccess().getTypeReferenceAssignment_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37432:1: ( rule__JvmUpperBoundAnded__TypeReferenceAssignment_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37432:2: rule__JvmUpperBoundAnded__TypeReferenceAssignment_1
{
pushFollow(FollowSets003.FOLLOW_rule__JvmUpperBoundAnded__TypeReferenceAssignment_1_in_rule__JvmUpperBoundAnded__Group__1__Impl75115);
rule__JvmUpperBoundAnded__TypeReferenceAssignment_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getJvmUpperBoundAndedAccess().getTypeReferenceAssignment_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__JvmUpperBoundAnded__Group__1__Impl"
// $ANTLR start "rule__JvmLowerBound__Group__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37446:1: rule__JvmLowerBound__Group__0 : rule__JvmLowerBound__Group__0__Impl rule__JvmLowerBound__Group__1 ;
public final void rule__JvmLowerBound__Group__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37450:1: ( rule__JvmLowerBound__Group__0__Impl rule__JvmLowerBound__Group__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37451:2: rule__JvmLowerBound__Group__0__Impl rule__JvmLowerBound__Group__1
{
pushFollow(FollowSets003.FOLLOW_rule__JvmLowerBound__Group__0__Impl_in_rule__JvmLowerBound__Group__075149);
rule__JvmLowerBound__Group__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets003.FOLLOW_rule__JvmLowerBound__Group__1_in_rule__JvmLowerBound__Group__075152);
rule__JvmLowerBound__Group__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__JvmLowerBound__Group__0"
// $ANTLR start "rule__JvmLowerBound__Group__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37458:1: rule__JvmLowerBound__Group__0__Impl : ( 'super' ) ;
public final void rule__JvmLowerBound__Group__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37462:1: ( ( 'super' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37463:1: ( 'super' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37463:1: ( 'super' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37464:1: 'super'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getJvmLowerBoundAccess().getSuperKeyword_0());
}
match(input,49,FollowSets003.FOLLOW_49_in_rule__JvmLowerBound__Group__0__Impl75180); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getJvmLowerBoundAccess().getSuperKeyword_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__JvmLowerBound__Group__0__Impl"
// $ANTLR start "rule__JvmLowerBound__Group__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37477:1: rule__JvmLowerBound__Group__1 : rule__JvmLowerBound__Group__1__Impl ;
public final void rule__JvmLowerBound__Group__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37481:1: ( rule__JvmLowerBound__Group__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37482:2: rule__JvmLowerBound__Group__1__Impl
{
pushFollow(FollowSets003.FOLLOW_rule__JvmLowerBound__Group__1__Impl_in_rule__JvmLowerBound__Group__175211);
rule__JvmLowerBound__Group__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__JvmLowerBound__Group__1"
// $ANTLR start "rule__JvmLowerBound__Group__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37488:1: rule__JvmLowerBound__Group__1__Impl : ( ( rule__JvmLowerBound__TypeReferenceAssignment_1 ) ) ;
public final void rule__JvmLowerBound__Group__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37492:1: ( ( ( rule__JvmLowerBound__TypeReferenceAssignment_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37493:1: ( ( rule__JvmLowerBound__TypeReferenceAssignment_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37493:1: ( ( rule__JvmLowerBound__TypeReferenceAssignment_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37494:1: ( rule__JvmLowerBound__TypeReferenceAssignment_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getJvmLowerBoundAccess().getTypeReferenceAssignment_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37495:1: ( rule__JvmLowerBound__TypeReferenceAssignment_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37495:2: rule__JvmLowerBound__TypeReferenceAssignment_1
{
pushFollow(FollowSets003.FOLLOW_rule__JvmLowerBound__TypeReferenceAssignment_1_in_rule__JvmLowerBound__Group__1__Impl75238);
rule__JvmLowerBound__TypeReferenceAssignment_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getJvmLowerBoundAccess().getTypeReferenceAssignment_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__JvmLowerBound__Group__1__Impl"
// $ANTLR start "rule__JvmLowerBoundAnded__Group__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37509:1: rule__JvmLowerBoundAnded__Group__0 : rule__JvmLowerBoundAnded__Group__0__Impl rule__JvmLowerBoundAnded__Group__1 ;
public final void rule__JvmLowerBoundAnded__Group__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37513:1: ( rule__JvmLowerBoundAnded__Group__0__Impl rule__JvmLowerBoundAnded__Group__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37514:2: rule__JvmLowerBoundAnded__Group__0__Impl rule__JvmLowerBoundAnded__Group__1
{
pushFollow(FollowSets003.FOLLOW_rule__JvmLowerBoundAnded__Group__0__Impl_in_rule__JvmLowerBoundAnded__Group__075272);
rule__JvmLowerBoundAnded__Group__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets003.FOLLOW_rule__JvmLowerBoundAnded__Group__1_in_rule__JvmLowerBoundAnded__Group__075275);
rule__JvmLowerBoundAnded__Group__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__JvmLowerBoundAnded__Group__0"
// $ANTLR start "rule__JvmLowerBoundAnded__Group__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37521:1: rule__JvmLowerBoundAnded__Group__0__Impl : ( '&' ) ;
public final void rule__JvmLowerBoundAnded__Group__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37525:1: ( ( '&' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37526:1: ( '&' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37526:1: ( '&' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37527:1: '&'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getJvmLowerBoundAndedAccess().getAmpersandKeyword_0());
}
match(input,178,FollowSets003.FOLLOW_178_in_rule__JvmLowerBoundAnded__Group__0__Impl75303); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getJvmLowerBoundAndedAccess().getAmpersandKeyword_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__JvmLowerBoundAnded__Group__0__Impl"
// $ANTLR start "rule__JvmLowerBoundAnded__Group__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37540:1: rule__JvmLowerBoundAnded__Group__1 : rule__JvmLowerBoundAnded__Group__1__Impl ;
public final void rule__JvmLowerBoundAnded__Group__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37544:1: ( rule__JvmLowerBoundAnded__Group__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37545:2: rule__JvmLowerBoundAnded__Group__1__Impl
{
pushFollow(FollowSets003.FOLLOW_rule__JvmLowerBoundAnded__Group__1__Impl_in_rule__JvmLowerBoundAnded__Group__175334);
rule__JvmLowerBoundAnded__Group__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__JvmLowerBoundAnded__Group__1"
// $ANTLR start "rule__JvmLowerBoundAnded__Group__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37551:1: rule__JvmLowerBoundAnded__Group__1__Impl : ( ( rule__JvmLowerBoundAnded__TypeReferenceAssignment_1 ) ) ;
public final void rule__JvmLowerBoundAnded__Group__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37555:1: ( ( ( rule__JvmLowerBoundAnded__TypeReferenceAssignment_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37556:1: ( ( rule__JvmLowerBoundAnded__TypeReferenceAssignment_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37556:1: ( ( rule__JvmLowerBoundAnded__TypeReferenceAssignment_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37557:1: ( rule__JvmLowerBoundAnded__TypeReferenceAssignment_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getJvmLowerBoundAndedAccess().getTypeReferenceAssignment_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37558:1: ( rule__JvmLowerBoundAnded__TypeReferenceAssignment_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37558:2: rule__JvmLowerBoundAnded__TypeReferenceAssignment_1
{
pushFollow(FollowSets003.FOLLOW_rule__JvmLowerBoundAnded__TypeReferenceAssignment_1_in_rule__JvmLowerBoundAnded__Group__1__Impl75361);
rule__JvmLowerBoundAnded__TypeReferenceAssignment_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getJvmLowerBoundAndedAccess().getTypeReferenceAssignment_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__JvmLowerBoundAnded__Group__1__Impl"
// $ANTLR start "rule__JvmTypeParameter__Group__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37572:1: rule__JvmTypeParameter__Group__0 : rule__JvmTypeParameter__Group__0__Impl rule__JvmTypeParameter__Group__1 ;
public final void rule__JvmTypeParameter__Group__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37576:1: ( rule__JvmTypeParameter__Group__0__Impl rule__JvmTypeParameter__Group__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37577:2: rule__JvmTypeParameter__Group__0__Impl rule__JvmTypeParameter__Group__1
{
pushFollow(FollowSets003.FOLLOW_rule__JvmTypeParameter__Group__0__Impl_in_rule__JvmTypeParameter__Group__075395);
rule__JvmTypeParameter__Group__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets003.FOLLOW_rule__JvmTypeParameter__Group__1_in_rule__JvmTypeParameter__Group__075398);
rule__JvmTypeParameter__Group__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__JvmTypeParameter__Group__0"
// $ANTLR start "rule__JvmTypeParameter__Group__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37584:1: rule__JvmTypeParameter__Group__0__Impl : ( ( rule__JvmTypeParameter__NameAssignment_0 ) ) ;
public final void rule__JvmTypeParameter__Group__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37588:1: ( ( ( rule__JvmTypeParameter__NameAssignment_0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37589:1: ( ( rule__JvmTypeParameter__NameAssignment_0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37589:1: ( ( rule__JvmTypeParameter__NameAssignment_0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37590:1: ( rule__JvmTypeParameter__NameAssignment_0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getJvmTypeParameterAccess().getNameAssignment_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37591:1: ( rule__JvmTypeParameter__NameAssignment_0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37591:2: rule__JvmTypeParameter__NameAssignment_0
{
pushFollow(FollowSets003.FOLLOW_rule__JvmTypeParameter__NameAssignment_0_in_rule__JvmTypeParameter__Group__0__Impl75425);
rule__JvmTypeParameter__NameAssignment_0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getJvmTypeParameterAccess().getNameAssignment_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__JvmTypeParameter__Group__0__Impl"
// $ANTLR start "rule__JvmTypeParameter__Group__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37601:1: rule__JvmTypeParameter__Group__1 : rule__JvmTypeParameter__Group__1__Impl ;
public final void rule__JvmTypeParameter__Group__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37605:1: ( rule__JvmTypeParameter__Group__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37606:2: rule__JvmTypeParameter__Group__1__Impl
{
pushFollow(FollowSets003.FOLLOW_rule__JvmTypeParameter__Group__1__Impl_in_rule__JvmTypeParameter__Group__175455);
rule__JvmTypeParameter__Group__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__JvmTypeParameter__Group__1"
// $ANTLR start "rule__JvmTypeParameter__Group__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37612:1: rule__JvmTypeParameter__Group__1__Impl : ( ( rule__JvmTypeParameter__Group_1__0 )? ) ;
public final void rule__JvmTypeParameter__Group__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37616:1: ( ( ( rule__JvmTypeParameter__Group_1__0 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37617:1: ( ( rule__JvmTypeParameter__Group_1__0 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37617:1: ( ( rule__JvmTypeParameter__Group_1__0 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37618:1: ( rule__JvmTypeParameter__Group_1__0 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getJvmTypeParameterAccess().getGroup_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37619:1: ( rule__JvmTypeParameter__Group_1__0 )?
int alt270=2;
int LA270_0 = input.LA(1);
if ( (LA270_0==45) ) {
alt270=1;
}
switch (alt270) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37619:2: rule__JvmTypeParameter__Group_1__0
{
pushFollow(FollowSets003.FOLLOW_rule__JvmTypeParameter__Group_1__0_in_rule__JvmTypeParameter__Group__1__Impl75482);
rule__JvmTypeParameter__Group_1__0();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getJvmTypeParameterAccess().getGroup_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__JvmTypeParameter__Group__1__Impl"
// $ANTLR start "rule__JvmTypeParameter__Group_1__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37633:1: rule__JvmTypeParameter__Group_1__0 : rule__JvmTypeParameter__Group_1__0__Impl rule__JvmTypeParameter__Group_1__1 ;
public final void rule__JvmTypeParameter__Group_1__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37637:1: ( rule__JvmTypeParameter__Group_1__0__Impl rule__JvmTypeParameter__Group_1__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37638:2: rule__JvmTypeParameter__Group_1__0__Impl rule__JvmTypeParameter__Group_1__1
{
pushFollow(FollowSets003.FOLLOW_rule__JvmTypeParameter__Group_1__0__Impl_in_rule__JvmTypeParameter__Group_1__075517);
rule__JvmTypeParameter__Group_1__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets003.FOLLOW_rule__JvmTypeParameter__Group_1__1_in_rule__JvmTypeParameter__Group_1__075520);
rule__JvmTypeParameter__Group_1__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__JvmTypeParameter__Group_1__0"
// $ANTLR start "rule__JvmTypeParameter__Group_1__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37645:1: rule__JvmTypeParameter__Group_1__0__Impl : ( ( rule__JvmTypeParameter__ConstraintsAssignment_1_0 ) ) ;
public final void rule__JvmTypeParameter__Group_1__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37649:1: ( ( ( rule__JvmTypeParameter__ConstraintsAssignment_1_0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37650:1: ( ( rule__JvmTypeParameter__ConstraintsAssignment_1_0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37650:1: ( ( rule__JvmTypeParameter__ConstraintsAssignment_1_0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37651:1: ( rule__JvmTypeParameter__ConstraintsAssignment_1_0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getJvmTypeParameterAccess().getConstraintsAssignment_1_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37652:1: ( rule__JvmTypeParameter__ConstraintsAssignment_1_0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37652:2: rule__JvmTypeParameter__ConstraintsAssignment_1_0
{
pushFollow(FollowSets003.FOLLOW_rule__JvmTypeParameter__ConstraintsAssignment_1_0_in_rule__JvmTypeParameter__Group_1__0__Impl75547);
rule__JvmTypeParameter__ConstraintsAssignment_1_0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getJvmTypeParameterAccess().getConstraintsAssignment_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__JvmTypeParameter__Group_1__0__Impl"
// $ANTLR start "rule__JvmTypeParameter__Group_1__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37662:1: rule__JvmTypeParameter__Group_1__1 : rule__JvmTypeParameter__Group_1__1__Impl ;
public final void rule__JvmTypeParameter__Group_1__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37666:1: ( rule__JvmTypeParameter__Group_1__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37667:2: rule__JvmTypeParameter__Group_1__1__Impl
{
pushFollow(FollowSets003.FOLLOW_rule__JvmTypeParameter__Group_1__1__Impl_in_rule__JvmTypeParameter__Group_1__175577);
rule__JvmTypeParameter__Group_1__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__JvmTypeParameter__Group_1__1"
// $ANTLR start "rule__JvmTypeParameter__Group_1__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37673:1: rule__JvmTypeParameter__Group_1__1__Impl : ( ( rule__JvmTypeParameter__ConstraintsAssignment_1_1 )* ) ;
public final void rule__JvmTypeParameter__Group_1__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37677:1: ( ( ( rule__JvmTypeParameter__ConstraintsAssignment_1_1 )* ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37678:1: ( ( rule__JvmTypeParameter__ConstraintsAssignment_1_1 )* )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37678:1: ( ( rule__JvmTypeParameter__ConstraintsAssignment_1_1 )* )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37679:1: ( rule__JvmTypeParameter__ConstraintsAssignment_1_1 )*
{
if ( state.backtracking==0 ) {
before(grammarAccess.getJvmTypeParameterAccess().getConstraintsAssignment_1_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37680:1: ( rule__JvmTypeParameter__ConstraintsAssignment_1_1 )*
loop271:
do {
int alt271=2;
int LA271_0 = input.LA(1);
if ( (LA271_0==178) ) {
alt271=1;
}
switch (alt271) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37680:2: rule__JvmTypeParameter__ConstraintsAssignment_1_1
{
pushFollow(FollowSets003.FOLLOW_rule__JvmTypeParameter__ConstraintsAssignment_1_1_in_rule__JvmTypeParameter__Group_1__1__Impl75604);
rule__JvmTypeParameter__ConstraintsAssignment_1_1();
state._fsp--;
if (state.failed) return ;
}
break;
default :
break loop271;
}
} while (true);
if ( state.backtracking==0 ) {
after(grammarAccess.getJvmTypeParameterAccess().getConstraintsAssignment_1_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__JvmTypeParameter__Group_1__1__Impl"
// $ANTLR start "rule__QualifiedNameWithWildcard__Group__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37694:1: rule__QualifiedNameWithWildcard__Group__0 : rule__QualifiedNameWithWildcard__Group__0__Impl rule__QualifiedNameWithWildcard__Group__1 ;
public final void rule__QualifiedNameWithWildcard__Group__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37698:1: ( rule__QualifiedNameWithWildcard__Group__0__Impl rule__QualifiedNameWithWildcard__Group__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37699:2: rule__QualifiedNameWithWildcard__Group__0__Impl rule__QualifiedNameWithWildcard__Group__1
{
pushFollow(FollowSets003.FOLLOW_rule__QualifiedNameWithWildcard__Group__0__Impl_in_rule__QualifiedNameWithWildcard__Group__075639);
rule__QualifiedNameWithWildcard__Group__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets003.FOLLOW_rule__QualifiedNameWithWildcard__Group__1_in_rule__QualifiedNameWithWildcard__Group__075642);
rule__QualifiedNameWithWildcard__Group__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__QualifiedNameWithWildcard__Group__0"
// $ANTLR start "rule__QualifiedNameWithWildcard__Group__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37706:1: rule__QualifiedNameWithWildcard__Group__0__Impl : ( ruleQualifiedName ) ;
public final void rule__QualifiedNameWithWildcard__Group__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37710:1: ( ( ruleQualifiedName ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37711:1: ( ruleQualifiedName )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37711:1: ( ruleQualifiedName )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37712:1: ruleQualifiedName
{
if ( state.backtracking==0 ) {
before(grammarAccess.getQualifiedNameWithWildcardAccess().getQualifiedNameParserRuleCall_0());
}
pushFollow(FollowSets003.FOLLOW_ruleQualifiedName_in_rule__QualifiedNameWithWildcard__Group__0__Impl75669);
ruleQualifiedName();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getQualifiedNameWithWildcardAccess().getQualifiedNameParserRuleCall_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__QualifiedNameWithWildcard__Group__0__Impl"
// $ANTLR start "rule__QualifiedNameWithWildcard__Group__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37723:1: rule__QualifiedNameWithWildcard__Group__1 : rule__QualifiedNameWithWildcard__Group__1__Impl rule__QualifiedNameWithWildcard__Group__2 ;
public final void rule__QualifiedNameWithWildcard__Group__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37727:1: ( rule__QualifiedNameWithWildcard__Group__1__Impl rule__QualifiedNameWithWildcard__Group__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37728:2: rule__QualifiedNameWithWildcard__Group__1__Impl rule__QualifiedNameWithWildcard__Group__2
{
pushFollow(FollowSets003.FOLLOW_rule__QualifiedNameWithWildcard__Group__1__Impl_in_rule__QualifiedNameWithWildcard__Group__175698);
rule__QualifiedNameWithWildcard__Group__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets003.FOLLOW_rule__QualifiedNameWithWildcard__Group__2_in_rule__QualifiedNameWithWildcard__Group__175701);
rule__QualifiedNameWithWildcard__Group__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__QualifiedNameWithWildcard__Group__1"
// $ANTLR start "rule__QualifiedNameWithWildcard__Group__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37735:1: rule__QualifiedNameWithWildcard__Group__1__Impl : ( '.' ) ;
public final void rule__QualifiedNameWithWildcard__Group__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37739:1: ( ( '.' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37740:1: ( '.' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37740:1: ( '.' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37741:1: '.'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getQualifiedNameWithWildcardAccess().getFullStopKeyword_1());
}
match(input,43,FollowSets003.FOLLOW_43_in_rule__QualifiedNameWithWildcard__Group__1__Impl75729); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getQualifiedNameWithWildcardAccess().getFullStopKeyword_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__QualifiedNameWithWildcard__Group__1__Impl"
// $ANTLR start "rule__QualifiedNameWithWildcard__Group__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37754:1: rule__QualifiedNameWithWildcard__Group__2 : rule__QualifiedNameWithWildcard__Group__2__Impl ;
public final void rule__QualifiedNameWithWildcard__Group__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37758:1: ( rule__QualifiedNameWithWildcard__Group__2__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37759:2: rule__QualifiedNameWithWildcard__Group__2__Impl
{
pushFollow(FollowSets003.FOLLOW_rule__QualifiedNameWithWildcard__Group__2__Impl_in_rule__QualifiedNameWithWildcard__Group__275760);
rule__QualifiedNameWithWildcard__Group__2__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__QualifiedNameWithWildcard__Group__2"
// $ANTLR start "rule__QualifiedNameWithWildcard__Group__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37765:1: rule__QualifiedNameWithWildcard__Group__2__Impl : ( '*' ) ;
public final void rule__QualifiedNameWithWildcard__Group__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37769:1: ( ( '*' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37770:1: ( '*' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37770:1: ( '*' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37771:1: '*'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getQualifiedNameWithWildcardAccess().getAsteriskKeyword_2());
}
match(input,36,FollowSets003.FOLLOW_36_in_rule__QualifiedNameWithWildcard__Group__2__Impl75788); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getQualifiedNameWithWildcardAccess().getAsteriskKeyword_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__QualifiedNameWithWildcard__Group__2__Impl"
// $ANTLR start "rule__XImportDeclaration__Group__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37790:1: rule__XImportDeclaration__Group__0 : rule__XImportDeclaration__Group__0__Impl rule__XImportDeclaration__Group__1 ;
public final void rule__XImportDeclaration__Group__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37794:1: ( rule__XImportDeclaration__Group__0__Impl rule__XImportDeclaration__Group__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37795:2: rule__XImportDeclaration__Group__0__Impl rule__XImportDeclaration__Group__1
{
pushFollow(FollowSets003.FOLLOW_rule__XImportDeclaration__Group__0__Impl_in_rule__XImportDeclaration__Group__075825);
rule__XImportDeclaration__Group__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets003.FOLLOW_rule__XImportDeclaration__Group__1_in_rule__XImportDeclaration__Group__075828);
rule__XImportDeclaration__Group__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XImportDeclaration__Group__0"
// $ANTLR start "rule__XImportDeclaration__Group__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37802:1: rule__XImportDeclaration__Group__0__Impl : ( 'import' ) ;
public final void rule__XImportDeclaration__Group__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37806:1: ( ( 'import' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37807:1: ( 'import' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37807:1: ( 'import' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37808:1: 'import'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXImportDeclarationAccess().getImportKeyword_0());
}
match(input,47,FollowSets003.FOLLOW_47_in_rule__XImportDeclaration__Group__0__Impl75856); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXImportDeclarationAccess().getImportKeyword_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XImportDeclaration__Group__0__Impl"
// $ANTLR start "rule__XImportDeclaration__Group__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37821:1: rule__XImportDeclaration__Group__1 : rule__XImportDeclaration__Group__1__Impl rule__XImportDeclaration__Group__2 ;
public final void rule__XImportDeclaration__Group__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37825:1: ( rule__XImportDeclaration__Group__1__Impl rule__XImportDeclaration__Group__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37826:2: rule__XImportDeclaration__Group__1__Impl rule__XImportDeclaration__Group__2
{
pushFollow(FollowSets003.FOLLOW_rule__XImportDeclaration__Group__1__Impl_in_rule__XImportDeclaration__Group__175887);
rule__XImportDeclaration__Group__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets003.FOLLOW_rule__XImportDeclaration__Group__2_in_rule__XImportDeclaration__Group__175890);
rule__XImportDeclaration__Group__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XImportDeclaration__Group__1"
// $ANTLR start "rule__XImportDeclaration__Group__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37833:1: rule__XImportDeclaration__Group__1__Impl : ( ( rule__XImportDeclaration__Alternatives_1 ) ) ;
public final void rule__XImportDeclaration__Group__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37837:1: ( ( ( rule__XImportDeclaration__Alternatives_1 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37838:1: ( ( rule__XImportDeclaration__Alternatives_1 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37838:1: ( ( rule__XImportDeclaration__Alternatives_1 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37839:1: ( rule__XImportDeclaration__Alternatives_1 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXImportDeclarationAccess().getAlternatives_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37840:1: ( rule__XImportDeclaration__Alternatives_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37840:2: rule__XImportDeclaration__Alternatives_1
{
pushFollow(FollowSets003.FOLLOW_rule__XImportDeclaration__Alternatives_1_in_rule__XImportDeclaration__Group__1__Impl75917);
rule__XImportDeclaration__Alternatives_1();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXImportDeclarationAccess().getAlternatives_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XImportDeclaration__Group__1__Impl"
// $ANTLR start "rule__XImportDeclaration__Group__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37850:1: rule__XImportDeclaration__Group__2 : rule__XImportDeclaration__Group__2__Impl ;
public final void rule__XImportDeclaration__Group__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37854:1: ( rule__XImportDeclaration__Group__2__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37855:2: rule__XImportDeclaration__Group__2__Impl
{
pushFollow(FollowSets003.FOLLOW_rule__XImportDeclaration__Group__2__Impl_in_rule__XImportDeclaration__Group__275947);
rule__XImportDeclaration__Group__2__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XImportDeclaration__Group__2"
// $ANTLR start "rule__XImportDeclaration__Group__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37861:1: rule__XImportDeclaration__Group__2__Impl : ( ( ';' )? ) ;
public final void rule__XImportDeclaration__Group__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37865:1: ( ( ( ';' )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37866:1: ( ( ';' )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37866:1: ( ( ';' )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37867:1: ( ';' )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXImportDeclarationAccess().getSemicolonKeyword_2());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37868:1: ( ';' )?
int alt272=2;
int LA272_0 = input.LA(1);
if ( (LA272_0==158) ) {
alt272=1;
}
switch (alt272) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37869:2: ';'
{
match(input,158,FollowSets003.FOLLOW_158_in_rule__XImportDeclaration__Group__2__Impl75976); if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXImportDeclarationAccess().getSemicolonKeyword_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XImportDeclaration__Group__2__Impl"
// $ANTLR start "rule__XImportDeclaration__Group_1_0__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37886:1: rule__XImportDeclaration__Group_1_0__0 : rule__XImportDeclaration__Group_1_0__0__Impl rule__XImportDeclaration__Group_1_0__1 ;
public final void rule__XImportDeclaration__Group_1_0__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37890:1: ( rule__XImportDeclaration__Group_1_0__0__Impl rule__XImportDeclaration__Group_1_0__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37891:2: rule__XImportDeclaration__Group_1_0__0__Impl rule__XImportDeclaration__Group_1_0__1
{
pushFollow(FollowSets003.FOLLOW_rule__XImportDeclaration__Group_1_0__0__Impl_in_rule__XImportDeclaration__Group_1_0__076015);
rule__XImportDeclaration__Group_1_0__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets003.FOLLOW_rule__XImportDeclaration__Group_1_0__1_in_rule__XImportDeclaration__Group_1_0__076018);
rule__XImportDeclaration__Group_1_0__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XImportDeclaration__Group_1_0__0"
// $ANTLR start "rule__XImportDeclaration__Group_1_0__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37898:1: rule__XImportDeclaration__Group_1_0__0__Impl : ( ( rule__XImportDeclaration__StaticAssignment_1_0_0 ) ) ;
public final void rule__XImportDeclaration__Group_1_0__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37902:1: ( ( ( rule__XImportDeclaration__StaticAssignment_1_0_0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37903:1: ( ( rule__XImportDeclaration__StaticAssignment_1_0_0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37903:1: ( ( rule__XImportDeclaration__StaticAssignment_1_0_0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37904:1: ( rule__XImportDeclaration__StaticAssignment_1_0_0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXImportDeclarationAccess().getStaticAssignment_1_0_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37905:1: ( rule__XImportDeclaration__StaticAssignment_1_0_0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37905:2: rule__XImportDeclaration__StaticAssignment_1_0_0
{
pushFollow(FollowSets003.FOLLOW_rule__XImportDeclaration__StaticAssignment_1_0_0_in_rule__XImportDeclaration__Group_1_0__0__Impl76045);
rule__XImportDeclaration__StaticAssignment_1_0_0();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXImportDeclarationAccess().getStaticAssignment_1_0_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XImportDeclaration__Group_1_0__0__Impl"
// $ANTLR start "rule__XImportDeclaration__Group_1_0__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37915:1: rule__XImportDeclaration__Group_1_0__1 : rule__XImportDeclaration__Group_1_0__1__Impl rule__XImportDeclaration__Group_1_0__2 ;
public final void rule__XImportDeclaration__Group_1_0__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37919:1: ( rule__XImportDeclaration__Group_1_0__1__Impl rule__XImportDeclaration__Group_1_0__2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37920:2: rule__XImportDeclaration__Group_1_0__1__Impl rule__XImportDeclaration__Group_1_0__2
{
pushFollow(FollowSets003.FOLLOW_rule__XImportDeclaration__Group_1_0__1__Impl_in_rule__XImportDeclaration__Group_1_0__176075);
rule__XImportDeclaration__Group_1_0__1__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets003.FOLLOW_rule__XImportDeclaration__Group_1_0__2_in_rule__XImportDeclaration__Group_1_0__176078);
rule__XImportDeclaration__Group_1_0__2();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XImportDeclaration__Group_1_0__1"
// $ANTLR start "rule__XImportDeclaration__Group_1_0__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37927:1: rule__XImportDeclaration__Group_1_0__1__Impl : ( ( rule__XImportDeclaration__ExtensionAssignment_1_0_1 )? ) ;
public final void rule__XImportDeclaration__Group_1_0__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37931:1: ( ( ( rule__XImportDeclaration__ExtensionAssignment_1_0_1 )? ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37932:1: ( ( rule__XImportDeclaration__ExtensionAssignment_1_0_1 )? )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37932:1: ( ( rule__XImportDeclaration__ExtensionAssignment_1_0_1 )? )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37933:1: ( rule__XImportDeclaration__ExtensionAssignment_1_0_1 )?
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXImportDeclarationAccess().getExtensionAssignment_1_0_1());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37934:1: ( rule__XImportDeclaration__ExtensionAssignment_1_0_1 )?
int alt273=2;
int LA273_0 = input.LA(1);
if ( (LA273_0==48) ) {
alt273=1;
}
switch (alt273) {
case 1 :
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37934:2: rule__XImportDeclaration__ExtensionAssignment_1_0_1
{
pushFollow(FollowSets003.FOLLOW_rule__XImportDeclaration__ExtensionAssignment_1_0_1_in_rule__XImportDeclaration__Group_1_0__1__Impl76105);
rule__XImportDeclaration__ExtensionAssignment_1_0_1();
state._fsp--;
if (state.failed) return ;
}
break;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXImportDeclarationAccess().getExtensionAssignment_1_0_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XImportDeclaration__Group_1_0__1__Impl"
// $ANTLR start "rule__XImportDeclaration__Group_1_0__2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37944:1: rule__XImportDeclaration__Group_1_0__2 : rule__XImportDeclaration__Group_1_0__2__Impl rule__XImportDeclaration__Group_1_0__3 ;
public final void rule__XImportDeclaration__Group_1_0__2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37948:1: ( rule__XImportDeclaration__Group_1_0__2__Impl rule__XImportDeclaration__Group_1_0__3 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37949:2: rule__XImportDeclaration__Group_1_0__2__Impl rule__XImportDeclaration__Group_1_0__3
{
pushFollow(FollowSets003.FOLLOW_rule__XImportDeclaration__Group_1_0__2__Impl_in_rule__XImportDeclaration__Group_1_0__276136);
rule__XImportDeclaration__Group_1_0__2__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets003.FOLLOW_rule__XImportDeclaration__Group_1_0__3_in_rule__XImportDeclaration__Group_1_0__276139);
rule__XImportDeclaration__Group_1_0__3();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XImportDeclaration__Group_1_0__2"
// $ANTLR start "rule__XImportDeclaration__Group_1_0__2__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37956:1: rule__XImportDeclaration__Group_1_0__2__Impl : ( ( rule__XImportDeclaration__ImportedTypeAssignment_1_0_2 ) ) ;
public final void rule__XImportDeclaration__Group_1_0__2__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37960:1: ( ( ( rule__XImportDeclaration__ImportedTypeAssignment_1_0_2 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37961:1: ( ( rule__XImportDeclaration__ImportedTypeAssignment_1_0_2 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37961:1: ( ( rule__XImportDeclaration__ImportedTypeAssignment_1_0_2 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37962:1: ( rule__XImportDeclaration__ImportedTypeAssignment_1_0_2 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXImportDeclarationAccess().getImportedTypeAssignment_1_0_2());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37963:1: ( rule__XImportDeclaration__ImportedTypeAssignment_1_0_2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37963:2: rule__XImportDeclaration__ImportedTypeAssignment_1_0_2
{
pushFollow(FollowSets003.FOLLOW_rule__XImportDeclaration__ImportedTypeAssignment_1_0_2_in_rule__XImportDeclaration__Group_1_0__2__Impl76166);
rule__XImportDeclaration__ImportedTypeAssignment_1_0_2();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXImportDeclarationAccess().getImportedTypeAssignment_1_0_2());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XImportDeclaration__Group_1_0__2__Impl"
// $ANTLR start "rule__XImportDeclaration__Group_1_0__3"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37973:1: rule__XImportDeclaration__Group_1_0__3 : rule__XImportDeclaration__Group_1_0__3__Impl ;
public final void rule__XImportDeclaration__Group_1_0__3() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37977:1: ( rule__XImportDeclaration__Group_1_0__3__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37978:2: rule__XImportDeclaration__Group_1_0__3__Impl
{
pushFollow(FollowSets003.FOLLOW_rule__XImportDeclaration__Group_1_0__3__Impl_in_rule__XImportDeclaration__Group_1_0__376196);
rule__XImportDeclaration__Group_1_0__3__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XImportDeclaration__Group_1_0__3"
// $ANTLR start "rule__XImportDeclaration__Group_1_0__3__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37984:1: rule__XImportDeclaration__Group_1_0__3__Impl : ( ( rule__XImportDeclaration__Alternatives_1_0_3 ) ) ;
public final void rule__XImportDeclaration__Group_1_0__3__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37988:1: ( ( ( rule__XImportDeclaration__Alternatives_1_0_3 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37989:1: ( ( rule__XImportDeclaration__Alternatives_1_0_3 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37989:1: ( ( rule__XImportDeclaration__Alternatives_1_0_3 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37990:1: ( rule__XImportDeclaration__Alternatives_1_0_3 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXImportDeclarationAccess().getAlternatives_1_0_3());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37991:1: ( rule__XImportDeclaration__Alternatives_1_0_3 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:37991:2: rule__XImportDeclaration__Alternatives_1_0_3
{
pushFollow(FollowSets003.FOLLOW_rule__XImportDeclaration__Alternatives_1_0_3_in_rule__XImportDeclaration__Group_1_0__3__Impl76223);
rule__XImportDeclaration__Alternatives_1_0_3();
state._fsp--;
if (state.failed) return ;
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXImportDeclarationAccess().getAlternatives_1_0_3());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XImportDeclaration__Group_1_0__3__Impl"
// $ANTLR start "rule__QualifiedNameInStaticImport__Group__0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38009:1: rule__QualifiedNameInStaticImport__Group__0 : rule__QualifiedNameInStaticImport__Group__0__Impl rule__QualifiedNameInStaticImport__Group__1 ;
public final void rule__QualifiedNameInStaticImport__Group__0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38013:1: ( rule__QualifiedNameInStaticImport__Group__0__Impl rule__QualifiedNameInStaticImport__Group__1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38014:2: rule__QualifiedNameInStaticImport__Group__0__Impl rule__QualifiedNameInStaticImport__Group__1
{
pushFollow(FollowSets003.FOLLOW_rule__QualifiedNameInStaticImport__Group__0__Impl_in_rule__QualifiedNameInStaticImport__Group__076261);
rule__QualifiedNameInStaticImport__Group__0__Impl();
state._fsp--;
if (state.failed) return ;
pushFollow(FollowSets003.FOLLOW_rule__QualifiedNameInStaticImport__Group__1_in_rule__QualifiedNameInStaticImport__Group__076264);
rule__QualifiedNameInStaticImport__Group__1();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__QualifiedNameInStaticImport__Group__0"
// $ANTLR start "rule__QualifiedNameInStaticImport__Group__0__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38021:1: rule__QualifiedNameInStaticImport__Group__0__Impl : ( ruleValidID ) ;
public final void rule__QualifiedNameInStaticImport__Group__0__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38025:1: ( ( ruleValidID ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38026:1: ( ruleValidID )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38026:1: ( ruleValidID )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38027:1: ruleValidID
{
if ( state.backtracking==0 ) {
before(grammarAccess.getQualifiedNameInStaticImportAccess().getValidIDParserRuleCall_0());
}
pushFollow(FollowSets003.FOLLOW_ruleValidID_in_rule__QualifiedNameInStaticImport__Group__0__Impl76291);
ruleValidID();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getQualifiedNameInStaticImportAccess().getValidIDParserRuleCall_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__QualifiedNameInStaticImport__Group__0__Impl"
// $ANTLR start "rule__QualifiedNameInStaticImport__Group__1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38038:1: rule__QualifiedNameInStaticImport__Group__1 : rule__QualifiedNameInStaticImport__Group__1__Impl ;
public final void rule__QualifiedNameInStaticImport__Group__1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38042:1: ( rule__QualifiedNameInStaticImport__Group__1__Impl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38043:2: rule__QualifiedNameInStaticImport__Group__1__Impl
{
pushFollow(FollowSets003.FOLLOW_rule__QualifiedNameInStaticImport__Group__1__Impl_in_rule__QualifiedNameInStaticImport__Group__176320);
rule__QualifiedNameInStaticImport__Group__1__Impl();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__QualifiedNameInStaticImport__Group__1"
// $ANTLR start "rule__QualifiedNameInStaticImport__Group__1__Impl"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38049:1: rule__QualifiedNameInStaticImport__Group__1__Impl : ( '.' ) ;
public final void rule__QualifiedNameInStaticImport__Group__1__Impl() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38053:1: ( ( '.' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38054:1: ( '.' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38054:1: ( '.' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38055:1: '.'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getQualifiedNameInStaticImportAccess().getFullStopKeyword_1());
}
match(input,43,FollowSets003.FOLLOW_43_in_rule__QualifiedNameInStaticImport__Group__1__Impl76348); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getQualifiedNameInStaticImportAccess().getFullStopKeyword_1());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__QualifiedNameInStaticImport__Group__1__Impl"
// $ANTLR start "rule__ModelRoot__ImportSectionAssignment_0_0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38073:1: rule__ModelRoot__ImportSectionAssignment_0_0 : ( ruleXImportSection ) ;
public final void rule__ModelRoot__ImportSectionAssignment_0_0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38077:1: ( ( ruleXImportSection ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38078:1: ( ruleXImportSection )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38078:1: ( ruleXImportSection )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38079:1: ruleXImportSection
{
if ( state.backtracking==0 ) {
before(grammarAccess.getModelRootAccess().getImportSectionXImportSectionParserRuleCall_0_0_0());
}
pushFollow(FollowSets003.FOLLOW_ruleXImportSection_in_rule__ModelRoot__ImportSectionAssignment_0_076388);
ruleXImportSection();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getModelRootAccess().getImportSectionXImportSectionParserRuleCall_0_0_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ModelRoot__ImportSectionAssignment_0_0"
// $ANTLR start "rule__ModelRoot__ModelRootAssignment_0_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38088:1: rule__ModelRoot__ModelRootAssignment_0_1 : ( ruleModel ) ;
public final void rule__ModelRoot__ModelRootAssignment_0_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38092:1: ( ( ruleModel ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38093:1: ( ruleModel )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38093:1: ( ruleModel )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38094:1: ruleModel
{
if ( state.backtracking==0 ) {
before(grammarAccess.getModelRootAccess().getModelRootModelParserRuleCall_0_1_0());
}
pushFollow(FollowSets003.FOLLOW_ruleModel_in_rule__ModelRoot__ModelRootAssignment_0_176419);
ruleModel();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getModelRootAccess().getModelRootModelParserRuleCall_0_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ModelRoot__ModelRootAssignment_0_1"
// $ANTLR start "rule__Model__ModelNameAssignment_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38103:1: rule__Model__ModelNameAssignment_1 : ( ruleValidID ) ;
public final void rule__Model__ModelNameAssignment_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38107:1: ( ( ruleValidID ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38108:1: ( ruleValidID )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38108:1: ( ruleValidID )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38109:1: ruleValidID
{
if ( state.backtracking==0 ) {
before(grammarAccess.getModelAccess().getModelNameValidIDParserRuleCall_1_0());
}
pushFollow(FollowSets003.FOLLOW_ruleValidID_in_rule__Model__ModelNameAssignment_176450);
ruleValidID();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getModelAccess().getModelNameValidIDParserRuleCall_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Model__ModelNameAssignment_1"
// $ANTLR start "rule__Model__ElementsAssignment_3"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38118:1: rule__Model__ElementsAssignment_3 : ( rulePackageDeclaration ) ;
public final void rule__Model__ElementsAssignment_3() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38122:1: ( ( rulePackageDeclaration ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38123:1: ( rulePackageDeclaration )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38123:1: ( rulePackageDeclaration )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38124:1: rulePackageDeclaration
{
if ( state.backtracking==0 ) {
before(grammarAccess.getModelAccess().getElementsPackageDeclarationParserRuleCall_3_0());
}
pushFollow(FollowSets003.FOLLOW_rulePackageDeclaration_in_rule__Model__ElementsAssignment_376481);
rulePackageDeclaration();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getModelAccess().getElementsPackageDeclarationParserRuleCall_3_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Model__ElementsAssignment_3"
// $ANTLR start "rule__PackageDeclaration__PackageNameAssignment_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38133:1: rule__PackageDeclaration__PackageNameAssignment_1 : ( ruleQualifiedName ) ;
public final void rule__PackageDeclaration__PackageNameAssignment_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38137:1: ( ( ruleQualifiedName ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38138:1: ( ruleQualifiedName )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38138:1: ( ruleQualifiedName )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38139:1: ruleQualifiedName
{
if ( state.backtracking==0 ) {
before(grammarAccess.getPackageDeclarationAccess().getPackageNameQualifiedNameParserRuleCall_1_0());
}
pushFollow(FollowSets003.FOLLOW_ruleQualifiedName_in_rule__PackageDeclaration__PackageNameAssignment_176512);
ruleQualifiedName();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getPackageDeclarationAccess().getPackageNameQualifiedNameParserRuleCall_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__PackageDeclaration__PackageNameAssignment_1"
// $ANTLR start "rule__PackageDeclaration__ElementsAssignment_3"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38148:1: rule__PackageDeclaration__ElementsAssignment_3 : ( ruleAbstractElement ) ;
public final void rule__PackageDeclaration__ElementsAssignment_3() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38152:1: ( ( ruleAbstractElement ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38153:1: ( ruleAbstractElement )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38153:1: ( ruleAbstractElement )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38154:1: ruleAbstractElement
{
if ( state.backtracking==0 ) {
before(grammarAccess.getPackageDeclarationAccess().getElementsAbstractElementParserRuleCall_3_0());
}
pushFollow(FollowSets003.FOLLOW_ruleAbstractElement_in_rule__PackageDeclaration__ElementsAssignment_376543);
ruleAbstractElement();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getPackageDeclarationAccess().getElementsAbstractElementParserRuleCall_3_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__PackageDeclaration__ElementsAssignment_3"
// $ANTLR start "rule__Enumeration__NameAssignment_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38163:1: rule__Enumeration__NameAssignment_1 : ( RULE_ID ) ;
public final void rule__Enumeration__NameAssignment_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38167:1: ( ( RULE_ID ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38168:1: ( RULE_ID )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38168:1: ( RULE_ID )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38169:1: RULE_ID
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEnumerationAccess().getNameIDTerminalRuleCall_1_0());
}
match(input,RULE_ID,FollowSets003.FOLLOW_RULE_ID_in_rule__Enumeration__NameAssignment_176574); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getEnumerationAccess().getNameIDTerminalRuleCall_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Enumeration__NameAssignment_1"
// $ANTLR start "rule__Enumeration__EnumerationValuesAssignment_3"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38178:1: rule__Enumeration__EnumerationValuesAssignment_3 : ( ruleEnumerationValue ) ;
public final void rule__Enumeration__EnumerationValuesAssignment_3() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38182:1: ( ( ruleEnumerationValue ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38183:1: ( ruleEnumerationValue )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38183:1: ( ruleEnumerationValue )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38184:1: ruleEnumerationValue
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEnumerationAccess().getEnumerationValuesEnumerationValueParserRuleCall_3_0());
}
pushFollow(FollowSets003.FOLLOW_ruleEnumerationValue_in_rule__Enumeration__EnumerationValuesAssignment_376605);
ruleEnumerationValue();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getEnumerationAccess().getEnumerationValuesEnumerationValueParserRuleCall_3_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Enumeration__EnumerationValuesAssignment_3"
// $ANTLR start "rule__EnumerationValue__NameAssignment_0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38193:1: rule__EnumerationValue__NameAssignment_0 : ( RULE_ID ) ;
public final void rule__EnumerationValue__NameAssignment_0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38197:1: ( ( RULE_ID ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38198:1: ( RULE_ID )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38198:1: ( RULE_ID )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38199:1: RULE_ID
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEnumerationValueAccess().getNameIDTerminalRuleCall_0_0());
}
match(input,RULE_ID,FollowSets003.FOLLOW_RULE_ID_in_rule__EnumerationValue__NameAssignment_076636); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getEnumerationValueAccess().getNameIDTerminalRuleCall_0_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EnumerationValue__NameAssignment_0"
// $ANTLR start "rule__EnumerationValue__ValueAssignment_1_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38208:1: rule__EnumerationValue__ValueAssignment_1_1 : ( RULE_STRING ) ;
public final void rule__EnumerationValue__ValueAssignment_1_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38212:1: ( ( RULE_STRING ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38213:1: ( RULE_STRING )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38213:1: ( RULE_STRING )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38214:1: RULE_STRING
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEnumerationValueAccess().getValueSTRINGTerminalRuleCall_1_1_0());
}
match(input,RULE_STRING,FollowSets003.FOLLOW_RULE_STRING_in_rule__EnumerationValue__ValueAssignment_1_176667); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getEnumerationValueAccess().getValueSTRINGTerminalRuleCall_1_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EnumerationValue__ValueAssignment_1_1"
// $ANTLR start "rule__EntityNaturalKeyFields__NaturalKeyAttributesAssignment_3"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38223:1: rule__EntityNaturalKeyFields__NaturalKeyAttributesAssignment_3 : ( ( ruleQualifiedName ) ) ;
public final void rule__EntityNaturalKeyFields__NaturalKeyAttributesAssignment_3() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38227:1: ( ( ( ruleQualifiedName ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38228:1: ( ( ruleQualifiedName ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38228:1: ( ( ruleQualifiedName ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38229:1: ( ruleQualifiedName )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEntityNaturalKeyFieldsAccess().getNaturalKeyAttributesEntityAttributeCrossReference_3_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38230:1: ( ruleQualifiedName )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38231:1: ruleQualifiedName
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEntityNaturalKeyFieldsAccess().getNaturalKeyAttributesEntityAttributeQualifiedNameParserRuleCall_3_0_1());
}
pushFollow(FollowSets003.FOLLOW_ruleQualifiedName_in_rule__EntityNaturalKeyFields__NaturalKeyAttributesAssignment_376702);
ruleQualifiedName();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getEntityNaturalKeyFieldsAccess().getNaturalKeyAttributesEntityAttributeQualifiedNameParserRuleCall_3_0_1());
}
}
if ( state.backtracking==0 ) {
after(grammarAccess.getEntityNaturalKeyFieldsAccess().getNaturalKeyAttributesEntityAttributeCrossReference_3_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EntityNaturalKeyFields__NaturalKeyAttributesAssignment_3"
// $ANTLR start "rule__EntityNaturalKeyFields__NaturalKeyAttributesAssignment_4_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38242:1: rule__EntityNaturalKeyFields__NaturalKeyAttributesAssignment_4_1 : ( ( ruleQualifiedName ) ) ;
public final void rule__EntityNaturalKeyFields__NaturalKeyAttributesAssignment_4_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38246:1: ( ( ( ruleQualifiedName ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38247:1: ( ( ruleQualifiedName ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38247:1: ( ( ruleQualifiedName ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38248:1: ( ruleQualifiedName )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEntityNaturalKeyFieldsAccess().getNaturalKeyAttributesEntityAttributeCrossReference_4_1_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38249:1: ( ruleQualifiedName )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38250:1: ruleQualifiedName
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEntityNaturalKeyFieldsAccess().getNaturalKeyAttributesEntityAttributeQualifiedNameParserRuleCall_4_1_0_1());
}
pushFollow(FollowSets003.FOLLOW_ruleQualifiedName_in_rule__EntityNaturalKeyFields__NaturalKeyAttributesAssignment_4_176741);
ruleQualifiedName();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getEntityNaturalKeyFieldsAccess().getNaturalKeyAttributesEntityAttributeQualifiedNameParserRuleCall_4_1_0_1());
}
}
if ( state.backtracking==0 ) {
after(grammarAccess.getEntityNaturalKeyFieldsAccess().getNaturalKeyAttributesEntityAttributeCrossReference_4_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EntityNaturalKeyFields__NaturalKeyAttributesAssignment_4_1"
// $ANTLR start "rule__EntityHierarchical__HierarchicalAssignment_2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38261:1: rule__EntityHierarchical__HierarchicalAssignment_2 : ( ruleXBooleanLiteral ) ;
public final void rule__EntityHierarchical__HierarchicalAssignment_2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38265:1: ( ( ruleXBooleanLiteral ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38266:1: ( ruleXBooleanLiteral )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38266:1: ( ruleXBooleanLiteral )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38267:1: ruleXBooleanLiteral
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEntityHierarchicalAccess().getHierarchicalXBooleanLiteralParserRuleCall_2_0());
}
pushFollow(FollowSets003.FOLLOW_ruleXBooleanLiteral_in_rule__EntityHierarchical__HierarchicalAssignment_276776);
ruleXBooleanLiteral();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getEntityHierarchicalAccess().getHierarchicalXBooleanLiteralParserRuleCall_2_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EntityHierarchical__HierarchicalAssignment_2"
// $ANTLR start "rule__EntityDisableIdField__DisableIdFieldAssignment_2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38276:1: rule__EntityDisableIdField__DisableIdFieldAssignment_2 : ( ruleXBooleanLiteral ) ;
public final void rule__EntityDisableIdField__DisableIdFieldAssignment_2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38280:1: ( ( ruleXBooleanLiteral ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38281:1: ( ruleXBooleanLiteral )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38281:1: ( ruleXBooleanLiteral )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38282:1: ruleXBooleanLiteral
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEntityDisableIdFieldAccess().getDisableIdFieldXBooleanLiteralParserRuleCall_2_0());
}
pushFollow(FollowSets003.FOLLOW_ruleXBooleanLiteral_in_rule__EntityDisableIdField__DisableIdFieldAssignment_276807);
ruleXBooleanLiteral();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getEntityDisableIdFieldAccess().getDisableIdFieldXBooleanLiteralParserRuleCall_2_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EntityDisableIdField__DisableIdFieldAssignment_2"
// $ANTLR start "rule__EntityLabelField__LabelAssignment_2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38291:1: rule__EntityLabelField__LabelAssignment_2 : ( RULE_STRING ) ;
public final void rule__EntityLabelField__LabelAssignment_2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38295:1: ( ( RULE_STRING ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38296:1: ( RULE_STRING )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38296:1: ( RULE_STRING )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38297:1: RULE_STRING
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEntityLabelFieldAccess().getLabelSTRINGTerminalRuleCall_2_0());
}
match(input,RULE_STRING,FollowSets003.FOLLOW_RULE_STRING_in_rule__EntityLabelField__LabelAssignment_276838); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getEntityLabelFieldAccess().getLabelSTRINGTerminalRuleCall_2_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EntityLabelField__LabelAssignment_2"
// $ANTLR start "rule__EntityPluralLabelField__PluralLabelAssignment_2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38306:1: rule__EntityPluralLabelField__PluralLabelAssignment_2 : ( RULE_STRING ) ;
public final void rule__EntityPluralLabelField__PluralLabelAssignment_2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38310:1: ( ( RULE_STRING ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38311:1: ( RULE_STRING )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38311:1: ( RULE_STRING )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38312:1: RULE_STRING
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEntityPluralLabelFieldAccess().getPluralLabelSTRINGTerminalRuleCall_2_0());
}
match(input,RULE_STRING,FollowSets003.FOLLOW_RULE_STRING_in_rule__EntityPluralLabelField__PluralLabelAssignment_276869); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getEntityPluralLabelFieldAccess().getPluralLabelSTRINGTerminalRuleCall_2_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EntityPluralLabelField__PluralLabelAssignment_2"
// $ANTLR start "rule__EntityOptionsContainer__OptionsAssignment_3"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38322:1: rule__EntityOptionsContainer__OptionsAssignment_3 : ( ruleEntityOptions ) ;
public final void rule__EntityOptionsContainer__OptionsAssignment_3() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38326:1: ( ( ruleEntityOptions ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38327:1: ( ruleEntityOptions )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38327:1: ( ruleEntityOptions )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38328:1: ruleEntityOptions
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEntityOptionsContainerAccess().getOptionsEntityOptionsParserRuleCall_3_0());
}
pushFollow(FollowSets003.FOLLOW_ruleEntityOptions_in_rule__EntityOptionsContainer__OptionsAssignment_376901);
ruleEntityOptions();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getEntityOptionsContainerAccess().getOptionsEntityOptionsParserRuleCall_3_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EntityOptionsContainer__OptionsAssignment_3"
// $ANTLR start "rule__Entity__NameAssignment_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38337:1: rule__Entity__NameAssignment_1 : ( RULE_ID ) ;
public final void rule__Entity__NameAssignment_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38341:1: ( ( RULE_ID ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38342:1: ( RULE_ID )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38342:1: ( RULE_ID )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38343:1: RULE_ID
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEntityAccess().getNameIDTerminalRuleCall_1_0());
}
match(input,RULE_ID,FollowSets003.FOLLOW_RULE_ID_in_rule__Entity__NameAssignment_176932); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getEntityAccess().getNameIDTerminalRuleCall_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Entity__NameAssignment_1"
// $ANTLR start "rule__Entity__ExtendsAssignment_2_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38352:1: rule__Entity__ExtendsAssignment_2_1 : ( ( ruleQualifiedName ) ) ;
public final void rule__Entity__ExtendsAssignment_2_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38356:1: ( ( ( ruleQualifiedName ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38357:1: ( ( ruleQualifiedName ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38357:1: ( ( ruleQualifiedName ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38358:1: ( ruleQualifiedName )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEntityAccess().getExtendsEntityCrossReference_2_1_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38359:1: ( ruleQualifiedName )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38360:1: ruleQualifiedName
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEntityAccess().getExtendsEntityQualifiedNameParserRuleCall_2_1_0_1());
}
pushFollow(FollowSets003.FOLLOW_ruleQualifiedName_in_rule__Entity__ExtendsAssignment_2_176967);
ruleQualifiedName();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getEntityAccess().getExtendsEntityQualifiedNameParserRuleCall_2_1_0_1());
}
}
if ( state.backtracking==0 ) {
after(grammarAccess.getEntityAccess().getExtendsEntityCrossReference_2_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Entity__ExtendsAssignment_2_1"
// $ANTLR start "rule__Entity__JvmtypeAssignment_3_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38371:1: rule__Entity__JvmtypeAssignment_3_1 : ( ruleJvmTypeReference ) ;
public final void rule__Entity__JvmtypeAssignment_3_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38375:1: ( ( ruleJvmTypeReference ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38376:1: ( ruleJvmTypeReference )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38376:1: ( ruleJvmTypeReference )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38377:1: ruleJvmTypeReference
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEntityAccess().getJvmtypeJvmTypeReferenceParserRuleCall_3_1_0());
}
pushFollow(FollowSets003.FOLLOW_ruleJvmTypeReference_in_rule__Entity__JvmtypeAssignment_3_177002);
ruleJvmTypeReference();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getEntityAccess().getJvmtypeJvmTypeReferenceParserRuleCall_3_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Entity__JvmtypeAssignment_3_1"
// $ANTLR start "rule__Entity__EntityOptionsAssignment_5"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38386:1: rule__Entity__EntityOptionsAssignment_5 : ( ruleEntityOptionsContainer ) ;
public final void rule__Entity__EntityOptionsAssignment_5() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38390:1: ( ( ruleEntityOptionsContainer ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38391:1: ( ruleEntityOptionsContainer )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38391:1: ( ruleEntityOptionsContainer )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38392:1: ruleEntityOptionsContainer
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEntityAccess().getEntityOptionsEntityOptionsContainerParserRuleCall_5_0());
}
pushFollow(FollowSets003.FOLLOW_ruleEntityOptionsContainer_in_rule__Entity__EntityOptionsAssignment_577033);
ruleEntityOptionsContainer();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getEntityAccess().getEntityOptionsEntityOptionsContainerParserRuleCall_5_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Entity__EntityOptionsAssignment_5"
// $ANTLR start "rule__Entity__AttributesAssignment_6"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38401:1: rule__Entity__AttributesAssignment_6 : ( ruleEntityAttribute ) ;
public final void rule__Entity__AttributesAssignment_6() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38405:1: ( ( ruleEntityAttribute ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38406:1: ( ruleEntityAttribute )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38406:1: ( ruleEntityAttribute )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38407:1: ruleEntityAttribute
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEntityAccess().getAttributesEntityAttributeParserRuleCall_6_0());
}
pushFollow(FollowSets003.FOLLOW_ruleEntityAttribute_in_rule__Entity__AttributesAssignment_677064);
ruleEntityAttribute();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getEntityAccess().getAttributesEntityAttributeParserRuleCall_6_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Entity__AttributesAssignment_6"
// $ANTLR start "rule__ValueObject__NameAssignment_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38416:1: rule__ValueObject__NameAssignment_1 : ( RULE_ID ) ;
public final void rule__ValueObject__NameAssignment_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38420:1: ( ( RULE_ID ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38421:1: ( RULE_ID )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38421:1: ( RULE_ID )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38422:1: RULE_ID
{
if ( state.backtracking==0 ) {
before(grammarAccess.getValueObjectAccess().getNameIDTerminalRuleCall_1_0());
}
match(input,RULE_ID,FollowSets003.FOLLOW_RULE_ID_in_rule__ValueObject__NameAssignment_177095); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getValueObjectAccess().getNameIDTerminalRuleCall_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ValueObject__NameAssignment_1"
// $ANTLR start "rule__ValueObject__ExtendsAssignment_2_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38431:1: rule__ValueObject__ExtendsAssignment_2_1 : ( ( ruleQualifiedName ) ) ;
public final void rule__ValueObject__ExtendsAssignment_2_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38435:1: ( ( ( ruleQualifiedName ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38436:1: ( ( ruleQualifiedName ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38436:1: ( ( ruleQualifiedName ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38437:1: ( ruleQualifiedName )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getValueObjectAccess().getExtendsValueObjectCrossReference_2_1_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38438:1: ( ruleQualifiedName )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38439:1: ruleQualifiedName
{
if ( state.backtracking==0 ) {
before(grammarAccess.getValueObjectAccess().getExtendsValueObjectQualifiedNameParserRuleCall_2_1_0_1());
}
pushFollow(FollowSets003.FOLLOW_ruleQualifiedName_in_rule__ValueObject__ExtendsAssignment_2_177130);
ruleQualifiedName();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getValueObjectAccess().getExtendsValueObjectQualifiedNameParserRuleCall_2_1_0_1());
}
}
if ( state.backtracking==0 ) {
after(grammarAccess.getValueObjectAccess().getExtendsValueObjectCrossReference_2_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ValueObject__ExtendsAssignment_2_1"
// $ANTLR start "rule__ValueObject__JvmtypeAssignment_3_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38450:1: rule__ValueObject__JvmtypeAssignment_3_1 : ( ruleJvmTypeReference ) ;
public final void rule__ValueObject__JvmtypeAssignment_3_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38454:1: ( ( ruleJvmTypeReference ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38455:1: ( ruleJvmTypeReference )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38455:1: ( ruleJvmTypeReference )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38456:1: ruleJvmTypeReference
{
if ( state.backtracking==0 ) {
before(grammarAccess.getValueObjectAccess().getJvmtypeJvmTypeReferenceParserRuleCall_3_1_0());
}
pushFollow(FollowSets003.FOLLOW_ruleJvmTypeReference_in_rule__ValueObject__JvmtypeAssignment_3_177165);
ruleJvmTypeReference();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getValueObjectAccess().getJvmtypeJvmTypeReferenceParserRuleCall_3_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ValueObject__JvmtypeAssignment_3_1"
// $ANTLR start "rule__ValueObject__AttributesAssignment_5"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38465:1: rule__ValueObject__AttributesAssignment_5 : ( ruleEntityAttribute ) ;
public final void rule__ValueObject__AttributesAssignment_5() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38469:1: ( ( ruleEntityAttribute ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38470:1: ( ruleEntityAttribute )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38470:1: ( ruleEntityAttribute )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38471:1: ruleEntityAttribute
{
if ( state.backtracking==0 ) {
before(grammarAccess.getValueObjectAccess().getAttributesEntityAttributeParserRuleCall_5_0());
}
pushFollow(FollowSets003.FOLLOW_ruleEntityAttribute_in_rule__ValueObject__AttributesAssignment_577196);
ruleEntityAttribute();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getValueObjectAccess().getAttributesEntityAttributeParserRuleCall_5_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ValueObject__AttributesAssignment_5"
// $ANTLR start "rule__BaseDataType__BaseDatatypePropertiesAssignment_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38484:1: rule__BaseDataType__BaseDatatypePropertiesAssignment_1 : ( ruleBaseDataTypeProperties ) ;
public final void rule__BaseDataType__BaseDatatypePropertiesAssignment_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38488:1: ( ( ruleBaseDataTypeProperties ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38489:1: ( ruleBaseDataTypeProperties )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38489:1: ( ruleBaseDataTypeProperties )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38490:1: ruleBaseDataTypeProperties
{
if ( state.backtracking==0 ) {
before(grammarAccess.getBaseDataTypeAccess().getBaseDatatypePropertiesBaseDataTypePropertiesParserRuleCall_1_0());
}
pushFollow(FollowSets003.FOLLOW_ruleBaseDataTypeProperties_in_rule__BaseDataType__BaseDatatypePropertiesAssignment_177231);
ruleBaseDataTypeProperties();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getBaseDataTypeAccess().getBaseDatatypePropertiesBaseDataTypePropertiesParserRuleCall_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__BaseDataType__BaseDatatypePropertiesAssignment_1"
// $ANTLR start "rule__BaseDataTypeWidth__WidthAssignment_2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38499:1: rule__BaseDataTypeWidth__WidthAssignment_2 : ( RULE_INT ) ;
public final void rule__BaseDataTypeWidth__WidthAssignment_2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38503:1: ( ( RULE_INT ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38504:1: ( RULE_INT )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38504:1: ( RULE_INT )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38505:1: RULE_INT
{
if ( state.backtracking==0 ) {
before(grammarAccess.getBaseDataTypeWidthAccess().getWidthINTTerminalRuleCall_2_0());
}
match(input,RULE_INT,FollowSets003.FOLLOW_RULE_INT_in_rule__BaseDataTypeWidth__WidthAssignment_277262); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getBaseDataTypeWidthAccess().getWidthINTTerminalRuleCall_2_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__BaseDataTypeWidth__WidthAssignment_2"
// $ANTLR start "rule__BaseDataTypeLabel__LabelAssignment_2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38514:1: rule__BaseDataTypeLabel__LabelAssignment_2 : ( RULE_STRING ) ;
public final void rule__BaseDataTypeLabel__LabelAssignment_2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38518:1: ( ( RULE_STRING ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38519:1: ( RULE_STRING )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38519:1: ( RULE_STRING )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38520:1: RULE_STRING
{
if ( state.backtracking==0 ) {
before(grammarAccess.getBaseDataTypeLabelAccess().getLabelSTRINGTerminalRuleCall_2_0());
}
match(input,RULE_STRING,FollowSets003.FOLLOW_RULE_STRING_in_rule__BaseDataTypeLabel__LabelAssignment_277293); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getBaseDataTypeLabelAccess().getLabelSTRINGTerminalRuleCall_2_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__BaseDataTypeLabel__LabelAssignment_2"
// $ANTLR start "rule__StringDataType__NameAssignment_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38529:1: rule__StringDataType__NameAssignment_1 : ( RULE_ID ) ;
public final void rule__StringDataType__NameAssignment_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38533:1: ( ( RULE_ID ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38534:1: ( RULE_ID )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38534:1: ( RULE_ID )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38535:1: RULE_ID
{
if ( state.backtracking==0 ) {
before(grammarAccess.getStringDataTypeAccess().getNameIDTerminalRuleCall_1_0());
}
match(input,RULE_ID,FollowSets003.FOLLOW_RULE_ID_in_rule__StringDataType__NameAssignment_177324); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getStringDataTypeAccess().getNameIDTerminalRuleCall_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__StringDataType__NameAssignment_1"
// $ANTLR start "rule__StringDataType__RefAssignment_2_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38544:1: rule__StringDataType__RefAssignment_2_1 : ( ( ruleQualifiedName ) ) ;
public final void rule__StringDataType__RefAssignment_2_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38548:1: ( ( ( ruleQualifiedName ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38549:1: ( ( ruleQualifiedName ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38549:1: ( ( ruleQualifiedName ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38550:1: ( ruleQualifiedName )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getStringDataTypeAccess().getRefStringDataTypeCrossReference_2_1_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38551:1: ( ruleQualifiedName )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38552:1: ruleQualifiedName
{
if ( state.backtracking==0 ) {
before(grammarAccess.getStringDataTypeAccess().getRefStringDataTypeQualifiedNameParserRuleCall_2_1_0_1());
}
pushFollow(FollowSets003.FOLLOW_ruleQualifiedName_in_rule__StringDataType__RefAssignment_2_177359);
ruleQualifiedName();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getStringDataTypeAccess().getRefStringDataTypeQualifiedNameParserRuleCall_2_1_0_1());
}
}
if ( state.backtracking==0 ) {
after(grammarAccess.getStringDataTypeAccess().getRefStringDataTypeCrossReference_2_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__StringDataType__RefAssignment_2_1"
// $ANTLR start "rule__StringDataType__BaseDataTypeAssignment_4"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38563:1: rule__StringDataType__BaseDataTypeAssignment_4 : ( ruleBaseDataType ) ;
public final void rule__StringDataType__BaseDataTypeAssignment_4() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38567:1: ( ( ruleBaseDataType ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38568:1: ( ruleBaseDataType )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38568:1: ( ruleBaseDataType )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38569:1: ruleBaseDataType
{
if ( state.backtracking==0 ) {
before(grammarAccess.getStringDataTypeAccess().getBaseDataTypeBaseDataTypeParserRuleCall_4_0());
}
pushFollow(FollowSets003.FOLLOW_ruleBaseDataType_in_rule__StringDataType__BaseDataTypeAssignment_477394);
ruleBaseDataType();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getStringDataTypeAccess().getBaseDataTypeBaseDataTypeParserRuleCall_4_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__StringDataType__BaseDataTypeAssignment_4"
// $ANTLR start "rule__StringDataType__MaxLengthAssignment_5_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38578:1: rule__StringDataType__MaxLengthAssignment_5_1 : ( RULE_INT ) ;
public final void rule__StringDataType__MaxLengthAssignment_5_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38582:1: ( ( RULE_INT ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38583:1: ( RULE_INT )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38583:1: ( RULE_INT )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38584:1: RULE_INT
{
if ( state.backtracking==0 ) {
before(grammarAccess.getStringDataTypeAccess().getMaxLengthINTTerminalRuleCall_5_1_0());
}
match(input,RULE_INT,FollowSets003.FOLLOW_RULE_INT_in_rule__StringDataType__MaxLengthAssignment_5_177425); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getStringDataTypeAccess().getMaxLengthINTTerminalRuleCall_5_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__StringDataType__MaxLengthAssignment_5_1"
// $ANTLR start "rule__StringDataType__MinLengthAssignment_6_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38593:1: rule__StringDataType__MinLengthAssignment_6_1 : ( RULE_INT ) ;
public final void rule__StringDataType__MinLengthAssignment_6_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38597:1: ( ( RULE_INT ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38598:1: ( RULE_INT )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38598:1: ( RULE_INT )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38599:1: RULE_INT
{
if ( state.backtracking==0 ) {
before(grammarAccess.getStringDataTypeAccess().getMinLengthINTTerminalRuleCall_6_1_0());
}
match(input,RULE_INT,FollowSets003.FOLLOW_RULE_INT_in_rule__StringDataType__MinLengthAssignment_6_177456); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getStringDataTypeAccess().getMinLengthINTTerminalRuleCall_6_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__StringDataType__MinLengthAssignment_6_1"
// $ANTLR start "rule__StringEntityAttribute__TypeAssignment_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38608:1: rule__StringEntityAttribute__TypeAssignment_1 : ( ( ruleQualifiedName ) ) ;
public final void rule__StringEntityAttribute__TypeAssignment_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38612:1: ( ( ( ruleQualifiedName ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38613:1: ( ( ruleQualifiedName ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38613:1: ( ( ruleQualifiedName ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38614:1: ( ruleQualifiedName )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getStringEntityAttributeAccess().getTypeStringDataTypeCrossReference_1_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38615:1: ( ruleQualifiedName )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38616:1: ruleQualifiedName
{
if ( state.backtracking==0 ) {
before(grammarAccess.getStringEntityAttributeAccess().getTypeStringDataTypeQualifiedNameParserRuleCall_1_0_1());
}
pushFollow(FollowSets003.FOLLOW_ruleQualifiedName_in_rule__StringEntityAttribute__TypeAssignment_177491);
ruleQualifiedName();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getStringEntityAttributeAccess().getTypeStringDataTypeQualifiedNameParserRuleCall_1_0_1());
}
}
if ( state.backtracking==0 ) {
after(grammarAccess.getStringEntityAttributeAccess().getTypeStringDataTypeCrossReference_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__StringEntityAttribute__TypeAssignment_1"
// $ANTLR start "rule__StringEntityAttribute__CardinalityAssignment_2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38627:1: rule__StringEntityAttribute__CardinalityAssignment_2 : ( ruleCardinality ) ;
public final void rule__StringEntityAttribute__CardinalityAssignment_2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38631:1: ( ( ruleCardinality ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38632:1: ( ruleCardinality )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38632:1: ( ruleCardinality )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38633:1: ruleCardinality
{
if ( state.backtracking==0 ) {
before(grammarAccess.getStringEntityAttributeAccess().getCardinalityCardinalityEnumRuleCall_2_0());
}
pushFollow(FollowSets003.FOLLOW_ruleCardinality_in_rule__StringEntityAttribute__CardinalityAssignment_277526);
ruleCardinality();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getStringEntityAttributeAccess().getCardinalityCardinalityEnumRuleCall_2_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__StringEntityAttribute__CardinalityAssignment_2"
// $ANTLR start "rule__StringEntityAttribute__NameAssignment_3"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38642:1: rule__StringEntityAttribute__NameAssignment_3 : ( RULE_ID ) ;
public final void rule__StringEntityAttribute__NameAssignment_3() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38646:1: ( ( RULE_ID ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38647:1: ( RULE_ID )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38647:1: ( RULE_ID )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38648:1: RULE_ID
{
if ( state.backtracking==0 ) {
before(grammarAccess.getStringEntityAttributeAccess().getNameIDTerminalRuleCall_3_0());
}
match(input,RULE_ID,FollowSets003.FOLLOW_RULE_ID_in_rule__StringEntityAttribute__NameAssignment_377557); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getStringEntityAttributeAccess().getNameIDTerminalRuleCall_3_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__StringEntityAttribute__NameAssignment_3"
// $ANTLR start "rule__MapEntityAttribute__TypeAssignment_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38662:1: rule__MapEntityAttribute__TypeAssignment_1 : ( ( ruleQualifiedName ) ) ;
public final void rule__MapEntityAttribute__TypeAssignment_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38666:1: ( ( ( ruleQualifiedName ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38667:1: ( ( ruleQualifiedName ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38667:1: ( ( ruleQualifiedName ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38668:1: ( ruleQualifiedName )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getMapEntityAttributeAccess().getTypeMapDataTypeCrossReference_1_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38669:1: ( ruleQualifiedName )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38670:1: ruleQualifiedName
{
if ( state.backtracking==0 ) {
before(grammarAccess.getMapEntityAttributeAccess().getTypeMapDataTypeQualifiedNameParserRuleCall_1_0_1());
}
pushFollow(FollowSets003.FOLLOW_ruleQualifiedName_in_rule__MapEntityAttribute__TypeAssignment_177597);
ruleQualifiedName();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getMapEntityAttributeAccess().getTypeMapDataTypeQualifiedNameParserRuleCall_1_0_1());
}
}
if ( state.backtracking==0 ) {
after(grammarAccess.getMapEntityAttributeAccess().getTypeMapDataTypeCrossReference_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__MapEntityAttribute__TypeAssignment_1"
// $ANTLR start "rule__MapEntityAttribute__KeyTypeAssignment_2_0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38681:1: rule__MapEntityAttribute__KeyTypeAssignment_2_0 : ( ruleSimpleTypes ) ;
public final void rule__MapEntityAttribute__KeyTypeAssignment_2_0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38685:1: ( ( ruleSimpleTypes ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38686:1: ( ruleSimpleTypes )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38686:1: ( ruleSimpleTypes )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38687:1: ruleSimpleTypes
{
if ( state.backtracking==0 ) {
before(grammarAccess.getMapEntityAttributeAccess().getKeyTypeSimpleTypesEnumRuleCall_2_0_0());
}
pushFollow(FollowSets003.FOLLOW_ruleSimpleTypes_in_rule__MapEntityAttribute__KeyTypeAssignment_2_077632);
ruleSimpleTypes();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getMapEntityAttributeAccess().getKeyTypeSimpleTypesEnumRuleCall_2_0_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__MapEntityAttribute__KeyTypeAssignment_2_0"
// $ANTLR start "rule__MapEntityAttribute__ValueTypeAssignment_2_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38696:1: rule__MapEntityAttribute__ValueTypeAssignment_2_1 : ( ruleSimpleTypes ) ;
public final void rule__MapEntityAttribute__ValueTypeAssignment_2_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38700:1: ( ( ruleSimpleTypes ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38701:1: ( ruleSimpleTypes )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38701:1: ( ruleSimpleTypes )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38702:1: ruleSimpleTypes
{
if ( state.backtracking==0 ) {
before(grammarAccess.getMapEntityAttributeAccess().getValueTypeSimpleTypesEnumRuleCall_2_1_0());
}
pushFollow(FollowSets003.FOLLOW_ruleSimpleTypes_in_rule__MapEntityAttribute__ValueTypeAssignment_2_177663);
ruleSimpleTypes();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getMapEntityAttributeAccess().getValueTypeSimpleTypesEnumRuleCall_2_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__MapEntityAttribute__ValueTypeAssignment_2_1"
// $ANTLR start "rule__MapEntityAttribute__NameAssignment_3"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38711:1: rule__MapEntityAttribute__NameAssignment_3 : ( RULE_ID ) ;
public final void rule__MapEntityAttribute__NameAssignment_3() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38715:1: ( ( RULE_ID ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38716:1: ( RULE_ID )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38716:1: ( RULE_ID )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38717:1: RULE_ID
{
if ( state.backtracking==0 ) {
before(grammarAccess.getMapEntityAttributeAccess().getNameIDTerminalRuleCall_3_0());
}
match(input,RULE_ID,FollowSets003.FOLLOW_RULE_ID_in_rule__MapEntityAttribute__NameAssignment_377694); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getMapEntityAttributeAccess().getNameIDTerminalRuleCall_3_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__MapEntityAttribute__NameAssignment_3"
// $ANTLR start "rule__BooleanDataType__NameAssignment_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38726:1: rule__BooleanDataType__NameAssignment_1 : ( RULE_ID ) ;
public final void rule__BooleanDataType__NameAssignment_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38730:1: ( ( RULE_ID ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38731:1: ( RULE_ID )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38731:1: ( RULE_ID )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38732:1: RULE_ID
{
if ( state.backtracking==0 ) {
before(grammarAccess.getBooleanDataTypeAccess().getNameIDTerminalRuleCall_1_0());
}
match(input,RULE_ID,FollowSets003.FOLLOW_RULE_ID_in_rule__BooleanDataType__NameAssignment_177725); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getBooleanDataTypeAccess().getNameIDTerminalRuleCall_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__BooleanDataType__NameAssignment_1"
// $ANTLR start "rule__BooleanDataType__RefAssignment_2_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38741:1: rule__BooleanDataType__RefAssignment_2_1 : ( ( ruleQualifiedName ) ) ;
public final void rule__BooleanDataType__RefAssignment_2_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38745:1: ( ( ( ruleQualifiedName ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38746:1: ( ( ruleQualifiedName ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38746:1: ( ( ruleQualifiedName ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38747:1: ( ruleQualifiedName )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getBooleanDataTypeAccess().getRefBooleanDataTypeCrossReference_2_1_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38748:1: ( ruleQualifiedName )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38749:1: ruleQualifiedName
{
if ( state.backtracking==0 ) {
before(grammarAccess.getBooleanDataTypeAccess().getRefBooleanDataTypeQualifiedNameParserRuleCall_2_1_0_1());
}
pushFollow(FollowSets003.FOLLOW_ruleQualifiedName_in_rule__BooleanDataType__RefAssignment_2_177760);
ruleQualifiedName();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getBooleanDataTypeAccess().getRefBooleanDataTypeQualifiedNameParserRuleCall_2_1_0_1());
}
}
if ( state.backtracking==0 ) {
after(grammarAccess.getBooleanDataTypeAccess().getRefBooleanDataTypeCrossReference_2_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__BooleanDataType__RefAssignment_2_1"
// $ANTLR start "rule__BooleanDataType__BaseDataTypeAssignment_4"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38760:1: rule__BooleanDataType__BaseDataTypeAssignment_4 : ( ruleBaseDataType ) ;
public final void rule__BooleanDataType__BaseDataTypeAssignment_4() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38764:1: ( ( ruleBaseDataType ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38765:1: ( ruleBaseDataType )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38765:1: ( ruleBaseDataType )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38766:1: ruleBaseDataType
{
if ( state.backtracking==0 ) {
before(grammarAccess.getBooleanDataTypeAccess().getBaseDataTypeBaseDataTypeParserRuleCall_4_0());
}
pushFollow(FollowSets003.FOLLOW_ruleBaseDataType_in_rule__BooleanDataType__BaseDataTypeAssignment_477795);
ruleBaseDataType();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getBooleanDataTypeAccess().getBaseDataTypeBaseDataTypeParserRuleCall_4_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__BooleanDataType__BaseDataTypeAssignment_4"
// $ANTLR start "rule__BooleanEntityAttribute__TypeAssignment_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38775:1: rule__BooleanEntityAttribute__TypeAssignment_1 : ( ( ruleQualifiedName ) ) ;
public final void rule__BooleanEntityAttribute__TypeAssignment_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38779:1: ( ( ( ruleQualifiedName ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38780:1: ( ( ruleQualifiedName ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38780:1: ( ( ruleQualifiedName ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38781:1: ( ruleQualifiedName )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getBooleanEntityAttributeAccess().getTypeBooleanDataTypeCrossReference_1_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38782:1: ( ruleQualifiedName )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38783:1: ruleQualifiedName
{
if ( state.backtracking==0 ) {
before(grammarAccess.getBooleanEntityAttributeAccess().getTypeBooleanDataTypeQualifiedNameParserRuleCall_1_0_1());
}
pushFollow(FollowSets003.FOLLOW_ruleQualifiedName_in_rule__BooleanEntityAttribute__TypeAssignment_177830);
ruleQualifiedName();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getBooleanEntityAttributeAccess().getTypeBooleanDataTypeQualifiedNameParserRuleCall_1_0_1());
}
}
if ( state.backtracking==0 ) {
after(grammarAccess.getBooleanEntityAttributeAccess().getTypeBooleanDataTypeCrossReference_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__BooleanEntityAttribute__TypeAssignment_1"
// $ANTLR start "rule__BooleanEntityAttribute__NameAssignment_2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38794:1: rule__BooleanEntityAttribute__NameAssignment_2 : ( RULE_ID ) ;
public final void rule__BooleanEntityAttribute__NameAssignment_2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38798:1: ( ( RULE_ID ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38799:1: ( RULE_ID )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38799:1: ( RULE_ID )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38800:1: RULE_ID
{
if ( state.backtracking==0 ) {
before(grammarAccess.getBooleanEntityAttributeAccess().getNameIDTerminalRuleCall_2_0());
}
match(input,RULE_ID,FollowSets003.FOLLOW_RULE_ID_in_rule__BooleanEntityAttribute__NameAssignment_277865); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getBooleanEntityAttributeAccess().getNameIDTerminalRuleCall_2_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__BooleanEntityAttribute__NameAssignment_2"
// $ANTLR start "rule__IntegerDataType__NameAssignment_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38809:1: rule__IntegerDataType__NameAssignment_1 : ( RULE_ID ) ;
public final void rule__IntegerDataType__NameAssignment_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38813:1: ( ( RULE_ID ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38814:1: ( RULE_ID )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38814:1: ( RULE_ID )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38815:1: RULE_ID
{
if ( state.backtracking==0 ) {
before(grammarAccess.getIntegerDataTypeAccess().getNameIDTerminalRuleCall_1_0());
}
match(input,RULE_ID,FollowSets003.FOLLOW_RULE_ID_in_rule__IntegerDataType__NameAssignment_177896); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getIntegerDataTypeAccess().getNameIDTerminalRuleCall_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__IntegerDataType__NameAssignment_1"
// $ANTLR start "rule__IntegerDataType__RefAssignment_2_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38824:1: rule__IntegerDataType__RefAssignment_2_1 : ( ( ruleQualifiedName ) ) ;
public final void rule__IntegerDataType__RefAssignment_2_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38828:1: ( ( ( ruleQualifiedName ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38829:1: ( ( ruleQualifiedName ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38829:1: ( ( ruleQualifiedName ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38830:1: ( ruleQualifiedName )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getIntegerDataTypeAccess().getRefIntegerDataTypeCrossReference_2_1_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38831:1: ( ruleQualifiedName )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38832:1: ruleQualifiedName
{
if ( state.backtracking==0 ) {
before(grammarAccess.getIntegerDataTypeAccess().getRefIntegerDataTypeQualifiedNameParserRuleCall_2_1_0_1());
}
pushFollow(FollowSets003.FOLLOW_ruleQualifiedName_in_rule__IntegerDataType__RefAssignment_2_177931);
ruleQualifiedName();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getIntegerDataTypeAccess().getRefIntegerDataTypeQualifiedNameParserRuleCall_2_1_0_1());
}
}
if ( state.backtracking==0 ) {
after(grammarAccess.getIntegerDataTypeAccess().getRefIntegerDataTypeCrossReference_2_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__IntegerDataType__RefAssignment_2_1"
// $ANTLR start "rule__IntegerDataType__BaseDataTypeAssignment_4"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38843:1: rule__IntegerDataType__BaseDataTypeAssignment_4 : ( ruleBaseDataType ) ;
public final void rule__IntegerDataType__BaseDataTypeAssignment_4() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38847:1: ( ( ruleBaseDataType ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38848:1: ( ruleBaseDataType )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38848:1: ( ruleBaseDataType )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38849:1: ruleBaseDataType
{
if ( state.backtracking==0 ) {
before(grammarAccess.getIntegerDataTypeAccess().getBaseDataTypeBaseDataTypeParserRuleCall_4_0());
}
pushFollow(FollowSets003.FOLLOW_ruleBaseDataType_in_rule__IntegerDataType__BaseDataTypeAssignment_477966);
ruleBaseDataType();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getIntegerDataTypeAccess().getBaseDataTypeBaseDataTypeParserRuleCall_4_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__IntegerDataType__BaseDataTypeAssignment_4"
// $ANTLR start "rule__IntegerEntityAttribute__TypeAssignment_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38858:1: rule__IntegerEntityAttribute__TypeAssignment_1 : ( ( ruleQualifiedName ) ) ;
public final void rule__IntegerEntityAttribute__TypeAssignment_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38862:1: ( ( ( ruleQualifiedName ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38863:1: ( ( ruleQualifiedName ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38863:1: ( ( ruleQualifiedName ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38864:1: ( ruleQualifiedName )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getIntegerEntityAttributeAccess().getTypeIntegerDataTypeCrossReference_1_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38865:1: ( ruleQualifiedName )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38866:1: ruleQualifiedName
{
if ( state.backtracking==0 ) {
before(grammarAccess.getIntegerEntityAttributeAccess().getTypeIntegerDataTypeQualifiedNameParserRuleCall_1_0_1());
}
pushFollow(FollowSets003.FOLLOW_ruleQualifiedName_in_rule__IntegerEntityAttribute__TypeAssignment_178001);
ruleQualifiedName();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getIntegerEntityAttributeAccess().getTypeIntegerDataTypeQualifiedNameParserRuleCall_1_0_1());
}
}
if ( state.backtracking==0 ) {
after(grammarAccess.getIntegerEntityAttributeAccess().getTypeIntegerDataTypeCrossReference_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__IntegerEntityAttribute__TypeAssignment_1"
// $ANTLR start "rule__IntegerEntityAttribute__NameAssignment_2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38877:1: rule__IntegerEntityAttribute__NameAssignment_2 : ( RULE_ID ) ;
public final void rule__IntegerEntityAttribute__NameAssignment_2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38881:1: ( ( RULE_ID ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38882:1: ( RULE_ID )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38882:1: ( RULE_ID )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38883:1: RULE_ID
{
if ( state.backtracking==0 ) {
before(grammarAccess.getIntegerEntityAttributeAccess().getNameIDTerminalRuleCall_2_0());
}
match(input,RULE_ID,FollowSets003.FOLLOW_RULE_ID_in_rule__IntegerEntityAttribute__NameAssignment_278036); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getIntegerEntityAttributeAccess().getNameIDTerminalRuleCall_2_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__IntegerEntityAttribute__NameAssignment_2"
// $ANTLR start "rule__DateDataType__NameAssignment_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38892:1: rule__DateDataType__NameAssignment_1 : ( RULE_ID ) ;
public final void rule__DateDataType__NameAssignment_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38896:1: ( ( RULE_ID ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38897:1: ( RULE_ID )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38897:1: ( RULE_ID )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38898:1: RULE_ID
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDateDataTypeAccess().getNameIDTerminalRuleCall_1_0());
}
match(input,RULE_ID,FollowSets003.FOLLOW_RULE_ID_in_rule__DateDataType__NameAssignment_178067); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDateDataTypeAccess().getNameIDTerminalRuleCall_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DateDataType__NameAssignment_1"
// $ANTLR start "rule__DateDataType__RefAssignment_2_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38907:1: rule__DateDataType__RefAssignment_2_1 : ( ( ruleQualifiedName ) ) ;
public final void rule__DateDataType__RefAssignment_2_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38911:1: ( ( ( ruleQualifiedName ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38912:1: ( ( ruleQualifiedName ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38912:1: ( ( ruleQualifiedName ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38913:1: ( ruleQualifiedName )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDateDataTypeAccess().getRefDateDataTypeCrossReference_2_1_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38914:1: ( ruleQualifiedName )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38915:1: ruleQualifiedName
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDateDataTypeAccess().getRefDateDataTypeQualifiedNameParserRuleCall_2_1_0_1());
}
pushFollow(FollowSets003.FOLLOW_ruleQualifiedName_in_rule__DateDataType__RefAssignment_2_178102);
ruleQualifiedName();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDateDataTypeAccess().getRefDateDataTypeQualifiedNameParserRuleCall_2_1_0_1());
}
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDateDataTypeAccess().getRefDateDataTypeCrossReference_2_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DateDataType__RefAssignment_2_1"
// $ANTLR start "rule__DateDataType__BaseDataTypeAssignment_4"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38926:1: rule__DateDataType__BaseDataTypeAssignment_4 : ( ruleBaseDataType ) ;
public final void rule__DateDataType__BaseDataTypeAssignment_4() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38930:1: ( ( ruleBaseDataType ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38931:1: ( ruleBaseDataType )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38931:1: ( ruleBaseDataType )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38932:1: ruleBaseDataType
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDateDataTypeAccess().getBaseDataTypeBaseDataTypeParserRuleCall_4_0());
}
pushFollow(FollowSets003.FOLLOW_ruleBaseDataType_in_rule__DateDataType__BaseDataTypeAssignment_478137);
ruleBaseDataType();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDateDataTypeAccess().getBaseDataTypeBaseDataTypeParserRuleCall_4_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DateDataType__BaseDataTypeAssignment_4"
// $ANTLR start "rule__DateEntityAttribute__TypeAssignment_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38941:1: rule__DateEntityAttribute__TypeAssignment_1 : ( ( ruleQualifiedName ) ) ;
public final void rule__DateEntityAttribute__TypeAssignment_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38945:1: ( ( ( ruleQualifiedName ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38946:1: ( ( ruleQualifiedName ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38946:1: ( ( ruleQualifiedName ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38947:1: ( ruleQualifiedName )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDateEntityAttributeAccess().getTypeDateDataTypeCrossReference_1_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38948:1: ( ruleQualifiedName )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38949:1: ruleQualifiedName
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDateEntityAttributeAccess().getTypeDateDataTypeQualifiedNameParserRuleCall_1_0_1());
}
pushFollow(FollowSets003.FOLLOW_ruleQualifiedName_in_rule__DateEntityAttribute__TypeAssignment_178172);
ruleQualifiedName();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDateEntityAttributeAccess().getTypeDateDataTypeQualifiedNameParserRuleCall_1_0_1());
}
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDateEntityAttributeAccess().getTypeDateDataTypeCrossReference_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DateEntityAttribute__TypeAssignment_1"
// $ANTLR start "rule__DateEntityAttribute__NameAssignment_2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38960:1: rule__DateEntityAttribute__NameAssignment_2 : ( RULE_ID ) ;
public final void rule__DateEntityAttribute__NameAssignment_2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38964:1: ( ( RULE_ID ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38965:1: ( RULE_ID )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38965:1: ( RULE_ID )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38966:1: RULE_ID
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDateEntityAttributeAccess().getNameIDTerminalRuleCall_2_0());
}
match(input,RULE_ID,FollowSets003.FOLLOW_RULE_ID_in_rule__DateEntityAttribute__NameAssignment_278207); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDateEntityAttributeAccess().getNameIDTerminalRuleCall_2_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DateEntityAttribute__NameAssignment_2"
// $ANTLR start "rule__DecimalDataType__NameAssignment_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38975:1: rule__DecimalDataType__NameAssignment_1 : ( RULE_ID ) ;
public final void rule__DecimalDataType__NameAssignment_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38979:1: ( ( RULE_ID ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38980:1: ( RULE_ID )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38980:1: ( RULE_ID )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38981:1: RULE_ID
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDecimalDataTypeAccess().getNameIDTerminalRuleCall_1_0());
}
match(input,RULE_ID,FollowSets003.FOLLOW_RULE_ID_in_rule__DecimalDataType__NameAssignment_178238); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDecimalDataTypeAccess().getNameIDTerminalRuleCall_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DecimalDataType__NameAssignment_1"
// $ANTLR start "rule__DecimalDataType__RefAssignment_2_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38990:1: rule__DecimalDataType__RefAssignment_2_1 : ( ( ruleQualifiedName ) ) ;
public final void rule__DecimalDataType__RefAssignment_2_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38994:1: ( ( ( ruleQualifiedName ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38995:1: ( ( ruleQualifiedName ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38995:1: ( ( ruleQualifiedName ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38996:1: ( ruleQualifiedName )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDecimalDataTypeAccess().getRefDecimalDataTypeCrossReference_2_1_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38997:1: ( ruleQualifiedName )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:38998:1: ruleQualifiedName
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDecimalDataTypeAccess().getRefDecimalDataTypeQualifiedNameParserRuleCall_2_1_0_1());
}
pushFollow(FollowSets003.FOLLOW_ruleQualifiedName_in_rule__DecimalDataType__RefAssignment_2_178273);
ruleQualifiedName();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDecimalDataTypeAccess().getRefDecimalDataTypeQualifiedNameParserRuleCall_2_1_0_1());
}
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDecimalDataTypeAccess().getRefDecimalDataTypeCrossReference_2_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DecimalDataType__RefAssignment_2_1"
// $ANTLR start "rule__DecimalDataType__BaseDataTypeAssignment_4"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39009:1: rule__DecimalDataType__BaseDataTypeAssignment_4 : ( ruleBaseDataType ) ;
public final void rule__DecimalDataType__BaseDataTypeAssignment_4() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39013:1: ( ( ruleBaseDataType ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39014:1: ( ruleBaseDataType )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39014:1: ( ruleBaseDataType )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39015:1: ruleBaseDataType
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDecimalDataTypeAccess().getBaseDataTypeBaseDataTypeParserRuleCall_4_0());
}
pushFollow(FollowSets003.FOLLOW_ruleBaseDataType_in_rule__DecimalDataType__BaseDataTypeAssignment_478308);
ruleBaseDataType();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDecimalDataTypeAccess().getBaseDataTypeBaseDataTypeParserRuleCall_4_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DecimalDataType__BaseDataTypeAssignment_4"
// $ANTLR start "rule__DecimalEntityAttribute__TypeAssignment_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39024:1: rule__DecimalEntityAttribute__TypeAssignment_1 : ( ( ruleQualifiedName ) ) ;
public final void rule__DecimalEntityAttribute__TypeAssignment_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39028:1: ( ( ( ruleQualifiedName ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39029:1: ( ( ruleQualifiedName ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39029:1: ( ( ruleQualifiedName ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39030:1: ( ruleQualifiedName )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDecimalEntityAttributeAccess().getTypeDecimalDataTypeCrossReference_1_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39031:1: ( ruleQualifiedName )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39032:1: ruleQualifiedName
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDecimalEntityAttributeAccess().getTypeDecimalDataTypeQualifiedNameParserRuleCall_1_0_1());
}
pushFollow(FollowSets003.FOLLOW_ruleQualifiedName_in_rule__DecimalEntityAttribute__TypeAssignment_178343);
ruleQualifiedName();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDecimalEntityAttributeAccess().getTypeDecimalDataTypeQualifiedNameParserRuleCall_1_0_1());
}
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDecimalEntityAttributeAccess().getTypeDecimalDataTypeCrossReference_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DecimalEntityAttribute__TypeAssignment_1"
// $ANTLR start "rule__DecimalEntityAttribute__NameAssignment_2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39043:1: rule__DecimalEntityAttribute__NameAssignment_2 : ( RULE_ID ) ;
public final void rule__DecimalEntityAttribute__NameAssignment_2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39047:1: ( ( RULE_ID ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39048:1: ( RULE_ID )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39048:1: ( RULE_ID )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39049:1: RULE_ID
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDecimalEntityAttributeAccess().getNameIDTerminalRuleCall_2_0());
}
match(input,RULE_ID,FollowSets003.FOLLOW_RULE_ID_in_rule__DecimalEntityAttribute__NameAssignment_278378); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDecimalEntityAttributeAccess().getNameIDTerminalRuleCall_2_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DecimalEntityAttribute__NameAssignment_2"
// $ANTLR start "rule__LongDataType__NameAssignment_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39058:1: rule__LongDataType__NameAssignment_1 : ( RULE_ID ) ;
public final void rule__LongDataType__NameAssignment_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39062:1: ( ( RULE_ID ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39063:1: ( RULE_ID )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39063:1: ( RULE_ID )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39064:1: RULE_ID
{
if ( state.backtracking==0 ) {
before(grammarAccess.getLongDataTypeAccess().getNameIDTerminalRuleCall_1_0());
}
match(input,RULE_ID,FollowSets003.FOLLOW_RULE_ID_in_rule__LongDataType__NameAssignment_178409); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getLongDataTypeAccess().getNameIDTerminalRuleCall_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__LongDataType__NameAssignment_1"
// $ANTLR start "rule__LongDataType__RefAssignment_2_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39073:1: rule__LongDataType__RefAssignment_2_1 : ( ( ruleQualifiedName ) ) ;
public final void rule__LongDataType__RefAssignment_2_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39077:1: ( ( ( ruleQualifiedName ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39078:1: ( ( ruleQualifiedName ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39078:1: ( ( ruleQualifiedName ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39079:1: ( ruleQualifiedName )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getLongDataTypeAccess().getRefLongDataTypeCrossReference_2_1_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39080:1: ( ruleQualifiedName )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39081:1: ruleQualifiedName
{
if ( state.backtracking==0 ) {
before(grammarAccess.getLongDataTypeAccess().getRefLongDataTypeQualifiedNameParserRuleCall_2_1_0_1());
}
pushFollow(FollowSets003.FOLLOW_ruleQualifiedName_in_rule__LongDataType__RefAssignment_2_178444);
ruleQualifiedName();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getLongDataTypeAccess().getRefLongDataTypeQualifiedNameParserRuleCall_2_1_0_1());
}
}
if ( state.backtracking==0 ) {
after(grammarAccess.getLongDataTypeAccess().getRefLongDataTypeCrossReference_2_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__LongDataType__RefAssignment_2_1"
// $ANTLR start "rule__LongDataType__BaseDataTypeAssignment_4"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39092:1: rule__LongDataType__BaseDataTypeAssignment_4 : ( ruleBaseDataType ) ;
public final void rule__LongDataType__BaseDataTypeAssignment_4() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39096:1: ( ( ruleBaseDataType ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39097:1: ( ruleBaseDataType )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39097:1: ( ruleBaseDataType )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39098:1: ruleBaseDataType
{
if ( state.backtracking==0 ) {
before(grammarAccess.getLongDataTypeAccess().getBaseDataTypeBaseDataTypeParserRuleCall_4_0());
}
pushFollow(FollowSets003.FOLLOW_ruleBaseDataType_in_rule__LongDataType__BaseDataTypeAssignment_478479);
ruleBaseDataType();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getLongDataTypeAccess().getBaseDataTypeBaseDataTypeParserRuleCall_4_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__LongDataType__BaseDataTypeAssignment_4"
// $ANTLR start "rule__LongEntityAttribute__TypeAssignment_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39107:1: rule__LongEntityAttribute__TypeAssignment_1 : ( ( ruleQualifiedName ) ) ;
public final void rule__LongEntityAttribute__TypeAssignment_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39111:1: ( ( ( ruleQualifiedName ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39112:1: ( ( ruleQualifiedName ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39112:1: ( ( ruleQualifiedName ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39113:1: ( ruleQualifiedName )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getLongEntityAttributeAccess().getTypeLongDataTypeCrossReference_1_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39114:1: ( ruleQualifiedName )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39115:1: ruleQualifiedName
{
if ( state.backtracking==0 ) {
before(grammarAccess.getLongEntityAttributeAccess().getTypeLongDataTypeQualifiedNameParserRuleCall_1_0_1());
}
pushFollow(FollowSets003.FOLLOW_ruleQualifiedName_in_rule__LongEntityAttribute__TypeAssignment_178514);
ruleQualifiedName();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getLongEntityAttributeAccess().getTypeLongDataTypeQualifiedNameParserRuleCall_1_0_1());
}
}
if ( state.backtracking==0 ) {
after(grammarAccess.getLongEntityAttributeAccess().getTypeLongDataTypeCrossReference_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__LongEntityAttribute__TypeAssignment_1"
// $ANTLR start "rule__LongEntityAttribute__NameAssignment_2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39126:1: rule__LongEntityAttribute__NameAssignment_2 : ( RULE_ID ) ;
public final void rule__LongEntityAttribute__NameAssignment_2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39130:1: ( ( RULE_ID ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39131:1: ( RULE_ID )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39131:1: ( RULE_ID )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39132:1: RULE_ID
{
if ( state.backtracking==0 ) {
before(grammarAccess.getLongEntityAttributeAccess().getNameIDTerminalRuleCall_2_0());
}
match(input,RULE_ID,FollowSets003.FOLLOW_RULE_ID_in_rule__LongEntityAttribute__NameAssignment_278549); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getLongEntityAttributeAccess().getNameIDTerminalRuleCall_2_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__LongEntityAttribute__NameAssignment_2"
// $ANTLR start "rule__FloatDataType__NameAssignment_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39141:1: rule__FloatDataType__NameAssignment_1 : ( RULE_ID ) ;
public final void rule__FloatDataType__NameAssignment_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39145:1: ( ( RULE_ID ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39146:1: ( RULE_ID )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39146:1: ( RULE_ID )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39147:1: RULE_ID
{
if ( state.backtracking==0 ) {
before(grammarAccess.getFloatDataTypeAccess().getNameIDTerminalRuleCall_1_0());
}
match(input,RULE_ID,FollowSets003.FOLLOW_RULE_ID_in_rule__FloatDataType__NameAssignment_178580); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getFloatDataTypeAccess().getNameIDTerminalRuleCall_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__FloatDataType__NameAssignment_1"
// $ANTLR start "rule__FloatDataType__RefAssignment_2_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39156:1: rule__FloatDataType__RefAssignment_2_1 : ( ( ruleQualifiedName ) ) ;
public final void rule__FloatDataType__RefAssignment_2_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39160:1: ( ( ( ruleQualifiedName ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39161:1: ( ( ruleQualifiedName ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39161:1: ( ( ruleQualifiedName ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39162:1: ( ruleQualifiedName )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getFloatDataTypeAccess().getRefFloatDataTypeCrossReference_2_1_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39163:1: ( ruleQualifiedName )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39164:1: ruleQualifiedName
{
if ( state.backtracking==0 ) {
before(grammarAccess.getFloatDataTypeAccess().getRefFloatDataTypeQualifiedNameParserRuleCall_2_1_0_1());
}
pushFollow(FollowSets003.FOLLOW_ruleQualifiedName_in_rule__FloatDataType__RefAssignment_2_178615);
ruleQualifiedName();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getFloatDataTypeAccess().getRefFloatDataTypeQualifiedNameParserRuleCall_2_1_0_1());
}
}
if ( state.backtracking==0 ) {
after(grammarAccess.getFloatDataTypeAccess().getRefFloatDataTypeCrossReference_2_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__FloatDataType__RefAssignment_2_1"
// $ANTLR start "rule__FloatDataType__BaseDataTypeAssignment_4"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39175:1: rule__FloatDataType__BaseDataTypeAssignment_4 : ( ruleBaseDataType ) ;
public final void rule__FloatDataType__BaseDataTypeAssignment_4() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39179:1: ( ( ruleBaseDataType ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39180:1: ( ruleBaseDataType )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39180:1: ( ruleBaseDataType )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39181:1: ruleBaseDataType
{
if ( state.backtracking==0 ) {
before(grammarAccess.getFloatDataTypeAccess().getBaseDataTypeBaseDataTypeParserRuleCall_4_0());
}
pushFollow(FollowSets003.FOLLOW_ruleBaseDataType_in_rule__FloatDataType__BaseDataTypeAssignment_478650);
ruleBaseDataType();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getFloatDataTypeAccess().getBaseDataTypeBaseDataTypeParserRuleCall_4_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__FloatDataType__BaseDataTypeAssignment_4"
// $ANTLR start "rule__FloatEntityAttribute__TypeAssignment_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39190:1: rule__FloatEntityAttribute__TypeAssignment_1 : ( ( ruleQualifiedName ) ) ;
public final void rule__FloatEntityAttribute__TypeAssignment_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39194:1: ( ( ( ruleQualifiedName ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39195:1: ( ( ruleQualifiedName ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39195:1: ( ( ruleQualifiedName ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39196:1: ( ruleQualifiedName )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getFloatEntityAttributeAccess().getTypeFloatDataTypeCrossReference_1_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39197:1: ( ruleQualifiedName )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39198:1: ruleQualifiedName
{
if ( state.backtracking==0 ) {
before(grammarAccess.getFloatEntityAttributeAccess().getTypeFloatDataTypeQualifiedNameParserRuleCall_1_0_1());
}
pushFollow(FollowSets003.FOLLOW_ruleQualifiedName_in_rule__FloatEntityAttribute__TypeAssignment_178685);
ruleQualifiedName();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getFloatEntityAttributeAccess().getTypeFloatDataTypeQualifiedNameParserRuleCall_1_0_1());
}
}
if ( state.backtracking==0 ) {
after(grammarAccess.getFloatEntityAttributeAccess().getTypeFloatDataTypeCrossReference_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__FloatEntityAttribute__TypeAssignment_1"
// $ANTLR start "rule__FloatEntityAttribute__NameAssignment_2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39209:1: rule__FloatEntityAttribute__NameAssignment_2 : ( RULE_ID ) ;
public final void rule__FloatEntityAttribute__NameAssignment_2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39213:1: ( ( RULE_ID ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39214:1: ( RULE_ID )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39214:1: ( RULE_ID )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39215:1: RULE_ID
{
if ( state.backtracking==0 ) {
before(grammarAccess.getFloatEntityAttributeAccess().getNameIDTerminalRuleCall_2_0());
}
match(input,RULE_ID,FollowSets003.FOLLOW_RULE_ID_in_rule__FloatEntityAttribute__NameAssignment_278720); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getFloatEntityAttributeAccess().getNameIDTerminalRuleCall_2_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__FloatEntityAttribute__NameAssignment_2"
// $ANTLR start "rule__DoubleDataType__NameAssignment_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39224:1: rule__DoubleDataType__NameAssignment_1 : ( RULE_ID ) ;
public final void rule__DoubleDataType__NameAssignment_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39228:1: ( ( RULE_ID ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39229:1: ( RULE_ID )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39229:1: ( RULE_ID )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39230:1: RULE_ID
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDoubleDataTypeAccess().getNameIDTerminalRuleCall_1_0());
}
match(input,RULE_ID,FollowSets003.FOLLOW_RULE_ID_in_rule__DoubleDataType__NameAssignment_178751); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDoubleDataTypeAccess().getNameIDTerminalRuleCall_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DoubleDataType__NameAssignment_1"
// $ANTLR start "rule__DoubleDataType__RefAssignment_2_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39239:1: rule__DoubleDataType__RefAssignment_2_1 : ( ( ruleQualifiedName ) ) ;
public final void rule__DoubleDataType__RefAssignment_2_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39243:1: ( ( ( ruleQualifiedName ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39244:1: ( ( ruleQualifiedName ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39244:1: ( ( ruleQualifiedName ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39245:1: ( ruleQualifiedName )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDoubleDataTypeAccess().getRefDoubleDataTypeCrossReference_2_1_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39246:1: ( ruleQualifiedName )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39247:1: ruleQualifiedName
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDoubleDataTypeAccess().getRefDoubleDataTypeQualifiedNameParserRuleCall_2_1_0_1());
}
pushFollow(FollowSets003.FOLLOW_ruleQualifiedName_in_rule__DoubleDataType__RefAssignment_2_178786);
ruleQualifiedName();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDoubleDataTypeAccess().getRefDoubleDataTypeQualifiedNameParserRuleCall_2_1_0_1());
}
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDoubleDataTypeAccess().getRefDoubleDataTypeCrossReference_2_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DoubleDataType__RefAssignment_2_1"
// $ANTLR start "rule__DoubleDataType__BaseDataTypeAssignment_4"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39258:1: rule__DoubleDataType__BaseDataTypeAssignment_4 : ( ruleBaseDataType ) ;
public final void rule__DoubleDataType__BaseDataTypeAssignment_4() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39262:1: ( ( ruleBaseDataType ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39263:1: ( ruleBaseDataType )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39263:1: ( ruleBaseDataType )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39264:1: ruleBaseDataType
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDoubleDataTypeAccess().getBaseDataTypeBaseDataTypeParserRuleCall_4_0());
}
pushFollow(FollowSets003.FOLLOW_ruleBaseDataType_in_rule__DoubleDataType__BaseDataTypeAssignment_478821);
ruleBaseDataType();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDoubleDataTypeAccess().getBaseDataTypeBaseDataTypeParserRuleCall_4_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DoubleDataType__BaseDataTypeAssignment_4"
// $ANTLR start "rule__DoubleEntityAttribute__TypeAssignment_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39273:1: rule__DoubleEntityAttribute__TypeAssignment_1 : ( ( ruleQualifiedName ) ) ;
public final void rule__DoubleEntityAttribute__TypeAssignment_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39277:1: ( ( ( ruleQualifiedName ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39278:1: ( ( ruleQualifiedName ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39278:1: ( ( ruleQualifiedName ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39279:1: ( ruleQualifiedName )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDoubleEntityAttributeAccess().getTypeDoubleDataTypeCrossReference_1_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39280:1: ( ruleQualifiedName )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39281:1: ruleQualifiedName
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDoubleEntityAttributeAccess().getTypeDoubleDataTypeQualifiedNameParserRuleCall_1_0_1());
}
pushFollow(FollowSets003.FOLLOW_ruleQualifiedName_in_rule__DoubleEntityAttribute__TypeAssignment_178856);
ruleQualifiedName();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDoubleEntityAttributeAccess().getTypeDoubleDataTypeQualifiedNameParserRuleCall_1_0_1());
}
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDoubleEntityAttributeAccess().getTypeDoubleDataTypeCrossReference_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DoubleEntityAttribute__TypeAssignment_1"
// $ANTLR start "rule__DoubleEntityAttribute__NameAssignment_2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39292:1: rule__DoubleEntityAttribute__NameAssignment_2 : ( RULE_ID ) ;
public final void rule__DoubleEntityAttribute__NameAssignment_2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39296:1: ( ( RULE_ID ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39297:1: ( RULE_ID )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39297:1: ( RULE_ID )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39298:1: RULE_ID
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDoubleEntityAttributeAccess().getNameIDTerminalRuleCall_2_0());
}
match(input,RULE_ID,FollowSets003.FOLLOW_RULE_ID_in_rule__DoubleEntityAttribute__NameAssignment_278891); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDoubleEntityAttributeAccess().getNameIDTerminalRuleCall_2_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DoubleEntityAttribute__NameAssignment_2"
// $ANTLR start "rule__BinaryDataType__NameAssignment_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39307:1: rule__BinaryDataType__NameAssignment_1 : ( RULE_ID ) ;
public final void rule__BinaryDataType__NameAssignment_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39311:1: ( ( RULE_ID ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39312:1: ( RULE_ID )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39312:1: ( RULE_ID )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39313:1: RULE_ID
{
if ( state.backtracking==0 ) {
before(grammarAccess.getBinaryDataTypeAccess().getNameIDTerminalRuleCall_1_0());
}
match(input,RULE_ID,FollowSets003.FOLLOW_RULE_ID_in_rule__BinaryDataType__NameAssignment_178922); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getBinaryDataTypeAccess().getNameIDTerminalRuleCall_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__BinaryDataType__NameAssignment_1"
// $ANTLR start "rule__BinaryDataType__RefAssignment_2_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39322:1: rule__BinaryDataType__RefAssignment_2_1 : ( ( ruleQualifiedName ) ) ;
public final void rule__BinaryDataType__RefAssignment_2_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39326:1: ( ( ( ruleQualifiedName ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39327:1: ( ( ruleQualifiedName ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39327:1: ( ( ruleQualifiedName ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39328:1: ( ruleQualifiedName )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getBinaryDataTypeAccess().getRefBinaryDataTypeCrossReference_2_1_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39329:1: ( ruleQualifiedName )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39330:1: ruleQualifiedName
{
if ( state.backtracking==0 ) {
before(grammarAccess.getBinaryDataTypeAccess().getRefBinaryDataTypeQualifiedNameParserRuleCall_2_1_0_1());
}
pushFollow(FollowSets003.FOLLOW_ruleQualifiedName_in_rule__BinaryDataType__RefAssignment_2_178957);
ruleQualifiedName();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getBinaryDataTypeAccess().getRefBinaryDataTypeQualifiedNameParserRuleCall_2_1_0_1());
}
}
if ( state.backtracking==0 ) {
after(grammarAccess.getBinaryDataTypeAccess().getRefBinaryDataTypeCrossReference_2_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__BinaryDataType__RefAssignment_2_1"
// $ANTLR start "rule__BinaryDataType__BaseDataTypeAssignment_4"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39341:1: rule__BinaryDataType__BaseDataTypeAssignment_4 : ( ruleBaseDataType ) ;
public final void rule__BinaryDataType__BaseDataTypeAssignment_4() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39345:1: ( ( ruleBaseDataType ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39346:1: ( ruleBaseDataType )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39346:1: ( ruleBaseDataType )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39347:1: ruleBaseDataType
{
if ( state.backtracking==0 ) {
before(grammarAccess.getBinaryDataTypeAccess().getBaseDataTypeBaseDataTypeParserRuleCall_4_0());
}
pushFollow(FollowSets003.FOLLOW_ruleBaseDataType_in_rule__BinaryDataType__BaseDataTypeAssignment_478992);
ruleBaseDataType();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getBinaryDataTypeAccess().getBaseDataTypeBaseDataTypeParserRuleCall_4_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__BinaryDataType__BaseDataTypeAssignment_4"
// $ANTLR start "rule__BinaryEntityAttribute__TypeAssignment_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39356:1: rule__BinaryEntityAttribute__TypeAssignment_1 : ( ( ruleQualifiedName ) ) ;
public final void rule__BinaryEntityAttribute__TypeAssignment_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39360:1: ( ( ( ruleQualifiedName ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39361:1: ( ( ruleQualifiedName ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39361:1: ( ( ruleQualifiedName ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39362:1: ( ruleQualifiedName )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getBinaryEntityAttributeAccess().getTypeBinaryDataTypeCrossReference_1_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39363:1: ( ruleQualifiedName )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39364:1: ruleQualifiedName
{
if ( state.backtracking==0 ) {
before(grammarAccess.getBinaryEntityAttributeAccess().getTypeBinaryDataTypeQualifiedNameParserRuleCall_1_0_1());
}
pushFollow(FollowSets003.FOLLOW_ruleQualifiedName_in_rule__BinaryEntityAttribute__TypeAssignment_179027);
ruleQualifiedName();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getBinaryEntityAttributeAccess().getTypeBinaryDataTypeQualifiedNameParserRuleCall_1_0_1());
}
}
if ( state.backtracking==0 ) {
after(grammarAccess.getBinaryEntityAttributeAccess().getTypeBinaryDataTypeCrossReference_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__BinaryEntityAttribute__TypeAssignment_1"
// $ANTLR start "rule__BinaryEntityAttribute__NameAssignment_2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39375:1: rule__BinaryEntityAttribute__NameAssignment_2 : ( RULE_ID ) ;
public final void rule__BinaryEntityAttribute__NameAssignment_2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39379:1: ( ( RULE_ID ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39380:1: ( RULE_ID )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39380:1: ( RULE_ID )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39381:1: RULE_ID
{
if ( state.backtracking==0 ) {
before(grammarAccess.getBinaryEntityAttributeAccess().getNameIDTerminalRuleCall_2_0());
}
match(input,RULE_ID,FollowSets003.FOLLOW_RULE_ID_in_rule__BinaryEntityAttribute__NameAssignment_279062); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getBinaryEntityAttributeAccess().getNameIDTerminalRuleCall_2_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__BinaryEntityAttribute__NameAssignment_2"
// $ANTLR start "rule__EntityDataType__NameAssignment_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39390:1: rule__EntityDataType__NameAssignment_1 : ( RULE_ID ) ;
public final void rule__EntityDataType__NameAssignment_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39394:1: ( ( RULE_ID ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39395:1: ( RULE_ID )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39395:1: ( RULE_ID )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39396:1: RULE_ID
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEntityDataTypeAccess().getNameIDTerminalRuleCall_1_0());
}
match(input,RULE_ID,FollowSets003.FOLLOW_RULE_ID_in_rule__EntityDataType__NameAssignment_179093); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getEntityDataTypeAccess().getNameIDTerminalRuleCall_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EntityDataType__NameAssignment_1"
// $ANTLR start "rule__EntityDataType__RefAssignment_2_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39405:1: rule__EntityDataType__RefAssignment_2_1 : ( ( ruleQualifiedName ) ) ;
public final void rule__EntityDataType__RefAssignment_2_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39409:1: ( ( ( ruleQualifiedName ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39410:1: ( ( ruleQualifiedName ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39410:1: ( ( ruleQualifiedName ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39411:1: ( ruleQualifiedName )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEntityDataTypeAccess().getRefEntityDataTypeCrossReference_2_1_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39412:1: ( ruleQualifiedName )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39413:1: ruleQualifiedName
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEntityDataTypeAccess().getRefEntityDataTypeQualifiedNameParserRuleCall_2_1_0_1());
}
pushFollow(FollowSets003.FOLLOW_ruleQualifiedName_in_rule__EntityDataType__RefAssignment_2_179128);
ruleQualifiedName();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getEntityDataTypeAccess().getRefEntityDataTypeQualifiedNameParserRuleCall_2_1_0_1());
}
}
if ( state.backtracking==0 ) {
after(grammarAccess.getEntityDataTypeAccess().getRefEntityDataTypeCrossReference_2_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EntityDataType__RefAssignment_2_1"
// $ANTLR start "rule__EntityDataType__BaseDataTypeAssignment_4"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39424:1: rule__EntityDataType__BaseDataTypeAssignment_4 : ( ruleBaseDataType ) ;
public final void rule__EntityDataType__BaseDataTypeAssignment_4() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39428:1: ( ( ruleBaseDataType ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39429:1: ( ruleBaseDataType )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39429:1: ( ruleBaseDataType )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39430:1: ruleBaseDataType
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEntityDataTypeAccess().getBaseDataTypeBaseDataTypeParserRuleCall_4_0());
}
pushFollow(FollowSets003.FOLLOW_ruleBaseDataType_in_rule__EntityDataType__BaseDataTypeAssignment_479163);
ruleBaseDataType();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getEntityDataTypeAccess().getBaseDataTypeBaseDataTypeParserRuleCall_4_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EntityDataType__BaseDataTypeAssignment_4"
// $ANTLR start "rule__EntityDataType__EntityAssignment_6"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39439:1: rule__EntityDataType__EntityAssignment_6 : ( ( ruleQualifiedName ) ) ;
public final void rule__EntityDataType__EntityAssignment_6() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39443:1: ( ( ( ruleQualifiedName ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39444:1: ( ( ruleQualifiedName ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39444:1: ( ( ruleQualifiedName ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39445:1: ( ruleQualifiedName )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEntityDataTypeAccess().getEntityEntityCrossReference_6_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39446:1: ( ruleQualifiedName )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39447:1: ruleQualifiedName
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEntityDataTypeAccess().getEntityEntityQualifiedNameParserRuleCall_6_0_1());
}
pushFollow(FollowSets003.FOLLOW_ruleQualifiedName_in_rule__EntityDataType__EntityAssignment_679198);
ruleQualifiedName();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getEntityDataTypeAccess().getEntityEntityQualifiedNameParserRuleCall_6_0_1());
}
}
if ( state.backtracking==0 ) {
after(grammarAccess.getEntityDataTypeAccess().getEntityEntityCrossReference_6_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EntityDataType__EntityAssignment_6"
// $ANTLR start "rule__EntityEntityAttribute__TypeAssignment_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39458:1: rule__EntityEntityAttribute__TypeAssignment_1 : ( ( ruleQualifiedName ) ) ;
public final void rule__EntityEntityAttribute__TypeAssignment_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39462:1: ( ( ( ruleQualifiedName ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39463:1: ( ( ruleQualifiedName ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39463:1: ( ( ruleQualifiedName ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39464:1: ( ruleQualifiedName )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEntityEntityAttributeAccess().getTypeEntityAttributeTypeCrossReference_1_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39465:1: ( ruleQualifiedName )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39466:1: ruleQualifiedName
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEntityEntityAttributeAccess().getTypeEntityAttributeTypeQualifiedNameParserRuleCall_1_0_1());
}
pushFollow(FollowSets003.FOLLOW_ruleQualifiedName_in_rule__EntityEntityAttribute__TypeAssignment_179237);
ruleQualifiedName();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getEntityEntityAttributeAccess().getTypeEntityAttributeTypeQualifiedNameParserRuleCall_1_0_1());
}
}
if ( state.backtracking==0 ) {
after(grammarAccess.getEntityEntityAttributeAccess().getTypeEntityAttributeTypeCrossReference_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EntityEntityAttribute__TypeAssignment_1"
// $ANTLR start "rule__EntityEntityAttribute__CardinalityAssignment_2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39477:1: rule__EntityEntityAttribute__CardinalityAssignment_2 : ( ruleCardinality ) ;
public final void rule__EntityEntityAttribute__CardinalityAssignment_2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39481:1: ( ( ruleCardinality ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39482:1: ( ruleCardinality )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39482:1: ( ruleCardinality )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39483:1: ruleCardinality
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEntityEntityAttributeAccess().getCardinalityCardinalityEnumRuleCall_2_0());
}
pushFollow(FollowSets003.FOLLOW_ruleCardinality_in_rule__EntityEntityAttribute__CardinalityAssignment_279272);
ruleCardinality();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getEntityEntityAttributeAccess().getCardinalityCardinalityEnumRuleCall_2_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EntityEntityAttribute__CardinalityAssignment_2"
// $ANTLR start "rule__EntityEntityAttribute__NameAssignment_3"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39492:1: rule__EntityEntityAttribute__NameAssignment_3 : ( RULE_ID ) ;
public final void rule__EntityEntityAttribute__NameAssignment_3() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39496:1: ( ( RULE_ID ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39497:1: ( RULE_ID )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39497:1: ( RULE_ID )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39498:1: RULE_ID
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEntityEntityAttributeAccess().getNameIDTerminalRuleCall_3_0());
}
match(input,RULE_ID,FollowSets003.FOLLOW_RULE_ID_in_rule__EntityEntityAttribute__NameAssignment_379303); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getEntityEntityAttributeAccess().getNameIDTerminalRuleCall_3_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EntityEntityAttribute__NameAssignment_3"
// $ANTLR start "rule__ValueObjectEntityAttribute__TypeAssignment_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39507:1: rule__ValueObjectEntityAttribute__TypeAssignment_1 : ( ( ruleQualifiedName ) ) ;
public final void rule__ValueObjectEntityAttribute__TypeAssignment_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39511:1: ( ( ( ruleQualifiedName ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39512:1: ( ( ruleQualifiedName ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39512:1: ( ( ruleQualifiedName ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39513:1: ( ruleQualifiedName )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getValueObjectEntityAttributeAccess().getTypeValueObjectCrossReference_1_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39514:1: ( ruleQualifiedName )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39515:1: ruleQualifiedName
{
if ( state.backtracking==0 ) {
before(grammarAccess.getValueObjectEntityAttributeAccess().getTypeValueObjectQualifiedNameParserRuleCall_1_0_1());
}
pushFollow(FollowSets003.FOLLOW_ruleQualifiedName_in_rule__ValueObjectEntityAttribute__TypeAssignment_179338);
ruleQualifiedName();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getValueObjectEntityAttributeAccess().getTypeValueObjectQualifiedNameParserRuleCall_1_0_1());
}
}
if ( state.backtracking==0 ) {
after(grammarAccess.getValueObjectEntityAttributeAccess().getTypeValueObjectCrossReference_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ValueObjectEntityAttribute__TypeAssignment_1"
// $ANTLR start "rule__ValueObjectEntityAttribute__CardinalityAssignment_2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39526:1: rule__ValueObjectEntityAttribute__CardinalityAssignment_2 : ( ruleCardinality ) ;
public final void rule__ValueObjectEntityAttribute__CardinalityAssignment_2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39530:1: ( ( ruleCardinality ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39531:1: ( ruleCardinality )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39531:1: ( ruleCardinality )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39532:1: ruleCardinality
{
if ( state.backtracking==0 ) {
before(grammarAccess.getValueObjectEntityAttributeAccess().getCardinalityCardinalityEnumRuleCall_2_0());
}
pushFollow(FollowSets003.FOLLOW_ruleCardinality_in_rule__ValueObjectEntityAttribute__CardinalityAssignment_279373);
ruleCardinality();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getValueObjectEntityAttributeAccess().getCardinalityCardinalityEnumRuleCall_2_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ValueObjectEntityAttribute__CardinalityAssignment_2"
// $ANTLR start "rule__ValueObjectEntityAttribute__NameAssignment_3"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39541:1: rule__ValueObjectEntityAttribute__NameAssignment_3 : ( RULE_ID ) ;
public final void rule__ValueObjectEntityAttribute__NameAssignment_3() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39545:1: ( ( RULE_ID ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39546:1: ( RULE_ID )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39546:1: ( RULE_ID )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39547:1: RULE_ID
{
if ( state.backtracking==0 ) {
before(grammarAccess.getValueObjectEntityAttributeAccess().getNameIDTerminalRuleCall_3_0());
}
match(input,RULE_ID,FollowSets003.FOLLOW_RULE_ID_in_rule__ValueObjectEntityAttribute__NameAssignment_379404); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getValueObjectEntityAttributeAccess().getNameIDTerminalRuleCall_3_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ValueObjectEntityAttribute__NameAssignment_3"
// $ANTLR start "rule__EnumerationDataType__NameAssignment_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39556:1: rule__EnumerationDataType__NameAssignment_1 : ( RULE_ID ) ;
public final void rule__EnumerationDataType__NameAssignment_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39560:1: ( ( RULE_ID ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39561:1: ( RULE_ID )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39561:1: ( RULE_ID )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39562:1: RULE_ID
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEnumerationDataTypeAccess().getNameIDTerminalRuleCall_1_0());
}
match(input,RULE_ID,FollowSets003.FOLLOW_RULE_ID_in_rule__EnumerationDataType__NameAssignment_179435); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getEnumerationDataTypeAccess().getNameIDTerminalRuleCall_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EnumerationDataType__NameAssignment_1"
// $ANTLR start "rule__EnumerationDataType__RefAssignment_2_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39571:1: rule__EnumerationDataType__RefAssignment_2_1 : ( ( ruleQualifiedName ) ) ;
public final void rule__EnumerationDataType__RefAssignment_2_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39575:1: ( ( ( ruleQualifiedName ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39576:1: ( ( ruleQualifiedName ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39576:1: ( ( ruleQualifiedName ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39577:1: ( ruleQualifiedName )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEnumerationDataTypeAccess().getRefEnumerationDataTypeCrossReference_2_1_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39578:1: ( ruleQualifiedName )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39579:1: ruleQualifiedName
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEnumerationDataTypeAccess().getRefEnumerationDataTypeQualifiedNameParserRuleCall_2_1_0_1());
}
pushFollow(FollowSets003.FOLLOW_ruleQualifiedName_in_rule__EnumerationDataType__RefAssignment_2_179470);
ruleQualifiedName();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getEnumerationDataTypeAccess().getRefEnumerationDataTypeQualifiedNameParserRuleCall_2_1_0_1());
}
}
if ( state.backtracking==0 ) {
after(grammarAccess.getEnumerationDataTypeAccess().getRefEnumerationDataTypeCrossReference_2_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EnumerationDataType__RefAssignment_2_1"
// $ANTLR start "rule__EnumerationDataType__BaseDataTypeAssignment_4"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39590:1: rule__EnumerationDataType__BaseDataTypeAssignment_4 : ( ruleBaseDataType ) ;
public final void rule__EnumerationDataType__BaseDataTypeAssignment_4() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39594:1: ( ( ruleBaseDataType ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39595:1: ( ruleBaseDataType )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39595:1: ( ruleBaseDataType )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39596:1: ruleBaseDataType
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEnumerationDataTypeAccess().getBaseDataTypeBaseDataTypeParserRuleCall_4_0());
}
pushFollow(FollowSets003.FOLLOW_ruleBaseDataType_in_rule__EnumerationDataType__BaseDataTypeAssignment_479505);
ruleBaseDataType();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getEnumerationDataTypeAccess().getBaseDataTypeBaseDataTypeParserRuleCall_4_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EnumerationDataType__BaseDataTypeAssignment_4"
// $ANTLR start "rule__EnumerationDataType__EnumerationAssignment_6"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39605:1: rule__EnumerationDataType__EnumerationAssignment_6 : ( ( ruleQualifiedName ) ) ;
public final void rule__EnumerationDataType__EnumerationAssignment_6() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39609:1: ( ( ( ruleQualifiedName ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39610:1: ( ( ruleQualifiedName ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39610:1: ( ( ruleQualifiedName ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39611:1: ( ruleQualifiedName )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEnumerationDataTypeAccess().getEnumerationEnumerationCrossReference_6_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39612:1: ( ruleQualifiedName )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39613:1: ruleQualifiedName
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEnumerationDataTypeAccess().getEnumerationEnumerationQualifiedNameParserRuleCall_6_0_1());
}
pushFollow(FollowSets003.FOLLOW_ruleQualifiedName_in_rule__EnumerationDataType__EnumerationAssignment_679540);
ruleQualifiedName();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getEnumerationDataTypeAccess().getEnumerationEnumerationQualifiedNameParserRuleCall_6_0_1());
}
}
if ( state.backtracking==0 ) {
after(grammarAccess.getEnumerationDataTypeAccess().getEnumerationEnumerationCrossReference_6_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EnumerationDataType__EnumerationAssignment_6"
// $ANTLR start "rule__EnumerationEntityAttribute__TypeAssignment_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39624:1: rule__EnumerationEntityAttribute__TypeAssignment_1 : ( ( ruleQualifiedName ) ) ;
public final void rule__EnumerationEntityAttribute__TypeAssignment_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39628:1: ( ( ( ruleQualifiedName ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39629:1: ( ( ruleQualifiedName ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39629:1: ( ( ruleQualifiedName ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39630:1: ( ruleQualifiedName )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEnumerationEntityAttributeAccess().getTypeEnumerationAttributeTypeCrossReference_1_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39631:1: ( ruleQualifiedName )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39632:1: ruleQualifiedName
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEnumerationEntityAttributeAccess().getTypeEnumerationAttributeTypeQualifiedNameParserRuleCall_1_0_1());
}
pushFollow(FollowSets003.FOLLOW_ruleQualifiedName_in_rule__EnumerationEntityAttribute__TypeAssignment_179579);
ruleQualifiedName();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getEnumerationEntityAttributeAccess().getTypeEnumerationAttributeTypeQualifiedNameParserRuleCall_1_0_1());
}
}
if ( state.backtracking==0 ) {
after(grammarAccess.getEnumerationEntityAttributeAccess().getTypeEnumerationAttributeTypeCrossReference_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EnumerationEntityAttribute__TypeAssignment_1"
// $ANTLR start "rule__EnumerationEntityAttribute__CardinalityAssignment_2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39643:1: rule__EnumerationEntityAttribute__CardinalityAssignment_2 : ( ruleCardinality ) ;
public final void rule__EnumerationEntityAttribute__CardinalityAssignment_2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39647:1: ( ( ruleCardinality ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39648:1: ( ruleCardinality )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39648:1: ( ruleCardinality )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39649:1: ruleCardinality
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEnumerationEntityAttributeAccess().getCardinalityCardinalityEnumRuleCall_2_0());
}
pushFollow(FollowSets003.FOLLOW_ruleCardinality_in_rule__EnumerationEntityAttribute__CardinalityAssignment_279614);
ruleCardinality();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getEnumerationEntityAttributeAccess().getCardinalityCardinalityEnumRuleCall_2_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EnumerationEntityAttribute__CardinalityAssignment_2"
// $ANTLR start "rule__EnumerationEntityAttribute__NameAssignment_3"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39658:1: rule__EnumerationEntityAttribute__NameAssignment_3 : ( RULE_ID ) ;
public final void rule__EnumerationEntityAttribute__NameAssignment_3() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39662:1: ( ( RULE_ID ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39663:1: ( RULE_ID )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39663:1: ( RULE_ID )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39664:1: RULE_ID
{
if ( state.backtracking==0 ) {
before(grammarAccess.getEnumerationEntityAttributeAccess().getNameIDTerminalRuleCall_3_0());
}
match(input,RULE_ID,FollowSets003.FOLLOW_RULE_ID_in_rule__EnumerationEntityAttribute__NameAssignment_379645); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getEnumerationEntityAttributeAccess().getNameIDTerminalRuleCall_3_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__EnumerationEntityAttribute__NameAssignment_3"
// $ANTLR start "rule__Dictionary__NameAssignment_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39673:1: rule__Dictionary__NameAssignment_1 : ( RULE_ID ) ;
public final void rule__Dictionary__NameAssignment_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39677:1: ( ( RULE_ID ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39678:1: ( RULE_ID )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39678:1: ( RULE_ID )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39679:1: RULE_ID
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryAccess().getNameIDTerminalRuleCall_1_0());
}
match(input,RULE_ID,FollowSets003.FOLLOW_RULE_ID_in_rule__Dictionary__NameAssignment_179676); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryAccess().getNameIDTerminalRuleCall_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Dictionary__NameAssignment_1"
// $ANTLR start "rule__Dictionary__EntityAssignment_4"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39688:1: rule__Dictionary__EntityAssignment_4 : ( ( ruleQualifiedName ) ) ;
public final void rule__Dictionary__EntityAssignment_4() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39692:1: ( ( ( ruleQualifiedName ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39693:1: ( ( ruleQualifiedName ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39693:1: ( ( ruleQualifiedName ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39694:1: ( ruleQualifiedName )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryAccess().getEntityEntityCrossReference_4_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39695:1: ( ruleQualifiedName )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39696:1: ruleQualifiedName
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryAccess().getEntityEntityQualifiedNameParserRuleCall_4_0_1());
}
pushFollow(FollowSets003.FOLLOW_ruleQualifiedName_in_rule__Dictionary__EntityAssignment_479711);
ruleQualifiedName();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryAccess().getEntityEntityQualifiedNameParserRuleCall_4_0_1());
}
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryAccess().getEntityEntityCrossReference_4_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Dictionary__EntityAssignment_4"
// $ANTLR start "rule__Dictionary__LabelAssignment_5_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39707:1: rule__Dictionary__LabelAssignment_5_1 : ( RULE_STRING ) ;
public final void rule__Dictionary__LabelAssignment_5_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39711:1: ( ( RULE_STRING ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39712:1: ( RULE_STRING )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39712:1: ( RULE_STRING )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39713:1: RULE_STRING
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryAccess().getLabelSTRINGTerminalRuleCall_5_1_0());
}
match(input,RULE_STRING,FollowSets003.FOLLOW_RULE_STRING_in_rule__Dictionary__LabelAssignment_5_179746); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryAccess().getLabelSTRINGTerminalRuleCall_5_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Dictionary__LabelAssignment_5_1"
// $ANTLR start "rule__Dictionary__PluralLabelAssignment_6_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39722:1: rule__Dictionary__PluralLabelAssignment_6_1 : ( RULE_STRING ) ;
public final void rule__Dictionary__PluralLabelAssignment_6_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39726:1: ( ( RULE_STRING ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39727:1: ( RULE_STRING )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39727:1: ( RULE_STRING )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39728:1: RULE_STRING
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryAccess().getPluralLabelSTRINGTerminalRuleCall_6_1_0());
}
match(input,RULE_STRING,FollowSets003.FOLLOW_RULE_STRING_in_rule__Dictionary__PluralLabelAssignment_6_179777); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryAccess().getPluralLabelSTRINGTerminalRuleCall_6_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Dictionary__PluralLabelAssignment_6_1"
// $ANTLR start "rule__Dictionary__DictionarycontrolsAssignment_7_2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39737:1: rule__Dictionary__DictionarycontrolsAssignment_7_2 : ( ruleDictionaryControl ) ;
public final void rule__Dictionary__DictionarycontrolsAssignment_7_2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39741:1: ( ( ruleDictionaryControl ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39742:1: ( ruleDictionaryControl )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39742:1: ( ruleDictionaryControl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39743:1: ruleDictionaryControl
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryAccess().getDictionarycontrolsDictionaryControlParserRuleCall_7_2_0());
}
pushFollow(FollowSets003.FOLLOW_ruleDictionaryControl_in_rule__Dictionary__DictionarycontrolsAssignment_7_279808);
ruleDictionaryControl();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryAccess().getDictionarycontrolsDictionaryControlParserRuleCall_7_2_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Dictionary__DictionarycontrolsAssignment_7_2"
// $ANTLR start "rule__Dictionary__LabelcontrolsAssignment_8_2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39752:1: rule__Dictionary__LabelcontrolsAssignment_8_2 : ( ruleDictionaryControl ) ;
public final void rule__Dictionary__LabelcontrolsAssignment_8_2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39756:1: ( ( ruleDictionaryControl ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39757:1: ( ruleDictionaryControl )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39757:1: ( ruleDictionaryControl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39758:1: ruleDictionaryControl
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryAccess().getLabelcontrolsDictionaryControlParserRuleCall_8_2_0());
}
pushFollow(FollowSets003.FOLLOW_ruleDictionaryControl_in_rule__Dictionary__LabelcontrolsAssignment_8_279839);
ruleDictionaryControl();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryAccess().getLabelcontrolsDictionaryControlParserRuleCall_8_2_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Dictionary__LabelcontrolsAssignment_8_2"
// $ANTLR start "rule__Dictionary__DictionarysearchAssignment_9"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39767:1: rule__Dictionary__DictionarysearchAssignment_9 : ( ruleDictionarySearch ) ;
public final void rule__Dictionary__DictionarysearchAssignment_9() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39771:1: ( ( ruleDictionarySearch ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39772:1: ( ruleDictionarySearch )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39772:1: ( ruleDictionarySearch )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39773:1: ruleDictionarySearch
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryAccess().getDictionarysearchDictionarySearchParserRuleCall_9_0());
}
pushFollow(FollowSets003.FOLLOW_ruleDictionarySearch_in_rule__Dictionary__DictionarysearchAssignment_979870);
ruleDictionarySearch();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryAccess().getDictionarysearchDictionarySearchParserRuleCall_9_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Dictionary__DictionarysearchAssignment_9"
// $ANTLR start "rule__Dictionary__DictionaryeditorAssignment_10"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39782:1: rule__Dictionary__DictionaryeditorAssignment_10 : ( ruleDictionaryEditor ) ;
public final void rule__Dictionary__DictionaryeditorAssignment_10() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39786:1: ( ( ruleDictionaryEditor ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39787:1: ( ruleDictionaryEditor )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39787:1: ( ruleDictionaryEditor )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39788:1: ruleDictionaryEditor
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryAccess().getDictionaryeditorDictionaryEditorParserRuleCall_10_0());
}
pushFollow(FollowSets003.FOLLOW_ruleDictionaryEditor_in_rule__Dictionary__DictionaryeditorAssignment_1079901);
ruleDictionaryEditor();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryAccess().getDictionaryeditorDictionaryEditorParserRuleCall_10_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Dictionary__DictionaryeditorAssignment_10"
// $ANTLR start "rule__DictionarySearch__NameAssignment_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39797:1: rule__DictionarySearch__NameAssignment_1 : ( RULE_ID ) ;
public final void rule__DictionarySearch__NameAssignment_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39801:1: ( ( RULE_ID ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39802:1: ( RULE_ID )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39802:1: ( RULE_ID )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39803:1: RULE_ID
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionarySearchAccess().getNameIDTerminalRuleCall_1_0());
}
match(input,RULE_ID,FollowSets003.FOLLOW_RULE_ID_in_rule__DictionarySearch__NameAssignment_179932); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionarySearchAccess().getNameIDTerminalRuleCall_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionarySearch__NameAssignment_1"
// $ANTLR start "rule__DictionarySearch__LabelAssignment_3_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39812:1: rule__DictionarySearch__LabelAssignment_3_1 : ( RULE_STRING ) ;
public final void rule__DictionarySearch__LabelAssignment_3_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39816:1: ( ( RULE_STRING ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39817:1: ( RULE_STRING )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39817:1: ( RULE_STRING )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39818:1: RULE_STRING
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionarySearchAccess().getLabelSTRINGTerminalRuleCall_3_1_0());
}
match(input,RULE_STRING,FollowSets003.FOLLOW_RULE_STRING_in_rule__DictionarySearch__LabelAssignment_3_179963); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionarySearchAccess().getLabelSTRINGTerminalRuleCall_3_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionarySearch__LabelAssignment_3_1"
// $ANTLR start "rule__DictionarySearch__DictionaryfiltersAssignment_4"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39827:1: rule__DictionarySearch__DictionaryfiltersAssignment_4 : ( ruleDictionaryFilter ) ;
public final void rule__DictionarySearch__DictionaryfiltersAssignment_4() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39831:1: ( ( ruleDictionaryFilter ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39832:1: ( ruleDictionaryFilter )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39832:1: ( ruleDictionaryFilter )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39833:1: ruleDictionaryFilter
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionarySearchAccess().getDictionaryfiltersDictionaryFilterParserRuleCall_4_0());
}
pushFollow(FollowSets003.FOLLOW_ruleDictionaryFilter_in_rule__DictionarySearch__DictionaryfiltersAssignment_479994);
ruleDictionaryFilter();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionarySearchAccess().getDictionaryfiltersDictionaryFilterParserRuleCall_4_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionarySearch__DictionaryfiltersAssignment_4"
// $ANTLR start "rule__DictionarySearch__DictionaryresultAssignment_5"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39842:1: rule__DictionarySearch__DictionaryresultAssignment_5 : ( ruleDictionaryResult ) ;
public final void rule__DictionarySearch__DictionaryresultAssignment_5() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39846:1: ( ( ruleDictionaryResult ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39847:1: ( ruleDictionaryResult )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39847:1: ( ruleDictionaryResult )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39848:1: ruleDictionaryResult
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionarySearchAccess().getDictionaryresultDictionaryResultParserRuleCall_5_0());
}
pushFollow(FollowSets003.FOLLOW_ruleDictionaryResult_in_rule__DictionarySearch__DictionaryresultAssignment_580025);
ruleDictionaryResult();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionarySearchAccess().getDictionaryresultDictionaryResultParserRuleCall_5_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionarySearch__DictionaryresultAssignment_5"
// $ANTLR start "rule__DictionaryEditor__NameAssignment_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39857:1: rule__DictionaryEditor__NameAssignment_1 : ( RULE_ID ) ;
public final void rule__DictionaryEditor__NameAssignment_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39861:1: ( ( RULE_ID ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39862:1: ( RULE_ID )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39862:1: ( RULE_ID )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39863:1: RULE_ID
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryEditorAccess().getNameIDTerminalRuleCall_1_0());
}
match(input,RULE_ID,FollowSets003.FOLLOW_RULE_ID_in_rule__DictionaryEditor__NameAssignment_180056); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryEditorAccess().getNameIDTerminalRuleCall_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryEditor__NameAssignment_1"
// $ANTLR start "rule__DictionaryEditor__LabelAssignment_3_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39872:1: rule__DictionaryEditor__LabelAssignment_3_1 : ( RULE_STRING ) ;
public final void rule__DictionaryEditor__LabelAssignment_3_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39876:1: ( ( RULE_STRING ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39877:1: ( RULE_STRING )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39877:1: ( RULE_STRING )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39878:1: RULE_STRING
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryEditorAccess().getLabelSTRINGTerminalRuleCall_3_1_0());
}
match(input,RULE_STRING,FollowSets003.FOLLOW_RULE_STRING_in_rule__DictionaryEditor__LabelAssignment_3_180087); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryEditorAccess().getLabelSTRINGTerminalRuleCall_3_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryEditor__LabelAssignment_3_1"
// $ANTLR start "rule__DictionaryEditor__LayoutdataAssignment_4"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39887:1: rule__DictionaryEditor__LayoutdataAssignment_4 : ( ruleColumnLayoutData ) ;
public final void rule__DictionaryEditor__LayoutdataAssignment_4() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39891:1: ( ( ruleColumnLayoutData ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39892:1: ( ruleColumnLayoutData )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39892:1: ( ruleColumnLayoutData )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39893:1: ruleColumnLayoutData
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryEditorAccess().getLayoutdataColumnLayoutDataParserRuleCall_4_0());
}
pushFollow(FollowSets003.FOLLOW_ruleColumnLayoutData_in_rule__DictionaryEditor__LayoutdataAssignment_480118);
ruleColumnLayoutData();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryEditorAccess().getLayoutdataColumnLayoutDataParserRuleCall_4_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryEditor__LayoutdataAssignment_4"
// $ANTLR start "rule__DictionaryEditor__LayoutAssignment_5"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39902:1: rule__DictionaryEditor__LayoutAssignment_5 : ( ruleColumnLayout ) ;
public final void rule__DictionaryEditor__LayoutAssignment_5() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39906:1: ( ( ruleColumnLayout ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39907:1: ( ruleColumnLayout )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39907:1: ( ruleColumnLayout )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39908:1: ruleColumnLayout
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryEditorAccess().getLayoutColumnLayoutParserRuleCall_5_0());
}
pushFollow(FollowSets003.FOLLOW_ruleColumnLayout_in_rule__DictionaryEditor__LayoutAssignment_580149);
ruleColumnLayout();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryEditorAccess().getLayoutColumnLayoutParserRuleCall_5_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryEditor__LayoutAssignment_5"
// $ANTLR start "rule__DictionaryEditor__ContainercontentsAssignment_6"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39917:1: rule__DictionaryEditor__ContainercontentsAssignment_6 : ( ruleDictionaryContainerContent ) ;
public final void rule__DictionaryEditor__ContainercontentsAssignment_6() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39921:1: ( ( ruleDictionaryContainerContent ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39922:1: ( ruleDictionaryContainerContent )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39922:1: ( ruleDictionaryContainerContent )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39923:1: ruleDictionaryContainerContent
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryEditorAccess().getContainercontentsDictionaryContainerContentParserRuleCall_6_0());
}
pushFollow(FollowSets003.FOLLOW_ruleDictionaryContainerContent_in_rule__DictionaryEditor__ContainercontentsAssignment_680180);
ruleDictionaryContainerContent();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryEditorAccess().getContainercontentsDictionaryContainerContentParserRuleCall_6_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryEditor__ContainercontentsAssignment_6"
// $ANTLR start "rule__DictionaryFilter__NameAssignment_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39932:1: rule__DictionaryFilter__NameAssignment_1 : ( RULE_ID ) ;
public final void rule__DictionaryFilter__NameAssignment_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39936:1: ( ( RULE_ID ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39937:1: ( RULE_ID )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39937:1: ( RULE_ID )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39938:1: RULE_ID
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryFilterAccess().getNameIDTerminalRuleCall_1_0());
}
match(input,RULE_ID,FollowSets003.FOLLOW_RULE_ID_in_rule__DictionaryFilter__NameAssignment_180211); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryFilterAccess().getNameIDTerminalRuleCall_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryFilter__NameAssignment_1"
// $ANTLR start "rule__DictionaryFilter__LayoutdataAssignment_3"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39947:1: rule__DictionaryFilter__LayoutdataAssignment_3 : ( ruleColumnLayoutData ) ;
public final void rule__DictionaryFilter__LayoutdataAssignment_3() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39951:1: ( ( ruleColumnLayoutData ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39952:1: ( ruleColumnLayoutData )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39952:1: ( ruleColumnLayoutData )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39953:1: ruleColumnLayoutData
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryFilterAccess().getLayoutdataColumnLayoutDataParserRuleCall_3_0());
}
pushFollow(FollowSets003.FOLLOW_ruleColumnLayoutData_in_rule__DictionaryFilter__LayoutdataAssignment_380242);
ruleColumnLayoutData();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryFilterAccess().getLayoutdataColumnLayoutDataParserRuleCall_3_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryFilter__LayoutdataAssignment_3"
// $ANTLR start "rule__DictionaryFilter__LayoutAssignment_4"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39962:1: rule__DictionaryFilter__LayoutAssignment_4 : ( ruleColumnLayout ) ;
public final void rule__DictionaryFilter__LayoutAssignment_4() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39966:1: ( ( ruleColumnLayout ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39967:1: ( ruleColumnLayout )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39967:1: ( ruleColumnLayout )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39968:1: ruleColumnLayout
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryFilterAccess().getLayoutColumnLayoutParserRuleCall_4_0());
}
pushFollow(FollowSets003.FOLLOW_ruleColumnLayout_in_rule__DictionaryFilter__LayoutAssignment_480273);
ruleColumnLayout();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryFilterAccess().getLayoutColumnLayoutParserRuleCall_4_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryFilter__LayoutAssignment_4"
// $ANTLR start "rule__DictionaryFilter__ContainercontentsAssignment_5"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39977:1: rule__DictionaryFilter__ContainercontentsAssignment_5 : ( ruleDictionaryContainerContent ) ;
public final void rule__DictionaryFilter__ContainercontentsAssignment_5() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39981:1: ( ( ruleDictionaryContainerContent ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39982:1: ( ruleDictionaryContainerContent )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39982:1: ( ruleDictionaryContainerContent )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39983:1: ruleDictionaryContainerContent
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryFilterAccess().getContainercontentsDictionaryContainerContentParserRuleCall_5_0());
}
pushFollow(FollowSets003.FOLLOW_ruleDictionaryContainerContent_in_rule__DictionaryFilter__ContainercontentsAssignment_580304);
ruleDictionaryContainerContent();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryFilterAccess().getContainercontentsDictionaryContainerContentParserRuleCall_5_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryFilter__ContainercontentsAssignment_5"
// $ANTLR start "rule__DictionaryResult__NameAssignment_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39992:1: rule__DictionaryResult__NameAssignment_1 : ( RULE_ID ) ;
public final void rule__DictionaryResult__NameAssignment_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39996:1: ( ( RULE_ID ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39997:1: ( RULE_ID )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39997:1: ( RULE_ID )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:39998:1: RULE_ID
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryResultAccess().getNameIDTerminalRuleCall_1_0());
}
match(input,RULE_ID,FollowSets003.FOLLOW_RULE_ID_in_rule__DictionaryResult__NameAssignment_180335); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryResultAccess().getNameIDTerminalRuleCall_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryResult__NameAssignment_1"
// $ANTLR start "rule__DictionaryResult__ResultcolumnsAssignment_3"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40007:1: rule__DictionaryResult__ResultcolumnsAssignment_3 : ( ruleDictionaryControl ) ;
public final void rule__DictionaryResult__ResultcolumnsAssignment_3() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40011:1: ( ( ruleDictionaryControl ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40012:1: ( ruleDictionaryControl )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40012:1: ( ruleDictionaryControl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40013:1: ruleDictionaryControl
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryResultAccess().getResultcolumnsDictionaryControlParserRuleCall_3_0());
}
pushFollow(FollowSets003.FOLLOW_ruleDictionaryControl_in_rule__DictionaryResult__ResultcolumnsAssignment_380366);
ruleDictionaryControl();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryResultAccess().getResultcolumnsDictionaryControlParserRuleCall_3_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryResult__ResultcolumnsAssignment_3"
// $ANTLR start "rule__ColumnLayout__ColumnsAssignment_3"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40022:1: rule__ColumnLayout__ColumnsAssignment_3 : ( RULE_INT ) ;
public final void rule__ColumnLayout__ColumnsAssignment_3() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40026:1: ( ( RULE_INT ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40027:1: ( RULE_INT )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40027:1: ( RULE_INT )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40028:1: RULE_INT
{
if ( state.backtracking==0 ) {
before(grammarAccess.getColumnLayoutAccess().getColumnsINTTerminalRuleCall_3_0());
}
match(input,RULE_INT,FollowSets003.FOLLOW_RULE_INT_in_rule__ColumnLayout__ColumnsAssignment_380397); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getColumnLayoutAccess().getColumnsINTTerminalRuleCall_3_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ColumnLayout__ColumnsAssignment_3"
// $ANTLR start "rule__ColumnLayoutData__ColumnspanAssignment_3"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40037:1: rule__ColumnLayoutData__ColumnspanAssignment_3 : ( RULE_INT ) ;
public final void rule__ColumnLayoutData__ColumnspanAssignment_3() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40041:1: ( ( RULE_INT ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40042:1: ( RULE_INT )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40042:1: ( RULE_INT )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40043:1: RULE_INT
{
if ( state.backtracking==0 ) {
before(grammarAccess.getColumnLayoutDataAccess().getColumnspanINTTerminalRuleCall_3_0());
}
match(input,RULE_INT,FollowSets003.FOLLOW_RULE_INT_in_rule__ColumnLayoutData__ColumnspanAssignment_380428); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getColumnLayoutDataAccess().getColumnspanINTTerminalRuleCall_3_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ColumnLayoutData__ColumnspanAssignment_3"
// $ANTLR start "rule__DictionaryComposite__NameAssignment_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40052:1: rule__DictionaryComposite__NameAssignment_1 : ( RULE_ID ) ;
public final void rule__DictionaryComposite__NameAssignment_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40056:1: ( ( RULE_ID ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40057:1: ( RULE_ID )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40057:1: ( RULE_ID )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40058:1: RULE_ID
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryCompositeAccess().getNameIDTerminalRuleCall_1_0());
}
match(input,RULE_ID,FollowSets003.FOLLOW_RULE_ID_in_rule__DictionaryComposite__NameAssignment_180459); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryCompositeAccess().getNameIDTerminalRuleCall_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryComposite__NameAssignment_1"
// $ANTLR start "rule__DictionaryComposite__LayoutdataAssignment_3"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40067:1: rule__DictionaryComposite__LayoutdataAssignment_3 : ( ruleColumnLayoutData ) ;
public final void rule__DictionaryComposite__LayoutdataAssignment_3() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40071:1: ( ( ruleColumnLayoutData ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40072:1: ( ruleColumnLayoutData )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40072:1: ( ruleColumnLayoutData )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40073:1: ruleColumnLayoutData
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryCompositeAccess().getLayoutdataColumnLayoutDataParserRuleCall_3_0());
}
pushFollow(FollowSets003.FOLLOW_ruleColumnLayoutData_in_rule__DictionaryComposite__LayoutdataAssignment_380490);
ruleColumnLayoutData();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryCompositeAccess().getLayoutdataColumnLayoutDataParserRuleCall_3_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryComposite__LayoutdataAssignment_3"
// $ANTLR start "rule__DictionaryComposite__LayoutAssignment_4"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40082:1: rule__DictionaryComposite__LayoutAssignment_4 : ( ruleColumnLayout ) ;
public final void rule__DictionaryComposite__LayoutAssignment_4() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40086:1: ( ( ruleColumnLayout ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40087:1: ( ruleColumnLayout )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40087:1: ( ruleColumnLayout )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40088:1: ruleColumnLayout
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryCompositeAccess().getLayoutColumnLayoutParserRuleCall_4_0());
}
pushFollow(FollowSets003.FOLLOW_ruleColumnLayout_in_rule__DictionaryComposite__LayoutAssignment_480521);
ruleColumnLayout();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryCompositeAccess().getLayoutColumnLayoutParserRuleCall_4_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryComposite__LayoutAssignment_4"
// $ANTLR start "rule__DictionaryComposite__ContainercontentsAssignment_5"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40097:1: rule__DictionaryComposite__ContainercontentsAssignment_5 : ( ruleDictionaryContainerContent ) ;
public final void rule__DictionaryComposite__ContainercontentsAssignment_5() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40101:1: ( ( ruleDictionaryContainerContent ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40102:1: ( ruleDictionaryContainerContent )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40102:1: ( ruleDictionaryContainerContent )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40103:1: ruleDictionaryContainerContent
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryCompositeAccess().getContainercontentsDictionaryContainerContentParserRuleCall_5_0());
}
pushFollow(FollowSets003.FOLLOW_ruleDictionaryContainerContent_in_rule__DictionaryComposite__ContainercontentsAssignment_580552);
ruleDictionaryContainerContent();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryCompositeAccess().getContainercontentsDictionaryContainerContentParserRuleCall_5_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryComposite__ContainercontentsAssignment_5"
// $ANTLR start "rule__DictionaryEditableTable__NameAssignment_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40112:1: rule__DictionaryEditableTable__NameAssignment_1 : ( RULE_ID ) ;
public final void rule__DictionaryEditableTable__NameAssignment_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40116:1: ( ( RULE_ID ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40117:1: ( RULE_ID )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40117:1: ( RULE_ID )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40118:1: RULE_ID
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryEditableTableAccess().getNameIDTerminalRuleCall_1_0());
}
match(input,RULE_ID,FollowSets003.FOLLOW_RULE_ID_in_rule__DictionaryEditableTable__NameAssignment_180583); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryEditableTableAccess().getNameIDTerminalRuleCall_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryEditableTable__NameAssignment_1"
// $ANTLR start "rule__DictionaryEditableTable__LayoutdataAssignment_3_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40127:1: rule__DictionaryEditableTable__LayoutdataAssignment_3_1 : ( ruleColumnLayoutData ) ;
public final void rule__DictionaryEditableTable__LayoutdataAssignment_3_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40131:1: ( ( ruleColumnLayoutData ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40132:1: ( ruleColumnLayoutData )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40132:1: ( ruleColumnLayoutData )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40133:1: ruleColumnLayoutData
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryEditableTableAccess().getLayoutdataColumnLayoutDataParserRuleCall_3_1_0());
}
pushFollow(FollowSets003.FOLLOW_ruleColumnLayoutData_in_rule__DictionaryEditableTable__LayoutdataAssignment_3_180614);
ruleColumnLayoutData();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryEditableTableAccess().getLayoutdataColumnLayoutDataParserRuleCall_3_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryEditableTable__LayoutdataAssignment_3_1"
// $ANTLR start "rule__DictionaryEditableTable__LayoutAssignment_4_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40142:1: rule__DictionaryEditableTable__LayoutAssignment_4_1 : ( ruleColumnLayout ) ;
public final void rule__DictionaryEditableTable__LayoutAssignment_4_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40146:1: ( ( ruleColumnLayout ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40147:1: ( ruleColumnLayout )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40147:1: ( ruleColumnLayout )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40148:1: ruleColumnLayout
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryEditableTableAccess().getLayoutColumnLayoutParserRuleCall_4_1_0());
}
pushFollow(FollowSets003.FOLLOW_ruleColumnLayout_in_rule__DictionaryEditableTable__LayoutAssignment_4_180645);
ruleColumnLayout();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryEditableTableAccess().getLayoutColumnLayoutParserRuleCall_4_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryEditableTable__LayoutAssignment_4_1"
// $ANTLR start "rule__DictionaryEditableTable__ContainercontentsAssignment_5"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40157:1: rule__DictionaryEditableTable__ContainercontentsAssignment_5 : ( ruleDictionaryContainerContent ) ;
public final void rule__DictionaryEditableTable__ContainercontentsAssignment_5() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40161:1: ( ( ruleDictionaryContainerContent ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40162:1: ( ruleDictionaryContainerContent )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40162:1: ( ruleDictionaryContainerContent )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40163:1: ruleDictionaryContainerContent
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryEditableTableAccess().getContainercontentsDictionaryContainerContentParserRuleCall_5_0());
}
pushFollow(FollowSets003.FOLLOW_ruleDictionaryContainerContent_in_rule__DictionaryEditableTable__ContainercontentsAssignment_580676);
ruleDictionaryContainerContent();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryEditableTableAccess().getContainercontentsDictionaryContainerContentParserRuleCall_5_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryEditableTable__ContainercontentsAssignment_5"
// $ANTLR start "rule__DictionaryEditableTable__EntityattributeAssignment_7"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40172:1: rule__DictionaryEditableTable__EntityattributeAssignment_7 : ( ( ruleQualifiedName ) ) ;
public final void rule__DictionaryEditableTable__EntityattributeAssignment_7() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40176:1: ( ( ( ruleQualifiedName ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40177:1: ( ( ruleQualifiedName ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40177:1: ( ( ruleQualifiedName ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40178:1: ( ruleQualifiedName )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryEditableTableAccess().getEntityattributeEntityAttributeCrossReference_7_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40179:1: ( ruleQualifiedName )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40180:1: ruleQualifiedName
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryEditableTableAccess().getEntityattributeEntityAttributeQualifiedNameParserRuleCall_7_0_1());
}
pushFollow(FollowSets003.FOLLOW_ruleQualifiedName_in_rule__DictionaryEditableTable__EntityattributeAssignment_780711);
ruleQualifiedName();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryEditableTableAccess().getEntityattributeEntityAttributeQualifiedNameParserRuleCall_7_0_1());
}
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryEditableTableAccess().getEntityattributeEntityAttributeCrossReference_7_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryEditableTable__EntityattributeAssignment_7"
// $ANTLR start "rule__DictionaryEditableTable__ColumncontrolsAssignment_10"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40191:1: rule__DictionaryEditableTable__ColumncontrolsAssignment_10 : ( ruleDictionaryControl ) ;
public final void rule__DictionaryEditableTable__ColumncontrolsAssignment_10() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40195:1: ( ( ruleDictionaryControl ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40196:1: ( ruleDictionaryControl )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40196:1: ( ruleDictionaryControl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40197:1: ruleDictionaryControl
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryEditableTableAccess().getColumncontrolsDictionaryControlParserRuleCall_10_0());
}
pushFollow(FollowSets003.FOLLOW_ruleDictionaryControl_in_rule__DictionaryEditableTable__ColumncontrolsAssignment_1080746);
ruleDictionaryControl();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryEditableTableAccess().getColumncontrolsDictionaryControlParserRuleCall_10_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryEditableTable__ColumncontrolsAssignment_10"
// $ANTLR start "rule__DictionaryAssignmentTable__NameAssignment_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40206:1: rule__DictionaryAssignmentTable__NameAssignment_1 : ( RULE_ID ) ;
public final void rule__DictionaryAssignmentTable__NameAssignment_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40210:1: ( ( RULE_ID ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40211:1: ( RULE_ID )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40211:1: ( RULE_ID )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40212:1: RULE_ID
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryAssignmentTableAccess().getNameIDTerminalRuleCall_1_0());
}
match(input,RULE_ID,FollowSets003.FOLLOW_RULE_ID_in_rule__DictionaryAssignmentTable__NameAssignment_180777); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryAssignmentTableAccess().getNameIDTerminalRuleCall_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryAssignmentTable__NameAssignment_1"
// $ANTLR start "rule__DictionaryAssignmentTable__LayoutdataAssignment_3_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40221:1: rule__DictionaryAssignmentTable__LayoutdataAssignment_3_1 : ( ruleColumnLayoutData ) ;
public final void rule__DictionaryAssignmentTable__LayoutdataAssignment_3_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40225:1: ( ( ruleColumnLayoutData ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40226:1: ( ruleColumnLayoutData )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40226:1: ( ruleColumnLayoutData )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40227:1: ruleColumnLayoutData
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryAssignmentTableAccess().getLayoutdataColumnLayoutDataParserRuleCall_3_1_0());
}
pushFollow(FollowSets003.FOLLOW_ruleColumnLayoutData_in_rule__DictionaryAssignmentTable__LayoutdataAssignment_3_180808);
ruleColumnLayoutData();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryAssignmentTableAccess().getLayoutdataColumnLayoutDataParserRuleCall_3_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryAssignmentTable__LayoutdataAssignment_3_1"
// $ANTLR start "rule__DictionaryAssignmentTable__LayoutAssignment_4_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40236:1: rule__DictionaryAssignmentTable__LayoutAssignment_4_1 : ( ruleColumnLayout ) ;
public final void rule__DictionaryAssignmentTable__LayoutAssignment_4_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40240:1: ( ( ruleColumnLayout ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40241:1: ( ruleColumnLayout )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40241:1: ( ruleColumnLayout )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40242:1: ruleColumnLayout
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryAssignmentTableAccess().getLayoutColumnLayoutParserRuleCall_4_1_0());
}
pushFollow(FollowSets003.FOLLOW_ruleColumnLayout_in_rule__DictionaryAssignmentTable__LayoutAssignment_4_180839);
ruleColumnLayout();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryAssignmentTableAccess().getLayoutColumnLayoutParserRuleCall_4_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryAssignmentTable__LayoutAssignment_4_1"
// $ANTLR start "rule__DictionaryAssignmentTable__ContainercontentsAssignment_5"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40251:1: rule__DictionaryAssignmentTable__ContainercontentsAssignment_5 : ( ruleDictionaryContainerContent ) ;
public final void rule__DictionaryAssignmentTable__ContainercontentsAssignment_5() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40255:1: ( ( ruleDictionaryContainerContent ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40256:1: ( ruleDictionaryContainerContent )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40256:1: ( ruleDictionaryContainerContent )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40257:1: ruleDictionaryContainerContent
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryAssignmentTableAccess().getContainercontentsDictionaryContainerContentParserRuleCall_5_0());
}
pushFollow(FollowSets003.FOLLOW_ruleDictionaryContainerContent_in_rule__DictionaryAssignmentTable__ContainercontentsAssignment_580870);
ruleDictionaryContainerContent();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryAssignmentTableAccess().getContainercontentsDictionaryContainerContentParserRuleCall_5_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryAssignmentTable__ContainercontentsAssignment_5"
// $ANTLR start "rule__DictionaryAssignmentTable__EntityattributeAssignment_7"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40266:1: rule__DictionaryAssignmentTable__EntityattributeAssignment_7 : ( ( ruleQualifiedName ) ) ;
public final void rule__DictionaryAssignmentTable__EntityattributeAssignment_7() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40270:1: ( ( ( ruleQualifiedName ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40271:1: ( ( ruleQualifiedName ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40271:1: ( ( ruleQualifiedName ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40272:1: ( ruleQualifiedName )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryAssignmentTableAccess().getEntityattributeEntityAttributeCrossReference_7_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40273:1: ( ruleQualifiedName )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40274:1: ruleQualifiedName
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryAssignmentTableAccess().getEntityattributeEntityAttributeQualifiedNameParserRuleCall_7_0_1());
}
pushFollow(FollowSets003.FOLLOW_ruleQualifiedName_in_rule__DictionaryAssignmentTable__EntityattributeAssignment_780905);
ruleQualifiedName();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryAssignmentTableAccess().getEntityattributeEntityAttributeQualifiedNameParserRuleCall_7_0_1());
}
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryAssignmentTableAccess().getEntityattributeEntityAttributeCrossReference_7_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryAssignmentTable__EntityattributeAssignment_7"
// $ANTLR start "rule__DictionaryAssignmentTable__DictionaryAssignment_9"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40285:1: rule__DictionaryAssignmentTable__DictionaryAssignment_9 : ( ( ruleQualifiedName ) ) ;
public final void rule__DictionaryAssignmentTable__DictionaryAssignment_9() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40289:1: ( ( ( ruleQualifiedName ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40290:1: ( ( ruleQualifiedName ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40290:1: ( ( ruleQualifiedName ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40291:1: ( ruleQualifiedName )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryAssignmentTableAccess().getDictionaryDictionaryCrossReference_9_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40292:1: ( ruleQualifiedName )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40293:1: ruleQualifiedName
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryAssignmentTableAccess().getDictionaryDictionaryQualifiedNameParserRuleCall_9_0_1());
}
pushFollow(FollowSets003.FOLLOW_ruleQualifiedName_in_rule__DictionaryAssignmentTable__DictionaryAssignment_980944);
ruleQualifiedName();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryAssignmentTableAccess().getDictionaryDictionaryQualifiedNameParserRuleCall_9_0_1());
}
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryAssignmentTableAccess().getDictionaryDictionaryCrossReference_9_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryAssignmentTable__DictionaryAssignment_9"
// $ANTLR start "rule__DictionaryAssignmentTable__ColumncontrolsAssignment_12"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40304:1: rule__DictionaryAssignmentTable__ColumncontrolsAssignment_12 : ( ruleDictionaryControl ) ;
public final void rule__DictionaryAssignmentTable__ColumncontrolsAssignment_12() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40308:1: ( ( ruleDictionaryControl ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40309:1: ( ruleDictionaryControl )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40309:1: ( ruleDictionaryControl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40310:1: ruleDictionaryControl
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryAssignmentTableAccess().getColumncontrolsDictionaryControlParserRuleCall_12_0());
}
pushFollow(FollowSets003.FOLLOW_ruleDictionaryControl_in_rule__DictionaryAssignmentTable__ColumncontrolsAssignment_1280979);
ruleDictionaryControl();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryAssignmentTableAccess().getColumncontrolsDictionaryControlParserRuleCall_12_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryAssignmentTable__ColumncontrolsAssignment_12"
// $ANTLR start "rule__Labels__LabelAssignment_1_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40319:1: rule__Labels__LabelAssignment_1_1 : ( RULE_STRING ) ;
public final void rule__Labels__LabelAssignment_1_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40323:1: ( ( RULE_STRING ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40324:1: ( RULE_STRING )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40324:1: ( RULE_STRING )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40325:1: RULE_STRING
{
if ( state.backtracking==0 ) {
before(grammarAccess.getLabelsAccess().getLabelSTRINGTerminalRuleCall_1_1_0());
}
match(input,RULE_STRING,FollowSets003.FOLLOW_RULE_STRING_in_rule__Labels__LabelAssignment_1_181010); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getLabelsAccess().getLabelSTRINGTerminalRuleCall_1_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Labels__LabelAssignment_1_1"
// $ANTLR start "rule__Labels__FilterLabelAssignment_2_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40334:1: rule__Labels__FilterLabelAssignment_2_1 : ( RULE_STRING ) ;
public final void rule__Labels__FilterLabelAssignment_2_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40338:1: ( ( RULE_STRING ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40339:1: ( RULE_STRING )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40339:1: ( RULE_STRING )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40340:1: RULE_STRING
{
if ( state.backtracking==0 ) {
before(grammarAccess.getLabelsAccess().getFilterLabelSTRINGTerminalRuleCall_2_1_0());
}
match(input,RULE_STRING,FollowSets003.FOLLOW_RULE_STRING_in_rule__Labels__FilterLabelAssignment_2_181041); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getLabelsAccess().getFilterLabelSTRINGTerminalRuleCall_2_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Labels__FilterLabelAssignment_2_1"
// $ANTLR start "rule__Labels__ColumnLabelAssignment_3_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40349:1: rule__Labels__ColumnLabelAssignment_3_1 : ( RULE_STRING ) ;
public final void rule__Labels__ColumnLabelAssignment_3_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40353:1: ( ( RULE_STRING ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40354:1: ( RULE_STRING )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40354:1: ( RULE_STRING )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40355:1: RULE_STRING
{
if ( state.backtracking==0 ) {
before(grammarAccess.getLabelsAccess().getColumnLabelSTRINGTerminalRuleCall_3_1_0());
}
match(input,RULE_STRING,FollowSets003.FOLLOW_RULE_STRING_in_rule__Labels__ColumnLabelAssignment_3_181072); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getLabelsAccess().getColumnLabelSTRINGTerminalRuleCall_3_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Labels__ColumnLabelAssignment_3_1"
// $ANTLR start "rule__Labels__EditorLabelAssignment_4_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40364:1: rule__Labels__EditorLabelAssignment_4_1 : ( RULE_STRING ) ;
public final void rule__Labels__EditorLabelAssignment_4_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40368:1: ( ( RULE_STRING ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40369:1: ( RULE_STRING )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40369:1: ( RULE_STRING )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40370:1: RULE_STRING
{
if ( state.backtracking==0 ) {
before(grammarAccess.getLabelsAccess().getEditorLabelSTRINGTerminalRuleCall_4_1_0());
}
match(input,RULE_STRING,FollowSets003.FOLLOW_RULE_STRING_in_rule__Labels__EditorLabelAssignment_4_181103); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getLabelsAccess().getEditorLabelSTRINGTerminalRuleCall_4_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Labels__EditorLabelAssignment_4_1"
// $ANTLR start "rule__Labels__ToolTipAssignment_5_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40379:1: rule__Labels__ToolTipAssignment_5_1 : ( RULE_STRING ) ;
public final void rule__Labels__ToolTipAssignment_5_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40383:1: ( ( RULE_STRING ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40384:1: ( RULE_STRING )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40384:1: ( RULE_STRING )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40385:1: RULE_STRING
{
if ( state.backtracking==0 ) {
before(grammarAccess.getLabelsAccess().getToolTipSTRINGTerminalRuleCall_5_1_0());
}
match(input,RULE_STRING,FollowSets003.FOLLOW_RULE_STRING_in_rule__Labels__ToolTipAssignment_5_181134); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getLabelsAccess().getToolTipSTRINGTerminalRuleCall_5_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Labels__ToolTipAssignment_5_1"
// $ANTLR start "rule__BaseDictionaryControl__EntityattributeAssignment_0_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40394:1: rule__BaseDictionaryControl__EntityattributeAssignment_0_1 : ( ( ruleQualifiedName ) ) ;
public final void rule__BaseDictionaryControl__EntityattributeAssignment_0_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40398:1: ( ( ( ruleQualifiedName ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40399:1: ( ( ruleQualifiedName ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40399:1: ( ( ruleQualifiedName ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40400:1: ( ruleQualifiedName )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getBaseDictionaryControlAccess().getEntityattributeEntityAttributeCrossReference_0_1_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40401:1: ( ruleQualifiedName )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40402:1: ruleQualifiedName
{
if ( state.backtracking==0 ) {
before(grammarAccess.getBaseDictionaryControlAccess().getEntityattributeEntityAttributeQualifiedNameParserRuleCall_0_1_0_1());
}
pushFollow(FollowSets003.FOLLOW_ruleQualifiedName_in_rule__BaseDictionaryControl__EntityattributeAssignment_0_181169);
ruleQualifiedName();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getBaseDictionaryControlAccess().getEntityattributeEntityAttributeQualifiedNameParserRuleCall_0_1_0_1());
}
}
if ( state.backtracking==0 ) {
after(grammarAccess.getBaseDictionaryControlAccess().getEntityattributeEntityAttributeCrossReference_0_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__BaseDictionaryControl__EntityattributeAssignment_0_1"
// $ANTLR start "rule__BaseDictionaryControl__TypeAssignment_1_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40413:1: rule__BaseDictionaryControl__TypeAssignment_1_1 : ( ( ruleQualifiedName ) ) ;
public final void rule__BaseDictionaryControl__TypeAssignment_1_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40417:1: ( ( ( ruleQualifiedName ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40418:1: ( ( ruleQualifiedName ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40418:1: ( ( ruleQualifiedName ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40419:1: ( ruleQualifiedName )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getBaseDictionaryControlAccess().getTypeDatatypeCrossReference_1_1_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40420:1: ( ruleQualifiedName )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40421:1: ruleQualifiedName
{
if ( state.backtracking==0 ) {
before(grammarAccess.getBaseDictionaryControlAccess().getTypeDatatypeQualifiedNameParserRuleCall_1_1_0_1());
}
pushFollow(FollowSets003.FOLLOW_ruleQualifiedName_in_rule__BaseDictionaryControl__TypeAssignment_1_181208);
ruleQualifiedName();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getBaseDictionaryControlAccess().getTypeDatatypeQualifiedNameParserRuleCall_1_1_0_1());
}
}
if ( state.backtracking==0 ) {
after(grammarAccess.getBaseDictionaryControlAccess().getTypeDatatypeCrossReference_1_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__BaseDictionaryControl__TypeAssignment_1_1"
// $ANTLR start "rule__BaseDictionaryControl__LabelsAssignment_2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40432:1: rule__BaseDictionaryControl__LabelsAssignment_2 : ( ruleLabels ) ;
public final void rule__BaseDictionaryControl__LabelsAssignment_2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40436:1: ( ( ruleLabels ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40437:1: ( ruleLabels )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40437:1: ( ruleLabels )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40438:1: ruleLabels
{
if ( state.backtracking==0 ) {
before(grammarAccess.getBaseDictionaryControlAccess().getLabelsLabelsParserRuleCall_2_0());
}
pushFollow(FollowSets003.FOLLOW_ruleLabels_in_rule__BaseDictionaryControl__LabelsAssignment_281243);
ruleLabels();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getBaseDictionaryControlAccess().getLabelsLabelsParserRuleCall_2_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__BaseDictionaryControl__LabelsAssignment_2"
// $ANTLR start "rule__BaseDictionaryControl__MandatoryAssignment_3"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40447:1: rule__BaseDictionaryControl__MandatoryAssignment_3 : ( ( 'mandatory' ) ) ;
public final void rule__BaseDictionaryControl__MandatoryAssignment_3() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40451:1: ( ( ( 'mandatory' ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40452:1: ( ( 'mandatory' ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40452:1: ( ( 'mandatory' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40453:1: ( 'mandatory' )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getBaseDictionaryControlAccess().getMandatoryMandatoryKeyword_3_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40454:1: ( 'mandatory' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40455:1: 'mandatory'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getBaseDictionaryControlAccess().getMandatoryMandatoryKeyword_3_0());
}
match(input,179,FollowSets003.FOLLOW_179_in_rule__BaseDictionaryControl__MandatoryAssignment_381279); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getBaseDictionaryControlAccess().getMandatoryMandatoryKeyword_3_0());
}
}
if ( state.backtracking==0 ) {
after(grammarAccess.getBaseDictionaryControlAccess().getMandatoryMandatoryKeyword_3_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__BaseDictionaryControl__MandatoryAssignment_3"
// $ANTLR start "rule__BaseDictionaryControl__WidthAssignment_4_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40470:1: rule__BaseDictionaryControl__WidthAssignment_4_1 : ( RULE_INT ) ;
public final void rule__BaseDictionaryControl__WidthAssignment_4_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40474:1: ( ( RULE_INT ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40475:1: ( RULE_INT )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40475:1: ( RULE_INT )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40476:1: RULE_INT
{
if ( state.backtracking==0 ) {
before(grammarAccess.getBaseDictionaryControlAccess().getWidthINTTerminalRuleCall_4_1_0());
}
match(input,RULE_INT,FollowSets003.FOLLOW_RULE_INT_in_rule__BaseDictionaryControl__WidthAssignment_4_181318); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getBaseDictionaryControlAccess().getWidthINTTerminalRuleCall_4_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__BaseDictionaryControl__WidthAssignment_4_1"
// $ANTLR start "rule__BaseDictionaryControl__ReadonlyAssignment_5_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40485:1: rule__BaseDictionaryControl__ReadonlyAssignment_5_1 : ( ruleXBooleanLiteral ) ;
public final void rule__BaseDictionaryControl__ReadonlyAssignment_5_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40489:1: ( ( ruleXBooleanLiteral ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40490:1: ( ruleXBooleanLiteral )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40490:1: ( ruleXBooleanLiteral )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40491:1: ruleXBooleanLiteral
{
if ( state.backtracking==0 ) {
before(grammarAccess.getBaseDictionaryControlAccess().getReadonlyXBooleanLiteralParserRuleCall_5_1_0());
}
pushFollow(FollowSets003.FOLLOW_ruleXBooleanLiteral_in_rule__BaseDictionaryControl__ReadonlyAssignment_5_181349);
ruleXBooleanLiteral();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getBaseDictionaryControlAccess().getReadonlyXBooleanLiteralParserRuleCall_5_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__BaseDictionaryControl__ReadonlyAssignment_5_1"
// $ANTLR start "rule__DictionaryControlGroupOptionMultiFilterField__MultiFilterFieldAssignment_1_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40500:1: rule__DictionaryControlGroupOptionMultiFilterField__MultiFilterFieldAssignment_1_1 : ( ruleXBooleanLiteral ) ;
public final void rule__DictionaryControlGroupOptionMultiFilterField__MultiFilterFieldAssignment_1_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40504:1: ( ( ruleXBooleanLiteral ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40505:1: ( ruleXBooleanLiteral )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40505:1: ( ruleXBooleanLiteral )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40506:1: ruleXBooleanLiteral
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryControlGroupOptionMultiFilterFieldAccess().getMultiFilterFieldXBooleanLiteralParserRuleCall_1_1_0());
}
pushFollow(FollowSets003.FOLLOW_ruleXBooleanLiteral_in_rule__DictionaryControlGroupOptionMultiFilterField__MultiFilterFieldAssignment_1_181380);
ruleXBooleanLiteral();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryControlGroupOptionMultiFilterFieldAccess().getMultiFilterFieldXBooleanLiteralParserRuleCall_1_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryControlGroupOptionMultiFilterField__MultiFilterFieldAssignment_1_1"
// $ANTLR start "rule__DictionaryControlGroupOptionsContainer__OptionsAssignment_3"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40515:1: rule__DictionaryControlGroupOptionsContainer__OptionsAssignment_3 : ( ruleDictionaryControlGroupOptions ) ;
public final void rule__DictionaryControlGroupOptionsContainer__OptionsAssignment_3() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40519:1: ( ( ruleDictionaryControlGroupOptions ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40520:1: ( ruleDictionaryControlGroupOptions )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40520:1: ( ruleDictionaryControlGroupOptions )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40521:1: ruleDictionaryControlGroupOptions
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryControlGroupOptionsContainerAccess().getOptionsDictionaryControlGroupOptionsParserRuleCall_3_0());
}
pushFollow(FollowSets003.FOLLOW_ruleDictionaryControlGroupOptions_in_rule__DictionaryControlGroupOptionsContainer__OptionsAssignment_381411);
ruleDictionaryControlGroupOptions();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryControlGroupOptionsContainerAccess().getOptionsDictionaryControlGroupOptionsParserRuleCall_3_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryControlGroupOptionsContainer__OptionsAssignment_3"
// $ANTLR start "rule__DictionaryControlGroup__NameAssignment_2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40530:1: rule__DictionaryControlGroup__NameAssignment_2 : ( RULE_ID ) ;
public final void rule__DictionaryControlGroup__NameAssignment_2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40534:1: ( ( RULE_ID ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40535:1: ( RULE_ID )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40535:1: ( RULE_ID )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40536:1: RULE_ID
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryControlGroupAccess().getNameIDTerminalRuleCall_2_0());
}
match(input,RULE_ID,FollowSets003.FOLLOW_RULE_ID_in_rule__DictionaryControlGroup__NameAssignment_281442); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryControlGroupAccess().getNameIDTerminalRuleCall_2_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryControlGroup__NameAssignment_2"
// $ANTLR start "rule__DictionaryControlGroup__RefAssignment_3_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40545:1: rule__DictionaryControlGroup__RefAssignment_3_1 : ( ( ruleQualifiedName ) ) ;
public final void rule__DictionaryControlGroup__RefAssignment_3_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40549:1: ( ( ( ruleQualifiedName ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40550:1: ( ( ruleQualifiedName ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40550:1: ( ( ruleQualifiedName ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40551:1: ( ruleQualifiedName )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryControlGroupAccess().getRefDictionaryControlGroupCrossReference_3_1_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40552:1: ( ruleQualifiedName )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40553:1: ruleQualifiedName
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryControlGroupAccess().getRefDictionaryControlGroupQualifiedNameParserRuleCall_3_1_0_1());
}
pushFollow(FollowSets003.FOLLOW_ruleQualifiedName_in_rule__DictionaryControlGroup__RefAssignment_3_181477);
ruleQualifiedName();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryControlGroupAccess().getRefDictionaryControlGroupQualifiedNameParserRuleCall_3_1_0_1());
}
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryControlGroupAccess().getRefDictionaryControlGroupCrossReference_3_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryControlGroup__RefAssignment_3_1"
// $ANTLR start "rule__DictionaryControlGroup__ControlGroupOptionsAssignment_4_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40564:1: rule__DictionaryControlGroup__ControlGroupOptionsAssignment_4_1 : ( ruleDictionaryControlGroupOptionsContainer ) ;
public final void rule__DictionaryControlGroup__ControlGroupOptionsAssignment_4_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40568:1: ( ( ruleDictionaryControlGroupOptionsContainer ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40569:1: ( ruleDictionaryControlGroupOptionsContainer )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40569:1: ( ruleDictionaryControlGroupOptionsContainer )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40570:1: ruleDictionaryControlGroupOptionsContainer
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryControlGroupAccess().getControlGroupOptionsDictionaryControlGroupOptionsContainerParserRuleCall_4_1_0());
}
pushFollow(FollowSets003.FOLLOW_ruleDictionaryControlGroupOptionsContainer_in_rule__DictionaryControlGroup__ControlGroupOptionsAssignment_4_181512);
ruleDictionaryControlGroupOptionsContainer();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryControlGroupAccess().getControlGroupOptionsDictionaryControlGroupOptionsContainerParserRuleCall_4_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryControlGroup__ControlGroupOptionsAssignment_4_1"
// $ANTLR start "rule__DictionaryControlGroup__BaseControlAssignment_4_2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40579:1: rule__DictionaryControlGroup__BaseControlAssignment_4_2 : ( ruleBaseDictionaryControl ) ;
public final void rule__DictionaryControlGroup__BaseControlAssignment_4_2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40583:1: ( ( ruleBaseDictionaryControl ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40584:1: ( ruleBaseDictionaryControl )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40584:1: ( ruleBaseDictionaryControl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40585:1: ruleBaseDictionaryControl
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryControlGroupAccess().getBaseControlBaseDictionaryControlParserRuleCall_4_2_0());
}
pushFollow(FollowSets003.FOLLOW_ruleBaseDictionaryControl_in_rule__DictionaryControlGroup__BaseControlAssignment_4_281543);
ruleBaseDictionaryControl();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryControlGroupAccess().getBaseControlBaseDictionaryControlParserRuleCall_4_2_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryControlGroup__BaseControlAssignment_4_2"
// $ANTLR start "rule__DictionaryControlGroup__GroupcontrolsAssignment_4_3"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40594:1: rule__DictionaryControlGroup__GroupcontrolsAssignment_4_3 : ( ruleDictionaryControl ) ;
public final void rule__DictionaryControlGroup__GroupcontrolsAssignment_4_3() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40598:1: ( ( ruleDictionaryControl ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40599:1: ( ruleDictionaryControl )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40599:1: ( ruleDictionaryControl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40600:1: ruleDictionaryControl
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryControlGroupAccess().getGroupcontrolsDictionaryControlParserRuleCall_4_3_0());
}
pushFollow(FollowSets003.FOLLOW_ruleDictionaryControl_in_rule__DictionaryControlGroup__GroupcontrolsAssignment_4_381574);
ruleDictionaryControl();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryControlGroupAccess().getGroupcontrolsDictionaryControlParserRuleCall_4_3_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryControlGroup__GroupcontrolsAssignment_4_3"
// $ANTLR start "rule__DictionaryHierarchicalControl__NameAssignment_2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40609:1: rule__DictionaryHierarchicalControl__NameAssignment_2 : ( RULE_ID ) ;
public final void rule__DictionaryHierarchicalControl__NameAssignment_2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40613:1: ( ( RULE_ID ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40614:1: ( RULE_ID )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40614:1: ( RULE_ID )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40615:1: RULE_ID
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryHierarchicalControlAccess().getNameIDTerminalRuleCall_2_0());
}
match(input,RULE_ID,FollowSets003.FOLLOW_RULE_ID_in_rule__DictionaryHierarchicalControl__NameAssignment_281605); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryHierarchicalControlAccess().getNameIDTerminalRuleCall_2_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryHierarchicalControl__NameAssignment_2"
// $ANTLR start "rule__DictionaryHierarchicalControl__RefAssignment_3_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40624:1: rule__DictionaryHierarchicalControl__RefAssignment_3_1 : ( ( ruleQualifiedName ) ) ;
public final void rule__DictionaryHierarchicalControl__RefAssignment_3_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40628:1: ( ( ( ruleQualifiedName ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40629:1: ( ( ruleQualifiedName ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40629:1: ( ( ruleQualifiedName ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40630:1: ( ruleQualifiedName )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryHierarchicalControlAccess().getRefDictionaryHierarchicalControlCrossReference_3_1_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40631:1: ( ruleQualifiedName )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40632:1: ruleQualifiedName
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryHierarchicalControlAccess().getRefDictionaryHierarchicalControlQualifiedNameParserRuleCall_3_1_0_1());
}
pushFollow(FollowSets003.FOLLOW_ruleQualifiedName_in_rule__DictionaryHierarchicalControl__RefAssignment_3_181640);
ruleQualifiedName();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryHierarchicalControlAccess().getRefDictionaryHierarchicalControlQualifiedNameParserRuleCall_3_1_0_1());
}
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryHierarchicalControlAccess().getRefDictionaryHierarchicalControlCrossReference_3_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryHierarchicalControl__RefAssignment_3_1"
// $ANTLR start "rule__DictionaryHierarchicalControl__BaseControlAssignment_4_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40643:1: rule__DictionaryHierarchicalControl__BaseControlAssignment_4_1 : ( ruleBaseDictionaryControl ) ;
public final void rule__DictionaryHierarchicalControl__BaseControlAssignment_4_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40647:1: ( ( ruleBaseDictionaryControl ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40648:1: ( ruleBaseDictionaryControl )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40648:1: ( ruleBaseDictionaryControl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40649:1: ruleBaseDictionaryControl
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryHierarchicalControlAccess().getBaseControlBaseDictionaryControlParserRuleCall_4_1_0());
}
pushFollow(FollowSets003.FOLLOW_ruleBaseDictionaryControl_in_rule__DictionaryHierarchicalControl__BaseControlAssignment_4_181675);
ruleBaseDictionaryControl();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryHierarchicalControlAccess().getBaseControlBaseDictionaryControlParserRuleCall_4_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryHierarchicalControl__BaseControlAssignment_4_1"
// $ANTLR start "rule__DictionaryHierarchicalControl__HierarchicalIdAssignment_4_3"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40658:1: rule__DictionaryHierarchicalControl__HierarchicalIdAssignment_4_3 : ( RULE_STRING ) ;
public final void rule__DictionaryHierarchicalControl__HierarchicalIdAssignment_4_3() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40662:1: ( ( RULE_STRING ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40663:1: ( RULE_STRING )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40663:1: ( RULE_STRING )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40664:1: RULE_STRING
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryHierarchicalControlAccess().getHierarchicalIdSTRINGTerminalRuleCall_4_3_0());
}
match(input,RULE_STRING,FollowSets003.FOLLOW_RULE_STRING_in_rule__DictionaryHierarchicalControl__HierarchicalIdAssignment_4_381706); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryHierarchicalControlAccess().getHierarchicalIdSTRINGTerminalRuleCall_4_3_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryHierarchicalControl__HierarchicalIdAssignment_4_3"
// $ANTLR start "rule__DictionaryTextControl__NameAssignment_2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40673:1: rule__DictionaryTextControl__NameAssignment_2 : ( RULE_ID ) ;
public final void rule__DictionaryTextControl__NameAssignment_2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40677:1: ( ( RULE_ID ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40678:1: ( RULE_ID )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40678:1: ( RULE_ID )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40679:1: RULE_ID
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryTextControlAccess().getNameIDTerminalRuleCall_2_0());
}
match(input,RULE_ID,FollowSets003.FOLLOW_RULE_ID_in_rule__DictionaryTextControl__NameAssignment_281737); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryTextControlAccess().getNameIDTerminalRuleCall_2_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryTextControl__NameAssignment_2"
// $ANTLR start "rule__DictionaryTextControl__RefAssignment_3_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40688:1: rule__DictionaryTextControl__RefAssignment_3_1 : ( ( ruleQualifiedName ) ) ;
public final void rule__DictionaryTextControl__RefAssignment_3_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40692:1: ( ( ( ruleQualifiedName ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40693:1: ( ( ruleQualifiedName ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40693:1: ( ( ruleQualifiedName ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40694:1: ( ruleQualifiedName )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryTextControlAccess().getRefDictionaryTextControlCrossReference_3_1_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40695:1: ( ruleQualifiedName )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40696:1: ruleQualifiedName
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryTextControlAccess().getRefDictionaryTextControlQualifiedNameParserRuleCall_3_1_0_1());
}
pushFollow(FollowSets003.FOLLOW_ruleQualifiedName_in_rule__DictionaryTextControl__RefAssignment_3_181772);
ruleQualifiedName();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryTextControlAccess().getRefDictionaryTextControlQualifiedNameParserRuleCall_3_1_0_1());
}
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryTextControlAccess().getRefDictionaryTextControlCrossReference_3_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryTextControl__RefAssignment_3_1"
// $ANTLR start "rule__DictionaryTextControl__BaseControlAssignment_4_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40707:1: rule__DictionaryTextControl__BaseControlAssignment_4_1 : ( ruleBaseDictionaryControl ) ;
public final void rule__DictionaryTextControl__BaseControlAssignment_4_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40711:1: ( ( ruleBaseDictionaryControl ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40712:1: ( ruleBaseDictionaryControl )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40712:1: ( ruleBaseDictionaryControl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40713:1: ruleBaseDictionaryControl
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryTextControlAccess().getBaseControlBaseDictionaryControlParserRuleCall_4_1_0());
}
pushFollow(FollowSets003.FOLLOW_ruleBaseDictionaryControl_in_rule__DictionaryTextControl__BaseControlAssignment_4_181807);
ruleBaseDictionaryControl();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryTextControlAccess().getBaseControlBaseDictionaryControlParserRuleCall_4_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryTextControl__BaseControlAssignment_4_1"
// $ANTLR start "rule__DictionaryIntegerControlInputType__InputtypeAssignment_2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40722:1: rule__DictionaryIntegerControlInputType__InputtypeAssignment_2 : ( ruleIntegerControlInputType ) ;
public final void rule__DictionaryIntegerControlInputType__InputtypeAssignment_2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40726:1: ( ( ruleIntegerControlInputType ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40727:1: ( ruleIntegerControlInputType )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40727:1: ( ruleIntegerControlInputType )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40728:1: ruleIntegerControlInputType
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryIntegerControlInputTypeAccess().getInputtypeIntegerControlInputTypeEnumRuleCall_2_0());
}
pushFollow(FollowSets003.FOLLOW_ruleIntegerControlInputType_in_rule__DictionaryIntegerControlInputType__InputtypeAssignment_281838);
ruleIntegerControlInputType();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryIntegerControlInputTypeAccess().getInputtypeIntegerControlInputTypeEnumRuleCall_2_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryIntegerControlInputType__InputtypeAssignment_2"
// $ANTLR start "rule__DictionaryIntegerControl__NameAssignment_2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40737:1: rule__DictionaryIntegerControl__NameAssignment_2 : ( RULE_ID ) ;
public final void rule__DictionaryIntegerControl__NameAssignment_2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40741:1: ( ( RULE_ID ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40742:1: ( RULE_ID )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40742:1: ( RULE_ID )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40743:1: RULE_ID
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryIntegerControlAccess().getNameIDTerminalRuleCall_2_0());
}
match(input,RULE_ID,FollowSets003.FOLLOW_RULE_ID_in_rule__DictionaryIntegerControl__NameAssignment_281869); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryIntegerControlAccess().getNameIDTerminalRuleCall_2_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryIntegerControl__NameAssignment_2"
// $ANTLR start "rule__DictionaryIntegerControl__RefAssignment_3_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40752:1: rule__DictionaryIntegerControl__RefAssignment_3_1 : ( ( ruleQualifiedName ) ) ;
public final void rule__DictionaryIntegerControl__RefAssignment_3_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40756:1: ( ( ( ruleQualifiedName ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40757:1: ( ( ruleQualifiedName ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40757:1: ( ( ruleQualifiedName ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40758:1: ( ruleQualifiedName )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryIntegerControlAccess().getRefDictionaryIntegerControlCrossReference_3_1_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40759:1: ( ruleQualifiedName )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40760:1: ruleQualifiedName
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryIntegerControlAccess().getRefDictionaryIntegerControlQualifiedNameParserRuleCall_3_1_0_1());
}
pushFollow(FollowSets003.FOLLOW_ruleQualifiedName_in_rule__DictionaryIntegerControl__RefAssignment_3_181904);
ruleQualifiedName();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryIntegerControlAccess().getRefDictionaryIntegerControlQualifiedNameParserRuleCall_3_1_0_1());
}
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryIntegerControlAccess().getRefDictionaryIntegerControlCrossReference_3_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryIntegerControl__RefAssignment_3_1"
// $ANTLR start "rule__DictionaryIntegerControl__BaseControlAssignment_4_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40771:1: rule__DictionaryIntegerControl__BaseControlAssignment_4_1 : ( ruleBaseDictionaryControl ) ;
public final void rule__DictionaryIntegerControl__BaseControlAssignment_4_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40775:1: ( ( ruleBaseDictionaryControl ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40776:1: ( ruleBaseDictionaryControl )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40776:1: ( ruleBaseDictionaryControl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40777:1: ruleBaseDictionaryControl
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryIntegerControlAccess().getBaseControlBaseDictionaryControlParserRuleCall_4_1_0());
}
pushFollow(FollowSets003.FOLLOW_ruleBaseDictionaryControl_in_rule__DictionaryIntegerControl__BaseControlAssignment_4_181939);
ruleBaseDictionaryControl();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryIntegerControlAccess().getBaseControlBaseDictionaryControlParserRuleCall_4_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryIntegerControl__BaseControlAssignment_4_1"
// $ANTLR start "rule__DictionaryIntegerControl__OptionsAssignment_4_2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40786:1: rule__DictionaryIntegerControl__OptionsAssignment_4_2 : ( ruleDictionaryIntegerControlOptions ) ;
public final void rule__DictionaryIntegerControl__OptionsAssignment_4_2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40790:1: ( ( ruleDictionaryIntegerControlOptions ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40791:1: ( ruleDictionaryIntegerControlOptions )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40791:1: ( ruleDictionaryIntegerControlOptions )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40792:1: ruleDictionaryIntegerControlOptions
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryIntegerControlAccess().getOptionsDictionaryIntegerControlOptionsParserRuleCall_4_2_0());
}
pushFollow(FollowSets003.FOLLOW_ruleDictionaryIntegerControlOptions_in_rule__DictionaryIntegerControl__OptionsAssignment_4_281970);
ruleDictionaryIntegerControlOptions();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryIntegerControlAccess().getOptionsDictionaryIntegerControlOptionsParserRuleCall_4_2_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryIntegerControl__OptionsAssignment_4_2"
// $ANTLR start "rule__DictionaryBigDecimalControl__NameAssignment_2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40801:1: rule__DictionaryBigDecimalControl__NameAssignment_2 : ( RULE_ID ) ;
public final void rule__DictionaryBigDecimalControl__NameAssignment_2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40805:1: ( ( RULE_ID ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40806:1: ( RULE_ID )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40806:1: ( RULE_ID )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40807:1: RULE_ID
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryBigDecimalControlAccess().getNameIDTerminalRuleCall_2_0());
}
match(input,RULE_ID,FollowSets003.FOLLOW_RULE_ID_in_rule__DictionaryBigDecimalControl__NameAssignment_282001); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryBigDecimalControlAccess().getNameIDTerminalRuleCall_2_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryBigDecimalControl__NameAssignment_2"
// $ANTLR start "rule__DictionaryBigDecimalControl__RefAssignment_3_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40816:1: rule__DictionaryBigDecimalControl__RefAssignment_3_1 : ( ( ruleQualifiedName ) ) ;
public final void rule__DictionaryBigDecimalControl__RefAssignment_3_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40820:1: ( ( ( ruleQualifiedName ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40821:1: ( ( ruleQualifiedName ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40821:1: ( ( ruleQualifiedName ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40822:1: ( ruleQualifiedName )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryBigDecimalControlAccess().getRefDictionaryBigDecimalControlCrossReference_3_1_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40823:1: ( ruleQualifiedName )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40824:1: ruleQualifiedName
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryBigDecimalControlAccess().getRefDictionaryBigDecimalControlQualifiedNameParserRuleCall_3_1_0_1());
}
pushFollow(FollowSets003.FOLLOW_ruleQualifiedName_in_rule__DictionaryBigDecimalControl__RefAssignment_3_182036);
ruleQualifiedName();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryBigDecimalControlAccess().getRefDictionaryBigDecimalControlQualifiedNameParserRuleCall_3_1_0_1());
}
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryBigDecimalControlAccess().getRefDictionaryBigDecimalControlCrossReference_3_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryBigDecimalControl__RefAssignment_3_1"
// $ANTLR start "rule__DictionaryBigDecimalControl__BaseControlAssignment_4_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40835:1: rule__DictionaryBigDecimalControl__BaseControlAssignment_4_1 : ( ruleBaseDictionaryControl ) ;
public final void rule__DictionaryBigDecimalControl__BaseControlAssignment_4_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40839:1: ( ( ruleBaseDictionaryControl ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40840:1: ( ruleBaseDictionaryControl )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40840:1: ( ruleBaseDictionaryControl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40841:1: ruleBaseDictionaryControl
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryBigDecimalControlAccess().getBaseControlBaseDictionaryControlParserRuleCall_4_1_0());
}
pushFollow(FollowSets003.FOLLOW_ruleBaseDictionaryControl_in_rule__DictionaryBigDecimalControl__BaseControlAssignment_4_182071);
ruleBaseDictionaryControl();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryBigDecimalControlAccess().getBaseControlBaseDictionaryControlParserRuleCall_4_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryBigDecimalControl__BaseControlAssignment_4_1"
// $ANTLR start "rule__DictionaryBooleanControl__NameAssignment_2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40850:1: rule__DictionaryBooleanControl__NameAssignment_2 : ( RULE_ID ) ;
public final void rule__DictionaryBooleanControl__NameAssignment_2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40854:1: ( ( RULE_ID ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40855:1: ( RULE_ID )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40855:1: ( RULE_ID )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40856:1: RULE_ID
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryBooleanControlAccess().getNameIDTerminalRuleCall_2_0());
}
match(input,RULE_ID,FollowSets003.FOLLOW_RULE_ID_in_rule__DictionaryBooleanControl__NameAssignment_282102); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryBooleanControlAccess().getNameIDTerminalRuleCall_2_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryBooleanControl__NameAssignment_2"
// $ANTLR start "rule__DictionaryBooleanControl__RefAssignment_3_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40865:1: rule__DictionaryBooleanControl__RefAssignment_3_1 : ( ( ruleQualifiedName ) ) ;
public final void rule__DictionaryBooleanControl__RefAssignment_3_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40869:1: ( ( ( ruleQualifiedName ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40870:1: ( ( ruleQualifiedName ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40870:1: ( ( ruleQualifiedName ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40871:1: ( ruleQualifiedName )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryBooleanControlAccess().getRefDictionaryBooleanControlCrossReference_3_1_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40872:1: ( ruleQualifiedName )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40873:1: ruleQualifiedName
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryBooleanControlAccess().getRefDictionaryBooleanControlQualifiedNameParserRuleCall_3_1_0_1());
}
pushFollow(FollowSets003.FOLLOW_ruleQualifiedName_in_rule__DictionaryBooleanControl__RefAssignment_3_182137);
ruleQualifiedName();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryBooleanControlAccess().getRefDictionaryBooleanControlQualifiedNameParserRuleCall_3_1_0_1());
}
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryBooleanControlAccess().getRefDictionaryBooleanControlCrossReference_3_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryBooleanControl__RefAssignment_3_1"
// $ANTLR start "rule__DictionaryBooleanControl__BaseControlAssignment_4_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40884:1: rule__DictionaryBooleanControl__BaseControlAssignment_4_1 : ( ruleBaseDictionaryControl ) ;
public final void rule__DictionaryBooleanControl__BaseControlAssignment_4_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40888:1: ( ( ruleBaseDictionaryControl ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40889:1: ( ruleBaseDictionaryControl )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40889:1: ( ruleBaseDictionaryControl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40890:1: ruleBaseDictionaryControl
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryBooleanControlAccess().getBaseControlBaseDictionaryControlParserRuleCall_4_1_0());
}
pushFollow(FollowSets003.FOLLOW_ruleBaseDictionaryControl_in_rule__DictionaryBooleanControl__BaseControlAssignment_4_182172);
ruleBaseDictionaryControl();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryBooleanControlAccess().getBaseControlBaseDictionaryControlParserRuleCall_4_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryBooleanControl__BaseControlAssignment_4_1"
// $ANTLR start "rule__DictionaryDateControl__NameAssignment_2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40899:1: rule__DictionaryDateControl__NameAssignment_2 : ( RULE_ID ) ;
public final void rule__DictionaryDateControl__NameAssignment_2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40903:1: ( ( RULE_ID ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40904:1: ( RULE_ID )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40904:1: ( RULE_ID )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40905:1: RULE_ID
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryDateControlAccess().getNameIDTerminalRuleCall_2_0());
}
match(input,RULE_ID,FollowSets003.FOLLOW_RULE_ID_in_rule__DictionaryDateControl__NameAssignment_282203); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryDateControlAccess().getNameIDTerminalRuleCall_2_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryDateControl__NameAssignment_2"
// $ANTLR start "rule__DictionaryDateControl__RefAssignment_3_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40914:1: rule__DictionaryDateControl__RefAssignment_3_1 : ( ( ruleQualifiedName ) ) ;
public final void rule__DictionaryDateControl__RefAssignment_3_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40918:1: ( ( ( ruleQualifiedName ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40919:1: ( ( ruleQualifiedName ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40919:1: ( ( ruleQualifiedName ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40920:1: ( ruleQualifiedName )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryDateControlAccess().getRefDictionaryDateControlCrossReference_3_1_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40921:1: ( ruleQualifiedName )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40922:1: ruleQualifiedName
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryDateControlAccess().getRefDictionaryDateControlQualifiedNameParserRuleCall_3_1_0_1());
}
pushFollow(FollowSets003.FOLLOW_ruleQualifiedName_in_rule__DictionaryDateControl__RefAssignment_3_182238);
ruleQualifiedName();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryDateControlAccess().getRefDictionaryDateControlQualifiedNameParserRuleCall_3_1_0_1());
}
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryDateControlAccess().getRefDictionaryDateControlCrossReference_3_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryDateControl__RefAssignment_3_1"
// $ANTLR start "rule__DictionaryDateControl__BaseControlAssignment_4_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40933:1: rule__DictionaryDateControl__BaseControlAssignment_4_1 : ( ruleBaseDictionaryControl ) ;
public final void rule__DictionaryDateControl__BaseControlAssignment_4_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40937:1: ( ( ruleBaseDictionaryControl ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40938:1: ( ruleBaseDictionaryControl )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40938:1: ( ruleBaseDictionaryControl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40939:1: ruleBaseDictionaryControl
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryDateControlAccess().getBaseControlBaseDictionaryControlParserRuleCall_4_1_0());
}
pushFollow(FollowSets003.FOLLOW_ruleBaseDictionaryControl_in_rule__DictionaryDateControl__BaseControlAssignment_4_182273);
ruleBaseDictionaryControl();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryDateControlAccess().getBaseControlBaseDictionaryControlParserRuleCall_4_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryDateControl__BaseControlAssignment_4_1"
// $ANTLR start "rule__DictionaryEnumerationControl__NameAssignment_2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40948:1: rule__DictionaryEnumerationControl__NameAssignment_2 : ( RULE_ID ) ;
public final void rule__DictionaryEnumerationControl__NameAssignment_2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40952:1: ( ( RULE_ID ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40953:1: ( RULE_ID )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40953:1: ( RULE_ID )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40954:1: RULE_ID
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryEnumerationControlAccess().getNameIDTerminalRuleCall_2_0());
}
match(input,RULE_ID,FollowSets003.FOLLOW_RULE_ID_in_rule__DictionaryEnumerationControl__NameAssignment_282304); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryEnumerationControlAccess().getNameIDTerminalRuleCall_2_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryEnumerationControl__NameAssignment_2"
// $ANTLR start "rule__DictionaryEnumerationControl__RefAssignment_3_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40963:1: rule__DictionaryEnumerationControl__RefAssignment_3_1 : ( ( ruleQualifiedName ) ) ;
public final void rule__DictionaryEnumerationControl__RefAssignment_3_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40967:1: ( ( ( ruleQualifiedName ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40968:1: ( ( ruleQualifiedName ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40968:1: ( ( ruleQualifiedName ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40969:1: ( ruleQualifiedName )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryEnumerationControlAccess().getRefDictionaryEnumerationControlCrossReference_3_1_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40970:1: ( ruleQualifiedName )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40971:1: ruleQualifiedName
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryEnumerationControlAccess().getRefDictionaryEnumerationControlQualifiedNameParserRuleCall_3_1_0_1());
}
pushFollow(FollowSets003.FOLLOW_ruleQualifiedName_in_rule__DictionaryEnumerationControl__RefAssignment_3_182339);
ruleQualifiedName();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryEnumerationControlAccess().getRefDictionaryEnumerationControlQualifiedNameParserRuleCall_3_1_0_1());
}
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryEnumerationControlAccess().getRefDictionaryEnumerationControlCrossReference_3_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryEnumerationControl__RefAssignment_3_1"
// $ANTLR start "rule__DictionaryEnumerationControl__BaseControlAssignment_4_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40982:1: rule__DictionaryEnumerationControl__BaseControlAssignment_4_1 : ( ruleBaseDictionaryControl ) ;
public final void rule__DictionaryEnumerationControl__BaseControlAssignment_4_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40986:1: ( ( ruleBaseDictionaryControl ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40987:1: ( ruleBaseDictionaryControl )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40987:1: ( ruleBaseDictionaryControl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40988:1: ruleBaseDictionaryControl
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryEnumerationControlAccess().getBaseControlBaseDictionaryControlParserRuleCall_4_1_0());
}
pushFollow(FollowSets003.FOLLOW_ruleBaseDictionaryControl_in_rule__DictionaryEnumerationControl__BaseControlAssignment_4_182374);
ruleBaseDictionaryControl();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryEnumerationControlAccess().getBaseControlBaseDictionaryControlParserRuleCall_4_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryEnumerationControl__BaseControlAssignment_4_1"
// $ANTLR start "rule__DictionaryReferenceControl__NameAssignment_2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:40997:1: rule__DictionaryReferenceControl__NameAssignment_2 : ( RULE_ID ) ;
public final void rule__DictionaryReferenceControl__NameAssignment_2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41001:1: ( ( RULE_ID ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41002:1: ( RULE_ID )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41002:1: ( RULE_ID )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41003:1: RULE_ID
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryReferenceControlAccess().getNameIDTerminalRuleCall_2_0());
}
match(input,RULE_ID,FollowSets003.FOLLOW_RULE_ID_in_rule__DictionaryReferenceControl__NameAssignment_282405); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryReferenceControlAccess().getNameIDTerminalRuleCall_2_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryReferenceControl__NameAssignment_2"
// $ANTLR start "rule__DictionaryReferenceControl__RefAssignment_3_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41012:1: rule__DictionaryReferenceControl__RefAssignment_3_1 : ( ( ruleQualifiedName ) ) ;
public final void rule__DictionaryReferenceControl__RefAssignment_3_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41016:1: ( ( ( ruleQualifiedName ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41017:1: ( ( ruleQualifiedName ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41017:1: ( ( ruleQualifiedName ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41018:1: ( ruleQualifiedName )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryReferenceControlAccess().getRefDictionaryReferenceControlCrossReference_3_1_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41019:1: ( ruleQualifiedName )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41020:1: ruleQualifiedName
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryReferenceControlAccess().getRefDictionaryReferenceControlQualifiedNameParserRuleCall_3_1_0_1());
}
pushFollow(FollowSets003.FOLLOW_ruleQualifiedName_in_rule__DictionaryReferenceControl__RefAssignment_3_182440);
ruleQualifiedName();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryReferenceControlAccess().getRefDictionaryReferenceControlQualifiedNameParserRuleCall_3_1_0_1());
}
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryReferenceControlAccess().getRefDictionaryReferenceControlCrossReference_3_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryReferenceControl__RefAssignment_3_1"
// $ANTLR start "rule__DictionaryReferenceControl__BaseControlAssignment_4_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41031:1: rule__DictionaryReferenceControl__BaseControlAssignment_4_1 : ( ruleBaseDictionaryControl ) ;
public final void rule__DictionaryReferenceControl__BaseControlAssignment_4_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41035:1: ( ( ruleBaseDictionaryControl ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41036:1: ( ruleBaseDictionaryControl )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41036:1: ( ruleBaseDictionaryControl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41037:1: ruleBaseDictionaryControl
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryReferenceControlAccess().getBaseControlBaseDictionaryControlParserRuleCall_4_1_0());
}
pushFollow(FollowSets003.FOLLOW_ruleBaseDictionaryControl_in_rule__DictionaryReferenceControl__BaseControlAssignment_4_182475);
ruleBaseDictionaryControl();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryReferenceControlAccess().getBaseControlBaseDictionaryControlParserRuleCall_4_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryReferenceControl__BaseControlAssignment_4_1"
// $ANTLR start "rule__DictionaryReferenceControl__DictionaryAssignment_4_2_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41046:1: rule__DictionaryReferenceControl__DictionaryAssignment_4_2_1 : ( ( ruleQualifiedName ) ) ;
public final void rule__DictionaryReferenceControl__DictionaryAssignment_4_2_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41050:1: ( ( ( ruleQualifiedName ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41051:1: ( ( ruleQualifiedName ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41051:1: ( ( ruleQualifiedName ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41052:1: ( ruleQualifiedName )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryReferenceControlAccess().getDictionaryDictionaryCrossReference_4_2_1_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41053:1: ( ruleQualifiedName )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41054:1: ruleQualifiedName
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryReferenceControlAccess().getDictionaryDictionaryQualifiedNameParserRuleCall_4_2_1_0_1());
}
pushFollow(FollowSets003.FOLLOW_ruleQualifiedName_in_rule__DictionaryReferenceControl__DictionaryAssignment_4_2_182510);
ruleQualifiedName();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryReferenceControlAccess().getDictionaryDictionaryQualifiedNameParserRuleCall_4_2_1_0_1());
}
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryReferenceControlAccess().getDictionaryDictionaryCrossReference_4_2_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryReferenceControl__DictionaryAssignment_4_2_1"
// $ANTLR start "rule__DictionaryReferenceControl__ControlTypeAssignment_4_3_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41065:1: rule__DictionaryReferenceControl__ControlTypeAssignment_4_3_1 : ( ruleReferenceControlType ) ;
public final void rule__DictionaryReferenceControl__ControlTypeAssignment_4_3_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41069:1: ( ( ruleReferenceControlType ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41070:1: ( ruleReferenceControlType )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41070:1: ( ruleReferenceControlType )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41071:1: ruleReferenceControlType
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryReferenceControlAccess().getControlTypeReferenceControlTypeEnumRuleCall_4_3_1_0());
}
pushFollow(FollowSets003.FOLLOW_ruleReferenceControlType_in_rule__DictionaryReferenceControl__ControlTypeAssignment_4_3_182545);
ruleReferenceControlType();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryReferenceControlAccess().getControlTypeReferenceControlTypeEnumRuleCall_4_3_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryReferenceControl__ControlTypeAssignment_4_3_1"
// $ANTLR start "rule__DictionaryReferenceControl__LabelcontrolsAssignment_4_4_2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41080:1: rule__DictionaryReferenceControl__LabelcontrolsAssignment_4_4_2 : ( ruleDictionaryControl ) ;
public final void rule__DictionaryReferenceControl__LabelcontrolsAssignment_4_4_2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41084:1: ( ( ruleDictionaryControl ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41085:1: ( ruleDictionaryControl )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41085:1: ( ruleDictionaryControl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41086:1: ruleDictionaryControl
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryReferenceControlAccess().getLabelcontrolsDictionaryControlParserRuleCall_4_4_2_0());
}
pushFollow(FollowSets003.FOLLOW_ruleDictionaryControl_in_rule__DictionaryReferenceControl__LabelcontrolsAssignment_4_4_282576);
ruleDictionaryControl();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryReferenceControlAccess().getLabelcontrolsDictionaryControlParserRuleCall_4_4_2_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryReferenceControl__LabelcontrolsAssignment_4_4_2"
// $ANTLR start "rule__DictionaryFileControl__NameAssignment_2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41095:1: rule__DictionaryFileControl__NameAssignment_2 : ( RULE_ID ) ;
public final void rule__DictionaryFileControl__NameAssignment_2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41099:1: ( ( RULE_ID ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41100:1: ( RULE_ID )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41100:1: ( RULE_ID )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41101:1: RULE_ID
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryFileControlAccess().getNameIDTerminalRuleCall_2_0());
}
match(input,RULE_ID,FollowSets003.FOLLOW_RULE_ID_in_rule__DictionaryFileControl__NameAssignment_282607); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryFileControlAccess().getNameIDTerminalRuleCall_2_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryFileControl__NameAssignment_2"
// $ANTLR start "rule__DictionaryFileControl__RefAssignment_3_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41110:1: rule__DictionaryFileControl__RefAssignment_3_1 : ( ( ruleQualifiedName ) ) ;
public final void rule__DictionaryFileControl__RefAssignment_3_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41114:1: ( ( ( ruleQualifiedName ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41115:1: ( ( ruleQualifiedName ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41115:1: ( ( ruleQualifiedName ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41116:1: ( ruleQualifiedName )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryFileControlAccess().getRefDictionaryFileControlCrossReference_3_1_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41117:1: ( ruleQualifiedName )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41118:1: ruleQualifiedName
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryFileControlAccess().getRefDictionaryFileControlQualifiedNameParserRuleCall_3_1_0_1());
}
pushFollow(FollowSets003.FOLLOW_ruleQualifiedName_in_rule__DictionaryFileControl__RefAssignment_3_182642);
ruleQualifiedName();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryFileControlAccess().getRefDictionaryFileControlQualifiedNameParserRuleCall_3_1_0_1());
}
}
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryFileControlAccess().getRefDictionaryFileControlCrossReference_3_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryFileControl__RefAssignment_3_1"
// $ANTLR start "rule__DictionaryFileControl__BaseControlAssignment_4_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41129:1: rule__DictionaryFileControl__BaseControlAssignment_4_1 : ( ruleBaseDictionaryControl ) ;
public final void rule__DictionaryFileControl__BaseControlAssignment_4_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41133:1: ( ( ruleBaseDictionaryControl ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41134:1: ( ruleBaseDictionaryControl )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41134:1: ( ruleBaseDictionaryControl )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41135:1: ruleBaseDictionaryControl
{
if ( state.backtracking==0 ) {
before(grammarAccess.getDictionaryFileControlAccess().getBaseControlBaseDictionaryControlParserRuleCall_4_1_0());
}
pushFollow(FollowSets003.FOLLOW_ruleBaseDictionaryControl_in_rule__DictionaryFileControl__BaseControlAssignment_4_182677);
ruleBaseDictionaryControl();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getDictionaryFileControlAccess().getBaseControlBaseDictionaryControlParserRuleCall_4_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__DictionaryFileControl__BaseControlAssignment_4_1"
// $ANTLR start "rule__Module__NameAssignment_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41144:1: rule__Module__NameAssignment_1 : ( RULE_ID ) ;
public final void rule__Module__NameAssignment_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41148:1: ( ( RULE_ID ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41149:1: ( RULE_ID )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41149:1: ( RULE_ID )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41150:1: RULE_ID
{
if ( state.backtracking==0 ) {
before(grammarAccess.getModuleAccess().getNameIDTerminalRuleCall_1_0());
}
match(input,RULE_ID,FollowSets003.FOLLOW_RULE_ID_in_rule__Module__NameAssignment_182708); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getModuleAccess().getNameIDTerminalRuleCall_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Module__NameAssignment_1"
// $ANTLR start "rule__Module__ModuledefinitionAssignment_4"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41159:1: rule__Module__ModuledefinitionAssignment_4 : ( ( ruleQualifiedName ) ) ;
public final void rule__Module__ModuledefinitionAssignment_4() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41163:1: ( ( ( ruleQualifiedName ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41164:1: ( ( ruleQualifiedName ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41164:1: ( ( ruleQualifiedName ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41165:1: ( ruleQualifiedName )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getModuleAccess().getModuledefinitionModuleDefinitionCrossReference_4_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41166:1: ( ruleQualifiedName )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41167:1: ruleQualifiedName
{
if ( state.backtracking==0 ) {
before(grammarAccess.getModuleAccess().getModuledefinitionModuleDefinitionQualifiedNameParserRuleCall_4_0_1());
}
pushFollow(FollowSets003.FOLLOW_ruleQualifiedName_in_rule__Module__ModuledefinitionAssignment_482743);
ruleQualifiedName();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getModuleAccess().getModuledefinitionModuleDefinitionQualifiedNameParserRuleCall_4_0_1());
}
}
if ( state.backtracking==0 ) {
after(grammarAccess.getModuleAccess().getModuledefinitionModuleDefinitionCrossReference_4_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Module__ModuledefinitionAssignment_4"
// $ANTLR start "rule__Module__ModuleParametersAssignment_5_2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41178:1: rule__Module__ModuleParametersAssignment_5_2 : ( ruleModuleParameter ) ;
public final void rule__Module__ModuleParametersAssignment_5_2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41182:1: ( ( ruleModuleParameter ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41183:1: ( ruleModuleParameter )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41183:1: ( ruleModuleParameter )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41184:1: ruleModuleParameter
{
if ( state.backtracking==0 ) {
before(grammarAccess.getModuleAccess().getModuleParametersModuleParameterParserRuleCall_5_2_0());
}
pushFollow(FollowSets003.FOLLOW_ruleModuleParameter_in_rule__Module__ModuleParametersAssignment_5_282778);
ruleModuleParameter();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getModuleAccess().getModuleParametersModuleParameterParserRuleCall_5_2_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Module__ModuleParametersAssignment_5_2"
// $ANTLR start "rule__ModuleParameter__ModuleDefinitionParameterAssignment_0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41193:1: rule__ModuleParameter__ModuleDefinitionParameterAssignment_0 : ( ( ruleQualifiedName ) ) ;
public final void rule__ModuleParameter__ModuleDefinitionParameterAssignment_0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41197:1: ( ( ( ruleQualifiedName ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41198:1: ( ( ruleQualifiedName ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41198:1: ( ( ruleQualifiedName ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41199:1: ( ruleQualifiedName )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getModuleParameterAccess().getModuleDefinitionParameterModuleDefinitionParameterCrossReference_0_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41200:1: ( ruleQualifiedName )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41201:1: ruleQualifiedName
{
if ( state.backtracking==0 ) {
before(grammarAccess.getModuleParameterAccess().getModuleDefinitionParameterModuleDefinitionParameterQualifiedNameParserRuleCall_0_0_1());
}
pushFollow(FollowSets003.FOLLOW_ruleQualifiedName_in_rule__ModuleParameter__ModuleDefinitionParameterAssignment_082813);
ruleQualifiedName();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getModuleParameterAccess().getModuleDefinitionParameterModuleDefinitionParameterQualifiedNameParserRuleCall_0_0_1());
}
}
if ( state.backtracking==0 ) {
after(grammarAccess.getModuleParameterAccess().getModuleDefinitionParameterModuleDefinitionParameterCrossReference_0_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ModuleParameter__ModuleDefinitionParameterAssignment_0"
// $ANTLR start "rule__ModuleParameter__ValueAssignment_2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41212:1: rule__ModuleParameter__ValueAssignment_2 : ( RULE_STRING ) ;
public final void rule__ModuleParameter__ValueAssignment_2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41216:1: ( ( RULE_STRING ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41217:1: ( RULE_STRING )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41217:1: ( RULE_STRING )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41218:1: RULE_STRING
{
if ( state.backtracking==0 ) {
before(grammarAccess.getModuleParameterAccess().getValueSTRINGTerminalRuleCall_2_0());
}
match(input,RULE_STRING,FollowSets003.FOLLOW_RULE_STRING_in_rule__ModuleParameter__ValueAssignment_282848); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getModuleParameterAccess().getValueSTRINGTerminalRuleCall_2_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ModuleParameter__ValueAssignment_2"
// $ANTLR start "rule__ModuleDefinition__NameAssignment_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41227:1: rule__ModuleDefinition__NameAssignment_1 : ( RULE_ID ) ;
public final void rule__ModuleDefinition__NameAssignment_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41231:1: ( ( RULE_ID ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41232:1: ( RULE_ID )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41232:1: ( RULE_ID )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41233:1: RULE_ID
{
if ( state.backtracking==0 ) {
before(grammarAccess.getModuleDefinitionAccess().getNameIDTerminalRuleCall_1_0());
}
match(input,RULE_ID,FollowSets003.FOLLOW_RULE_ID_in_rule__ModuleDefinition__NameAssignment_182879); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getModuleDefinitionAccess().getNameIDTerminalRuleCall_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ModuleDefinition__NameAssignment_1"
// $ANTLR start "rule__ModuleDefinition__ModuleDefinitionParametersAssignment_3_2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41242:1: rule__ModuleDefinition__ModuleDefinitionParametersAssignment_3_2 : ( ruleModuleDefinitionParameter ) ;
public final void rule__ModuleDefinition__ModuleDefinitionParametersAssignment_3_2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41246:1: ( ( ruleModuleDefinitionParameter ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41247:1: ( ruleModuleDefinitionParameter )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41247:1: ( ruleModuleDefinitionParameter )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41248:1: ruleModuleDefinitionParameter
{
if ( state.backtracking==0 ) {
before(grammarAccess.getModuleDefinitionAccess().getModuleDefinitionParametersModuleDefinitionParameterParserRuleCall_3_2_0());
}
pushFollow(FollowSets003.FOLLOW_ruleModuleDefinitionParameter_in_rule__ModuleDefinition__ModuleDefinitionParametersAssignment_3_282910);
ruleModuleDefinitionParameter();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getModuleDefinitionAccess().getModuleDefinitionParametersModuleDefinitionParameterParserRuleCall_3_2_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ModuleDefinition__ModuleDefinitionParametersAssignment_3_2"
// $ANTLR start "rule__ModuleDefinitionParameter__NameAssignment_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41257:1: rule__ModuleDefinitionParameter__NameAssignment_1 : ( RULE_ID ) ;
public final void rule__ModuleDefinitionParameter__NameAssignment_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41261:1: ( ( RULE_ID ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41262:1: ( RULE_ID )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41262:1: ( RULE_ID )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41263:1: RULE_ID
{
if ( state.backtracking==0 ) {
before(grammarAccess.getModuleDefinitionParameterAccess().getNameIDTerminalRuleCall_1_0());
}
match(input,RULE_ID,FollowSets003.FOLLOW_RULE_ID_in_rule__ModuleDefinitionParameter__NameAssignment_182941); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getModuleDefinitionParameterAccess().getNameIDTerminalRuleCall_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ModuleDefinitionParameter__NameAssignment_1"
// $ANTLR start "rule__ModuleDefinitionParameter__TypeAssignment_4"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41272:1: rule__ModuleDefinitionParameter__TypeAssignment_4 : ( ruleSimpleTypes ) ;
public final void rule__ModuleDefinitionParameter__TypeAssignment_4() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41276:1: ( ( ruleSimpleTypes ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41277:1: ( ruleSimpleTypes )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41277:1: ( ruleSimpleTypes )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41278:1: ruleSimpleTypes
{
if ( state.backtracking==0 ) {
before(grammarAccess.getModuleDefinitionParameterAccess().getTypeSimpleTypesEnumRuleCall_4_0());
}
pushFollow(FollowSets003.FOLLOW_ruleSimpleTypes_in_rule__ModuleDefinitionParameter__TypeAssignment_482972);
ruleSimpleTypes();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getModuleDefinitionParameterAccess().getTypeSimpleTypesEnumRuleCall_4_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ModuleDefinitionParameter__TypeAssignment_4"
// $ANTLR start "rule__ServiceOptions__NonpublicAssignment_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41287:1: rule__ServiceOptions__NonpublicAssignment_1 : ( ( 'nonpublic' ) ) ;
public final void rule__ServiceOptions__NonpublicAssignment_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41291:1: ( ( ( 'nonpublic' ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41292:1: ( ( 'nonpublic' ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41292:1: ( ( 'nonpublic' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41293:1: ( 'nonpublic' )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getServiceOptionsAccess().getNonpublicNonpublicKeyword_1_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41294:1: ( 'nonpublic' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41295:1: 'nonpublic'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getServiceOptionsAccess().getNonpublicNonpublicKeyword_1_0());
}
match(input,180,FollowSets003.FOLLOW_180_in_rule__ServiceOptions__NonpublicAssignment_183008); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getServiceOptionsAccess().getNonpublicNonpublicKeyword_1_0());
}
}
if ( state.backtracking==0 ) {
after(grammarAccess.getServiceOptionsAccess().getNonpublicNonpublicKeyword_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ServiceOptions__NonpublicAssignment_1"
// $ANTLR start "rule__ServiceMethod__TypeParameterAssignment_1_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41310:1: rule__ServiceMethod__TypeParameterAssignment_1_1 : ( ruleJvmTypeParameter ) ;
public final void rule__ServiceMethod__TypeParameterAssignment_1_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41314:1: ( ( ruleJvmTypeParameter ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41315:1: ( ruleJvmTypeParameter )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41315:1: ( ruleJvmTypeParameter )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41316:1: ruleJvmTypeParameter
{
if ( state.backtracking==0 ) {
before(grammarAccess.getServiceMethodAccess().getTypeParameterJvmTypeParameterParserRuleCall_1_1_0());
}
pushFollow(FollowSets003.FOLLOW_ruleJvmTypeParameter_in_rule__ServiceMethod__TypeParameterAssignment_1_183047);
ruleJvmTypeParameter();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getServiceMethodAccess().getTypeParameterJvmTypeParameterParserRuleCall_1_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ServiceMethod__TypeParameterAssignment_1_1"
// $ANTLR start "rule__ServiceMethod__ReturnTypeAssignment_2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41325:1: rule__ServiceMethod__ReturnTypeAssignment_2 : ( ruleJvmTypeReference ) ;
public final void rule__ServiceMethod__ReturnTypeAssignment_2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41329:1: ( ( ruleJvmTypeReference ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41330:1: ( ruleJvmTypeReference )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41330:1: ( ruleJvmTypeReference )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41331:1: ruleJvmTypeReference
{
if ( state.backtracking==0 ) {
before(grammarAccess.getServiceMethodAccess().getReturnTypeJvmTypeReferenceParserRuleCall_2_0());
}
pushFollow(FollowSets003.FOLLOW_ruleJvmTypeReference_in_rule__ServiceMethod__ReturnTypeAssignment_283078);
ruleJvmTypeReference();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getServiceMethodAccess().getReturnTypeJvmTypeReferenceParserRuleCall_2_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ServiceMethod__ReturnTypeAssignment_2"
// $ANTLR start "rule__ServiceMethod__NameAssignment_3"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41340:1: rule__ServiceMethod__NameAssignment_3 : ( ruleValidID ) ;
public final void rule__ServiceMethod__NameAssignment_3() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41344:1: ( ( ruleValidID ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41345:1: ( ruleValidID )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41345:1: ( ruleValidID )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41346:1: ruleValidID
{
if ( state.backtracking==0 ) {
before(grammarAccess.getServiceMethodAccess().getNameValidIDParserRuleCall_3_0());
}
pushFollow(FollowSets003.FOLLOW_ruleValidID_in_rule__ServiceMethod__NameAssignment_383109);
ruleValidID();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getServiceMethodAccess().getNameValidIDParserRuleCall_3_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ServiceMethod__NameAssignment_3"
// $ANTLR start "rule__ServiceMethod__ParamsAssignment_5_0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41355:1: rule__ServiceMethod__ParamsAssignment_5_0 : ( ruleFullJvmFormalParameter ) ;
public final void rule__ServiceMethod__ParamsAssignment_5_0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41359:1: ( ( ruleFullJvmFormalParameter ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41360:1: ( ruleFullJvmFormalParameter )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41360:1: ( ruleFullJvmFormalParameter )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41361:1: ruleFullJvmFormalParameter
{
if ( state.backtracking==0 ) {
before(grammarAccess.getServiceMethodAccess().getParamsFullJvmFormalParameterParserRuleCall_5_0_0());
}
pushFollow(FollowSets003.FOLLOW_ruleFullJvmFormalParameter_in_rule__ServiceMethod__ParamsAssignment_5_083140);
ruleFullJvmFormalParameter();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getServiceMethodAccess().getParamsFullJvmFormalParameterParserRuleCall_5_0_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ServiceMethod__ParamsAssignment_5_0"
// $ANTLR start "rule__ServiceMethod__ParamsAssignment_5_1_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41370:1: rule__ServiceMethod__ParamsAssignment_5_1_1 : ( ruleFullJvmFormalParameter ) ;
public final void rule__ServiceMethod__ParamsAssignment_5_1_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41374:1: ( ( ruleFullJvmFormalParameter ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41375:1: ( ruleFullJvmFormalParameter )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41375:1: ( ruleFullJvmFormalParameter )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41376:1: ruleFullJvmFormalParameter
{
if ( state.backtracking==0 ) {
before(grammarAccess.getServiceMethodAccess().getParamsFullJvmFormalParameterParserRuleCall_5_1_1_0());
}
pushFollow(FollowSets003.FOLLOW_ruleFullJvmFormalParameter_in_rule__ServiceMethod__ParamsAssignment_5_1_183171);
ruleFullJvmFormalParameter();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getServiceMethodAccess().getParamsFullJvmFormalParameterParserRuleCall_5_1_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__ServiceMethod__ParamsAssignment_5_1_1"
// $ANTLR start "rule__Service__NameAssignment_2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41385:1: rule__Service__NameAssignment_2 : ( RULE_ID ) ;
public final void rule__Service__NameAssignment_2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41389:1: ( ( RULE_ID ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41390:1: ( RULE_ID )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41390:1: ( RULE_ID )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41391:1: RULE_ID
{
if ( state.backtracking==0 ) {
before(grammarAccess.getServiceAccess().getNameIDTerminalRuleCall_2_0());
}
match(input,RULE_ID,FollowSets003.FOLLOW_RULE_ID_in_rule__Service__NameAssignment_283202); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getServiceAccess().getNameIDTerminalRuleCall_2_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Service__NameAssignment_2"
// $ANTLR start "rule__Service__RemoteServiceOptionsAssignment_4_2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41400:1: rule__Service__RemoteServiceOptionsAssignment_4_2 : ( ruleServiceOptions ) ;
public final void rule__Service__RemoteServiceOptionsAssignment_4_2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41404:1: ( ( ruleServiceOptions ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41405:1: ( ruleServiceOptions )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41405:1: ( ruleServiceOptions )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41406:1: ruleServiceOptions
{
if ( state.backtracking==0 ) {
before(grammarAccess.getServiceAccess().getRemoteServiceOptionsServiceOptionsParserRuleCall_4_2_0());
}
pushFollow(FollowSets003.FOLLOW_ruleServiceOptions_in_rule__Service__RemoteServiceOptionsAssignment_4_283233);
ruleServiceOptions();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getServiceAccess().getRemoteServiceOptionsServiceOptionsParserRuleCall_4_2_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Service__RemoteServiceOptionsAssignment_4_2"
// $ANTLR start "rule__Service__RemoteMethodsAssignment_5"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41415:1: rule__Service__RemoteMethodsAssignment_5 : ( ruleServiceMethod ) ;
public final void rule__Service__RemoteMethodsAssignment_5() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41419:1: ( ( ruleServiceMethod ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41420:1: ( ruleServiceMethod )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41420:1: ( ruleServiceMethod )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41421:1: ruleServiceMethod
{
if ( state.backtracking==0 ) {
before(grammarAccess.getServiceAccess().getRemoteMethodsServiceMethodParserRuleCall_5_0());
}
pushFollow(FollowSets003.FOLLOW_ruleServiceMethod_in_rule__Service__RemoteMethodsAssignment_583264);
ruleServiceMethod();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getServiceAccess().getRemoteMethodsServiceMethodParserRuleCall_5_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__Service__RemoteMethodsAssignment_5"
// $ANTLR start "rule__NavigationNode__NameAssignment_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41430:1: rule__NavigationNode__NameAssignment_1 : ( RULE_ID ) ;
public final void rule__NavigationNode__NameAssignment_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41434:1: ( ( RULE_ID ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41435:1: ( RULE_ID )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41435:1: ( RULE_ID )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41436:1: RULE_ID
{
if ( state.backtracking==0 ) {
before(grammarAccess.getNavigationNodeAccess().getNameIDTerminalRuleCall_1_0());
}
match(input,RULE_ID,FollowSets003.FOLLOW_RULE_ID_in_rule__NavigationNode__NameAssignment_183295); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getNavigationNodeAccess().getNameIDTerminalRuleCall_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__NavigationNode__NameAssignment_1"
// $ANTLR start "rule__NavigationNode__LabelAssignment_3_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41445:1: rule__NavigationNode__LabelAssignment_3_1 : ( RULE_STRING ) ;
public final void rule__NavigationNode__LabelAssignment_3_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41449:1: ( ( RULE_STRING ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41450:1: ( RULE_STRING )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41450:1: ( RULE_STRING )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41451:1: RULE_STRING
{
if ( state.backtracking==0 ) {
before(grammarAccess.getNavigationNodeAccess().getLabelSTRINGTerminalRuleCall_3_1_0());
}
match(input,RULE_STRING,FollowSets003.FOLLOW_RULE_STRING_in_rule__NavigationNode__LabelAssignment_3_183326); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getNavigationNodeAccess().getLabelSTRINGTerminalRuleCall_3_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__NavigationNode__LabelAssignment_3_1"
// $ANTLR start "rule__NavigationNode__ModuleDefinitionAssignment_4_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41460:1: rule__NavigationNode__ModuleDefinitionAssignment_4_1 : ( ( ruleQualifiedName ) ) ;
public final void rule__NavigationNode__ModuleDefinitionAssignment_4_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41464:1: ( ( ( ruleQualifiedName ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41465:1: ( ( ruleQualifiedName ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41465:1: ( ( ruleQualifiedName ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41466:1: ( ruleQualifiedName )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getNavigationNodeAccess().getModuleDefinitionModuleDefinitionCrossReference_4_1_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41467:1: ( ruleQualifiedName )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41468:1: ruleQualifiedName
{
if ( state.backtracking==0 ) {
before(grammarAccess.getNavigationNodeAccess().getModuleDefinitionModuleDefinitionQualifiedNameParserRuleCall_4_1_0_1());
}
pushFollow(FollowSets003.FOLLOW_ruleQualifiedName_in_rule__NavigationNode__ModuleDefinitionAssignment_4_183361);
ruleQualifiedName();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getNavigationNodeAccess().getModuleDefinitionModuleDefinitionQualifiedNameParserRuleCall_4_1_0_1());
}
}
if ( state.backtracking==0 ) {
after(grammarAccess.getNavigationNodeAccess().getModuleDefinitionModuleDefinitionCrossReference_4_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__NavigationNode__ModuleDefinitionAssignment_4_1"
// $ANTLR start "rule__NavigationNode__ModuleAssignment_5_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41479:1: rule__NavigationNode__ModuleAssignment_5_1 : ( ( ruleQualifiedName ) ) ;
public final void rule__NavigationNode__ModuleAssignment_5_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41483:1: ( ( ( ruleQualifiedName ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41484:1: ( ( ruleQualifiedName ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41484:1: ( ( ruleQualifiedName ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41485:1: ( ruleQualifiedName )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getNavigationNodeAccess().getModuleModuleCrossReference_5_1_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41486:1: ( ruleQualifiedName )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41487:1: ruleQualifiedName
{
if ( state.backtracking==0 ) {
before(grammarAccess.getNavigationNodeAccess().getModuleModuleQualifiedNameParserRuleCall_5_1_0_1());
}
pushFollow(FollowSets003.FOLLOW_ruleQualifiedName_in_rule__NavigationNode__ModuleAssignment_5_183400);
ruleQualifiedName();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getNavigationNodeAccess().getModuleModuleQualifiedNameParserRuleCall_5_1_0_1());
}
}
if ( state.backtracking==0 ) {
after(grammarAccess.getNavigationNodeAccess().getModuleModuleCrossReference_5_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__NavigationNode__ModuleAssignment_5_1"
// $ANTLR start "rule__NavigationNode__DictionaryEditorAssignment_6_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41498:1: rule__NavigationNode__DictionaryEditorAssignment_6_1 : ( ( ruleQualifiedName ) ) ;
public final void rule__NavigationNode__DictionaryEditorAssignment_6_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41502:1: ( ( ( ruleQualifiedName ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41503:1: ( ( ruleQualifiedName ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41503:1: ( ( ruleQualifiedName ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41504:1: ( ruleQualifiedName )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getNavigationNodeAccess().getDictionaryEditorDictionaryEditorCrossReference_6_1_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41505:1: ( ruleQualifiedName )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41506:1: ruleQualifiedName
{
if ( state.backtracking==0 ) {
before(grammarAccess.getNavigationNodeAccess().getDictionaryEditorDictionaryEditorQualifiedNameParserRuleCall_6_1_0_1());
}
pushFollow(FollowSets003.FOLLOW_ruleQualifiedName_in_rule__NavigationNode__DictionaryEditorAssignment_6_183439);
ruleQualifiedName();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getNavigationNodeAccess().getDictionaryEditorDictionaryEditorQualifiedNameParserRuleCall_6_1_0_1());
}
}
if ( state.backtracking==0 ) {
after(grammarAccess.getNavigationNodeAccess().getDictionaryEditorDictionaryEditorCrossReference_6_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__NavigationNode__DictionaryEditorAssignment_6_1"
// $ANTLR start "rule__NavigationNode__DictionarySearchAssignment_7_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41517:1: rule__NavigationNode__DictionarySearchAssignment_7_1 : ( ( ruleQualifiedName ) ) ;
public final void rule__NavigationNode__DictionarySearchAssignment_7_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41521:1: ( ( ( ruleQualifiedName ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41522:1: ( ( ruleQualifiedName ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41522:1: ( ( ruleQualifiedName ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41523:1: ( ruleQualifiedName )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getNavigationNodeAccess().getDictionarySearchDictionarySearchCrossReference_7_1_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41524:1: ( ruleQualifiedName )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41525:1: ruleQualifiedName
{
if ( state.backtracking==0 ) {
before(grammarAccess.getNavigationNodeAccess().getDictionarySearchDictionarySearchQualifiedNameParserRuleCall_7_1_0_1());
}
pushFollow(FollowSets003.FOLLOW_ruleQualifiedName_in_rule__NavigationNode__DictionarySearchAssignment_7_183478);
ruleQualifiedName();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getNavigationNodeAccess().getDictionarySearchDictionarySearchQualifiedNameParserRuleCall_7_1_0_1());
}
}
if ( state.backtracking==0 ) {
after(grammarAccess.getNavigationNodeAccess().getDictionarySearchDictionarySearchCrossReference_7_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__NavigationNode__DictionarySearchAssignment_7_1"
// $ANTLR start "rule__NavigationNode__NavigationNodesAssignment_8"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41536:1: rule__NavigationNode__NavigationNodesAssignment_8 : ( ruleNavigationNode ) ;
public final void rule__NavigationNode__NavigationNodesAssignment_8() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41540:1: ( ( ruleNavigationNode ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41541:1: ( ruleNavigationNode )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41541:1: ( ruleNavigationNode )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41542:1: ruleNavigationNode
{
if ( state.backtracking==0 ) {
before(grammarAccess.getNavigationNodeAccess().getNavigationNodesNavigationNodeParserRuleCall_8_0());
}
pushFollow(FollowSets003.FOLLOW_ruleNavigationNode_in_rule__NavigationNode__NavigationNodesAssignment_883513);
ruleNavigationNode();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getNavigationNodeAccess().getNavigationNodesNavigationNodeParserRuleCall_8_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__NavigationNode__NavigationNodesAssignment_8"
// $ANTLR start "rule__XAssignment__FeatureAssignment_0_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41551:1: rule__XAssignment__FeatureAssignment_0_1 : ( ( ruleFeatureCallID ) ) ;
public final void rule__XAssignment__FeatureAssignment_0_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41555:1: ( ( ( ruleFeatureCallID ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41556:1: ( ( ruleFeatureCallID ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41556:1: ( ( ruleFeatureCallID ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41557:1: ( ruleFeatureCallID )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXAssignmentAccess().getFeatureJvmIdentifiableElementCrossReference_0_1_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41558:1: ( ruleFeatureCallID )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41559:1: ruleFeatureCallID
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXAssignmentAccess().getFeatureJvmIdentifiableElementFeatureCallIDParserRuleCall_0_1_0_1());
}
pushFollow(FollowSets003.FOLLOW_ruleFeatureCallID_in_rule__XAssignment__FeatureAssignment_0_183548);
ruleFeatureCallID();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXAssignmentAccess().getFeatureJvmIdentifiableElementFeatureCallIDParserRuleCall_0_1_0_1());
}
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXAssignmentAccess().getFeatureJvmIdentifiableElementCrossReference_0_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XAssignment__FeatureAssignment_0_1"
// $ANTLR start "rule__XAssignment__ValueAssignment_0_3"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41570:1: rule__XAssignment__ValueAssignment_0_3 : ( ruleXAssignment ) ;
public final void rule__XAssignment__ValueAssignment_0_3() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41574:1: ( ( ruleXAssignment ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41575:1: ( ruleXAssignment )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41575:1: ( ruleXAssignment )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41576:1: ruleXAssignment
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXAssignmentAccess().getValueXAssignmentParserRuleCall_0_3_0());
}
pushFollow(FollowSets003.FOLLOW_ruleXAssignment_in_rule__XAssignment__ValueAssignment_0_383583);
ruleXAssignment();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXAssignmentAccess().getValueXAssignmentParserRuleCall_0_3_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XAssignment__ValueAssignment_0_3"
// $ANTLR start "rule__XAssignment__FeatureAssignment_1_1_0_0_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41585:1: rule__XAssignment__FeatureAssignment_1_1_0_0_1 : ( ( ruleOpMultiAssign ) ) ;
public final void rule__XAssignment__FeatureAssignment_1_1_0_0_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41589:1: ( ( ( ruleOpMultiAssign ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41590:1: ( ( ruleOpMultiAssign ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41590:1: ( ( ruleOpMultiAssign ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41591:1: ( ruleOpMultiAssign )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXAssignmentAccess().getFeatureJvmIdentifiableElementCrossReference_1_1_0_0_1_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41592:1: ( ruleOpMultiAssign )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41593:1: ruleOpMultiAssign
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXAssignmentAccess().getFeatureJvmIdentifiableElementOpMultiAssignParserRuleCall_1_1_0_0_1_0_1());
}
pushFollow(FollowSets003.FOLLOW_ruleOpMultiAssign_in_rule__XAssignment__FeatureAssignment_1_1_0_0_183618);
ruleOpMultiAssign();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXAssignmentAccess().getFeatureJvmIdentifiableElementOpMultiAssignParserRuleCall_1_1_0_0_1_0_1());
}
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXAssignmentAccess().getFeatureJvmIdentifiableElementCrossReference_1_1_0_0_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XAssignment__FeatureAssignment_1_1_0_0_1"
// $ANTLR start "rule__XAssignment__RightOperandAssignment_1_1_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41604:1: rule__XAssignment__RightOperandAssignment_1_1_1 : ( ruleXAssignment ) ;
public final void rule__XAssignment__RightOperandAssignment_1_1_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41608:1: ( ( ruleXAssignment ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41609:1: ( ruleXAssignment )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41609:1: ( ruleXAssignment )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41610:1: ruleXAssignment
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXAssignmentAccess().getRightOperandXAssignmentParserRuleCall_1_1_1_0());
}
pushFollow(FollowSets003.FOLLOW_ruleXAssignment_in_rule__XAssignment__RightOperandAssignment_1_1_183653);
ruleXAssignment();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXAssignmentAccess().getRightOperandXAssignmentParserRuleCall_1_1_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XAssignment__RightOperandAssignment_1_1_1"
// $ANTLR start "rule__XOrExpression__FeatureAssignment_1_0_0_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41619:1: rule__XOrExpression__FeatureAssignment_1_0_0_1 : ( ( ruleOpOr ) ) ;
public final void rule__XOrExpression__FeatureAssignment_1_0_0_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41623:1: ( ( ( ruleOpOr ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41624:1: ( ( ruleOpOr ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41624:1: ( ( ruleOpOr ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41625:1: ( ruleOpOr )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXOrExpressionAccess().getFeatureJvmIdentifiableElementCrossReference_1_0_0_1_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41626:1: ( ruleOpOr )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41627:1: ruleOpOr
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXOrExpressionAccess().getFeatureJvmIdentifiableElementOpOrParserRuleCall_1_0_0_1_0_1());
}
pushFollow(FollowSets003.FOLLOW_ruleOpOr_in_rule__XOrExpression__FeatureAssignment_1_0_0_183688);
ruleOpOr();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXOrExpressionAccess().getFeatureJvmIdentifiableElementOpOrParserRuleCall_1_0_0_1_0_1());
}
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXOrExpressionAccess().getFeatureJvmIdentifiableElementCrossReference_1_0_0_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XOrExpression__FeatureAssignment_1_0_0_1"
// $ANTLR start "rule__XOrExpression__RightOperandAssignment_1_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41638:1: rule__XOrExpression__RightOperandAssignment_1_1 : ( ruleXAndExpression ) ;
public final void rule__XOrExpression__RightOperandAssignment_1_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41642:1: ( ( ruleXAndExpression ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41643:1: ( ruleXAndExpression )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41643:1: ( ruleXAndExpression )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41644:1: ruleXAndExpression
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXOrExpressionAccess().getRightOperandXAndExpressionParserRuleCall_1_1_0());
}
pushFollow(FollowSets003.FOLLOW_ruleXAndExpression_in_rule__XOrExpression__RightOperandAssignment_1_183723);
ruleXAndExpression();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXOrExpressionAccess().getRightOperandXAndExpressionParserRuleCall_1_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XOrExpression__RightOperandAssignment_1_1"
// $ANTLR start "rule__XAndExpression__FeatureAssignment_1_0_0_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41653:1: rule__XAndExpression__FeatureAssignment_1_0_0_1 : ( ( ruleOpAnd ) ) ;
public final void rule__XAndExpression__FeatureAssignment_1_0_0_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41657:1: ( ( ( ruleOpAnd ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41658:1: ( ( ruleOpAnd ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41658:1: ( ( ruleOpAnd ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41659:1: ( ruleOpAnd )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXAndExpressionAccess().getFeatureJvmIdentifiableElementCrossReference_1_0_0_1_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41660:1: ( ruleOpAnd )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41661:1: ruleOpAnd
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXAndExpressionAccess().getFeatureJvmIdentifiableElementOpAndParserRuleCall_1_0_0_1_0_1());
}
pushFollow(FollowSets003.FOLLOW_ruleOpAnd_in_rule__XAndExpression__FeatureAssignment_1_0_0_183758);
ruleOpAnd();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXAndExpressionAccess().getFeatureJvmIdentifiableElementOpAndParserRuleCall_1_0_0_1_0_1());
}
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXAndExpressionAccess().getFeatureJvmIdentifiableElementCrossReference_1_0_0_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XAndExpression__FeatureAssignment_1_0_0_1"
// $ANTLR start "rule__XAndExpression__RightOperandAssignment_1_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41672:1: rule__XAndExpression__RightOperandAssignment_1_1 : ( ruleXEqualityExpression ) ;
public final void rule__XAndExpression__RightOperandAssignment_1_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41676:1: ( ( ruleXEqualityExpression ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41677:1: ( ruleXEqualityExpression )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41677:1: ( ruleXEqualityExpression )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41678:1: ruleXEqualityExpression
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXAndExpressionAccess().getRightOperandXEqualityExpressionParserRuleCall_1_1_0());
}
pushFollow(FollowSets003.FOLLOW_ruleXEqualityExpression_in_rule__XAndExpression__RightOperandAssignment_1_183793);
ruleXEqualityExpression();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXAndExpressionAccess().getRightOperandXEqualityExpressionParserRuleCall_1_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XAndExpression__RightOperandAssignment_1_1"
// $ANTLR start "rule__XEqualityExpression__FeatureAssignment_1_0_0_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41687:1: rule__XEqualityExpression__FeatureAssignment_1_0_0_1 : ( ( ruleOpEquality ) ) ;
public final void rule__XEqualityExpression__FeatureAssignment_1_0_0_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41691:1: ( ( ( ruleOpEquality ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41692:1: ( ( ruleOpEquality ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41692:1: ( ( ruleOpEquality ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41693:1: ( ruleOpEquality )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXEqualityExpressionAccess().getFeatureJvmIdentifiableElementCrossReference_1_0_0_1_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41694:1: ( ruleOpEquality )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41695:1: ruleOpEquality
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXEqualityExpressionAccess().getFeatureJvmIdentifiableElementOpEqualityParserRuleCall_1_0_0_1_0_1());
}
pushFollow(FollowSets003.FOLLOW_ruleOpEquality_in_rule__XEqualityExpression__FeatureAssignment_1_0_0_183828);
ruleOpEquality();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXEqualityExpressionAccess().getFeatureJvmIdentifiableElementOpEqualityParserRuleCall_1_0_0_1_0_1());
}
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXEqualityExpressionAccess().getFeatureJvmIdentifiableElementCrossReference_1_0_0_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XEqualityExpression__FeatureAssignment_1_0_0_1"
// $ANTLR start "rule__XEqualityExpression__RightOperandAssignment_1_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41706:1: rule__XEqualityExpression__RightOperandAssignment_1_1 : ( ruleXRelationalExpression ) ;
public final void rule__XEqualityExpression__RightOperandAssignment_1_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41710:1: ( ( ruleXRelationalExpression ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41711:1: ( ruleXRelationalExpression )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41711:1: ( ruleXRelationalExpression )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41712:1: ruleXRelationalExpression
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXEqualityExpressionAccess().getRightOperandXRelationalExpressionParserRuleCall_1_1_0());
}
pushFollow(FollowSets003.FOLLOW_ruleXRelationalExpression_in_rule__XEqualityExpression__RightOperandAssignment_1_183863);
ruleXRelationalExpression();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXEqualityExpressionAccess().getRightOperandXRelationalExpressionParserRuleCall_1_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XEqualityExpression__RightOperandAssignment_1_1"
// $ANTLR start "rule__XRelationalExpression__TypeAssignment_1_0_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41721:1: rule__XRelationalExpression__TypeAssignment_1_0_1 : ( ruleJvmTypeReference ) ;
public final void rule__XRelationalExpression__TypeAssignment_1_0_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41725:1: ( ( ruleJvmTypeReference ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41726:1: ( ruleJvmTypeReference )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41726:1: ( ruleJvmTypeReference )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41727:1: ruleJvmTypeReference
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXRelationalExpressionAccess().getTypeJvmTypeReferenceParserRuleCall_1_0_1_0());
}
pushFollow(FollowSets003.FOLLOW_ruleJvmTypeReference_in_rule__XRelationalExpression__TypeAssignment_1_0_183894);
ruleJvmTypeReference();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXRelationalExpressionAccess().getTypeJvmTypeReferenceParserRuleCall_1_0_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XRelationalExpression__TypeAssignment_1_0_1"
// $ANTLR start "rule__XRelationalExpression__FeatureAssignment_1_1_0_0_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41736:1: rule__XRelationalExpression__FeatureAssignment_1_1_0_0_1 : ( ( ruleOpCompare ) ) ;
public final void rule__XRelationalExpression__FeatureAssignment_1_1_0_0_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41740:1: ( ( ( ruleOpCompare ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41741:1: ( ( ruleOpCompare ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41741:1: ( ( ruleOpCompare ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41742:1: ( ruleOpCompare )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXRelationalExpressionAccess().getFeatureJvmIdentifiableElementCrossReference_1_1_0_0_1_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41743:1: ( ruleOpCompare )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41744:1: ruleOpCompare
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXRelationalExpressionAccess().getFeatureJvmIdentifiableElementOpCompareParserRuleCall_1_1_0_0_1_0_1());
}
pushFollow(FollowSets003.FOLLOW_ruleOpCompare_in_rule__XRelationalExpression__FeatureAssignment_1_1_0_0_183929);
ruleOpCompare();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXRelationalExpressionAccess().getFeatureJvmIdentifiableElementOpCompareParserRuleCall_1_1_0_0_1_0_1());
}
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXRelationalExpressionAccess().getFeatureJvmIdentifiableElementCrossReference_1_1_0_0_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XRelationalExpression__FeatureAssignment_1_1_0_0_1"
// $ANTLR start "rule__XRelationalExpression__RightOperandAssignment_1_1_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41755:1: rule__XRelationalExpression__RightOperandAssignment_1_1_1 : ( ruleXOtherOperatorExpression ) ;
public final void rule__XRelationalExpression__RightOperandAssignment_1_1_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41759:1: ( ( ruleXOtherOperatorExpression ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41760:1: ( ruleXOtherOperatorExpression )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41760:1: ( ruleXOtherOperatorExpression )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41761:1: ruleXOtherOperatorExpression
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXRelationalExpressionAccess().getRightOperandXOtherOperatorExpressionParserRuleCall_1_1_1_0());
}
pushFollow(FollowSets003.FOLLOW_ruleXOtherOperatorExpression_in_rule__XRelationalExpression__RightOperandAssignment_1_1_183964);
ruleXOtherOperatorExpression();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXRelationalExpressionAccess().getRightOperandXOtherOperatorExpressionParserRuleCall_1_1_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XRelationalExpression__RightOperandAssignment_1_1_1"
// $ANTLR start "rule__XOtherOperatorExpression__FeatureAssignment_1_0_0_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41770:1: rule__XOtherOperatorExpression__FeatureAssignment_1_0_0_1 : ( ( ruleOpOther ) ) ;
public final void rule__XOtherOperatorExpression__FeatureAssignment_1_0_0_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41774:1: ( ( ( ruleOpOther ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41775:1: ( ( ruleOpOther ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41775:1: ( ( ruleOpOther ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41776:1: ( ruleOpOther )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXOtherOperatorExpressionAccess().getFeatureJvmIdentifiableElementCrossReference_1_0_0_1_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41777:1: ( ruleOpOther )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41778:1: ruleOpOther
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXOtherOperatorExpressionAccess().getFeatureJvmIdentifiableElementOpOtherParserRuleCall_1_0_0_1_0_1());
}
pushFollow(FollowSets003.FOLLOW_ruleOpOther_in_rule__XOtherOperatorExpression__FeatureAssignment_1_0_0_183999);
ruleOpOther();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXOtherOperatorExpressionAccess().getFeatureJvmIdentifiableElementOpOtherParserRuleCall_1_0_0_1_0_1());
}
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXOtherOperatorExpressionAccess().getFeatureJvmIdentifiableElementCrossReference_1_0_0_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XOtherOperatorExpression__FeatureAssignment_1_0_0_1"
// $ANTLR start "rule__XOtherOperatorExpression__RightOperandAssignment_1_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41789:1: rule__XOtherOperatorExpression__RightOperandAssignment_1_1 : ( ruleXAdditiveExpression ) ;
public final void rule__XOtherOperatorExpression__RightOperandAssignment_1_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41793:1: ( ( ruleXAdditiveExpression ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41794:1: ( ruleXAdditiveExpression )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41794:1: ( ruleXAdditiveExpression )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41795:1: ruleXAdditiveExpression
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXOtherOperatorExpressionAccess().getRightOperandXAdditiveExpressionParserRuleCall_1_1_0());
}
pushFollow(FollowSets003.FOLLOW_ruleXAdditiveExpression_in_rule__XOtherOperatorExpression__RightOperandAssignment_1_184034);
ruleXAdditiveExpression();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXOtherOperatorExpressionAccess().getRightOperandXAdditiveExpressionParserRuleCall_1_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XOtherOperatorExpression__RightOperandAssignment_1_1"
// $ANTLR start "rule__XAdditiveExpression__FeatureAssignment_1_0_0_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41804:1: rule__XAdditiveExpression__FeatureAssignment_1_0_0_1 : ( ( ruleOpAdd ) ) ;
public final void rule__XAdditiveExpression__FeatureAssignment_1_0_0_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41808:1: ( ( ( ruleOpAdd ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41809:1: ( ( ruleOpAdd ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41809:1: ( ( ruleOpAdd ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41810:1: ( ruleOpAdd )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXAdditiveExpressionAccess().getFeatureJvmIdentifiableElementCrossReference_1_0_0_1_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41811:1: ( ruleOpAdd )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41812:1: ruleOpAdd
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXAdditiveExpressionAccess().getFeatureJvmIdentifiableElementOpAddParserRuleCall_1_0_0_1_0_1());
}
pushFollow(FollowSets003.FOLLOW_ruleOpAdd_in_rule__XAdditiveExpression__FeatureAssignment_1_0_0_184069);
ruleOpAdd();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXAdditiveExpressionAccess().getFeatureJvmIdentifiableElementOpAddParserRuleCall_1_0_0_1_0_1());
}
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXAdditiveExpressionAccess().getFeatureJvmIdentifiableElementCrossReference_1_0_0_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XAdditiveExpression__FeatureAssignment_1_0_0_1"
// $ANTLR start "rule__XAdditiveExpression__RightOperandAssignment_1_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41823:1: rule__XAdditiveExpression__RightOperandAssignment_1_1 : ( ruleXMultiplicativeExpression ) ;
public final void rule__XAdditiveExpression__RightOperandAssignment_1_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41827:1: ( ( ruleXMultiplicativeExpression ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41828:1: ( ruleXMultiplicativeExpression )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41828:1: ( ruleXMultiplicativeExpression )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41829:1: ruleXMultiplicativeExpression
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXAdditiveExpressionAccess().getRightOperandXMultiplicativeExpressionParserRuleCall_1_1_0());
}
pushFollow(FollowSets003.FOLLOW_ruleXMultiplicativeExpression_in_rule__XAdditiveExpression__RightOperandAssignment_1_184104);
ruleXMultiplicativeExpression();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXAdditiveExpressionAccess().getRightOperandXMultiplicativeExpressionParserRuleCall_1_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XAdditiveExpression__RightOperandAssignment_1_1"
// $ANTLR start "rule__XMultiplicativeExpression__FeatureAssignment_1_0_0_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41838:1: rule__XMultiplicativeExpression__FeatureAssignment_1_0_0_1 : ( ( ruleOpMulti ) ) ;
public final void rule__XMultiplicativeExpression__FeatureAssignment_1_0_0_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41842:1: ( ( ( ruleOpMulti ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41843:1: ( ( ruleOpMulti ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41843:1: ( ( ruleOpMulti ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41844:1: ( ruleOpMulti )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXMultiplicativeExpressionAccess().getFeatureJvmIdentifiableElementCrossReference_1_0_0_1_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41845:1: ( ruleOpMulti )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41846:1: ruleOpMulti
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXMultiplicativeExpressionAccess().getFeatureJvmIdentifiableElementOpMultiParserRuleCall_1_0_0_1_0_1());
}
pushFollow(FollowSets003.FOLLOW_ruleOpMulti_in_rule__XMultiplicativeExpression__FeatureAssignment_1_0_0_184139);
ruleOpMulti();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXMultiplicativeExpressionAccess().getFeatureJvmIdentifiableElementOpMultiParserRuleCall_1_0_0_1_0_1());
}
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXMultiplicativeExpressionAccess().getFeatureJvmIdentifiableElementCrossReference_1_0_0_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XMultiplicativeExpression__FeatureAssignment_1_0_0_1"
// $ANTLR start "rule__XMultiplicativeExpression__RightOperandAssignment_1_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41857:1: rule__XMultiplicativeExpression__RightOperandAssignment_1_1 : ( ruleXUnaryOperation ) ;
public final void rule__XMultiplicativeExpression__RightOperandAssignment_1_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41861:1: ( ( ruleXUnaryOperation ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41862:1: ( ruleXUnaryOperation )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41862:1: ( ruleXUnaryOperation )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41863:1: ruleXUnaryOperation
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXMultiplicativeExpressionAccess().getRightOperandXUnaryOperationParserRuleCall_1_1_0());
}
pushFollow(FollowSets003.FOLLOW_ruleXUnaryOperation_in_rule__XMultiplicativeExpression__RightOperandAssignment_1_184174);
ruleXUnaryOperation();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXMultiplicativeExpressionAccess().getRightOperandXUnaryOperationParserRuleCall_1_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XMultiplicativeExpression__RightOperandAssignment_1_1"
// $ANTLR start "rule__XUnaryOperation__FeatureAssignment_0_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41872:1: rule__XUnaryOperation__FeatureAssignment_0_1 : ( ( ruleOpUnary ) ) ;
public final void rule__XUnaryOperation__FeatureAssignment_0_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41876:1: ( ( ( ruleOpUnary ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41877:1: ( ( ruleOpUnary ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41877:1: ( ( ruleOpUnary ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41878:1: ( ruleOpUnary )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXUnaryOperationAccess().getFeatureJvmIdentifiableElementCrossReference_0_1_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41879:1: ( ruleOpUnary )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41880:1: ruleOpUnary
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXUnaryOperationAccess().getFeatureJvmIdentifiableElementOpUnaryParserRuleCall_0_1_0_1());
}
pushFollow(FollowSets003.FOLLOW_ruleOpUnary_in_rule__XUnaryOperation__FeatureAssignment_0_184209);
ruleOpUnary();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXUnaryOperationAccess().getFeatureJvmIdentifiableElementOpUnaryParserRuleCall_0_1_0_1());
}
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXUnaryOperationAccess().getFeatureJvmIdentifiableElementCrossReference_0_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XUnaryOperation__FeatureAssignment_0_1"
// $ANTLR start "rule__XUnaryOperation__OperandAssignment_0_2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41891:1: rule__XUnaryOperation__OperandAssignment_0_2 : ( ruleXUnaryOperation ) ;
public final void rule__XUnaryOperation__OperandAssignment_0_2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41895:1: ( ( ruleXUnaryOperation ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41896:1: ( ruleXUnaryOperation )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41896:1: ( ruleXUnaryOperation )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41897:1: ruleXUnaryOperation
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXUnaryOperationAccess().getOperandXUnaryOperationParserRuleCall_0_2_0());
}
pushFollow(FollowSets003.FOLLOW_ruleXUnaryOperation_in_rule__XUnaryOperation__OperandAssignment_0_284244);
ruleXUnaryOperation();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXUnaryOperationAccess().getOperandXUnaryOperationParserRuleCall_0_2_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XUnaryOperation__OperandAssignment_0_2"
// $ANTLR start "rule__XCastedExpression__TypeAssignment_1_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41906:1: rule__XCastedExpression__TypeAssignment_1_1 : ( ruleJvmTypeReference ) ;
public final void rule__XCastedExpression__TypeAssignment_1_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41910:1: ( ( ruleJvmTypeReference ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41911:1: ( ruleJvmTypeReference )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41911:1: ( ruleJvmTypeReference )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41912:1: ruleJvmTypeReference
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXCastedExpressionAccess().getTypeJvmTypeReferenceParserRuleCall_1_1_0());
}
pushFollow(FollowSets003.FOLLOW_ruleJvmTypeReference_in_rule__XCastedExpression__TypeAssignment_1_184275);
ruleJvmTypeReference();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXCastedExpressionAccess().getTypeJvmTypeReferenceParserRuleCall_1_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XCastedExpression__TypeAssignment_1_1"
// $ANTLR start "rule__XPostfixOperation__FeatureAssignment_1_0_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41921:1: rule__XPostfixOperation__FeatureAssignment_1_0_1 : ( ( ruleOpPostfix ) ) ;
public final void rule__XPostfixOperation__FeatureAssignment_1_0_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41925:1: ( ( ( ruleOpPostfix ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41926:1: ( ( ruleOpPostfix ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41926:1: ( ( ruleOpPostfix ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41927:1: ( ruleOpPostfix )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXPostfixOperationAccess().getFeatureJvmIdentifiableElementCrossReference_1_0_1_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41928:1: ( ruleOpPostfix )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41929:1: ruleOpPostfix
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXPostfixOperationAccess().getFeatureJvmIdentifiableElementOpPostfixParserRuleCall_1_0_1_0_1());
}
pushFollow(FollowSets003.FOLLOW_ruleOpPostfix_in_rule__XPostfixOperation__FeatureAssignment_1_0_184310);
ruleOpPostfix();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXPostfixOperationAccess().getFeatureJvmIdentifiableElementOpPostfixParserRuleCall_1_0_1_0_1());
}
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXPostfixOperationAccess().getFeatureJvmIdentifiableElementCrossReference_1_0_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XPostfixOperation__FeatureAssignment_1_0_1"
// $ANTLR start "rule__XMemberFeatureCall__ExplicitStaticAssignment_1_0_0_0_1_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41940:1: rule__XMemberFeatureCall__ExplicitStaticAssignment_1_0_0_0_1_1 : ( ( '::' ) ) ;
public final void rule__XMemberFeatureCall__ExplicitStaticAssignment_1_0_0_0_1_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41944:1: ( ( ( '::' ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41945:1: ( ( '::' ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41945:1: ( ( '::' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41946:1: ( '::' )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXMemberFeatureCallAccess().getExplicitStaticColonColonKeyword_1_0_0_0_1_1_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41947:1: ( '::' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41948:1: '::'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXMemberFeatureCallAccess().getExplicitStaticColonColonKeyword_1_0_0_0_1_1_0());
}
match(input,181,FollowSets003.FOLLOW_181_in_rule__XMemberFeatureCall__ExplicitStaticAssignment_1_0_0_0_1_184350); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXMemberFeatureCallAccess().getExplicitStaticColonColonKeyword_1_0_0_0_1_1_0());
}
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXMemberFeatureCallAccess().getExplicitStaticColonColonKeyword_1_0_0_0_1_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XMemberFeatureCall__ExplicitStaticAssignment_1_0_0_0_1_1"
// $ANTLR start "rule__XMemberFeatureCall__FeatureAssignment_1_0_0_0_2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41963:1: rule__XMemberFeatureCall__FeatureAssignment_1_0_0_0_2 : ( ( ruleFeatureCallID ) ) ;
public final void rule__XMemberFeatureCall__FeatureAssignment_1_0_0_0_2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41967:1: ( ( ( ruleFeatureCallID ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41968:1: ( ( ruleFeatureCallID ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41968:1: ( ( ruleFeatureCallID ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41969:1: ( ruleFeatureCallID )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXMemberFeatureCallAccess().getFeatureJvmIdentifiableElementCrossReference_1_0_0_0_2_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41970:1: ( ruleFeatureCallID )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41971:1: ruleFeatureCallID
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXMemberFeatureCallAccess().getFeatureJvmIdentifiableElementFeatureCallIDParserRuleCall_1_0_0_0_2_0_1());
}
pushFollow(FollowSets003.FOLLOW_ruleFeatureCallID_in_rule__XMemberFeatureCall__FeatureAssignment_1_0_0_0_284393);
ruleFeatureCallID();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXMemberFeatureCallAccess().getFeatureJvmIdentifiableElementFeatureCallIDParserRuleCall_1_0_0_0_2_0_1());
}
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXMemberFeatureCallAccess().getFeatureJvmIdentifiableElementCrossReference_1_0_0_0_2_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XMemberFeatureCall__FeatureAssignment_1_0_0_0_2"
// $ANTLR start "rule__XMemberFeatureCall__ValueAssignment_1_0_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41982:1: rule__XMemberFeatureCall__ValueAssignment_1_0_1 : ( ruleXAssignment ) ;
public final void rule__XMemberFeatureCall__ValueAssignment_1_0_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41986:1: ( ( ruleXAssignment ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41987:1: ( ruleXAssignment )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41987:1: ( ruleXAssignment )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41988:1: ruleXAssignment
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXMemberFeatureCallAccess().getValueXAssignmentParserRuleCall_1_0_1_0());
}
pushFollow(FollowSets003.FOLLOW_ruleXAssignment_in_rule__XMemberFeatureCall__ValueAssignment_1_0_184428);
ruleXAssignment();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXMemberFeatureCallAccess().getValueXAssignmentParserRuleCall_1_0_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XMemberFeatureCall__ValueAssignment_1_0_1"
// $ANTLR start "rule__XMemberFeatureCall__NullSafeAssignment_1_1_0_0_1_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:41997:1: rule__XMemberFeatureCall__NullSafeAssignment_1_1_0_0_1_1 : ( ( '?.' ) ) ;
public final void rule__XMemberFeatureCall__NullSafeAssignment_1_1_0_0_1_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42001:1: ( ( ( '?.' ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42002:1: ( ( '?.' ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42002:1: ( ( '?.' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42003:1: ( '?.' )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXMemberFeatureCallAccess().getNullSafeQuestionMarkFullStopKeyword_1_1_0_0_1_1_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42004:1: ( '?.' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42005:1: '?.'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXMemberFeatureCallAccess().getNullSafeQuestionMarkFullStopKeyword_1_1_0_0_1_1_0());
}
match(input,182,FollowSets003.FOLLOW_182_in_rule__XMemberFeatureCall__NullSafeAssignment_1_1_0_0_1_184464); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXMemberFeatureCallAccess().getNullSafeQuestionMarkFullStopKeyword_1_1_0_0_1_1_0());
}
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXMemberFeatureCallAccess().getNullSafeQuestionMarkFullStopKeyword_1_1_0_0_1_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XMemberFeatureCall__NullSafeAssignment_1_1_0_0_1_1"
// $ANTLR start "rule__XMemberFeatureCall__ExplicitStaticAssignment_1_1_0_0_1_2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42020:1: rule__XMemberFeatureCall__ExplicitStaticAssignment_1_1_0_0_1_2 : ( ( '::' ) ) ;
public final void rule__XMemberFeatureCall__ExplicitStaticAssignment_1_1_0_0_1_2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42024:1: ( ( ( '::' ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42025:1: ( ( '::' ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42025:1: ( ( '::' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42026:1: ( '::' )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXMemberFeatureCallAccess().getExplicitStaticColonColonKeyword_1_1_0_0_1_2_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42027:1: ( '::' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42028:1: '::'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXMemberFeatureCallAccess().getExplicitStaticColonColonKeyword_1_1_0_0_1_2_0());
}
match(input,181,FollowSets003.FOLLOW_181_in_rule__XMemberFeatureCall__ExplicitStaticAssignment_1_1_0_0_1_284508); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXMemberFeatureCallAccess().getExplicitStaticColonColonKeyword_1_1_0_0_1_2_0());
}
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXMemberFeatureCallAccess().getExplicitStaticColonColonKeyword_1_1_0_0_1_2_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XMemberFeatureCall__ExplicitStaticAssignment_1_1_0_0_1_2"
// $ANTLR start "rule__XMemberFeatureCall__TypeArgumentsAssignment_1_1_1_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42043:1: rule__XMemberFeatureCall__TypeArgumentsAssignment_1_1_1_1 : ( ruleJvmArgumentTypeReference ) ;
public final void rule__XMemberFeatureCall__TypeArgumentsAssignment_1_1_1_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42047:1: ( ( ruleJvmArgumentTypeReference ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42048:1: ( ruleJvmArgumentTypeReference )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42048:1: ( ruleJvmArgumentTypeReference )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42049:1: ruleJvmArgumentTypeReference
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXMemberFeatureCallAccess().getTypeArgumentsJvmArgumentTypeReferenceParserRuleCall_1_1_1_1_0());
}
pushFollow(FollowSets003.FOLLOW_ruleJvmArgumentTypeReference_in_rule__XMemberFeatureCall__TypeArgumentsAssignment_1_1_1_184547);
ruleJvmArgumentTypeReference();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXMemberFeatureCallAccess().getTypeArgumentsJvmArgumentTypeReferenceParserRuleCall_1_1_1_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XMemberFeatureCall__TypeArgumentsAssignment_1_1_1_1"
// $ANTLR start "rule__XMemberFeatureCall__TypeArgumentsAssignment_1_1_1_2_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42058:1: rule__XMemberFeatureCall__TypeArgumentsAssignment_1_1_1_2_1 : ( ruleJvmArgumentTypeReference ) ;
public final void rule__XMemberFeatureCall__TypeArgumentsAssignment_1_1_1_2_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42062:1: ( ( ruleJvmArgumentTypeReference ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42063:1: ( ruleJvmArgumentTypeReference )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42063:1: ( ruleJvmArgumentTypeReference )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42064:1: ruleJvmArgumentTypeReference
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXMemberFeatureCallAccess().getTypeArgumentsJvmArgumentTypeReferenceParserRuleCall_1_1_1_2_1_0());
}
pushFollow(FollowSets003.FOLLOW_ruleJvmArgumentTypeReference_in_rule__XMemberFeatureCall__TypeArgumentsAssignment_1_1_1_2_184578);
ruleJvmArgumentTypeReference();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXMemberFeatureCallAccess().getTypeArgumentsJvmArgumentTypeReferenceParserRuleCall_1_1_1_2_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XMemberFeatureCall__TypeArgumentsAssignment_1_1_1_2_1"
// $ANTLR start "rule__XMemberFeatureCall__FeatureAssignment_1_1_2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42073:1: rule__XMemberFeatureCall__FeatureAssignment_1_1_2 : ( ( ruleIdOrSuper ) ) ;
public final void rule__XMemberFeatureCall__FeatureAssignment_1_1_2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42077:1: ( ( ( ruleIdOrSuper ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42078:1: ( ( ruleIdOrSuper ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42078:1: ( ( ruleIdOrSuper ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42079:1: ( ruleIdOrSuper )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXMemberFeatureCallAccess().getFeatureJvmIdentifiableElementCrossReference_1_1_2_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42080:1: ( ruleIdOrSuper )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42081:1: ruleIdOrSuper
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXMemberFeatureCallAccess().getFeatureJvmIdentifiableElementIdOrSuperParserRuleCall_1_1_2_0_1());
}
pushFollow(FollowSets003.FOLLOW_ruleIdOrSuper_in_rule__XMemberFeatureCall__FeatureAssignment_1_1_284613);
ruleIdOrSuper();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXMemberFeatureCallAccess().getFeatureJvmIdentifiableElementIdOrSuperParserRuleCall_1_1_2_0_1());
}
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXMemberFeatureCallAccess().getFeatureJvmIdentifiableElementCrossReference_1_1_2_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XMemberFeatureCall__FeatureAssignment_1_1_2"
// $ANTLR start "rule__XMemberFeatureCall__ExplicitOperationCallAssignment_1_1_3_0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42092:1: rule__XMemberFeatureCall__ExplicitOperationCallAssignment_1_1_3_0 : ( ( '(' ) ) ;
public final void rule__XMemberFeatureCall__ExplicitOperationCallAssignment_1_1_3_0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42096:1: ( ( ( '(' ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42097:1: ( ( '(' ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42097:1: ( ( '(' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42098:1: ( '(' )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXMemberFeatureCallAccess().getExplicitOperationCallLeftParenthesisKeyword_1_1_3_0_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42099:1: ( '(' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42100:1: '('
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXMemberFeatureCallAccess().getExplicitOperationCallLeftParenthesisKeyword_1_1_3_0_0());
}
match(input,144,FollowSets003.FOLLOW_144_in_rule__XMemberFeatureCall__ExplicitOperationCallAssignment_1_1_3_084653); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXMemberFeatureCallAccess().getExplicitOperationCallLeftParenthesisKeyword_1_1_3_0_0());
}
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXMemberFeatureCallAccess().getExplicitOperationCallLeftParenthesisKeyword_1_1_3_0_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XMemberFeatureCall__ExplicitOperationCallAssignment_1_1_3_0"
// $ANTLR start "rule__XMemberFeatureCall__MemberCallArgumentsAssignment_1_1_3_1_0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42115:1: rule__XMemberFeatureCall__MemberCallArgumentsAssignment_1_1_3_1_0 : ( ruleXShortClosure ) ;
public final void rule__XMemberFeatureCall__MemberCallArgumentsAssignment_1_1_3_1_0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42119:1: ( ( ruleXShortClosure ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42120:1: ( ruleXShortClosure )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42120:1: ( ruleXShortClosure )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42121:1: ruleXShortClosure
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXMemberFeatureCallAccess().getMemberCallArgumentsXShortClosureParserRuleCall_1_1_3_1_0_0());
}
pushFollow(FollowSets003.FOLLOW_ruleXShortClosure_in_rule__XMemberFeatureCall__MemberCallArgumentsAssignment_1_1_3_1_084692);
ruleXShortClosure();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXMemberFeatureCallAccess().getMemberCallArgumentsXShortClosureParserRuleCall_1_1_3_1_0_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XMemberFeatureCall__MemberCallArgumentsAssignment_1_1_3_1_0"
// $ANTLR start "rule__XMemberFeatureCall__MemberCallArgumentsAssignment_1_1_3_1_1_0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42130:1: rule__XMemberFeatureCall__MemberCallArgumentsAssignment_1_1_3_1_1_0 : ( ruleXExpression ) ;
public final void rule__XMemberFeatureCall__MemberCallArgumentsAssignment_1_1_3_1_1_0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42134:1: ( ( ruleXExpression ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42135:1: ( ruleXExpression )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42135:1: ( ruleXExpression )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42136:1: ruleXExpression
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXMemberFeatureCallAccess().getMemberCallArgumentsXExpressionParserRuleCall_1_1_3_1_1_0_0());
}
pushFollow(FollowSets003.FOLLOW_ruleXExpression_in_rule__XMemberFeatureCall__MemberCallArgumentsAssignment_1_1_3_1_1_084723);
ruleXExpression();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXMemberFeatureCallAccess().getMemberCallArgumentsXExpressionParserRuleCall_1_1_3_1_1_0_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XMemberFeatureCall__MemberCallArgumentsAssignment_1_1_3_1_1_0"
// $ANTLR start "rule__XMemberFeatureCall__MemberCallArgumentsAssignment_1_1_3_1_1_1_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42145:1: rule__XMemberFeatureCall__MemberCallArgumentsAssignment_1_1_3_1_1_1_1 : ( ruleXExpression ) ;
public final void rule__XMemberFeatureCall__MemberCallArgumentsAssignment_1_1_3_1_1_1_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42149:1: ( ( ruleXExpression ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42150:1: ( ruleXExpression )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42150:1: ( ruleXExpression )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42151:1: ruleXExpression
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXMemberFeatureCallAccess().getMemberCallArgumentsXExpressionParserRuleCall_1_1_3_1_1_1_1_0());
}
pushFollow(FollowSets003.FOLLOW_ruleXExpression_in_rule__XMemberFeatureCall__MemberCallArgumentsAssignment_1_1_3_1_1_1_184754);
ruleXExpression();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXMemberFeatureCallAccess().getMemberCallArgumentsXExpressionParserRuleCall_1_1_3_1_1_1_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XMemberFeatureCall__MemberCallArgumentsAssignment_1_1_3_1_1_1_1"
// $ANTLR start "rule__XMemberFeatureCall__MemberCallArgumentsAssignment_1_1_4"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42160:1: rule__XMemberFeatureCall__MemberCallArgumentsAssignment_1_1_4 : ( ruleXClosure ) ;
public final void rule__XMemberFeatureCall__MemberCallArgumentsAssignment_1_1_4() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42164:1: ( ( ruleXClosure ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42165:1: ( ruleXClosure )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42165:1: ( ruleXClosure )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42166:1: ruleXClosure
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXMemberFeatureCallAccess().getMemberCallArgumentsXClosureParserRuleCall_1_1_4_0());
}
pushFollow(FollowSets003.FOLLOW_ruleXClosure_in_rule__XMemberFeatureCall__MemberCallArgumentsAssignment_1_1_484785);
ruleXClosure();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXMemberFeatureCallAccess().getMemberCallArgumentsXClosureParserRuleCall_1_1_4_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XMemberFeatureCall__MemberCallArgumentsAssignment_1_1_4"
// $ANTLR start "rule__XSetLiteral__ElementsAssignment_3_0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42175:1: rule__XSetLiteral__ElementsAssignment_3_0 : ( ruleXExpression ) ;
public final void rule__XSetLiteral__ElementsAssignment_3_0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42179:1: ( ( ruleXExpression ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42180:1: ( ruleXExpression )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42180:1: ( ruleXExpression )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42181:1: ruleXExpression
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXSetLiteralAccess().getElementsXExpressionParserRuleCall_3_0_0());
}
pushFollow(FollowSets003.FOLLOW_ruleXExpression_in_rule__XSetLiteral__ElementsAssignment_3_084816);
ruleXExpression();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXSetLiteralAccess().getElementsXExpressionParserRuleCall_3_0_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XSetLiteral__ElementsAssignment_3_0"
// $ANTLR start "rule__XSetLiteral__ElementsAssignment_3_1_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42190:1: rule__XSetLiteral__ElementsAssignment_3_1_1 : ( ruleXExpression ) ;
public final void rule__XSetLiteral__ElementsAssignment_3_1_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42194:1: ( ( ruleXExpression ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42195:1: ( ruleXExpression )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42195:1: ( ruleXExpression )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42196:1: ruleXExpression
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXSetLiteralAccess().getElementsXExpressionParserRuleCall_3_1_1_0());
}
pushFollow(FollowSets003.FOLLOW_ruleXExpression_in_rule__XSetLiteral__ElementsAssignment_3_1_184847);
ruleXExpression();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXSetLiteralAccess().getElementsXExpressionParserRuleCall_3_1_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XSetLiteral__ElementsAssignment_3_1_1"
// $ANTLR start "rule__XListLiteral__ElementsAssignment_3_0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42205:1: rule__XListLiteral__ElementsAssignment_3_0 : ( ruleXExpression ) ;
public final void rule__XListLiteral__ElementsAssignment_3_0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42209:1: ( ( ruleXExpression ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42210:1: ( ruleXExpression )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42210:1: ( ruleXExpression )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42211:1: ruleXExpression
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXListLiteralAccess().getElementsXExpressionParserRuleCall_3_0_0());
}
pushFollow(FollowSets003.FOLLOW_ruleXExpression_in_rule__XListLiteral__ElementsAssignment_3_084878);
ruleXExpression();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXListLiteralAccess().getElementsXExpressionParserRuleCall_3_0_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XListLiteral__ElementsAssignment_3_0"
// $ANTLR start "rule__XListLiteral__ElementsAssignment_3_1_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42220:1: rule__XListLiteral__ElementsAssignment_3_1_1 : ( ruleXExpression ) ;
public final void rule__XListLiteral__ElementsAssignment_3_1_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42224:1: ( ( ruleXExpression ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42225:1: ( ruleXExpression )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42225:1: ( ruleXExpression )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42226:1: ruleXExpression
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXListLiteralAccess().getElementsXExpressionParserRuleCall_3_1_1_0());
}
pushFollow(FollowSets003.FOLLOW_ruleXExpression_in_rule__XListLiteral__ElementsAssignment_3_1_184909);
ruleXExpression();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXListLiteralAccess().getElementsXExpressionParserRuleCall_3_1_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XListLiteral__ElementsAssignment_3_1_1"
// $ANTLR start "rule__XClosure__DeclaredFormalParametersAssignment_1_0_0_0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42235:1: rule__XClosure__DeclaredFormalParametersAssignment_1_0_0_0 : ( ruleJvmFormalParameter ) ;
public final void rule__XClosure__DeclaredFormalParametersAssignment_1_0_0_0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42239:1: ( ( ruleJvmFormalParameter ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42240:1: ( ruleJvmFormalParameter )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42240:1: ( ruleJvmFormalParameter )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42241:1: ruleJvmFormalParameter
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXClosureAccess().getDeclaredFormalParametersJvmFormalParameterParserRuleCall_1_0_0_0_0());
}
pushFollow(FollowSets003.FOLLOW_ruleJvmFormalParameter_in_rule__XClosure__DeclaredFormalParametersAssignment_1_0_0_084940);
ruleJvmFormalParameter();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXClosureAccess().getDeclaredFormalParametersJvmFormalParameterParserRuleCall_1_0_0_0_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XClosure__DeclaredFormalParametersAssignment_1_0_0_0"
// $ANTLR start "rule__XClosure__DeclaredFormalParametersAssignment_1_0_0_1_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42250:1: rule__XClosure__DeclaredFormalParametersAssignment_1_0_0_1_1 : ( ruleJvmFormalParameter ) ;
public final void rule__XClosure__DeclaredFormalParametersAssignment_1_0_0_1_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42254:1: ( ( ruleJvmFormalParameter ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42255:1: ( ruleJvmFormalParameter )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42255:1: ( ruleJvmFormalParameter )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42256:1: ruleJvmFormalParameter
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXClosureAccess().getDeclaredFormalParametersJvmFormalParameterParserRuleCall_1_0_0_1_1_0());
}
pushFollow(FollowSets003.FOLLOW_ruleJvmFormalParameter_in_rule__XClosure__DeclaredFormalParametersAssignment_1_0_0_1_184971);
ruleJvmFormalParameter();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXClosureAccess().getDeclaredFormalParametersJvmFormalParameterParserRuleCall_1_0_0_1_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XClosure__DeclaredFormalParametersAssignment_1_0_0_1_1"
// $ANTLR start "rule__XClosure__ExplicitSyntaxAssignment_1_0_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42265:1: rule__XClosure__ExplicitSyntaxAssignment_1_0_1 : ( ( '|' ) ) ;
public final void rule__XClosure__ExplicitSyntaxAssignment_1_0_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42269:1: ( ( ( '|' ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42270:1: ( ( '|' ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42270:1: ( ( '|' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42271:1: ( '|' )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXClosureAccess().getExplicitSyntaxVerticalLineKeyword_1_0_1_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42272:1: ( '|' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42273:1: '|'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXClosureAccess().getExplicitSyntaxVerticalLineKeyword_1_0_1_0());
}
match(input,183,FollowSets003.FOLLOW_183_in_rule__XClosure__ExplicitSyntaxAssignment_1_0_185007); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXClosureAccess().getExplicitSyntaxVerticalLineKeyword_1_0_1_0());
}
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXClosureAccess().getExplicitSyntaxVerticalLineKeyword_1_0_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XClosure__ExplicitSyntaxAssignment_1_0_1"
// $ANTLR start "rule__XClosure__ExpressionAssignment_2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42288:1: rule__XClosure__ExpressionAssignment_2 : ( ruleXExpressionInClosure ) ;
public final void rule__XClosure__ExpressionAssignment_2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42292:1: ( ( ruleXExpressionInClosure ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42293:1: ( ruleXExpressionInClosure )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42293:1: ( ruleXExpressionInClosure )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42294:1: ruleXExpressionInClosure
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXClosureAccess().getExpressionXExpressionInClosureParserRuleCall_2_0());
}
pushFollow(FollowSets003.FOLLOW_ruleXExpressionInClosure_in_rule__XClosure__ExpressionAssignment_285046);
ruleXExpressionInClosure();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXClosureAccess().getExpressionXExpressionInClosureParserRuleCall_2_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XClosure__ExpressionAssignment_2"
// $ANTLR start "rule__XExpressionInClosure__ExpressionsAssignment_1_0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42303:1: rule__XExpressionInClosure__ExpressionsAssignment_1_0 : ( ruleXExpressionOrVarDeclaration ) ;
public final void rule__XExpressionInClosure__ExpressionsAssignment_1_0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42307:1: ( ( ruleXExpressionOrVarDeclaration ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42308:1: ( ruleXExpressionOrVarDeclaration )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42308:1: ( ruleXExpressionOrVarDeclaration )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42309:1: ruleXExpressionOrVarDeclaration
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXExpressionInClosureAccess().getExpressionsXExpressionOrVarDeclarationParserRuleCall_1_0_0());
}
pushFollow(FollowSets003.FOLLOW_ruleXExpressionOrVarDeclaration_in_rule__XExpressionInClosure__ExpressionsAssignment_1_085077);
ruleXExpressionOrVarDeclaration();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXExpressionInClosureAccess().getExpressionsXExpressionOrVarDeclarationParserRuleCall_1_0_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XExpressionInClosure__ExpressionsAssignment_1_0"
// $ANTLR start "rule__XShortClosure__DeclaredFormalParametersAssignment_0_0_1_0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42318:1: rule__XShortClosure__DeclaredFormalParametersAssignment_0_0_1_0 : ( ruleJvmFormalParameter ) ;
public final void rule__XShortClosure__DeclaredFormalParametersAssignment_0_0_1_0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42322:1: ( ( ruleJvmFormalParameter ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42323:1: ( ruleJvmFormalParameter )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42323:1: ( ruleJvmFormalParameter )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42324:1: ruleJvmFormalParameter
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXShortClosureAccess().getDeclaredFormalParametersJvmFormalParameterParserRuleCall_0_0_1_0_0());
}
pushFollow(FollowSets003.FOLLOW_ruleJvmFormalParameter_in_rule__XShortClosure__DeclaredFormalParametersAssignment_0_0_1_085108);
ruleJvmFormalParameter();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXShortClosureAccess().getDeclaredFormalParametersJvmFormalParameterParserRuleCall_0_0_1_0_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XShortClosure__DeclaredFormalParametersAssignment_0_0_1_0"
// $ANTLR start "rule__XShortClosure__DeclaredFormalParametersAssignment_0_0_1_1_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42333:1: rule__XShortClosure__DeclaredFormalParametersAssignment_0_0_1_1_1 : ( ruleJvmFormalParameter ) ;
public final void rule__XShortClosure__DeclaredFormalParametersAssignment_0_0_1_1_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42337:1: ( ( ruleJvmFormalParameter ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42338:1: ( ruleJvmFormalParameter )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42338:1: ( ruleJvmFormalParameter )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42339:1: ruleJvmFormalParameter
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXShortClosureAccess().getDeclaredFormalParametersJvmFormalParameterParserRuleCall_0_0_1_1_1_0());
}
pushFollow(FollowSets003.FOLLOW_ruleJvmFormalParameter_in_rule__XShortClosure__DeclaredFormalParametersAssignment_0_0_1_1_185139);
ruleJvmFormalParameter();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXShortClosureAccess().getDeclaredFormalParametersJvmFormalParameterParserRuleCall_0_0_1_1_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XShortClosure__DeclaredFormalParametersAssignment_0_0_1_1_1"
// $ANTLR start "rule__XShortClosure__ExplicitSyntaxAssignment_0_0_2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42348:1: rule__XShortClosure__ExplicitSyntaxAssignment_0_0_2 : ( ( '|' ) ) ;
public final void rule__XShortClosure__ExplicitSyntaxAssignment_0_0_2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42352:1: ( ( ( '|' ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42353:1: ( ( '|' ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42353:1: ( ( '|' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42354:1: ( '|' )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXShortClosureAccess().getExplicitSyntaxVerticalLineKeyword_0_0_2_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42355:1: ( '|' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42356:1: '|'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXShortClosureAccess().getExplicitSyntaxVerticalLineKeyword_0_0_2_0());
}
match(input,183,FollowSets003.FOLLOW_183_in_rule__XShortClosure__ExplicitSyntaxAssignment_0_0_285175); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXShortClosureAccess().getExplicitSyntaxVerticalLineKeyword_0_0_2_0());
}
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXShortClosureAccess().getExplicitSyntaxVerticalLineKeyword_0_0_2_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XShortClosure__ExplicitSyntaxAssignment_0_0_2"
// $ANTLR start "rule__XShortClosure__ExpressionAssignment_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42371:1: rule__XShortClosure__ExpressionAssignment_1 : ( ruleXExpression ) ;
public final void rule__XShortClosure__ExpressionAssignment_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42375:1: ( ( ruleXExpression ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42376:1: ( ruleXExpression )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42376:1: ( ruleXExpression )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42377:1: ruleXExpression
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXShortClosureAccess().getExpressionXExpressionParserRuleCall_1_0());
}
pushFollow(FollowSets003.FOLLOW_ruleXExpression_in_rule__XShortClosure__ExpressionAssignment_185214);
ruleXExpression();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXShortClosureAccess().getExpressionXExpressionParserRuleCall_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XShortClosure__ExpressionAssignment_1"
// $ANTLR start "rule__XIfExpression__IfAssignment_3"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42386:1: rule__XIfExpression__IfAssignment_3 : ( ruleXExpression ) ;
public final void rule__XIfExpression__IfAssignment_3() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42390:1: ( ( ruleXExpression ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42391:1: ( ruleXExpression )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42391:1: ( ruleXExpression )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42392:1: ruleXExpression
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXIfExpressionAccess().getIfXExpressionParserRuleCall_3_0());
}
pushFollow(FollowSets003.FOLLOW_ruleXExpression_in_rule__XIfExpression__IfAssignment_385245);
ruleXExpression();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXIfExpressionAccess().getIfXExpressionParserRuleCall_3_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XIfExpression__IfAssignment_3"
// $ANTLR start "rule__XIfExpression__ThenAssignment_5"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42401:1: rule__XIfExpression__ThenAssignment_5 : ( ruleXExpression ) ;
public final void rule__XIfExpression__ThenAssignment_5() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42405:1: ( ( ruleXExpression ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42406:1: ( ruleXExpression )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42406:1: ( ruleXExpression )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42407:1: ruleXExpression
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXIfExpressionAccess().getThenXExpressionParserRuleCall_5_0());
}
pushFollow(FollowSets003.FOLLOW_ruleXExpression_in_rule__XIfExpression__ThenAssignment_585276);
ruleXExpression();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXIfExpressionAccess().getThenXExpressionParserRuleCall_5_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XIfExpression__ThenAssignment_5"
// $ANTLR start "rule__XIfExpression__ElseAssignment_6_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42416:1: rule__XIfExpression__ElseAssignment_6_1 : ( ruleXExpression ) ;
public final void rule__XIfExpression__ElseAssignment_6_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42420:1: ( ( ruleXExpression ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42421:1: ( ruleXExpression )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42421:1: ( ruleXExpression )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42422:1: ruleXExpression
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXIfExpressionAccess().getElseXExpressionParserRuleCall_6_1_0());
}
pushFollow(FollowSets003.FOLLOW_ruleXExpression_in_rule__XIfExpression__ElseAssignment_6_185307);
ruleXExpression();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXIfExpressionAccess().getElseXExpressionParserRuleCall_6_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XIfExpression__ElseAssignment_6_1"
// $ANTLR start "rule__XSwitchExpression__DeclaredParamAssignment_2_0_0_0_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42431:1: rule__XSwitchExpression__DeclaredParamAssignment_2_0_0_0_1 : ( ruleJvmFormalParameter ) ;
public final void rule__XSwitchExpression__DeclaredParamAssignment_2_0_0_0_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42435:1: ( ( ruleJvmFormalParameter ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42436:1: ( ruleJvmFormalParameter )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42436:1: ( ruleJvmFormalParameter )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42437:1: ruleJvmFormalParameter
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXSwitchExpressionAccess().getDeclaredParamJvmFormalParameterParserRuleCall_2_0_0_0_1_0());
}
pushFollow(FollowSets003.FOLLOW_ruleJvmFormalParameter_in_rule__XSwitchExpression__DeclaredParamAssignment_2_0_0_0_185338);
ruleJvmFormalParameter();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXSwitchExpressionAccess().getDeclaredParamJvmFormalParameterParserRuleCall_2_0_0_0_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XSwitchExpression__DeclaredParamAssignment_2_0_0_0_1"
// $ANTLR start "rule__XSwitchExpression__SwitchAssignment_2_0_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42446:1: rule__XSwitchExpression__SwitchAssignment_2_0_1 : ( ruleXExpression ) ;
public final void rule__XSwitchExpression__SwitchAssignment_2_0_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42450:1: ( ( ruleXExpression ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42451:1: ( ruleXExpression )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42451:1: ( ruleXExpression )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42452:1: ruleXExpression
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXSwitchExpressionAccess().getSwitchXExpressionParserRuleCall_2_0_1_0());
}
pushFollow(FollowSets003.FOLLOW_ruleXExpression_in_rule__XSwitchExpression__SwitchAssignment_2_0_185369);
ruleXExpression();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXSwitchExpressionAccess().getSwitchXExpressionParserRuleCall_2_0_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XSwitchExpression__SwitchAssignment_2_0_1"
// $ANTLR start "rule__XSwitchExpression__DeclaredParamAssignment_2_1_0_0_0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42461:1: rule__XSwitchExpression__DeclaredParamAssignment_2_1_0_0_0 : ( ruleJvmFormalParameter ) ;
public final void rule__XSwitchExpression__DeclaredParamAssignment_2_1_0_0_0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42465:1: ( ( ruleJvmFormalParameter ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42466:1: ( ruleJvmFormalParameter )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42466:1: ( ruleJvmFormalParameter )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42467:1: ruleJvmFormalParameter
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXSwitchExpressionAccess().getDeclaredParamJvmFormalParameterParserRuleCall_2_1_0_0_0_0());
}
pushFollow(FollowSets003.FOLLOW_ruleJvmFormalParameter_in_rule__XSwitchExpression__DeclaredParamAssignment_2_1_0_0_085400);
ruleJvmFormalParameter();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXSwitchExpressionAccess().getDeclaredParamJvmFormalParameterParserRuleCall_2_1_0_0_0_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XSwitchExpression__DeclaredParamAssignment_2_1_0_0_0"
// $ANTLR start "rule__XSwitchExpression__SwitchAssignment_2_1_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42476:1: rule__XSwitchExpression__SwitchAssignment_2_1_1 : ( ruleXExpression ) ;
public final void rule__XSwitchExpression__SwitchAssignment_2_1_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42480:1: ( ( ruleXExpression ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42481:1: ( ruleXExpression )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42481:1: ( ruleXExpression )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42482:1: ruleXExpression
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXSwitchExpressionAccess().getSwitchXExpressionParserRuleCall_2_1_1_0());
}
pushFollow(FollowSets003.FOLLOW_ruleXExpression_in_rule__XSwitchExpression__SwitchAssignment_2_1_185431);
ruleXExpression();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXSwitchExpressionAccess().getSwitchXExpressionParserRuleCall_2_1_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XSwitchExpression__SwitchAssignment_2_1_1"
// $ANTLR start "rule__XSwitchExpression__CasesAssignment_4"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42491:1: rule__XSwitchExpression__CasesAssignment_4 : ( ruleXCasePart ) ;
public final void rule__XSwitchExpression__CasesAssignment_4() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42495:1: ( ( ruleXCasePart ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42496:1: ( ruleXCasePart )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42496:1: ( ruleXCasePart )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42497:1: ruleXCasePart
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXSwitchExpressionAccess().getCasesXCasePartParserRuleCall_4_0());
}
pushFollow(FollowSets003.FOLLOW_ruleXCasePart_in_rule__XSwitchExpression__CasesAssignment_485462);
ruleXCasePart();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXSwitchExpressionAccess().getCasesXCasePartParserRuleCall_4_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XSwitchExpression__CasesAssignment_4"
// $ANTLR start "rule__XSwitchExpression__DefaultAssignment_5_2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42506:1: rule__XSwitchExpression__DefaultAssignment_5_2 : ( ruleXExpression ) ;
public final void rule__XSwitchExpression__DefaultAssignment_5_2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42510:1: ( ( ruleXExpression ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42511:1: ( ruleXExpression )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42511:1: ( ruleXExpression )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42512:1: ruleXExpression
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXSwitchExpressionAccess().getDefaultXExpressionParserRuleCall_5_2_0());
}
pushFollow(FollowSets003.FOLLOW_ruleXExpression_in_rule__XSwitchExpression__DefaultAssignment_5_285493);
ruleXExpression();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXSwitchExpressionAccess().getDefaultXExpressionParserRuleCall_5_2_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XSwitchExpression__DefaultAssignment_5_2"
// $ANTLR start "rule__XCasePart__TypeGuardAssignment_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42521:1: rule__XCasePart__TypeGuardAssignment_1 : ( ruleJvmTypeReference ) ;
public final void rule__XCasePart__TypeGuardAssignment_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42525:1: ( ( ruleJvmTypeReference ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42526:1: ( ruleJvmTypeReference )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42526:1: ( ruleJvmTypeReference )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42527:1: ruleJvmTypeReference
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXCasePartAccess().getTypeGuardJvmTypeReferenceParserRuleCall_1_0());
}
pushFollow(FollowSets003.FOLLOW_ruleJvmTypeReference_in_rule__XCasePart__TypeGuardAssignment_185524);
ruleJvmTypeReference();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXCasePartAccess().getTypeGuardJvmTypeReferenceParserRuleCall_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XCasePart__TypeGuardAssignment_1"
// $ANTLR start "rule__XCasePart__CaseAssignment_2_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42536:1: rule__XCasePart__CaseAssignment_2_1 : ( ruleXExpression ) ;
public final void rule__XCasePart__CaseAssignment_2_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42540:1: ( ( ruleXExpression ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42541:1: ( ruleXExpression )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42541:1: ( ruleXExpression )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42542:1: ruleXExpression
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXCasePartAccess().getCaseXExpressionParserRuleCall_2_1_0());
}
pushFollow(FollowSets003.FOLLOW_ruleXExpression_in_rule__XCasePart__CaseAssignment_2_185555);
ruleXExpression();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXCasePartAccess().getCaseXExpressionParserRuleCall_2_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XCasePart__CaseAssignment_2_1"
// $ANTLR start "rule__XCasePart__ThenAssignment_3_0_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42551:1: rule__XCasePart__ThenAssignment_3_0_1 : ( ruleXExpression ) ;
public final void rule__XCasePart__ThenAssignment_3_0_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42555:1: ( ( ruleXExpression ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42556:1: ( ruleXExpression )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42556:1: ( ruleXExpression )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42557:1: ruleXExpression
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXCasePartAccess().getThenXExpressionParserRuleCall_3_0_1_0());
}
pushFollow(FollowSets003.FOLLOW_ruleXExpression_in_rule__XCasePart__ThenAssignment_3_0_185586);
ruleXExpression();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXCasePartAccess().getThenXExpressionParserRuleCall_3_0_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XCasePart__ThenAssignment_3_0_1"
// $ANTLR start "rule__XCasePart__FallThroughAssignment_3_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42566:1: rule__XCasePart__FallThroughAssignment_3_1 : ( ( ',' ) ) ;
public final void rule__XCasePart__FallThroughAssignment_3_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42570:1: ( ( ( ',' ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42571:1: ( ( ',' ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42571:1: ( ( ',' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42572:1: ( ',' )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXCasePartAccess().getFallThroughCommaKeyword_3_1_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42573:1: ( ',' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42574:1: ','
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXCasePartAccess().getFallThroughCommaKeyword_3_1_0());
}
match(input,154,FollowSets003.FOLLOW_154_in_rule__XCasePart__FallThroughAssignment_3_185622); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXCasePartAccess().getFallThroughCommaKeyword_3_1_0());
}
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXCasePartAccess().getFallThroughCommaKeyword_3_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XCasePart__FallThroughAssignment_3_1"
// $ANTLR start "rule__XForLoopExpression__DeclaredParamAssignment_0_0_3"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42589:1: rule__XForLoopExpression__DeclaredParamAssignment_0_0_3 : ( ruleJvmFormalParameter ) ;
public final void rule__XForLoopExpression__DeclaredParamAssignment_0_0_3() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42593:1: ( ( ruleJvmFormalParameter ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42594:1: ( ruleJvmFormalParameter )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42594:1: ( ruleJvmFormalParameter )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42595:1: ruleJvmFormalParameter
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXForLoopExpressionAccess().getDeclaredParamJvmFormalParameterParserRuleCall_0_0_3_0());
}
pushFollow(FollowSets003.FOLLOW_ruleJvmFormalParameter_in_rule__XForLoopExpression__DeclaredParamAssignment_0_0_385661);
ruleJvmFormalParameter();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXForLoopExpressionAccess().getDeclaredParamJvmFormalParameterParserRuleCall_0_0_3_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XForLoopExpression__DeclaredParamAssignment_0_0_3"
// $ANTLR start "rule__XForLoopExpression__ForExpressionAssignment_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42604:1: rule__XForLoopExpression__ForExpressionAssignment_1 : ( ruleXExpression ) ;
public final void rule__XForLoopExpression__ForExpressionAssignment_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42608:1: ( ( ruleXExpression ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42609:1: ( ruleXExpression )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42609:1: ( ruleXExpression )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42610:1: ruleXExpression
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXForLoopExpressionAccess().getForExpressionXExpressionParserRuleCall_1_0());
}
pushFollow(FollowSets003.FOLLOW_ruleXExpression_in_rule__XForLoopExpression__ForExpressionAssignment_185692);
ruleXExpression();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXForLoopExpressionAccess().getForExpressionXExpressionParserRuleCall_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XForLoopExpression__ForExpressionAssignment_1"
// $ANTLR start "rule__XForLoopExpression__EachExpressionAssignment_3"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42619:1: rule__XForLoopExpression__EachExpressionAssignment_3 : ( ruleXExpression ) ;
public final void rule__XForLoopExpression__EachExpressionAssignment_3() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42623:1: ( ( ruleXExpression ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42624:1: ( ruleXExpression )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42624:1: ( ruleXExpression )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42625:1: ruleXExpression
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXForLoopExpressionAccess().getEachExpressionXExpressionParserRuleCall_3_0());
}
pushFollow(FollowSets003.FOLLOW_ruleXExpression_in_rule__XForLoopExpression__EachExpressionAssignment_385723);
ruleXExpression();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXForLoopExpressionAccess().getEachExpressionXExpressionParserRuleCall_3_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XForLoopExpression__EachExpressionAssignment_3"
// $ANTLR start "rule__XBasicForLoopExpression__InitExpressionsAssignment_3_0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42634:1: rule__XBasicForLoopExpression__InitExpressionsAssignment_3_0 : ( ruleXExpressionOrVarDeclaration ) ;
public final void rule__XBasicForLoopExpression__InitExpressionsAssignment_3_0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42638:1: ( ( ruleXExpressionOrVarDeclaration ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42639:1: ( ruleXExpressionOrVarDeclaration )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42639:1: ( ruleXExpressionOrVarDeclaration )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42640:1: ruleXExpressionOrVarDeclaration
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXBasicForLoopExpressionAccess().getInitExpressionsXExpressionOrVarDeclarationParserRuleCall_3_0_0());
}
pushFollow(FollowSets003.FOLLOW_ruleXExpressionOrVarDeclaration_in_rule__XBasicForLoopExpression__InitExpressionsAssignment_3_085754);
ruleXExpressionOrVarDeclaration();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXBasicForLoopExpressionAccess().getInitExpressionsXExpressionOrVarDeclarationParserRuleCall_3_0_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XBasicForLoopExpression__InitExpressionsAssignment_3_0"
// $ANTLR start "rule__XBasicForLoopExpression__InitExpressionsAssignment_3_1_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42649:1: rule__XBasicForLoopExpression__InitExpressionsAssignment_3_1_1 : ( ruleXExpressionOrVarDeclaration ) ;
public final void rule__XBasicForLoopExpression__InitExpressionsAssignment_3_1_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42653:1: ( ( ruleXExpressionOrVarDeclaration ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42654:1: ( ruleXExpressionOrVarDeclaration )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42654:1: ( ruleXExpressionOrVarDeclaration )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42655:1: ruleXExpressionOrVarDeclaration
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXBasicForLoopExpressionAccess().getInitExpressionsXExpressionOrVarDeclarationParserRuleCall_3_1_1_0());
}
pushFollow(FollowSets003.FOLLOW_ruleXExpressionOrVarDeclaration_in_rule__XBasicForLoopExpression__InitExpressionsAssignment_3_1_185785);
ruleXExpressionOrVarDeclaration();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXBasicForLoopExpressionAccess().getInitExpressionsXExpressionOrVarDeclarationParserRuleCall_3_1_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XBasicForLoopExpression__InitExpressionsAssignment_3_1_1"
// $ANTLR start "rule__XBasicForLoopExpression__ExpressionAssignment_5"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42664:1: rule__XBasicForLoopExpression__ExpressionAssignment_5 : ( ruleXExpression ) ;
public final void rule__XBasicForLoopExpression__ExpressionAssignment_5() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42668:1: ( ( ruleXExpression ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42669:1: ( ruleXExpression )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42669:1: ( ruleXExpression )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42670:1: ruleXExpression
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXBasicForLoopExpressionAccess().getExpressionXExpressionParserRuleCall_5_0());
}
pushFollow(FollowSets003.FOLLOW_ruleXExpression_in_rule__XBasicForLoopExpression__ExpressionAssignment_585816);
ruleXExpression();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXBasicForLoopExpressionAccess().getExpressionXExpressionParserRuleCall_5_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XBasicForLoopExpression__ExpressionAssignment_5"
// $ANTLR start "rule__XBasicForLoopExpression__UpdateExpressionsAssignment_7_0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42679:1: rule__XBasicForLoopExpression__UpdateExpressionsAssignment_7_0 : ( ruleXExpression ) ;
public final void rule__XBasicForLoopExpression__UpdateExpressionsAssignment_7_0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42683:1: ( ( ruleXExpression ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42684:1: ( ruleXExpression )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42684:1: ( ruleXExpression )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42685:1: ruleXExpression
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXBasicForLoopExpressionAccess().getUpdateExpressionsXExpressionParserRuleCall_7_0_0());
}
pushFollow(FollowSets003.FOLLOW_ruleXExpression_in_rule__XBasicForLoopExpression__UpdateExpressionsAssignment_7_085847);
ruleXExpression();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXBasicForLoopExpressionAccess().getUpdateExpressionsXExpressionParserRuleCall_7_0_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XBasicForLoopExpression__UpdateExpressionsAssignment_7_0"
// $ANTLR start "rule__XBasicForLoopExpression__UpdateExpressionsAssignment_7_1_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42694:1: rule__XBasicForLoopExpression__UpdateExpressionsAssignment_7_1_1 : ( ruleXExpression ) ;
public final void rule__XBasicForLoopExpression__UpdateExpressionsAssignment_7_1_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42698:1: ( ( ruleXExpression ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42699:1: ( ruleXExpression )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42699:1: ( ruleXExpression )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42700:1: ruleXExpression
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXBasicForLoopExpressionAccess().getUpdateExpressionsXExpressionParserRuleCall_7_1_1_0());
}
pushFollow(FollowSets003.FOLLOW_ruleXExpression_in_rule__XBasicForLoopExpression__UpdateExpressionsAssignment_7_1_185878);
ruleXExpression();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXBasicForLoopExpressionAccess().getUpdateExpressionsXExpressionParserRuleCall_7_1_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XBasicForLoopExpression__UpdateExpressionsAssignment_7_1_1"
// $ANTLR start "rule__XBasicForLoopExpression__EachExpressionAssignment_9"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42709:1: rule__XBasicForLoopExpression__EachExpressionAssignment_9 : ( ruleXExpression ) ;
public final void rule__XBasicForLoopExpression__EachExpressionAssignment_9() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42713:1: ( ( ruleXExpression ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42714:1: ( ruleXExpression )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42714:1: ( ruleXExpression )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42715:1: ruleXExpression
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXBasicForLoopExpressionAccess().getEachExpressionXExpressionParserRuleCall_9_0());
}
pushFollow(FollowSets003.FOLLOW_ruleXExpression_in_rule__XBasicForLoopExpression__EachExpressionAssignment_985909);
ruleXExpression();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXBasicForLoopExpressionAccess().getEachExpressionXExpressionParserRuleCall_9_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XBasicForLoopExpression__EachExpressionAssignment_9"
// $ANTLR start "rule__XWhileExpression__PredicateAssignment_3"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42724:1: rule__XWhileExpression__PredicateAssignment_3 : ( ruleXExpression ) ;
public final void rule__XWhileExpression__PredicateAssignment_3() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42728:1: ( ( ruleXExpression ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42729:1: ( ruleXExpression )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42729:1: ( ruleXExpression )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42730:1: ruleXExpression
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXWhileExpressionAccess().getPredicateXExpressionParserRuleCall_3_0());
}
pushFollow(FollowSets003.FOLLOW_ruleXExpression_in_rule__XWhileExpression__PredicateAssignment_385940);
ruleXExpression();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXWhileExpressionAccess().getPredicateXExpressionParserRuleCall_3_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XWhileExpression__PredicateAssignment_3"
// $ANTLR start "rule__XWhileExpression__BodyAssignment_5"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42739:1: rule__XWhileExpression__BodyAssignment_5 : ( ruleXExpression ) ;
public final void rule__XWhileExpression__BodyAssignment_5() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42743:1: ( ( ruleXExpression ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42744:1: ( ruleXExpression )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42744:1: ( ruleXExpression )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42745:1: ruleXExpression
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXWhileExpressionAccess().getBodyXExpressionParserRuleCall_5_0());
}
pushFollow(FollowSets003.FOLLOW_ruleXExpression_in_rule__XWhileExpression__BodyAssignment_585971);
ruleXExpression();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXWhileExpressionAccess().getBodyXExpressionParserRuleCall_5_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XWhileExpression__BodyAssignment_5"
// $ANTLR start "rule__XDoWhileExpression__BodyAssignment_2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42754:1: rule__XDoWhileExpression__BodyAssignment_2 : ( ruleXExpression ) ;
public final void rule__XDoWhileExpression__BodyAssignment_2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42758:1: ( ( ruleXExpression ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42759:1: ( ruleXExpression )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42759:1: ( ruleXExpression )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42760:1: ruleXExpression
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXDoWhileExpressionAccess().getBodyXExpressionParserRuleCall_2_0());
}
pushFollow(FollowSets003.FOLLOW_ruleXExpression_in_rule__XDoWhileExpression__BodyAssignment_286002);
ruleXExpression();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXDoWhileExpressionAccess().getBodyXExpressionParserRuleCall_2_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XDoWhileExpression__BodyAssignment_2"
// $ANTLR start "rule__XDoWhileExpression__PredicateAssignment_5"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42769:1: rule__XDoWhileExpression__PredicateAssignment_5 : ( ruleXExpression ) ;
public final void rule__XDoWhileExpression__PredicateAssignment_5() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42773:1: ( ( ruleXExpression ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42774:1: ( ruleXExpression )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42774:1: ( ruleXExpression )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42775:1: ruleXExpression
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXDoWhileExpressionAccess().getPredicateXExpressionParserRuleCall_5_0());
}
pushFollow(FollowSets003.FOLLOW_ruleXExpression_in_rule__XDoWhileExpression__PredicateAssignment_586033);
ruleXExpression();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXDoWhileExpressionAccess().getPredicateXExpressionParserRuleCall_5_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XDoWhileExpression__PredicateAssignment_5"
// $ANTLR start "rule__XBlockExpression__ExpressionsAssignment_2_0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42784:1: rule__XBlockExpression__ExpressionsAssignment_2_0 : ( ruleXExpressionOrVarDeclaration ) ;
public final void rule__XBlockExpression__ExpressionsAssignment_2_0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42788:1: ( ( ruleXExpressionOrVarDeclaration ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42789:1: ( ruleXExpressionOrVarDeclaration )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42789:1: ( ruleXExpressionOrVarDeclaration )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42790:1: ruleXExpressionOrVarDeclaration
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXBlockExpressionAccess().getExpressionsXExpressionOrVarDeclarationParserRuleCall_2_0_0());
}
pushFollow(FollowSets003.FOLLOW_ruleXExpressionOrVarDeclaration_in_rule__XBlockExpression__ExpressionsAssignment_2_086064);
ruleXExpressionOrVarDeclaration();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXBlockExpressionAccess().getExpressionsXExpressionOrVarDeclarationParserRuleCall_2_0_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XBlockExpression__ExpressionsAssignment_2_0"
// $ANTLR start "rule__XVariableDeclaration__WriteableAssignment_1_0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42799:1: rule__XVariableDeclaration__WriteableAssignment_1_0 : ( ( 'var' ) ) ;
public final void rule__XVariableDeclaration__WriteableAssignment_1_0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42803:1: ( ( ( 'var' ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42804:1: ( ( 'var' ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42804:1: ( ( 'var' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42805:1: ( 'var' )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXVariableDeclarationAccess().getWriteableVarKeyword_1_0_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42806:1: ( 'var' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42807:1: 'var'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXVariableDeclarationAccess().getWriteableVarKeyword_1_0_0());
}
match(input,184,FollowSets003.FOLLOW_184_in_rule__XVariableDeclaration__WriteableAssignment_1_086100); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXVariableDeclarationAccess().getWriteableVarKeyword_1_0_0());
}
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXVariableDeclarationAccess().getWriteableVarKeyword_1_0_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XVariableDeclaration__WriteableAssignment_1_0"
// $ANTLR start "rule__XVariableDeclaration__TypeAssignment_2_0_0_0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42822:1: rule__XVariableDeclaration__TypeAssignment_2_0_0_0 : ( ruleJvmTypeReference ) ;
public final void rule__XVariableDeclaration__TypeAssignment_2_0_0_0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42826:1: ( ( ruleJvmTypeReference ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42827:1: ( ruleJvmTypeReference )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42827:1: ( ruleJvmTypeReference )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42828:1: ruleJvmTypeReference
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXVariableDeclarationAccess().getTypeJvmTypeReferenceParserRuleCall_2_0_0_0_0());
}
pushFollow(FollowSets003.FOLLOW_ruleJvmTypeReference_in_rule__XVariableDeclaration__TypeAssignment_2_0_0_086139);
ruleJvmTypeReference();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXVariableDeclarationAccess().getTypeJvmTypeReferenceParserRuleCall_2_0_0_0_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XVariableDeclaration__TypeAssignment_2_0_0_0"
// $ANTLR start "rule__XVariableDeclaration__NameAssignment_2_0_0_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42837:1: rule__XVariableDeclaration__NameAssignment_2_0_0_1 : ( ruleValidID ) ;
public final void rule__XVariableDeclaration__NameAssignment_2_0_0_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42841:1: ( ( ruleValidID ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42842:1: ( ruleValidID )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42842:1: ( ruleValidID )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42843:1: ruleValidID
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXVariableDeclarationAccess().getNameValidIDParserRuleCall_2_0_0_1_0());
}
pushFollow(FollowSets003.FOLLOW_ruleValidID_in_rule__XVariableDeclaration__NameAssignment_2_0_0_186170);
ruleValidID();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXVariableDeclarationAccess().getNameValidIDParserRuleCall_2_0_0_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XVariableDeclaration__NameAssignment_2_0_0_1"
// $ANTLR start "rule__XVariableDeclaration__NameAssignment_2_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42852:1: rule__XVariableDeclaration__NameAssignment_2_1 : ( ruleValidID ) ;
public final void rule__XVariableDeclaration__NameAssignment_2_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42856:1: ( ( ruleValidID ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42857:1: ( ruleValidID )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42857:1: ( ruleValidID )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42858:1: ruleValidID
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXVariableDeclarationAccess().getNameValidIDParserRuleCall_2_1_0());
}
pushFollow(FollowSets003.FOLLOW_ruleValidID_in_rule__XVariableDeclaration__NameAssignment_2_186201);
ruleValidID();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXVariableDeclarationAccess().getNameValidIDParserRuleCall_2_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XVariableDeclaration__NameAssignment_2_1"
// $ANTLR start "rule__XVariableDeclaration__RightAssignment_3_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42867:1: rule__XVariableDeclaration__RightAssignment_3_1 : ( ruleXExpression ) ;
public final void rule__XVariableDeclaration__RightAssignment_3_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42871:1: ( ( ruleXExpression ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42872:1: ( ruleXExpression )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42872:1: ( ruleXExpression )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42873:1: ruleXExpression
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXVariableDeclarationAccess().getRightXExpressionParserRuleCall_3_1_0());
}
pushFollow(FollowSets003.FOLLOW_ruleXExpression_in_rule__XVariableDeclaration__RightAssignment_3_186232);
ruleXExpression();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXVariableDeclarationAccess().getRightXExpressionParserRuleCall_3_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XVariableDeclaration__RightAssignment_3_1"
// $ANTLR start "rule__JvmFormalParameter__ParameterTypeAssignment_0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42882:1: rule__JvmFormalParameter__ParameterTypeAssignment_0 : ( ruleJvmTypeReference ) ;
public final void rule__JvmFormalParameter__ParameterTypeAssignment_0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42886:1: ( ( ruleJvmTypeReference ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42887:1: ( ruleJvmTypeReference )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42887:1: ( ruleJvmTypeReference )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42888:1: ruleJvmTypeReference
{
if ( state.backtracking==0 ) {
before(grammarAccess.getJvmFormalParameterAccess().getParameterTypeJvmTypeReferenceParserRuleCall_0_0());
}
pushFollow(FollowSets003.FOLLOW_ruleJvmTypeReference_in_rule__JvmFormalParameter__ParameterTypeAssignment_086263);
ruleJvmTypeReference();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getJvmFormalParameterAccess().getParameterTypeJvmTypeReferenceParserRuleCall_0_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__JvmFormalParameter__ParameterTypeAssignment_0"
// $ANTLR start "rule__JvmFormalParameter__NameAssignment_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42897:1: rule__JvmFormalParameter__NameAssignment_1 : ( ruleValidID ) ;
public final void rule__JvmFormalParameter__NameAssignment_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42901:1: ( ( ruleValidID ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42902:1: ( ruleValidID )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42902:1: ( ruleValidID )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42903:1: ruleValidID
{
if ( state.backtracking==0 ) {
before(grammarAccess.getJvmFormalParameterAccess().getNameValidIDParserRuleCall_1_0());
}
pushFollow(FollowSets003.FOLLOW_ruleValidID_in_rule__JvmFormalParameter__NameAssignment_186294);
ruleValidID();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getJvmFormalParameterAccess().getNameValidIDParserRuleCall_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__JvmFormalParameter__NameAssignment_1"
// $ANTLR start "rule__FullJvmFormalParameter__ParameterTypeAssignment_0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42912:1: rule__FullJvmFormalParameter__ParameterTypeAssignment_0 : ( ruleJvmTypeReference ) ;
public final void rule__FullJvmFormalParameter__ParameterTypeAssignment_0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42916:1: ( ( ruleJvmTypeReference ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42917:1: ( ruleJvmTypeReference )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42917:1: ( ruleJvmTypeReference )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42918:1: ruleJvmTypeReference
{
if ( state.backtracking==0 ) {
before(grammarAccess.getFullJvmFormalParameterAccess().getParameterTypeJvmTypeReferenceParserRuleCall_0_0());
}
pushFollow(FollowSets003.FOLLOW_ruleJvmTypeReference_in_rule__FullJvmFormalParameter__ParameterTypeAssignment_086325);
ruleJvmTypeReference();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getFullJvmFormalParameterAccess().getParameterTypeJvmTypeReferenceParserRuleCall_0_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__FullJvmFormalParameter__ParameterTypeAssignment_0"
// $ANTLR start "rule__FullJvmFormalParameter__NameAssignment_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42927:1: rule__FullJvmFormalParameter__NameAssignment_1 : ( ruleValidID ) ;
public final void rule__FullJvmFormalParameter__NameAssignment_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42931:1: ( ( ruleValidID ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42932:1: ( ruleValidID )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42932:1: ( ruleValidID )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42933:1: ruleValidID
{
if ( state.backtracking==0 ) {
before(grammarAccess.getFullJvmFormalParameterAccess().getNameValidIDParserRuleCall_1_0());
}
pushFollow(FollowSets003.FOLLOW_ruleValidID_in_rule__FullJvmFormalParameter__NameAssignment_186356);
ruleValidID();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getFullJvmFormalParameterAccess().getNameValidIDParserRuleCall_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__FullJvmFormalParameter__NameAssignment_1"
// $ANTLR start "rule__XFeatureCall__TypeArgumentsAssignment_1_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42942:1: rule__XFeatureCall__TypeArgumentsAssignment_1_1 : ( ruleJvmArgumentTypeReference ) ;
public final void rule__XFeatureCall__TypeArgumentsAssignment_1_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42946:1: ( ( ruleJvmArgumentTypeReference ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42947:1: ( ruleJvmArgumentTypeReference )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42947:1: ( ruleJvmArgumentTypeReference )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42948:1: ruleJvmArgumentTypeReference
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXFeatureCallAccess().getTypeArgumentsJvmArgumentTypeReferenceParserRuleCall_1_1_0());
}
pushFollow(FollowSets003.FOLLOW_ruleJvmArgumentTypeReference_in_rule__XFeatureCall__TypeArgumentsAssignment_1_186387);
ruleJvmArgumentTypeReference();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXFeatureCallAccess().getTypeArgumentsJvmArgumentTypeReferenceParserRuleCall_1_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XFeatureCall__TypeArgumentsAssignment_1_1"
// $ANTLR start "rule__XFeatureCall__TypeArgumentsAssignment_1_2_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42957:1: rule__XFeatureCall__TypeArgumentsAssignment_1_2_1 : ( ruleJvmArgumentTypeReference ) ;
public final void rule__XFeatureCall__TypeArgumentsAssignment_1_2_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42961:1: ( ( ruleJvmArgumentTypeReference ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42962:1: ( ruleJvmArgumentTypeReference )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42962:1: ( ruleJvmArgumentTypeReference )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42963:1: ruleJvmArgumentTypeReference
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXFeatureCallAccess().getTypeArgumentsJvmArgumentTypeReferenceParserRuleCall_1_2_1_0());
}
pushFollow(FollowSets003.FOLLOW_ruleJvmArgumentTypeReference_in_rule__XFeatureCall__TypeArgumentsAssignment_1_2_186418);
ruleJvmArgumentTypeReference();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXFeatureCallAccess().getTypeArgumentsJvmArgumentTypeReferenceParserRuleCall_1_2_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XFeatureCall__TypeArgumentsAssignment_1_2_1"
// $ANTLR start "rule__XFeatureCall__FeatureAssignment_2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42972:1: rule__XFeatureCall__FeatureAssignment_2 : ( ( ruleIdOrSuper ) ) ;
public final void rule__XFeatureCall__FeatureAssignment_2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42976:1: ( ( ( ruleIdOrSuper ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42977:1: ( ( ruleIdOrSuper ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42977:1: ( ( ruleIdOrSuper ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42978:1: ( ruleIdOrSuper )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXFeatureCallAccess().getFeatureJvmIdentifiableElementCrossReference_2_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42979:1: ( ruleIdOrSuper )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42980:1: ruleIdOrSuper
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXFeatureCallAccess().getFeatureJvmIdentifiableElementIdOrSuperParserRuleCall_2_0_1());
}
pushFollow(FollowSets003.FOLLOW_ruleIdOrSuper_in_rule__XFeatureCall__FeatureAssignment_286453);
ruleIdOrSuper();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXFeatureCallAccess().getFeatureJvmIdentifiableElementIdOrSuperParserRuleCall_2_0_1());
}
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXFeatureCallAccess().getFeatureJvmIdentifiableElementCrossReference_2_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XFeatureCall__FeatureAssignment_2"
// $ANTLR start "rule__XFeatureCall__ExplicitOperationCallAssignment_3_0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42991:1: rule__XFeatureCall__ExplicitOperationCallAssignment_3_0 : ( ( '(' ) ) ;
public final void rule__XFeatureCall__ExplicitOperationCallAssignment_3_0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42995:1: ( ( ( '(' ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42996:1: ( ( '(' ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42996:1: ( ( '(' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42997:1: ( '(' )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXFeatureCallAccess().getExplicitOperationCallLeftParenthesisKeyword_3_0_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42998:1: ( '(' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:42999:1: '('
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXFeatureCallAccess().getExplicitOperationCallLeftParenthesisKeyword_3_0_0());
}
match(input,144,FollowSets003.FOLLOW_144_in_rule__XFeatureCall__ExplicitOperationCallAssignment_3_086493); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXFeatureCallAccess().getExplicitOperationCallLeftParenthesisKeyword_3_0_0());
}
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXFeatureCallAccess().getExplicitOperationCallLeftParenthesisKeyword_3_0_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XFeatureCall__ExplicitOperationCallAssignment_3_0"
// $ANTLR start "rule__XFeatureCall__FeatureCallArgumentsAssignment_3_1_0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43014:1: rule__XFeatureCall__FeatureCallArgumentsAssignment_3_1_0 : ( ruleXShortClosure ) ;
public final void rule__XFeatureCall__FeatureCallArgumentsAssignment_3_1_0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43018:1: ( ( ruleXShortClosure ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43019:1: ( ruleXShortClosure )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43019:1: ( ruleXShortClosure )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43020:1: ruleXShortClosure
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXFeatureCallAccess().getFeatureCallArgumentsXShortClosureParserRuleCall_3_1_0_0());
}
pushFollow(FollowSets003.FOLLOW_ruleXShortClosure_in_rule__XFeatureCall__FeatureCallArgumentsAssignment_3_1_086532);
ruleXShortClosure();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXFeatureCallAccess().getFeatureCallArgumentsXShortClosureParserRuleCall_3_1_0_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XFeatureCall__FeatureCallArgumentsAssignment_3_1_0"
// $ANTLR start "rule__XFeatureCall__FeatureCallArgumentsAssignment_3_1_1_0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43029:1: rule__XFeatureCall__FeatureCallArgumentsAssignment_3_1_1_0 : ( ruleXExpression ) ;
public final void rule__XFeatureCall__FeatureCallArgumentsAssignment_3_1_1_0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43033:1: ( ( ruleXExpression ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43034:1: ( ruleXExpression )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43034:1: ( ruleXExpression )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43035:1: ruleXExpression
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXFeatureCallAccess().getFeatureCallArgumentsXExpressionParserRuleCall_3_1_1_0_0());
}
pushFollow(FollowSets003.FOLLOW_ruleXExpression_in_rule__XFeatureCall__FeatureCallArgumentsAssignment_3_1_1_086563);
ruleXExpression();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXFeatureCallAccess().getFeatureCallArgumentsXExpressionParserRuleCall_3_1_1_0_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XFeatureCall__FeatureCallArgumentsAssignment_3_1_1_0"
// $ANTLR start "rule__XFeatureCall__FeatureCallArgumentsAssignment_3_1_1_1_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43044:1: rule__XFeatureCall__FeatureCallArgumentsAssignment_3_1_1_1_1 : ( ruleXExpression ) ;
public final void rule__XFeatureCall__FeatureCallArgumentsAssignment_3_1_1_1_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43048:1: ( ( ruleXExpression ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43049:1: ( ruleXExpression )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43049:1: ( ruleXExpression )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43050:1: ruleXExpression
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXFeatureCallAccess().getFeatureCallArgumentsXExpressionParserRuleCall_3_1_1_1_1_0());
}
pushFollow(FollowSets003.FOLLOW_ruleXExpression_in_rule__XFeatureCall__FeatureCallArgumentsAssignment_3_1_1_1_186594);
ruleXExpression();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXFeatureCallAccess().getFeatureCallArgumentsXExpressionParserRuleCall_3_1_1_1_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XFeatureCall__FeatureCallArgumentsAssignment_3_1_1_1_1"
// $ANTLR start "rule__XFeatureCall__FeatureCallArgumentsAssignment_4"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43059:1: rule__XFeatureCall__FeatureCallArgumentsAssignment_4 : ( ruleXClosure ) ;
public final void rule__XFeatureCall__FeatureCallArgumentsAssignment_4() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43063:1: ( ( ruleXClosure ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43064:1: ( ruleXClosure )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43064:1: ( ruleXClosure )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43065:1: ruleXClosure
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXFeatureCallAccess().getFeatureCallArgumentsXClosureParserRuleCall_4_0());
}
pushFollow(FollowSets003.FOLLOW_ruleXClosure_in_rule__XFeatureCall__FeatureCallArgumentsAssignment_486625);
ruleXClosure();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXFeatureCallAccess().getFeatureCallArgumentsXClosureParserRuleCall_4_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XFeatureCall__FeatureCallArgumentsAssignment_4"
// $ANTLR start "rule__XConstructorCall__ConstructorAssignment_2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43074:1: rule__XConstructorCall__ConstructorAssignment_2 : ( ( ruleQualifiedName ) ) ;
public final void rule__XConstructorCall__ConstructorAssignment_2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43078:1: ( ( ( ruleQualifiedName ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43079:1: ( ( ruleQualifiedName ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43079:1: ( ( ruleQualifiedName ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43080:1: ( ruleQualifiedName )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXConstructorCallAccess().getConstructorJvmConstructorCrossReference_2_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43081:1: ( ruleQualifiedName )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43082:1: ruleQualifiedName
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXConstructorCallAccess().getConstructorJvmConstructorQualifiedNameParserRuleCall_2_0_1());
}
pushFollow(FollowSets003.FOLLOW_ruleQualifiedName_in_rule__XConstructorCall__ConstructorAssignment_286660);
ruleQualifiedName();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXConstructorCallAccess().getConstructorJvmConstructorQualifiedNameParserRuleCall_2_0_1());
}
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXConstructorCallAccess().getConstructorJvmConstructorCrossReference_2_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XConstructorCall__ConstructorAssignment_2"
// $ANTLR start "rule__XConstructorCall__TypeArgumentsAssignment_3_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43093:1: rule__XConstructorCall__TypeArgumentsAssignment_3_1 : ( ruleJvmArgumentTypeReference ) ;
public final void rule__XConstructorCall__TypeArgumentsAssignment_3_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43097:1: ( ( ruleJvmArgumentTypeReference ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43098:1: ( ruleJvmArgumentTypeReference )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43098:1: ( ruleJvmArgumentTypeReference )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43099:1: ruleJvmArgumentTypeReference
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXConstructorCallAccess().getTypeArgumentsJvmArgumentTypeReferenceParserRuleCall_3_1_0());
}
pushFollow(FollowSets003.FOLLOW_ruleJvmArgumentTypeReference_in_rule__XConstructorCall__TypeArgumentsAssignment_3_186695);
ruleJvmArgumentTypeReference();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXConstructorCallAccess().getTypeArgumentsJvmArgumentTypeReferenceParserRuleCall_3_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XConstructorCall__TypeArgumentsAssignment_3_1"
// $ANTLR start "rule__XConstructorCall__TypeArgumentsAssignment_3_2_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43108:1: rule__XConstructorCall__TypeArgumentsAssignment_3_2_1 : ( ruleJvmArgumentTypeReference ) ;
public final void rule__XConstructorCall__TypeArgumentsAssignment_3_2_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43112:1: ( ( ruleJvmArgumentTypeReference ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43113:1: ( ruleJvmArgumentTypeReference )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43113:1: ( ruleJvmArgumentTypeReference )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43114:1: ruleJvmArgumentTypeReference
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXConstructorCallAccess().getTypeArgumentsJvmArgumentTypeReferenceParserRuleCall_3_2_1_0());
}
pushFollow(FollowSets003.FOLLOW_ruleJvmArgumentTypeReference_in_rule__XConstructorCall__TypeArgumentsAssignment_3_2_186726);
ruleJvmArgumentTypeReference();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXConstructorCallAccess().getTypeArgumentsJvmArgumentTypeReferenceParserRuleCall_3_2_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XConstructorCall__TypeArgumentsAssignment_3_2_1"
// $ANTLR start "rule__XConstructorCall__ExplicitConstructorCallAssignment_4_0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43123:1: rule__XConstructorCall__ExplicitConstructorCallAssignment_4_0 : ( ( '(' ) ) ;
public final void rule__XConstructorCall__ExplicitConstructorCallAssignment_4_0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43127:1: ( ( ( '(' ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43128:1: ( ( '(' ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43128:1: ( ( '(' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43129:1: ( '(' )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXConstructorCallAccess().getExplicitConstructorCallLeftParenthesisKeyword_4_0_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43130:1: ( '(' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43131:1: '('
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXConstructorCallAccess().getExplicitConstructorCallLeftParenthesisKeyword_4_0_0());
}
match(input,144,FollowSets003.FOLLOW_144_in_rule__XConstructorCall__ExplicitConstructorCallAssignment_4_086762); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXConstructorCallAccess().getExplicitConstructorCallLeftParenthesisKeyword_4_0_0());
}
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXConstructorCallAccess().getExplicitConstructorCallLeftParenthesisKeyword_4_0_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XConstructorCall__ExplicitConstructorCallAssignment_4_0"
// $ANTLR start "rule__XConstructorCall__ArgumentsAssignment_4_1_0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43146:1: rule__XConstructorCall__ArgumentsAssignment_4_1_0 : ( ruleXShortClosure ) ;
public final void rule__XConstructorCall__ArgumentsAssignment_4_1_0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43150:1: ( ( ruleXShortClosure ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43151:1: ( ruleXShortClosure )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43151:1: ( ruleXShortClosure )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43152:1: ruleXShortClosure
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXConstructorCallAccess().getArgumentsXShortClosureParserRuleCall_4_1_0_0());
}
pushFollow(FollowSets003.FOLLOW_ruleXShortClosure_in_rule__XConstructorCall__ArgumentsAssignment_4_1_086801);
ruleXShortClosure();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXConstructorCallAccess().getArgumentsXShortClosureParserRuleCall_4_1_0_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XConstructorCall__ArgumentsAssignment_4_1_0"
// $ANTLR start "rule__XConstructorCall__ArgumentsAssignment_4_1_1_0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43161:1: rule__XConstructorCall__ArgumentsAssignment_4_1_1_0 : ( ruleXExpression ) ;
public final void rule__XConstructorCall__ArgumentsAssignment_4_1_1_0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43165:1: ( ( ruleXExpression ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43166:1: ( ruleXExpression )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43166:1: ( ruleXExpression )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43167:1: ruleXExpression
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXConstructorCallAccess().getArgumentsXExpressionParserRuleCall_4_1_1_0_0());
}
pushFollow(FollowSets003.FOLLOW_ruleXExpression_in_rule__XConstructorCall__ArgumentsAssignment_4_1_1_086832);
ruleXExpression();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXConstructorCallAccess().getArgumentsXExpressionParserRuleCall_4_1_1_0_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XConstructorCall__ArgumentsAssignment_4_1_1_0"
// $ANTLR start "rule__XConstructorCall__ArgumentsAssignment_4_1_1_1_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43176:1: rule__XConstructorCall__ArgumentsAssignment_4_1_1_1_1 : ( ruleXExpression ) ;
public final void rule__XConstructorCall__ArgumentsAssignment_4_1_1_1_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43180:1: ( ( ruleXExpression ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43181:1: ( ruleXExpression )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43181:1: ( ruleXExpression )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43182:1: ruleXExpression
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXConstructorCallAccess().getArgumentsXExpressionParserRuleCall_4_1_1_1_1_0());
}
pushFollow(FollowSets003.FOLLOW_ruleXExpression_in_rule__XConstructorCall__ArgumentsAssignment_4_1_1_1_186863);
ruleXExpression();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXConstructorCallAccess().getArgumentsXExpressionParserRuleCall_4_1_1_1_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XConstructorCall__ArgumentsAssignment_4_1_1_1_1"
// $ANTLR start "rule__XConstructorCall__ArgumentsAssignment_5"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43191:1: rule__XConstructorCall__ArgumentsAssignment_5 : ( ruleXClosure ) ;
public final void rule__XConstructorCall__ArgumentsAssignment_5() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43195:1: ( ( ruleXClosure ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43196:1: ( ruleXClosure )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43196:1: ( ruleXClosure )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43197:1: ruleXClosure
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXConstructorCallAccess().getArgumentsXClosureParserRuleCall_5_0());
}
pushFollow(FollowSets003.FOLLOW_ruleXClosure_in_rule__XConstructorCall__ArgumentsAssignment_586894);
ruleXClosure();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXConstructorCallAccess().getArgumentsXClosureParserRuleCall_5_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XConstructorCall__ArgumentsAssignment_5"
// $ANTLR start "rule__XBooleanLiteral__IsTrueAssignment_1_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43206:1: rule__XBooleanLiteral__IsTrueAssignment_1_1 : ( ( 'true' ) ) ;
public final void rule__XBooleanLiteral__IsTrueAssignment_1_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43210:1: ( ( ( 'true' ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43211:1: ( ( 'true' ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43211:1: ( ( 'true' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43212:1: ( 'true' )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXBooleanLiteralAccess().getIsTrueTrueKeyword_1_1_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43213:1: ( 'true' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43214:1: 'true'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXBooleanLiteralAccess().getIsTrueTrueKeyword_1_1_0());
}
match(input,185,FollowSets003.FOLLOW_185_in_rule__XBooleanLiteral__IsTrueAssignment_1_186930); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXBooleanLiteralAccess().getIsTrueTrueKeyword_1_1_0());
}
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXBooleanLiteralAccess().getIsTrueTrueKeyword_1_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XBooleanLiteral__IsTrueAssignment_1_1"
// $ANTLR start "rule__XNumberLiteral__ValueAssignment_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43229:1: rule__XNumberLiteral__ValueAssignment_1 : ( ruleNumber ) ;
public final void rule__XNumberLiteral__ValueAssignment_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43233:1: ( ( ruleNumber ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43234:1: ( ruleNumber )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43234:1: ( ruleNumber )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43235:1: ruleNumber
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXNumberLiteralAccess().getValueNumberParserRuleCall_1_0());
}
pushFollow(FollowSets003.FOLLOW_ruleNumber_in_rule__XNumberLiteral__ValueAssignment_186969);
ruleNumber();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXNumberLiteralAccess().getValueNumberParserRuleCall_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XNumberLiteral__ValueAssignment_1"
// $ANTLR start "rule__XStringLiteral__ValueAssignment_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43244:1: rule__XStringLiteral__ValueAssignment_1 : ( RULE_STRING ) ;
public final void rule__XStringLiteral__ValueAssignment_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43248:1: ( ( RULE_STRING ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43249:1: ( RULE_STRING )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43249:1: ( RULE_STRING )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43250:1: RULE_STRING
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXStringLiteralAccess().getValueSTRINGTerminalRuleCall_1_0());
}
match(input,RULE_STRING,FollowSets003.FOLLOW_RULE_STRING_in_rule__XStringLiteral__ValueAssignment_187000); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXStringLiteralAccess().getValueSTRINGTerminalRuleCall_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XStringLiteral__ValueAssignment_1"
// $ANTLR start "rule__XTypeLiteral__TypeAssignment_3"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43259:1: rule__XTypeLiteral__TypeAssignment_3 : ( ( ruleQualifiedName ) ) ;
public final void rule__XTypeLiteral__TypeAssignment_3() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43263:1: ( ( ( ruleQualifiedName ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43264:1: ( ( ruleQualifiedName ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43264:1: ( ( ruleQualifiedName ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43265:1: ( ruleQualifiedName )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXTypeLiteralAccess().getTypeJvmTypeCrossReference_3_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43266:1: ( ruleQualifiedName )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43267:1: ruleQualifiedName
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXTypeLiteralAccess().getTypeJvmTypeQualifiedNameParserRuleCall_3_0_1());
}
pushFollow(FollowSets003.FOLLOW_ruleQualifiedName_in_rule__XTypeLiteral__TypeAssignment_387035);
ruleQualifiedName();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXTypeLiteralAccess().getTypeJvmTypeQualifiedNameParserRuleCall_3_0_1());
}
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXTypeLiteralAccess().getTypeJvmTypeCrossReference_3_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XTypeLiteral__TypeAssignment_3"
// $ANTLR start "rule__XTypeLiteral__ArrayDimensionsAssignment_4"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43278:1: rule__XTypeLiteral__ArrayDimensionsAssignment_4 : ( ruleArrayBrackets ) ;
public final void rule__XTypeLiteral__ArrayDimensionsAssignment_4() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43282:1: ( ( ruleArrayBrackets ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43283:1: ( ruleArrayBrackets )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43283:1: ( ruleArrayBrackets )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43284:1: ruleArrayBrackets
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXTypeLiteralAccess().getArrayDimensionsArrayBracketsParserRuleCall_4_0());
}
pushFollow(FollowSets003.FOLLOW_ruleArrayBrackets_in_rule__XTypeLiteral__ArrayDimensionsAssignment_487070);
ruleArrayBrackets();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXTypeLiteralAccess().getArrayDimensionsArrayBracketsParserRuleCall_4_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XTypeLiteral__ArrayDimensionsAssignment_4"
// $ANTLR start "rule__XThrowExpression__ExpressionAssignment_2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43293:1: rule__XThrowExpression__ExpressionAssignment_2 : ( ruleXExpression ) ;
public final void rule__XThrowExpression__ExpressionAssignment_2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43297:1: ( ( ruleXExpression ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43298:1: ( ruleXExpression )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43298:1: ( ruleXExpression )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43299:1: ruleXExpression
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXThrowExpressionAccess().getExpressionXExpressionParserRuleCall_2_0());
}
pushFollow(FollowSets003.FOLLOW_ruleXExpression_in_rule__XThrowExpression__ExpressionAssignment_287101);
ruleXExpression();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXThrowExpressionAccess().getExpressionXExpressionParserRuleCall_2_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XThrowExpression__ExpressionAssignment_2"
// $ANTLR start "rule__XReturnExpression__ExpressionAssignment_2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43308:1: rule__XReturnExpression__ExpressionAssignment_2 : ( ruleXExpression ) ;
public final void rule__XReturnExpression__ExpressionAssignment_2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43312:1: ( ( ruleXExpression ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43313:1: ( ruleXExpression )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43313:1: ( ruleXExpression )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43314:1: ruleXExpression
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXReturnExpressionAccess().getExpressionXExpressionParserRuleCall_2_0());
}
pushFollow(FollowSets003.FOLLOW_ruleXExpression_in_rule__XReturnExpression__ExpressionAssignment_287132);
ruleXExpression();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXReturnExpressionAccess().getExpressionXExpressionParserRuleCall_2_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XReturnExpression__ExpressionAssignment_2"
// $ANTLR start "rule__XTryCatchFinallyExpression__ExpressionAssignment_2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43323:1: rule__XTryCatchFinallyExpression__ExpressionAssignment_2 : ( ruleXExpression ) ;
public final void rule__XTryCatchFinallyExpression__ExpressionAssignment_2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43327:1: ( ( ruleXExpression ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43328:1: ( ruleXExpression )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43328:1: ( ruleXExpression )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43329:1: ruleXExpression
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXTryCatchFinallyExpressionAccess().getExpressionXExpressionParserRuleCall_2_0());
}
pushFollow(FollowSets003.FOLLOW_ruleXExpression_in_rule__XTryCatchFinallyExpression__ExpressionAssignment_287163);
ruleXExpression();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXTryCatchFinallyExpressionAccess().getExpressionXExpressionParserRuleCall_2_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XTryCatchFinallyExpression__ExpressionAssignment_2"
// $ANTLR start "rule__XTryCatchFinallyExpression__CatchClausesAssignment_3_0_0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43338:1: rule__XTryCatchFinallyExpression__CatchClausesAssignment_3_0_0 : ( ruleXCatchClause ) ;
public final void rule__XTryCatchFinallyExpression__CatchClausesAssignment_3_0_0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43342:1: ( ( ruleXCatchClause ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43343:1: ( ruleXCatchClause )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43343:1: ( ruleXCatchClause )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43344:1: ruleXCatchClause
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXTryCatchFinallyExpressionAccess().getCatchClausesXCatchClauseParserRuleCall_3_0_0_0());
}
pushFollow(FollowSets003.FOLLOW_ruleXCatchClause_in_rule__XTryCatchFinallyExpression__CatchClausesAssignment_3_0_087194);
ruleXCatchClause();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXTryCatchFinallyExpressionAccess().getCatchClausesXCatchClauseParserRuleCall_3_0_0_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XTryCatchFinallyExpression__CatchClausesAssignment_3_0_0"
// $ANTLR start "rule__XTryCatchFinallyExpression__FinallyExpressionAssignment_3_0_1_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43353:1: rule__XTryCatchFinallyExpression__FinallyExpressionAssignment_3_0_1_1 : ( ruleXExpression ) ;
public final void rule__XTryCatchFinallyExpression__FinallyExpressionAssignment_3_0_1_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43357:1: ( ( ruleXExpression ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43358:1: ( ruleXExpression )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43358:1: ( ruleXExpression )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43359:1: ruleXExpression
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXTryCatchFinallyExpressionAccess().getFinallyExpressionXExpressionParserRuleCall_3_0_1_1_0());
}
pushFollow(FollowSets003.FOLLOW_ruleXExpression_in_rule__XTryCatchFinallyExpression__FinallyExpressionAssignment_3_0_1_187225);
ruleXExpression();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXTryCatchFinallyExpressionAccess().getFinallyExpressionXExpressionParserRuleCall_3_0_1_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XTryCatchFinallyExpression__FinallyExpressionAssignment_3_0_1_1"
// $ANTLR start "rule__XTryCatchFinallyExpression__FinallyExpressionAssignment_3_1_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43368:1: rule__XTryCatchFinallyExpression__FinallyExpressionAssignment_3_1_1 : ( ruleXExpression ) ;
public final void rule__XTryCatchFinallyExpression__FinallyExpressionAssignment_3_1_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43372:1: ( ( ruleXExpression ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43373:1: ( ruleXExpression )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43373:1: ( ruleXExpression )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43374:1: ruleXExpression
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXTryCatchFinallyExpressionAccess().getFinallyExpressionXExpressionParserRuleCall_3_1_1_0());
}
pushFollow(FollowSets003.FOLLOW_ruleXExpression_in_rule__XTryCatchFinallyExpression__FinallyExpressionAssignment_3_1_187256);
ruleXExpression();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXTryCatchFinallyExpressionAccess().getFinallyExpressionXExpressionParserRuleCall_3_1_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XTryCatchFinallyExpression__FinallyExpressionAssignment_3_1_1"
// $ANTLR start "rule__XSynchronizedExpression__ParamAssignment_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43383:1: rule__XSynchronizedExpression__ParamAssignment_1 : ( ruleXExpression ) ;
public final void rule__XSynchronizedExpression__ParamAssignment_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43387:1: ( ( ruleXExpression ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43388:1: ( ruleXExpression )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43388:1: ( ruleXExpression )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43389:1: ruleXExpression
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXSynchronizedExpressionAccess().getParamXExpressionParserRuleCall_1_0());
}
pushFollow(FollowSets003.FOLLOW_ruleXExpression_in_rule__XSynchronizedExpression__ParamAssignment_187287);
ruleXExpression();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXSynchronizedExpressionAccess().getParamXExpressionParserRuleCall_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XSynchronizedExpression__ParamAssignment_1"
// $ANTLR start "rule__XSynchronizedExpression__ExpressionAssignment_3"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43398:1: rule__XSynchronizedExpression__ExpressionAssignment_3 : ( ruleXExpression ) ;
public final void rule__XSynchronizedExpression__ExpressionAssignment_3() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43402:1: ( ( ruleXExpression ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43403:1: ( ruleXExpression )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43403:1: ( ruleXExpression )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43404:1: ruleXExpression
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXSynchronizedExpressionAccess().getExpressionXExpressionParserRuleCall_3_0());
}
pushFollow(FollowSets003.FOLLOW_ruleXExpression_in_rule__XSynchronizedExpression__ExpressionAssignment_387318);
ruleXExpression();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXSynchronizedExpressionAccess().getExpressionXExpressionParserRuleCall_3_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XSynchronizedExpression__ExpressionAssignment_3"
// $ANTLR start "rule__XCatchClause__DeclaredParamAssignment_2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43413:1: rule__XCatchClause__DeclaredParamAssignment_2 : ( ruleFullJvmFormalParameter ) ;
public final void rule__XCatchClause__DeclaredParamAssignment_2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43417:1: ( ( ruleFullJvmFormalParameter ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43418:1: ( ruleFullJvmFormalParameter )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43418:1: ( ruleFullJvmFormalParameter )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43419:1: ruleFullJvmFormalParameter
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXCatchClauseAccess().getDeclaredParamFullJvmFormalParameterParserRuleCall_2_0());
}
pushFollow(FollowSets003.FOLLOW_ruleFullJvmFormalParameter_in_rule__XCatchClause__DeclaredParamAssignment_287349);
ruleFullJvmFormalParameter();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXCatchClauseAccess().getDeclaredParamFullJvmFormalParameterParserRuleCall_2_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XCatchClause__DeclaredParamAssignment_2"
// $ANTLR start "rule__XCatchClause__ExpressionAssignment_4"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43428:1: rule__XCatchClause__ExpressionAssignment_4 : ( ruleXExpression ) ;
public final void rule__XCatchClause__ExpressionAssignment_4() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43432:1: ( ( ruleXExpression ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43433:1: ( ruleXExpression )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43433:1: ( ruleXExpression )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43434:1: ruleXExpression
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXCatchClauseAccess().getExpressionXExpressionParserRuleCall_4_0());
}
pushFollow(FollowSets003.FOLLOW_ruleXExpression_in_rule__XCatchClause__ExpressionAssignment_487380);
ruleXExpression();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXCatchClauseAccess().getExpressionXExpressionParserRuleCall_4_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XCatchClause__ExpressionAssignment_4"
// $ANTLR start "rule__XFunctionTypeRef__ParamTypesAssignment_0_1_0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43443:1: rule__XFunctionTypeRef__ParamTypesAssignment_0_1_0 : ( ruleJvmTypeReference ) ;
public final void rule__XFunctionTypeRef__ParamTypesAssignment_0_1_0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43447:1: ( ( ruleJvmTypeReference ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43448:1: ( ruleJvmTypeReference )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43448:1: ( ruleJvmTypeReference )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43449:1: ruleJvmTypeReference
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXFunctionTypeRefAccess().getParamTypesJvmTypeReferenceParserRuleCall_0_1_0_0());
}
pushFollow(FollowSets003.FOLLOW_ruleJvmTypeReference_in_rule__XFunctionTypeRef__ParamTypesAssignment_0_1_087411);
ruleJvmTypeReference();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXFunctionTypeRefAccess().getParamTypesJvmTypeReferenceParserRuleCall_0_1_0_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XFunctionTypeRef__ParamTypesAssignment_0_1_0"
// $ANTLR start "rule__XFunctionTypeRef__ParamTypesAssignment_0_1_1_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43458:1: rule__XFunctionTypeRef__ParamTypesAssignment_0_1_1_1 : ( ruleJvmTypeReference ) ;
public final void rule__XFunctionTypeRef__ParamTypesAssignment_0_1_1_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43462:1: ( ( ruleJvmTypeReference ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43463:1: ( ruleJvmTypeReference )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43463:1: ( ruleJvmTypeReference )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43464:1: ruleJvmTypeReference
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXFunctionTypeRefAccess().getParamTypesJvmTypeReferenceParserRuleCall_0_1_1_1_0());
}
pushFollow(FollowSets003.FOLLOW_ruleJvmTypeReference_in_rule__XFunctionTypeRef__ParamTypesAssignment_0_1_1_187442);
ruleJvmTypeReference();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXFunctionTypeRefAccess().getParamTypesJvmTypeReferenceParserRuleCall_0_1_1_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XFunctionTypeRef__ParamTypesAssignment_0_1_1_1"
// $ANTLR start "rule__XFunctionTypeRef__ReturnTypeAssignment_2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43473:1: rule__XFunctionTypeRef__ReturnTypeAssignment_2 : ( ruleJvmTypeReference ) ;
public final void rule__XFunctionTypeRef__ReturnTypeAssignment_2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43477:1: ( ( ruleJvmTypeReference ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43478:1: ( ruleJvmTypeReference )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43478:1: ( ruleJvmTypeReference )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43479:1: ruleJvmTypeReference
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXFunctionTypeRefAccess().getReturnTypeJvmTypeReferenceParserRuleCall_2_0());
}
pushFollow(FollowSets003.FOLLOW_ruleJvmTypeReference_in_rule__XFunctionTypeRef__ReturnTypeAssignment_287473);
ruleJvmTypeReference();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXFunctionTypeRefAccess().getReturnTypeJvmTypeReferenceParserRuleCall_2_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XFunctionTypeRef__ReturnTypeAssignment_2"
// $ANTLR start "rule__JvmParameterizedTypeReference__TypeAssignment_0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43488:1: rule__JvmParameterizedTypeReference__TypeAssignment_0 : ( ( ruleQualifiedName ) ) ;
public final void rule__JvmParameterizedTypeReference__TypeAssignment_0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43492:1: ( ( ( ruleQualifiedName ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43493:1: ( ( ruleQualifiedName ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43493:1: ( ( ruleQualifiedName ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43494:1: ( ruleQualifiedName )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getJvmParameterizedTypeReferenceAccess().getTypeJvmTypeCrossReference_0_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43495:1: ( ruleQualifiedName )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43496:1: ruleQualifiedName
{
if ( state.backtracking==0 ) {
before(grammarAccess.getJvmParameterizedTypeReferenceAccess().getTypeJvmTypeQualifiedNameParserRuleCall_0_0_1());
}
pushFollow(FollowSets003.FOLLOW_ruleQualifiedName_in_rule__JvmParameterizedTypeReference__TypeAssignment_087508);
ruleQualifiedName();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getJvmParameterizedTypeReferenceAccess().getTypeJvmTypeQualifiedNameParserRuleCall_0_0_1());
}
}
if ( state.backtracking==0 ) {
after(grammarAccess.getJvmParameterizedTypeReferenceAccess().getTypeJvmTypeCrossReference_0_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__JvmParameterizedTypeReference__TypeAssignment_0"
// $ANTLR start "rule__JvmParameterizedTypeReference__ArgumentsAssignment_1_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43507:1: rule__JvmParameterizedTypeReference__ArgumentsAssignment_1_1 : ( ruleJvmArgumentTypeReference ) ;
public final void rule__JvmParameterizedTypeReference__ArgumentsAssignment_1_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43511:1: ( ( ruleJvmArgumentTypeReference ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43512:1: ( ruleJvmArgumentTypeReference )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43512:1: ( ruleJvmArgumentTypeReference )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43513:1: ruleJvmArgumentTypeReference
{
if ( state.backtracking==0 ) {
before(grammarAccess.getJvmParameterizedTypeReferenceAccess().getArgumentsJvmArgumentTypeReferenceParserRuleCall_1_1_0());
}
pushFollow(FollowSets003.FOLLOW_ruleJvmArgumentTypeReference_in_rule__JvmParameterizedTypeReference__ArgumentsAssignment_1_187543);
ruleJvmArgumentTypeReference();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getJvmParameterizedTypeReferenceAccess().getArgumentsJvmArgumentTypeReferenceParserRuleCall_1_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__JvmParameterizedTypeReference__ArgumentsAssignment_1_1"
// $ANTLR start "rule__JvmParameterizedTypeReference__ArgumentsAssignment_1_2_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43522:1: rule__JvmParameterizedTypeReference__ArgumentsAssignment_1_2_1 : ( ruleJvmArgumentTypeReference ) ;
public final void rule__JvmParameterizedTypeReference__ArgumentsAssignment_1_2_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43526:1: ( ( ruleJvmArgumentTypeReference ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43527:1: ( ruleJvmArgumentTypeReference )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43527:1: ( ruleJvmArgumentTypeReference )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43528:1: ruleJvmArgumentTypeReference
{
if ( state.backtracking==0 ) {
before(grammarAccess.getJvmParameterizedTypeReferenceAccess().getArgumentsJvmArgumentTypeReferenceParserRuleCall_1_2_1_0());
}
pushFollow(FollowSets003.FOLLOW_ruleJvmArgumentTypeReference_in_rule__JvmParameterizedTypeReference__ArgumentsAssignment_1_2_187574);
ruleJvmArgumentTypeReference();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getJvmParameterizedTypeReferenceAccess().getArgumentsJvmArgumentTypeReferenceParserRuleCall_1_2_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__JvmParameterizedTypeReference__ArgumentsAssignment_1_2_1"
// $ANTLR start "rule__JvmParameterizedTypeReference__TypeAssignment_1_4_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43537:1: rule__JvmParameterizedTypeReference__TypeAssignment_1_4_1 : ( ( ruleValidID ) ) ;
public final void rule__JvmParameterizedTypeReference__TypeAssignment_1_4_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43541:1: ( ( ( ruleValidID ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43542:1: ( ( ruleValidID ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43542:1: ( ( ruleValidID ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43543:1: ( ruleValidID )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getJvmParameterizedTypeReferenceAccess().getTypeJvmTypeCrossReference_1_4_1_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43544:1: ( ruleValidID )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43545:1: ruleValidID
{
if ( state.backtracking==0 ) {
before(grammarAccess.getJvmParameterizedTypeReferenceAccess().getTypeJvmTypeValidIDParserRuleCall_1_4_1_0_1());
}
pushFollow(FollowSets003.FOLLOW_ruleValidID_in_rule__JvmParameterizedTypeReference__TypeAssignment_1_4_187609);
ruleValidID();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getJvmParameterizedTypeReferenceAccess().getTypeJvmTypeValidIDParserRuleCall_1_4_1_0_1());
}
}
if ( state.backtracking==0 ) {
after(grammarAccess.getJvmParameterizedTypeReferenceAccess().getTypeJvmTypeCrossReference_1_4_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__JvmParameterizedTypeReference__TypeAssignment_1_4_1"
// $ANTLR start "rule__JvmParameterizedTypeReference__ArgumentsAssignment_1_4_2_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43556:1: rule__JvmParameterizedTypeReference__ArgumentsAssignment_1_4_2_1 : ( ruleJvmArgumentTypeReference ) ;
public final void rule__JvmParameterizedTypeReference__ArgumentsAssignment_1_4_2_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43560:1: ( ( ruleJvmArgumentTypeReference ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43561:1: ( ruleJvmArgumentTypeReference )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43561:1: ( ruleJvmArgumentTypeReference )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43562:1: ruleJvmArgumentTypeReference
{
if ( state.backtracking==0 ) {
before(grammarAccess.getJvmParameterizedTypeReferenceAccess().getArgumentsJvmArgumentTypeReferenceParserRuleCall_1_4_2_1_0());
}
pushFollow(FollowSets003.FOLLOW_ruleJvmArgumentTypeReference_in_rule__JvmParameterizedTypeReference__ArgumentsAssignment_1_4_2_187644);
ruleJvmArgumentTypeReference();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getJvmParameterizedTypeReferenceAccess().getArgumentsJvmArgumentTypeReferenceParserRuleCall_1_4_2_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__JvmParameterizedTypeReference__ArgumentsAssignment_1_4_2_1"
// $ANTLR start "rule__JvmParameterizedTypeReference__ArgumentsAssignment_1_4_2_2_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43571:1: rule__JvmParameterizedTypeReference__ArgumentsAssignment_1_4_2_2_1 : ( ruleJvmArgumentTypeReference ) ;
public final void rule__JvmParameterizedTypeReference__ArgumentsAssignment_1_4_2_2_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43575:1: ( ( ruleJvmArgumentTypeReference ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43576:1: ( ruleJvmArgumentTypeReference )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43576:1: ( ruleJvmArgumentTypeReference )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43577:1: ruleJvmArgumentTypeReference
{
if ( state.backtracking==0 ) {
before(grammarAccess.getJvmParameterizedTypeReferenceAccess().getArgumentsJvmArgumentTypeReferenceParserRuleCall_1_4_2_2_1_0());
}
pushFollow(FollowSets003.FOLLOW_ruleJvmArgumentTypeReference_in_rule__JvmParameterizedTypeReference__ArgumentsAssignment_1_4_2_2_187675);
ruleJvmArgumentTypeReference();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getJvmParameterizedTypeReferenceAccess().getArgumentsJvmArgumentTypeReferenceParserRuleCall_1_4_2_2_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__JvmParameterizedTypeReference__ArgumentsAssignment_1_4_2_2_1"
// $ANTLR start "rule__JvmWildcardTypeReference__ConstraintsAssignment_2_0_0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43586:1: rule__JvmWildcardTypeReference__ConstraintsAssignment_2_0_0 : ( ruleJvmUpperBound ) ;
public final void rule__JvmWildcardTypeReference__ConstraintsAssignment_2_0_0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43590:1: ( ( ruleJvmUpperBound ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43591:1: ( ruleJvmUpperBound )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43591:1: ( ruleJvmUpperBound )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43592:1: ruleJvmUpperBound
{
if ( state.backtracking==0 ) {
before(grammarAccess.getJvmWildcardTypeReferenceAccess().getConstraintsJvmUpperBoundParserRuleCall_2_0_0_0());
}
pushFollow(FollowSets003.FOLLOW_ruleJvmUpperBound_in_rule__JvmWildcardTypeReference__ConstraintsAssignment_2_0_087706);
ruleJvmUpperBound();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getJvmWildcardTypeReferenceAccess().getConstraintsJvmUpperBoundParserRuleCall_2_0_0_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__JvmWildcardTypeReference__ConstraintsAssignment_2_0_0"
// $ANTLR start "rule__JvmWildcardTypeReference__ConstraintsAssignment_2_0_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43601:1: rule__JvmWildcardTypeReference__ConstraintsAssignment_2_0_1 : ( ruleJvmUpperBoundAnded ) ;
public final void rule__JvmWildcardTypeReference__ConstraintsAssignment_2_0_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43605:1: ( ( ruleJvmUpperBoundAnded ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43606:1: ( ruleJvmUpperBoundAnded )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43606:1: ( ruleJvmUpperBoundAnded )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43607:1: ruleJvmUpperBoundAnded
{
if ( state.backtracking==0 ) {
before(grammarAccess.getJvmWildcardTypeReferenceAccess().getConstraintsJvmUpperBoundAndedParserRuleCall_2_0_1_0());
}
pushFollow(FollowSets003.FOLLOW_ruleJvmUpperBoundAnded_in_rule__JvmWildcardTypeReference__ConstraintsAssignment_2_0_187737);
ruleJvmUpperBoundAnded();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getJvmWildcardTypeReferenceAccess().getConstraintsJvmUpperBoundAndedParserRuleCall_2_0_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__JvmWildcardTypeReference__ConstraintsAssignment_2_0_1"
// $ANTLR start "rule__JvmWildcardTypeReference__ConstraintsAssignment_2_1_0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43616:1: rule__JvmWildcardTypeReference__ConstraintsAssignment_2_1_0 : ( ruleJvmLowerBound ) ;
public final void rule__JvmWildcardTypeReference__ConstraintsAssignment_2_1_0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43620:1: ( ( ruleJvmLowerBound ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43621:1: ( ruleJvmLowerBound )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43621:1: ( ruleJvmLowerBound )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43622:1: ruleJvmLowerBound
{
if ( state.backtracking==0 ) {
before(grammarAccess.getJvmWildcardTypeReferenceAccess().getConstraintsJvmLowerBoundParserRuleCall_2_1_0_0());
}
pushFollow(FollowSets003.FOLLOW_ruleJvmLowerBound_in_rule__JvmWildcardTypeReference__ConstraintsAssignment_2_1_087768);
ruleJvmLowerBound();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getJvmWildcardTypeReferenceAccess().getConstraintsJvmLowerBoundParserRuleCall_2_1_0_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__JvmWildcardTypeReference__ConstraintsAssignment_2_1_0"
// $ANTLR start "rule__JvmWildcardTypeReference__ConstraintsAssignment_2_1_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43631:1: rule__JvmWildcardTypeReference__ConstraintsAssignment_2_1_1 : ( ruleJvmLowerBoundAnded ) ;
public final void rule__JvmWildcardTypeReference__ConstraintsAssignment_2_1_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43635:1: ( ( ruleJvmLowerBoundAnded ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43636:1: ( ruleJvmLowerBoundAnded )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43636:1: ( ruleJvmLowerBoundAnded )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43637:1: ruleJvmLowerBoundAnded
{
if ( state.backtracking==0 ) {
before(grammarAccess.getJvmWildcardTypeReferenceAccess().getConstraintsJvmLowerBoundAndedParserRuleCall_2_1_1_0());
}
pushFollow(FollowSets003.FOLLOW_ruleJvmLowerBoundAnded_in_rule__JvmWildcardTypeReference__ConstraintsAssignment_2_1_187799);
ruleJvmLowerBoundAnded();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getJvmWildcardTypeReferenceAccess().getConstraintsJvmLowerBoundAndedParserRuleCall_2_1_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__JvmWildcardTypeReference__ConstraintsAssignment_2_1_1"
// $ANTLR start "rule__JvmUpperBound__TypeReferenceAssignment_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43646:1: rule__JvmUpperBound__TypeReferenceAssignment_1 : ( ruleJvmTypeReference ) ;
public final void rule__JvmUpperBound__TypeReferenceAssignment_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43650:1: ( ( ruleJvmTypeReference ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43651:1: ( ruleJvmTypeReference )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43651:1: ( ruleJvmTypeReference )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43652:1: ruleJvmTypeReference
{
if ( state.backtracking==0 ) {
before(grammarAccess.getJvmUpperBoundAccess().getTypeReferenceJvmTypeReferenceParserRuleCall_1_0());
}
pushFollow(FollowSets003.FOLLOW_ruleJvmTypeReference_in_rule__JvmUpperBound__TypeReferenceAssignment_187830);
ruleJvmTypeReference();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getJvmUpperBoundAccess().getTypeReferenceJvmTypeReferenceParserRuleCall_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__JvmUpperBound__TypeReferenceAssignment_1"
// $ANTLR start "rule__JvmUpperBoundAnded__TypeReferenceAssignment_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43661:1: rule__JvmUpperBoundAnded__TypeReferenceAssignment_1 : ( ruleJvmTypeReference ) ;
public final void rule__JvmUpperBoundAnded__TypeReferenceAssignment_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43665:1: ( ( ruleJvmTypeReference ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43666:1: ( ruleJvmTypeReference )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43666:1: ( ruleJvmTypeReference )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43667:1: ruleJvmTypeReference
{
if ( state.backtracking==0 ) {
before(grammarAccess.getJvmUpperBoundAndedAccess().getTypeReferenceJvmTypeReferenceParserRuleCall_1_0());
}
pushFollow(FollowSets003.FOLLOW_ruleJvmTypeReference_in_rule__JvmUpperBoundAnded__TypeReferenceAssignment_187861);
ruleJvmTypeReference();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getJvmUpperBoundAndedAccess().getTypeReferenceJvmTypeReferenceParserRuleCall_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__JvmUpperBoundAnded__TypeReferenceAssignment_1"
// $ANTLR start "rule__JvmLowerBound__TypeReferenceAssignment_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43676:1: rule__JvmLowerBound__TypeReferenceAssignment_1 : ( ruleJvmTypeReference ) ;
public final void rule__JvmLowerBound__TypeReferenceAssignment_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43680:1: ( ( ruleJvmTypeReference ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43681:1: ( ruleJvmTypeReference )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43681:1: ( ruleJvmTypeReference )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43682:1: ruleJvmTypeReference
{
if ( state.backtracking==0 ) {
before(grammarAccess.getJvmLowerBoundAccess().getTypeReferenceJvmTypeReferenceParserRuleCall_1_0());
}
pushFollow(FollowSets003.FOLLOW_ruleJvmTypeReference_in_rule__JvmLowerBound__TypeReferenceAssignment_187892);
ruleJvmTypeReference();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getJvmLowerBoundAccess().getTypeReferenceJvmTypeReferenceParserRuleCall_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__JvmLowerBound__TypeReferenceAssignment_1"
// $ANTLR start "rule__JvmLowerBoundAnded__TypeReferenceAssignment_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43691:1: rule__JvmLowerBoundAnded__TypeReferenceAssignment_1 : ( ruleJvmTypeReference ) ;
public final void rule__JvmLowerBoundAnded__TypeReferenceAssignment_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43695:1: ( ( ruleJvmTypeReference ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43696:1: ( ruleJvmTypeReference )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43696:1: ( ruleJvmTypeReference )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43697:1: ruleJvmTypeReference
{
if ( state.backtracking==0 ) {
before(grammarAccess.getJvmLowerBoundAndedAccess().getTypeReferenceJvmTypeReferenceParserRuleCall_1_0());
}
pushFollow(FollowSets003.FOLLOW_ruleJvmTypeReference_in_rule__JvmLowerBoundAnded__TypeReferenceAssignment_187923);
ruleJvmTypeReference();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getJvmLowerBoundAndedAccess().getTypeReferenceJvmTypeReferenceParserRuleCall_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__JvmLowerBoundAnded__TypeReferenceAssignment_1"
// $ANTLR start "rule__JvmTypeParameter__NameAssignment_0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43706:1: rule__JvmTypeParameter__NameAssignment_0 : ( ruleValidID ) ;
public final void rule__JvmTypeParameter__NameAssignment_0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43710:1: ( ( ruleValidID ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43711:1: ( ruleValidID )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43711:1: ( ruleValidID )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43712:1: ruleValidID
{
if ( state.backtracking==0 ) {
before(grammarAccess.getJvmTypeParameterAccess().getNameValidIDParserRuleCall_0_0());
}
pushFollow(FollowSets003.FOLLOW_ruleValidID_in_rule__JvmTypeParameter__NameAssignment_087954);
ruleValidID();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getJvmTypeParameterAccess().getNameValidIDParserRuleCall_0_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__JvmTypeParameter__NameAssignment_0"
// $ANTLR start "rule__JvmTypeParameter__ConstraintsAssignment_1_0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43721:1: rule__JvmTypeParameter__ConstraintsAssignment_1_0 : ( ruleJvmUpperBound ) ;
public final void rule__JvmTypeParameter__ConstraintsAssignment_1_0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43725:1: ( ( ruleJvmUpperBound ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43726:1: ( ruleJvmUpperBound )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43726:1: ( ruleJvmUpperBound )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43727:1: ruleJvmUpperBound
{
if ( state.backtracking==0 ) {
before(grammarAccess.getJvmTypeParameterAccess().getConstraintsJvmUpperBoundParserRuleCall_1_0_0());
}
pushFollow(FollowSets003.FOLLOW_ruleJvmUpperBound_in_rule__JvmTypeParameter__ConstraintsAssignment_1_087985);
ruleJvmUpperBound();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getJvmTypeParameterAccess().getConstraintsJvmUpperBoundParserRuleCall_1_0_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__JvmTypeParameter__ConstraintsAssignment_1_0"
// $ANTLR start "rule__JvmTypeParameter__ConstraintsAssignment_1_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43736:1: rule__JvmTypeParameter__ConstraintsAssignment_1_1 : ( ruleJvmUpperBoundAnded ) ;
public final void rule__JvmTypeParameter__ConstraintsAssignment_1_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43740:1: ( ( ruleJvmUpperBoundAnded ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43741:1: ( ruleJvmUpperBoundAnded )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43741:1: ( ruleJvmUpperBoundAnded )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43742:1: ruleJvmUpperBoundAnded
{
if ( state.backtracking==0 ) {
before(grammarAccess.getJvmTypeParameterAccess().getConstraintsJvmUpperBoundAndedParserRuleCall_1_1_0());
}
pushFollow(FollowSets003.FOLLOW_ruleJvmUpperBoundAnded_in_rule__JvmTypeParameter__ConstraintsAssignment_1_188016);
ruleJvmUpperBoundAnded();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getJvmTypeParameterAccess().getConstraintsJvmUpperBoundAndedParserRuleCall_1_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__JvmTypeParameter__ConstraintsAssignment_1_1"
// $ANTLR start "rule__XImportSection__ImportDeclarationsAssignment"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43751:1: rule__XImportSection__ImportDeclarationsAssignment : ( ruleXImportDeclaration ) ;
public final void rule__XImportSection__ImportDeclarationsAssignment() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43755:1: ( ( ruleXImportDeclaration ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43756:1: ( ruleXImportDeclaration )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43756:1: ( ruleXImportDeclaration )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43757:1: ruleXImportDeclaration
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXImportSectionAccess().getImportDeclarationsXImportDeclarationParserRuleCall_0());
}
pushFollow(FollowSets003.FOLLOW_ruleXImportDeclaration_in_rule__XImportSection__ImportDeclarationsAssignment88047);
ruleXImportDeclaration();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXImportSectionAccess().getImportDeclarationsXImportDeclarationParserRuleCall_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XImportSection__ImportDeclarationsAssignment"
// $ANTLR start "rule__XImportDeclaration__StaticAssignment_1_0_0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43766:1: rule__XImportDeclaration__StaticAssignment_1_0_0 : ( ( 'static' ) ) ;
public final void rule__XImportDeclaration__StaticAssignment_1_0_0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43770:1: ( ( ( 'static' ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43771:1: ( ( 'static' ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43771:1: ( ( 'static' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43772:1: ( 'static' )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXImportDeclarationAccess().getStaticStaticKeyword_1_0_0_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43773:1: ( 'static' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43774:1: 'static'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXImportDeclarationAccess().getStaticStaticKeyword_1_0_0_0());
}
match(input,46,FollowSets003.FOLLOW_46_in_rule__XImportDeclaration__StaticAssignment_1_0_088083); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXImportDeclarationAccess().getStaticStaticKeyword_1_0_0_0());
}
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXImportDeclarationAccess().getStaticStaticKeyword_1_0_0_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XImportDeclaration__StaticAssignment_1_0_0"
// $ANTLR start "rule__XImportDeclaration__ExtensionAssignment_1_0_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43789:1: rule__XImportDeclaration__ExtensionAssignment_1_0_1 : ( ( 'extension' ) ) ;
public final void rule__XImportDeclaration__ExtensionAssignment_1_0_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43793:1: ( ( ( 'extension' ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43794:1: ( ( 'extension' ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43794:1: ( ( 'extension' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43795:1: ( 'extension' )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXImportDeclarationAccess().getExtensionExtensionKeyword_1_0_1_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43796:1: ( 'extension' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43797:1: 'extension'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXImportDeclarationAccess().getExtensionExtensionKeyword_1_0_1_0());
}
match(input,48,FollowSets003.FOLLOW_48_in_rule__XImportDeclaration__ExtensionAssignment_1_0_188127); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXImportDeclarationAccess().getExtensionExtensionKeyword_1_0_1_0());
}
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXImportDeclarationAccess().getExtensionExtensionKeyword_1_0_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XImportDeclaration__ExtensionAssignment_1_0_1"
// $ANTLR start "rule__XImportDeclaration__ImportedTypeAssignment_1_0_2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43812:1: rule__XImportDeclaration__ImportedTypeAssignment_1_0_2 : ( ( ruleQualifiedNameInStaticImport ) ) ;
public final void rule__XImportDeclaration__ImportedTypeAssignment_1_0_2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43816:1: ( ( ( ruleQualifiedNameInStaticImport ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43817:1: ( ( ruleQualifiedNameInStaticImport ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43817:1: ( ( ruleQualifiedNameInStaticImport ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43818:1: ( ruleQualifiedNameInStaticImport )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXImportDeclarationAccess().getImportedTypeJvmDeclaredTypeCrossReference_1_0_2_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43819:1: ( ruleQualifiedNameInStaticImport )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43820:1: ruleQualifiedNameInStaticImport
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXImportDeclarationAccess().getImportedTypeJvmDeclaredTypeQualifiedNameInStaticImportParserRuleCall_1_0_2_0_1());
}
pushFollow(FollowSets003.FOLLOW_ruleQualifiedNameInStaticImport_in_rule__XImportDeclaration__ImportedTypeAssignment_1_0_288170);
ruleQualifiedNameInStaticImport();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXImportDeclarationAccess().getImportedTypeJvmDeclaredTypeQualifiedNameInStaticImportParserRuleCall_1_0_2_0_1());
}
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXImportDeclarationAccess().getImportedTypeJvmDeclaredTypeCrossReference_1_0_2_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XImportDeclaration__ImportedTypeAssignment_1_0_2"
// $ANTLR start "rule__XImportDeclaration__WildcardAssignment_1_0_3_0"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43831:1: rule__XImportDeclaration__WildcardAssignment_1_0_3_0 : ( ( '*' ) ) ;
public final void rule__XImportDeclaration__WildcardAssignment_1_0_3_0() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43835:1: ( ( ( '*' ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43836:1: ( ( '*' ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43836:1: ( ( '*' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43837:1: ( '*' )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXImportDeclarationAccess().getWildcardAsteriskKeyword_1_0_3_0_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43838:1: ( '*' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43839:1: '*'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXImportDeclarationAccess().getWildcardAsteriskKeyword_1_0_3_0_0());
}
match(input,36,FollowSets003.FOLLOW_36_in_rule__XImportDeclaration__WildcardAssignment_1_0_3_088210); if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXImportDeclarationAccess().getWildcardAsteriskKeyword_1_0_3_0_0());
}
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXImportDeclarationAccess().getWildcardAsteriskKeyword_1_0_3_0_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XImportDeclaration__WildcardAssignment_1_0_3_0"
// $ANTLR start "rule__XImportDeclaration__MemberNameAssignment_1_0_3_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43854:1: rule__XImportDeclaration__MemberNameAssignment_1_0_3_1 : ( ruleValidID ) ;
public final void rule__XImportDeclaration__MemberNameAssignment_1_0_3_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43858:1: ( ( ruleValidID ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43859:1: ( ruleValidID )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43859:1: ( ruleValidID )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43860:1: ruleValidID
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXImportDeclarationAccess().getMemberNameValidIDParserRuleCall_1_0_3_1_0());
}
pushFollow(FollowSets003.FOLLOW_ruleValidID_in_rule__XImportDeclaration__MemberNameAssignment_1_0_3_188249);
ruleValidID();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXImportDeclarationAccess().getMemberNameValidIDParserRuleCall_1_0_3_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XImportDeclaration__MemberNameAssignment_1_0_3_1"
// $ANTLR start "rule__XImportDeclaration__ImportedTypeAssignment_1_1"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43869:1: rule__XImportDeclaration__ImportedTypeAssignment_1_1 : ( ( ruleQualifiedName ) ) ;
public final void rule__XImportDeclaration__ImportedTypeAssignment_1_1() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43873:1: ( ( ( ruleQualifiedName ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43874:1: ( ( ruleQualifiedName ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43874:1: ( ( ruleQualifiedName ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43875:1: ( ruleQualifiedName )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXImportDeclarationAccess().getImportedTypeJvmDeclaredTypeCrossReference_1_1_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43876:1: ( ruleQualifiedName )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43877:1: ruleQualifiedName
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXImportDeclarationAccess().getImportedTypeJvmDeclaredTypeQualifiedNameParserRuleCall_1_1_0_1());
}
pushFollow(FollowSets003.FOLLOW_ruleQualifiedName_in_rule__XImportDeclaration__ImportedTypeAssignment_1_188284);
ruleQualifiedName();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXImportDeclarationAccess().getImportedTypeJvmDeclaredTypeQualifiedNameParserRuleCall_1_1_0_1());
}
}
if ( state.backtracking==0 ) {
after(grammarAccess.getXImportDeclarationAccess().getImportedTypeJvmDeclaredTypeCrossReference_1_1_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XImportDeclaration__ImportedTypeAssignment_1_1"
// $ANTLR start "rule__XImportDeclaration__ImportedNamespaceAssignment_1_2"
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43888:1: rule__XImportDeclaration__ImportedNamespaceAssignment_1_2 : ( ruleQualifiedNameWithWildcard ) ;
public final void rule__XImportDeclaration__ImportedNamespaceAssignment_1_2() throws RecognitionException {
int stackSize = keepStackSize();
try {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43892:1: ( ( ruleQualifiedNameWithWildcard ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43893:1: ( ruleQualifiedNameWithWildcard )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43893:1: ( ruleQualifiedNameWithWildcard )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:43894:1: ruleQualifiedNameWithWildcard
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXImportDeclarationAccess().getImportedNamespaceQualifiedNameWithWildcardParserRuleCall_1_2_0());
}
pushFollow(FollowSets003.FOLLOW_ruleQualifiedNameWithWildcard_in_rule__XImportDeclaration__ImportedNamespaceAssignment_1_288319);
ruleQualifiedNameWithWildcard();
state._fsp--;
if (state.failed) return ;
if ( state.backtracking==0 ) {
after(grammarAccess.getXImportDeclarationAccess().getImportedNamespaceQualifiedNameWithWildcardParserRuleCall_1_2_0());
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
restoreStackSize(stackSize);
}
return ;
}
// $ANTLR end "rule__XImportDeclaration__ImportedNamespaceAssignment_1_2"
// $ANTLR start synpred75_InternalMango
public final void synpred75_InternalMango_fragment() throws RecognitionException {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5499:1: ( ( ( rule__OpOther__Group_6_1_0__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5499:1: ( ( rule__OpOther__Group_6_1_0__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5499:1: ( ( rule__OpOther__Group_6_1_0__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5500:1: ( rule__OpOther__Group_6_1_0__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getOpOtherAccess().getGroup_6_1_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5501:1: ( rule__OpOther__Group_6_1_0__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5501:2: rule__OpOther__Group_6_1_0__0
{
pushFollow(FollowSets003.FOLLOW_rule__OpOther__Group_6_1_0__0_in_synpred75_InternalMango11906);
rule__OpOther__Group_6_1_0__0();
state._fsp--;
if (state.failed) return ;
}
}
}
}
// $ANTLR end synpred75_InternalMango
// $ANTLR start synpred76_InternalMango
public final void synpred76_InternalMango_fragment() throws RecognitionException {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5505:6: ( ( '<' ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5505:6: ( '<' )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5505:6: ( '<' )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5506:1: '<'
{
if ( state.backtracking==0 ) {
before(grammarAccess.getOpOtherAccess().getLessThanSignKeyword_6_1_1());
}
match(input,27,FollowSets003.FOLLOW_27_in_synpred76_InternalMango11925); if (state.failed) return ;
}
}
}
// $ANTLR end synpred76_InternalMango
// $ANTLR start synpred89_InternalMango
public final void synpred89_InternalMango_fragment() throws RecognitionException {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5757:1: ( ( ( rule__XMemberFeatureCall__MemberCallArgumentsAssignment_1_1_3_1_0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5757:1: ( ( rule__XMemberFeatureCall__MemberCallArgumentsAssignment_1_1_3_1_0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5757:1: ( ( rule__XMemberFeatureCall__MemberCallArgumentsAssignment_1_1_3_1_0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5758:1: ( rule__XMemberFeatureCall__MemberCallArgumentsAssignment_1_1_3_1_0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXMemberFeatureCallAccess().getMemberCallArgumentsAssignment_1_1_3_1_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5759:1: ( rule__XMemberFeatureCall__MemberCallArgumentsAssignment_1_1_3_1_0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5759:2: rule__XMemberFeatureCall__MemberCallArgumentsAssignment_1_1_3_1_0
{
pushFollow(FollowSets003.FOLLOW_rule__XMemberFeatureCall__MemberCallArgumentsAssignment_1_1_3_1_0_in_synpred89_InternalMango12484);
rule__XMemberFeatureCall__MemberCallArgumentsAssignment_1_1_3_1_0();
state._fsp--;
if (state.failed) return ;
}
}
}
}
// $ANTLR end synpred89_InternalMango
// $ANTLR start synpred97_InternalMango
public final void synpred97_InternalMango_fragment() throws RecognitionException {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5821:6: ( ( ( ruleXForLoopExpression ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5821:6: ( ( ruleXForLoopExpression ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5821:6: ( ( ruleXForLoopExpression ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5822:1: ( ruleXForLoopExpression )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXPrimaryExpressionAccess().getXForLoopExpressionParserRuleCall_7());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5823:1: ( ruleXForLoopExpression )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5823:3: ruleXForLoopExpression
{
pushFollow(FollowSets003.FOLLOW_ruleXForLoopExpression_in_synpred97_InternalMango12657);
ruleXForLoopExpression();
state._fsp--;
if (state.failed) return ;
}
}
}
}
// $ANTLR end synpred97_InternalMango
// $ANTLR start synpred98_InternalMango
public final void synpred98_InternalMango_fragment() throws RecognitionException {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5827:6: ( ( ruleXBasicForLoopExpression ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5827:6: ( ruleXBasicForLoopExpression )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5827:6: ( ruleXBasicForLoopExpression )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5828:1: ruleXBasicForLoopExpression
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXPrimaryExpressionAccess().getXBasicForLoopExpressionParserRuleCall_8());
}
pushFollow(FollowSets003.FOLLOW_ruleXBasicForLoopExpression_in_synpred98_InternalMango12675);
ruleXBasicForLoopExpression();
state._fsp--;
if (state.failed) return ;
}
}
}
// $ANTLR end synpred98_InternalMango
// $ANTLR start synpred111_InternalMango
public final void synpred111_InternalMango_fragment() throws RecognitionException {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5953:1: ( ( ( rule__XSwitchExpression__Group_2_0__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5953:1: ( ( rule__XSwitchExpression__Group_2_0__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5953:1: ( ( rule__XSwitchExpression__Group_2_0__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5954:1: ( rule__XSwitchExpression__Group_2_0__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXSwitchExpressionAccess().getGroup_2_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5955:1: ( rule__XSwitchExpression__Group_2_0__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:5955:2: rule__XSwitchExpression__Group_2_0__0
{
pushFollow(FollowSets003.FOLLOW_rule__XSwitchExpression__Group_2_0__0_in_synpred111_InternalMango12994);
rule__XSwitchExpression__Group_2_0__0();
state._fsp--;
if (state.failed) return ;
}
}
}
}
// $ANTLR end synpred111_InternalMango
// $ANTLR start synpred115_InternalMango
public final void synpred115_InternalMango_fragment() throws RecognitionException {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6043:1: ( ( ( rule__XVariableDeclaration__Group_2_0__0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6043:1: ( ( rule__XVariableDeclaration__Group_2_0__0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6043:1: ( ( rule__XVariableDeclaration__Group_2_0__0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6044:1: ( rule__XVariableDeclaration__Group_2_0__0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXVariableDeclarationAccess().getGroup_2_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6045:1: ( rule__XVariableDeclaration__Group_2_0__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6045:2: rule__XVariableDeclaration__Group_2_0__0
{
pushFollow(FollowSets003.FOLLOW_rule__XVariableDeclaration__Group_2_0__0_in_synpred115_InternalMango13198);
rule__XVariableDeclaration__Group_2_0__0();
state._fsp--;
if (state.failed) return ;
}
}
}
}
// $ANTLR end synpred115_InternalMango
// $ANTLR start synpred116_InternalMango
public final void synpred116_InternalMango_fragment() throws RecognitionException {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6065:1: ( ( ( rule__XFeatureCall__FeatureCallArgumentsAssignment_3_1_0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6065:1: ( ( rule__XFeatureCall__FeatureCallArgumentsAssignment_3_1_0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6065:1: ( ( rule__XFeatureCall__FeatureCallArgumentsAssignment_3_1_0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6066:1: ( rule__XFeatureCall__FeatureCallArgumentsAssignment_3_1_0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXFeatureCallAccess().getFeatureCallArgumentsAssignment_3_1_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6067:1: ( rule__XFeatureCall__FeatureCallArgumentsAssignment_3_1_0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6067:2: rule__XFeatureCall__FeatureCallArgumentsAssignment_3_1_0
{
pushFollow(FollowSets003.FOLLOW_rule__XFeatureCall__FeatureCallArgumentsAssignment_3_1_0_in_synpred116_InternalMango13249);
rule__XFeatureCall__FeatureCallArgumentsAssignment_3_1_0();
state._fsp--;
if (state.failed) return ;
}
}
}
}
// $ANTLR end synpred116_InternalMango
// $ANTLR start synpred122_InternalMango
public final void synpred122_InternalMango_fragment() throws RecognitionException {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6159:1: ( ( ( rule__XConstructorCall__ArgumentsAssignment_4_1_0 ) ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6159:1: ( ( rule__XConstructorCall__ArgumentsAssignment_4_1_0 ) )
{
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6159:1: ( ( rule__XConstructorCall__ArgumentsAssignment_4_1_0 ) )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6160:1: ( rule__XConstructorCall__ArgumentsAssignment_4_1_0 )
{
if ( state.backtracking==0 ) {
before(grammarAccess.getXConstructorCallAccess().getArgumentsAssignment_4_1_0());
}
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6161:1: ( rule__XConstructorCall__ArgumentsAssignment_4_1_0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:6161:2: rule__XConstructorCall__ArgumentsAssignment_4_1_0
{
pushFollow(FollowSets003.FOLLOW_rule__XConstructorCall__ArgumentsAssignment_4_1_0_in_synpred122_InternalMango13464);
rule__XConstructorCall__ArgumentsAssignment_4_1_0();
state._fsp--;
if (state.failed) return ;
}
}
}
}
// $ANTLR end synpred122_InternalMango
// $ANTLR start synpred282_InternalMango
public final void synpred282_InternalMango_fragment() throws RecognitionException {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24591:2: ( rule__XAssignment__Group_1_1__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:24591:2: rule__XAssignment__Group_1_1__0
{
pushFollow(FollowSets003.FOLLOW_rule__XAssignment__Group_1_1__0_in_synpred282_InternalMango49886);
rule__XAssignment__Group_1_1__0();
state._fsp--;
if (state.failed) return ;
}
}
// $ANTLR end synpred282_InternalMango
// $ANTLR start synpred284_InternalMango
public final void synpred284_InternalMango_fragment() throws RecognitionException {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25002:2: ( rule__XOrExpression__Group_1__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25002:2: rule__XOrExpression__Group_1__0
{
pushFollow(FollowSets003.FOLLOW_rule__XOrExpression__Group_1__0_in_synpred284_InternalMango50690);
rule__XOrExpression__Group_1__0();
state._fsp--;
if (state.failed) return ;
}
}
// $ANTLR end synpred284_InternalMango
// $ANTLR start synpred285_InternalMango
public final void synpred285_InternalMango_fragment() throws RecognitionException {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25217:2: ( rule__XAndExpression__Group_1__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25217:2: rule__XAndExpression__Group_1__0
{
pushFollow(FollowSets003.FOLLOW_rule__XAndExpression__Group_1__0_in_synpred285_InternalMango51113);
rule__XAndExpression__Group_1__0();
state._fsp--;
if (state.failed) return ;
}
}
// $ANTLR end synpred285_InternalMango
// $ANTLR start synpred286_InternalMango
public final void synpred286_InternalMango_fragment() throws RecognitionException {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25432:2: ( rule__XEqualityExpression__Group_1__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25432:2: rule__XEqualityExpression__Group_1__0
{
pushFollow(FollowSets003.FOLLOW_rule__XEqualityExpression__Group_1__0_in_synpred286_InternalMango51536);
rule__XEqualityExpression__Group_1__0();
state._fsp--;
if (state.failed) return ;
}
}
// $ANTLR end synpred286_InternalMango
// $ANTLR start synpred287_InternalMango
public final void synpred287_InternalMango_fragment() throws RecognitionException {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25647:2: ( rule__XRelationalExpression__Alternatives_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:25647:2: rule__XRelationalExpression__Alternatives_1
{
pushFollow(FollowSets003.FOLLOW_rule__XRelationalExpression__Alternatives_1_in_synpred287_InternalMango51959);
rule__XRelationalExpression__Alternatives_1();
state._fsp--;
if (state.failed) return ;
}
}
// $ANTLR end synpred287_InternalMango
// $ANTLR start synpred288_InternalMango
public final void synpred288_InternalMango_fragment() throws RecognitionException {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26083:2: ( rule__XOtherOperatorExpression__Group_1__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26083:2: rule__XOtherOperatorExpression__Group_1__0
{
pushFollow(FollowSets003.FOLLOW_rule__XOtherOperatorExpression__Group_1__0_in_synpred288_InternalMango52811);
rule__XOtherOperatorExpression__Group_1__0();
state._fsp--;
if (state.failed) return ;
}
}
// $ANTLR end synpred288_InternalMango
// $ANTLR start synpred289_InternalMango
public final void synpred289_InternalMango_fragment() throws RecognitionException {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26679:2: ( rule__XAdditiveExpression__Group_1__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26679:2: rule__XAdditiveExpression__Group_1__0
{
pushFollow(FollowSets003.FOLLOW_rule__XAdditiveExpression__Group_1__0_in_synpred289_InternalMango53973);
rule__XAdditiveExpression__Group_1__0();
state._fsp--;
if (state.failed) return ;
}
}
// $ANTLR end synpred289_InternalMango
// $ANTLR start synpred290_InternalMango
public final void synpred290_InternalMango_fragment() throws RecognitionException {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26894:2: ( rule__XMultiplicativeExpression__Group_1__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:26894:2: rule__XMultiplicativeExpression__Group_1__0
{
pushFollow(FollowSets003.FOLLOW_rule__XMultiplicativeExpression__Group_1__0_in_synpred290_InternalMango54396);
rule__XMultiplicativeExpression__Group_1__0();
state._fsp--;
if (state.failed) return ;
}
}
// $ANTLR end synpred290_InternalMango
// $ANTLR start synpred291_InternalMango
public final void synpred291_InternalMango_fragment() throws RecognitionException {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27203:2: ( rule__XCastedExpression__Group_1__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27203:2: rule__XCastedExpression__Group_1__0
{
pushFollow(FollowSets003.FOLLOW_rule__XCastedExpression__Group_1__0_in_synpred291_InternalMango55003);
rule__XCastedExpression__Group_1__0();
state._fsp--;
if (state.failed) return ;
}
}
// $ANTLR end synpred291_InternalMango
// $ANTLR start synpred292_InternalMango
public final void synpred292_InternalMango_fragment() throws RecognitionException {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27420:2: ( rule__XPostfixOperation__Group_1__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27420:2: rule__XPostfixOperation__Group_1__0
{
pushFollow(FollowSets003.FOLLOW_rule__XPostfixOperation__Group_1__0_in_synpred292_InternalMango55428);
rule__XPostfixOperation__Group_1__0();
state._fsp--;
if (state.failed) return ;
}
}
// $ANTLR end synpred292_InternalMango
// $ANTLR start synpred293_InternalMango
public final void synpred293_InternalMango_fragment() throws RecognitionException {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27574:2: ( rule__XMemberFeatureCall__Alternatives_1 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27574:2: rule__XMemberFeatureCall__Alternatives_1
{
pushFollow(FollowSets003.FOLLOW_rule__XMemberFeatureCall__Alternatives_1_in_synpred293_InternalMango55730);
rule__XMemberFeatureCall__Alternatives_1();
state._fsp--;
if (state.failed) return ;
}
}
// $ANTLR end synpred293_InternalMango
// $ANTLR start synpred295_InternalMango
public final void synpred295_InternalMango_fragment() throws RecognitionException {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27910:2: ( rule__XMemberFeatureCall__Group_1_1_3__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27910:2: rule__XMemberFeatureCall__Group_1_1_3__0
{
pushFollow(FollowSets003.FOLLOW_rule__XMemberFeatureCall__Group_1_1_3__0_in_synpred295_InternalMango56401);
rule__XMemberFeatureCall__Group_1_1_3__0();
state._fsp--;
if (state.failed) return ;
}
}
// $ANTLR end synpred295_InternalMango
// $ANTLR start synpred296_InternalMango
public final void synpred296_InternalMango_fragment() throws RecognitionException {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27938:2: ( rule__XMemberFeatureCall__MemberCallArgumentsAssignment_1_1_4 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:27938:2: rule__XMemberFeatureCall__MemberCallArgumentsAssignment_1_1_4
{
pushFollow(FollowSets003.FOLLOW_rule__XMemberFeatureCall__MemberCallArgumentsAssignment_1_1_4_in_synpred296_InternalMango56459);
rule__XMemberFeatureCall__MemberCallArgumentsAssignment_1_1_4();
state._fsp--;
if (state.failed) return ;
}
}
// $ANTLR end synpred296_InternalMango
// $ANTLR start synpred304_InternalMango
public final void synpred304_InternalMango_fragment() throws RecognitionException {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29079:2: ( rule__XClosure__Group_1__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:29079:2: rule__XClosure__Group_1__0
{
pushFollow(FollowSets003.FOLLOW_rule__XClosure__Group_1__0_in_synpred304_InternalMango58695);
rule__XClosure__Group_1__0();
state._fsp--;
if (state.failed) return ;
}
}
// $ANTLR end synpred304_InternalMango
// $ANTLR start synpred311_InternalMango
public final void synpred311_InternalMango_fragment() throws RecognitionException {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30197:2: ( rule__XIfExpression__Group_6__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30197:2: rule__XIfExpression__Group_6__0
{
pushFollow(FollowSets003.FOLLOW_rule__XIfExpression__Group_6__0_in_synpred311_InternalMango60901);
rule__XIfExpression__Group_6__0();
state._fsp--;
if (state.failed) return ;
}
}
// $ANTLR end synpred311_InternalMango
// $ANTLR start synpred314_InternalMango
public final void synpred314_InternalMango_fragment() throws RecognitionException {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30747:2: ( rule__XSwitchExpression__Group_2_1_0__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:30747:2: rule__XSwitchExpression__Group_2_1_0__0
{
pushFollow(FollowSets003.FOLLOW_rule__XSwitchExpression__Group_2_1_0__0_in_synpred314_InternalMango61972);
rule__XSwitchExpression__Group_2_1_0__0();
state._fsp--;
if (state.failed) return ;
}
}
// $ANTLR end synpred314_InternalMango
// $ANTLR start synpred327_InternalMango
public final void synpred327_InternalMango_fragment() throws RecognitionException {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33235:2: ( rule__XFeatureCall__Group_3__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33235:2: rule__XFeatureCall__Group_3__0
{
pushFollow(FollowSets003.FOLLOW_rule__XFeatureCall__Group_3__0_in_synpred327_InternalMango66866);
rule__XFeatureCall__Group_3__0();
state._fsp--;
if (state.failed) return ;
}
}
// $ANTLR end synpred327_InternalMango
// $ANTLR start synpred328_InternalMango
public final void synpred328_InternalMango_fragment() throws RecognitionException {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33263:2: ( rule__XFeatureCall__FeatureCallArgumentsAssignment_4 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33263:2: rule__XFeatureCall__FeatureCallArgumentsAssignment_4
{
pushFollow(FollowSets003.FOLLOW_rule__XFeatureCall__FeatureCallArgumentsAssignment_4_in_synpred328_InternalMango66924);
rule__XFeatureCall__FeatureCallArgumentsAssignment_4();
state._fsp--;
if (state.failed) return ;
}
}
// $ANTLR end synpred328_InternalMango
// $ANTLR start synpred332_InternalMango
public final void synpred332_InternalMango_fragment() throws RecognitionException {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33801:2: ( rule__XConstructorCall__Group_3__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33801:2: rule__XConstructorCall__Group_3__0
{
pushFollow(FollowSets003.FOLLOW_rule__XConstructorCall__Group_3__0_in_synpred332_InternalMango67982);
rule__XConstructorCall__Group_3__0();
state._fsp--;
if (state.failed) return ;
}
}
// $ANTLR end synpred332_InternalMango
// $ANTLR start synpred333_InternalMango
public final void synpred333_InternalMango_fragment() throws RecognitionException {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33830:2: ( rule__XConstructorCall__Group_4__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33830:2: rule__XConstructorCall__Group_4__0
{
pushFollow(FollowSets003.FOLLOW_rule__XConstructorCall__Group_4__0_in_synpred333_InternalMango68043);
rule__XConstructorCall__Group_4__0();
state._fsp--;
if (state.failed) return ;
}
}
// $ANTLR end synpred333_InternalMango
// $ANTLR start synpred334_InternalMango
public final void synpred334_InternalMango_fragment() throws RecognitionException {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33858:2: ( rule__XConstructorCall__ArgumentsAssignment_5 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:33858:2: rule__XConstructorCall__ArgumentsAssignment_5
{
pushFollow(FollowSets003.FOLLOW_rule__XConstructorCall__ArgumentsAssignment_5_in_synpred334_InternalMango68101);
rule__XConstructorCall__ArgumentsAssignment_5();
state._fsp--;
if (state.failed) return ;
}
}
// $ANTLR end synpred334_InternalMango
// $ANTLR start synpred339_InternalMango
public final void synpred339_InternalMango_fragment() throws RecognitionException {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34911:2: ( rule__XReturnExpression__ExpressionAssignment_2 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:34911:2: rule__XReturnExpression__ExpressionAssignment_2
{
pushFollow(FollowSets003.FOLLOW_rule__XReturnExpression__ExpressionAssignment_2_in_synpred339_InternalMango70153);
rule__XReturnExpression__ExpressionAssignment_2();
state._fsp--;
if (state.failed) return ;
}
}
// $ANTLR end synpred339_InternalMango
// $ANTLR start synpred340_InternalMango
public final void synpred340_InternalMango_fragment() throws RecognitionException {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35079:2: ( rule__XTryCatchFinallyExpression__CatchClausesAssignment_3_0_0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35079:2: rule__XTryCatchFinallyExpression__CatchClausesAssignment_3_0_0
{
pushFollow(FollowSets003.FOLLOW_rule__XTryCatchFinallyExpression__CatchClausesAssignment_3_0_0_in_synpred340_InternalMango70482);
rule__XTryCatchFinallyExpression__CatchClausesAssignment_3_0_0();
state._fsp--;
if (state.failed) return ;
}
}
// $ANTLR end synpred340_InternalMango
// $ANTLR start synpred341_InternalMango
public final void synpred341_InternalMango_fragment() throws RecognitionException {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35108:2: ( rule__XTryCatchFinallyExpression__Group_3_0_1__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35108:2: rule__XTryCatchFinallyExpression__Group_3_0_1__0
{
pushFollow(FollowSets003.FOLLOW_rule__XTryCatchFinallyExpression__Group_3_0_1__0_in_synpred341_InternalMango70542);
rule__XTryCatchFinallyExpression__Group_3_0_1__0();
state._fsp--;
if (state.failed) return ;
}
}
// $ANTLR end synpred341_InternalMango
// $ANTLR start synpred342_InternalMango
public final void synpred342_InternalMango_fragment() throws RecognitionException {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35708:2: ( rule__QualifiedName__Group_1__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35708:2: rule__QualifiedName__Group_1__0
{
pushFollow(FollowSets003.FOLLOW_rule__QualifiedName__Group_1__0_in_synpred342_InternalMango71720);
rule__QualifiedName__Group_1__0();
state._fsp--;
if (state.failed) return ;
}
}
// $ANTLR end synpred342_InternalMango
// $ANTLR start synpred344_InternalMango
public final void synpred344_InternalMango_fragment() throws RecognitionException {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35957:2: ( rule__JvmTypeReference__Group_0_1__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:35957:2: rule__JvmTypeReference__Group_0_1__0
{
pushFollow(FollowSets003.FOLLOW_rule__JvmTypeReference__Group_0_1__0_in_synpred344_InternalMango72211);
rule__JvmTypeReference__Group_0_1__0();
state._fsp--;
if (state.failed) return ;
}
}
// $ANTLR end synpred344_InternalMango
// $ANTLR start synpred348_InternalMango
public final void synpred348_InternalMango_fragment() throws RecognitionException {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36490:2: ( rule__JvmParameterizedTypeReference__Group_1__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36490:2: rule__JvmParameterizedTypeReference__Group_1__0
{
pushFollow(FollowSets003.FOLLOW_rule__JvmParameterizedTypeReference__Group_1__0_in_synpred348_InternalMango73257);
rule__JvmParameterizedTypeReference__Group_1__0();
state._fsp--;
if (state.failed) return ;
}
}
// $ANTLR end synpred348_InternalMango
// $ANTLR start synpred350_InternalMango
public final void synpred350_InternalMango_fragment() throws RecognitionException {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36642:2: ( rule__JvmParameterizedTypeReference__Group_1_4__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36642:2: rule__JvmParameterizedTypeReference__Group_1_4__0
{
pushFollow(FollowSets003.FOLLOW_rule__JvmParameterizedTypeReference__Group_1_4__0_in_synpred350_InternalMango73566);
rule__JvmParameterizedTypeReference__Group_1_4__0();
state._fsp--;
if (state.failed) return ;
}
}
// $ANTLR end synpred350_InternalMango
// $ANTLR start synpred351_InternalMango
public final void synpred351_InternalMango_fragment() throws RecognitionException {
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36801:2: ( rule__JvmParameterizedTypeReference__Group_1_4_2__0 )
// ../mango-dsl-ui/src-gen/io/pelle/mango/dsl/ui/contentassist/antlr/internal/InternalMango.g:36801:2: rule__JvmParameterizedTypeReference__Group_1_4_2__0
{
pushFollow(FollowSets003.FOLLOW_rule__JvmParameterizedTypeReference__Group_1_4_2__0_in_synpred351_InternalMango73877);
rule__JvmParameterizedTypeReference__Group_1_4_2__0();
state._fsp--;
if (state.failed) return ;
}
}
// $ANTLR end synpred351_InternalMango
}
@SuppressWarnings("all")
public class InternalMangoParser extends InternalMangoParser4 {
public InternalMangoParser(TokenStream input) {
this(input, new RecognizerSharedState());
}
public InternalMangoParser(TokenStream input, RecognizerSharedState state) {
super(input, state);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy