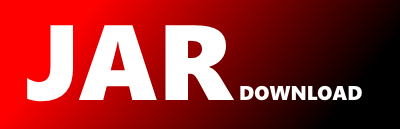
io.perfmark.impl.Storage Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of perfmark-impl Show documentation
Show all versions of perfmark-impl Show documentation
PerfMark Implementation API
The newest version!
/*
* Copyright 2019 Google LLC
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.perfmark.impl;
import java.lang.ref.Reference;
import java.lang.ref.SoftReference;
import java.lang.ref.WeakReference;
import java.util.ArrayList;
import java.util.Collections;
import java.util.HashSet;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.Set;
import java.util.concurrent.ConcurrentHashMap;
import java.util.concurrent.ConcurrentMap;
/**
* Storage is responsible for storing and returning recorded marks. This is a low level class and
* not intended for use by users. Instead, the {@code TraceEventWriter} and {@code TraceEventViewer}
* classes provide easier to use APIs for accessing PerfMark data.
*
* This code is NOT API stable, and may be removed in the future, or changed
* without notice.
*/
public final class Storage {
/*
* Invariants:
*
* - A Thread may have at most one MarkRecorder at a time.
* - If a MarkHolder is detached from its Thread, it cannot be written to again.
*
*/
// The order of initialization here matters. If a logger invokes PerfMark, it will be re-entrant
// and need to use these static variables.
private static final ConcurrentMap
© 2015 - 2025 Weber Informatics LLC | Privacy Policy