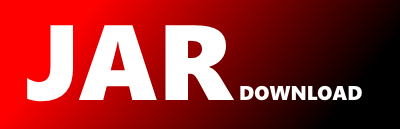
io.permazen.kv.raft.MostRecentView Maven / Gradle / Ivy
/*
* Copyright (C) 2015 Archie L. Cobbs. All rights reserved.
*/
package io.permazen.kv.raft;
import io.permazen.kv.CloseableKVStore;
import io.permazen.kv.KVStore;
import io.permazen.kv.mvcc.MutableView;
import io.permazen.kv.mvcc.Writes;
import io.permazen.kv.util.PrefixKVStore;
import java.util.HashMap;
import java.util.Map;
/**
* A view of the database based on the most recent log entry, if any, otherwise directly on the committed key/value store.
* Caller is responsible for eventually closing the snapshot.
*/
class MostRecentView {
private final CloseableKVStore snapshot;
private final long term;
private final long index;
private final HashMap config;
private final MutableView view;
MostRecentView(RaftKVDatabase raft) {
this(raft, -1);
}
MostRecentView(RaftKVDatabase raft, long maxIndex) {
// Sanity check
assert raft != null;
assert Thread.holdsLock(raft);
assert maxIndex >= -1;
// Grab a snapshot of the key/value store
this.snapshot = raft.kv.snapshot();
// Create a view of just the state machine keys and values and successively layer unapplied log entries
// If we require a committed view, then stop when we get to the first uncomitted log entry
KVStore kview = PrefixKVStore.create(snapshot, raft.getStateMachinePrefix());
this.config = new HashMap<>(raft.lastAppliedConfig);
long viewIndex = raft.lastAppliedIndex;
long viewTerm = raft.lastAppliedTerm;
for (LogEntry logEntry : raft.raftLog) {
if (maxIndex != -1 && logEntry.getIndex() > maxIndex)
break;
final Writes writes = logEntry.getWrites();
if (!writes.isEmpty())
kview = new MutableView(kview, null, writes);
logEntry.applyConfigChange(this.config);
viewIndex = logEntry.getIndex();
viewTerm = logEntry.getTerm();
}
// Finalize
this.view = new MutableView(kview);
this.term = viewTerm;
this.index = viewIndex;
}
public long getTerm() {
return this.term;
}
public long getIndex() {
return this.index;
}
public Map getConfig() {
return this.config;
}
public CloseableKVStore getSnapshot() {
return this.snapshot;
}
public MutableView getView() {
return this.view;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy