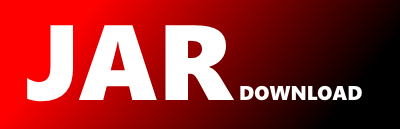
io.permazen.EnumConverter Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of permazen-main Show documentation
Show all versions of permazen-main Show documentation
Permazen classes that map Java model classes onto the core API.
/*
* Copyright (C) 2015 Archie L. Cobbs. All rights reserved.
*/
package io.permazen;
import com.google.common.base.Converter;
import com.google.common.base.Preconditions;
import com.google.common.collect.EnumHashBiMap;
import com.google.common.collect.Maps;
import io.permazen.core.EnumValue;
import java.util.EnumSet;
/**
* Converts between core database {@link EnumValue} objects and the corresponding Java {@link Enum} model values.
*/
public class EnumConverter> extends Converter {
private final Class enumType;
private final EnumHashBiMap valueMap;
/**
* Constructor.
*
* @param enumType {@link Enum} type
* @throws IllegalArgumentException if {@code enumType} is null
*/
public EnumConverter(Class enumType) {
Preconditions.checkArgument(enumType != null, "null enumType");
enumType.asSubclass(Enum.class); // verify it's really an Enum
this.enumType = enumType;
this.valueMap = EnumHashBiMap.create(Maps.asMap(EnumSet.allOf(this.enumType), EnumValue::new));
}
@Override
protected EnumValue doForward(T value) {
if (value == null)
return null;
final EnumValue enumValue = this.valueMap.get(value);
if (enumValue == null)
throw new IllegalArgumentException("invalid enum value " + value + " not an instance of " + this.enumType);
return enumValue;
}
@Override
protected T doBackward(EnumValue enumValue) {
if (enumValue == null)
return null;
final T value = this.valueMap.inverse().get(enumValue);
if (value == null)
throw new IllegalArgumentException("invalid value " + enumValue + " not found in " + this.enumType);
return value;
}
/**
* Get the {@link Enum} type associated with this instance.
*
* @return associated {@link Enum} type
*/
public Class getEnumType() {
return this.enumType;
}
/**
* Convenience "constructor" allowing wildcard caller {@link Enum} types.
*
* @param enumType type for the created converter
* @return new converter
*/
@SuppressWarnings({ "unchecked", "rawtypes" })
public static EnumConverter> createEnumConverter(Class extends Enum>> enumType) {
return new EnumConverter(enumType);
}
// Object
@Override
public boolean equals(Object obj) {
if (obj == this)
return true;
if (obj == null || obj.getClass() != this.getClass())
return false;
final EnumConverter> that = (EnumConverter>)obj;
return this.enumType == that.enumType;
}
@Override
public int hashCode() {
return this.enumType.hashCode();
}
@Override
public String toString() {
return this.getClass().getSimpleName() + "[type=" + this.enumType.getName() + "]";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy