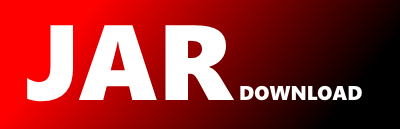
io.permazen.ExportContext Maven / Gradle / Ivy
Show all versions of permazen-main Show documentation
/*
* Copyright (C) 2015 Archie L. Cobbs. All rights reserved.
*/
package io.permazen;
import com.google.common.base.Preconditions;
import io.permazen.core.ObjId;
import io.permazen.core.util.ObjIdMap;
import java.util.Collections;
import java.util.Iterator;
import java.util.Map;
import java.util.function.Function;
/**
* Context for exporting plain (POJO) objects from a {@link JTransaction}.
*
*
* Plain objects (POJO's) can be exported from a {@link JTransaction} to the extent that the Permazen model class and
* the corresponding target POJO class share the same properties. The simplest example of this is when the Permazen model class
* is also the POJO class (implying a non-abstract class; see also
* {@link io.permazen.annotation.PermazenType#autogenNonAbstract @PermazenType.autogenNonAbstract()}). Also possible are POJO
* classes and model classes that implement common Java interfaces.
*
*
* The POJO corresponding to an exported database object is supplied by the configured {@code objectMapper}.
* If {@code objectMapper} returns null, the database object is not exported, and nulls replace any copied references to it.
* If {@code objectMapper} is null, the default behavior is to create a new POJO using the model class' default constructor,
* which of course implies the model class cannot be abstract.
*
*
* Instances ensure that an already-exported database object will be recognized and not exported twice.
* The {@code objectMapper} is invoked at most once for any object ID.
*
*
* When a database objext is exported, its fields are copied to the POJO. Fields for which no corresponding
* POJO property exists are omitted.
*
*
* Reference fields are traversed and the referenced objects are automatically exported as POJO's, recursively.
* In other words, the entire transitive closure of objects reachable from an exported object is exported.
* Cycles in the graph of references are handled properly.
*
*
Conversion Details
*
* {@link Counter} fields export to any {@link Number} property. Collection fields export to an existing collection,
* so that a setter method is not required; however, if the getter returns null, a setter is required and the export
* will attempt to use an appropriate collection class ({@link java.util.HashSet} for property of type {@link java.util.Set},
* {@link java.util.TreeSet} for a property of type {@link java.util.SortedSet}, etc). To avoid potential mismatch with
* collection types, initialize collection properties.
*
* @see ImportContext
*/
public class ExportContext {
private final JTransaction jtx;
private final Function objectMapper;
private final ObjIdMap