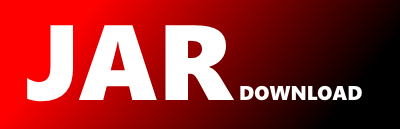
io.permazen.NavigableMapConverter Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of permazen-main Show documentation
Show all versions of permazen-main Show documentation
Permazen classes that map Java model classes onto the core API.
/*
* Copyright (C) 2015 Archie L. Cobbs. All rights reserved.
*/
package io.permazen;
import com.google.common.base.Converter;
import com.google.common.base.Preconditions;
import io.permazen.util.ConvertedNavigableMap;
import java.util.NavigableMap;
/**
* Converts {@link NavigableMap}s into {@link ConvertedNavigableMap}s using the provided element {@link Converter}.
*
* @param key type of converted maps
* @param value type of converted maps
* @param key type of unconverted (wrapped) maps
* @param value type of unconverted (wrapped) maps
*/
class NavigableMapConverter extends Converter, NavigableMap> {
private final Converter keyConverter;
private final Converter valueConverter;
NavigableMapConverter(Converter keyConverter, Converter valueConverter) {
Preconditions.checkArgument(keyConverter != null, "null keyConverter");
Preconditions.checkArgument(valueConverter != null, "null valueConverter");
this.keyConverter = keyConverter;
this.valueConverter = valueConverter;
}
@Override
protected NavigableMap doForward(NavigableMap map) {
if (map == null)
return null;
return new ConvertedNavigableMap(map, this.keyConverter.reverse(), this.valueConverter.reverse());
}
@Override
protected NavigableMap doBackward(NavigableMap map) {
if (map == null)
return null;
return new ConvertedNavigableMap<>(map, this.keyConverter, this.valueConverter);
}
// Object
@Override
public boolean equals(Object obj) {
if (obj == this)
return true;
if (obj == null || obj.getClass() != this.getClass())
return false;
final NavigableMapConverter, ?, ?, ?> that = (NavigableMapConverter, ?, ?, ?>)obj;
return this.keyConverter.equals(that.keyConverter) && this.valueConverter.equals(that.valueConverter);
}
@Override
public int hashCode() {
return this.keyConverter.hashCode() ^ this.valueConverter.hashCode();
}
@Override
public String toString() {
return this.getClass().getSimpleName() + "[keyConverter=" + this.keyConverter
+ ",valueConverter=" + this.valueConverter + "]";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy