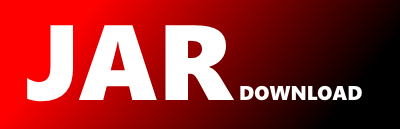
io.permazen.change.MapFieldAdd Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of permazen-main Show documentation
Show all versions of permazen-main Show documentation
Permazen classes that map Java model classes onto the core API.
/*
* Copyright (C) 2015 Archie L. Cobbs. All rights reserved.
*/
package io.permazen.change;
import io.permazen.JObject;
import io.permazen.JTransaction;
import java.util.Map;
import java.util.Objects;
/**
* Notification object that gets passed to {@link io.permazen.annotation.OnChange @OnChange}-annotated methods
* when a new key/value pair is added to a map field.
*
* @param the type of the object containing the changed field
* @param the type of the changed map's key
* @param the type of the changed map's value
*/
public class MapFieldAdd extends MapFieldChange {
private final K key;
private final V value;
/**
* Constructor.
*
* @param jobj Java object containing the map field that changed
* @param storageId the storage ID of the affected field
* @param fieldName the name of the field that changed
* @param key the key of the new key/value pair
* @param value the value of the new key/value pair
* @throws IllegalArgumentException if {@code jobj} or {@code fieldName} is null
*/
public MapFieldAdd(T jobj, int storageId, String fieldName, K key, V value) {
super(jobj, storageId, fieldName);
this.key = key;
this.value = value;
}
@Override
public R visit(ChangeSwitch target) {
return target.caseMapFieldAdd(this);
}
@Override
@SuppressWarnings("unchecked")
public void apply(JTransaction jtx, JObject jobj) {
((Map)jtx.readMapField(jobj.getObjId(), this.getStorageId(), false)).put(this.key, this.value);
}
/**
* Get the key of the new key/value pair that was added.
*
* @return the key of the newly added key/value pair
*/
public K getKey() {
return this.key;
}
/**
* Get the value of the new key/value pair that was added.
*
* @return the value of the newly added key/value pair
*/
public V getValue() {
return this.value;
}
// Object
@Override
public boolean equals(Object obj) {
if (obj == this)
return true;
if (!super.equals(obj))
return false;
final MapFieldAdd, ?, ?> that = (MapFieldAdd, ?, ?>)obj;
return Objects.equals(this.key, that.key)
&& Objects.equals(this.value, that.value);
}
@Override
public int hashCode() {
return super.hashCode() ^ Objects.hashCode(this.key) ^ Objects.hashCode(this.value);
}
@Override
public String toString() {
return "MapFieldAdd[object=" + this.getObject() + ",field=\"" + this.getFieldName() + "\",key="
+ this.key + ",value=" + this.value + "]";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy