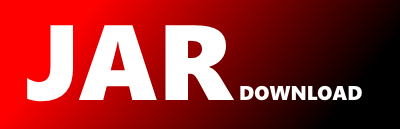
io.permazen.JCollectionField Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of permazen-main Show documentation
Show all versions of permazen-main Show documentation
Permazen classes that map Java model classes onto the core API.
/*
* Copyright (C) 2015 Archie L. Cobbs. All rights reserved.
*/
package io.permazen;
import com.google.common.base.Preconditions;
import com.google.common.reflect.TypeToken;
import io.permazen.core.CollectionField;
import io.permazen.core.ObjId;
import io.permazen.core.Transaction;
import io.permazen.schema.CollectionSchemaField;
import java.lang.annotation.Annotation;
import java.lang.reflect.Method;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
/**
* Represents a collection field in a {@link JClass}.
*/
public abstract class JCollectionField extends JComplexField {
final JSimpleField elementField;
JCollectionField(Permazen jdb, String name, int storageId,
Annotation annotation, JSimpleField elementField, String description, Method getter) {
super(jdb, name, storageId, annotation, description, getter);
Preconditions.checkArgument(elementField != null, "null elementField");
this.elementField = elementField;
}
/**
* Get the element sub-field.
*
* @return this instance's element sub-field
*/
public JSimpleField getElementField() {
return this.elementField;
}
@Override
public abstract Collection> getValue(JObject jobj);
@Override
public List getSubFields() {
return Collections.singletonList(this.elementField);
}
@Override
public JSimpleField getSubField(String name) {
if (CollectionField.ELEMENT_FIELD_NAME.equals(name))
return this.elementField;
throw new IllegalArgumentException("unknown sub-field `"
+ name + "' (did you mean `" + CollectionField.ELEMENT_FIELD_NAME + "' instead?)");
}
@Override
boolean isSameAs(JField that0) {
if (!super.isSameAs(that0))
return false;
final JCollectionField that = (JCollectionField)that0;
if (!this.elementField.isSameAs(that.elementField))
return false;
return true;
}
@Override
String getSubFieldName(JSimpleField subField) {
if (subField == this.elementField)
return CollectionField.ELEMENT_FIELD_NAME;
throw new IllegalArgumentException("unknown sub-field");
}
@Override
abstract CollectionSchemaField toSchemaItem(Permazen jdb);
void initialize(Permazen jdb, CollectionSchemaField schemaField) {
super.initialize(jdb, schemaField);
schemaField.setElementField(this.elementField.toSchemaItem(jdb));
}
@Override
public TypeToken> getTypeToken() {
return this.buildTypeToken(this.elementField.getTypeToken().wrap());
}
abstract TypeToken extends Collection> buildTypeToken(TypeToken elementType);
@Override
void addChangeParameterTypes(List> types, Class targetType) {
this.addChangeParameterTypes(types, targetType, this.elementField.getTypeToken());
}
abstract void addChangeParameterTypes(List> types, Class targetType, TypeToken elementType);
// POJO import/export
@Override
@SuppressWarnings("unchecked")
void importPlain(ImportContext context, Object obj, ObjId id) {
// Get POJO collection
final Collection> objCollection;
try {
objCollection = (Collection>)obj.getClass().getMethod(this.getter.getName()).invoke(obj);
} catch (Exception e) {
return;
}
if (objCollection == null)
return;
// Get JCollectionField collection
final Collection
© 2015 - 2025 Weber Informatics LLC | Privacy Policy